PHP Hypertext Preprocessor What is a PHP File
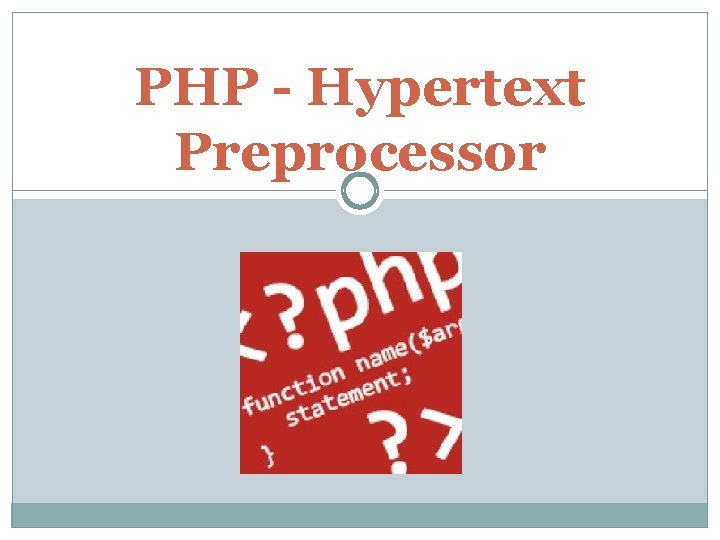
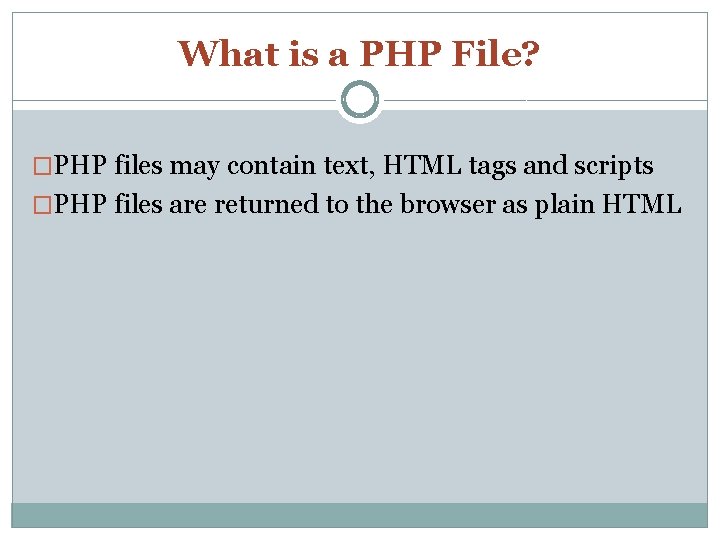
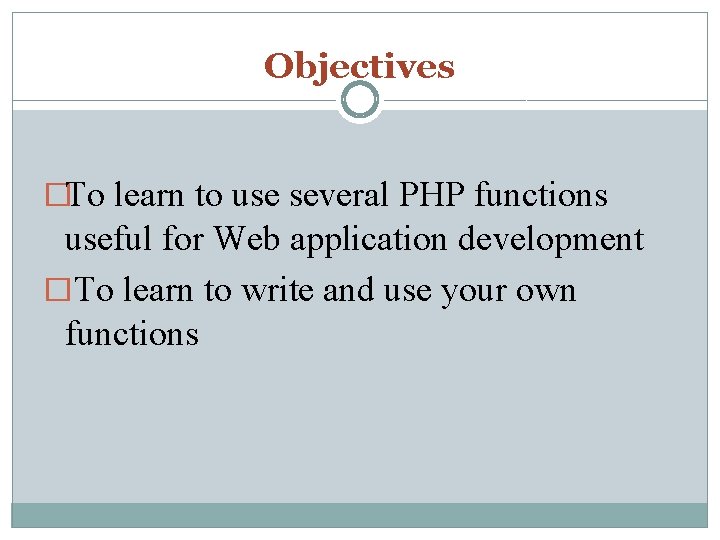
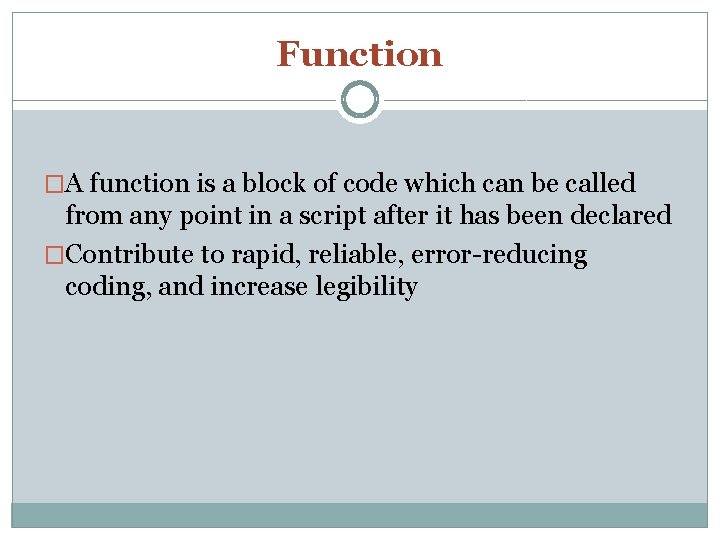
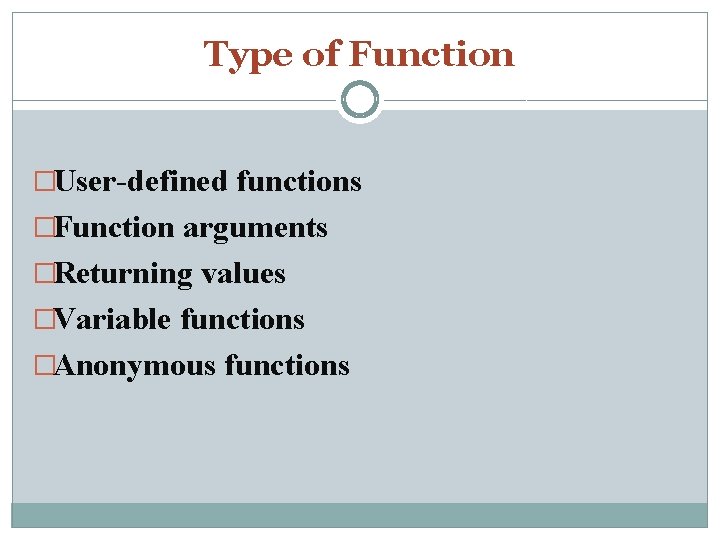
![Function �Some basic numeric PHP functions—E. g. , i) absolute value[abs()], ii) square root Function �Some basic numeric PHP functions—E. g. , i) absolute value[abs()], ii) square root](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-6.jpg)
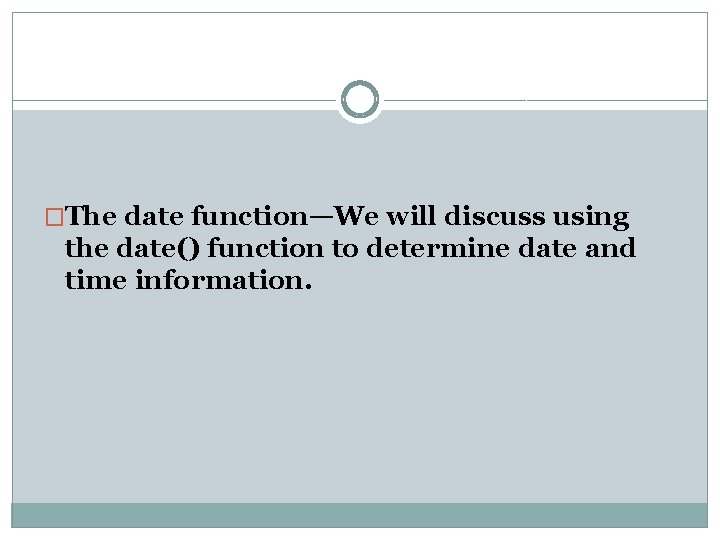
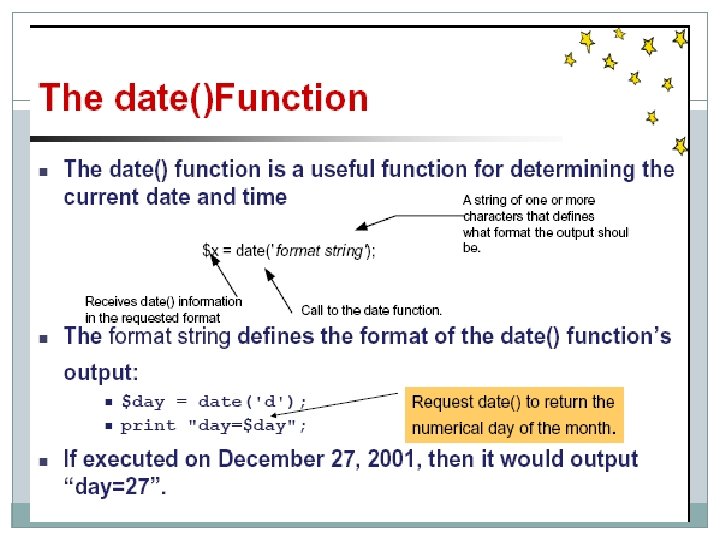
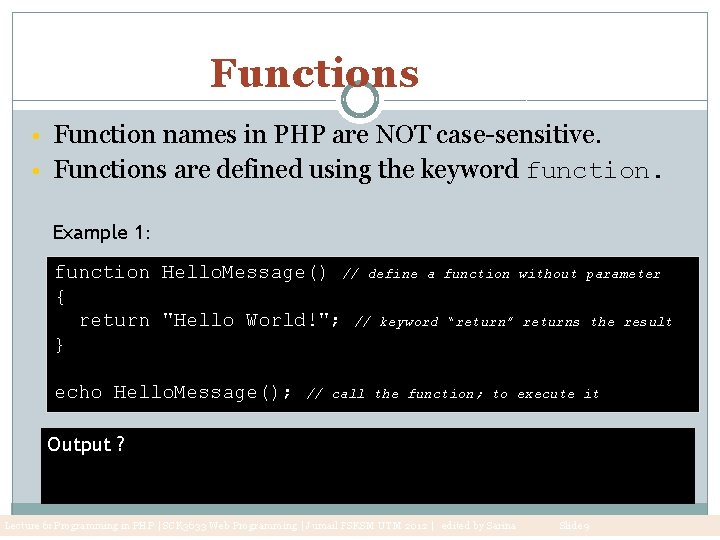
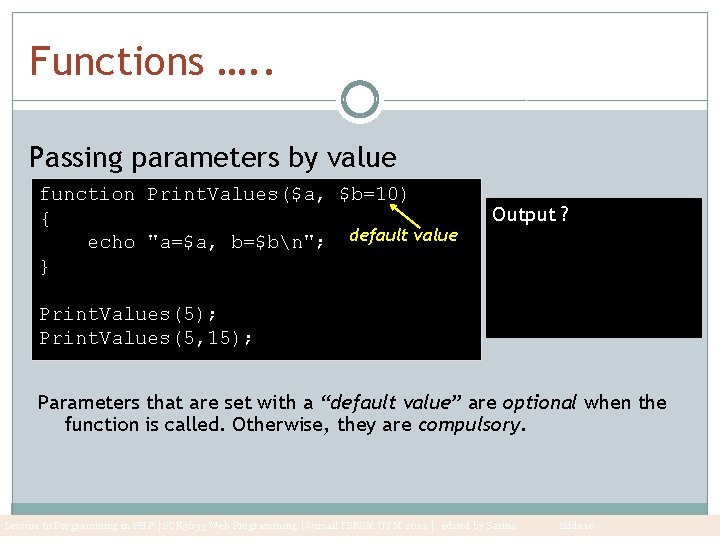
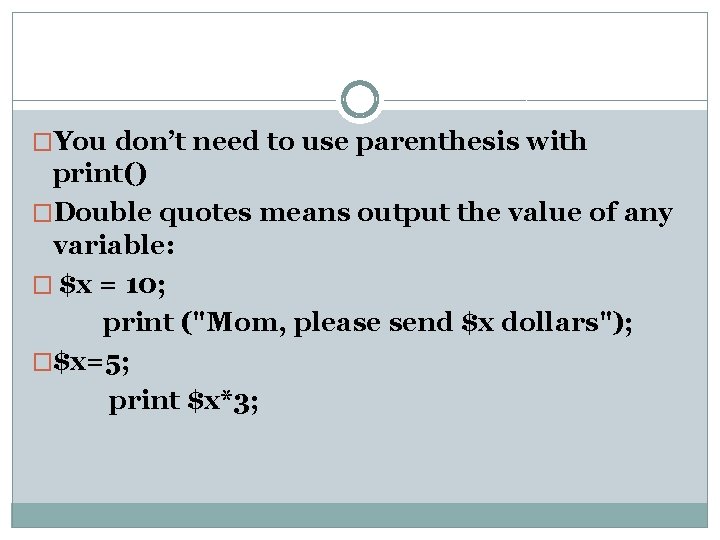
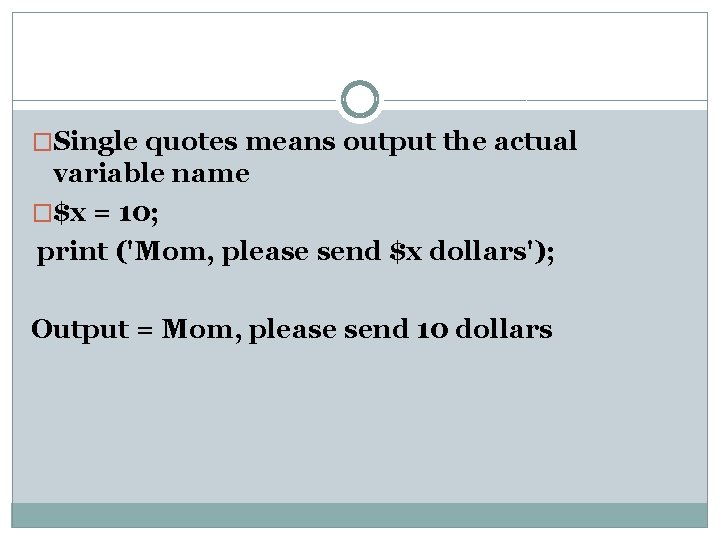
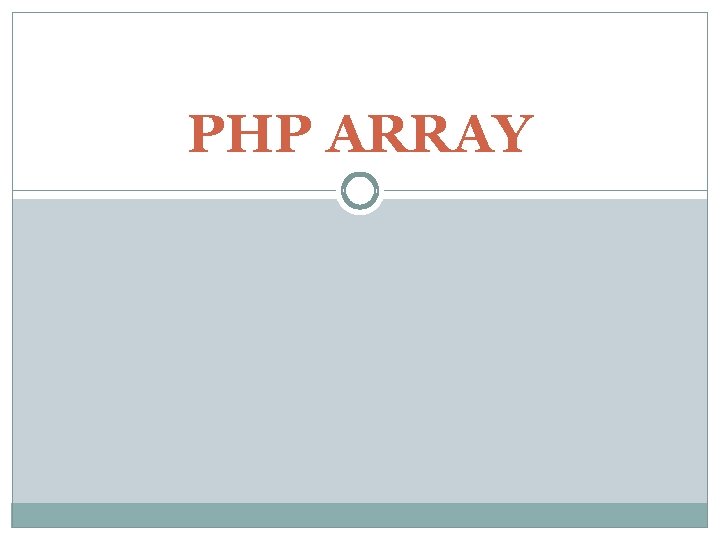
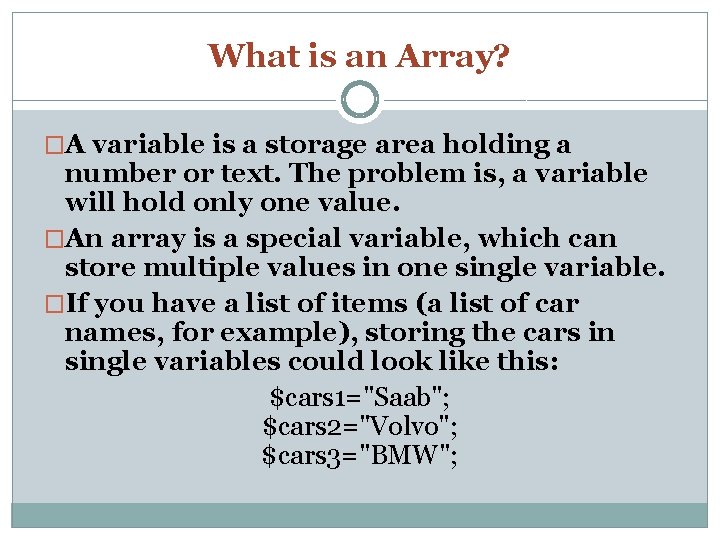
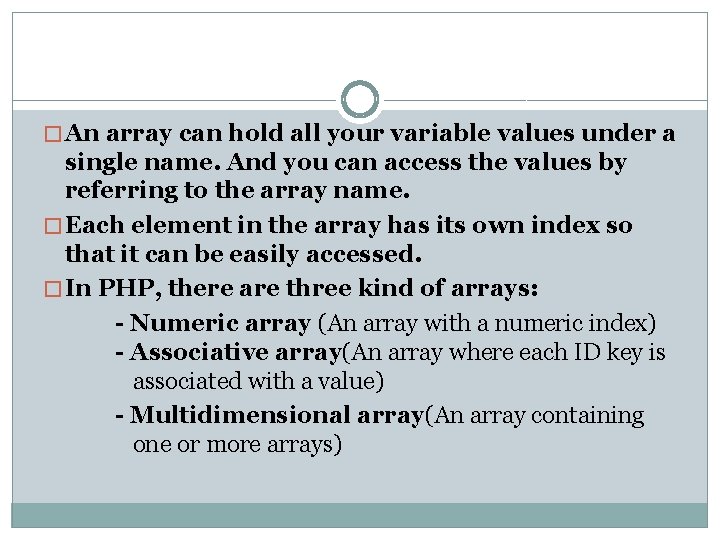
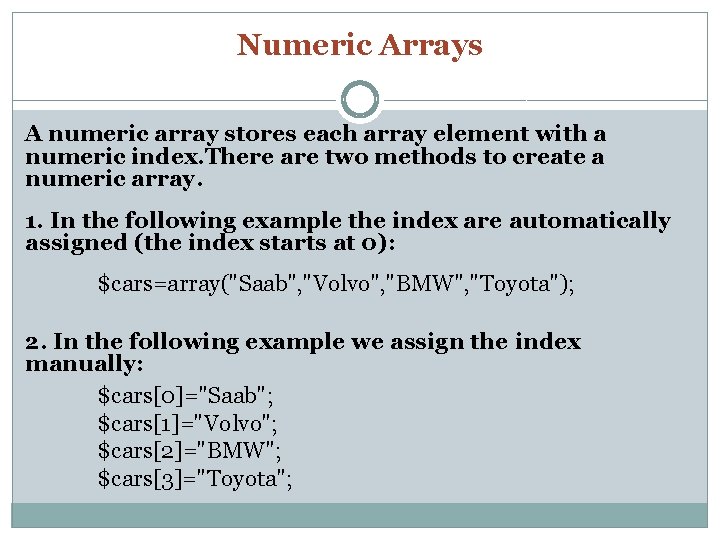
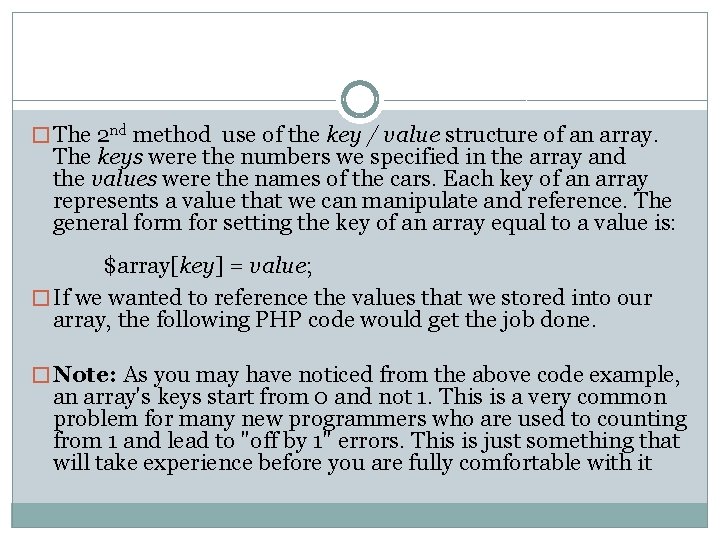
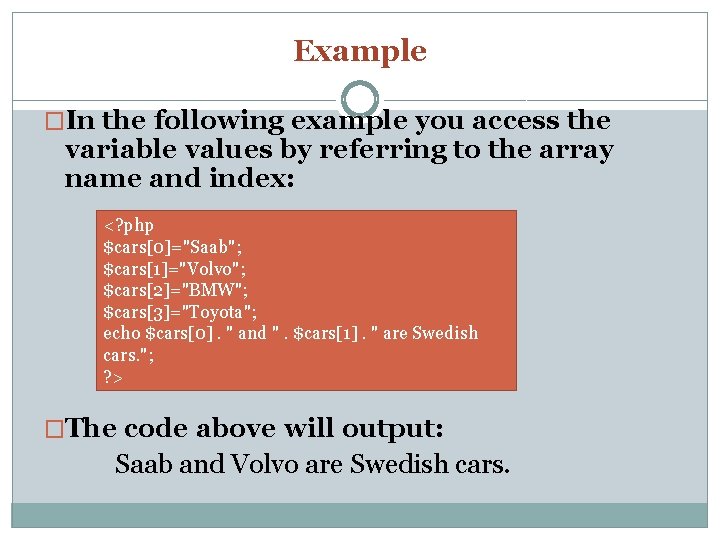
![PHP array (Numeric) Numeric Arrays $names = array("Peter", "Quagmire", "Joe"); Assign manualy: $names[0] = PHP array (Numeric) Numeric Arrays $names = array("Peter", "Quagmire", "Joe"); Assign manualy: $names[0] =](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-19.jpg)
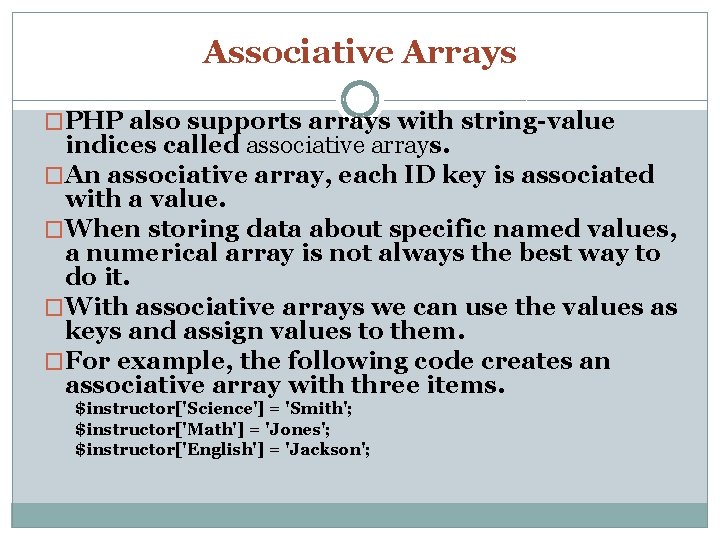
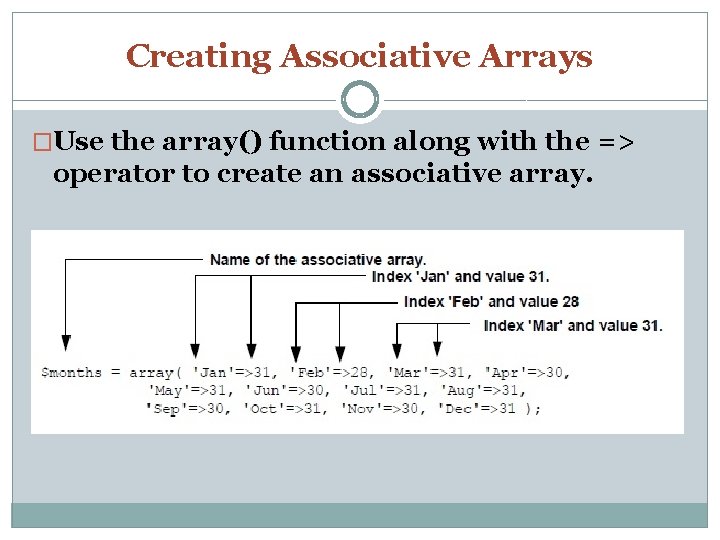
