Perl Introduction Outline What is Perl n What
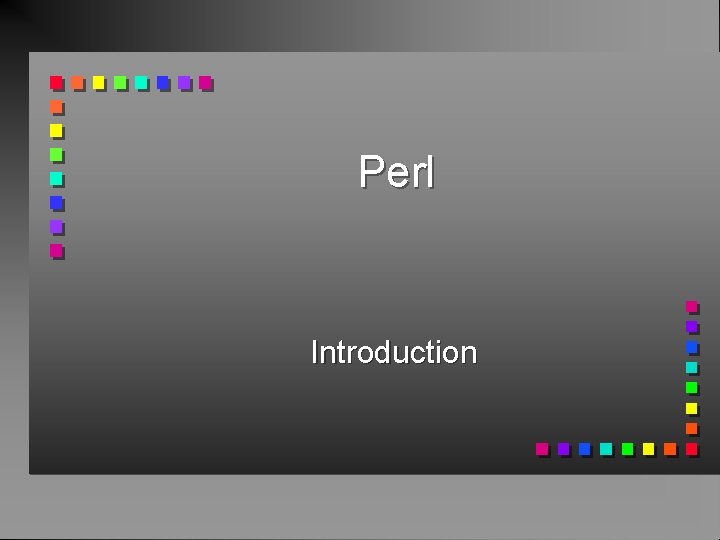
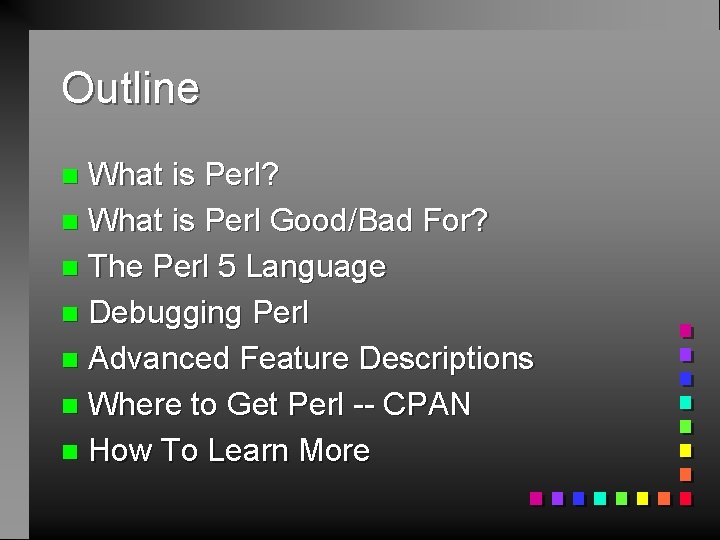
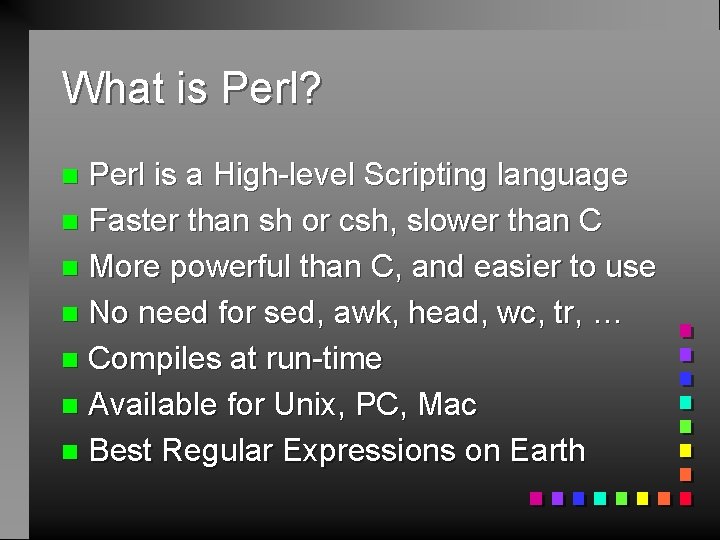
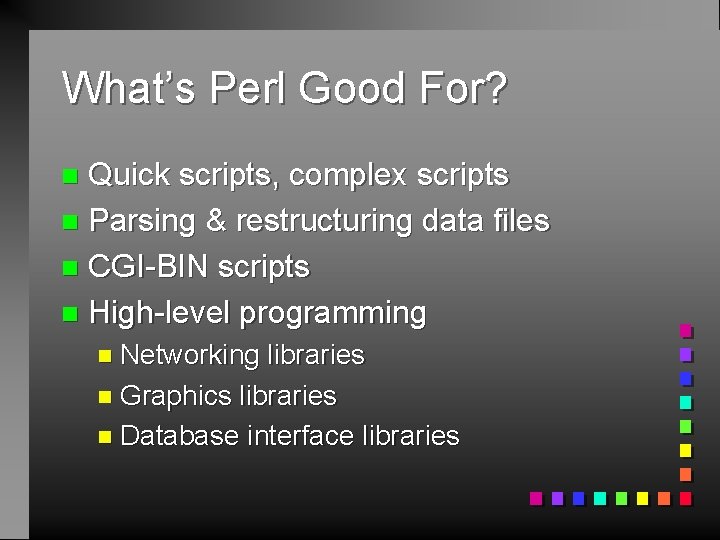
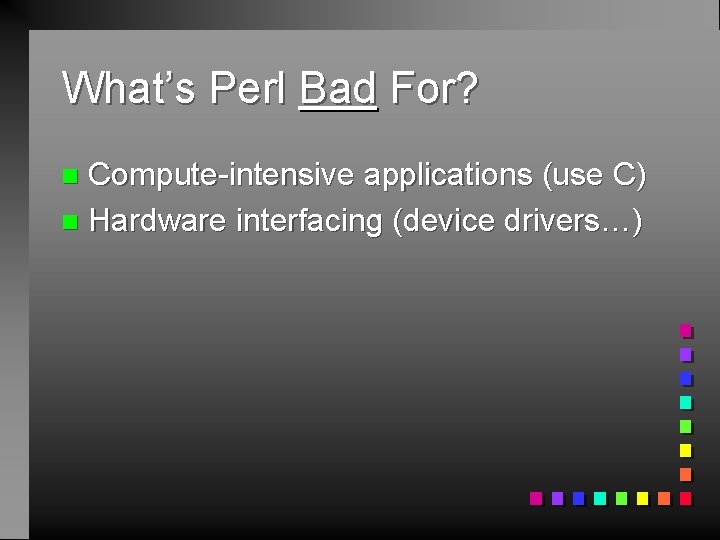
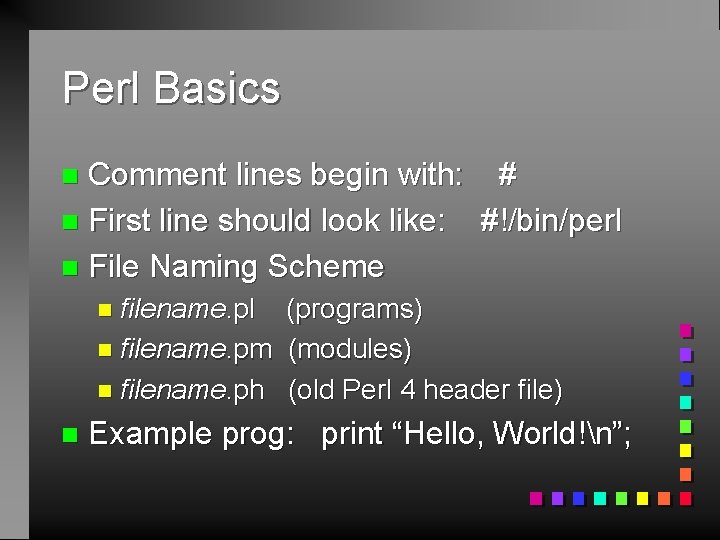
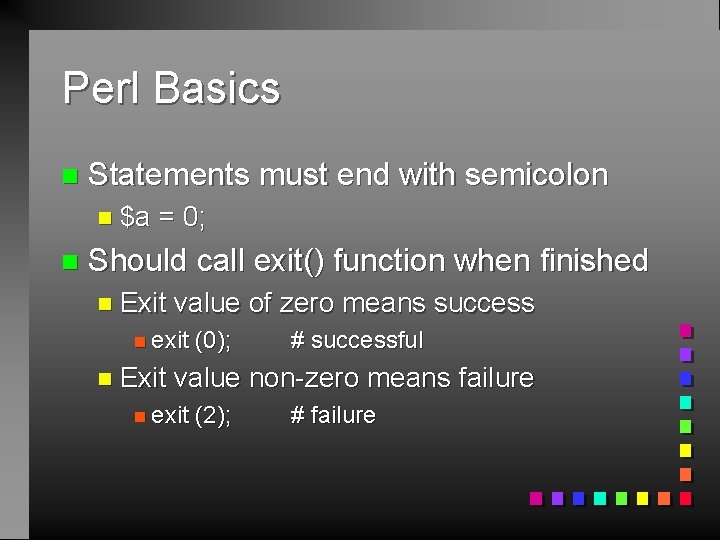
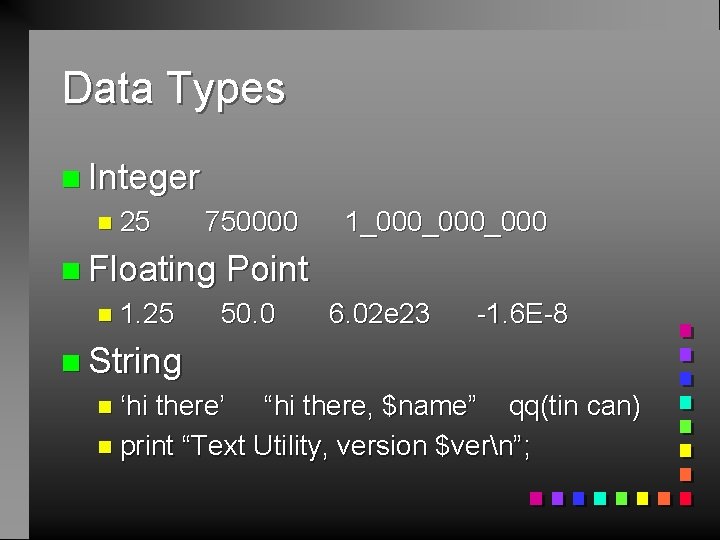
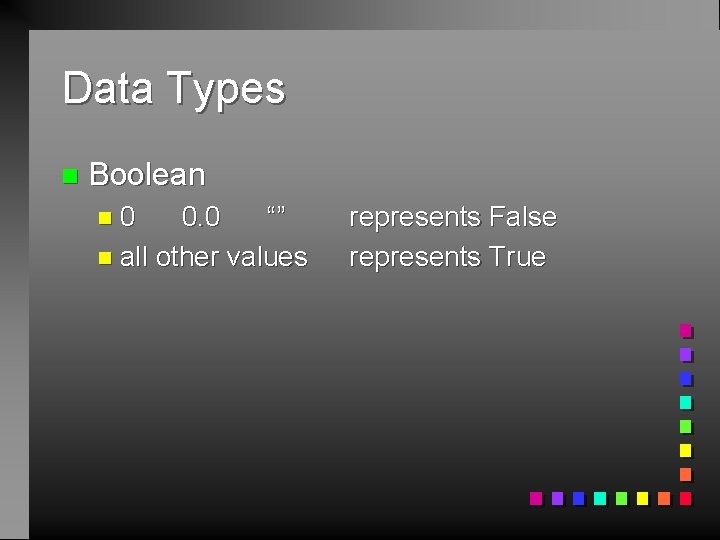
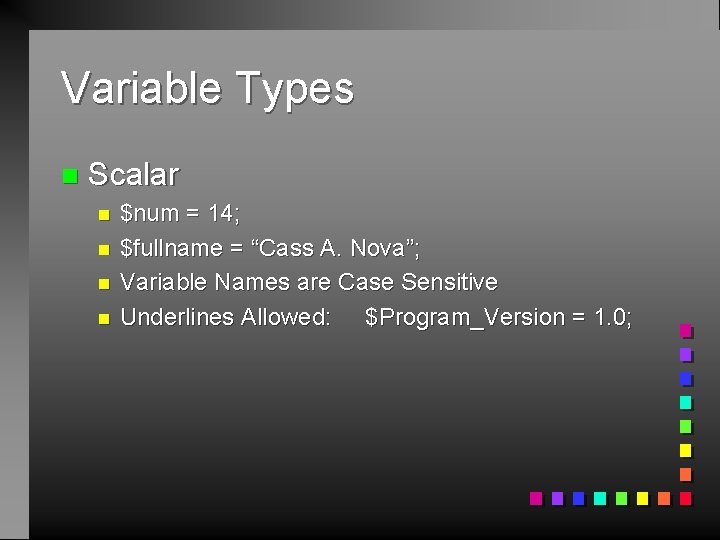
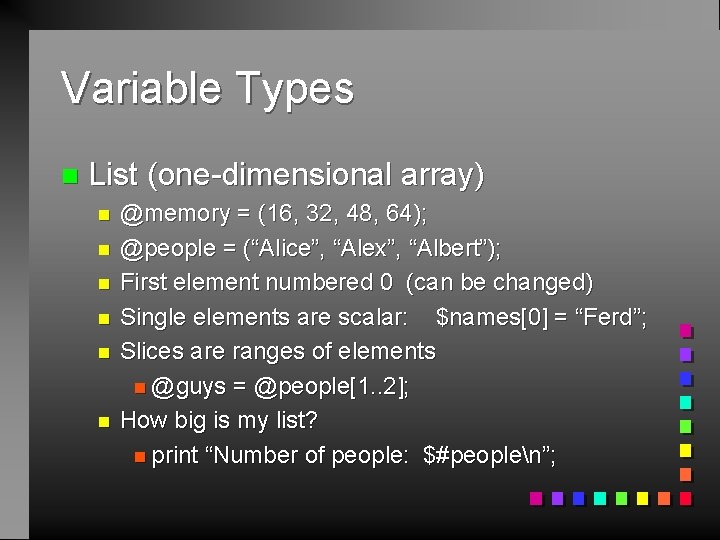
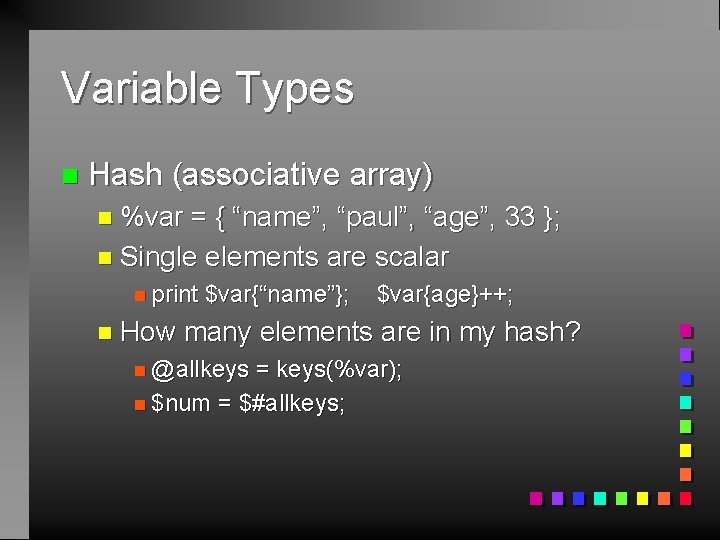
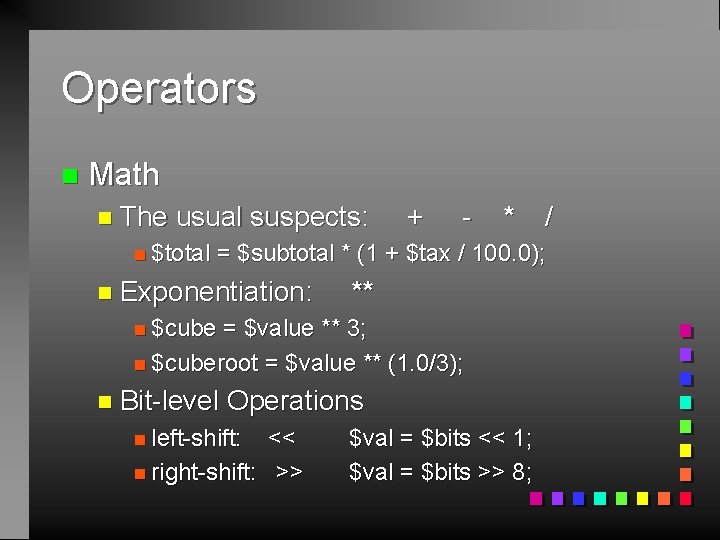
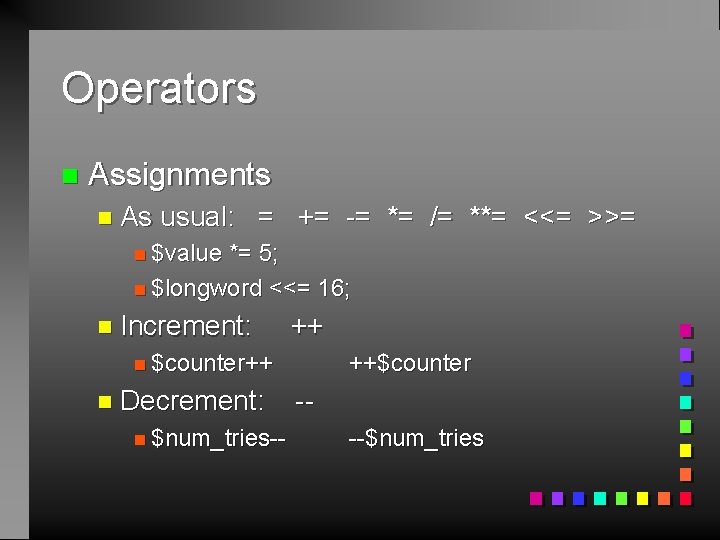
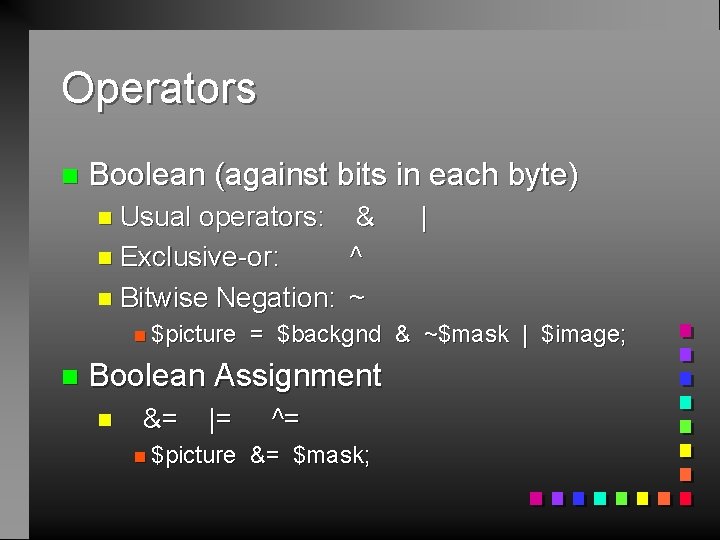
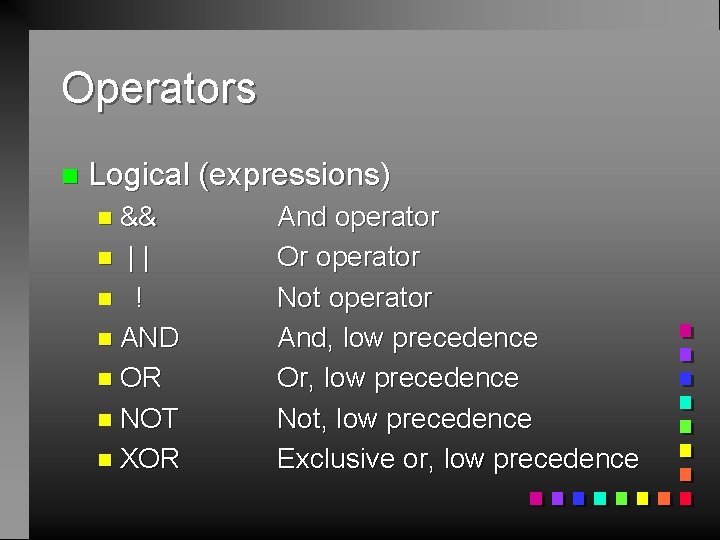
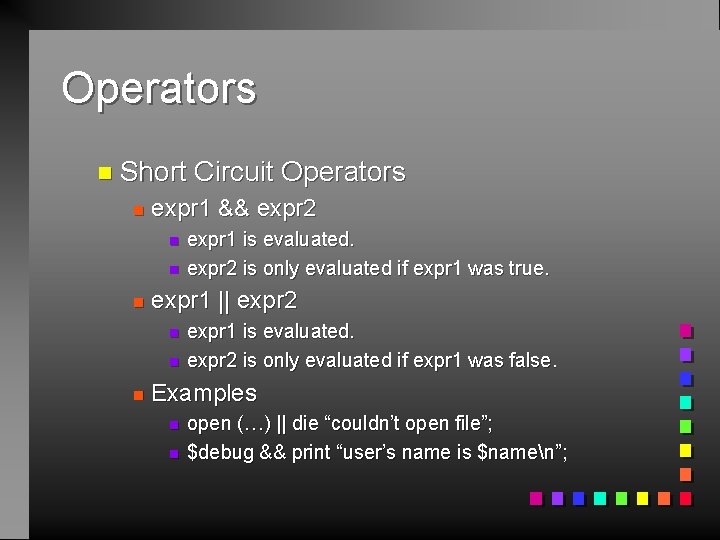
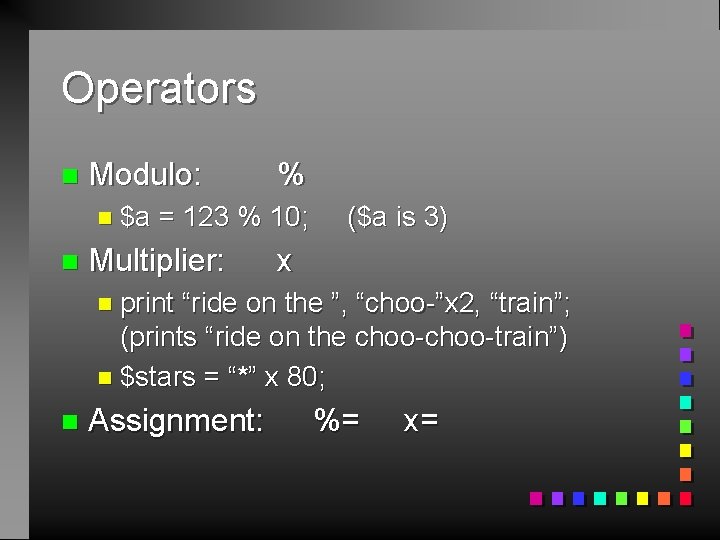
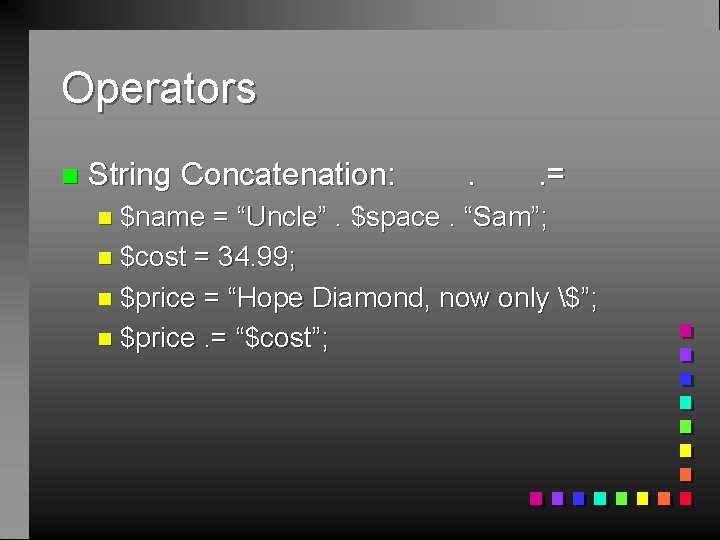
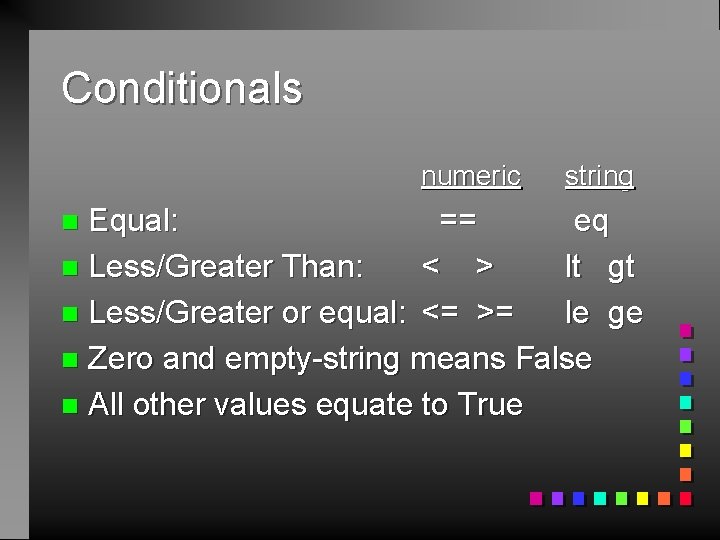
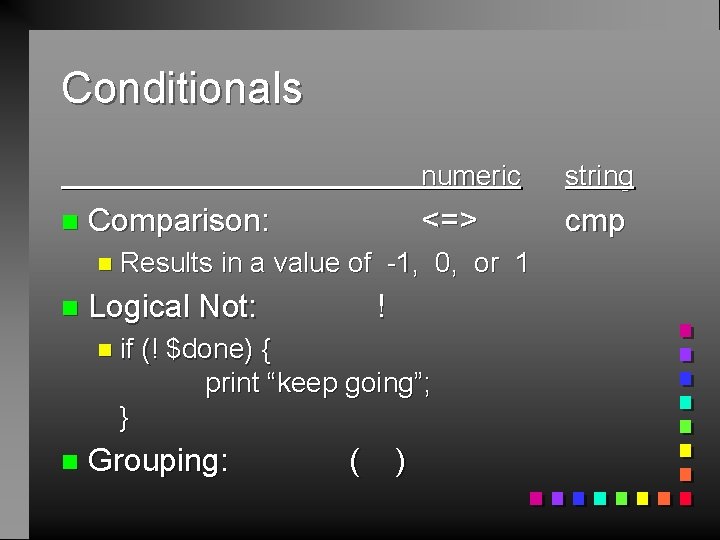
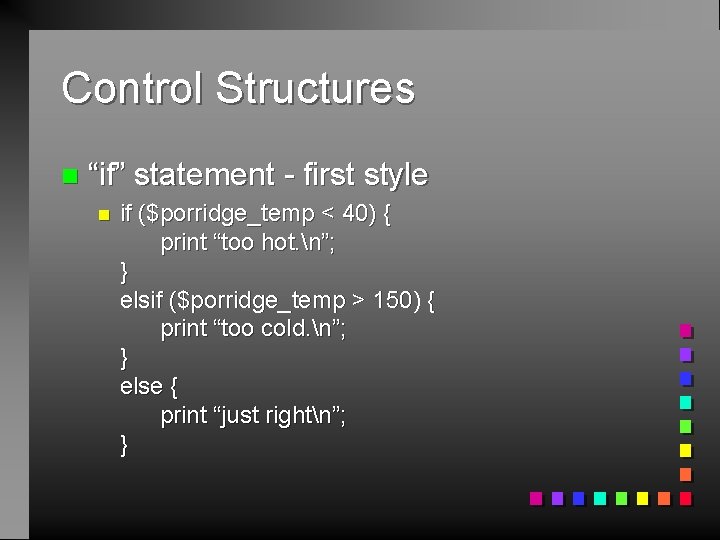
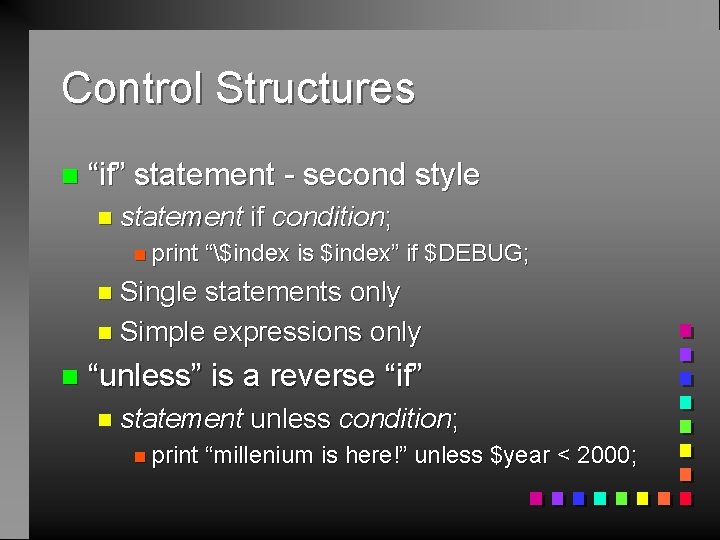
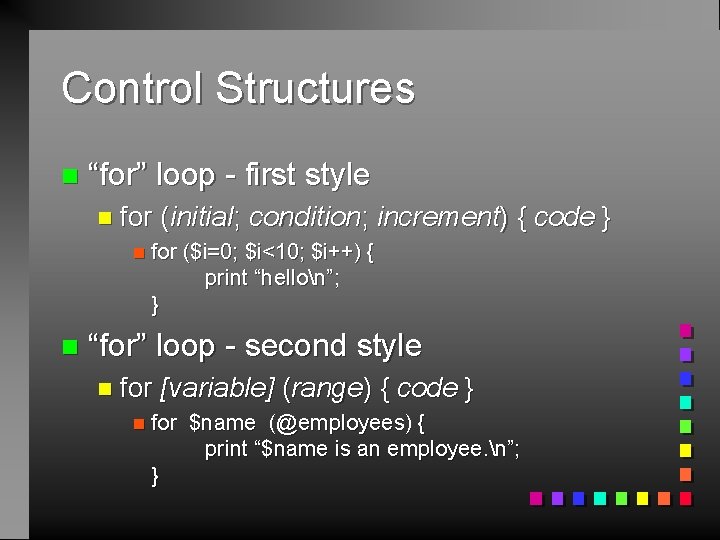
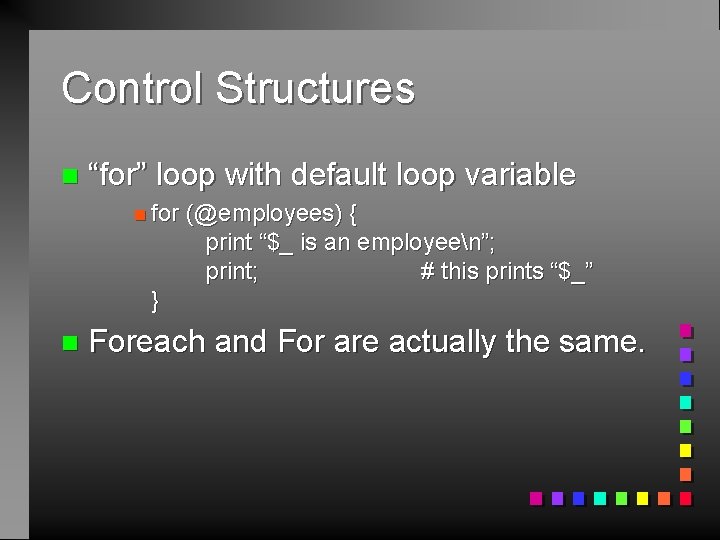
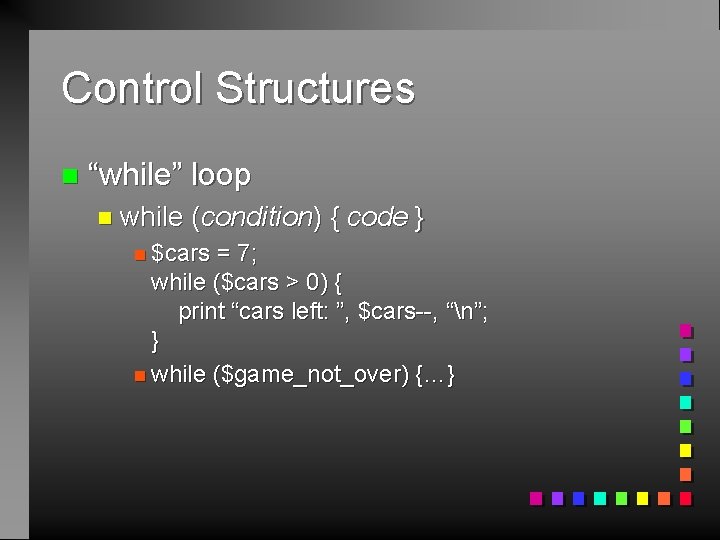
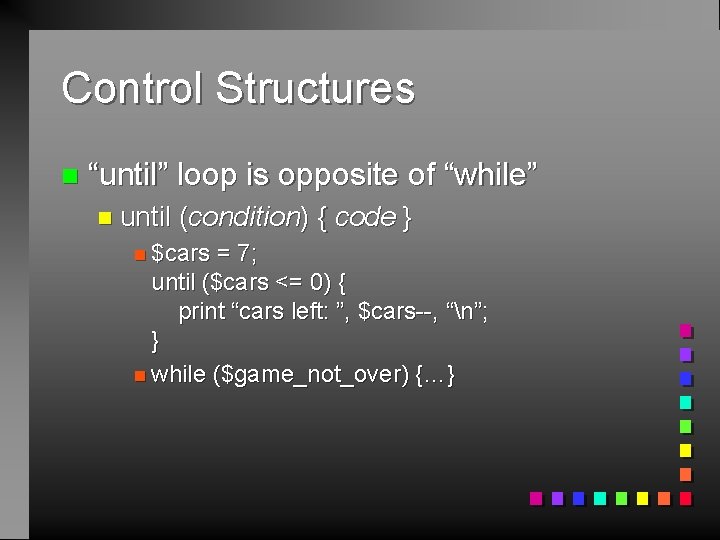
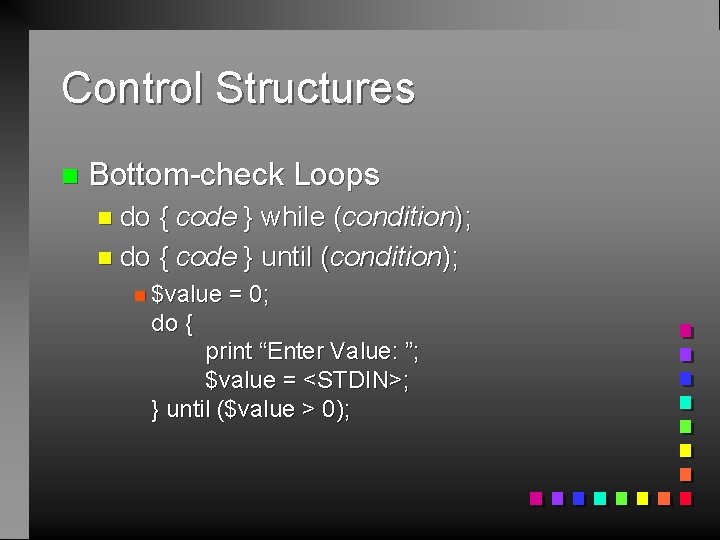
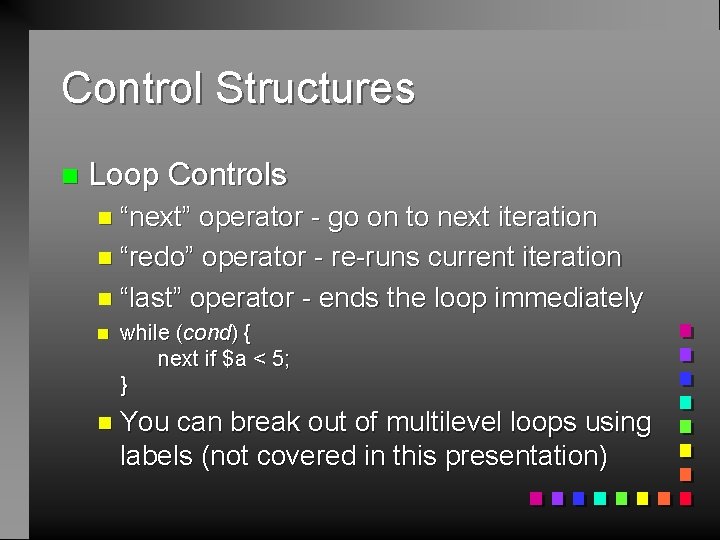
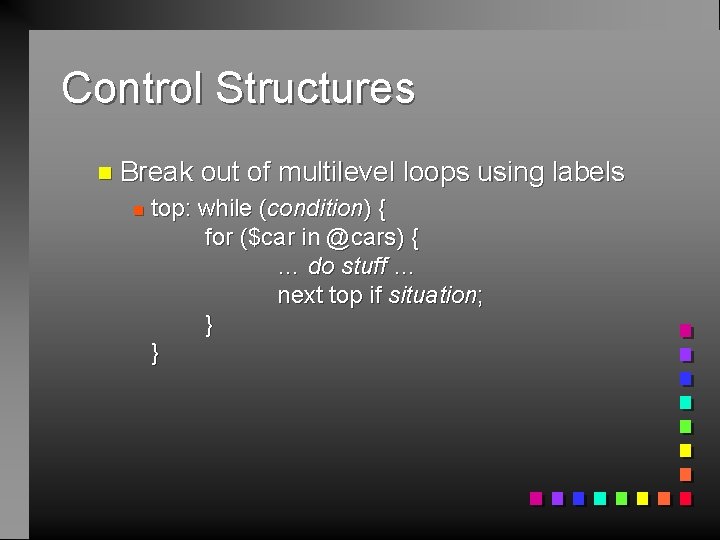
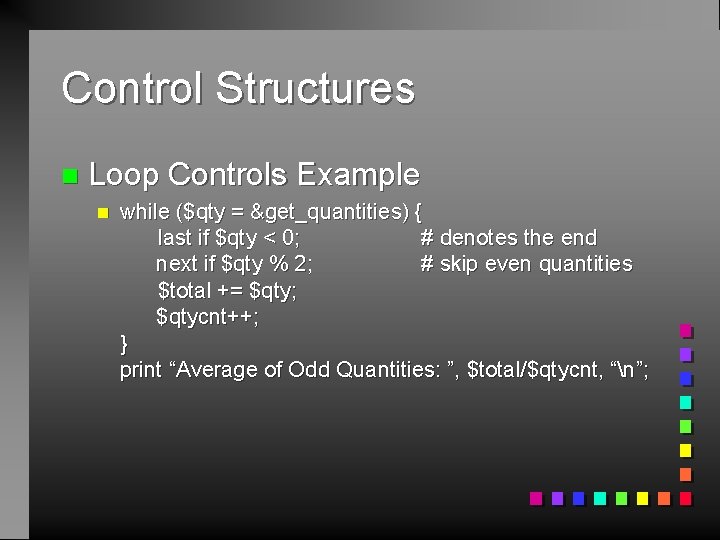
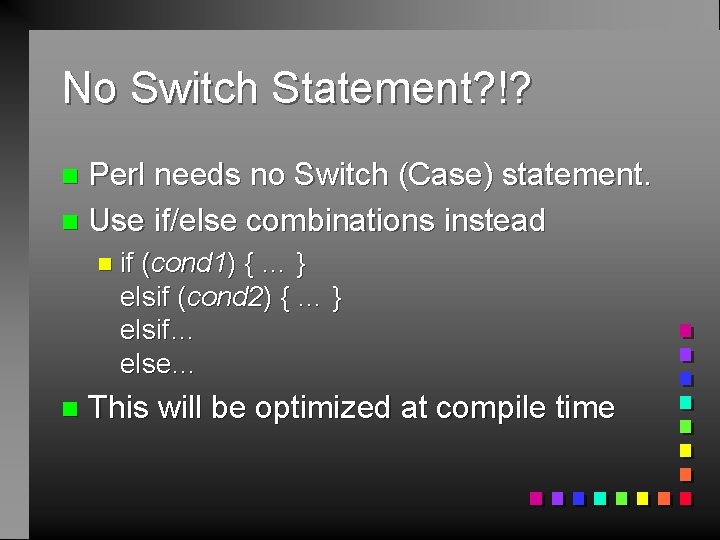
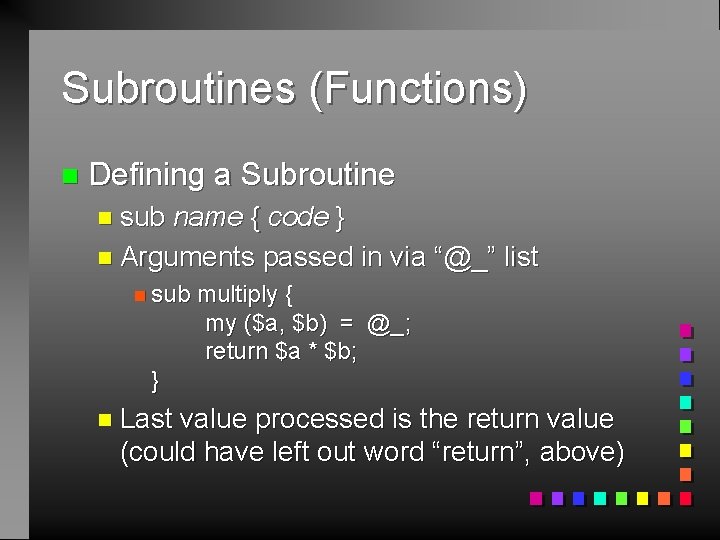
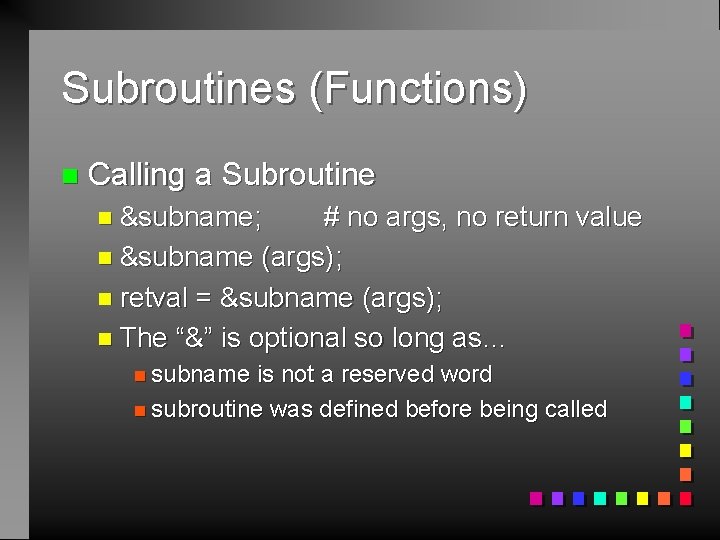
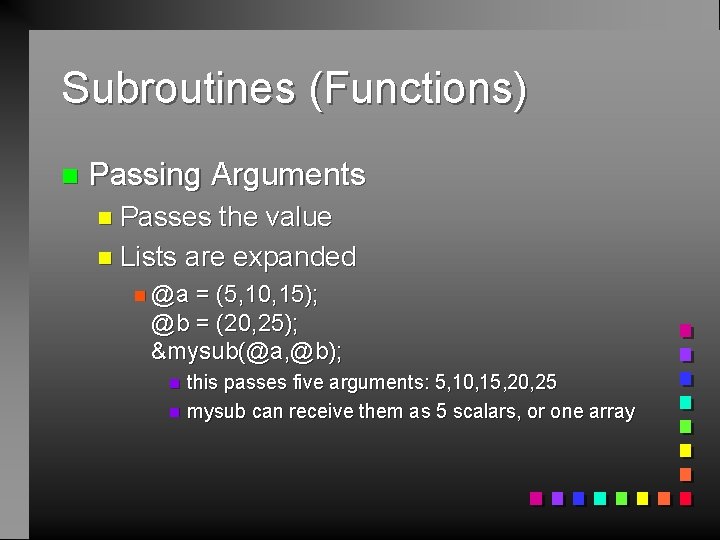
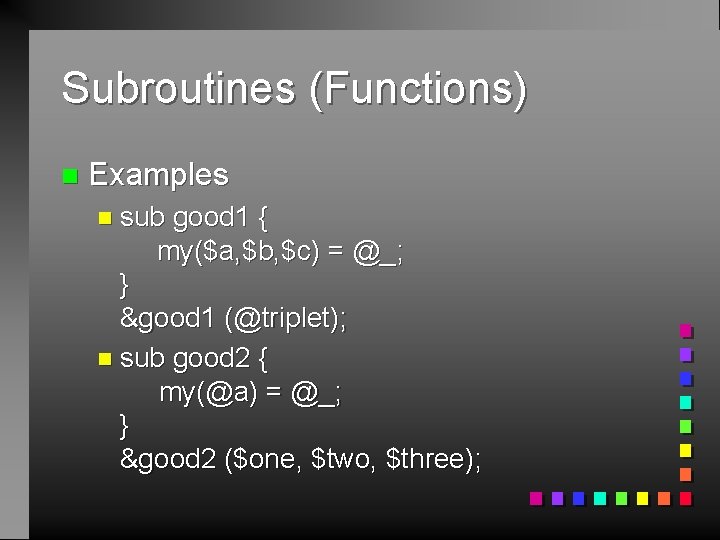
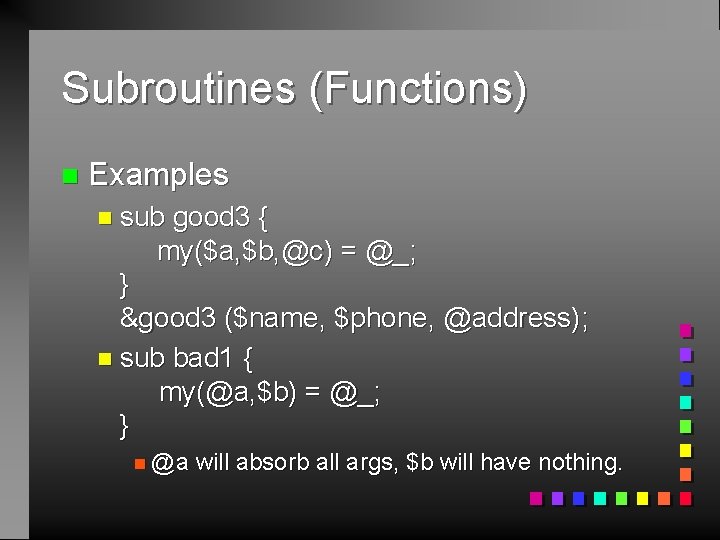
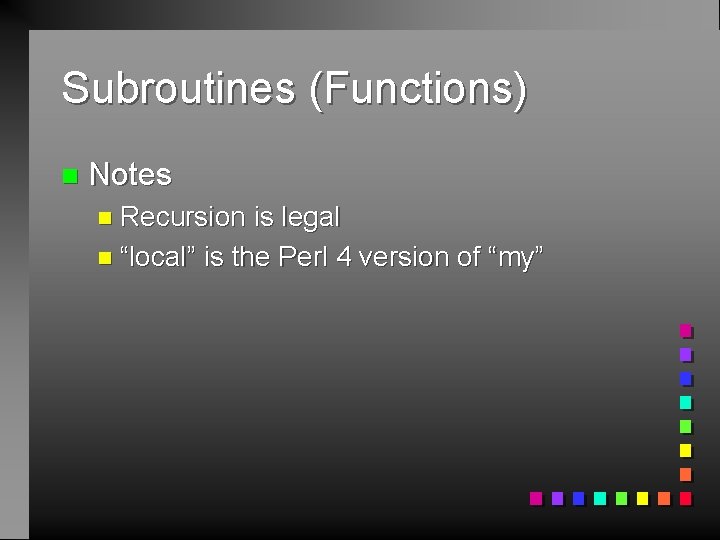
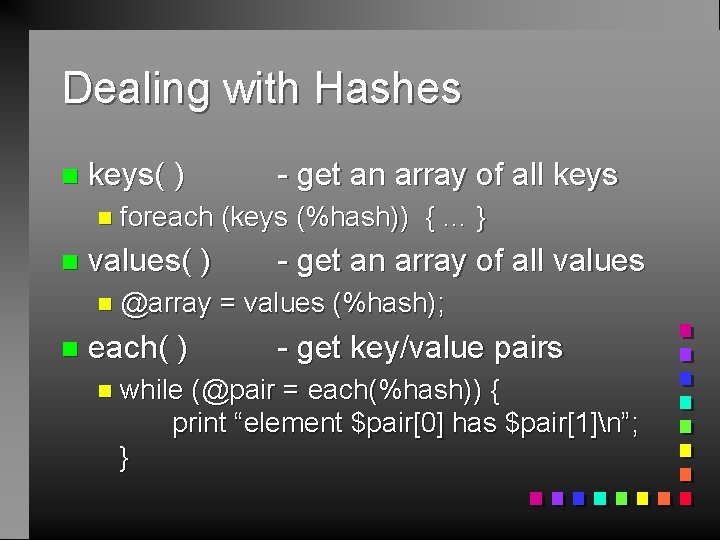
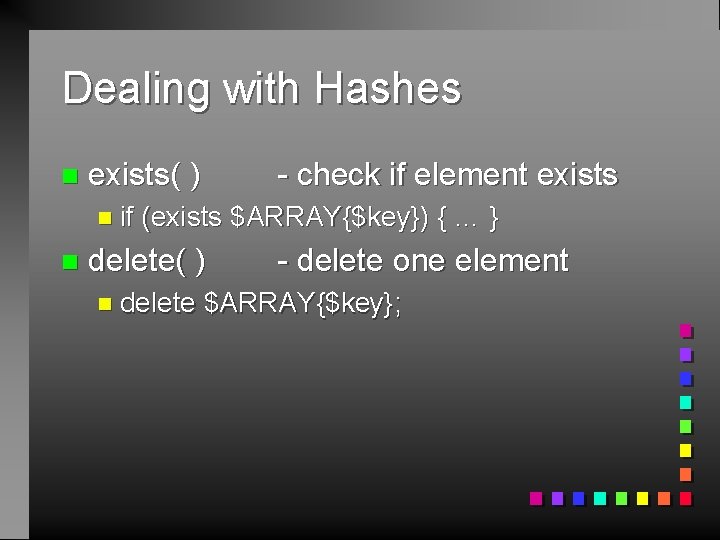
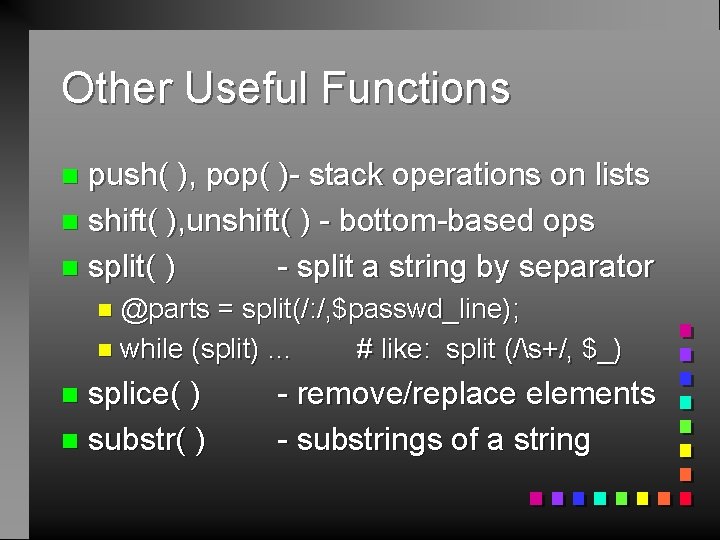
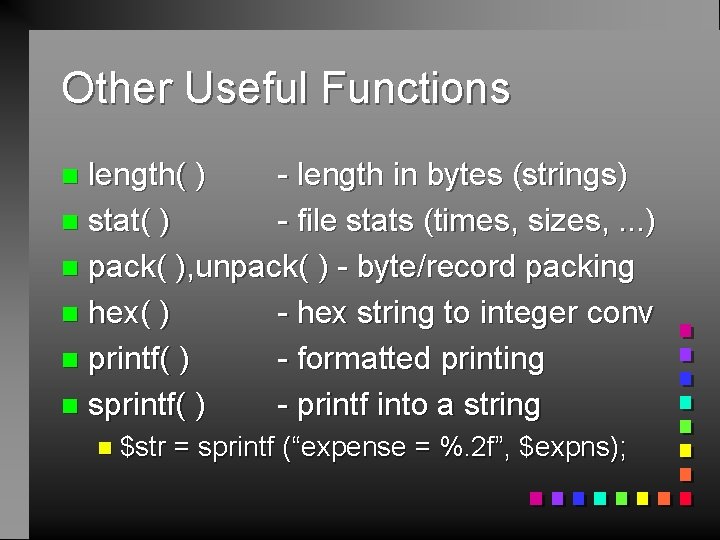
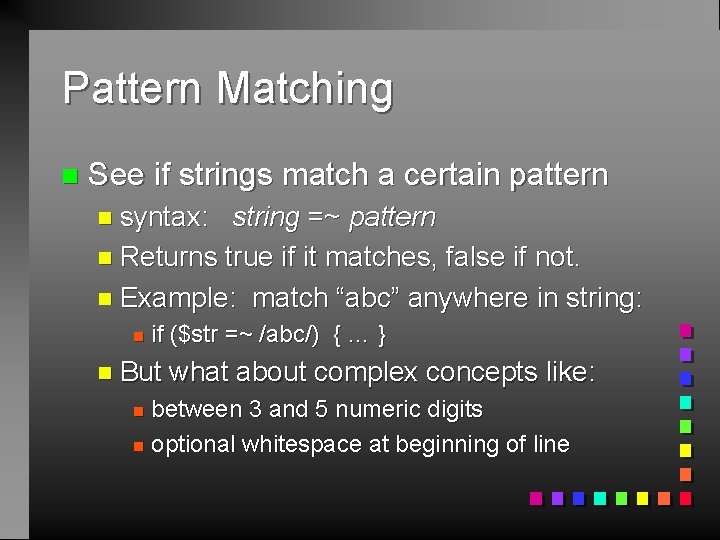
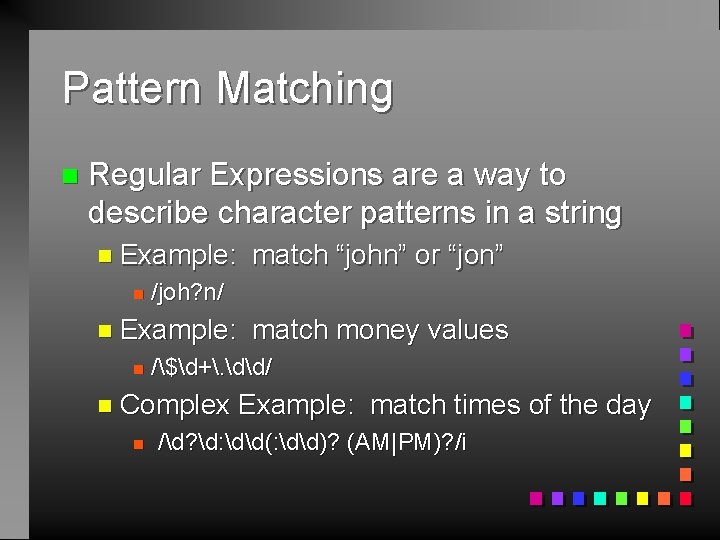
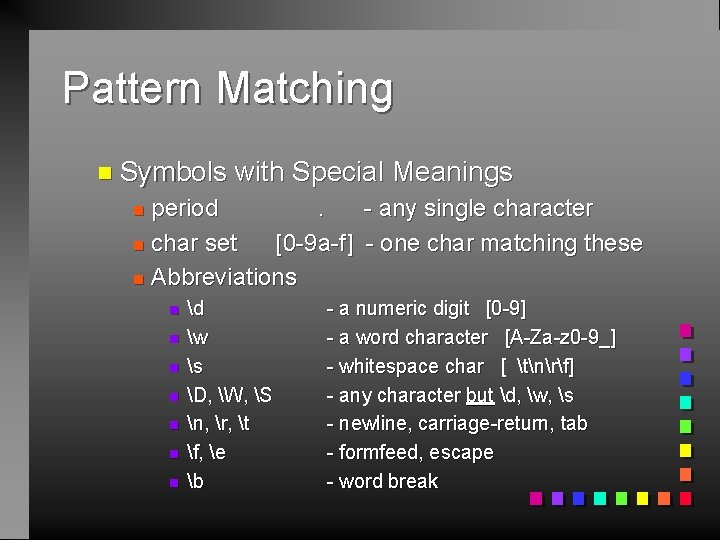
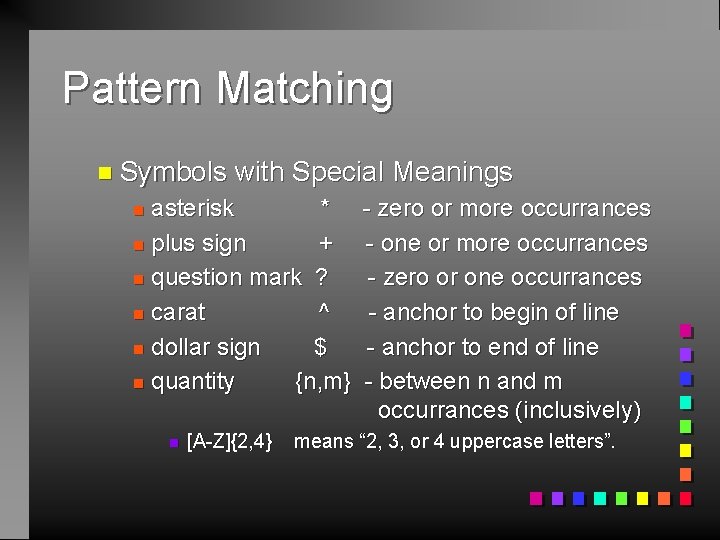
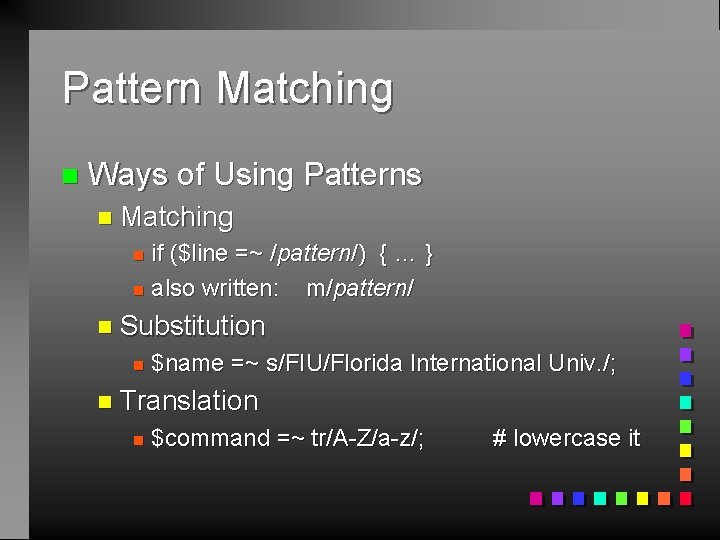
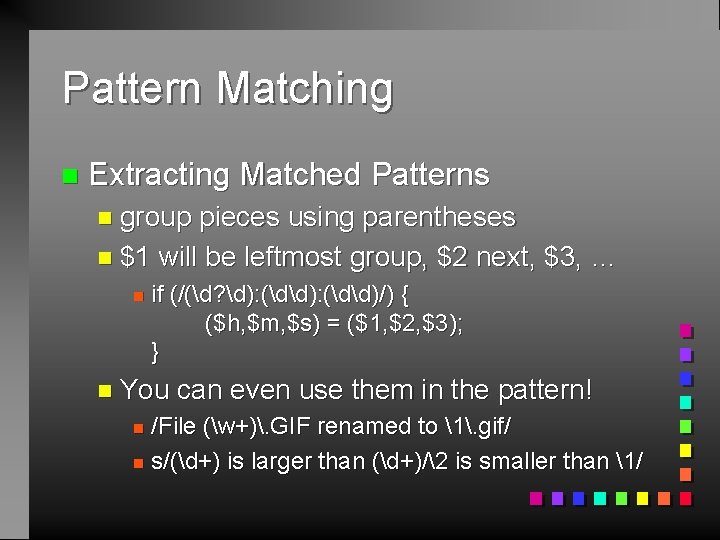
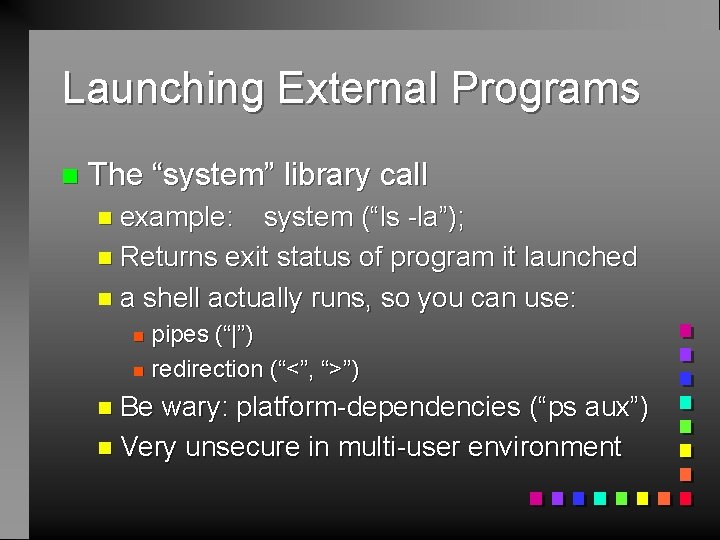
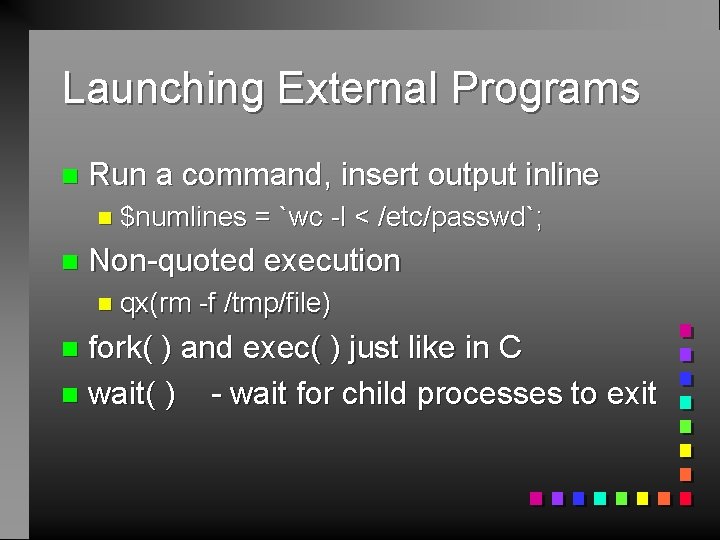
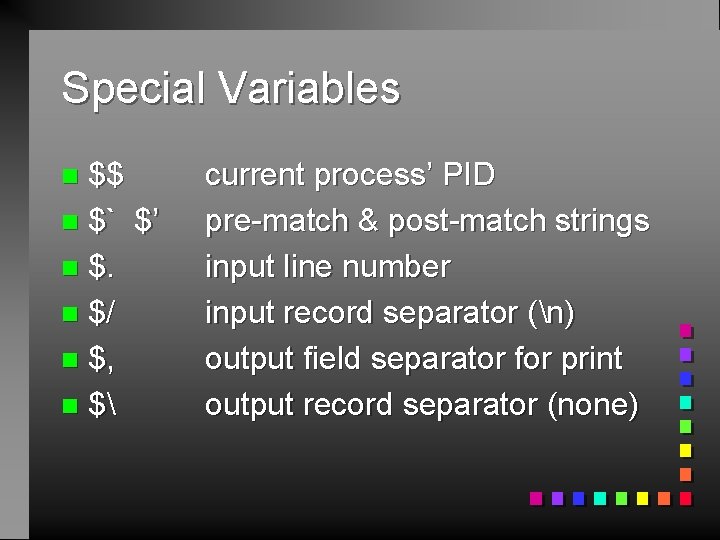
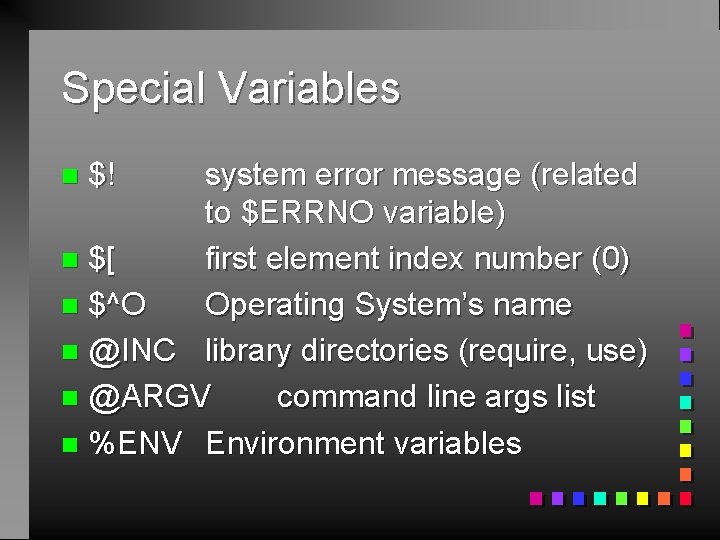
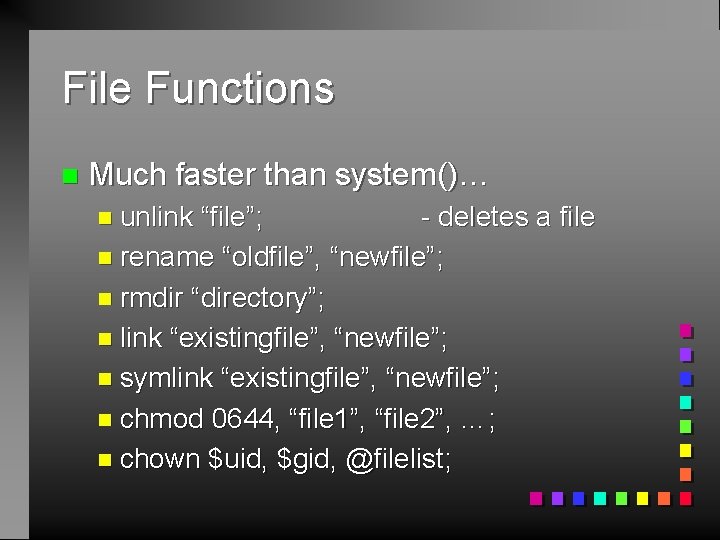
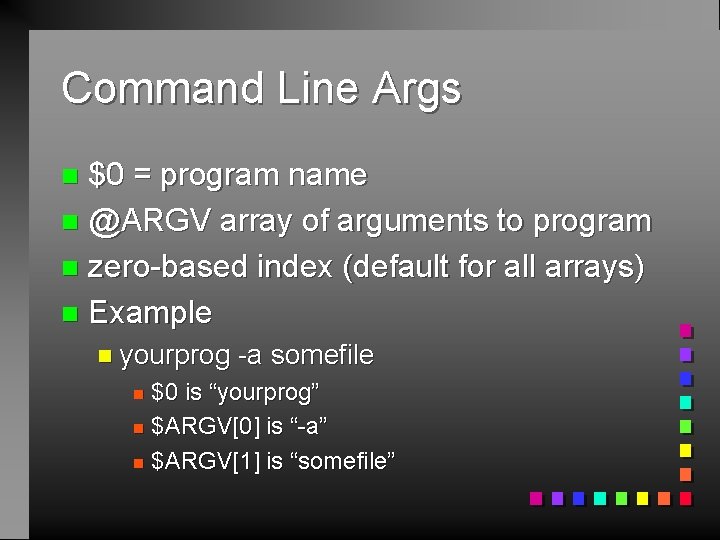
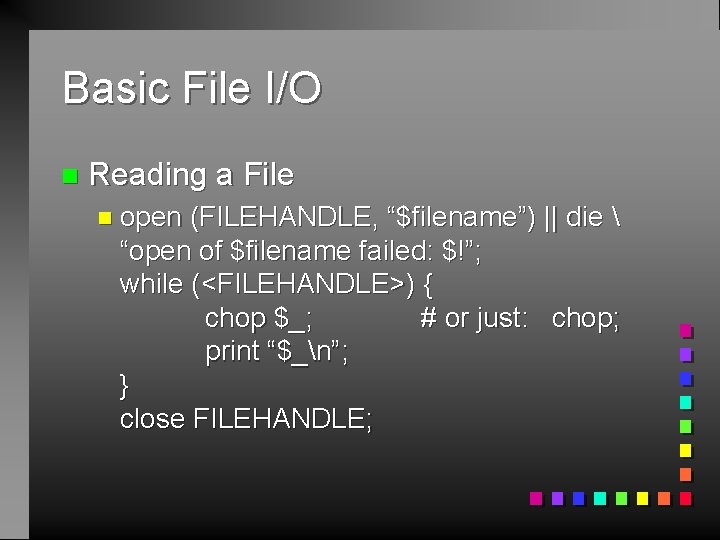
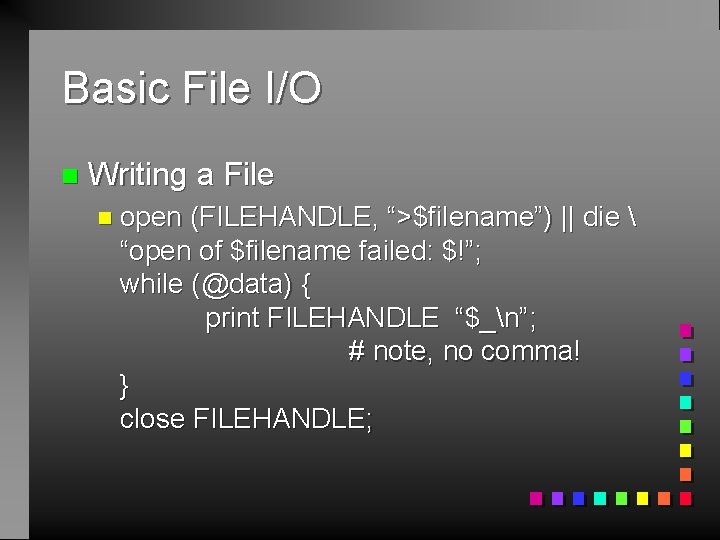
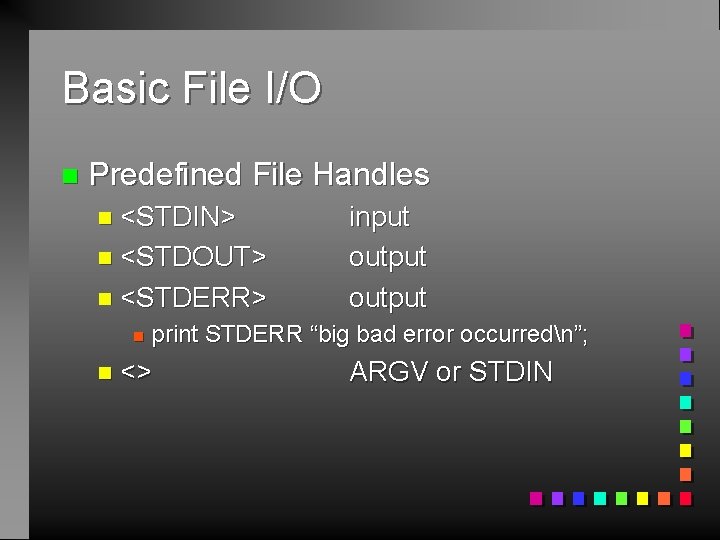
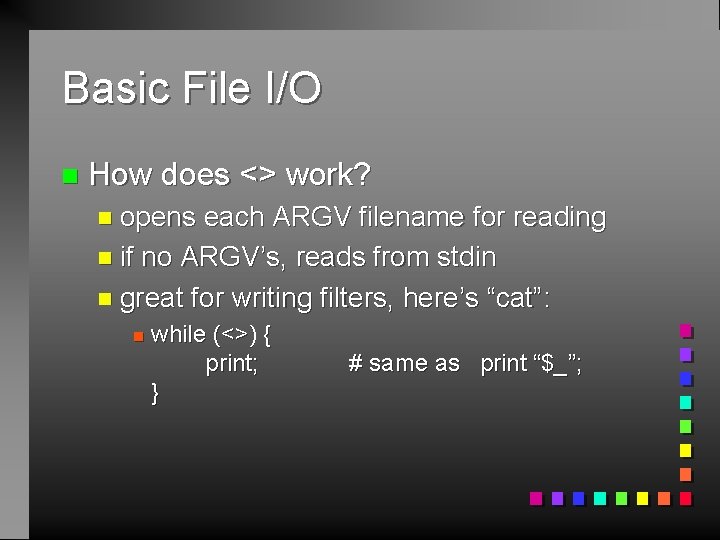
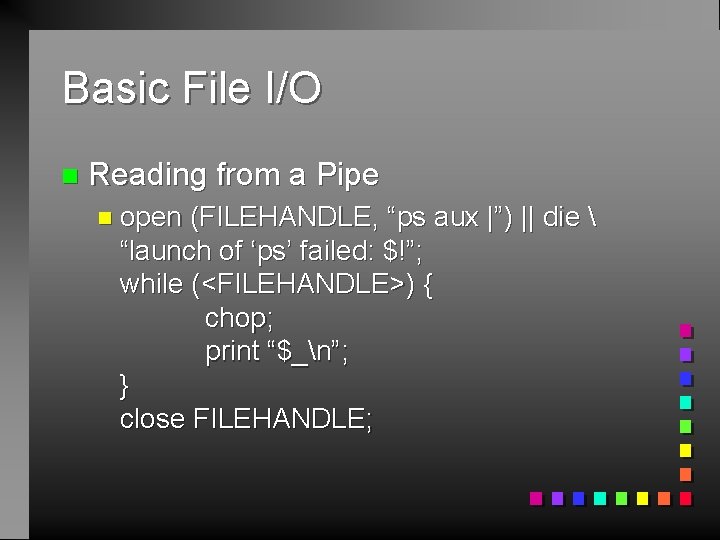
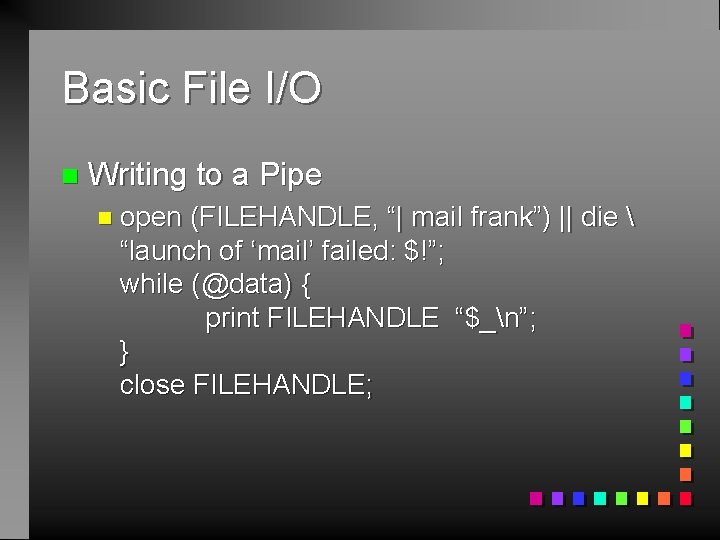
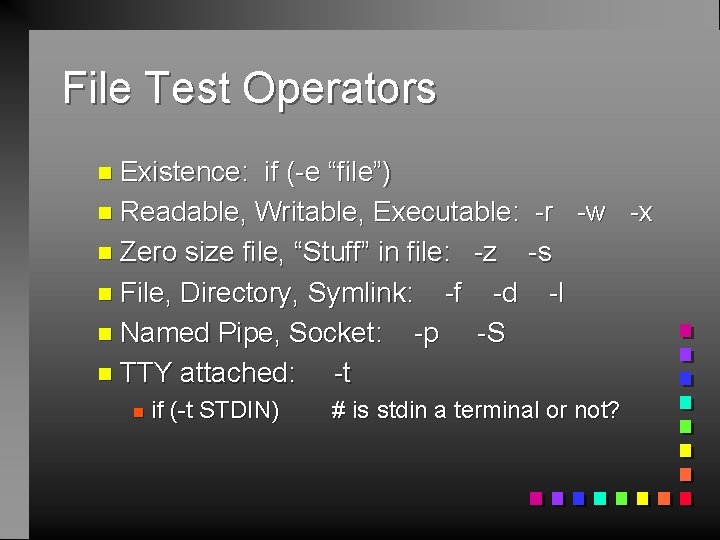
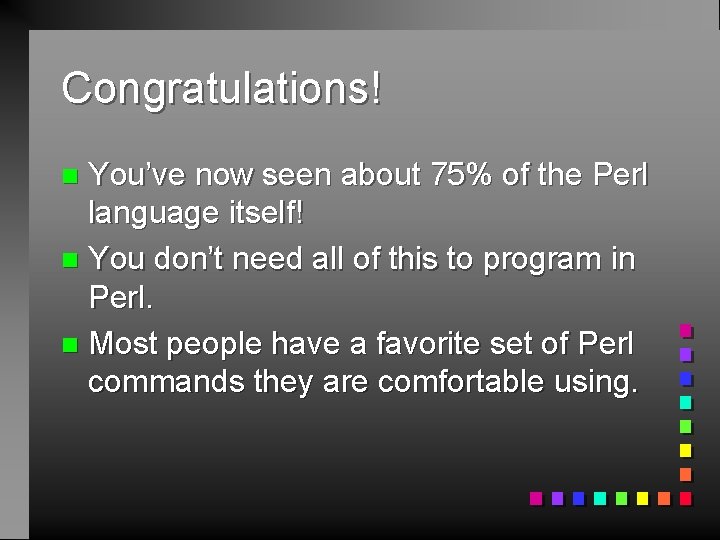
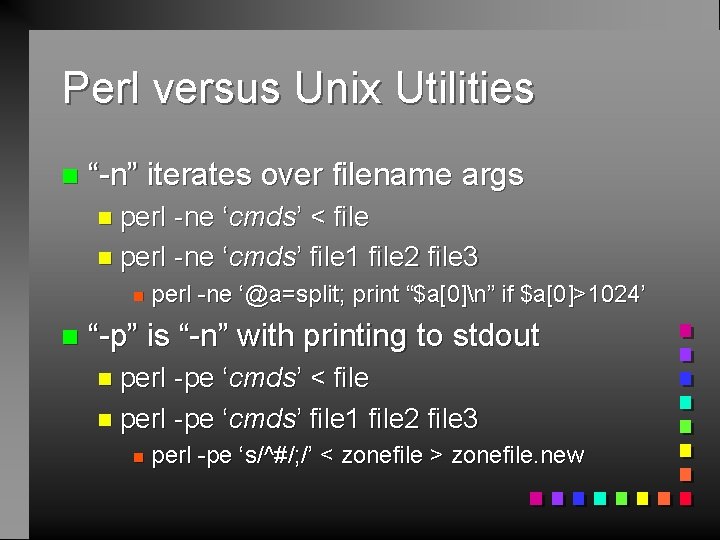
