Operating Systems Practical Session 12 Deadlocks Deadlocks The
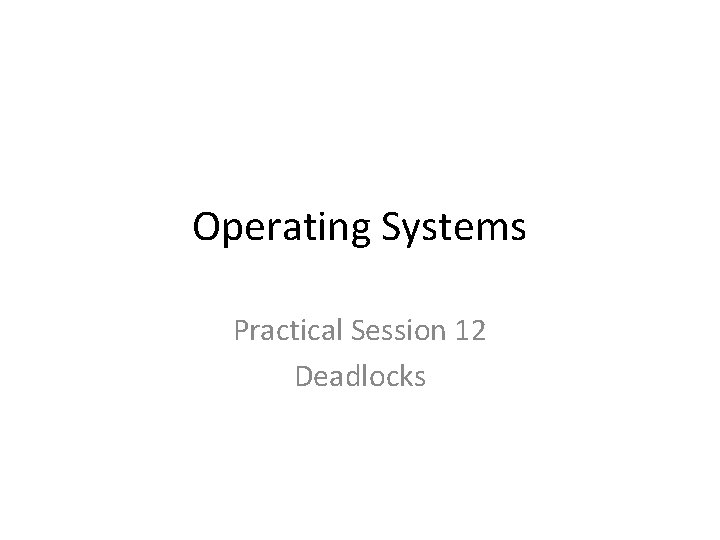
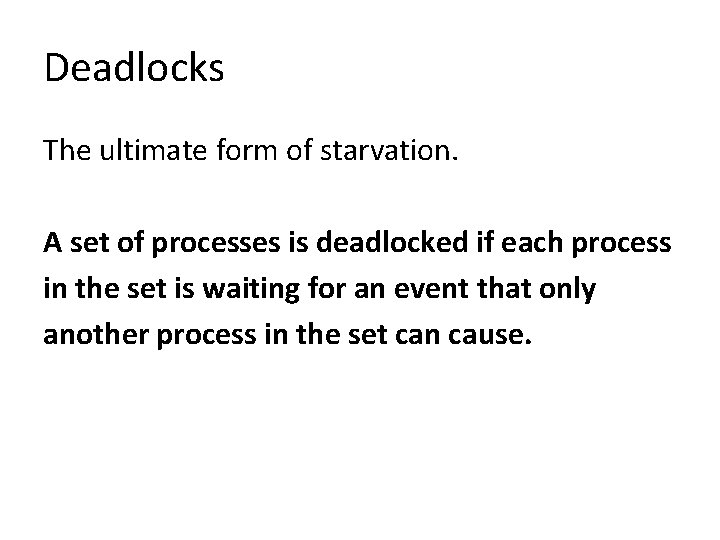
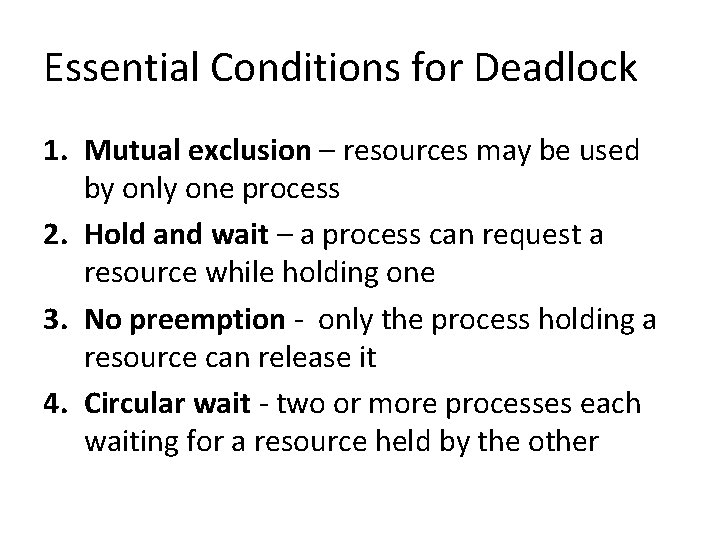
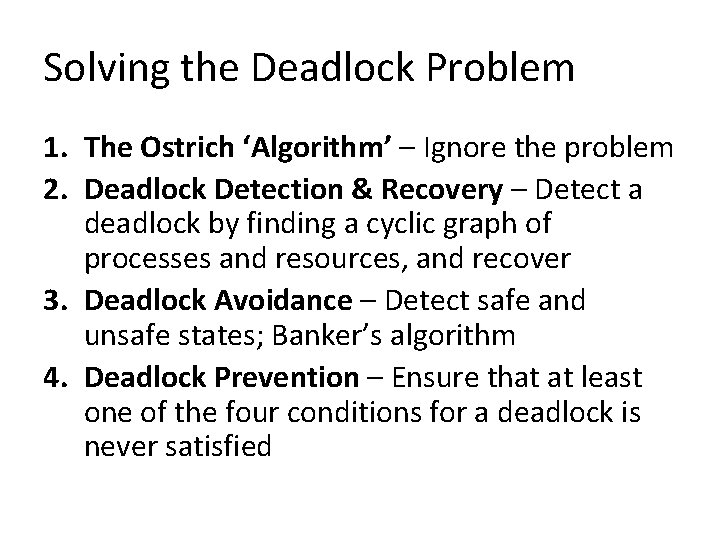
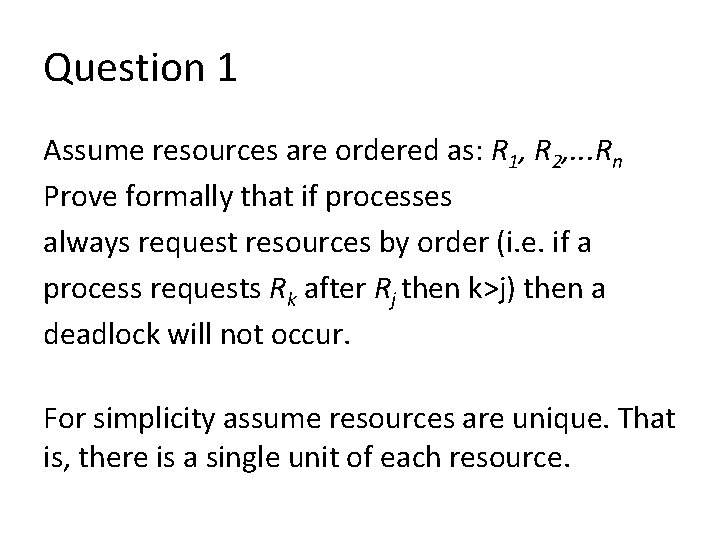
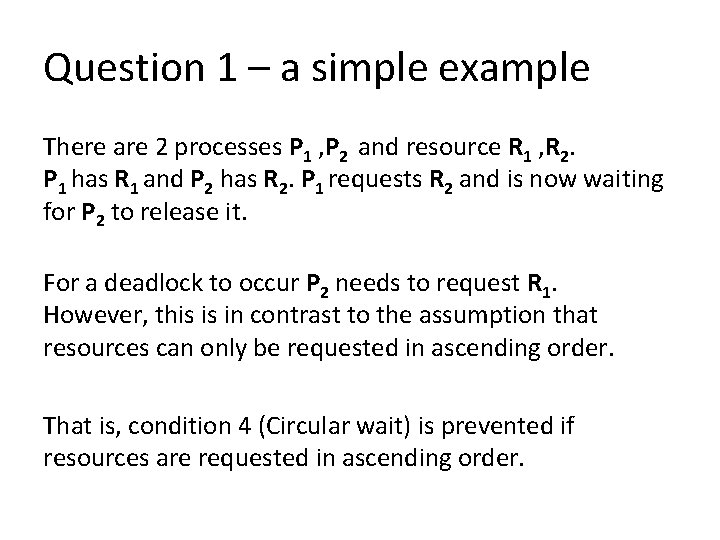
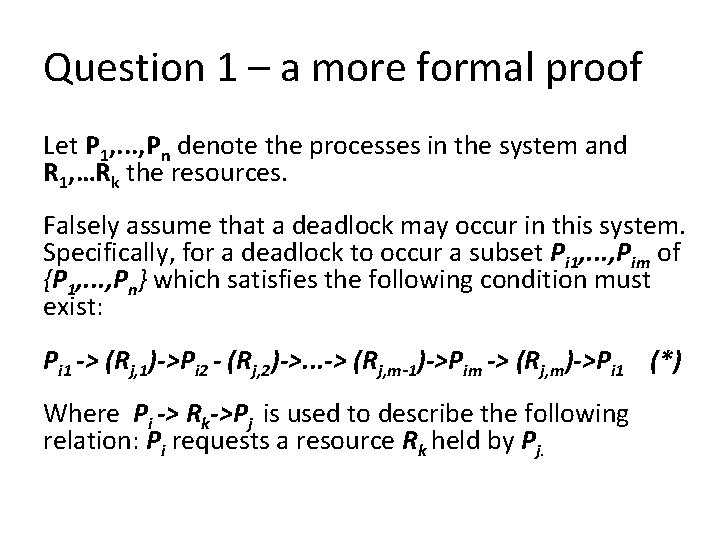
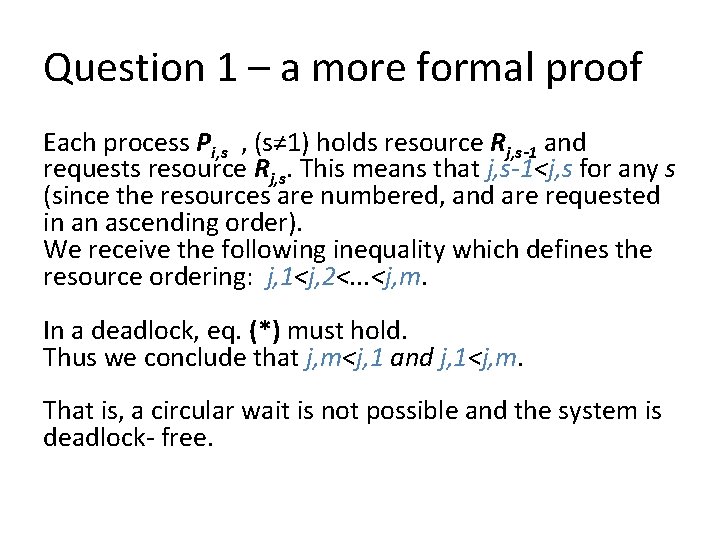
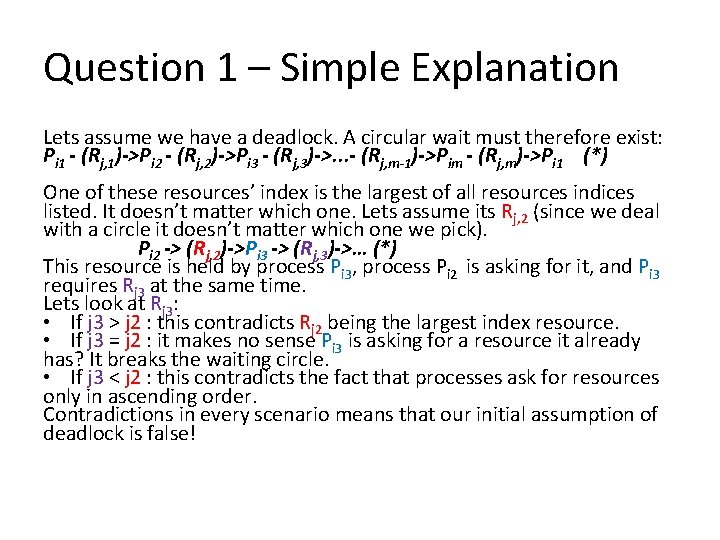
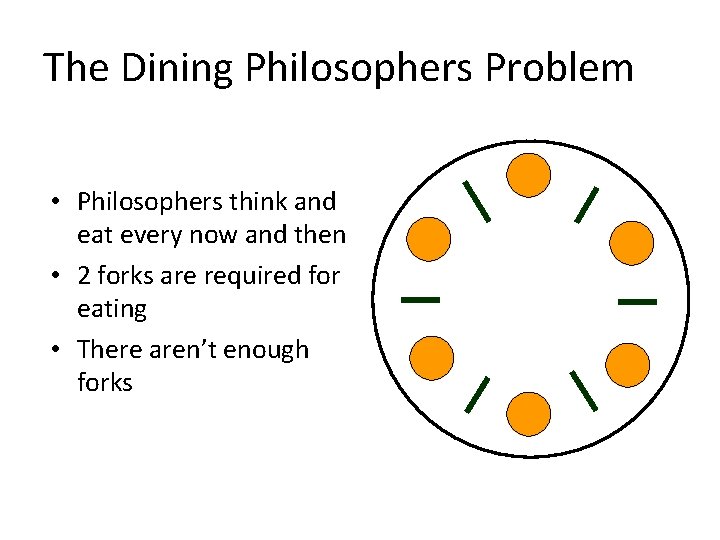
