PLT 101 Computer Programming CHAPTER 1 INTRODUCTION TO
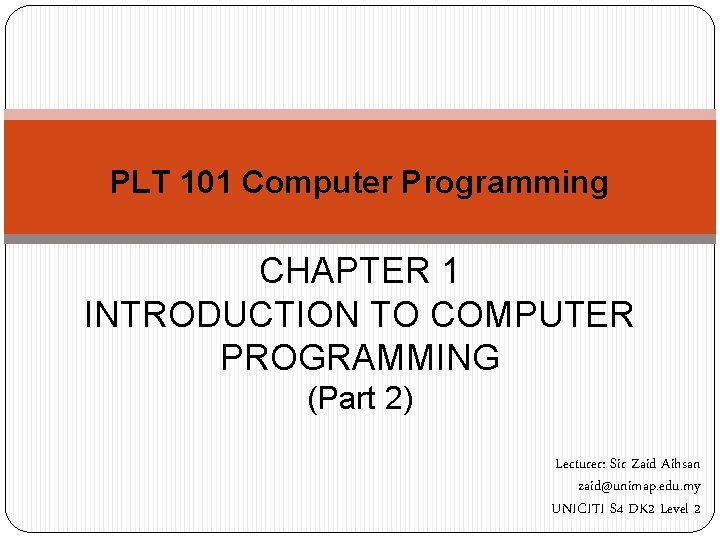
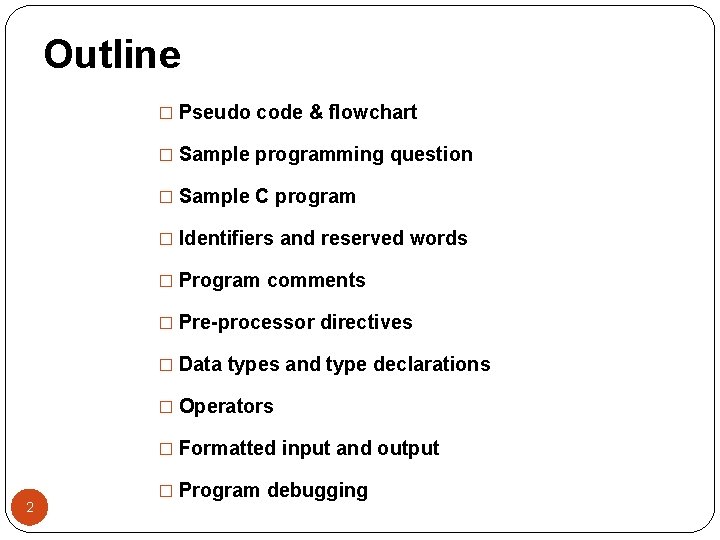
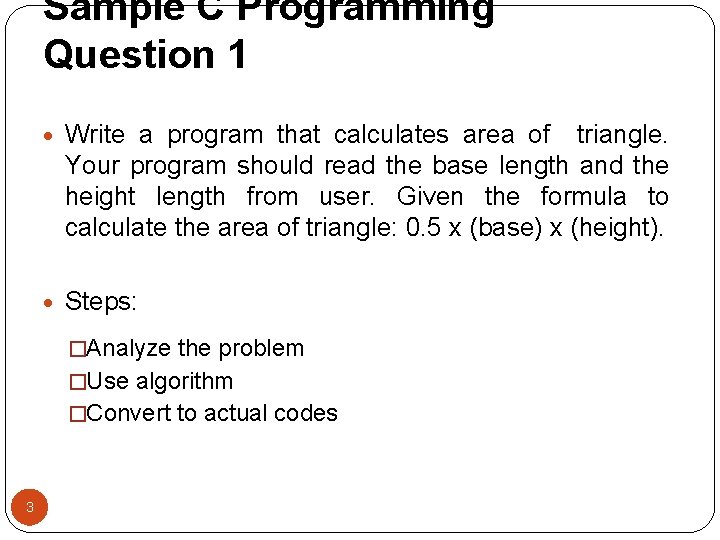
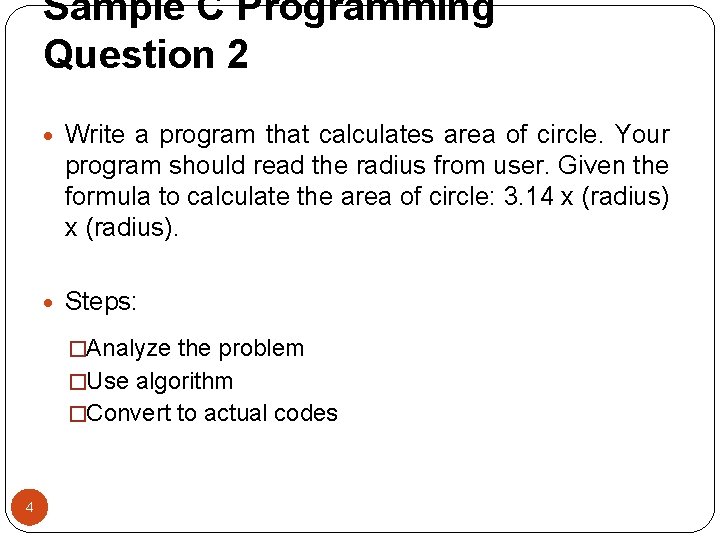
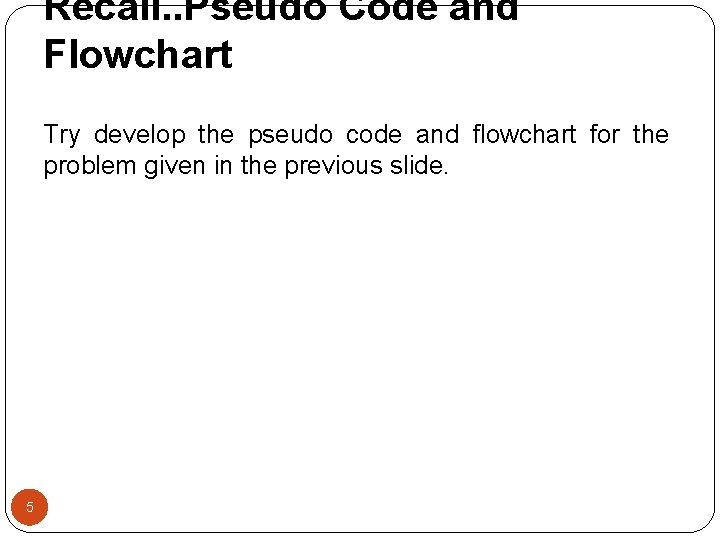
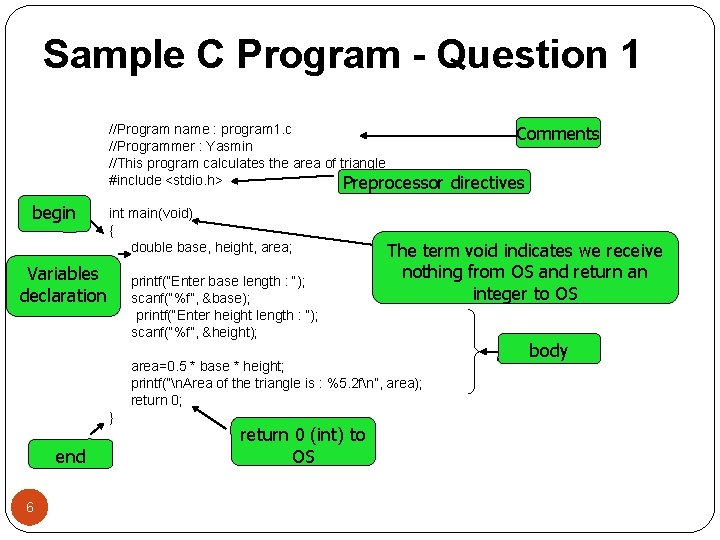
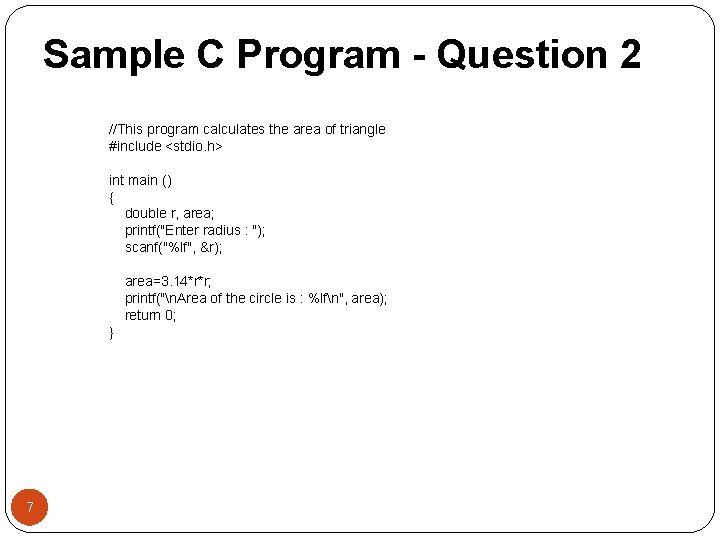
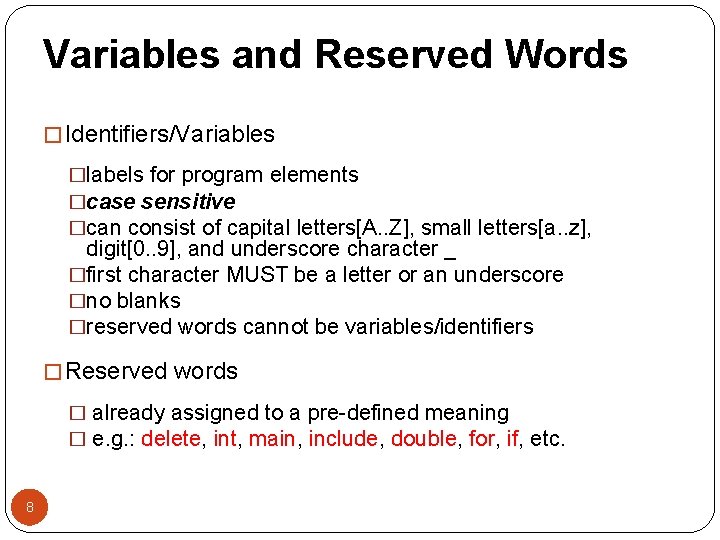
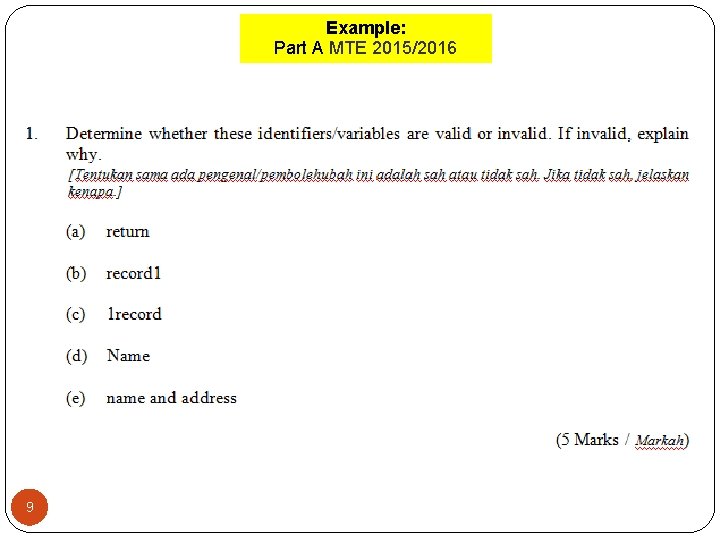
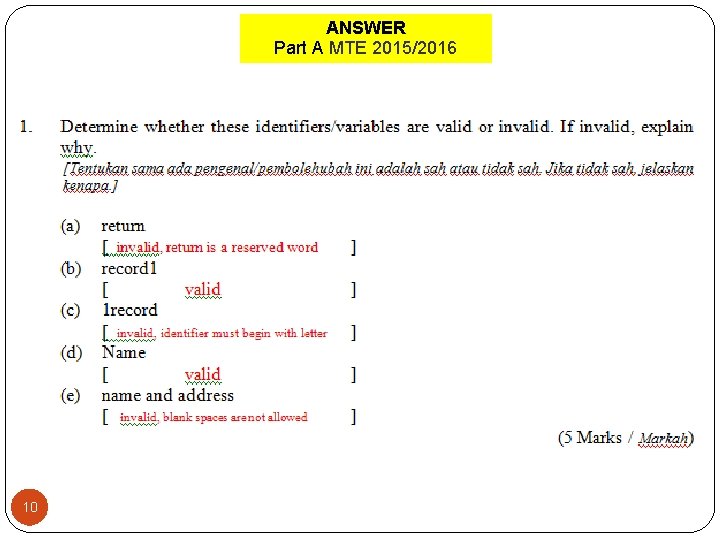
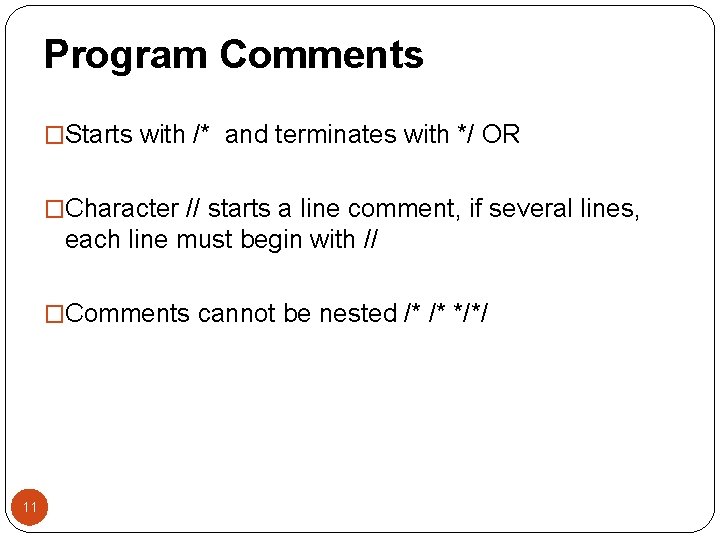
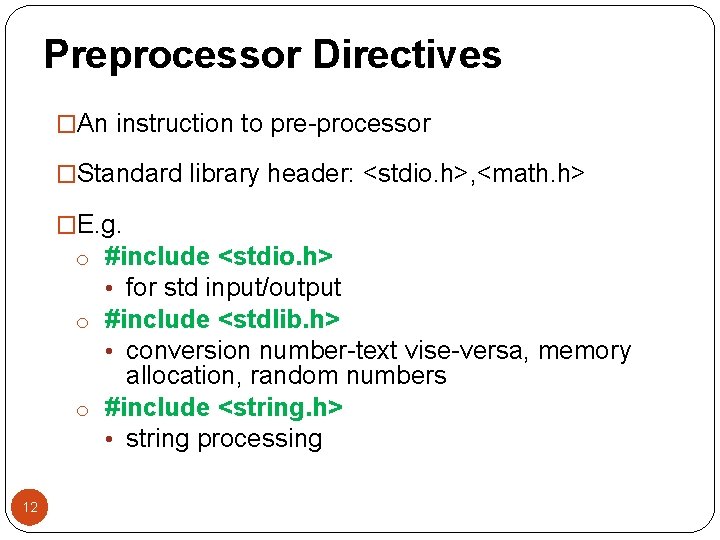
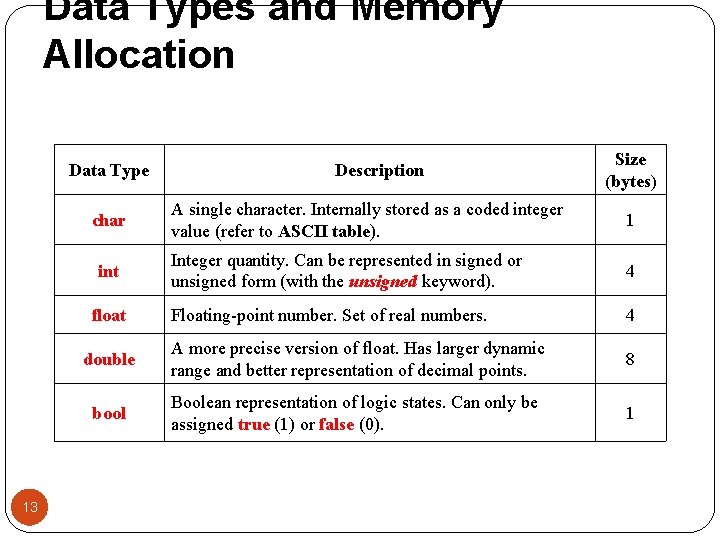
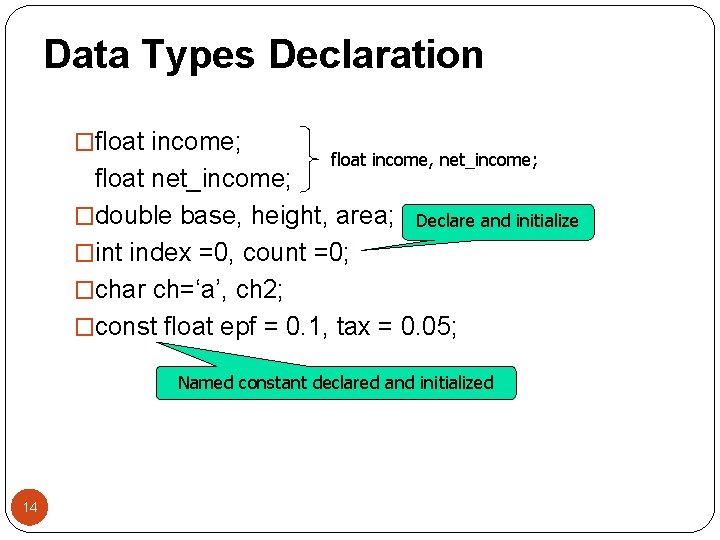
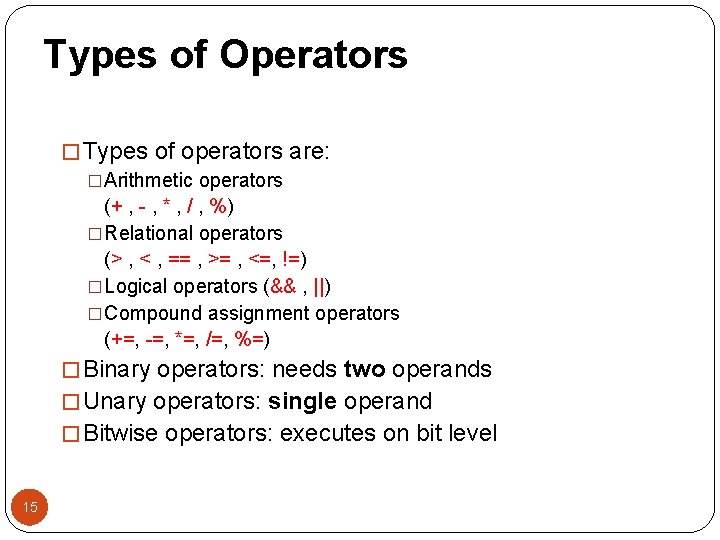
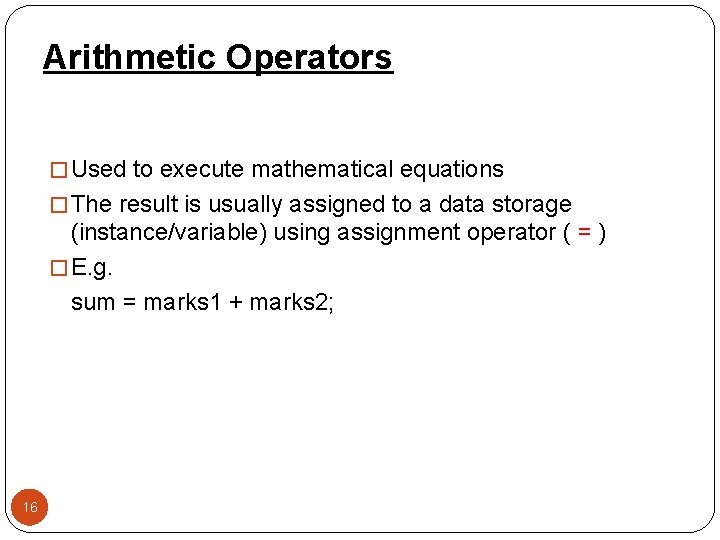
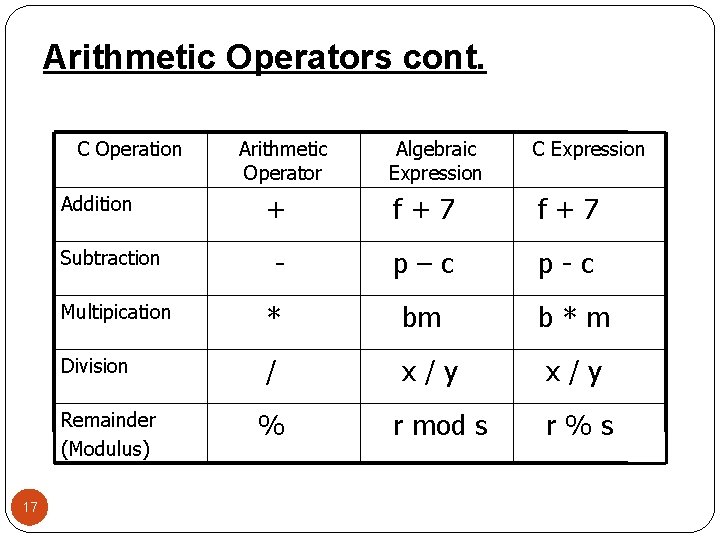
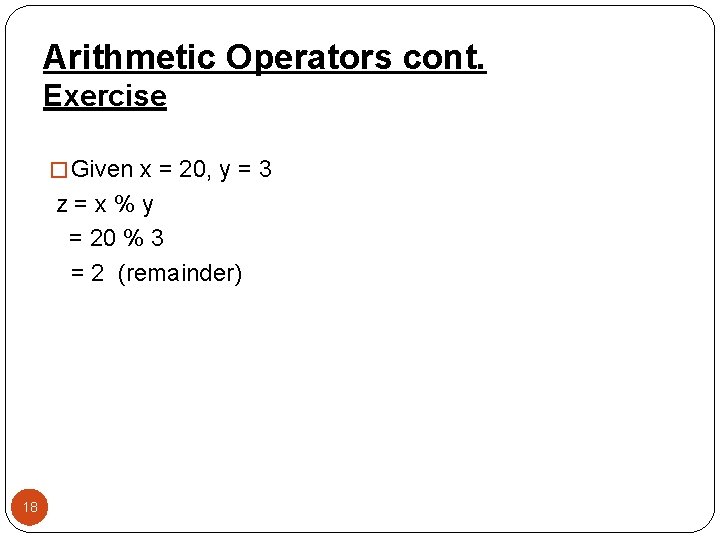
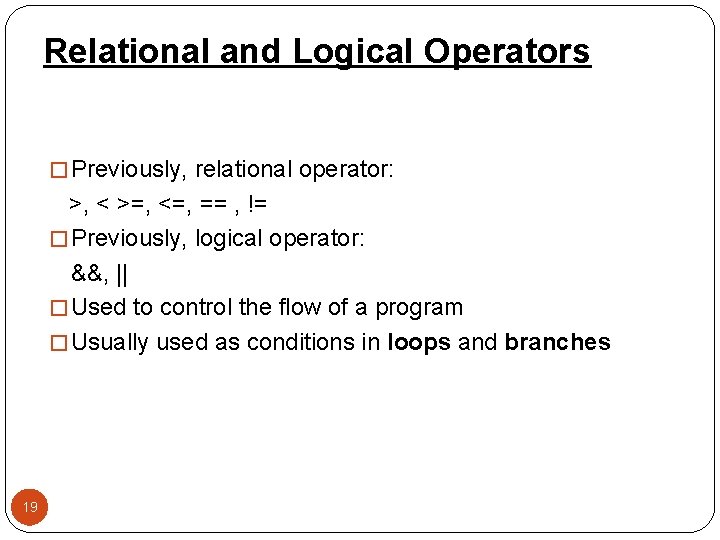
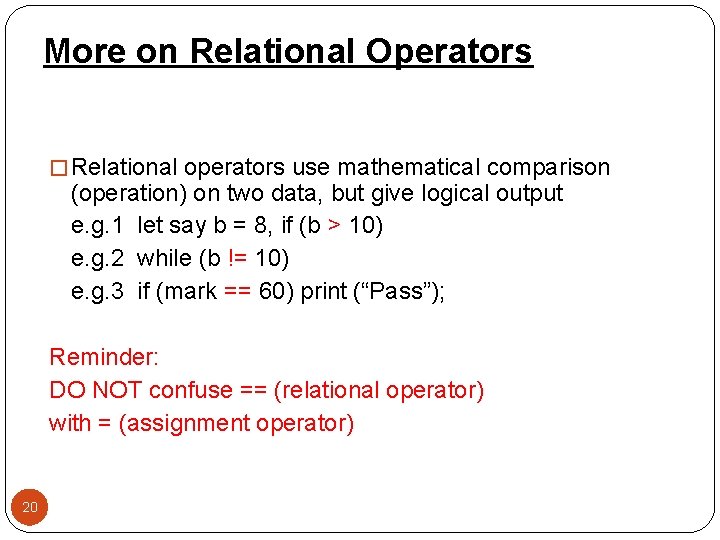
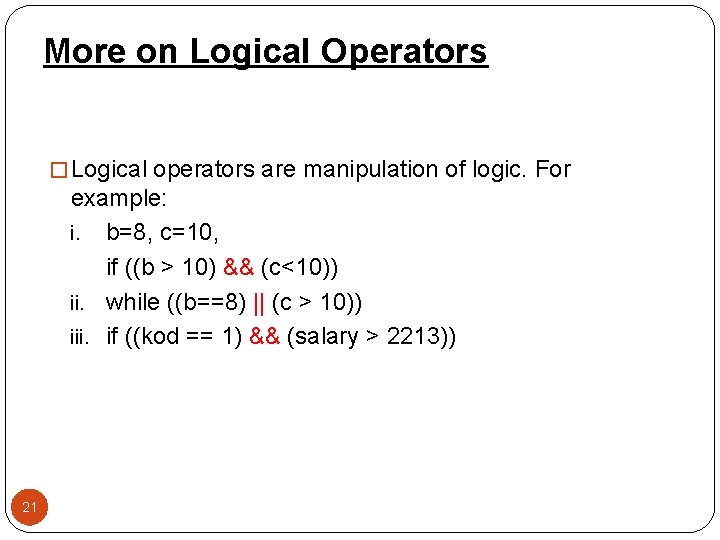
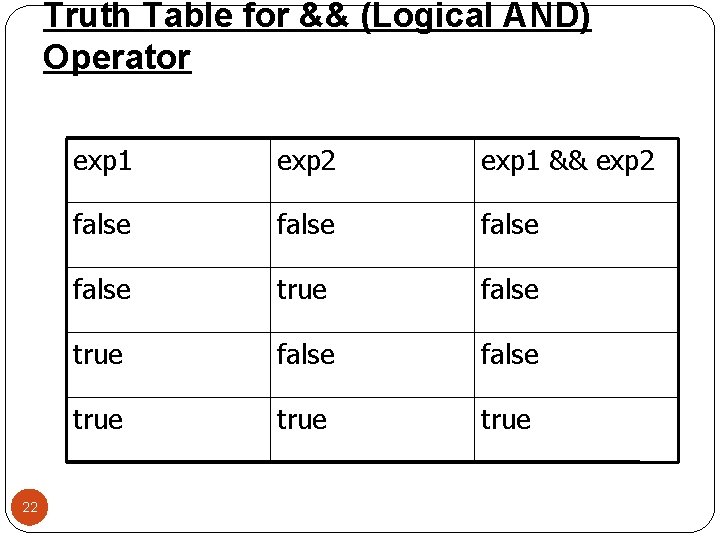
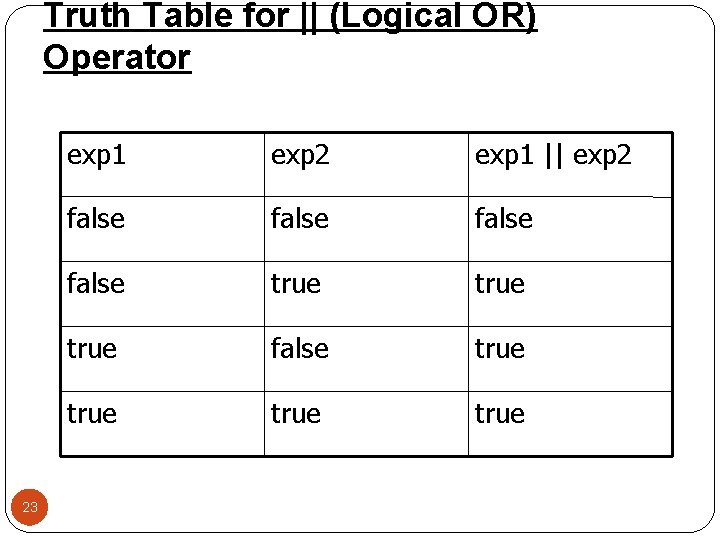
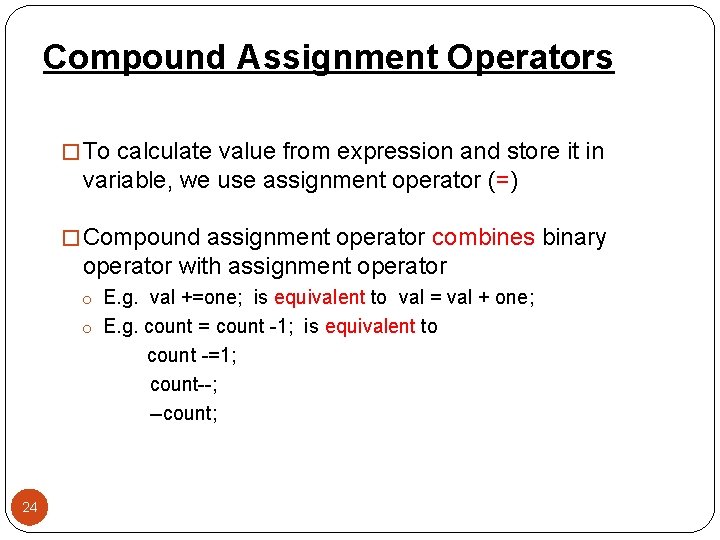
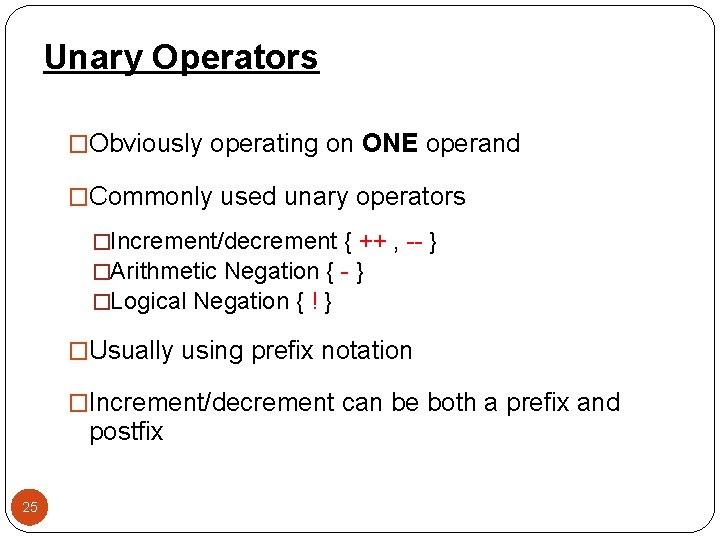
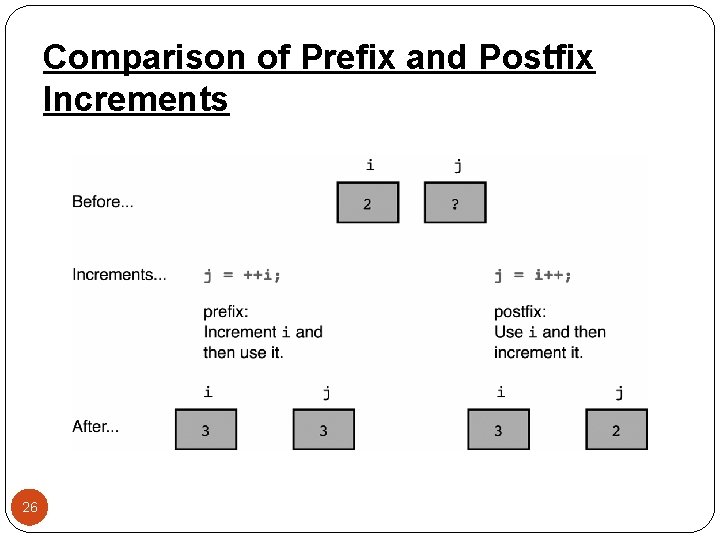
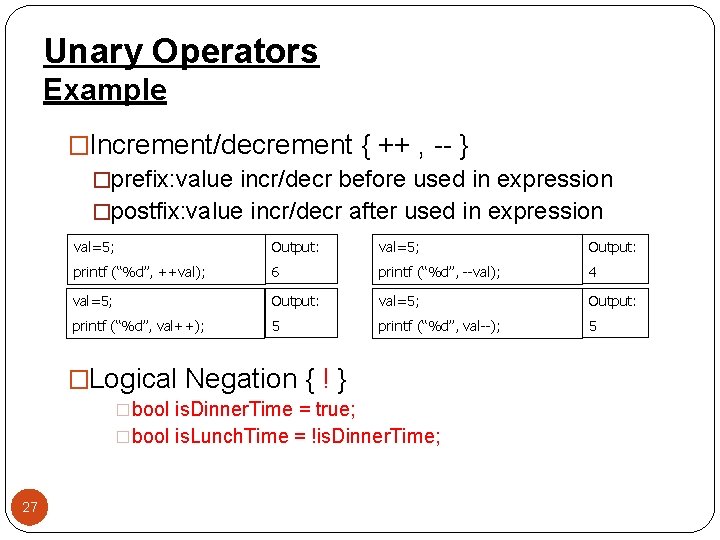
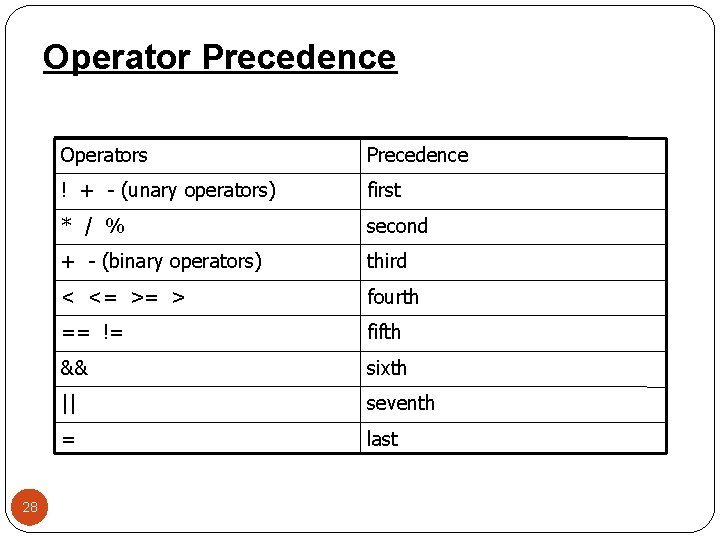
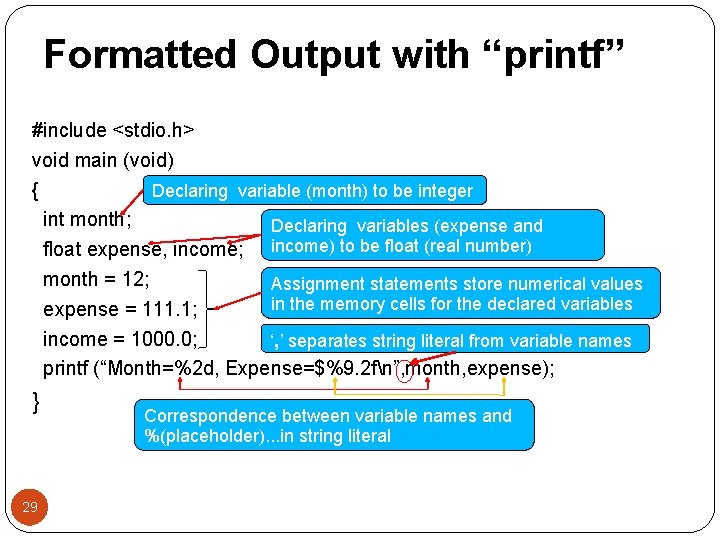
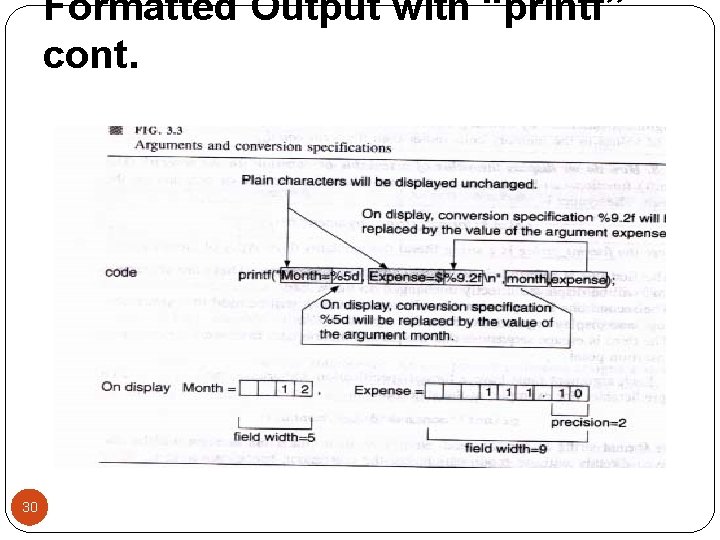
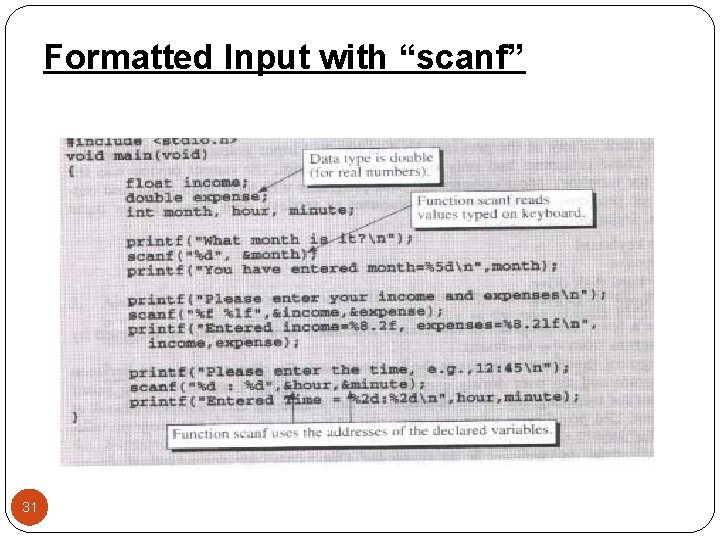
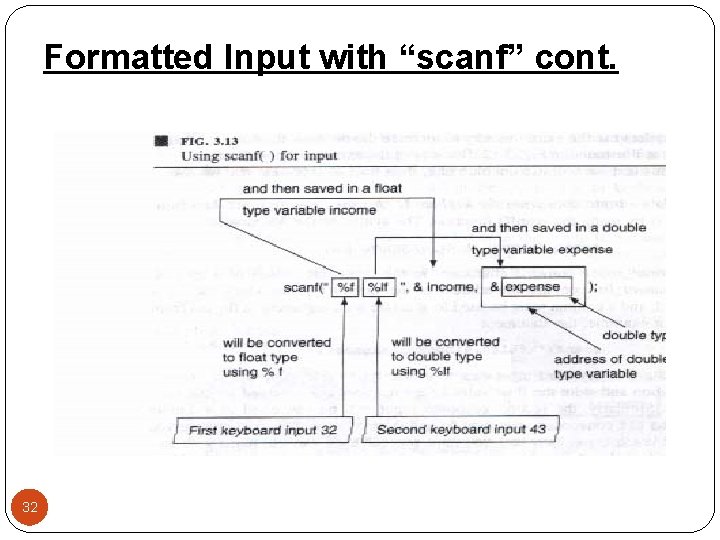
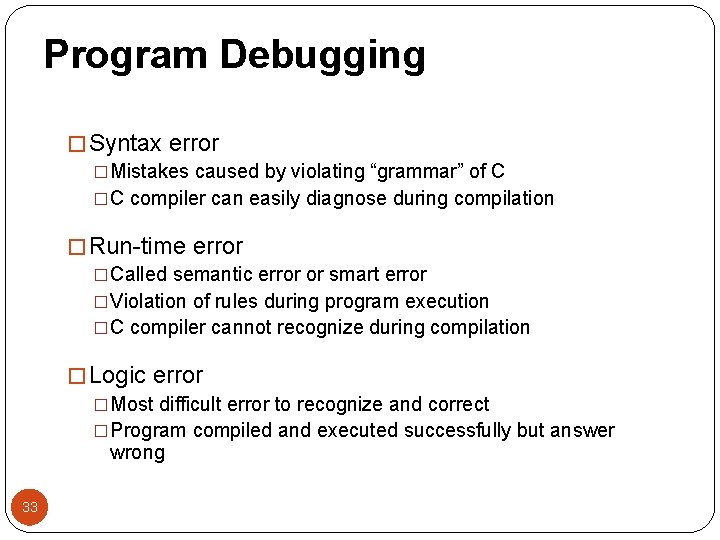
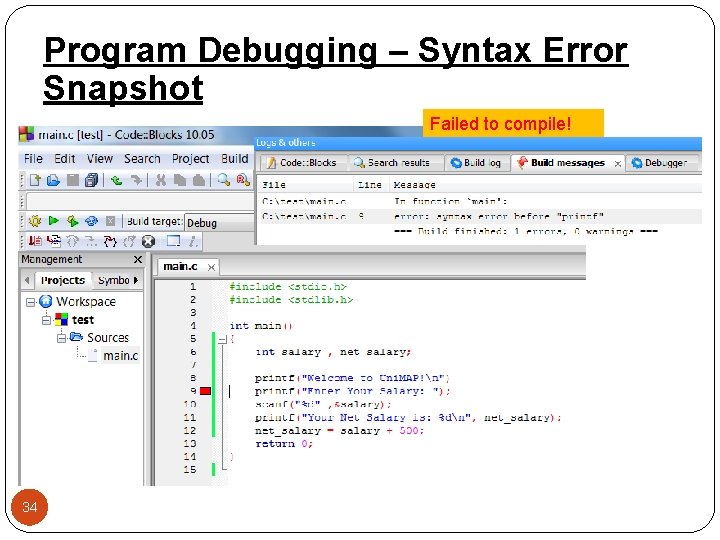
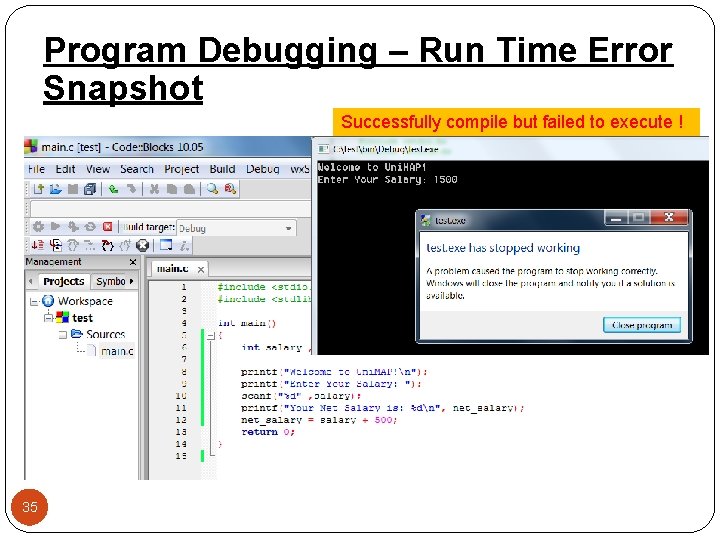
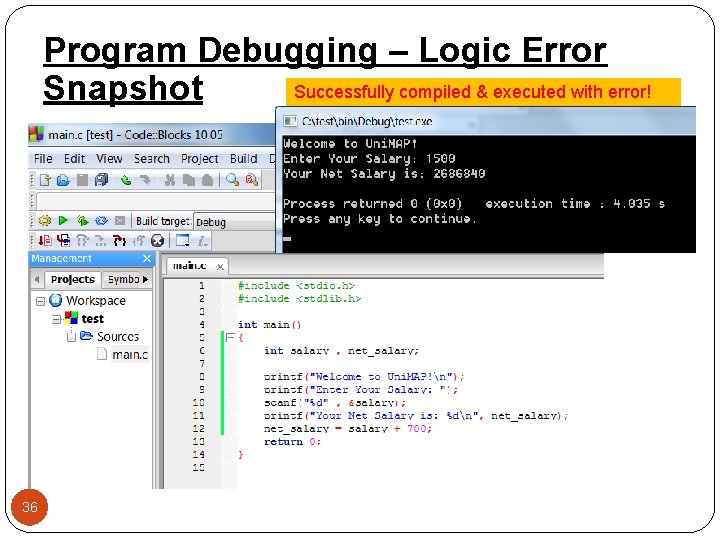
