Operators Identifiers The Data Elements Arithmetic Operators exponentiation
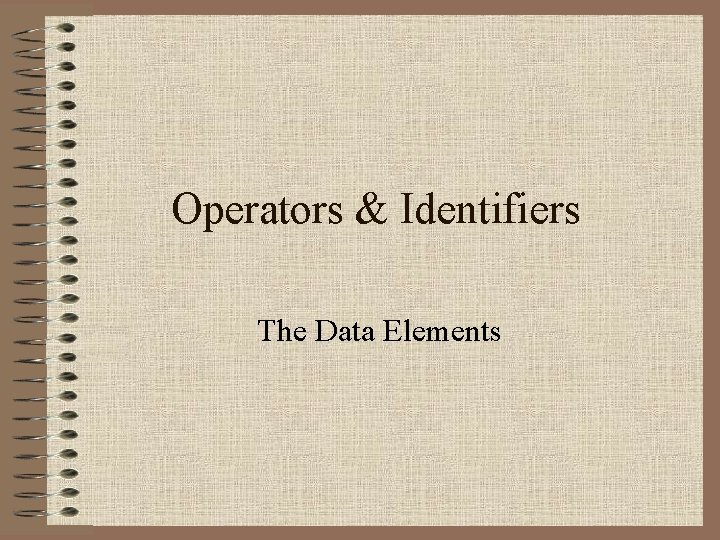
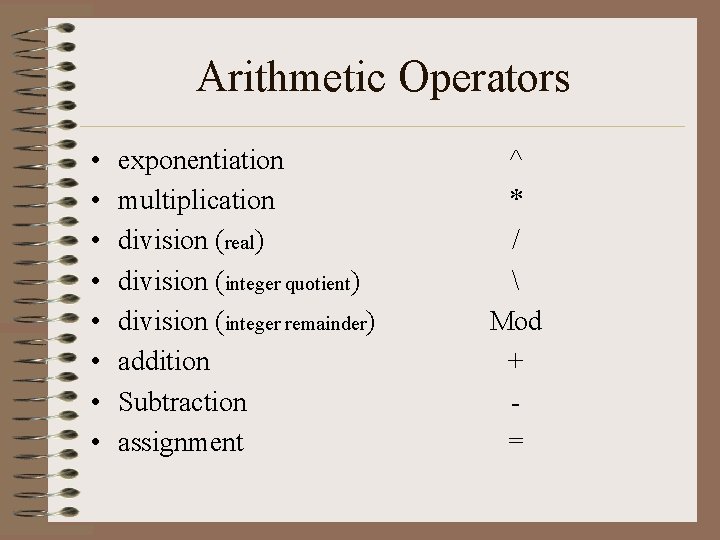
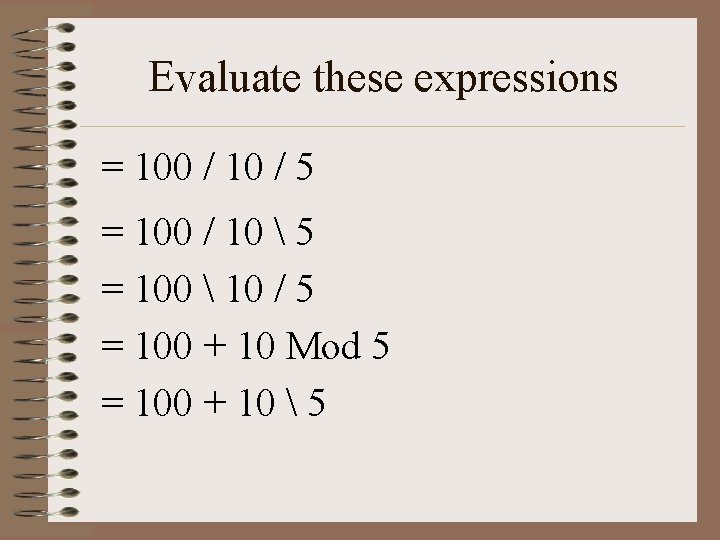
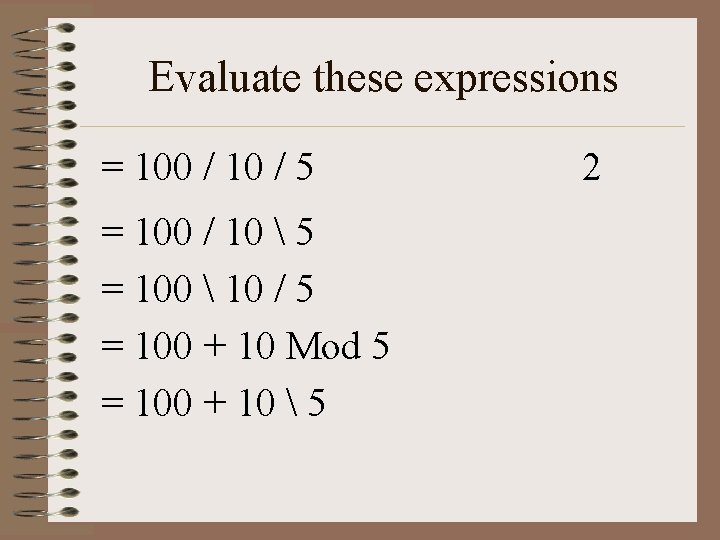
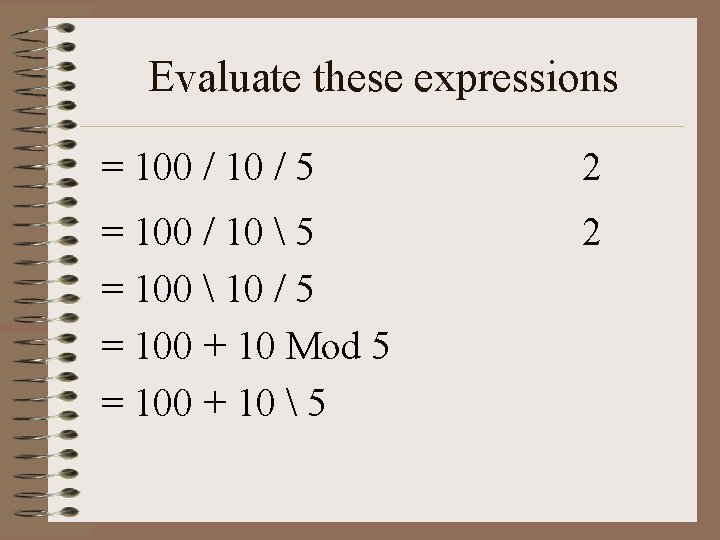
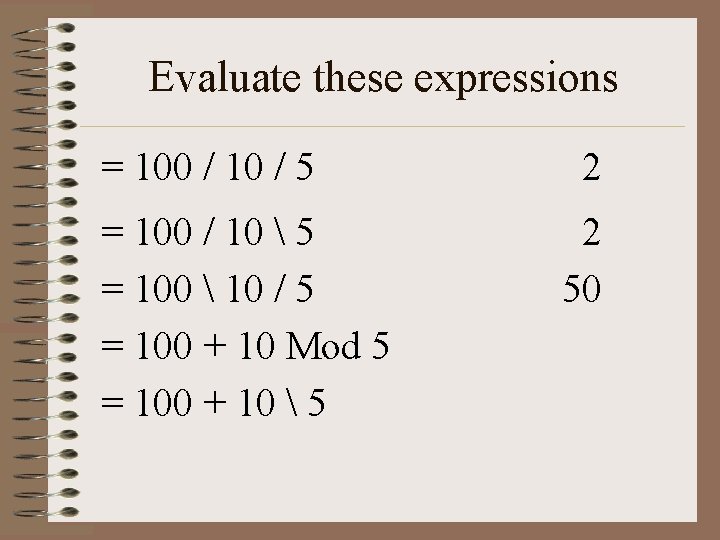
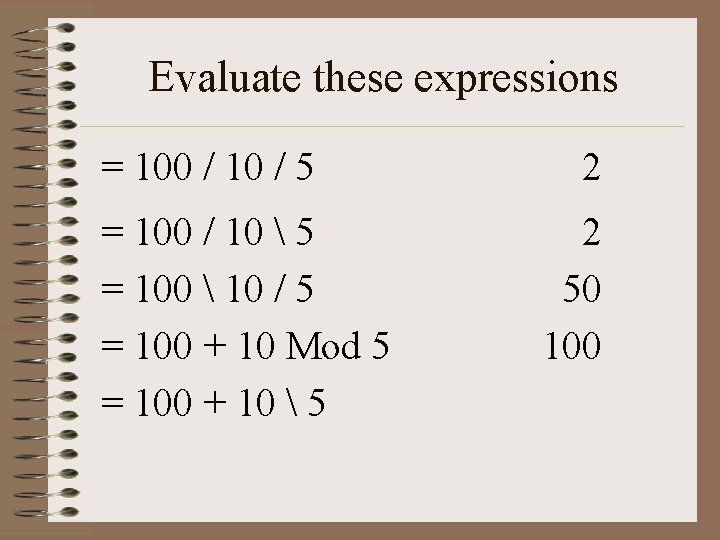
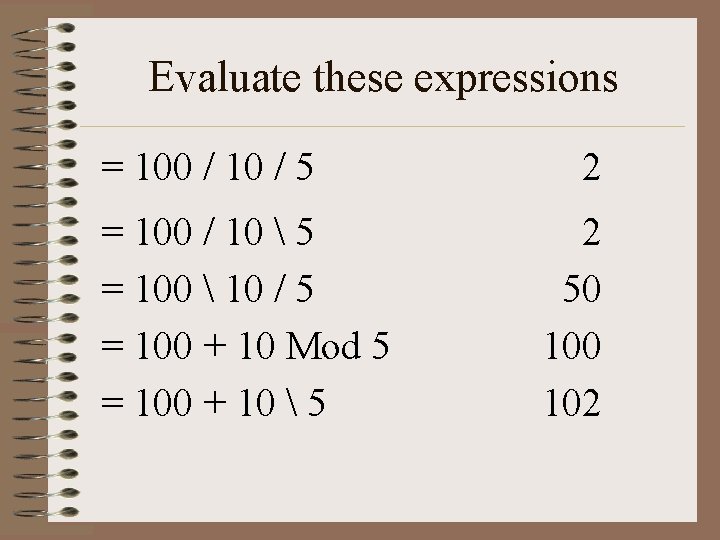
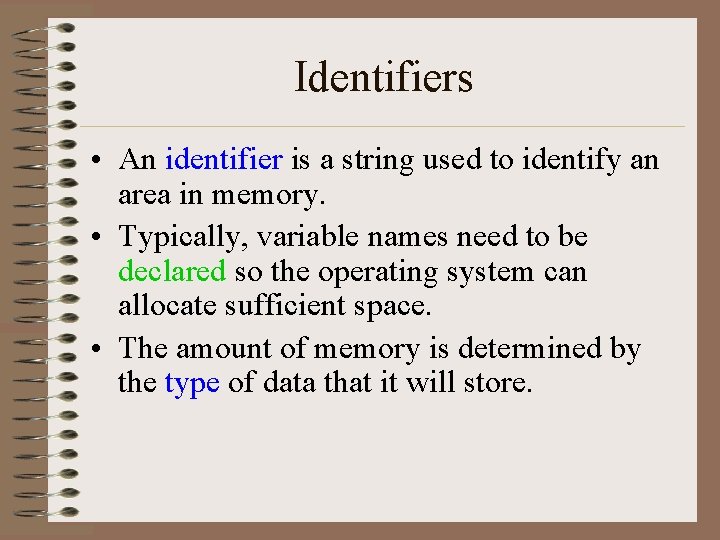
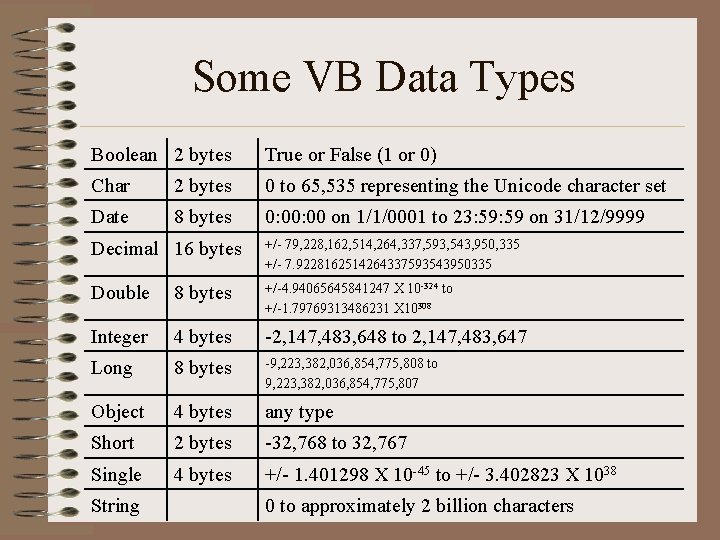
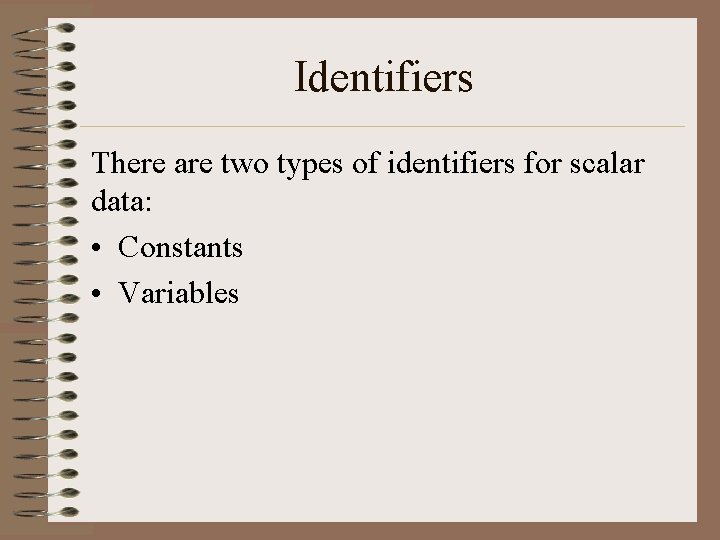
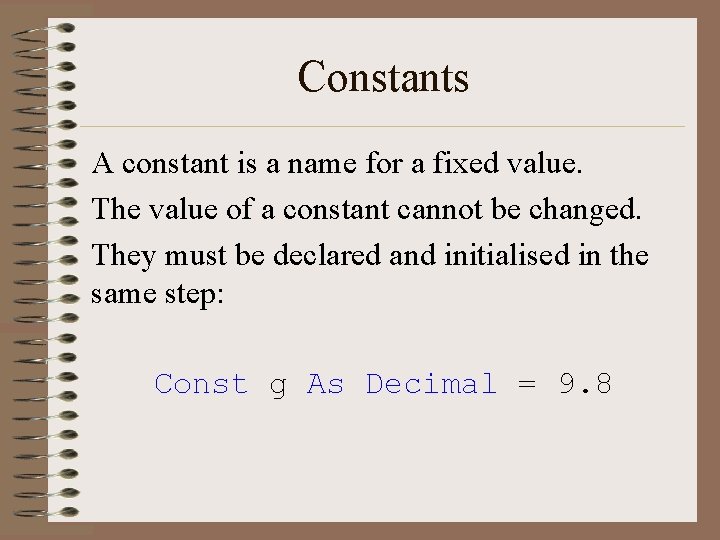
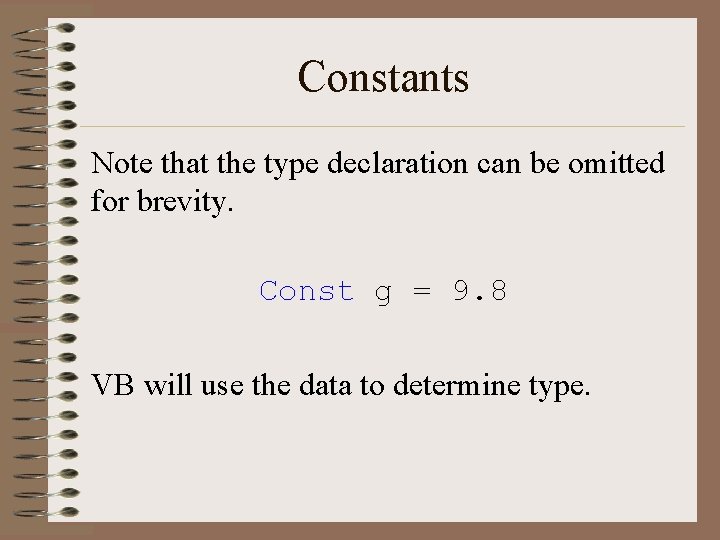
