Perform File IO using file pointers FILE datatype
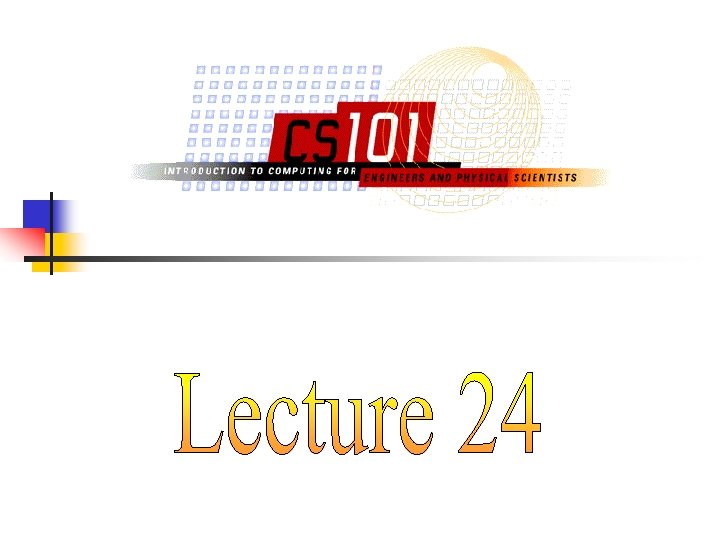
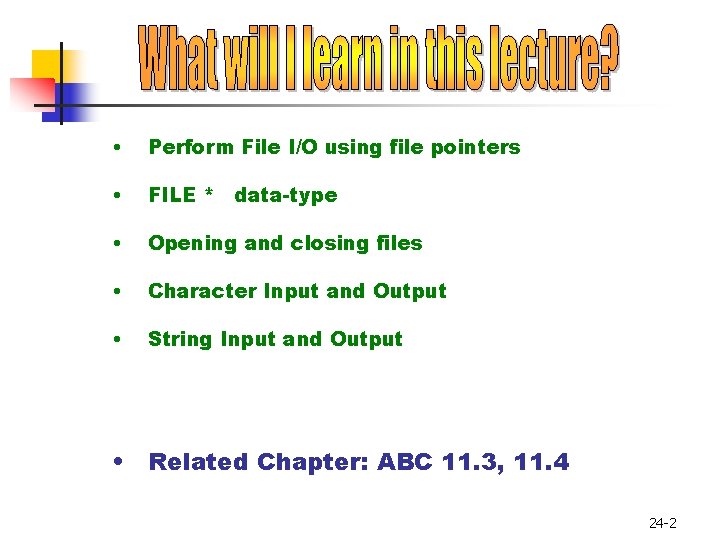
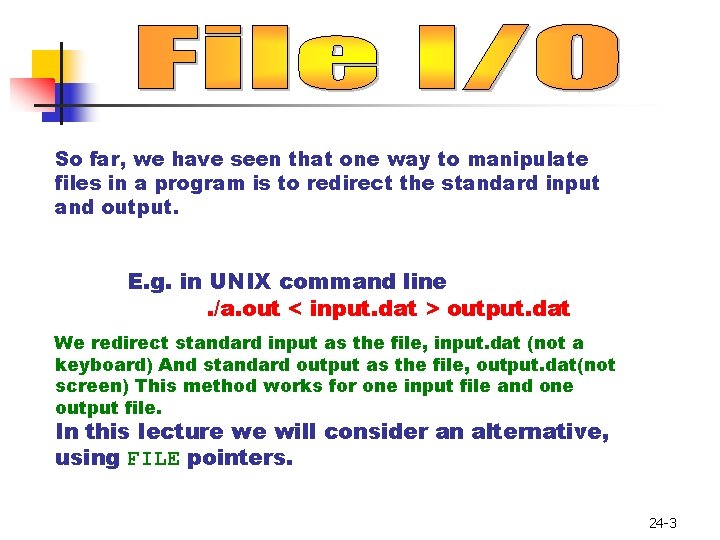
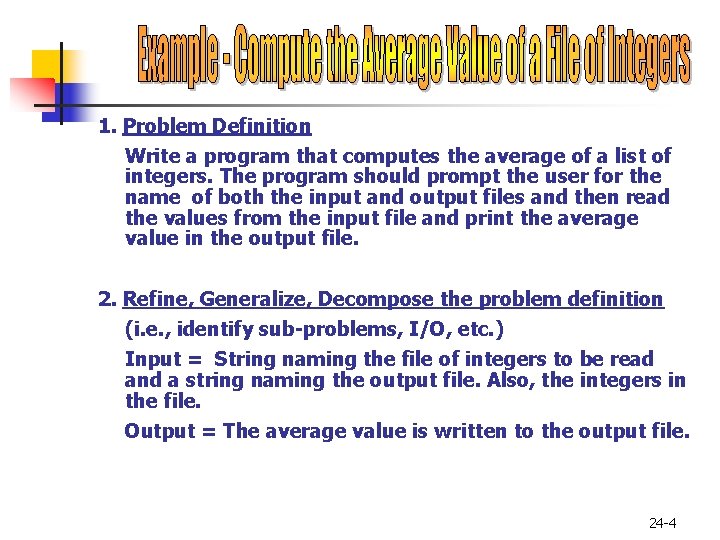
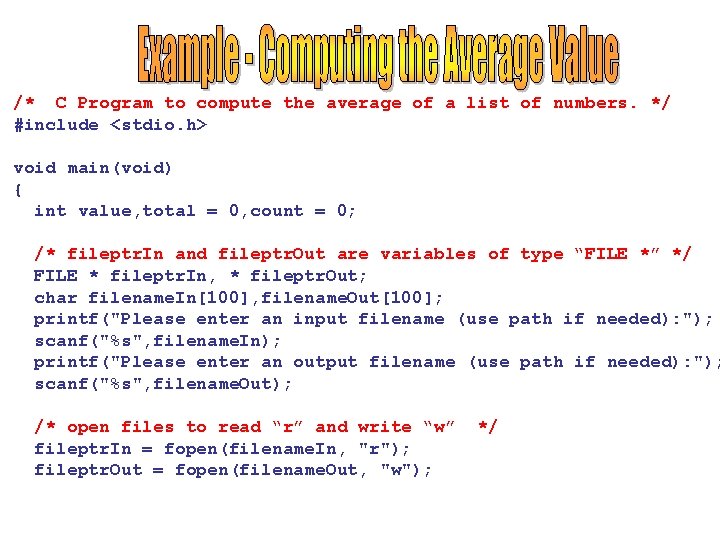
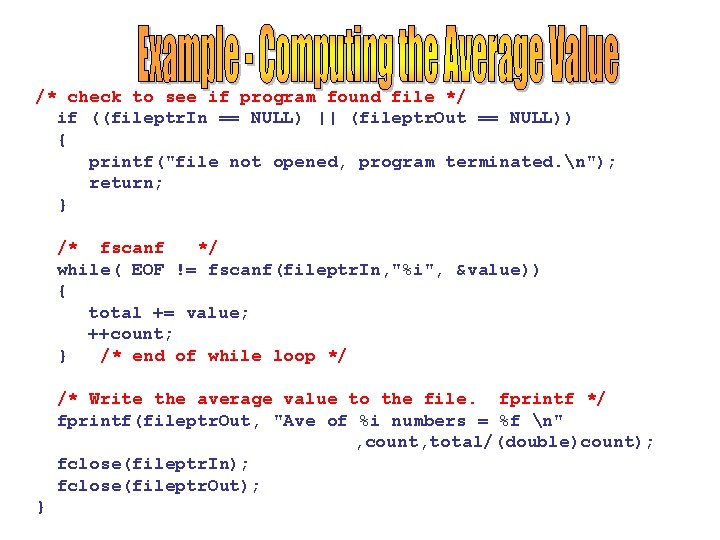
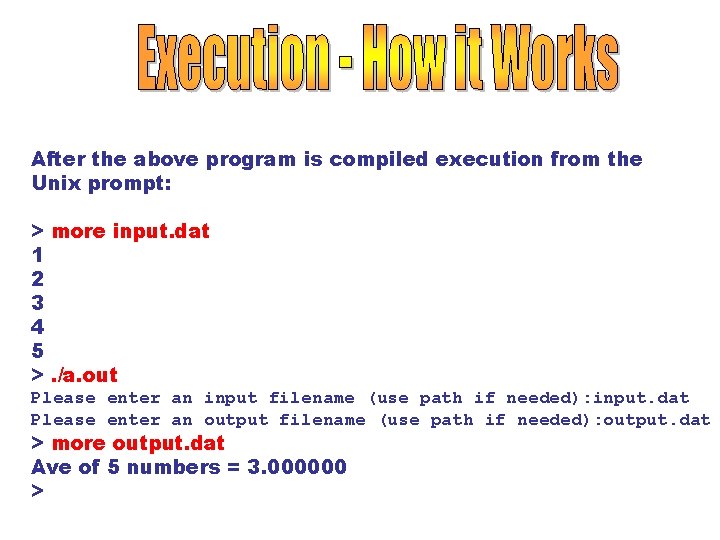
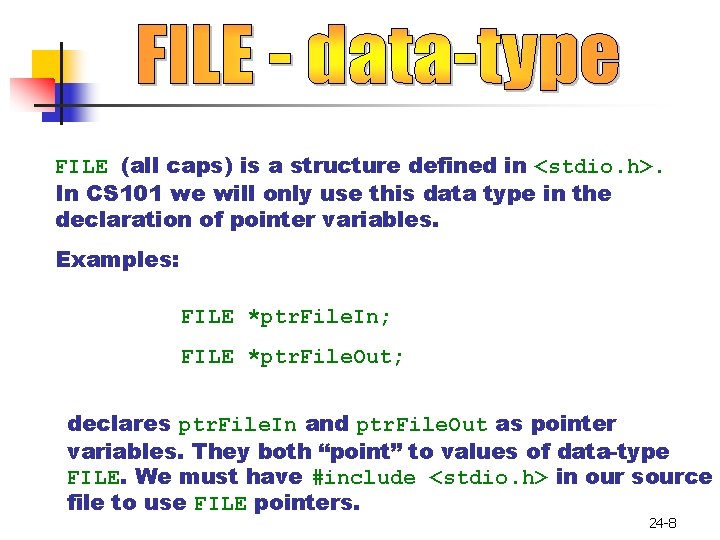
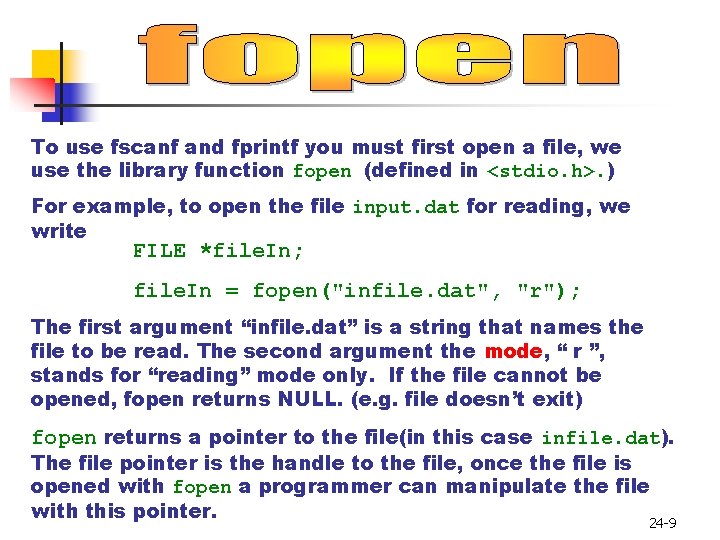
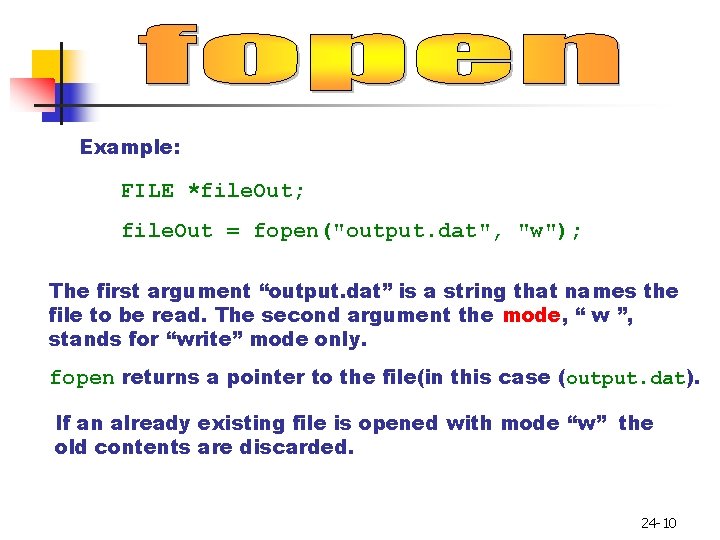
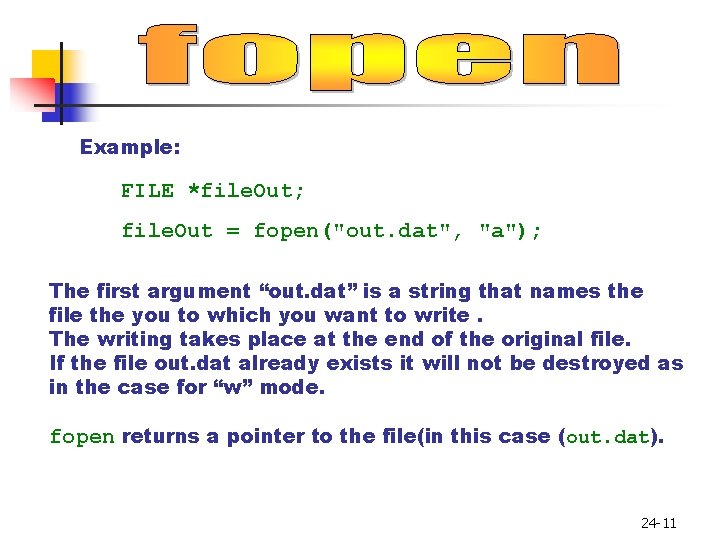
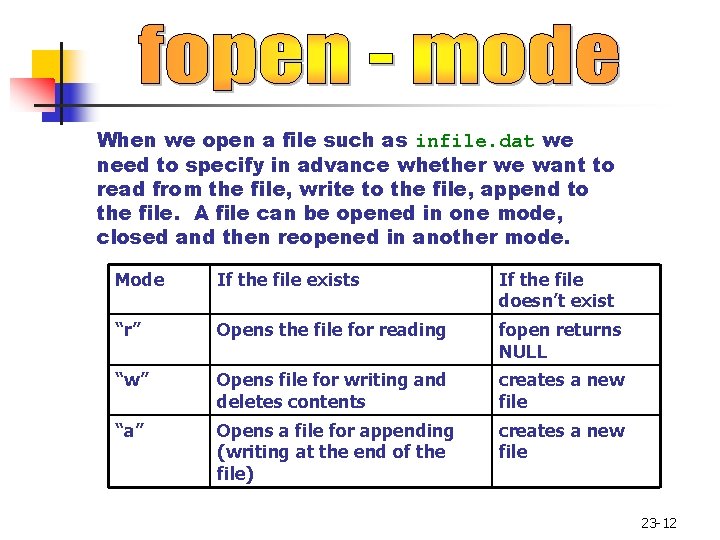
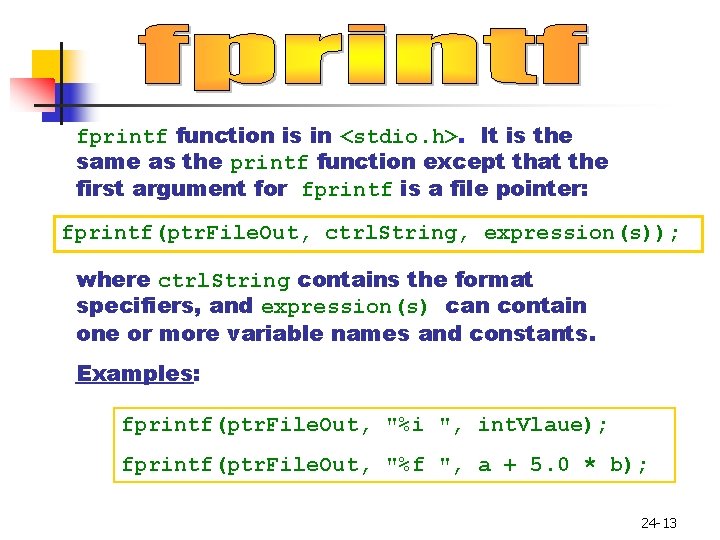
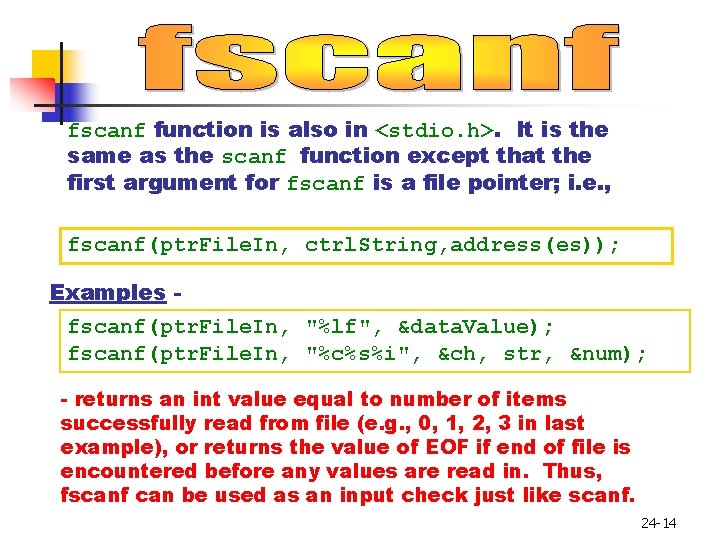
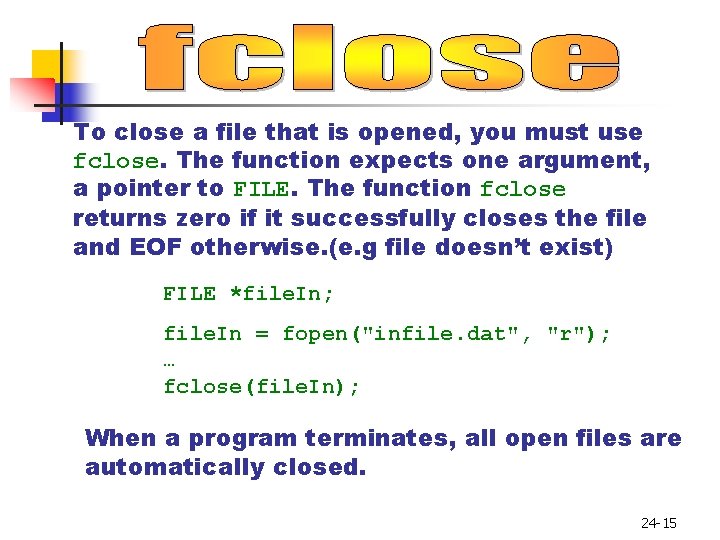
- Slides: 15
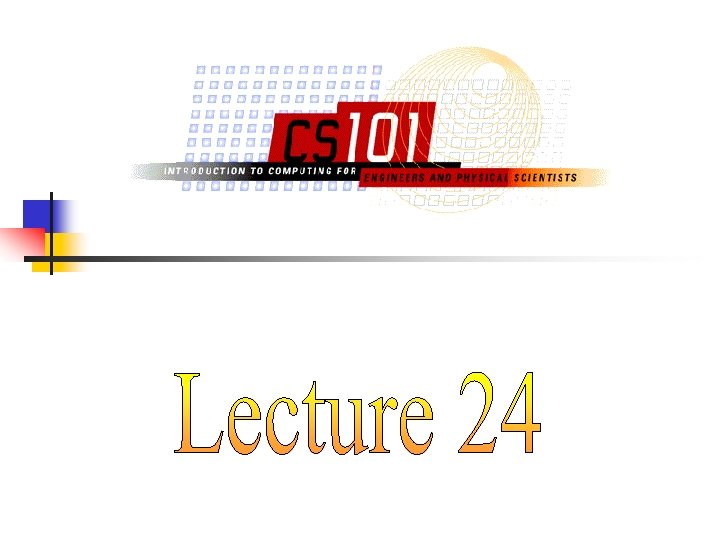
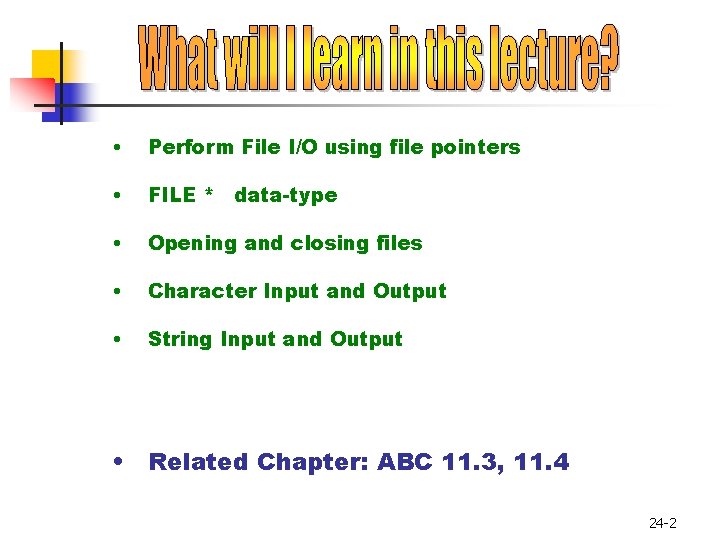
• Perform File I/O using file pointers • FILE * data-type • Opening and closing files • Character Input and Output • String Input and Output • Related Chapter: ABC 11. 3, 11. 4 24 -2
