Pointers Chapter 9 Savitch Chapter 9 Gaddis Chapter
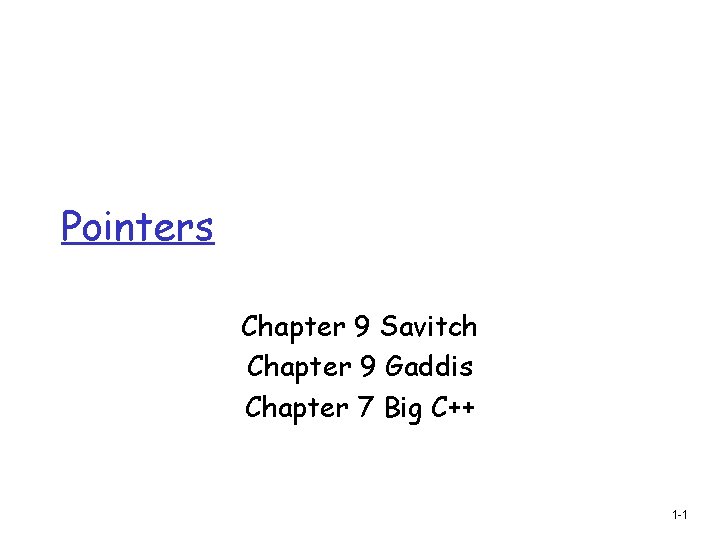
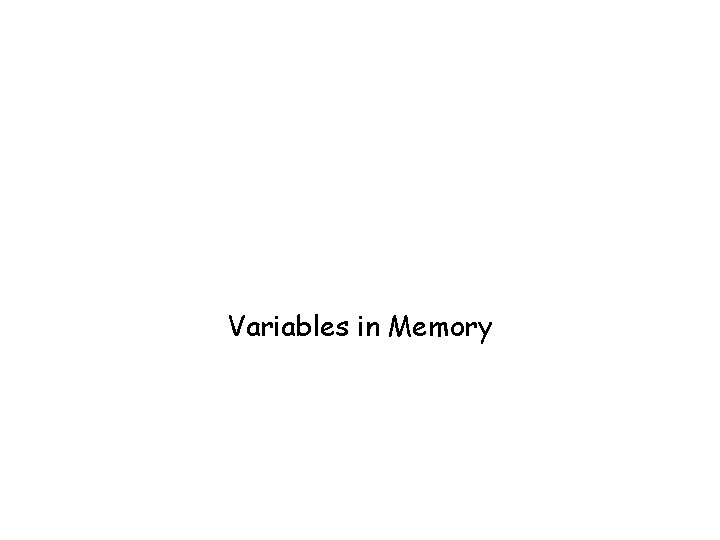
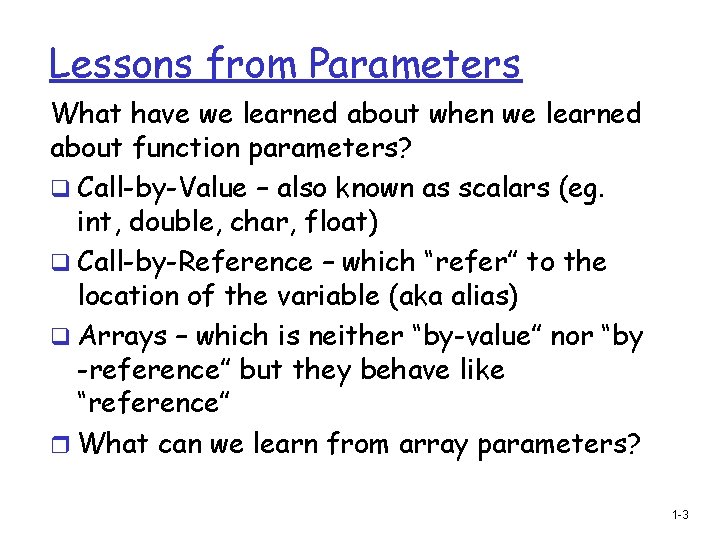
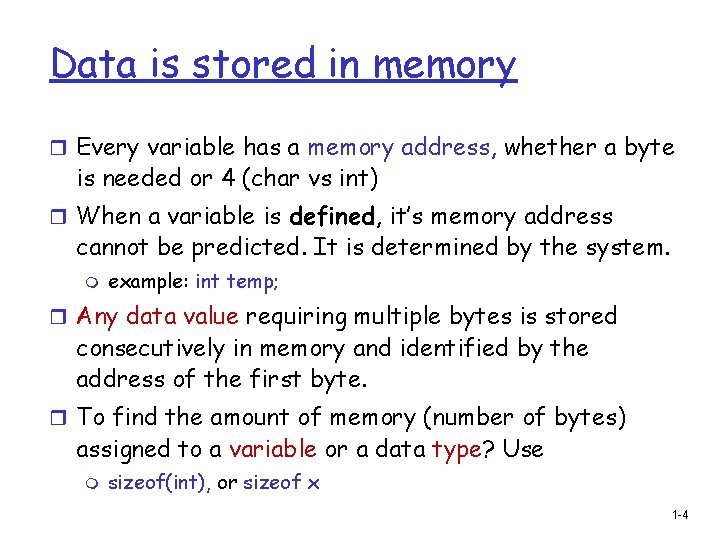
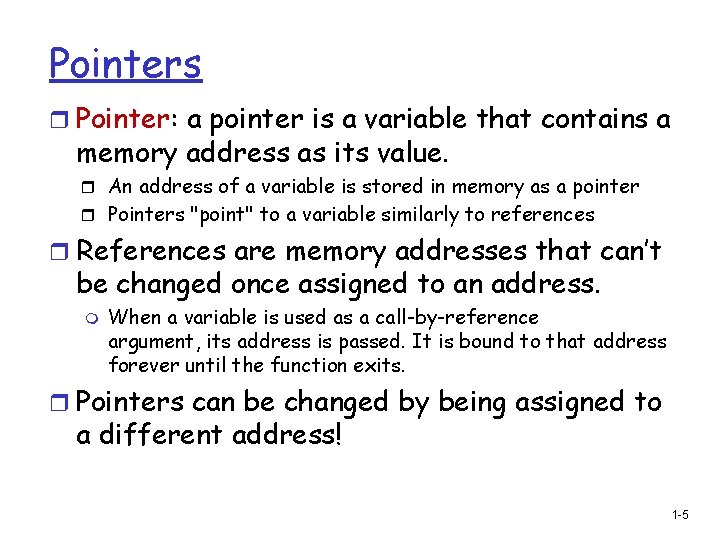
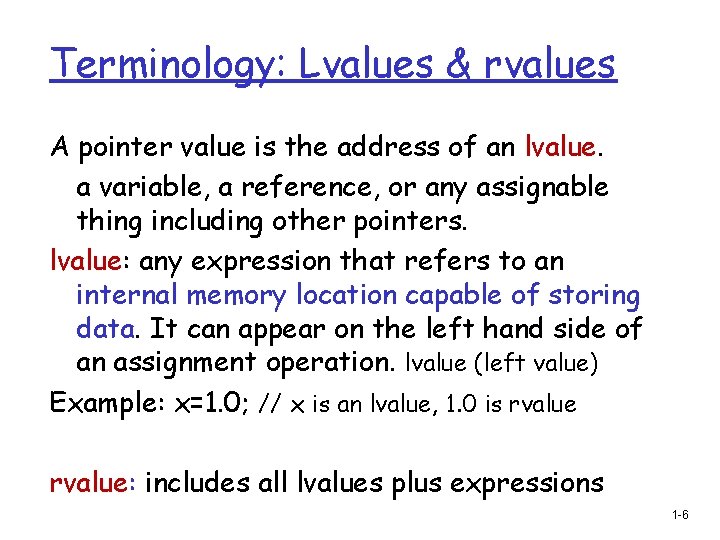
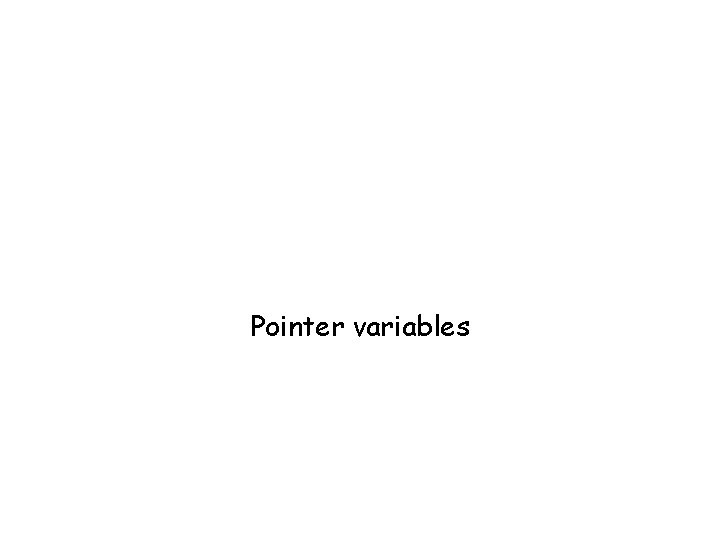
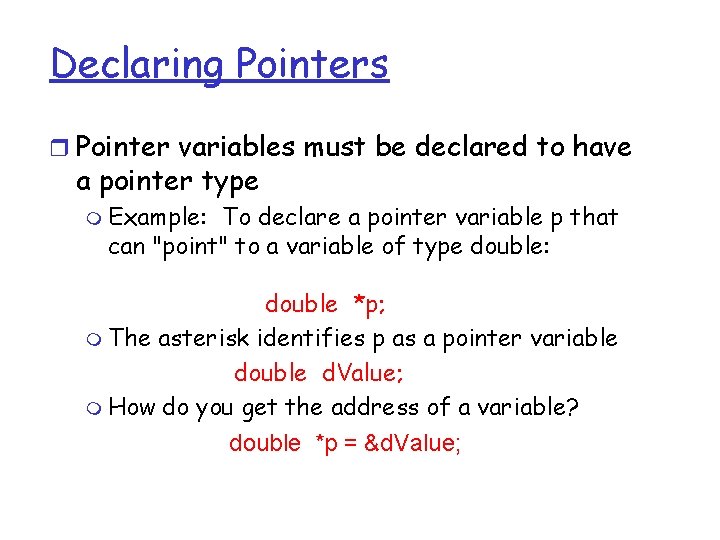
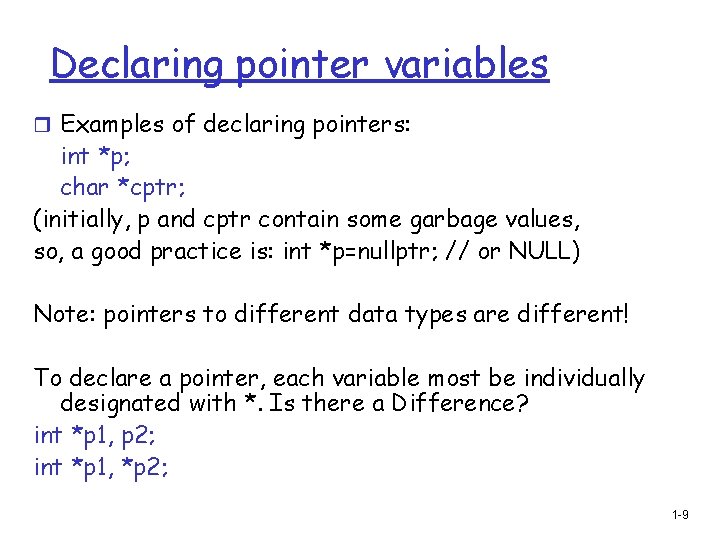
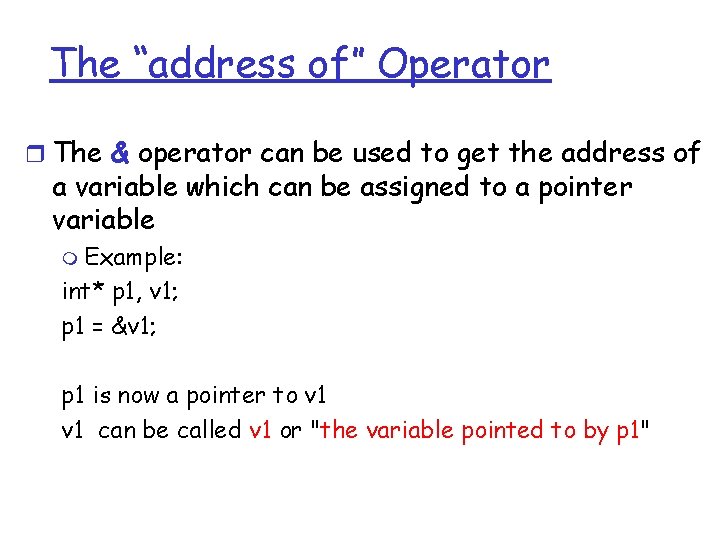
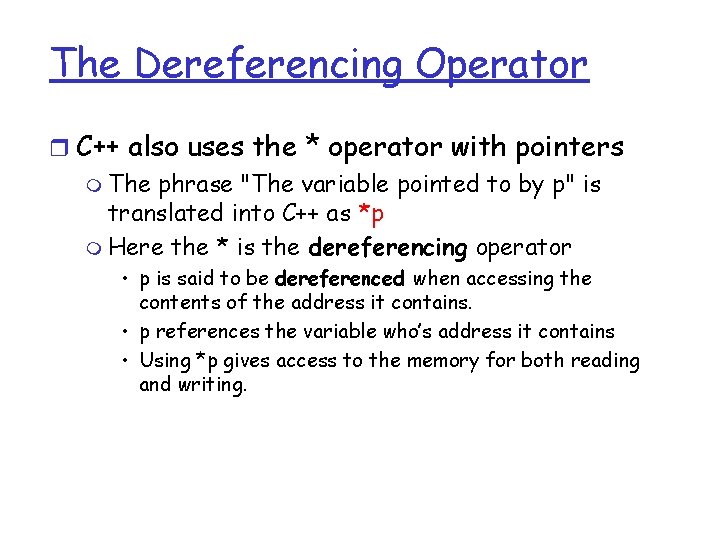
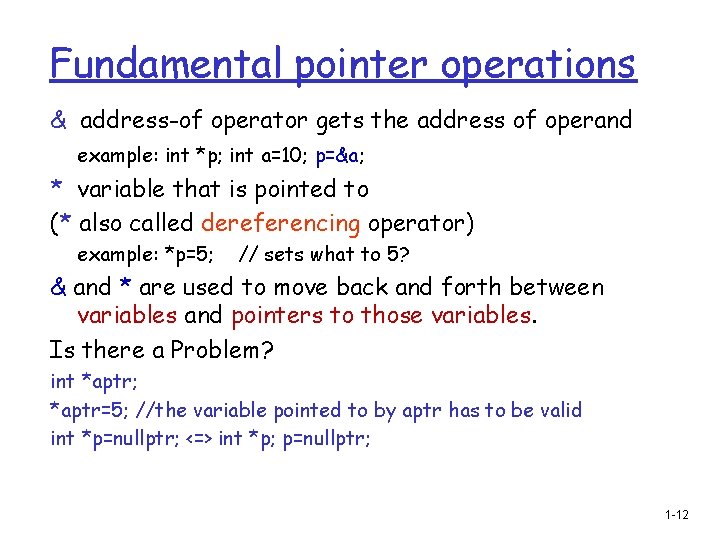
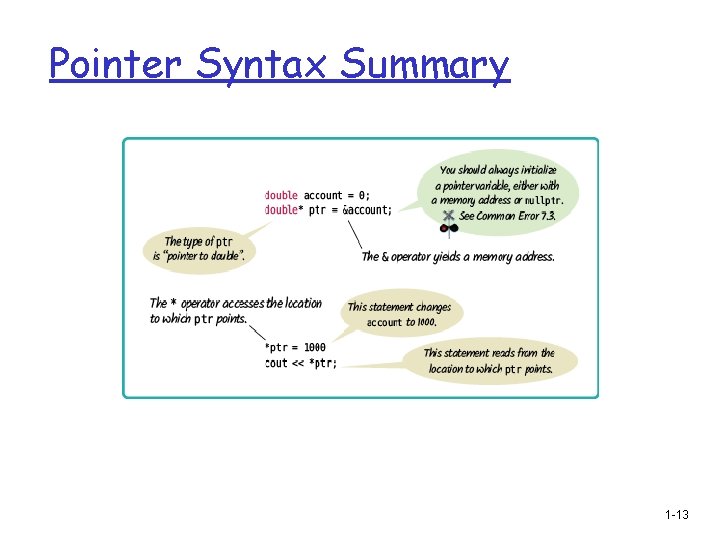
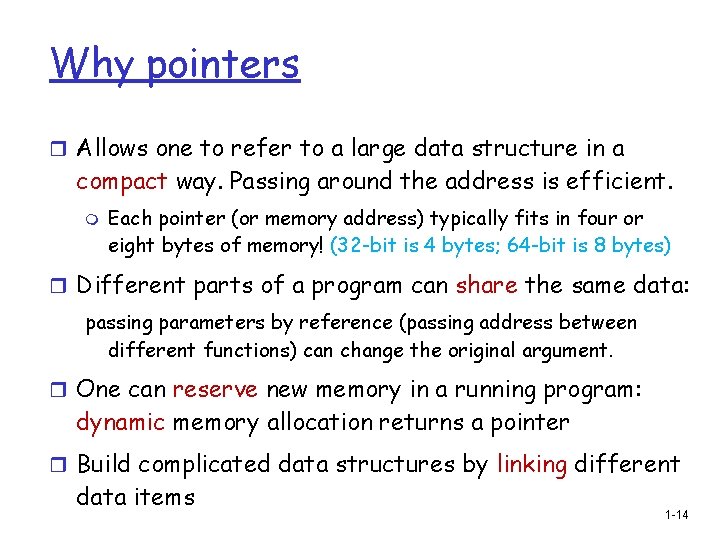
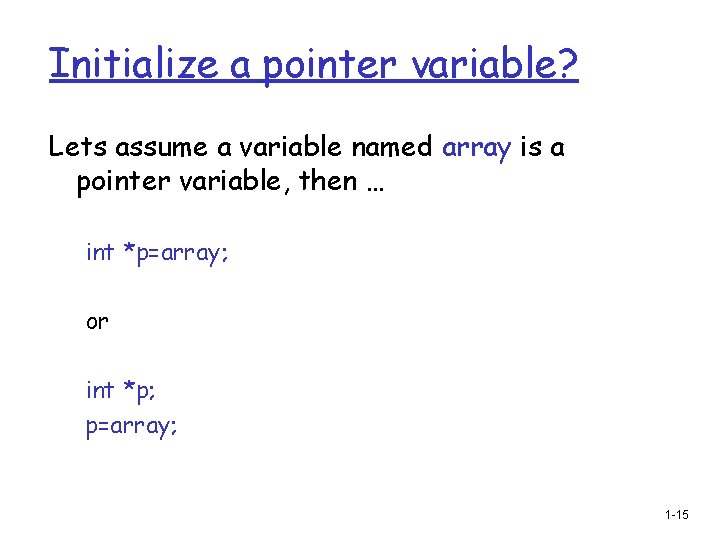
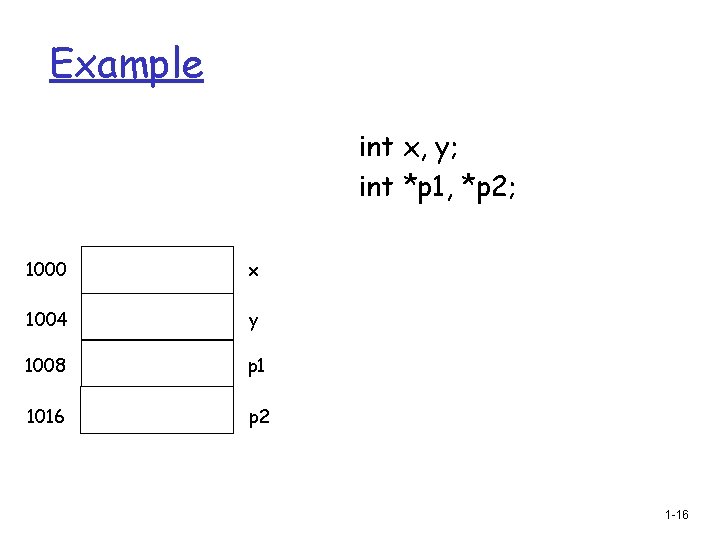
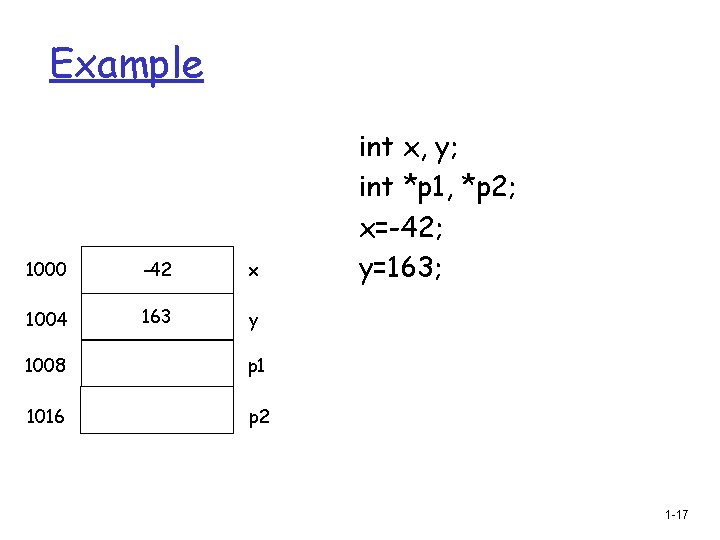
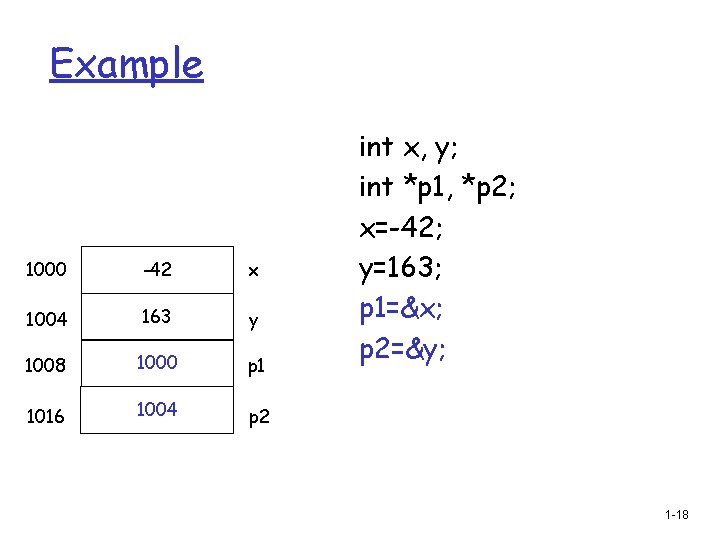
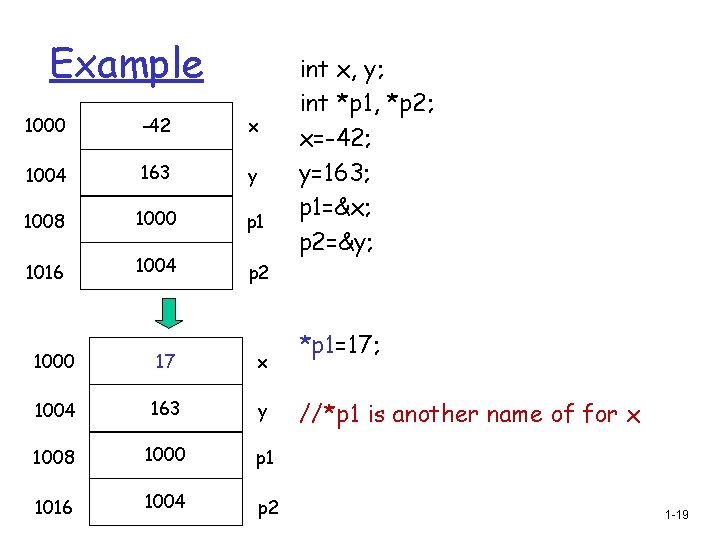
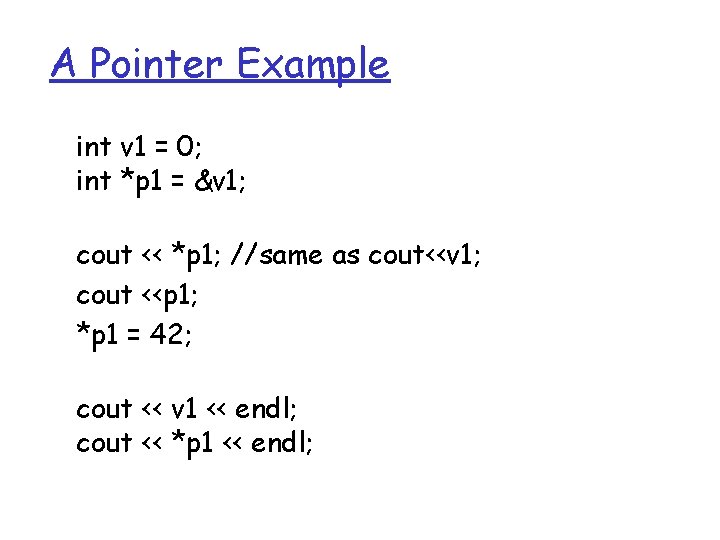
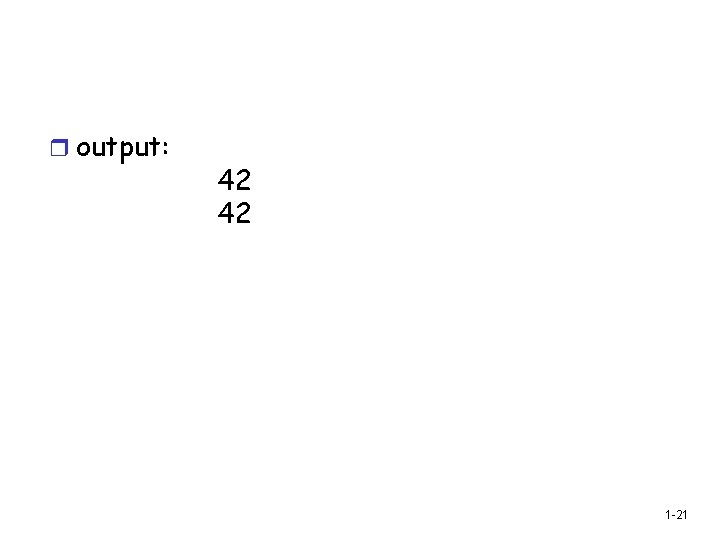
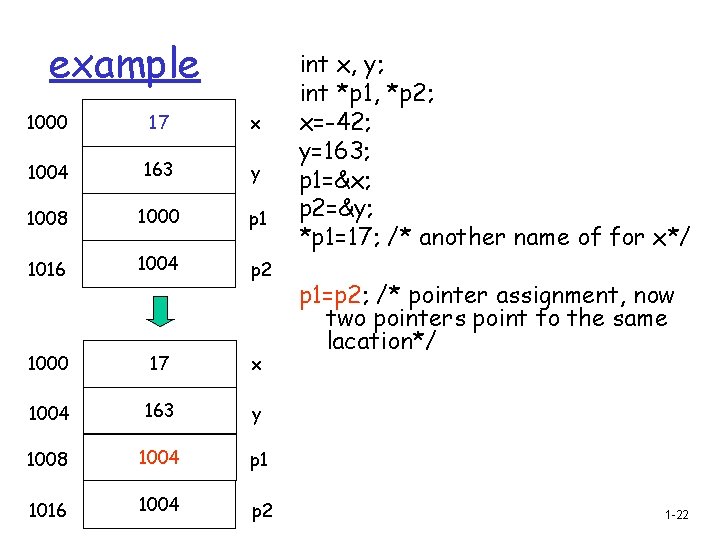
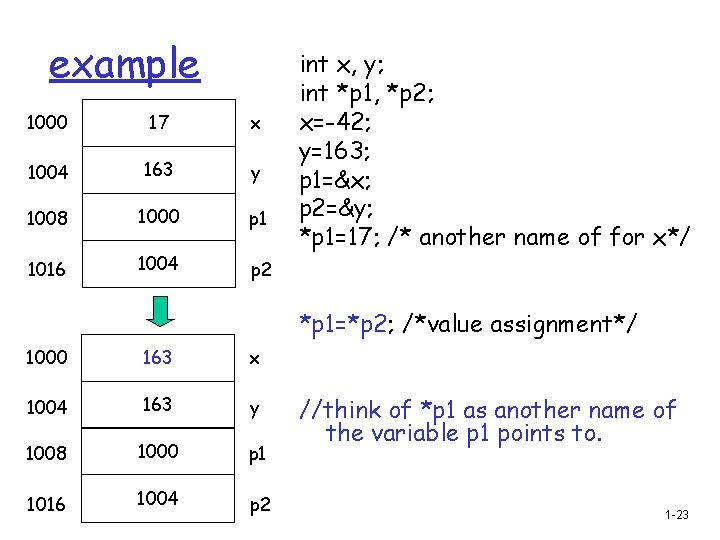
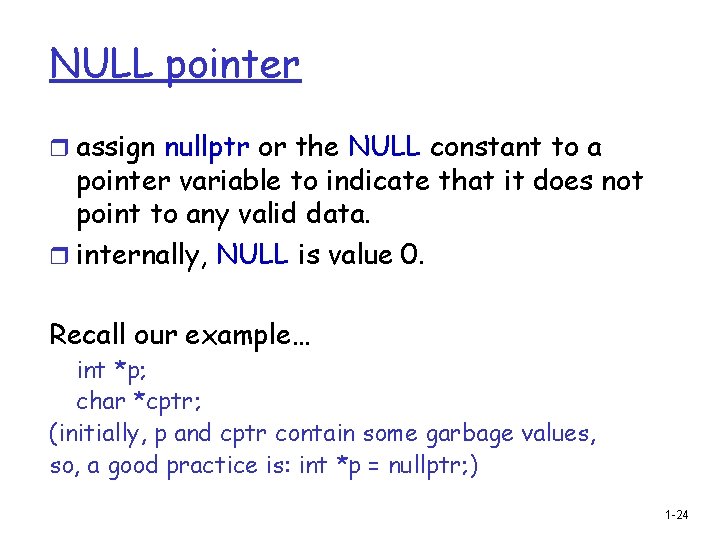
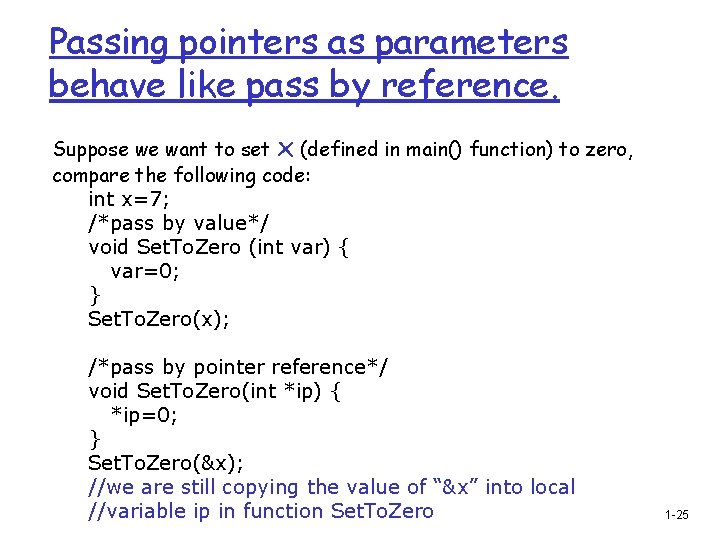
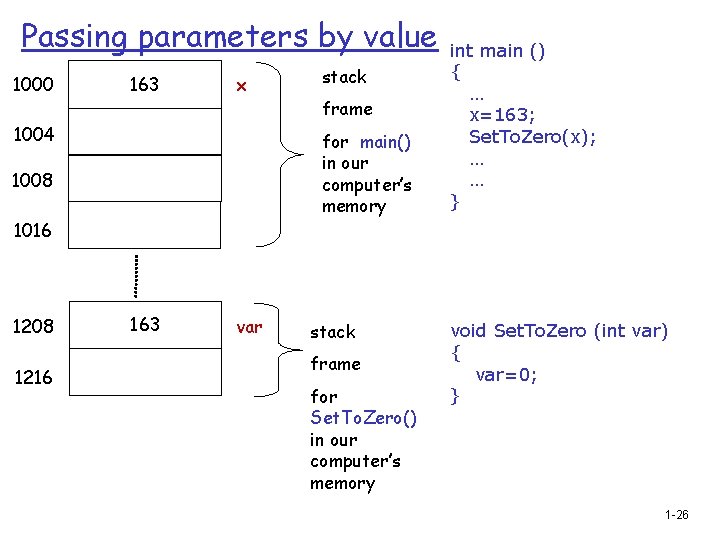
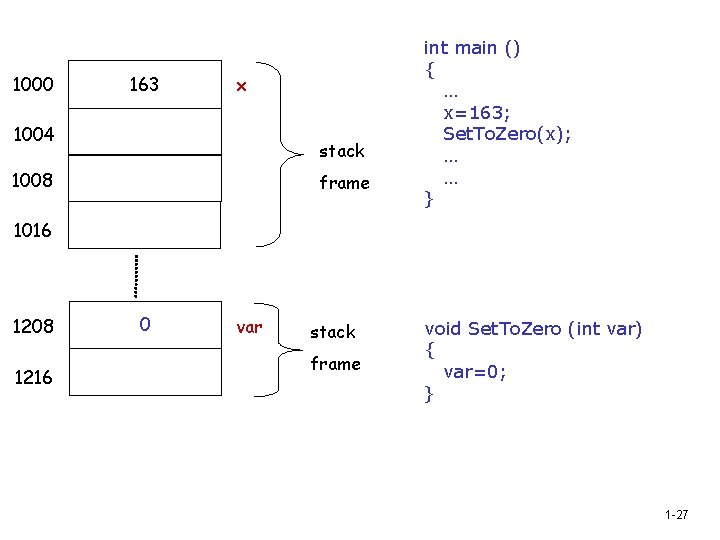
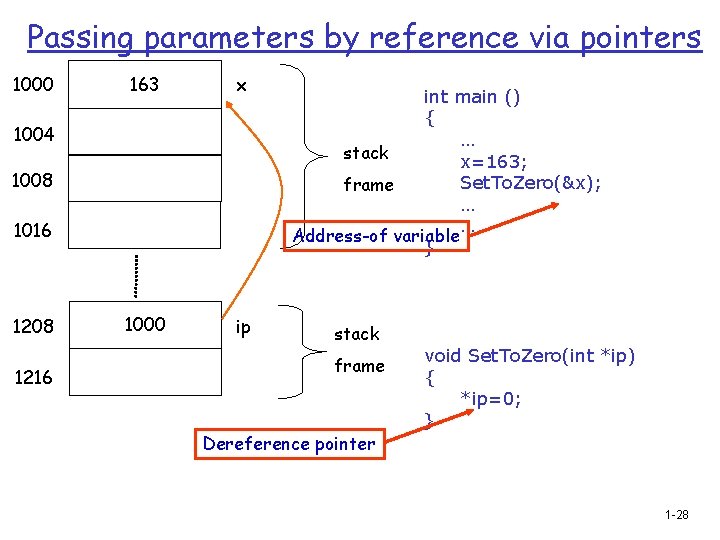
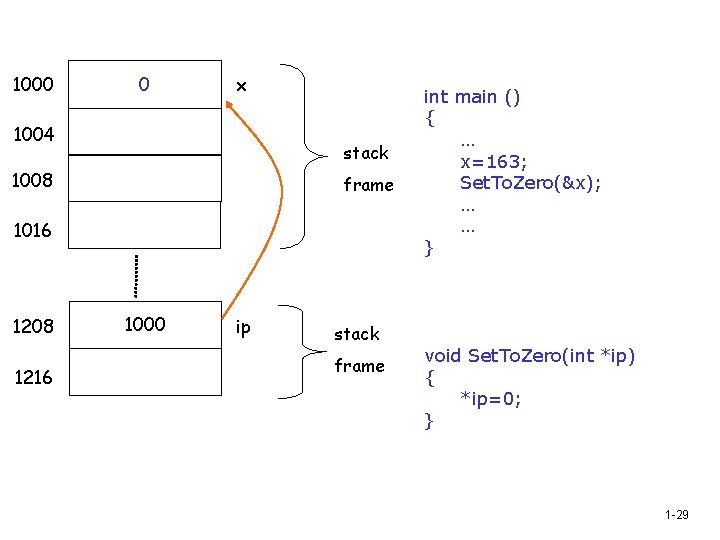
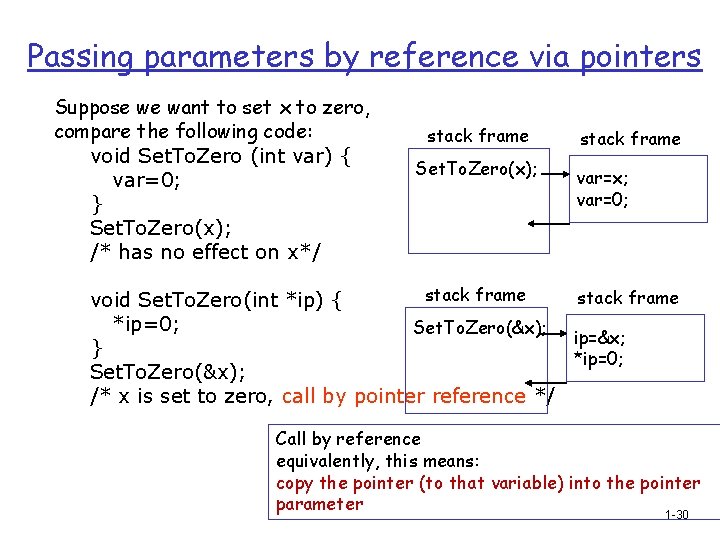
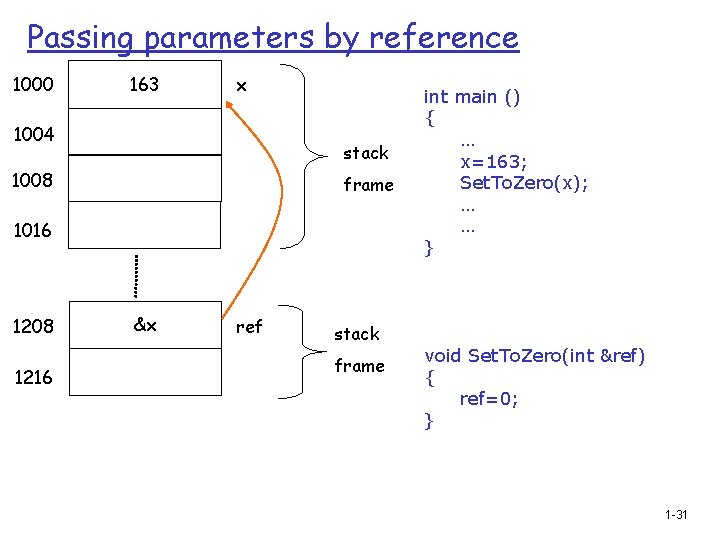
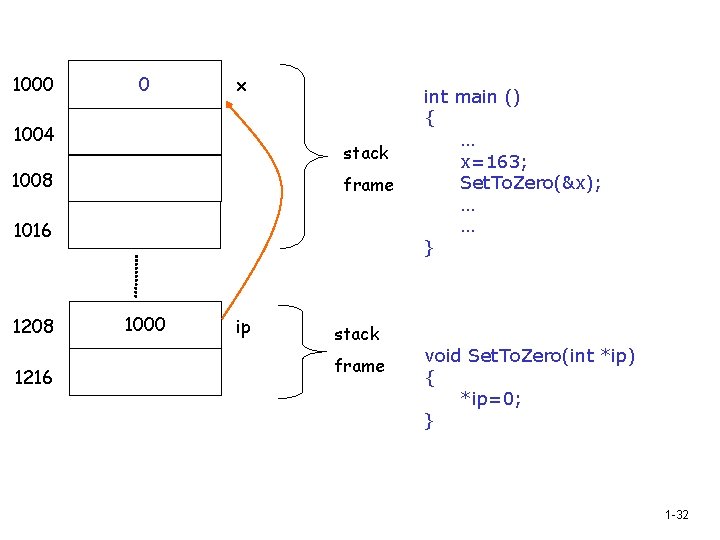
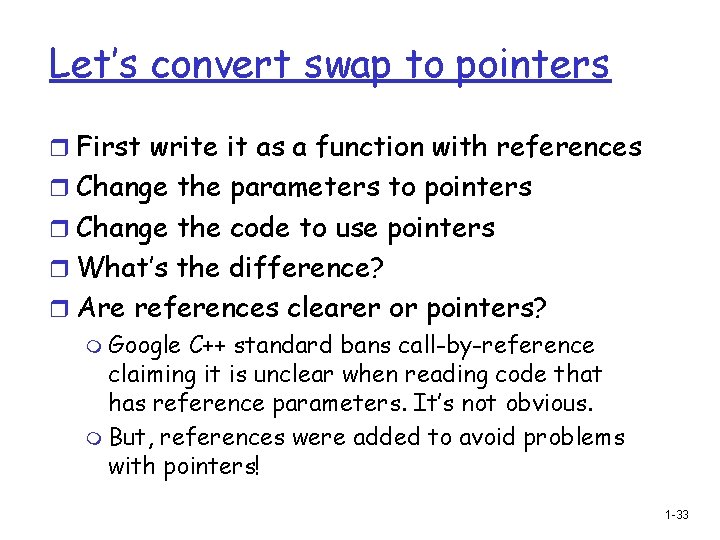
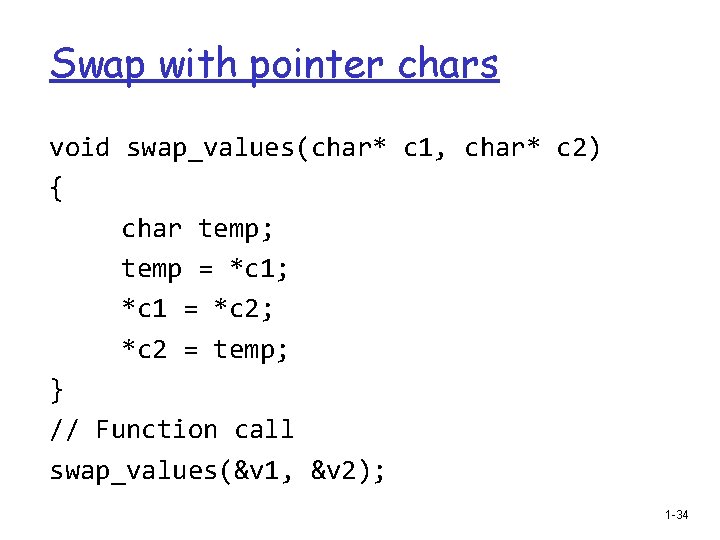
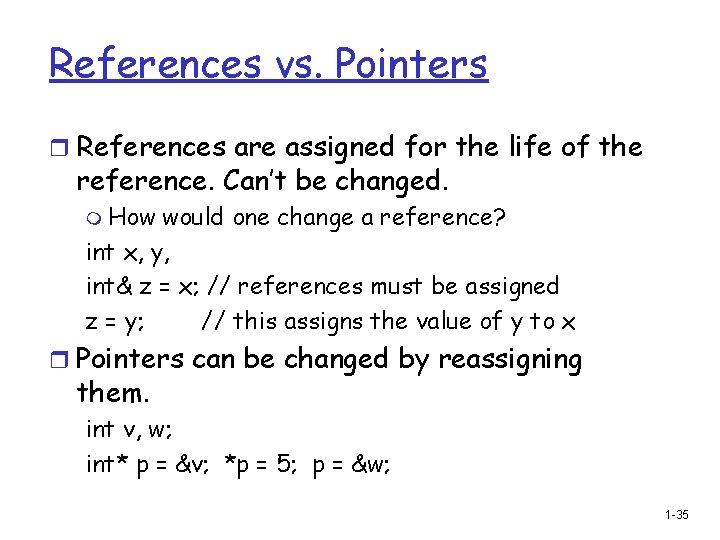
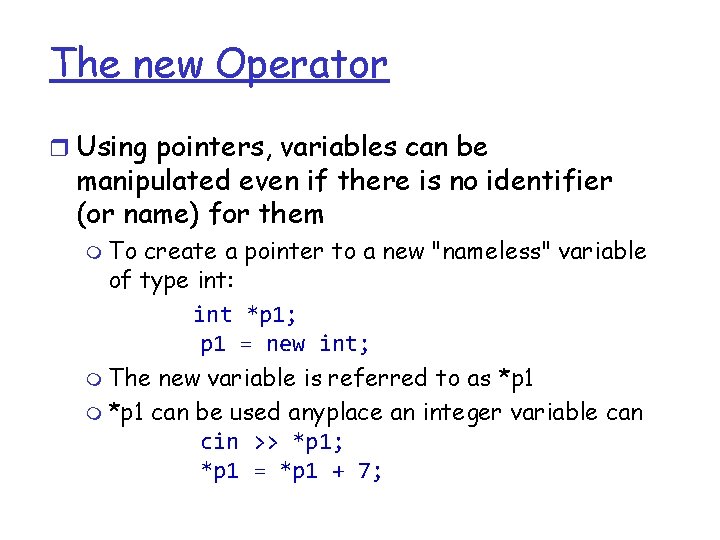
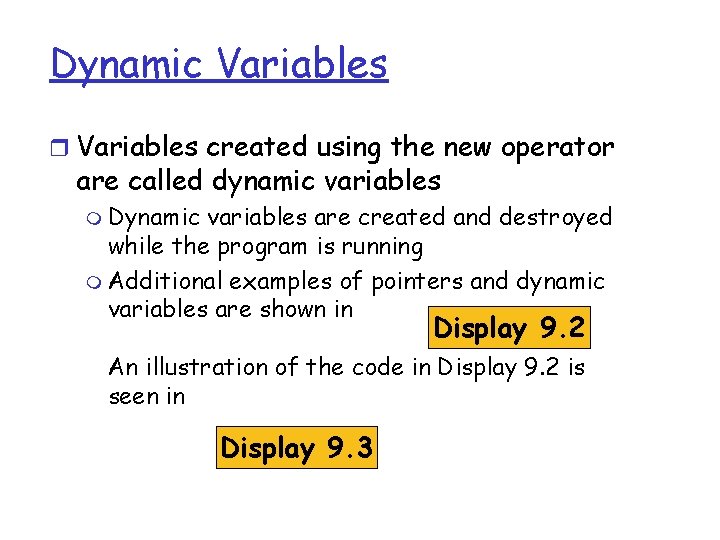
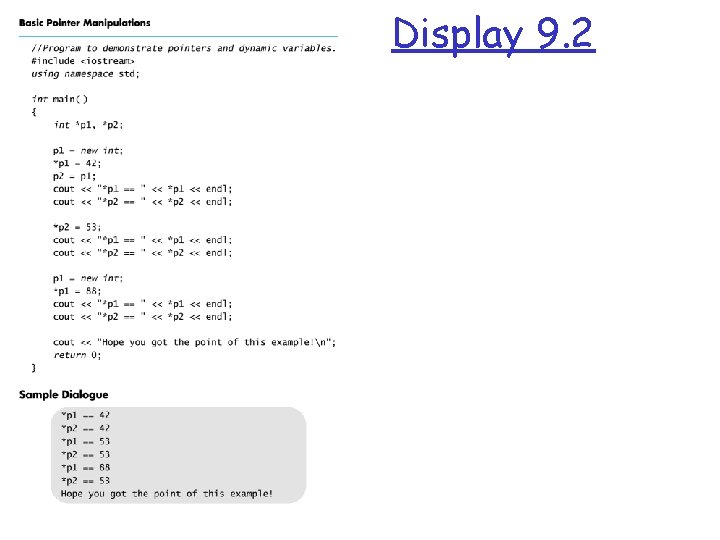
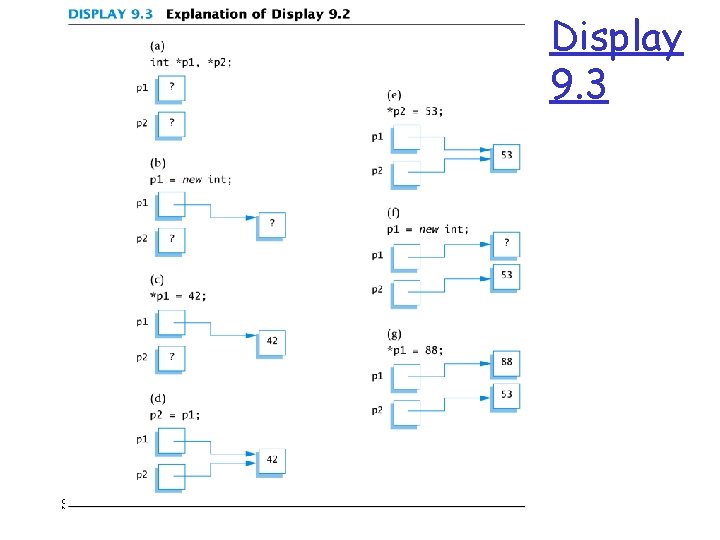
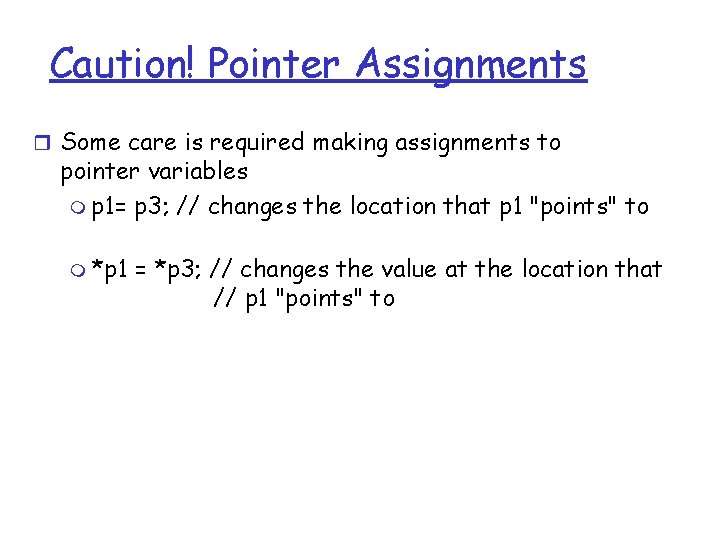
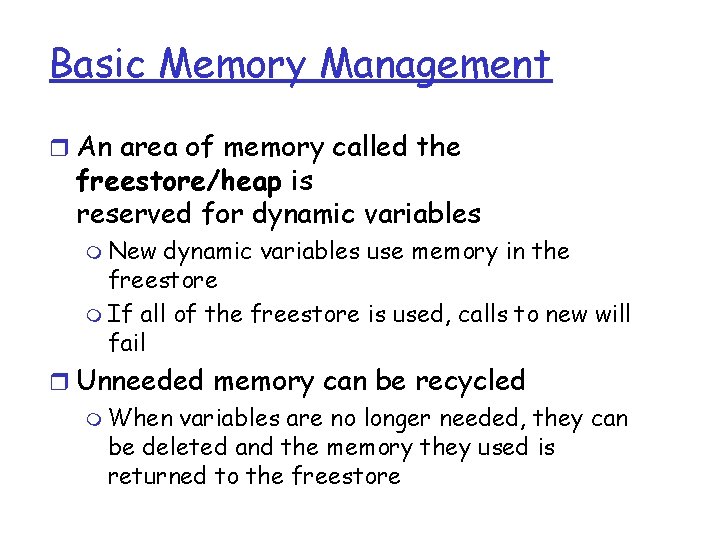
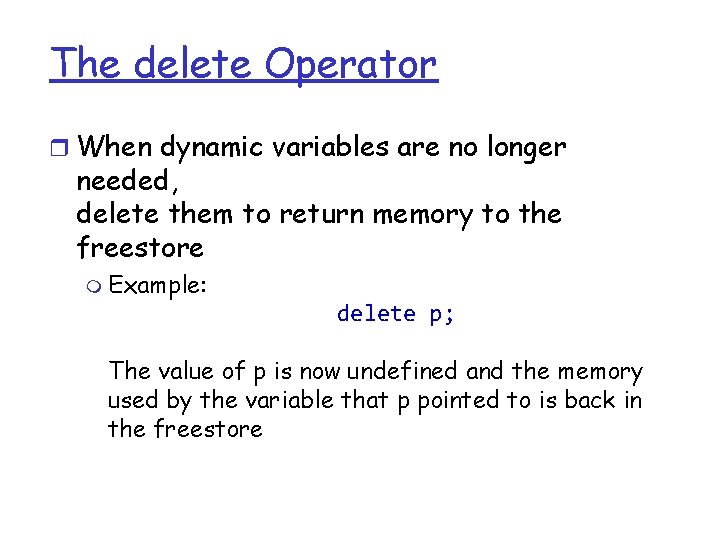
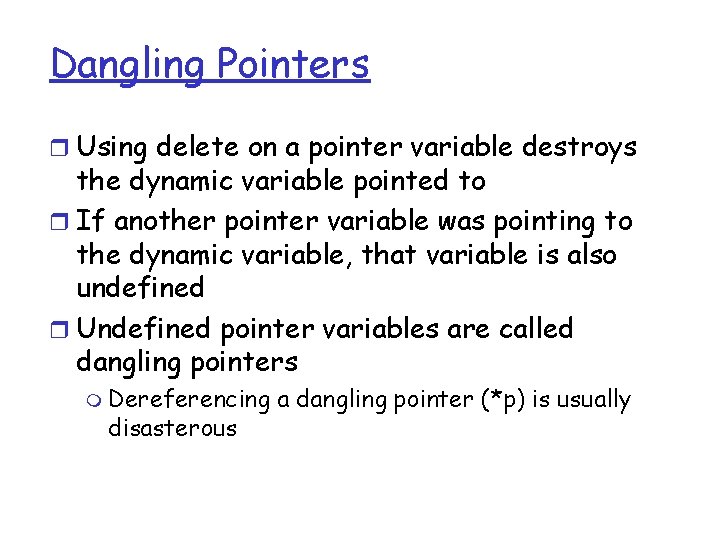
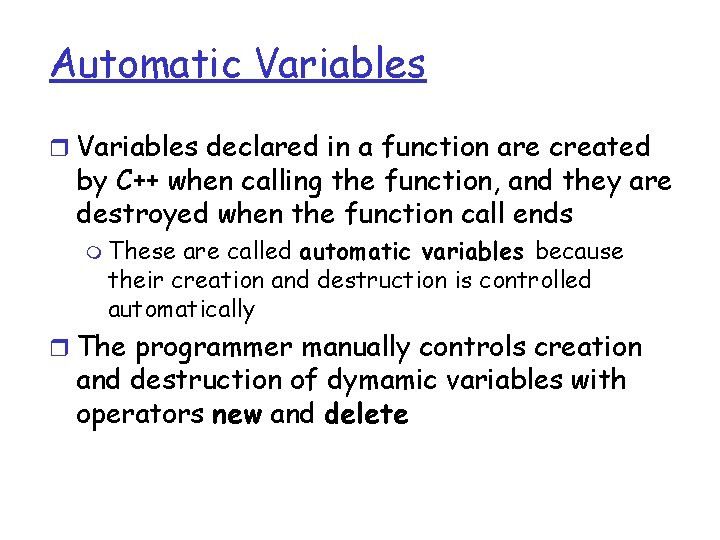
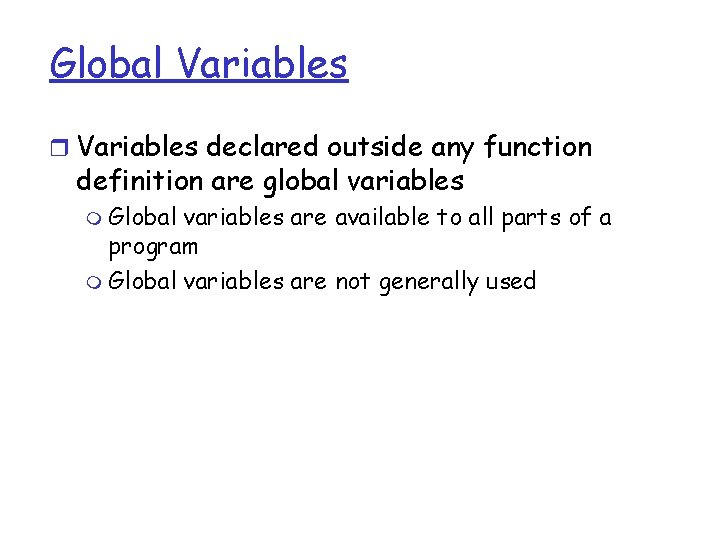
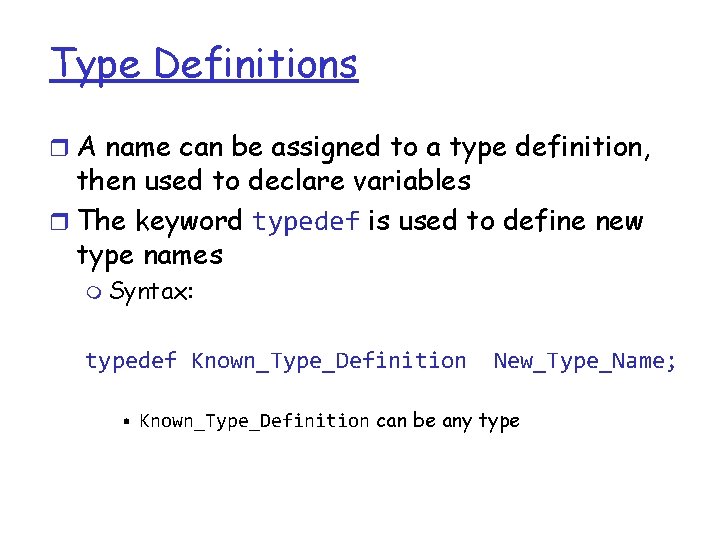
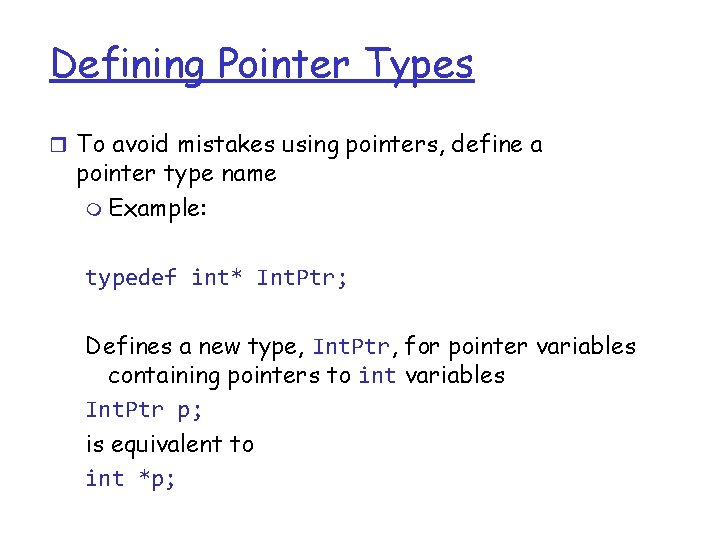
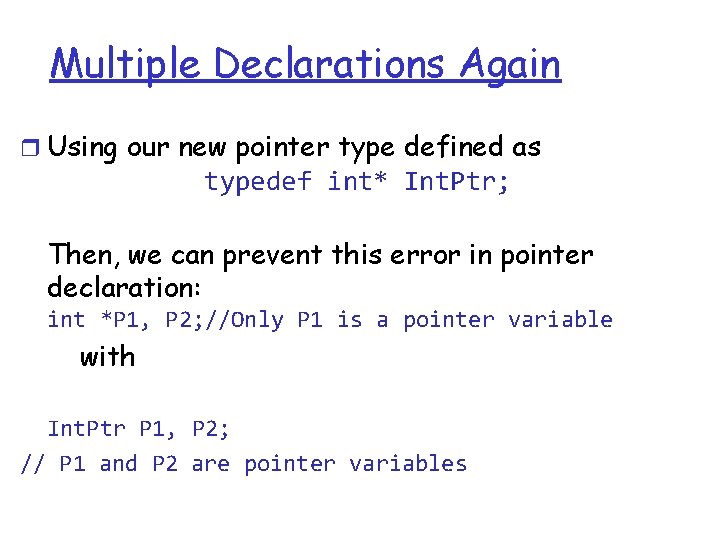
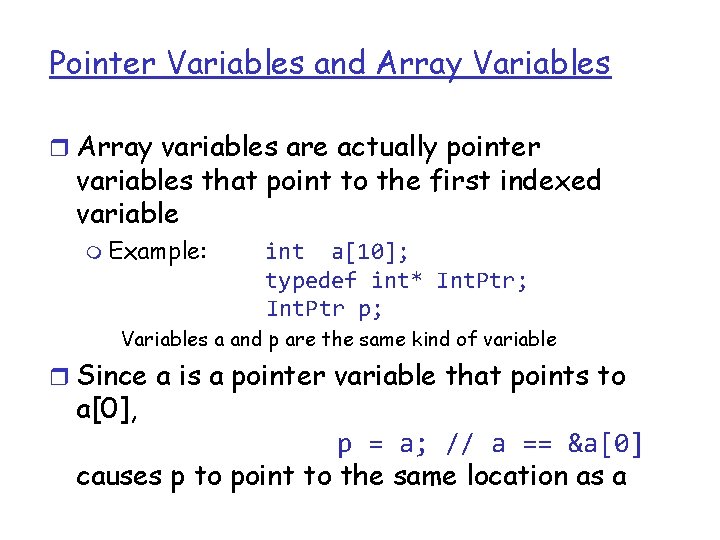
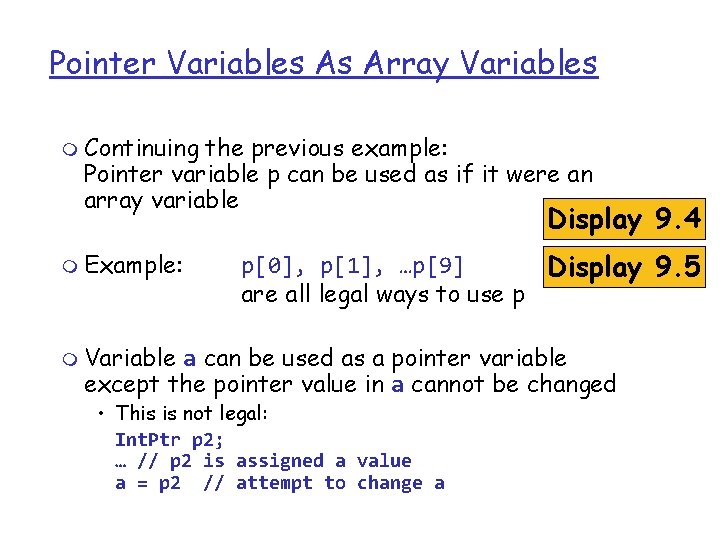
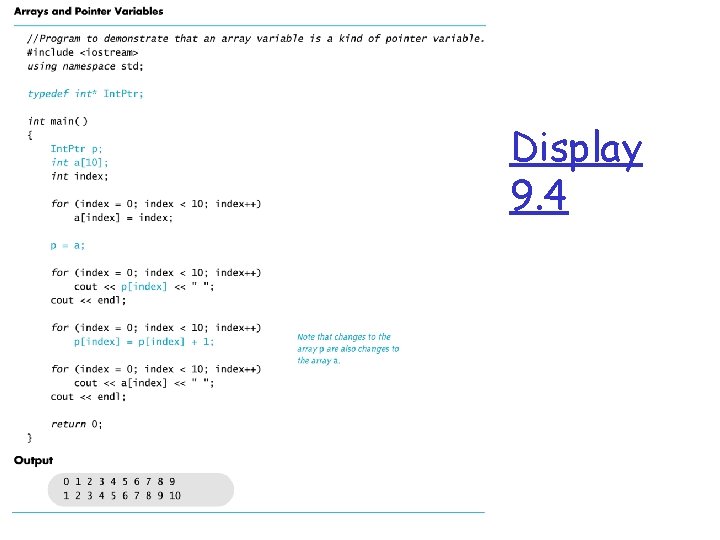
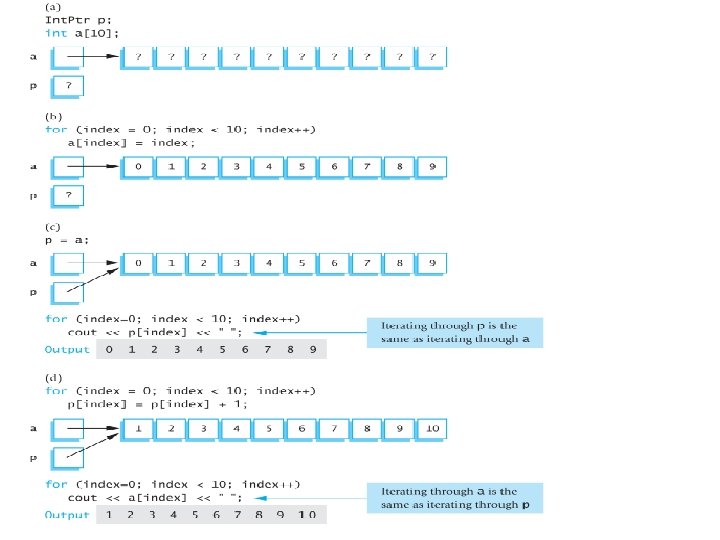
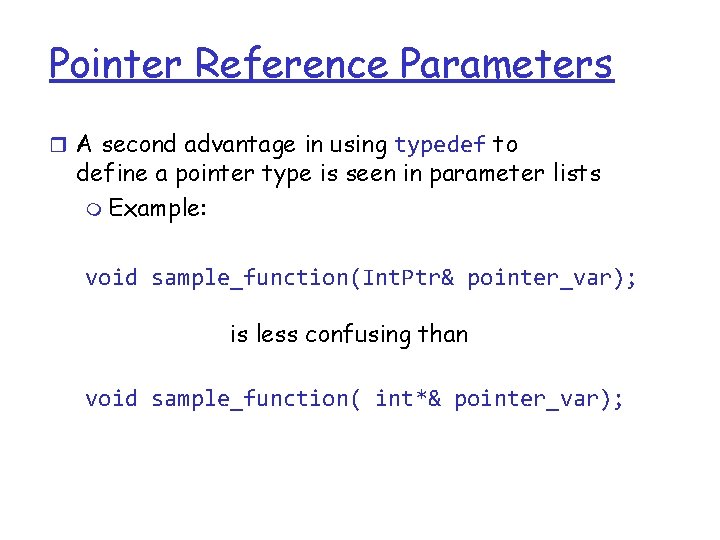
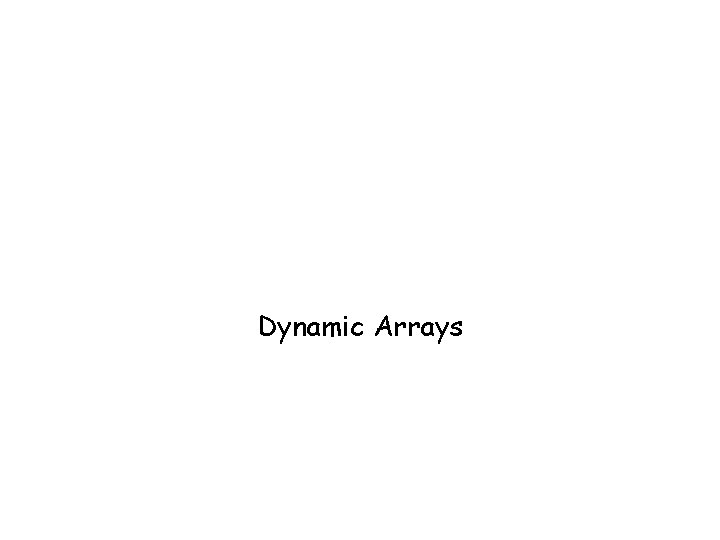
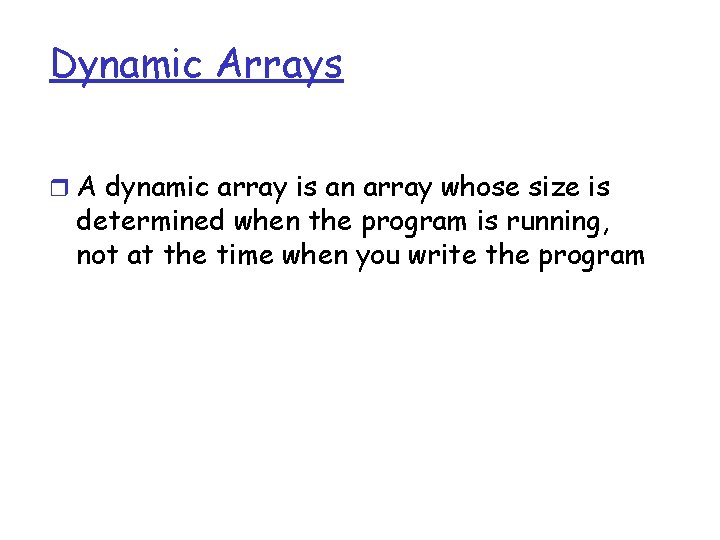
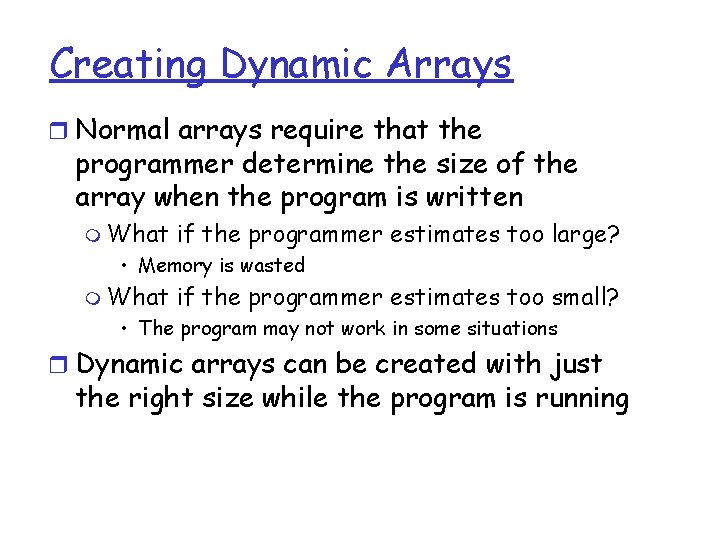
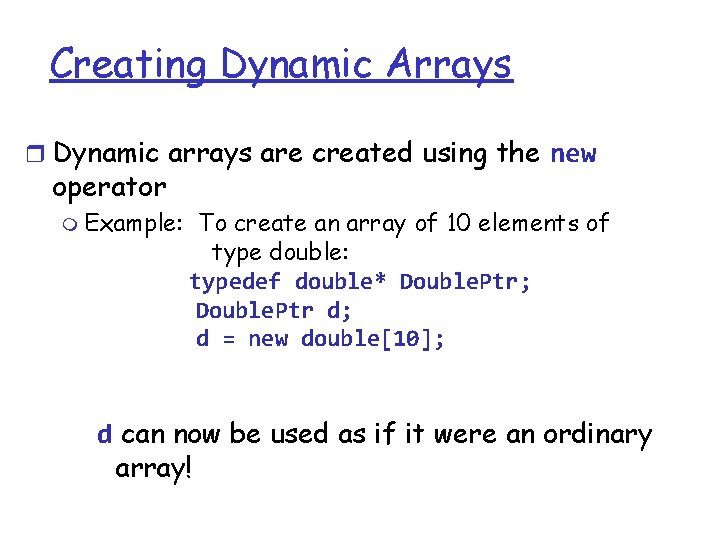
![Dynamic Arrays (cont. ) r Pointer variable d is a pointer to d[0] r Dynamic Arrays (cont. ) r Pointer variable d is a pointer to d[0] r](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-58.jpg)
