PIC 18 Timer Programming Explain the assembly language
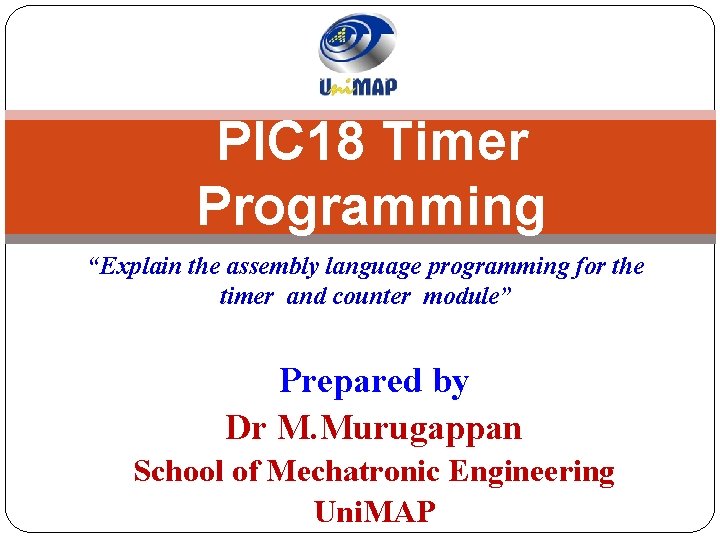
PIC 18 Timer Programming “Explain the assembly language programming for the timer and counter module” Prepared by Dr M. Murugappan School of Mechatronic Engineering Uni. MAP
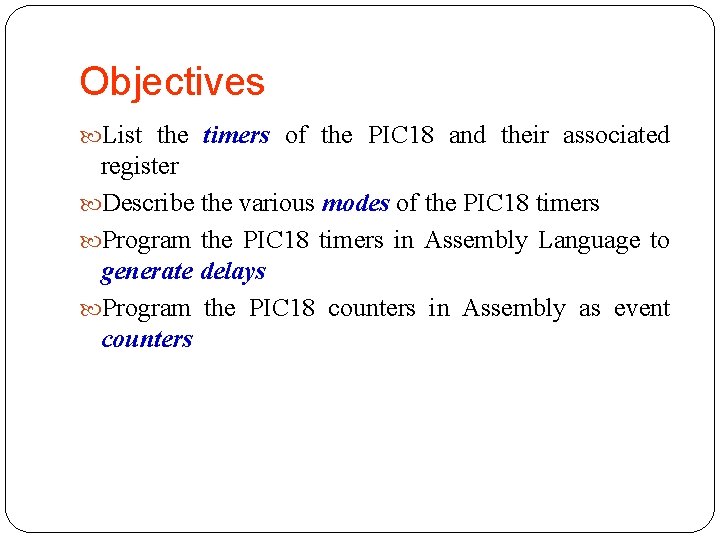
Objectives List the timers of the PIC 18 and their associated register Describe the various modes of the PIC 18 timers Program the PIC 18 timers in Assembly Language to generate delays Program the PIC 18 counters in Assembly as event counters
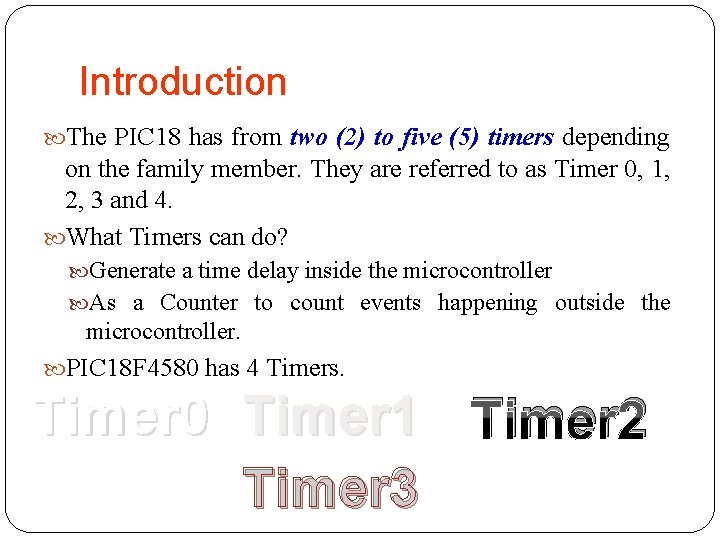
Introduction The PIC 18 has from two (2) to five (5) timers depending on the family member. They are referred to as Timer 0, 1, 2, 3 and 4. What Timers can do? Generate a time delay inside the microcontroller As a Counter to count events happening outside the microcontroller. PIC 18 F 4580 has 4 Timers. Timer 0 Timer 1 Timer 2 Timer 3
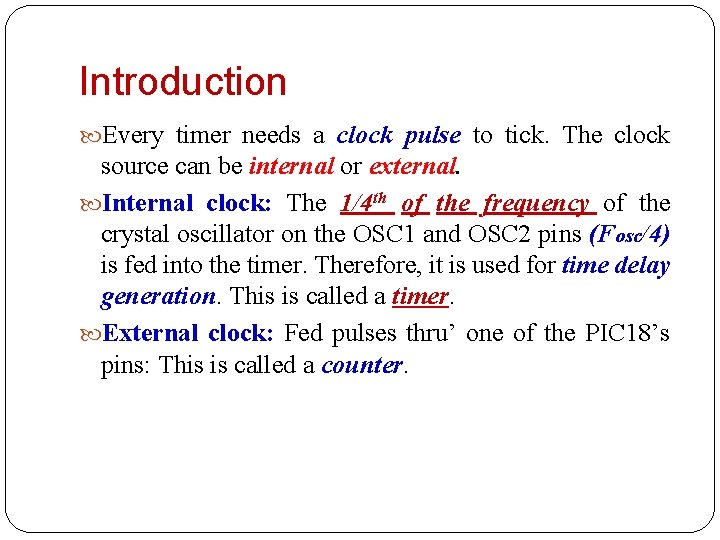
Introduction Every timer needs a clock pulse to tick. The clock source can be internal or external. Internal clock: The 1/4 th of the frequency of the crystal oscillator on the OSC 1 and OSC 2 pins (Fosc/4) is fed into the timer. Therefore, it is used for time delay generation. This is called a timer. External clock: Fed pulses thru’ one of the PIC 18’s pins: This is called a counter.
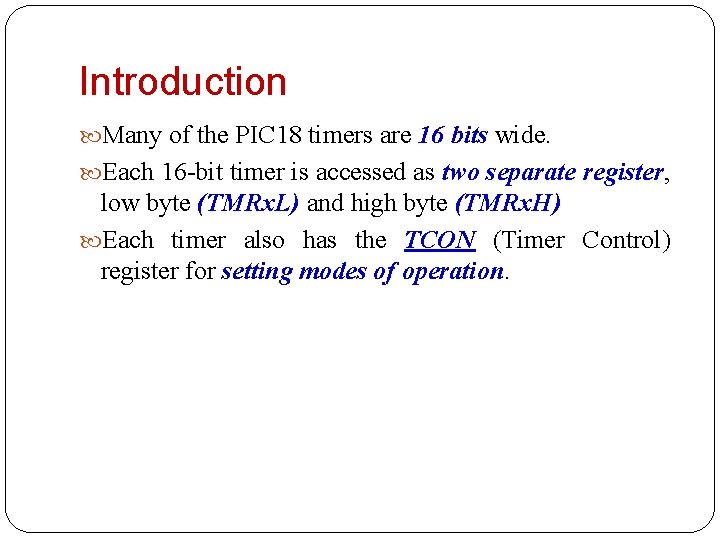
Introduction Many of the PIC 18 timers are 16 bits wide. Each 16 -bit timer is accessed as two separate register, low byte (TMRx. L) and high byte (TMRx. H) Each timer also has the TCON (Timer Control) register for setting modes of operation.
