Polymorphism CSCI 201 Principles of Software Development Jeffrey
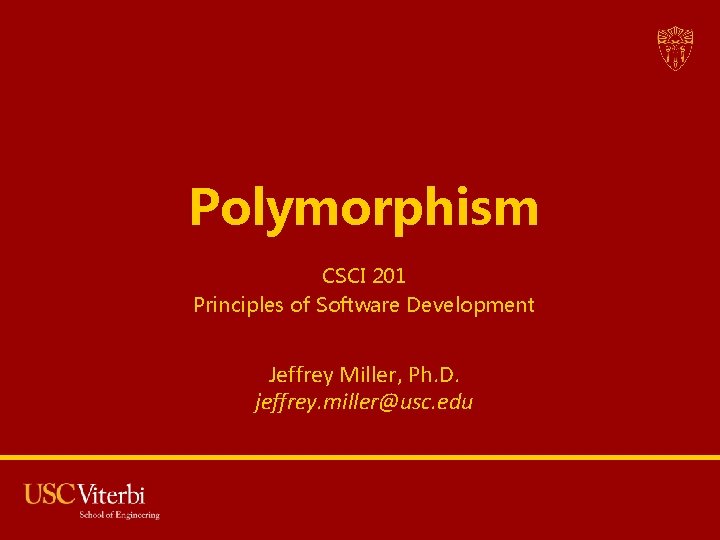
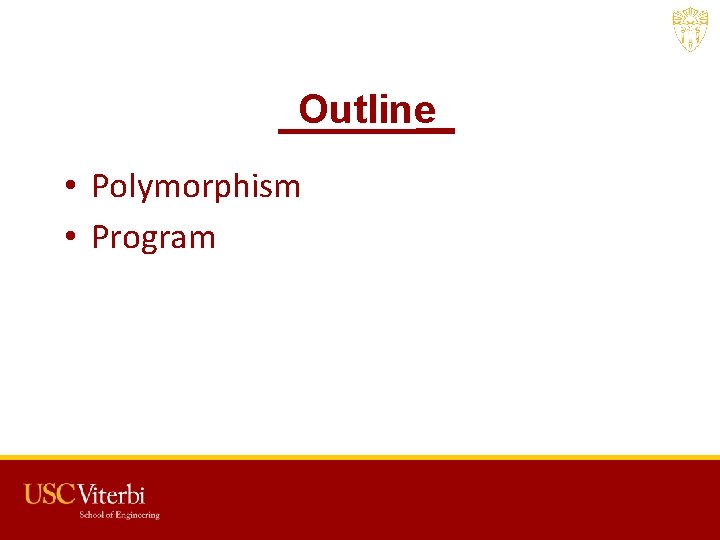
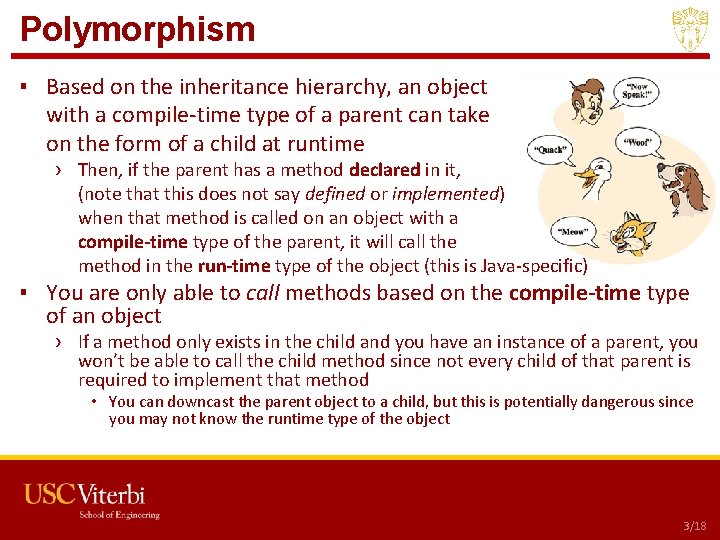
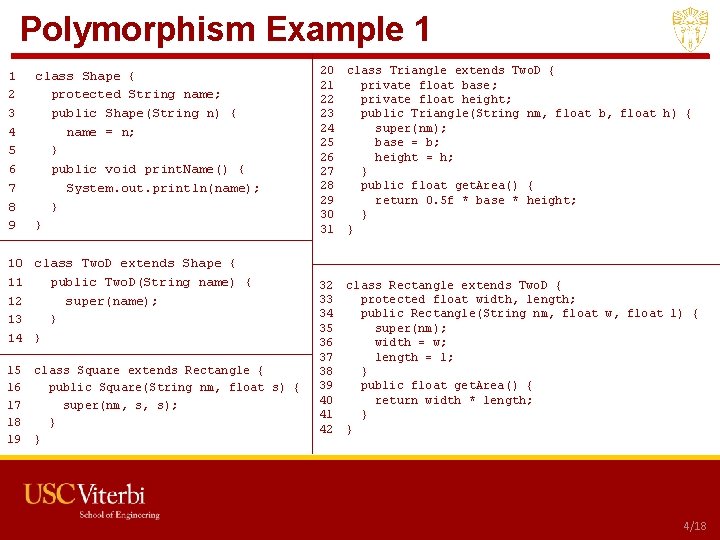
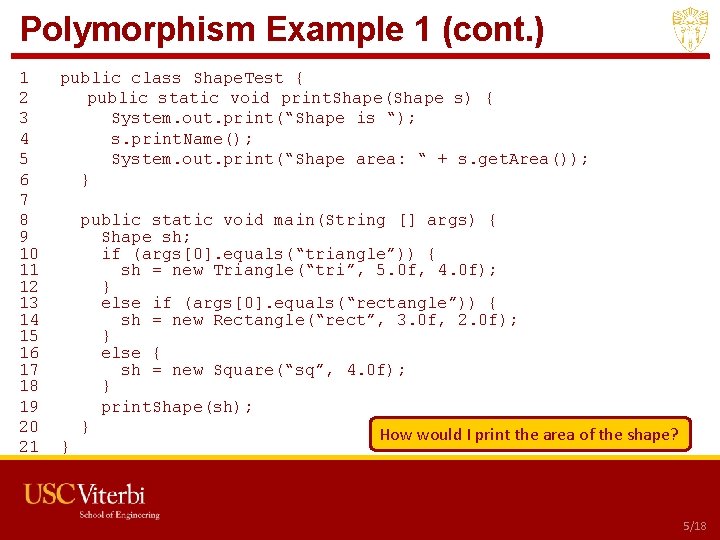
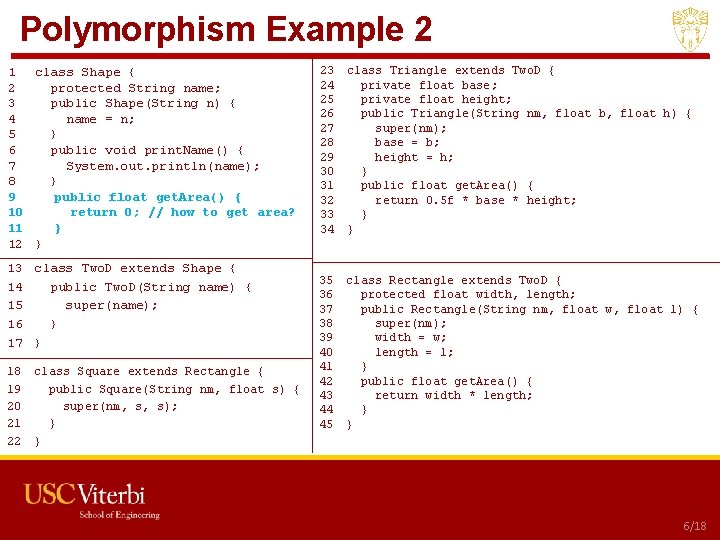
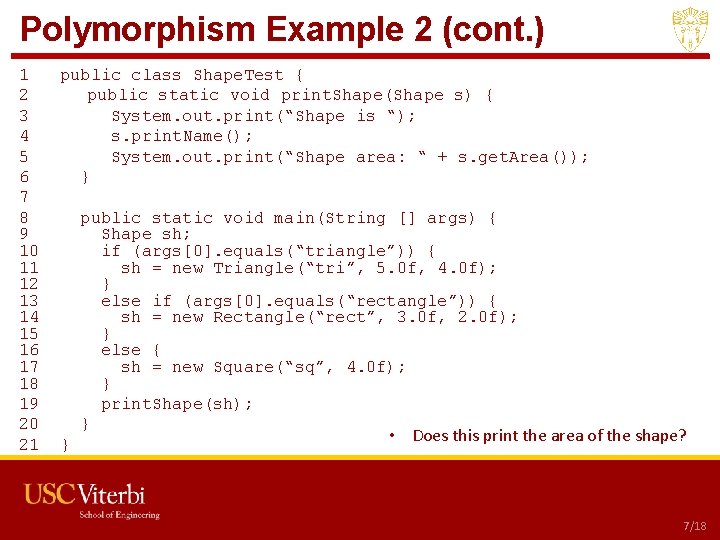
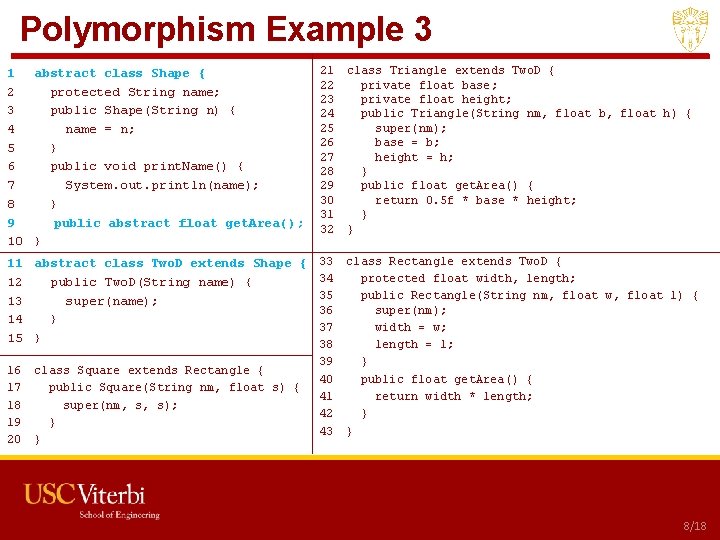
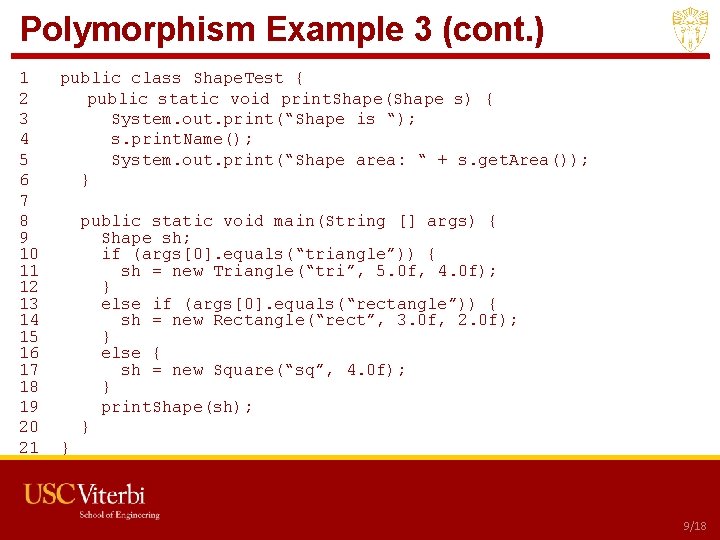
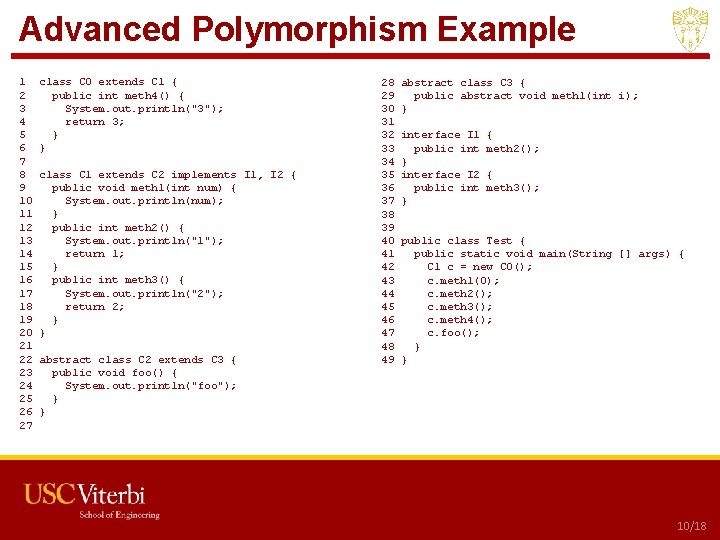
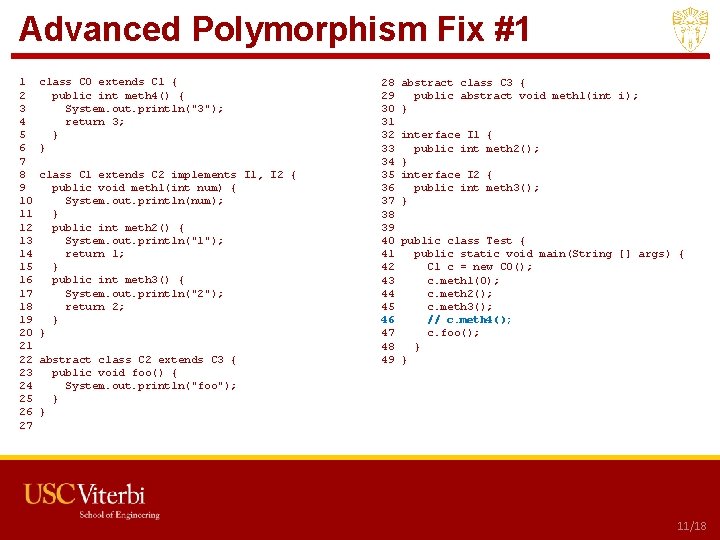
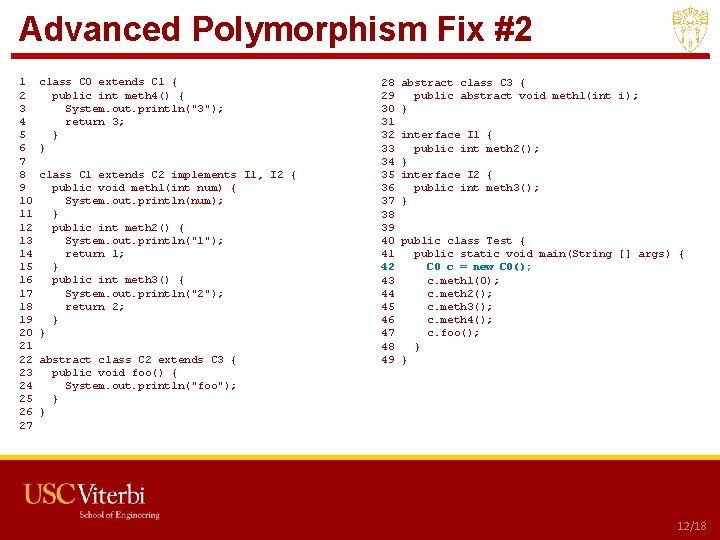
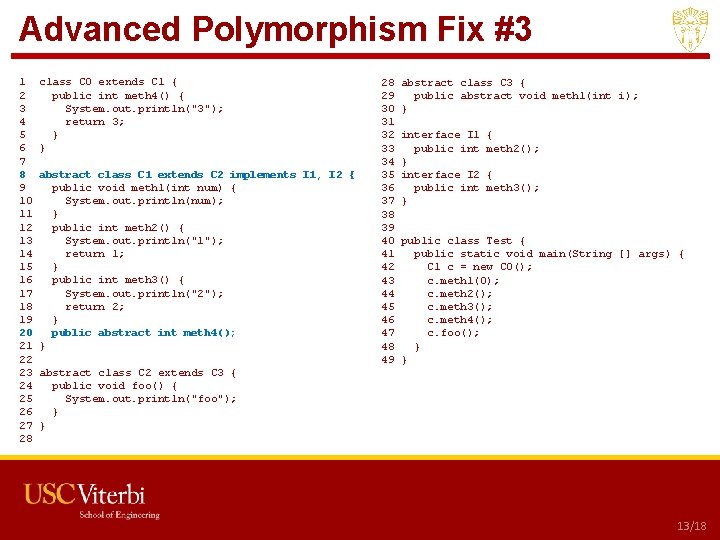
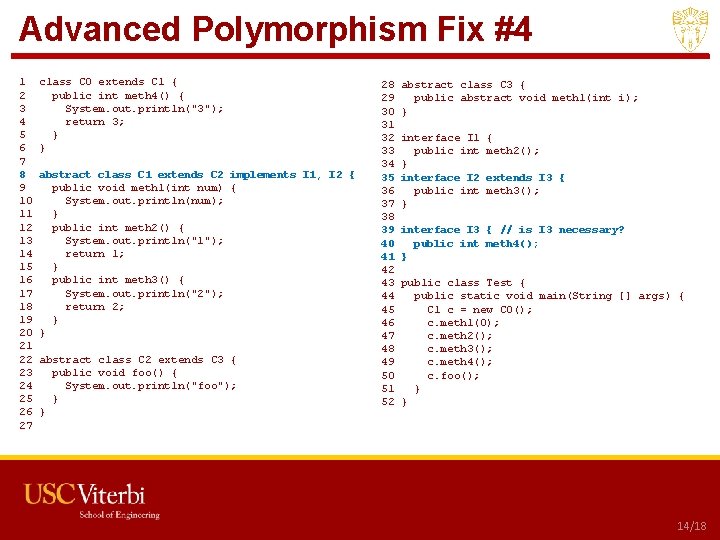
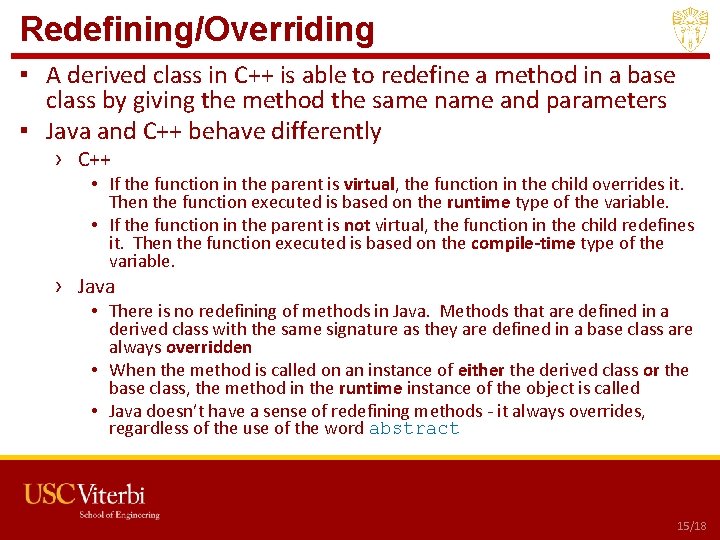
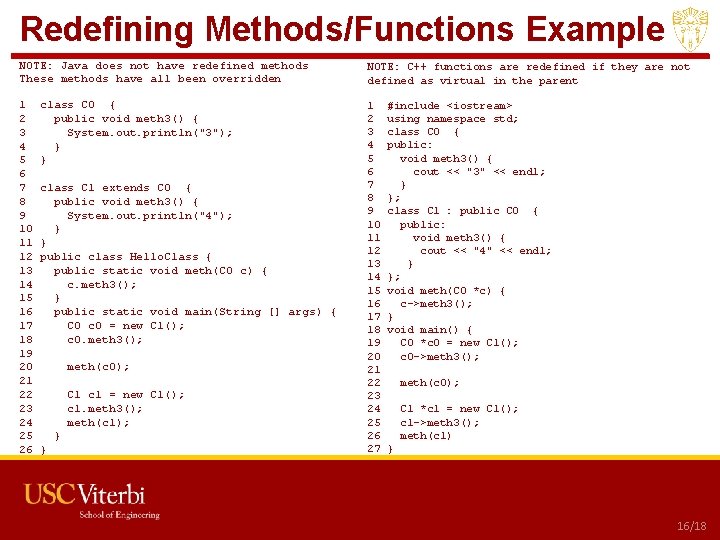
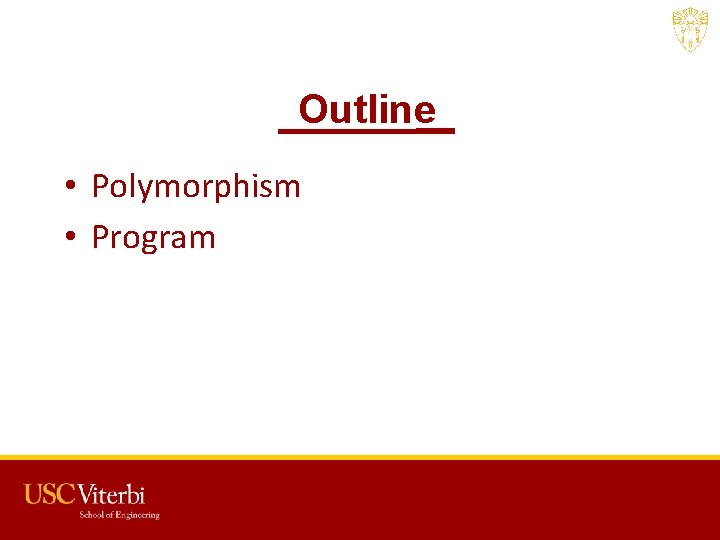
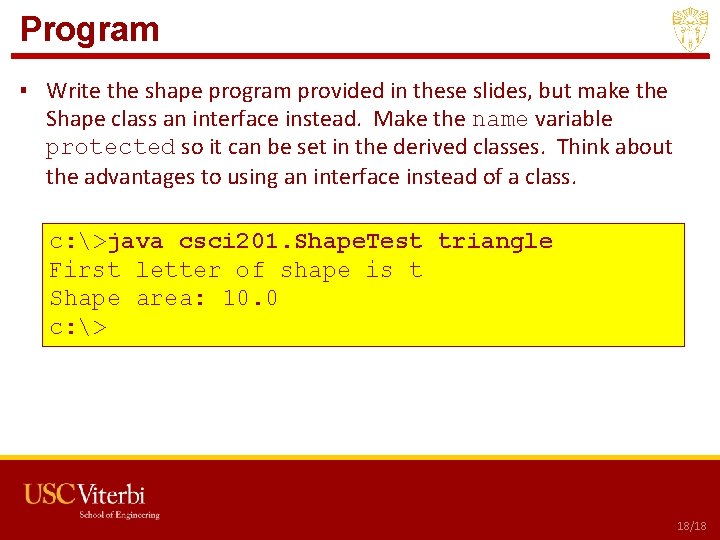
- Slides: 18
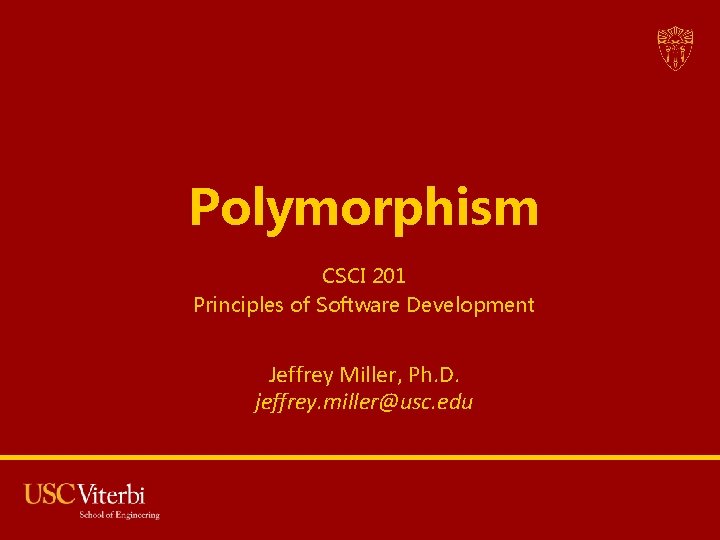
Polymorphism CSCI 201 Principles of Software Development Jeffrey Miller, Ph. D. jeffrey. miller@usc. edu
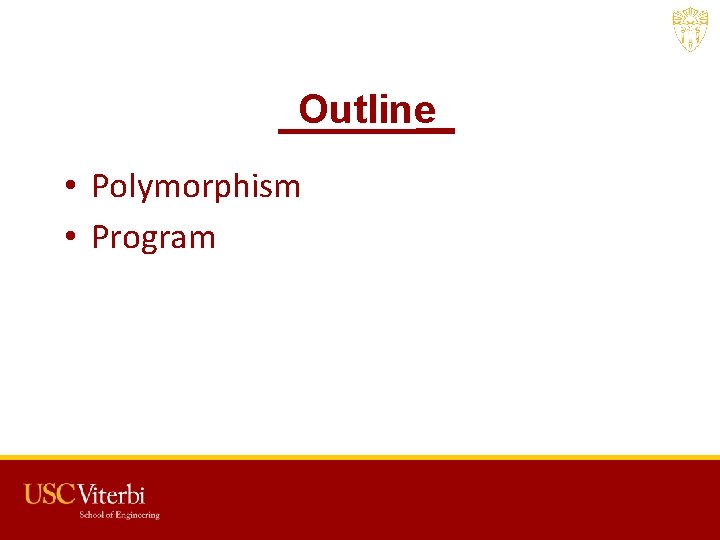
Outline • Polymorphism • Program USC CSCI 201 L
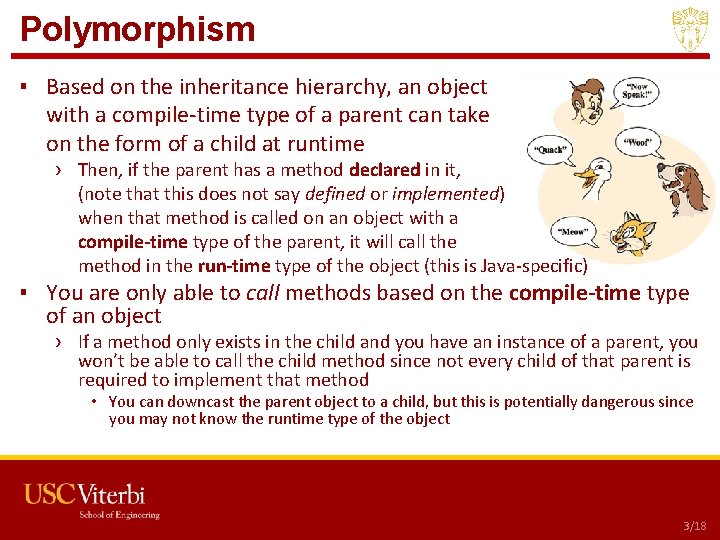
Polymorphism ▪ Based on the inheritance hierarchy, an object with a compile-time type of a parent can take on the form of a child at runtime › Then, if the parent has a method declared in it, (note that this does not say defined or implemented) when that method is called on an object with a compile-time type of the parent, it will call the method in the run-time type of the object (this is Java-specific) ▪ You are only able to call methods based on the compile-time type of an object › If a method only exists in the child and you have an instance of a parent, you won’t be able to call the child method since not every child of that parent is required to implement that method • You can downcast the parent object to a child, but this is potentially dangerous since you may not know the runtime type of the object • Polymorphism USC CSCI 201 L 3/18