![Dining Philosophers: a possible implementation Semaphore fork[N]; procedure pick_sticks(i) pick_sticks down(fork[LEFT]); down(fork[RIGHT]); procedure put_sticks(i) Dining Philosophers: a possible implementation Semaphore fork[N]; procedure pick_sticks(i) pick_sticks down(fork[LEFT]); down(fork[RIGHT]); procedure put_sticks(i)](https://slidetodoc.com/presentation_image/474ca96b4a20df71494fd932c1196ae8/image-11.jpg)
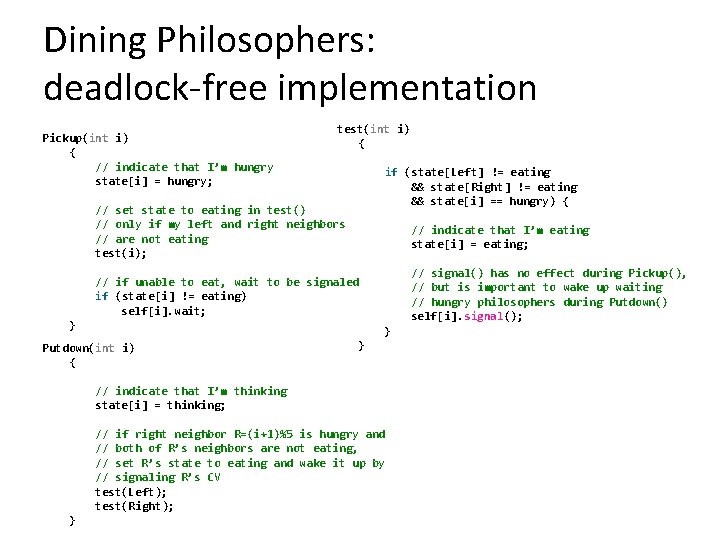
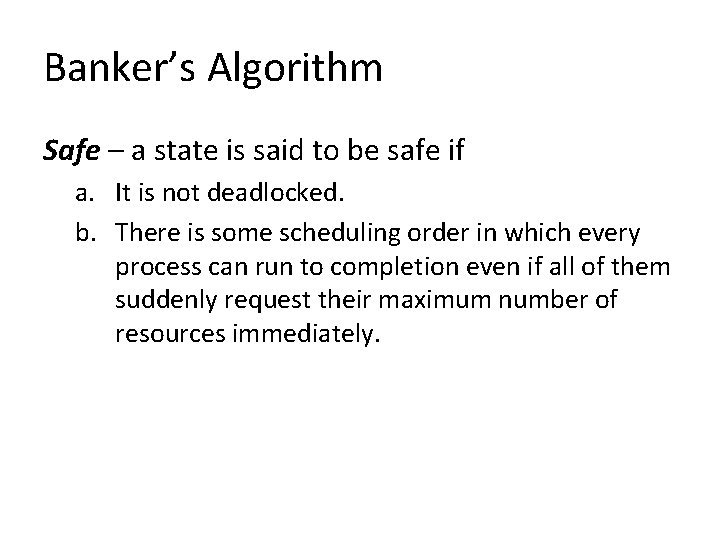