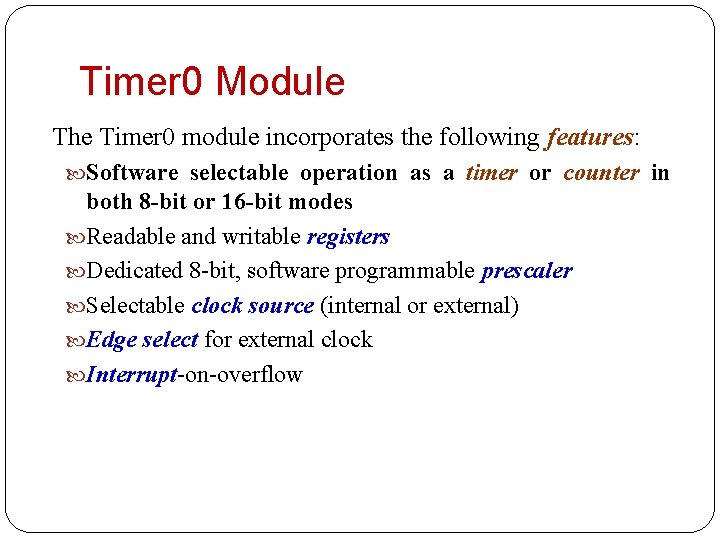
Timer 0 Module The Timer 0 module incorporates the following features: Software selectable operation as a timer or counter in both 8 -bit or 16 -bit modes Readable and writable registers Dedicated 8 -bit, software programmable prescaler Selectable clock source (internal or external) Edge select for external clock Interrupt-on-overflow
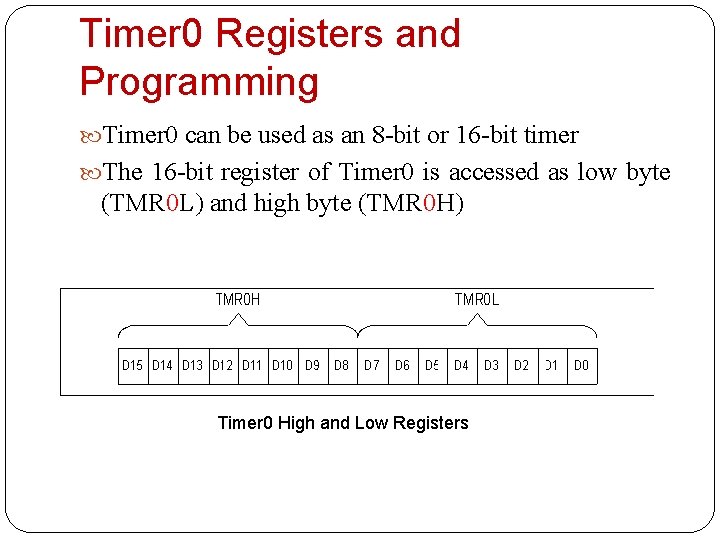
Timer 0 Registers and Programming Timer 0 can be used as an 8 -bit or 16 -bit timer The 16 -bit register of Timer 0 is accessed as low byte (TMR 0 L) and high byte (TMR 0 H) Timer 0 High and Low Registers
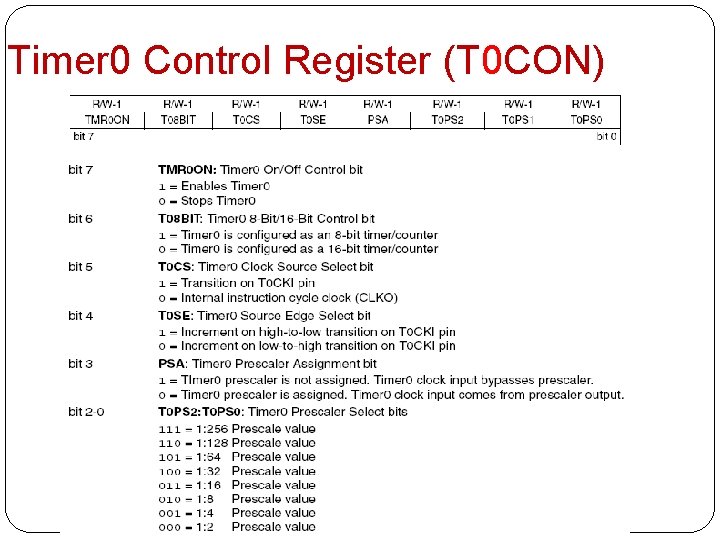
Timer 0 Control Register (T 0 CON)
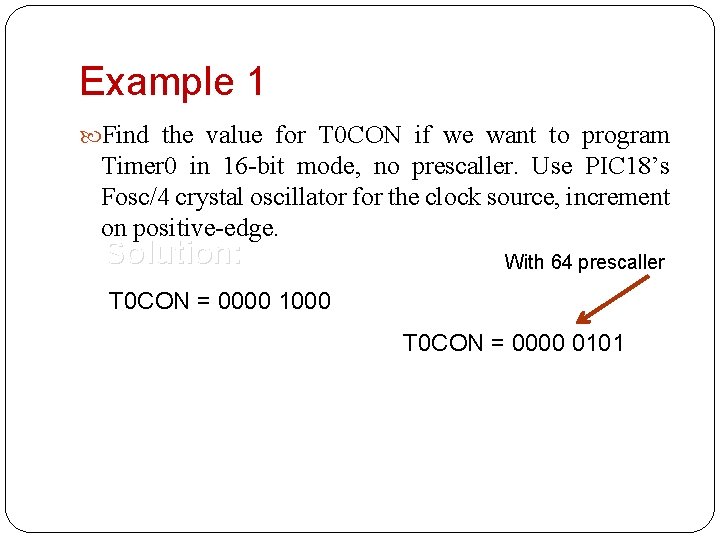
Example 1 Find the value for T 0 CON if we want to program Timer 0 in 16 -bit mode, no prescaller. Use PIC 18’s Fosc/4 crystal oscillator for the clock source, increment on positive-edge. Solution: With 64 prescaller T 0 CON = 0000 1000 T 0 CON = 0000 0101
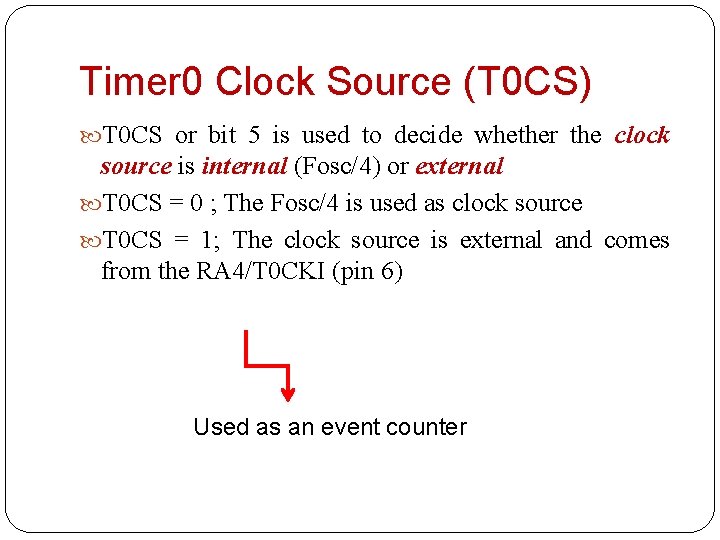
Timer 0 Clock Source (T 0 CS) T 0 CS or bit 5 is used to decide whether the clock source is internal (Fosc/4) or external T 0 CS = 0 ; The Fosc/4 is used as clock source T 0 CS = 1; The clock source is external and comes from the RA 4/T 0 CKI (pin 6) Used as an event counter
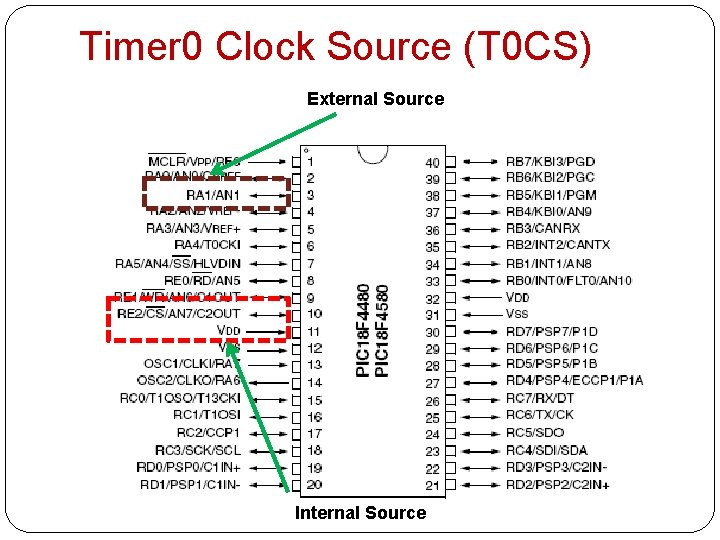
Timer 0 Clock Source (T 0 CS) External Source Internal Source
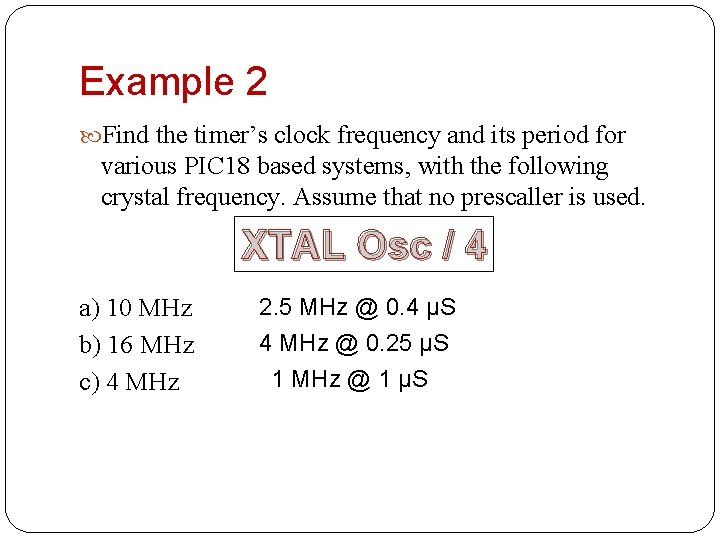