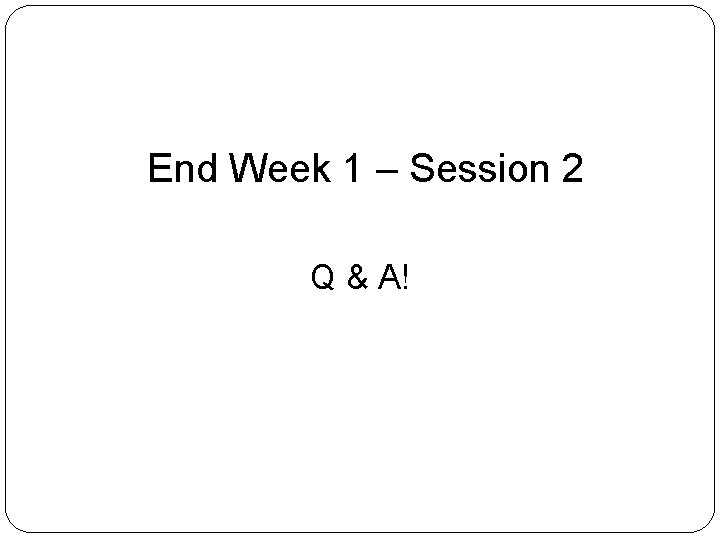
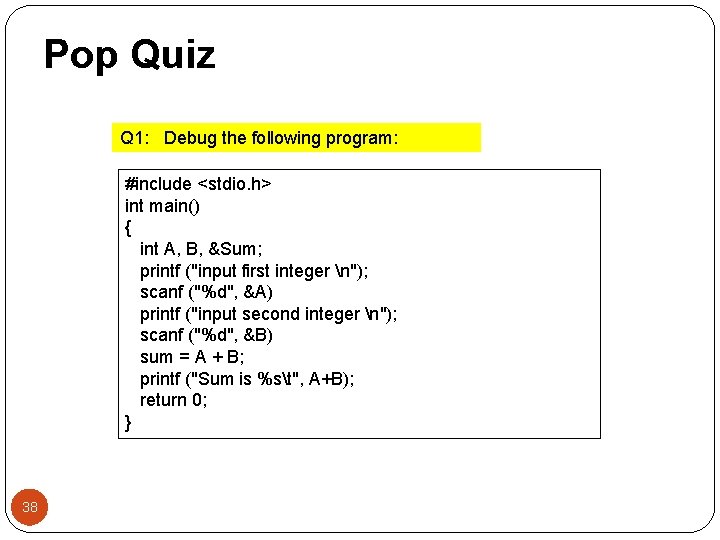
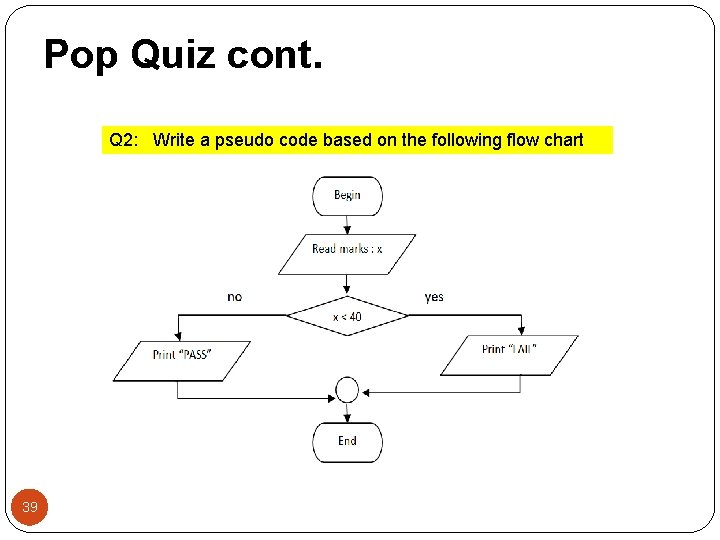
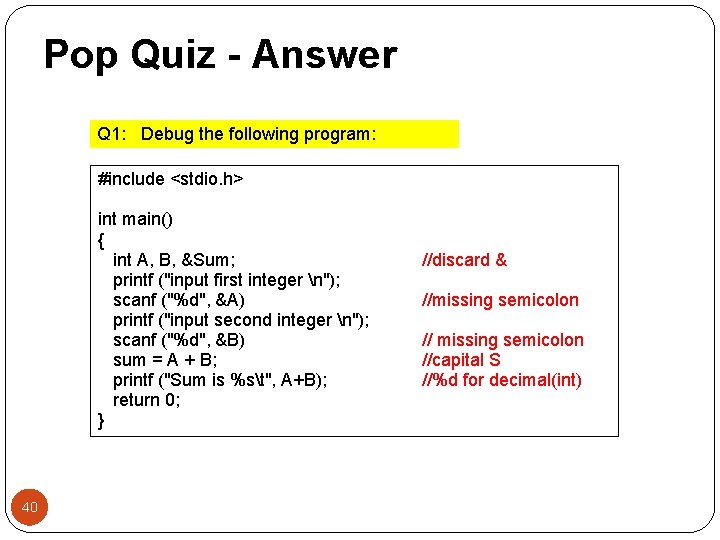
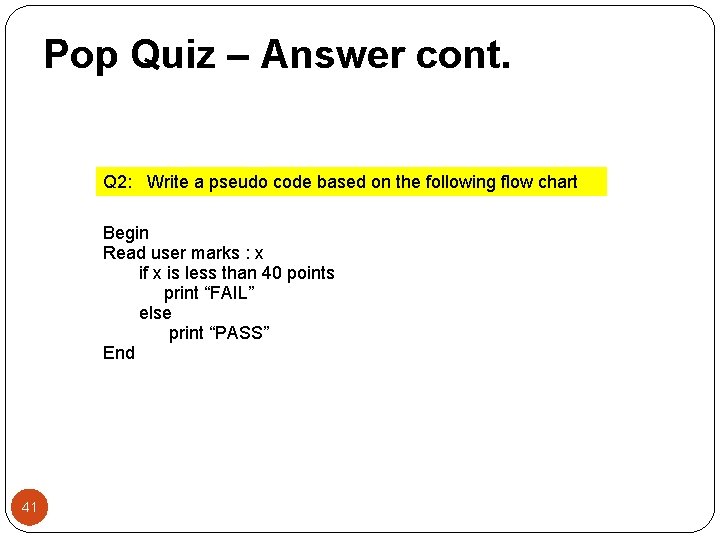
- Slides: 41
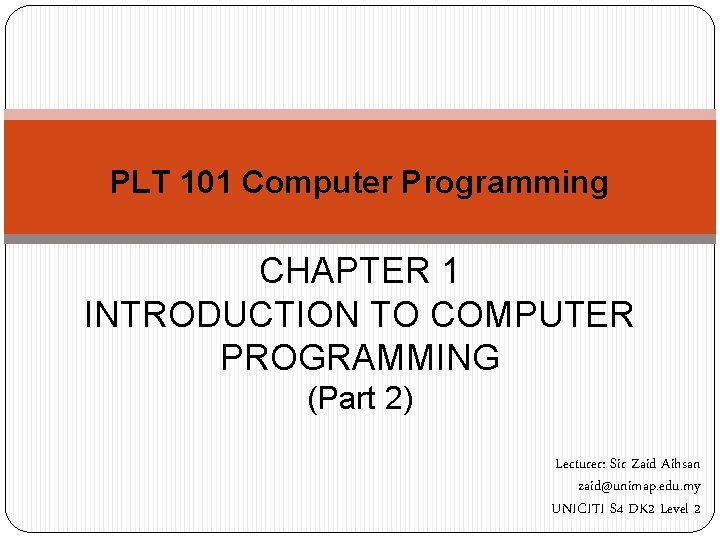
PLT 101 Computer Programming CHAPTER 1 INTRODUCTION TO COMPUTER PROGRAMMING (Part 2) Lecturer: Sir Zaid Aihsan zaid@unimap. edu. my UNICITI S 4 DK 2 Level 2
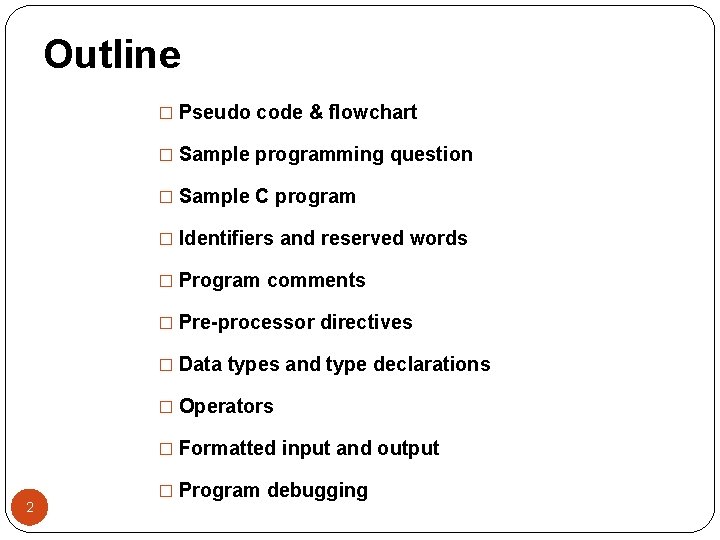
Outline � Pseudo code & flowchart � Sample programming question � Sample C program � Identifiers and reserved words � Program comments � Pre-processor directives � Data types and type declarations � Operators � Formatted input and output 2 � Program debugging
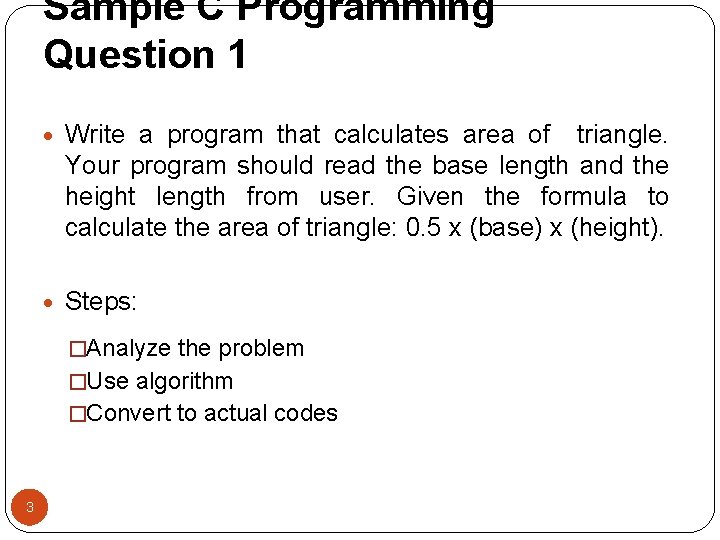
Sample C Programming Question 1 • Write a program that calculates area of triangle. Your program should read the base length and the height length from user. Given the formula to calculate the area of triangle: 0. 5 x (base) x (height). • Steps: �Analyze the problem �Use algorithm �Convert to actual codes 3
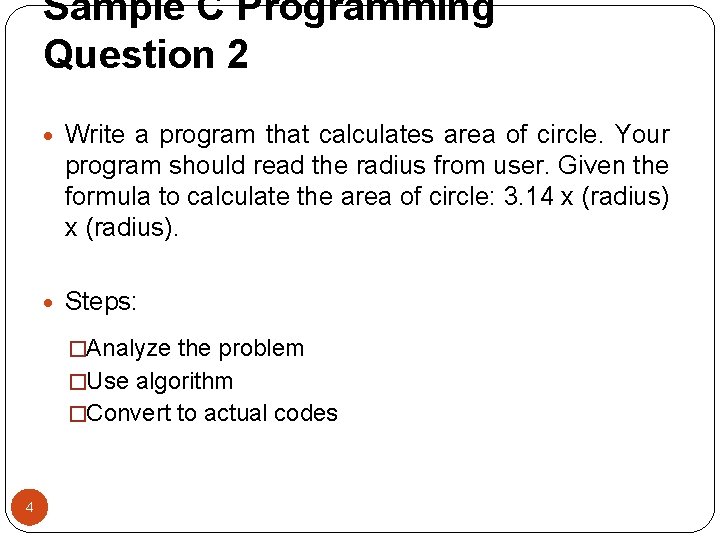
Sample C Programming Question 2 • Write a program that calculates area of circle. Your program should read the radius from user. Given the formula to calculate the area of circle: 3. 14 x (radius). • Steps: �Analyze the problem �Use algorithm �Convert to actual codes 4
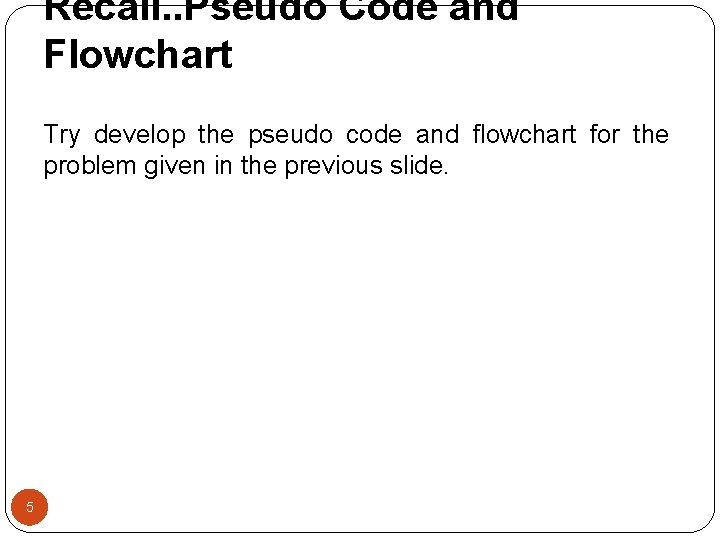
Recall. . Pseudo Code and Flowchart Try develop the pseudo code and flowchart for the problem given in the previous slide. 5