![Perl versus Unix Utilities n What’s n perl -ne ‘@a=split; print “$a[0]n” if $a[0]>1024’ Perl versus Unix Utilities n What’s n perl -ne ‘@a=split; print “$a[0]n” if $a[0]>1024’](https://slidetodoc.com/presentation_image_h2/59e30b6cb5887a59386ef47bfaf7304e/image-64.jpg)
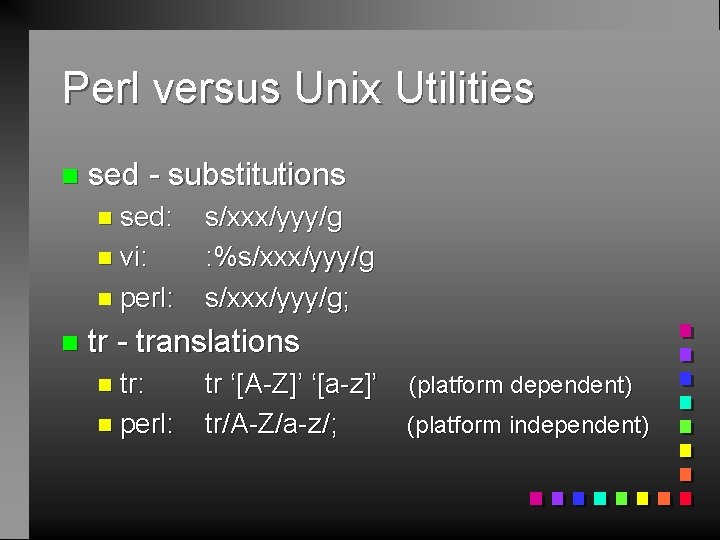
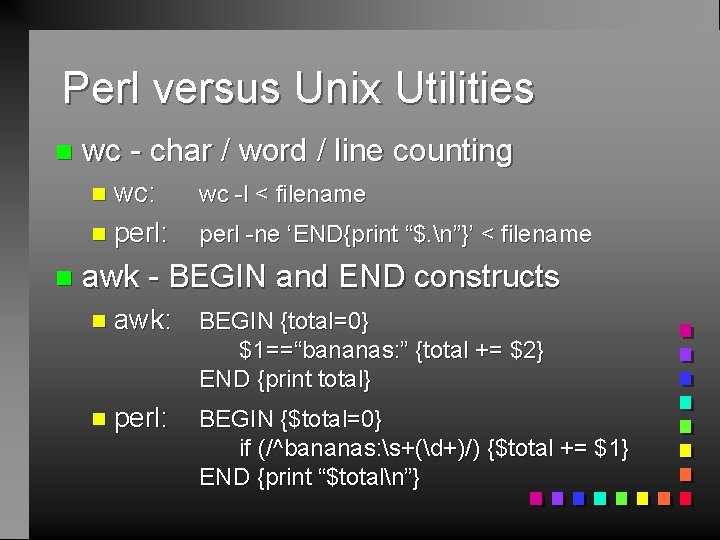
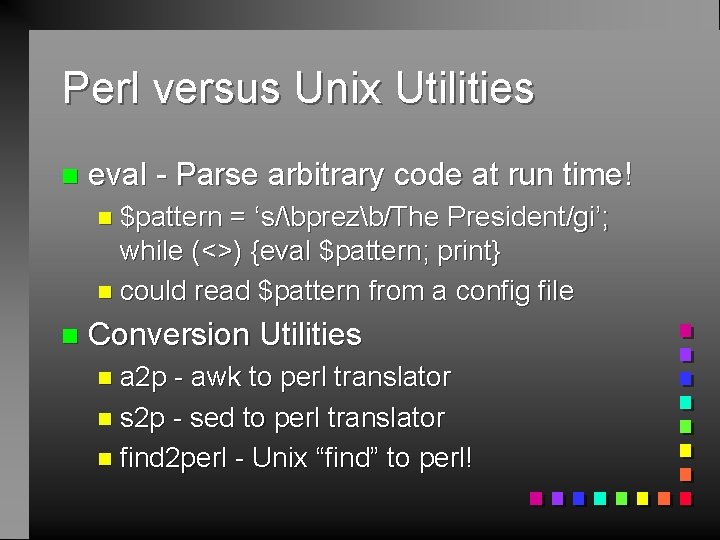
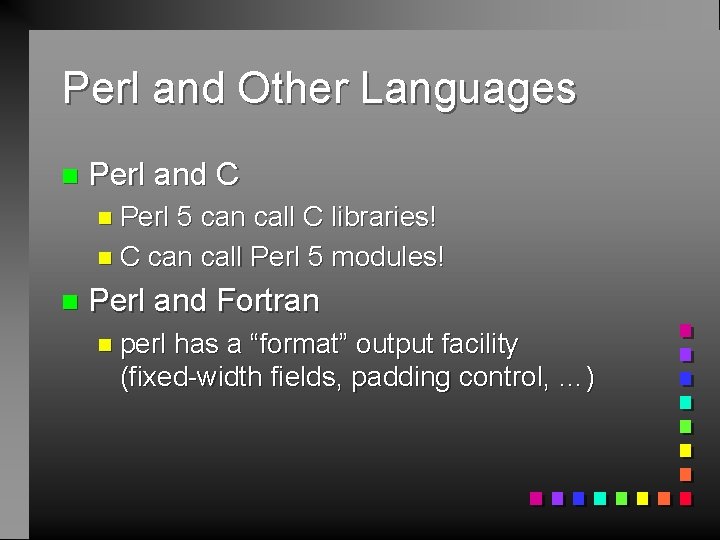
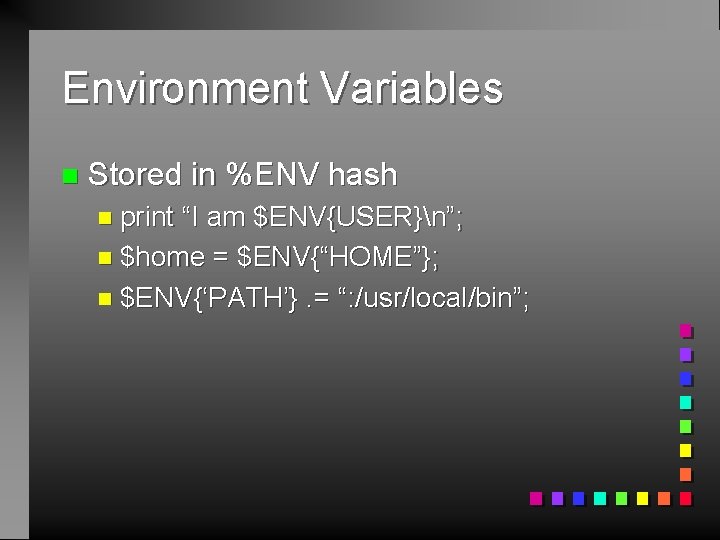
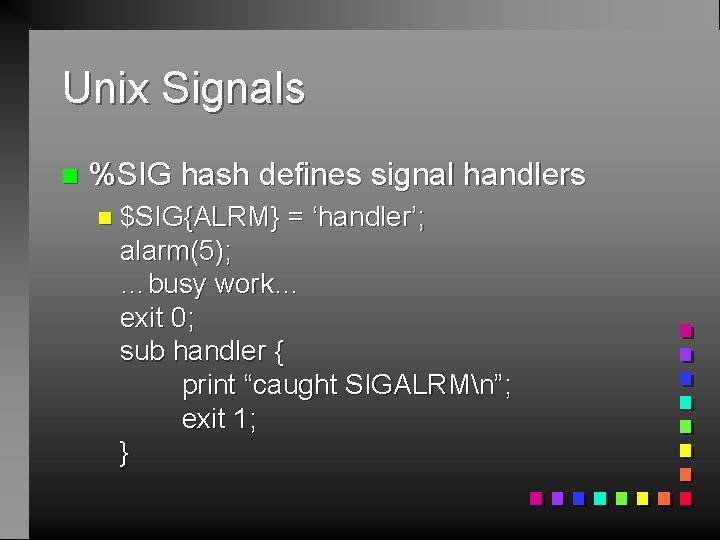
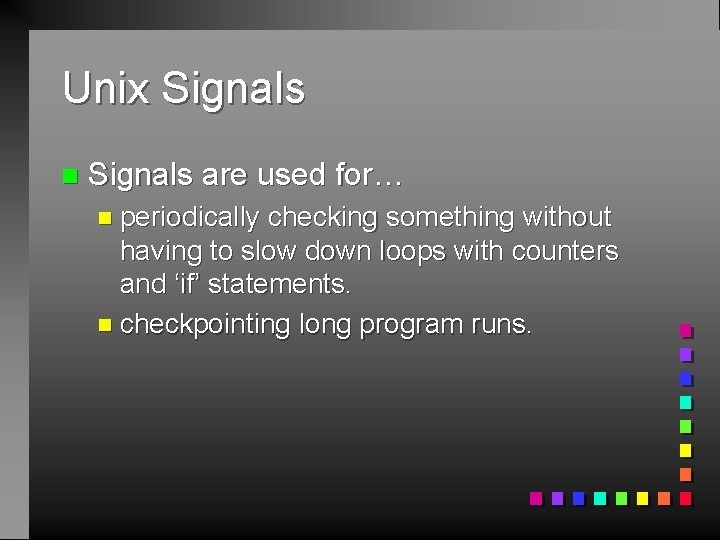
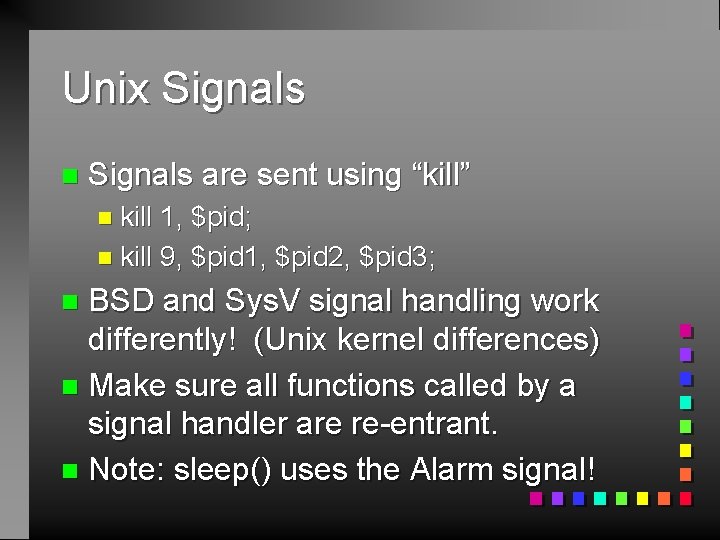
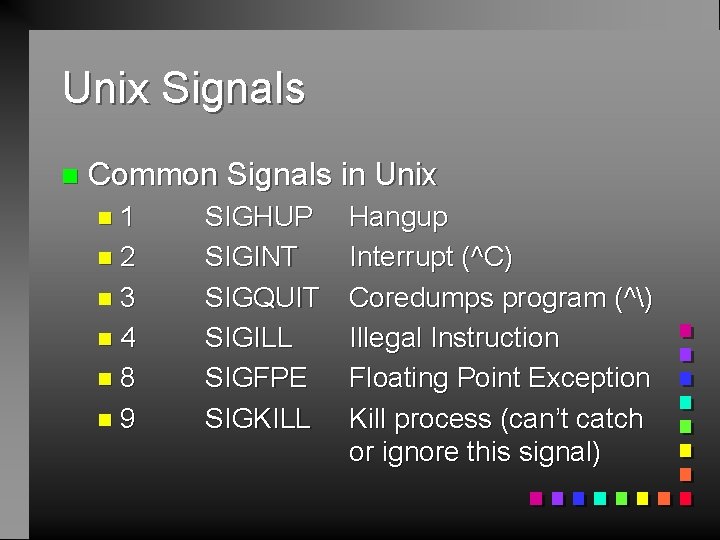
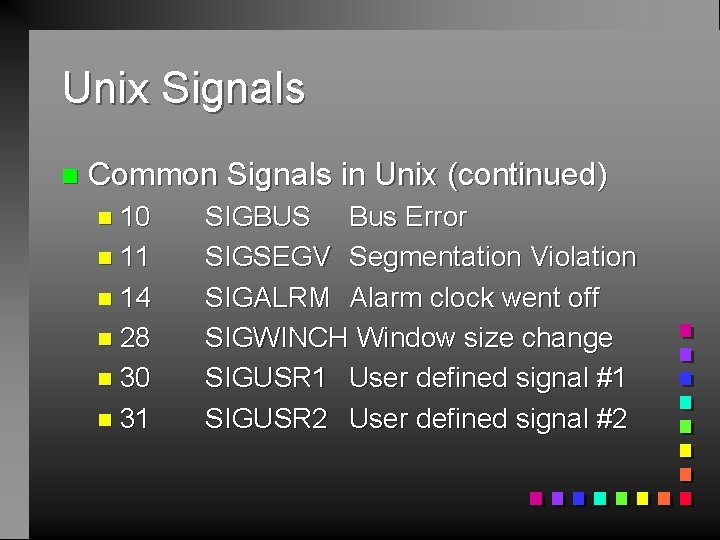
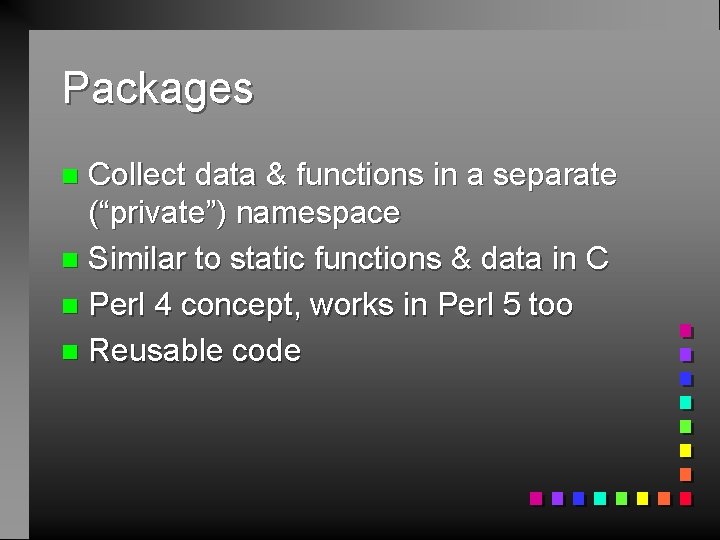
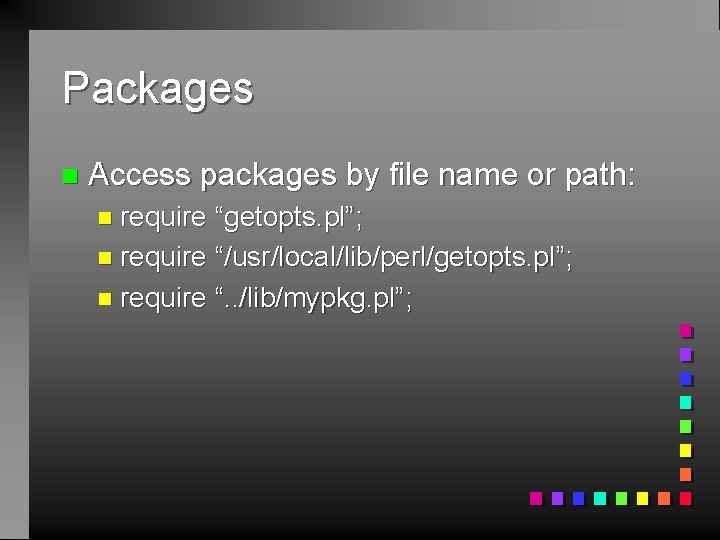
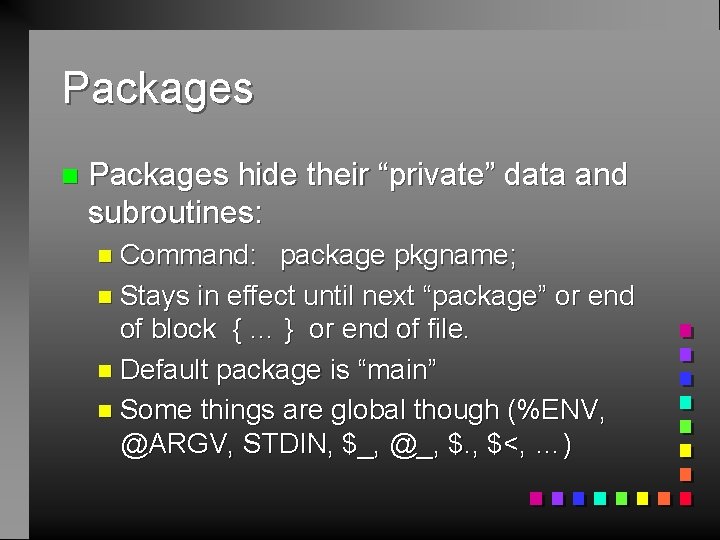
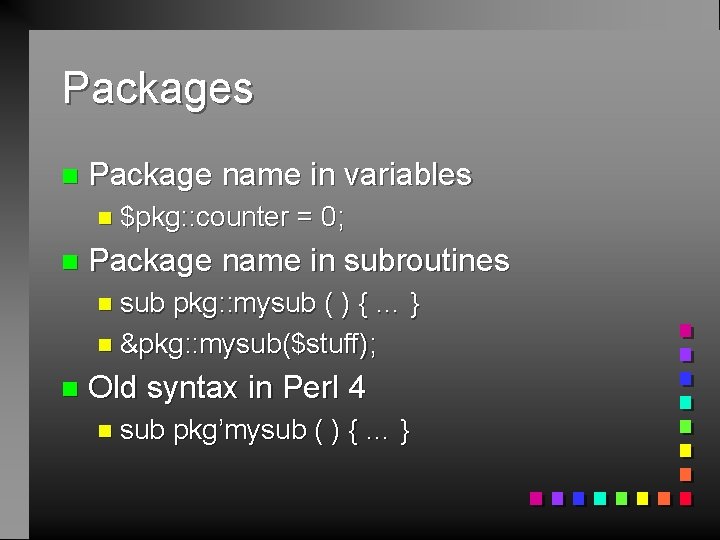
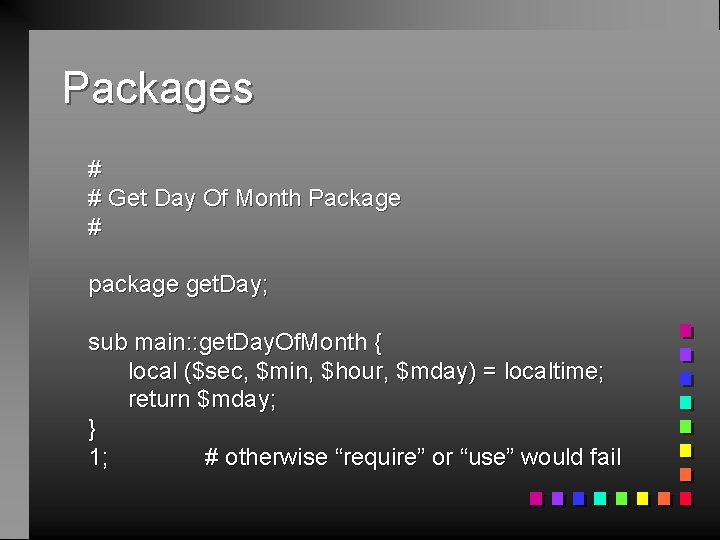
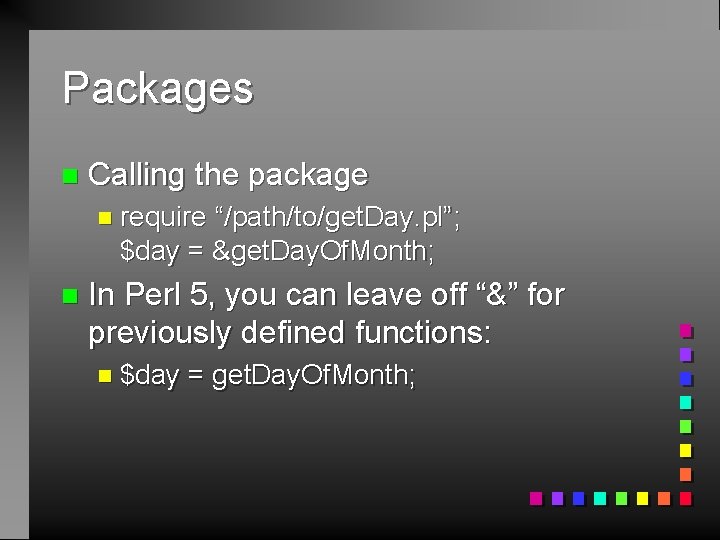
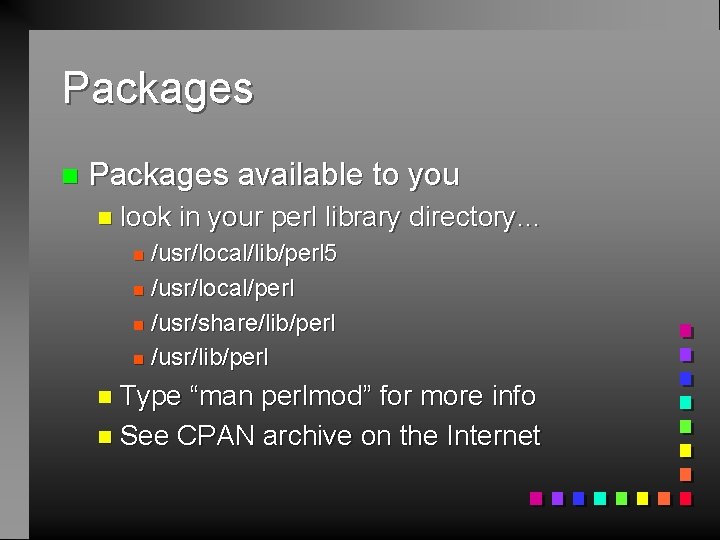
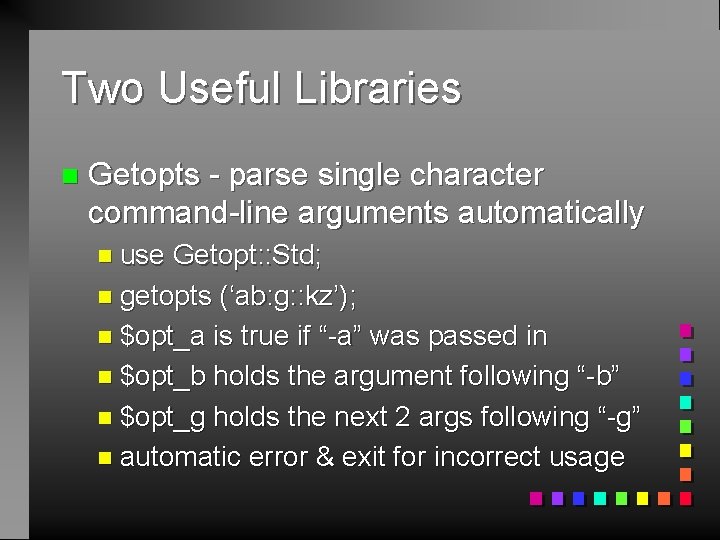
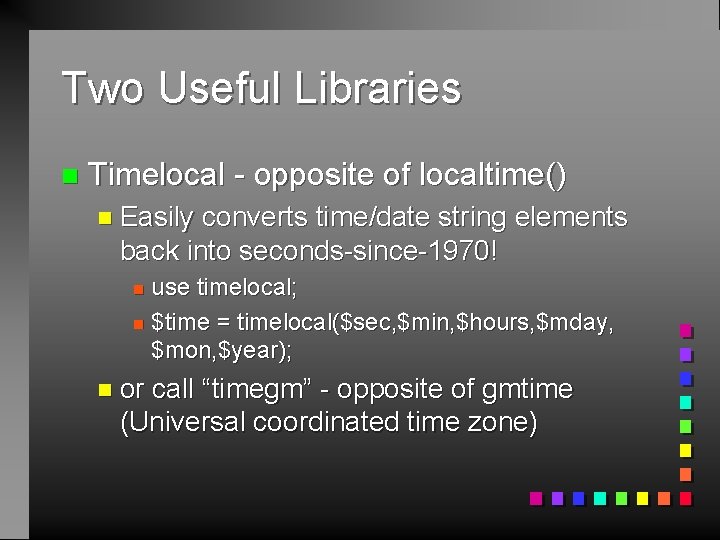
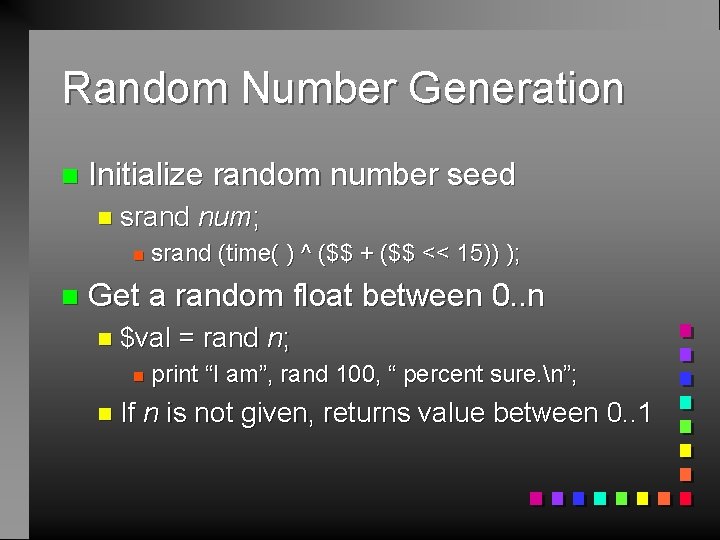
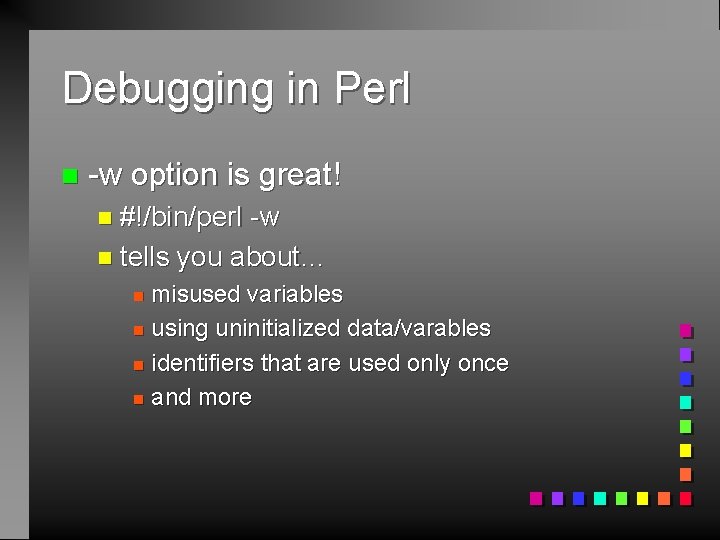
![Debugging in Perl n Debug mode: perl -d filename [args] n Display Commands h Debugging in Perl n Debug mode: perl -d filename [args] n Display Commands h](https://slidetodoc.com/presentation_image_h2/59e30b6cb5887a59386ef47bfaf7304e/image-86.jpg)
![Debugging in Perl n Display Commands (continued) w n. S n V [pkg] n Debugging in Perl n Display Commands (continued) w n. S n V [pkg] n](https://slidetodoc.com/presentation_image_h2/59e30b6cb5887a59386ef47bfaf7304e/image-87.jpg)
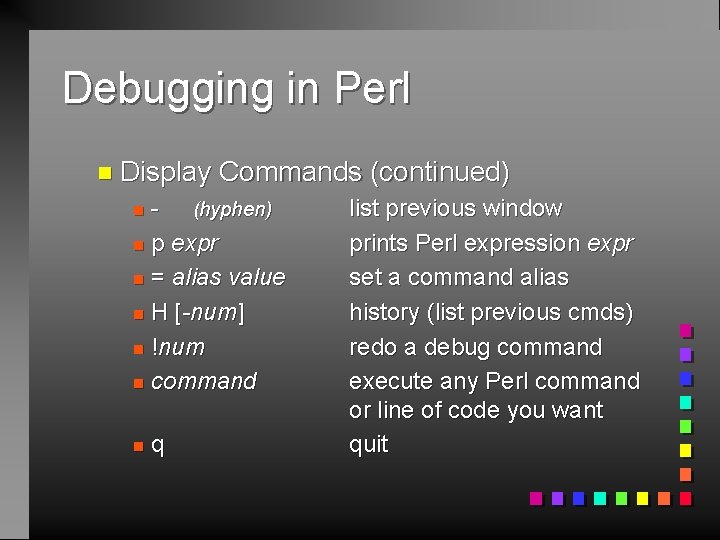
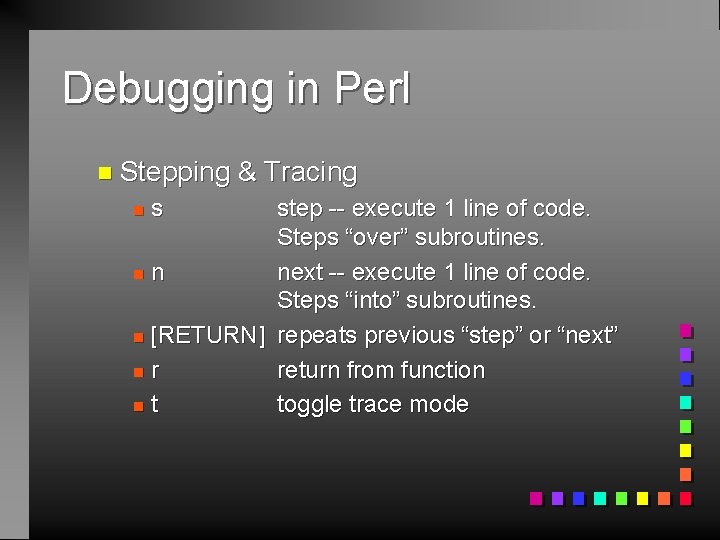
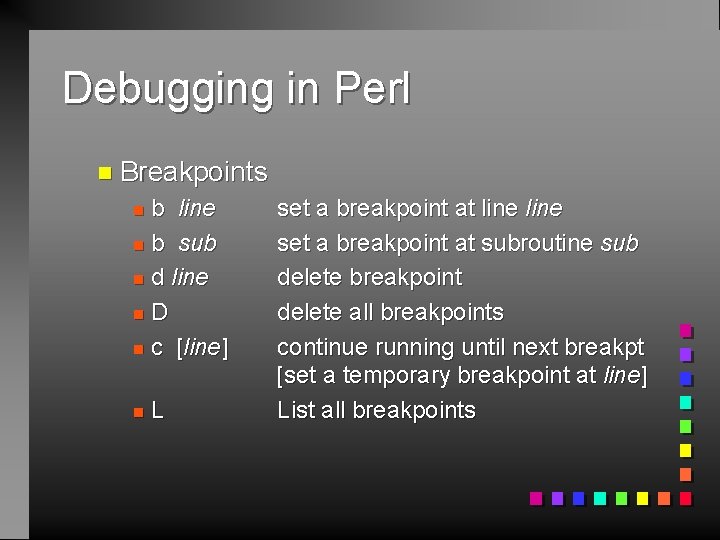
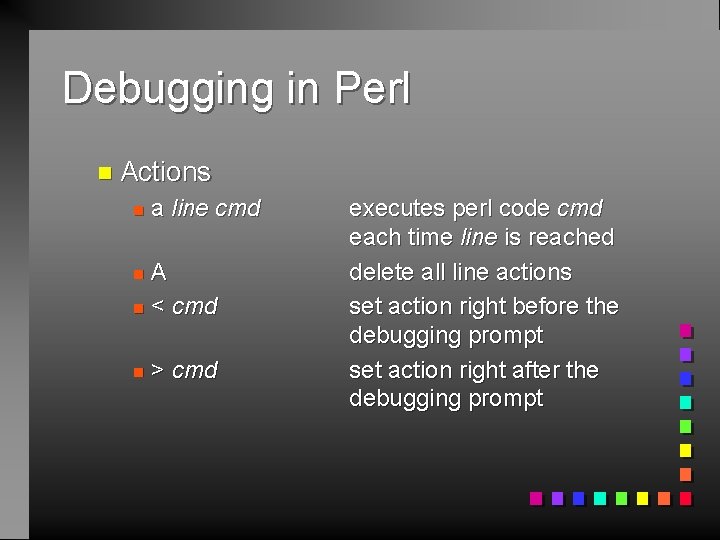
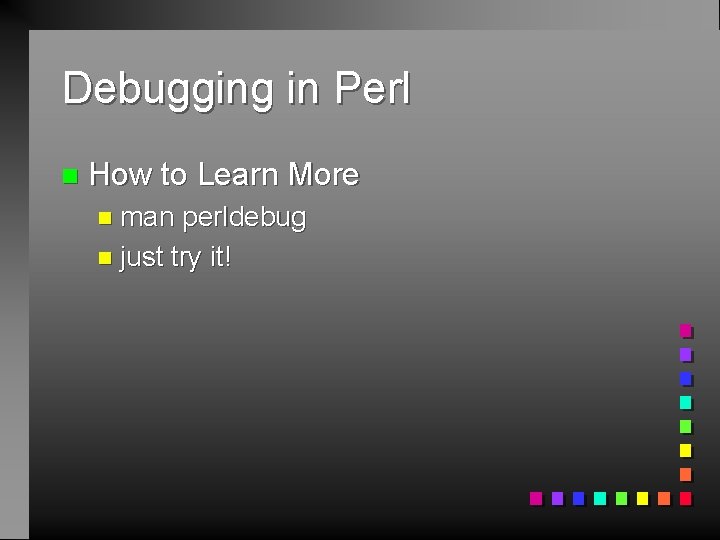
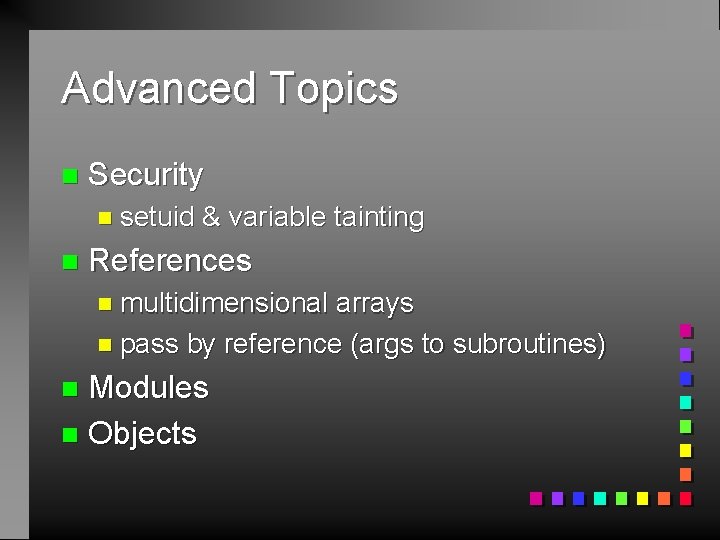
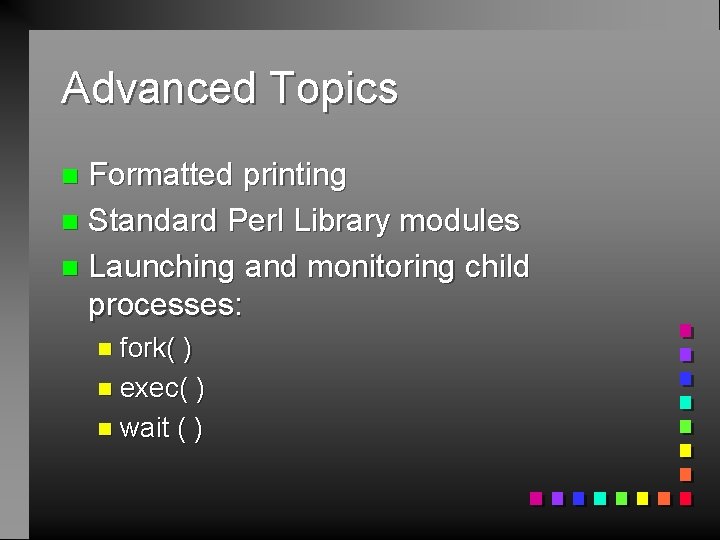
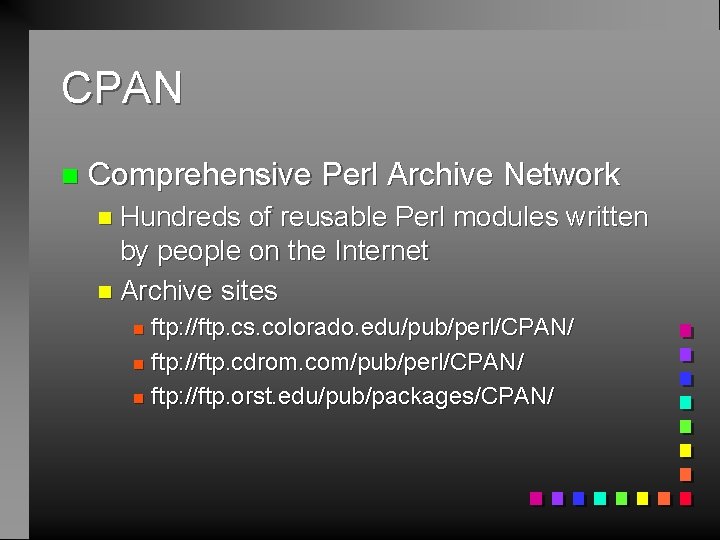
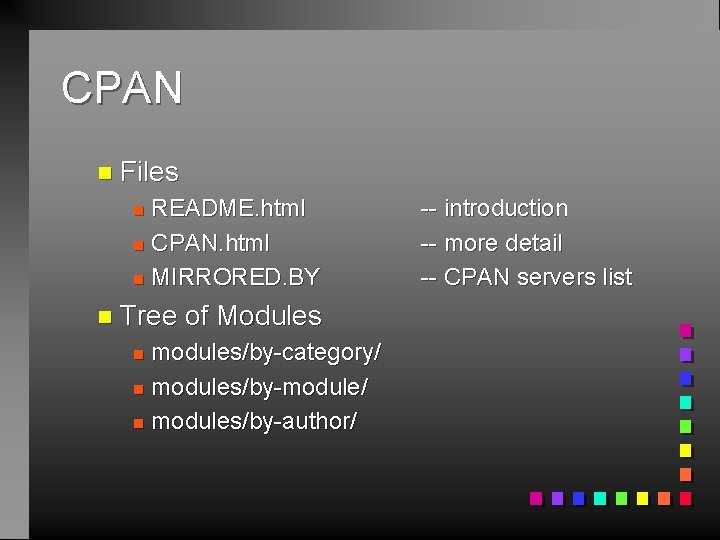
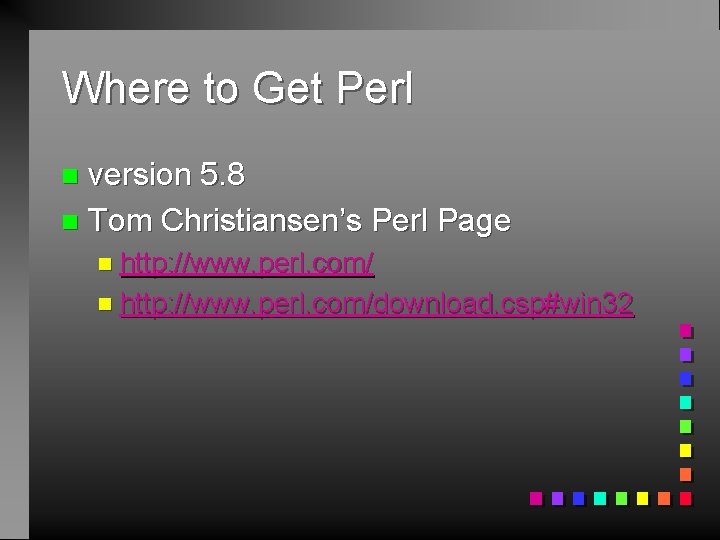
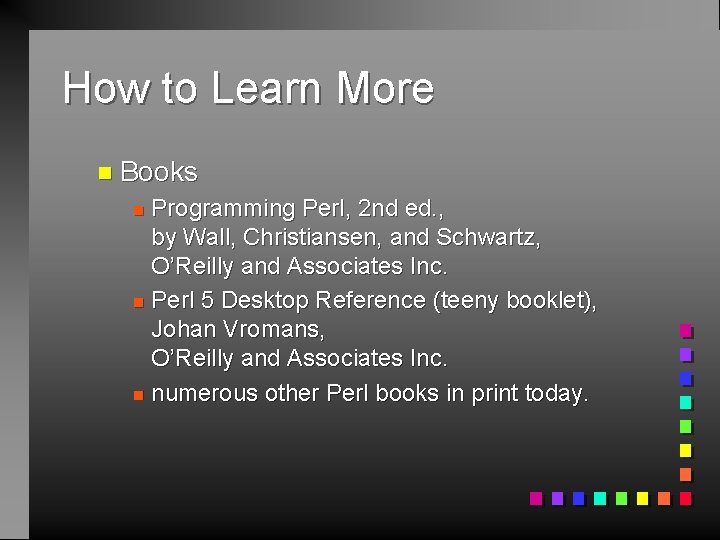
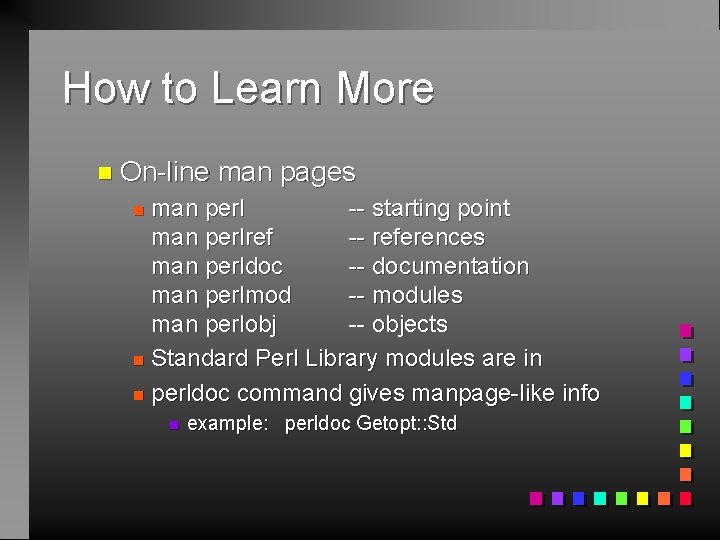
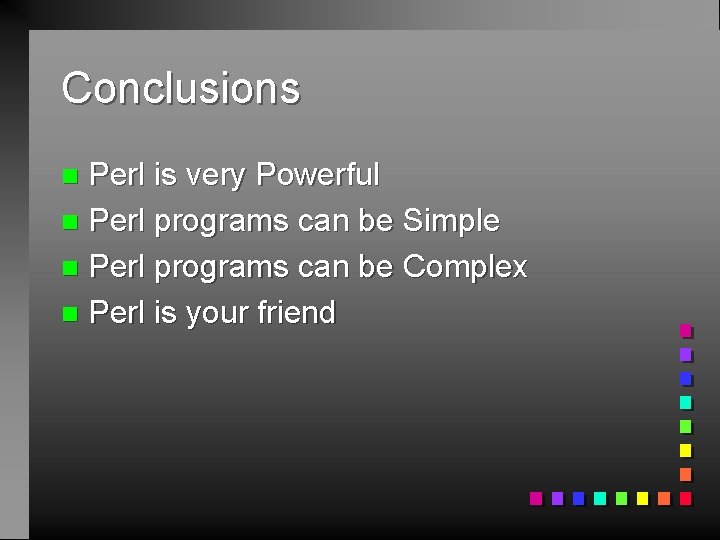
- Slides: 100
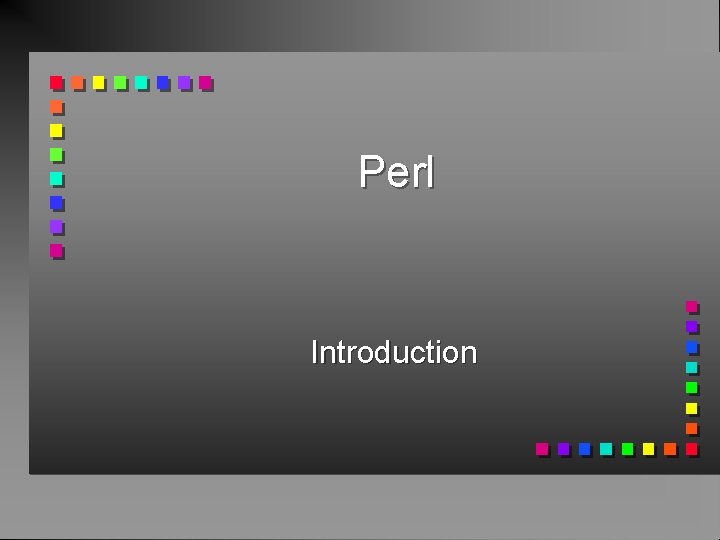
Perl Introduction
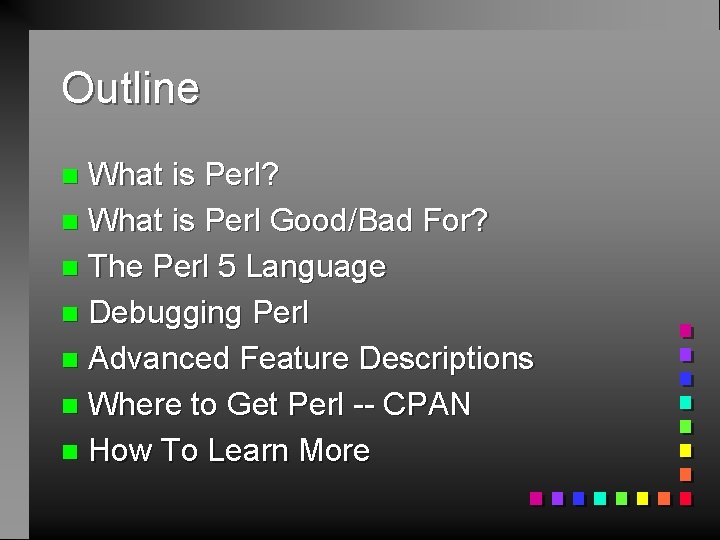
Outline What is Perl? n What is Perl Good/Bad For? n The Perl 5 Language n Debugging Perl n Advanced Feature Descriptions n Where to Get Perl -- CPAN n How To Learn More n
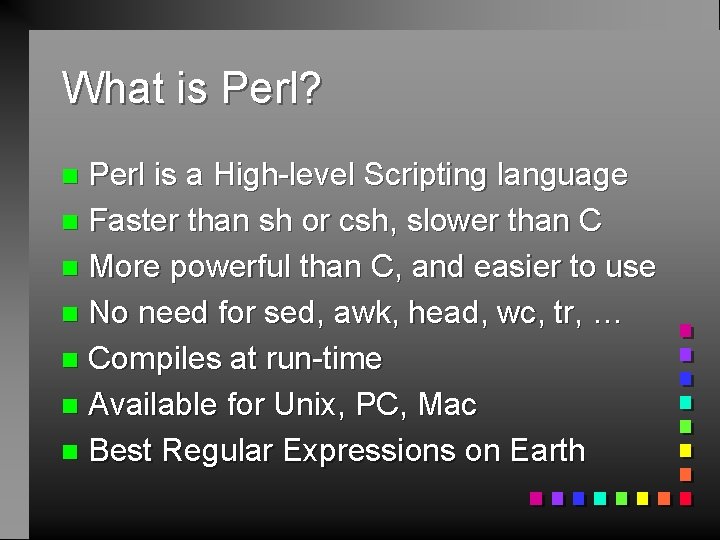
What is Perl? Perl is a High-level Scripting language n Faster than sh or csh, slower than C n More powerful than C, and easier to use n No need for sed, awk, head, wc, tr, … n Compiles at run-time n Available for Unix, PC, Mac n Best Regular Expressions on Earth n
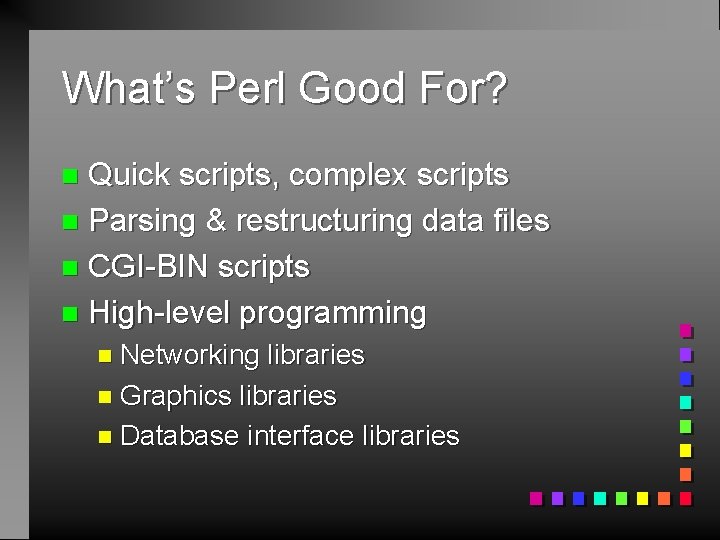