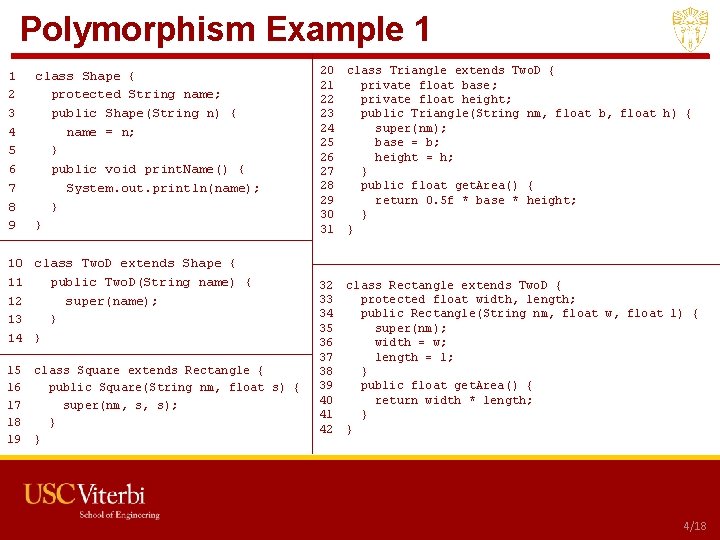
Polymorphism Example 1 1 2 3 4 5 6 7 8 9 class Shape { protected String name; public Shape(String n) { name = n; } public void print. Name() { System. out. println(name); } } 10 class Two. D extends Shape { 11 public Two. D(String name) { 12 super(name); 13 } 14 } 15 16 17 18 19 class Square extends Rectangle { public Square(String nm, float s) { super(nm, s, s); } } 20 21 22 23 24 25 26 27 28 29 30 31 class Triangle extends Two. D { private float base; private float height; public Triangle(String nm, float b, float h) { super(nm); base = b; height = h; } public float get. Area() { return 0. 5 f * base * height; } } 32 33 34 35 36 37 38 39 40 41 42 class Rectangle extends Two. D { protected float width, length; public Rectangle(String nm, float w, float l) { super(nm); width = w; length = l; } public float get. Area() { return width * length; } } USC CSCI 201 L 4/18
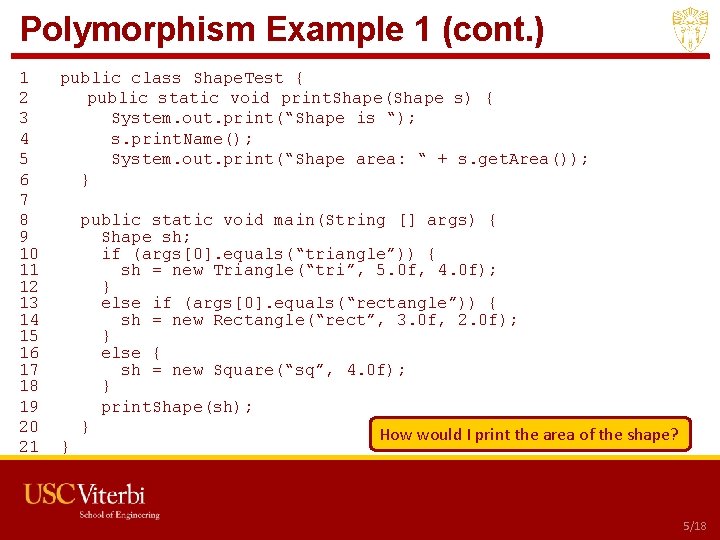
Polymorphism Example 1 (cont. ) 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 • public class Shape. Test { public static void print. Shape(Shape s) { System. out. print(“Shape is “); s. print. Name(); System. out. print(“Shape area: “ + s. get. Area()); } } public static void main(String [] args) { Shape sh; if (args[0]. equals(“triangle”)) { sh = new Triangle(“tri”, 5. 0 f, 4. 0 f); } else if (args[0]. equals(“rectangle”)) { sh = new Rectangle(“rect”, 3. 0 f, 2. 0 f); } else { sh = new Square(“sq”, 4. 0 f); } print. Shape(sh); } How would I print the area of the shape? Polymorphism USC CSCI 201 L 5/18
