Parameter Example A value passed into a method
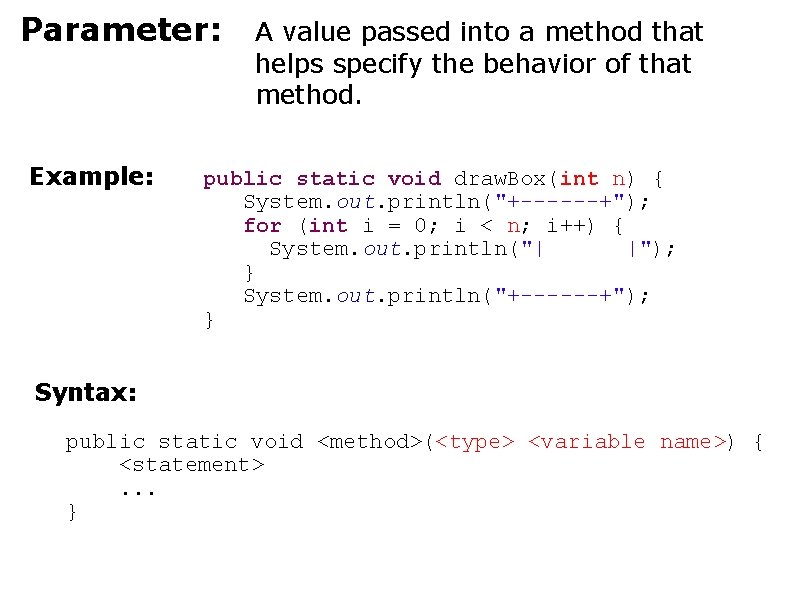
![public static void main(String[] args) { for (int row = 1; row <= 6; public static void main(String[] args) { for (int row = 1; row <= 6;](https://slidetodoc.com/presentation_image_h2/bce85a0ee3082f6869e52d5f68411dca/image-2.jpg)
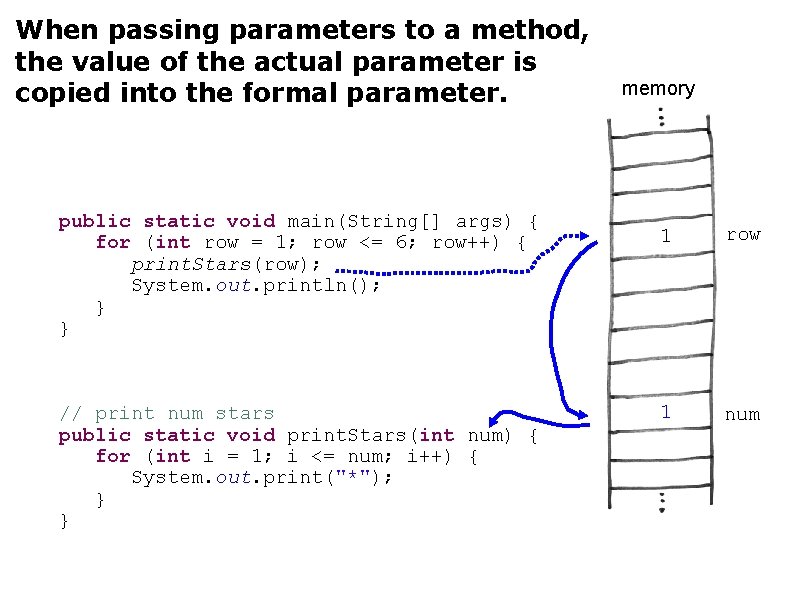
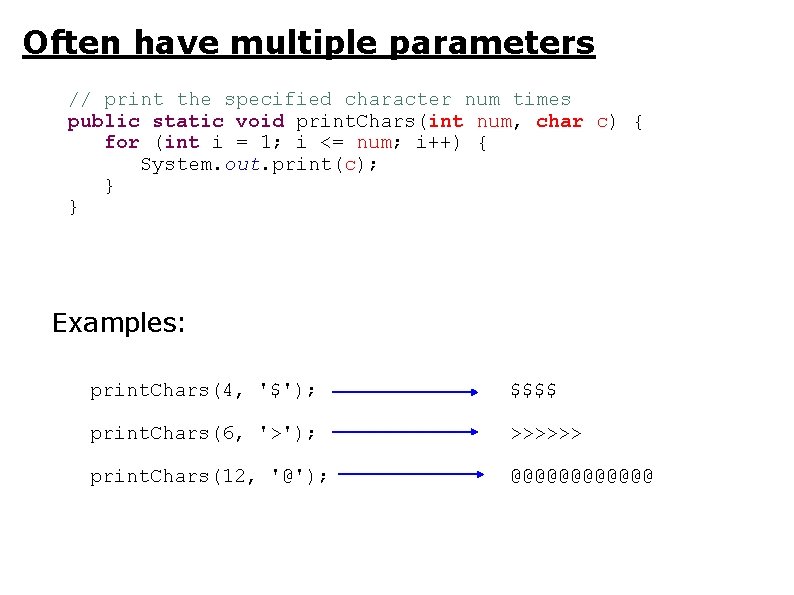
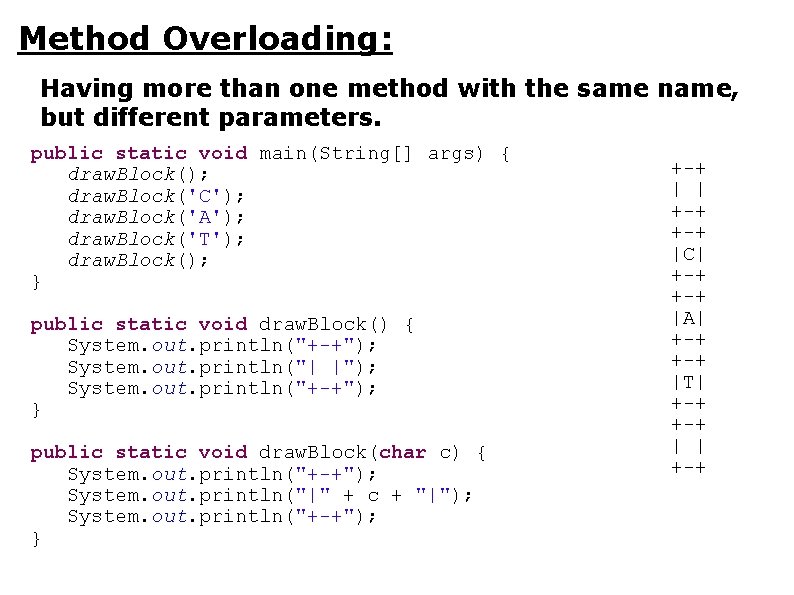
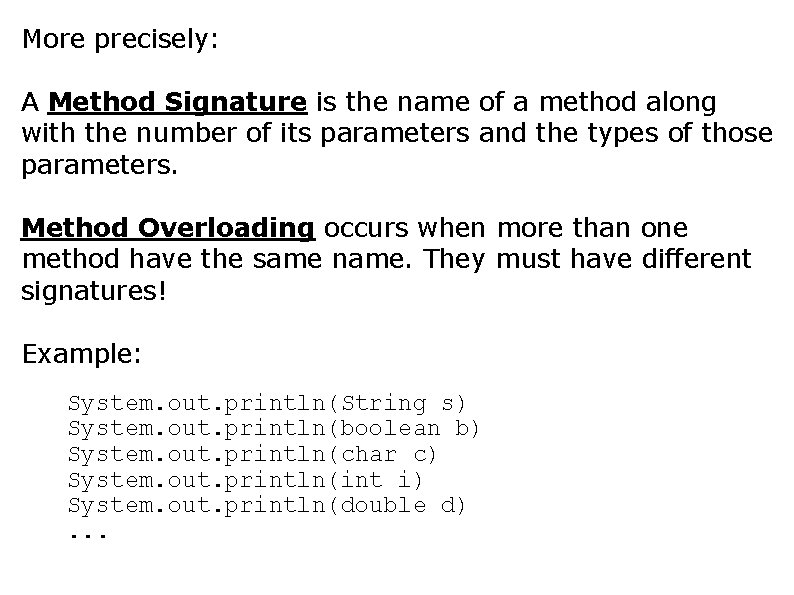
![Methods can return a result to the caller. Example: public static void main(String[] args) Methods can return a result to the caller. Example: public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/bce85a0ee3082f6869e52d5f68411dca/image-7.jpg)
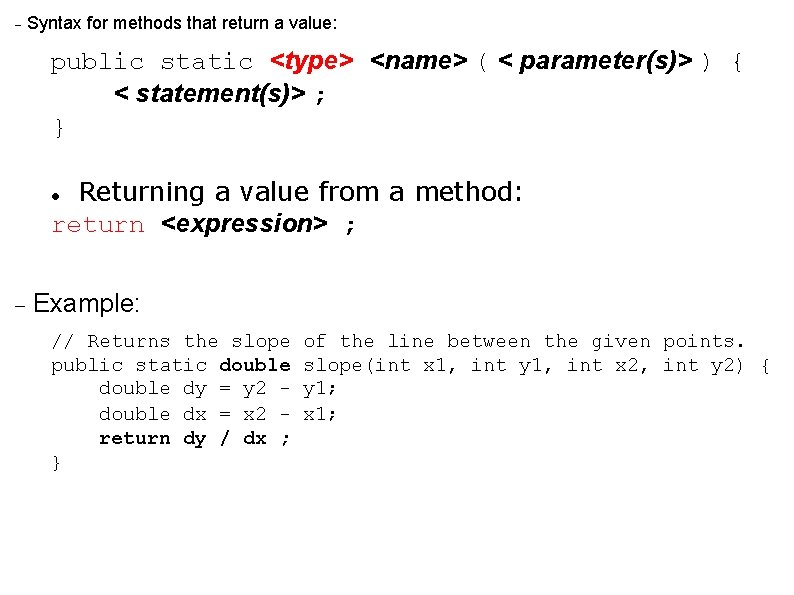
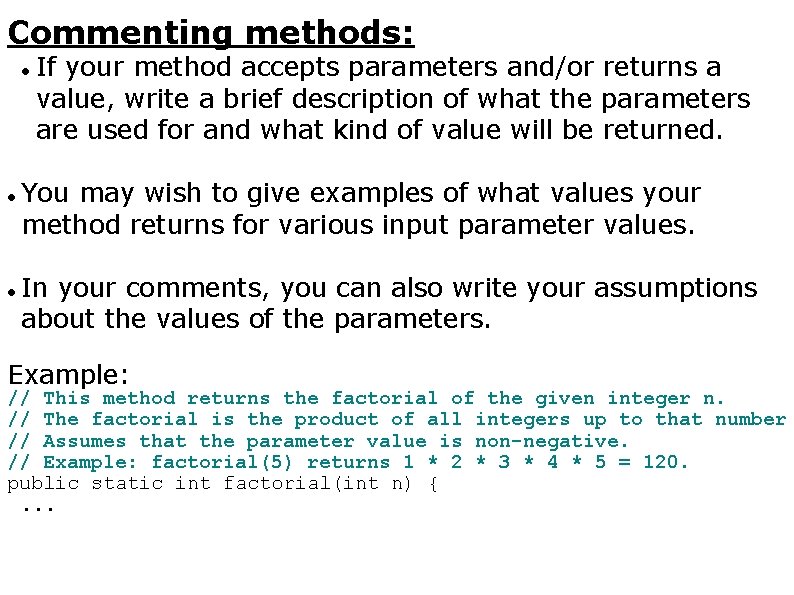