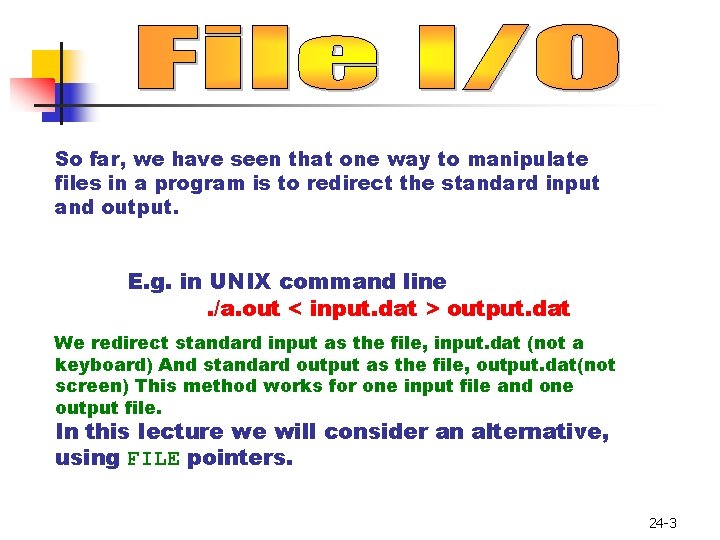
So far, we have seen that one way to manipulate files in a program is to redirect the standard input and output. E. g. in UNIX command line. /a. out < input. dat > output. dat We redirect standard input as the file, input. dat (not a keyboard) And standard output as the file, output. dat(not screen) This method works for one input file and one output file. In this lecture we will consider an alternative, using FILE pointers. 24 -3
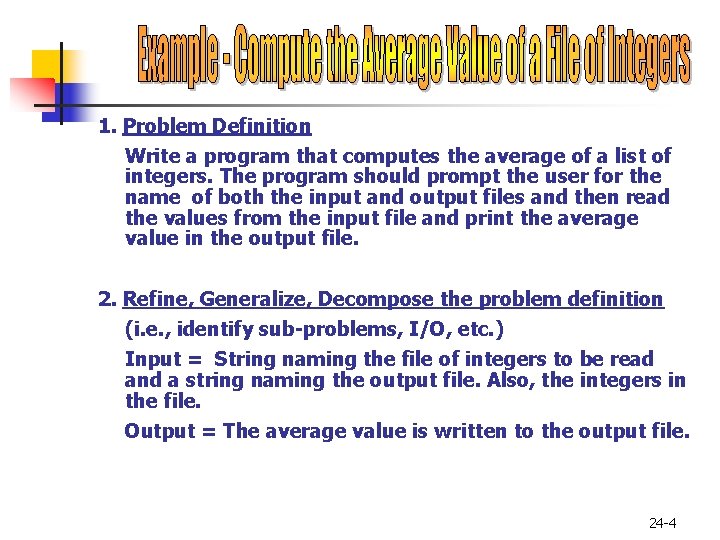
1. Problem Definition Write a program that computes the average of a list of integers. The program should prompt the user for the name of both the input and output files and then read the values from the input file and print the average value in the output file. 2. Refine, Generalize, Decompose the problem definition (i. e. , identify sub-problems, I/O, etc. ) Input = String naming the