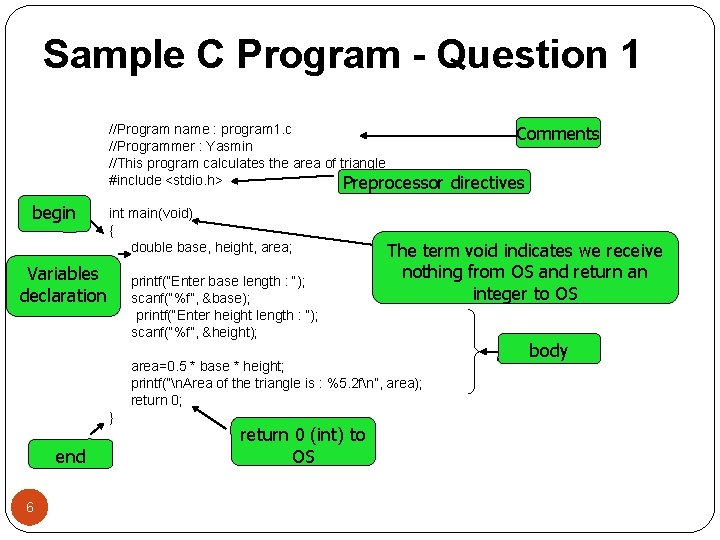
Sample C Program - Question 1 //Program name : program 1. c //Programmer : Yasmin //This program calculates the area of triangle #include <stdio. h> Preprocessor begin int main(void) { double base, height, area; Variables declaration printf(“Enter base length : “); scanf(“%f”, &base); printf(“Enter height length : “); scanf(“%f”, &height); end 6 return 0 (int) to OS directives The term void indicates we receive nothing from OS and return an integer to OS area=0. 5 * base * height; printf(“n. Area of the triangle is : %5. 2 fn”, area); return 0; } Comments body
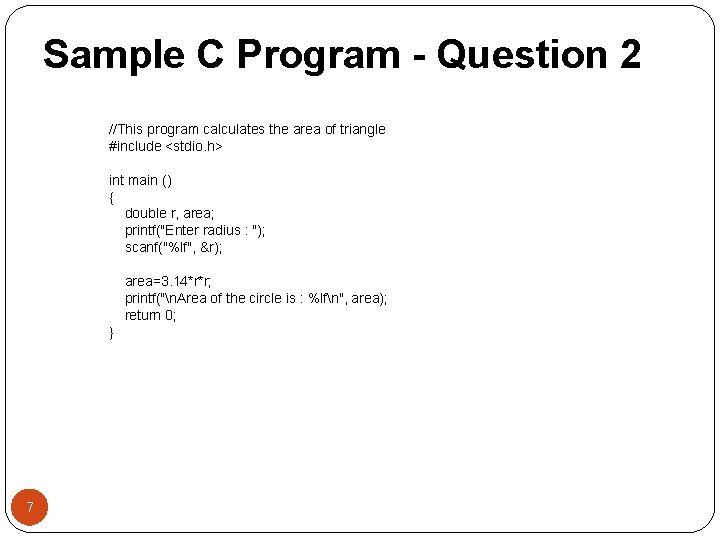
Sample C Program - Question 2 //This program calculates the area of triangle #include <stdio. h> int main () { double r, area; printf("Enter radius : "); scanf("%lf", &r); area=3. 14*r*r; printf("n. Area of the circle is : %lfn", area); return 0; } 7
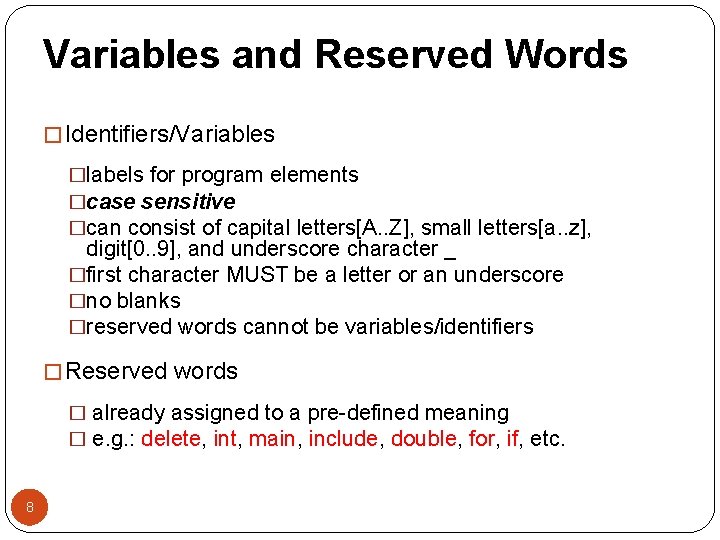
Variables and Reserved Words � Identifiers/Variables �labels for program elements �case sensitive �can consist of capital letters[A. . Z], small letters[a. . z], digit[0. . 9], and underscore character _ �first character MUST be a letter or an underscore �no blanks �reserved words cannot be variables/identifiers � Reserved words � already assigned to a pre-defined meaning � e. g. : delete, int, main, include, double, for, if, etc. 8
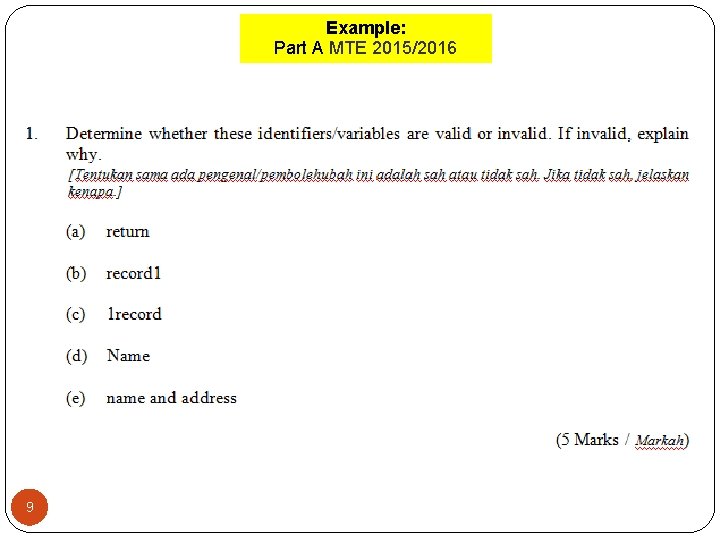
Example: Part A MTE 2015/2016 9
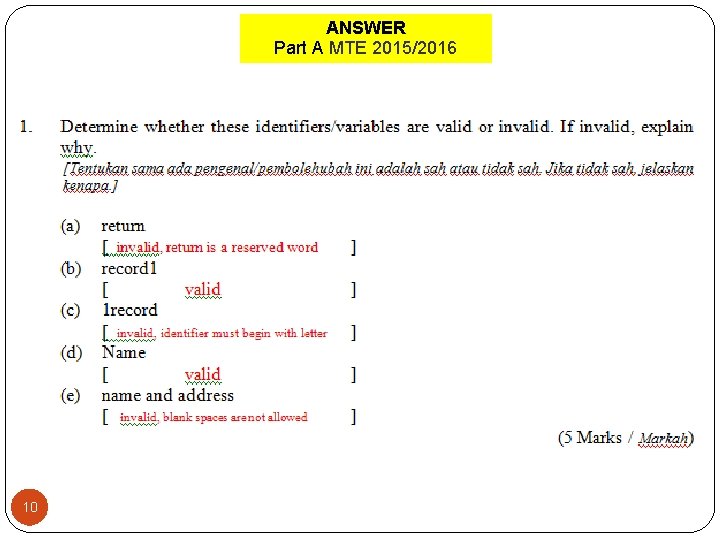
ANSWER Part A MTE 2015/2016 10