Example 2 Find the timer’s clock frequency and its period for various PIC 18 based systems, with the following crystal frequency. Assume that no prescaller is used. XTAL Osc / 4 a) 10 MHz b) 16 MHz c) 4 MHz 2. 5 MHz @ 0. 4 µS 4 MHz @ 0. 25 µS 1 MHz @ 1 µS
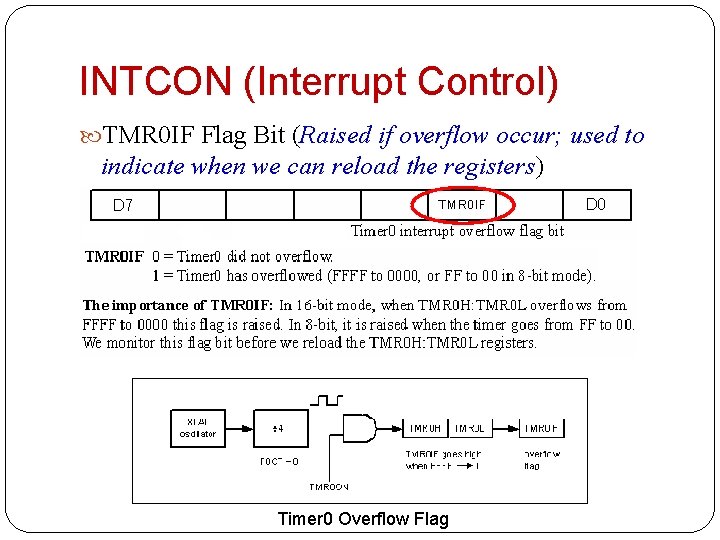
INTCON (Interrupt Control) TMR 0 IF Flag Bit (Raised if overflow occur; used to indicate when we can reload the registers) D 0 D 7 Timer 0 Overflow Flag
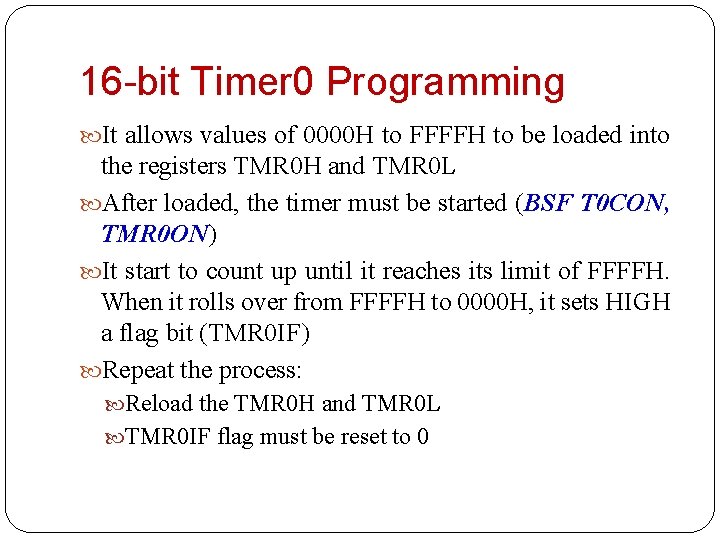
16 -bit Timer 0 Programming It allows values of 0000 H to FFFFH to be loaded into the registers TMR 0 H and TMR 0 L After loaded, the timer must be started (BSF T 0 CON, TMR 0 ON) It start to count up until it reaches its limit of FFFFH. When it rolls over from FFFFH to 0000 H, it sets HIGH a flag bit (TMR 0 IF) Repeat the process: Reload the TMR 0 H and TMR 0 L TMR 0 IF flag must be reset to 0
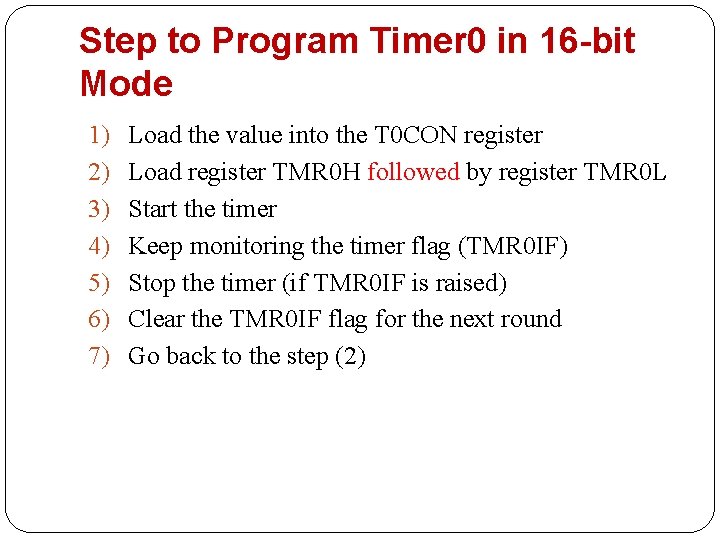
Step to Program Timer 0 in 16 -bit Mode 1) 2) 3) 4) 5) 6) 7) Load the value into the T 0 CON register Load register TMR 0 H followed by register TMR 0 L Start the timer Keep monitoring the timer flag (TMR 0 IF) Stop the timer (if TMR 0 IF is raised) Clear the TMR 0 IF flag for the next round Go back to the step (2)
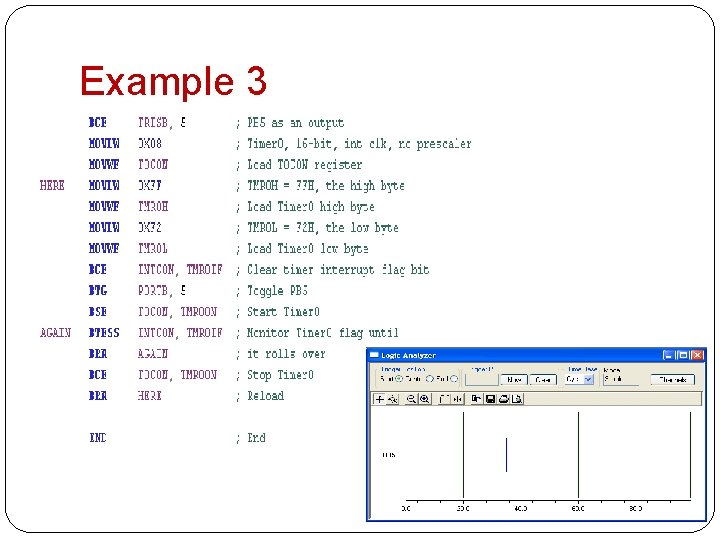
Example 3
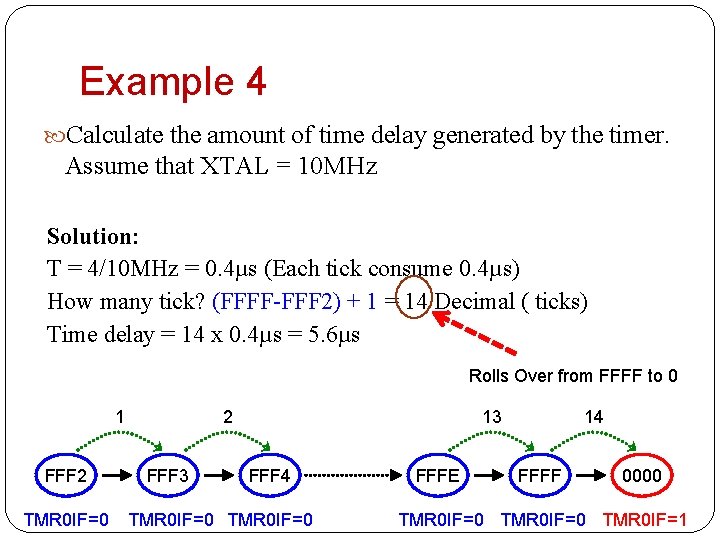