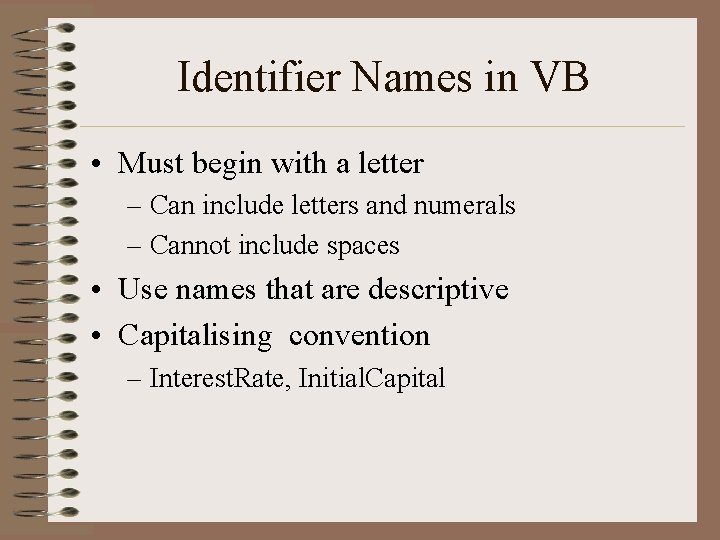
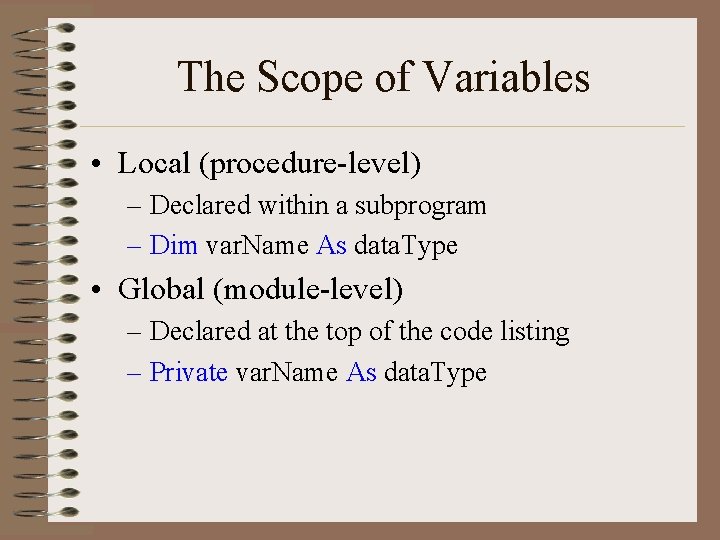
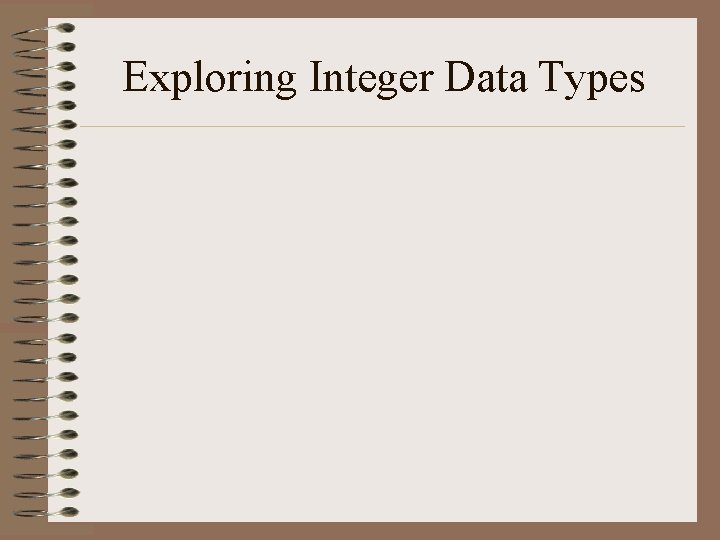
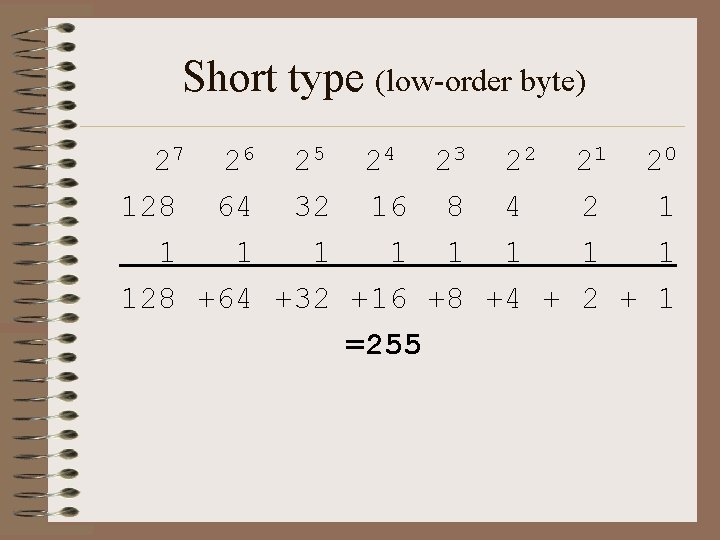
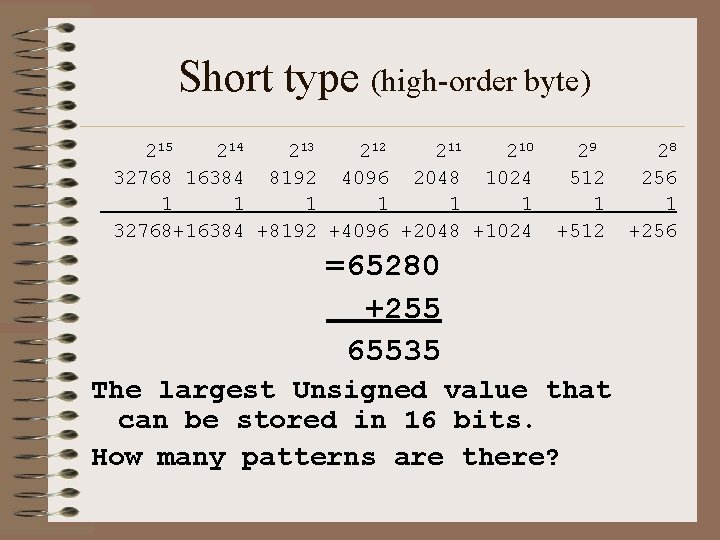
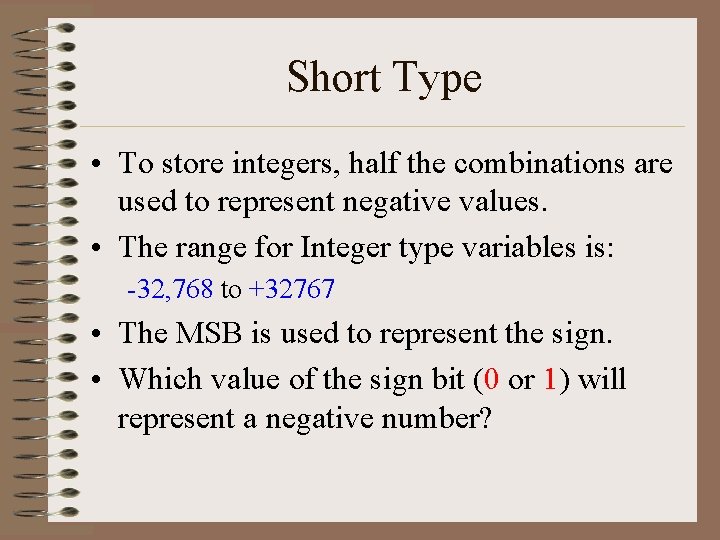
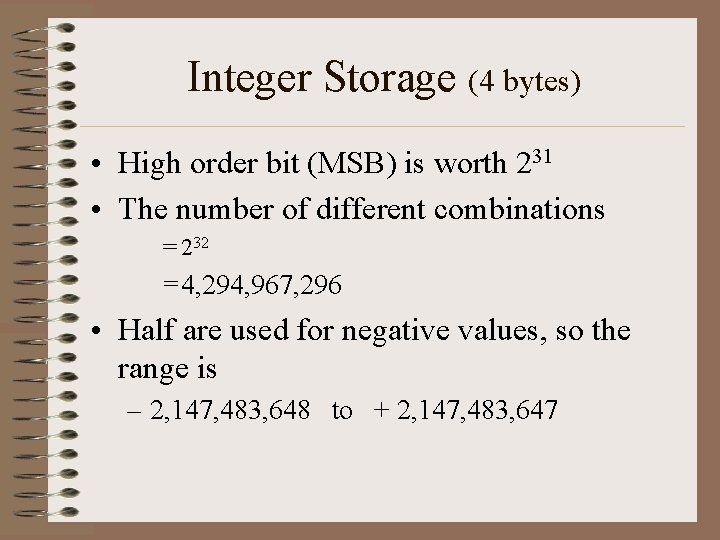
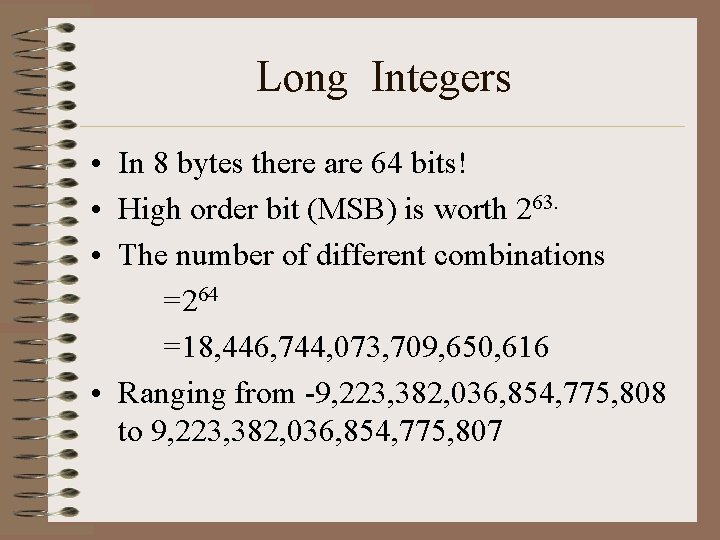
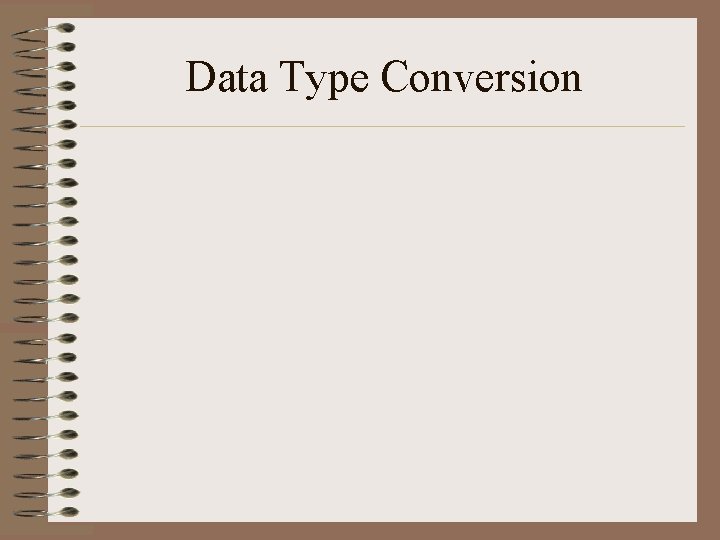
- Slides: 22
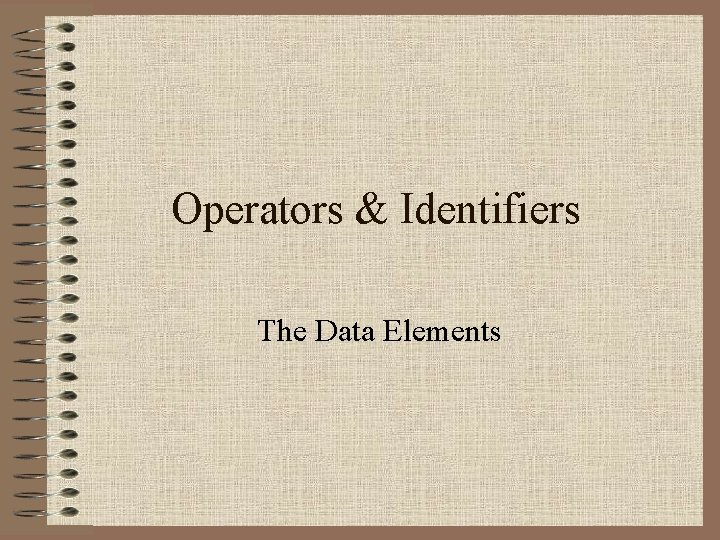
Operators & Identifiers The Data Elements
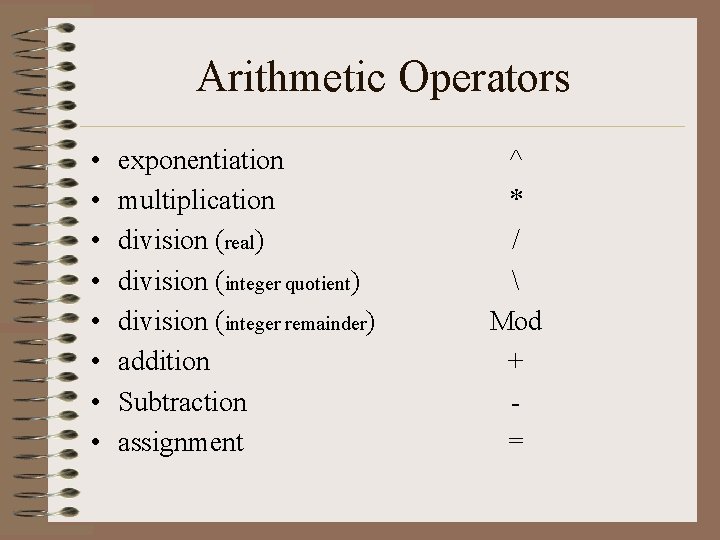
Arithmetic Operators • • exponentiation multiplication division (real) division (integer quotient) division (integer remainder) addition Subtraction assignment ^ * / Mod + =