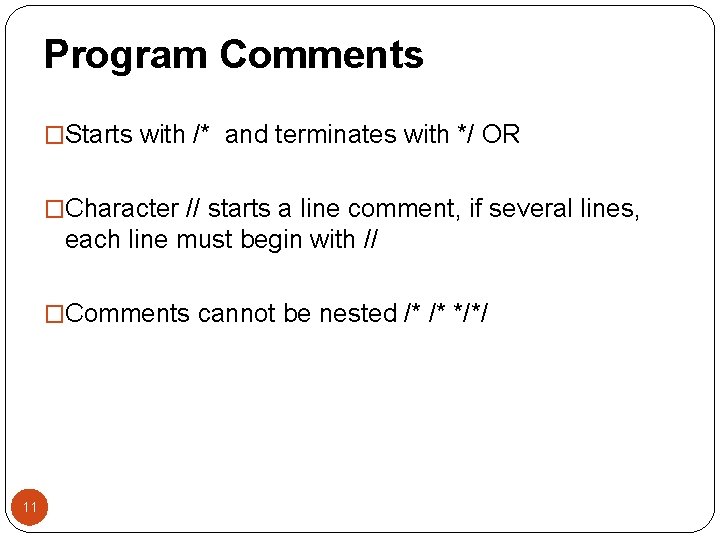
Program Comments �Starts with /* and terminates with */ OR �Character // starts a line comment, if several lines, each line must begin with // �Comments cannot be nested /* /* */*/ 11
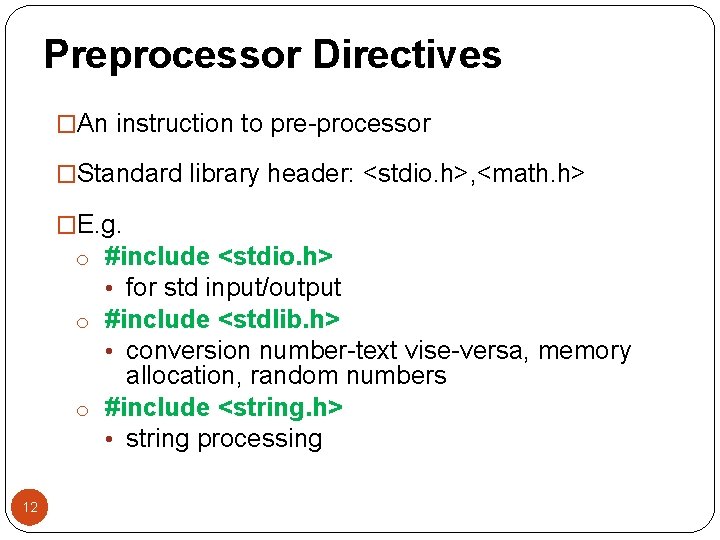
Preprocessor Directives �An instruction to pre-processor �Standard library header: <stdio. h>, <math. h> �E. g. o #include <stdio. h> • for std input/output o #include <stdlib. h> • conversion number-text vise-versa, memory allocation, random numbers o #include <string. h> • string processing 12
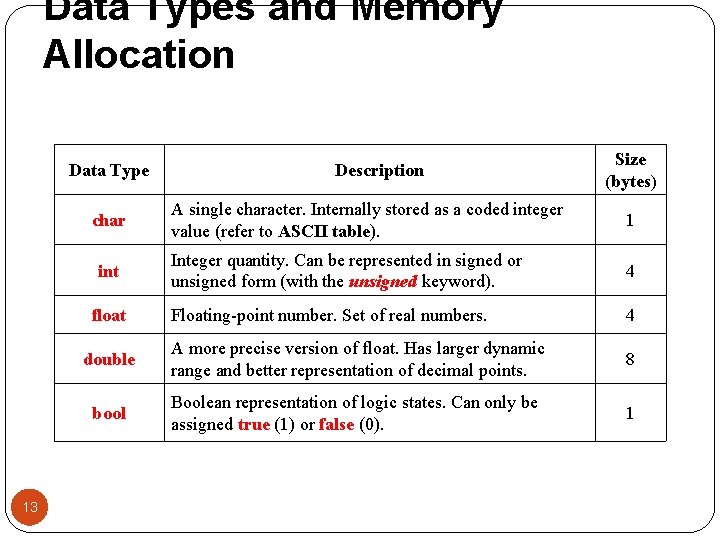
Data Types and Memory Allocation Data Type Size (bytes) A single character. Internally stored as a coded integer value (refer to ASCII table). 1 Integer quantity. Can be represented in signed or unsigned form (with the unsigned keyword). 4 Floating-point number. Set of real numbers. 4 double A more precise version of float. Has larger dynamic range and better representation of decimal points. 8 bool Boolean representation of logic states. Can only be assigned true (1) or false (0). 1 char int float 13 Description
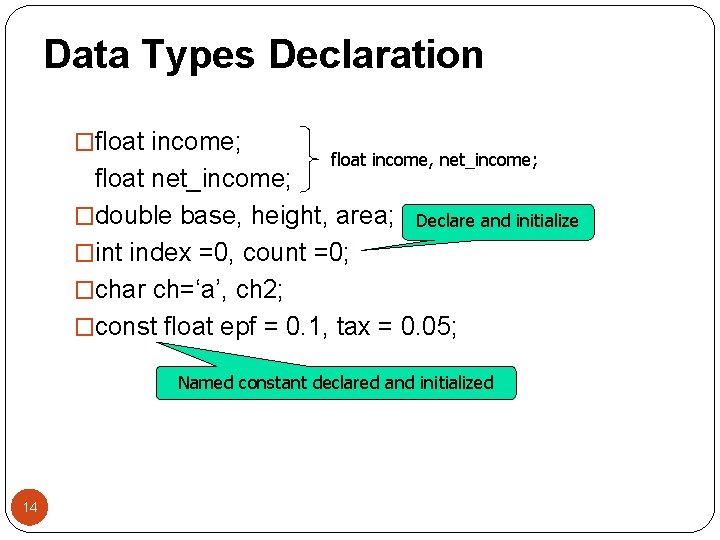
Data Types Declaration �float income; float income, net_income; float net_income; �double base, height, area; Declare and initialize �int index =0, count =0; �char ch=‘a’, ch 2; �const float epf = 0. 1, tax = 0. 05; Named constant declared and initialized 14
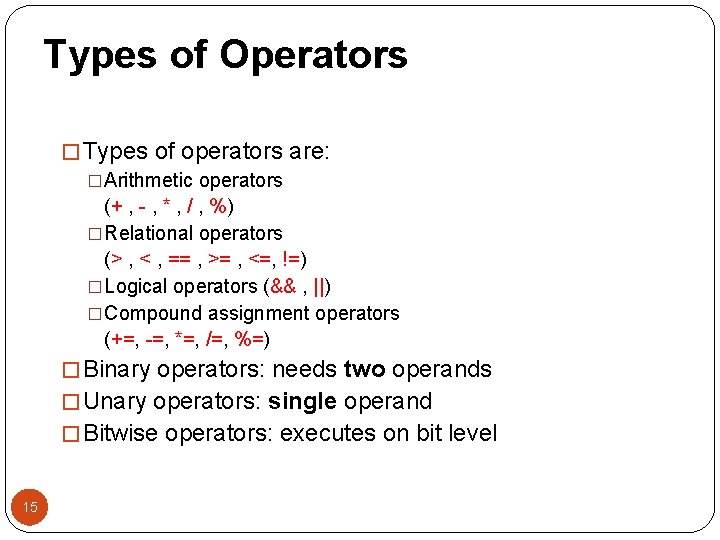
