Parameters Overview A Reminder Why Parameters are Needed
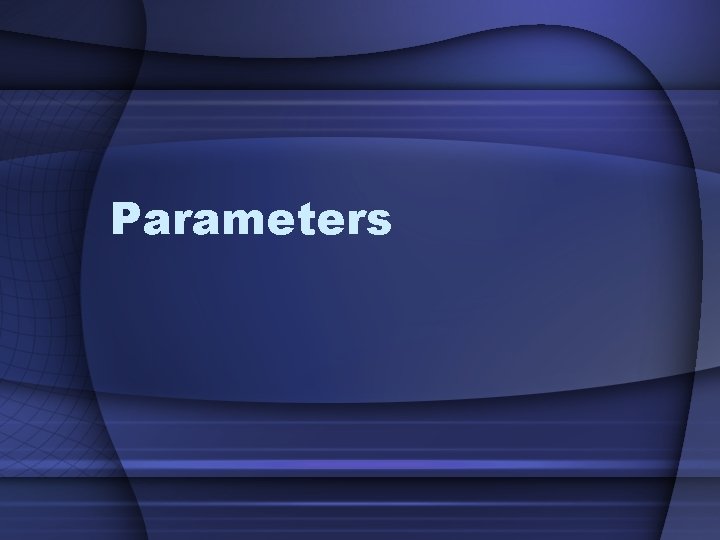
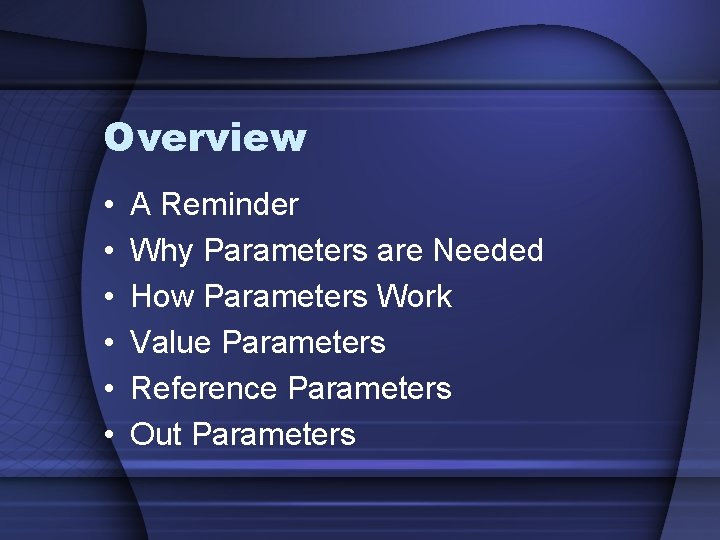
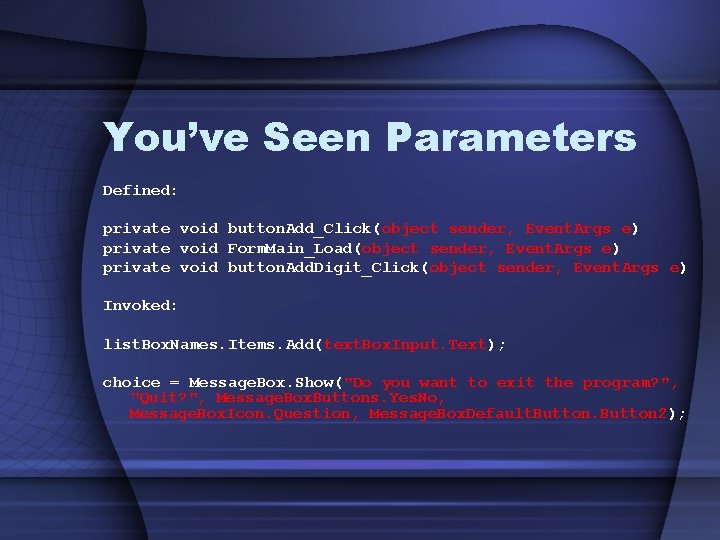
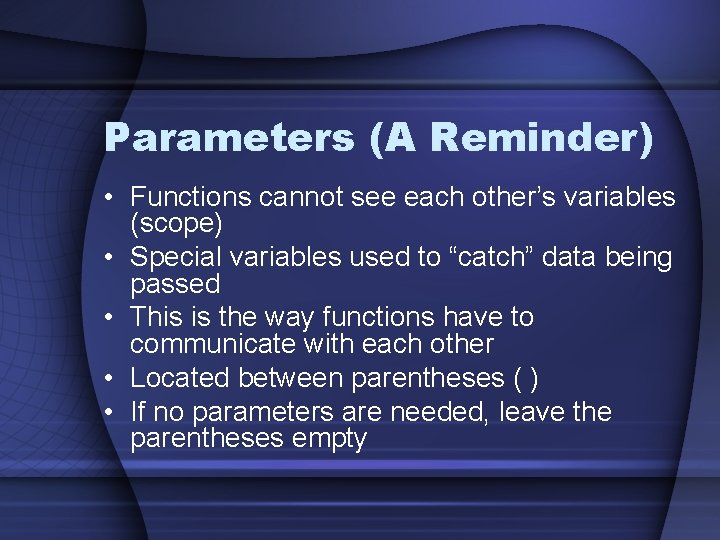
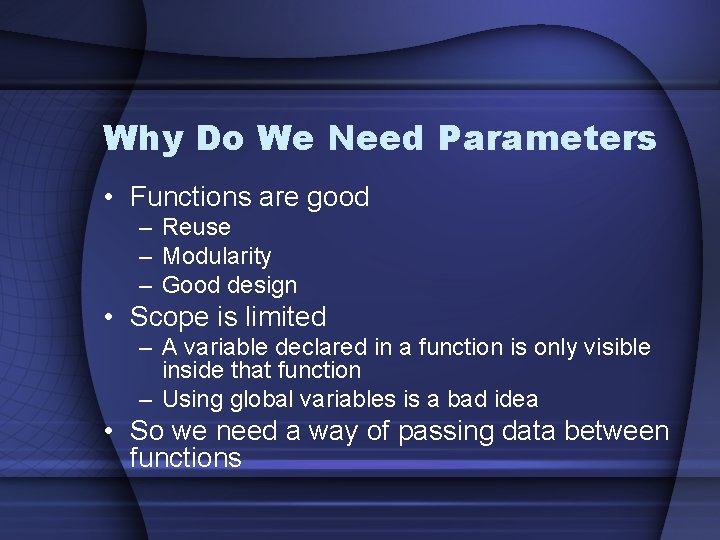
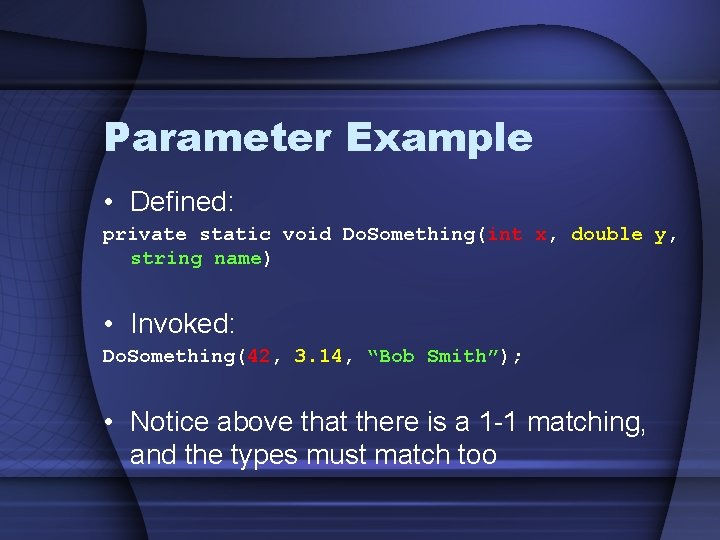
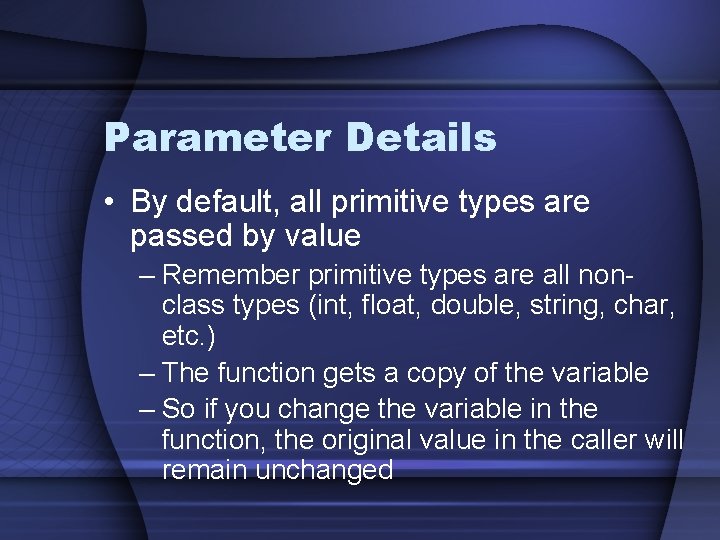
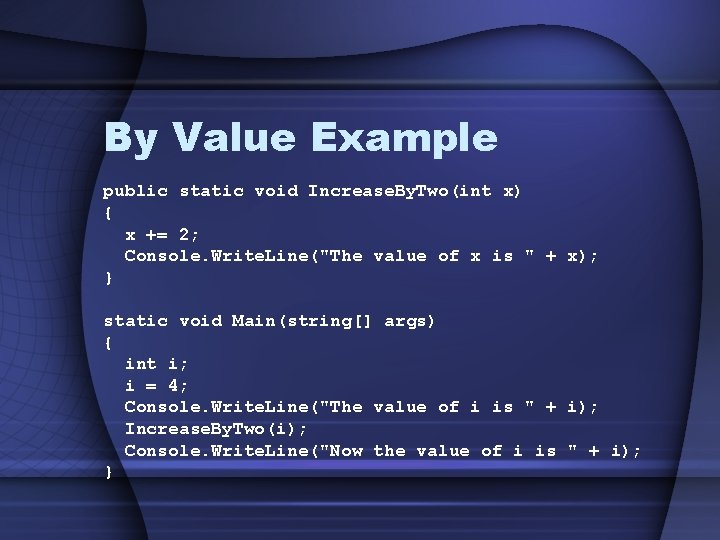
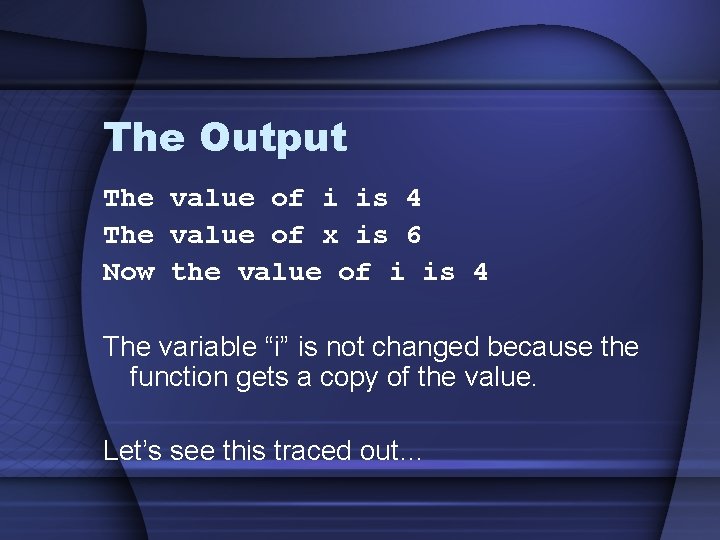
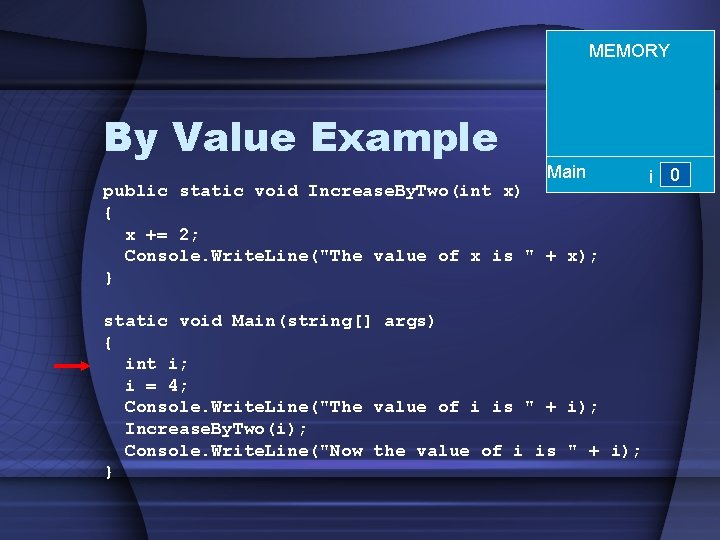
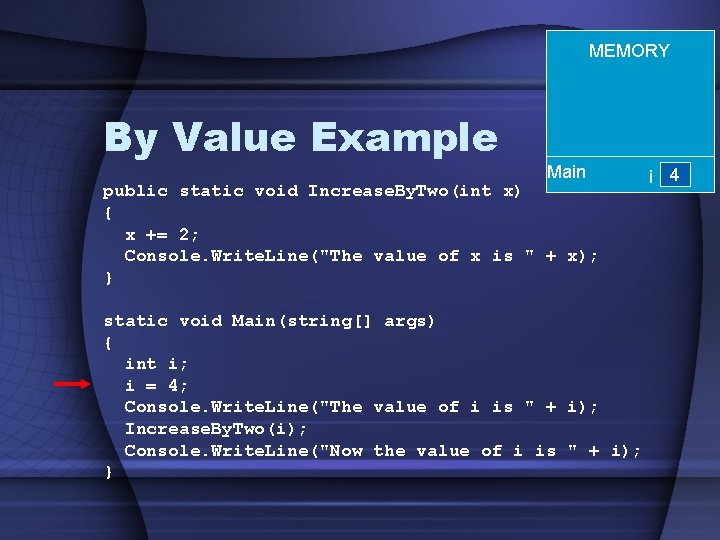
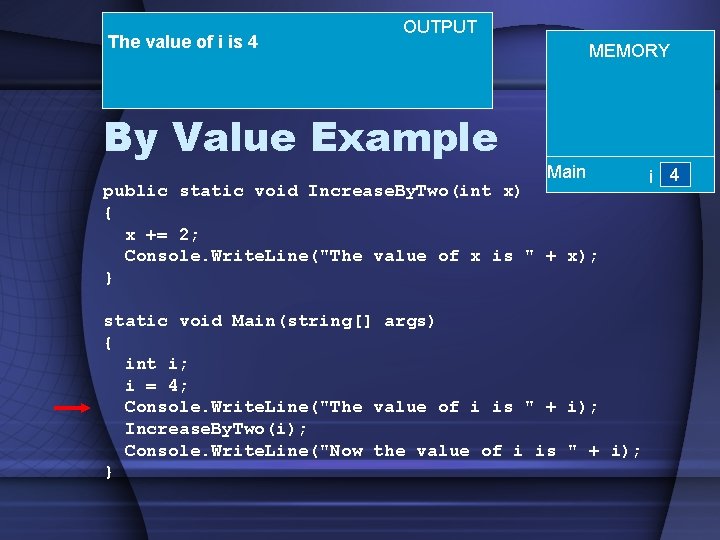
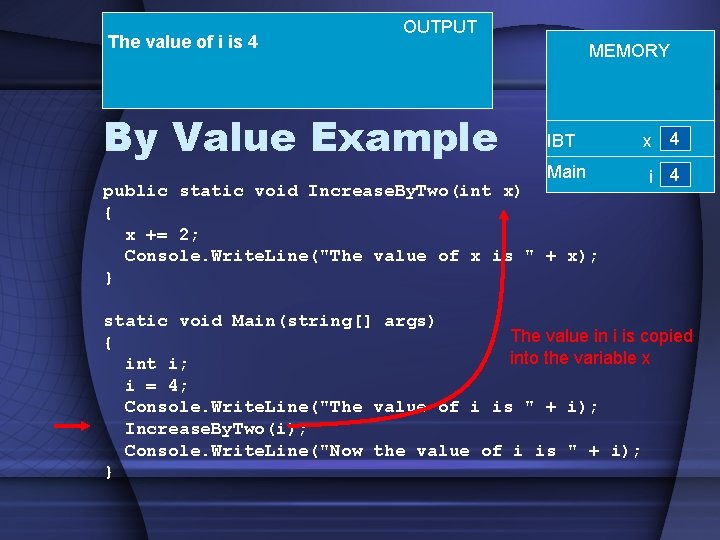
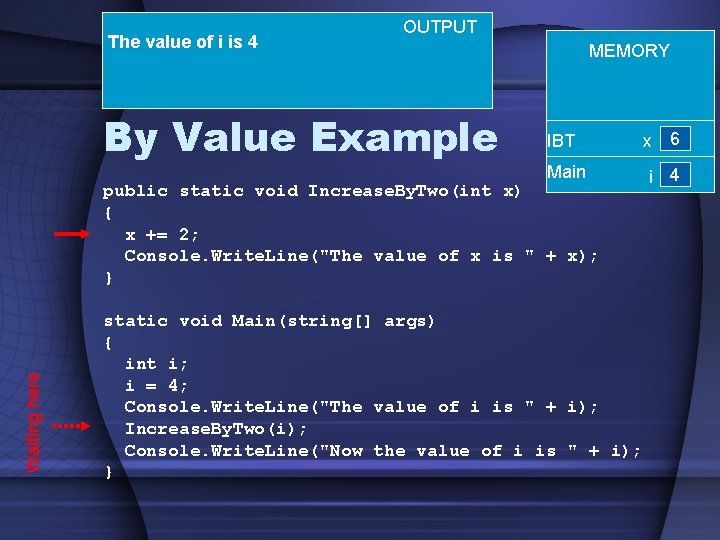
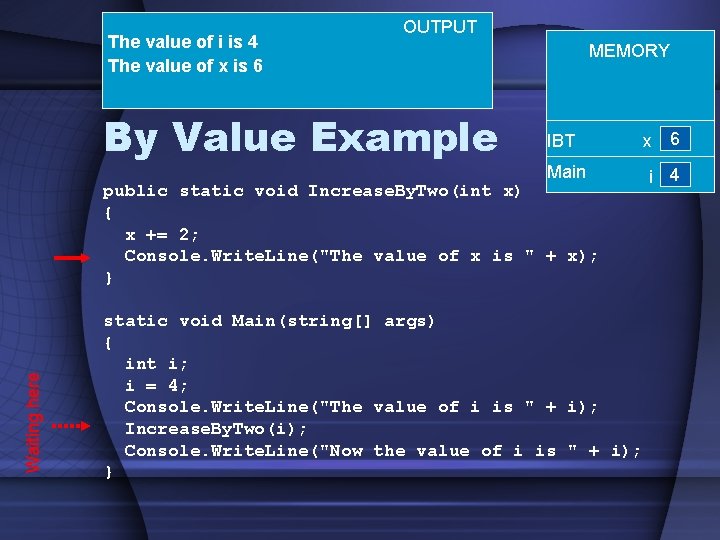
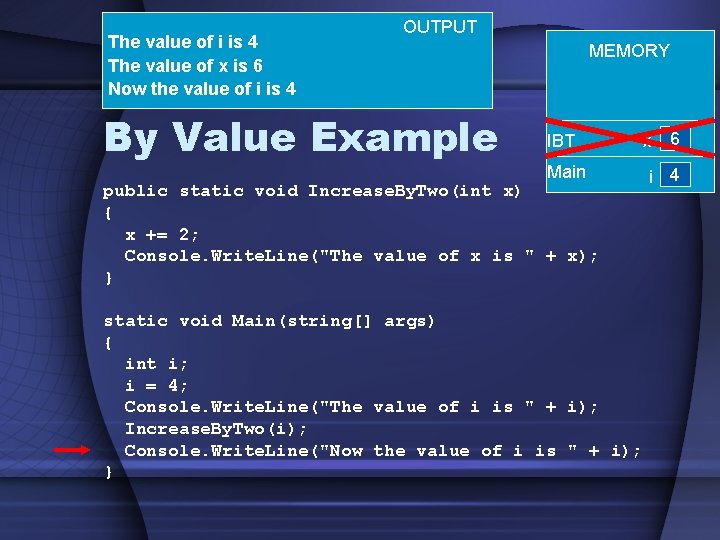
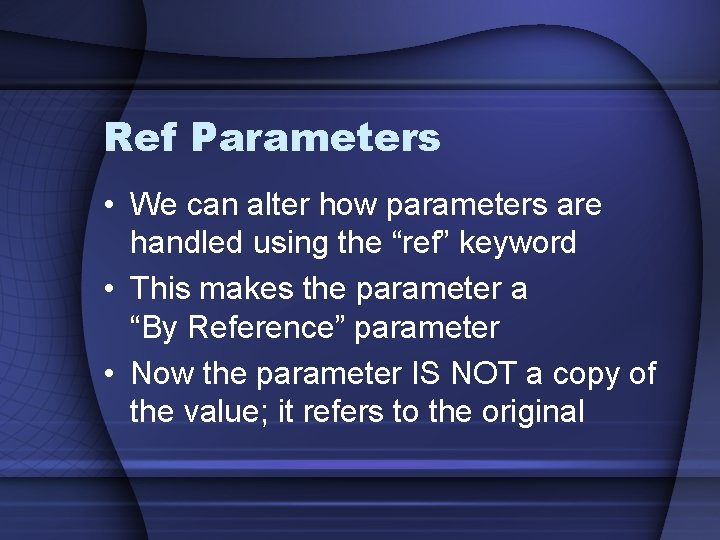
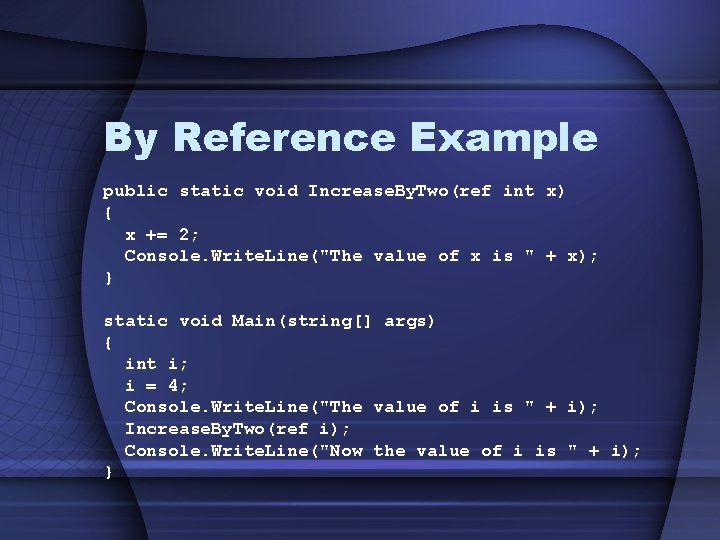
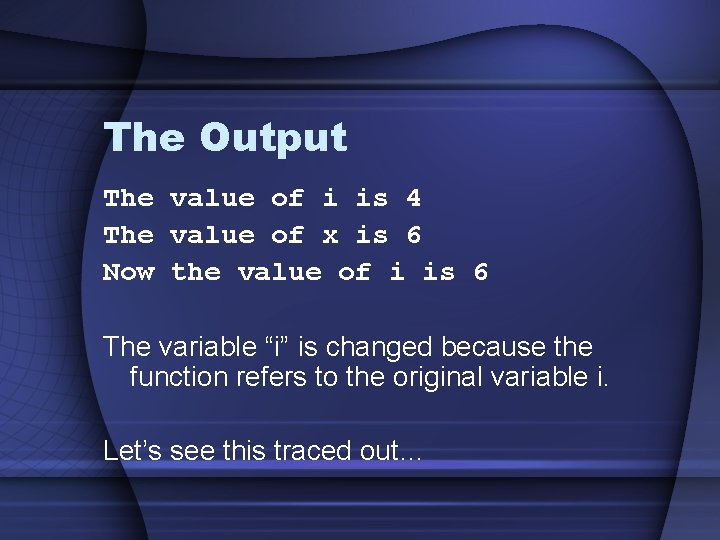
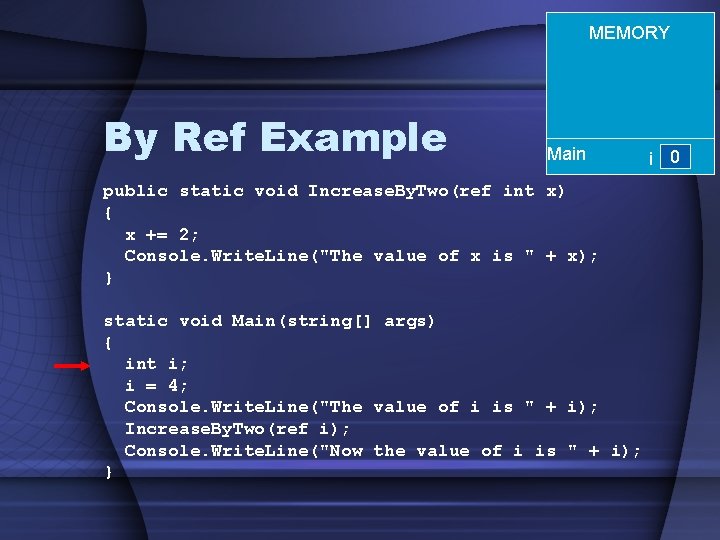
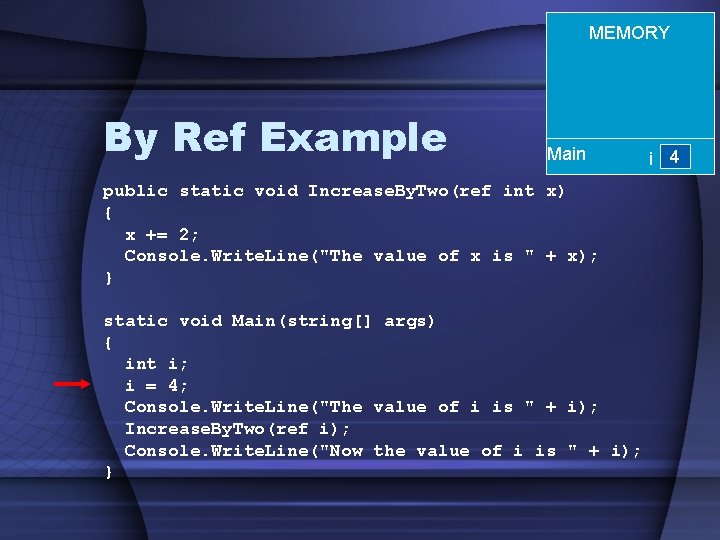
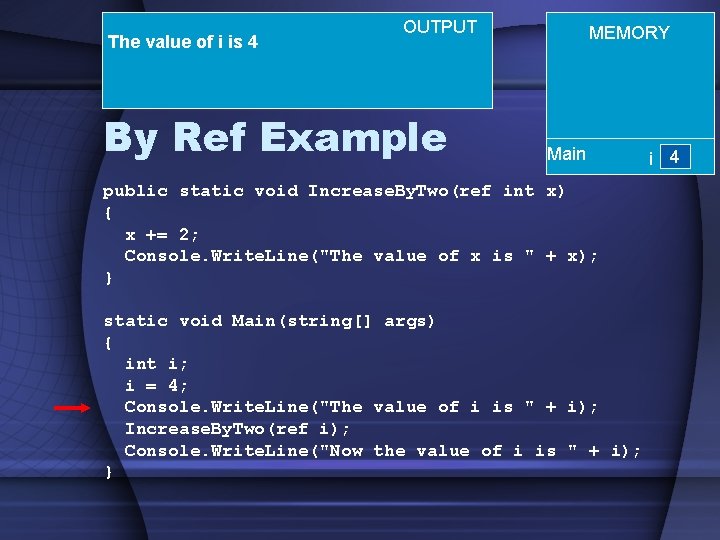
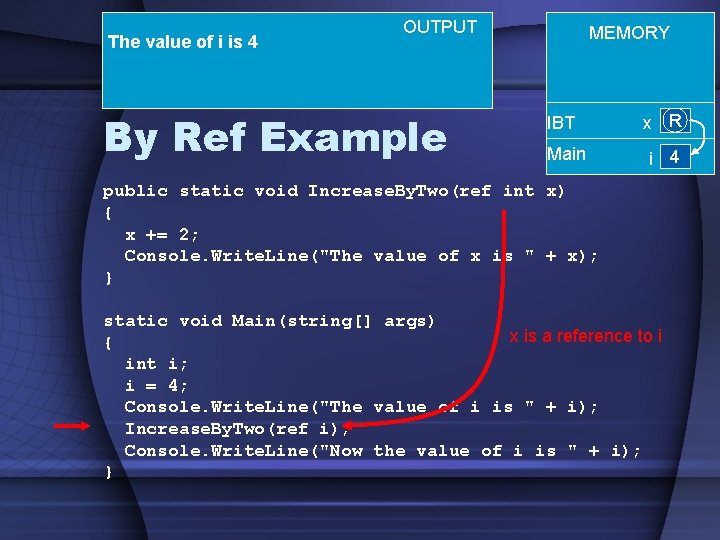
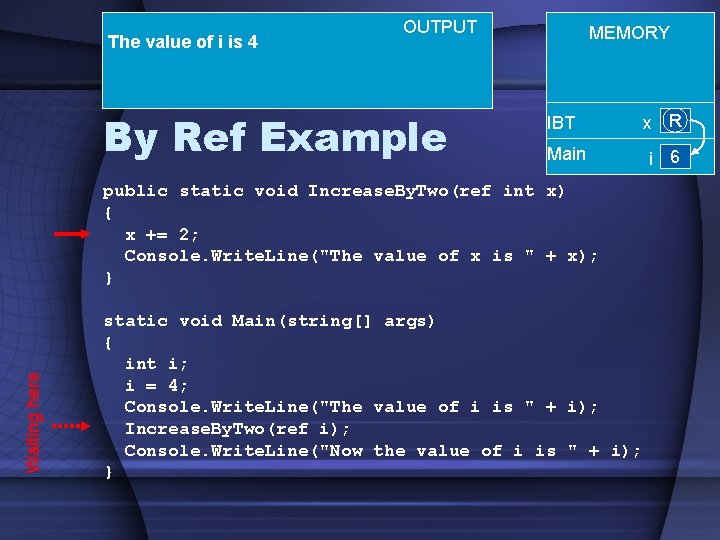
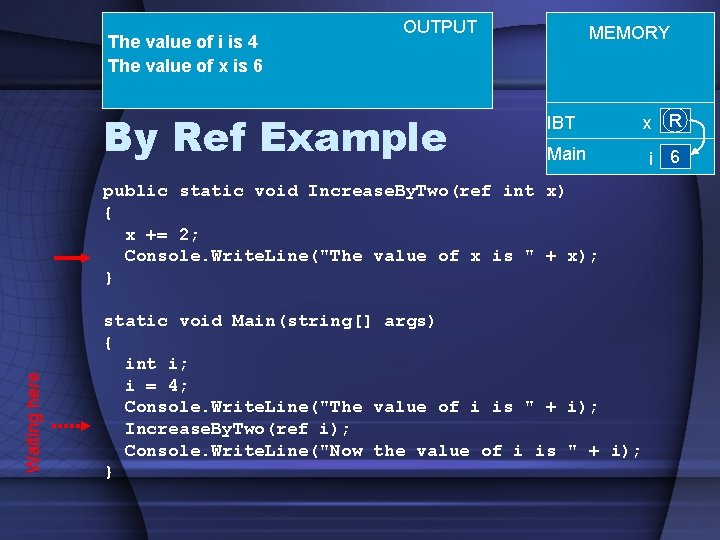
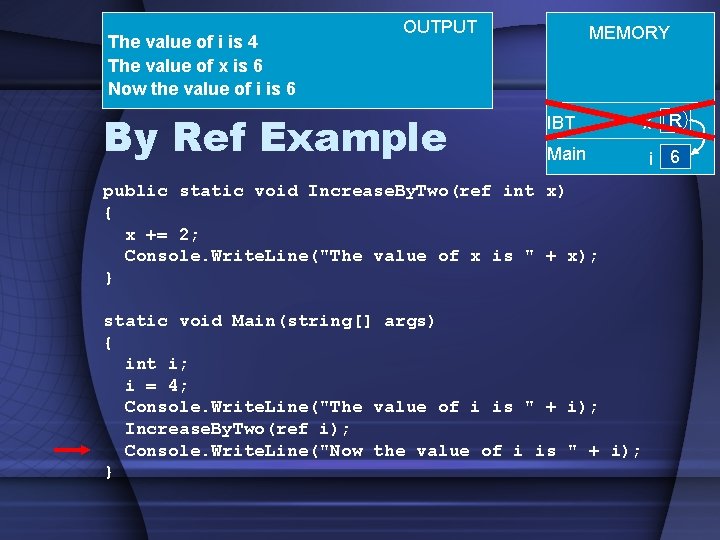
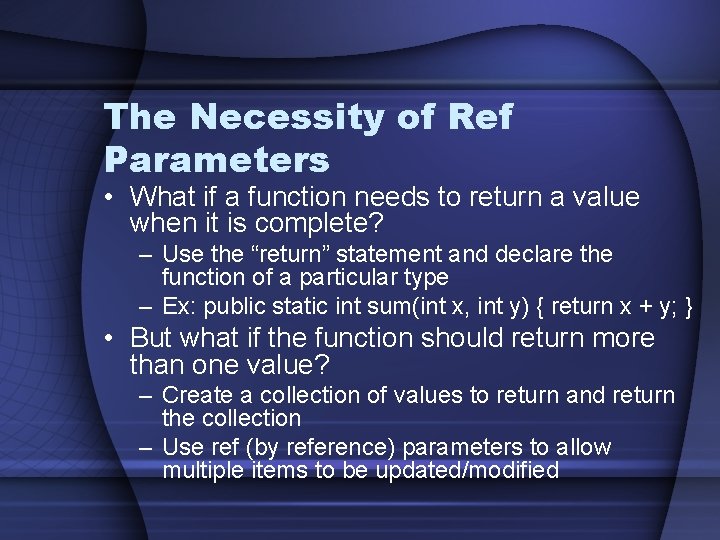
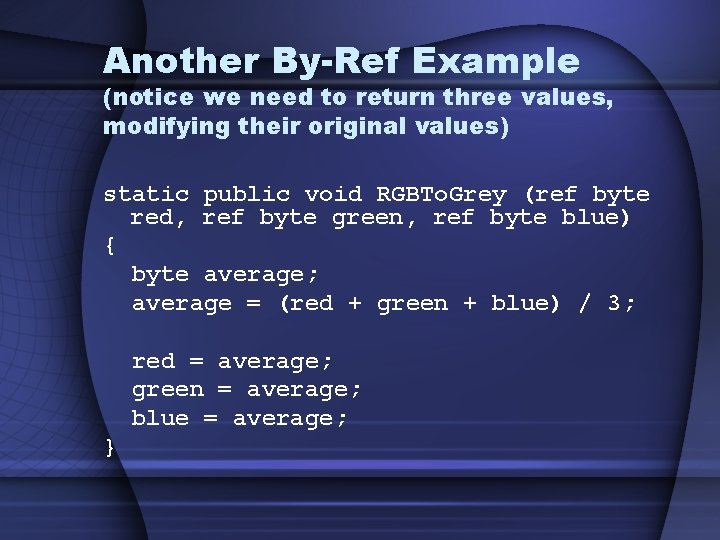
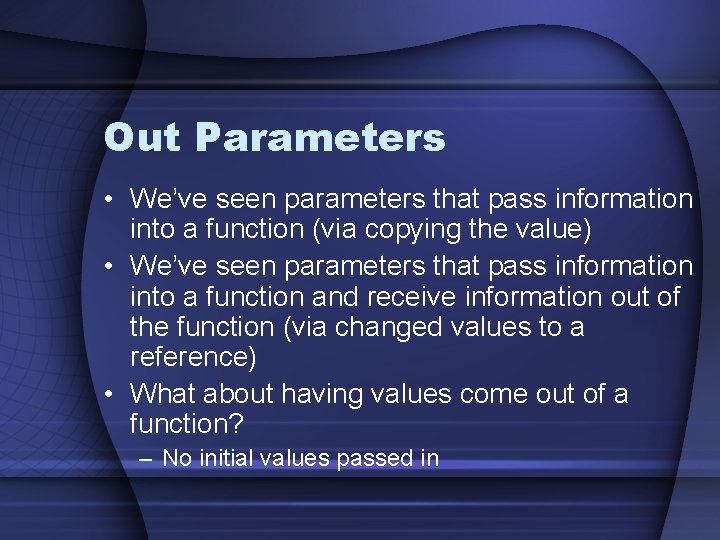
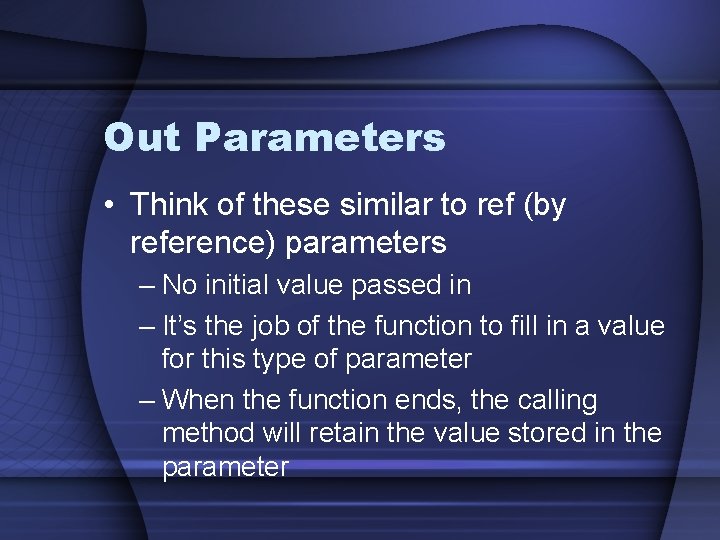
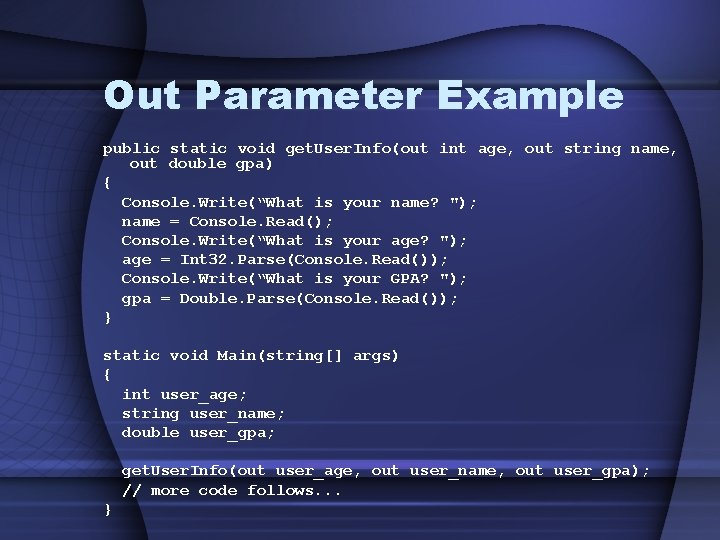
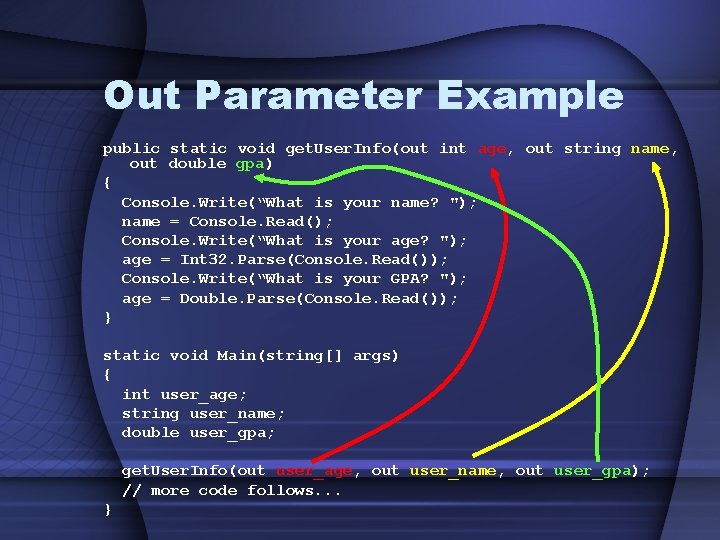
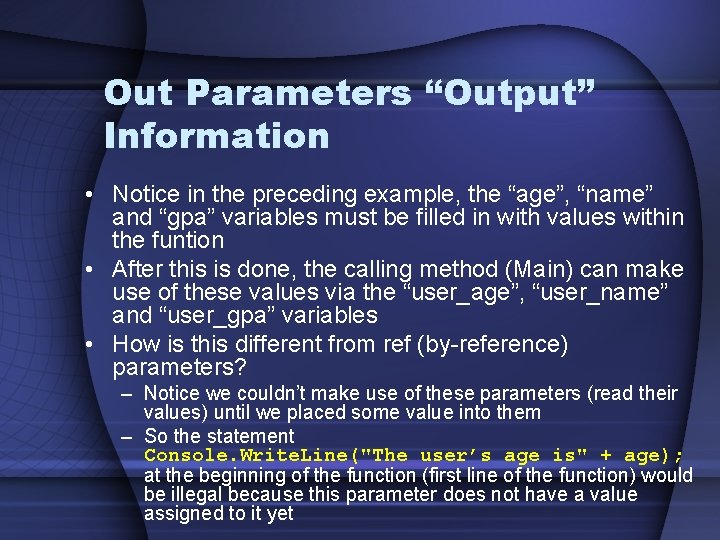
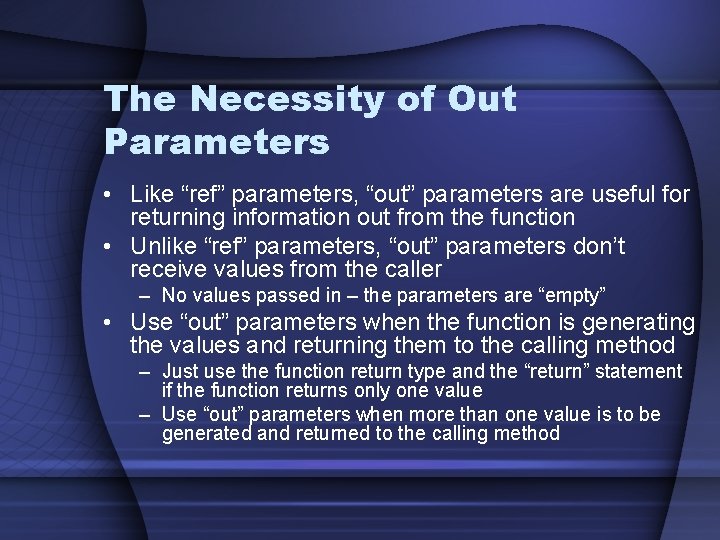
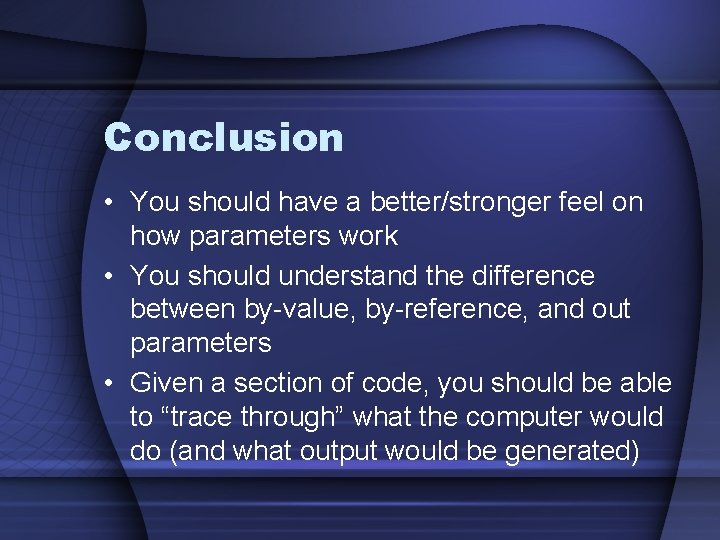
- Slides: 35
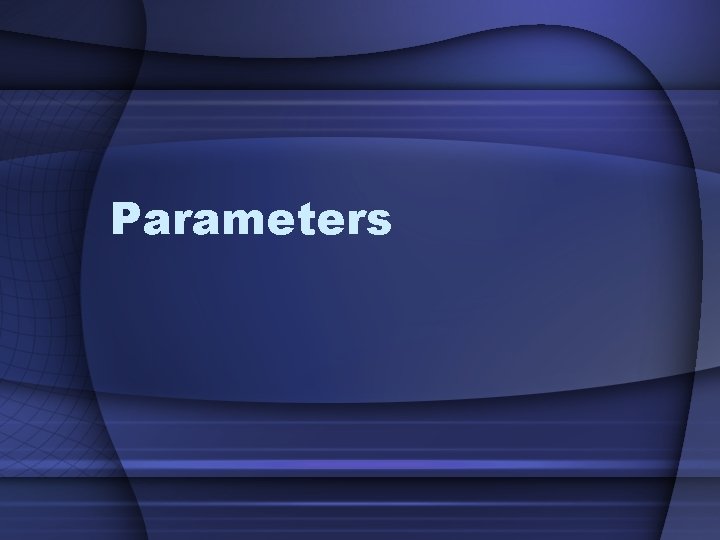
Parameters
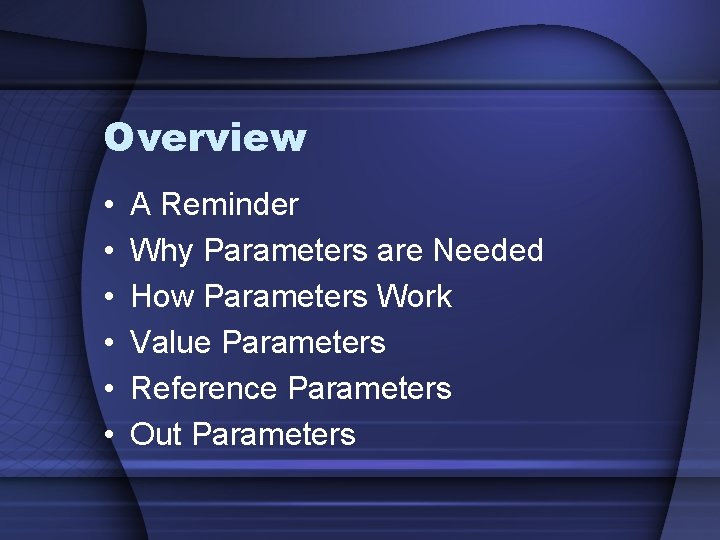
