Parallel execution Programming Language Design and Implementation 4
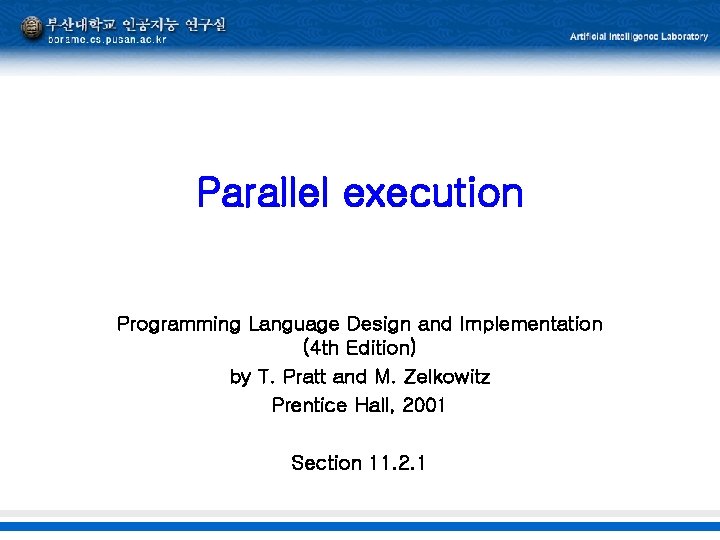
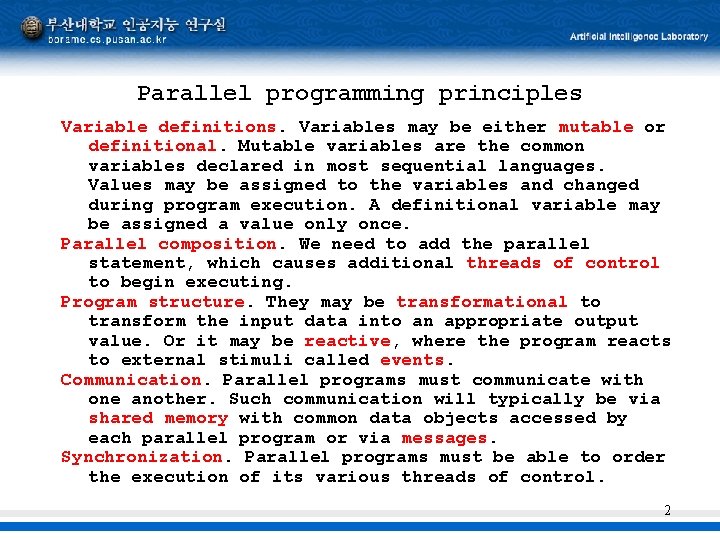
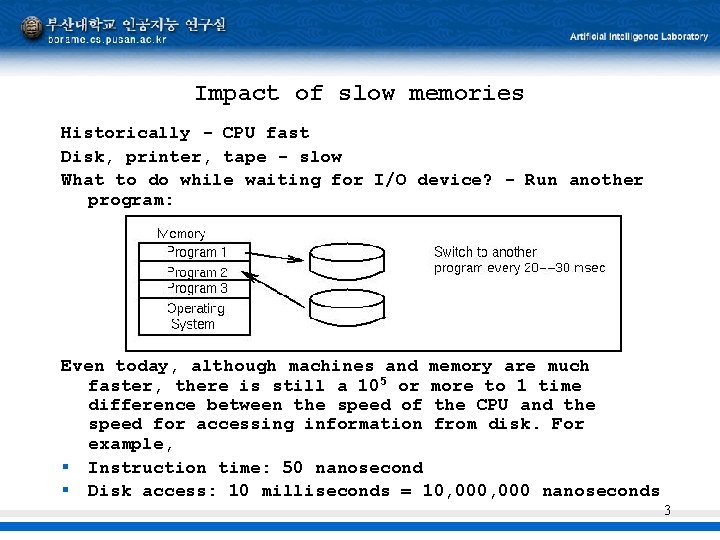
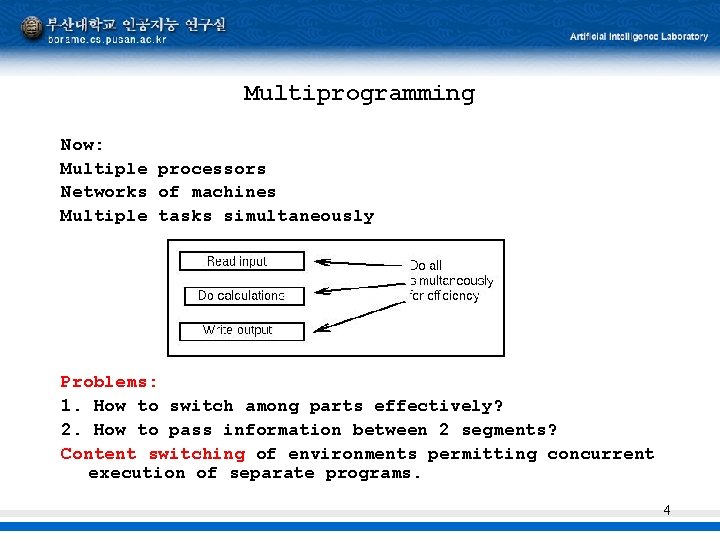
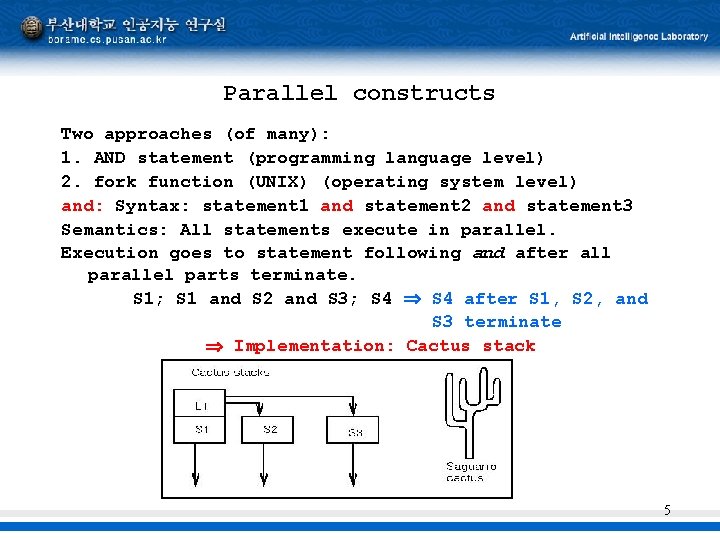
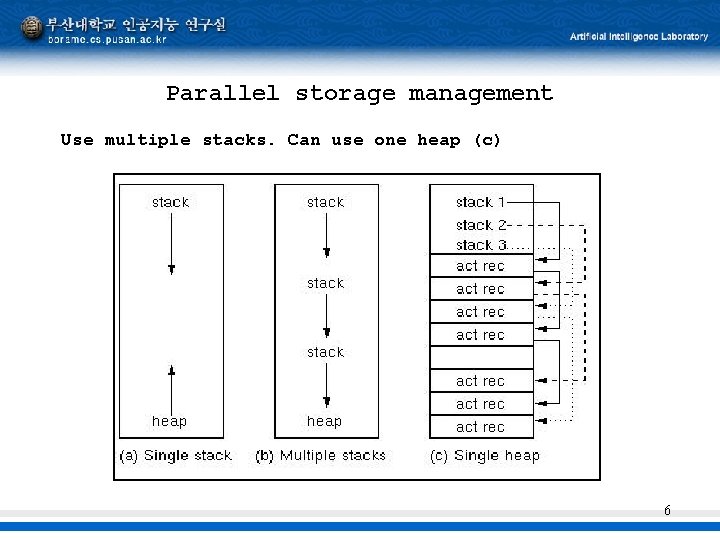
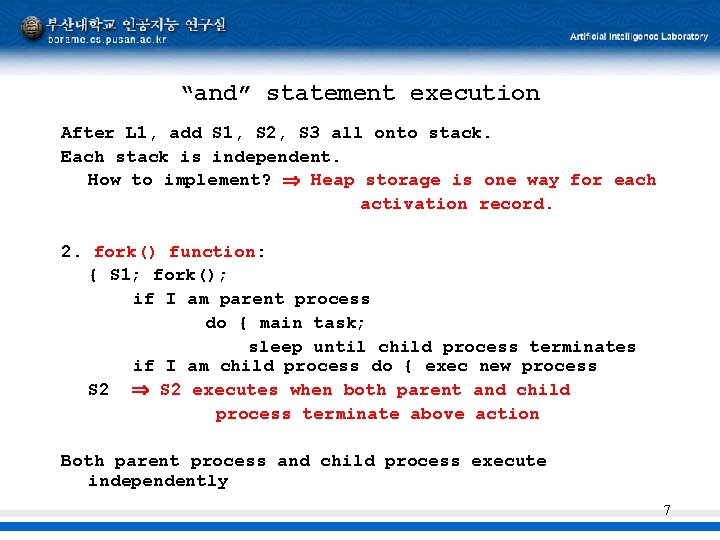
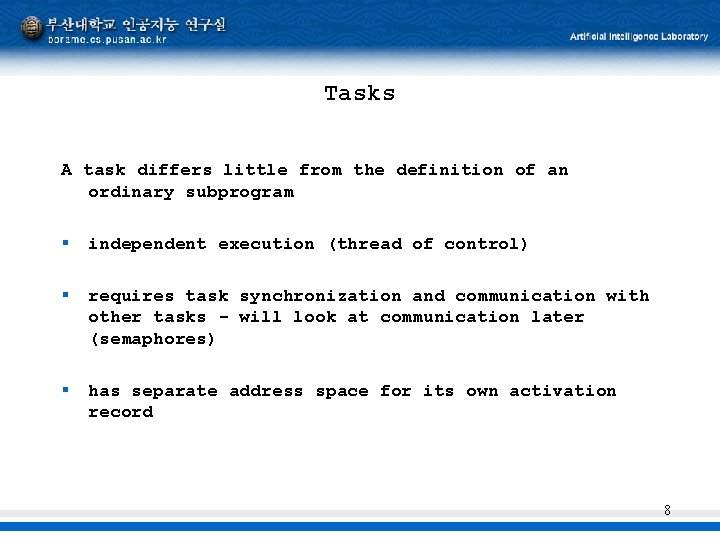
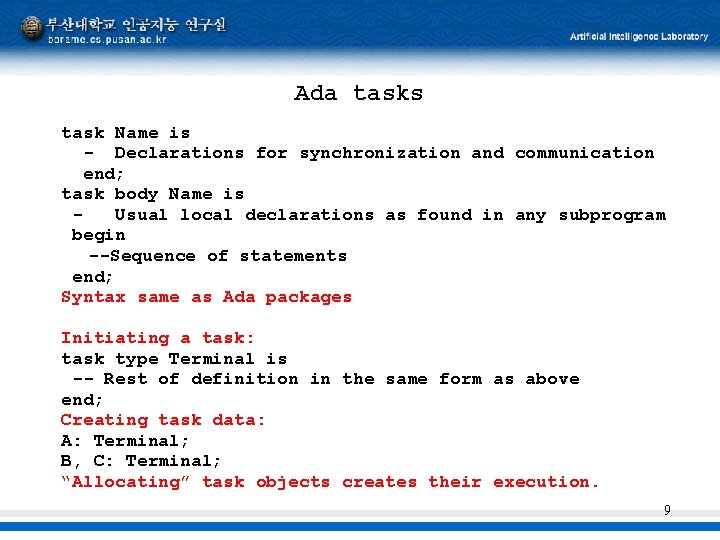
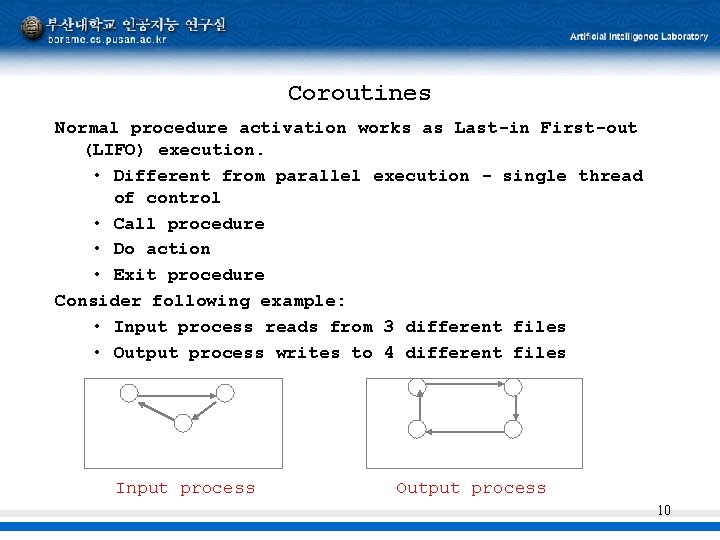
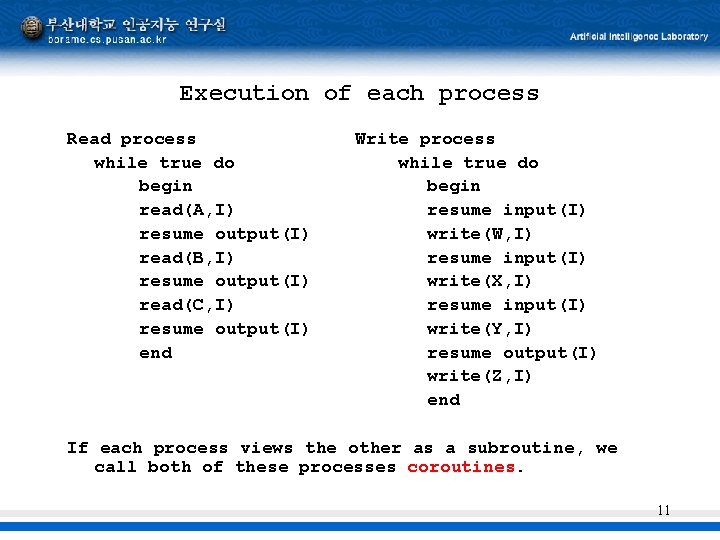
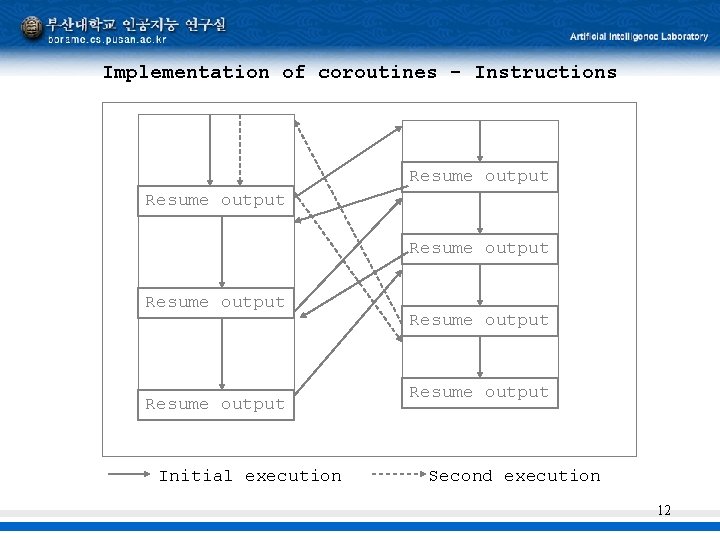
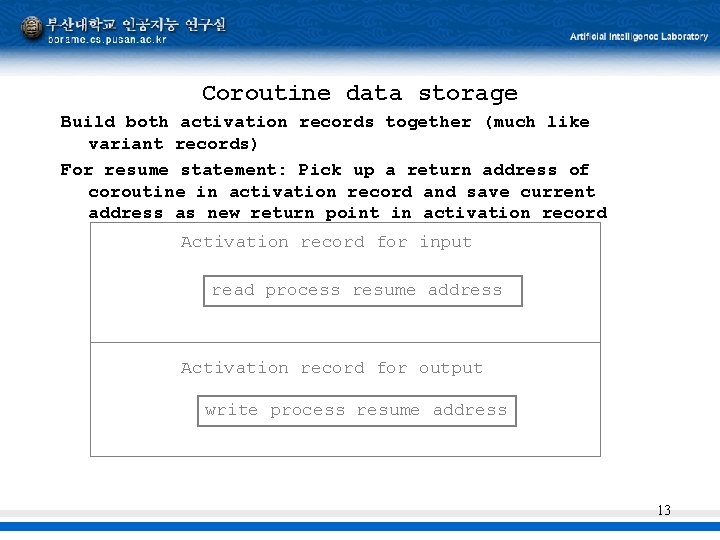
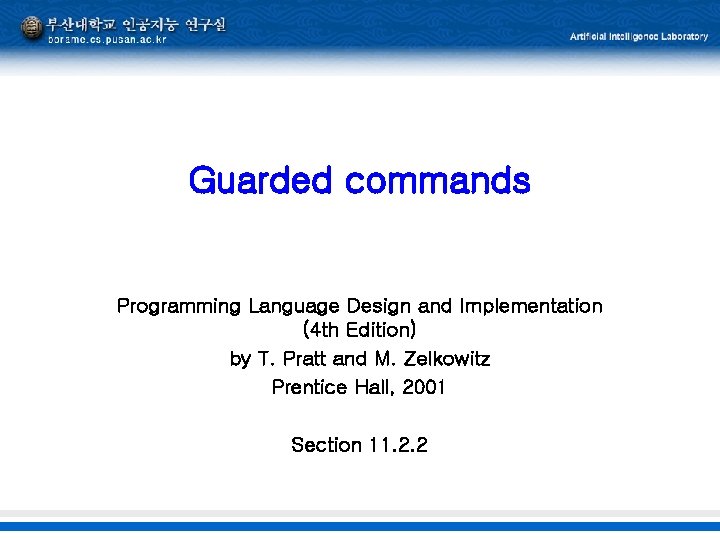
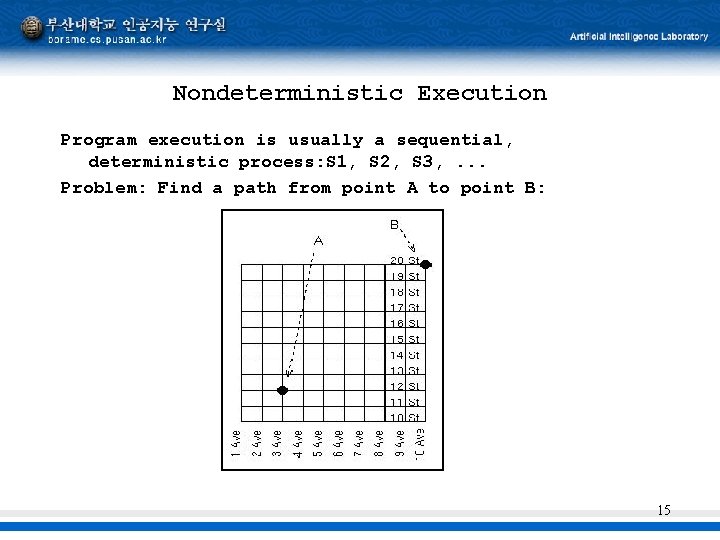
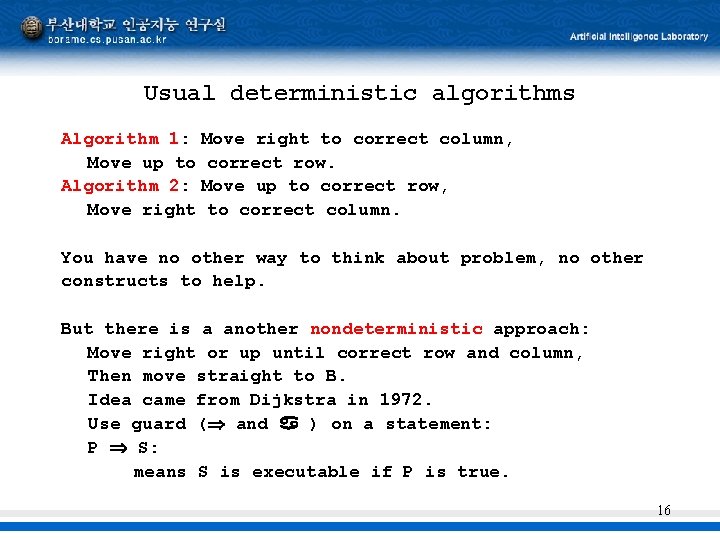
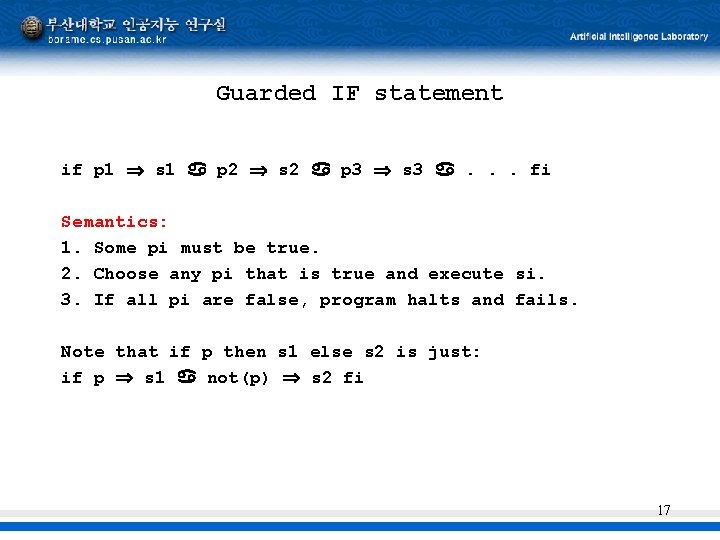
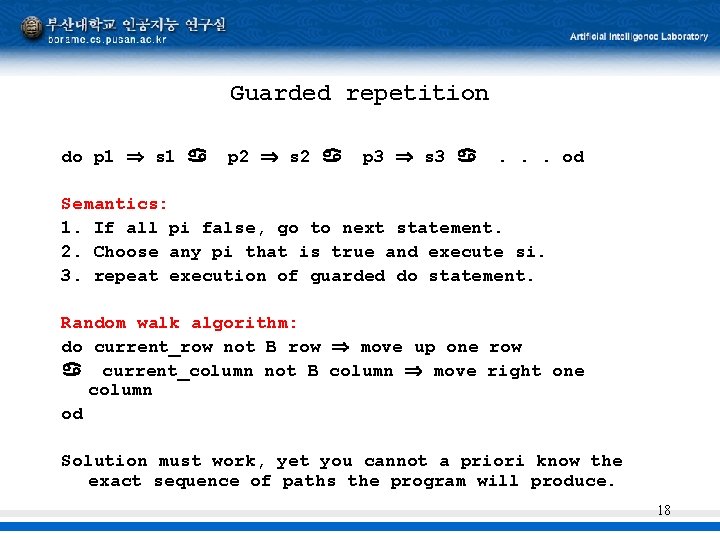
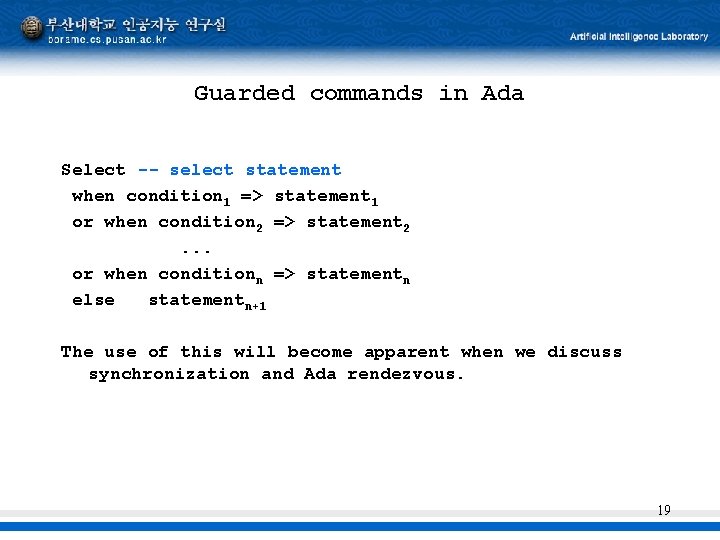
- Slides: 19
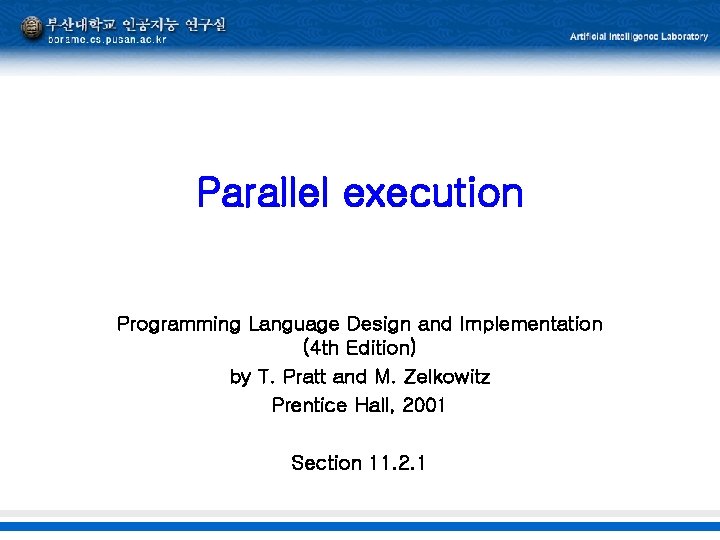
Parallel execution Programming Language Design and Implementation (4 th Edition) by T. Pratt and M. Zelkowitz Prentice Hall, 2001 Section 11. 2. 1