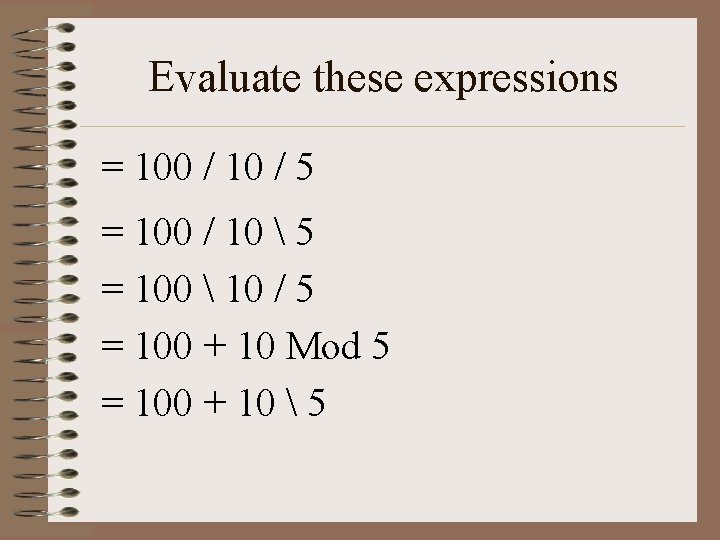
Evaluate these expressions = 100 / 10 / 5 = 100 / 10 5 = 100 10 / 5 = 100 + 10 Mod 5 = 100 + 10 5
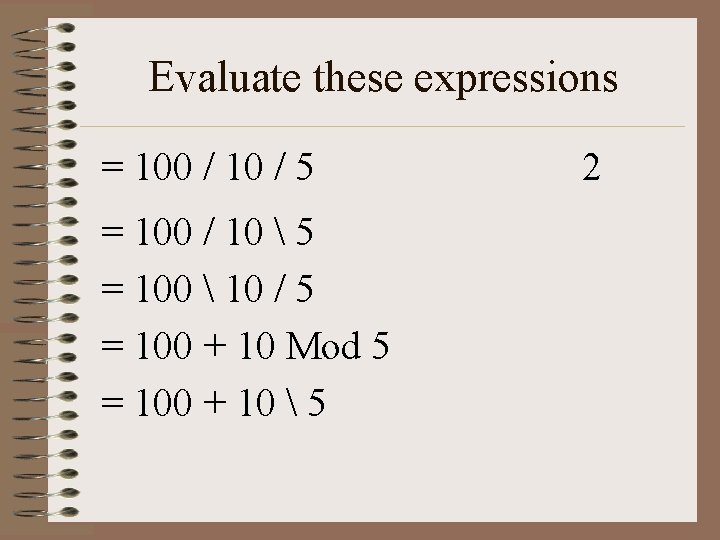
Evaluate these expressions = 100 / 10 / 5 = 100 / 10 5 = 100 10 / 5 = 100 + 10 Mod 5 = 100 + 10 5 2
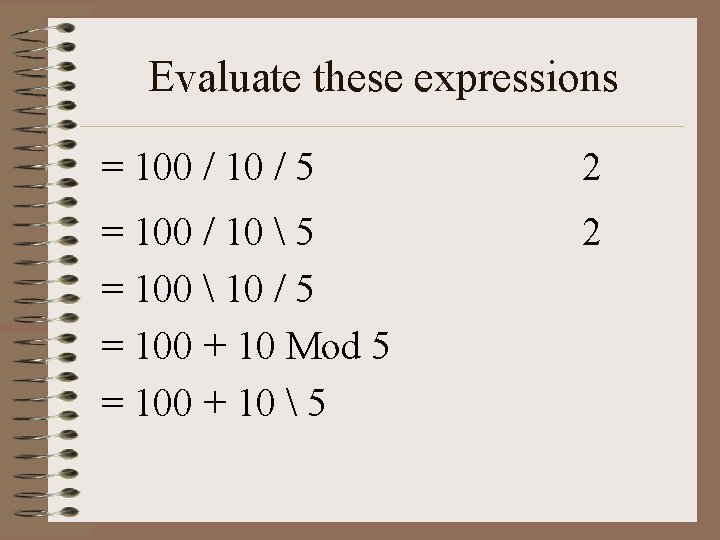
Evaluate these expressions = 100 / 10 / 5 2 = 100 / 10 5 = 100 10 / 5 = 100 + 10 Mod 5 = 100 + 10 5 2
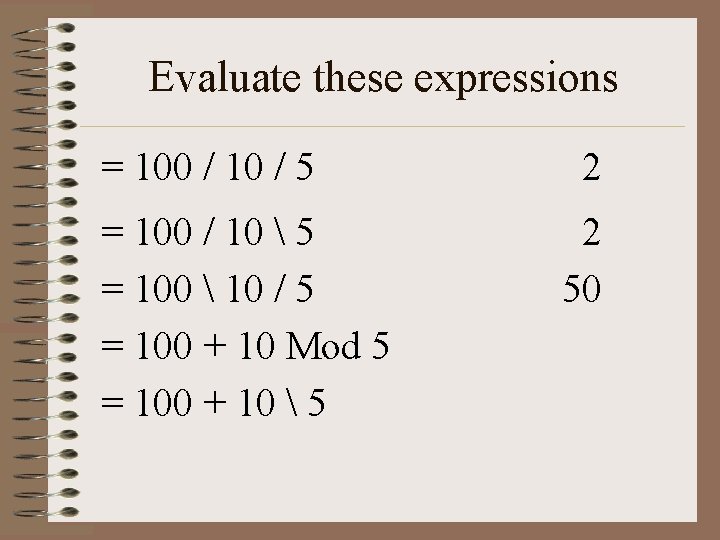
Evaluate these expressions = 100 / 10 / 5 = 100 / 10 5 = 100 10 / 5 = 100 + 10 Mod 5 = 100 + 10 5 2 2 50