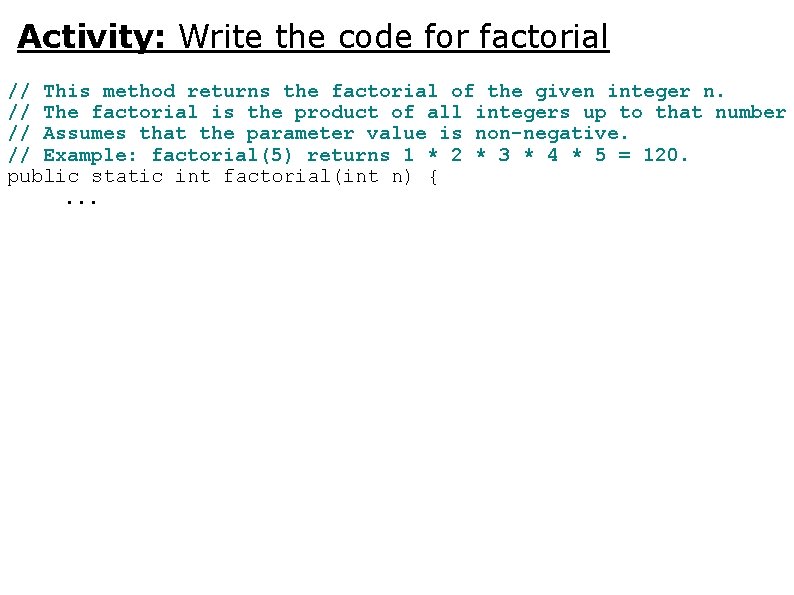
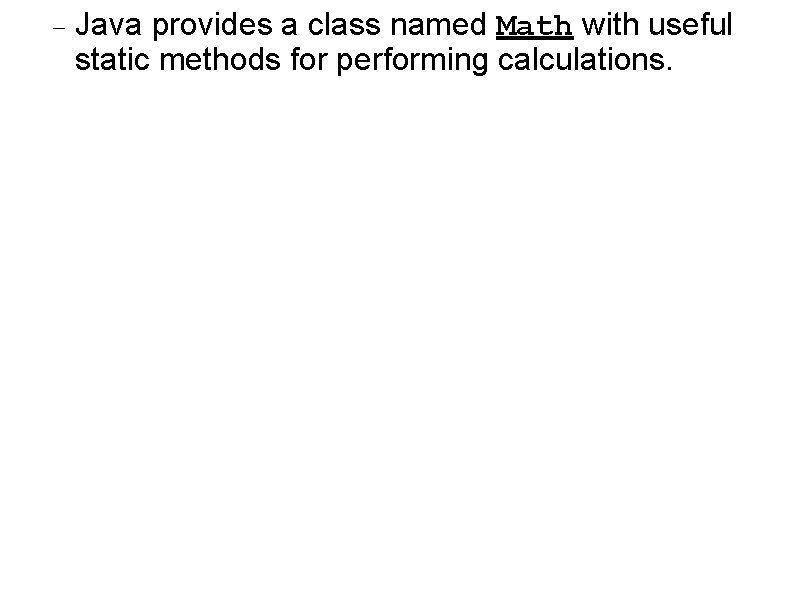
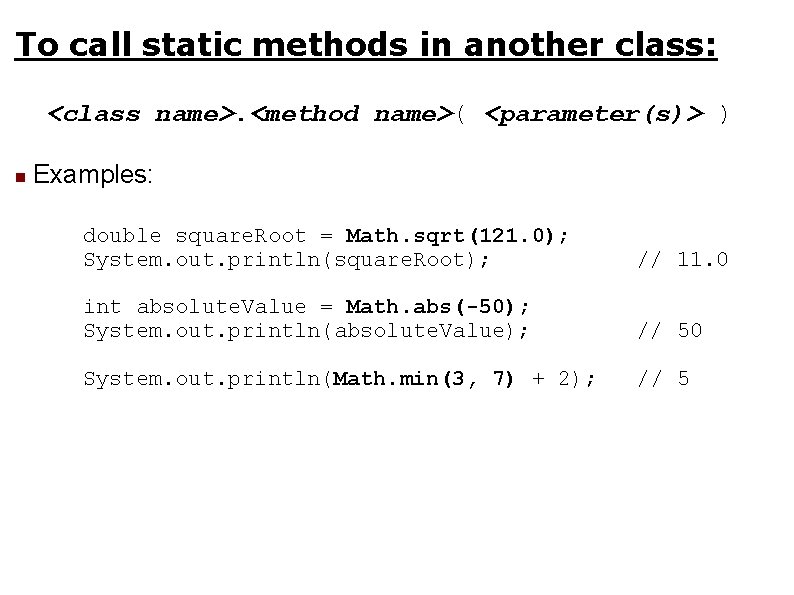
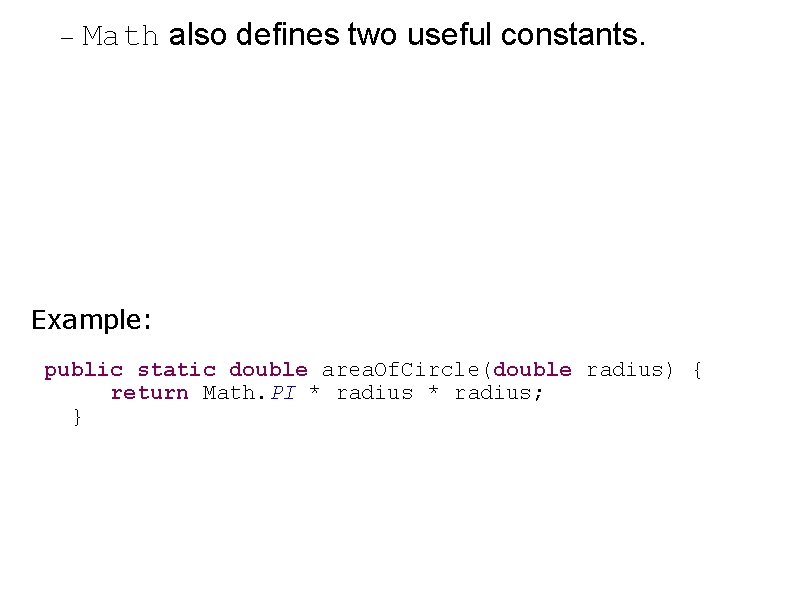
![Example: Printing an old fashion sine table. public static void main(String[] args) { System. Example: Printing an old fashion sine table. public static void main(String[] args) { System.](https://slidetodoc.com/presentation_image_h2/bce85a0ee3082f6869e52d5f68411dca/image-14.jpg)
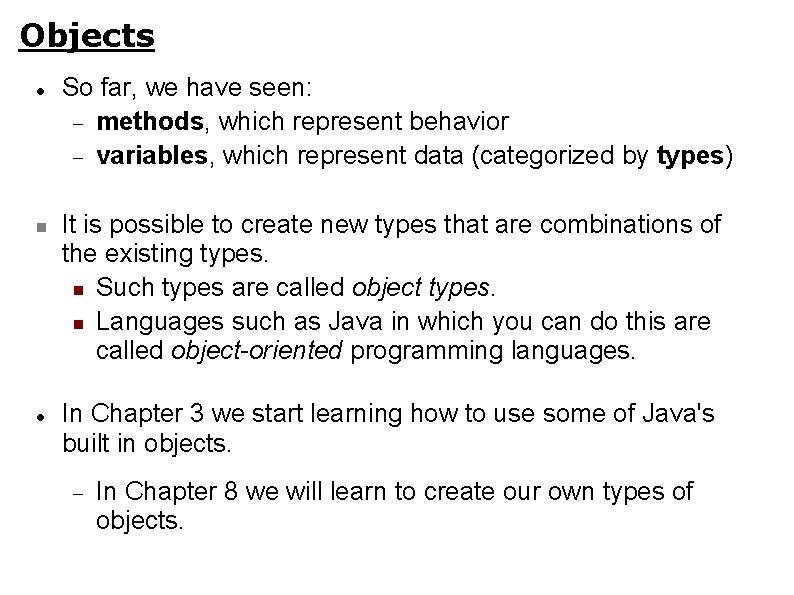
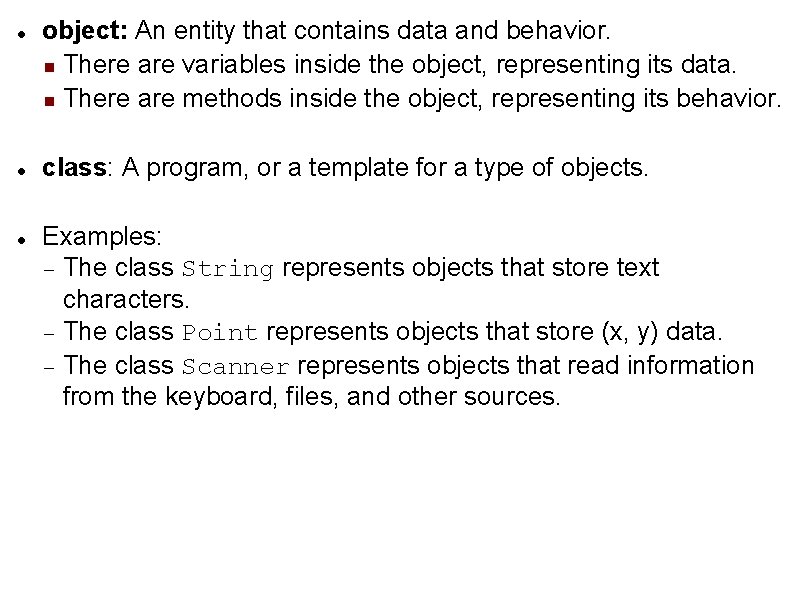
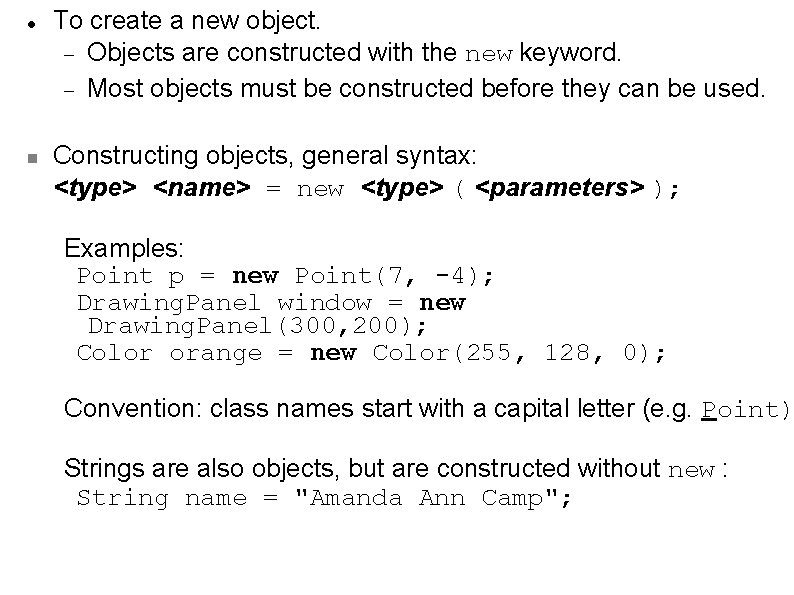
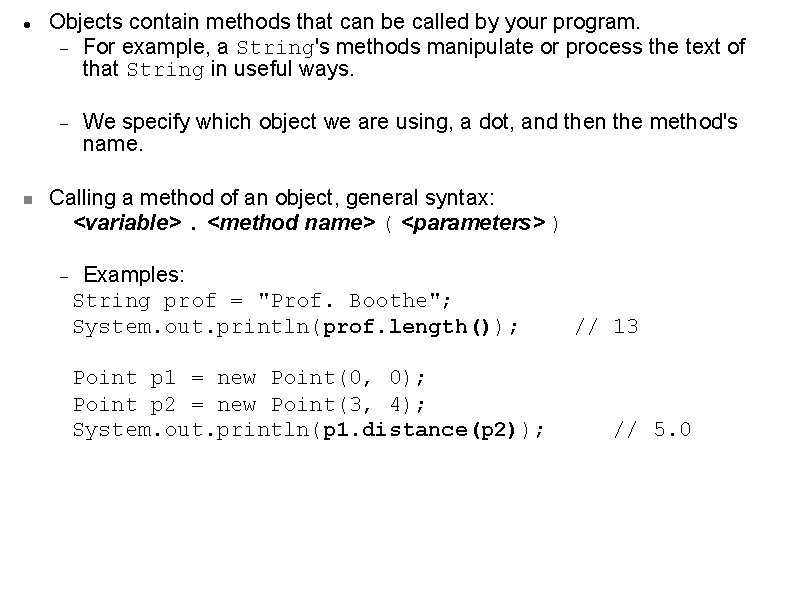
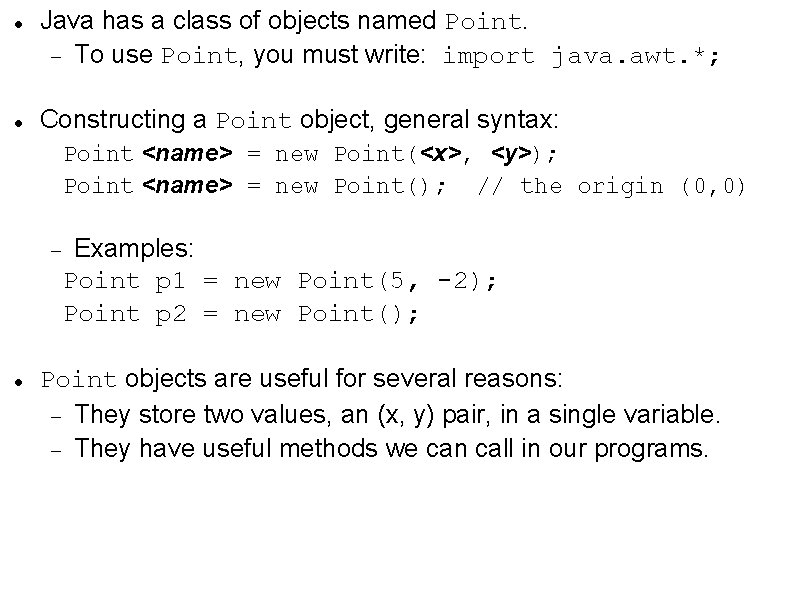
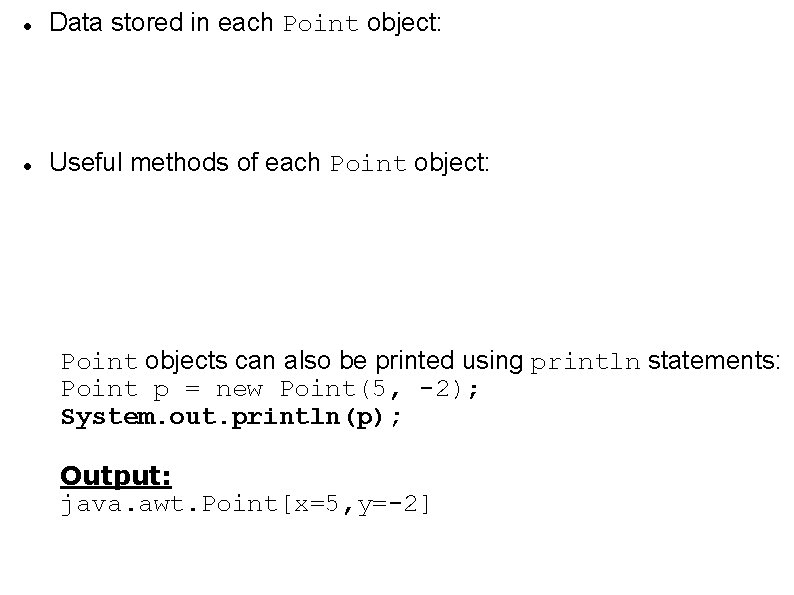
- Slides: 20
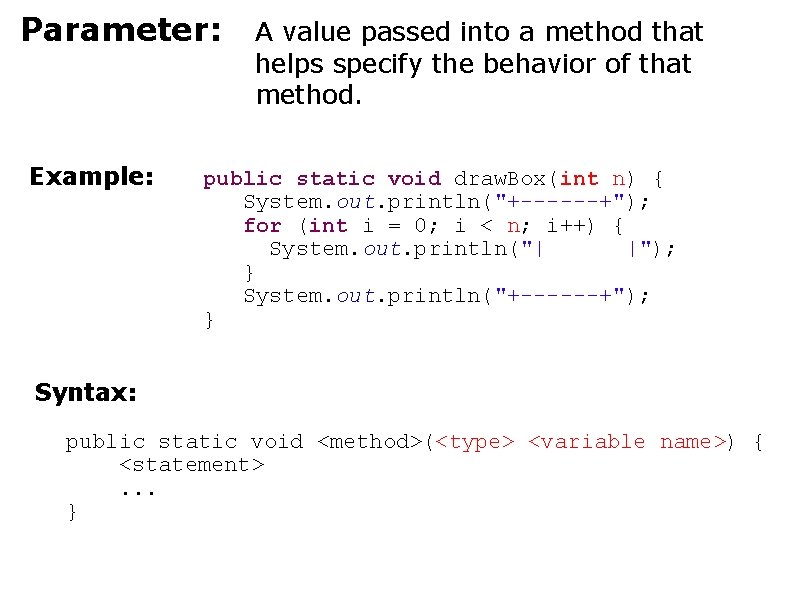