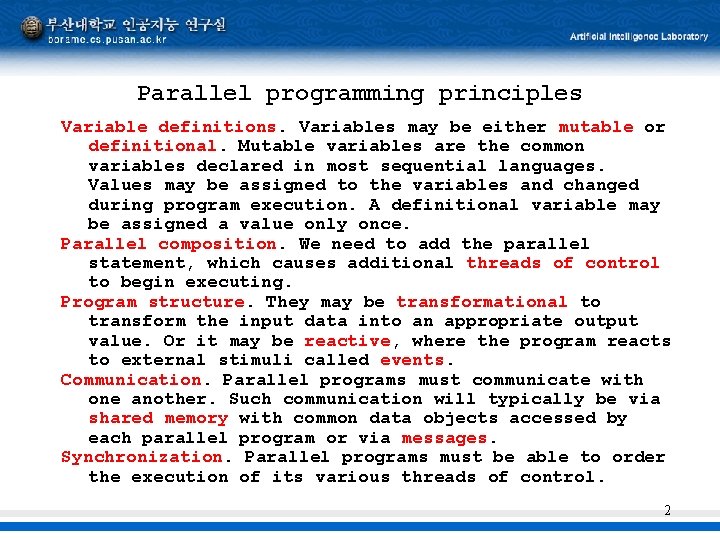
Parallel programming principles Variable definitions. Variables may be either mutable or definitional. Mutable variables are the common variables declared in most sequential languages. Values may be assigned to the variables and changed during program execution. A definitional variable may be assigned a value only once. Parallel composition. We need to add the parallel statement, which causes additional threads of control to begin executing. Program structure. They may be transformational to transform the input data into an appropriate output value. Or it may be reactive, where the program reacts to external stimuli called events. Communication. Parallel programs must communicate with one another. Such communication will typically be via shared memory with common data objects accessed by each parallel program or via messages. Synchronization. Parallel programs must be able to order the execution of its various threads of control. 2