Overview • • • A Reminder Why Parameters are Needed How Parameters Work Value Parameters Reference Parameters Out Parameters
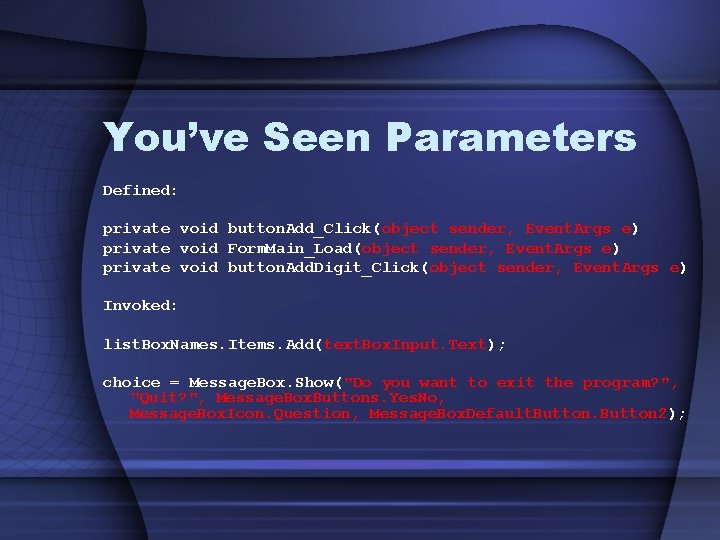
You’ve Seen Parameters Defined: private void button. Add_Click(object sender, Event. Args e) private void Form. Main_Load(object sender, Event. Args e) private void button. Add. Digit_Click(object sender, Event. Args e) Invoked: list. Box. Names. Items. Add(text. Box. Input. Text); choice = Message. Box. Show("Do you want to exit the program? ", "Quit? ", Message. Box. Buttons. Yes. No, Message. Box. Icon. Question, Message. Box. Default. Button 2);
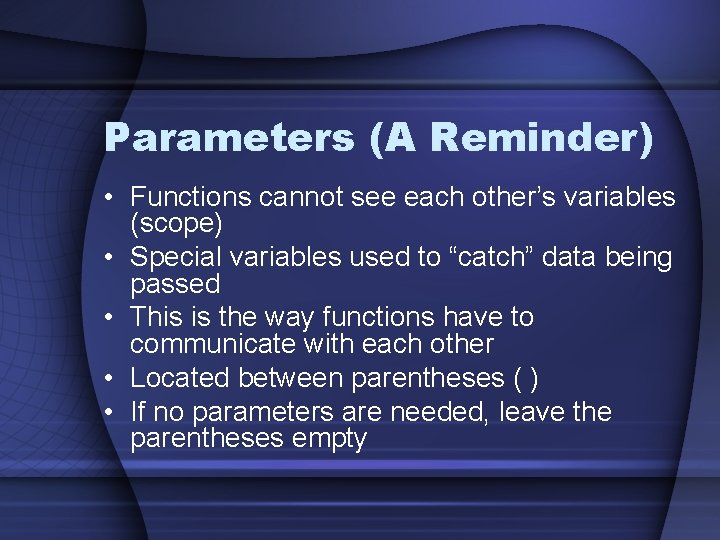
Parameters (A Reminder) • Functions cannot see each other’s variables (scope) • Special variables used to “catch” data being passed • This is the way functions have to communicate with each other • Located between parentheses ( ) • If no parameters are needed, leave the parentheses empty
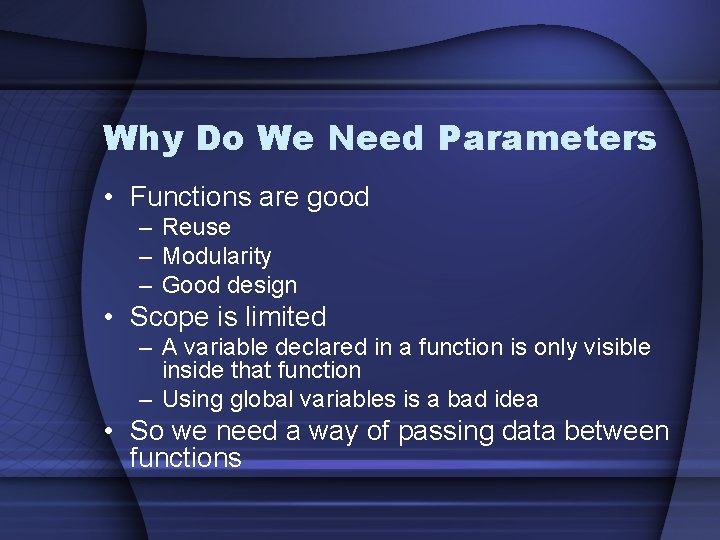
Why Do We Need Parameters • Functions are good – Reuse – Modularity – Good design • Scope is limited – A variable declared in a function is only visible inside that function – Using global variables is a bad idea • So we need a way of passing data between functions
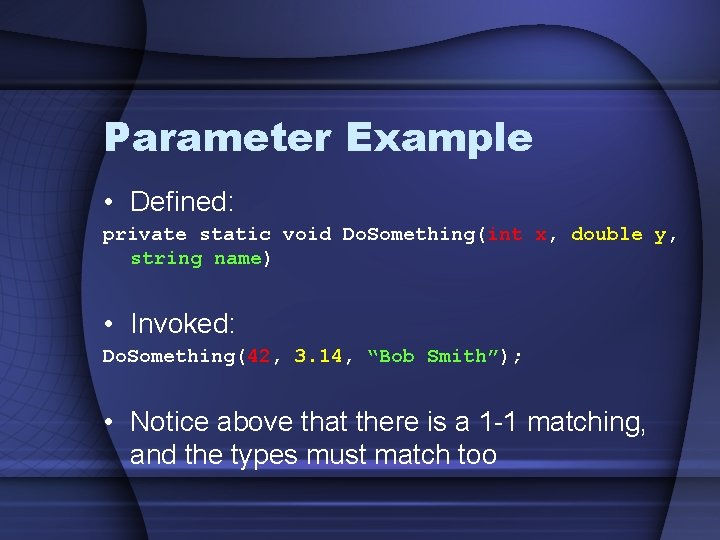
Parameter Example • Defined: private static void Do. Something(int x, double y, string name) • Invoked: Do. Something(42, 3. 14, “Bob Smith”); • Notice above that there is a 1 -1 matching, and the types must match too
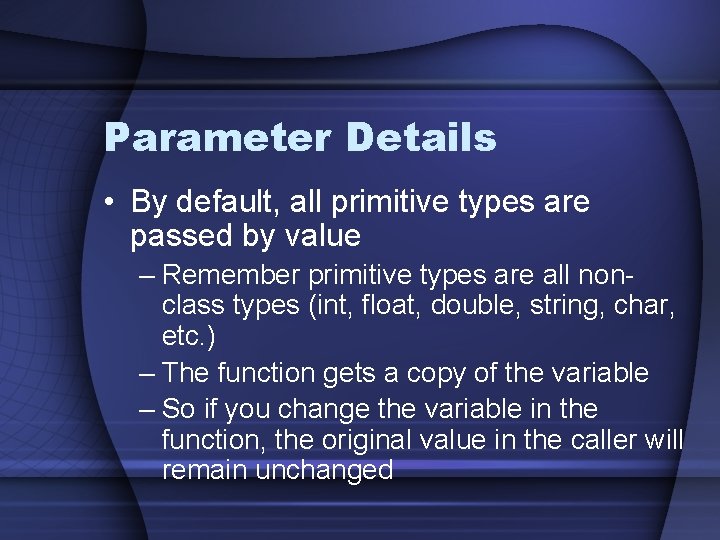