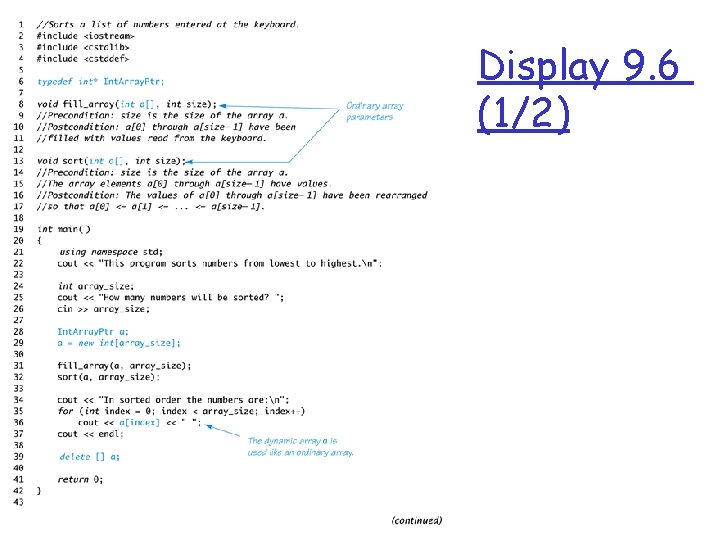
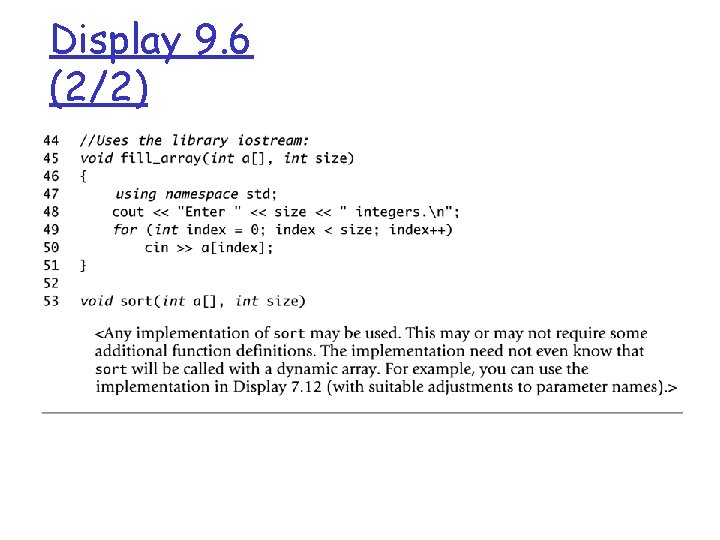
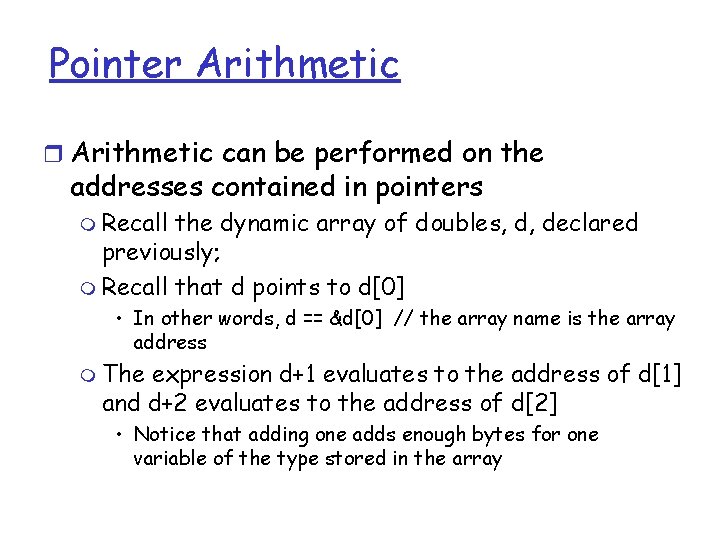
![pointers and arrays 1000 list[0] 1008 list[1] 1016 list[2] 1024 double list[3]; &list[1] ? pointers and arrays 1000 list[0] 1008 list[1] 1016 list[2] 1024 double list[3]; &list[1] ?](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-62.jpg)
![pointer arithmetic must take into account the size of the base type. double list[3]={1. pointer arithmetic must take into account the size of the base type. double list[3]={1.](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-63.jpg)
![pointer arithmetic must take into account the size of the base type. p=&list[0]; p=p+2; pointer arithmetic must take into account the size of the base type. p=&list[0]; p=p+2;](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-64.jpg)
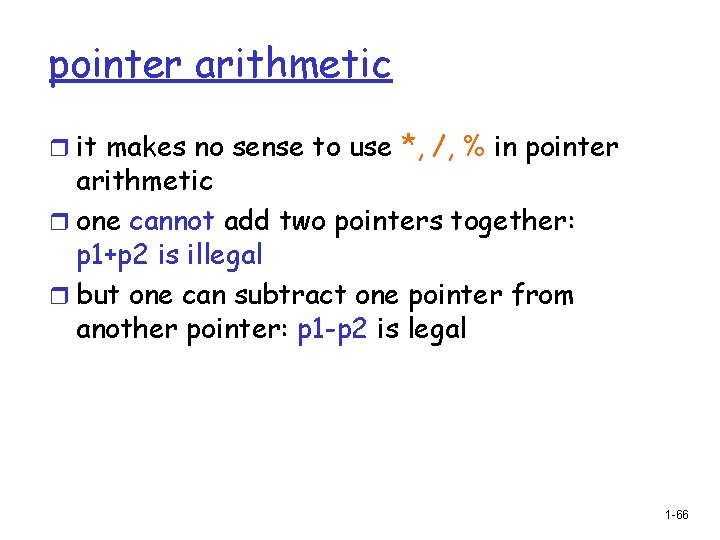
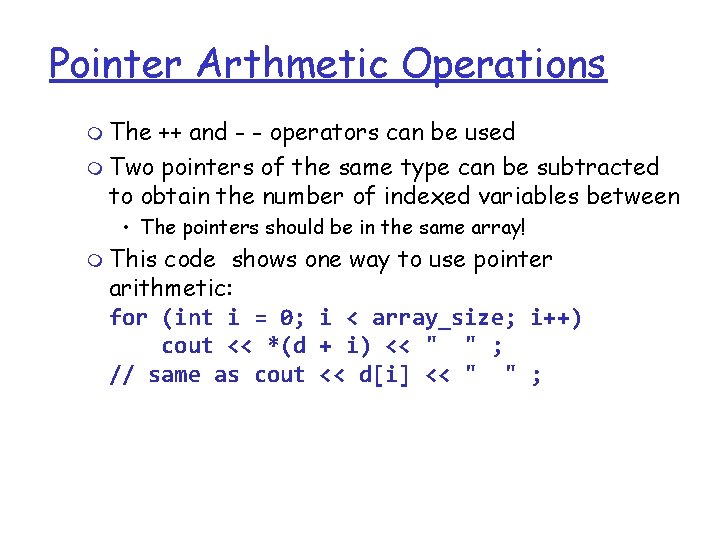
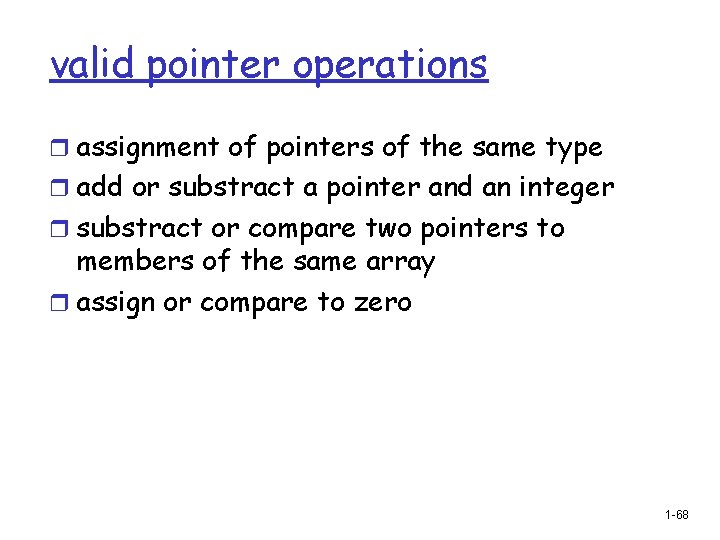
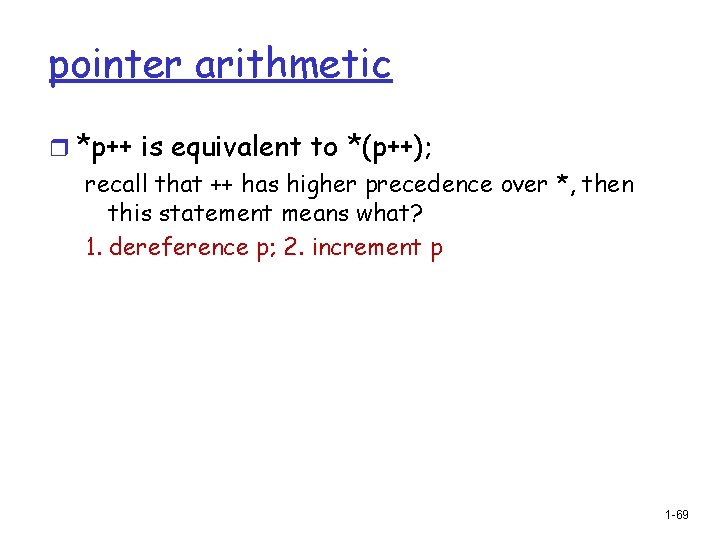
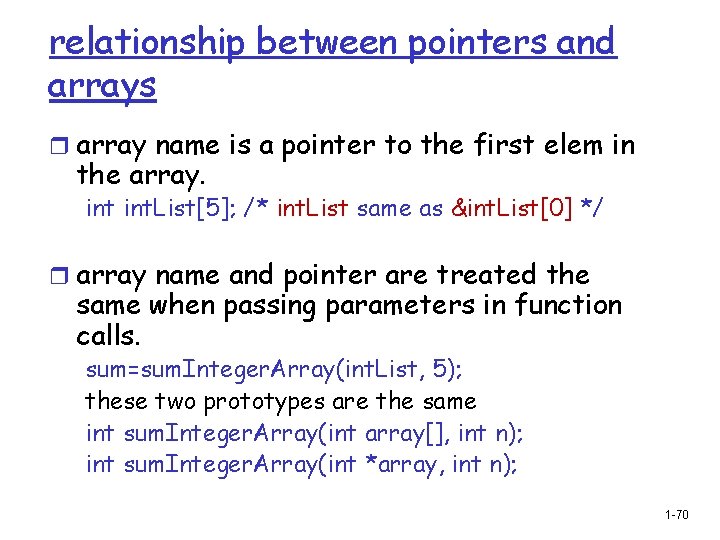
![differences between pointers and arrays declarations int array[5]; /*memory has been allocated for 5 differences between pointers and arrays declarations int array[5]; /*memory has been allocated for 5](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-70.jpg)
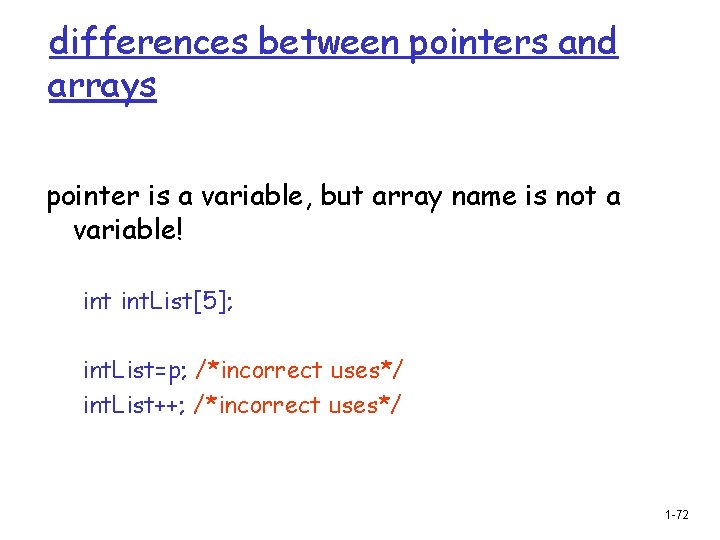
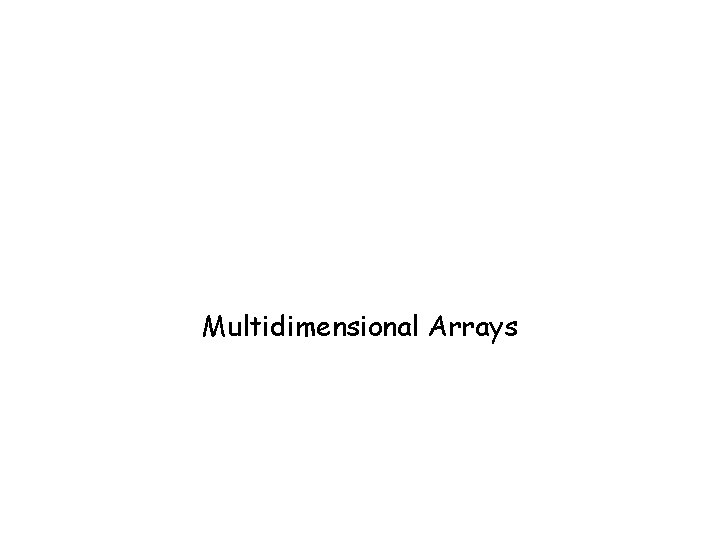
![Multi-Dimensional Arrays r C++ allows arrays with multiple index values m char page [30] Multi-Dimensional Arrays r C++ allows arrays with multiple index values m char page [30]](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-73.jpg)
![Index Values of page r The indexed variables for array page are page[0][0], page[0][1], Index Values of page r The indexed variables for array page are page[0][0], page[0][1],](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-74.jpg)
![Multidimensional Array Parameters r Recall when declaring a[] as a parameter, size is a Multidimensional Array Parameters r Recall when declaring a[] as a parameter, size is a](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-75.jpg)
![A Two Dimensional Array in C++ is an array of arrays: int a[4][5]; A A Two Dimensional Array in C++ is an array of arrays: int a[4][5]; A](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-76.jpg)
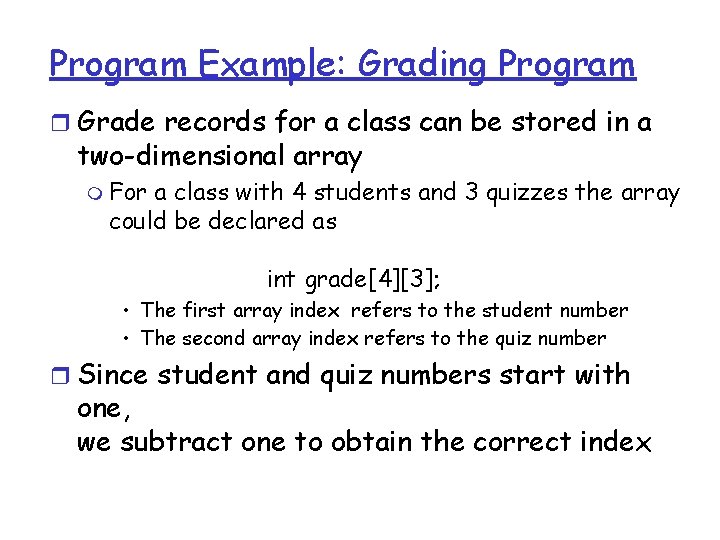
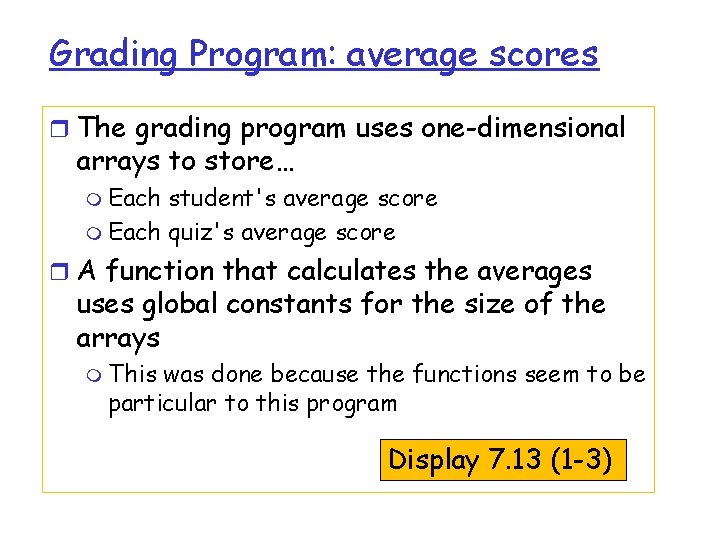
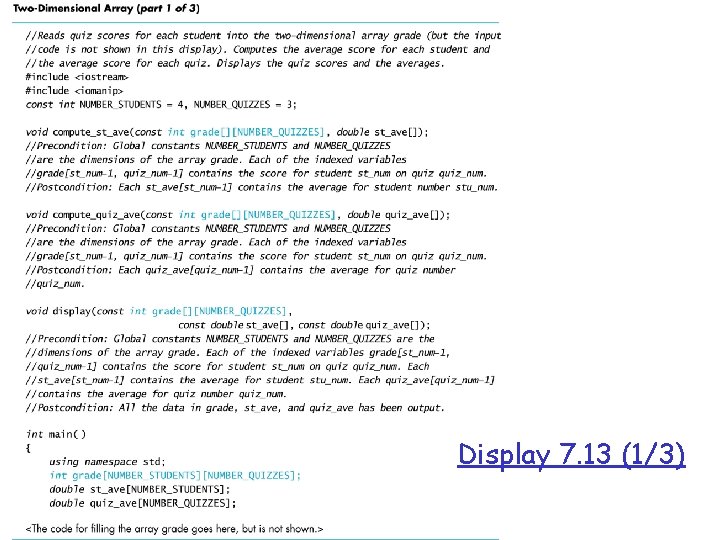