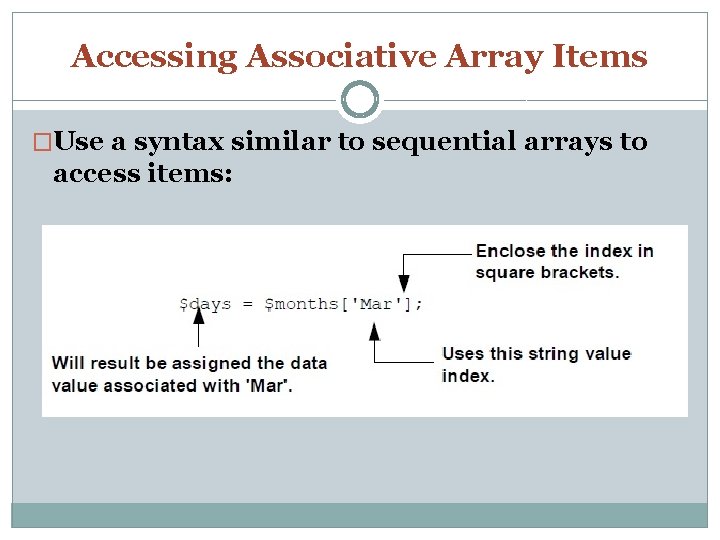
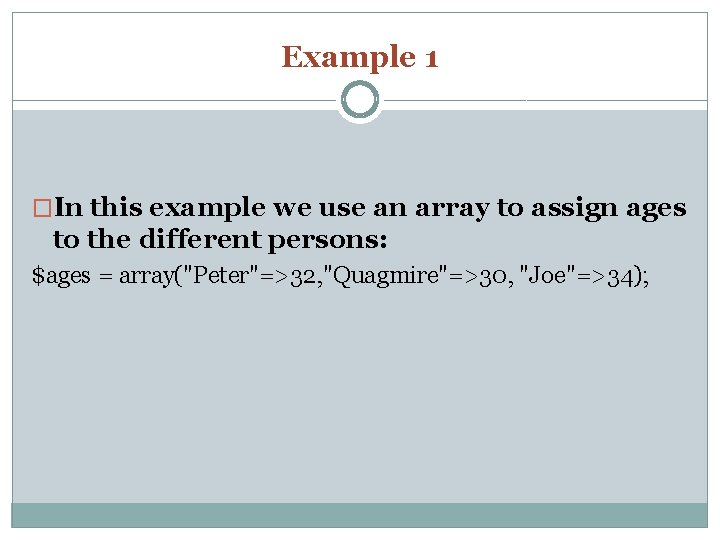
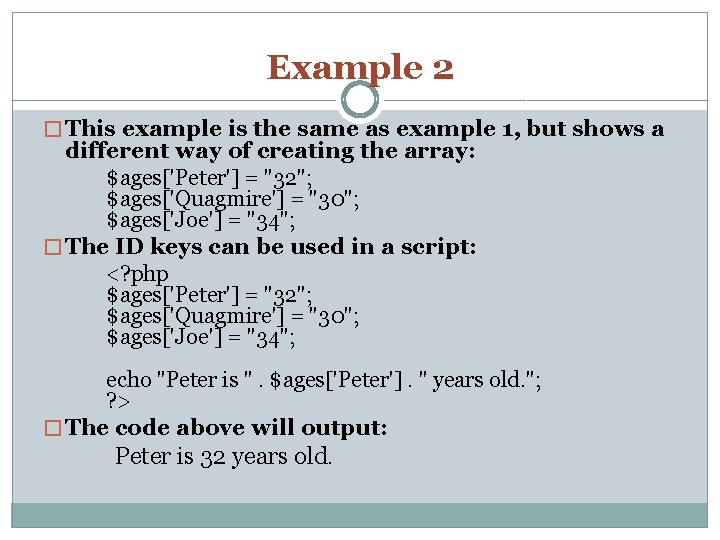
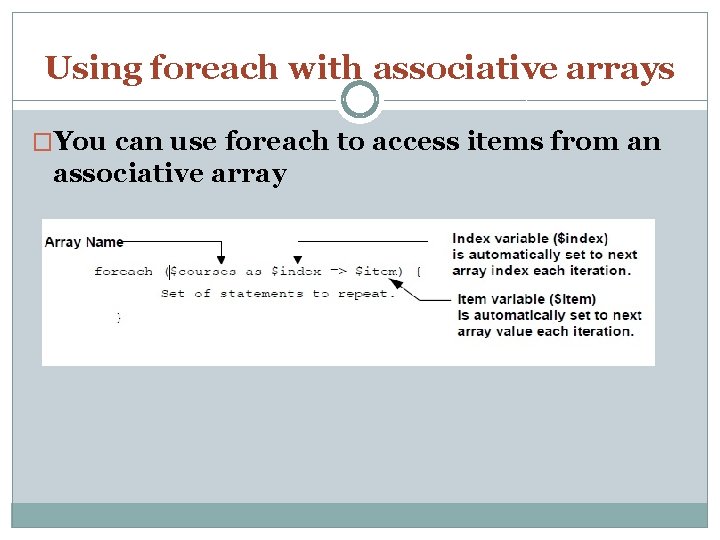
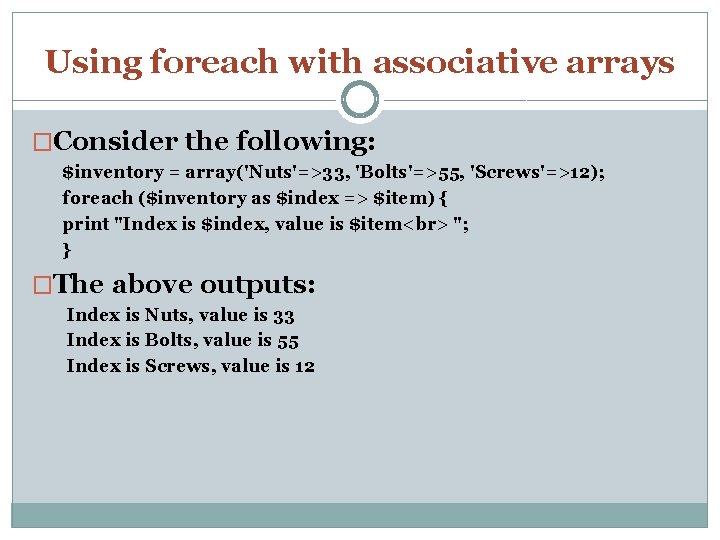
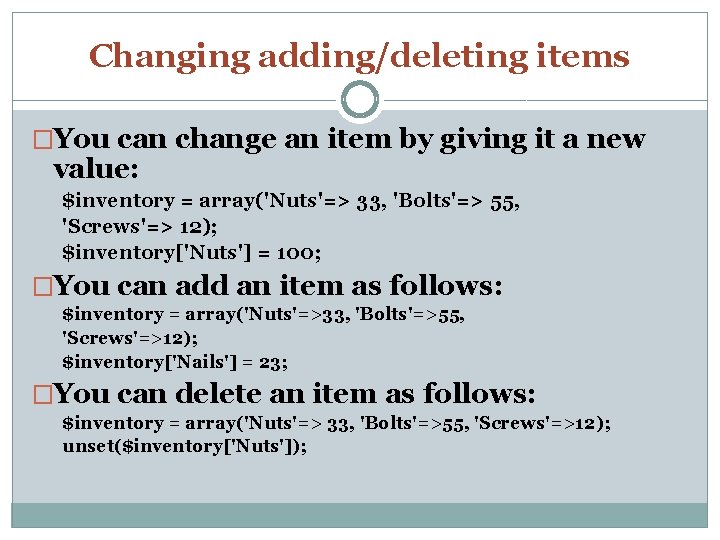
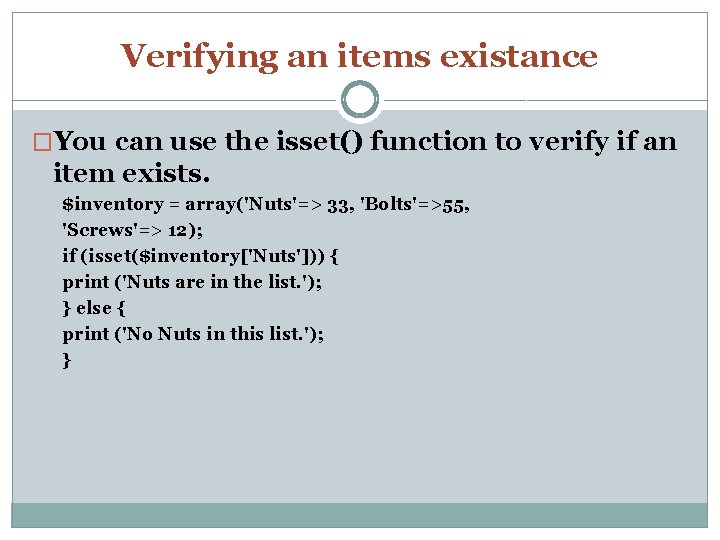
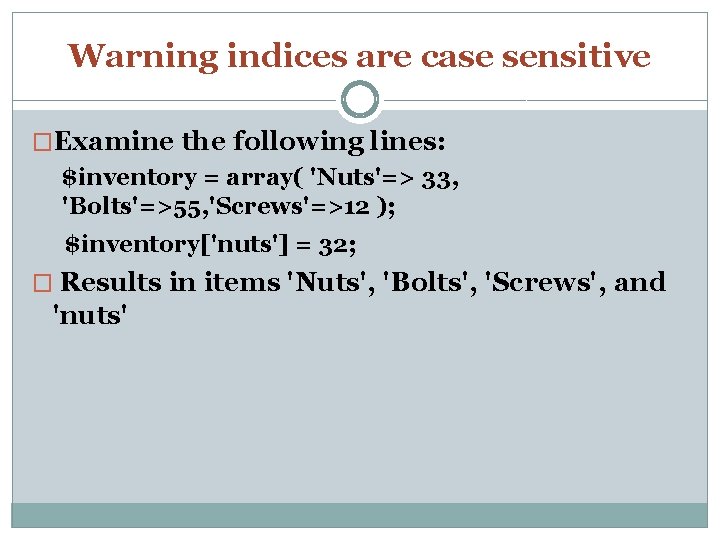
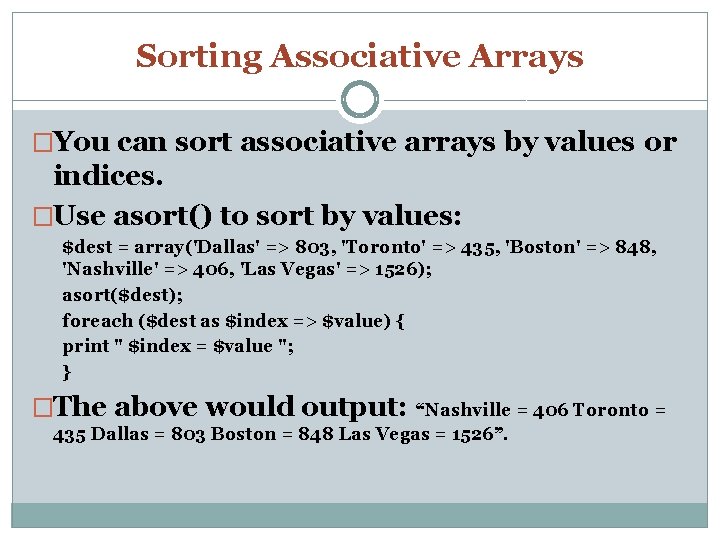
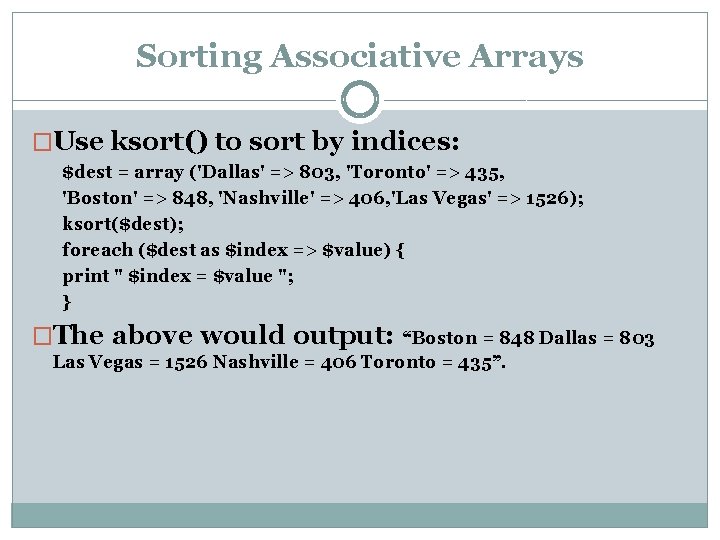
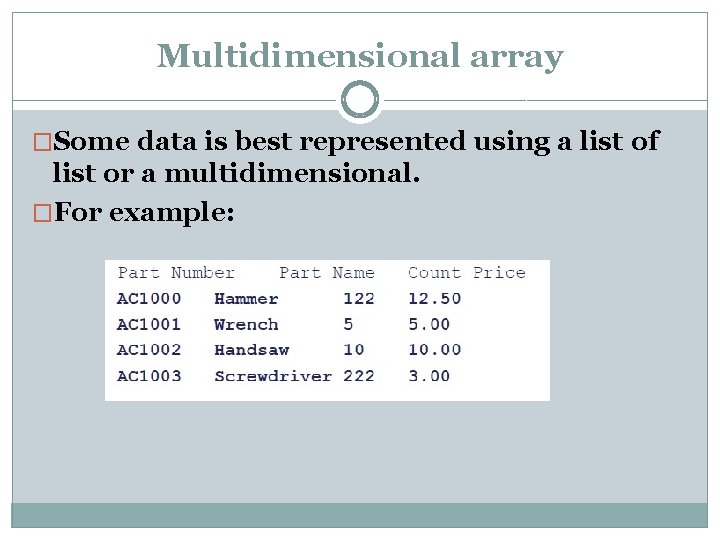
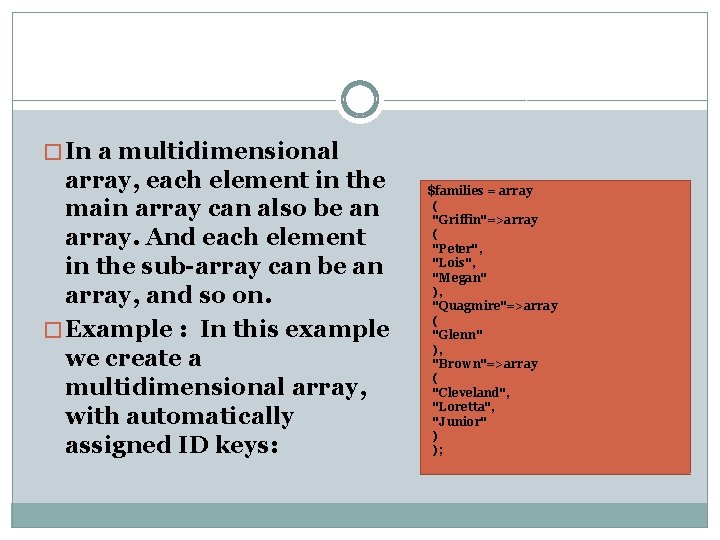
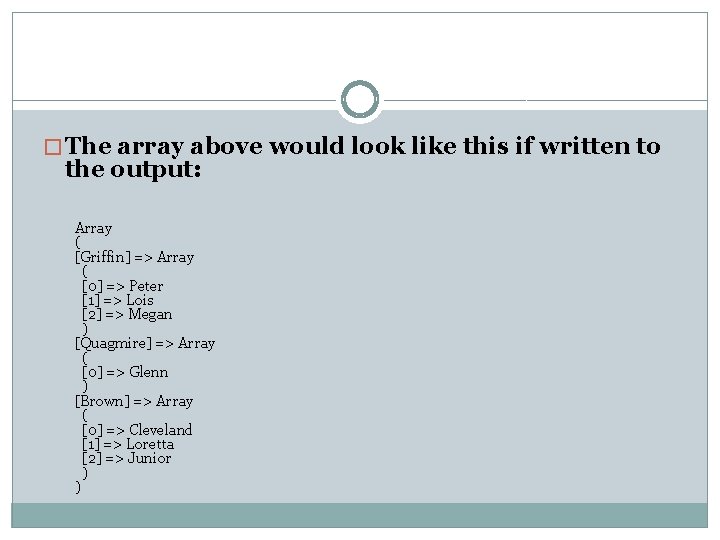
![Creating Multidimensional Lists �You can create multidimensional arrays with the array() function $inventory['AC 1000']['Part'] Creating Multidimensional Lists �You can create multidimensional arrays with the array() function $inventory['AC 1000']['Part']](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-35.jpg)
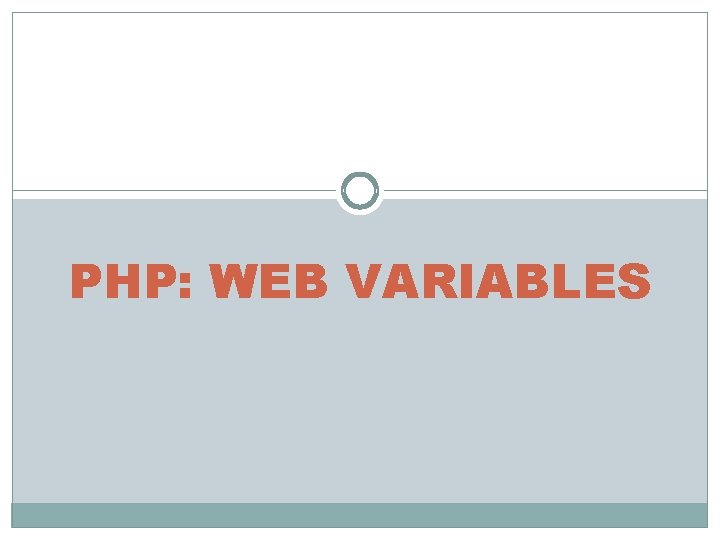
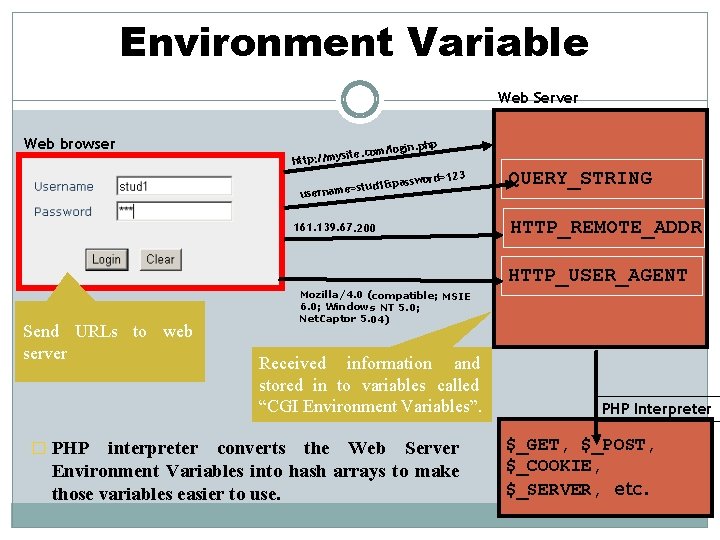
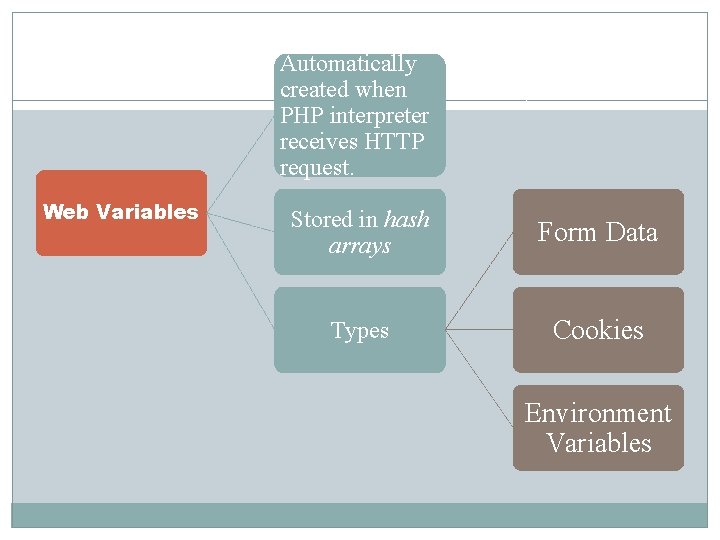
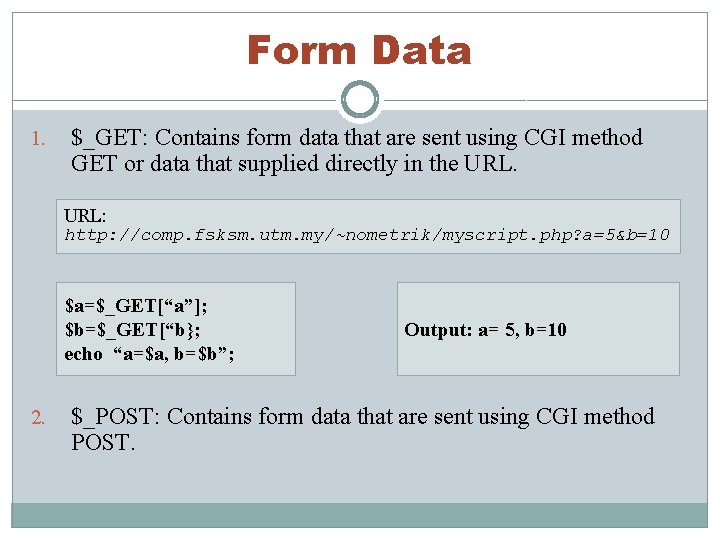
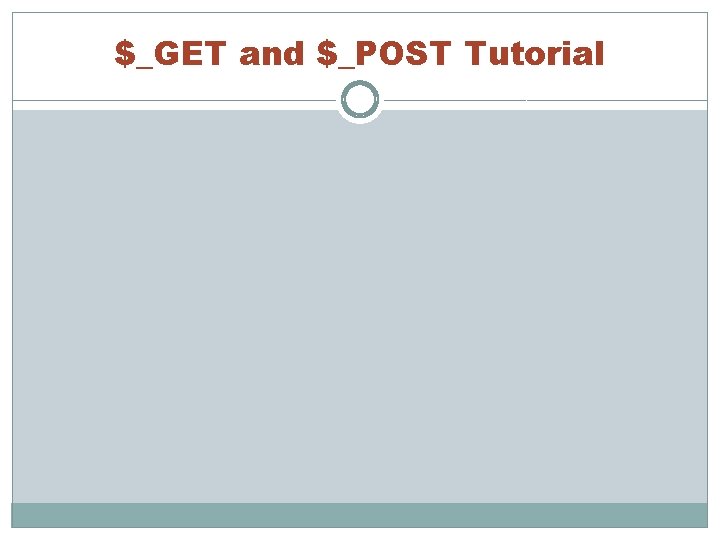
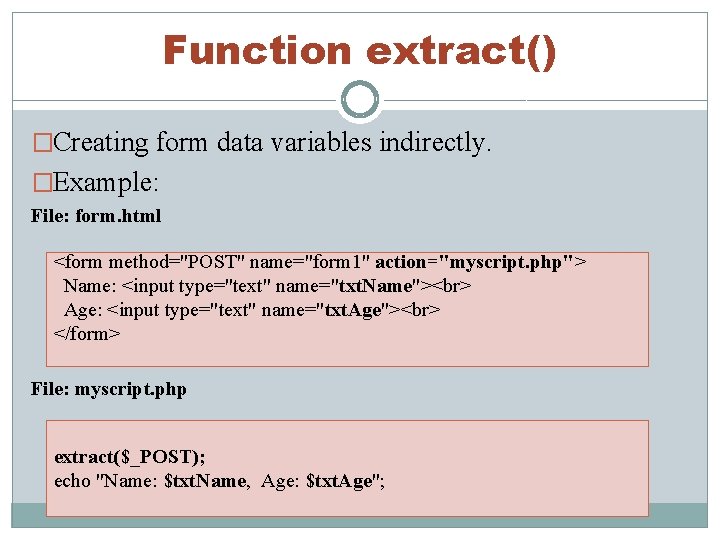
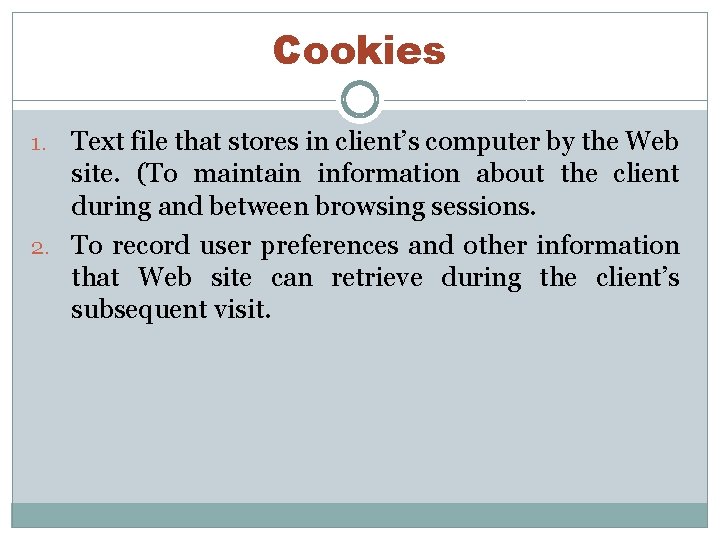
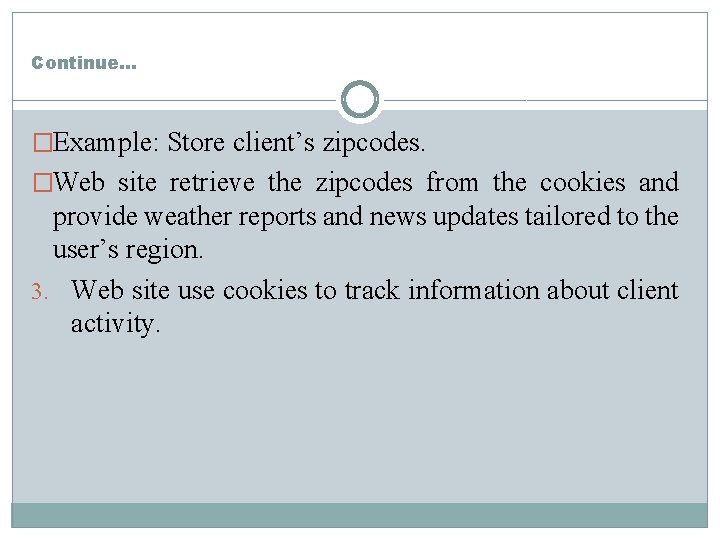
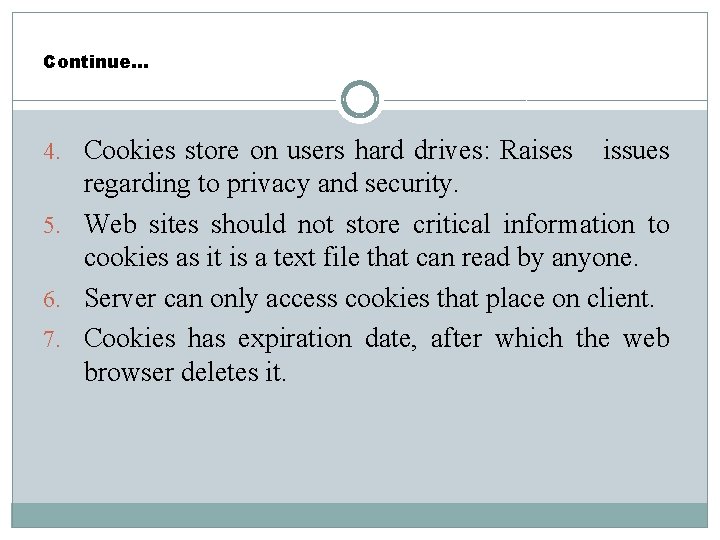
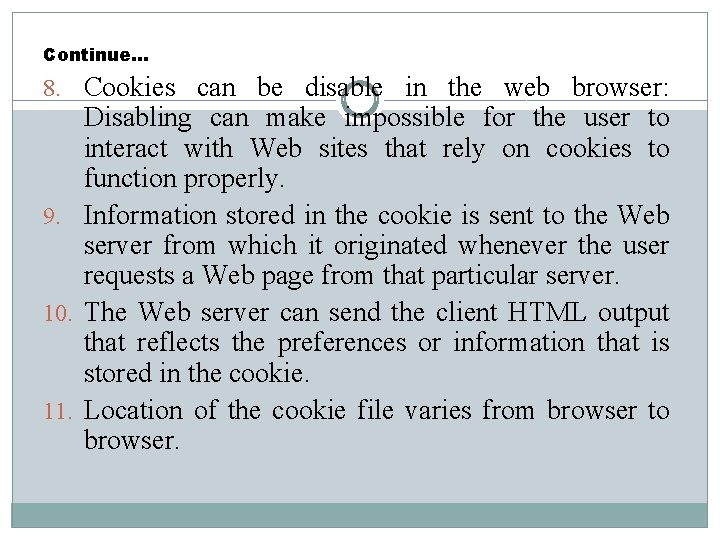
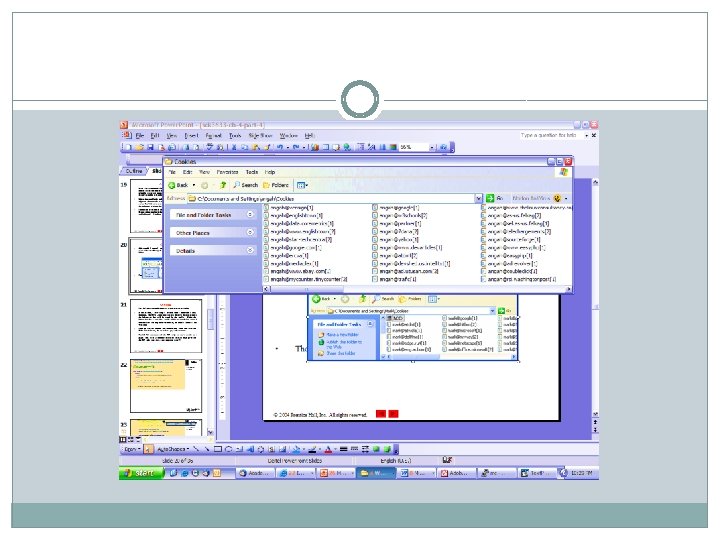
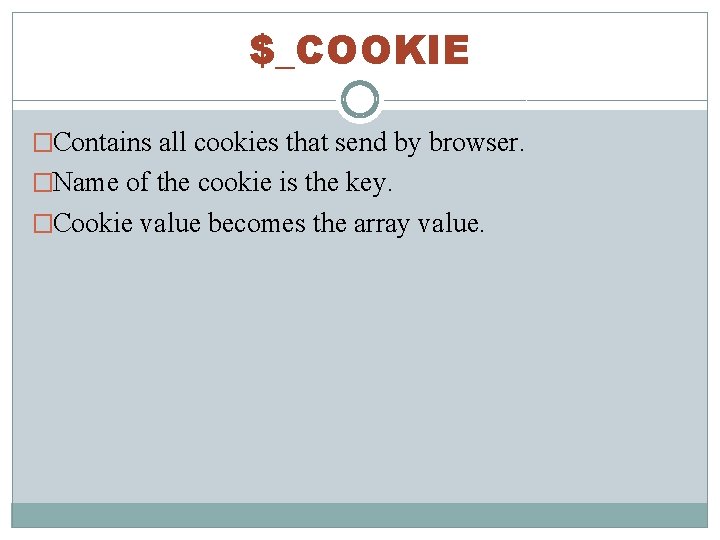