Parameter Details • By default, all primitive types are passed by value – Remember primitive types are all nonclass types (int, float, double, string, char, etc. ) – The function gets a copy of the variable – So if you change the variable in the function, the original value in the caller will remain unchanged
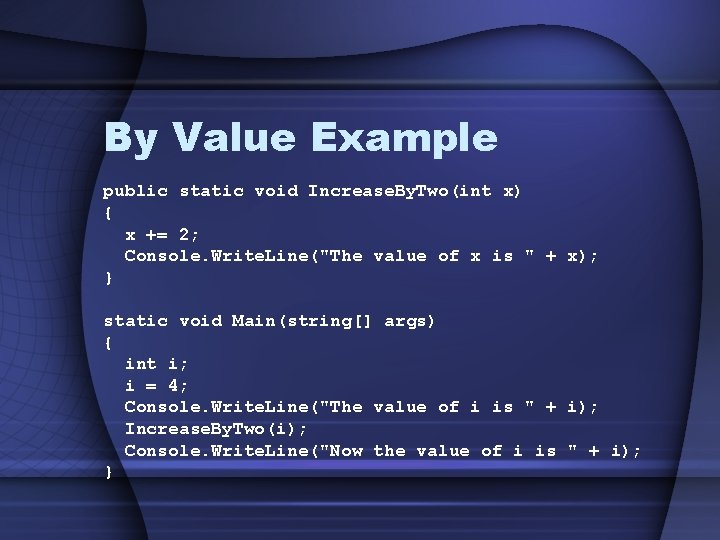
By Value Example public static void Increase. By. Two(int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(i); Console. Write. Line("Now the value of i is " + i); }
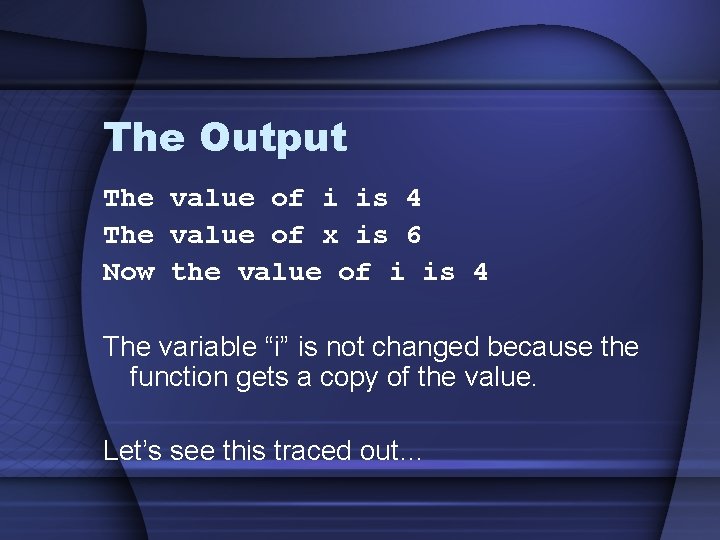
The Output The value of i is 4 The value of x is 6 Now the value of i is 4 The variable “i” is not changed because the function gets a copy of the value. Let’s see this traced out…
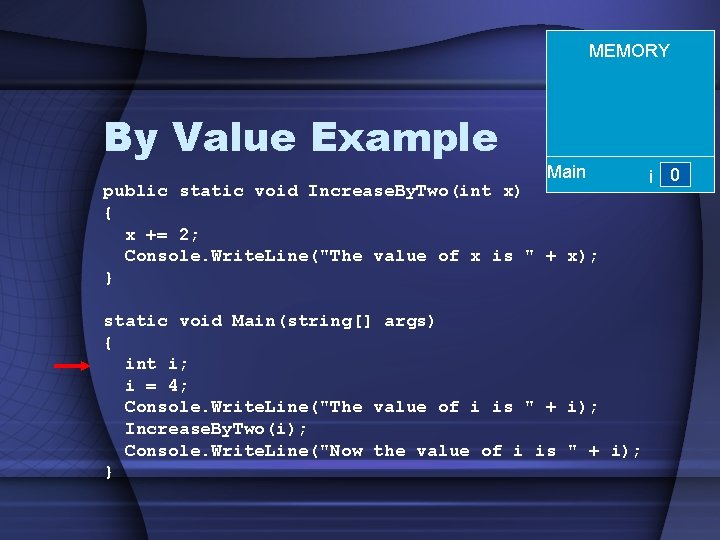
MEMORY By Value Example Main public static void Increase. By. Two(int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(i); Console. Write. Line("Now the value of i is " + i); } i 0
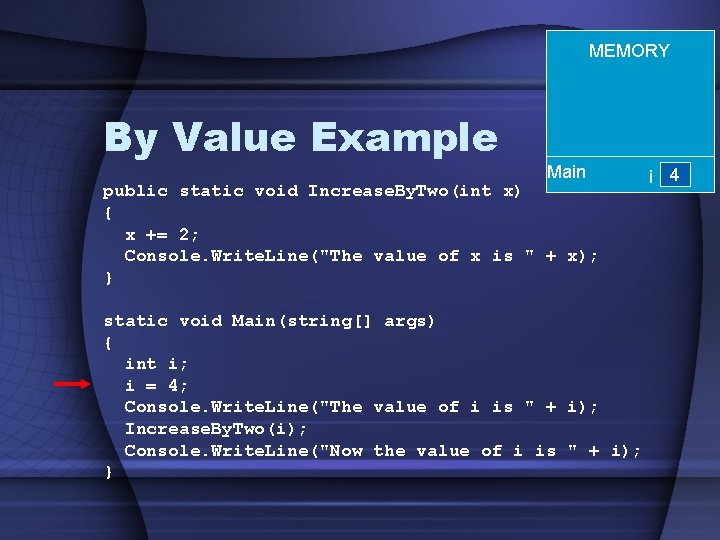
MEMORY By Value Example Main public static void Increase. By. Two(int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(i); Console. Write. Line("Now the value of i is " + i); } i 4
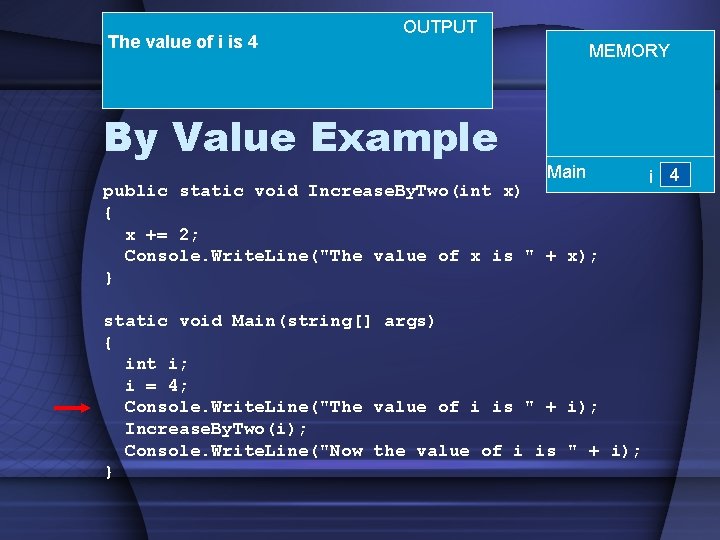
The value of i is 4 OUTPUT By Value Example MEMORY Main public static void Increase. By. Two(int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(i); Console. Write. Line("Now the value of i is " + i); } i 4
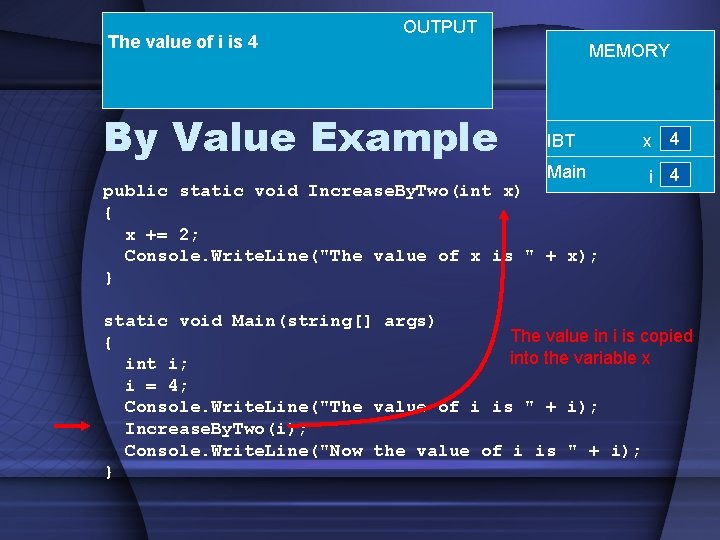
The value of i is 4 OUTPUT By Value Example MEMORY IBT Main public static void Increase. By. Two(int x) { x += 2; Console. Write. Line("The value of x is " + x); } x 4 i 4 static void Main(string[] args) The value in i is copied { into the variable x int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(i); Console. Write. Line("Now the value of i is " + i); }