Types of Operators � Types of operators are: �Arithmetic operators (+ , - , * , / , %) �Relational operators (> , < , == , >= , <=, !=) �Logical operators (&& , ||) �Compound assignment operators (+=, -=, *=, /=, %=) � Binary operators: needs two operands � Unary operators: single operand � Bitwise operators: executes on bit level 15
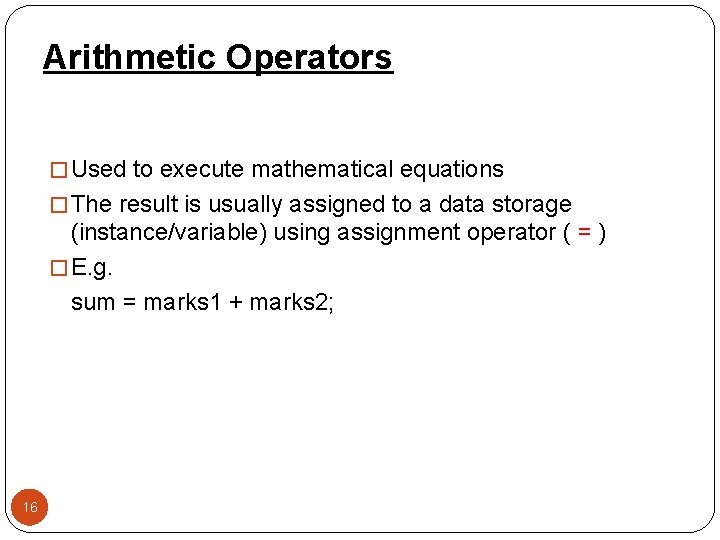
Arithmetic Operators � Used to execute mathematical equations � The result is usually assigned to a data storage (instance/variable) using assignment operator ( = ) � E. g. sum = marks 1 + marks 2; 16
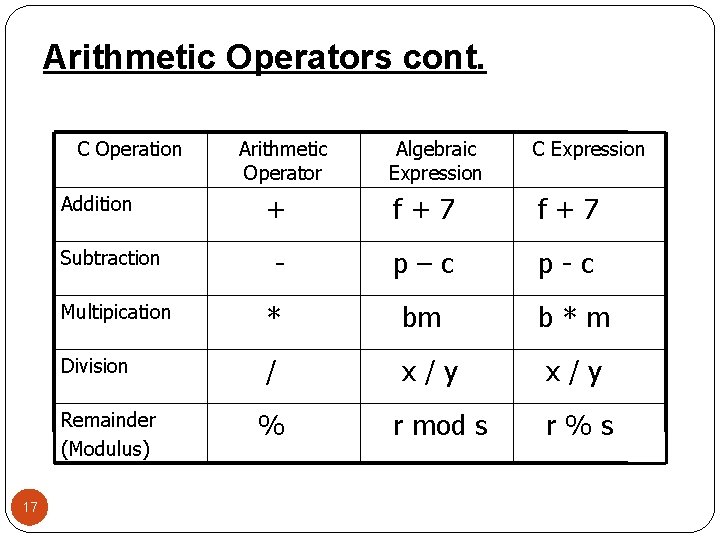
Arithmetic Operators cont. C Operation Algebraic Expression C Expression + f+7 - p–c p-c Multipication * bm b*m Division / x/y Addition Subtraction Remainder (Modulus) 17 Arithmetic Operator % r mod s x/y r%s
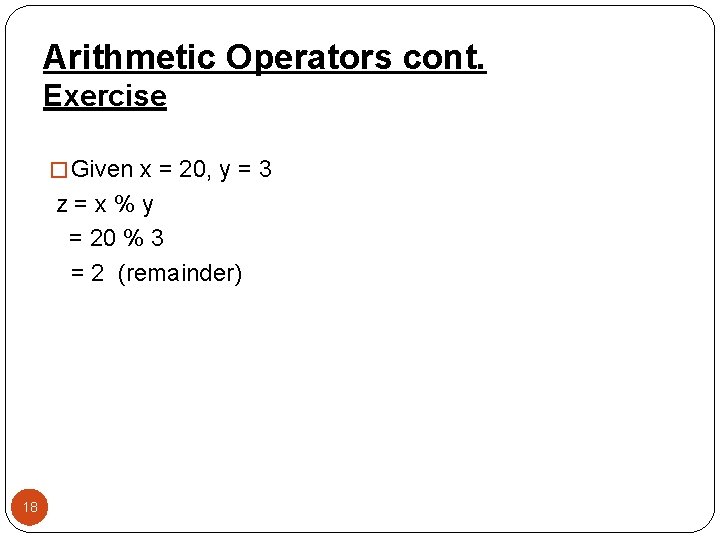
Arithmetic Operators cont. Exercise � Given x = 20, y = 3 z=x%y = 20 % 3 = 2 (remainder) 18
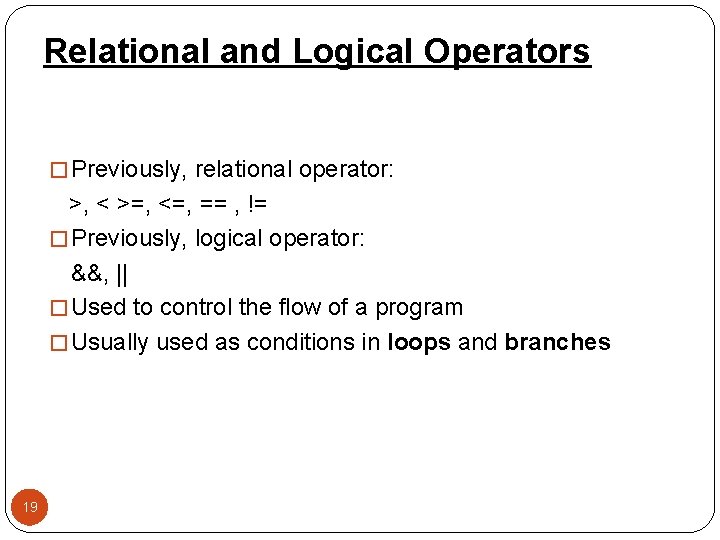
Relational and Logical Operators � Previously, relational operator: >, < >=, <=, == , != � Previously, logical operator: &&, || � Used to control the flow of a program � Usually used as conditions in loops and branches 19
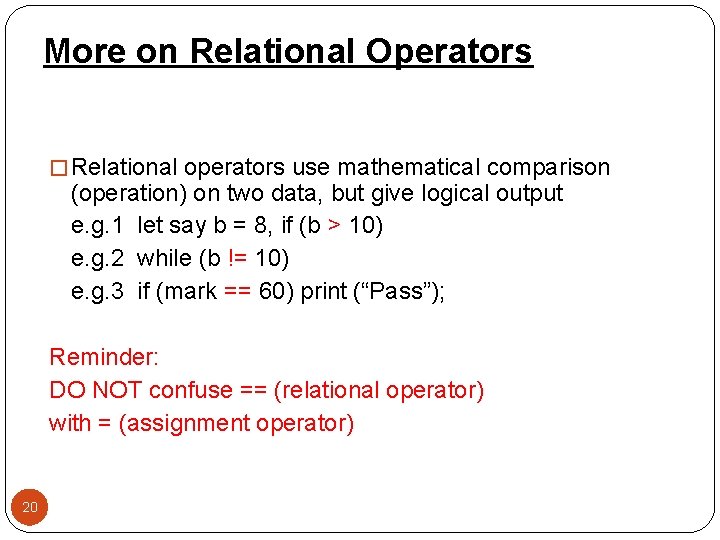
More on Relational Operators � Relational operators use mathematical comparison (operation) on two data, but give logical output e. g. 1 let say b = 8, if (b > 10) e. g. 2 while (b != 10) e. g. 3 if (mark == 60) print (“Pass”); Reminder: DO NOT confuse == (relational operator) with = (assignment operator) 20