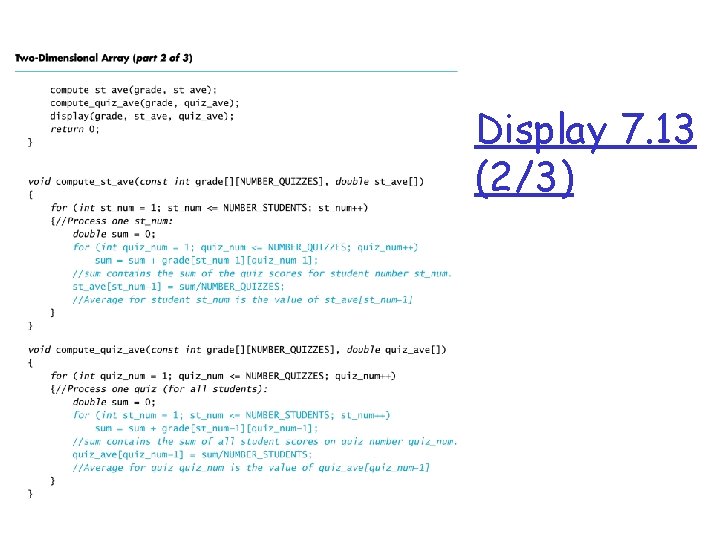
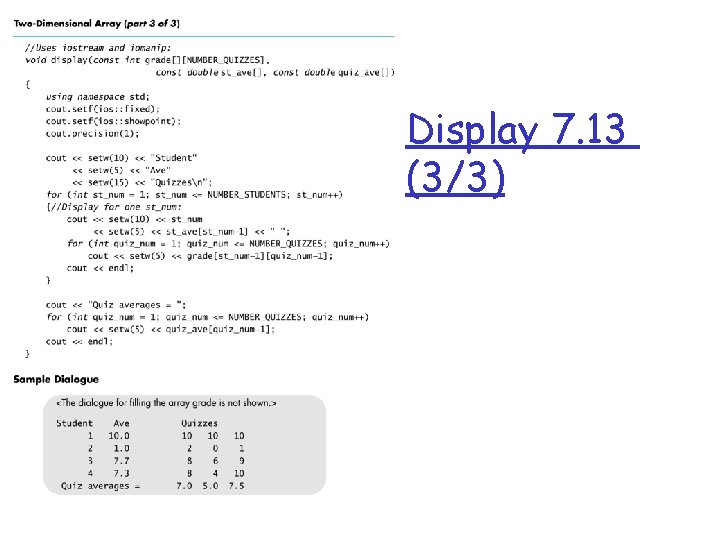
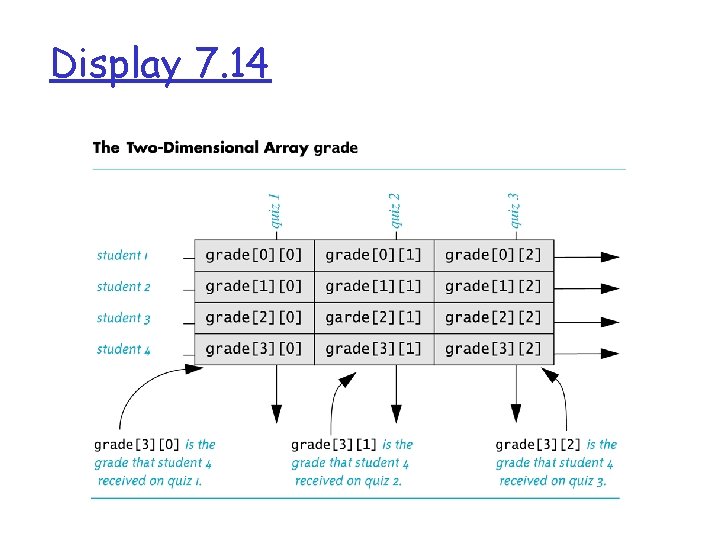
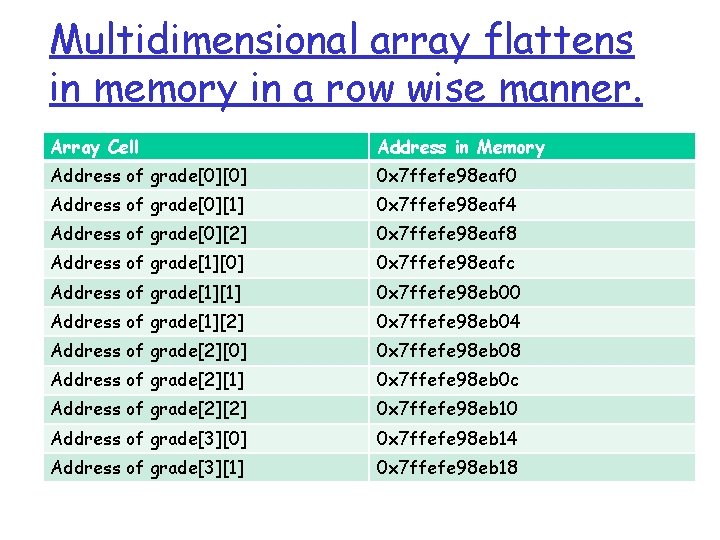
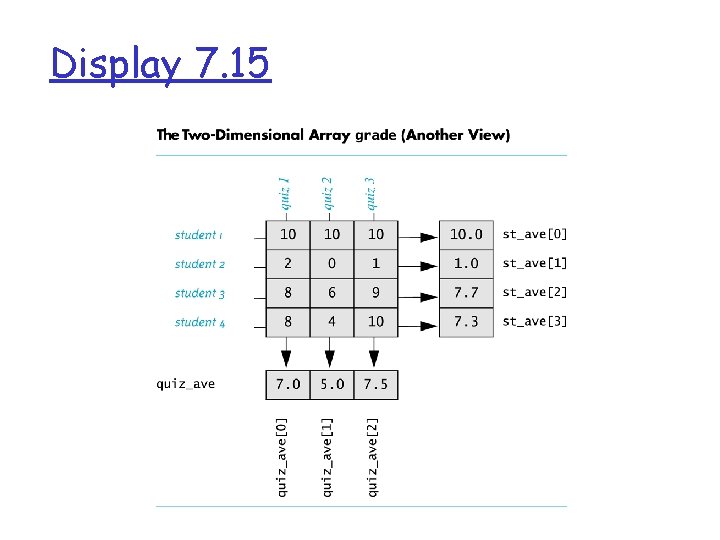
![Multidimensional Arrays r Recall that multidimension arrays are just arrays of arrays: int a[4][5]; Multidimensional Arrays r Recall that multidimension arrays are just arrays of arrays: int a[4][5];](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-85.jpg)
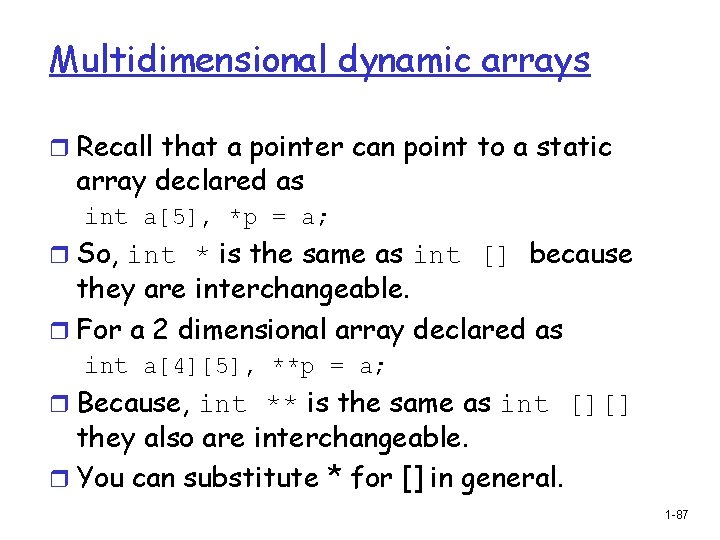
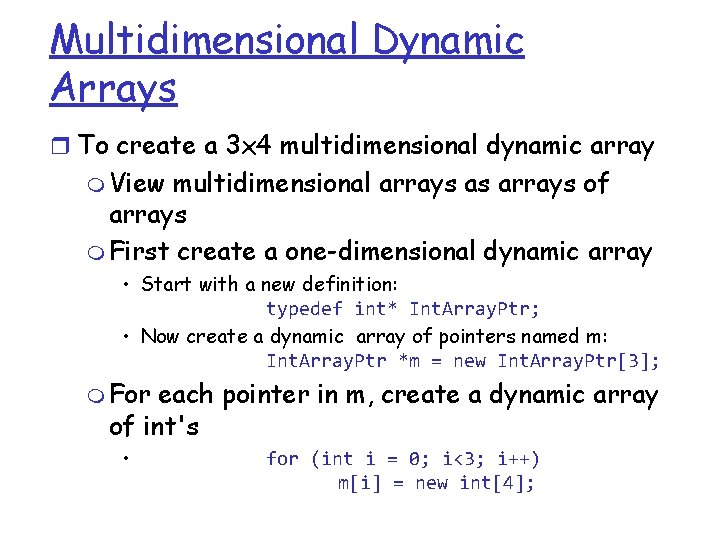
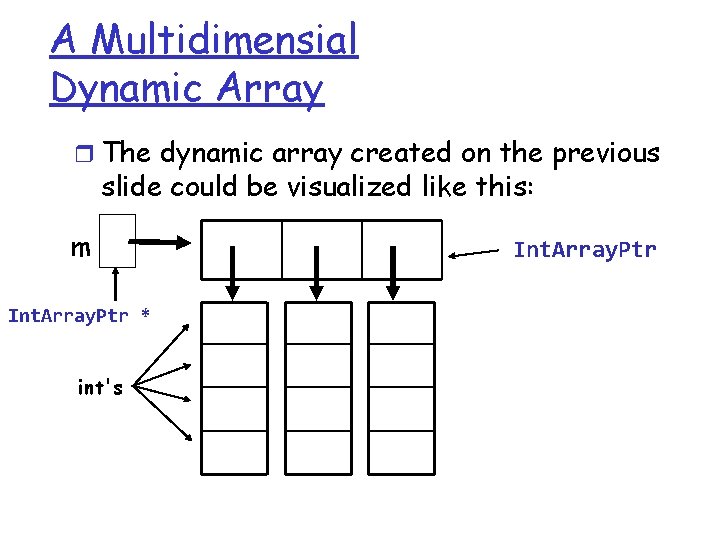
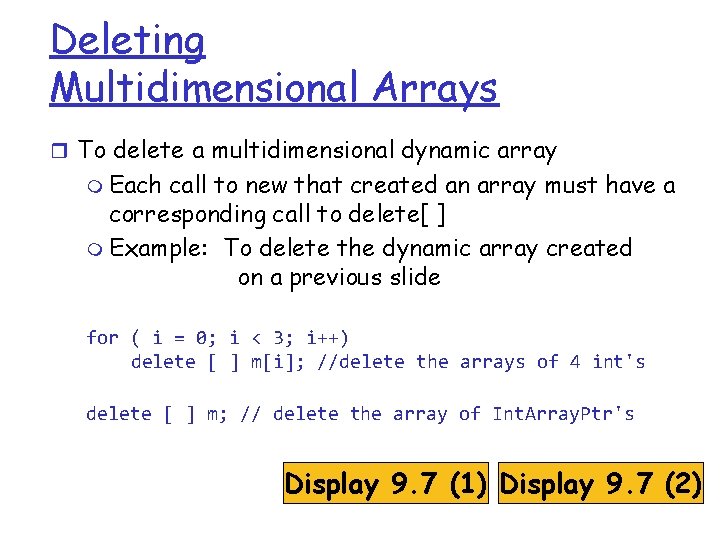
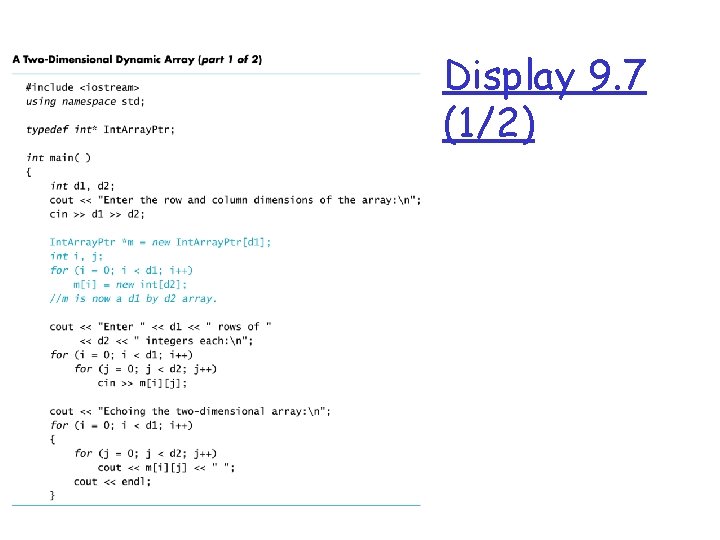
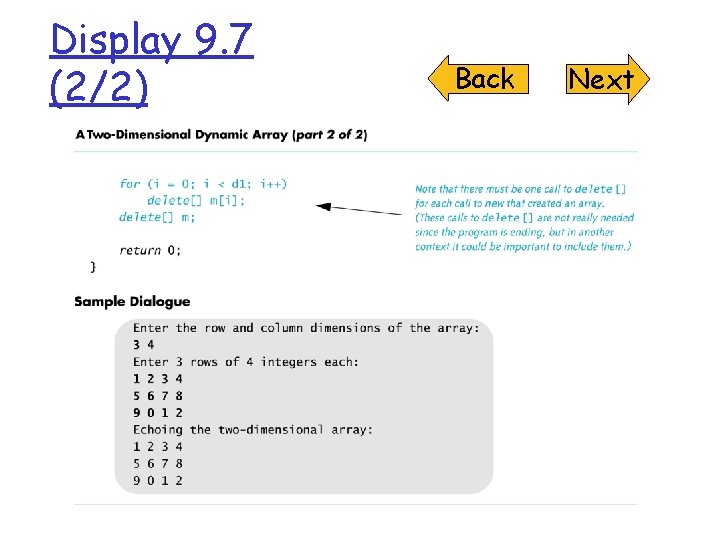
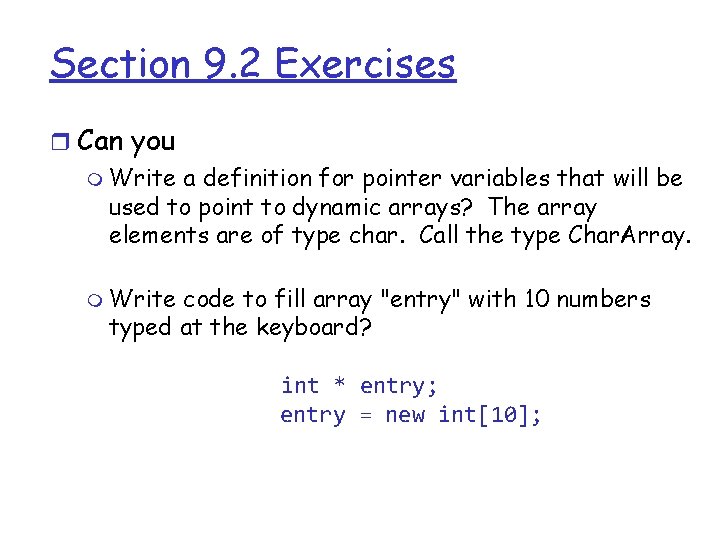
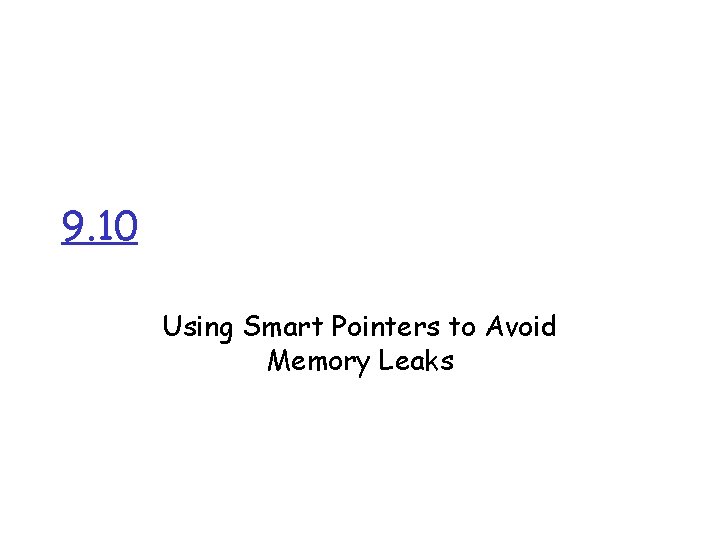
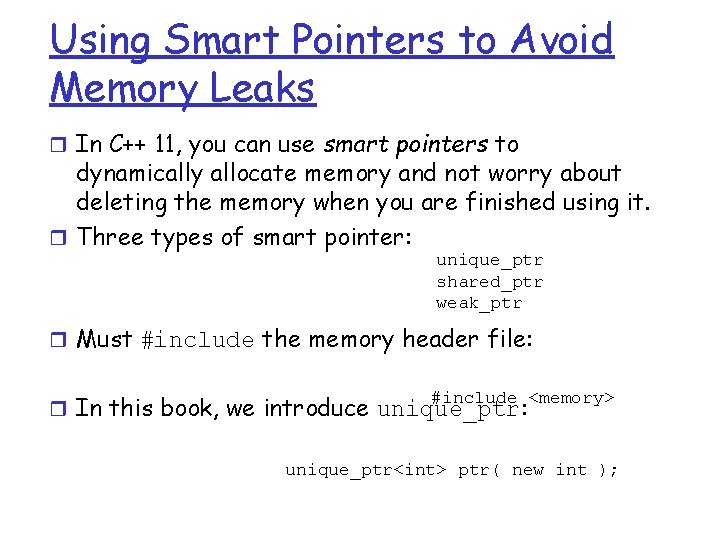
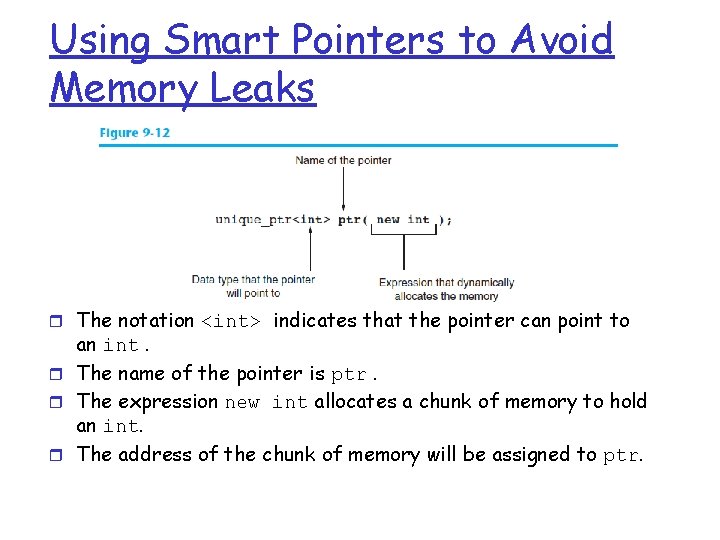
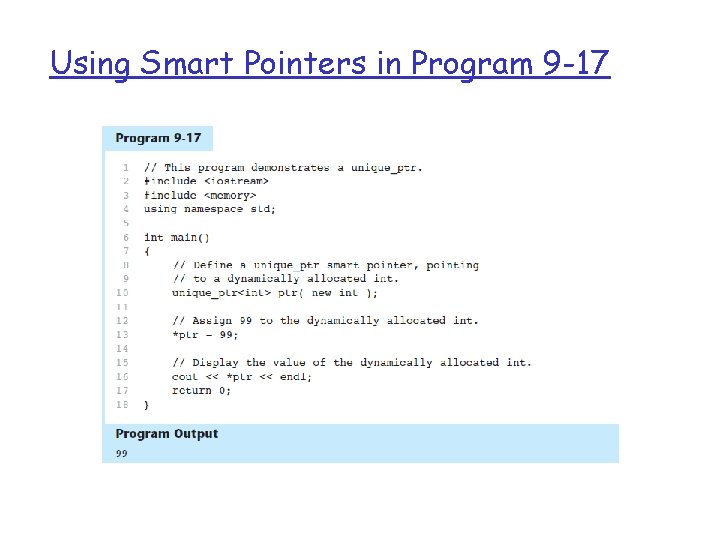
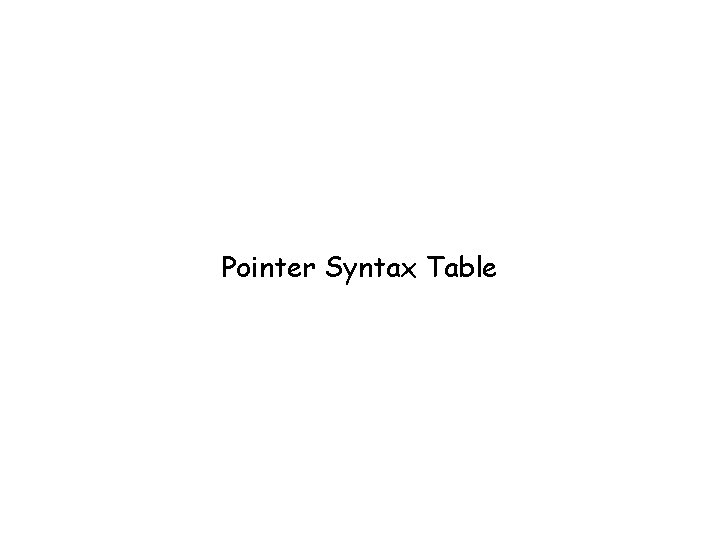
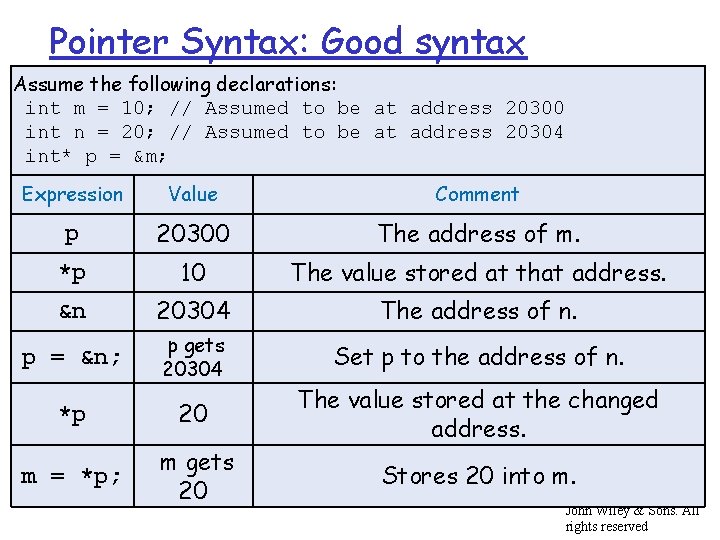
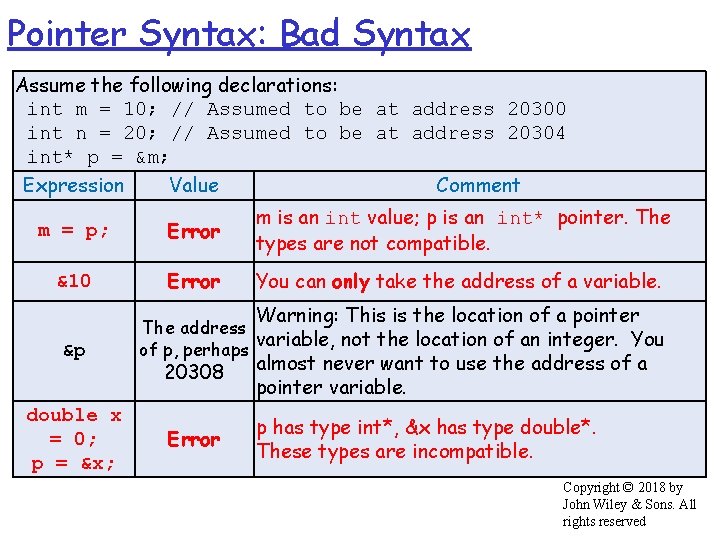
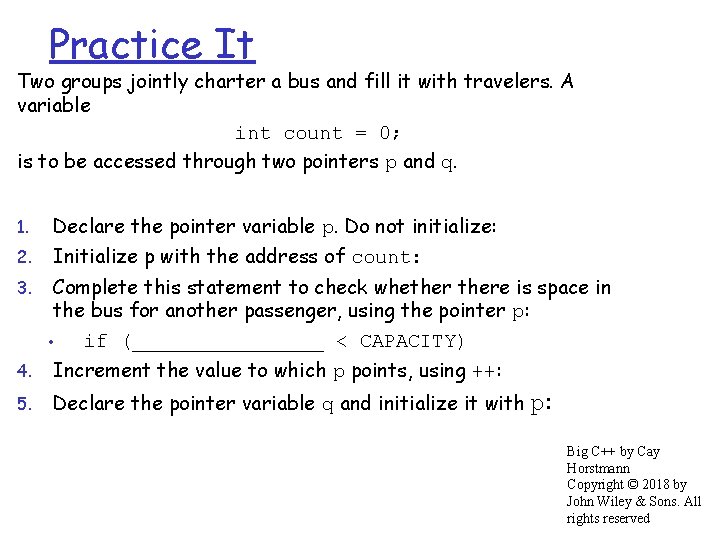
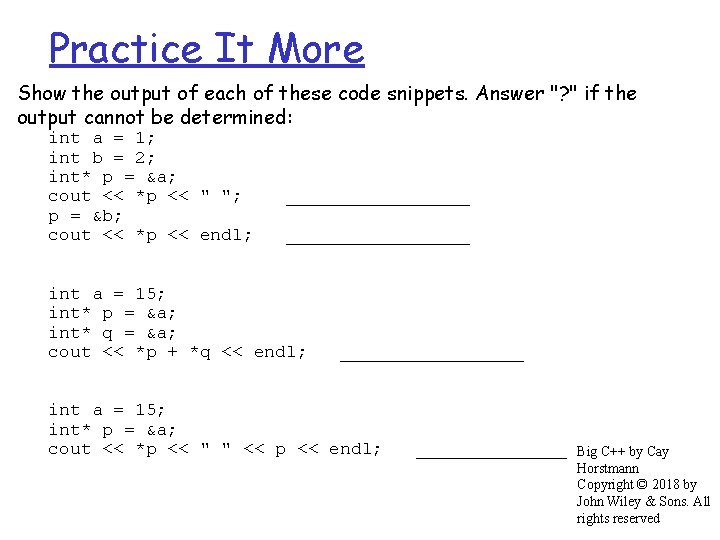
![Array / Pointer Ex: double d[10] Expression d Value Comment 20300 Starting address of Array / Pointer Ex: double d[10] Expression d Value Comment 20300 Starting address of](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-102.jpg)
- Slides: 102
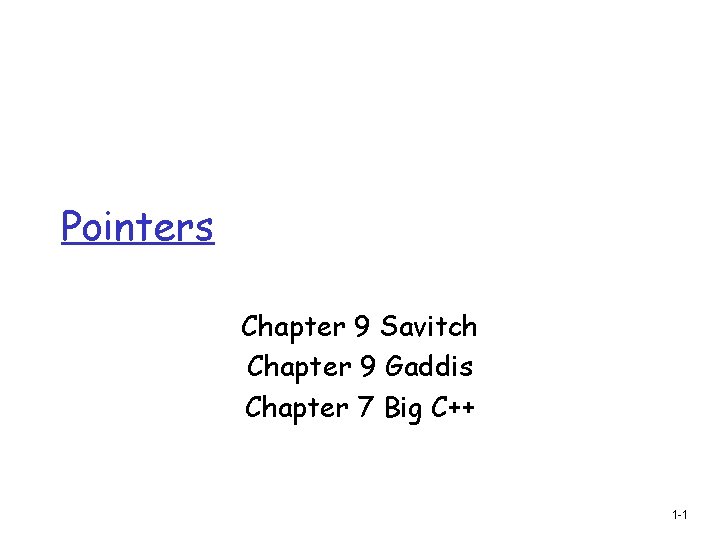
Pointers Chapter 9 Savitch Chapter 9 Gaddis Chapter 7 Big C++ 1 -1
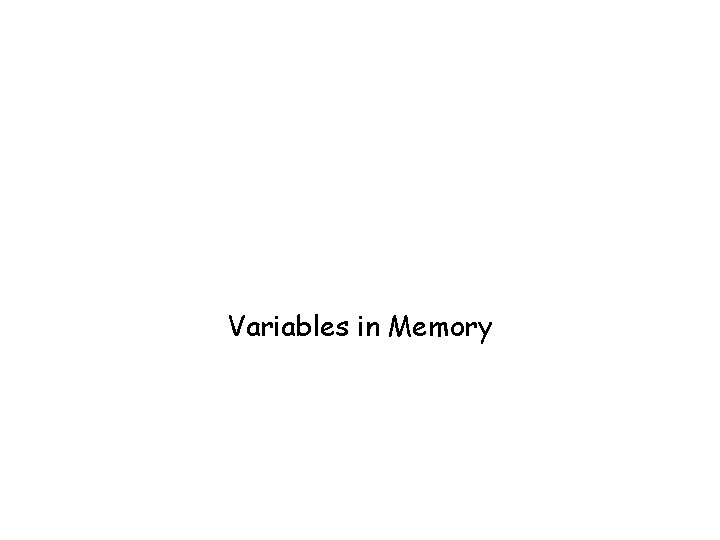
Variables in Memory
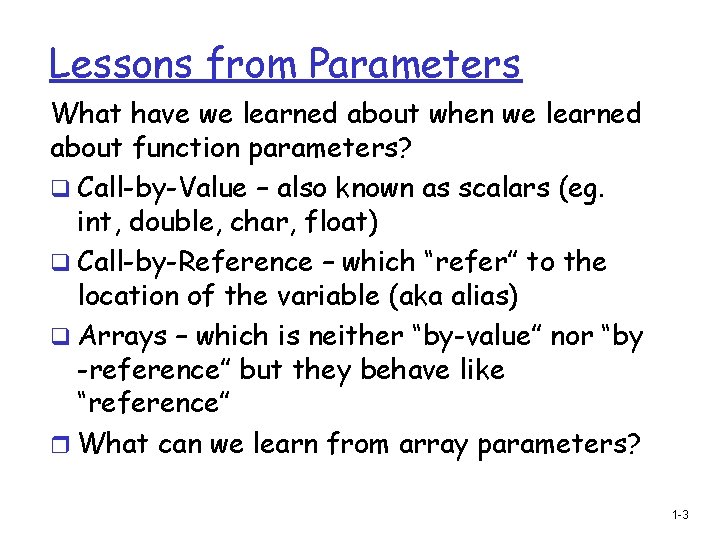
Lessons from Parameters What have we learned about when we learned about function parameters? q Call-by-Value – also known as scalars (eg. int, double, char, float) q Call-by-Reference – which “refer” to the location of the variable (aka alias) q Arrays – which is neither “by-value” nor “by -reference” but they behave like “reference” r What can we learn from array parameters? 1 -3
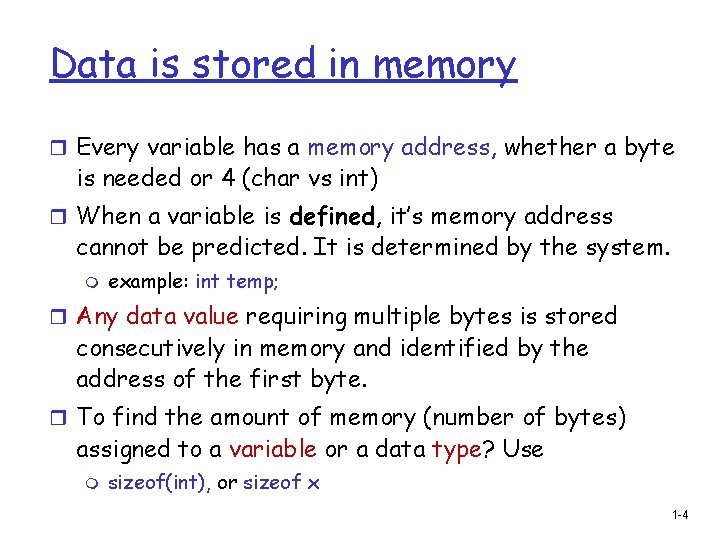
Data is stored in memory r Every variable has a memory address, whether a byte is needed or 4 (char vs int) r When a variable is defined, it’s memory address cannot be predicted. It is determined by the system. m example: int temp; r Any data value requiring multiple bytes is stored consecutively in memory and identified by the address of the first byte. r To find the amount of memory (number of bytes) assigned to a variable or a data type? Use m sizeof(int), or sizeof x 1 -4
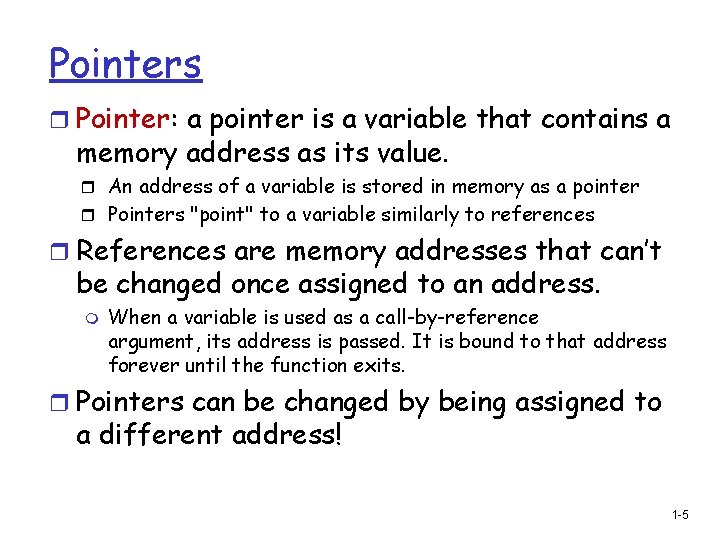