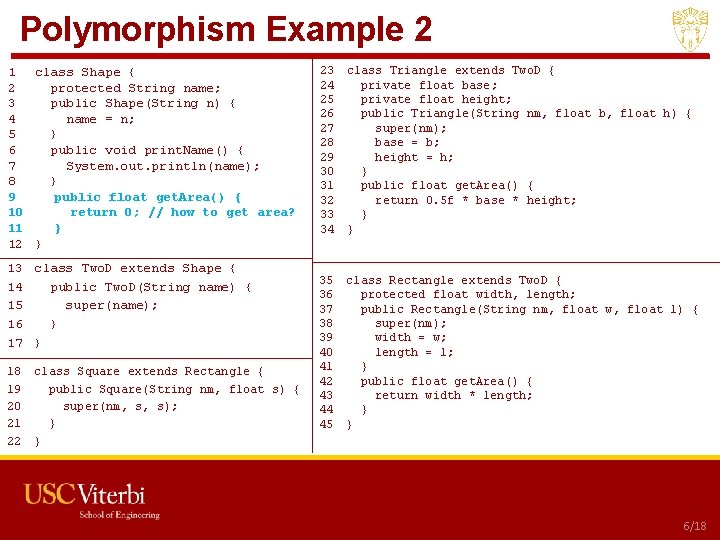
Polymorphism Example 2 1 class Shape { 2 protected String name; 3 public Shape(String n) { 4 name = n; 5 } 6 public void print. Name() { 7 System. out. println(name); 8 } 9 public float get. Area() { 10 return 0; // how to get area? 11 } 12 } 13 class Two. D extends Shape { 14 public Two. D(String name) { 15 super(name); 16 } 17 } 18 19 20 21 22 class Square extends Rectangle { public Square(String nm, float s) { super(nm, s, s); } } 23 24 25 26 27 28 29 30 31 32 33 34 class Triangle extends Two. D { private float base; private float height; public Triangle(String nm, float b, float h) { super(nm); base = b; height = h; } public float get. Area() { return 0. 5 f * base * height; } } 35 36 37 38 39 40 41 42 43 44 45 class Rectangle extends Two. D { protected float width, length; public Rectangle(String nm, float w, float l) { super(nm); width = w; length = l; } public float get. Area() { return width * length; } } USC CSCI 201 L 6/18
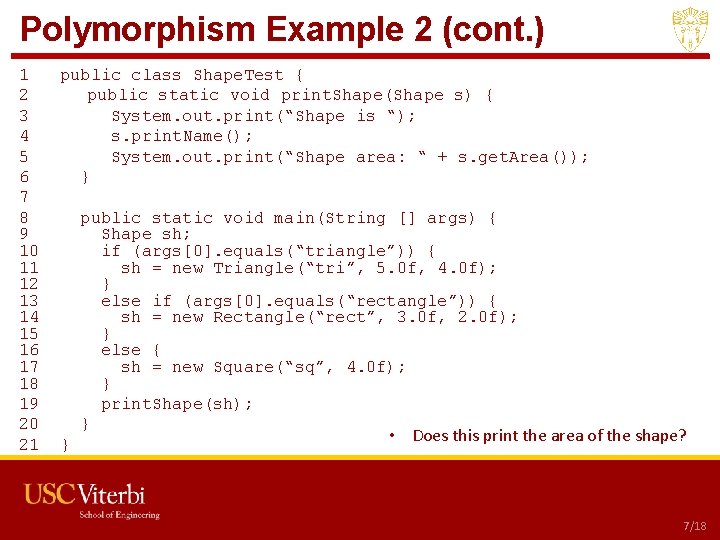
Polymorphism Example 2 (cont. ) 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 • public class Shape. Test { public static void print. Shape(Shape s) { System. out. print(“Shape is “); s. print. Name(); System. out. print(“Shape area: “ + s. get. Area()); } public static void main(String [] args) { Shape sh; if (args[0]. equals(“triangle”)) { sh = new Triangle(“tri”, 5. 0 f, 4. 0 f); } else if (args[0]. equals(“rectangle”)) { sh = new Rectangle(“rect”, 3. 0 f, 2. 0 f); } else { sh = new Square(“sq”, 4. 0 f); } print. Shape(sh); } } Polymorphism • Does this print the area of the shape? USC CSCI 201 L 7/18
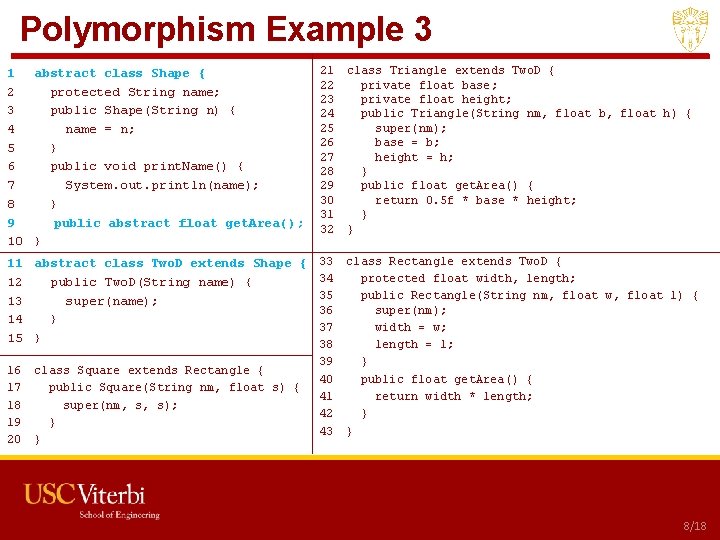