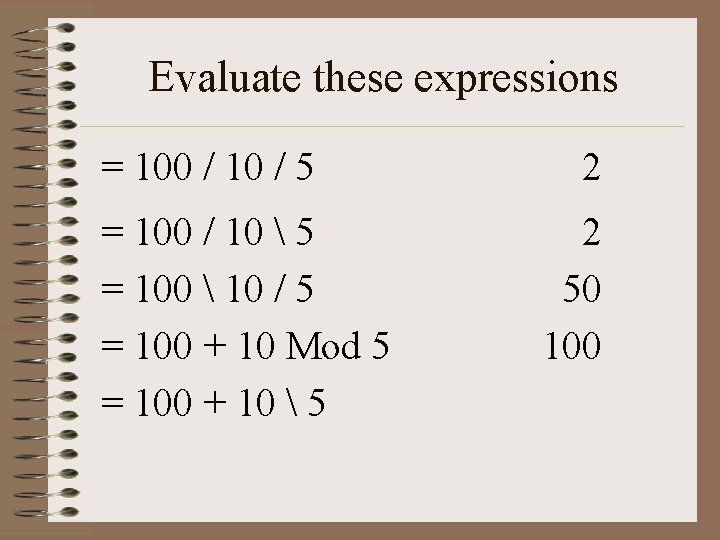
Evaluate these expressions = 100 / 10 / 5 = 100 / 10 5 = 100 10 / 5 = 100 + 10 Mod 5 = 100 + 10 5 2 2 50 100
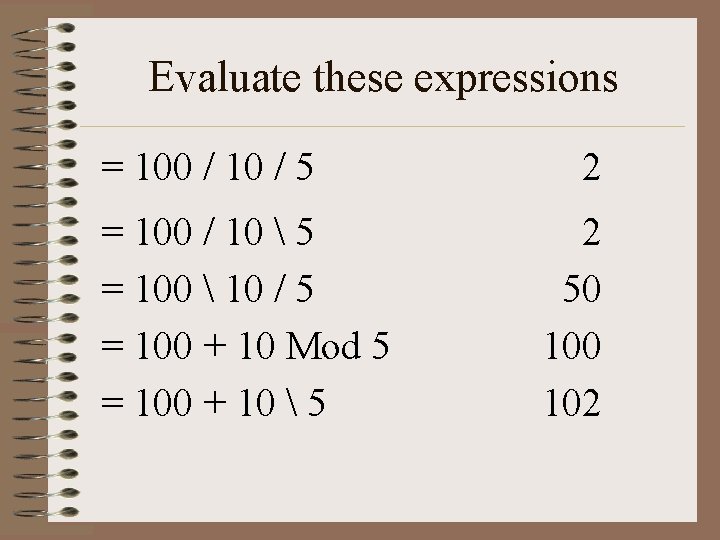
Evaluate these expressions = 100 / 10 / 5 = 100 / 10 5 = 100 10 / 5 = 100 + 10 Mod 5 = 100 + 10 5 2 2 50 102
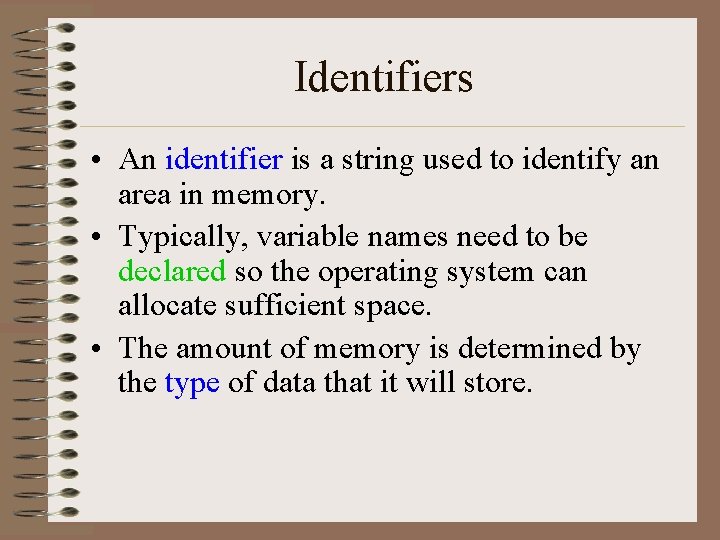
Identifiers • An identifier is a string used to identify an area in memory. • Typically, variable names need to be declared so the operating system can allocate sufficient space. • The amount of memory is determined by the type of data that it will store.