What’s Perl Good For? Quick scripts, complex scripts n Parsing & restructuring data files n CGI-BIN scripts n High-level programming n n Networking libraries n Graphics libraries n Database interface libraries
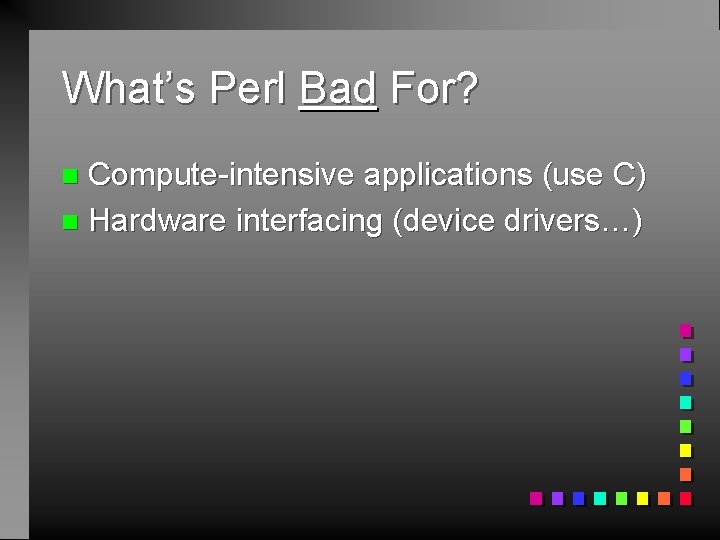
What’s Perl Bad For? Compute-intensive applications (use C) n Hardware interfacing (device drivers…) n
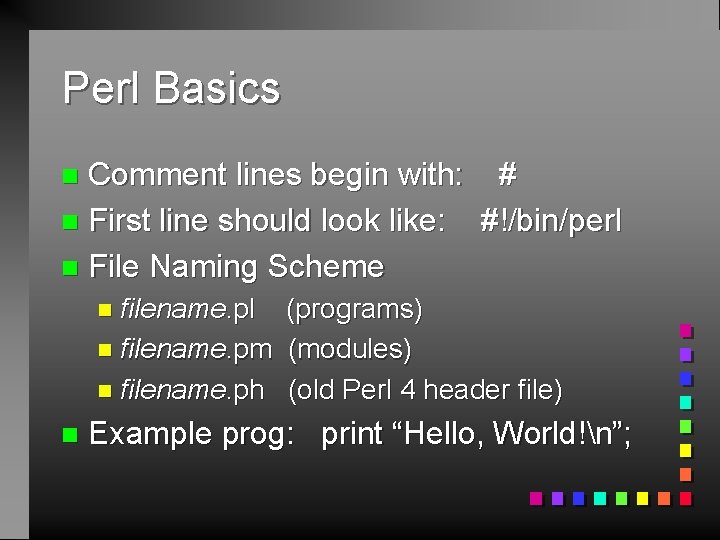
Perl Basics Comment lines begin with: # n First line should look like: #!/bin/perl n File Naming Scheme n n filename. pl (programs) n filename. pm (modules) n filename. ph (old Perl 4 header file) n Example prog: print “Hello, World!n”;
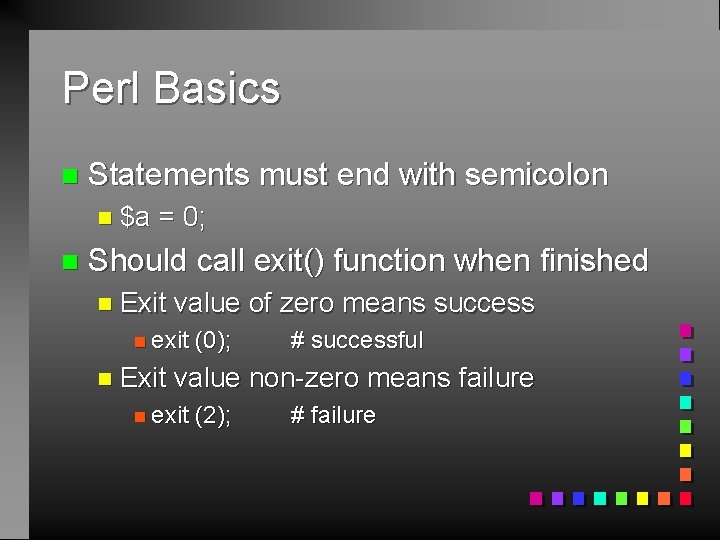
Perl Basics n Statements must end with semicolon n $a n = 0; Should call exit() function when finished n Exit value of zero means success n exit n Exit (0); # successful value non-zero means failure n exit (2); # failure
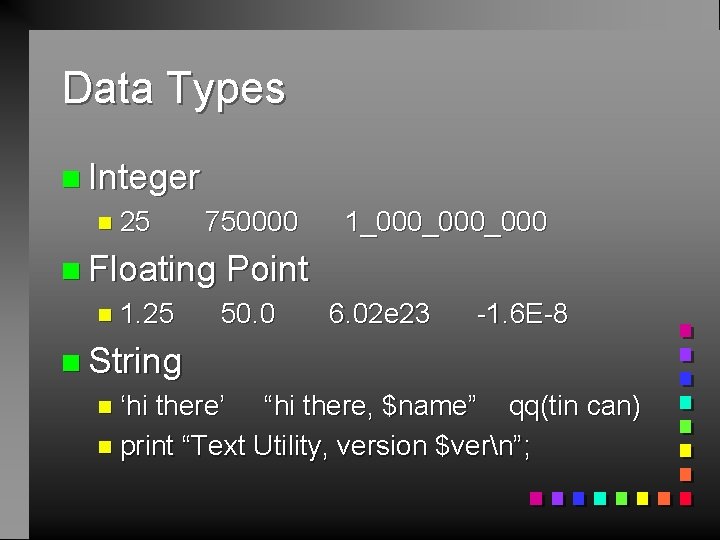
Data Types n Integer n 25 750000 1_000_000 n Floating Point n 1. 25 50. 0 6. 02 e 23 -1. 6 E-8 n String n ‘hi there’ “hi there, $name” qq(tin can) n print “Text Utility, version $vern”;
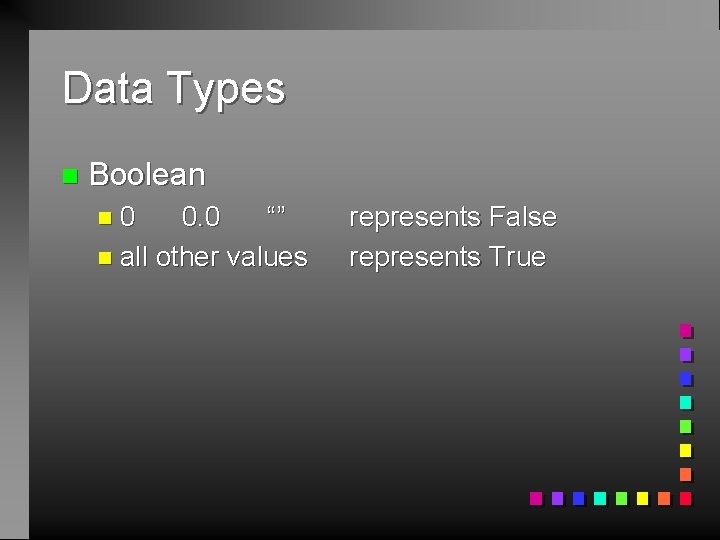
Data Types n Boolean n 0 0. 0 “” n all other values represents False represents True
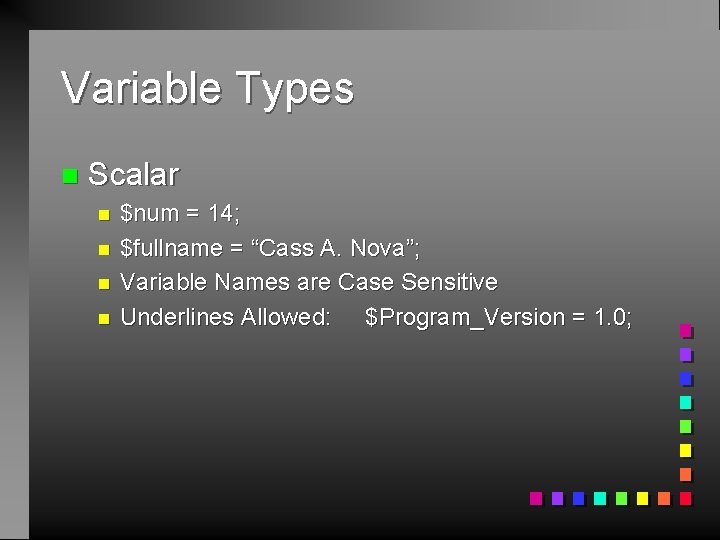
Variable Types n Scalar n n $num = 14; $fullname = “Cass A. Nova”; Variable Names are Case Sensitive Underlines Allowed: $Program_Version = 1. 0;
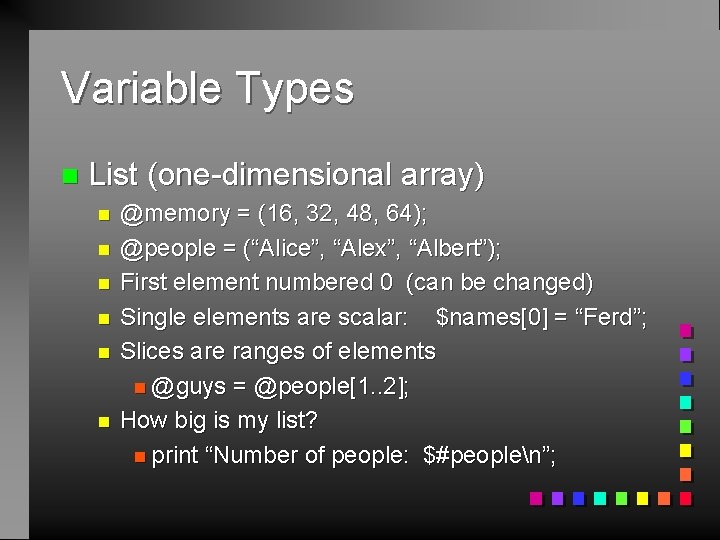
Variable Types n List (one-dimensional array) n n n @memory = (16, 32, 48, 64); @people = (“Alice”, “Alex”, “Albert”); First element numbered 0 (can be changed) Single elements are scalar: $names[0] = “Ferd”; Slices are ranges of elements n @guys = @people[1. . 2]; How big is my list? n print “Number of people: $#peoplen”;
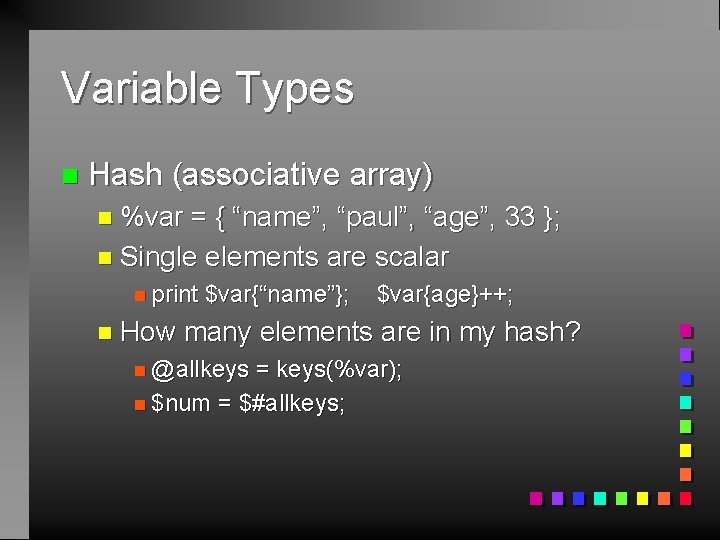
Variable Types n Hash (associative array) n %var = { “name”, “paul”, “age”, 33 }; n Single elements are scalar n print n How $var{“name”}; $var{age}++; many elements are in my hash? n @allkeys = keys(%var); n $num = $#allkeys;
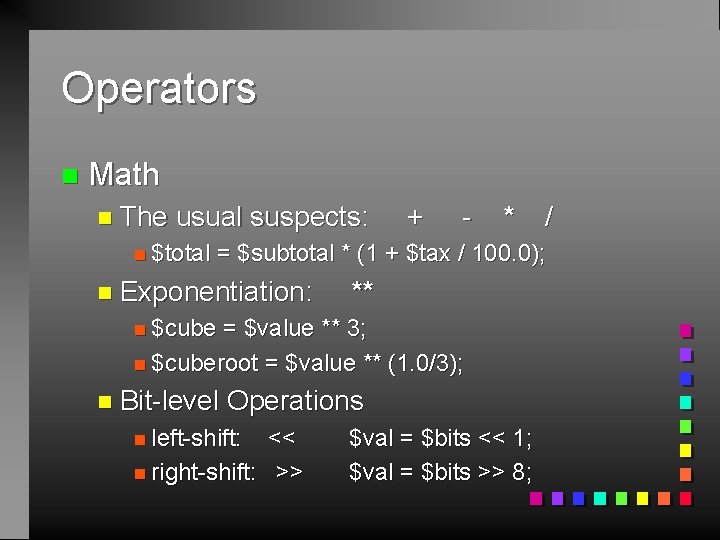
Operators n Math n The usual suspects: n $total + - * / = $subtotal * (1 + $tax / 100. 0); n Exponentiation: ** n $cube = $value ** 3; n $cuberoot = $value ** (1. 0/3); n Bit-level Operations n left-shift: << n right-shift: >> $val = $bits << 1; $val = $bits >> 8;
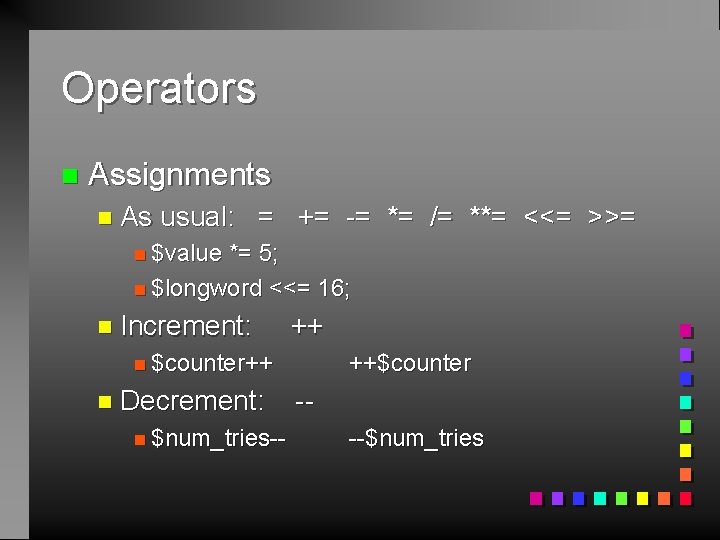
Operators n Assignments n As usual: = += -= *= /= **= <<= >>= n $value *= 5; n $longword <<= 16; n Increment: ++ n $counter++ n Decrement: n $num_tries-- ++$counter ---$num_tries
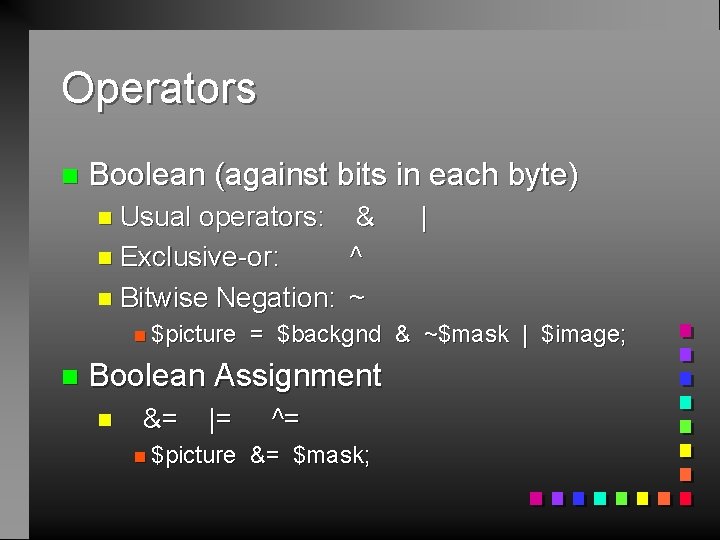
Operators n Boolean (against bits in each byte) n Usual operators: & n Exclusive-or: ^ n Bitwise Negation: ~ n $picture n = $backgnd & ~$mask | $image; Boolean Assignment n &= |= n $picture | ^= &= $mask;