Pointers r Pointer: a pointer is a variable that contains a memory address as its value. r An address of a variable is stored in memory as a pointer r Pointers "point" to a variable similarly to references r References are memory addresses that can’t be changed once assigned to an address. m When a variable is used as a call-by-reference argument, its address is passed. It is bound to that address forever until the function exits. r Pointers can be changed by being assigned to a different address! 1 -5
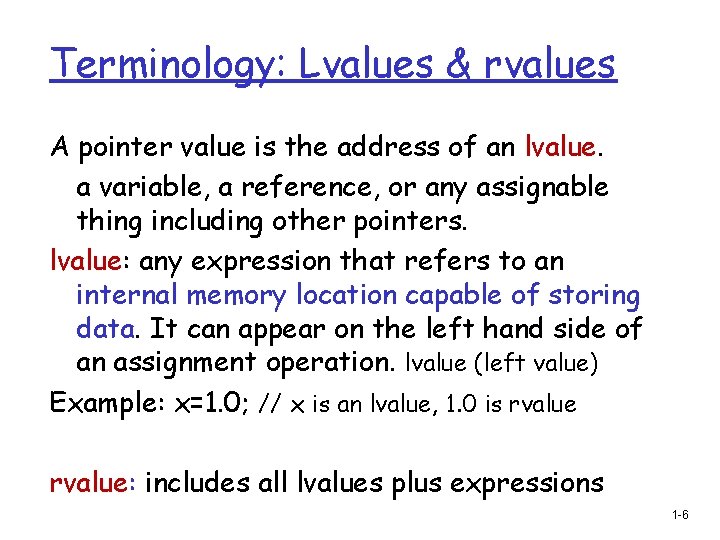
Terminology: Lvalues & rvalues A pointer value is the address of an lvalue. a variable, a reference, or any assignable thing including other pointers. lvalue: any expression that refers to an internal memory location capable of storing data. It can appear on the left hand side of an assignment operation. lvalue (left value) Example: x=1. 0; // x is an lvalue, 1. 0 is rvalue: includes all lvalues plus expressions 1 -6
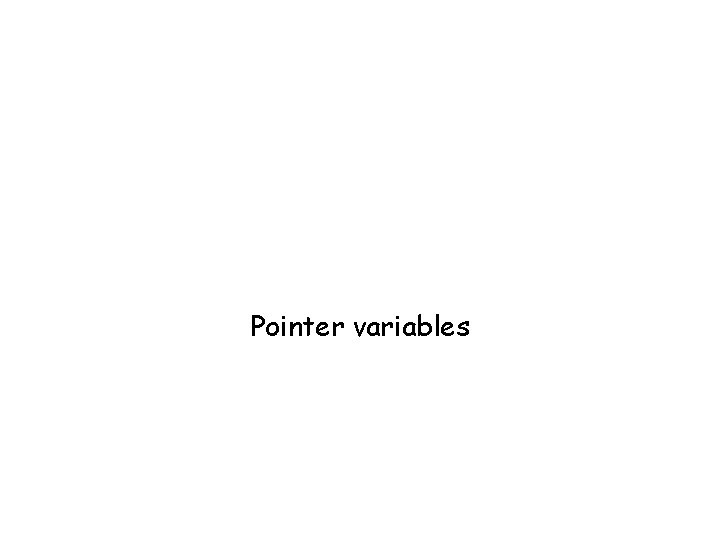
Pointer variables
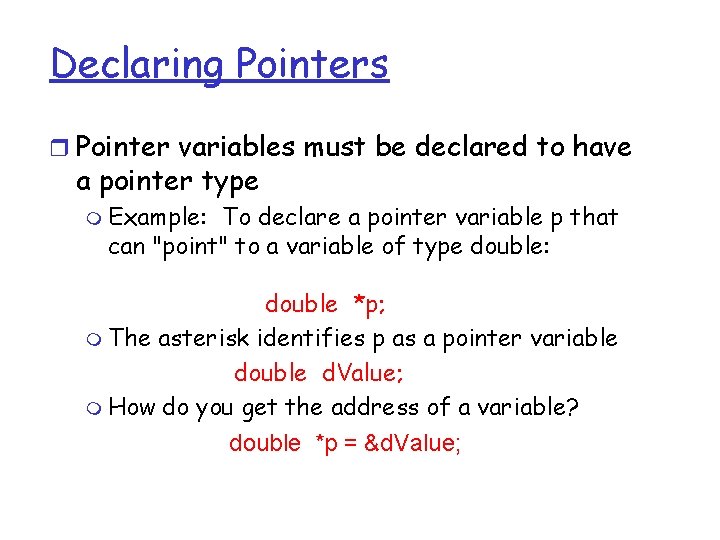
Declaring Pointers r Pointer variables must be declared to have a pointer type m Example: To declare a pointer variable p that can "point" to a variable of type double: double *p; m The asterisk identifies p as a pointer variable double d. Value; m How do you get the address of a variable? double *p = &d. Value;
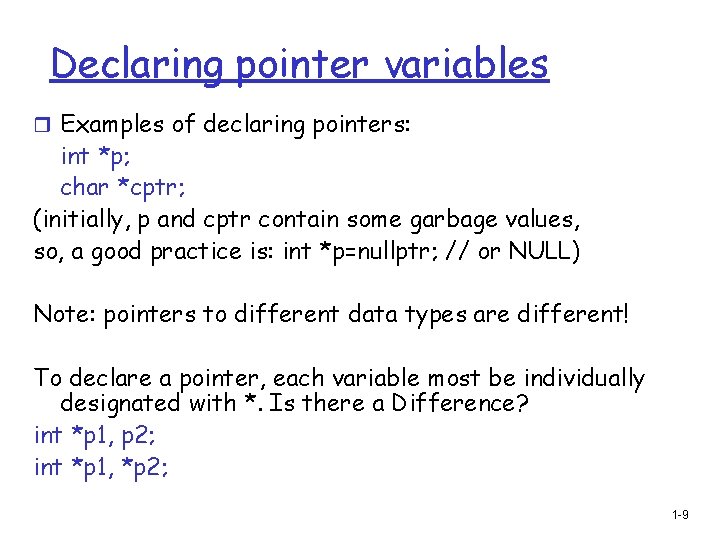
Declaring pointer variables r Examples of declaring pointers: int *p; char *cptr; (initially, p and cptr contain some garbage values, so, a good practice is: int *p=nullptr; // or NULL) Note: pointers to different data types are different! To declare a pointer, each variable most be individually designated with *. Is there a Difference? int *p 1, p 2; int *p 1, *p 2; 1 -9
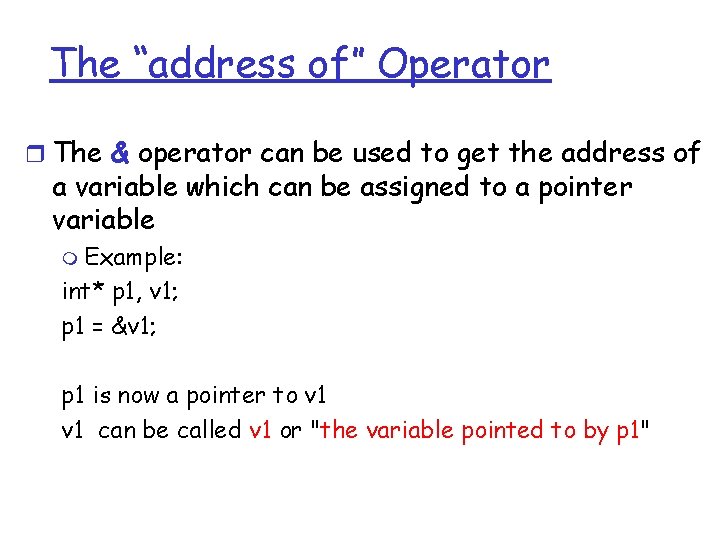
The “address of” Operator r The & operator can be used to get the address of a variable which can be assigned to a pointer variable m Example: int* p 1, v 1; p 1 = &v 1; p 1 is now a pointer to v 1 can be called v 1 or "the variable pointed to by p 1"
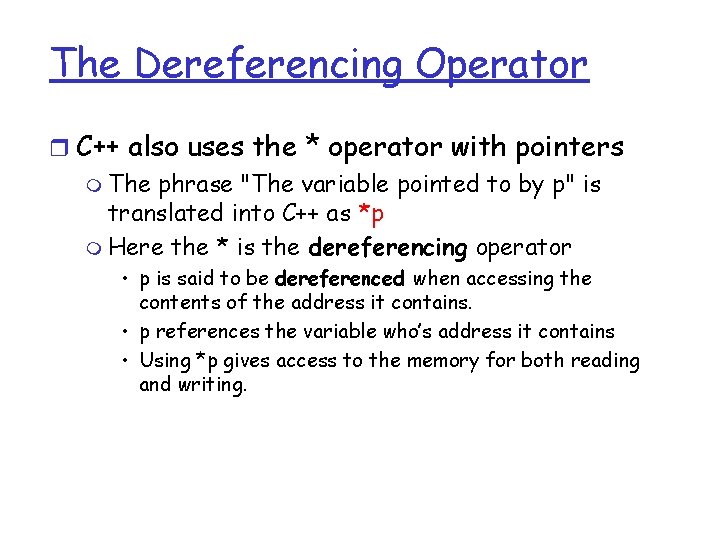
The Dereferencing Operator r C++ also uses the * operator with pointers m The phrase "The variable pointed to by p" is translated into C++ as *p m Here the * is the dereferencing operator • p is said to be dereferenced when accessing the contents of the address it contains. • p references the variable who’s address it contains • Using *p gives access to the memory for both reading and writing.