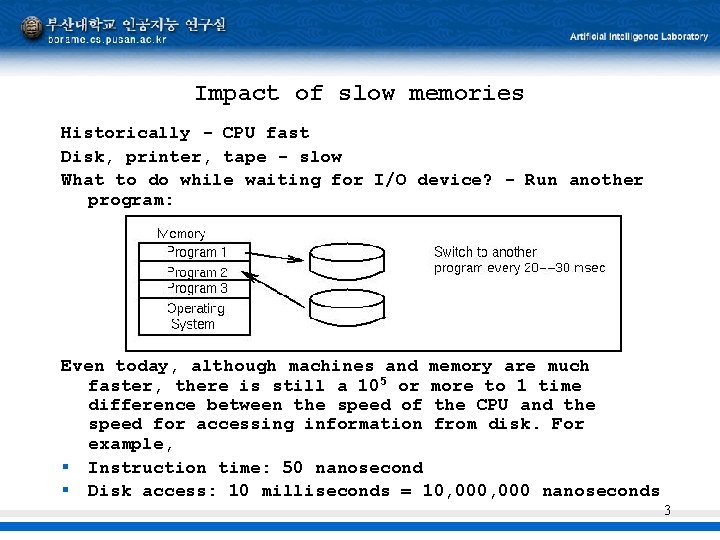
Impact of slow memories Historically - CPU fast Disk, printer, tape - slow What to do while waiting for I/O device? - Run another program: Even today, although machines and memory are much faster, there is still a 105 or more to 1 time difference between the speed of the CPU and the speed for accessing information from disk. For example, § Instruction time: 50 nanosecond § Disk access: 10 milliseconds = 10, 000 nanoseconds 3
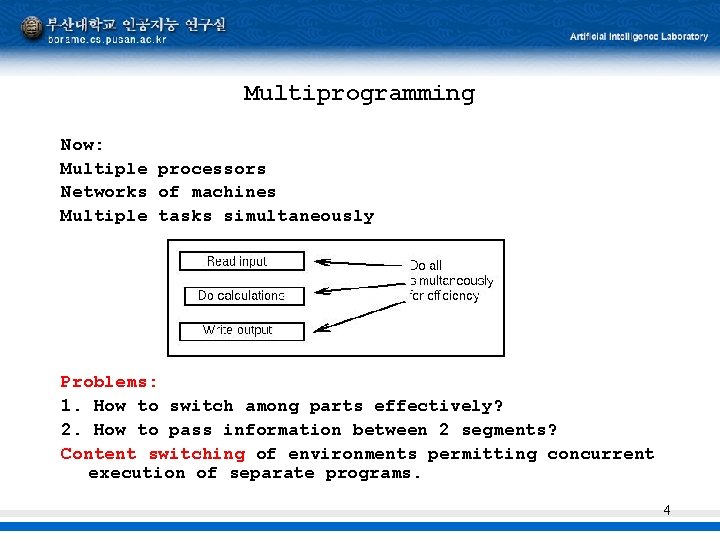
Multiprogramming Now: Multiple processors Networks of machines Multiple tasks simultaneously Problems: 1. How to switch among parts effectively? 2. How to pass information between 2 segments? Content switching of environments permitting concurrent execution of separate programs. 4
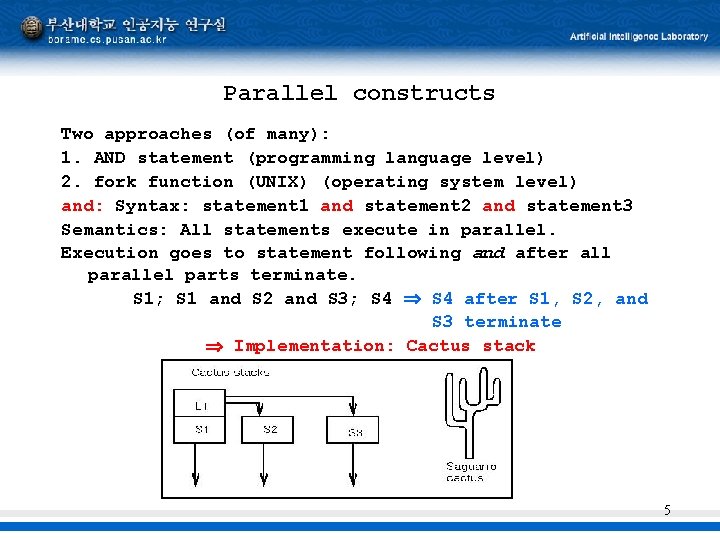