file of integers to be read and a string naming the output file. Also, the integers in the file. Output = The average value is written to the output file. 24 -4
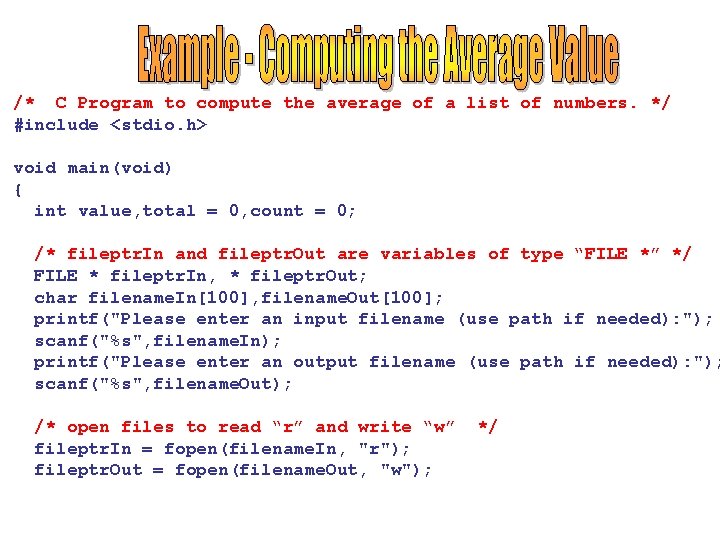
/* C Program to compute the average of a list of numbers. */ #include <stdio. h> void main(void) { int value, total = 0, count = 0; /* fileptr. In and fileptr. Out are variables of type “FILE *” */ FILE * fileptr. In, * fileptr. Out; char filename. In[100], filename. Out[100]; printf("Please enter an input filename (use path if needed): "); scanf("%s", filename. In); printf("Please enter an output filename (use path if needed): "); scanf("%s", filename. Out); /* open files to read “r” and write “w” fileptr. In = fopen(filename. In, "r"); fileptr. Out = fopen(filename. Out, "w"); */
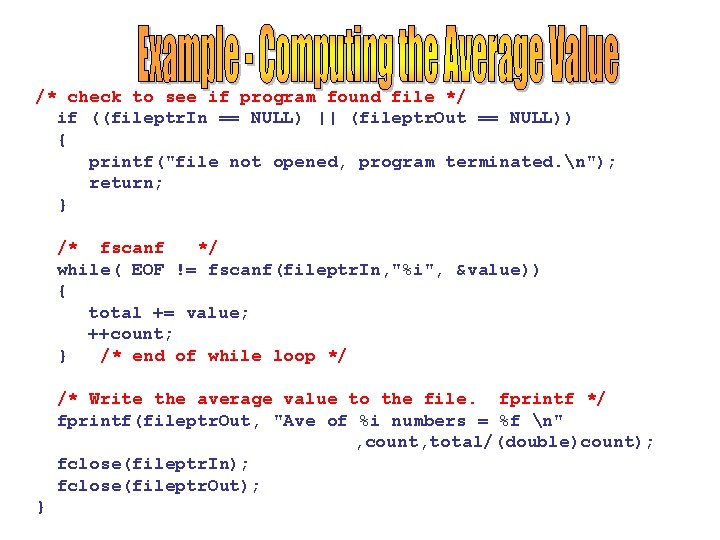