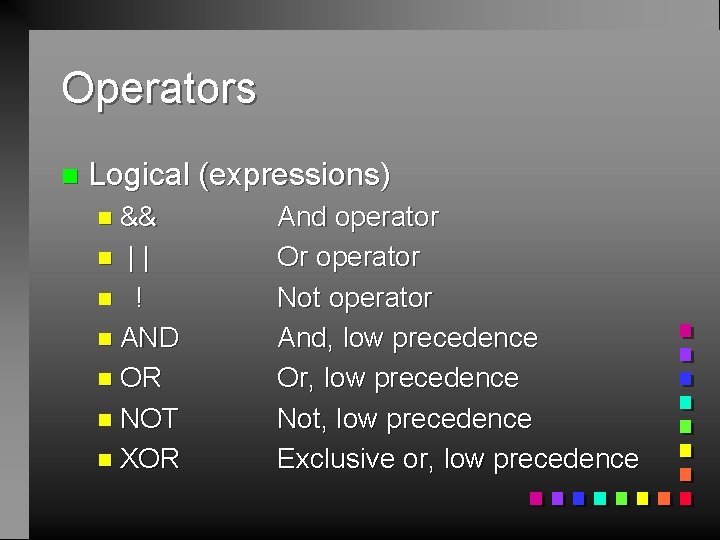
Operators n Logical (expressions) n && || n ! n AND n OR n NOT n XOR n And operator Or operator Not operator And, low precedence Or, low precedence Not, low precedence Exclusive or, low precedence
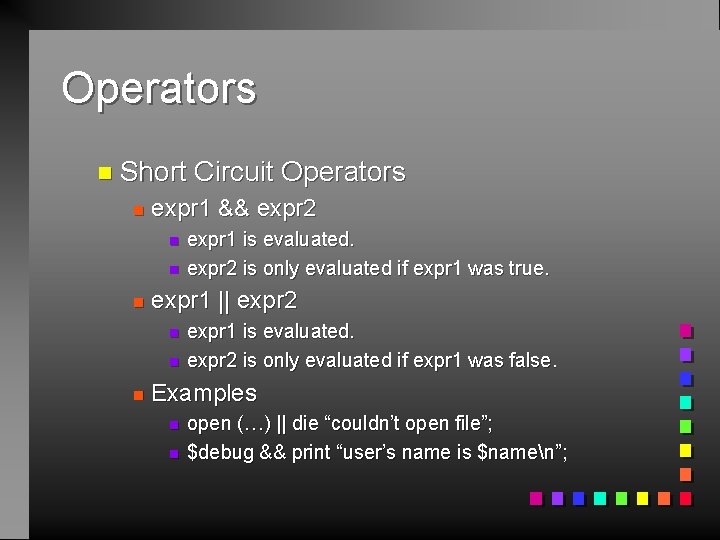
Operators n Short n expr 1 && expr 2 n n n expr 1 is evaluated. expr 2 is only evaluated if expr 1 was true. expr 1 || expr 2 n n n Circuit Operators expr 1 is evaluated. expr 2 is only evaluated if expr 1 was false. Examples n n open (…) || die “couldn’t open file”; $debug && print “user’s name is $namen”;
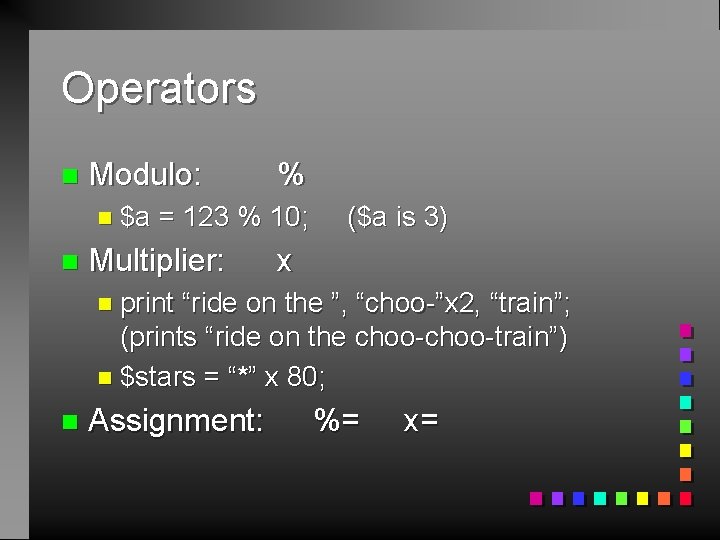
Operators n Modulo: n $a n % = 123 % 10; Multiplier: ($a is 3) x n print “ride on the ”, “choo-”x 2, “train”; (prints “ride on the choo-train”) n $stars = “*” x 80; n Assignment: %= x=
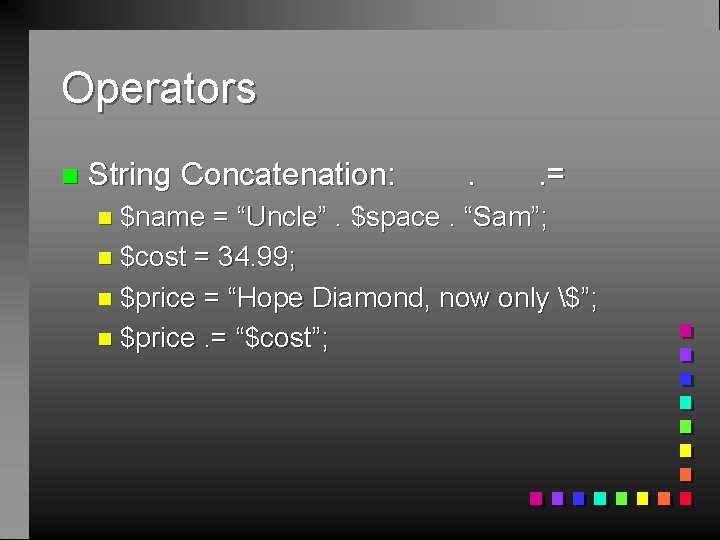
Operators n String Concatenation: n $name . . = = “Uncle”. $space. “Sam”; n $cost = 34. 99; n $price = “Hope Diamond, now only $”; n $price. = “$cost”;
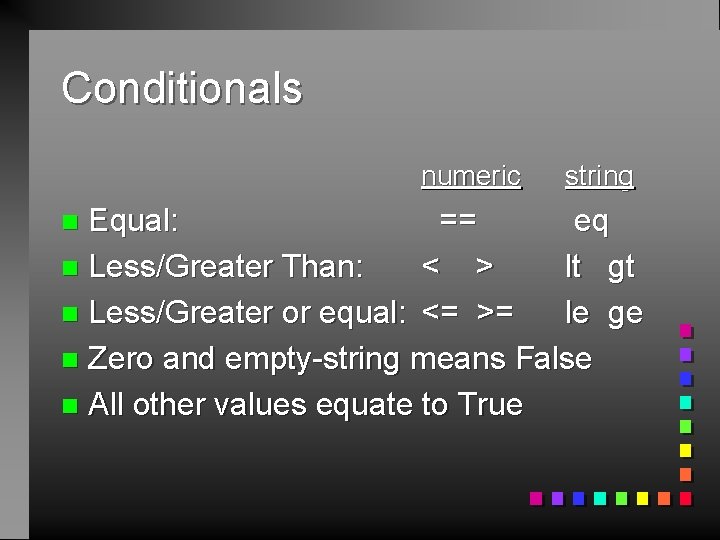
Conditionals numeric string Equal: == eq n Less/Greater Than: < > lt gt n Less/Greater or equal: <= >= le ge n Zero and empty-string means False n All other values equate to True n
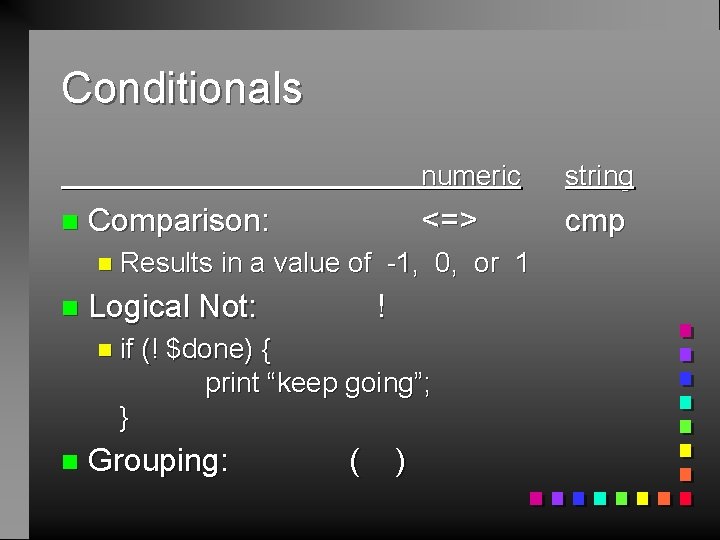
Conditionals n Comparison: n Results n <=> cmp ! (! $done) { print “keep going”; } n string in a value of -1, 0, or 1 Logical Not: n if numeric Grouping: ( )
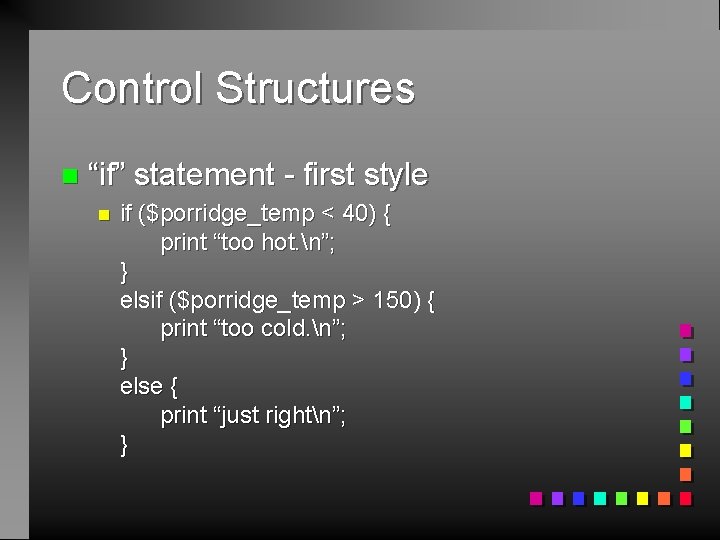
Control Structures n “if” statement - first style n if ($porridge_temp < 40) { print “too hot. n”; } elsif ($porridge_temp > 150) { print “too cold. n”; } else { print “just rightn”; }
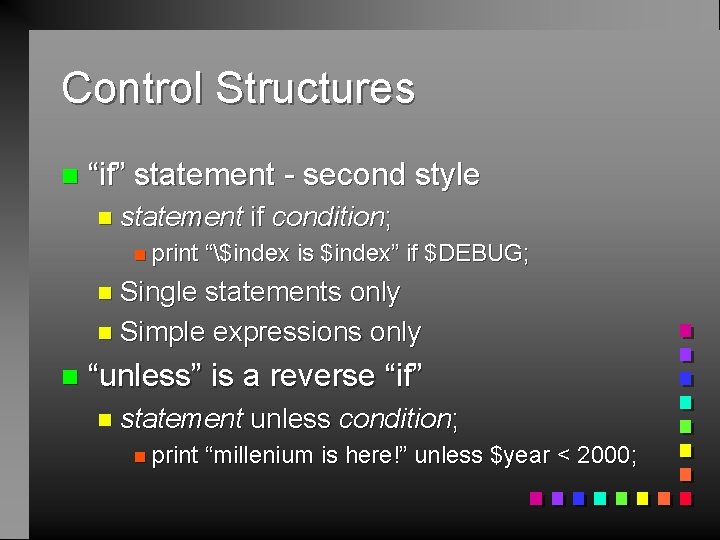
Control Structures n “if” statement - second style n statement n print if condition; “$index is $index” if $DEBUG; n Single statements only n Simple expressions only n “unless” is a reverse “if” n statement n print unless condition; “millenium is here!” unless $year < 2000;
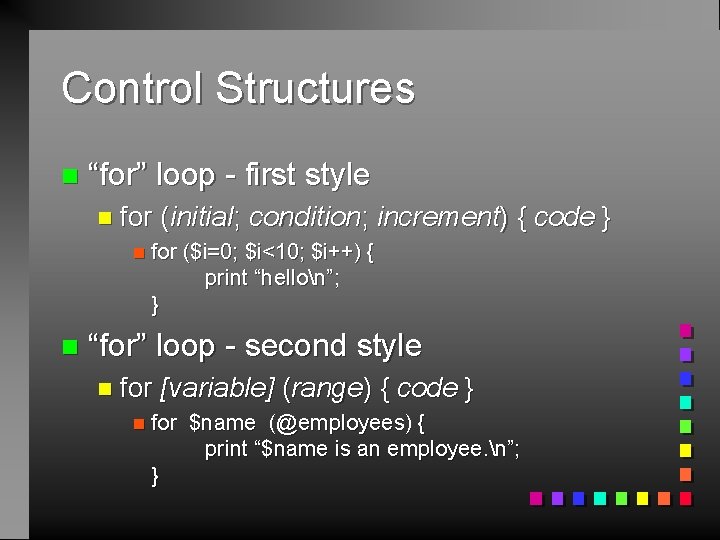
Control Structures n “for” loop - first style n for (initial; condition; increment) n for ($i=0; $i<10; $i++) { print “hellon”; } n { code } “for” loop - second style n for [variable] (range) { code } n for $name (@employees) { print “$name is an employee. n”; }
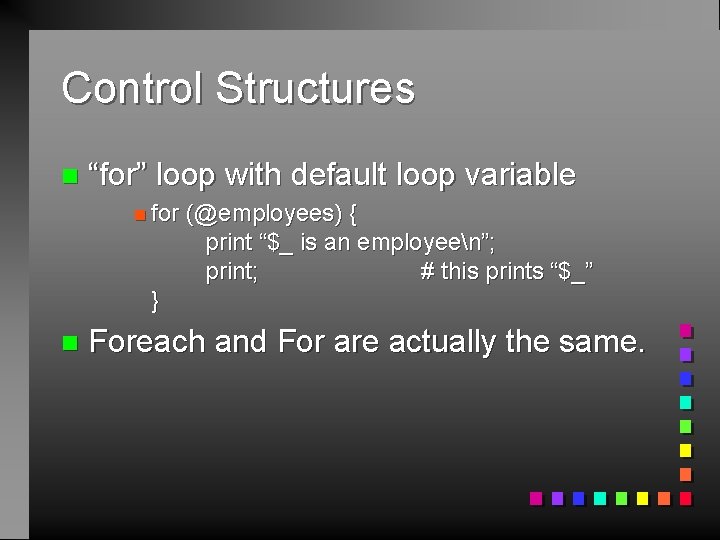
Control Structures n “for” loop with default loop variable n for (@employees) { print “$_ is an employeen”; print; # this prints “$_” } n Foreach and For are actually the same.
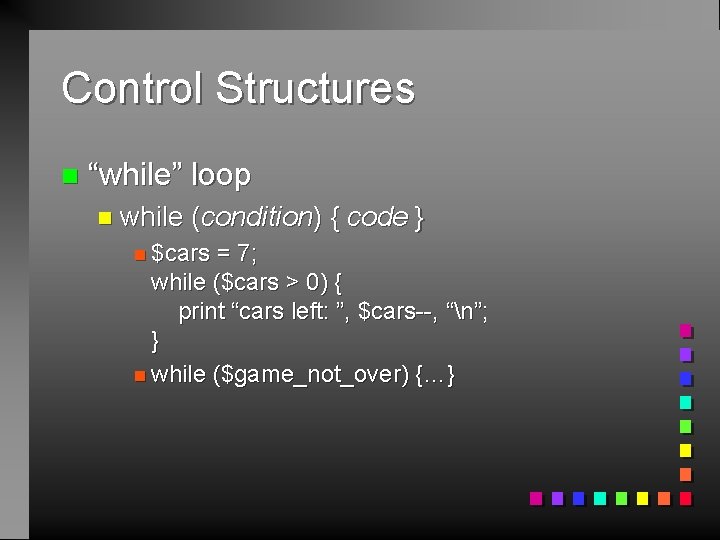
Control Structures n “while” loop n while (condition) { code } n $cars = 7; while ($cars > 0) { print “cars left: ”, $cars--, “n”; } n while ($game_not_over) {…}
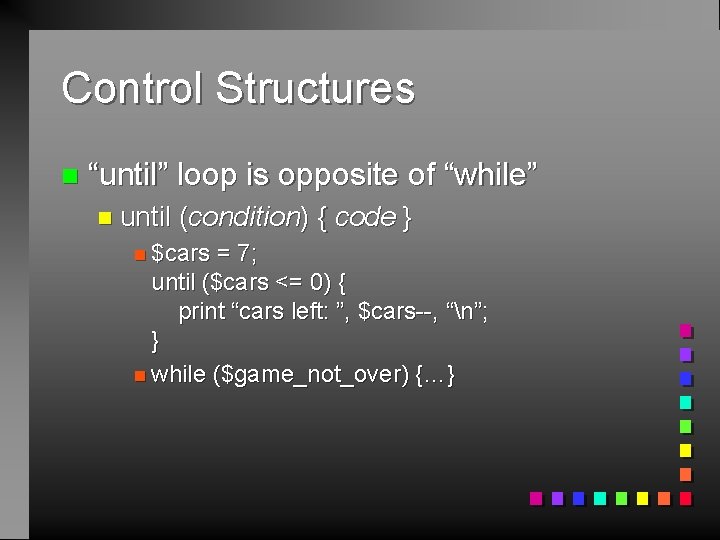