Example 4 Calculate the amount of time delay generated by the timer. Assume that XTAL = 10 MHz Solution: T = 4/10 MHz = 0. 4µs (Each tick consume 0. 4µs) How many tick? (FFFF-FFF 2) + 1 = 14 Decimal ( ticks) Time delay = 14 x 0. 4µs = 5. 6µs Rolls Over from FFFF to 0 1 FFF 2 TMR 0 IF=0 2 FFF 3 13 FFF 4 TMR 0 IF=0 FFFE 14 FFFF 0000 TMR 0 IF=0 TMR 0 IF=1
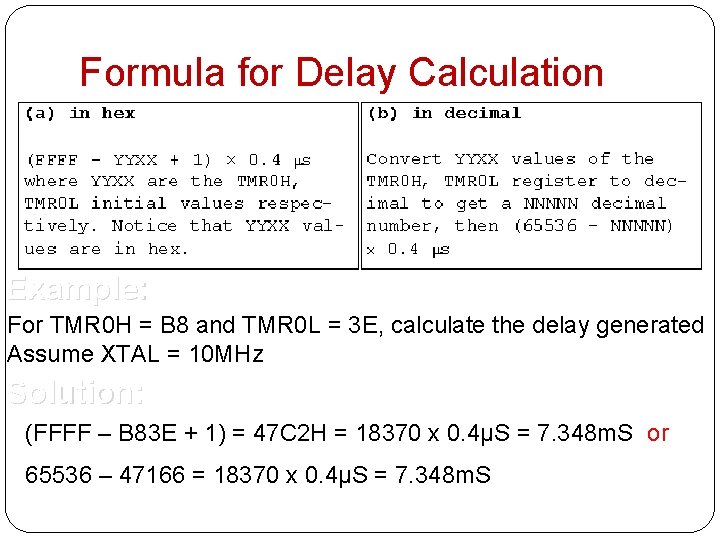
Formula for Delay Calculation Example: For TMR 0 H = B 8 and TMR 0 L = 3 E, calculate the delay generated Assume XTAL = 10 MHz Solution: (FFFF – B 83 E + 1) = 47 C 2 H = 18370 x 0. 4µS = 7. 348 m. S or 65536 – 47166 = 18370 x 0. 4µS = 7. 348 m. S
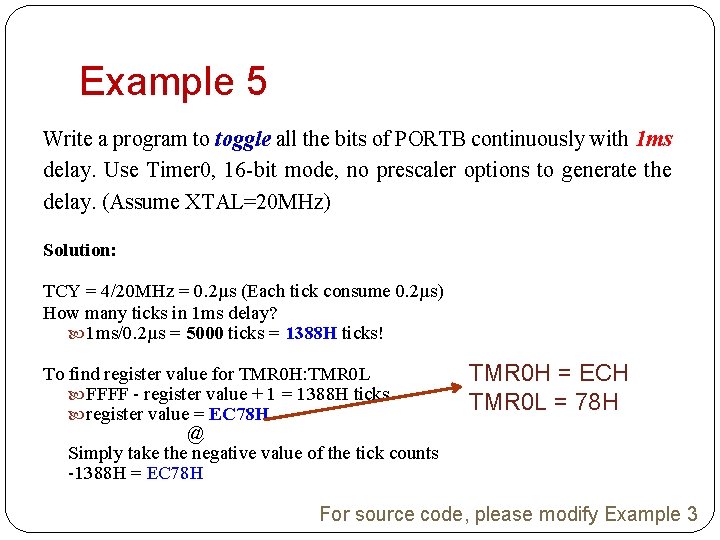
Example 5 Write a program to toggle all the bits of PORTB continuously with 1 ms delay. Use Timer 0, 16 -bit mode, no prescaler options to generate the delay. (Assume XTAL=20 MHz) Solution: TCY = 4/20 MHz = 0. 2µs (Each tick consume 0. 2µs) How many ticks in 1 ms delay? 1 ms/0. 2µs = 5000 ticks = 1388 H ticks! To find register value for TMR 0 H: TMR 0 L FFFF - register value + 1 = 1388 H ticks register value = EC 78 H @ Simply take the negative value of the tick counts -1388 H = EC 78 H TMR 0 H = ECH TMR 0 L = 78 H For source code, please modify Example 3
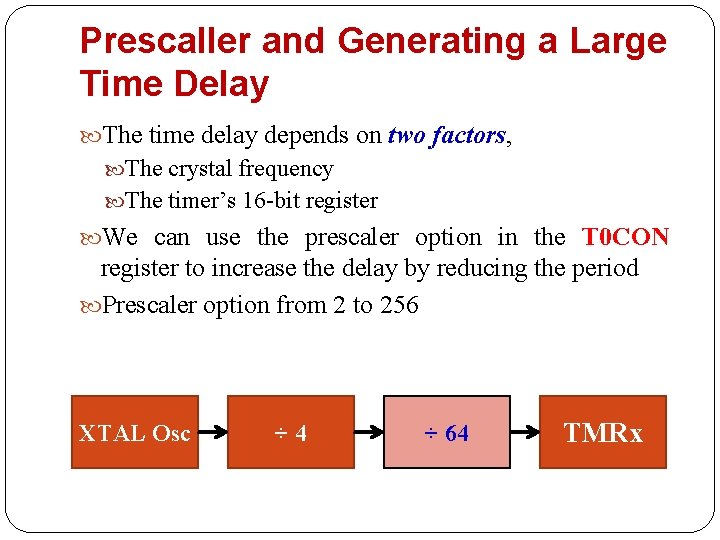
Prescaller and Generating a Large Time Delay The time delay depends on two factors, The crystal frequency The timer’s 16 -bit register We can use the prescaler option in the T 0 CON register to increase the delay by reducing the period Prescaler option from 2 to 256 XTAL Osc ÷ 4 ÷ 64 TMRx