/* check to see if program found file */ if ((fileptr. In == NULL) || (fileptr. Out == NULL)) { printf("file not opened, program terminated. n"); return; } /* fscanf */ while( EOF != fscanf(fileptr. In, "%i", &value)) { total += value; ++count; } /* end of while loop */ /* Write the average value to the file. fprintf */ fprintf(fileptr. Out, "Ave of %i numbers = %f n" , count, total/(double)count); fclose(fileptr. In); fclose(fileptr. Out); }
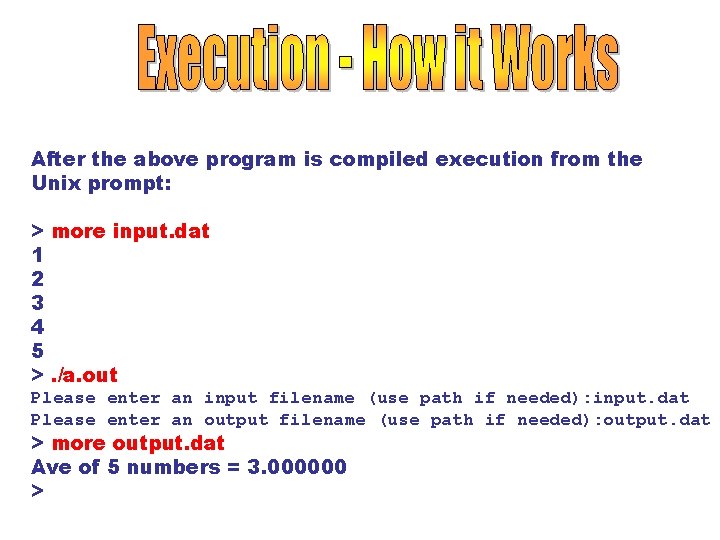
After the above program is compiled execution from the Unix prompt: > more input. dat 1 2 3 4 5 >. /a. out Please enter an input filename (use path if needed): input. dat Please enter an output filename (use path if needed): output. dat > more output. dat Ave of 5 numbers = 3. 000000 >
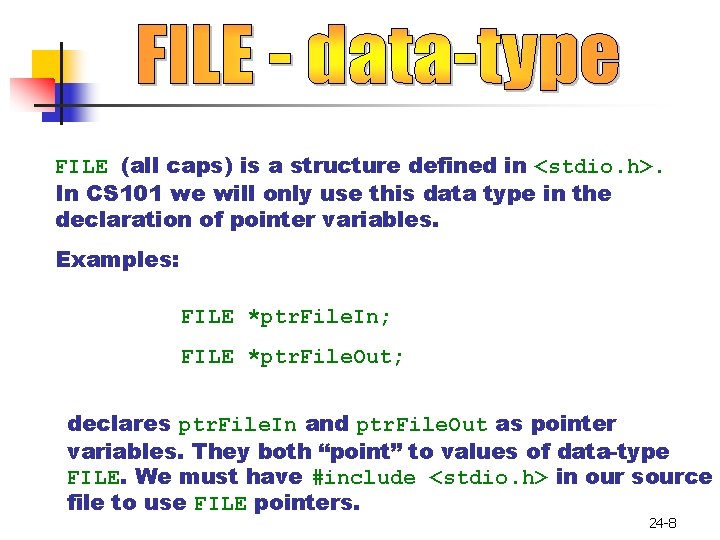
FILE (all caps) is a structure defined in <stdio. h>. In CS 101 we will only use this data type in the declaration of pointer variables. Examples: FILE *ptr. File. In; FILE *ptr. File. Out; declares