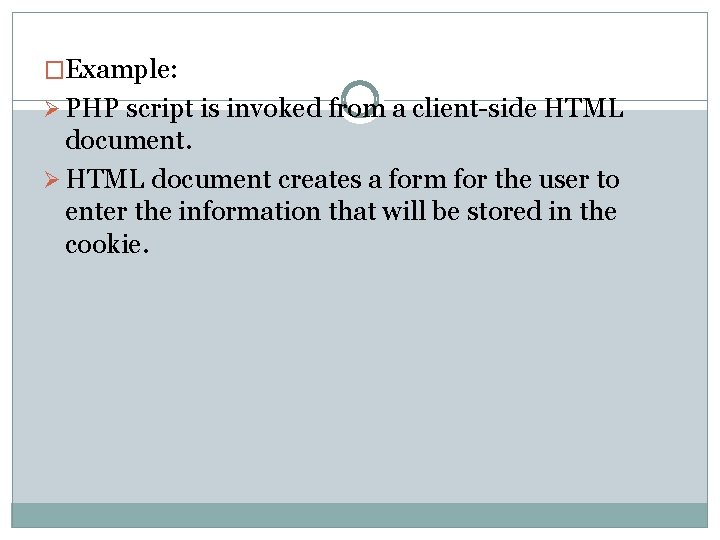
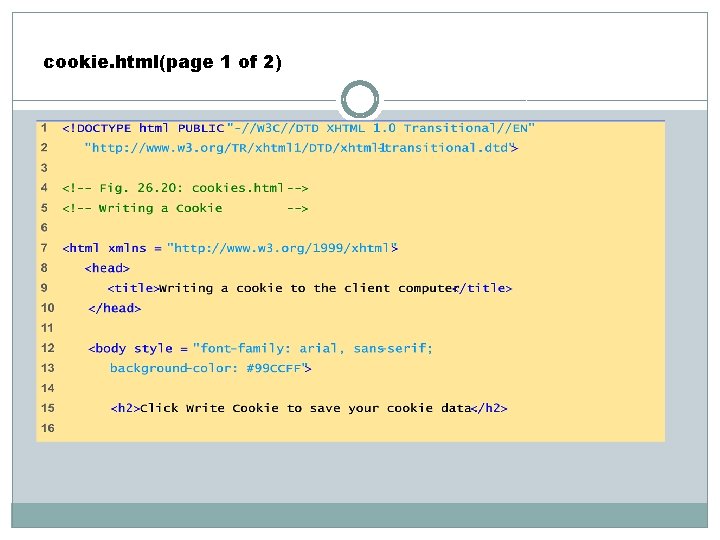
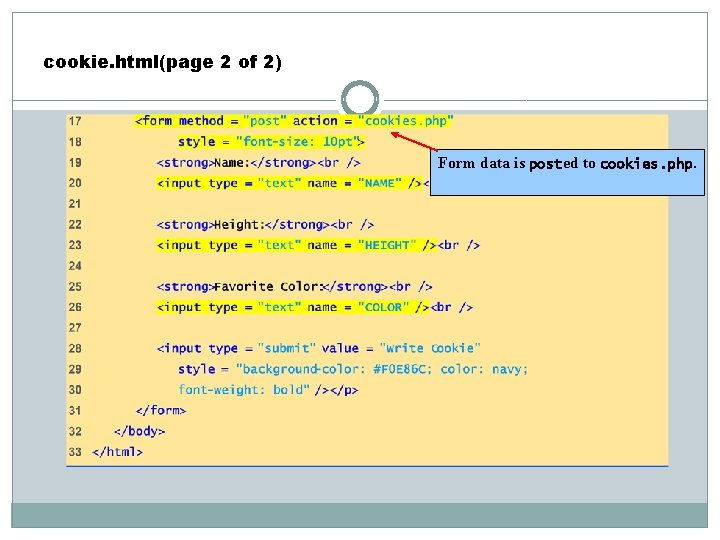
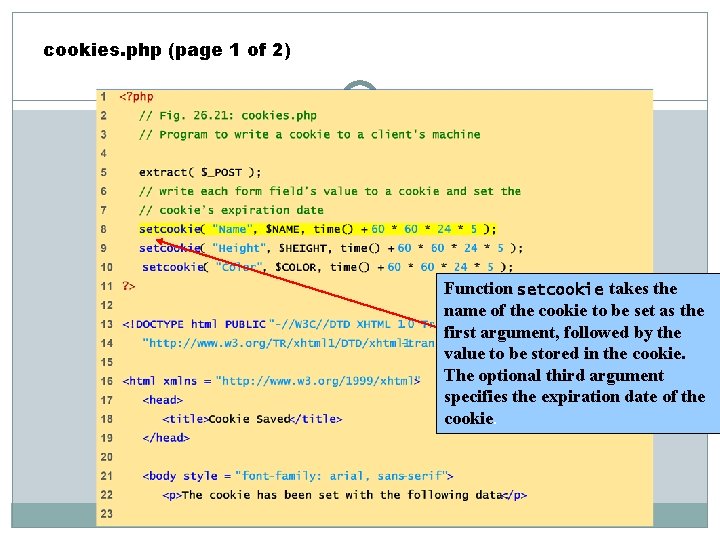
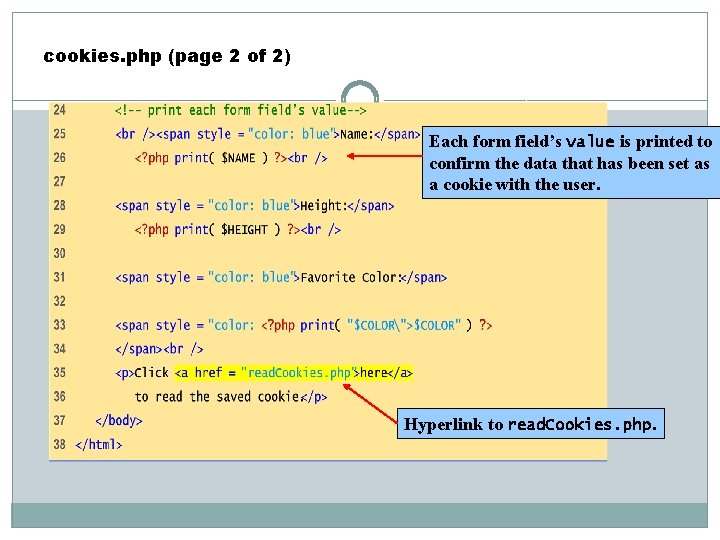
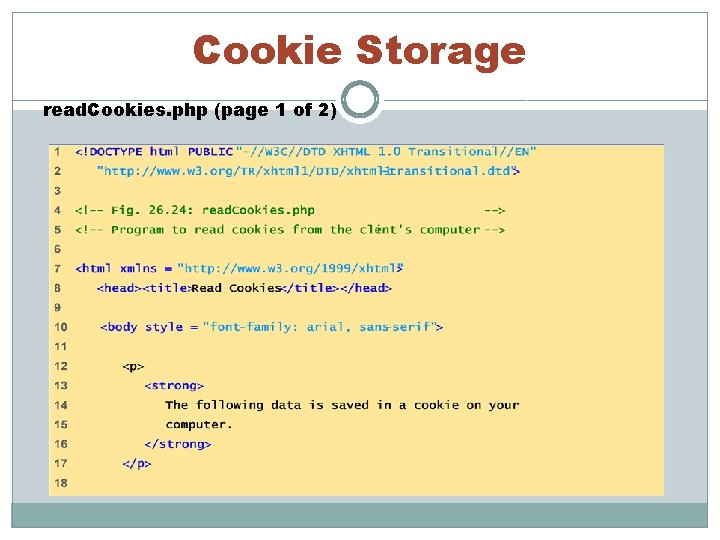
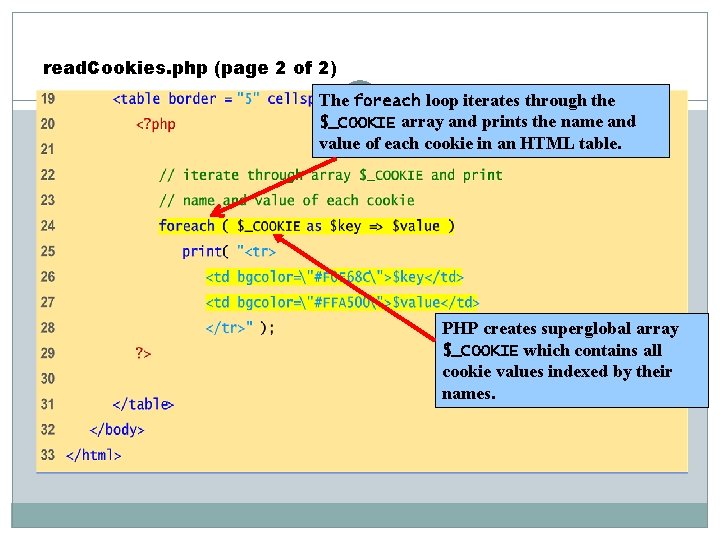
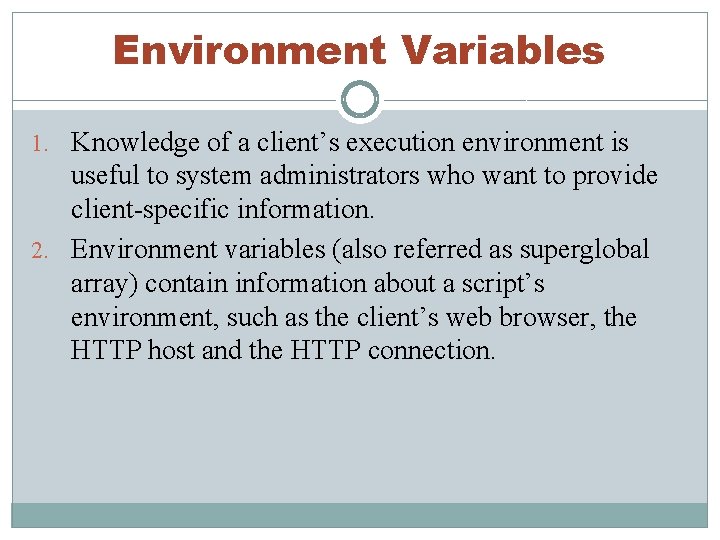
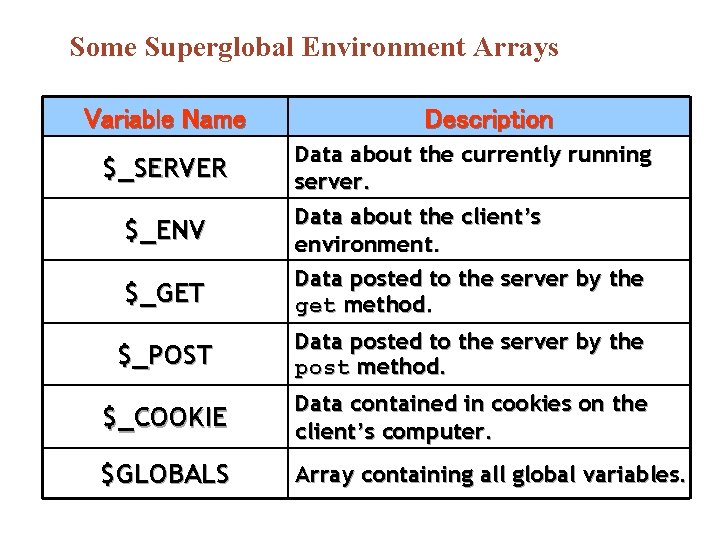
![Some Common $_SERVER Elements Variable Contains Example $_SERVER['PHP_SELF'] The current script. Suitable for use Some Common $_SERVER Elements Variable Contains Example $_SERVER['PHP_SELF'] The current script. Suitable for use](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-57.jpg)
![Some Common $_SERVER Elements Variable Contains Example $_SERVER['QUERY_STR ING'] For GET requests, the name=matt&address=u Some Common $_SERVER Elements Variable Contains Example $_SERVER['QUERY_STR ING'] For GET requests, the name=matt&address=u](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-58.jpg)
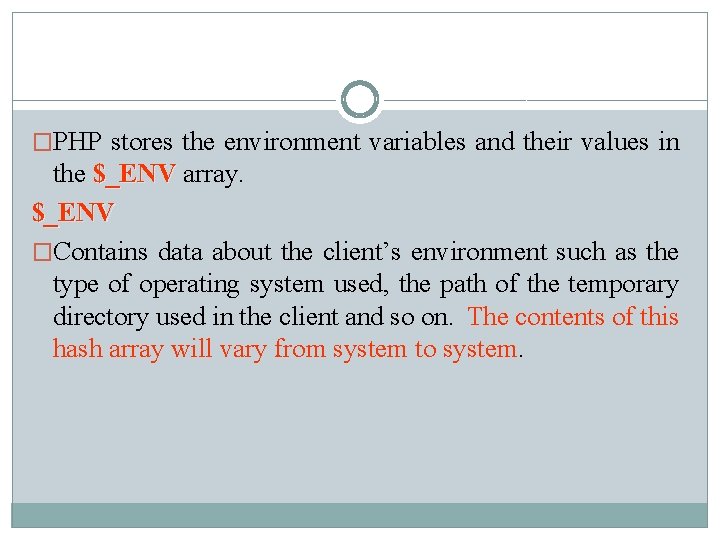
![Example: getting the OS used by the client $os_type = $_ENV[“OS"]; Example: getting the OS used by the client $os_type = $_ENV[“OS"];](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-60.jpg)
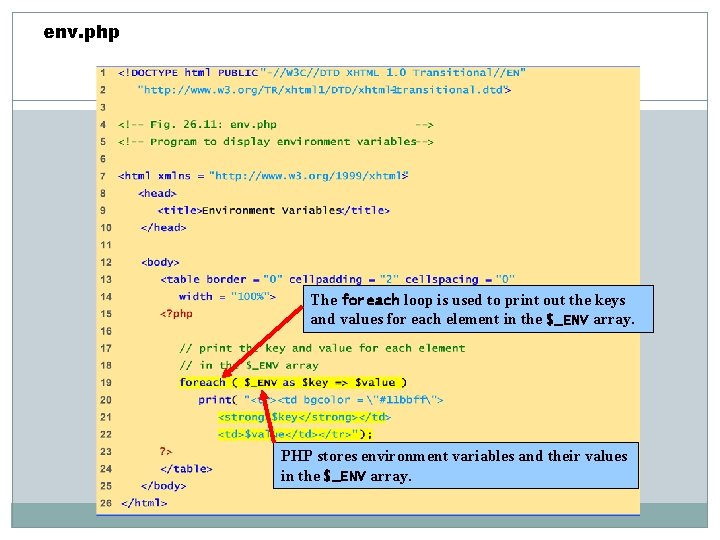
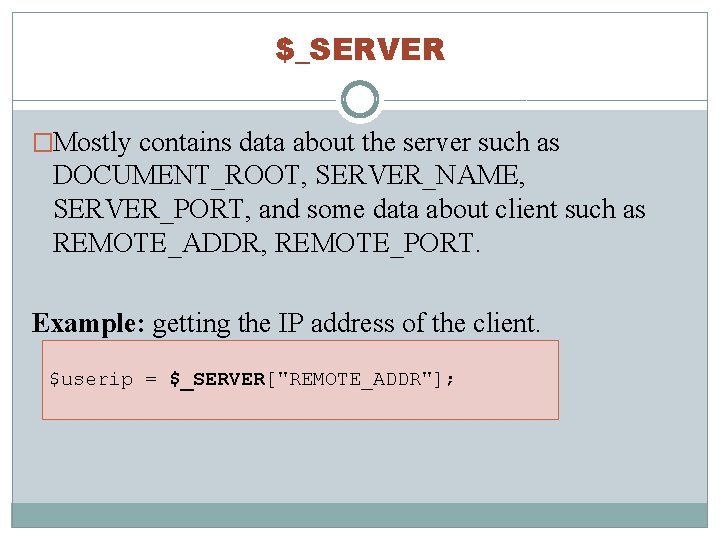
- Slides: 62
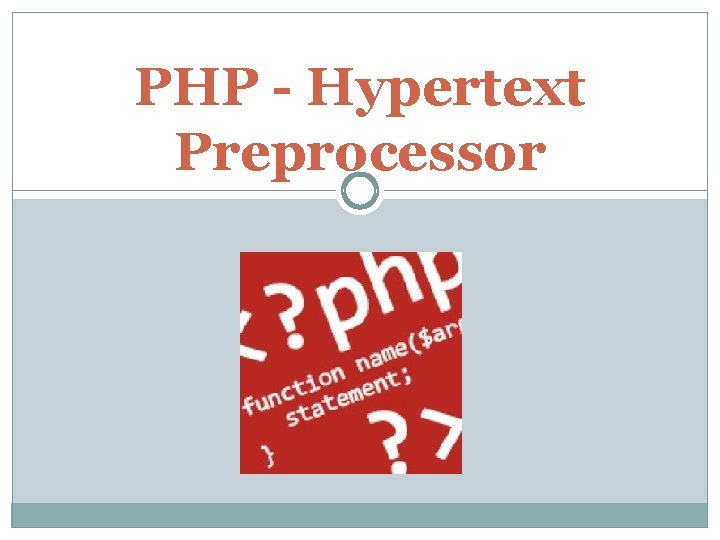