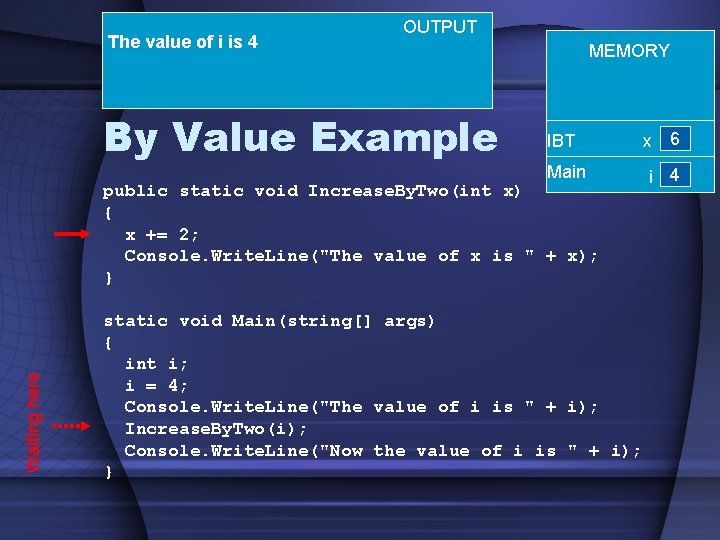
The value of i is 4 OUTPUT By Value Example MEMORY IBT Main Waiting here public static void Increase. By. Two(int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(i); Console. Write. Line("Now the value of i is " + i); } x 6 i 4
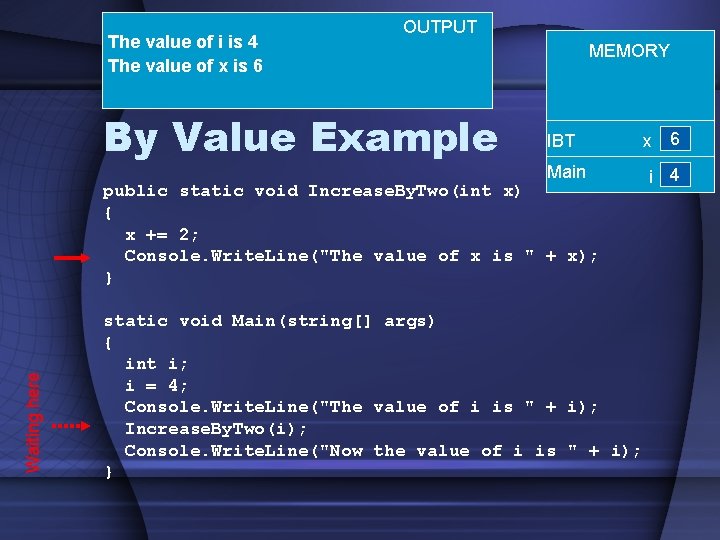
The value of i is 4 The value of x is 6 OUTPUT By Value Example MEMORY IBT Main Waiting here public static void Increase. By. Two(int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(i); Console. Write. Line("Now the value of i is " + i); } x 6 i 4
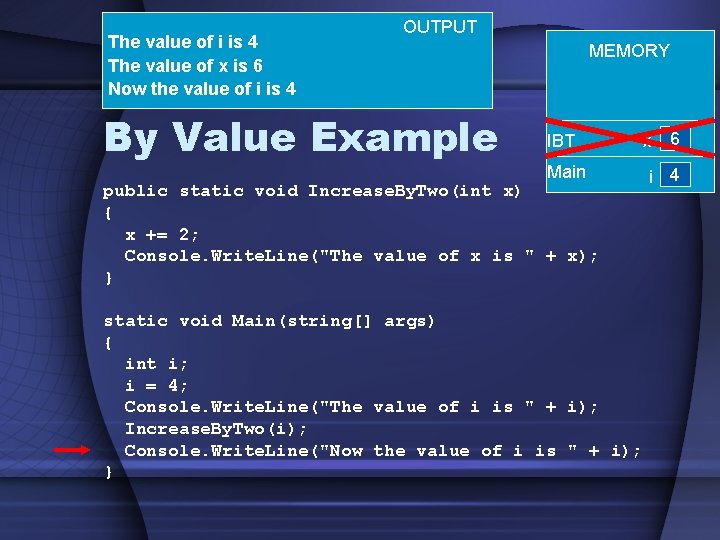
The value of i is 4 The value of x is 6 Now the value of i is 4 OUTPUT By Value Example MEMORY IBT Main public static void Increase. By. Two(int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(i); Console. Write. Line("Now the value of i is " + i); } x 6 i 4
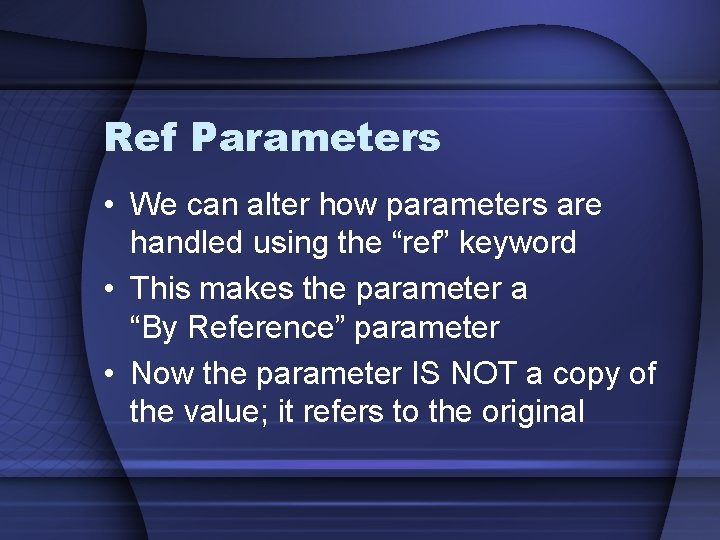
Ref Parameters • We can alter how parameters are handled using the “ref” keyword • This makes the parameter a “By Reference” parameter • Now the parameter IS NOT a copy of the value; it refers to the original
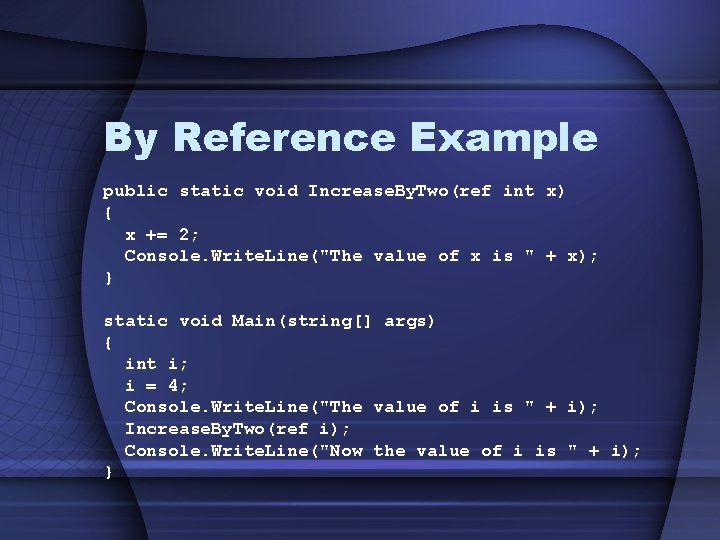
By Reference Example public static void Increase. By. Two(ref int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(ref i); Console. Write. Line("Now the value of i is " + i); }
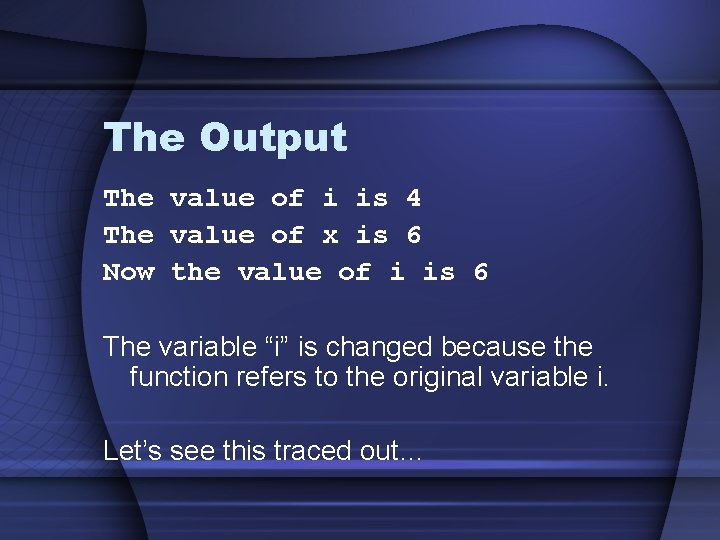