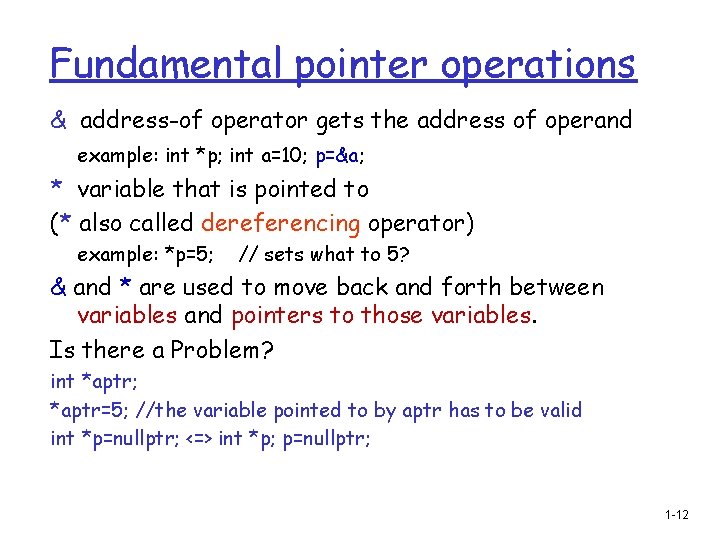
Fundamental pointer operations & address-of operator gets the address of operand example: int *p; int a=10; p=&a; * variable that is pointed to (* also called dereferencing operator) example: *p=5; // sets what to 5? & and * are used to move back and forth between variables and pointers to those variables. Is there a Problem? int *aptr; *aptr=5; //the variable pointed to by aptr has to be valid int *p=nullptr; <=> int *p; p=nullptr; 1 -12
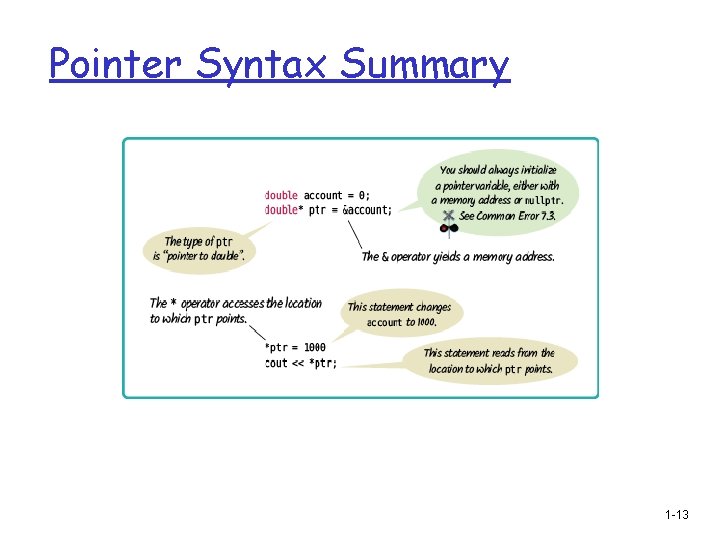
Pointer Syntax Summary 1 -13
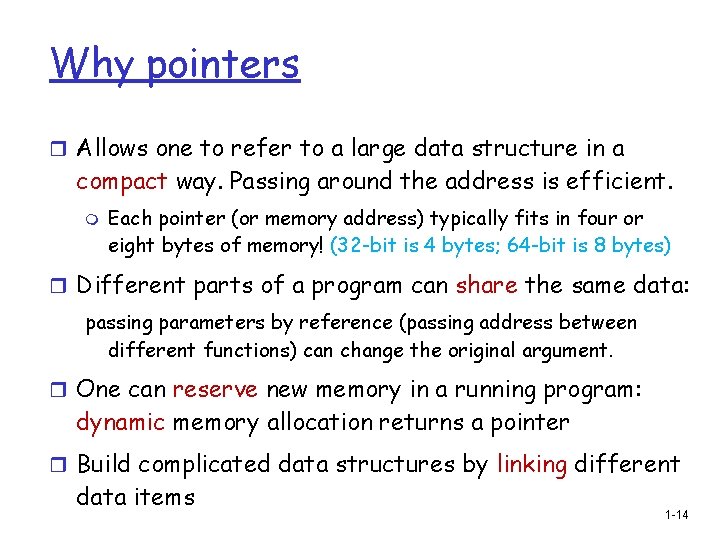
Why pointers r Allows one to refer to a large data structure in a compact way. Passing around the address is efficient. m Each pointer (or memory address) typically fits in four or eight bytes of memory! (32 -bit is 4 bytes; 64 -bit is 8 bytes) r Different parts of a program can share the same data: passing parameters by reference (passing address between different functions) can change the original argument. r One can reserve new memory in a running program: dynamic memory allocation returns a pointer r Build complicated data structures by linking different data items 1 -14
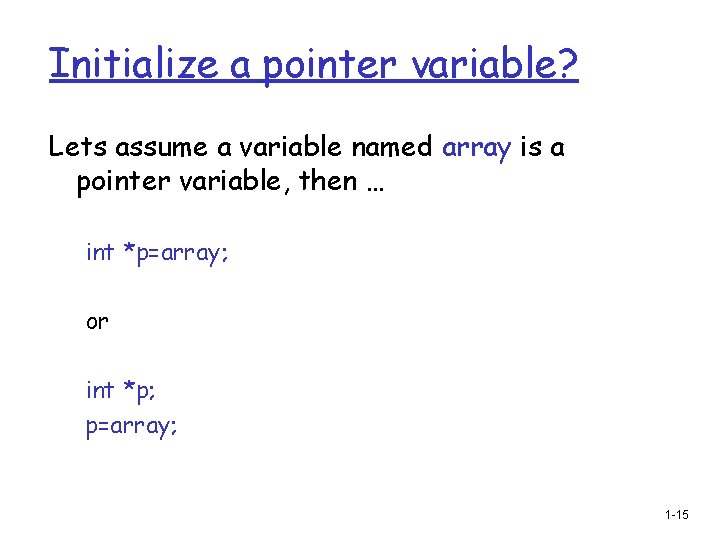
Initialize a pointer variable? Lets assume a variable named array is a pointer variable, then … int *p=array; or int *p; p=array; 1 -15
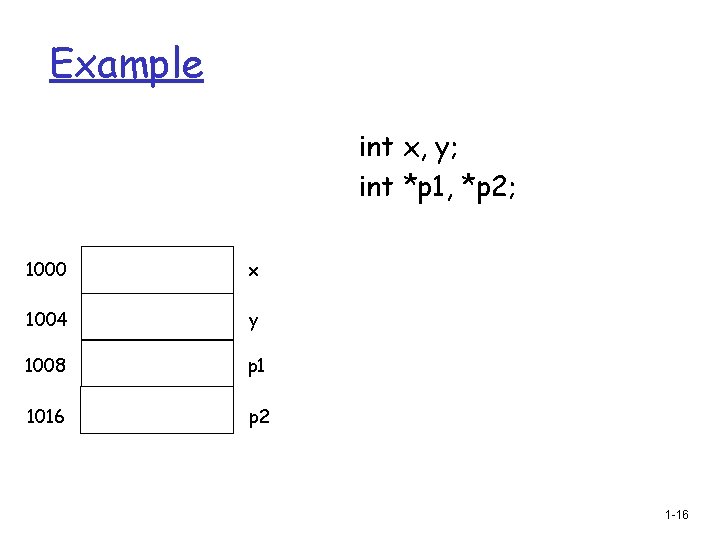
Example int x, y; int *p 1, *p 2; 1000 x 1004 y 1008 p 1 1016 p 2 1 -16
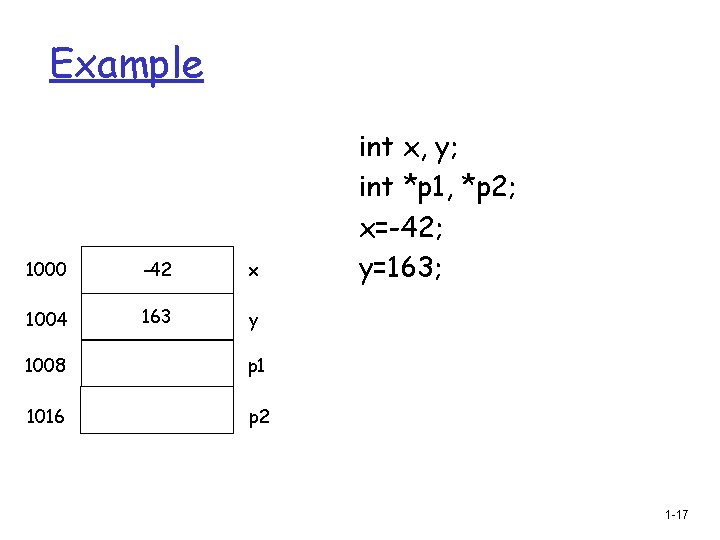
Example 1000 -42 x 1004 163 y 1008 p 1 1016 p 2 int x, y; int *p 1, *p 2; x=-42; y=163; 1 -17
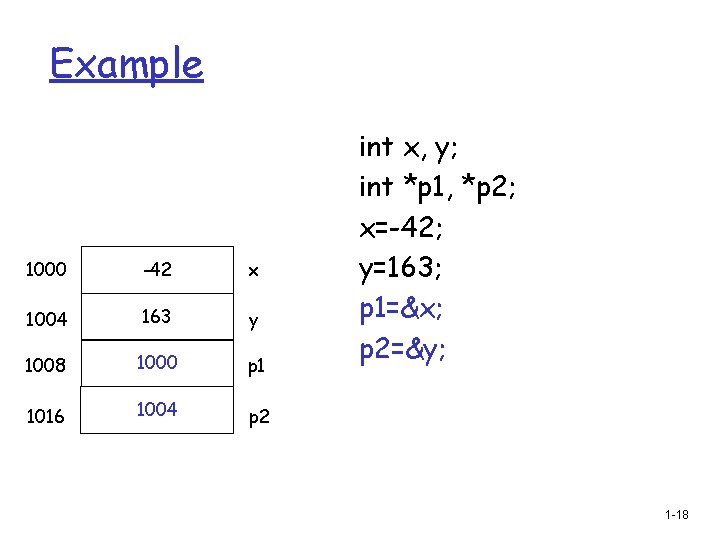
Example 1000 -42 x 1004 163 y 1008 1000 p 1 1016 1004 p 2 int x, y; int *p 1, *p 2; x=-42; y=163; p 1=&x; p 2=&y; 1 -18
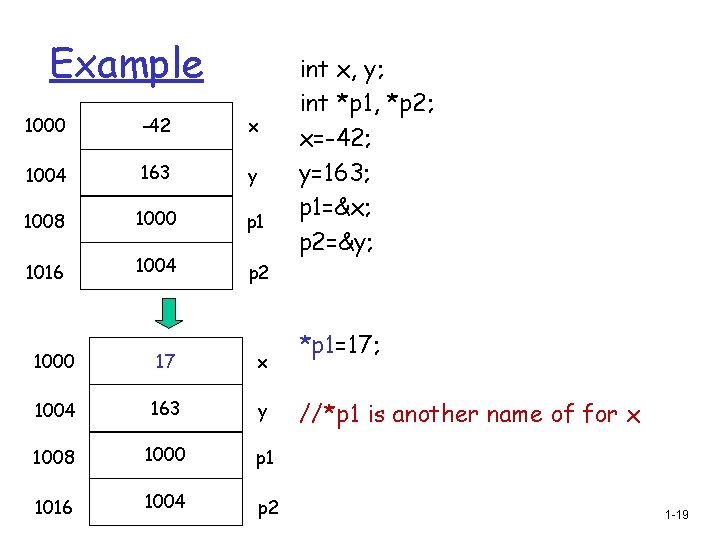
Example 1000 -42 x 1004 163 y 1008 1000 p 1 1016 1004 p 2 1000 17 x 1004 163 y 1008 1000 p 1 1016 1004 p 2 int x, y; int *p 1, *p 2; x=-42; y=163; p 1=&x; p 2=&y; *p 1=17; //*p 1 is another name of for x 1 -19
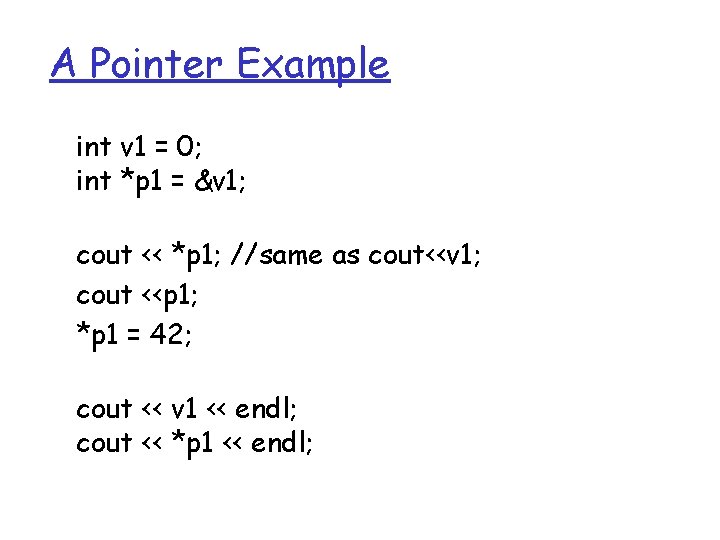
A Pointer Example int v 1 = 0; int *p 1 = &v 1; cout << *p 1; //same as cout<<v 1; cout <<p 1; *p 1 = 42; cout << v 1 << endl; cout << *p 1 << endl;
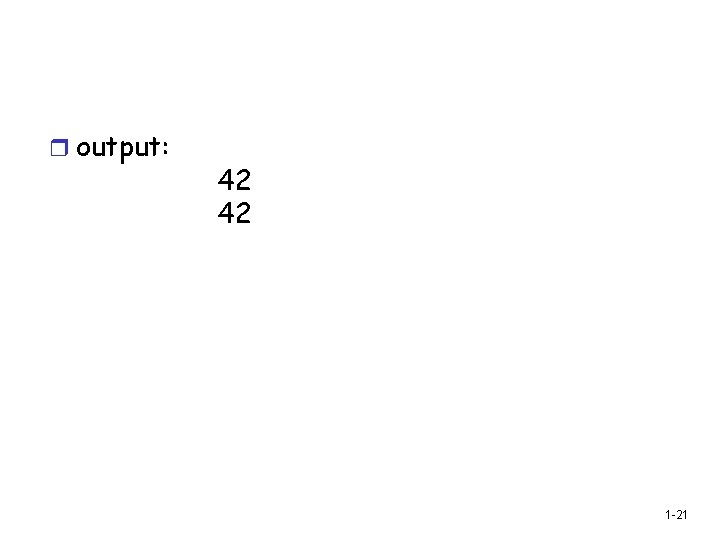
r output: 42 42 1 -21
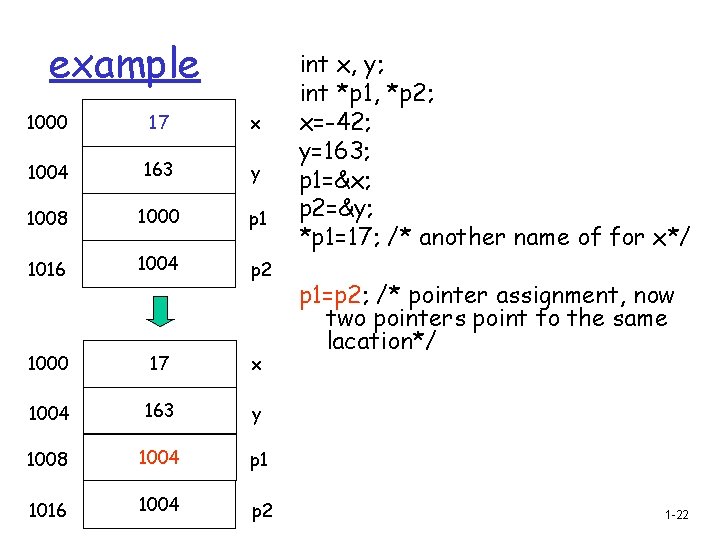
example 1000 17 x 1004 163 y 1008 1000 p 1 1016 1004 p 2 1000 17 x 1004 163 y 1008 1004 p 1 1016 1004 p 2 int x, y; int *p 1, *p 2; x=-42; y=163; p 1=&x; p 2=&y; *p 1=17; /* another name of for x*/ p 1=p 2; /* pointer assignment, now two pointers point to the same lacation*/ 1 -22
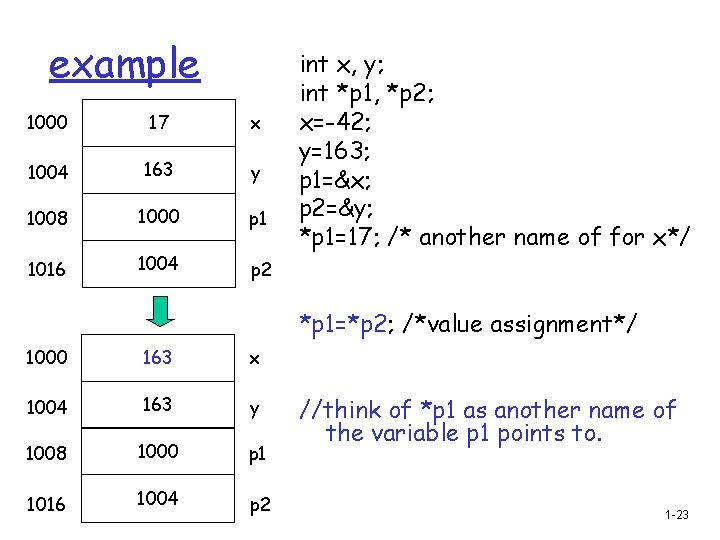
example 1000 17 x 1004 163 y 1008 1000 p 1 1016 1004 p 2 int x, y; int *p 1, *p 2; x=-42; y=163; p 1=&x; p 2=&y; *p 1=17; /* another name of for x*/ *p 1=*p 2; /*value assignment*/ 1000 163 x 1004 163 y 1008 1000 p 1 1016 1004 p 2 //think of *p 1 as another name of the variable p 1 points to. 1 -23
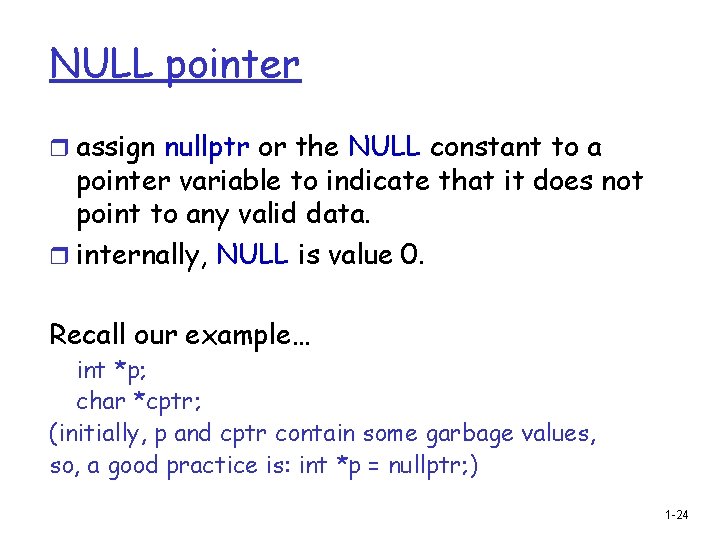
NULL pointer r assign nullptr or the NULL constant to a pointer variable to indicate that it does not point to any valid data. r internally, NULL is value 0. Recall our example… int *p; char *cptr; (initially, p and cptr contain some garbage values, so, a good practice is: int *p = nullptr; ) 1 -24
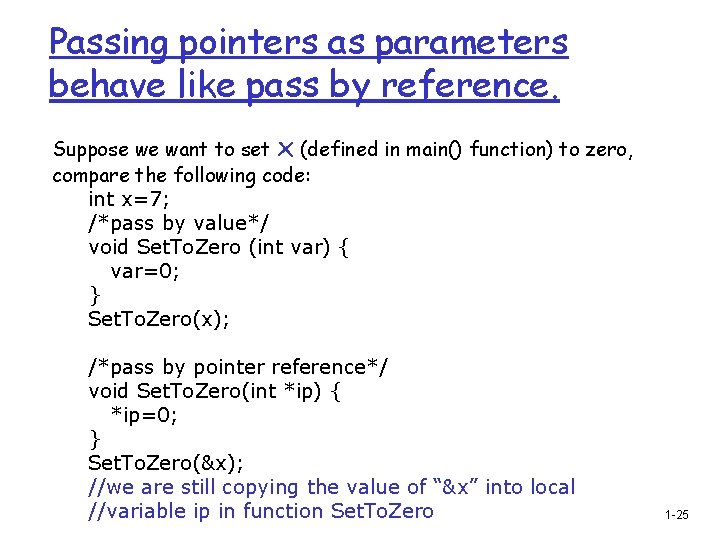
Passing pointers as parameters behave like pass by reference. Suppose we want to set x (defined in main() function) to zero, compare the following code: int x=7; /*pass by value*/ void Set. To. Zero (int var) { var=0; } Set. To. Zero(x); /*pass by pointer reference*/ void Set. To. Zero(int *ip) { *ip=0; } Set. To. Zero(&x); //we are still copying the value of “&x” into local //variable ip in function Set. To. Zero 1 -25
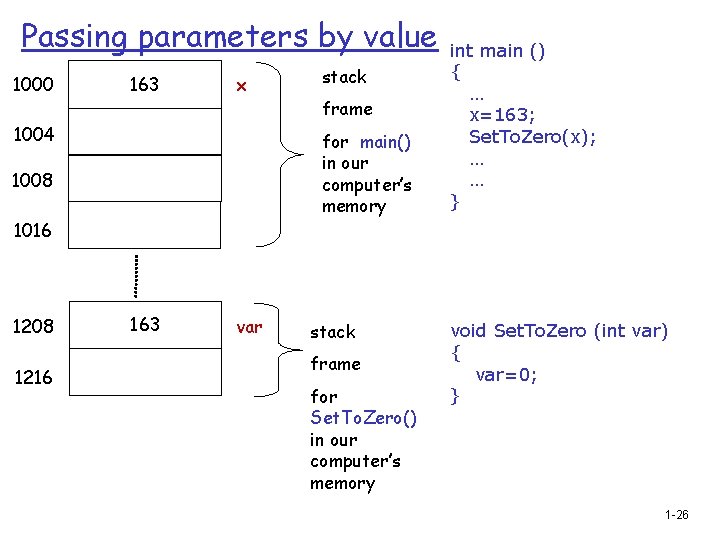
Passing parameters by value 1000 163 x 1004 1016 1216 frame for main() in our computer’s memory 1008 1208 stack 163 var stack frame for Set. To. Zero() in our computer’s memory int main () { … x=163; Set. To. Zero(x); … … } void Set. To. Zero (int var) { var=0; } 1 -26
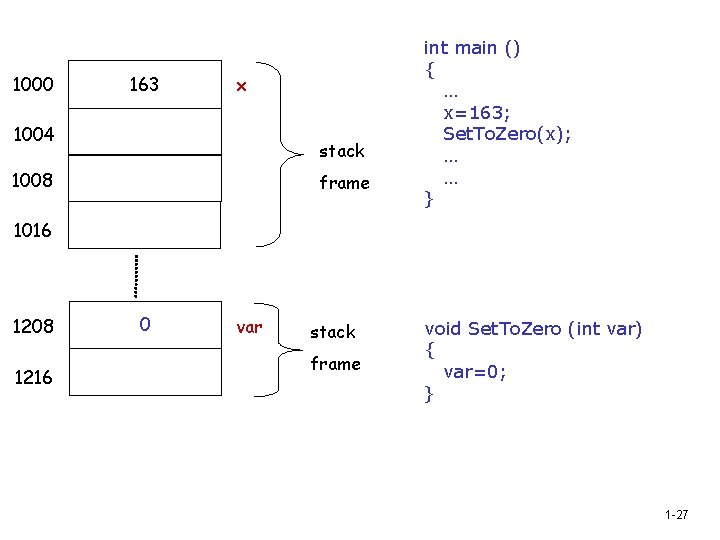
1000 163 x 1004 stack 1008 frame int main () { … x=163; Set. To. Zero(x); … … } 1016 1208 1216 0 var stack frame void Set. To. Zero (int var) { var=0; } 1 -27
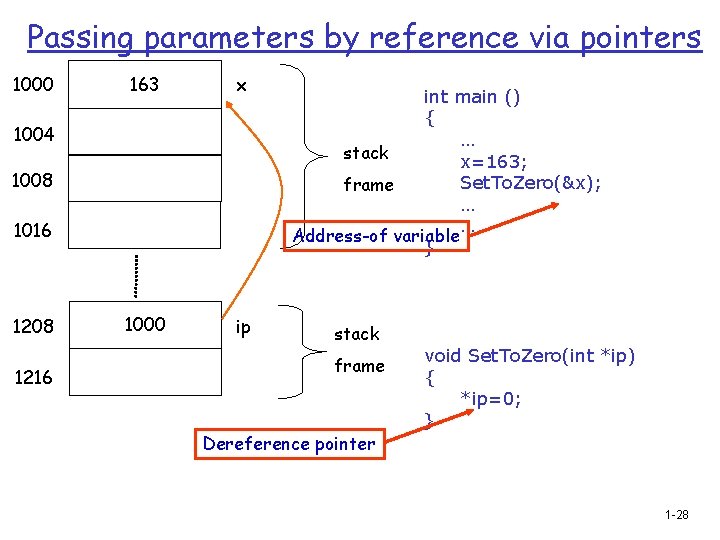
Passing parameters by reference via pointers 1000 163 x 1000 ip 1004 1008 1016 1208 1216 int main () { … stack x=163; Set. To. Zero(&x); frame … … Address-of variable } stack frame Dereference pointer void Set. To. Zero(int *ip) { *ip=0; } 1 -28
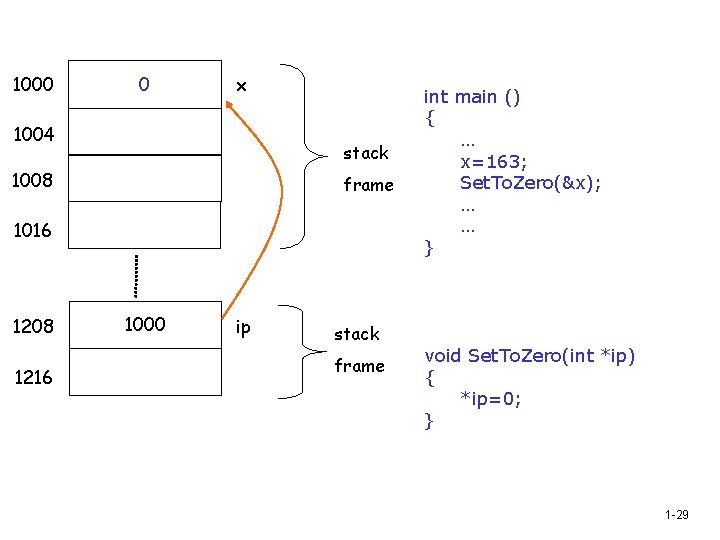
1000 0 x 1004 stack 1008 frame 1016 1208 1216 1000 ip stack frame int main () { … x=163; Set. To. Zero(&x); … … } void Set. To. Zero(int *ip) { *ip=0; } 1 -29
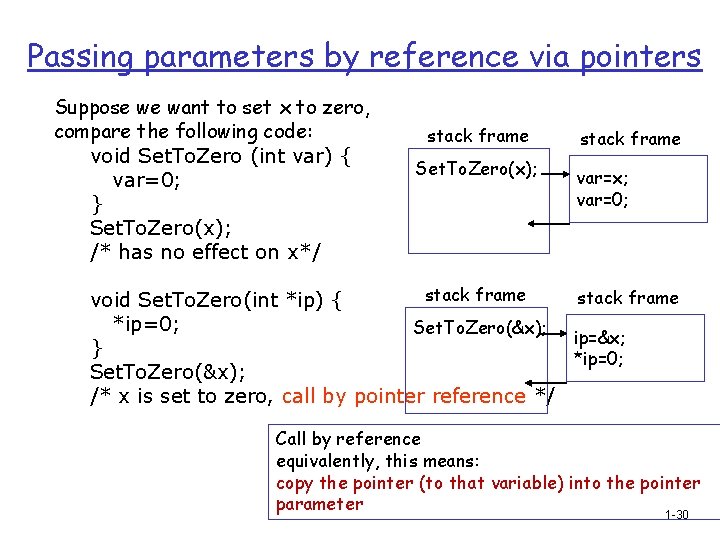
Passing parameters by reference via pointers Suppose we want to set x to zero, compare the following code: void Set. To. Zero (int var) { var=0; } Set. To. Zero(x); /* has no effect on x*/ stack frame Set. To. Zero(x); stack frame var=x; var=0; stack frame void Set. To. Zero(int *ip) { *ip=0; Set. To. Zero(&x); ip=&x; } *ip=0; Set. To. Zero(&x); /* x is set to zero, call by pointer reference */ Call by reference equivalently, this means: copy the pointer (to that variable) into the pointer parameter 1 -30
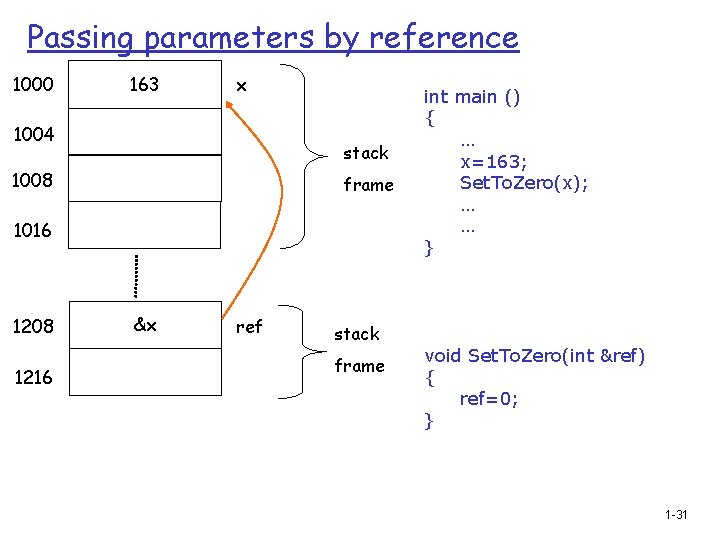
Passing parameters by reference 1000 163 x 1004 stack 1008 frame 1016 1208 1216 &x ref stack frame int main () { … x=163; Set. To. Zero(x); … … } void Set. To. Zero(int &ref) { ref=0; } 1 -31
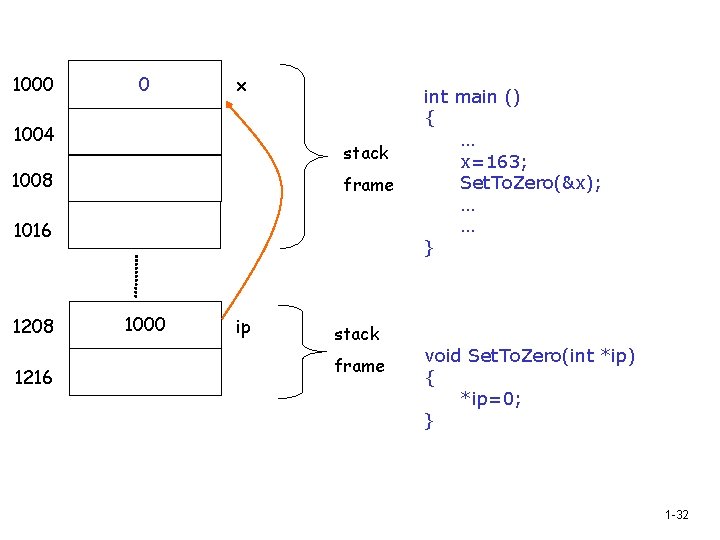
1000 0 x 1004 stack 1008 frame 1016 1208 1216 1000 ip stack frame int main () { … x=163; Set. To. Zero(&x); … … } void Set. To. Zero(int *ip) { *ip=0; } 1 -32
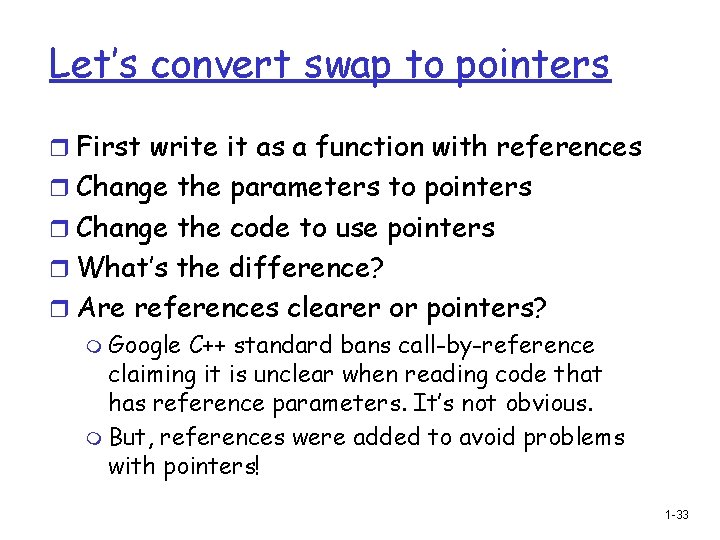
Let’s convert swap to pointers r First write it as a function with references r Change the parameters to pointers r Change the code to use pointers r What’s the difference? r Are references clearer or pointers? m Google C++ standard bans call-by-reference claiming it is unclear when reading code that has reference parameters. It’s not obvious. m But, references were added to avoid problems with pointers! 1 -33
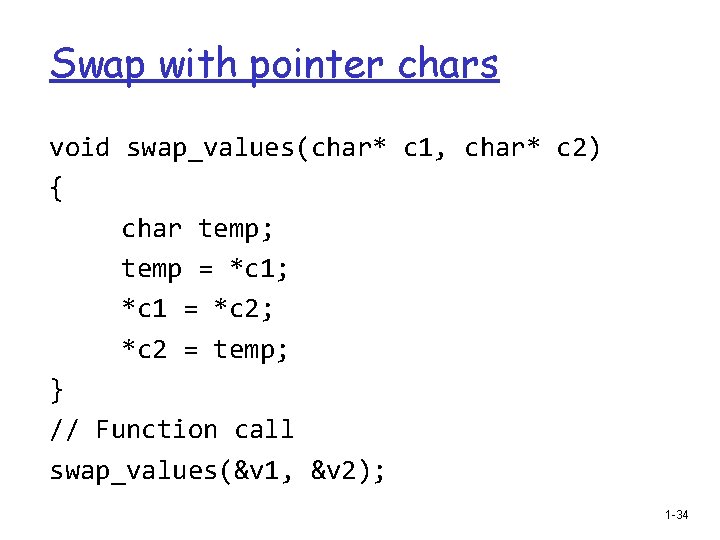
Swap with pointer chars void swap_values(char* c 1, char* c 2) { char temp; temp = *c 1; *c 1 = *c 2; *c 2 = temp; } // Function call swap_values(&v 1, &v 2); 1 -34