Parameter: Example: A value passed into a method that helps specify the behavior of that method. public static void draw. Box(int n) { System. out. println("+------+"); for (int i = 0; i < n; i++) { System. out. println("| |"); } System. out. println("+------+"); } Syntax: public static void <method>(<type> <variable name>) { <statement>. . . }
![public static void mainString args for int row 1 row 6 public static void main(String[] args) { for (int row = 1; row <= 6;](https://slidetodoc.com/presentation_image_h2/bce85a0ee3082f6869e52d5f68411dca/image-2.jpg)
public static void main(String[] args) { for (int row = 1; row <= 6; row++) { print. Stars(row); System. out. println(); } } Actual Parameter or Argument Formal // print num stars public static void print. Stars(int num) { for (int i = 1; i <= num; i++) { System. out. print("*"); } } Parameter
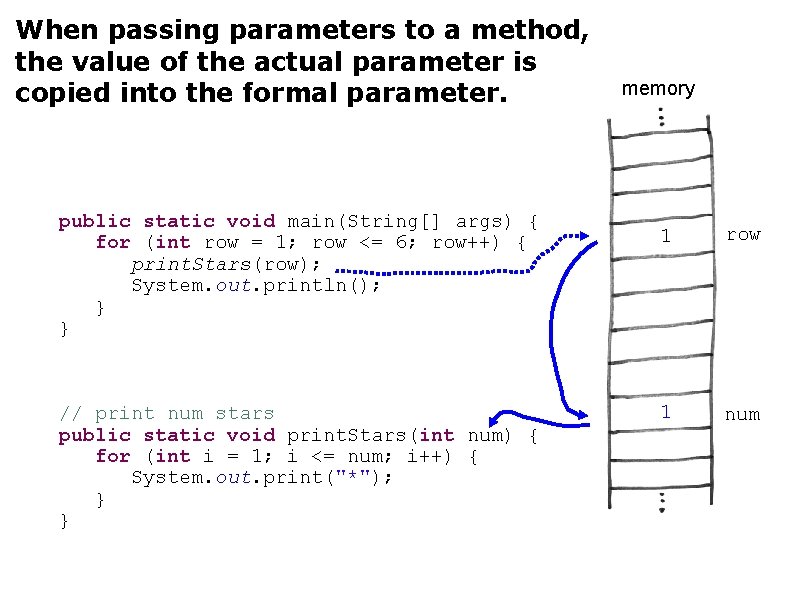