ptr. File. In and ptr. File. Out as pointer variables. They both “point” to values of data-type FILE. We must have #include <stdio. h> in our source file to use FILE pointers. 24 -8
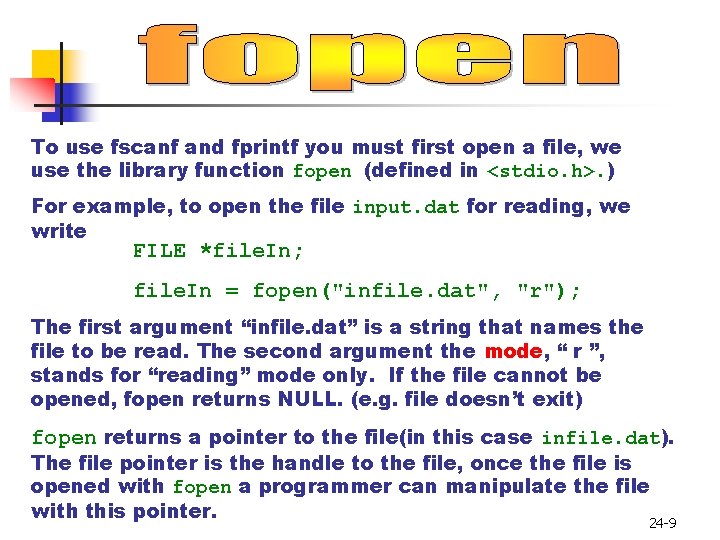
To use fscanf and fprintf you must first open a file, we use the library function fopen (defined in <stdio. h>. ) For example, to open the file input. dat for reading, we write FILE *file. In; file. In = fopen("infile. dat", "r"); The first argument “infile. dat” is a string that names the file to be read. The second argument the mode, “ r ”, stands for “reading” mode only. If the file cannot be opened, fopen returns NULL. (e. g. file doesn’t exit) fopen returns a pointer to the file(in this case infile. dat). The file pointer is the handle to the file, once the file is opened with fopen a programmer can manipulate the file with this pointer. 24 -9
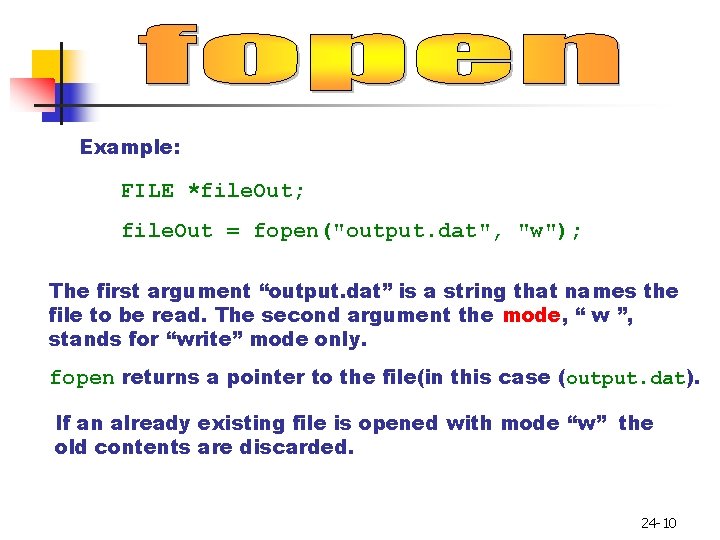