Polymorphism Example 3 1 abstract class Shape { 2 protected String name; 3 public Shape(String n) { 4 name = n; 5 } 6 public void print. Name() { 7 System. out. println(name); 8 } 9 public abstract float get. Area(); 10 } 21 22 23 24 25 26 27 28 29 30 31 32 class Triangle extends Two. D { private float base; private float height; public Triangle(String nm, float b, float h) { super(nm); base = b; height = h; } public float get. Area() { return 0. 5 f * base * height; } } 11 abstract class Two. D extends Shape { 33 class Rectangle extends Two. D { 34 protected float width, length; 12 public Two. D(String name) { 35 public Rectangle(String nm, float w, float l) { 13 super(name); 36 super(nm); 14 } 37 width = w; 15 } 38 length = l; 16 17 18 19 20 class Square extends Rectangle { public Square(String nm, float s) { super(nm, s, s); } } • Polymorphism 39 40 41 42 43 } public float get. Area() { return width * length; } } USC CSCI 201 L 8/18
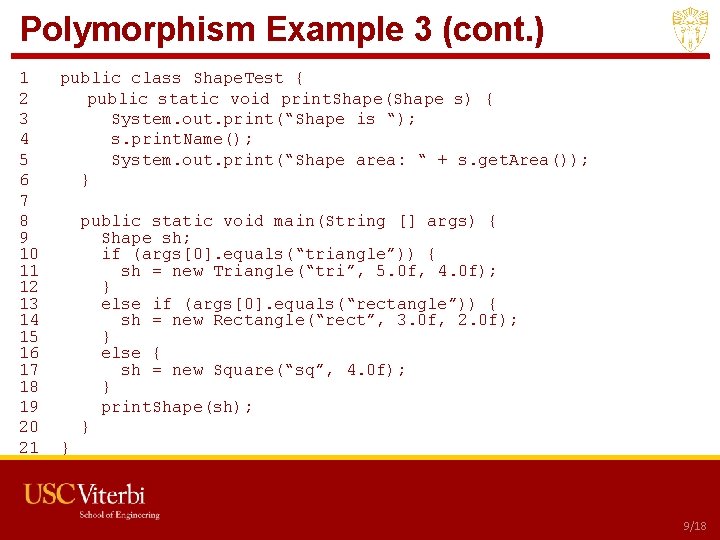
Polymorphism Example 3 (cont. ) 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 • public class Shape. Test { public static void print. Shape(Shape s) { System. out. print(“Shape is “); s. print. Name(); System. out. print(“Shape area: “ + s. get. Area()); } public static void main(String [] args) { Shape sh; if (args[0]. equals(“triangle”)) { sh = new Triangle(“tri”, 5. 0 f, 4. 0 f); } else if (args[0]. equals(“rectangle”)) { sh = new Rectangle(“rect”, 3. 0 f, 2. 0 f); } else { sh = new Square(“sq”, 4. 0 f); } print. Shape(sh); } } Polymorphism USC CSCI 201 L 9/18
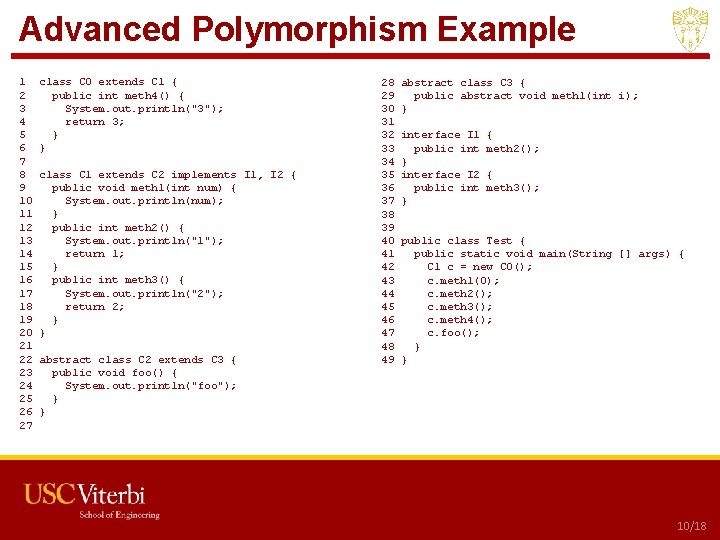