When passing parameters to a method, the value of the actual parameter is copied into the formal parameter. public static void main(String[] args) { for (int row = 1; row <= 6; row++) { print. Stars(row); System. out. println(); } } // print num stars public static void print. Stars(int num) { for (int i = 1; i <= num; i++) { System. out. print("*"); } } memory 1 row 1 num
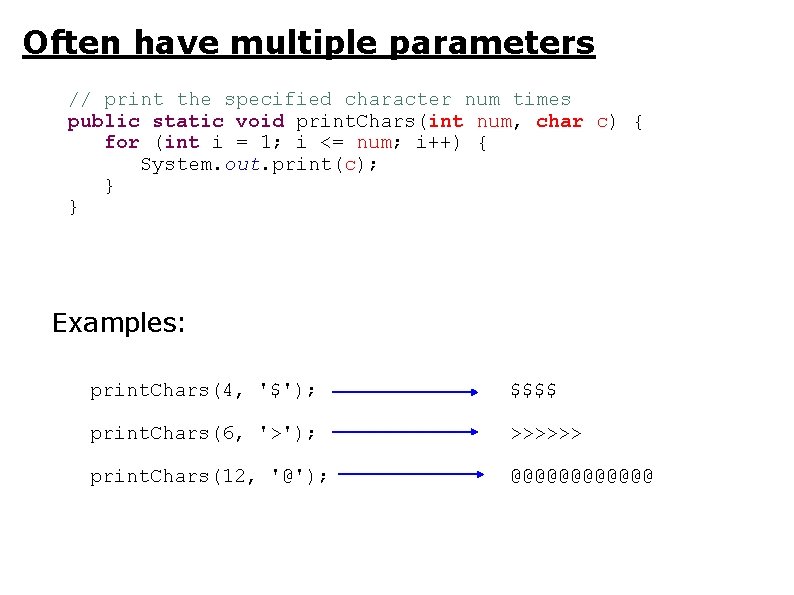
Often have multiple parameters // print the specified character num times public static void print. Chars(int num, char c) { for (int i = 1; i <= num; i++) { System. out. print(c); } } Examples: print. Chars(4, '$'); $$$$ print. Chars(6, '>'); >>>>>> print. Chars(12, '@'); @@@@@@
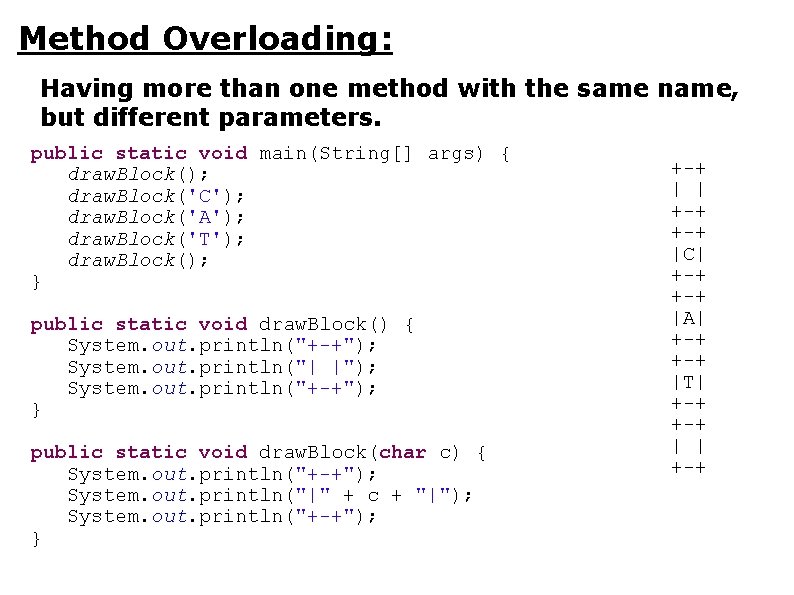
Method Overloading: Having more than one method with the same name, but different parameters. public static void