Example: FILE *file. Out; file. Out = fopen("output. dat", "w"); The first argument “output. dat” is a string that names the file to be read. The second argument the mode, “ w ”, stands for “write” mode only. fopen returns a pointer to the file(in this case (output. dat). If an already existing file is opened with mode “w” the old contents are discarded. 24 -10
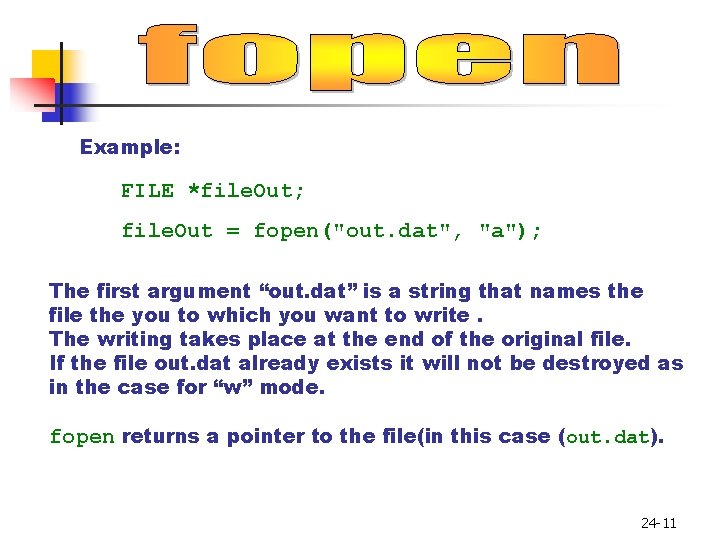
Example: FILE *file. Out; file. Out = fopen("out. dat", "a"); The first argument “out. dat” is a string that names the file the you to which you want to write. The writing takes place at the end of the original file. If the file out. dat already exists it will not be destroyed as in the case for “w” mode. fopen returns a pointer to the file(in this case (out. dat). 24 -11
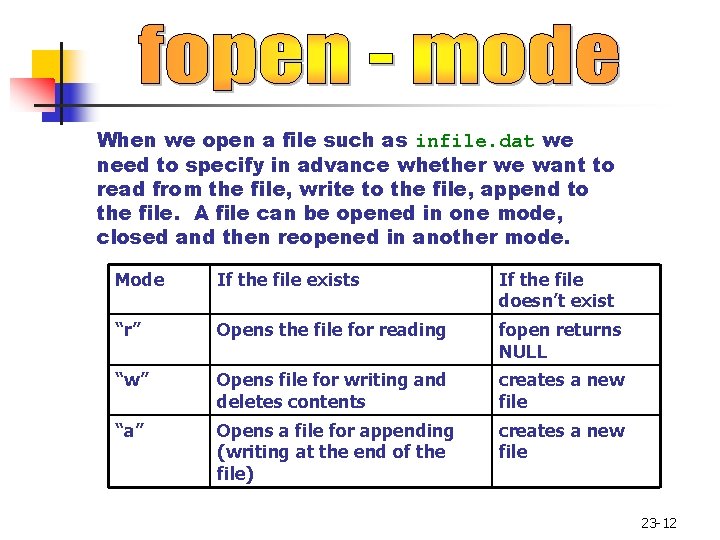