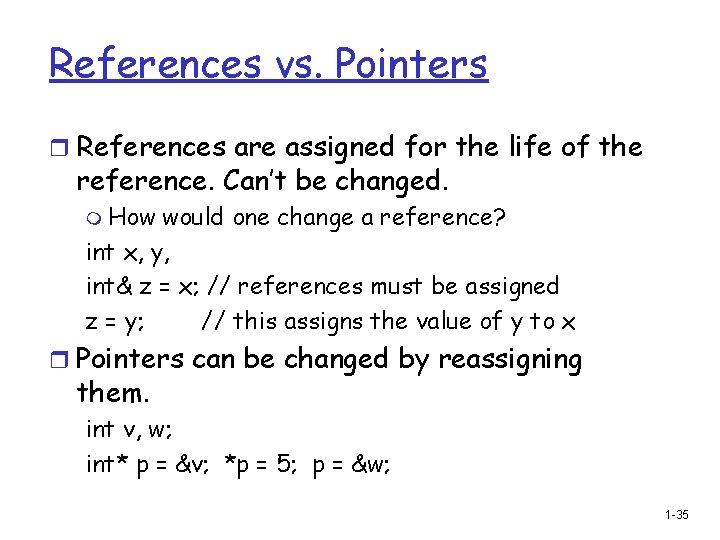
References vs. Pointers r References are assigned for the life of the reference. Can’t be changed. m How would one change a reference? int x, y, int& z = x; // references must be assigned z = y; // this assigns the value of y to x r Pointers can be changed by reassigning them. int v, w; int* p = &v; *p = 5; p = &w; 1 -35
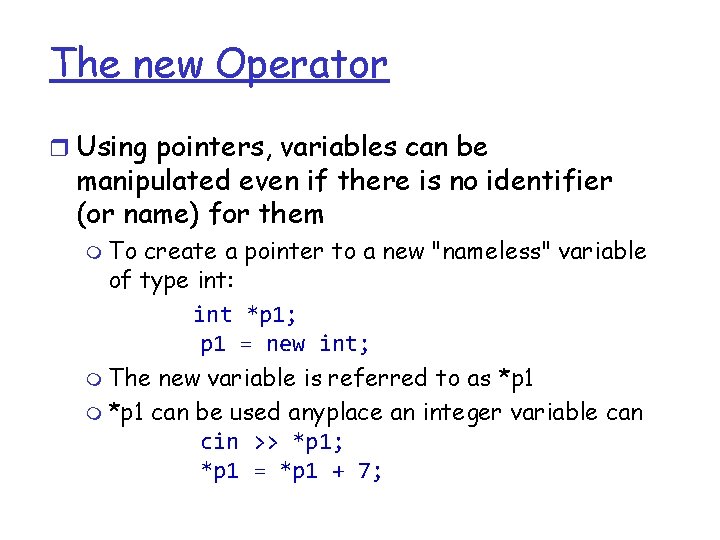
The new Operator r Using pointers, variables can be manipulated even if there is no identifier (or name) for them m To create a pointer to a new "nameless" variable of type int: int *p 1; p 1 = new int; m The new variable is referred to as *p 1 m *p 1 can be used anyplace an integer variable can cin >> *p 1; *p 1 = *p 1 + 7;
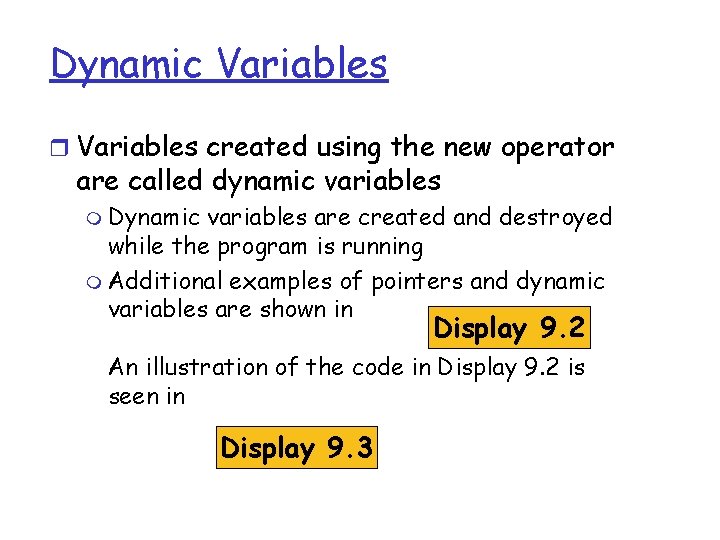
Dynamic Variables r Variables created using the new operator are called dynamic variables m Dynamic variables are created and destroyed while the program is running m Additional examples of pointers and dynamic variables are shown in Display 9. 2 An illustration of the code in Display 9. 2 is seen in Display 9. 3
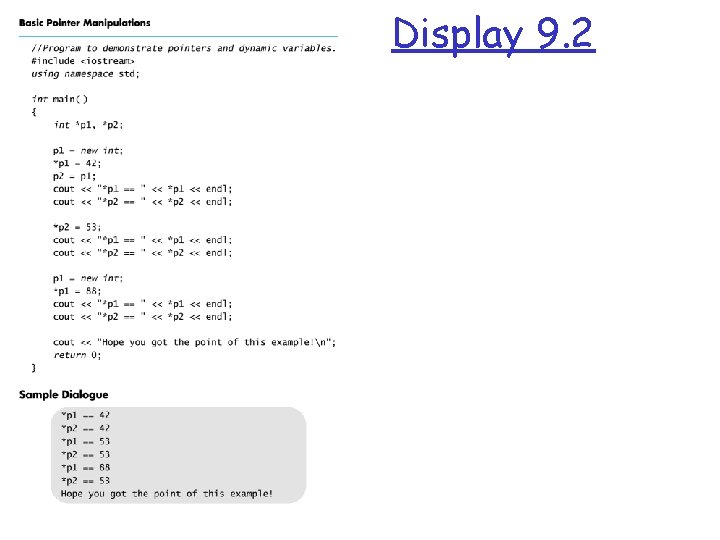
Display 9. 2 Slide 9 - 39
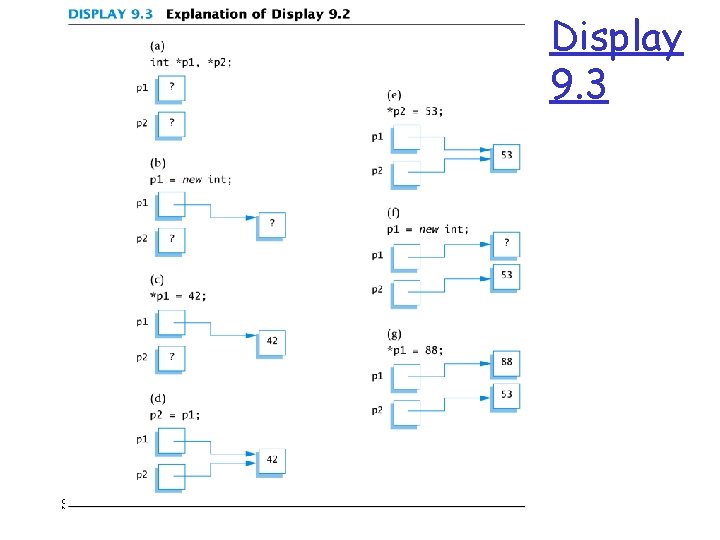
Display 9. 3 Slide 9 - 40
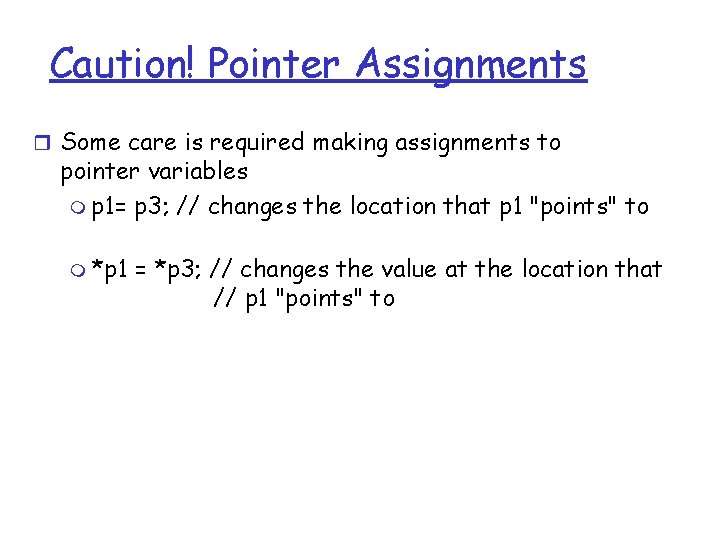
Caution! Pointer Assignments r Some care is required making assignments to pointer variables m p 1= p 3; // changes the location that p 1 "points" to m *p 1 = *p 3; // changes the value at the location that // p 1 "points" to
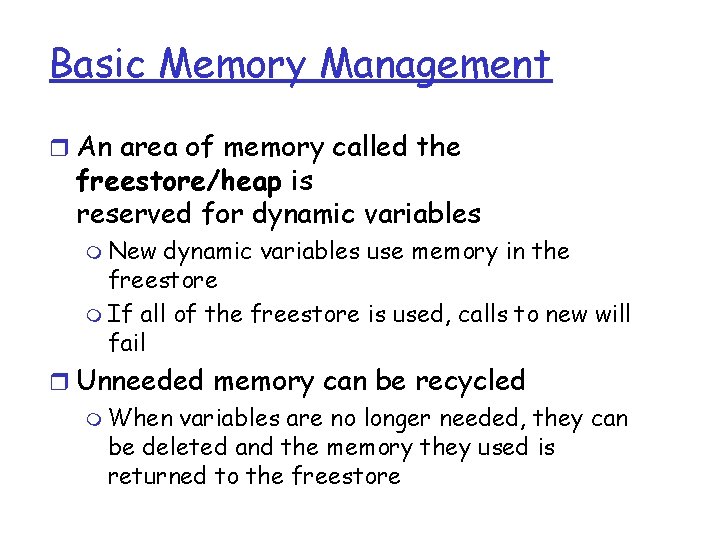
Basic Memory Management r An area of memory called the freestore/heap is reserved for dynamic variables m New dynamic variables use memory in the freestore m If all of the freestore is used, calls to new will fail r Unneeded memory can be recycled m When variables are no longer needed, they can be deleted and the memory they used is returned to the freestore
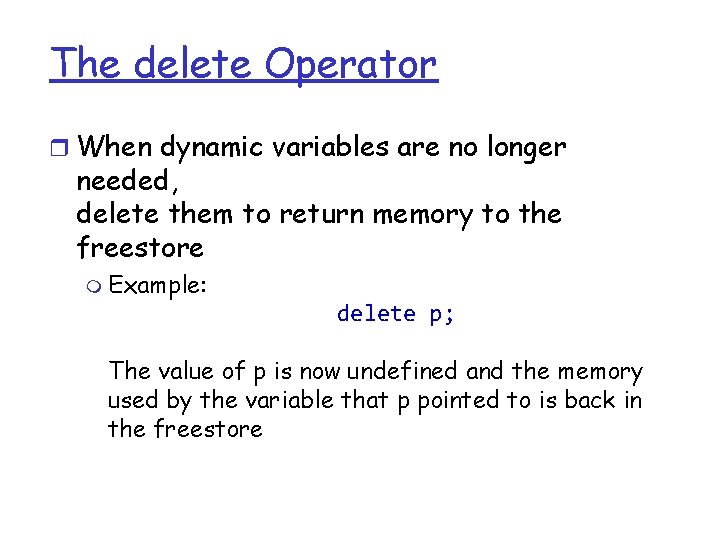
The delete Operator r When dynamic variables are no longer needed, delete them to return memory to the freestore m Example: delete p; The value of p is now undefined and the memory used by the variable that p pointed to is back in the freestore
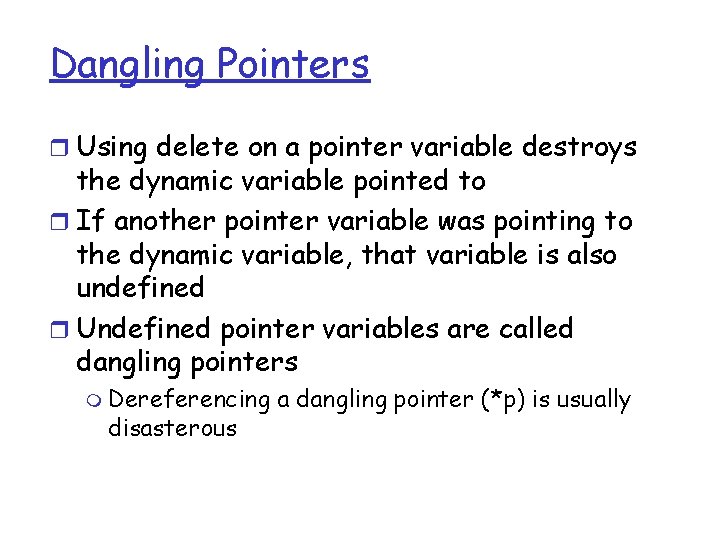
Dangling Pointers r Using delete on a pointer variable destroys the dynamic variable pointed to r If another pointer variable was pointing to the dynamic variable, that variable is also undefined r Undefined pointer variables are called dangling pointers m Dereferencing disasterous a dangling pointer (*p) is usually
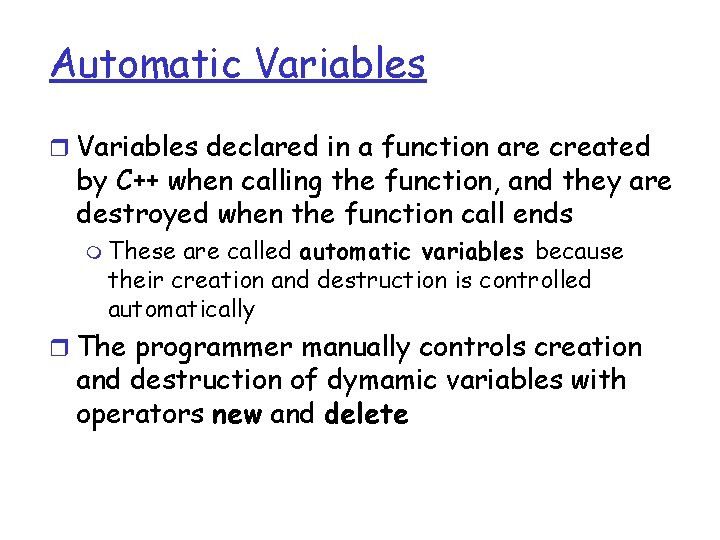
Automatic Variables r Variables declared in a function are created by C++ when calling the function, and they are destroyed when the function call ends m These are called automatic variables because their creation and destruction is controlled automatically r The programmer manually controls creation and destruction of dymamic variables with operators new and delete
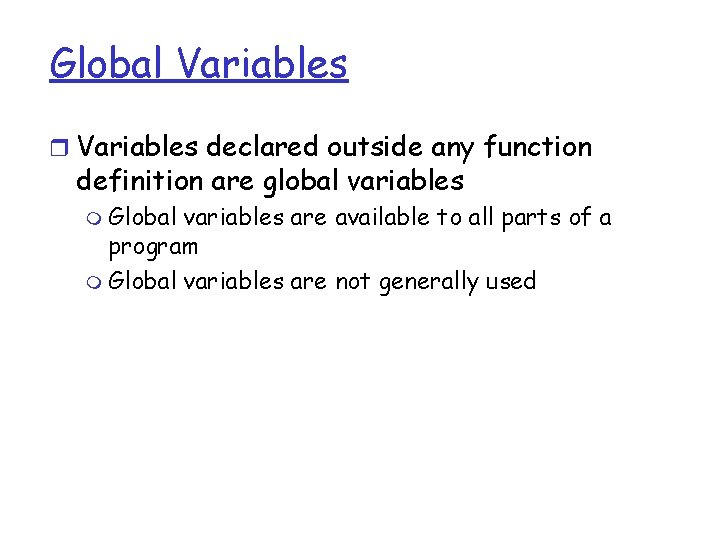
Global Variables r Variables declared outside any function definition are global variables m Global variables are available to all parts of a program m Global variables are not generally used
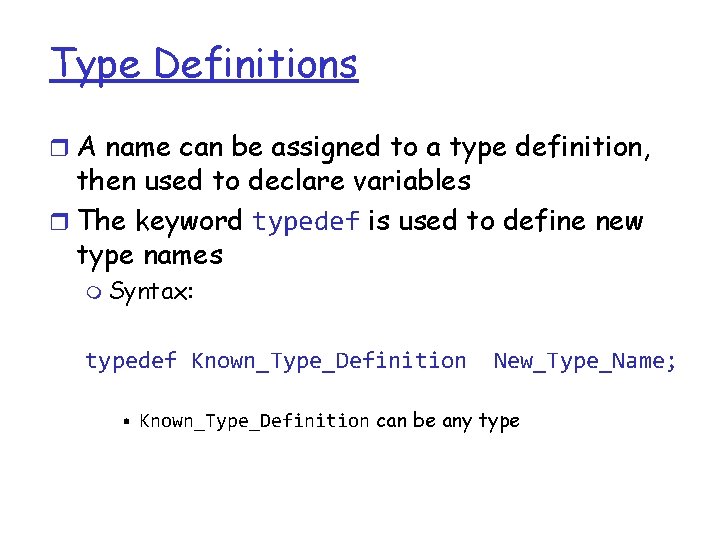
Type Definitions r A name can be assigned to a type definition, then used to declare variables r The keyword typedef is used to define new type names m Syntax: typedef Known_Type_Definition New_Type_Name; • Known_Type_Definition can be any type
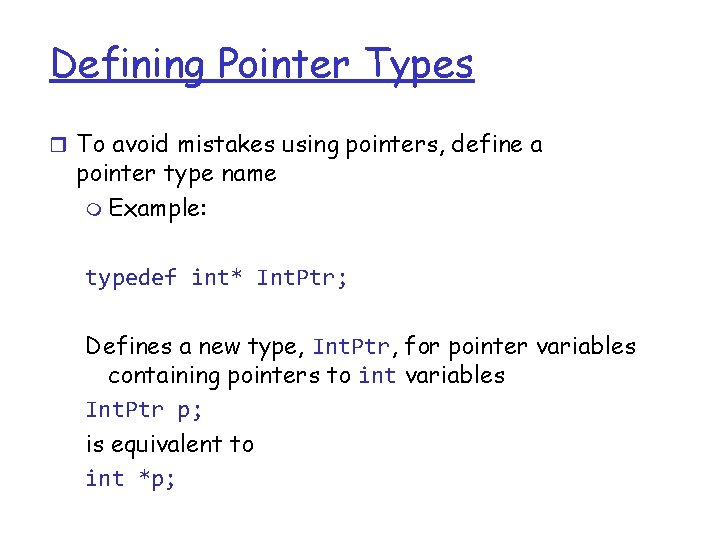
Defining Pointer Types r To avoid mistakes using pointers, define a pointer type name m Example: typedef int* Int. Ptr; Defines a new type, Int. Ptr, for pointer variables containing pointers to int variables Int. Ptr p; is equivalent to int *p;
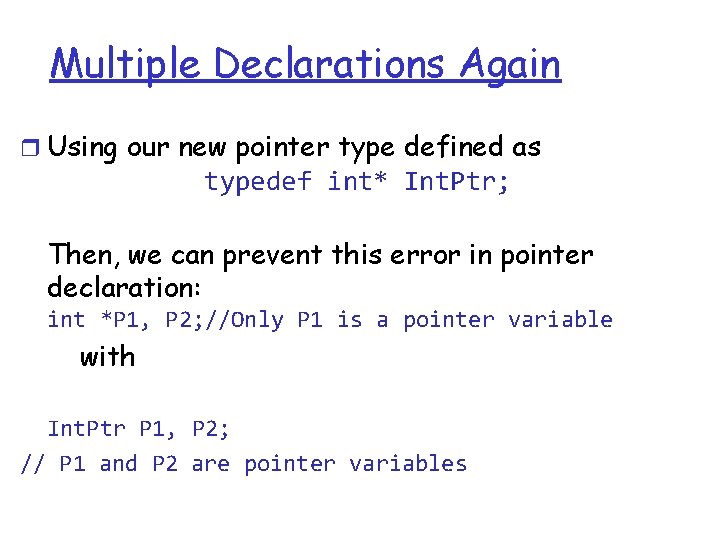
Multiple Declarations Again r Using our new pointer type defined as typedef int* Int. Ptr; Then, we can prevent this error in pointer declaration: int *P 1, P 2; //Only P 1 is a pointer variable with Int. Ptr P 1, P 2; // P 1 and P 2 are pointer variables
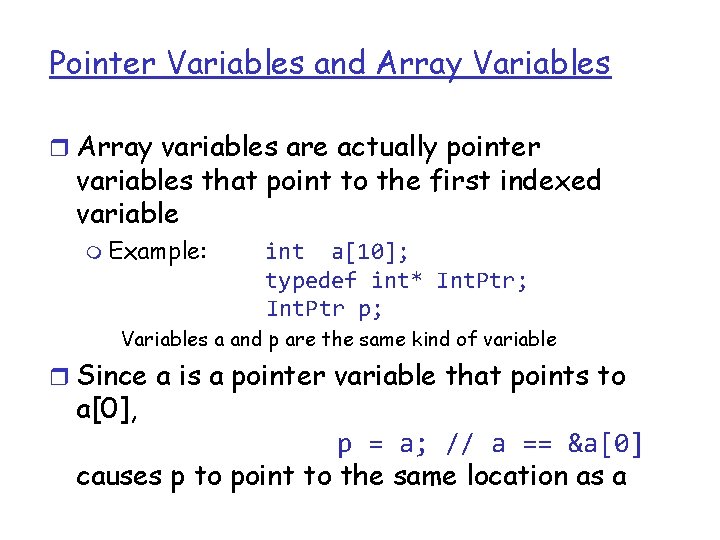
Pointer Variables and Array Variables r Array variables are actually pointer variables that point to the first indexed variable m Example: int a[10]; typedef int* Int. Ptr; Int. Ptr p; Variables a and p are the same kind of variable r Since a is a pointer variable that points to a[0], p = a; // a == &a[0] causes p to point to the same location as a
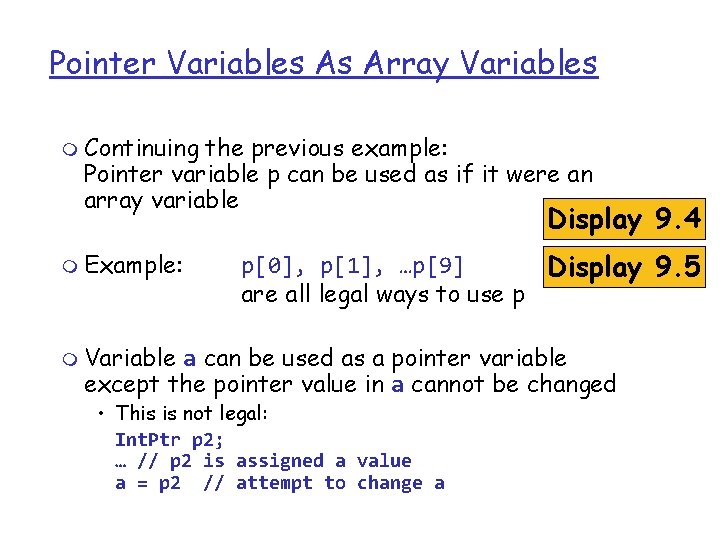
Pointer Variables As Array Variables m Continuing the previous example: Pointer variable p can be used as if it were an array variable Display 9. 4 m Example: p[0], p[1], …p[9] Display 9. 5 are all legal ways to use p m Variable a can be used as a pointer variable except the pointer value in a cannot be changed • This is not legal: Int. Ptr p 2; … // p 2 is assigned a value a = p 2 // attempt to change a
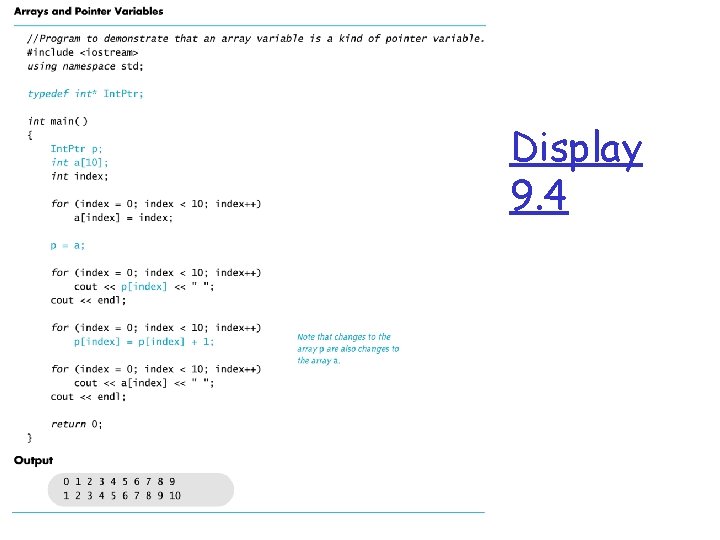
Display 9. 4 Slide 9 - 52
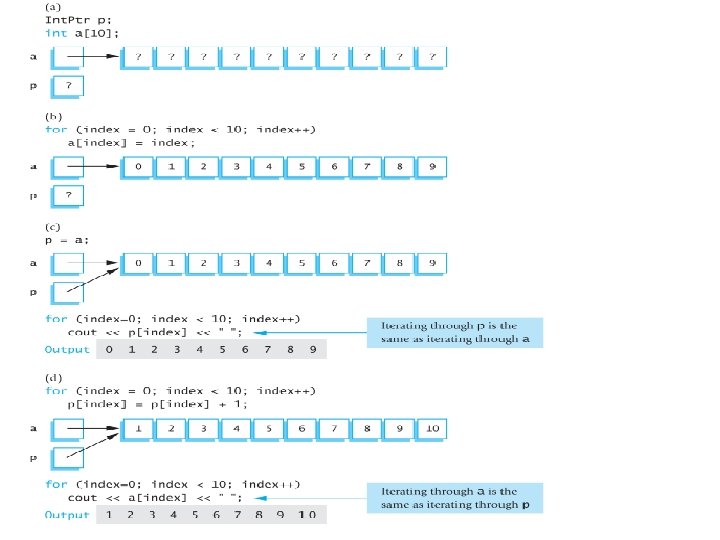
Slide 9 - 53
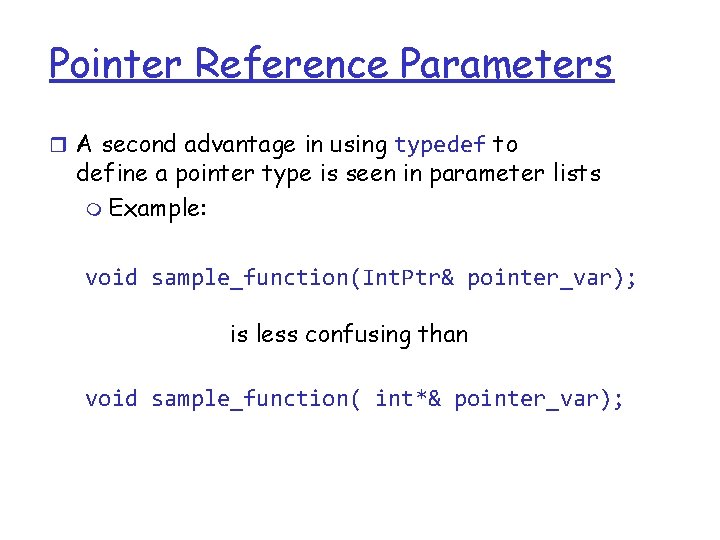
Pointer Reference Parameters r A second advantage in using typedef to define a pointer type is seen in parameter lists m Example: void sample_function(Int. Ptr& pointer_var); is less confusing than void sample_function( int*& pointer_var);
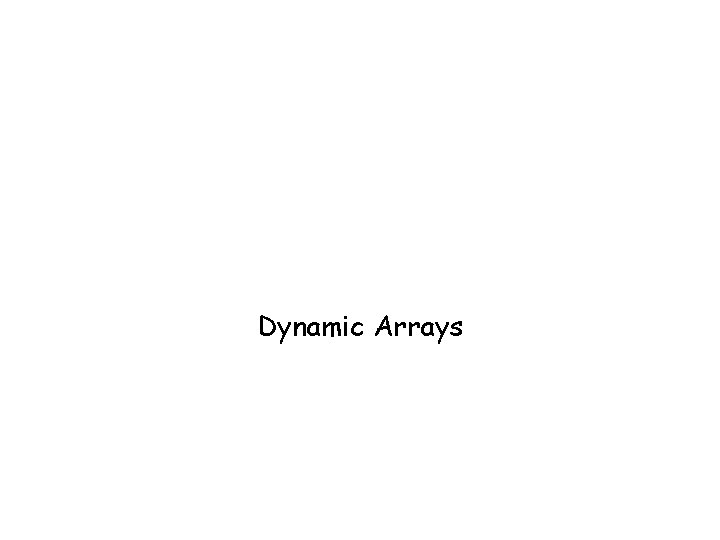
Dynamic Arrays
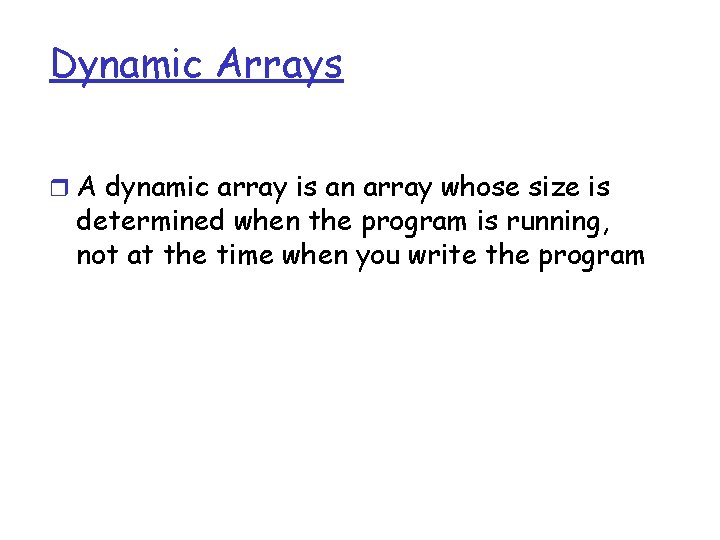
Dynamic Arrays r A dynamic array is an array whose size is determined when the program is running, not at the time when you write the program
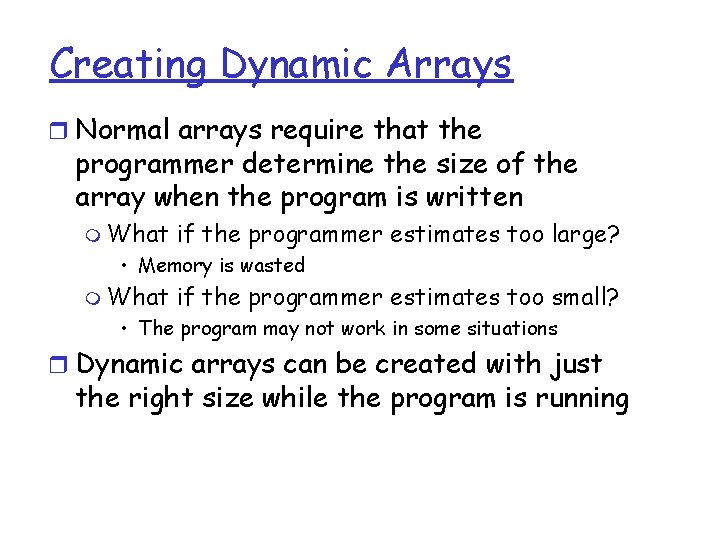
Creating Dynamic Arrays r Normal arrays require that the programmer determine the size of the array when the program is written m What if the programmer estimates too large? • Memory is wasted m What if the programmer estimates too small? • The program may not work in some situations r Dynamic arrays can be created with just the right size while the program is running
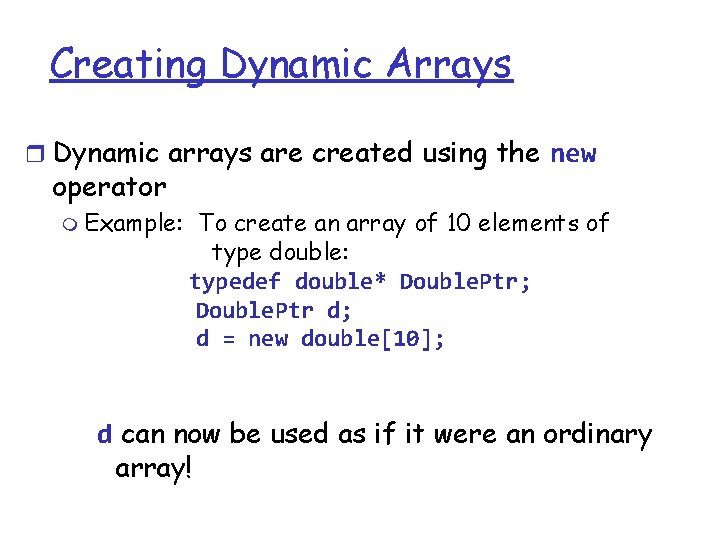
Creating Dynamic Arrays r Dynamic arrays are created using the new operator m Example: To create an array of 10 elements of type double: typedef double* Double. Ptr; Double. Ptr d; d = new double[10]; d can now be used as if it were an ordinary array!