Parallel constructs Two approaches (of many): 1. AND statement (programming language level) 2. fork function (UNIX) (operating system level) and: Syntax: statement 1 and statement 2 and statement 3 Semantics: All statements execute in parallel. Execution goes to statement following and after all parallel parts terminate. S 1; S 1 and S 2 and S 3; S 4 after S 1, S 2, and S 3 terminate Implementation: Cactus stack 5
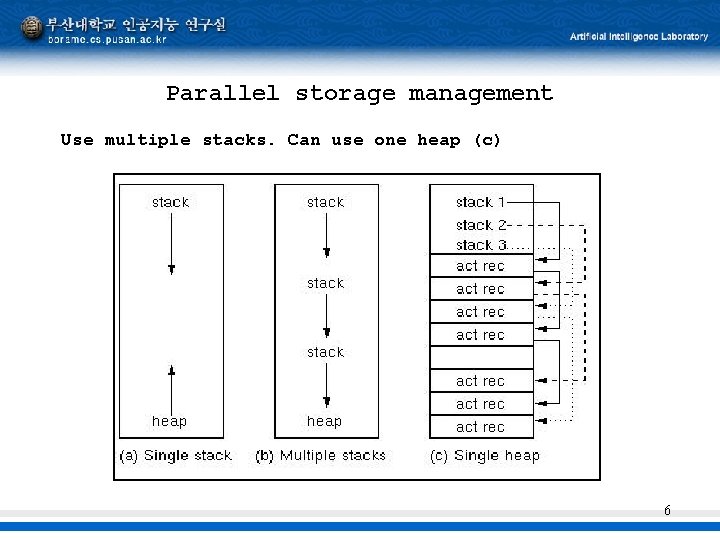
Parallel storage management Use multiple stacks. Can use one heap (c) 6
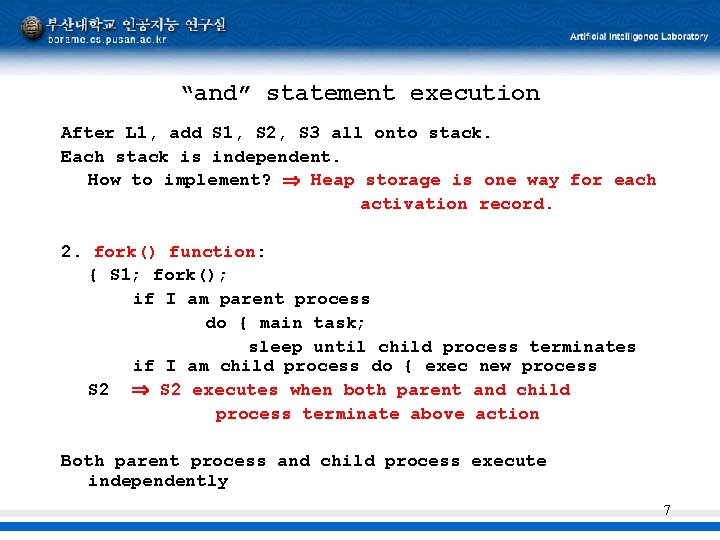
“and” statement execution After L 1, add S 1, S 2, S 3 all onto stack. Each stack is independent. How to implement? Heap storage is one way for each activation record. 2. fork() function: { S 1; fork(); if I am parent process do { main task; sleep until child process terminates if I am child process do { exec new process S 2 executes when both parent and child process terminate above action Both parent process and child process execute independently 7
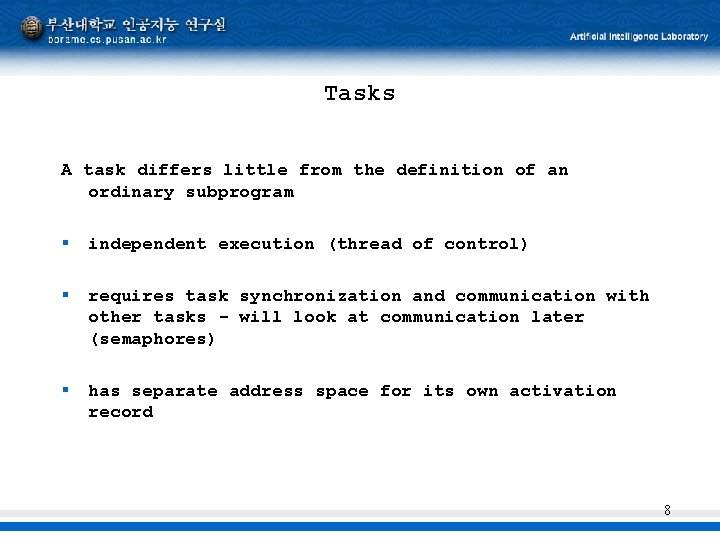