When we open a file such as infile. dat we need to specify in advance whether we want to read from the file, write to the file, append to the file. A file can be opened in one mode, closed and then reopened in another mode. Mode If the file exists If the file doesn’t exist “r” Opens the file for reading fopen returns NULL “w” Opens file for writing and deletes contents creates a new file “a” Opens a file for appending (writing at the end of the file) creates a new file 23 -12
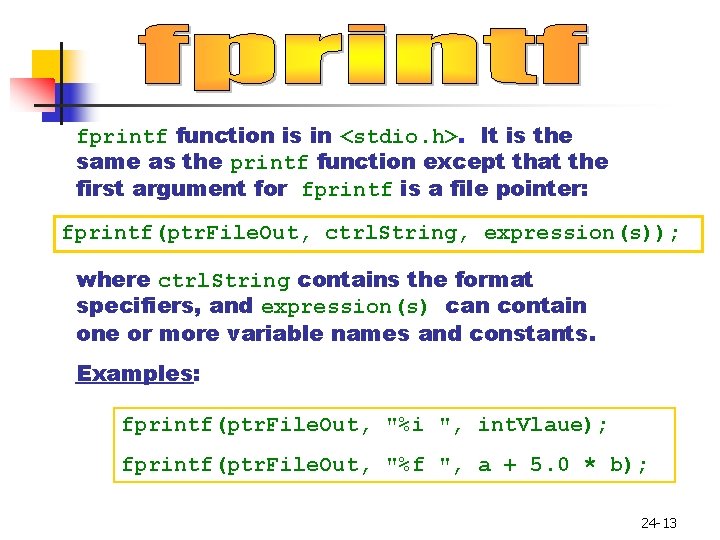
fprintf function is in <stdio. h>. It is the same as the printf function except that the first argument for fprintf is a file pointer: fprintf(ptr. File. Out, ctrl. String, expression(s)); where ctrl. String contains the format specifiers, and expression(s) can contain one or more variable names and constants. Examples: fprintf(ptr. File. Out, "%i ", int. Vlaue); fprintf(ptr. File. Out, "%f ", a + 5. 0 * b); 24 -13
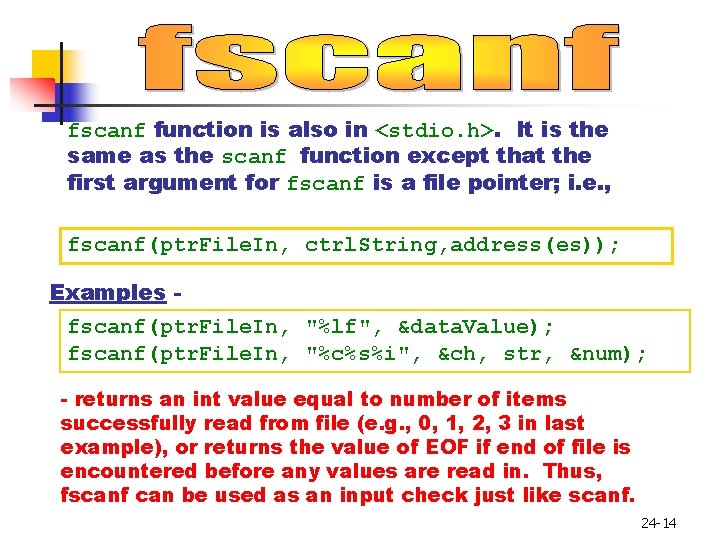