![Dynamic Arrays cont r Pointer variable d is a pointer to d0 r Dynamic Arrays (cont. ) r Pointer variable d is a pointer to d[0] r](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-58.jpg)
Dynamic Arrays (cont. ) r Pointer variable d is a pointer to d[0] r When finished with the array, it should be deleted to return memory to the freestore m Example: delete [ ] d; • The brackets tell C++ a dynamic array is being deleted so it must check the size to know how many indexed variables to remove • If forget to write the brackets, it would tell the computer to Display 9. 6 (1) remove only one variable Display 9. 6 (2)
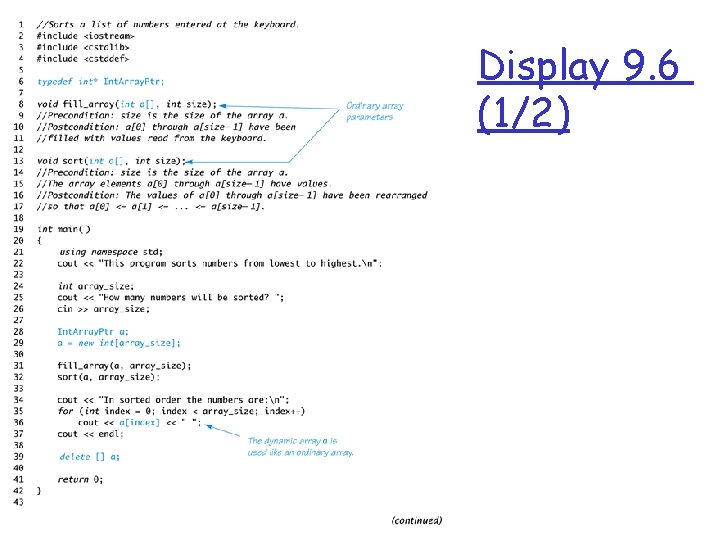
Display 9. 6 (1/2)
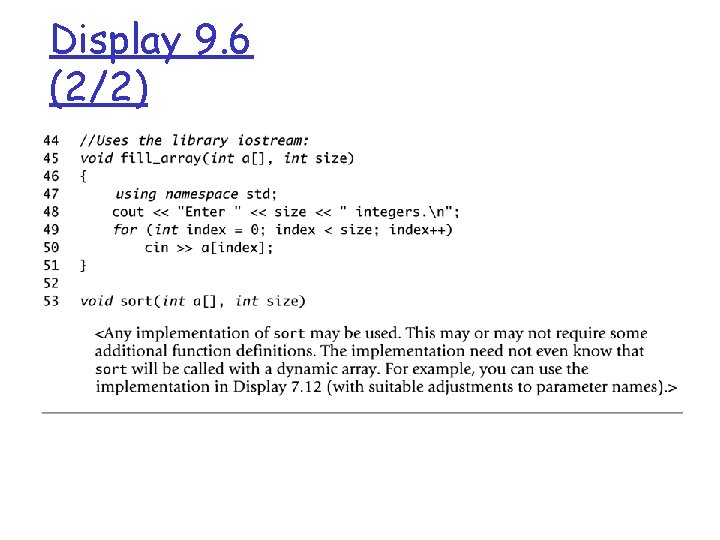
Display 9. 6 (2/2)
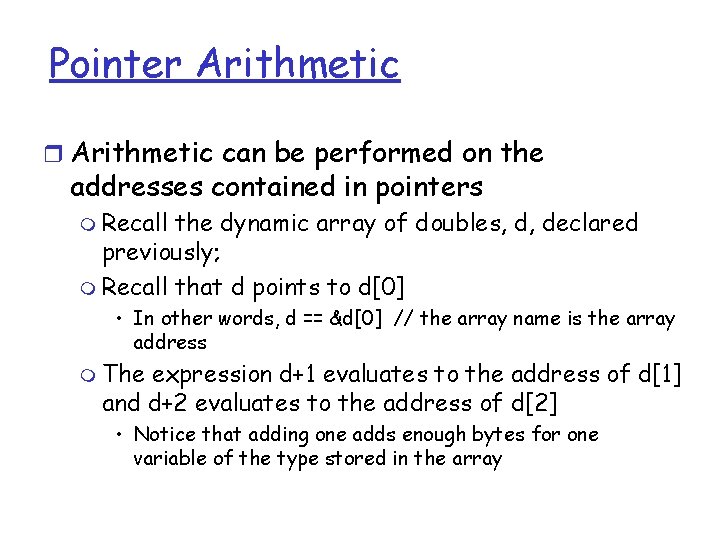
Pointer Arithmetic can be performed on the addresses contained in pointers m Recall the dynamic array of doubles, d, declared previously; m Recall that d points to d[0] • In other words, d == &d[0] // the array name is the array address m The expression d+1 evaluates to the address of d[1] and d+2 evaluates to the address of d[2] • Notice that adding one adds enough bytes for one variable of the type stored in the array
![pointers and arrays 1000 list0 1008 list1 1016 list2 1024 double list3 list1 pointers and arrays 1000 list[0] 1008 list[1] 1016 list[2] 1024 double list[3]; &list[1] ?](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-62.jpg)
pointers and arrays 1000 list[0] 1008 list[1] 1016 list[2] 1024 double list[3]; &list[1] ? 1008 how does the system find out this address? 1000+1*8 1 -63
![pointer arithmetic must take into account the size of the base type double list31 pointer arithmetic must take into account the size of the base type. double list[3]={1.](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-63.jpg)
pointer arithmetic must take into account the size of the base type. double list[3]={1. 0, 1. 1, 1. 2}; double *p; what’s in p after the following operations? p=&list[0]; p=p+2; /*recall each double value takes 8 bytes*/ p=p-1; 1000 1. 0 list[0] 1008 1. 1 list[1] 1016 1. 2 list[2] 1024 Here, implicitly the compiler knows the base type that p is pointing to. p 1 -64
![pointer arithmetic must take into account the size of the base type plist0 pp2 pointer arithmetic must take into account the size of the base type. p=&list[0]; p=p+2;](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-64.jpg)
pointer arithmetic must take into account the size of the base type. p=&list[0]; p=p+2; /*recall each double value takes 8 bytes*/ p=p-1; 1000 list[0] 1008 list[1] 1016 list[2] 1024 p 1 -65
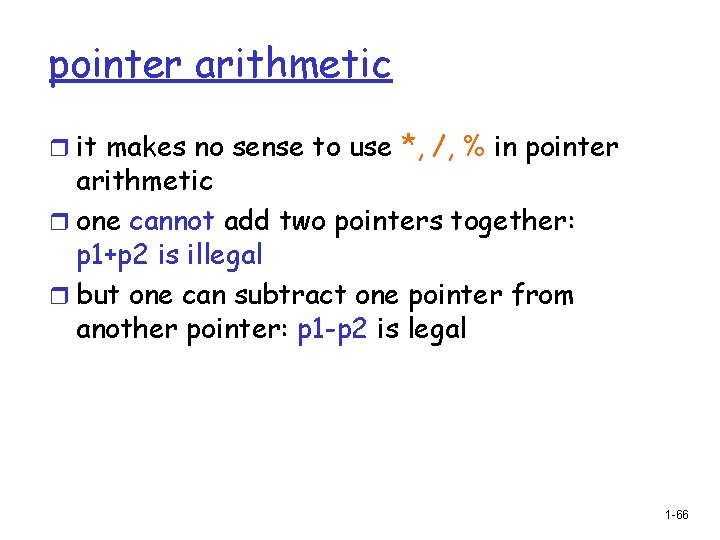
pointer arithmetic r it makes no sense to use *, /, % in pointer arithmetic r one cannot add two pointers together: p 1+p 2 is illegal r but one can subtract one pointer from another pointer: p 1 -p 2 is legal 1 -66
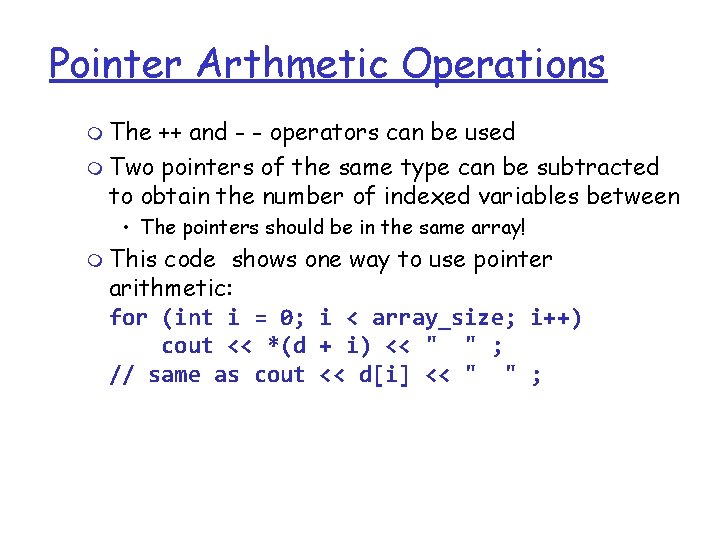
Pointer Arthmetic Operations m The ++ and - - operators can be used m Two pointers of the same type can be subtracted to obtain the number of indexed variables between • The pointers should be in the same array! m This code shows one way to use pointer arithmetic: for (int i = 0; i < array_size; i++) cout << *(d + i) << " " ; // same as cout << d[i] << " " ;
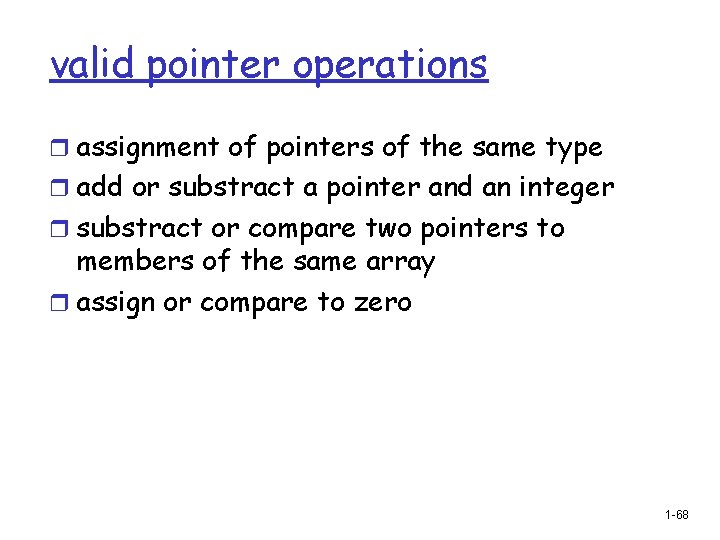
valid pointer operations r assignment of pointers of the same type r add or substract a pointer and an integer r substract or compare two pointers to members of the same array r assign or compare to zero 1 -68
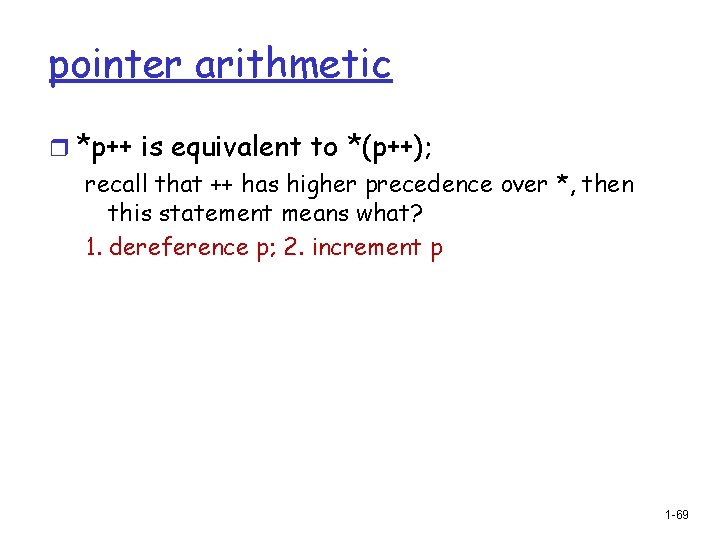
pointer arithmetic r *p++ is equivalent to *(p++); recall that ++ has higher precedence over *, then this statement means what? 1. dereference p; 2. increment p 1 -69
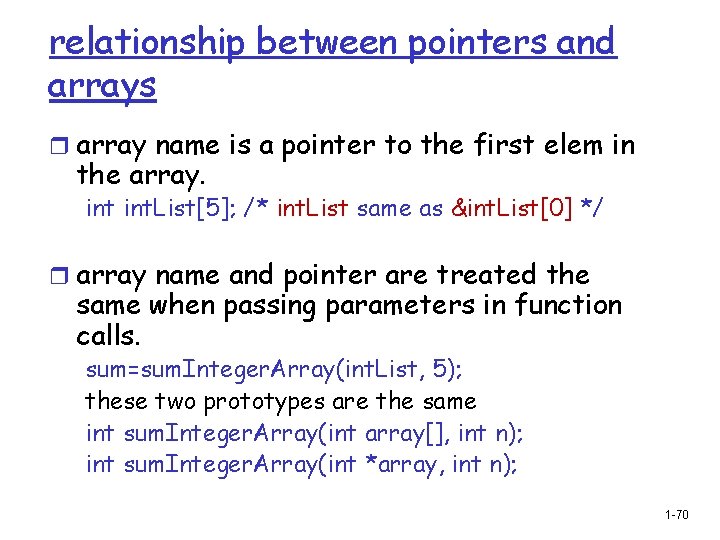
relationship between pointers and arrays r array name is a pointer to the first elem in the array. int. List[5]; /* int. List same as &int. List[0] */ r array name and pointer are treated the same when passing parameters in function calls. sum=sum. Integer. Array(int. List, 5); these two prototypes are the same int sum. Integer. Array(int array[], int n); int sum. Integer. Array(int *array, int n); 1 -70
![differences between pointers and arrays declarations int array5 memory has been allocated for 5 differences between pointers and arrays declarations int array[5]; /*memory has been allocated for 5](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-70.jpg)
differences between pointers and arrays declarations int array[5]; /*memory has been allocated for 5 integers*/ int *p; /*memory has been allocated for 1 pointer to integer, but content or value of this pointer is some garbage number initially. */ 1 -71
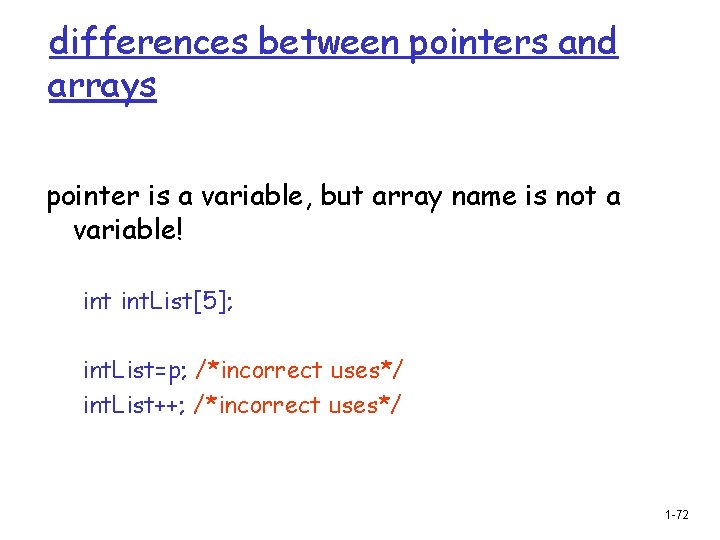
differences between pointers and arrays pointer is a variable, but array name is not a variable! int. List[5]; int. List=p; /*incorrect uses*/ int. List++; /*incorrect uses*/ 1 -72
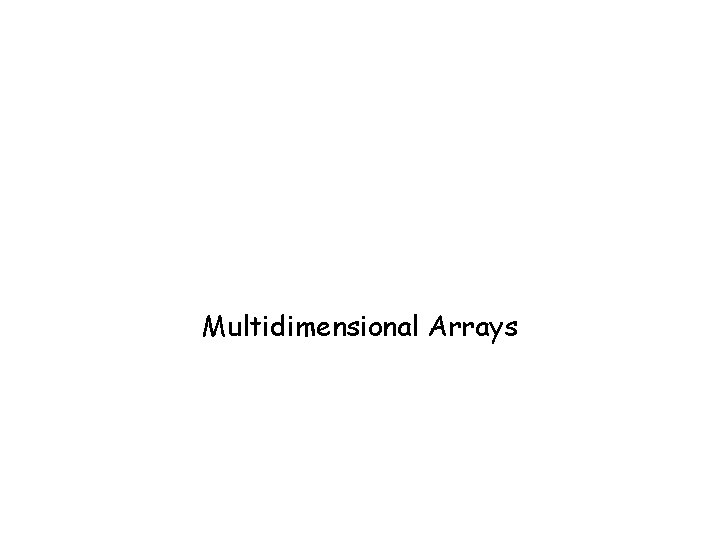
Multidimensional Arrays
![MultiDimensional Arrays r C allows arrays with multiple index values m char page 30 Multi-Dimensional Arrays r C++ allows arrays with multiple index values m char page [30]](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-73.jpg)
Multi-Dimensional Arrays r C++ allows arrays with multiple index values m char page [30] [100]; declares an array of characters named page • page has two index values: The first ranges from 0 to 29 The second ranges from 0 to 99 m Each index in enclosed in its own brackets m Page can be visualized as an array of 30 rows and 100 columns
![Index Values of page r The indexed variables for array page are page00 page01 Index Values of page r The indexed variables for array page are page[0][0], page[0][1],](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-74.jpg)
Index Values of page r The indexed variables for array page are page[0][0], page[0][1], …, page[0][99] page[1][0], page[1][1], …, page[1][99] … page[29][0], page[29][1], … , page[29][99] r page is actually an array of size 30 m page's base type is an array of 100 characters m A Multidimensional array is really an array of arrays.
![Multidimensional Array Parameters r Recall when declaring a as a parameter size is a Multidimensional Array Parameters r Recall when declaring a[] as a parameter, size is a](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-75.jpg)
Multidimensional Array Parameters r Recall when declaring a[] as a parameter, size is a separate parameter: void display_line(const char a[ ], int size); r C++ treats page as an array of arrays m void display_page(const char page[ ] [100], int size_dimension_1); r The base type of a multi-dimensional array must be completely specified in the parameter declaration: char a[100]
![A Two Dimensional Array in C is an array of arrays int a45 A A Two Dimensional Array in C++ is an array of arrays: int a[4][5]; A](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-76.jpg)
A Two Dimensional Array in C++ is an array of arrays: int a[4][5]; A two dimensional array is really an array of pointers to arrays Ø When declared this way, it is guaranteed to be in sequential memory. Ø The first index is the row index, the second index is the column index.