Tasks A task differs little from the definition of an ordinary subprogram § independent execution (thread of control) § requires task synchronization and communication with other tasks - will look at communication later (semaphores) § has separate address space for its own activation record 8
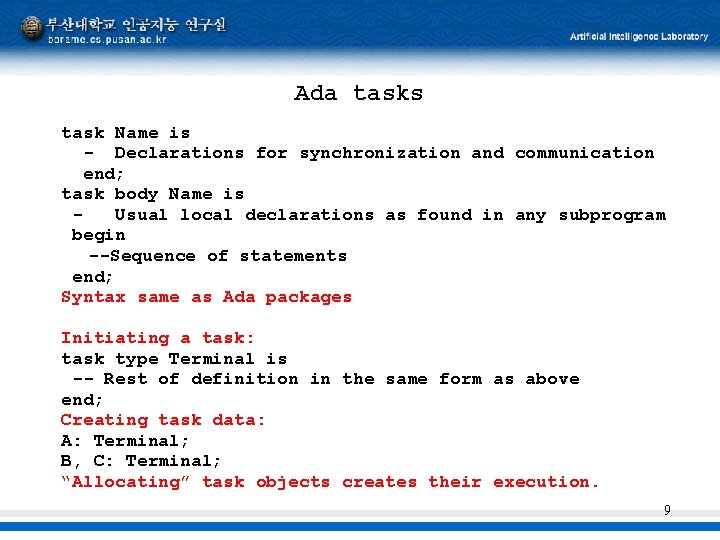
Ada tasks task Name is - Declarations for synchronization and communication end; task body Name is Usual local declarations as found in any subprogram begin --Sequence of statements end; Syntax same as Ada packages Initiating a task: task type Terminal is -- Rest of definition in the same form as above end; Creating task data: A: Terminal; B, C: Terminal; “Allocating” task objects creates their execution. 9
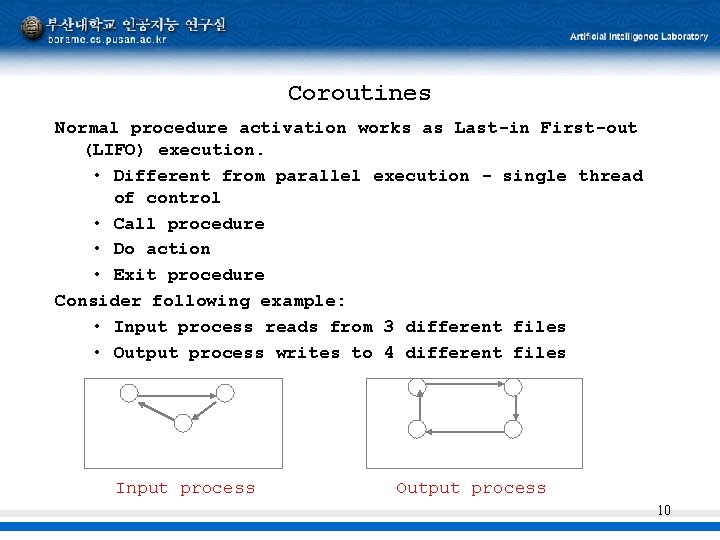
Coroutines Normal procedure activation works as Last-in First-out (LIFO) execution. • Different from parallel execution - single thread of control • Call procedure • Do action • Exit procedure Consider following example: • Input process reads from 3 different files • Output process writes to 4 different files Input process Output process 10