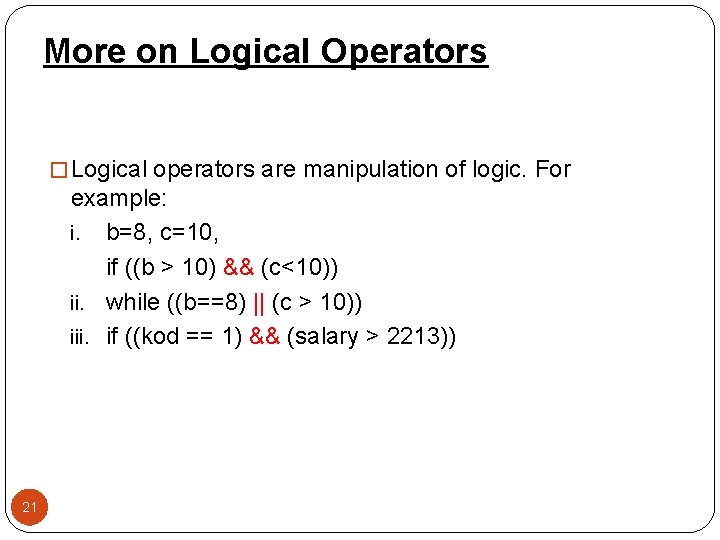
More on Logical Operators � Logical operators are manipulation of logic. For example: i. b=8, c=10, if ((b > 10) && (c<10)) ii. while ((b==8) || (c > 10)) iii. if ((kod == 1) && (salary > 2213)) 21
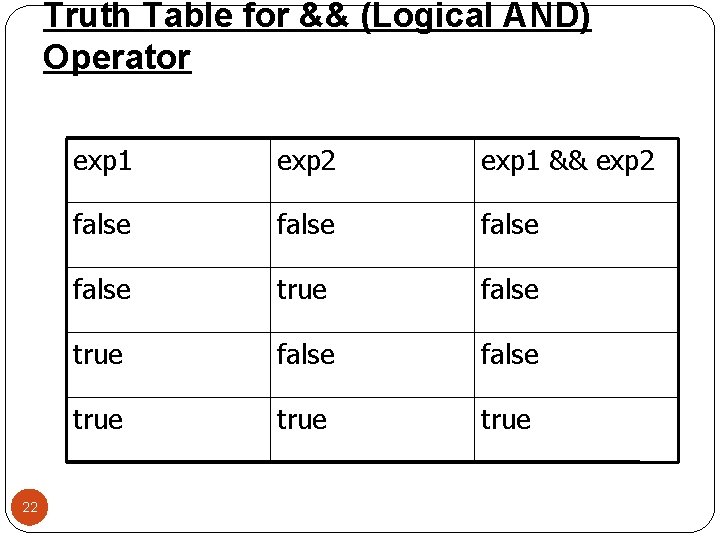
Truth Table for && (Logical AND) Operator 22 exp 1 exp 2 exp 1 && exp 2 false false true true
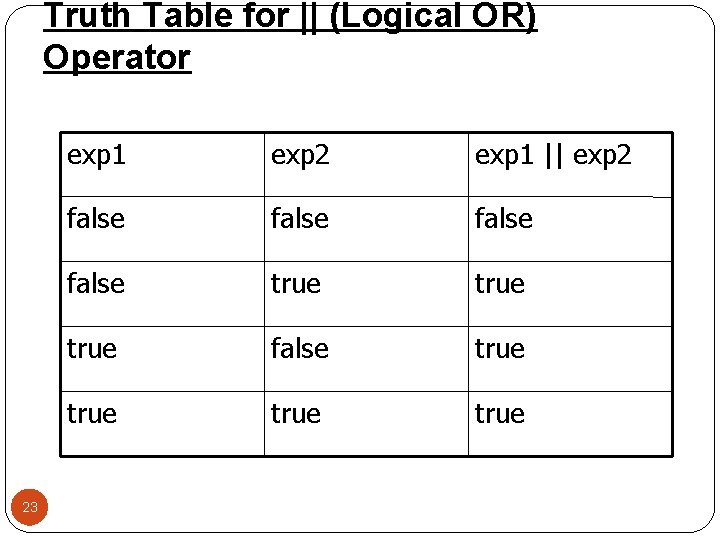
Truth Table for || (Logical OR) Operator 23 exp 1 exp 2 exp 1 || exp 2 false true false true
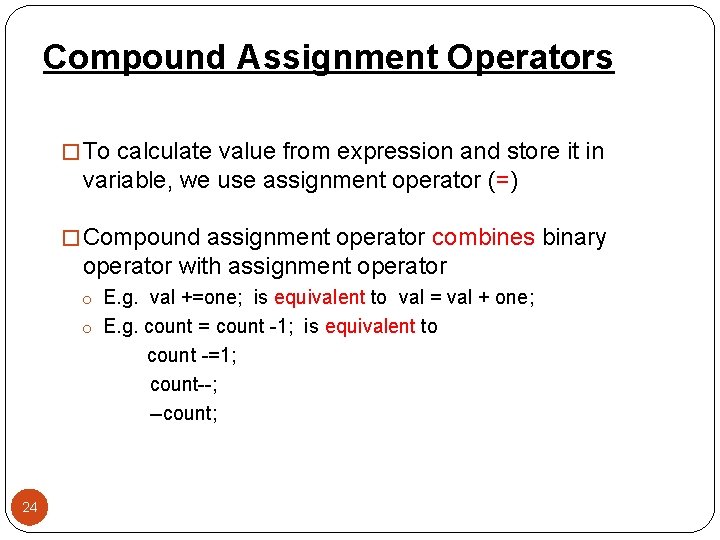
Compound Assignment Operators � To calculate value from expression and store it in variable, we use assignment operator (=) � Compound assignment operator combines binary operator with assignment operator o E. g. val +=one; is equivalent to val = val + one; o E. g. count = count -1; is equivalent to count -=1; count--; --count; 24
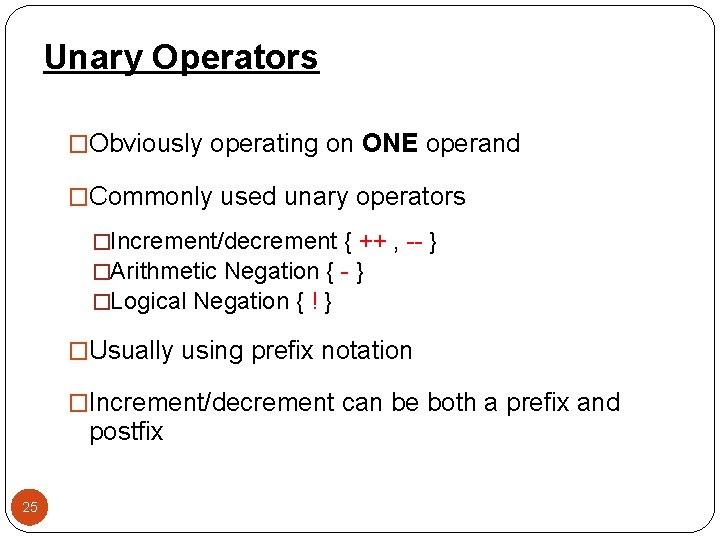
Unary Operators �Obviously operating on ONE operand �Commonly used unary operators �Increment/decrement { ++ , -- } �Arithmetic Negation { - } �Logical Negation { ! } �Usually using prefix notation �Increment/decrement can be both a prefix and postfix 25
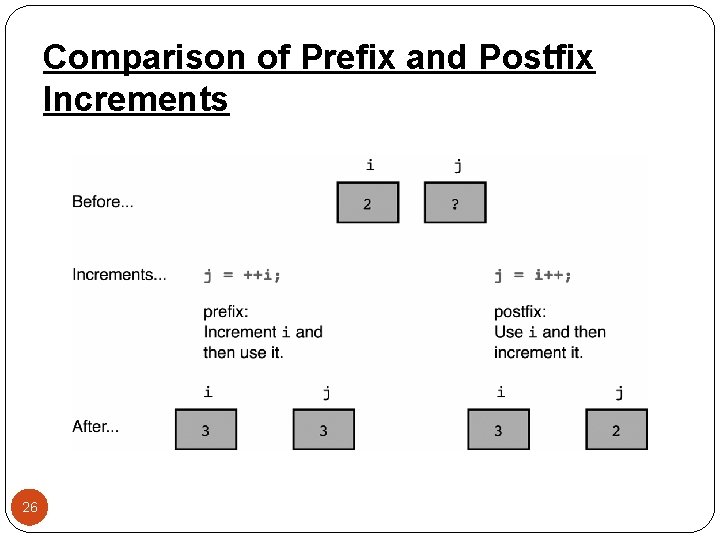
Comparison of Prefix and Postfix Increments 26
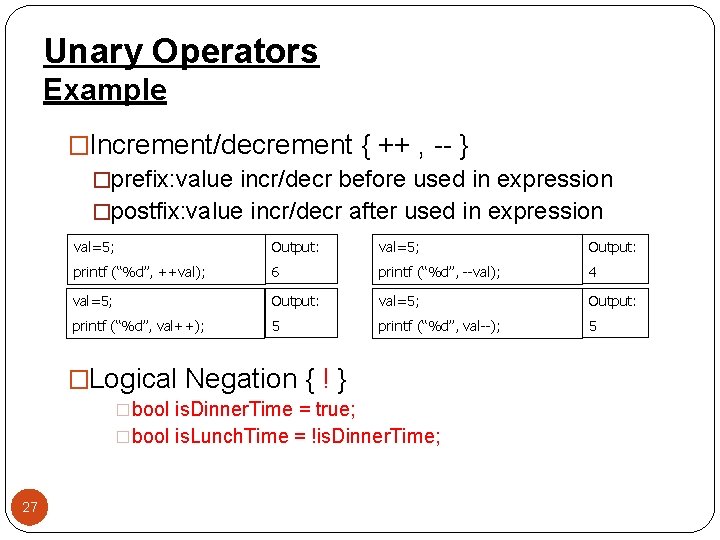
Unary Operators Example �Increment/decrement { ++ , -- } �prefix: value incr/decr before used in expression �postfix: value incr/decr after used in expression val=5; Output: printf (“%d”, ++val); 6 printf (“%d”, --val); 4 val=5; Output: printf (“%d”, val++); 5 printf (“%d”, val--); 5 �Logical Negation { ! } �bool is. Dinner. Time = true; �bool is. Lunch. Time = !is. Dinner. Time; 27