Advanced Polymorphism Example 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 • class C 0 extends C 1 { public int meth 4() { System. out. println("3"); return 3; } } class C 1 extends C 2 implements I 1, I 2 { public void meth 1(int num) { System. out. println(num); } public int meth 2() { System. out. println("1"); return 1; } public int meth 3() { System. out. println("2"); return 2; } } abstract class C 2 extends C 3 { public void foo() { System. out. println("foo"); } } Polymorphism 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 abstract class C 3 { public abstract void meth 1(int i); } interface I 1 public int } interface I 2 public int } { meth 2(); { meth 3(); public class Test { public static void main(String [] args) { C 1 c = new C 0(); c. meth 1(0); c. meth 2(); c. meth 3(); c. meth 4(); c. foo(); } } USC CSCI 201 L 10/18
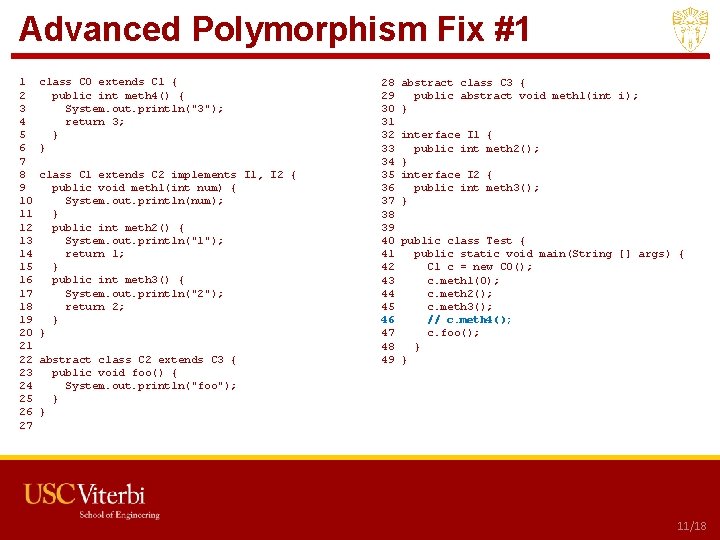
Advanced Polymorphism Fix #1 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 • class C 0 extends C 1 { public int meth 4() { System. out. println("3"); return 3; } } class C 1 extends C 2 implements I 1, I 2 { public void meth 1(int num) { System. out. println(num); } public int meth 2() { System. out. println("1"); return 1; } public int meth 3() { System. out. println("2"); return 2; } } abstract class C 2 extends C 3 { public void foo() { System. out. println("foo"); } } Polymorphism 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 abstract class C 3 { public abstract void meth 1(int i); } interface I 1 public int } interface I 2 public int } { meth 2(); { meth 3(); public class Test { public static void main(String [] args) { C 1 c = new C 0(); c. meth 1(0); c. meth 2(); c. meth 3(); // c. meth 4(); c. foo(); } } USC CSCI 201 L 11/18
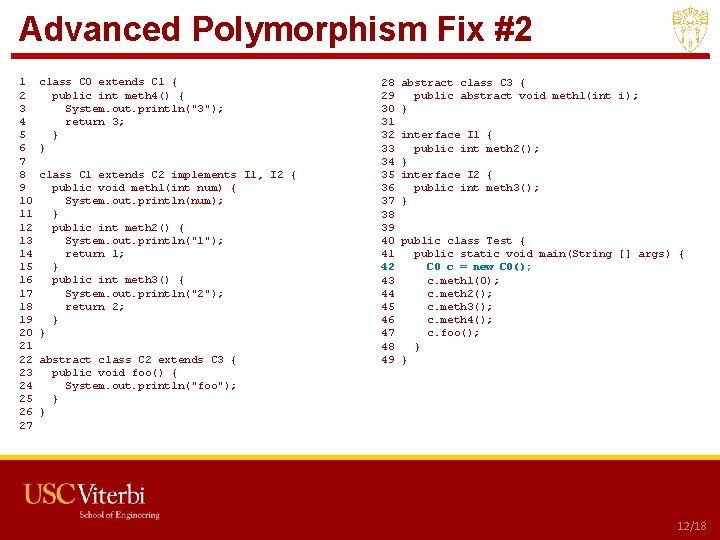