The Output The value of i is 4 The value of x is 6 Now the value of i is 6 The variable “i” is changed because the function refers to the original variable i. Let’s see this traced out…
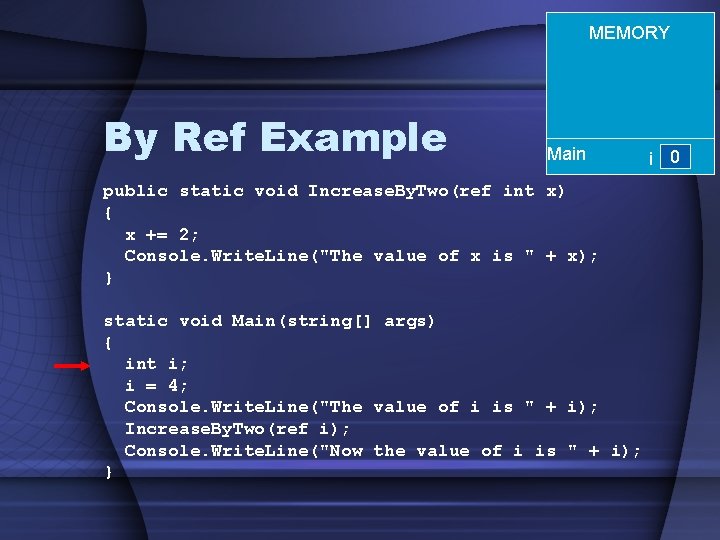
MEMORY By Ref Example Main public static void Increase. By. Two(ref int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(ref i); Console. Write. Line("Now the value of i is " + i); } i 0
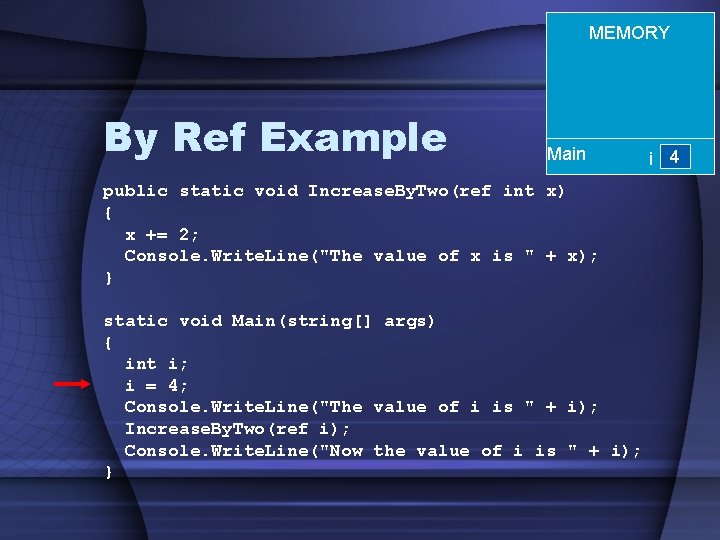
MEMORY By Ref Example Main public static void Increase. By. Two(ref int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(ref i); Console. Write. Line("Now the value of i is " + i); } i 4
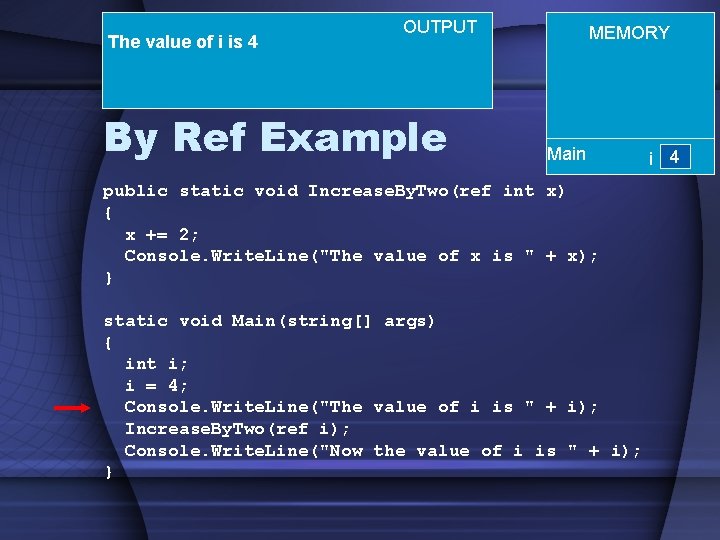
The value of i is 4 OUTPUT By Ref Example MEMORY Main public static void Increase. By. Two(ref int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(ref i); Console. Write. Line("Now the value of i is " + i); } i 4
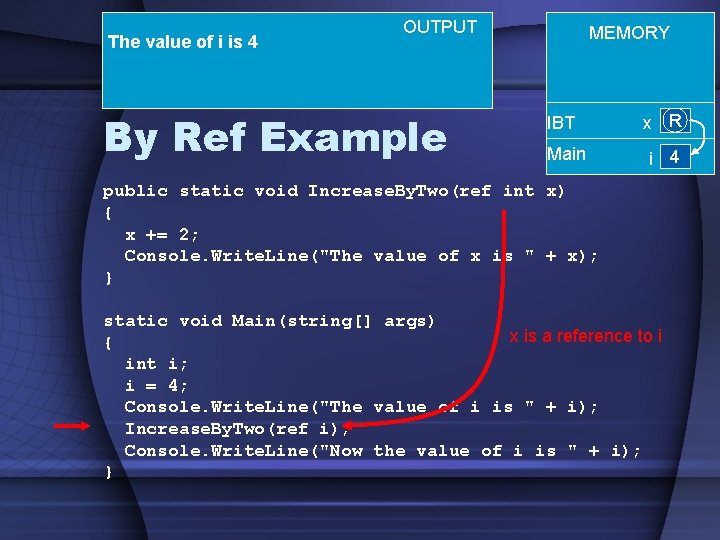
The value of i is 4 OUTPUT By Ref Example MEMORY IBT Main x R i 4 public static void Increase. By. Two(ref int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) x is a reference to i { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(ref i); Console. Write. Line("Now the value of i is " + i); }
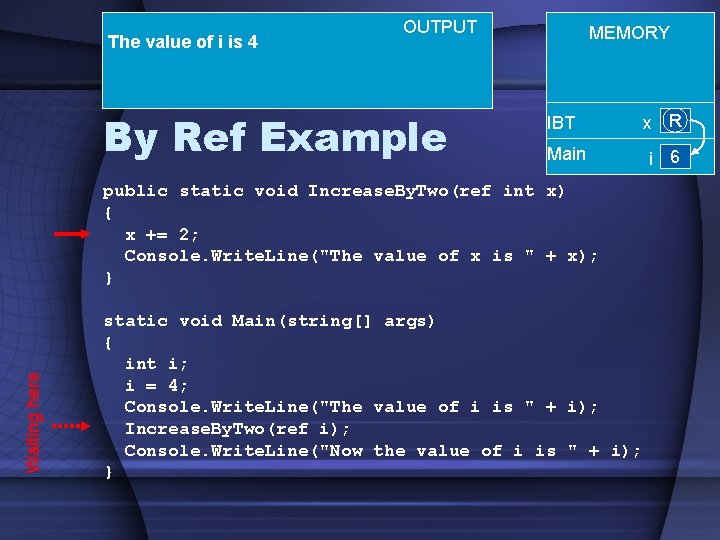
The value of i is 4 OUTPUT By Ref Example MEMORY IBT Main Waiting here public static void Increase. By. Two(ref int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(ref i); Console. Write. Line("Now the value of i is " + i); } x R i 6
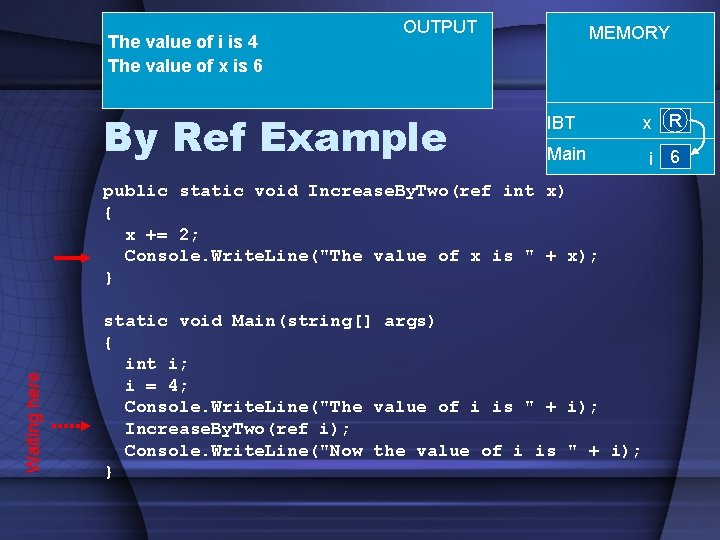