PHP - Hypertext Preprocessor
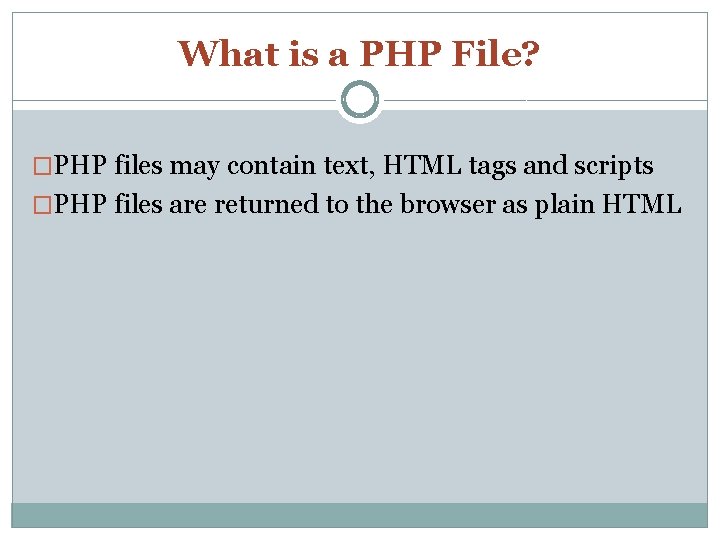
What is a PHP File? �PHP files may contain text, HTML tags and scripts �PHP files are returned to the browser as plain HTML
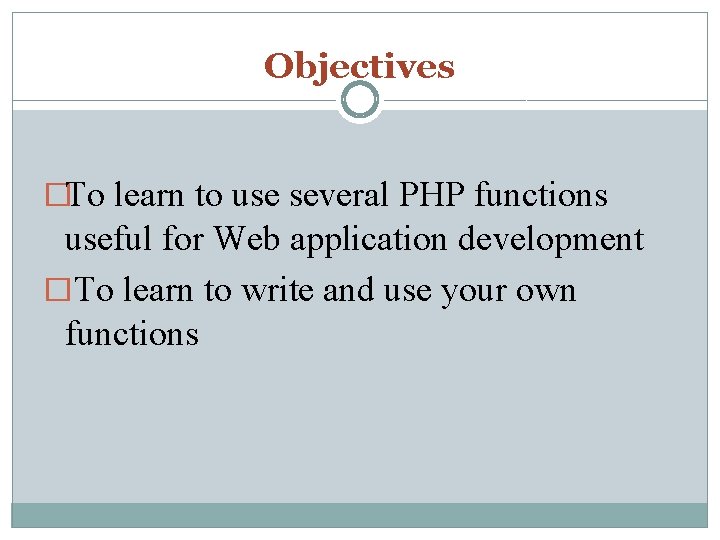
Objectives �To learn to use several PHP functions useful for Web application development �To learn to write and use your own functions
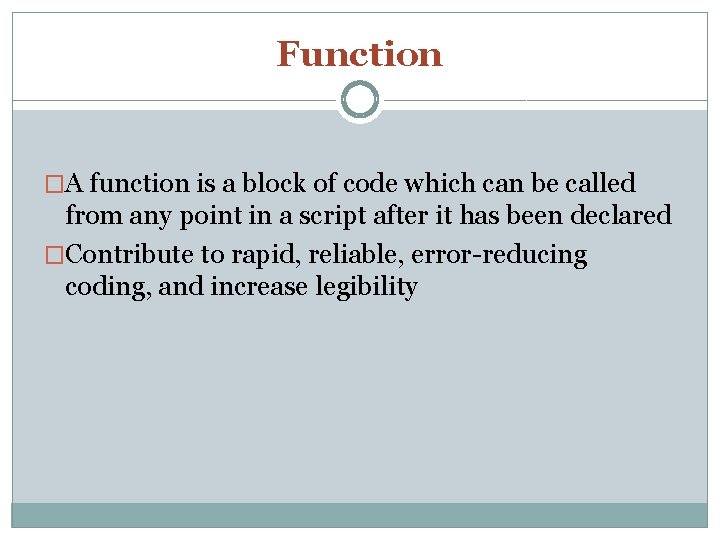
Function �A function is a block of code which can be called from any point in a script after it has been declared �Contribute to rapid, reliable, error-reducing coding, and increase legibility
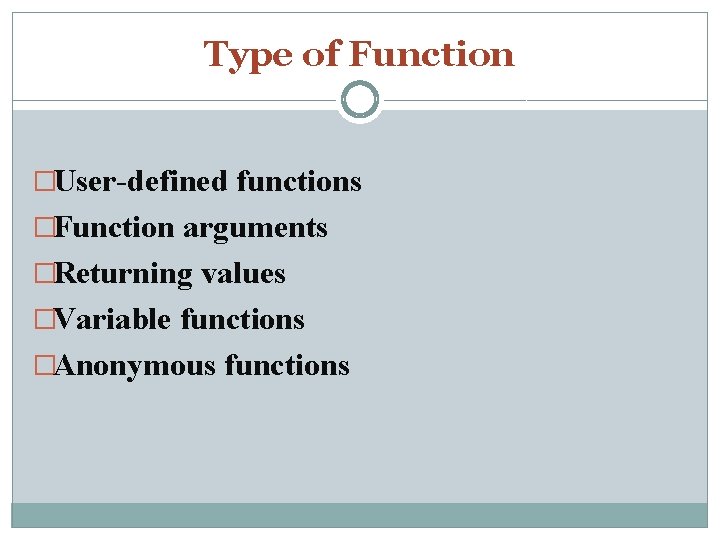
Type of Function �User-defined functions �Function arguments �Returning values �Variable functions �Anonymous functions
![Function Some basic numeric PHP functionsE g i absolute valueabs ii square root Function �Some basic numeric PHP functions—E. g. , i) absolute value[abs()], ii) square root](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-6.jpg)
Function �Some basic numeric PHP functions—E. g. , i) absolute value[abs()], ii) square root [sqrt()], iii) round [round()], iv) integer checker[is_numeric()], v) random number generation [rand()] functions
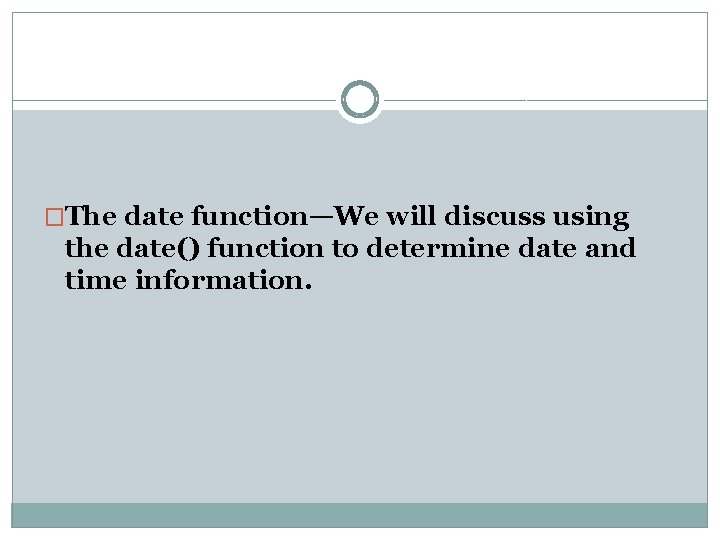
�The date function—We will discuss using the date() function to determine date and time information.