Advanced Polymorphism Fix #2 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 • class C 0 extends C 1 { public int meth 4() { System. out. println("3"); return 3; } } class C 1 extends C 2 implements I 1, I 2 { public void meth 1(int num) { System. out. println(num); } public int meth 2() { System. out. println("1"); return 1; } public int meth 3() { System. out. println("2"); return 2; } } abstract class C 2 extends C 3 { public void foo() { System. out. println("foo"); } } Polymorphism 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 abstract class C 3 { public abstract void meth 1(int i); } interface I 1 public int } interface I 2 public int } { meth 2(); { meth 3(); public class Test { public static void main(String [] args) { C 0 c = new C 0(); c. meth 1(0); c. meth 2(); c. meth 3(); c. meth 4(); c. foo(); } } USC CSCI 201 L 12/18
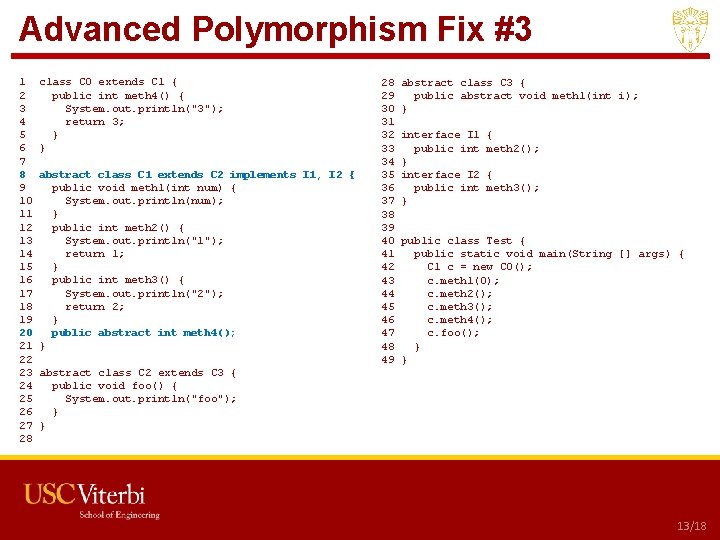
Advanced Polymorphism Fix #3 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 • class C 0 extends C 1 { public int meth 4() { System. out. println("3"); return 3; } } abstract class C 1 extends C 2 implements I 1, I 2 { public void meth 1(int num) { System. out. println(num); } public int meth 2() { System. out. println("1"); return 1; } public int meth 3() { System. out. println("2"); return 2; } public abstract int meth 4(); } 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 abstract class C 3 { public abstract void meth 1(int i); } interface I 1 public int } interface I 2 public int } { meth 2(); { meth 3(); public class Test { public static void main(String [] args) { C 1 c = new C 0(); c. meth 1(0); c. meth 2(); c. meth 3(); c. meth 4(); c. foo(); } } abstract class C 2 extends C 3 { public void foo() { System. out. println("foo"); } } Polymorphism USC CSCI 201 L 13/18
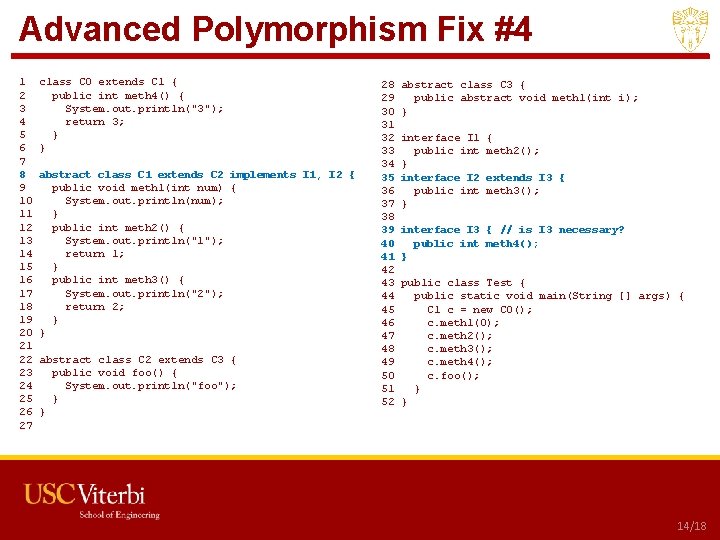