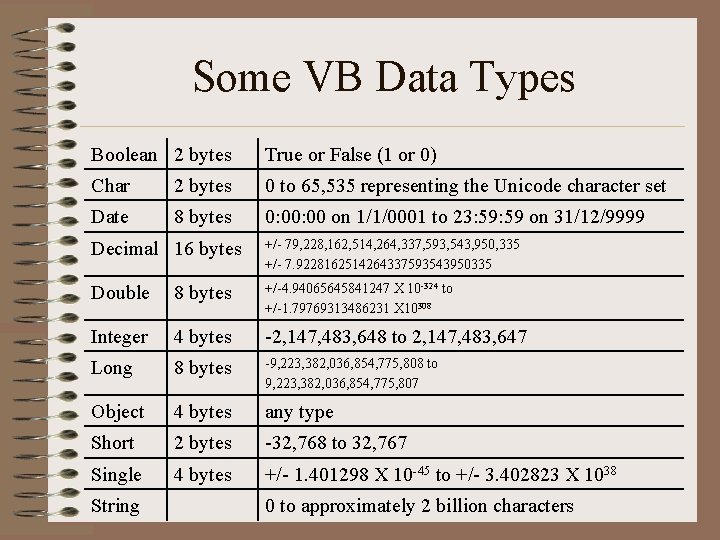
Some VB Data Types Boolean 2 bytes True or False (1 or 0) Char 2 bytes 0 to 65, 535 representing the Unicode character set Date 8 bytes 0: 00 on 1/1/0001 to 23: 59 on 31/12/9999 Decimal 16 bytes +/- 79, 228, 162, 514, 264, 337, 593, 543, 950, 335 +/- 7. 9228162514264337593543950335 Double 8 bytes +/-4. 94065645841247 X 10 -324 to +/-1. 79769313486231 X 10308 Integer 4 bytes -2, 147, 483, 648 to 2, 147, 483, 647 Long 8 bytes -9, 223, 382, 036, 854, 775, 808 to 9, 223, 382, 036, 854, 775, 807 Object 4 bytes any type Short 2 bytes -32, 768 to 32, 767 Single 4 bytes +/- 1. 