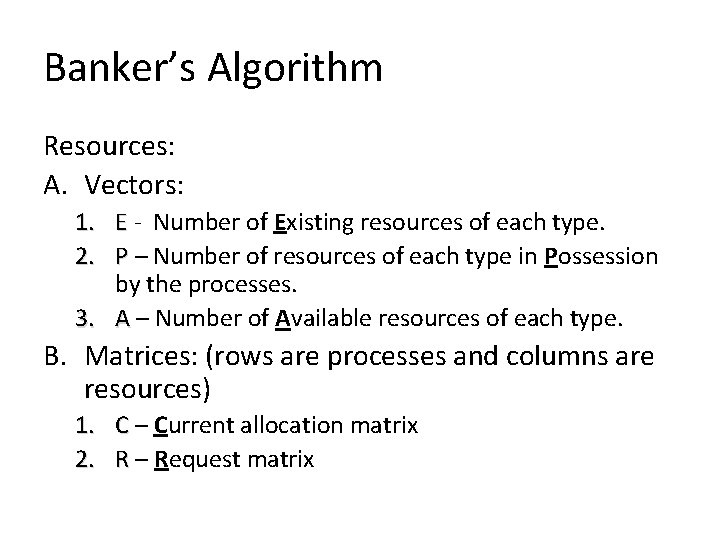
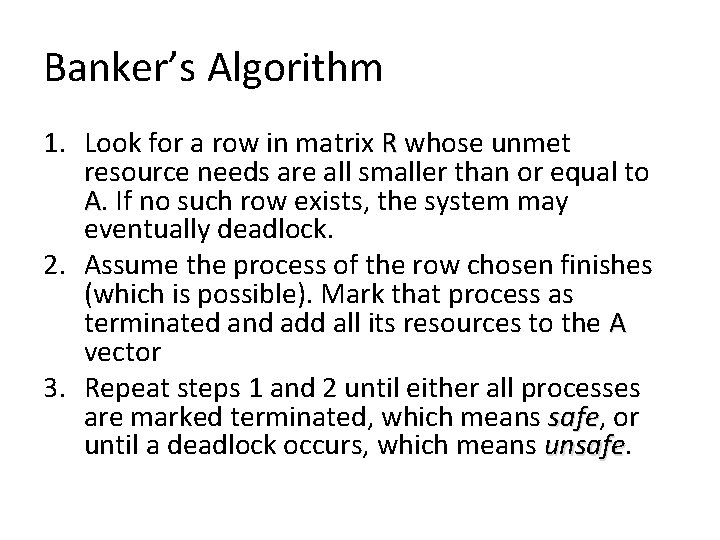
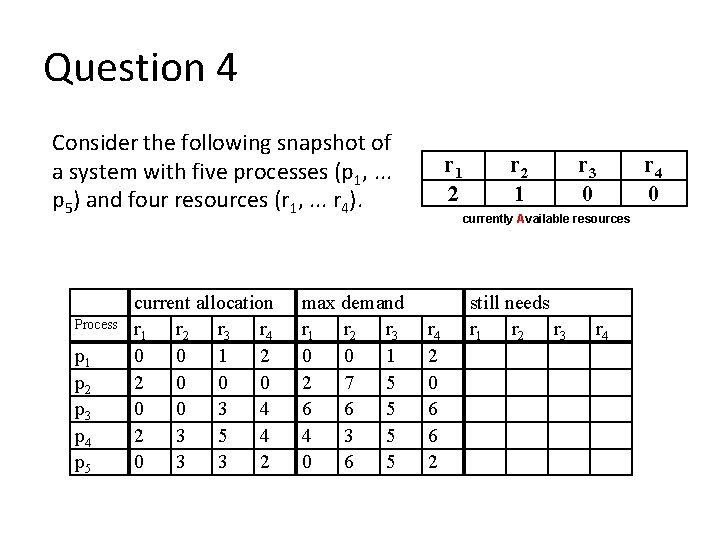
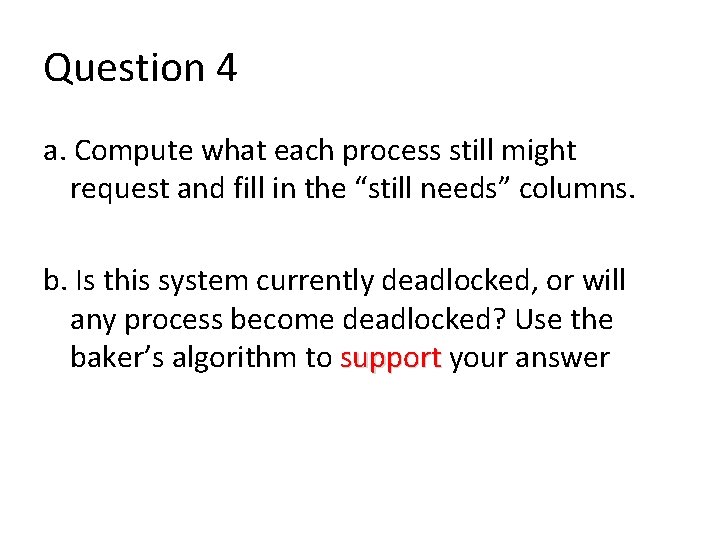
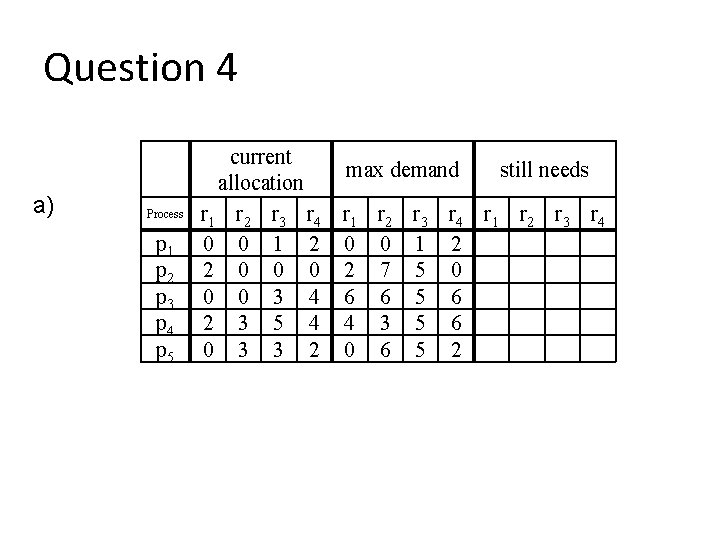
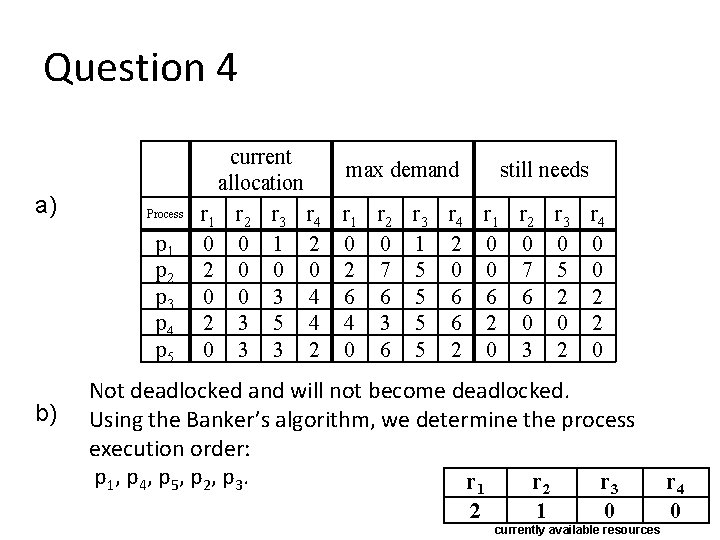
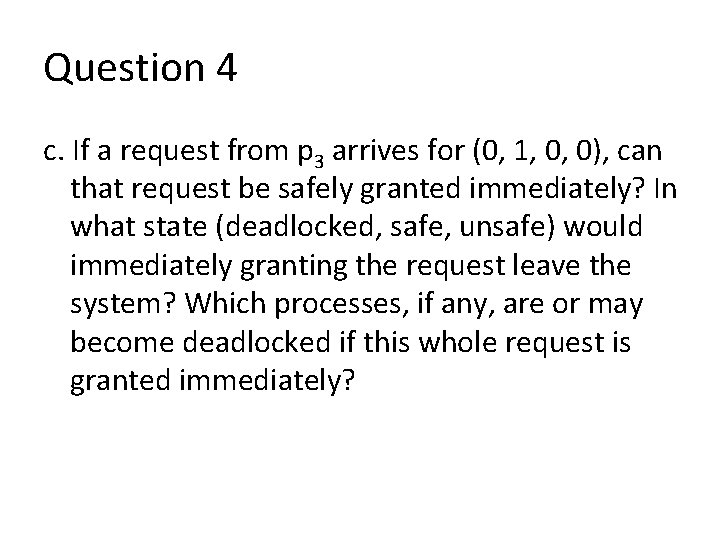
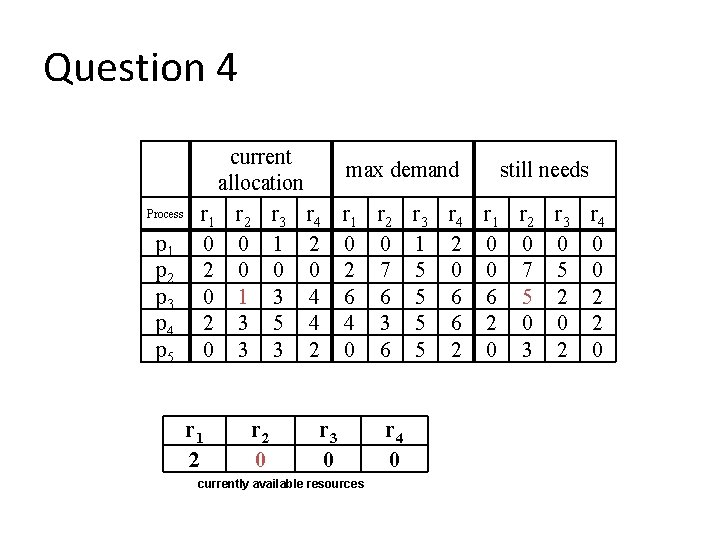
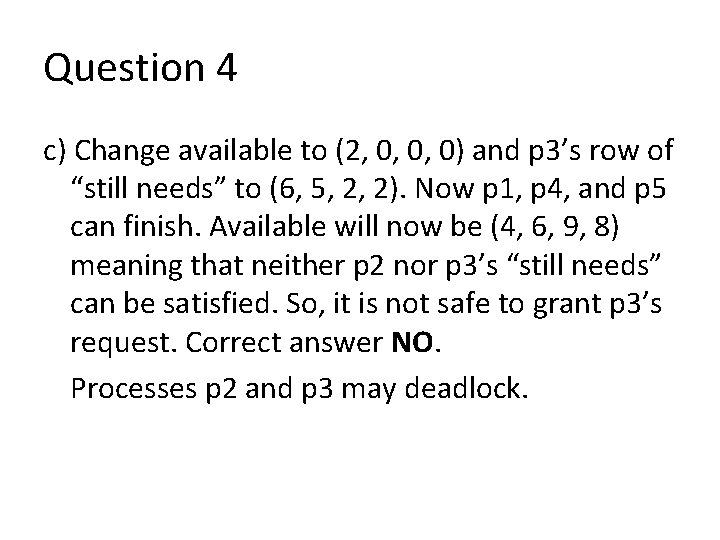
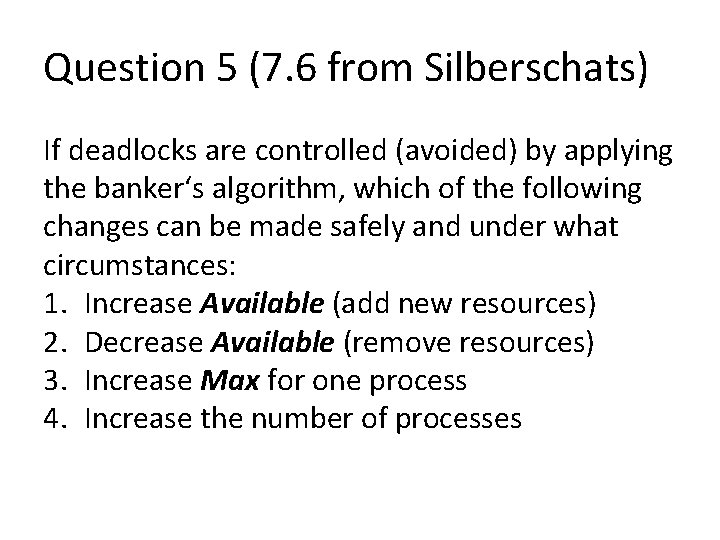
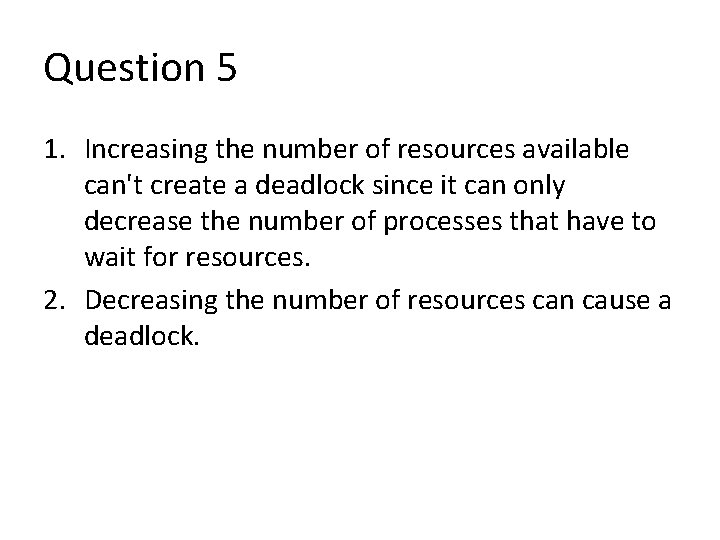
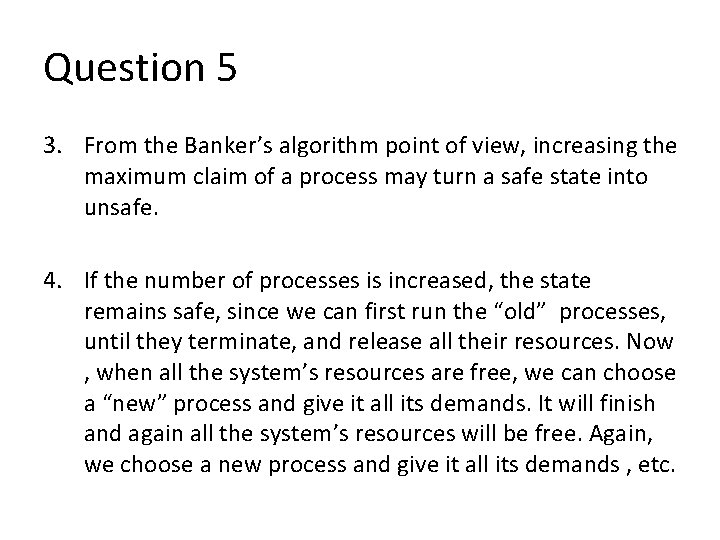