main(String[] args) { draw. Block(); draw. Block('C'); draw. Block('A'); draw. Block('T'); draw. Block(); } public static void draw. Block() { System. out. println("+-+"); System. out. println("| |"); System. out. println("+-+"); } public static void draw. Block(char c) { System. out. println("+-+"); System. out. println("|" + c + "|"); System. out. println("+-+"); } +-+ | | +-+ |C| +-+ |A| +-+ |T| +-+ | | +-+
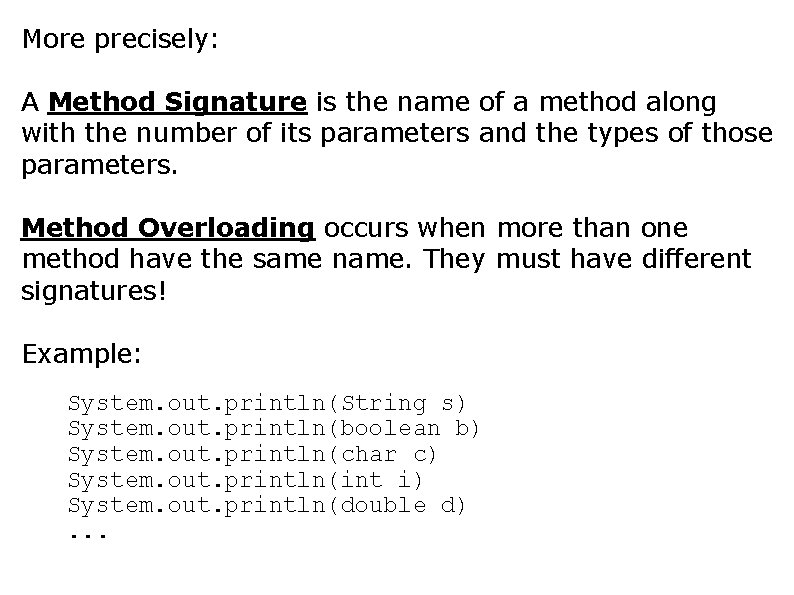
More precisely: A Method Signature is the name of a method along with the number of its parameters and the types of those parameters. Method Overloading occurs when more than one method have the same name. They must have different signatures! Example: System. out. println(String s) System. out. println(boolean b) System. out. println(char c) System. out. println(int i) System. out. println(double d). . .