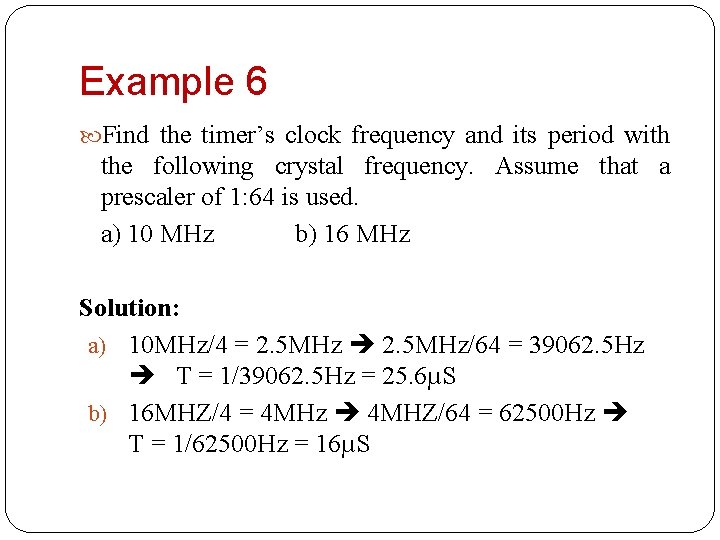
Example 6 Find the timer’s clock frequency and its period with the following crystal frequency. Assume that a prescaler of 1: 64 is used. a) 10 MHz b) 16 MHz Solution: a) 10 MHz/4 = 2. 5 MHz/64 = 39062. 5 Hz T = 1/39062. 5 Hz = 25. 6µS b) 16 MHZ/4 = 4 MHz 4 MHZ/64 = 62500 Hz T = 1/62500 Hz = 16µS
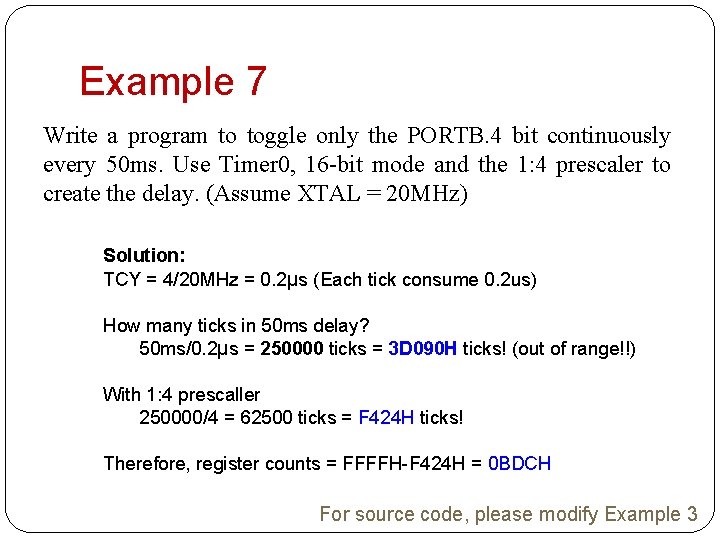
Example 7 Write a program to toggle only the PORTB. 4 bit continuously every 50 ms. Use Timer 0, 16 -bit mode and the 1: 4 prescaler to create the delay. (Assume XTAL = 20 MHz) Solution: TCY = 4/20 MHz = 0. 2µs (Each tick consume 0. 2 us) How many ticks in 50 ms delay? 50 ms/0. 2µs = 250000 ticks = 3 D 090 H ticks! (out of range!!) With 1: 4 prescaller 250000/4 = 62500 ticks = F 424 H ticks! Therefore, register counts = FFFFH-F 424 H = 0 BDCH For source code, please modify Example 3
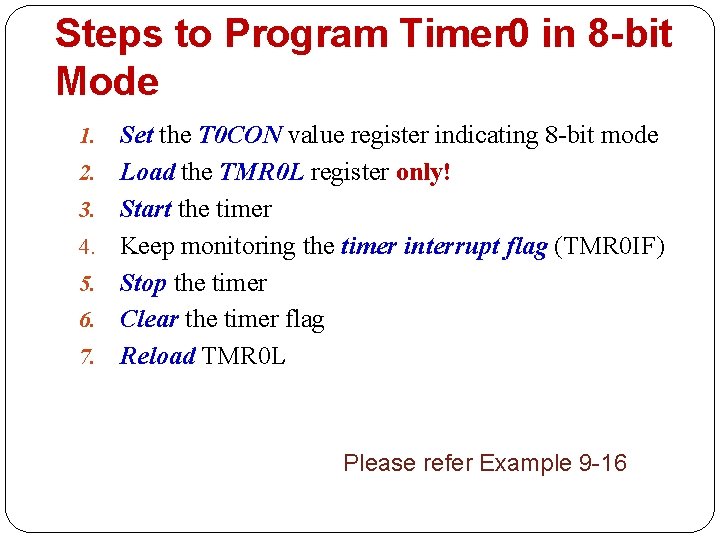
Steps to Program Timer 0 in 8 -bit Mode 1. 2. 3. 4. 5. 6. 7. Set the T 0 CON value register indicating 8 -bit mode Load the TMR 0 L register only! Start the timer Keep monitoring the timer interrupt flag (TMR 0 IF) Stop the timer Clear the timer flag Reload TMR 0 L Please refer Example 9 -16
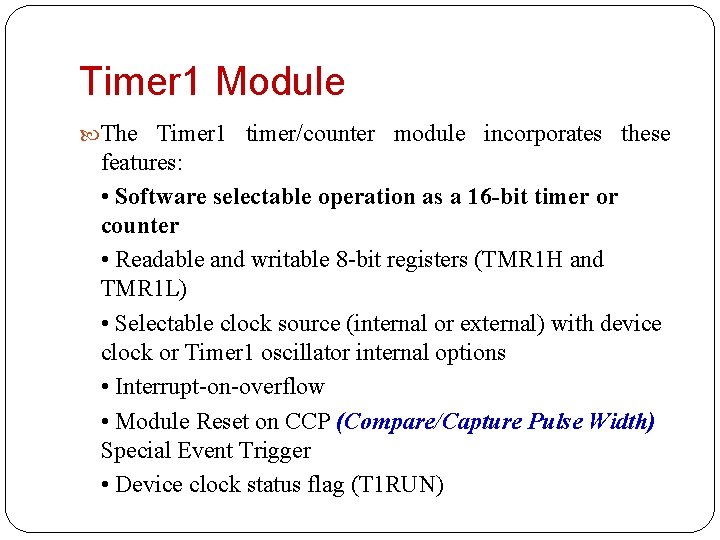