The value of i is 4 The value of x is 6 OUTPUT By Ref Example MEMORY IBT Main Waiting here public static void Increase. By. Two(ref int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(ref i); Console. Write. Line("Now the value of i is " + i); } x R i 6
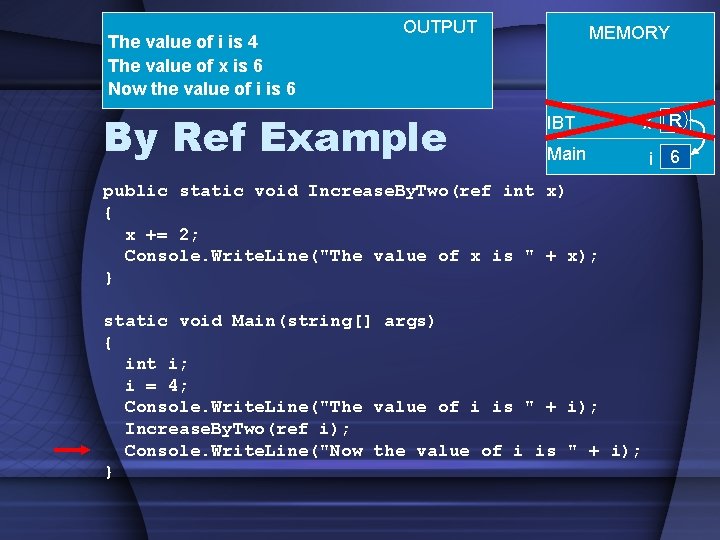
The value of i is 4 The value of x is 6 Now the value of i is 6 OUTPUT By Ref Example MEMORY IBT Main public static void Increase. By. Two(ref int x) { x += 2; Console. Write. Line("The value of x is " + x); } static void Main(string[] args) { int i; i = 4; Console. Write. Line("The value of i is " + i); Increase. By. Two(ref i); Console. Write. Line("Now the value of i is " + i); } x R i 6
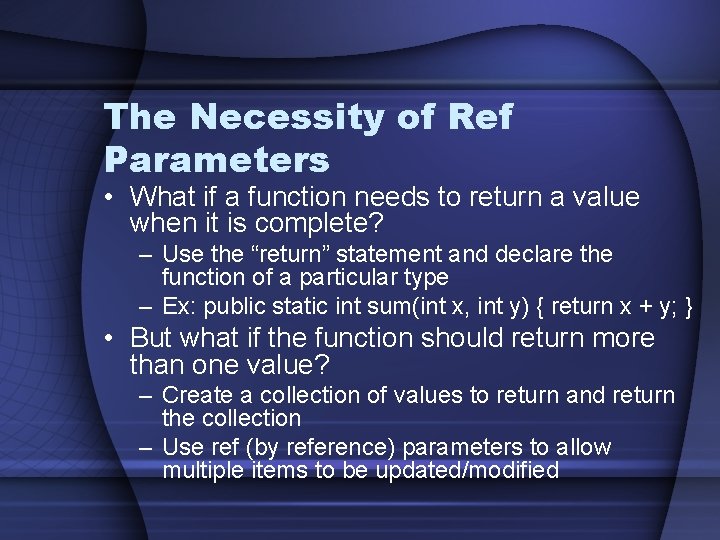
The Necessity of Ref Parameters • What if a function needs to return a value when it is complete? – Use the “return” statement and declare the function of a particular type – Ex: public static int sum(int x, int y) { return x + y; } • But what if the function should return more than one value? – Create a collection of values to return and return the collection – Use ref (by reference) parameters to allow multiple items to be updated/modified
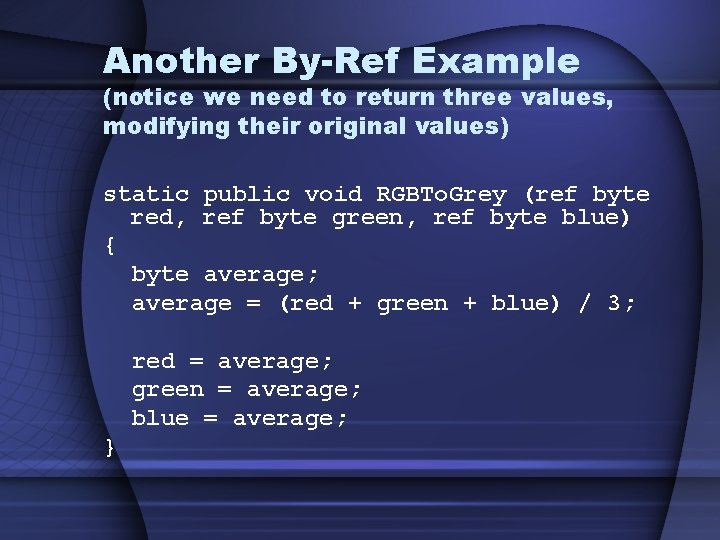
Another By-Ref Example (notice we need to return three values, modifying their original values) static public void RGBTo. Grey (ref byte red, ref byte green, ref byte blue) { byte average; average = (red + green + blue) / 3; red = average; green = average; blue = average; }
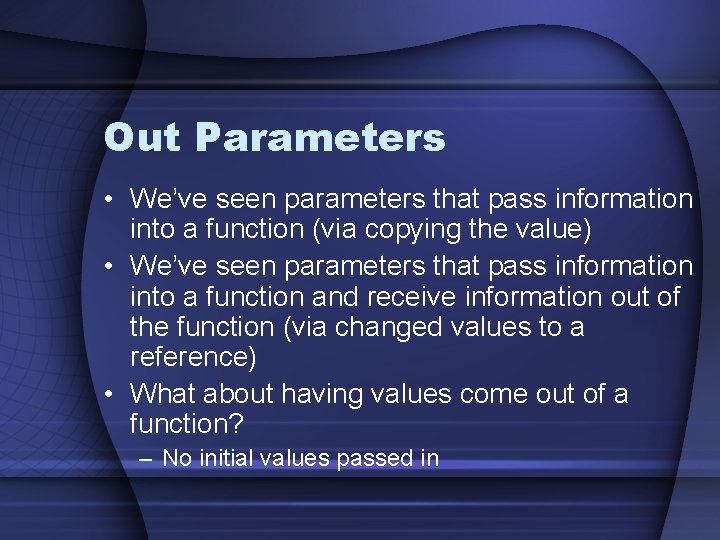