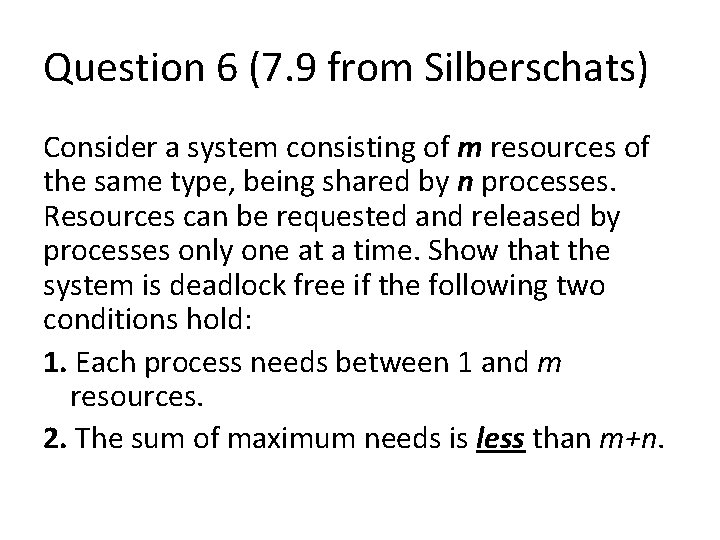
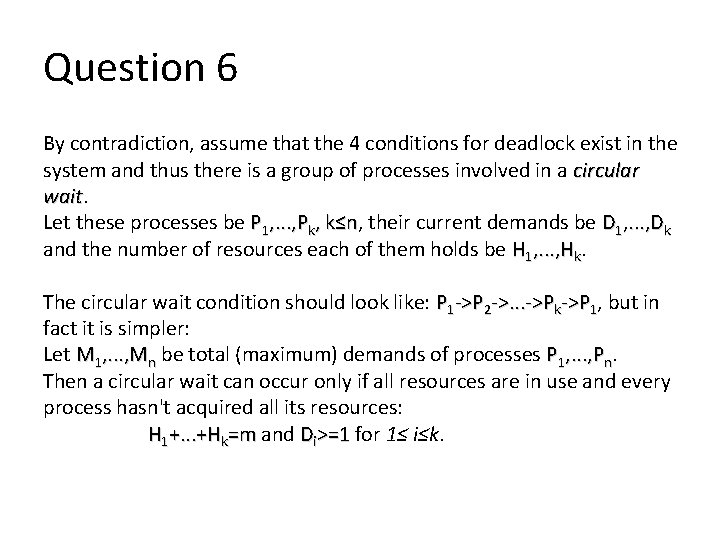
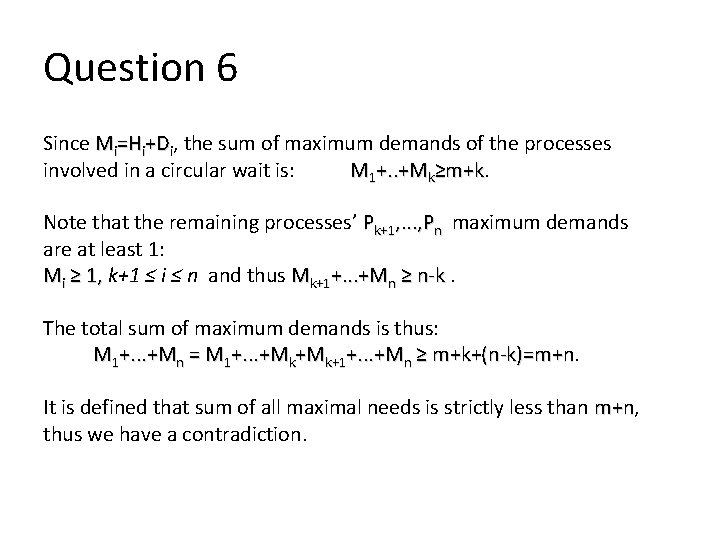
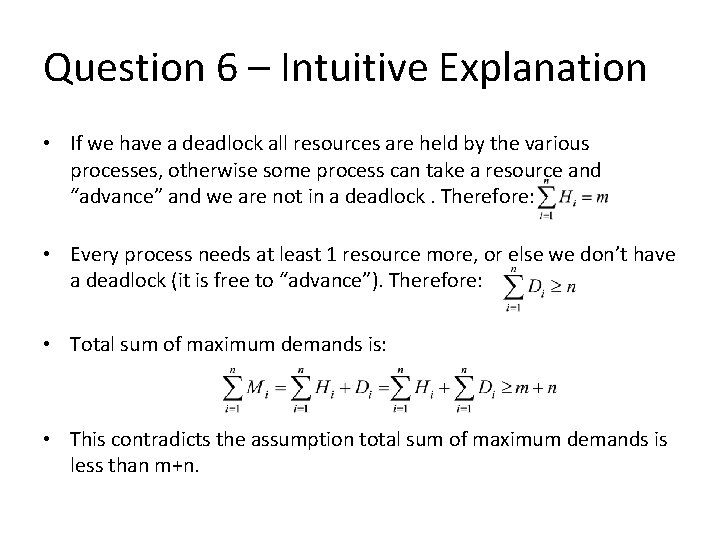
- Slides: 29
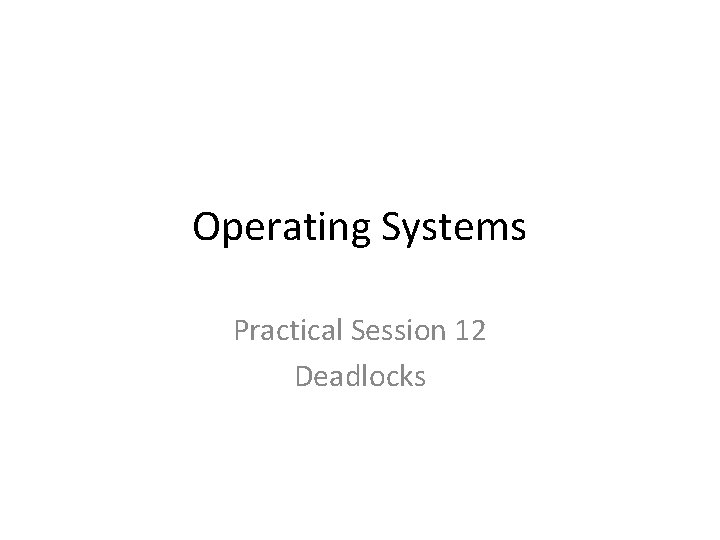
Operating Systems Practical Session 12 Deadlocks
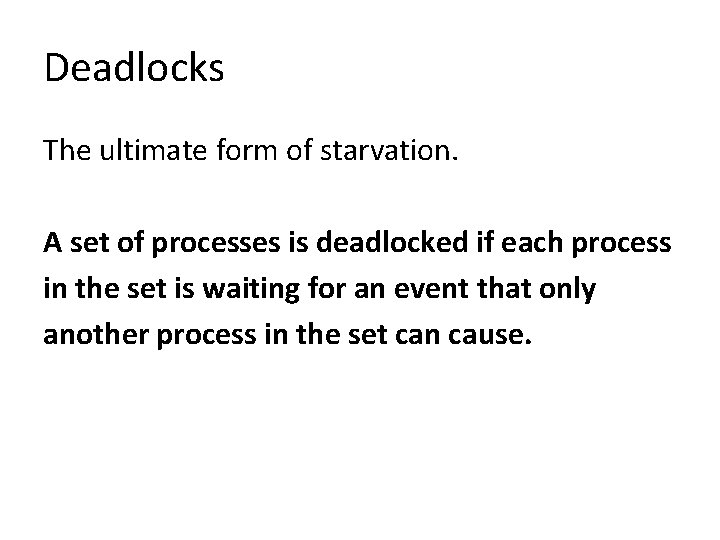
Deadlocks The ultimate form of starvation. A set of processes is deadlocked if each process in the set is waiting for an event that only another process in the set can cause.