![Methods can return a result to the caller Example public static void mainString args Methods can return a result to the caller. Example: public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/bce85a0ee3082f6869e52d5f68411dca/image-7.jpg)
Methods can return a result to the caller. Example: public static void main(String[] args) { double answer = average(95. 0, 80. 0); System. out. println(answer); } public static double average(double a, double b) { double avg = (a+b)/2. 0; return avg; } Output: 87. 5
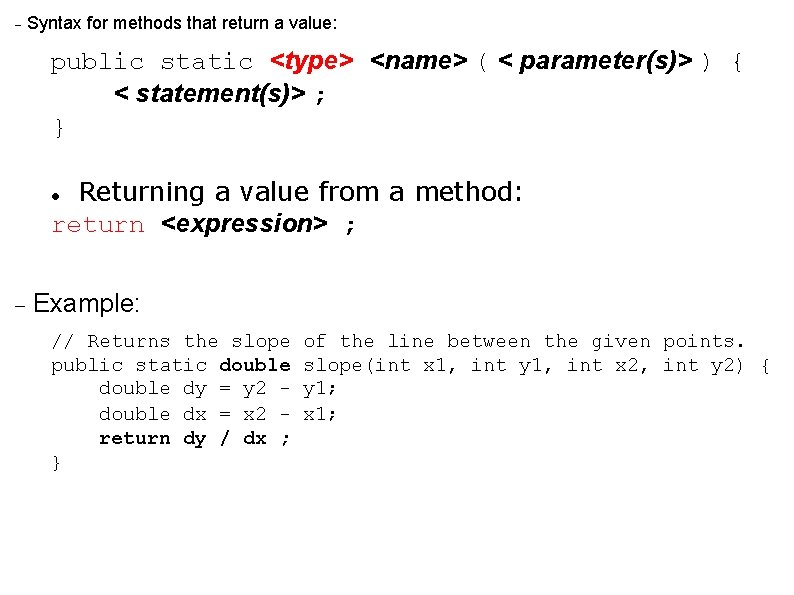
Syntax for methods that return a value: public static <type> <name> ( < parameter(s)> ) { < statement(s)> ; } Returning a value from a method: return <expression> ; Example: // Returns the slope public static double dy = y 2 double dx = x 2 return dy / dx ; } of the line between the given points. slope(int x 1, int y 1, int x 2, int y 2) { y 1; x 1;
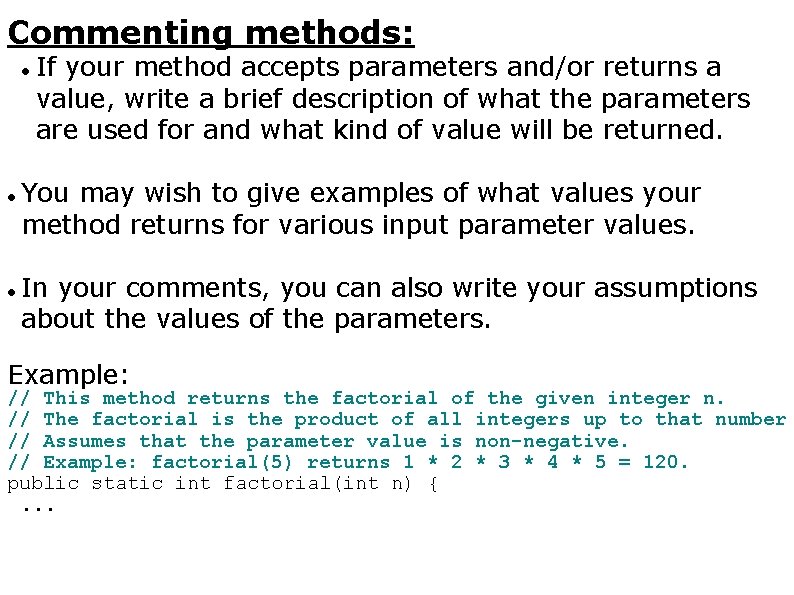
Commenting methods: If your method accepts parameters and/or returns a value, write a brief description of what the parameters are used for and what kind of value will be returned. You may wish to give examples of what values your method returns for various input parameter values. In your comments, you can also write your assumptions about the values of the parameters. Example: // This method returns the factorial of the given integer n. // The factorial is the product of all integers up to that number // Assumes that the parameter value is non-negative. // Example: factorial(5) returns 1 * 2 * 3 * 4 * 5 = 120. public static int factorial(int n) {. . .
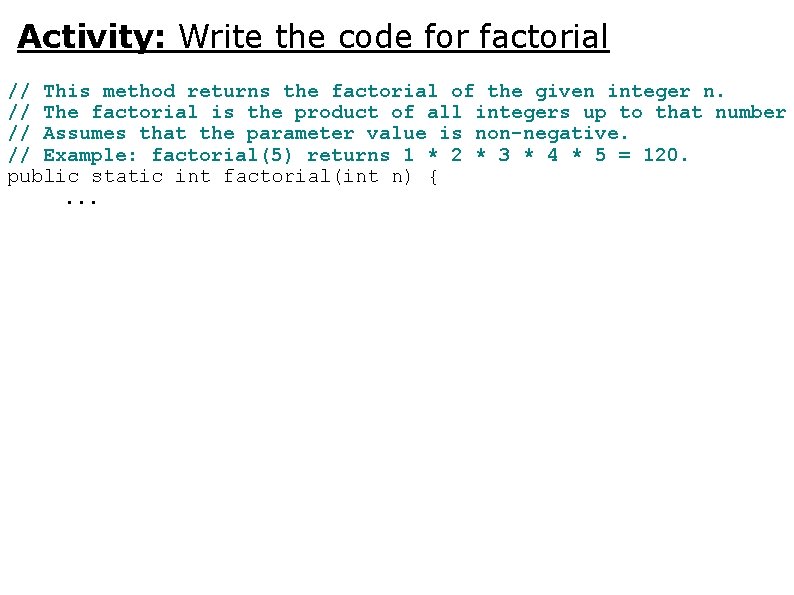
Activity: Write the code for factorial // This method returns the factorial of the given integer n. // The factorial is the product of all integers up to that number // Assumes that the parameter value is non-negative. // Example: factorial(5) returns 1 * 2 * 3 * 4 * 5 = 120. public static int factorial(int n) {. . .
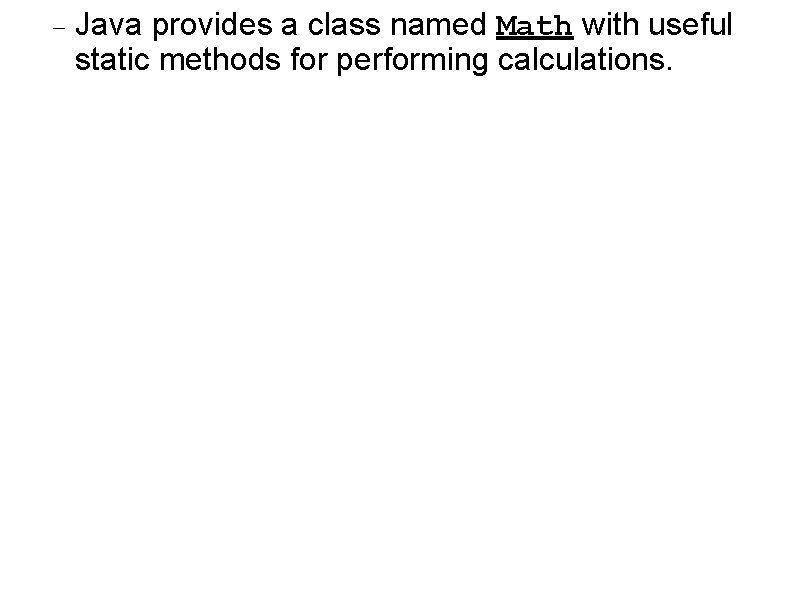
Java provides a class named Math with useful static methods for performing calculations.