Advanced Polymorphism Fix #4 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 • class C 0 extends C 1 { public int meth 4() { System. out. println("3"); return 3; } } abstract class C 1 extends C 2 implements I 1, I 2 { public void meth 1(int num) { System. out. println(num); } public int meth 2() { System. out. println("1"); return 1; } public int meth 3() { System. out. println("2"); return 2; } } abstract class C 2 extends C 3 { public void foo() { System. out. println("foo"); } } Polymorphism 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 abstract class C 3 { public abstract void meth 1(int i); } interface I 1 public int } interface I 2 public int } { meth 2(); extends I 3 { meth 3(); interface I 3 { // is I 3 necessary? public int meth 4(); } public class Test { public static void main(String [] args) { C 1 c = new C 0(); c. meth 1(0); c. meth 2(); c. meth 3(); c. meth 4(); c. foo(); } } USC CSCI 201 L 14/18
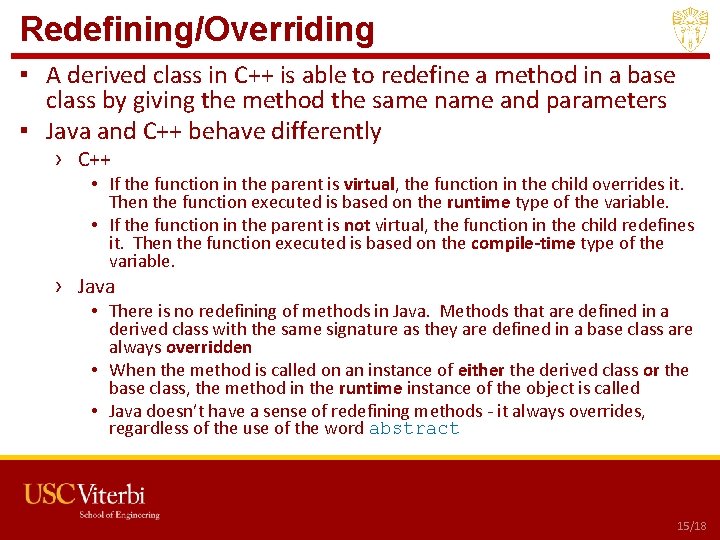
Redefining/Overriding ▪ A derived class in C++ is able to redefine a method in a base class by giving the method the same name and parameters ▪ Java and C++ behave differently › C++ • If the function in the parent is virtual, the function in the child overrides it. Then the function executed is based on the runtime type of the variable. • If the function in the parent is not virtual, the function in the child redefines it. Then the function executed is based on the compile-time type of the variable. › Java • There is no redefining of methods in Java. Methods that are defined in a derived class with the same signature as they are defined in a base class are always overridden • When the method is called on an instance of either the derived class or the base class, the method in the runtime instance of the object is called • Java doesn’t have a sense of redefining methods - it always overrides, regardless of the use of the word abstract • Polymorphism USC CSCI 201 L 15/18