Out Parameters • We’ve seen parameters that pass information into a function (via copying the value) • We’ve seen parameters that pass information into a function and receive information out of the function (via changed values to a reference) • What about having values come out of a function? – No initial values passed in
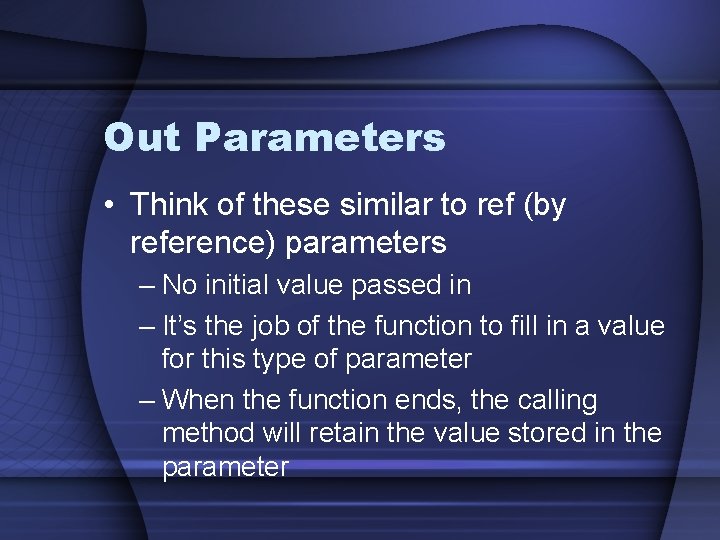
Out Parameters • Think of these similar to ref (by reference) parameters – No initial value passed in – It’s the job of the function to fill in a value for this type of parameter – When the function ends, the calling method will retain the value stored in the parameter
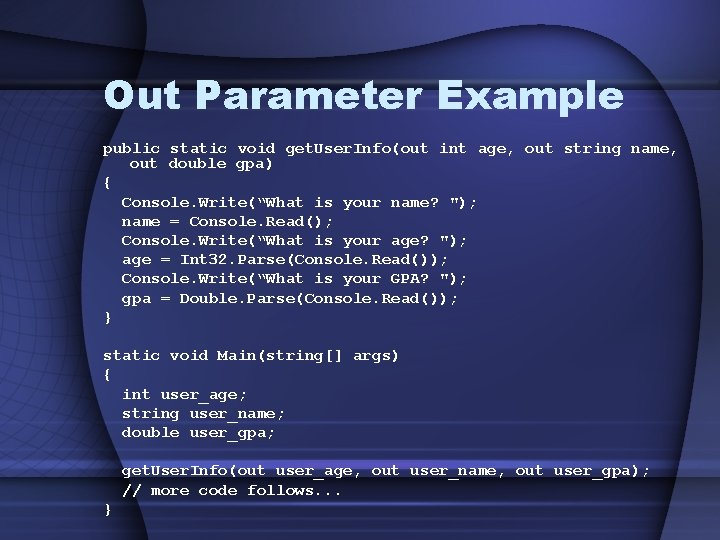
Out Parameter Example public static void get. User. Info(out int age, out string name, out double gpa) { Console. Write(“What is your name? "); name = Console. Read(); Console. Write(“What is your age? "); age = Int 32. Parse(Console. Read()); Console. Write(“What is your GPA? "); gpa = Double. Parse(Console. Read()); } static void Main(string[] args) { int user_age; string user_name; double user_gpa; get. User. Info(out user_age, out user_name, out user_gpa); // more code follows. . . }
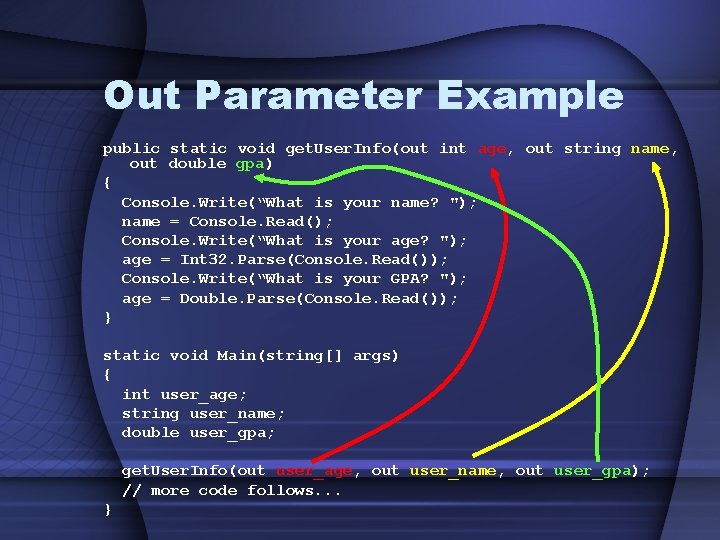
Out Parameter Example public static void get. User. Info(out int age, out string name, out double gpa) { Console. Write(“What is your name? "); name = Console. Read(); Console. Write(“What is your age? "); age = Int 32. Parse(Console. Read()); Console. Write(“What is your GPA? "); age = Double. Parse(Console. Read()); } static void Main(string[] args) { int user_age; string user_name; double user_gpa; get. User. Info(out user_age, out user_name, out user_gpa); // more code follows. . . }
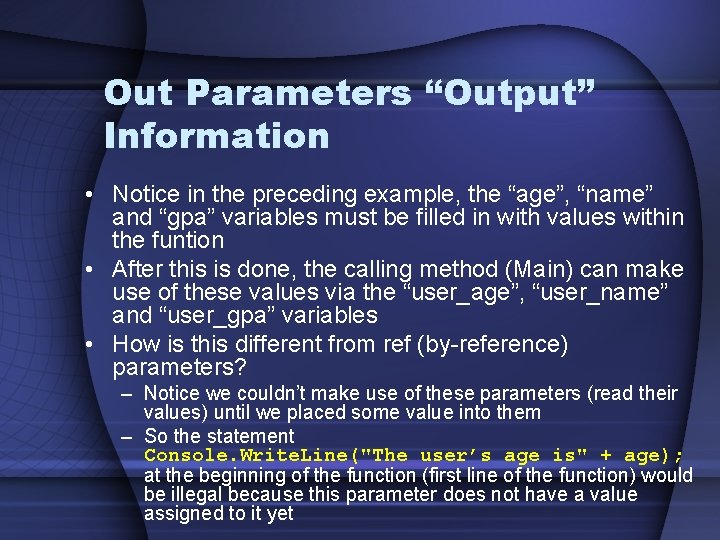