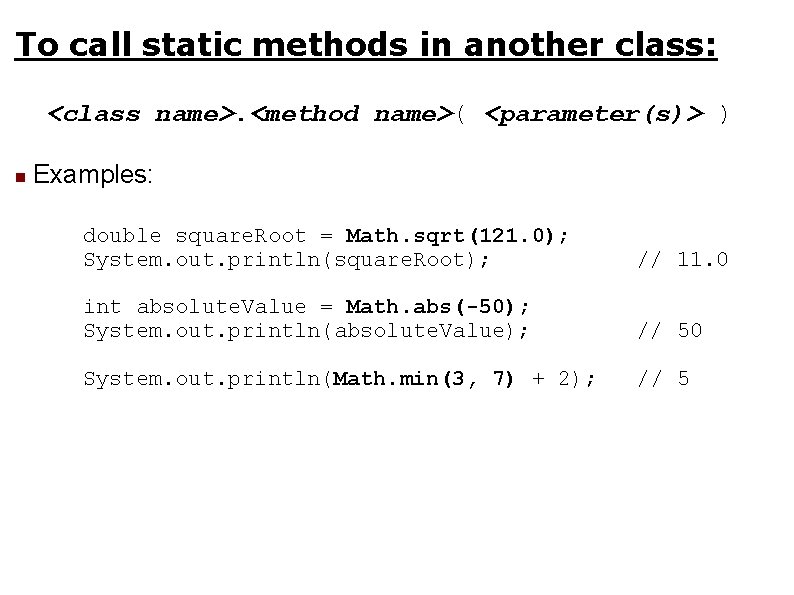
To call static methods in another class: <class name>. <method name>( <parameter(s)> ) Examples: double square. Root = Math. sqrt(121. 0); System. out. println(square. Root); // 11. 0 int absolute. Value = Math. abs(-50); System. out. println(absolute. Value); // 50 System. out. println(Math. min(3, 7) + 2); // 5
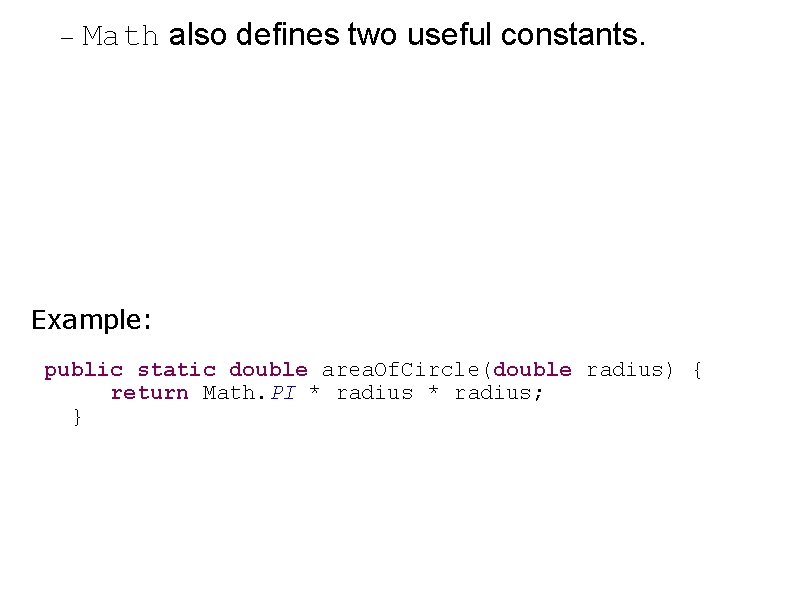
Math also defines two useful constants. Example: public static double area. Of. Circle(double radius) { return Math. PI * radius; }
![Example Printing an old fashion sine table public static void mainString args System Example: Printing an old fashion sine table. public static void main(String[] args) { System.](https://slidetodoc.com/presentation_image_h2/bce85a0ee3082f6869e52d5f68411dca/image-14.jpg)
Example: Printing an old fashion sine table. public static void main(String[] args) { System. out. println("angletsin"); for (double angle = 0. 0; angle <= 90. 0; angle++) { // convert angle to radians double radians = angle/90. 0*(Math. PI/2. 0); double sin = Math. sin(radians); // round to 4 digits - Math. round() returns a long sin = Math. round(sin*10000. 0)/10000. 0; System. out. println(angle+"t"+sin); } } angle 0. 0 1. 0 2. 0 3. 0 4. 0 5. 0 6. 0 7. 0 8. 0. . . sin 0. 0175 0. 0349 0. 0523 0. 0698 0. 0872 0. 1045 0. 1219 0. 1392 . . . 82. 0 83. 0 84. 0 85. 0 86. 0 87. 0 88. 0 89. 0 90. 0 0. 9903 0. 9925 0. 9945 0. 9962 0. 9976 0. 9986 0. 9994 0. 9998 1. 0
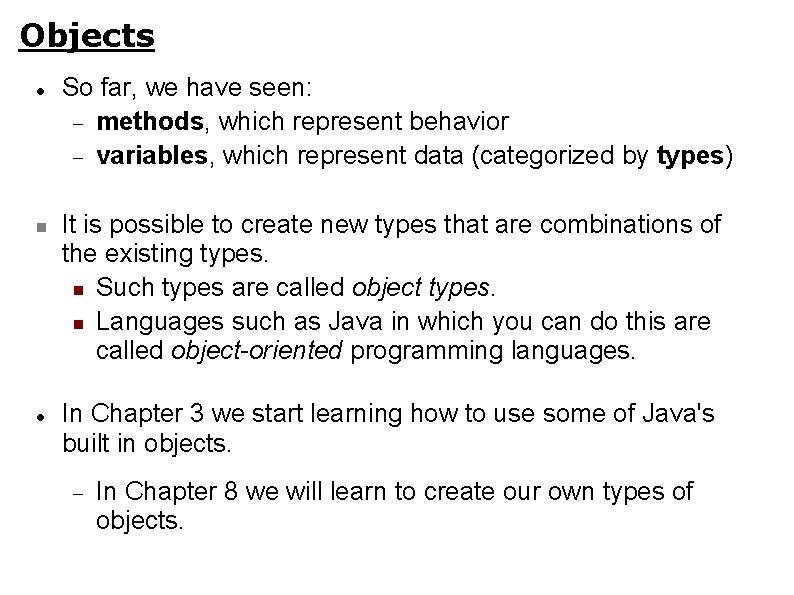
Objects So far, we have seen: methods, which represent behavior variables, which represent data (categorized by types) It is possible to create new types that are combinations of the existing types. Such types are called object types. Languages such as Java in which you can do this are called object-oriented programming languages. In Chapter 3 we start learning how to use some of Java's built in objects. In Chapter 8 we will learn to create our own types of objects.
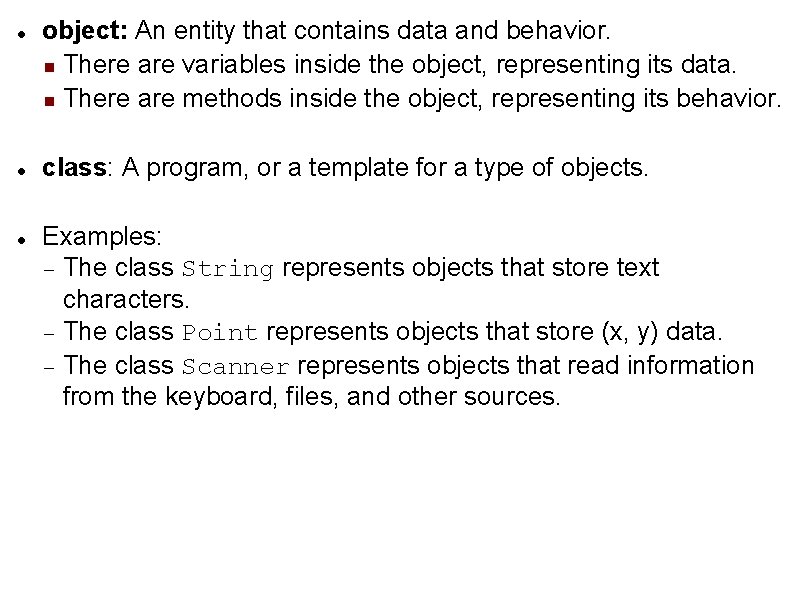
object: An entity that contains data and behavior. There are variables inside the object, representing its data. There are methods inside the object, representing its behavior. class: A program, or a template for a type of objects. Examples: The class String represents objects that store text characters. The class Point represents objects that store (x, y) data. The class Scanner represents objects that read information from the keyboard, files, and other sources.