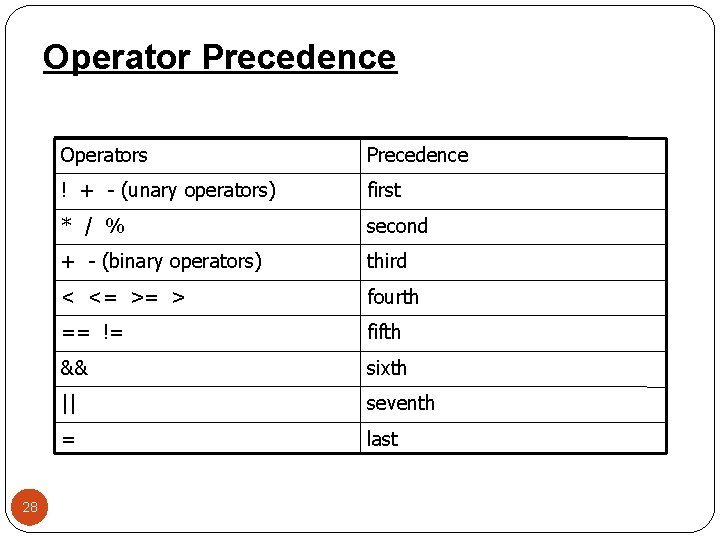
Operator Precedence 28 Operators Precedence ! + - (unary operators) first * / % second + - (binary operators) third < <= >= > fourth == != fifth && sixth || seventh = last
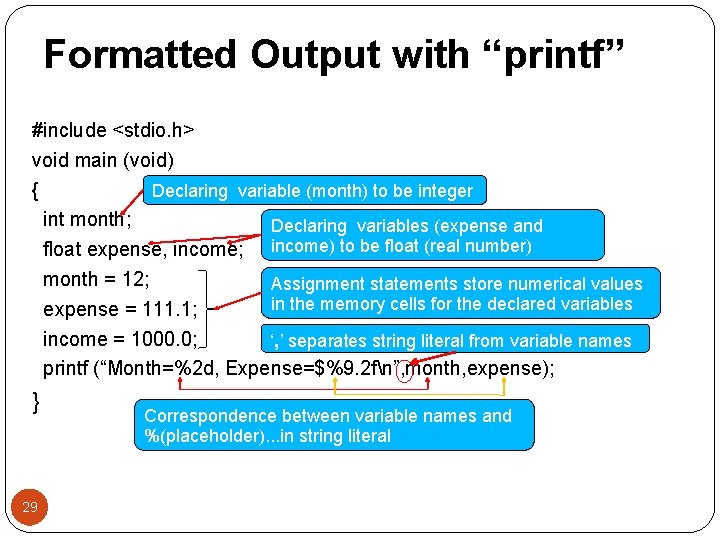
Formatted Output with “printf” #include <stdio. h> void main (void) { Declaring variable (month) to be integer int month; Declaring variables (expense and float expense, income; income) to be float (real number) month = 12; Assignment statements store numerical values in the memory cells for the declared variables expense = 111. 1; income = 1000. 0; ‘, ’ separates string literal from variable names printf (“Month=%2 d, Expense=$%9. 2 fn”, month, expense); } 29 Correspondence between variable names and %(placeholder). . . in string literal
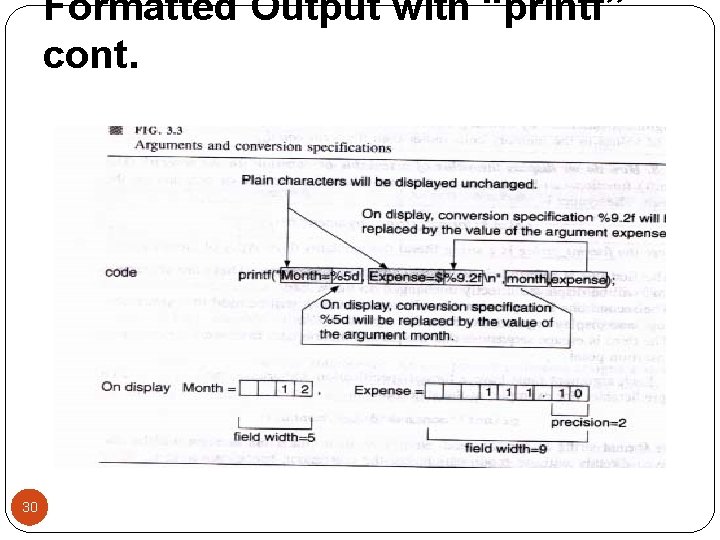
Formatted Output with “printf” cont. 30
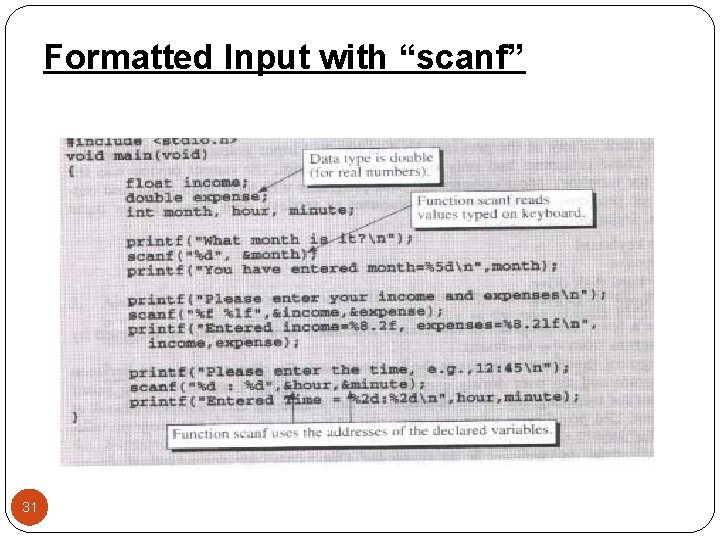
Formatted Input with “scanf” 31
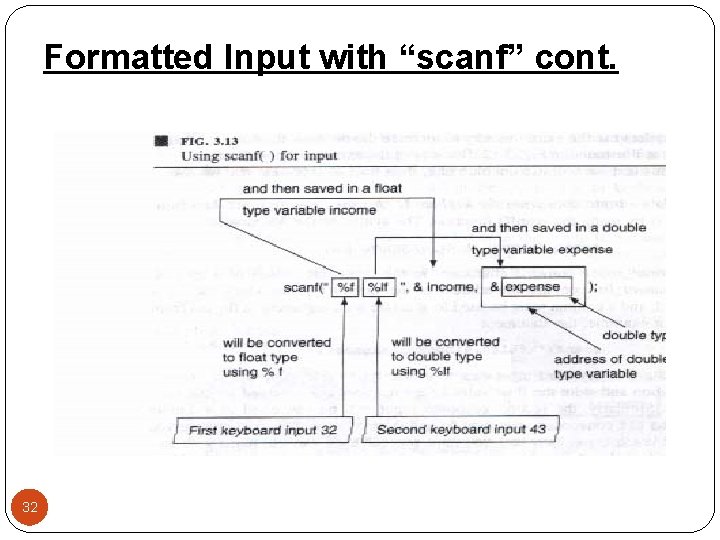
Formatted Input with “scanf” cont. 32
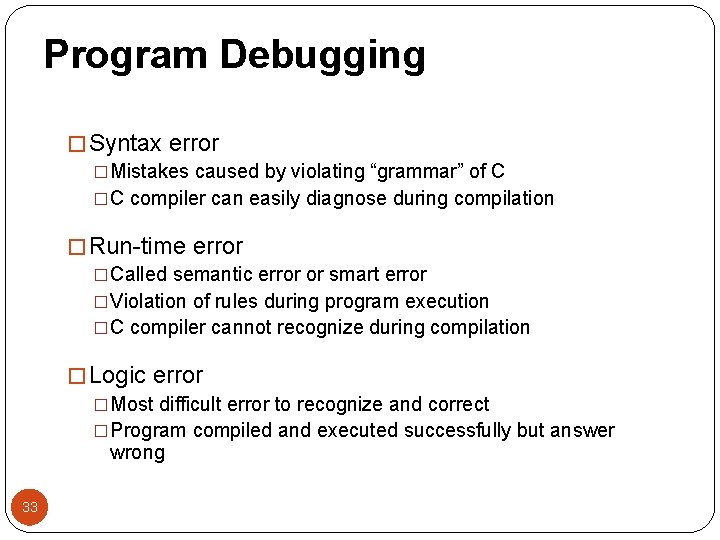
Program Debugging � Syntax error �Mistakes caused by violating “grammar” of C �C compiler can easily diagnose during compilation � Run-time error �Called semantic error or smart error �Violation of rules during program execution �C compiler cannot recognize during compilation � Logic error �Most difficult error to recognize and correct �Program compiled and executed successfully but answer wrong 33