fscanf function is also in <stdio. h>. It is the same as the scanf function except that the first argument for fscanf is a file pointer; i. e. , fscanf(ptr. File. In, ctrl. String, address(es)); Examples fscanf(ptr. File. In, "%lf", &data. Value); fscanf(ptr. File. In, "%c%s%i", &ch, str, &num); - returns an int value equal to number of items successfully read from file (e. g. , 0, 1, 2, 3 in last example), or returns the value of EOF if end of file is encountered before any values are read in. Thus, fscanf can be used as an input check just like scanf. 24 -14
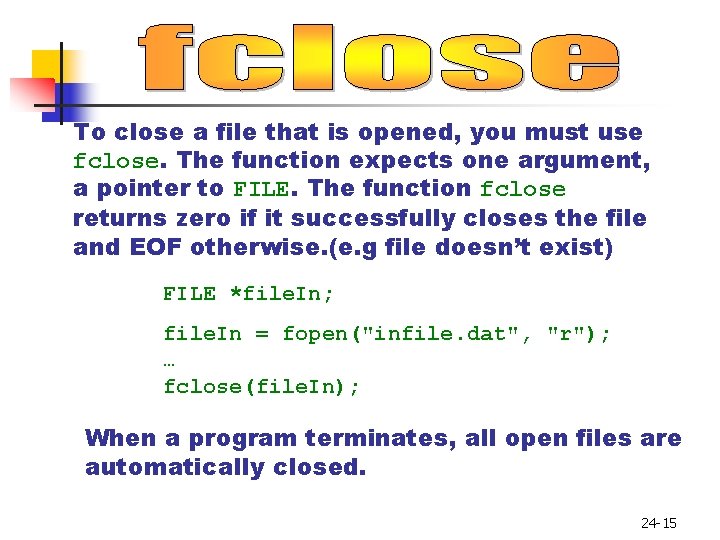
To close a file that is opened, you must use fclose. The function expects one argument, a pointer to FILE. The function fclose returns zero if it successfully closes the file and EOF otherwise. (e. g file doesn’t exist) FILE *file. In; file. In = fopen("infile. dat", "r"); … fclose(file. In); When a program terminates, all open files are automatically closed. 24 -15