Out Parameters “Output” Information • Notice in the preceding example, the “age”, “name” and “gpa” variables must be filled in with values within the funtion • After this is done, the calling method (Main) can make use of these values via the “user_age”, “user_name” and “user_gpa” variables • How is this different from ref (by-reference) parameters? – Notice we couldn’t make use of these parameters (read their values) until we placed some value into them – So the statement Console. Write. Line("The user’s age is" + age); at the beginning of the function (first line of the function) would be illegal because this parameter does not have a value assigned to it yet
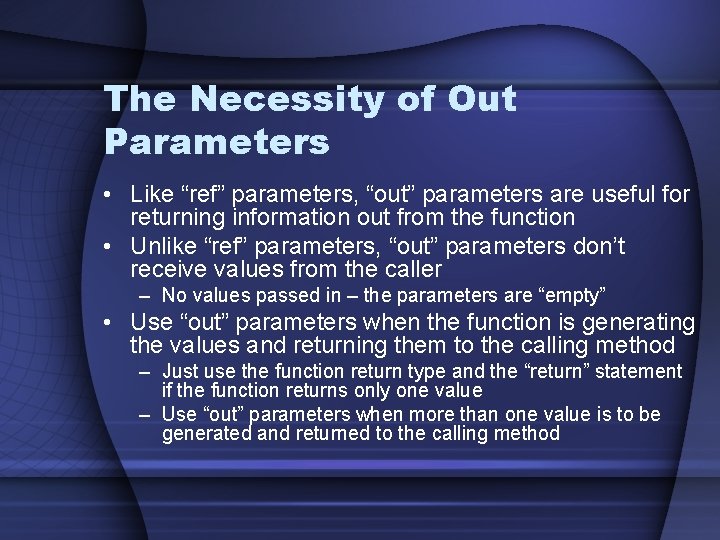
The Necessity of Out Parameters • Like “ref” parameters, “out” parameters are useful for returning information out from the function • Unlike “ref” parameters, “out” parameters don’t receive values from the caller – No values passed in – the parameters are “empty” • Use “out” parameters when the function is generating the values and returning them to the calling method – Just use the function return type and the “return” statement if the function returns only one value – Use “out” parameters when more than one value is to be generated and returned to the calling method
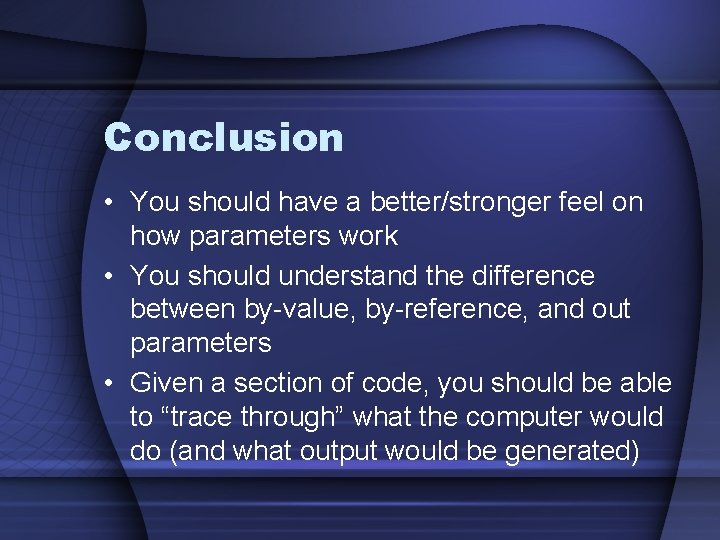
Conclusion • You should have a better/stronger feel on how parameters work • You should understand the difference between by-value, by-reference, and out parameters • Given a section of code, you should be able to “trace through” what the computer would do (and what output would be generated)