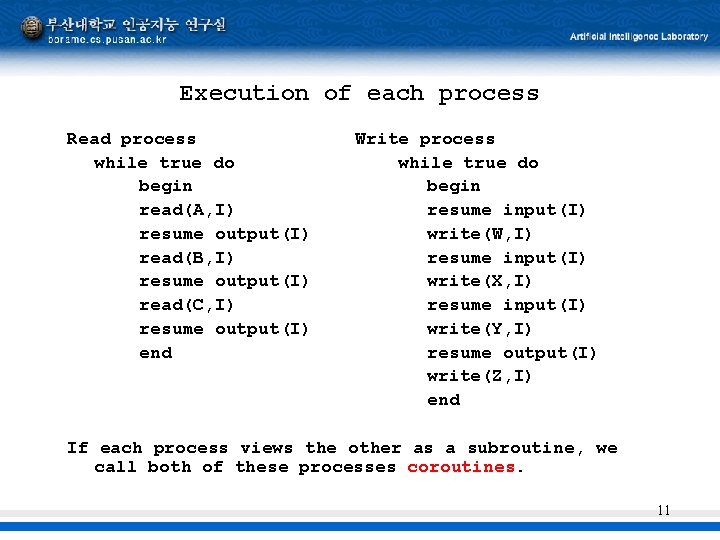
Execution of each process Read process while true do begin read(A, I) resume output(I) read(B, I) resume output(I) read(C, I) resume output(I) end Write process while true do begin resume input(I) write(W, I) resume input(I) write(X, I) resume input(I) write(Y, I) resume output(I) write(Z, I) end If each process views the other as a subroutine, we call both of these processes coroutines. 11
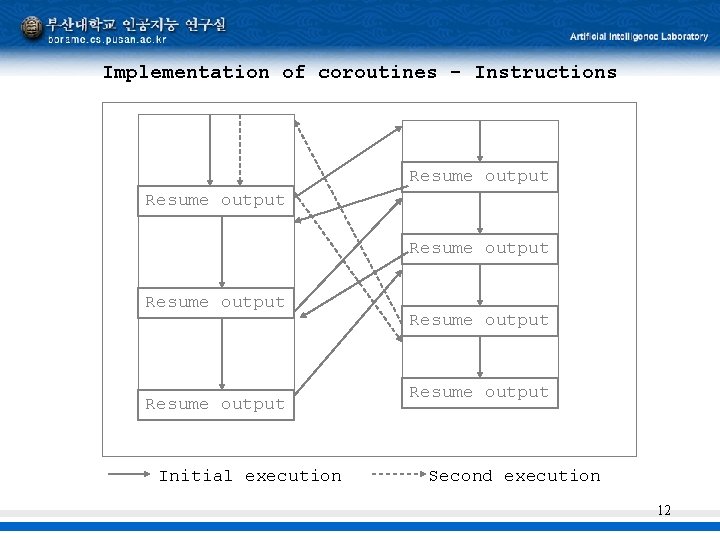
Implementation of coroutines - Instructions Resume output Resume output Initial execution Resume output Second execution 12
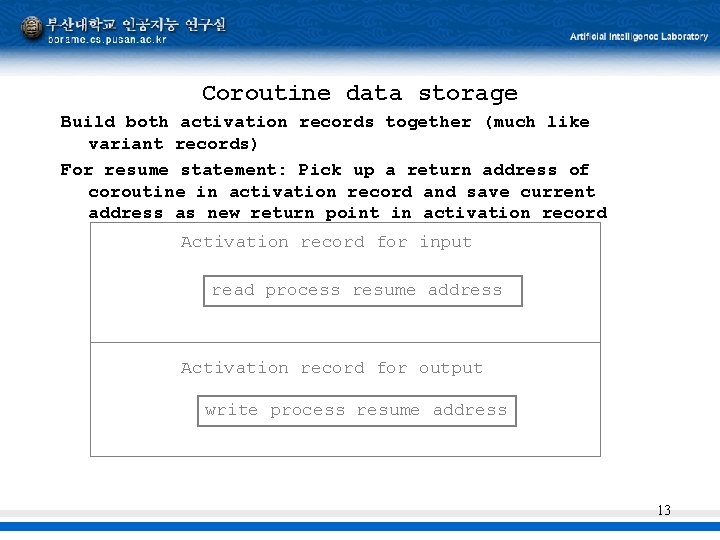
Coroutine data storage Build both activation records together (much like variant records) For resume statement: Pick up a return address of coroutine in activation record and save current address as new return point in activation record Activation record for input read process resume address Activation record for output write process resume address 13