Control Structures n “until” loop is opposite of “while” n until (condition) { code } n $cars = 7; until ($cars <= 0) { print “cars left: ”, $cars--, “n”; } n while ($game_not_over) {…}
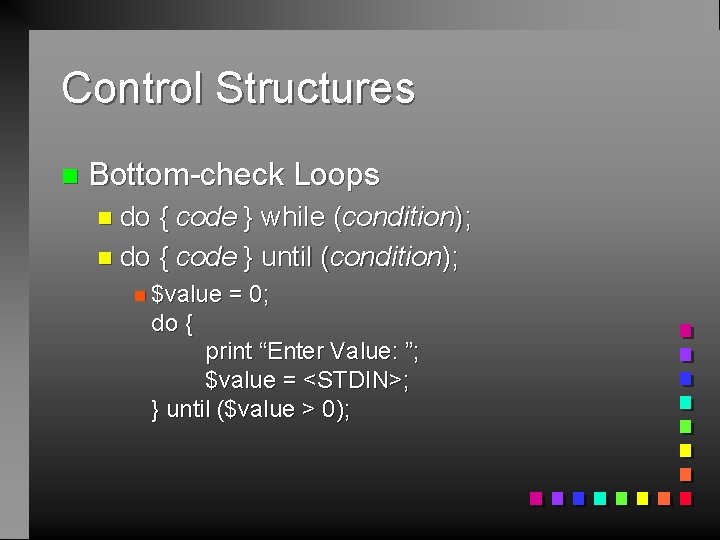
Control Structures n Bottom-check Loops n do { code } while (condition); n do { code } until (condition); n $value = 0; do { print “Enter Value: ”; $value = <STDIN>; } until ($value > 0);
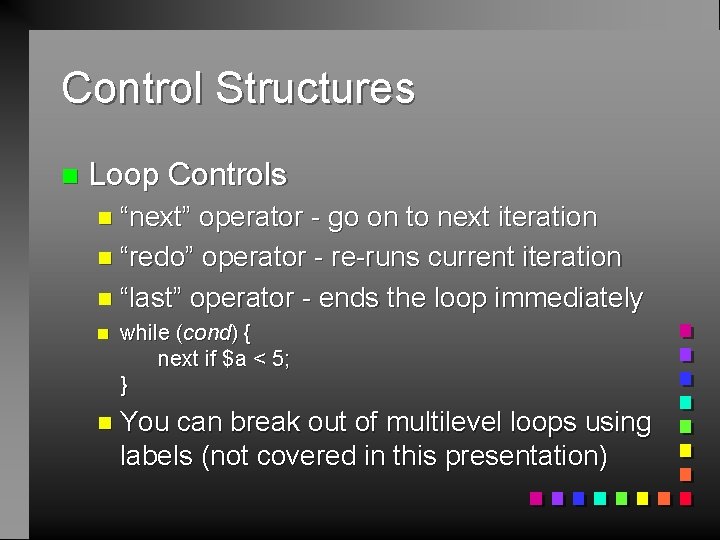
Control Structures n Loop Controls n “next” operator - go on to next iteration n “redo” operator - re-runs current iteration n “last” operator - ends the loop immediately n while (cond) { next if $a < 5; } n You can break out of multilevel loops using labels (not covered in this presentation)
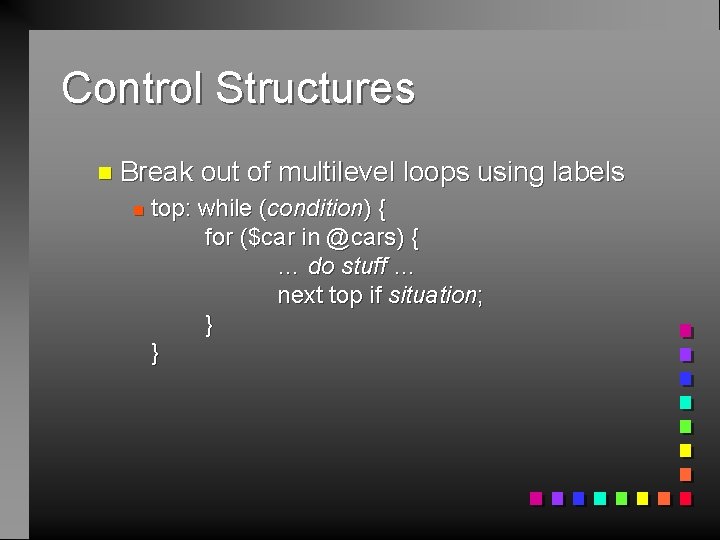
Control Structures n Break n out of multilevel loops using labels top: while (condition) { for ($car in @cars) { … do stuff … next top if situation; } }
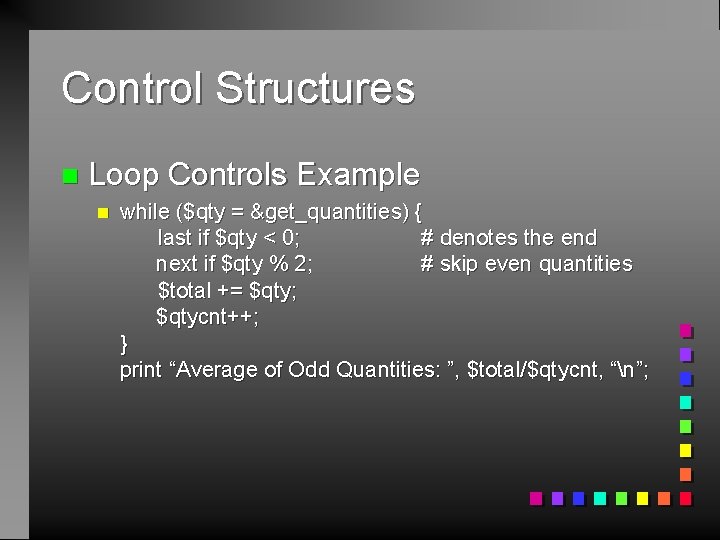
Control Structures n Loop Controls Example n while ($qty = &get_quantities) { last if $qty < 0; # denotes the end next if $qty % 2; # skip even quantities $total += $qty; $qtycnt++; } print “Average of Odd Quantities: ”, $total/$qtycnt, “n”;
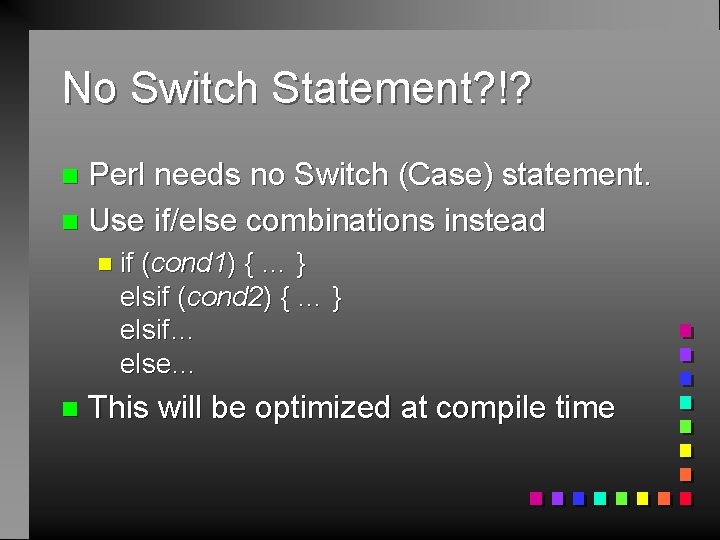
No Switch Statement? !? Perl needs no Switch (Case) statement. n Use if/else combinations instead n n if (cond 1) { … } elsif (cond 2) { … } elsif… else… n This will be optimized at compile time
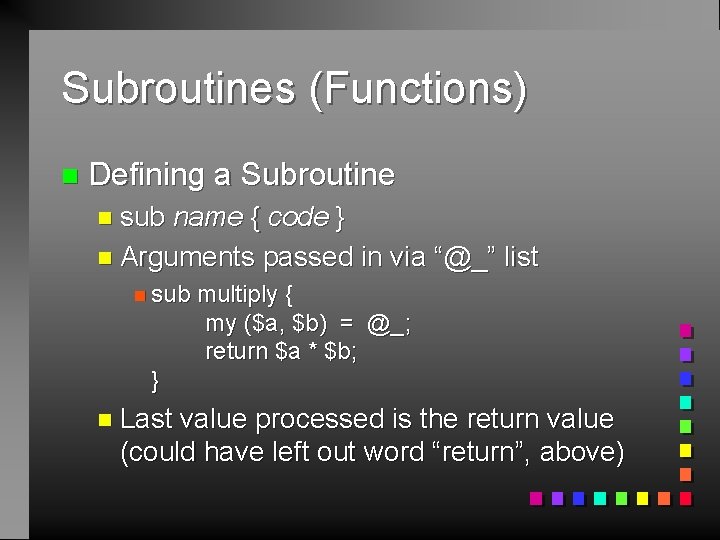
Subroutines (Functions) n Defining a Subroutine n sub name { code } n Arguments passed in via “@_” list n sub multiply { my ($a, $b) = @_; return $a * $b; } n Last value processed is the return value (could have left out word “return”, above)
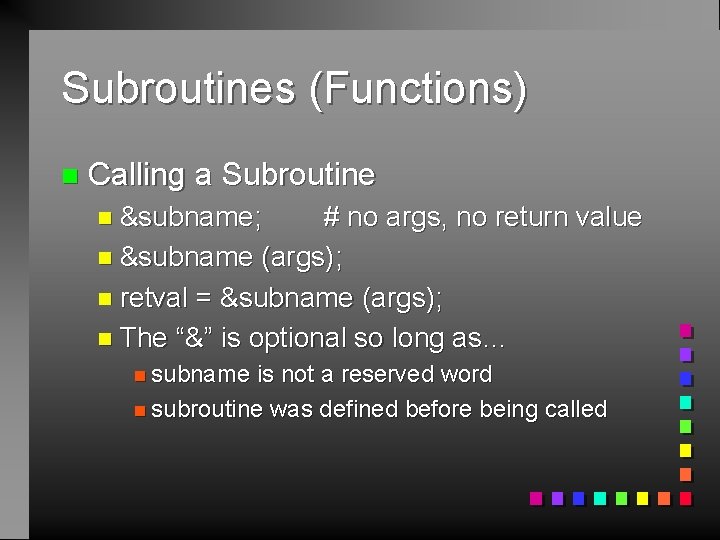
Subroutines (Functions) n Calling a Subroutine n &subname; # no args, no return value n &subname (args); n retval = &subname (args); n The “&” is optional so long as… n subname is not a reserved word n subroutine was defined before being called
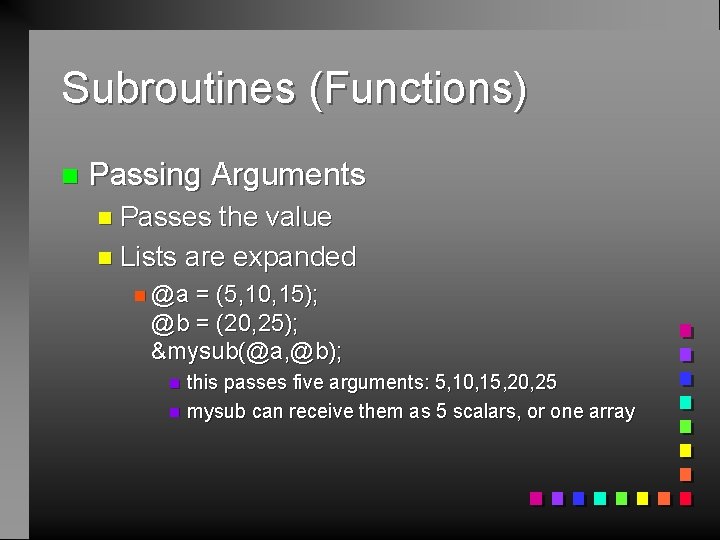
Subroutines (Functions) n Passing Arguments n Passes the value n Lists are expanded n @a = (5, 10, 15); @b = (20, 25); &mysub(@a, @b); this passes five arguments: 5, 10, 15, 20, 25 n mysub can receive them as 5 scalars, or one array n
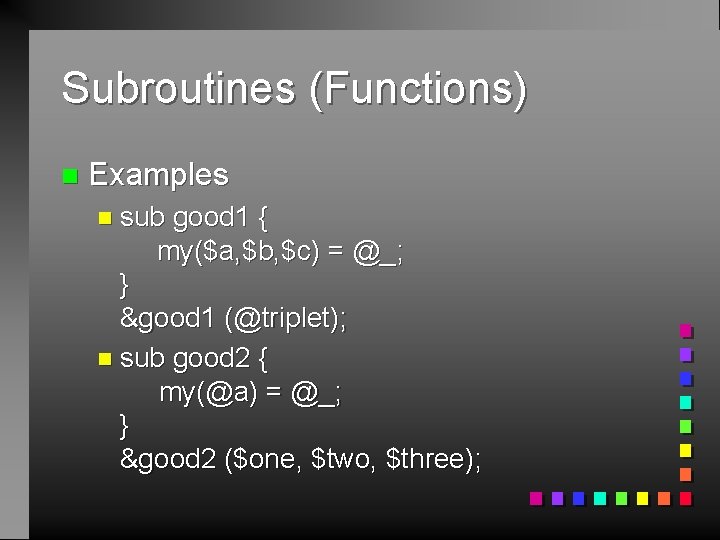
Subroutines (Functions) n Examples n sub good 1 { my($a, $b, $c) = @_; } &good 1 (@triplet); n sub good 2 { my(@a) = @_; } &good 2 ($one, $two, $three);
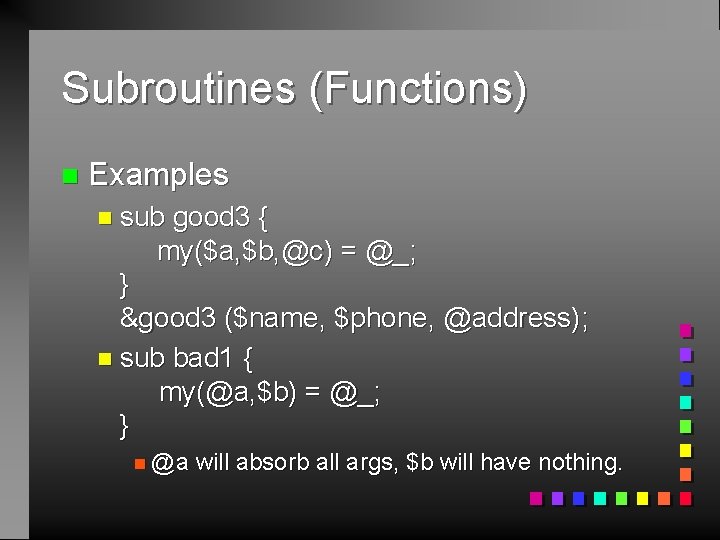
Subroutines (Functions) n Examples n sub good 3 { my($a, $b, @c) = @_; } &good 3 ($name, $phone, @address); n sub bad 1 { my(@a, $b) = @_; } n @a will absorb all args, $b will have nothing.