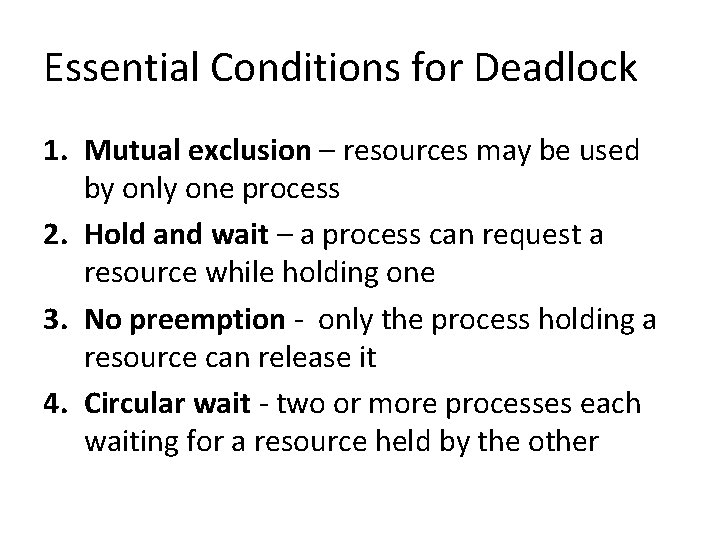
Essential Conditions for Deadlock 1. Mutual exclusion – resources may be used by only one process 2. Hold and wait – a process can request a resource while holding one 3. No preemption - only the process holding a resource can release it 4. Circular wait - two or more processes each waiting for a resource held by the other
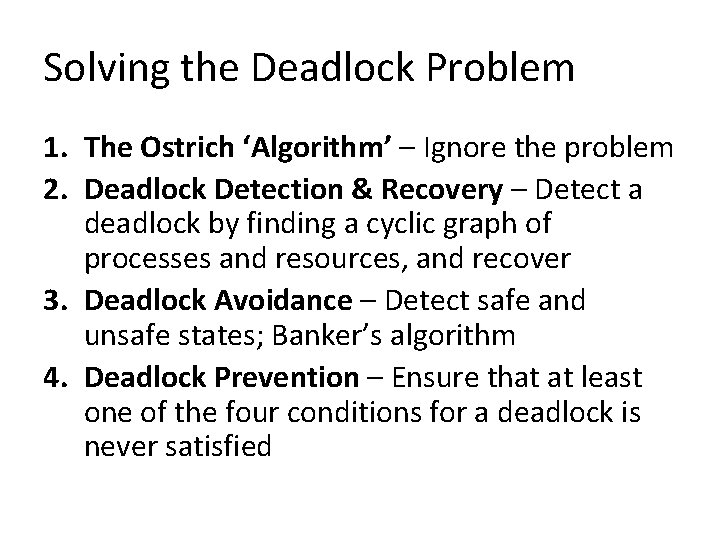
Solving the Deadlock Problem 1. The Ostrich ‘Algorithm’ – Ignore the problem 2. Deadlock Detection & Recovery – Detect a deadlock by finding a cyclic graph of processes and resources, and recover 3. Deadlock Avoidance – Detect safe and unsafe states; Banker’s algorithm 4. Deadlock Prevention – Ensure that at least one of the four conditions for a deadlock is never satisfied
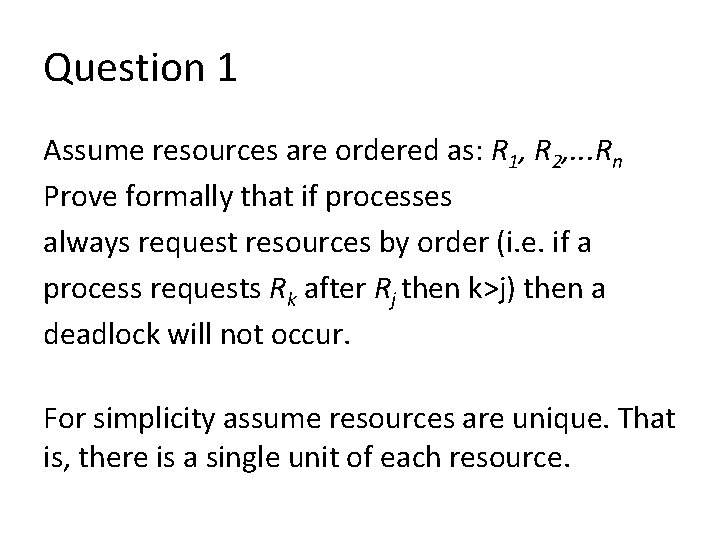
Question 1 Assume resources are ordered as: R 1, R 2, . . . Rn Prove formally that if processes always request resources by order (i. e. if a process requests Rk after Rj then k>j) then a deadlock will not occur. For simplicity assume resources are unique. That is, there is a single unit of each resource.
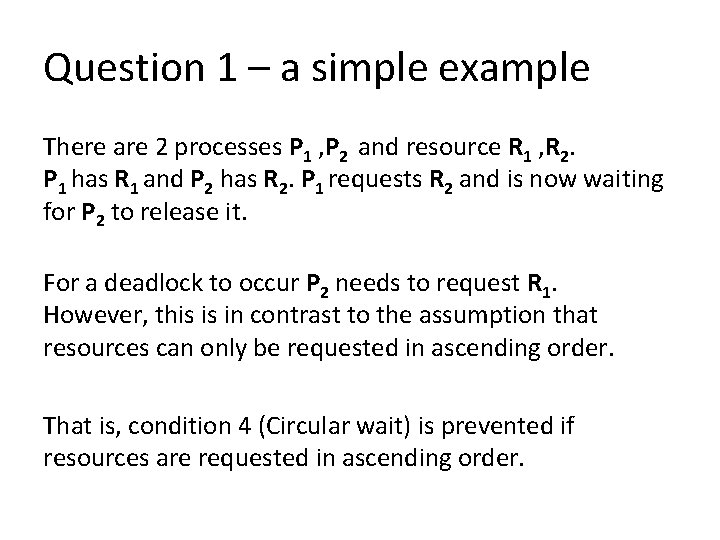
Question 1 – a simple example There are 2 processes P 1 , P 2 and resource R 1 , R 2. P 1 has R 1 and P 2 has R 2. P 1 requests R 2 and is now waiting for P 2 to release it. For a deadlock to occur P 2 needs to request R 1. However, this is in contrast to the assumption that resources can only be requested in ascending order. That is, condition 4 (Circular wait) is prevented if resources are requested in ascending order.
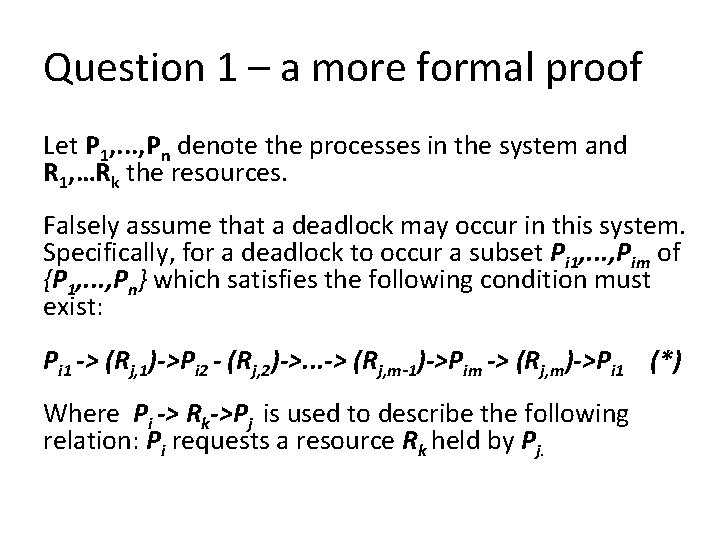
Question 1 – a more formal proof Let P 1, . . . , Pn denote the processes in the system and R 1, …Rk the resources. Falsely assume that a deadlock may occur in this system. Specifically, for a deadlock to occur a subset Pi 1, . . . , Pim of {P 1, . . . , Pn} which satisfies the following condition must exist: Pi 1 -> (Rj, 1)->Pi 2 - (Rj, 2)->. . . -> (Rj, m-1)->Pim -> (Rj, m)->Pi 1 (*) Where Pi -> Rk->Pj is used to describe the following relation: Pi requests a resource Rk held by Pj.
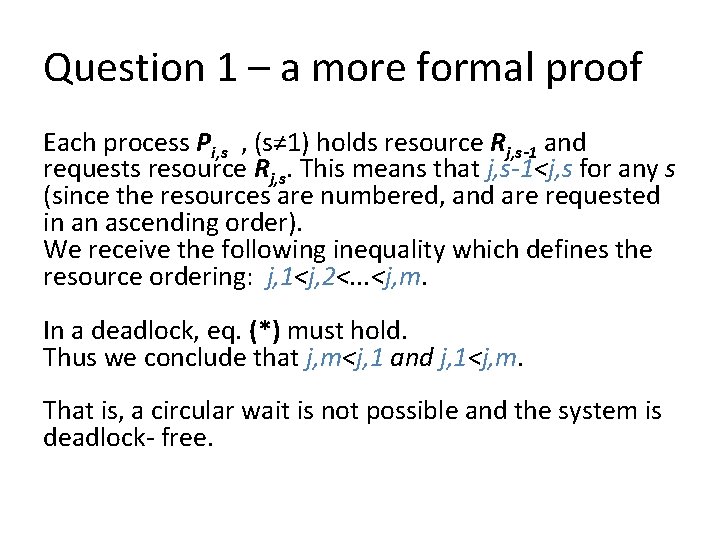
Question 1 – a more formal proof Each process Pi, s , (s≠ 1) holds resource Rj, s-1 and requests resource Rj, s. This means that j, s-1<j, s for any s (since the resources are numbered, and are requested in an ascending order). We receive the following inequality which defines the resource ordering: j, 1<j, 2<. . . <j, m. In a deadlock, eq. (*) must hold. Thus we conclude that j, m<j, 1 and j, 1<j, m. That is, a circular wait is not possible and the system is deadlock- free.
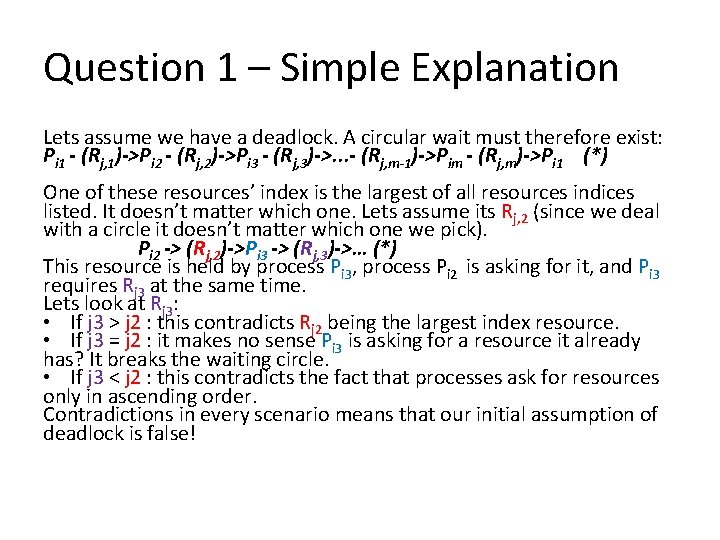
Question 1 – Simple Explanation Lets assume we have a deadlock. A circular wait must therefore exist: Pi 1 - (Rj, 1)->Pi 2 - (Rj, 2)->Pi 3 - (Rj, 3)->. . . - (Rj, m-1)->Pim - (Rj, m)->Pi 1 (*) One of these resources’ index is the largest of all resources indices listed. It doesn’t matter which one. Lets assume its Rj, 2 (since we deal with a circle it doesn’t matter which one we pick). Pi 2 -> (Rj, 2)->Pi 3 -> (Rj, 3)->… (*) This resource is held by process Pi 3, process Pi 2 is asking for it, and Pi 3 requires Rj 3 at the same time. Lets look at Rj 3: • If j 3 > j 2 : this contradicts Rj 2 being the largest index resource. • If j 3 = j 2 : it makes no sense Pi 3 is asking for a resource it already has? It breaks the waiting circle. • If j 3 < j 2 : this contradicts the fact that processes ask for resources only in ascending order. Contradictions in every scenario means that our initial assumption of deadlock is false!