Timer 1 Module The Timer 1 timer/counter module incorporates these features: • Software selectable operation as a 16 -bit timer or counter • Readable and writable 8 -bit registers (TMR 1 H and TMR 1 L) • Selectable clock source (internal or external) with device clock or Timer 1 oscillator internal options • Interrupt-on-overflow • Module Reset on CCP (Compare/Capture Pulse Width) Special Event Trigger • Device clock status flag (T 1 RUN)
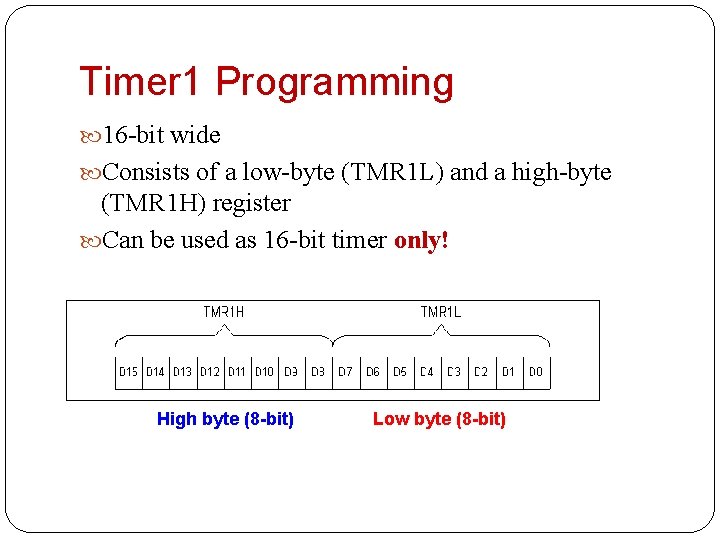
Timer 1 Programming 16 -bit wide Consists of a low-byte (TMR 1 L) and a high-byte (TMR 1 H) register Can be used as 16 -bit timer only! High byte (8 -bit) Low byte (8 -bit)
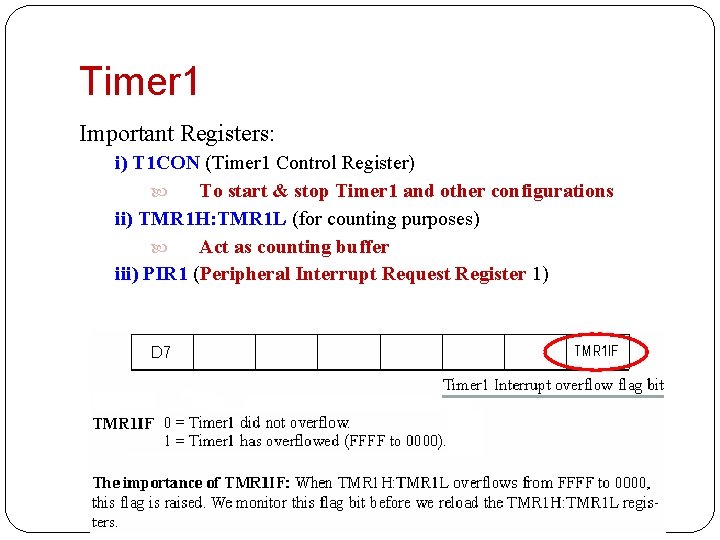
Timer 1 Important Registers: i) T 1 CON (Timer 1 Control Register) To start & stop Timer 1 and other configurations ii) TMR 1 H: TMR 1 L (for counting purposes) Act as counting buffer iii) PIR 1 (Peripheral Interrupt Request Register 1) D 7
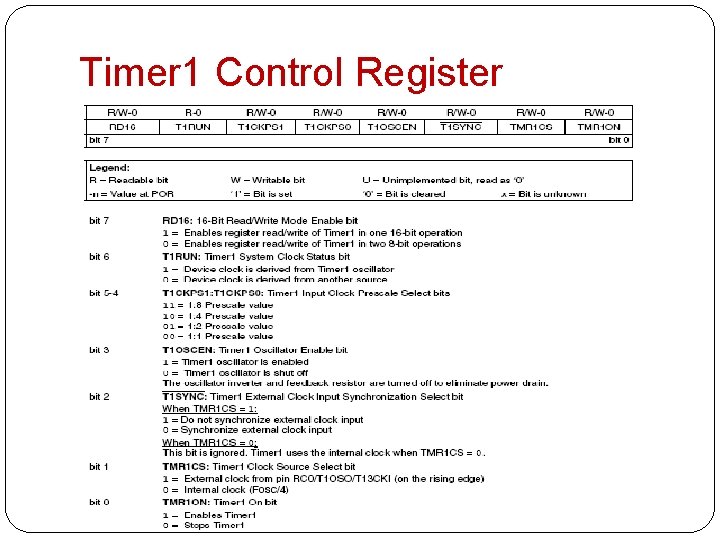
Timer 1 Control Register
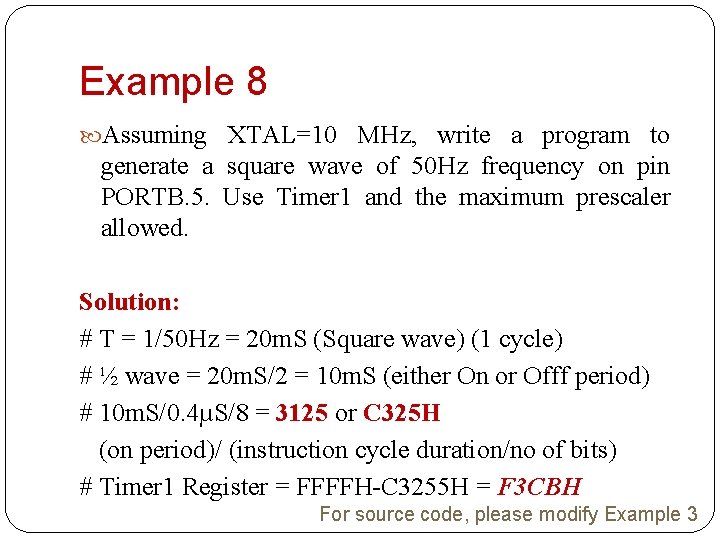
Example 8 Assuming XTAL=10 MHz, write a program to generate a square wave of 50 Hz frequency on pin PORTB. 5. Use Timer 1 and the maximum prescaler allowed. Solution: # T = 1/50 Hz = 20 m. S (Square wave) (1 cycle) # ½ wave = 20 m. S/2 = 10 m. S (either On or Offf period) # 10 m. S/0. 4µS/8 = 3125 or C 325 H (on period)/ (instruction cycle duration/no of bits) # Timer 1 Register = FFFFH-C 3255 H = F 3 CBH For source code, please modify Example 3
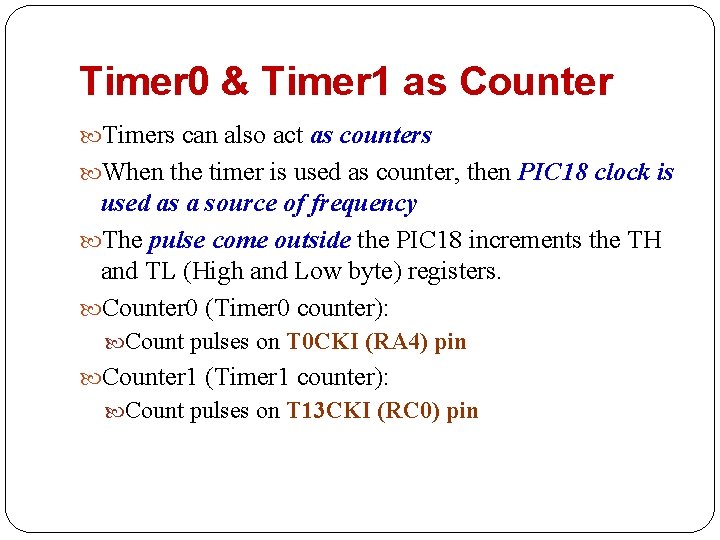