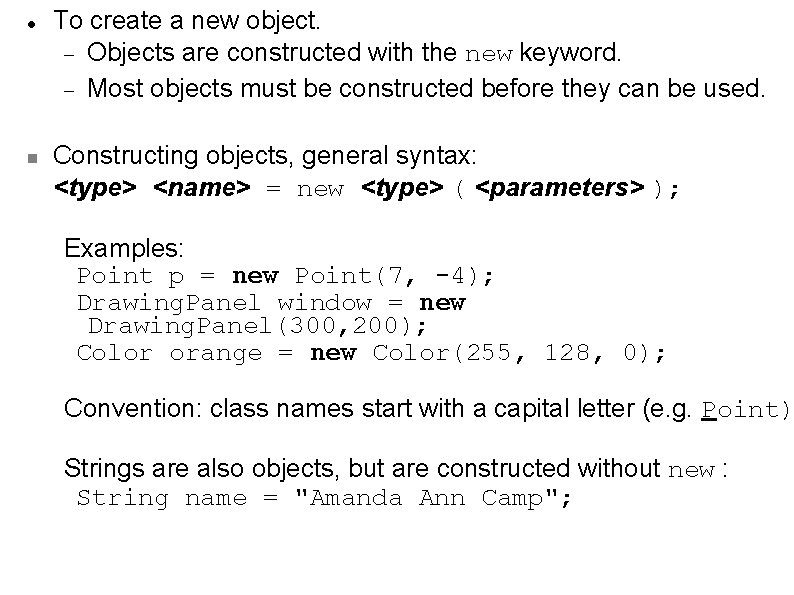
To create a new object. Objects are constructed with the new keyword. Most objects must be constructed before they can be used. Constructing objects, general syntax: <type> <name> = new <type> ( <parameters> ); Examples: Point p = new Point(7, -4); Drawing. Panel window = new Drawing. Panel(300, 200); Color orange = new Color(255, 128, 0); Convention: class names start with a capital letter (e. g. Point) Strings are also objects, but are constructed without new : String name = "Amanda Ann Camp";
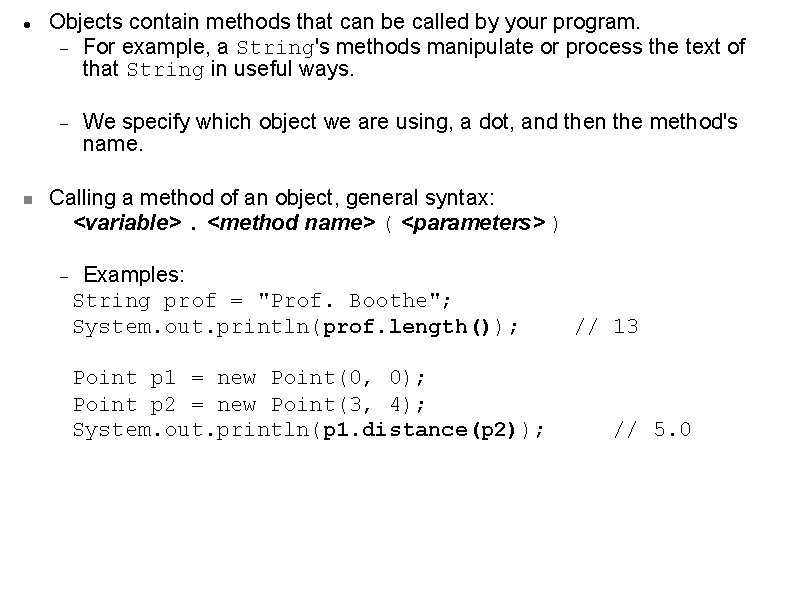
Objects contain methods that can be called by your program. For example, a String's methods manipulate or process the text of that String in useful ways. We specify which object we are using, a dot, and then the method's name. Calling a method of an object, general syntax: <variable>. <method name> ( <parameters> ) Examples: String prof = "Prof. Boothe"; System. out. println(prof. length()); Point p 1 = new Point(0, 0); Point p 2 = new Point(3, 4); System. out. println(p 1. distance(p 2)); // 13 // 5. 0
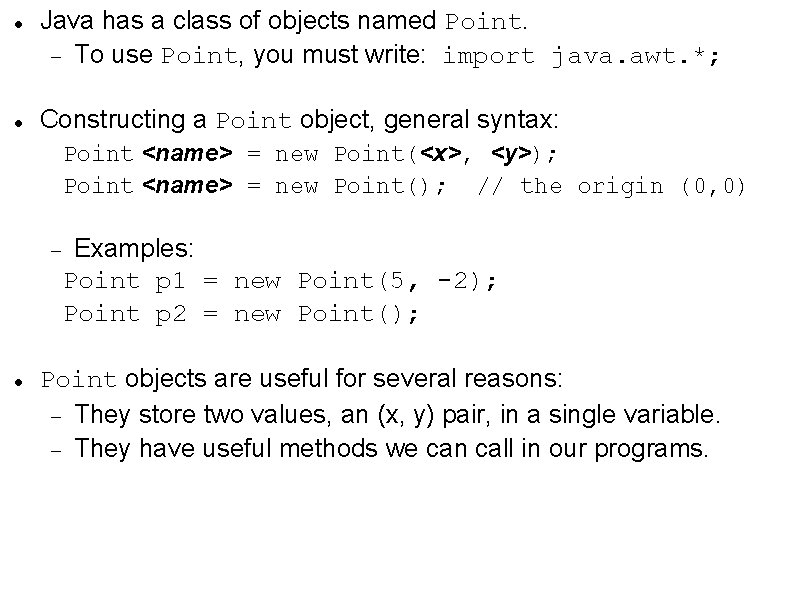
Java has a class of objects named Point. To use Point, you must write: import java. awt. *; Constructing a Point object, general syntax: Point <name> = new Point(<x>, <y>); Point <name> = new Point(); // the origin (0, 0) Examples: Point p 1 = new Point(5, -2); Point p 2 = new Point(); Point objects are useful for several reasons: They store two values, an (x, y) pair, in a single variable. They have useful methods we can call in our programs.
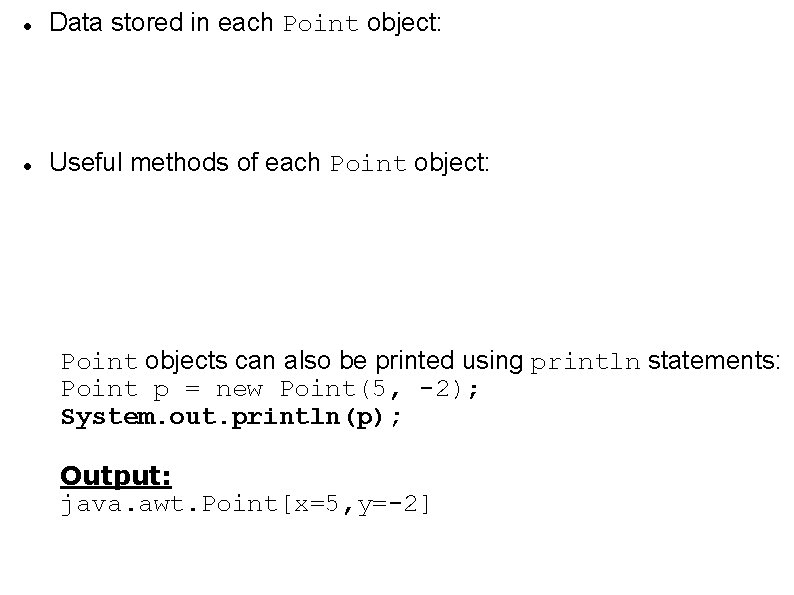
Data stored in each Point object: Useful methods of each Point object: Point objects can also be printed using println statements: Point p = new Point(5, -2); System. out. println(p); Output: java. awt. Point[x=5, y=-2]