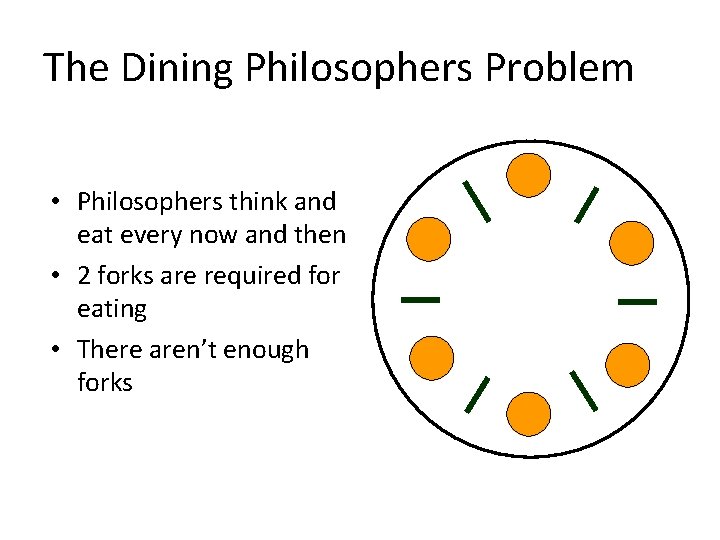
The Dining Philosophers Problem • Philosophers think and eat every now and then • 2 forks are required for eating • There aren’t enough forks
![Dining Philosophers a possible implementation Semaphore forkN procedure picksticksi picksticks downforkLEFT downforkRIGHT procedure putsticksi Dining Philosophers: a possible implementation Semaphore fork[N]; procedure pick_sticks(i) pick_sticks down(fork[LEFT]); down(fork[RIGHT]); procedure put_sticks(i)](https://slidetodoc.com/presentation_image/474ca96b4a20df71494fd932c1196ae8/image-11.jpg)
Dining Philosophers: a possible implementation Semaphore fork[N]; procedure pick_sticks(i) pick_sticks down(fork[LEFT]); down(fork[RIGHT]); procedure put_sticks(i) put_sticks up(fork[RIGHT]); up(fork[LEFT]);
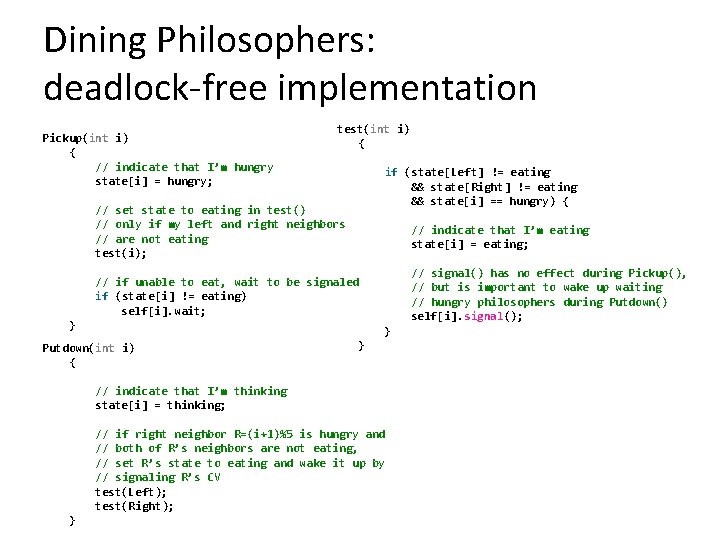
Dining Philosophers: deadlock-free implementation test(int i) Pickup(int i) { { // indicate that I’m hungry if (state[Left] != eating state[i] = hungry; && state[Right] != eating && state[i] == hungry) { // set state to eating in test() // only if my left and right neighbors // indicate that I’m eating // are not eating state[i] = eating; test(i); // signal() has no effect during Pickup(), // if unable to eat, wait to be signaled // but is important to wake up waiting if (state[i] != eating) // hungry philosophers during Putdown() self[i]. wait; self[i]. signal(); } } Putdown(int i) { // indicate that I’m thinking state[i] = thinking; // if right neighbor R=(i+1)%5 is hungry and // both of R’s neighbors are not eating, // set R’s state to eating and wake it up by // signaling R’s CV test(Left); test(Right); }
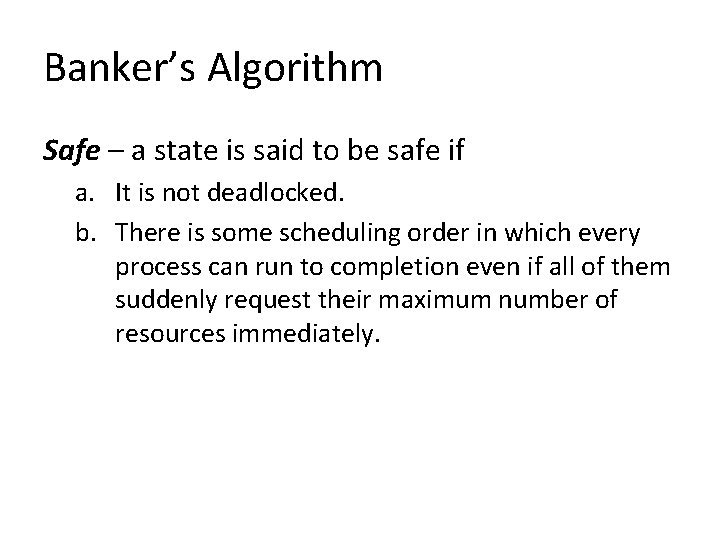
Banker’s Algorithm Safe – a state is said to be safe if a. It is not deadlocked. b. There is some scheduling order in which every process can run to completion even if all of them suddenly request their maximum number of resources immediately.
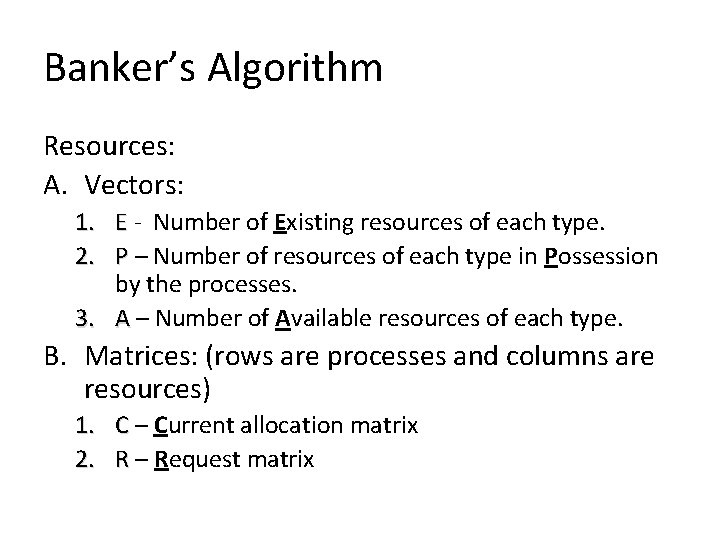
Banker’s Algorithm Resources: A. Vectors: 1. E - Number of Existing resources of each type. 2. P – Number of resources of each type in Possession by the processes. 3. A – Number of Available resources of each type. B. Matrices: (rows are processes and columns are resources) 1. C – Current allocation matrix 2. R – Request matrix
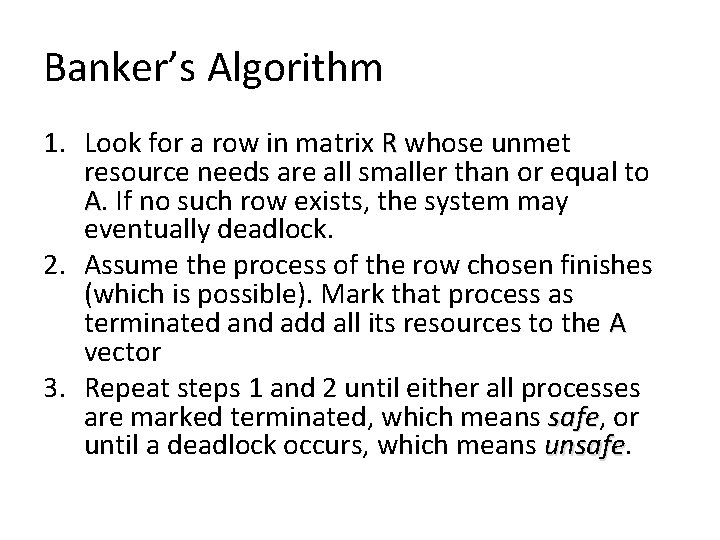