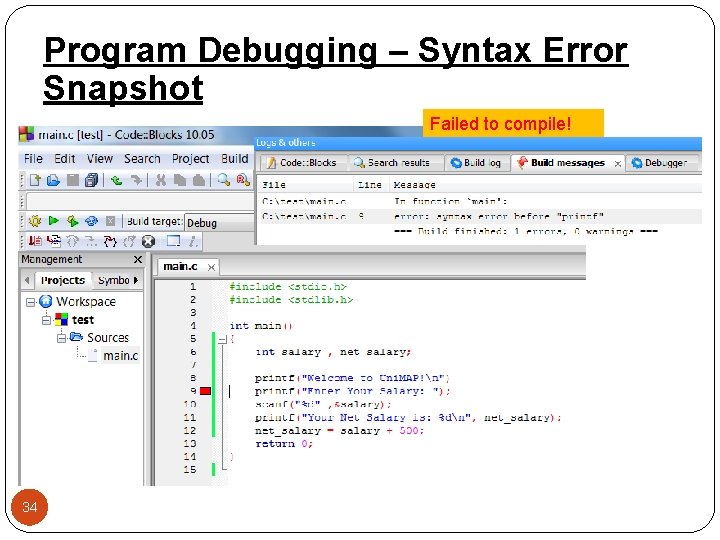
Program Debugging – Syntax Error Snapshot Failed to compile! 34
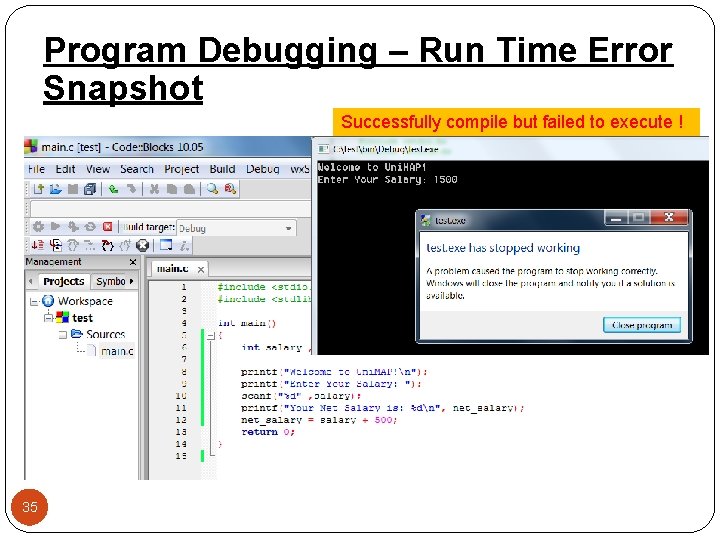
Program Debugging – Run Time Error Snapshot Successfully compile but failed to execute ! 35
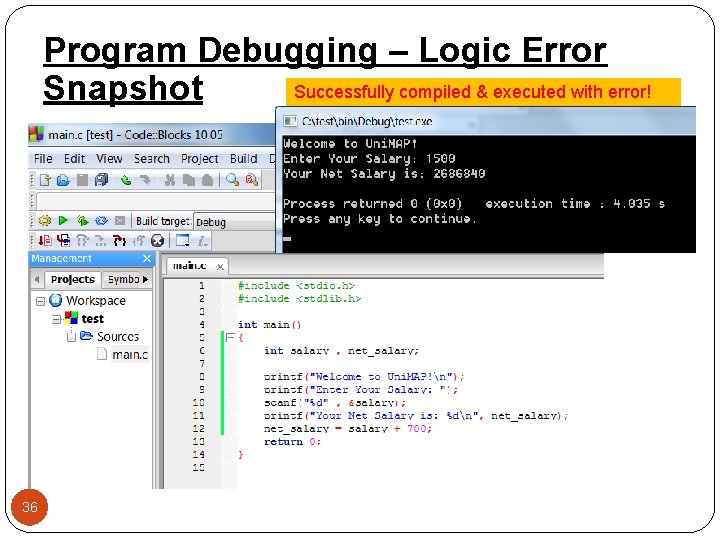
Program Debugging – Logic Error Successfully compiled & executed with error! Snapshot PLT 101 2013/2014 36
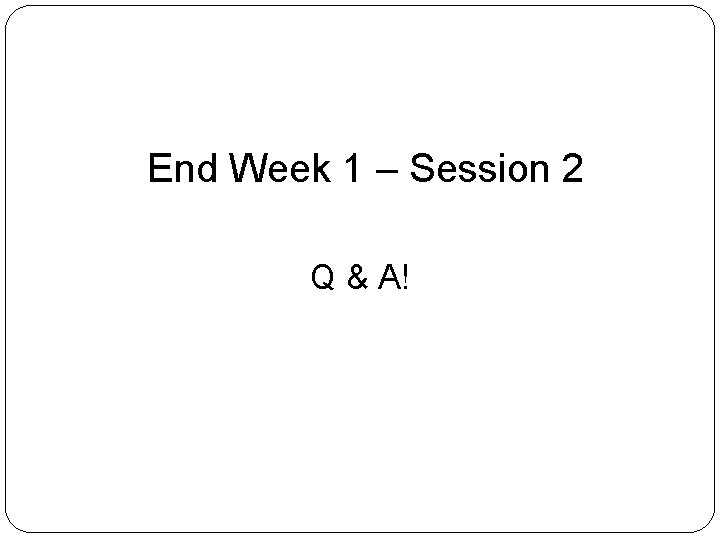
End Week 1 – Session 2 Q & A! 3 7
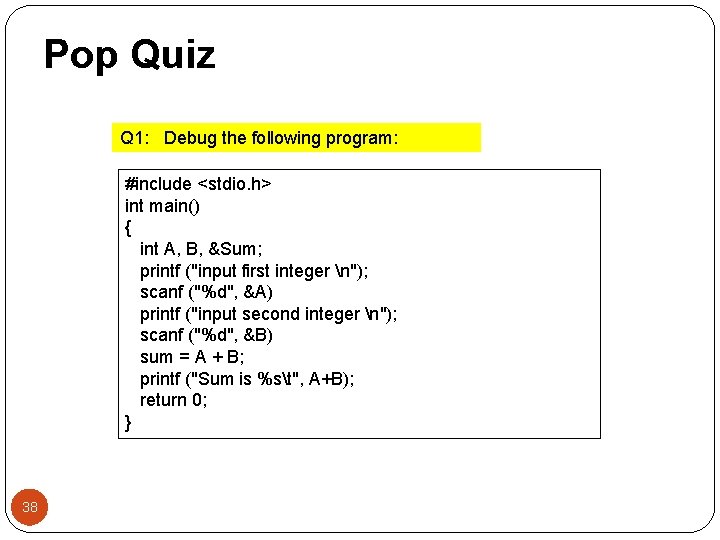
Pop Quiz Q 1: Debug the following program: #include <stdio. h> int main() { int A, B, ∑ printf ("input first integer n"); scanf ("%d", &A) printf ("input second integer n"); scanf ("%d", &B) sum = A + B; printf ("Sum is %st", A+B); return 0; } 38
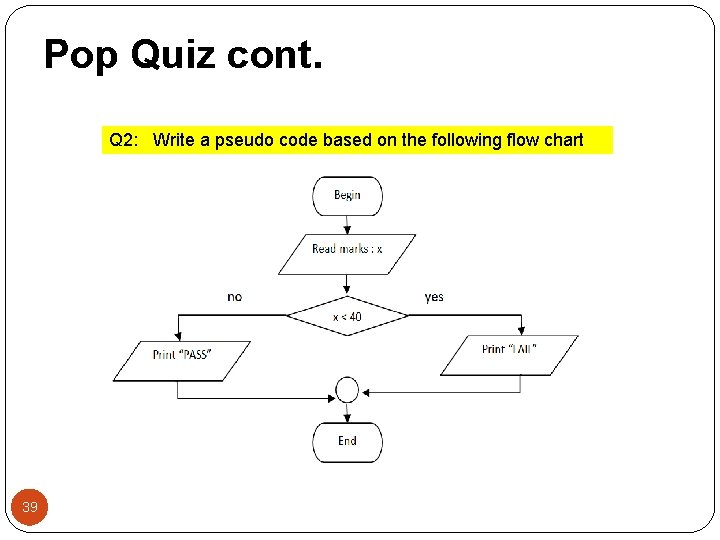
Pop Quiz cont. Q 2: Write a pseudo code based on the following flow chart 39
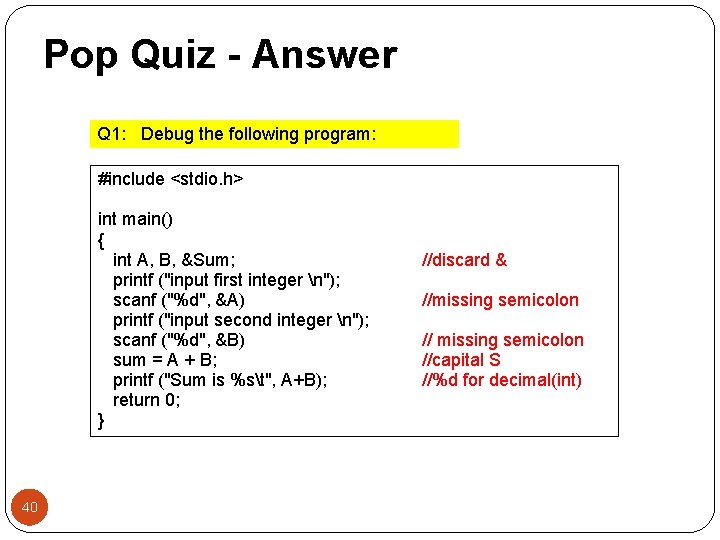
Pop Quiz - Answer Q 1: Debug the following program: #include <stdio. h> int main() { int A, B, ∑ printf ("input first integer n"); scanf ("%d", &A) printf ("input second integer n"); scanf ("%d", &B) sum = A + B; printf ("Sum is %st", A+B); return 0; } 40 //discard & //missing semicolon //capital S //%d for decimal(int)
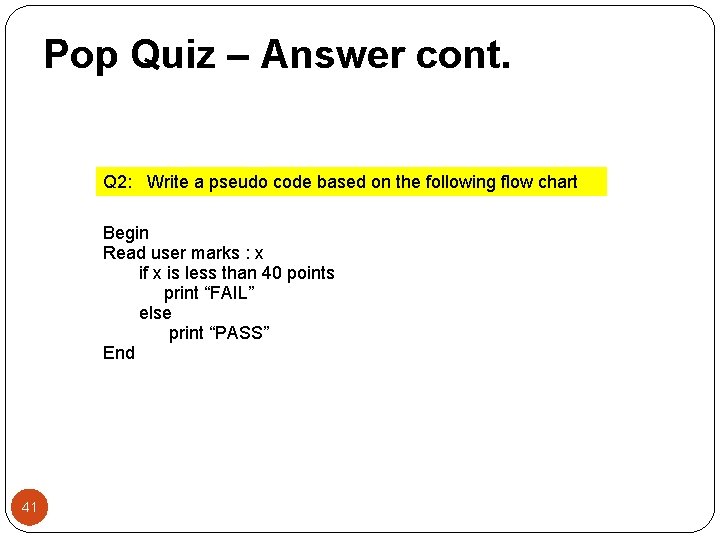
Pop Quiz – Answer cont. Q 2: Write a pseudo code based on the following flow chart Begin Read user marks : x if x is less than 40 points print “FAIL” else print “PASS” End 41