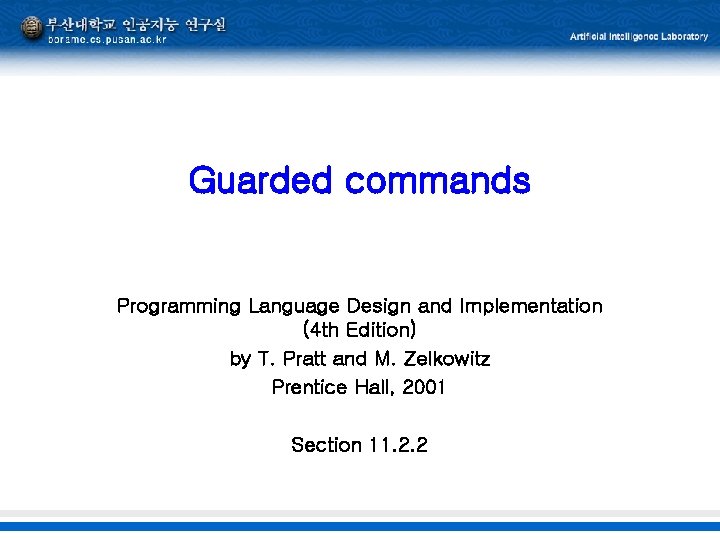
Guarded commands Programming Language Design and Implementation (4 th Edition) by T. Pratt and M. Zelkowitz Prentice Hall, 2001 Section 11. 2. 2
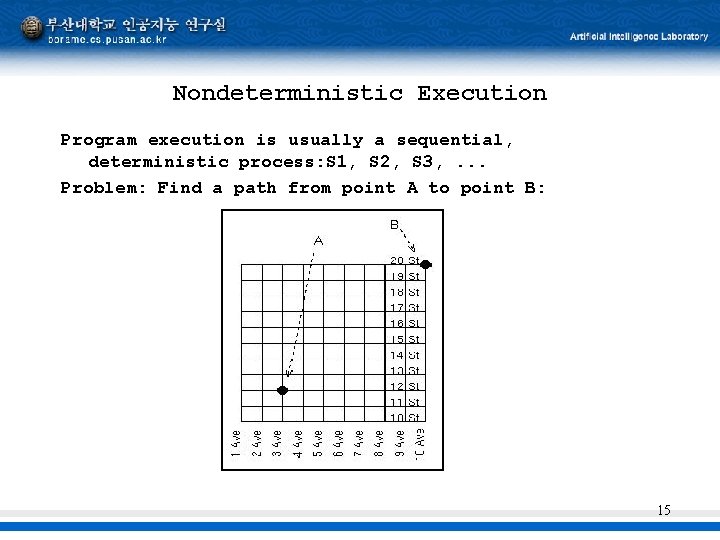
Nondeterministic Execution Program execution is usually a sequential, deterministic process: S 1, S 2, S 3, . . . Problem: Find a path from point A to point B: 15
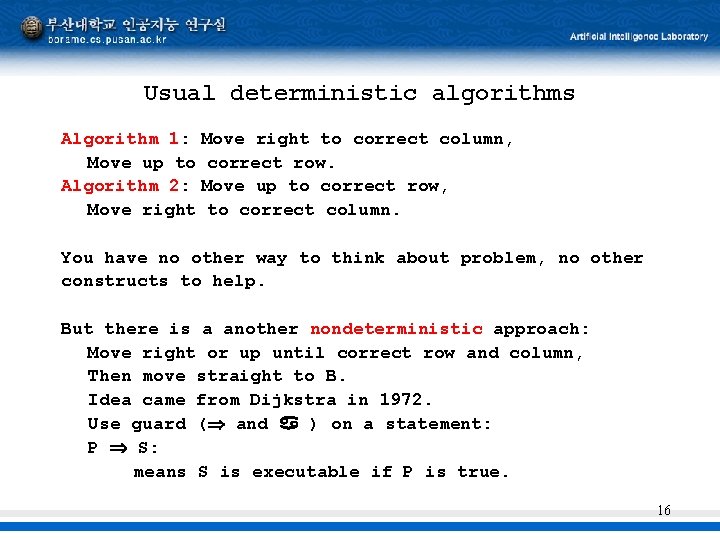
Usual deterministic algorithms Algorithm 1: Move up to Algorithm 2: Move right to correct column, correct row. Move up to correct row, to correct column. You have no other way to think about problem, no other constructs to help. But there is a another nondeterministic approach: Move right or up until correct row and column, Then move straight to B. Idea came from Dijkstra in 1972. Use guard ( and ) on a statement: P S: means S is executable if P is true. 16
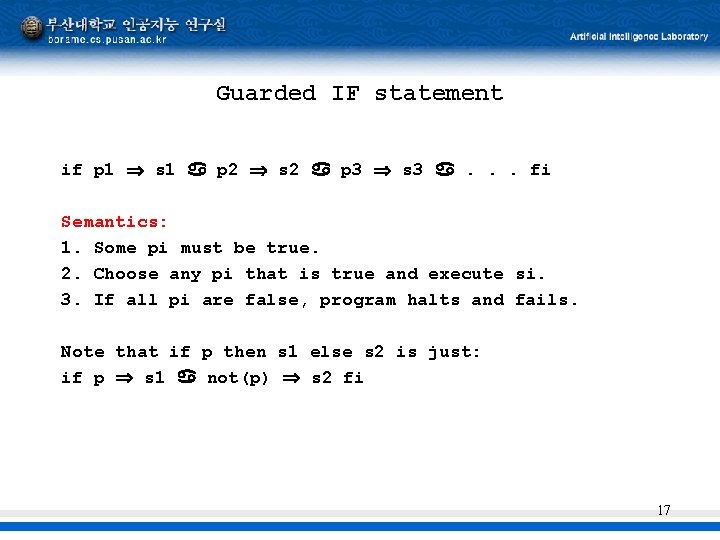