Banker’s Algorithm 1. Look for a row in matrix R whose unmet resource needs are all smaller than or equal to A. If no such row exists, the system may eventually deadlock. 2. Assume the process of the row chosen finishes (which is possible). Mark that process as terminated and add all its resources to the A vector 3. Repeat steps 1 and 2 until either all processes are marked terminated, which means safe, or safe until a deadlock occurs, which means unsafe
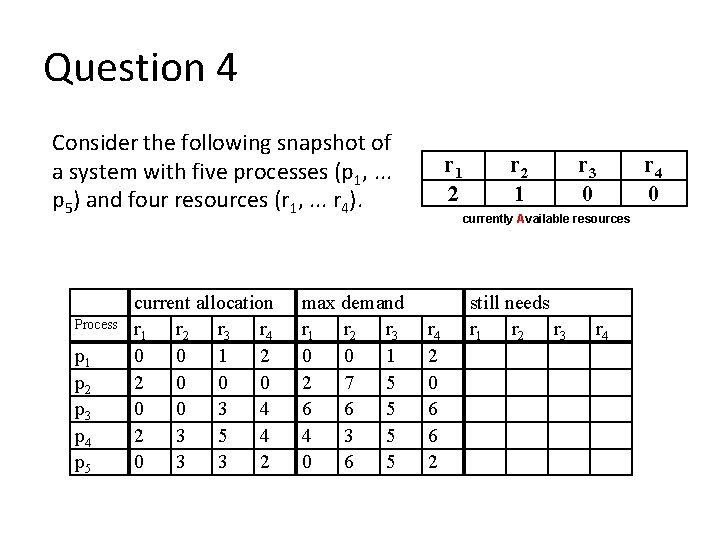
Question 4 Consider the following snapshot of a system with five processes (p 1, . . . p 5) and four resources (r 1, . . . r 4). Process p 1 p 2 p 3 p 4 p 5 current allocation r 1 r 2 r 3 r 4 0 0 1 2 2 0 0 0 3 4 2 3 5 4 0 3 3 2 max demand r 1 r 2 r 3 0 0 1 2 7 5 6 6 5 4 3 5 0 6 5 r 1 2 r 2 1 r 3 0 currently Available resources r 4 2 0 6 6 2 still needs r 1 r 2 r 3 r 4 0
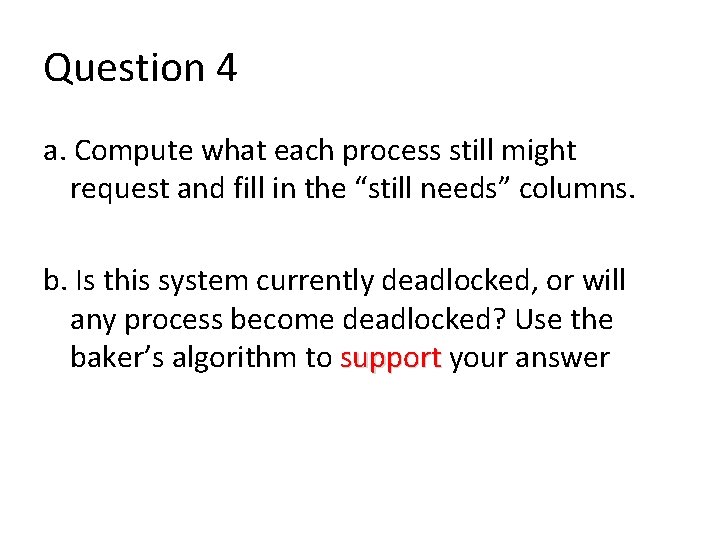
Question 4 a. Compute what each process still might request and fill in the “still needs” columns. b. Is this system currently deadlocked, or will any process become deadlocked? Use the baker’s algorithm to support your answer
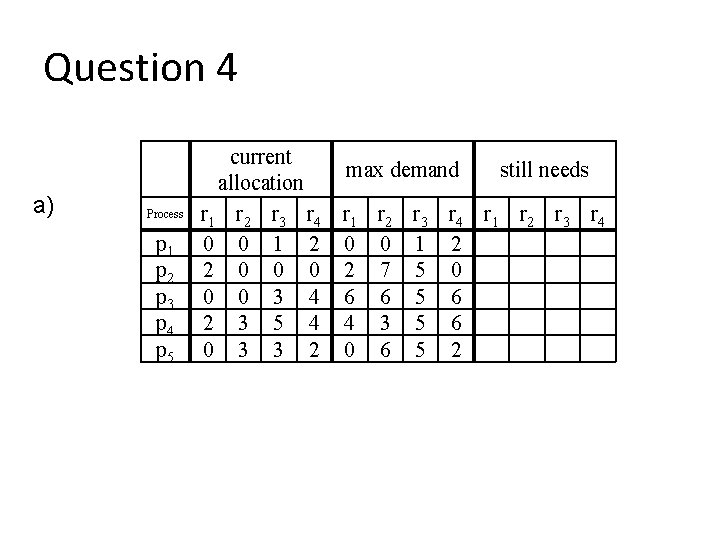
Question 4 a) Process p 1 p 2 p 3 p 4 p 5 current max demand still needs allocation r 1 r 2 r 3 r 4 0 0 1 2 2 0 0 0 2 7 5 0 0 0 3 4 6 6 5 6 2 3 5 4 4 3 5 6 0 3 3 2 0 6 5 2
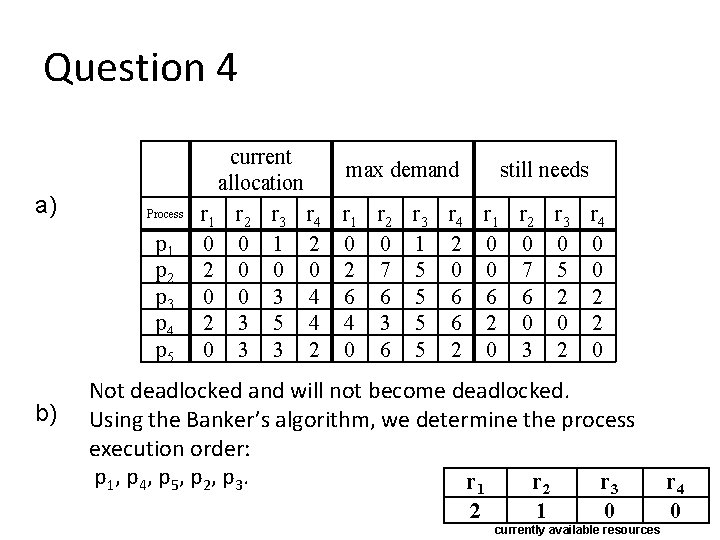
Question 4 a) Process p 1 p 2 p 3 p 4 p 5 b) current max demand still needs allocation r 1 r 2 r 3 r 4 0 0 1 2 0 0 0 2 7 5 0 0 0 3 4 6 6 5 6 6 6 2 2 2 3 5 4 4 3 5 6 2 0 0 2 0 3 3 2 0 6 5 2 0 3 2 0 Not deadlocked and will not become deadlocked. Using the Banker’s algorithm, we determine the process execution order: p 1, p 4, p 5, p 2, p 3. r 1 r 2 r 3 2 1 0 currently available resources r 4 0
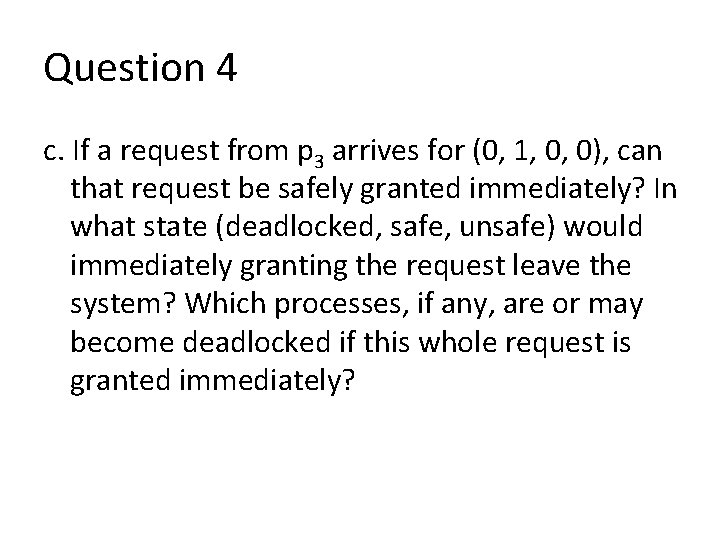
Question 4 c. If a request from p 3 arrives for (0, 1, 0, 0), can that request be safely granted immediately? In what state (deadlocked, safe, unsafe) would immediately granting the request leave the system? Which processes, if any, are or may become deadlocked if this whole request is granted immediately?
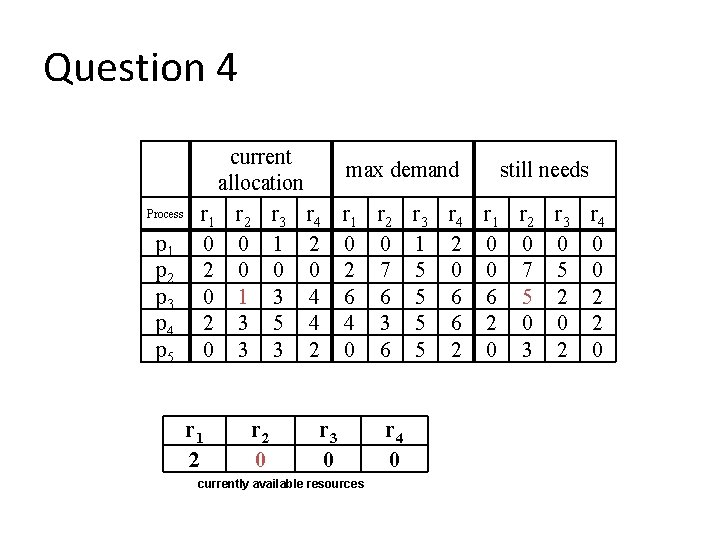
Question 4 Process p 1 p 2 p 3 p 4 p 5 current max demand still needs allocation r 1 r 2 r 3 r 4 0 0 1 2 0 0 0 2 7 5 0 0 1 3 4 6 6 5 2 2 2 3 5 4 4 3 5 6 2 0 0 2 0 3 3 2 0 6 5 2 0 3 2 0 r 1 2 r 2 0 r 3 0 currently available resources r 4 0
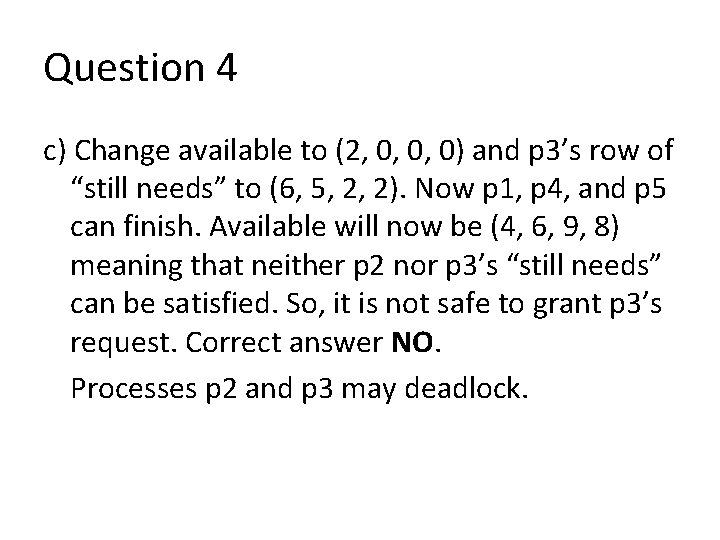
Question 4 c) Change available to (2, 0, 0, 0) and p 3’s row of “still needs” to (6, 5, 2, 2). Now p 1, p 4, and p 5 can finish. Available will now be (4, 6, 9, 8) meaning that neither p 2 nor p 3’s “still needs” can be satisfied. So, it is not safe to grant p 3’s request. Correct answer NO. Processes p 2 and p 3 may deadlock.