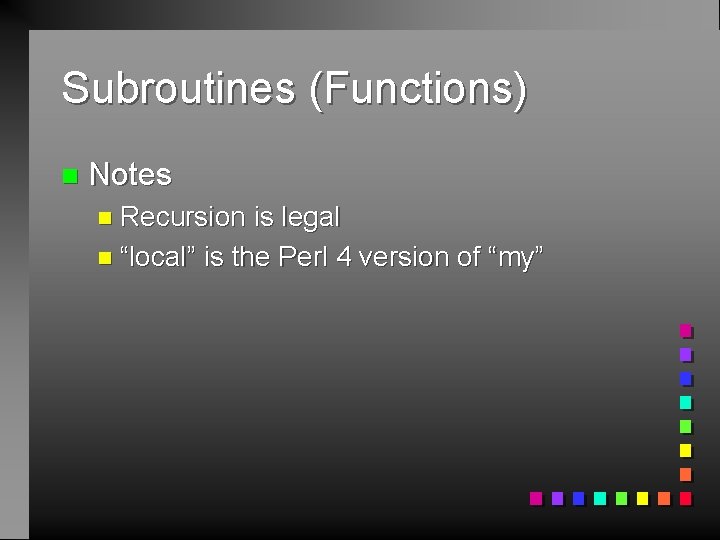
Subroutines (Functions) n Notes n Recursion is legal n “local” is the Perl 4 version of “my”
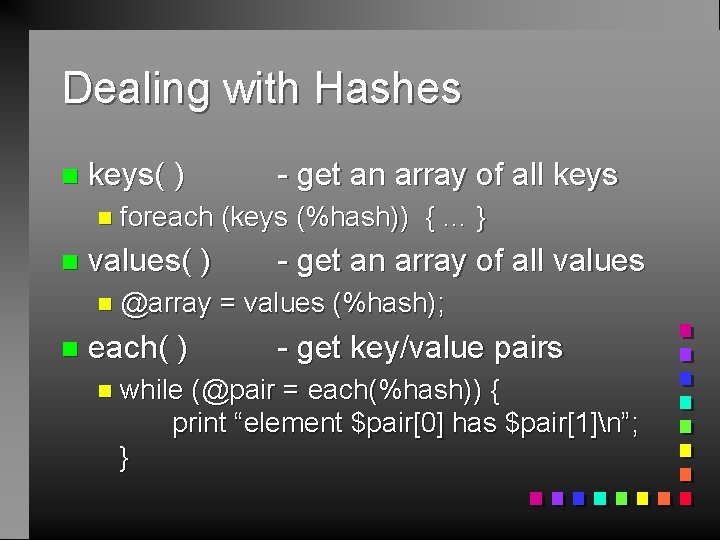
Dealing with Hashes n keys( ) n foreach n values( ) n @array n each( ) n while - get an array of all keys (%hash)) { … } - get an array of all values = values (%hash); - get key/value pairs (@pair = each(%hash)) { print “element $pair[0] has $pair[1]n”; }
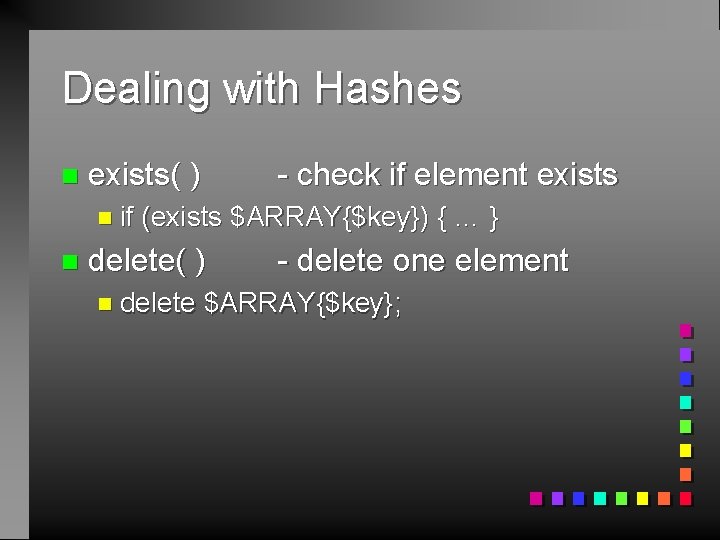
Dealing with Hashes n exists( ) n if n - check if element exists (exists $ARRAY{$key}) { … } delete( ) n delete - delete one element $ARRAY{$key};
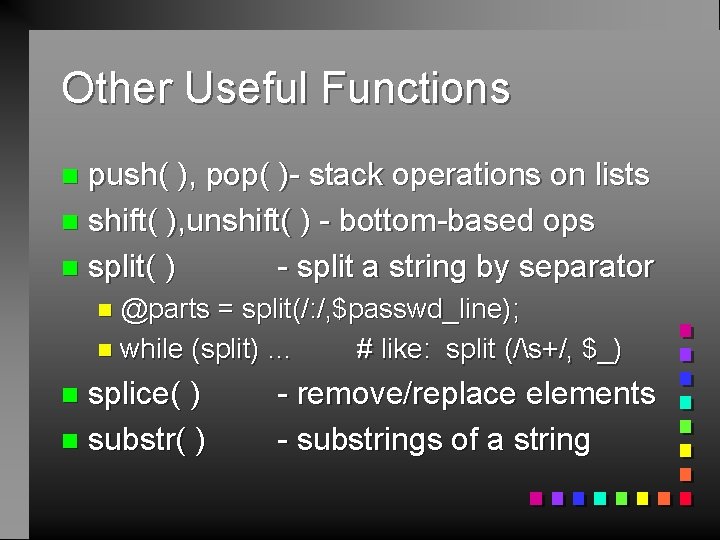
Other Useful Functions push( ), pop( )- stack operations on lists n shift( ), unshift( ) - bottom-based ops n split( ) - split a string by separator n n @parts = split(/: /, $passwd_line); n while (split) … # like: split (/s+/, $_) splice( ) n substr( ) n - remove/replace elements - substrings of a string
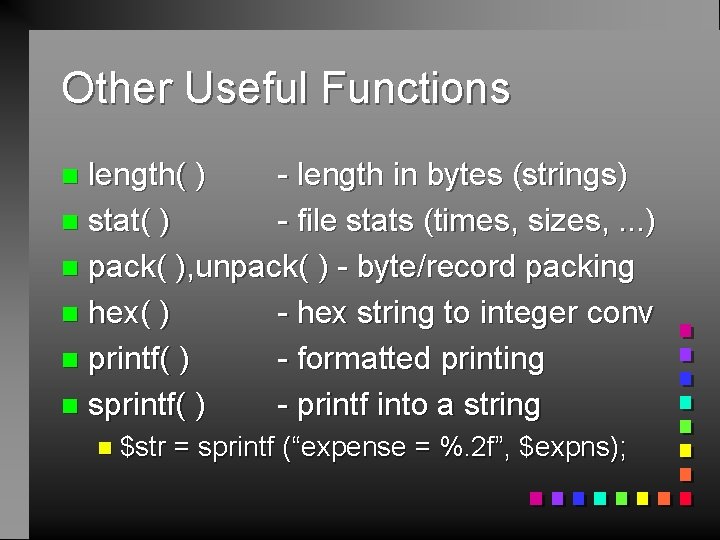
Other Useful Functions length( ) - length in bytes (strings) n stat( ) - file stats (times, sizes, . . . ) n pack( ), unpack( ) - byte/record packing n hex( ) - hex string to integer conv n printf( ) - formatted printing n sprintf( ) - printf into a string n n $str = sprintf (“expense = %. 2 f”, $expns);
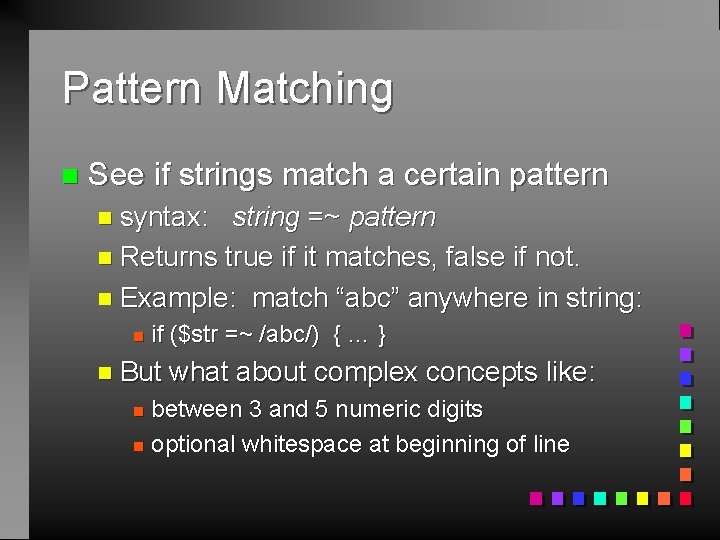
Pattern Matching n See if strings match a certain pattern n syntax: string =~ pattern n Returns true if it matches, false if not. n Example: match “abc” anywhere in string: n if ($str =~ /abc/) { … } n But what about complex concepts like: between 3 and 5 numeric digits n optional whitespace at beginning of line n
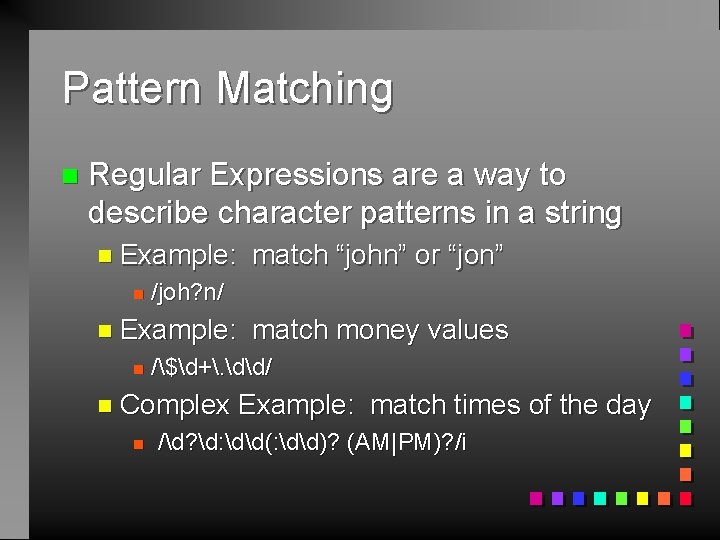
Pattern Matching n Regular Expressions are a way to describe character patterns in a string n Example: n /joh? n/ n Example: n match money values /$d+. dd/ n Complex n match “john” or “jon” Example: match times of the day /d? d: dd(: dd)? (AM|PM)? /i
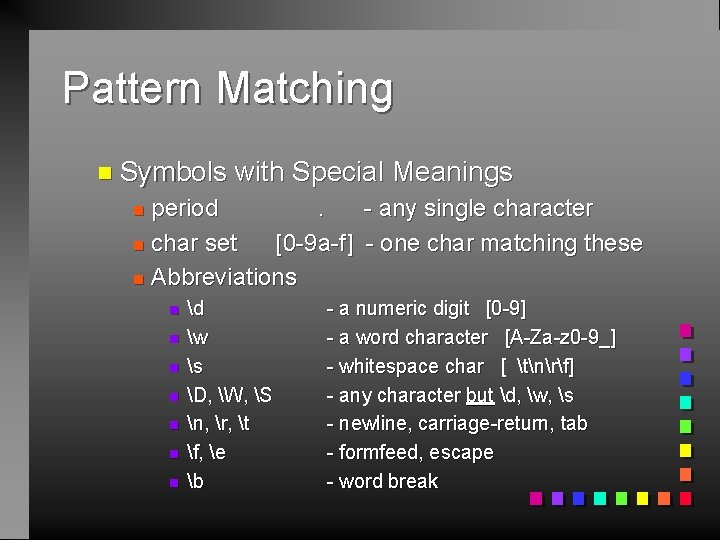
Pattern Matching n Symbols with Special Meanings period. - any single character n char set [0 -9 a-f] - one char matching these n Abbreviations n n n n d w s D, W, S n, r, t f, e b - a numeric digit [0 -9] - a word character [A-Za-z 0 -9_] - whitespace char [ tnrf] - any character but d, w, s - newline, carriage-return, tab - formfeed, escape - word break
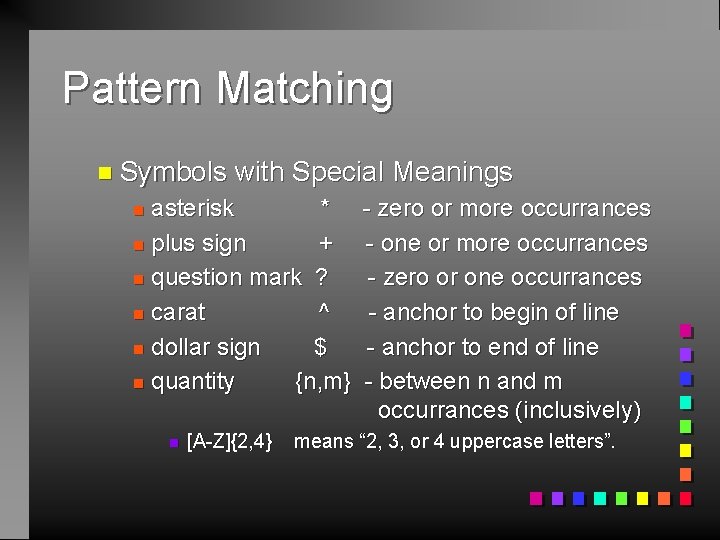
Pattern Matching n Symbols with Special Meanings asterisk * n plus sign + n question mark ? n carat ^ n dollar sign $ n quantity {n, m} n n [A-Z]{2, 4} - zero or more occurrances - one or more occurrances - zero or one occurrances - anchor to begin of line - anchor to end of line - between n and m occurrances (inclusively) means “ 2, 3, or 4 uppercase letters”.
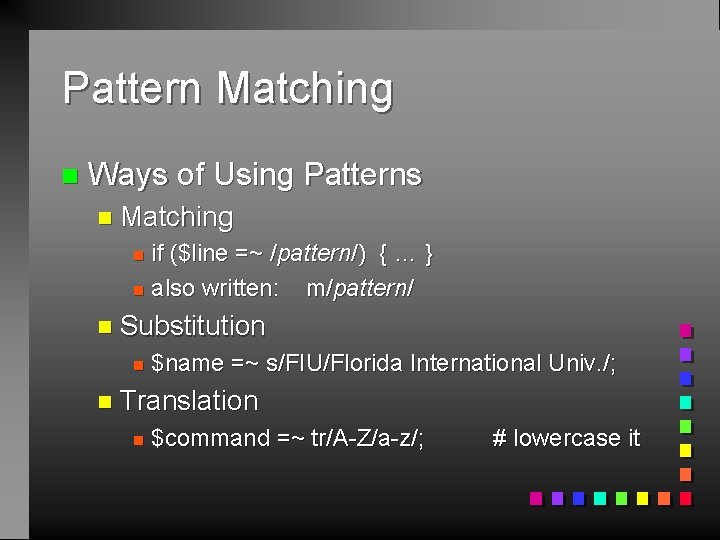
Pattern Matching n Ways of Using Patterns n Matching if ($line =~ /pattern/) { … } n also written: m/pattern/ n n Substitution n $name =~ s/FIU/Florida International Univ. /; n Translation n $command =~ tr/A-Z/a-z/; # lowercase it
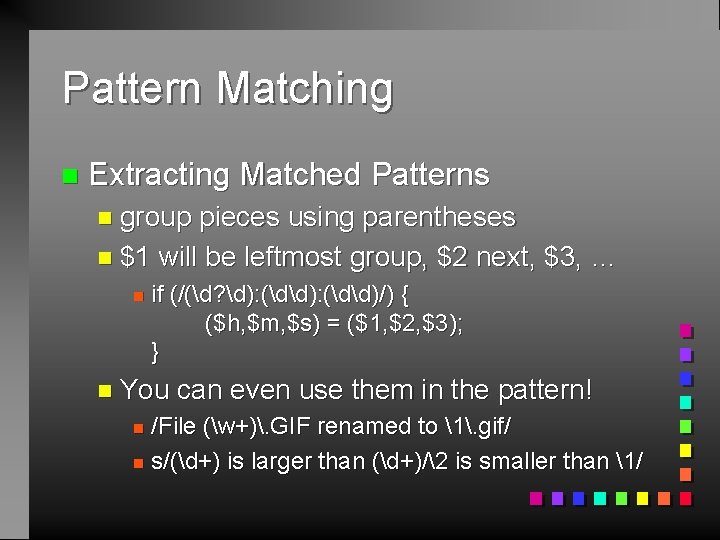
Pattern Matching n Extracting Matched Patterns n group pieces using parentheses n $1 will be leftmost group, $2 next, $3, … n if (/(d? d): (dd)/) { ($h, $m, $s) = ($1, $2, $3); } n You can even use them in the pattern! /File (w+). GIF renamed to 1. gif/ n s/(d+) is larger than (d+)/2 is smaller than 1/ n
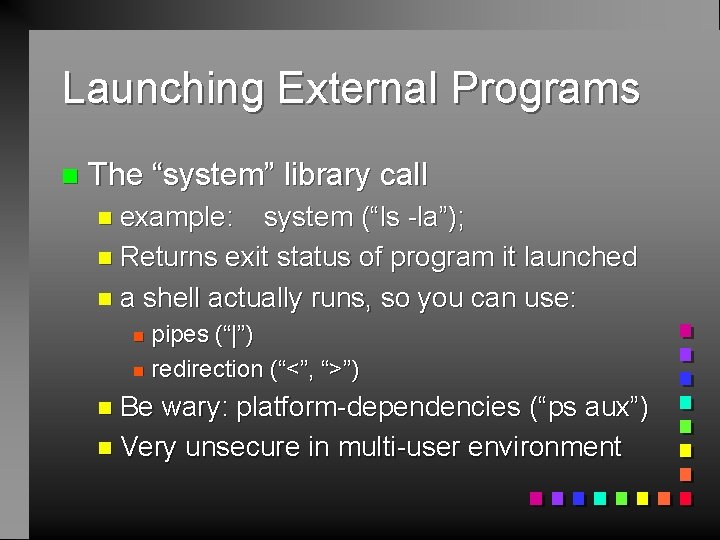
Launching External Programs n The “system” library call n example: system (“ls -la”); n Returns exit status of program it launched n a shell actually runs, so you can use: pipes (“|”) n redirection (“<”, “>”) n n Be wary: platform-dependencies (“ps aux”) n Very unsecure in multi-user environment
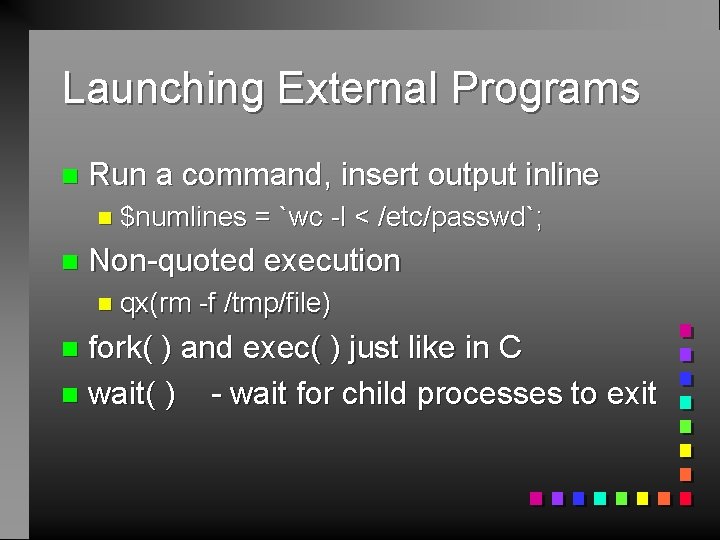
Launching External Programs n Run a command, insert output inline n $numlines n = `wc -l < /etc/passwd`; Non-quoted execution n qx(rm -f /tmp/file) fork( ) and exec( ) just like in C n wait( ) - wait for child processes to exit n
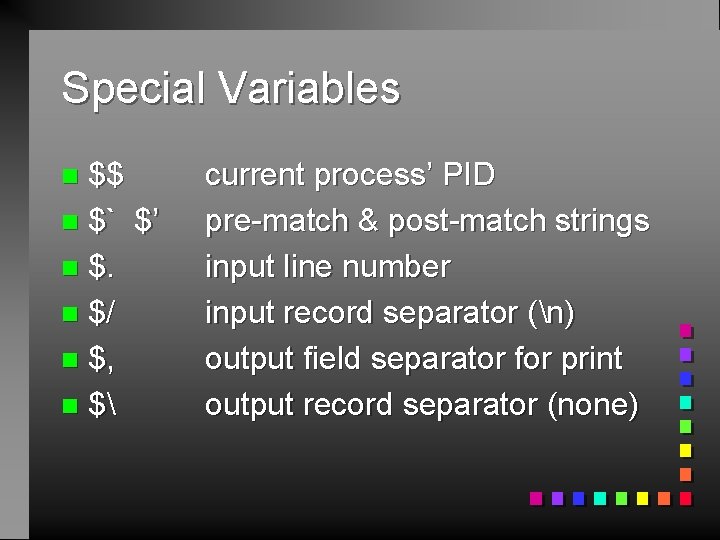
Special Variables $$ n $` $’ n $/ n $, n $ n current process’ PID pre-match & post-match strings input line number input record separator (n) output field separator for print output record separator (none)
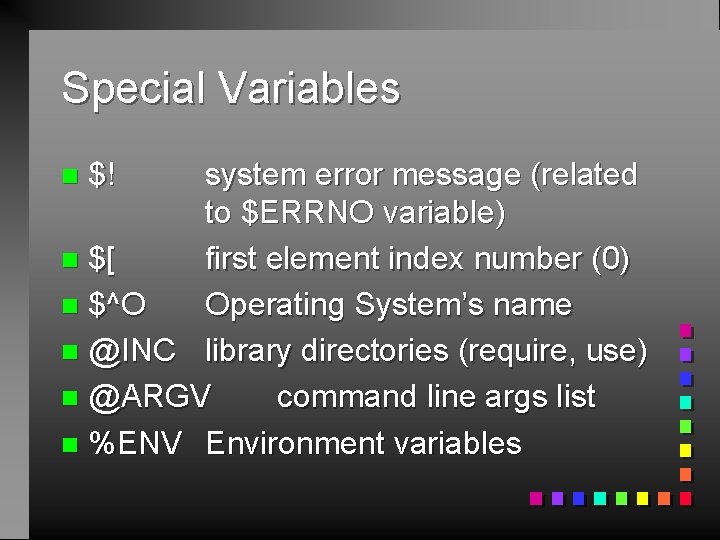
Special Variables system error message (related to $ERRNO variable) n $[ first element index number (0) n $^O Operating System’s name n @INC library directories (require, use) n @ARGV command line args list n %ENV Environment variables n $!