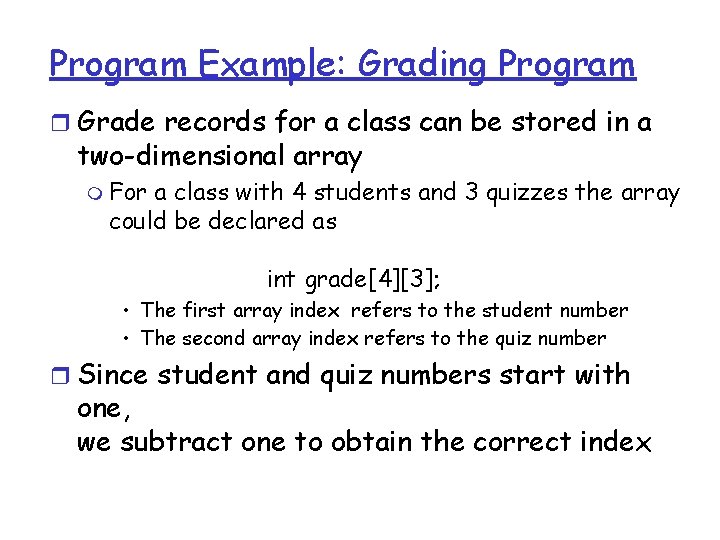
Program Example: Grading Program r Grade records for a class can be stored in a two-dimensional array m For a class with 4 students and 3 quizzes the array could be declared as int grade[4][3]; • The first array index refers to the student number • The second array index refers to the quiz number r Since student and quiz numbers start with one, we subtract one to obtain the correct index
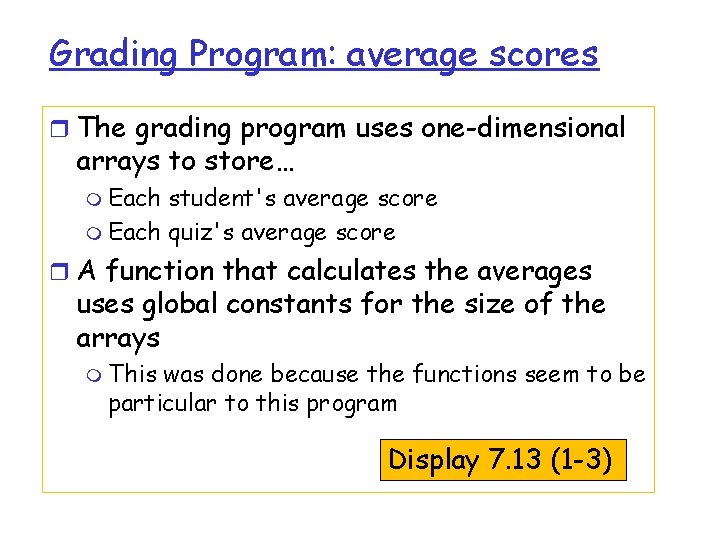
Grading Program: average scores r The grading program uses one-dimensional arrays to store… m Each student's average score m Each quiz's average score r A function that calculates the averages uses global constants for the size of the arrays m This was done because the functions seem to be particular to this program Display 7. 13 (1 -3)
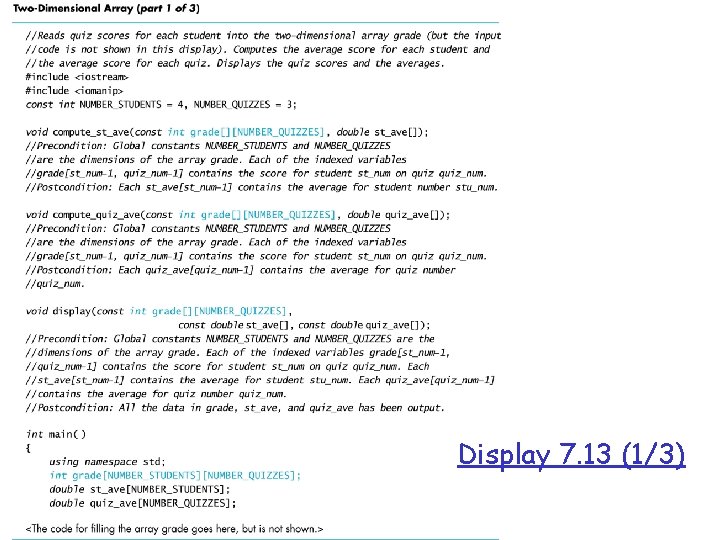
Display 7. 13 (1/3)
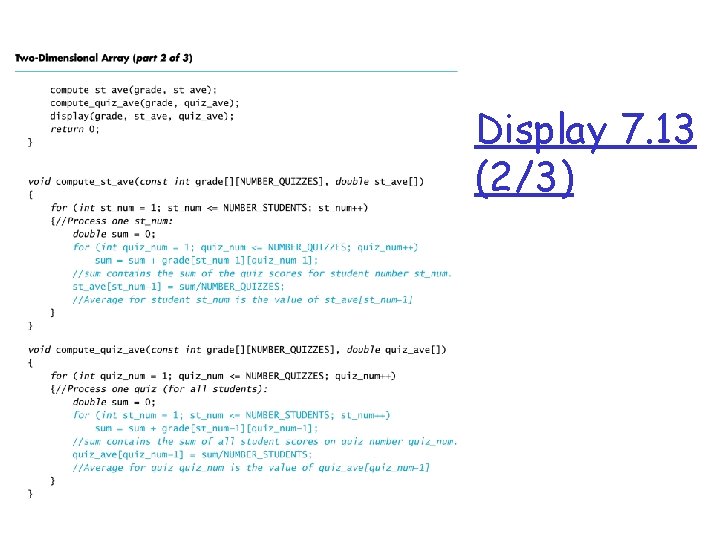
Display 7. 13 (2/3)
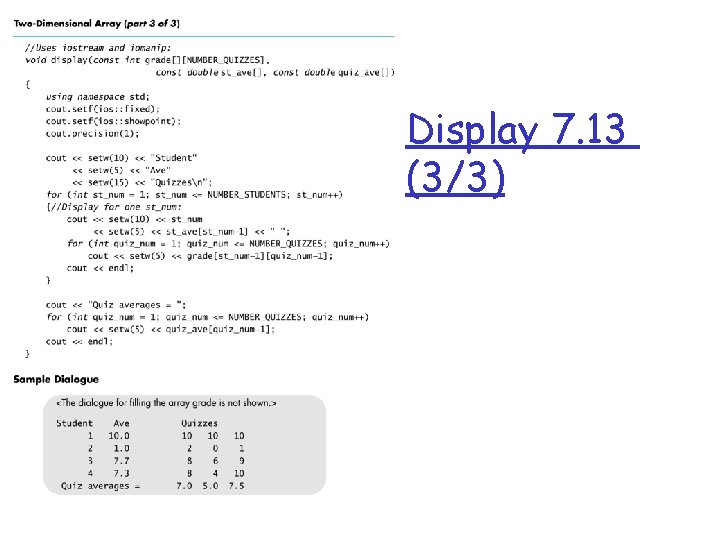
Display 7. 13 (3/3)
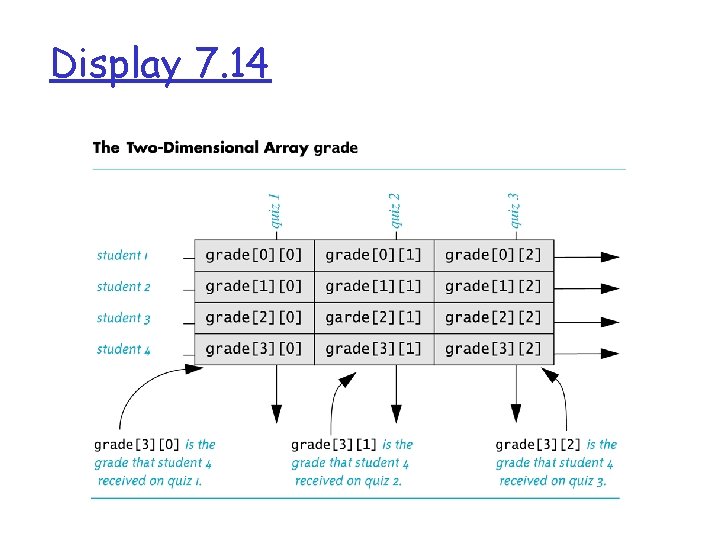
Display 7. 14
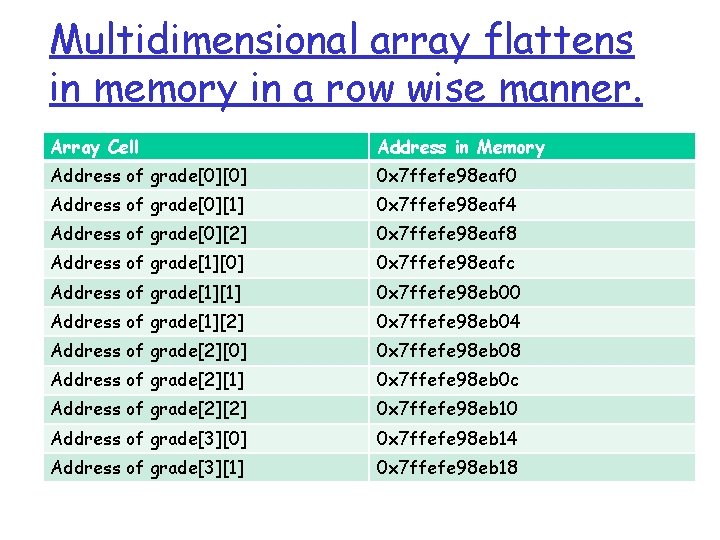
Multidimensional array flattens in memory in a row wise manner. Array Cell Address in Memory Address of grade[0][0] 0 x 7 ffefe 98 eaf 0 Address of grade[0][1] 0 x 7 ffefe 98 eaf 4 Address of grade[0][2] 0 x 7 ffefe 98 eaf 8 Address of grade[1][0] 0 x 7 ffefe 98 eafc Address of grade[1][1] 0 x 7 ffefe 98 eb 00 Address of grade[1][2] 0 x 7 ffefe 98 eb 04 Address of grade[2][0] 0 x 7 ffefe 98 eb 08 Address of grade[2][1] 0 x 7 ffefe 98 eb 0 c Address of grade[2][2] 0 x 7 ffefe 98 eb 10 Address of grade[3][0] 0 x 7 ffefe 98 eb 14 Address of grade[3][1] 0 x 7 ffefe 98 eb 18
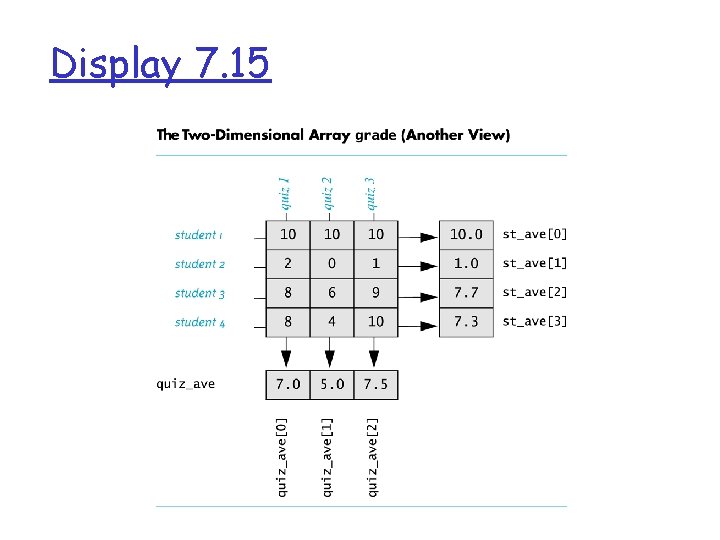
Display 7. 15
![Multidimensional Arrays r Recall that multidimension arrays are just arrays of arrays int a45 Multidimensional Arrays r Recall that multidimension arrays are just arrays of arrays: int a[4][5];](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-85.jpg)
Multidimensional Arrays r Recall that multidimension arrays are just arrays of arrays: int a[4][5]; 1 -86
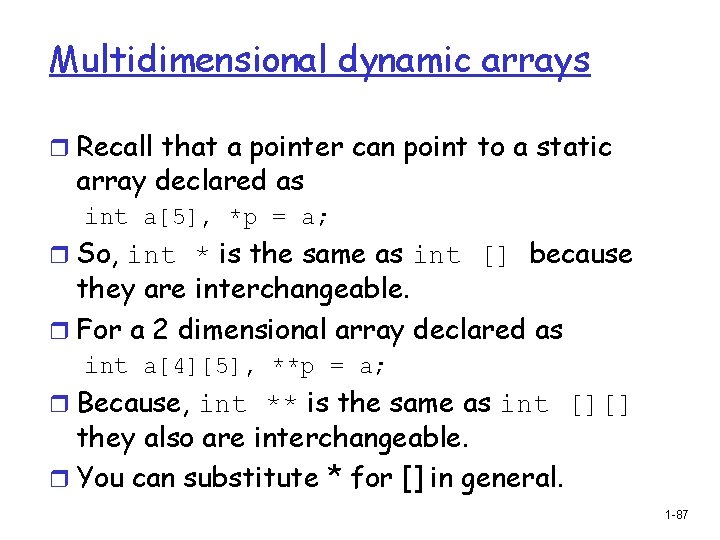
Multidimensional dynamic arrays r Recall that a pointer can point to a static array declared as int a[5], *p = a; r So, int * is the same as int [] because they are interchangeable. r For a 2 dimensional array declared as int a[4][5], **p = a; r Because, int ** is the same as int [][] they also are interchangeable. r You can substitute * for [] in general. 1 -87
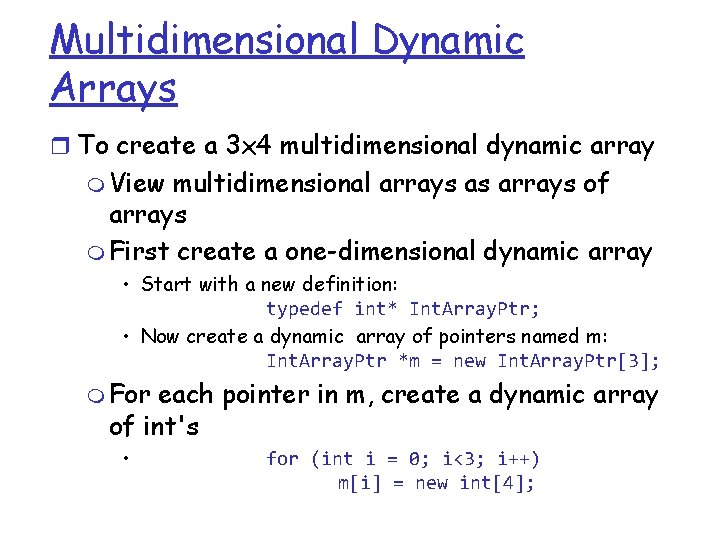
Multidimensional Dynamic Arrays r To create a 3 x 4 multidimensional dynamic array m View multidimensional arrays as arrays of arrays m First create a one-dimensional dynamic array • Start with a new definition: typedef int* Int. Array. Ptr; • Now create a dynamic array of pointers named m: Int. Array. Ptr *m = new Int. Array. Ptr[3]; m For each pointer in m, create a dynamic array of int's • for (int i = 0; i<3; i++) m[i] = new int[4];
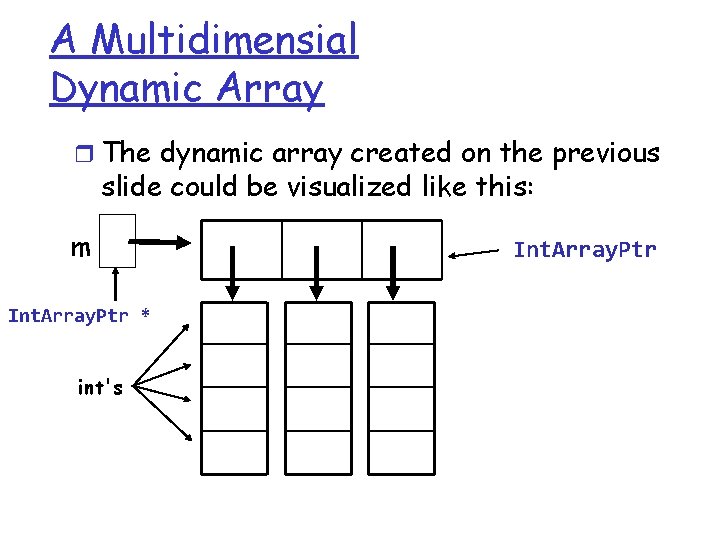
A Multidimensial Dynamic Array r The dynamic array created on the previous slide could be visualized like this: m Int. Array. Ptr * int's Int. Array. Ptr
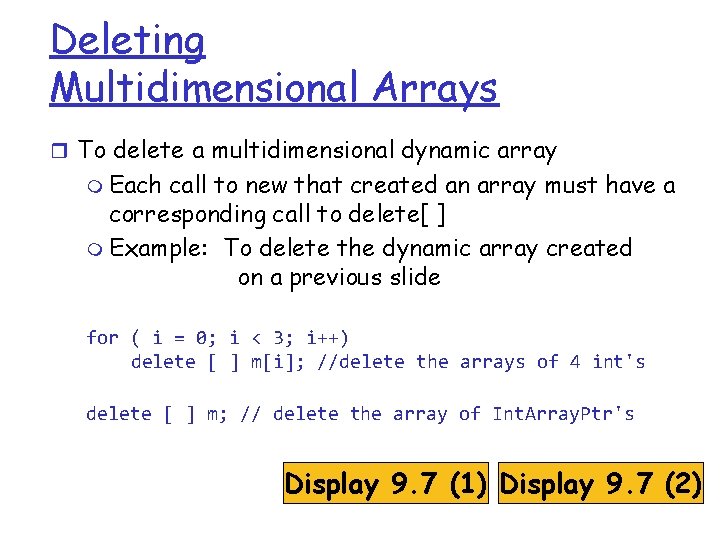
Deleting Multidimensional Arrays r To delete a multidimensional dynamic array m Each call to new that created an array must have a corresponding call to delete[ ] m Example: To delete the dynamic array created on a previous slide for ( i = 0; i < 3; i++) delete [ ] m[i]; //delete the arrays of 4 int's delete [ ] m; // delete the array of Int. Array. Ptr's Display 9. 7 (1) Display 9. 7 (2)
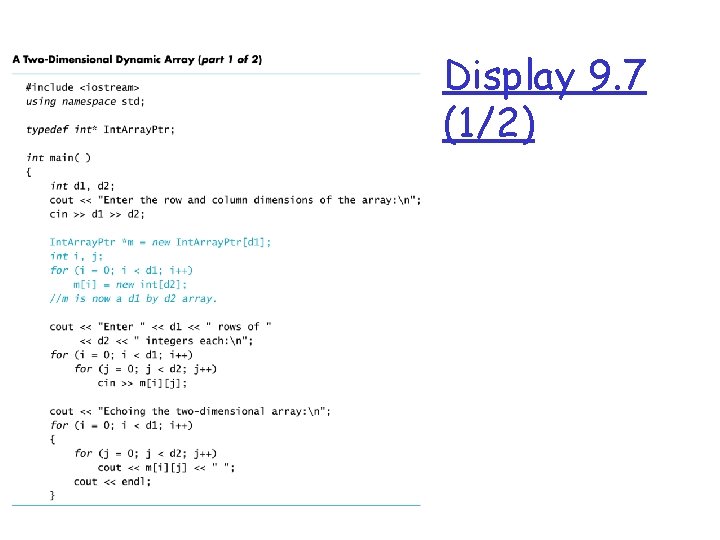
Display 9. 7 (1/2)
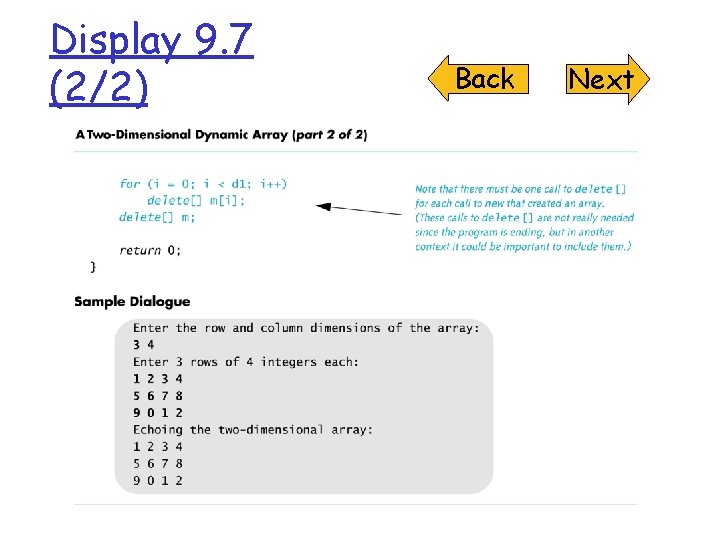
Display 9. 7 (2/2) Back Next
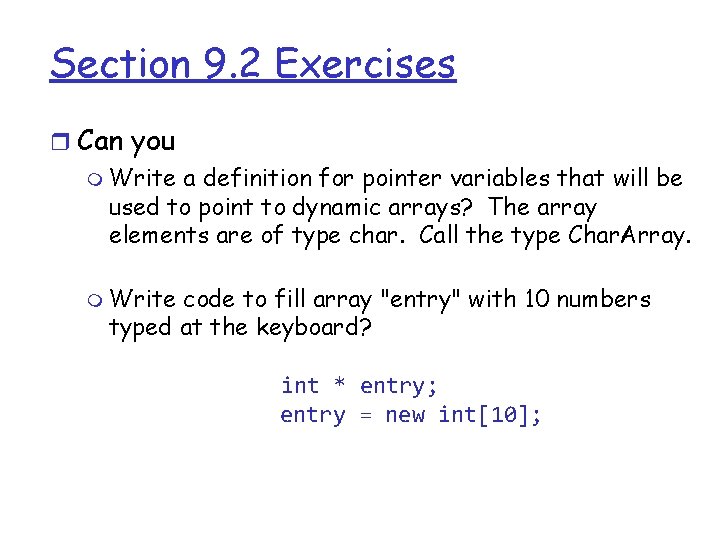
Section 9. 2 Exercises r Can you m Write a definition for pointer variables that will be used to point to dynamic arrays? The array elements are of type char. Call the type Char. Array. m Write code to fill array "entry" with 10 numbers typed at the keyboard? int * entry; entry = new int[10];
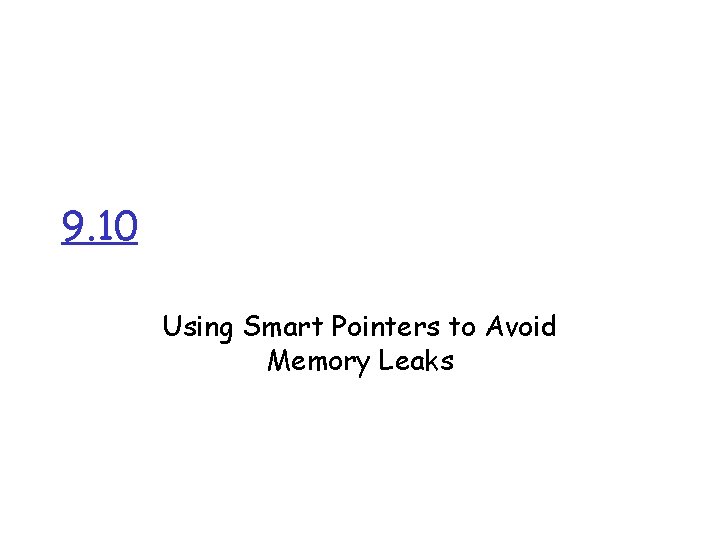
9. 10 Using Smart Pointers to Avoid Memory Leaks
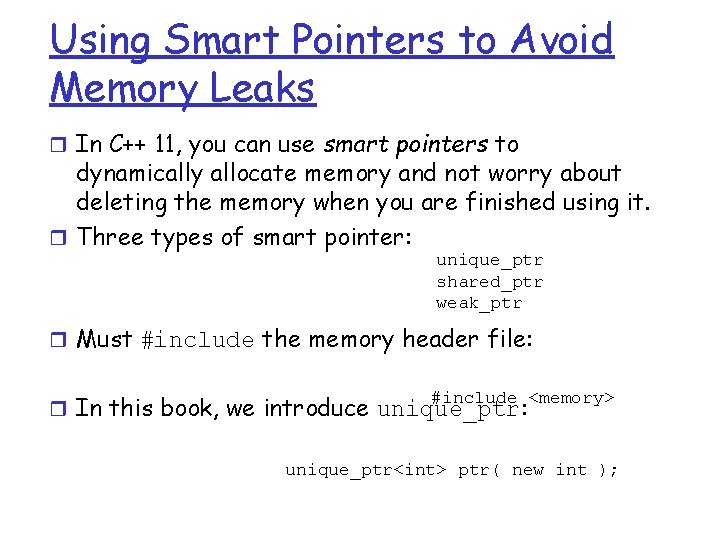
Using Smart Pointers to Avoid Memory Leaks r In C++ 11, you can use smart pointers to dynamically allocate memory and not worry about deleting the memory when you are finished using it. r Three types of smart pointer: unique_ptr shared_ptr weak_ptr r Must #include the memory header file: #include <memory> r In this book, we introduce unique_ptr: unique_ptr<int> ptr( new int );
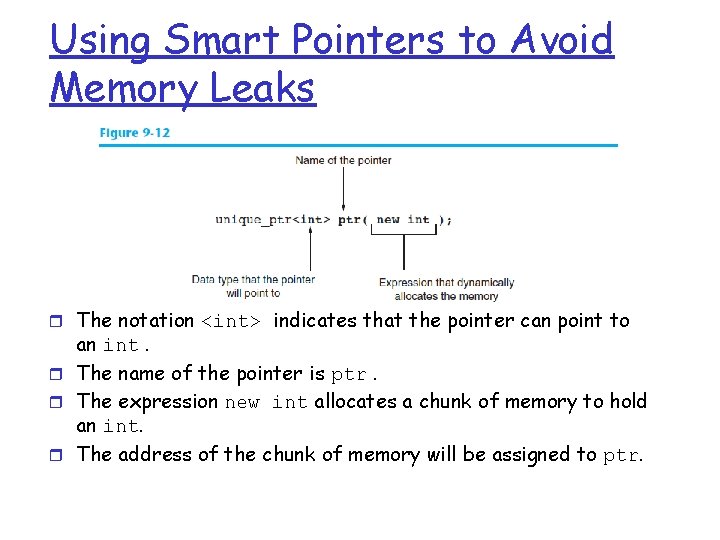
Using Smart Pointers to Avoid Memory Leaks r The notation <int> indicates that the pointer can point to an int. r The name of the pointer is ptr. r The expression new int allocates a chunk of memory to hold an int. r The address of the chunk of memory will be assigned to ptr.
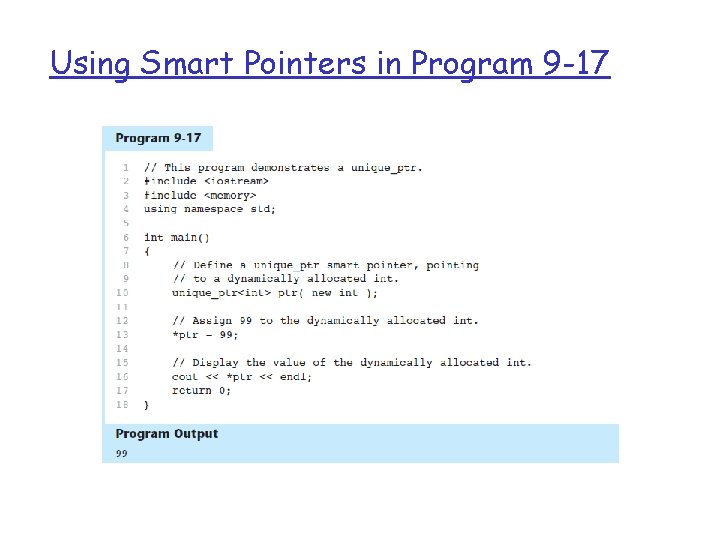
Using Smart Pointers in Program 9 -17
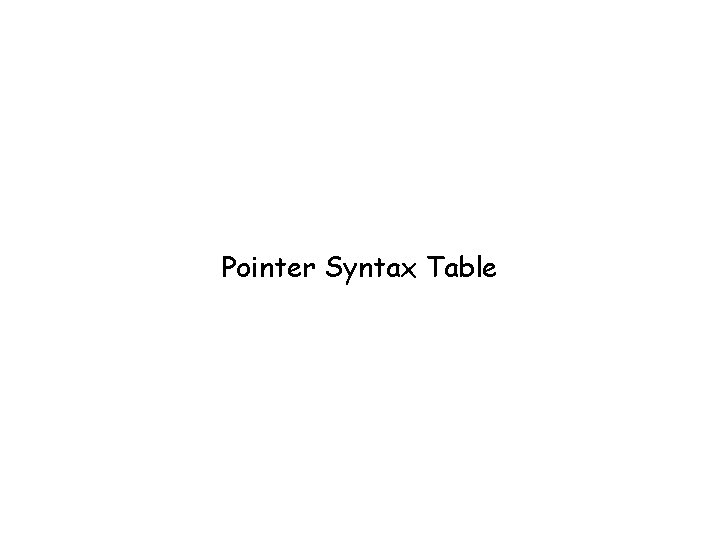
Pointer Syntax Table
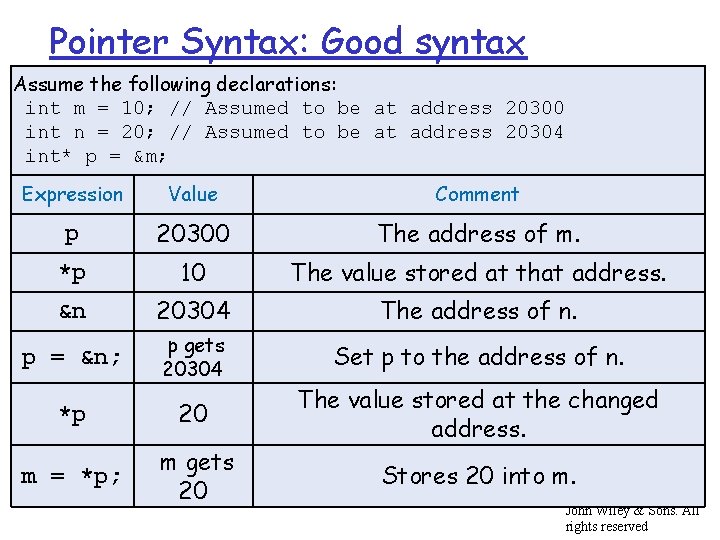
Pointer Syntax: Good syntax Assume the following declarations: int m = 10; // Assumed to be at address 20300 int n = 20; // Assumed to be at address 20304 int* p = &m; Expression Value Comment p 20300 The address of m. *p 10 The value stored at that address. &n 20304 The address of n. p = &n; p gets 20304 Set p to the address of n. *p 20 m = *p; m gets 20 The value stored at the changed address. Big C++ by Cay Horstmann Copyright © 2018 by John Wiley & Sons. All rights reserved Stores 20 into m.
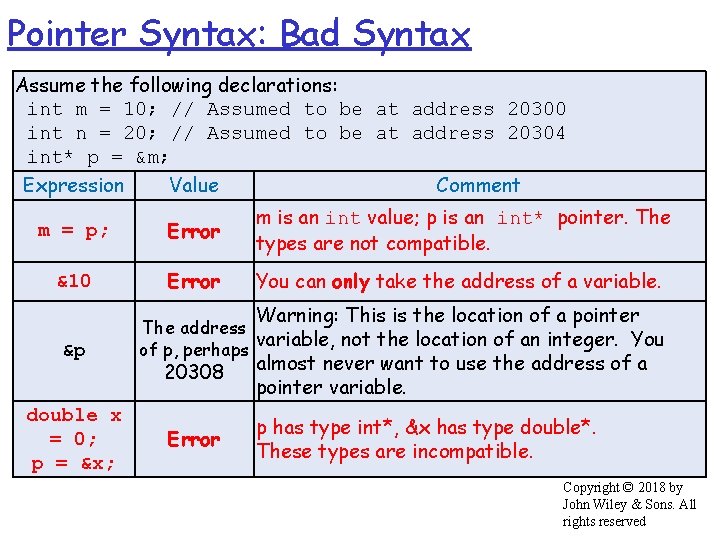
Pointer Syntax: Bad Syntax Assume the following declarations: int m = 10; // Assumed to be at address 20300 int n = 20; // Assumed to be at address 20304 int* p = &m; Expression Value Comment m = p; Error m is an int value; p is an int* pointer. The types are not compatible. &10 Error You can only take the address of a variable. Warning: This is the location of a pointer &p double x = 0; p = &x; The address variable, not the location of an integer. You of p, perhaps 20308 almost never want to use the address of a pointer variable. Error p has type int*, &x has type double*. These types are incompatible. Big C++ by Cay Horstmann Copyright © 2018 by John Wiley & Sons. All rights reserved
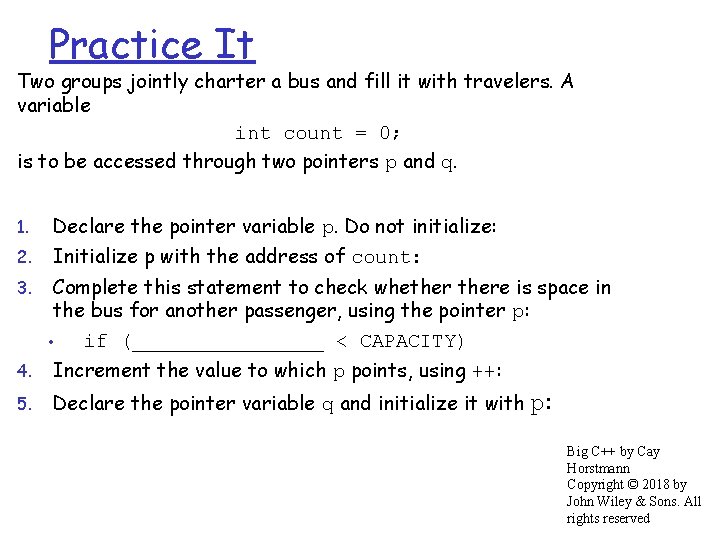
Practice It Two groups jointly charter a bus and fill it with travelers. A variable int count = 0; is to be accessed through two pointers p and q. 1. 2. Declare the pointer variable p. Do not initialize: Initialize p with the address of count: Complete this statement to check whethere is space in the bus for another passenger, using the pointer p: • if (________ < CAPACITY) 4. Increment the value to which p points, using ++: 3. 5. Declare the pointer variable q and initialize it with p: Big C++ by Cay Horstmann Copyright © 2018 by John Wiley & Sons. All rights reserved
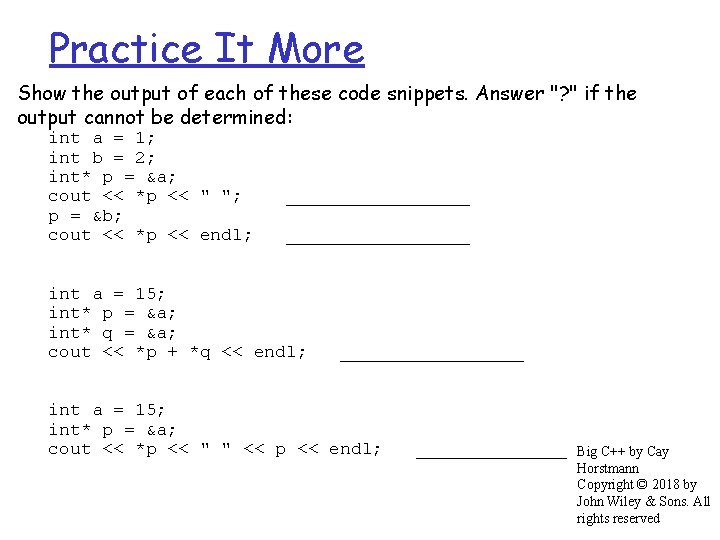
Practice It More Show the output of each of these code snippets. Answer "? " if the output cannot be determined: int a = 1; int b = 2; int* p = &a; cout << *p << " "; p = &b; cout << *p << endl; _________________ int a = 15; int* p = &a; int* q = &a; cout << *p + *q << endl; _________ int a = 15; int* p = &a; cout << *p << " " << p << endl; _______ Big C++ by Cay Horstmann Copyright © 2018 by John Wiley & Sons. All rights reserved
![Array Pointer Ex double d10 Expression d Value Comment 20300 Starting address of Array / Pointer Ex: double d[10] Expression d Value Comment 20300 Starting address of](https://slidetodoc.com/presentation_image_h2/24eb19cbc77062823fbf905e422ece85/image-102.jpg)
Array / Pointer Ex: double d[10] Expression d Value Comment 20300 Starting address of the array, here assumed 20300. The value stored at that address. (The array contains values 0, 1, 4, 9, . . ) *d 0 d + 1 20308 The address of the next double value in the array. A double occupies 8 bytes. d + 3 20324 The address of the element with index 3, obtained by skipping past 3 × 8 bytes. *(a+3) 9 The value stored at address 20324. a[3] 9 The same as *(a + 3) by array/pointer duality. *a + 3 3 The sum of *a and 3. Because there are no parentheses, the * refers only to a. &a[3] Big 3, C++the by Cay The address of the element with index same Horstmann 20324 as a + 3. Copyright © 2018 by John Wiley & Sons. All rights reserved
Gaddis and westad saq
Savitch theorem
Caedb
Importance of pointers in c
C array of pointers to structs
Pointers basics
Which is a good idea for using skip pointers?
Recall skip pointers: what is skip span?
Which is a good idea for using skip pointers
Explicit pointers
Pointers and strings
Java array pointer
Inter function communication in c
Swizzling glsl
Shuffle left algorithm
Hazard pointers
12 pointers
I-need-a-few-pointers-98hpou5
Logbook example science fair
Science fair logbook setup
Pointers are variables that contain
What is the fundamental of pointers?
Pointers are variables that contain as their values
Converging pointers algorithm
Advanced pointers in c
Constant pointer and pointer to constant
Pointers are variables that contain
& vs * in c
Xkcd
Pointers symbol
Skip pointers
Shuffle left algorithm
Advanced pointers in c
& vs * in c
File pointers
Pointers in assembly
Red tent summary
Does nick like gatsby chapter 8
Chapter 10 chemical reactions
Chapter 11 assessment chemistry
Chapter 9 chemical reactions test answers
Similarity ratio definition
Chapter 6 career readiness chapter review answers
Chapter 7 ionic and metallic bonding chapter answer key
Chapter 9 surface water chapter assessment answer key
Representing motion physics answers chapter 2
Chemistry the central science 14th edition
Chapter 7 ionic and metallic bonding answer key
Chapter test a chapter 4 population ecology answer key
Chapter 2 chapter assessment
Summary of the book of philippians chapter by chapter
Properties of ionic bonds
7 ionic and metallic bonding practice problems
Representative metal
Zeta phi beta mip process
Working with young children chapter 1
Wuthering heights summary chapter 4
Wuthering heights chapter 6
Chapter 10 qualitative research designs
Gbyeg
Chapter 16 world war looms vocabulary
Chapter 27 world war 1 and the russian revolution
Chapter 27 lesson 4 world war 1 ends
Chapter 17 section 3 luther leads the reformation
Chapter 32 assessment world history
Chapter 30 section 2 world history
Chapter 8 nationalist revolutions sweep the west
What was the counter-reformation?
Chapter 10 section 2 central america and the caribbean
Ap human geography chapter 11 vocab
World geography chapter 3 climates of the earth answers
Work measurement techniques
Chapter 14 section 1 work and power
Chapter 7 wood joints
Glue
Chapter 19 section 4 wilson fights for peace
Why study financial market
Wnhen
Where the red fern grows chapter 10
Ap psychology chapter 14
The great gatsby quiz
Is chalk natural or manmade
Great expectations chapter 20 quotes
Chapter 6 great expectations summary
Great expectations chapter 8
Great expectations chapter 6
Matthew chapter 7
Chapter 20 weather patterns and severe storms
Mechanical wave
Chapter 15 section 1 types of waves answer key
Chapter 14 study guide vibrations and waves
Wave by eric walters summary
Chapter 24 section 2 watergate answers
Walk two moons chapter 29 summary
Chapter 18 revenue recognition
Chapter 12 speech vocabulary
My family
The total exterior of a business includes the entranceways
Chapter 14 vibrations and waves
Chapter 9 vehicle information
Chapter 6 varieties of drama
Chapter 11 section 4 using water wisely answer key
Using water wisely