401298 X 10 -45 to +/- 3. 402823 X 1038 String 0 to approximately 2 billion characters
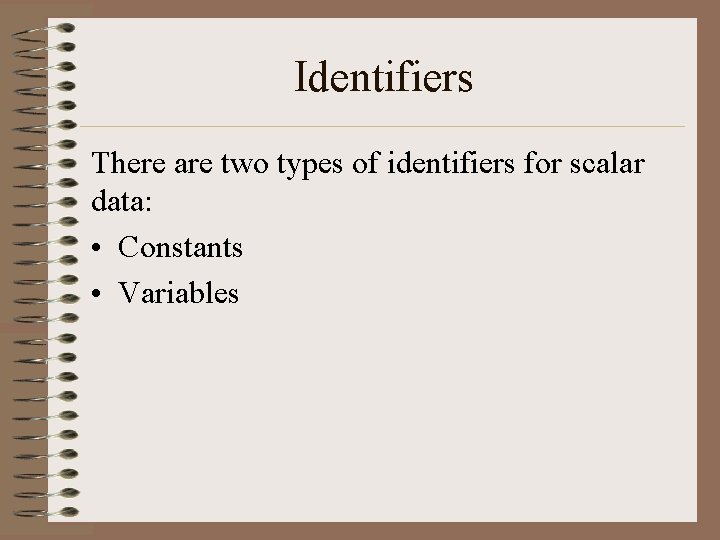
Identifiers There are two types of identifiers for scalar data: • Constants • Variables
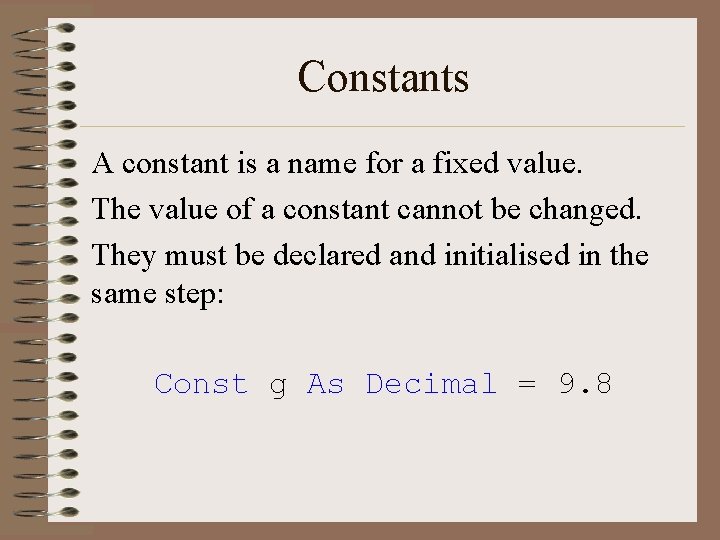
Constants A constant is a name for a fixed value. The value of a constant cannot be changed. They must be declared and initialised in the same step: Const g As Decimal = 9. 8
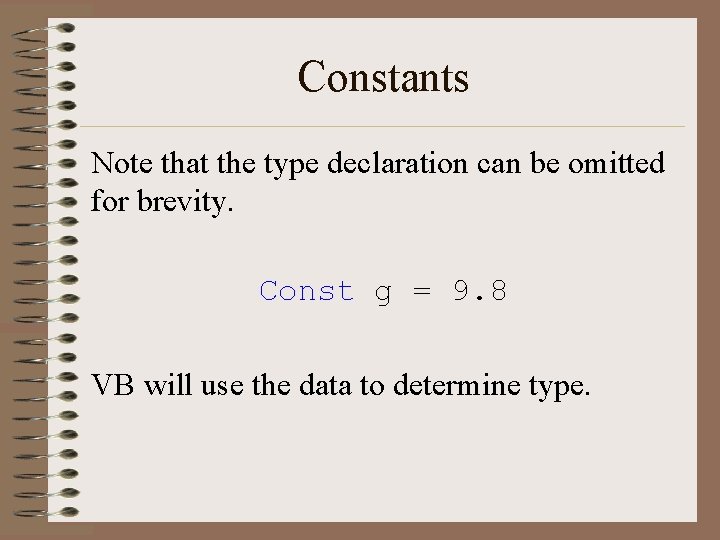
Constants Note that the type declaration can be omitted for brevity. Const g = 9. 8 VB will use the data to determine type.