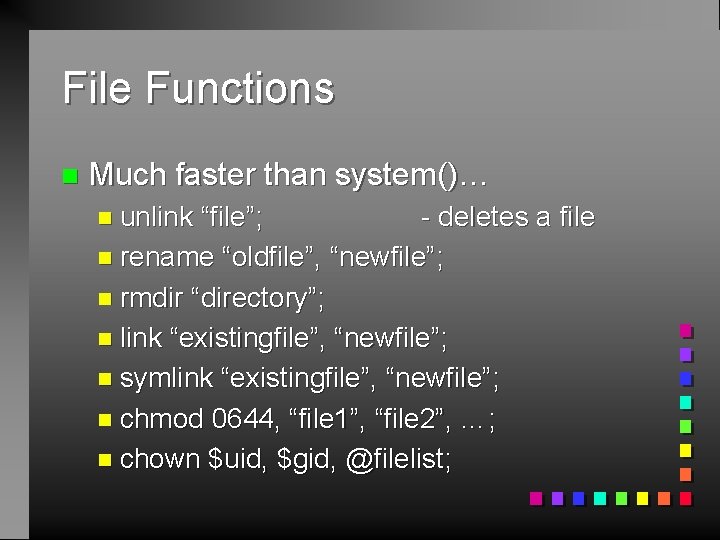
File Functions n Much faster than system()… n unlink “file”; - deletes a file n rename “oldfile”, “newfile”; n rmdir “directory”; n link “existingfile”, “newfile”; n symlink “existingfile”, “newfile”; n chmod 0644, “file 1”, “file 2”, …; n chown $uid, $gid, @filelist;
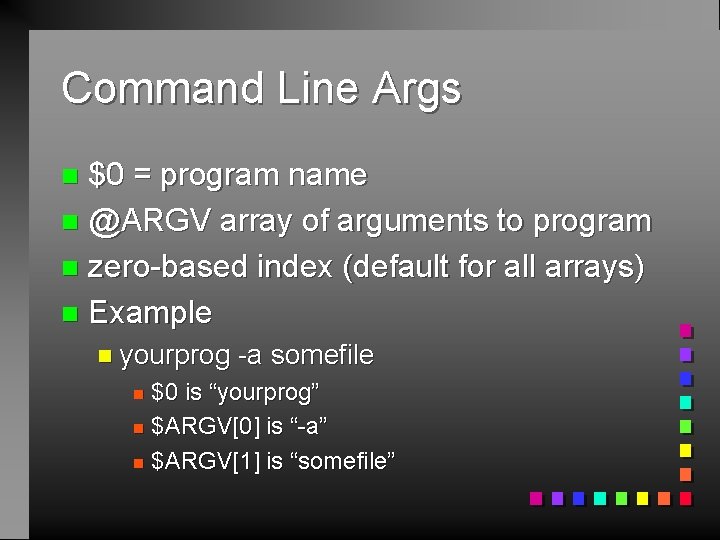
Command Line Args $0 = program name n @ARGV array of arguments to program n zero-based index (default for all arrays) n Example n n yourprog -a somefile $0 is “yourprog” n $ARGV[0] is “-a” n $ARGV[1] is “somefile” n
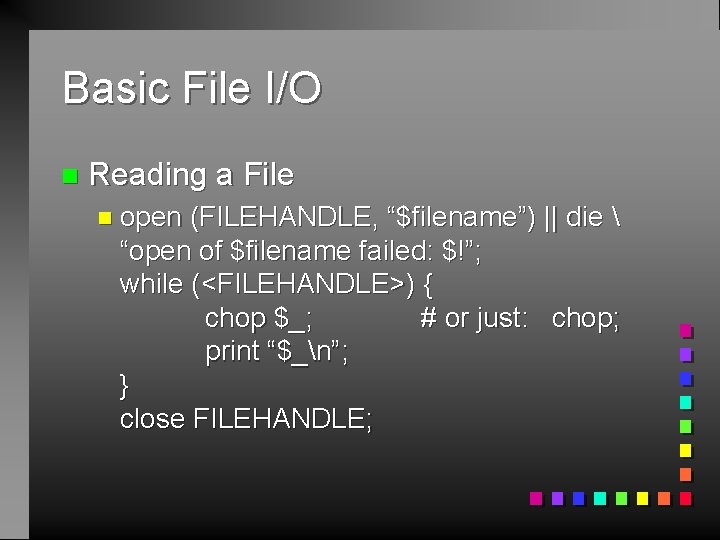
Basic File I/O n Reading a File n open (FILEHANDLE, “$filename”) || die “open of $filename failed: $!”; while (<FILEHANDLE>) { chop $_; # or just: chop; print “$_n”; } close FILEHANDLE;
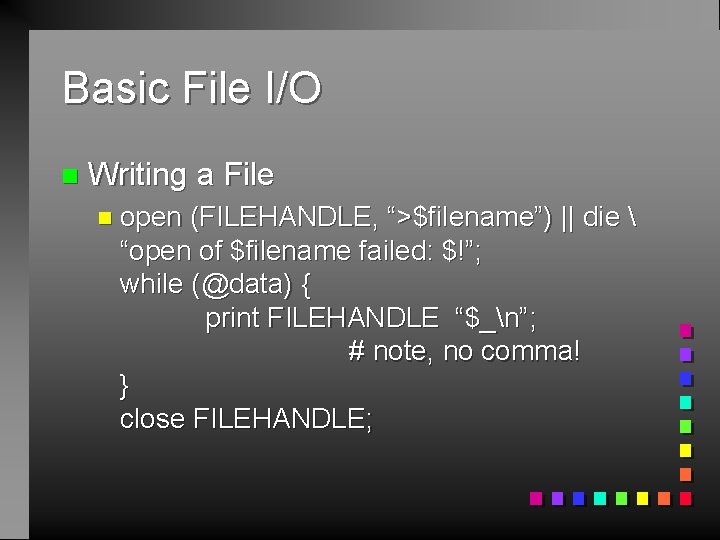
Basic File I/O n Writing a File n open (FILEHANDLE, “>$filename”) || die “open of $filename failed: $!”; while (@data) { print FILEHANDLE “$_n”; # note, no comma! } close FILEHANDLE;
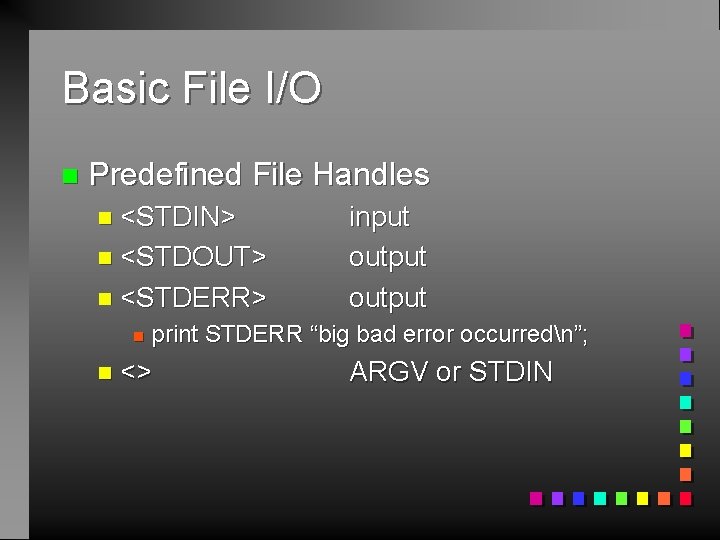
Basic File I/O n Predefined File Handles n <STDIN> n <STDOUT> n <STDERR> n input output print STDERR “big bad error occurredn”; n <> ARGV or STDIN
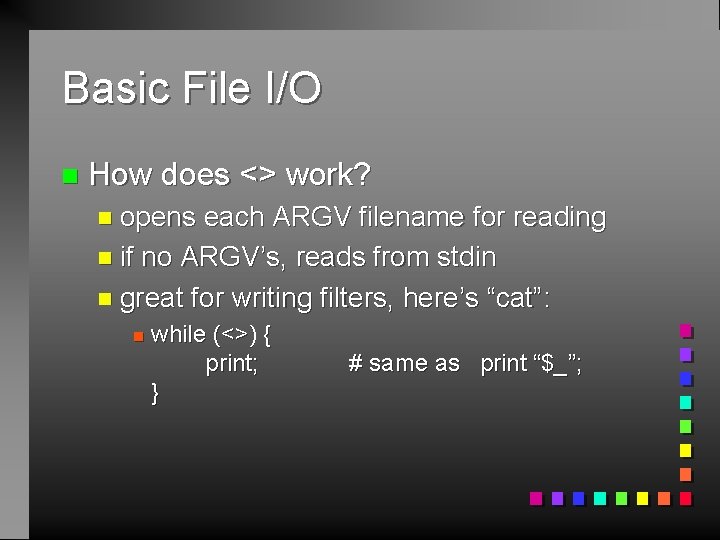
Basic File I/O n How does <> work? n opens each ARGV filename for reading n if no ARGV’s, reads from stdin n great for writing filters, here’s “cat”: n while (<>) { print; } # same as print “$_”;
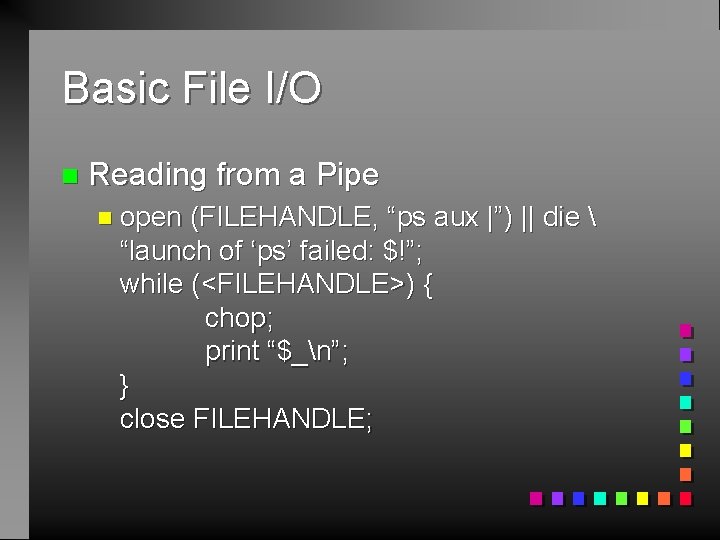
Basic File I/O n Reading from a Pipe n open (FILEHANDLE, “ps aux |”) || die “launch of ‘ps’ failed: $!”; while (<FILEHANDLE>) { chop; print “$_n”; } close FILEHANDLE;
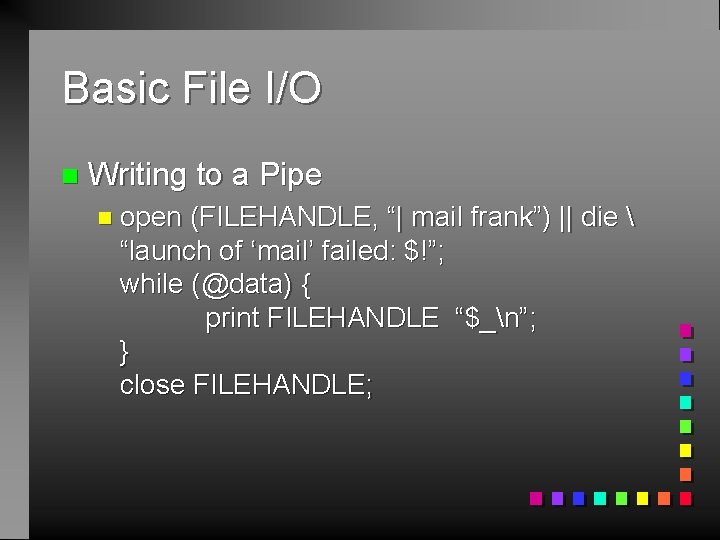
Basic File I/O n Writing to a Pipe n open (FILEHANDLE, “| mail frank”) || die “launch of ‘mail’ failed: $!”; while (@data) { print FILEHANDLE “$_n”; } close FILEHANDLE;
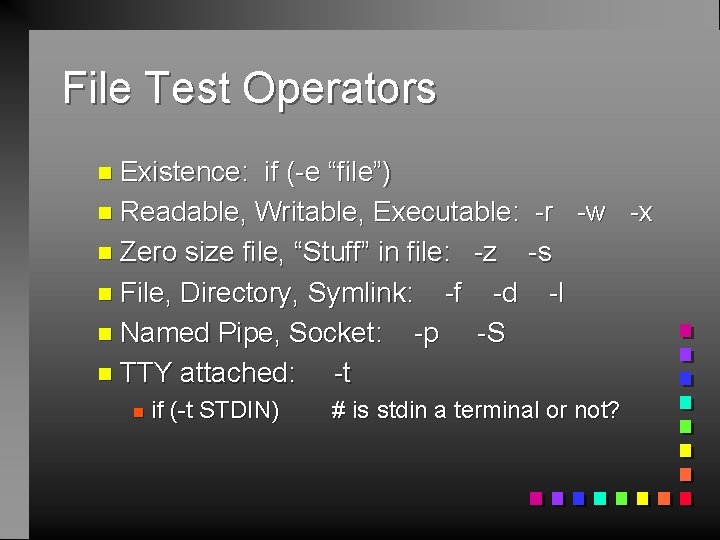
File Test Operators n Existence: if (-e “file”) n Readable, Writable, Executable: -r -w -x n Zero size file, “Stuff” in file: -z -s n File, Directory, Symlink: -f -d -l n Named Pipe, Socket: -p -S n TTY attached: -t n if (-t STDIN) # is stdin a terminal or not?
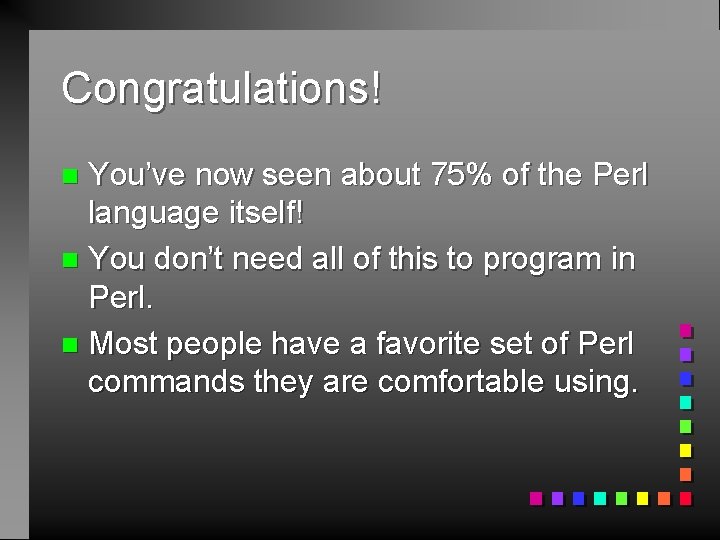
Congratulations! You’ve now seen about 75% of the Perl language itself! n You don’t need all of this to program in Perl. n Most people have a favorite set of Perl commands they are comfortable using. n
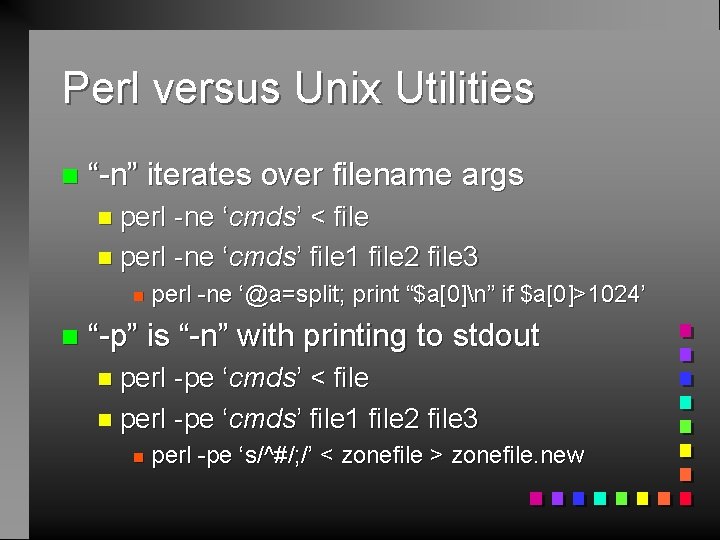
Perl versus Unix Utilities n “-n” iterates over filename args n perl -ne ‘cmds’ < file n perl -ne ‘cmds’ file 1 file 2 file 3 n n perl -ne ‘@a=split; print “$a[0]n” if $a[0]>1024’ “-p” is “-n” with printing to stdout n perl -pe ‘cmds’ < file n perl -pe ‘cmds’ file 1 file 2 file 3 n perl -pe ‘s/^#/; /’ < zonefile > zonefile. new
![Perl versus Unix Utilities n Whats n perl ne asplit print a0n if a01024 Perl versus Unix Utilities n What’s n perl -ne ‘@a=split; print “$a[0]n” if $a[0]>1024’](https://slidetodoc.com/presentation_image_h2/59e30b6cb5887a59386ef47bfaf7304e/image-64.jpg)
Perl versus Unix Utilities n What’s n perl -ne ‘@a=split; print “$a[0]n” if $a[0]>1024’ n …is n that again? the same as this program: #!/bin/perl while (<>) { @a=split; if ($a[0] > 1024) { print “$a[0]n”; } }
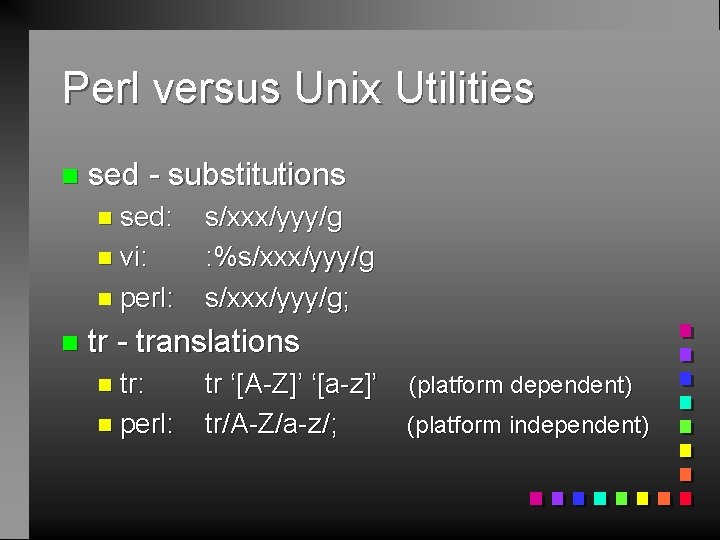
Perl versus Unix Utilities n sed - substitutions n sed: n vi: n perl: n s/xxx/yyy/g : %s/xxx/yyy/g; tr - translations n tr: n perl: tr ‘[A-Z]’ ‘[a-z]’ tr/A-Z/a-z/; (platform dependent) (platform independent)
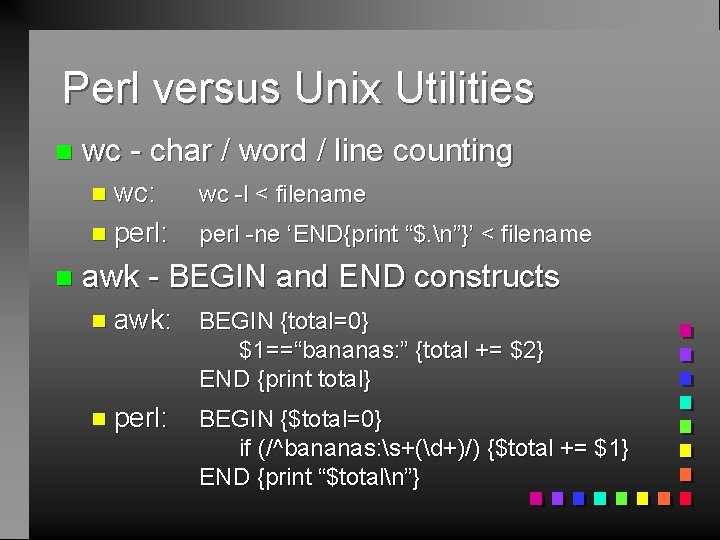
Perl versus Unix Utilities n n wc - char / word / line counting n wc: wc -l < filename n perl: perl -ne ‘END{print “$. n”}’ < filename awk - BEGIN and END constructs n awk: BEGIN {total=0} $1==“bananas: ” {total += $2} END {print total} n perl: BEGIN {$total=0} if (/^bananas: s+(d+)/) {$total += $1} END {print “$totaln”}
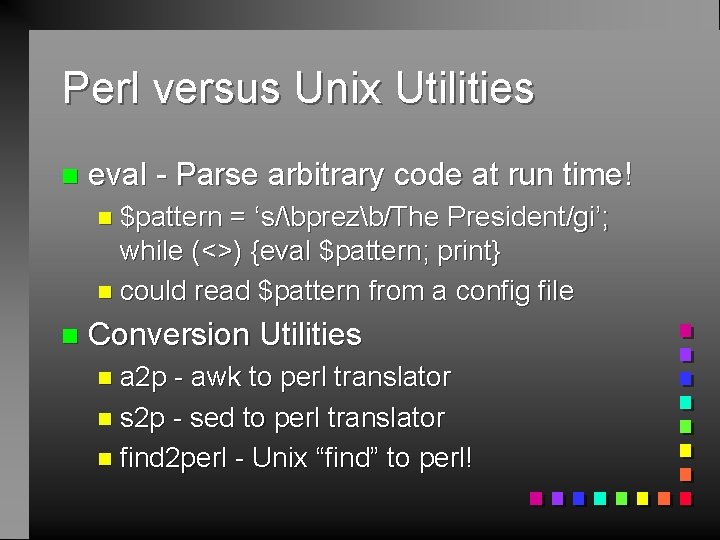
Perl versus Unix Utilities n eval - Parse arbitrary code at run time! n $pattern = ‘s/bprezb/The President/gi’; while (<>) {eval $pattern; print} n could read $pattern from a config file n Conversion Utilities n a 2 p - awk to perl translator n s 2 p - sed to perl translator n find 2 perl - Unix “find” to perl!
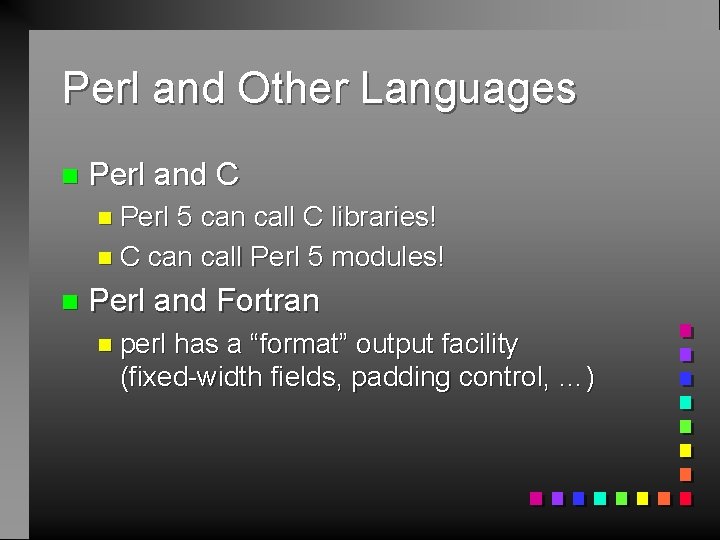
Perl and Other Languages n Perl and C n Perl 5 can call C libraries! n C can call Perl 5 modules! n Perl and Fortran n perl has a “format” output facility (fixed-width fields, padding control, …)
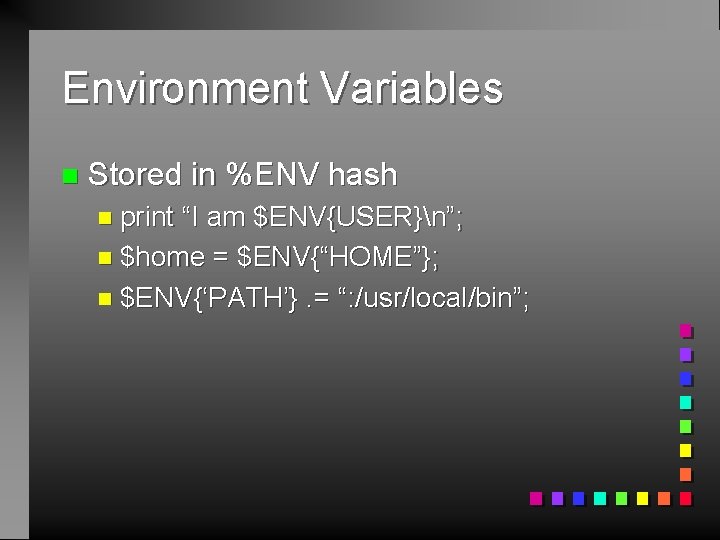
Environment Variables n Stored in %ENV hash n print “I am $ENV{USER}n”; n $home = $ENV{“HOME”}; n $ENV{‘PATH’}. = “: /usr/local/bin”;
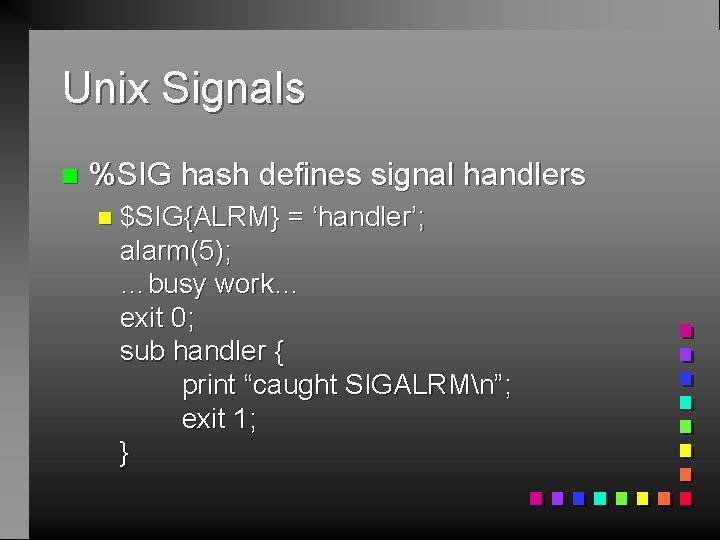
Unix Signals n %SIG hash defines signal handlers n $SIG{ALRM} = ‘handler’; alarm(5); …busy work… exit 0; sub handler { print “caught SIGALRMn”; exit 1; }
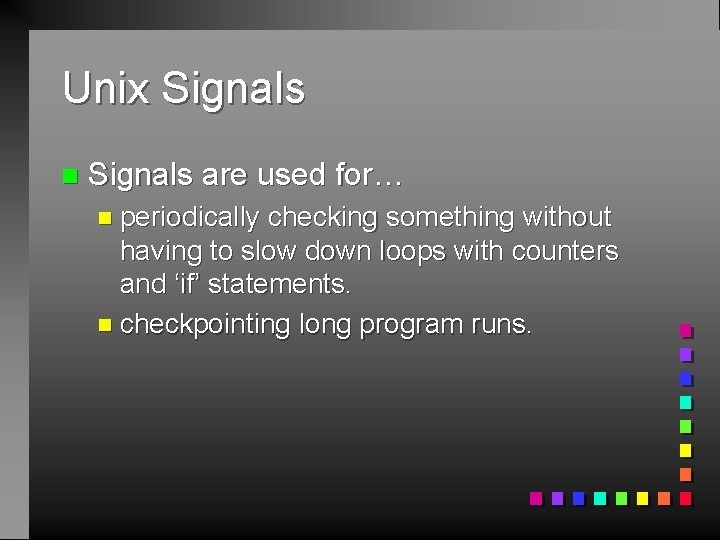
Unix Signals n Signals are used for… n periodically checking something without having to slow down loops with counters and ‘if’ statements. n checkpointing long program runs.