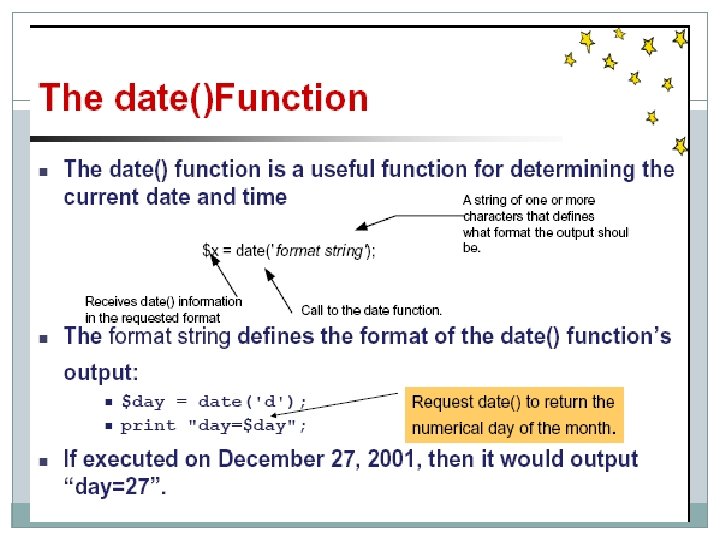
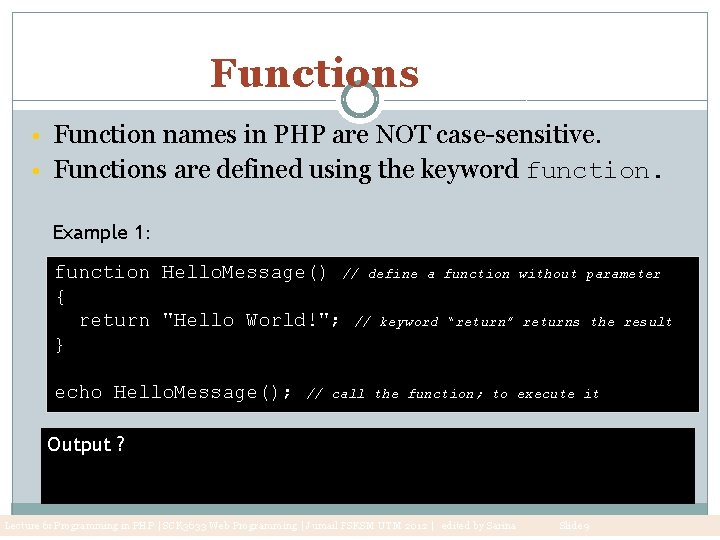
Functions • Function names in PHP are NOT case-sensitive. • Functions are defined using the keyword function. Example 1: function Hello. Message() // define a function without parameter { return "Hello World!"; // keyword “return” returns the result } echo Hello. Message(); // call the function; to execute it Output ? Lecture 6: Programming in PHP | SCK 3633 Web Programming | Jumail FSKSM UTM 2012 | edited by Sarina Slide 9
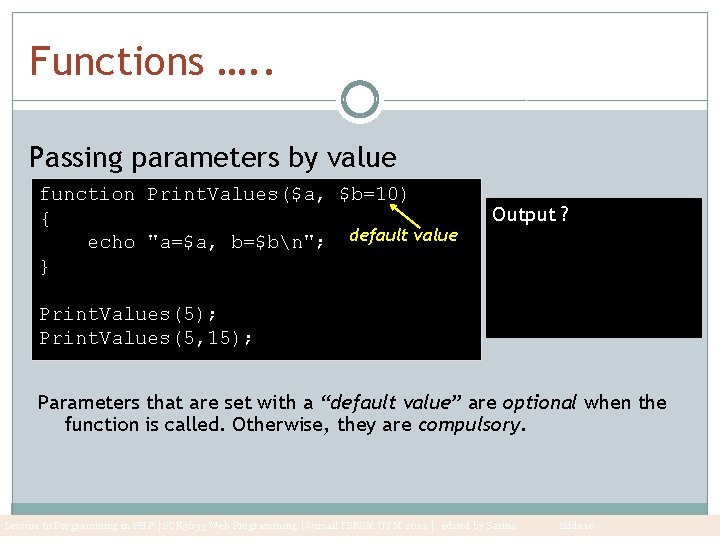
Functions …. . Passing parameters by value function Print. Values($a, $b=10) { echo "a=$a, b=$bn"; default value } Output ? Print. Values(5); Print. Values(5, 15); Parameters that are set with a “default value” are optional when the function is called. Otherwise, they are compulsory. Lecture 6: Programming in PHP | SCK 3633 Web Programming | Jumail FSKSM UTM 2012 | edited by Sarina Slide 10
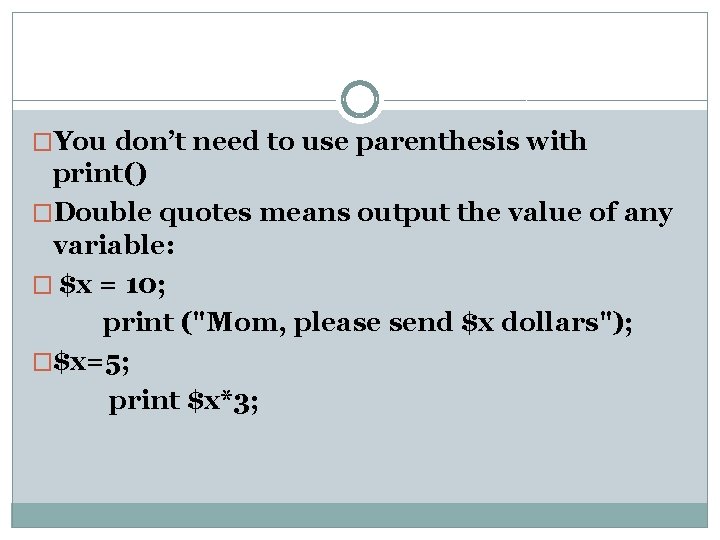
�You don’t need to use parenthesis with print() �Double quotes means output the value of any variable: � $x = 10; print ("Mom, please send $x dollars"); �$x=5; print $x*3;
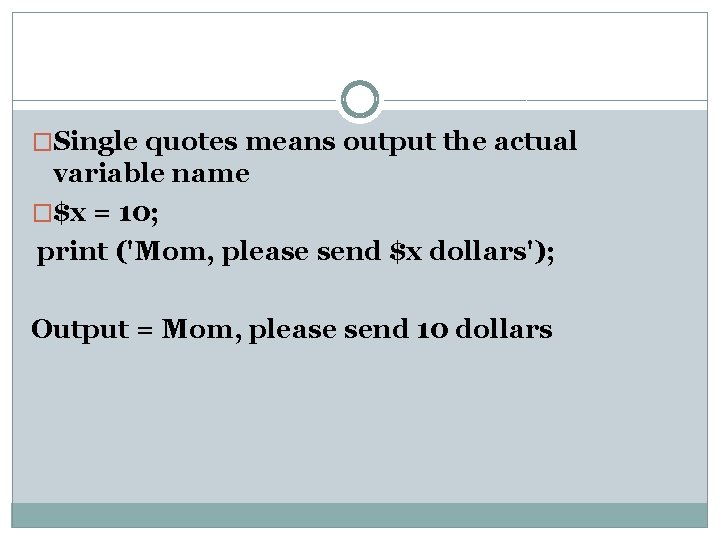
�Single quotes means output the actual variable name �$x = 10; print ('Mom, please send $x dollars'); Output = Mom, please send 10 dollars
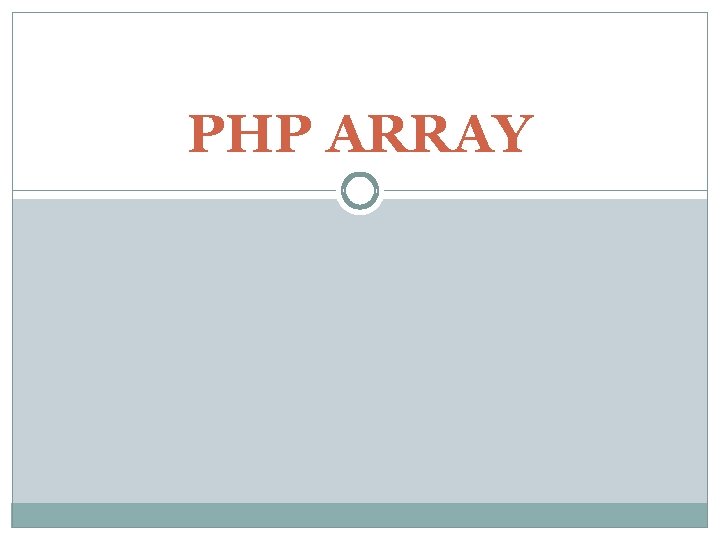
PHP ARRAY
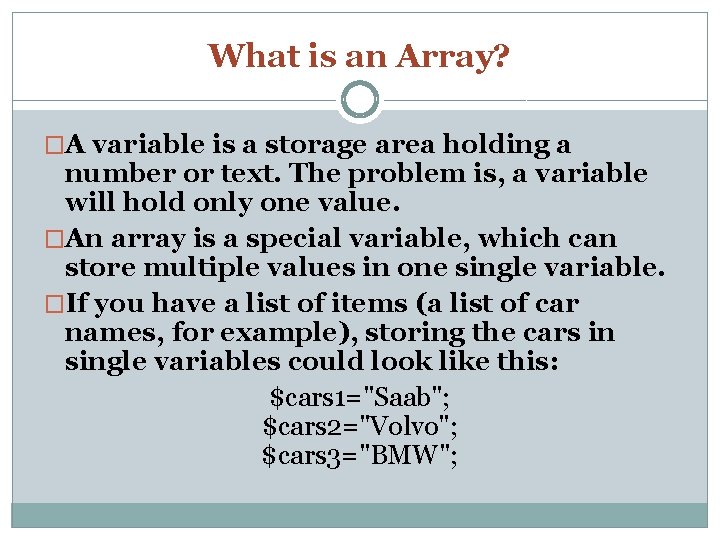
What is an Array? �A variable is a storage area holding a number or text. The problem is, a variable will hold only one value. �An array is a special variable, which can store multiple values in one single variable. �If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this: $cars 1="Saab"; $cars 2="Volvo"; $cars 3="BMW";
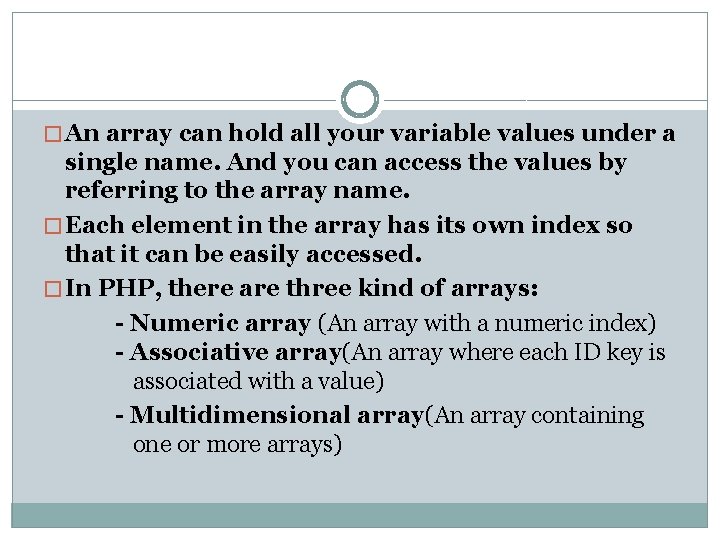
� An array can hold all your variable values under a single name. And you can access the values by referring to the array name. � Each element in the array has its own index so that it can be easily accessed. � In PHP, there are three kind of arrays: - Numeric array (An array with a numeric index) - Associative array(An array where each ID key is associated with a value) - Multidimensional array(An array containing one or more arrays)
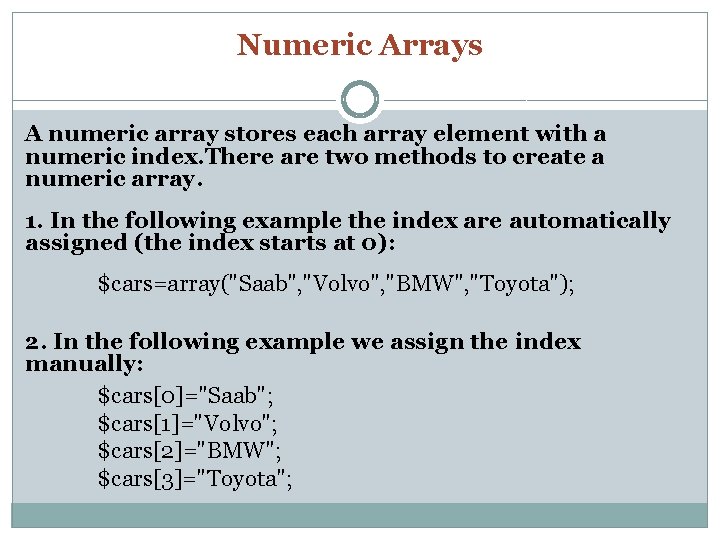
Numeric Arrays A numeric array stores each array element with a numeric index. There are two methods to create a numeric array. 1. In the following example the index are automatically assigned (the index starts at 0): $cars=array("Saab", "Volvo", "BMW", "Toyota"); 2. In the following example we assign the index manually: $cars[0]="Saab"; $cars[1]="Volvo"; $cars[2]="BMW"; $cars[3]="Toyota";
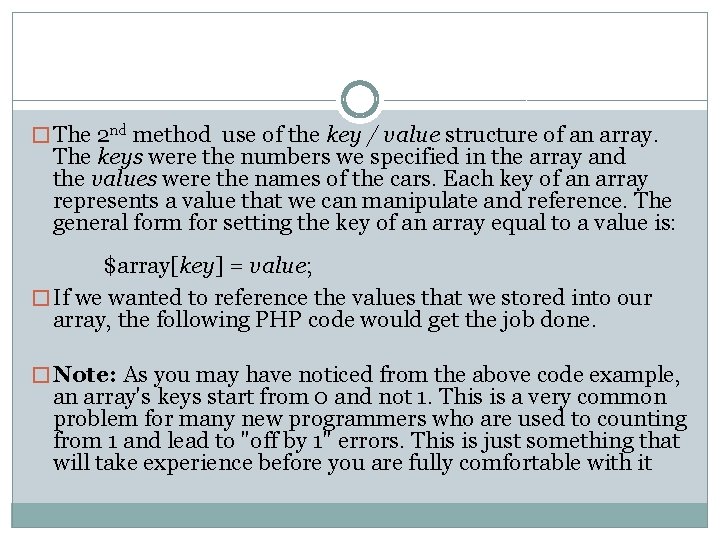
� The 2 nd method use of the key / value structure of an array. The keys were the numbers we specified in the array and the values were the names of the cars. Each key of an array represents a value that we can manipulate and reference. The general form for setting the key of an array equal to a value is: $array[key] = value; � If we wanted to reference the values that we stored into our array, the following PHP code would get the job done. � Note: As you may have noticed from the above code example, an array's keys start from 0 and not 1. This is a very common problem for many new programmers who are used to counting from 1 and lead to "off by 1" errors. This is just something that will take experience before you are fully comfortable with it
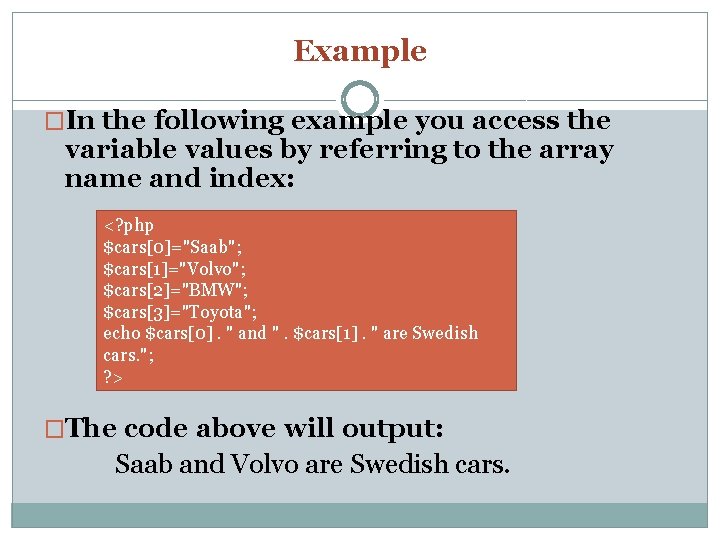
Example �In the following example you access the variable values by referring to the array name and index: <? php $cars[0]="Saab"; $cars[1]="Volvo"; $cars[2]="BMW"; $cars[3]="Toyota"; echo $cars[0]. " and ". $cars[1]. " are Swedish cars. "; ? > �The code above will output: Saab and Volvo are Swedish cars.