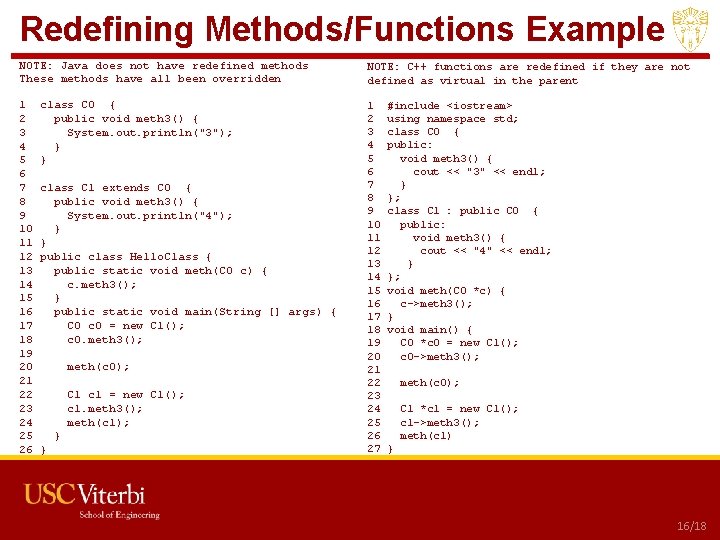
Redefining Methods/Functions Example • NOTE: Java does not have redefined methods These methods have all been overridden NOTE: C++ functions are redefined if they are not defined as virtual in the parent 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 class C 0 { public void meth 3() { System. out. println("3"); } } class C 1 extends C 0 { public void meth 3() { System. out. println("4"); } } public class Hello. Class { public static void meth(C 0 c) { c. meth 3(); } public static void main(String [] args) { C 0 c 0 = new C 1(); c 0. meth 3(); meth(c 0); C 1 c 1 = new C 1(); c 1. meth 3(); meth(c 1); } } Polymorphism #include <iostream> using namespace std; class C 0 { public: void meth 3() { cout << "3" << endl; } }; class C 1 : public C 0 { public: void meth 3() { cout << "4" << endl; } }; void meth(C 0 *c) { c->meth 3(); } void main() { C 0 *c 0 = new C 1(); c 0 ->meth 3(); meth(c 0); C 1 *c 1 = new C 1(); c 1 ->meth 3(); meth(c 1) } USC CSCI 201 L 16/18
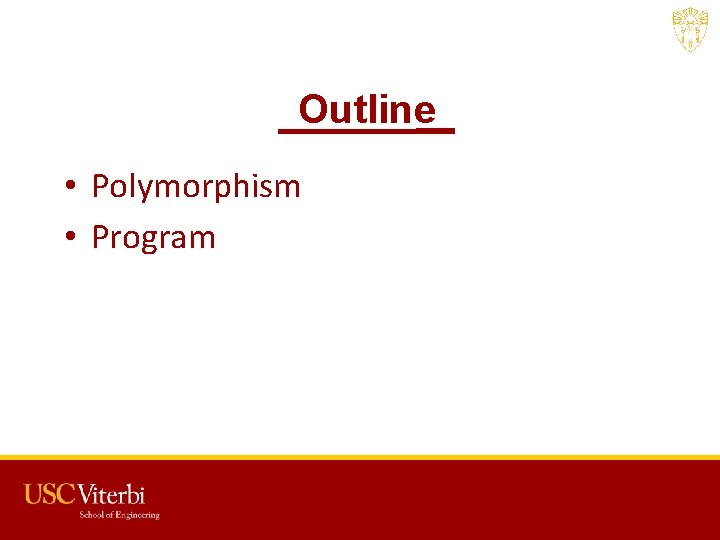
Outline • Polymorphism • Program USC CSCI 201 L
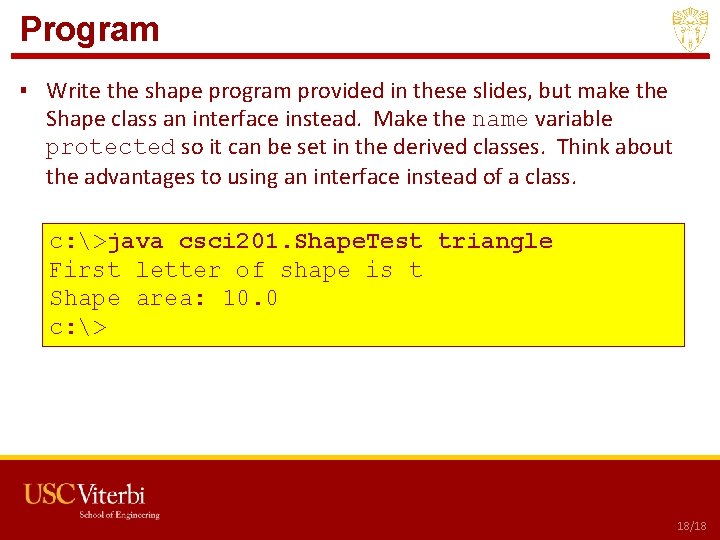
Program ▪ Write the shape program provided in these slides, but make the Shape class an interface instead. Make the name variable protected so it can be set in the derived classes. Think about the advantages to using an interface instead of a class. c: >java csci 201. Shape. Test triangle First letter of shape is t Shape area: 10. 0 c: > • Program USC CSCI 201 L 18/18