Timer 0 & Timer 1 as Counter Timers can also act as counters When the timer is used as counter, then PIC 18 clock is used as a source of frequency The pulse come outside the PIC 18 increments the TH and TL (High and Low byte) registers. Counter 0 (Timer 0 counter): Count pulses on T 0 CKI (RA 4) pin Counter 1 (Timer 1 counter): Count pulses on T 13 CKI (RC 0) pin
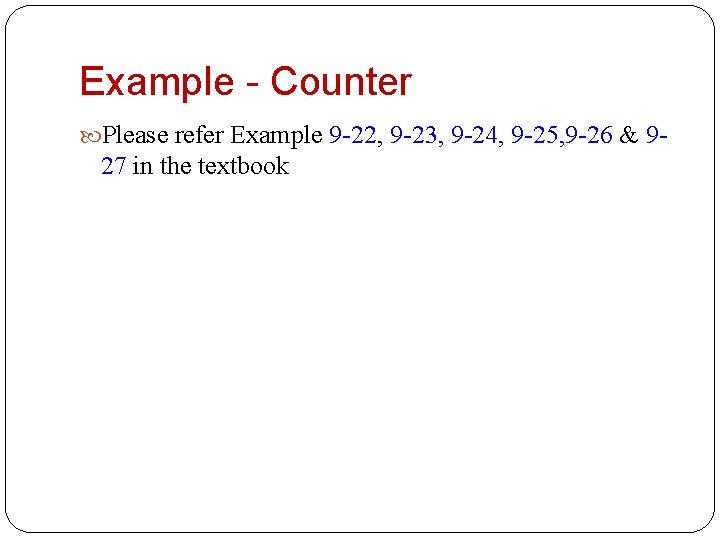
Example - Counter Please refer Example 9 -22, 9 -23, 9 -24, 9 -25, 9 -26 & 9 - 27 in the textbook
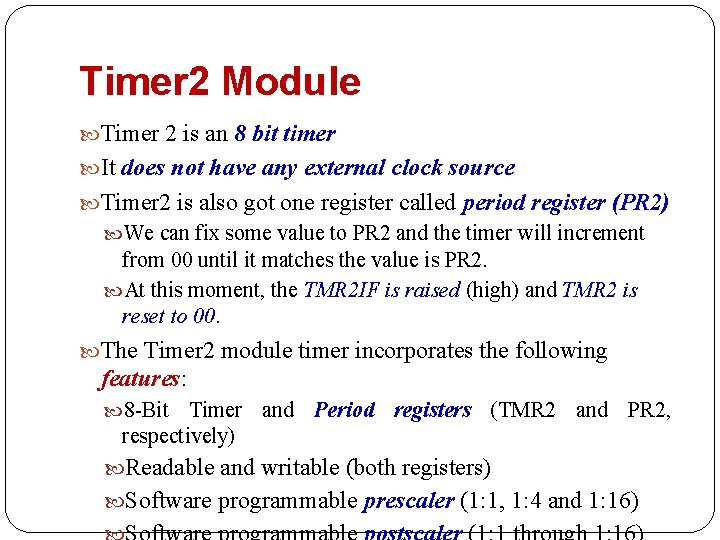
Timer 2 Module Timer 2 is an 8 bit timer It does not have any external clock source Timer 2 is also got one register called period register (PR 2) We can fix some value to PR 2 and the timer will increment from 00 until it matches the value is PR 2. At this moment, the TMR 2 IF is raised (high) and TMR 2 is reset to 00. The Timer 2 module timer incorporates the following features: 8 -Bit Timer and Period registers (TMR 2 and PR 2, respectively) Readable and writable (both registers) Software programmable prescaler (1: 1, 1: 4 and 1: 16)
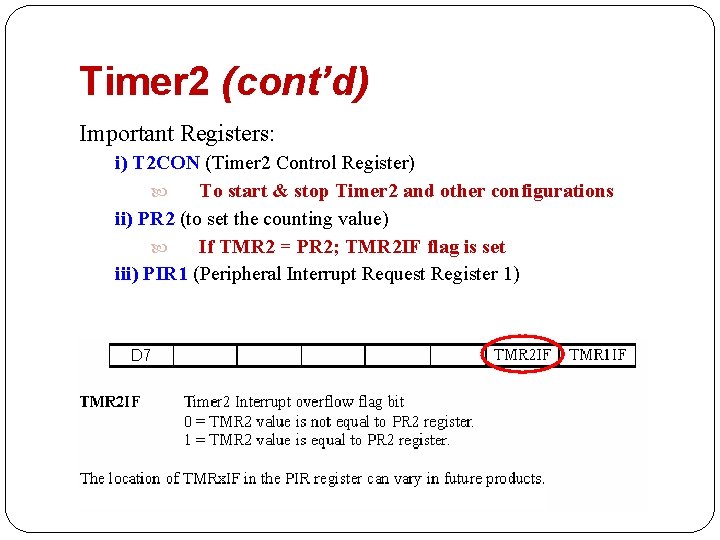
Timer 2 (cont’d) Important Registers: i) T 2 CON (Timer 2 Control Register) To start & stop Timer 2 and other configurations ii) PR 2 (to set the counting value) If TMR 2 = PR 2; TMR 2 IF flag is set iii) PIR 1 (Peripheral Interrupt Request Register 1) D 7
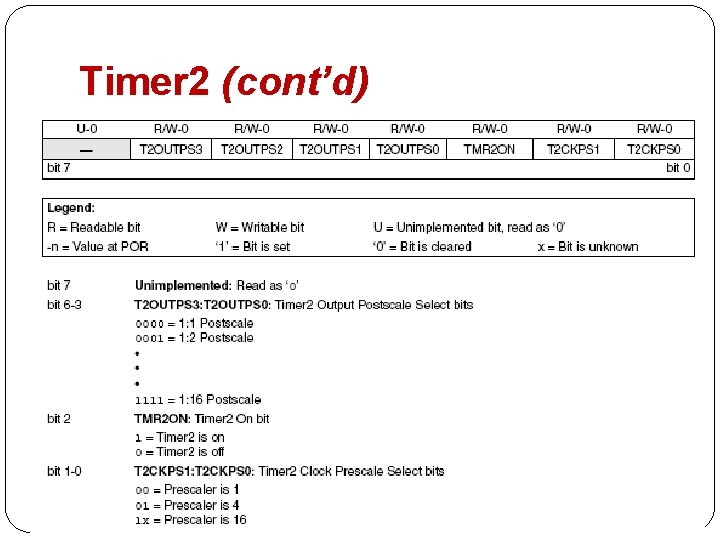
Timer 2 (cont’d)
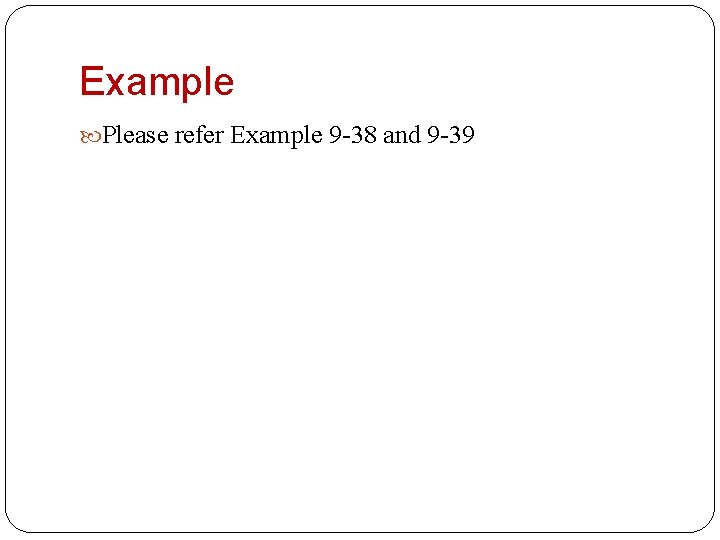