![PHP array Numeric Numeric Arrays names arrayPeter Quagmire Joe Assign manualy names0 PHP array (Numeric) Numeric Arrays $names = array("Peter", "Quagmire", "Joe"); Assign manualy: $names[0] =](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-19.jpg)
PHP array (Numeric) Numeric Arrays $names = array("Peter", "Quagmire", "Joe"); Assign manualy: $names[0] = "Peter"; $names[1] = "Quagmire"; $names[2] = "Joe";
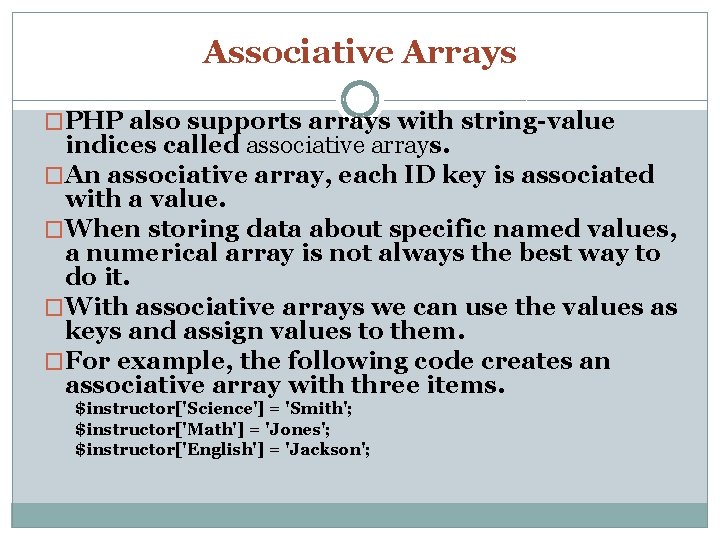
Associative Arrays �PHP also supports arrays with string-value indices called associative arrays. �An associative array, each ID key is associated with a value. �When storing data about specific named values, a numerical array is not always the best way to do it. �With associative arrays we can use the values as keys and assign values to them. �For example, the following code creates an associative array with three items. $instructor['Science'] = 'Smith'; $instructor['Math'] = 'Jones'; $instructor['English'] = 'Jackson';
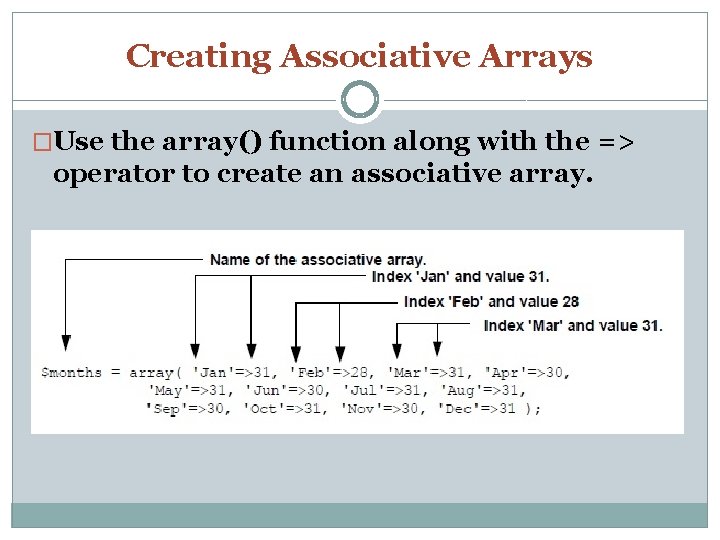
Creating Associative Arrays �Use the array() function along with the => operator to create an associative array.
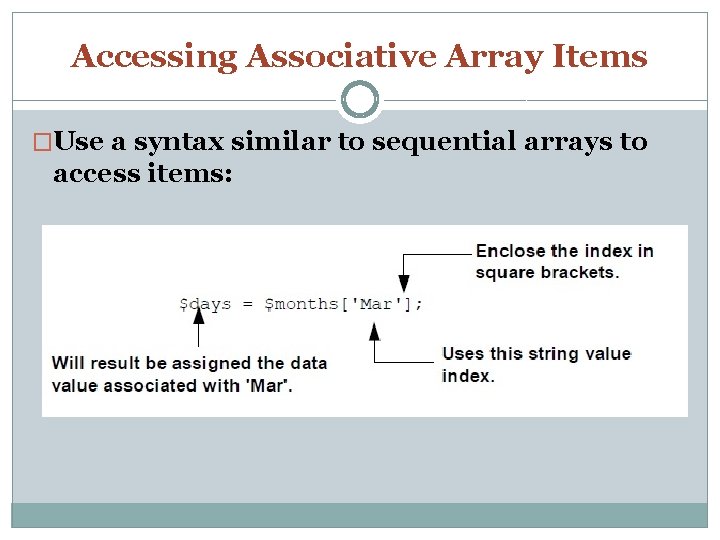
Accessing Associative Array Items �Use a syntax similar to sequential arrays to access items:
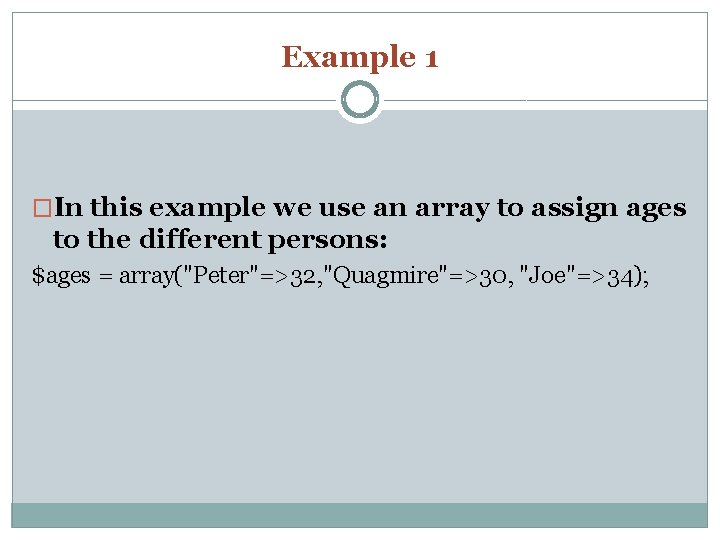
Example 1 �In this example we use an array to assign ages to the different persons: $ages = array("Peter"=>32, "Quagmire"=>30, "Joe"=>34);
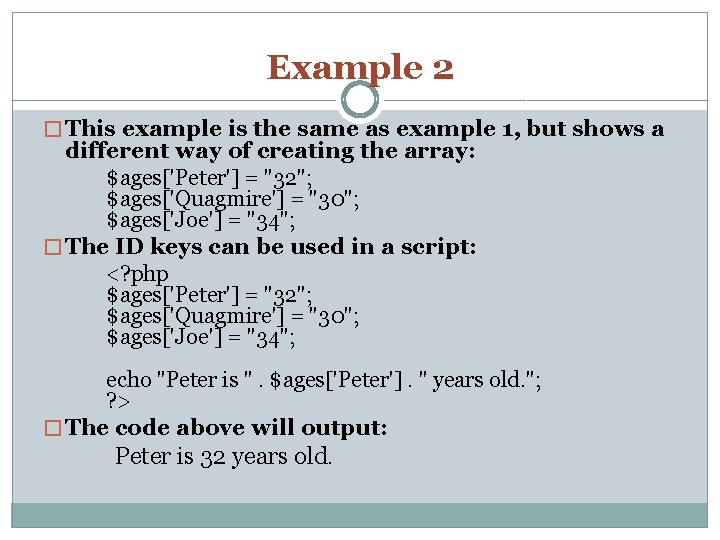
Example 2 � This example is the same as example 1, but shows a different way of creating the array: $ages['Peter'] = "32"; $ages['Quagmire'] = "30"; $ages['Joe'] = "34"; � The ID keys can be used in a script: <? php $ages['Peter'] = "32"; $ages['Quagmire'] = "30"; $ages['Joe'] = "34"; echo "Peter is ". $ages['Peter']. " years old. "; ? > � The code above will output: Peter is 32 years old.
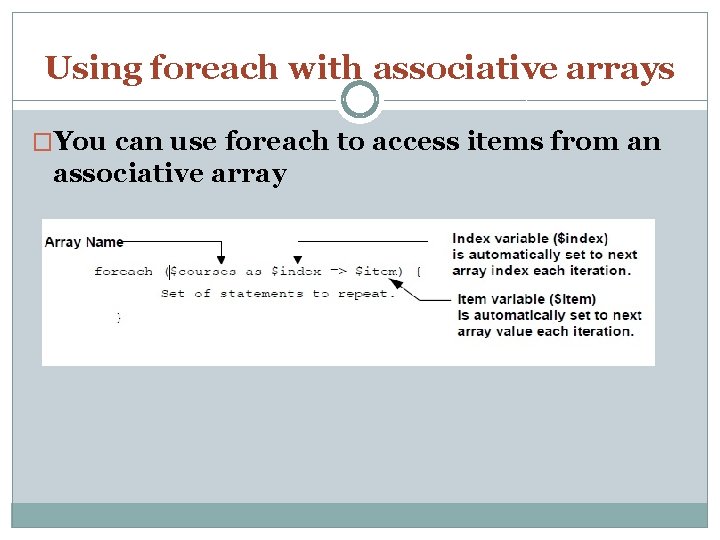
Using foreach with associative arrays �You can use foreach to access items from an associative array
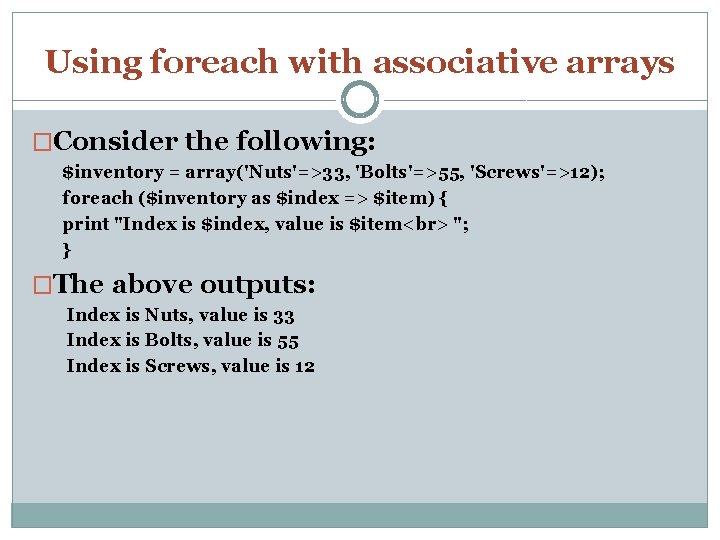
Using foreach with associative arrays �Consider the following: $inventory = array('Nuts'=>33, 'Bolts'=>55, 'Screws'=>12); foreach ($inventory as $index => $item) { print "Index is $index, value is $item "; } �The above outputs: Index is Nuts, value is 33 Index is Bolts, value is 55 Index is Screws, value is 12
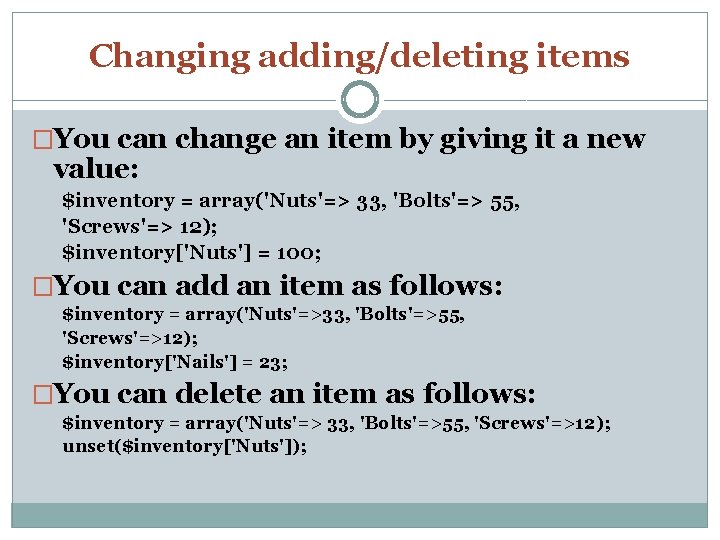
Changing adding/deleting items �You can change an item by giving it a new value: $inventory = array('Nuts'=> 33, 'Bolts'=> 55, 'Screws'=> 12); $inventory['Nuts'] = 100; �You can add an item as follows: $inventory = array('Nuts'=>33, 'Bolts'=>55, 'Screws'=>12); $inventory['Nails'] = 23; �You can delete an item as follows: $inventory = array('Nuts'=> 33, 'Bolts'=>55, 'Screws'=>12); unset($inventory['Nuts']);
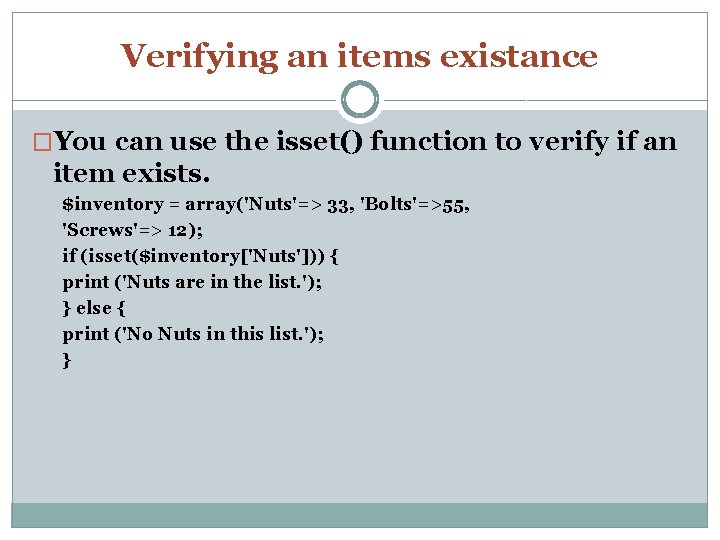
Verifying an items existance �You can use the isset() function to verify if an item exists. $inventory = array('Nuts'=> 33, 'Bolts'=>55, 'Screws'=> 12); if (isset($inventory['Nuts'])) { print ('Nuts are in the list. '); } else { print ('No Nuts in this list. '); }
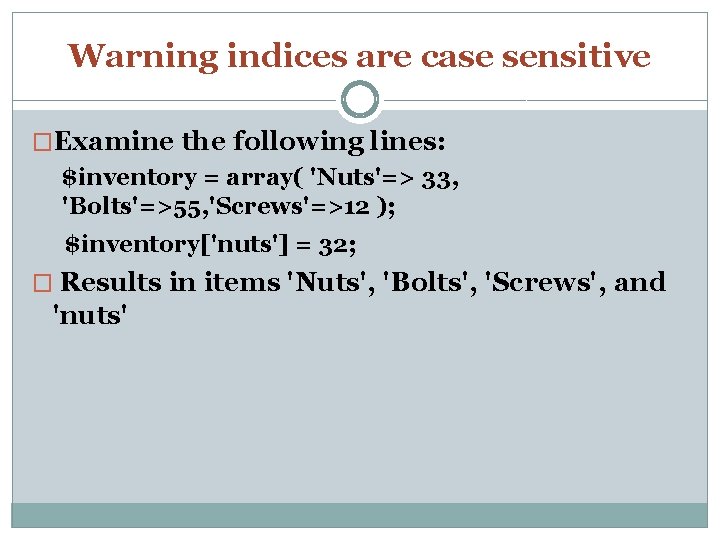
Warning indices are case sensitive �Examine the following lines: $inventory = array( 'Nuts'=> 33, 'Bolts'=>55, 'Screws'=>12 ); $inventory['nuts'] = 32; � Results in items 'Nuts', 'Bolts', 'Screws', and 'nuts'
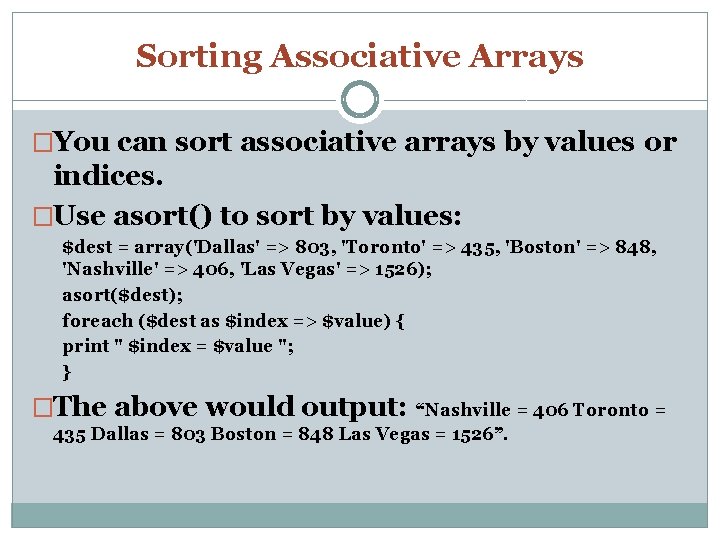
Sorting Associative Arrays �You can sort associative arrays by values or indices. �Use asort() to sort by values: $dest = array('Dallas' => 803, 'Toronto' => 435, 'Boston' => 848, 'Nashville' => 406, 'Las Vegas' => 1526); asort($dest); foreach ($dest as $index => $value) { print " $index = $value "; } �The above would output: “Nashville = 406 Toronto = 435 Dallas = 803 Boston = 848 Las Vegas = 1526”.
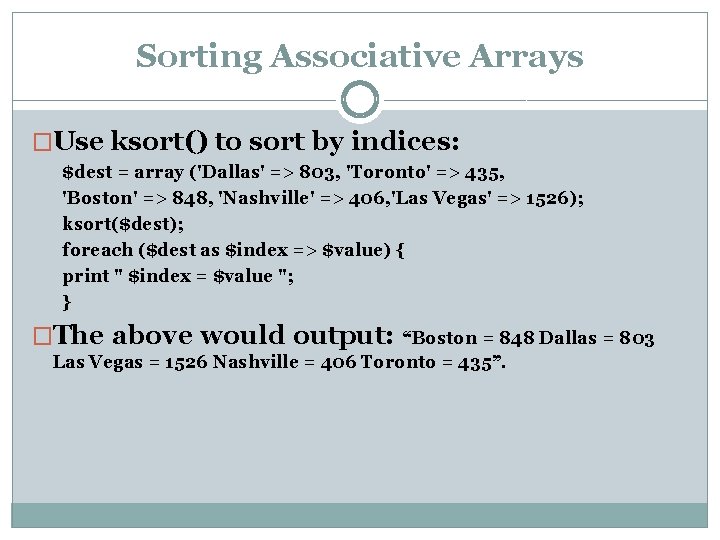
Sorting Associative Arrays �Use ksort() to sort by indices: $dest = array ('Dallas' => 803, 'Toronto' => 435, 'Boston' => 848, 'Nashville' => 406, 'Las Vegas' => 1526); ksort($dest); foreach ($dest as $index => $value) { print " $index = $value "; } �The above would output: “Boston = 848 Dallas = 803 Las Vegas = 1526 Nashville = 406 Toronto = 435”.