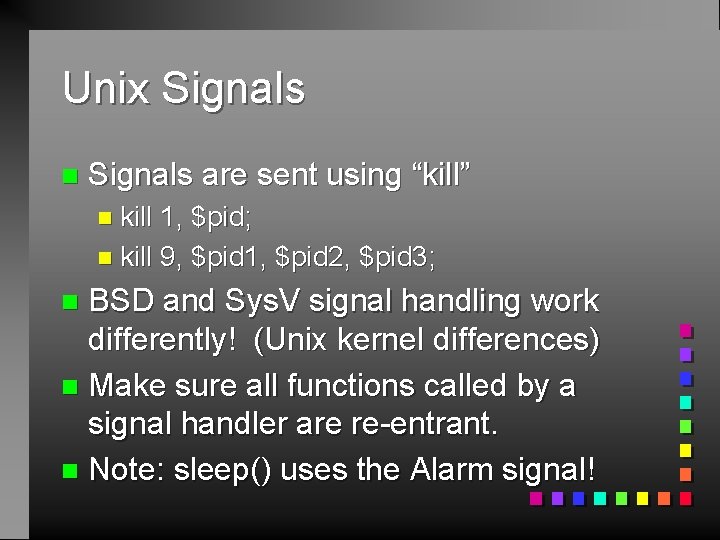
Unix Signals n Signals are sent using “kill” n kill 1, $pid; n kill 9, $pid 1, $pid 2, $pid 3; BSD and Sys. V signal handling work differently! (Unix kernel differences) n Make sure all functions called by a signal handler are re-entrant. n Note: sleep() uses the Alarm signal! n
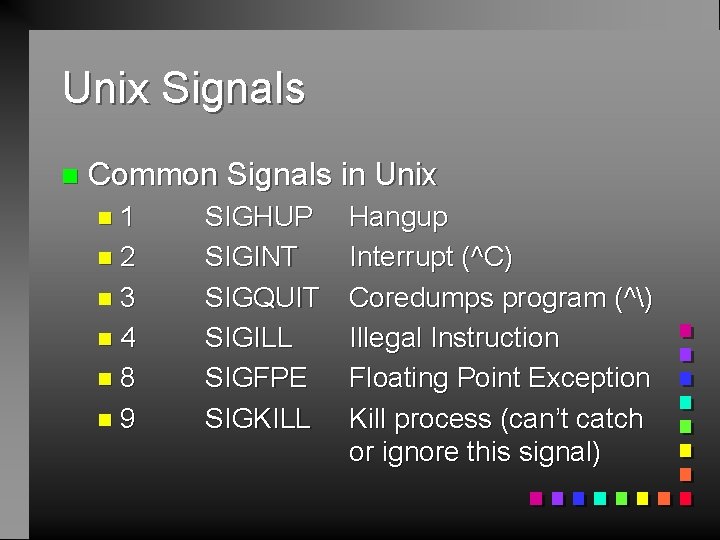
Unix Signals n Common Signals in Unix n 1 n 2 n 3 n 4 n 8 n 9 SIGHUP SIGINT SIGQUIT SIGILL SIGFPE SIGKILL Hangup Interrupt (^C) Coredumps program (^) Illegal Instruction Floating Point Exception Kill process (can’t catch or ignore this signal)
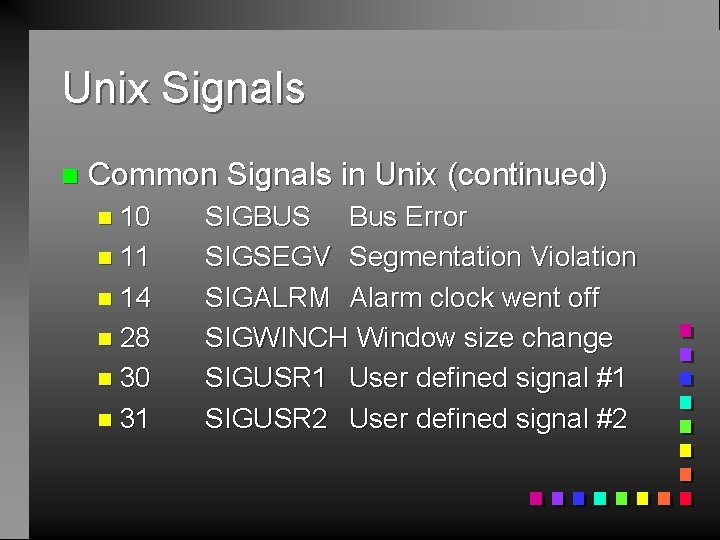
Unix Signals n Common Signals in Unix (continued) n 10 n 11 n 14 n 28 n 30 n 31 SIGBUS Bus Error SIGSEGV Segmentation Violation SIGALRM Alarm clock went off SIGWINCH Window size change SIGUSR 1 User defined signal #1 SIGUSR 2 User defined signal #2
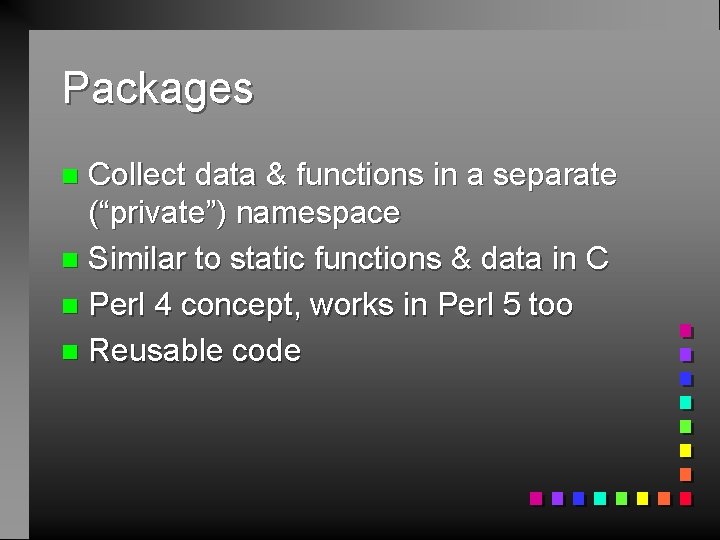
Packages Collect data & functions in a separate (“private”) namespace n Similar to static functions & data in C n Perl 4 concept, works in Perl 5 too n Reusable code n
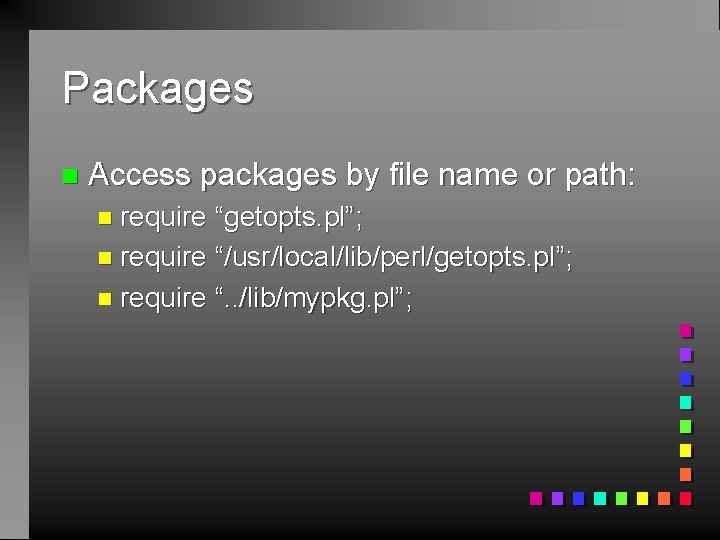
Packages n Access packages by file name or path: n require “getopts. pl”; n require “/usr/local/lib/perl/getopts. pl”; n require “. . /lib/mypkg. pl”;
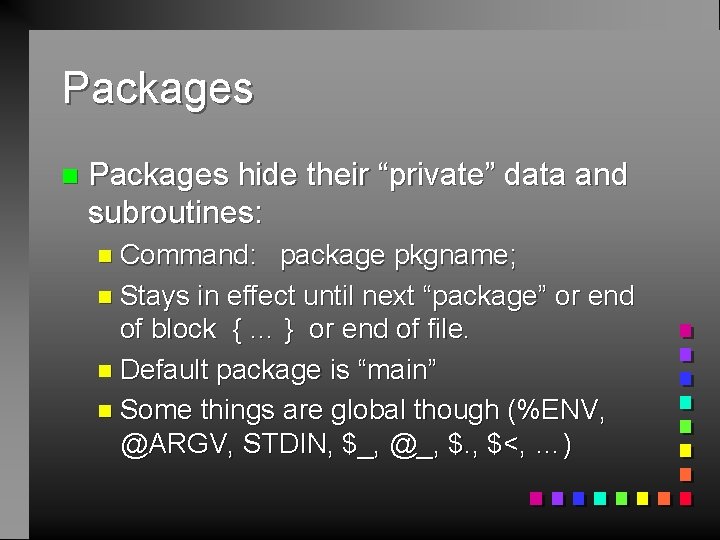
Packages n Packages hide their “private” data and subroutines: n Command: package pkgname; n Stays in effect until next “package” or end of block { … } or end of file. n Default package is “main” n Some things are global though (%ENV, @ARGV, STDIN, $_, @_, $. , $<, …)
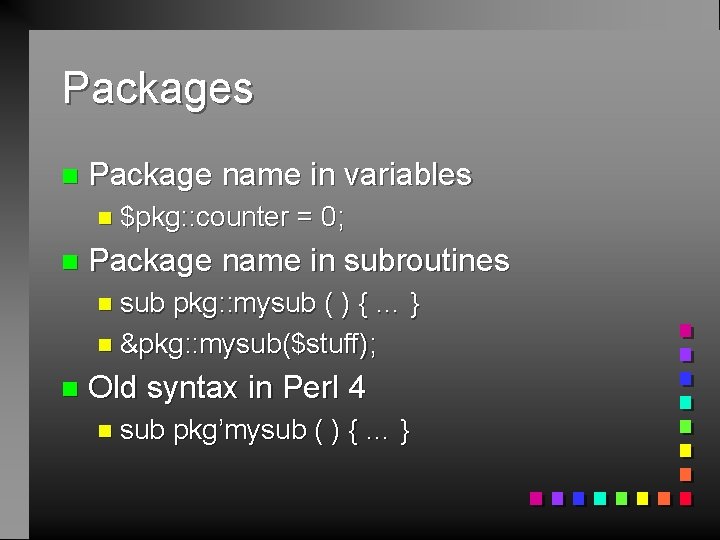
Packages n Package name in variables n $pkg: : counter n = 0; Package name in subroutines n sub pkg: : mysub ( ) { … } n &pkg: : mysub($stuff); n Old syntax in Perl 4 n sub pkg’mysub ( ) { … }
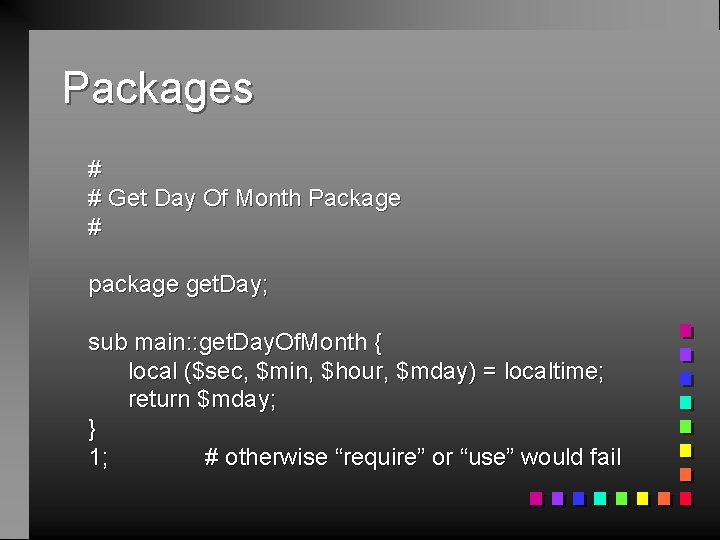
Packages # # Get Day Of Month Package # package get. Day; sub main: : get. Day. Of. Month { local ($sec, $min, $hour, $mday) = localtime; return $mday; } 1; # otherwise “require” or “use” would fail
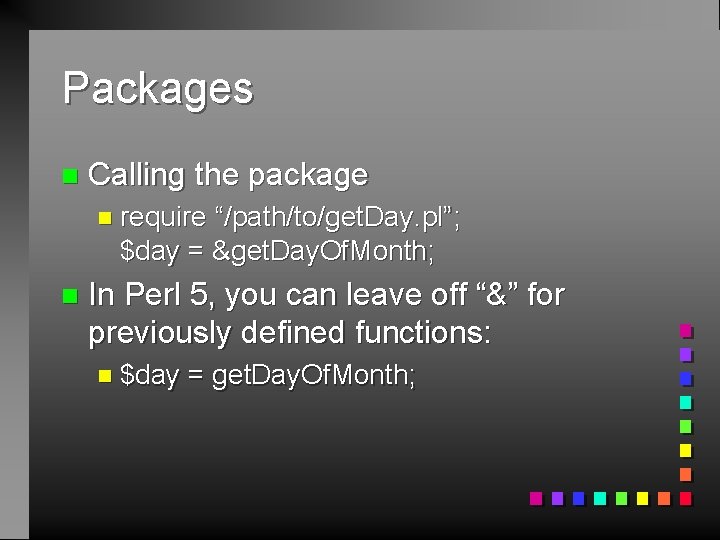
Packages n Calling the package n require “/path/to/get. Day. pl”; $day = &get. Day. Of. Month; n In Perl 5, you can leave off “&” for previously defined functions: n $day = get. Day. Of. Month;
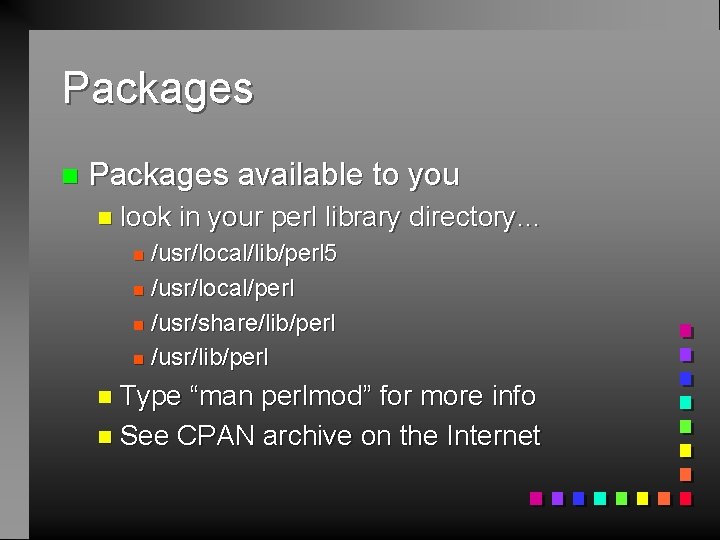
Packages n Packages available to you n look in your perl library directory… /usr/local/lib/perl 5 n /usr/local/perl n /usr/share/lib/perl n /usr/lib/perl n n Type “man perlmod” for more info n See CPAN archive on the Internet
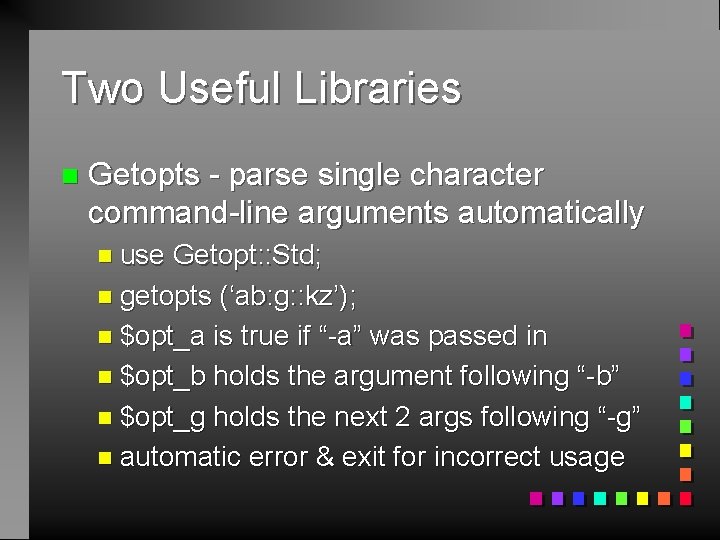
Two Useful Libraries n Getopts - parse single character command-line arguments automatically n use Getopt: : Std; n getopts (‘ab: g: : kz’); n $opt_a is true if “-a” was passed in n $opt_b holds the argument following “-b” n $opt_g holds the next 2 args following “-g” n automatic error & exit for incorrect usage
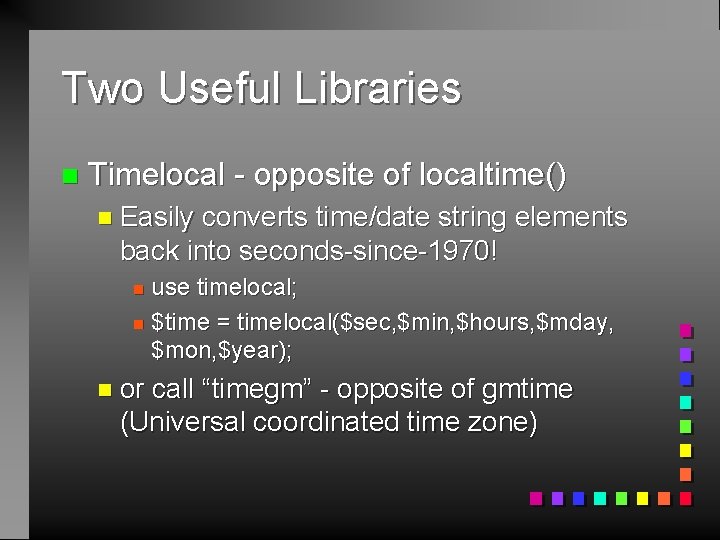
Two Useful Libraries n Timelocal - opposite of localtime() n Easily converts time/date string elements back into seconds-since-1970! use timelocal; n $time = timelocal($sec, $min, $hours, $mday, $mon, $year); n n or call “timegm” - opposite of gmtime (Universal coordinated time zone)
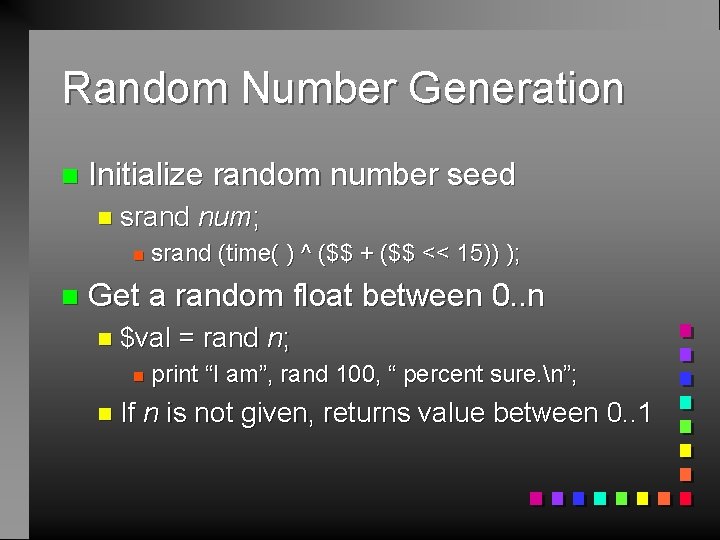
Random Number Generation n Initialize random number seed n srand n n num; srand (time( ) ^ ($$ + ($$ << 15)) ); Get a random float between 0. . n n $val n n If = rand n; print “I am”, rand 100, “ percent sure. n”; n is not given, returns value between 0. . 1
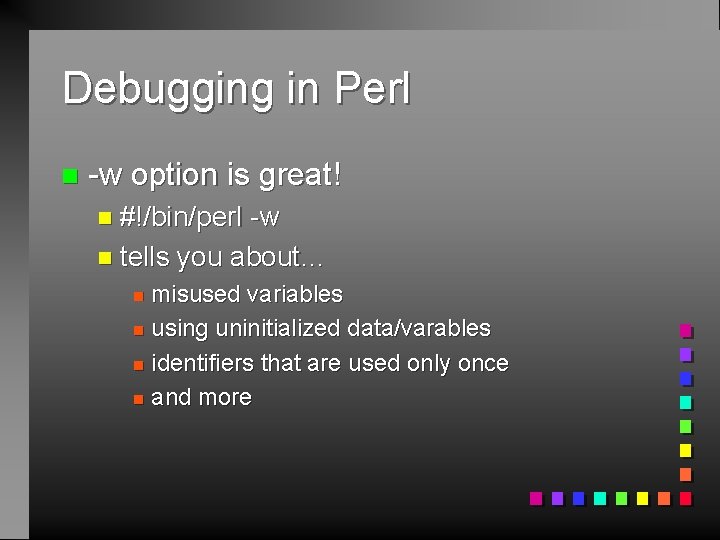
Debugging in Perl n -w option is great! n #!/bin/perl -w n tells you about… misused variables n using uninitialized data/varables n identifiers that are used only once n and more n
![Debugging in Perl n Debug mode perl d filename args n Display Commands h Debugging in Perl n Debug mode: perl -d filename [args] n Display Commands h](https://slidetodoc.com/presentation_image_h2/59e30b6cb5887a59386ef47bfaf7304e/image-86.jpg)
Debugging in Perl n Debug mode: perl -d filename [args] n Display Commands h nh h nl (lowercase-L) n n n l sub l 5 l 3 -6 l Extended help Abbreviated help list lines of code list subroutine sub list line 5 list lines 3 through 6 inclusive list next window of lines
![Debugging in Perl n Display Commands continued w n S n V pkg n Debugging in Perl n Display Commands (continued) w n. S n V [pkg] n](https://slidetodoc.com/presentation_image_h2/59e30b6cb5887a59386ef47bfaf7304e/image-87.jpg)
Debugging in Perl n Display Commands (continued) w n. S n V [pkg] n X [vars] n /pat n n ? pat list window around current location list all subroutines w/ packages list all variables [in package pkg] list variables in this package search forwards for pattern pat (regular expressions legal) search backwards for pattern pat (regular expressions legal)
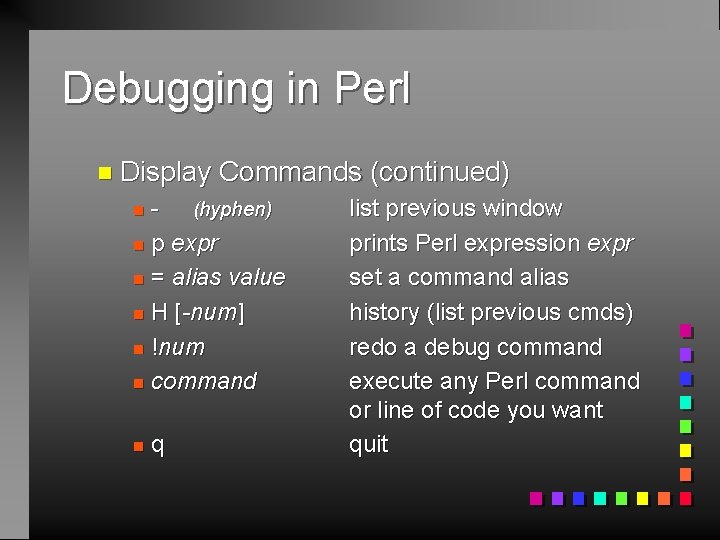
Debugging in Perl n Display Commands (continued) - (hyphen) n p expr n = alias value n H [-num] n !num n command n n q list previous window prints Perl expression expr set a command alias history (list previous cmds) redo a debug command execute any Perl command or line of code you want quit
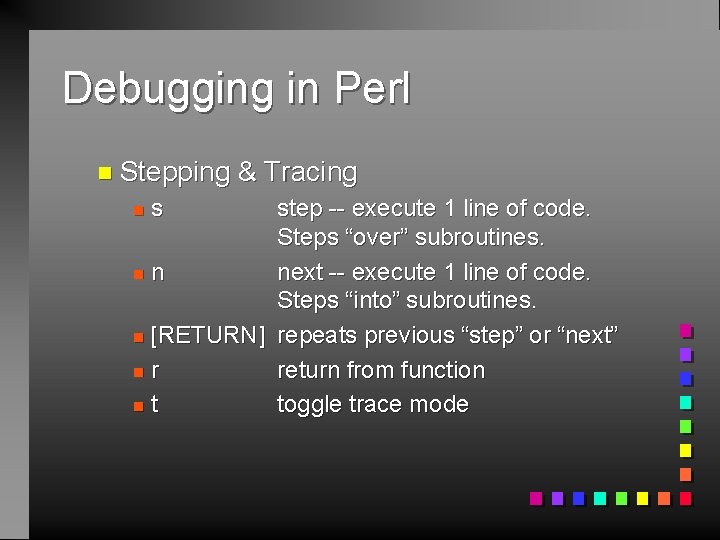
Debugging in Perl n Stepping step -- execute 1 line of code. Steps “over” subroutines. nn next -- execute 1 line of code. Steps “into” subroutines. n [RETURN] repeats previous “step” or “next” nr return from function nt toggle trace mode n s & Tracing
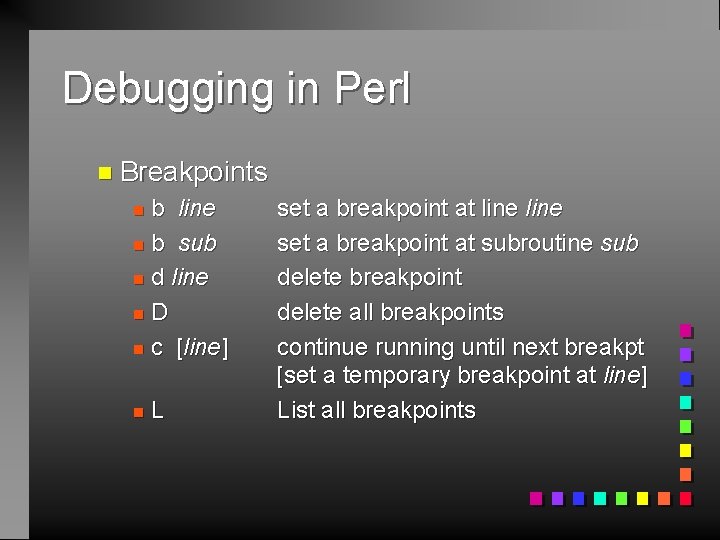
Debugging in Perl n Breakpoints b line n b sub n d line n. D n c [line] n n L set a breakpoint at line set a breakpoint at subroutine sub delete breakpoint delete all breakpoints continue running until next breakpt [set a temporary breakpoint at line] List all breakpoints
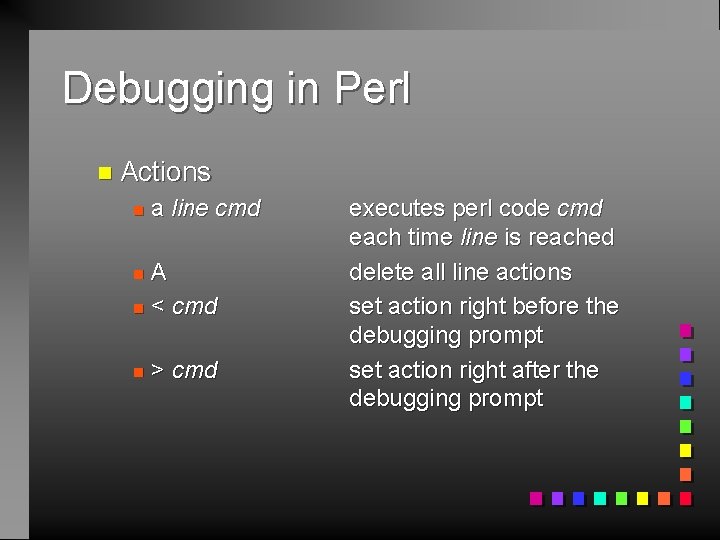
Debugging in Perl n Actions n a line cmd A n < cmd n n > cmd executes perl code cmd each time line is reached delete all line actions set action right before the debugging prompt set action right after the debugging prompt
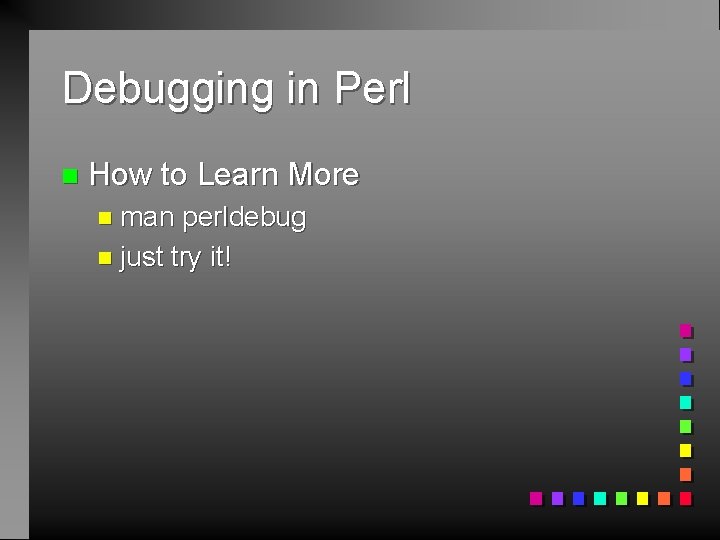
Debugging in Perl n How to Learn More n man perldebug n just try it!
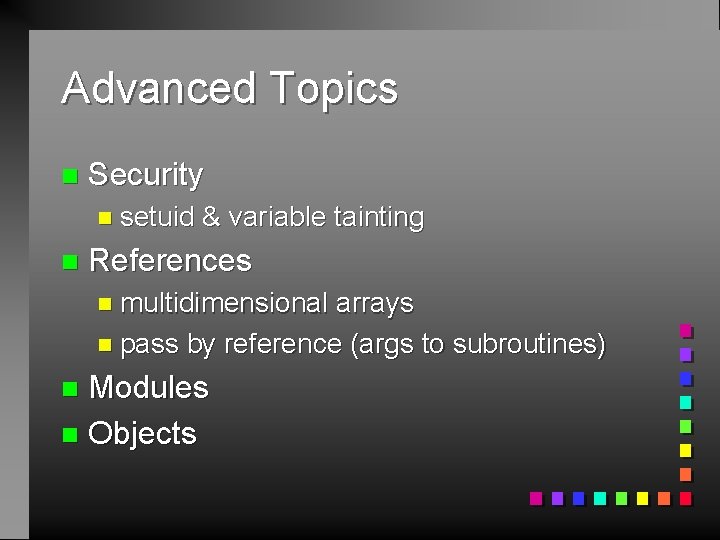
Advanced Topics n Security n setuid n & variable tainting References n multidimensional arrays n pass by reference (args to subroutines) Modules n Objects n
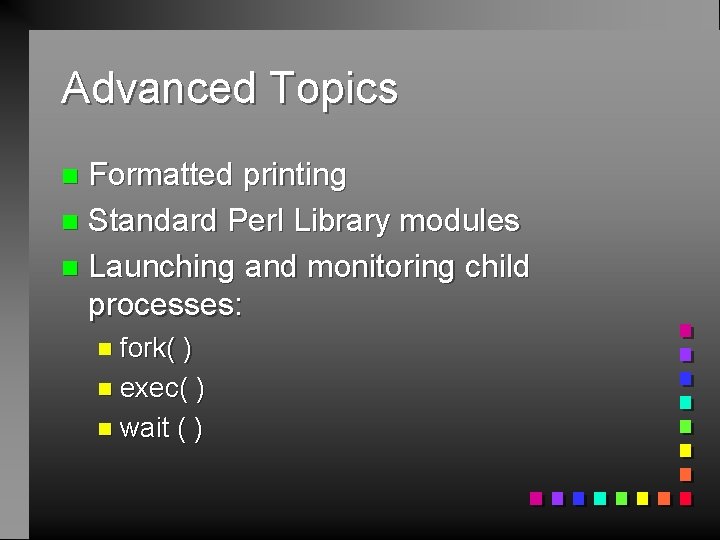
Advanced Topics Formatted printing n Standard Perl Library modules n Launching and monitoring child processes: n n fork( ) n exec( ) n wait ( )
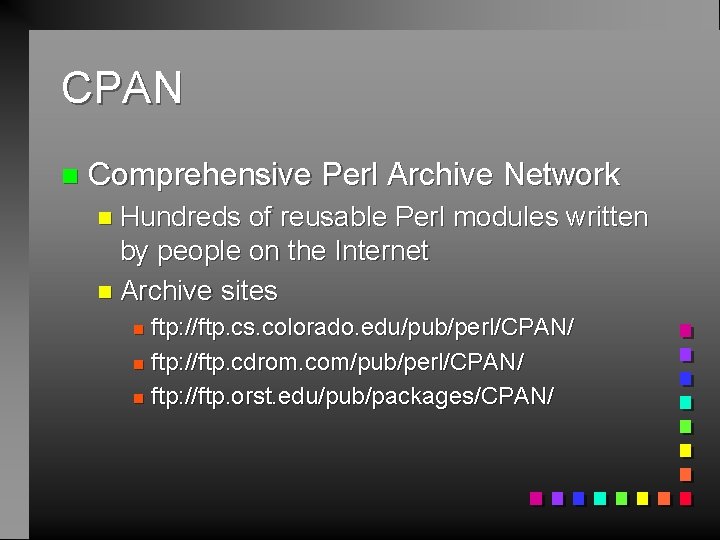
CPAN n Comprehensive Perl Archive Network n Hundreds of reusable Perl modules written by people on the Internet n Archive sites ftp: //ftp. cs. colorado. edu/pub/perl/CPAN/ n ftp: //ftp. cdrom. com/pub/perl/CPAN/ n ftp: //ftp. orst. edu/pub/packages/CPAN/ n
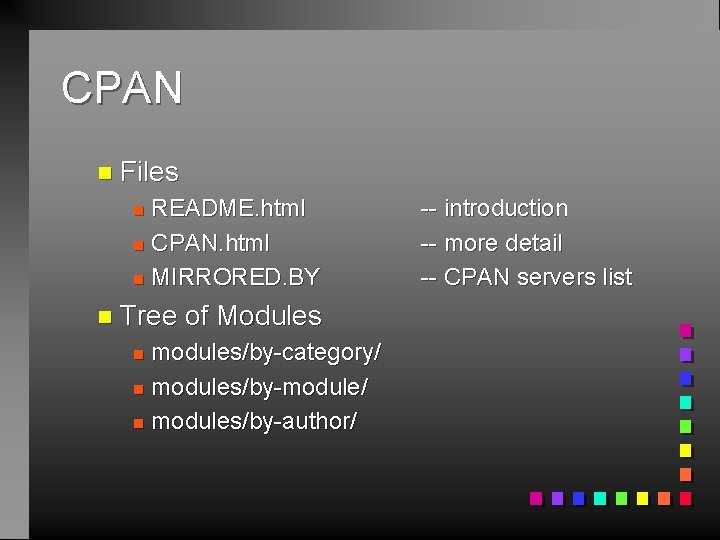
CPAN n Files README. html n CPAN. html n MIRRORED. BY n n Tree of Modules modules/by-category/ n modules/by-module/ n modules/by-author/ n -- introduction -- more detail -- CPAN servers list
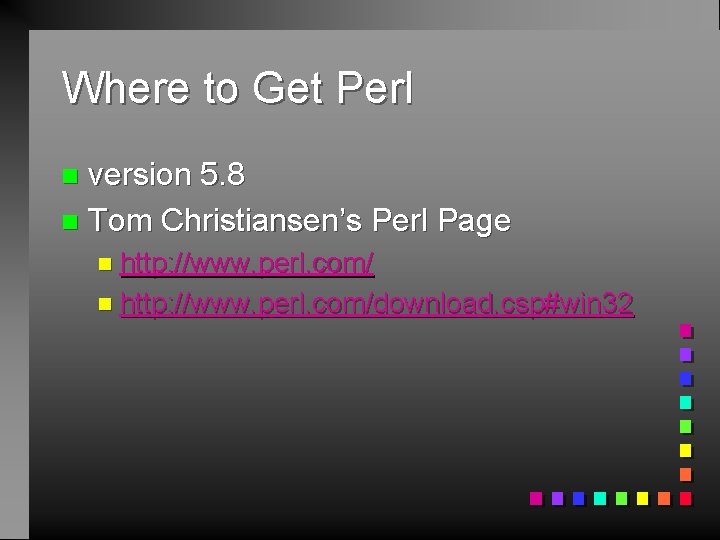
Where to Get Perl version 5. 8 n Tom Christiansen’s Perl Page n n http: //www. perl. com/download. csp#win 32
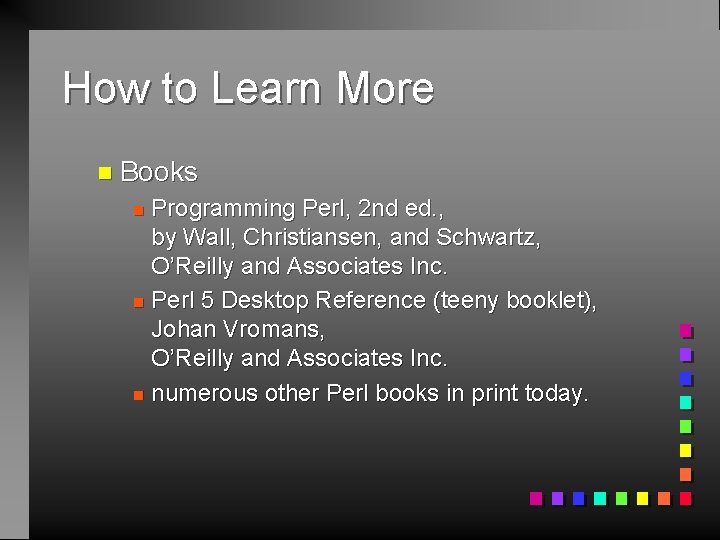
How to Learn More n Books Programming Perl, 2 nd ed. , by Wall, Christiansen, and Schwartz, O’Reilly and Associates Inc. n Perl 5 Desktop Reference (teeny booklet), Johan Vromans, O’Reilly and Associates Inc. n numerous other Perl books in print today. n
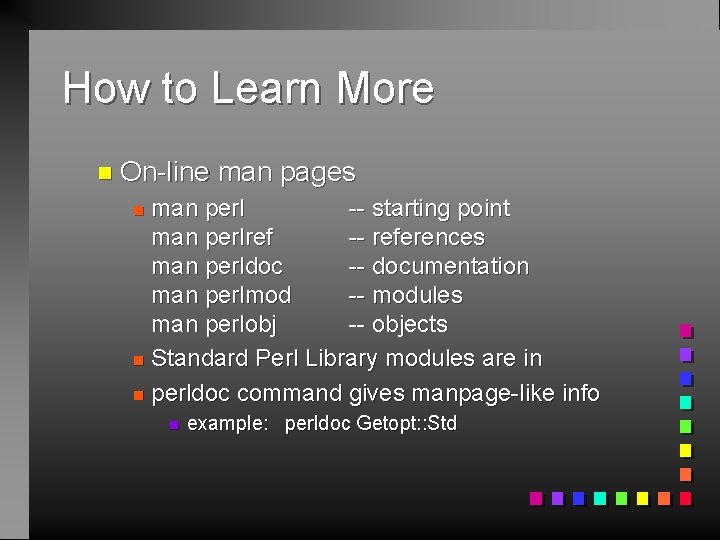
How to Learn More n On-line man pages man perl -- starting point man perlref -- references man perldoc -- documentation man perlmod -- modules man perlobj -- objects n Standard Perl Library modules are in n perldoc command gives manpage-like info n n example: perldoc Getopt: : Std
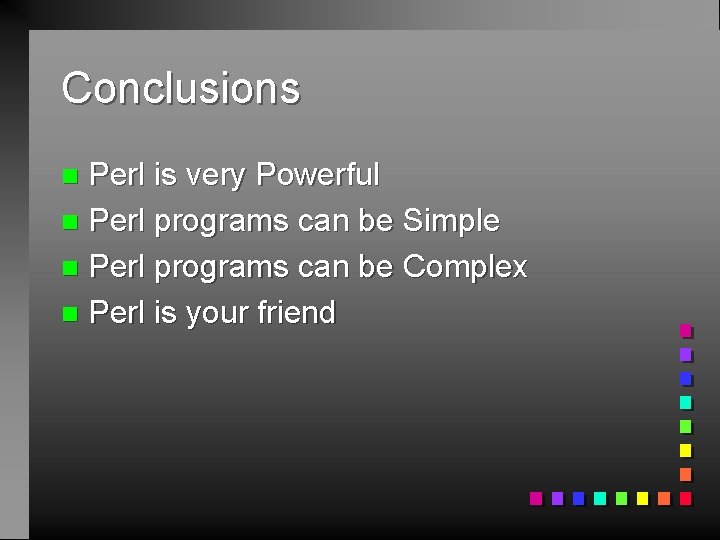
Conclusions Perl is very Powerful n Perl programs can be Simple n Perl programs can be Complex n Perl is your friend n
Slidetodoc.com
Citation sandwich example
"conclusion introduction" "introductory paragraph"
5 paragraph essay
Software engineering 1 course outline
Perl logger
Perl defined 廃止
Perl diamond operator
Tk
Peal soap
Obfuscated perl
Perl boolean operators
Perl framework web
Cgi linkage
Perl cgi table
Elongday
Beginning perl for bioinformatics
Nassim zellal
Perl data types
Perl hash table
Four perfect pebbles chapter 2
Perl bioinformatics
Language
Talend
Perl random number generator
Perl log analysis
Intro to perl
Dr jeffrey roach
Chromosomes examples in real life
Language
Perl conditionals
Man perlrun
Perl paradigma
Perl round
Cgi linkage in perl
Swigxs
Perl camel
Php global exception handler
Practical extraction and reporting language
Perl text manipulation
Dan perl
Esb9999
The oneweek perl.com
Perl tutorial javatpoint
Perl println
Perl bioinformatics
Schengenlyzeum perl
Cgi perl
"commercial" perl or tcl or python
Piper perl
Body paragraph
Rhetorical precis template
Ap world leq structure
Comparison and contrast outline
Social psychology class
Lesson outline lesson 3 describing circuits answers
What are protists lesson outline
Outline of the book of numbers
The physical outline of a display
Kind of fault
Lesson outline lesson 2 aquatic ecosystems answer key
Enduring issue thesis statement
Usbank developer portal
Army training outline
Psalm 61 sermon outline
Psalm 46 sermon outline
Psalm 40 sermon
Pathology outline
Components of a thesis statement
Jesus vs ganesha
Toulmin argument essay outline
What is a synthesis essay
Outline of colossians
Mandt system crisis cycle
Presentation outline slide
Student case brief example
2 corinthians outline
2 corinthians outline
1 corinthians outline
Essay on cognitive approach
Book of joel outline
Outline of joel
Evangelism explosion outline
Kings outline
Rogerian model
Spatial outline
Labeling theory examples
Short story outline
Short answer questions examples
Senior project outline
Capstone project outline
Project presentation outline
Science investigatory project sample
The rogerian argument model is named after
Rogerian argument outline
Romans outline by chapter
Work plan for research proposal
Research paper body paragraph outline
Pathology outline
Outline plagiarism
Outline ratio