Example Please refer Example 9 -38 and 9 -39
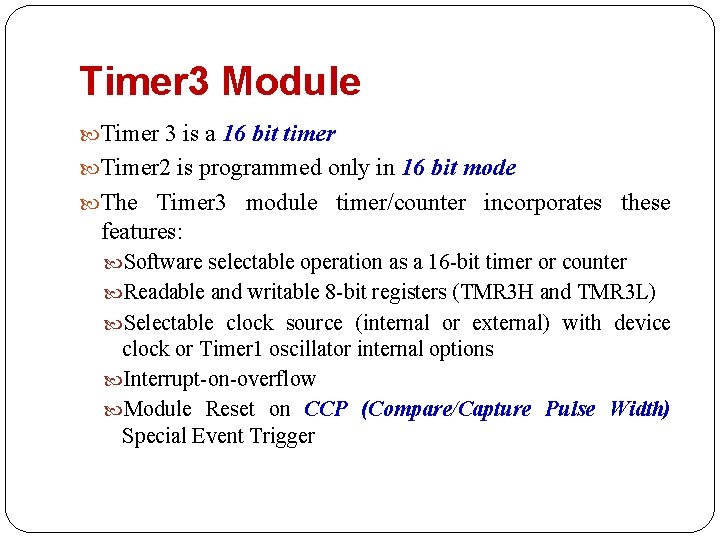
Timer 3 Module Timer 3 is a 16 bit timer Timer 2 is programmed only in 16 bit mode The Timer 3 module timer/counter incorporates these features: Software selectable operation as a 16 -bit timer or counter Readable and writable 8 -bit registers (TMR 3 H and TMR 3 L) Selectable clock source (internal or external) with device clock or Timer 1 oscillator internal options Interrupt-on-overflow Module Reset on CCP (Compare/Capture Pulse Width) Special Event Trigger
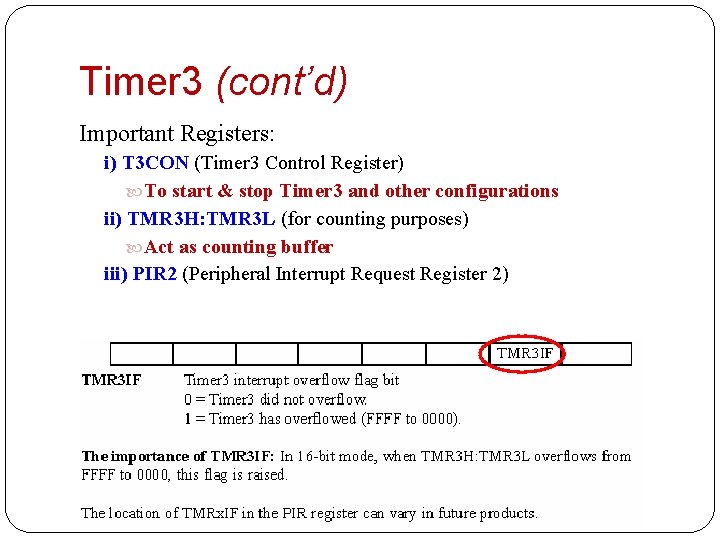
Timer 3 (cont’d) Important Registers: i) T 3 CON (Timer 3 Control Register) To start & stop Timer 3 and other configurations ii) TMR 3 H: TMR 3 L (for counting purposes) Act as counting buffer iii) PIR 2 (Peripheral Interrupt Request Register 2)
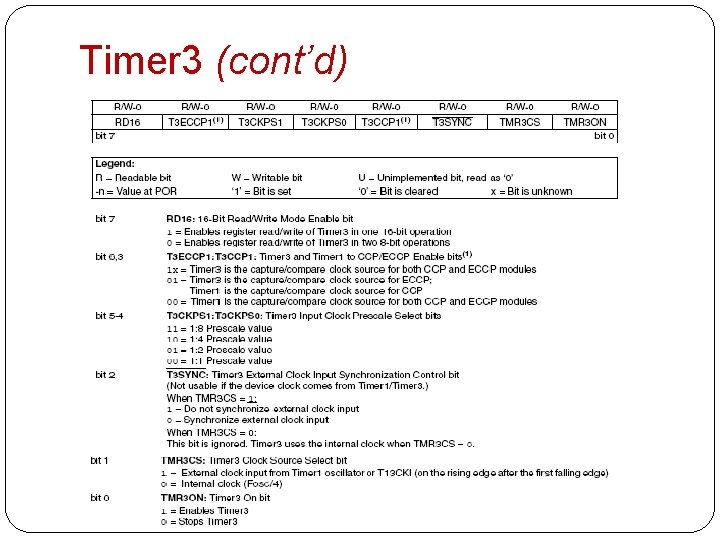
Timer 3 (cont’d)
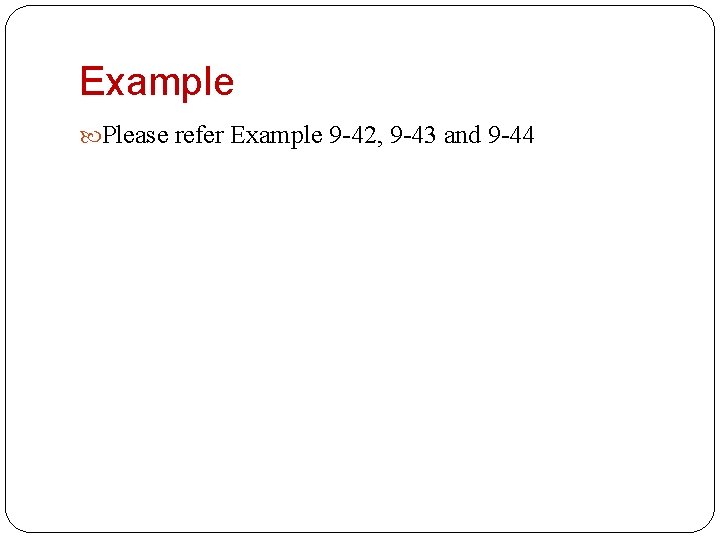
Example Please refer Example 9 -42, 9 -43 and 9 -44
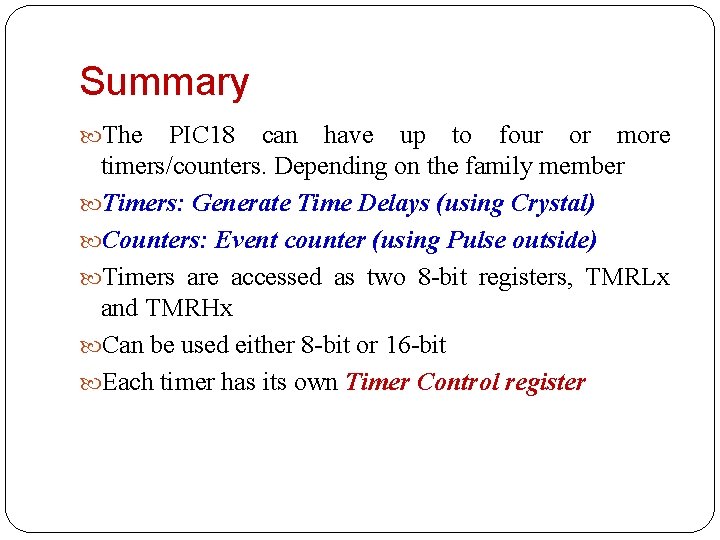
Summary The PIC 18 can have up to four or more timers/counters. Depending on the family member Timers: Generate Time Delays (using Crystal) Counters: Event counter (using Pulse outside) Timers are accessed as two 8 -bit registers, TMRLx and TMRHx Can be used either 8 -bit or 16 -bit Each timer has its own Timer Control register
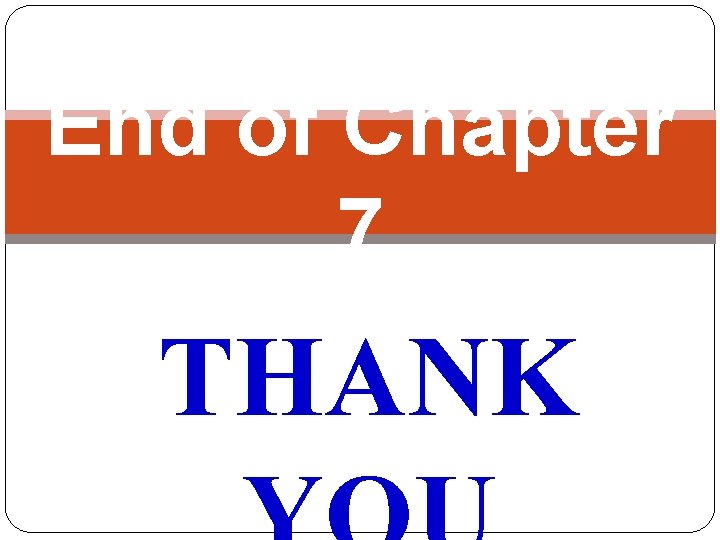
End of Chapter 7 THANK
- Slides: 40