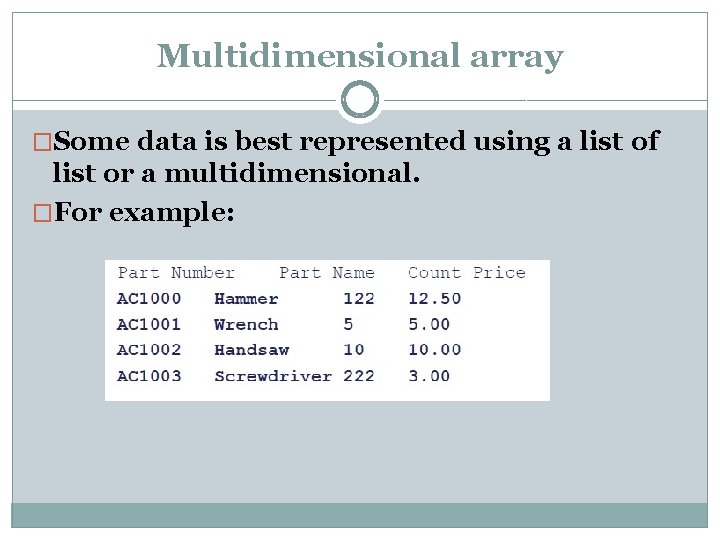
Multidimensional array �Some data is best represented using a list of list or a multidimensional. �For example:
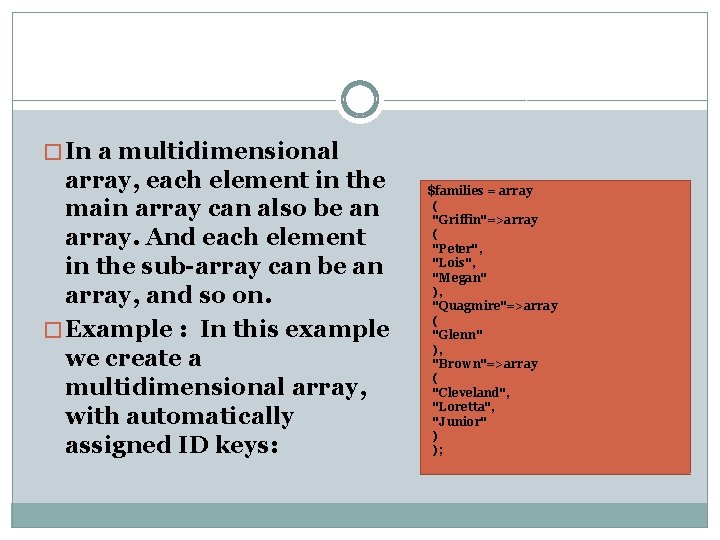
� In a multidimensional array, each element in the main array can also be an array. And each element in the sub-array can be an array, and so on. � Example : In this example we create a multidimensional array, with automatically assigned ID keys: $families = array ( "Griffin"=>array ( "Peter", "Lois", "Megan" ), "Quagmire"=>array ( "Glenn" ), "Brown"=>array ( "Cleveland", "Loretta", "Junior" ) );
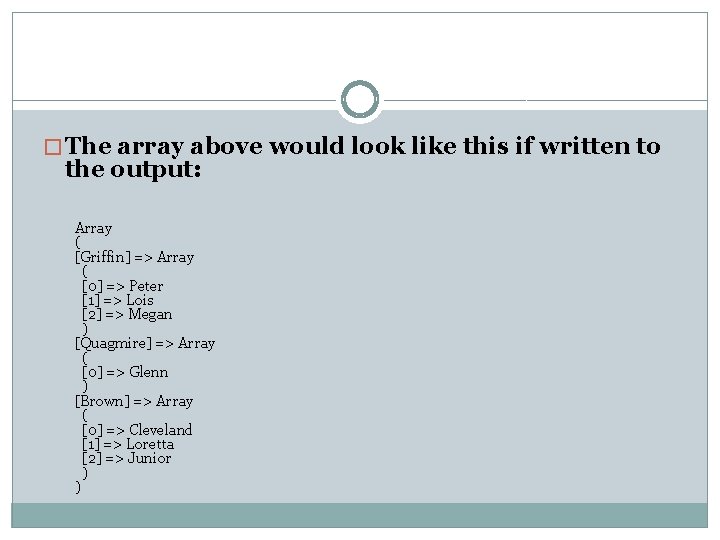
� The array above would look like this if written to the output: Array ( [Griffin] => Array ( [0] => Peter [1] => Lois [2] => Megan ) [Quagmire] => Array ( [0] => Glenn ) [Brown] => Array ( [0] => Cleveland [1] => Loretta [2] => Junior ) )
![Creating Multidimensional Lists You can create multidimensional arrays with the array function inventoryAC 1000Part Creating Multidimensional Lists �You can create multidimensional arrays with the array() function $inventory['AC 1000']['Part']](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-35.jpg)
Creating Multidimensional Lists �You can create multidimensional arrays with the array() function $inventory['AC 1000']['Part'] has the value Hammer, $inventory['AC 1001']['Count'] has the value 5, and $inventory['AC 1002']['Price'] has the value 10. 00
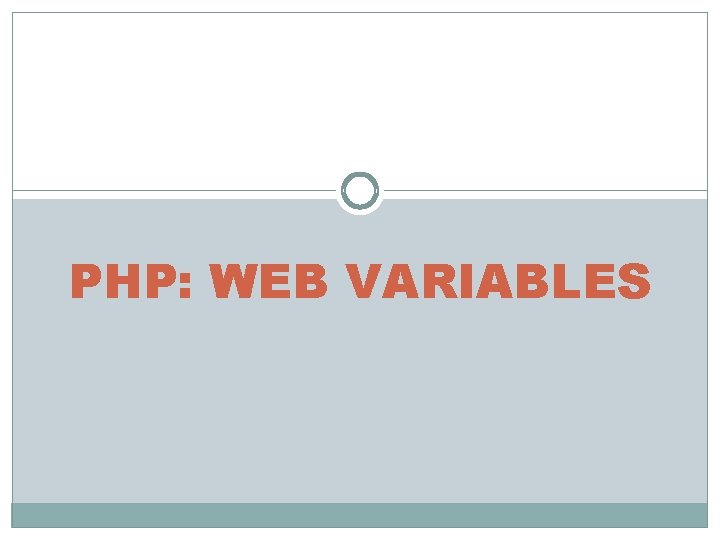
PHP: WEB VARIABLES
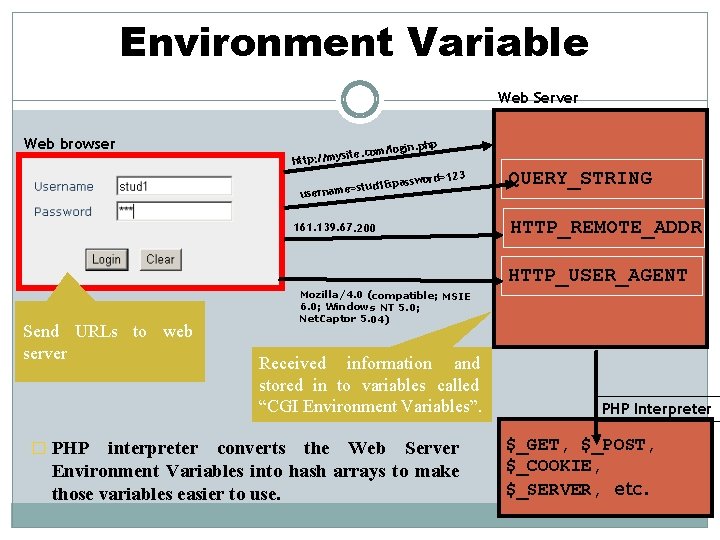
Environment Variable Web Server Web browser . php in e. com/log sit http: //my tud 1&pa ername=s us 3 2 ssword=1 161. 139. 67. 200 QUERY_STRING HTTP_REMOTE_ADDR HTTP_USER_AGENT Send URLs to web server � PHP Mozilla/4. 0 (compatible; MSIE 6. 0; Windows NT 5. 0; Net. Captor 5. 04) Received information and stored in to variables called “CGI Environment Variables”. interpreter converts the Web Server Environment Variables into hash arrays to make those variables easier to use. PHP Interpreter $_GET, $_POST, $_COOKIE, $_SERVER, etc.
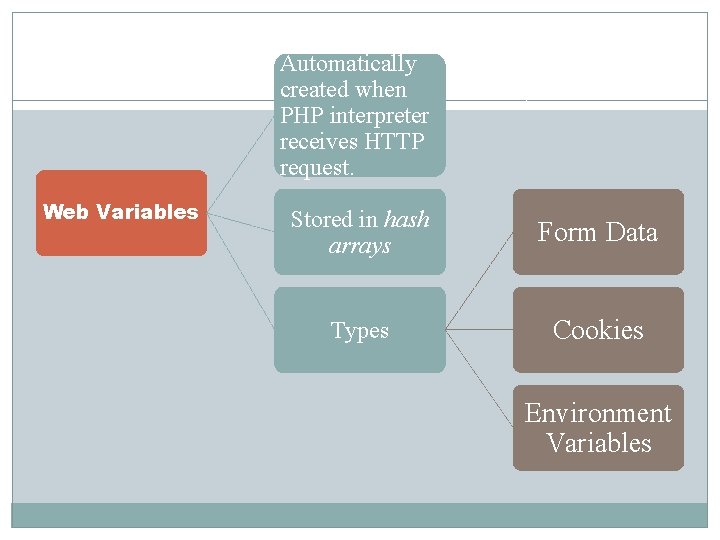
Automatically created when PHP interpreter receives HTTP request. Web Variables Stored in hash arrays Form Data Types Cookies Environment Variables
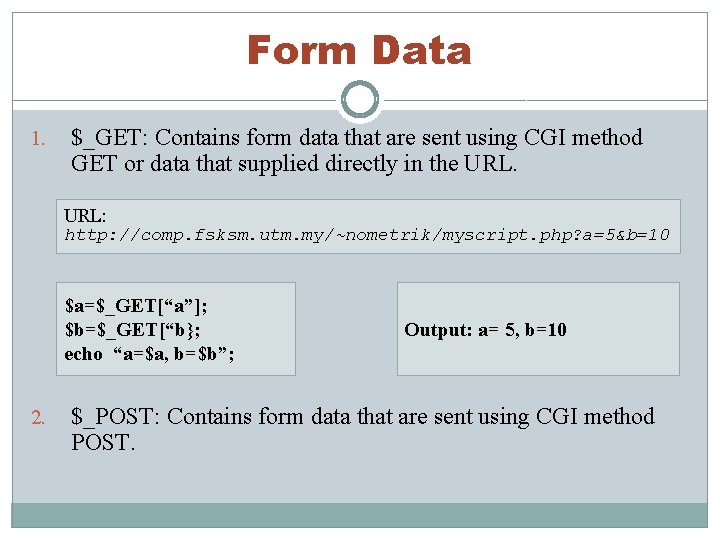
Form Data 1. $_GET: Contains form data that are sent using CGI method GET or data that supplied directly in the URL: http: //comp. fsksm. utm. my/~nometrik/myscript. php? a=5&b=10 $a=$_GET[“a”]; $b=$_GET[“b}; echo “a=$a, b=$b”; 2. Output: a= 5, b=10 $_POST: Contains form data that are sent using CGI method POST.
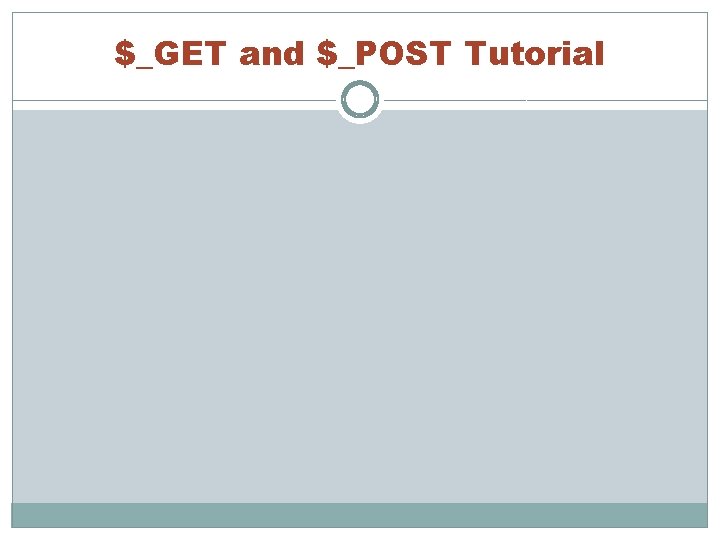
$_GET and $_POST Tutorial
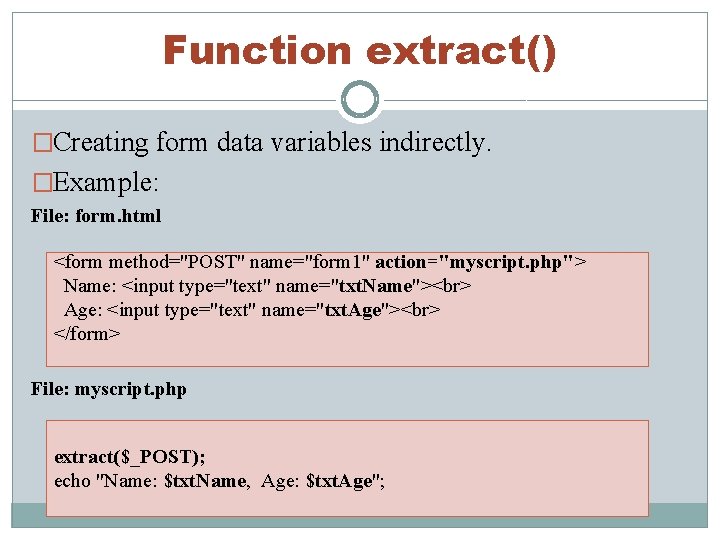
Function extract() �Creating form data variables indirectly. �Example: File: form. html <form method="POST" name="form 1" action="myscript. php"> Name: <input type="text" name="txt. Name"> Age: <input type="text" name="txt. Age"> </form> File: myscript. php extract($_POST); echo "Name: $txt. Name, Age: $txt. Age";
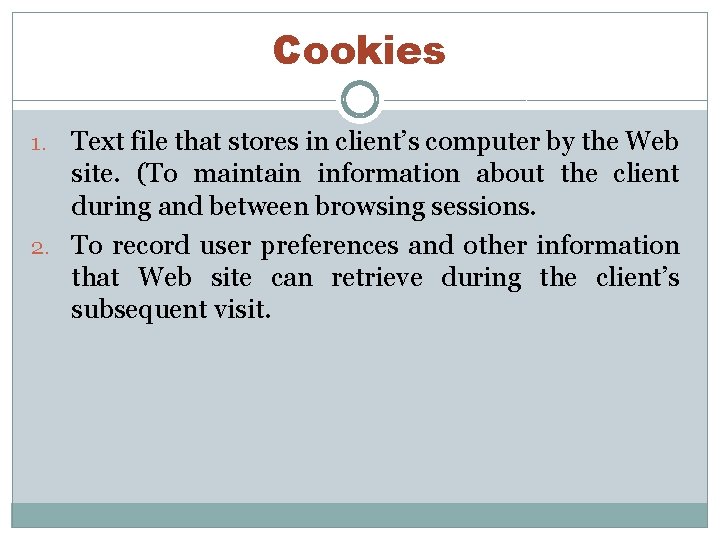
Cookies Text file that stores in client’s computer by the Web site. (To maintain information about the client during and between browsing sessions. 2. To record user preferences and other information that Web site can retrieve during the client’s subsequent visit. 1.
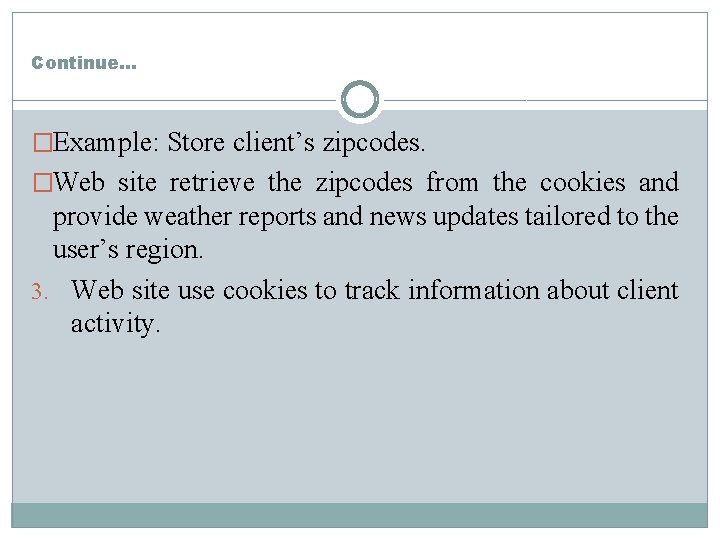
Continue… �Example: Store client’s zipcodes. �Web site retrieve the zipcodes from the cookies and provide weather reports and news updates tailored to the user’s region. 3. Web site use cookies to track information about client activity.
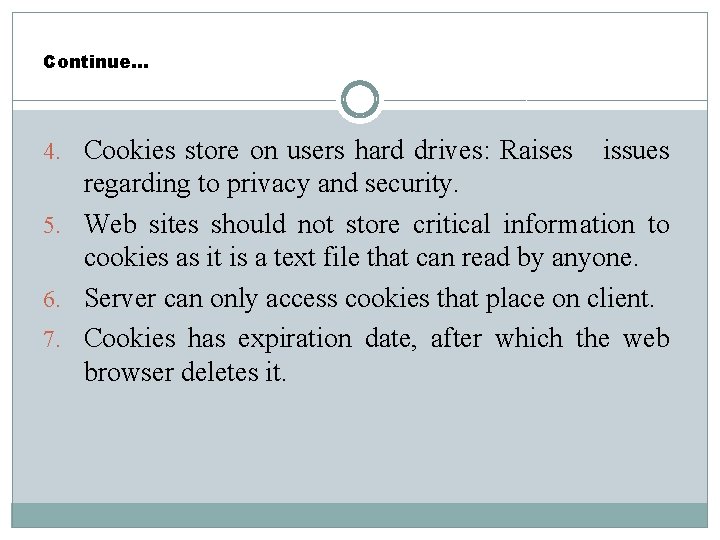
Continue… 4. Cookies store on users hard drives: Raises issues regarding to privacy and security. 5. Web sites should not store critical information to cookies as it is a text file that can read by anyone. 6. Server can only access cookies that place on client. 7. Cookies has expiration date, after which the web browser deletes it.
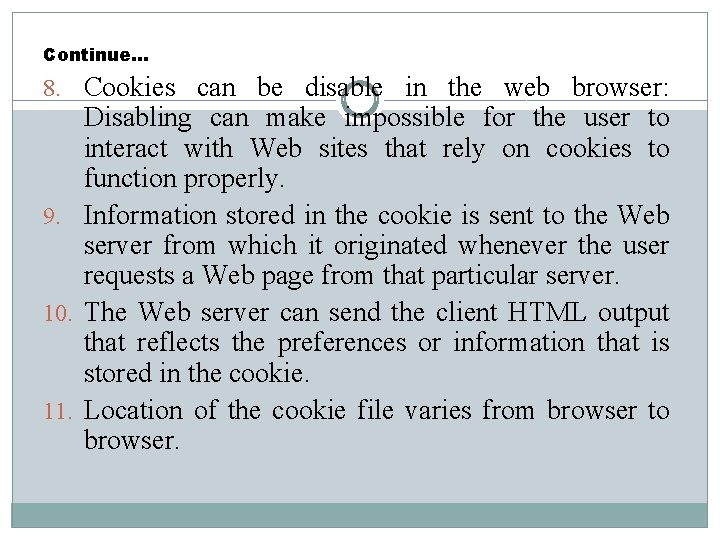
Continue… 8. Cookies can be disable in the web browser: Disabling can make impossible for the user to interact with Web sites that rely on cookies to function properly. 9. Information stored in the cookie is sent to the Web server from which it originated whenever the user requests a Web page from that particular server. 10. The Web server can send the client HTML output that reflects the preferences or information that is stored in the cookie. 11. Location of the cookie file varies from browser to browser.
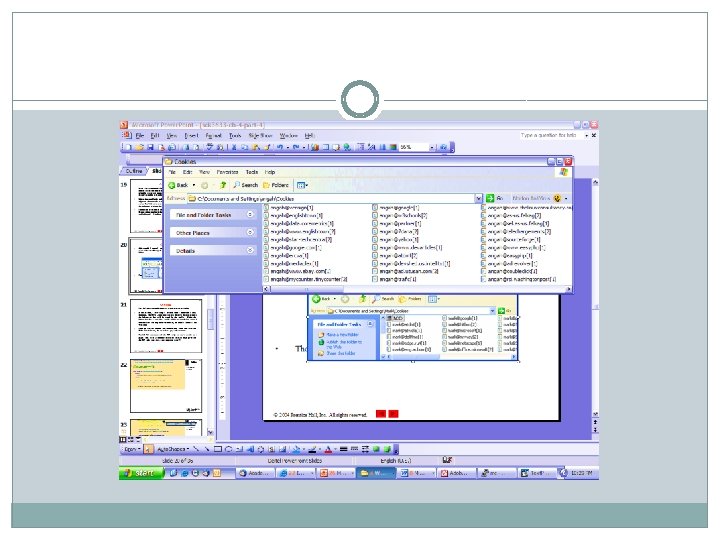
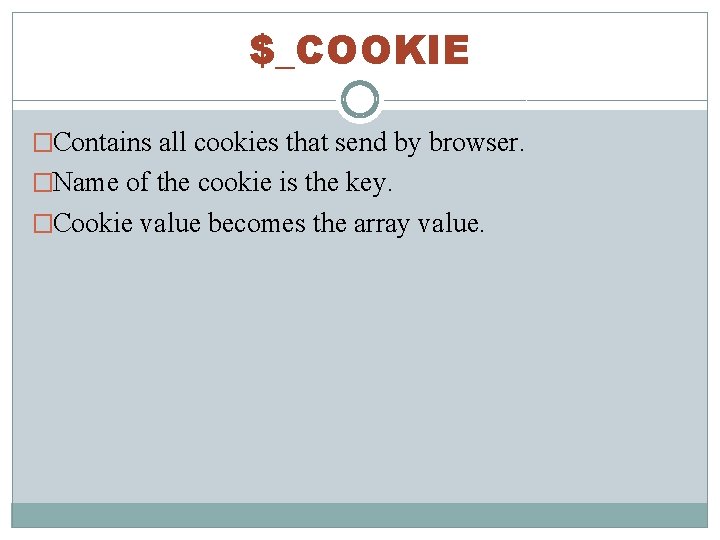
$_COOKIE �Contains all cookies that send by browser. �Name of the cookie is the key. �Cookie value becomes the array value.