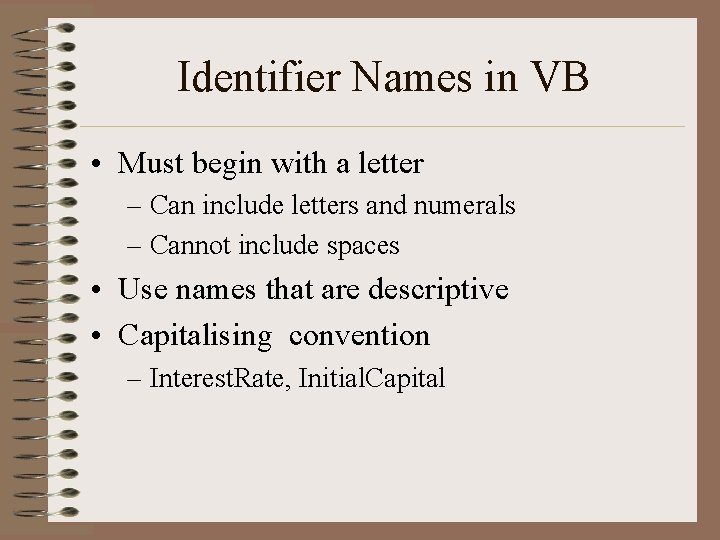
Identifier Names in VB • Must begin with a letter – Can include letters and numerals – Cannot include spaces • Use names that are descriptive • Capitalising convention – Interest. Rate, Initial. Capital
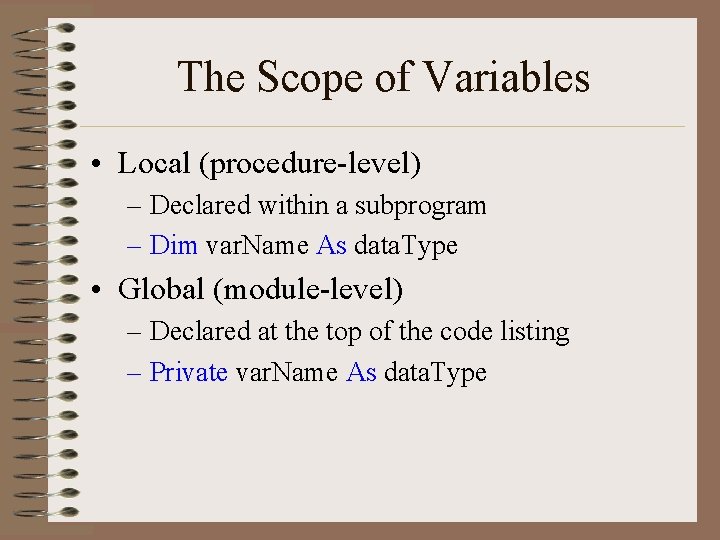
The Scope of Variables • Local (procedure-level) – Declared within a subprogram – Dim var. Name As data. Type • Global (module-level) – Declared at the top of the code listing – Private var. Name As data. Type
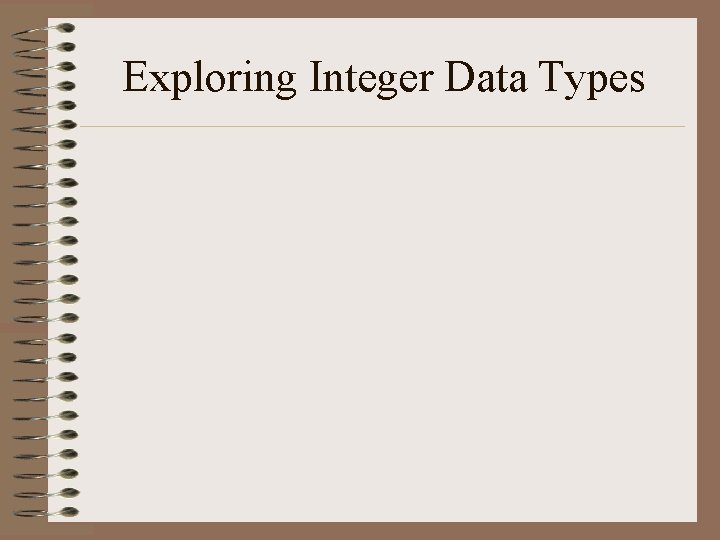
Exploring Integer Data Types
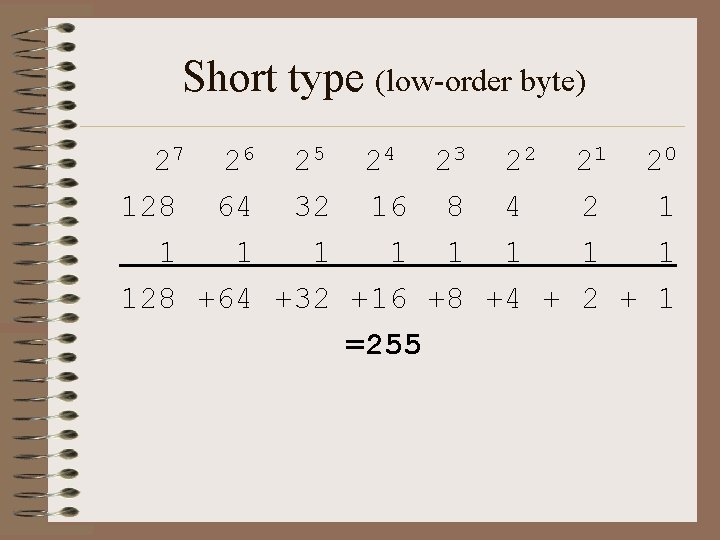
Short type (low-order byte) 27 2 6 2 5 2 4 2 3 2 2 128 64 32 16 8 4 1 1 1 128 +64 +32 +16 +8 +4 + =255 21 20 2 1 1 1 2 + 1
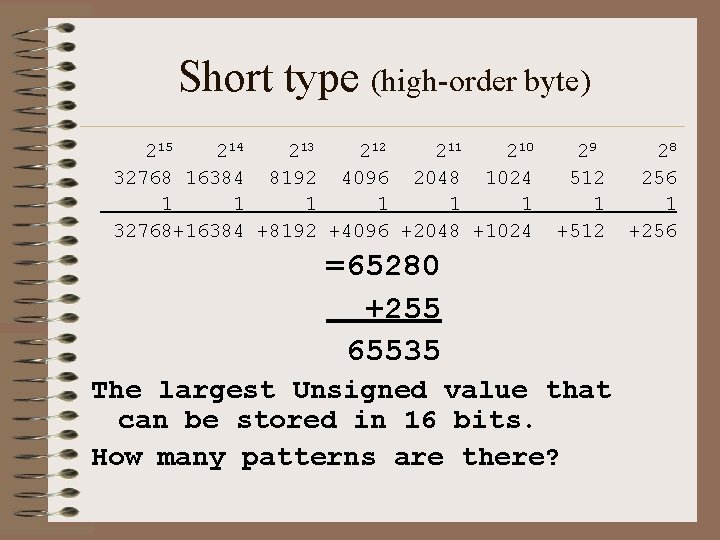
Short type (high-order byte) 215 214 213 212 211 210 32768 16384 8192 4096 2048 1024 1 1 1 32768+16384 +8192 +4096 +2048 +1024 29 512 1 +512 =65280 +255 65535 The largest Unsigned value that can be stored in 16 bits. How many patterns are there? 28 256 1 +256