Guarded IF statement if p 1 s 1 p 2 s 2 p 3 s 3 . . . fi Semantics: 1. Some pi must be true. 2. Choose any pi that is true and execute si. 3. If all pi are false, program halts and fails. Note that if p then s 1 else s 2 is just: if p s 1 not(p) s 2 fi 17
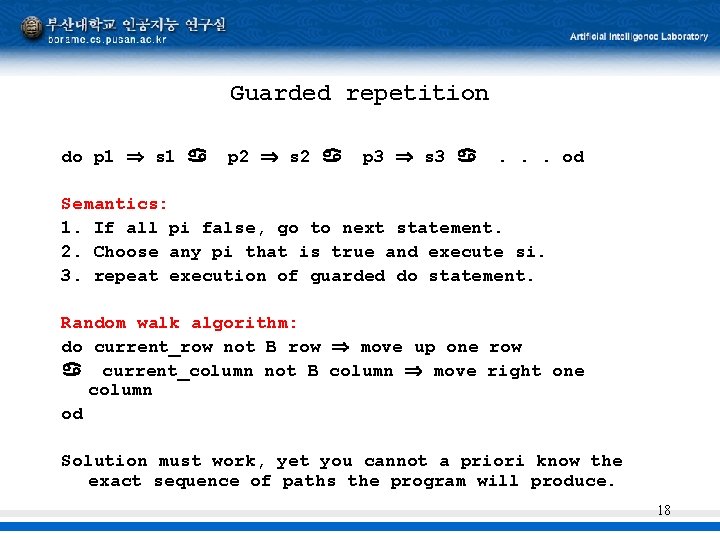
Guarded repetition do p 1 s 1 p 2 s 2 p 3 s 3 . . . od Semantics: 1. If all pi false, go to next statement. 2. Choose any pi that is true and execute si. 3. repeat execution of guarded do statement. Random walk algorithm: do current_row not B row move up one row current_column not B column move right one column od Solution must work, yet you cannot a priori know the exact sequence of paths the program will produce. 18
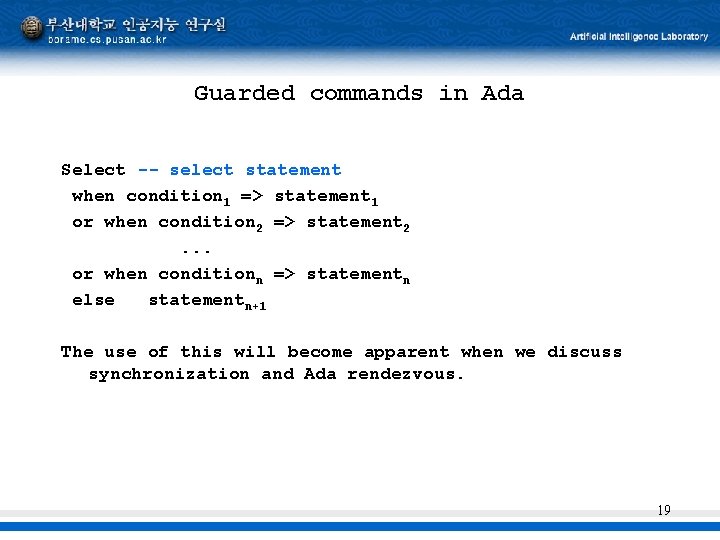
Guarded commands in Ada Select -- select statement when condition 1 => statement 1 or when condition 2 => statement 2. . . or when conditionn => statementn else statementn+1 The use of this will become apparent when we discuss synchronization and Ada rendezvous. 19