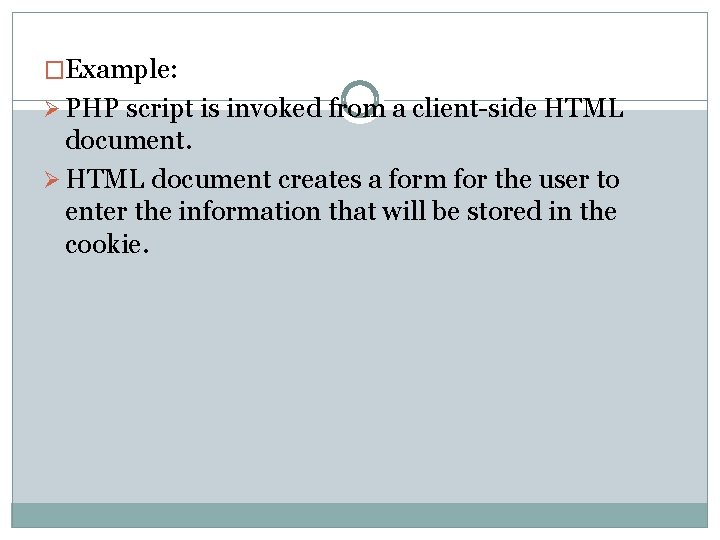
�Example: Ø PHP script is invoked from a client-side HTML document. Ø HTML document creates a form for the user to enter the information that will be stored in the cookie.
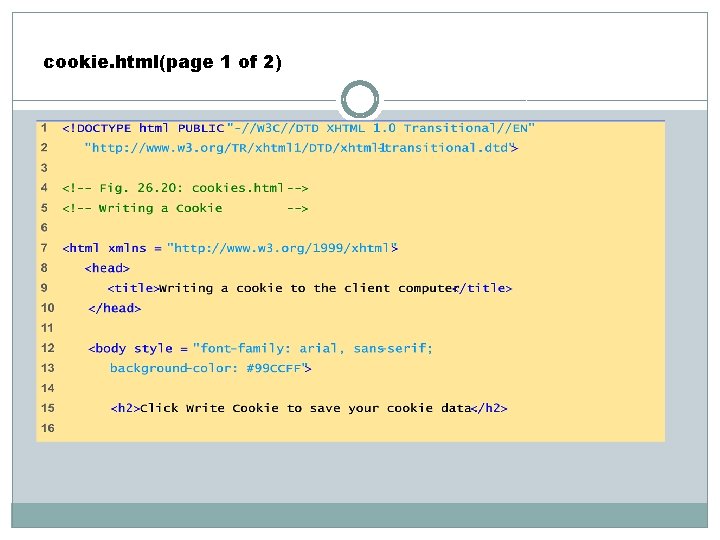
cookie. html(page 1 of 2)
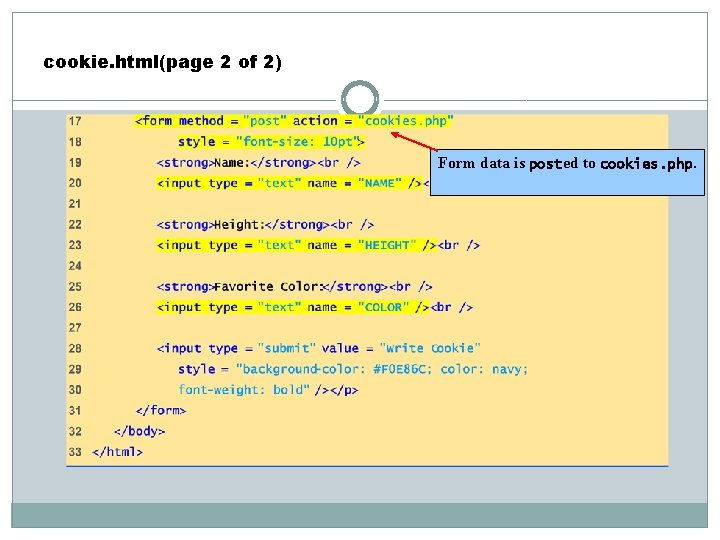
cookie. html(page 2 of 2) Form data is posted to cookies. php.
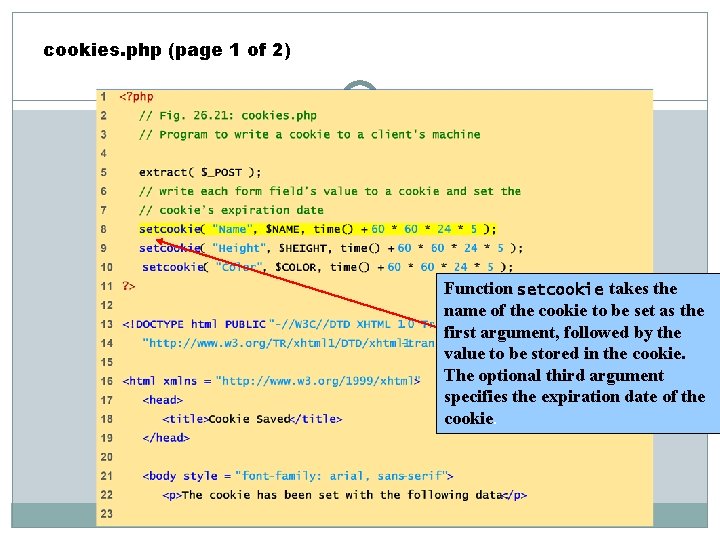
cookies. php (page 1 of 2) Function setcookie takes the name of the cookie to be set as the first argument, followed by the value to be stored in the cookie. The optional third argument specifies the expiration date of the cookie.
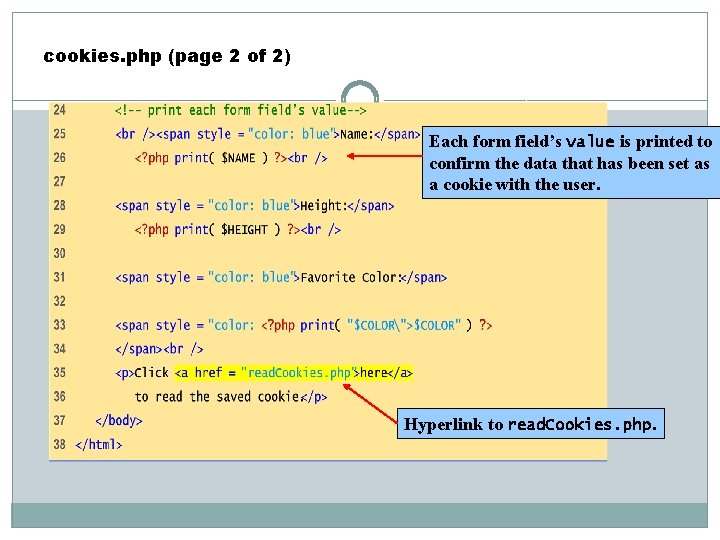
cookies. php (page 2 of 2) Each form field’s value is printed to confirm the data that has been set as a cookie with the user. Hyperlink to read. Cookies. php.
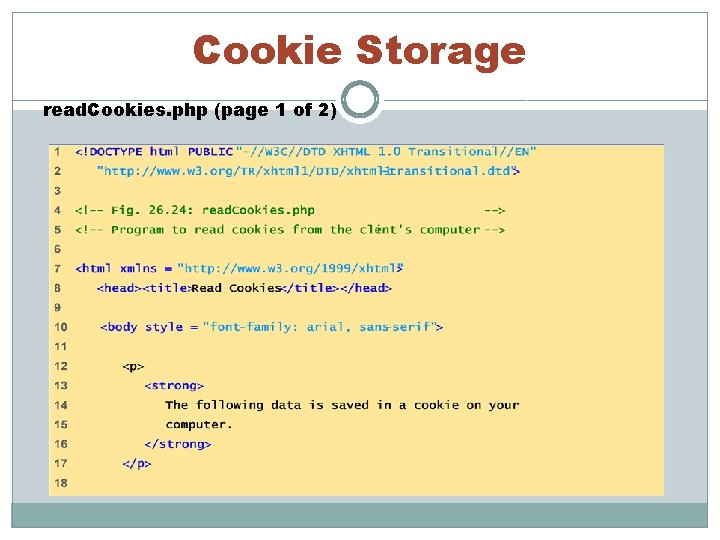
Cookie Storage read. Cookies. php (page 1 of 2)
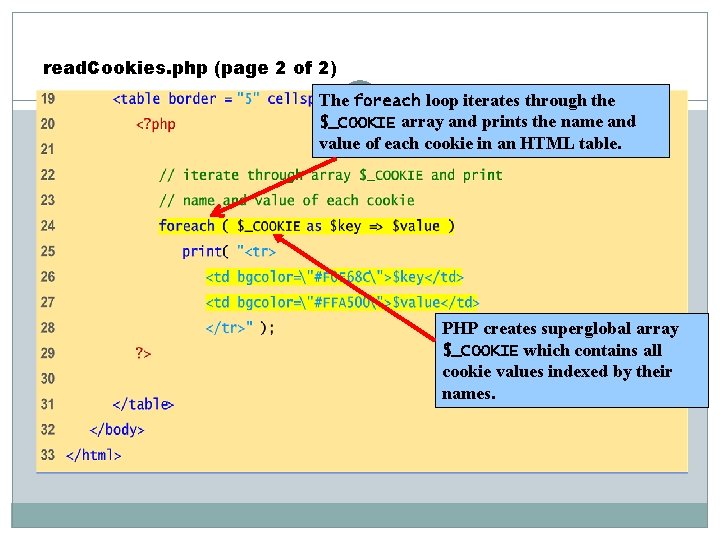
read. Cookies. php (page 2 of 2) The foreach loop iterates through the $_COOKIE array and prints the name and value of each cookie in an HTML table. PHP creates superglobal array $_COOKIE which contains all cookie values indexed by their names.
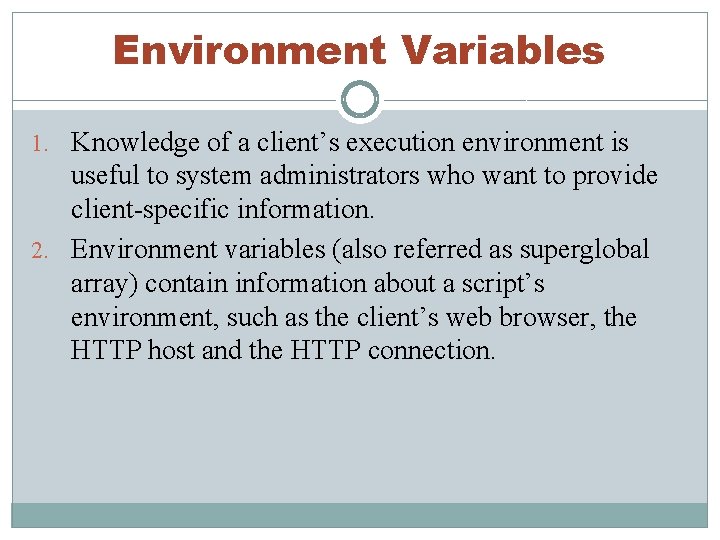
Environment Variables 1. Knowledge of a client’s execution environment is useful to system administrators who want to provide client-specific information. 2. Environment variables (also referred as superglobal array) contain information about a script’s environment, such as the client’s web browser, the HTTP host and the HTTP connection.
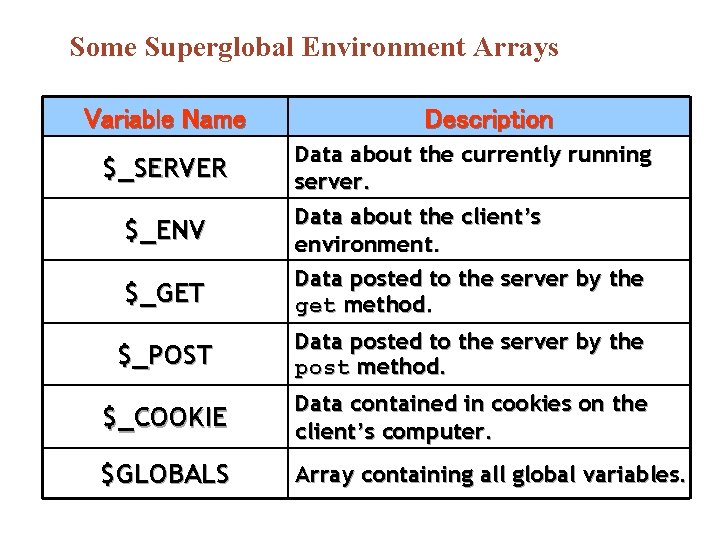
Some Superglobal Environment Arrays Variable Name Description $_GET Data about the currently running server. Data about the client’s environment. Data posted to the server by the get method. $_POST Data posted to the server by the post method. $_COOKIE Data contained in cookies on the client’s computer. $GLOBALS Array containing all global variables. $_SERVER $_ENV
![Some Common SERVER Elements Variable Contains Example SERVERPHPSELF The current script Suitable for use Some Common $_SERVER Elements Variable Contains Example $_SERVER['PHP_SELF'] The current script. Suitable for use](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-57.jpg)
Some Common $_SERVER Elements Variable Contains Example $_SERVER['PHP_SELF'] The current script. Suitable for use in links and form element action arguments. $_SERVER['HTTP_USER_ AGENT'] The name and version Mozilla/4. 6 –(X 11; I; Linux 2. 2. 6 of the client. /phpbook/source/list ing 10. 1. php 15 apmac ppc) $_SERVER['REMOTE_ADD R'] The IP address of the client. 158. 152. 55. 35 $_SERVER['REQUEST_ME THOD'] Whether the request was GET or POST
![Some Common SERVER Elements Variable Contains Example SERVERQUERYSTR ING For GET requests the namemattaddressu Some Common $_SERVER Elements Variable Contains Example $_SERVER['QUERY_STR ING'] For GET requests, the name=matt&address=u](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-58.jpg)
Some Common $_SERVER Elements Variable Contains Example $_SERVER['QUERY_STR ING'] For GET requests, the name=matt&address=u nknown encoded data sent appended to the URL. $_SERVER['REQUEST_U RI'] /phpbook/source/lis The full address of ting 10. 1. php? the request, name=matt including query string. $_SERVER['HTTP_REFE RER'] The address of the page from which the request was made. http: //p 24. corrosiv e. co. uk/ref. html
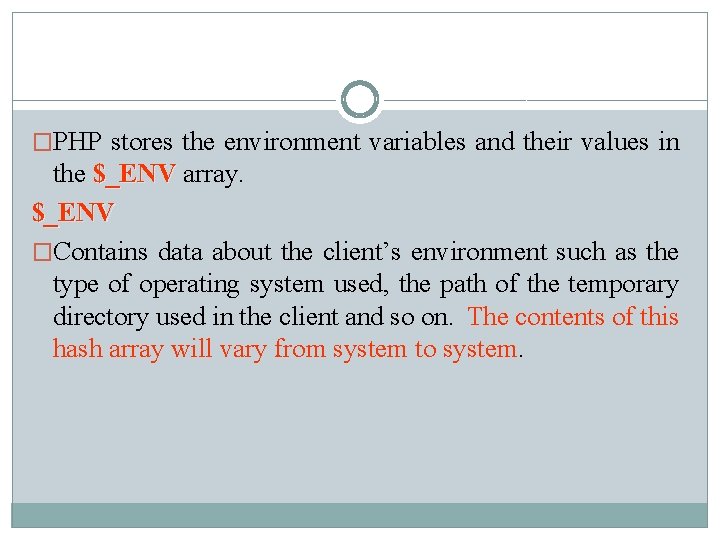
�PHP stores the environment variables and their values in the $_ENV array. $_ENV �Contains data about the client’s environment such as the type of operating system used, the path of the temporary directory used in the client and so on. The contents of this hash array will vary from system to system.
![Example getting the OS used by the client ostype ENVOS Example: getting the OS used by the client $os_type = $_ENV[“OS"];](https://slidetodoc.com/presentation_image_h2/3512b41d12829f10923f1b7462cfb99d/image-60.jpg)
Example: getting the OS used by the client $os_type = $_ENV[“OS"];
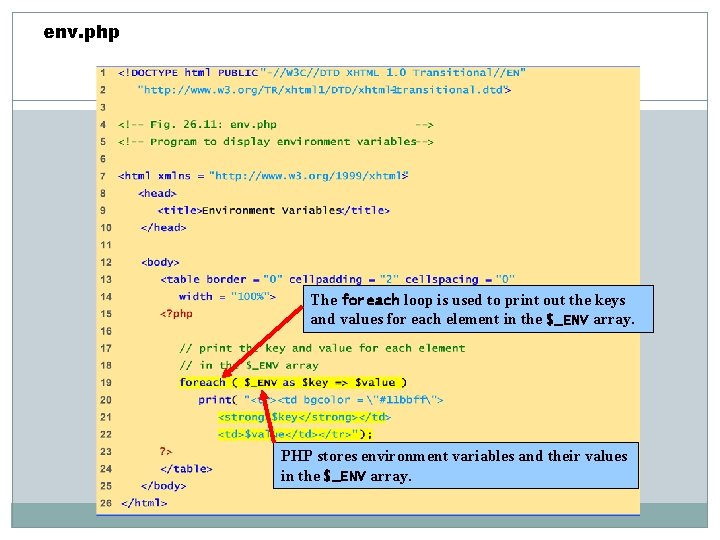
env. php The foreach loop is used to print out the keys and values for each element in the $_ENV array. PHP stores environment variables and their values in the $_ENV array.
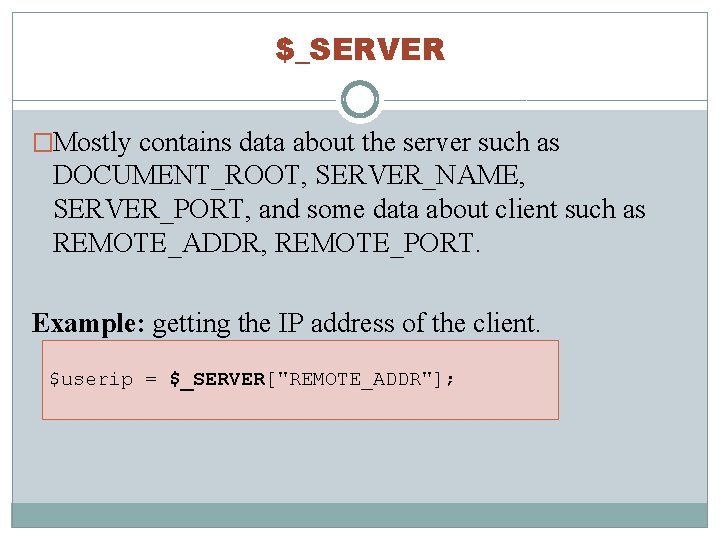
$_SERVER �Mostly contains data about the server such as DOCUMENT_ROOT, SERVER_NAME, SERVER_PORT, and some data about client such as REMOTE_ADDR, REMOTE_PORT. Example: getting the IP address of the client. $userip = $_SERVER["REMOTE_ADDR"];