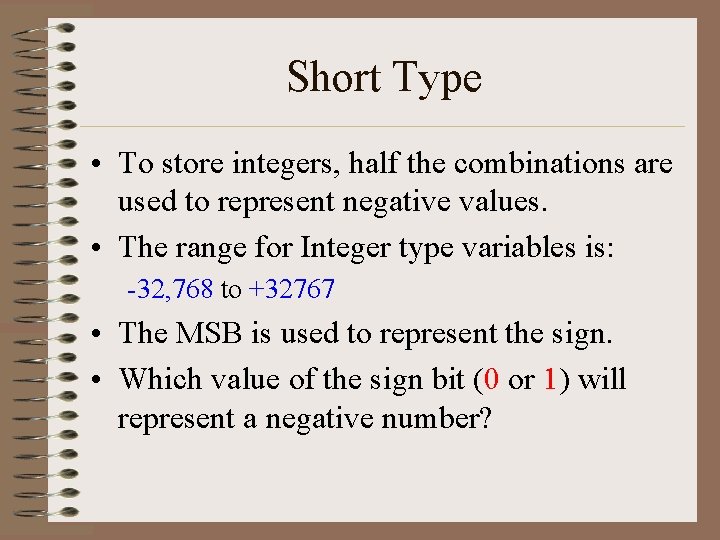
Short Type • To store integers, half the combinations are used to represent negative values. • The range for Integer type variables is: -32, 768 to +32767 • The MSB is used to represent the sign. • Which value of the sign bit (0 or 1) will represent a negative number?
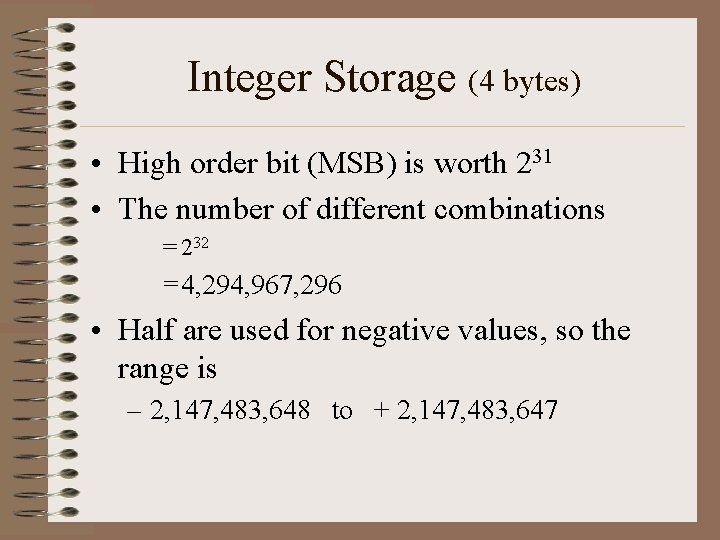
Integer Storage (4 bytes) • High order bit (MSB) is worth 231 • The number of different combinations = 232 = 4, 294, 967, 296 • Half are used for negative values, so the range is – 2, 147, 483, 648 to + 2, 147, 483, 647
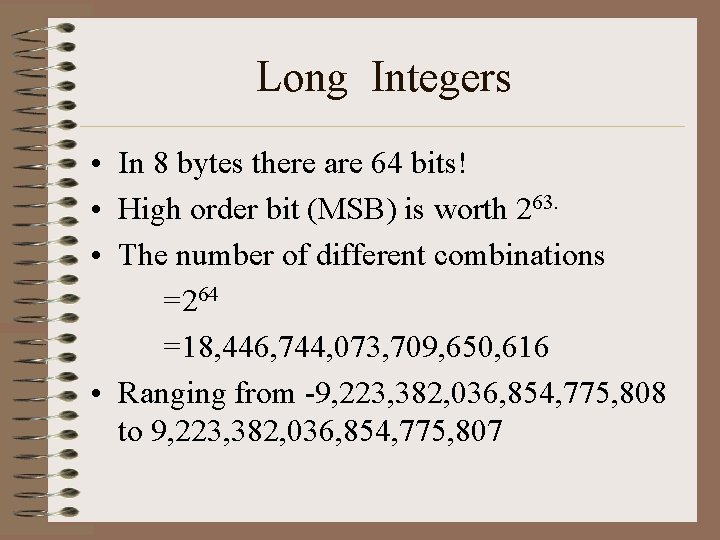
Long Integers • In 8 bytes there are 64 bits! • High order bit (MSB) is worth 263. • The number of different combinations =264 =18, 446, 744, 073, 709, 650, 616 • Ranging from -9, 223, 382, 036, 854, 775, 808 to 9, 223, 382, 036, 854, 775, 807
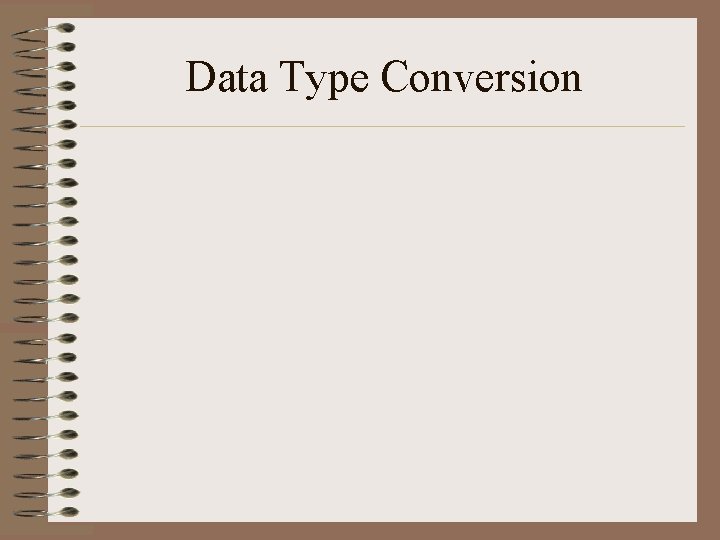
Data Type Conversion