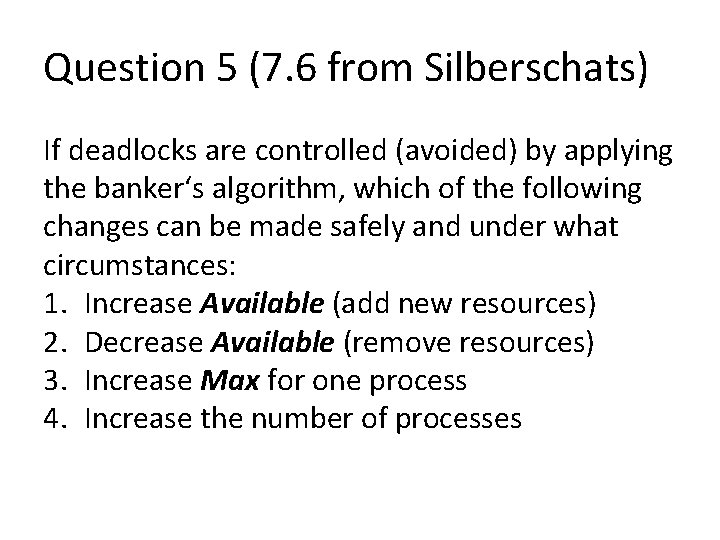
Question 5 (7. 6 from Silberschats) If deadlocks are controlled (avoided) by applying the banker‘s algorithm, which of the following changes can be made safely and under what circumstances: 1. Increase Available (add new resources) 2. Decrease Available (remove resources) 3. Increase Max for one process 4. Increase the number of processes
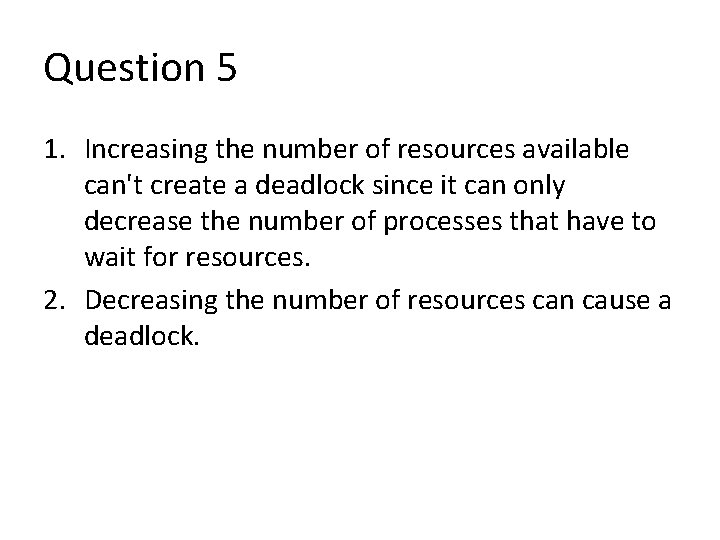
Question 5 1. Increasing the number of resources available can't create a deadlock since it can only decrease the number of processes that have to wait for resources. 2. Decreasing the number of resources can cause a deadlock.
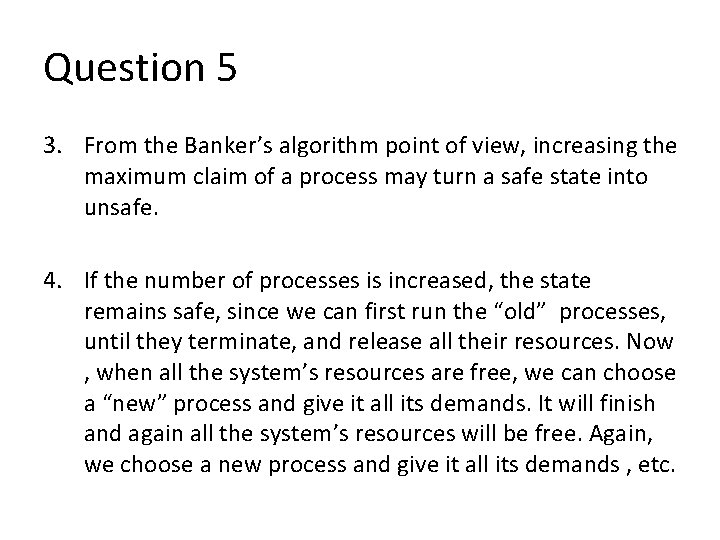
Question 5 3. From the Banker’s algorithm point of view, increasing the maximum claim of a process may turn a safe state into unsafe. 4. If the number of processes is increased, the state remains safe, since we can first run the “old” processes, until they terminate, and release all their resources. Now , when all the system’s resources are free, we can choose a “new” process and give it all its demands. It will finish and again all the system’s resources will be free. Again, we choose a new process and give it all its demands , etc.
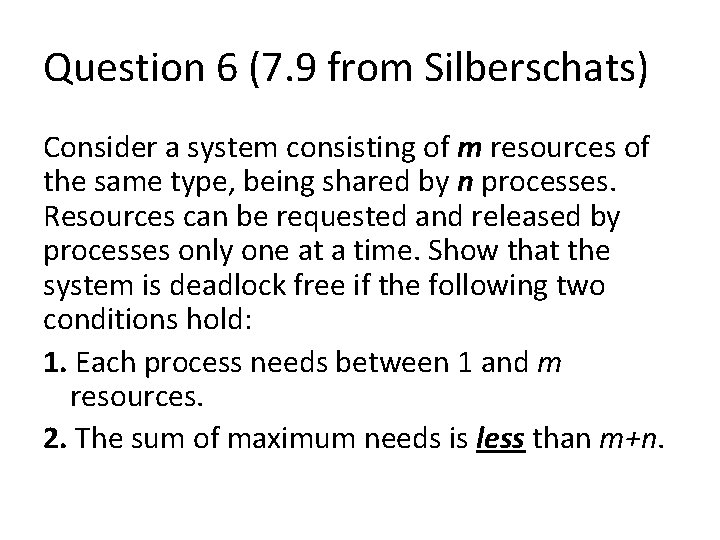
Question 6 (7. 9 from Silberschats) Consider a system consisting of m resources of the same type, being shared by n processes. Resources can be requested and released by processes only one at a time. Show that the system is deadlock free if the following two conditions hold: 1. Each process needs between 1 and m resources. 2. The sum of maximum needs is less than m+n.
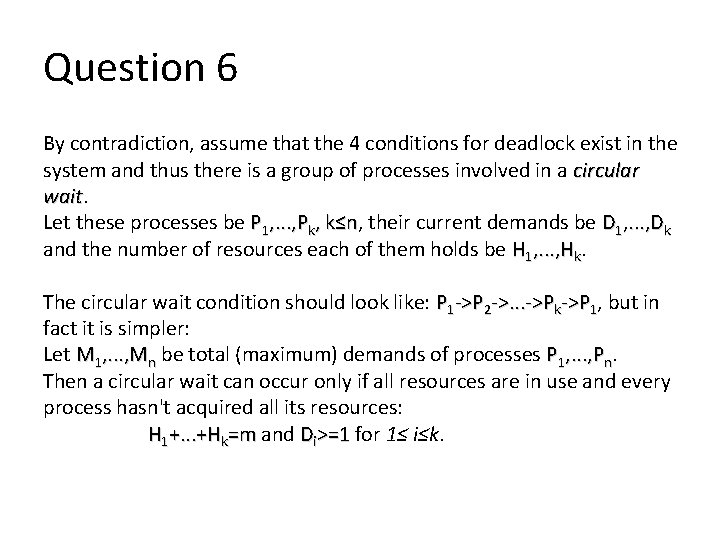
Question 6 By contradiction, assume that the 4 conditions for deadlock exist in the system and thus there is a group of processes involved in a circular wait Let these processes be P 1, . . . , Pk, k≤n, their current demands be D 1, . . . , Dk , k≤n and the number of resources each of them holds be H 1, . . . , Hk. The circular wait condition should look like: P 1 ->P 2 ->. . . ->Pk->P 1, but in fact it is simpler: Let M 1, . . . , Mn be total (maximum) demands of processes P 1, . . . , Pn. Then a circular wait can occur only if all resources are in use and every process hasn't acquired all its resources: H 1+. . . +Hk=m and Di>=1 for 1≤ i≤k. =m >=1
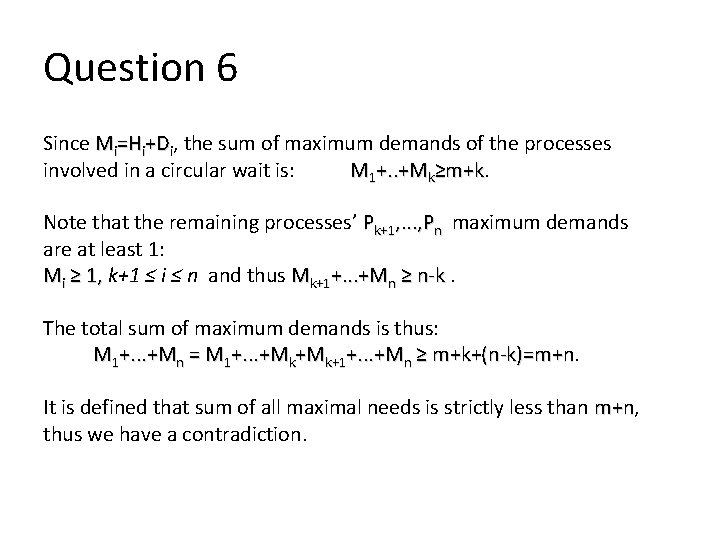
Question 6 Since Mi=Hi+Di, the sum of maximum demands of the processes involved in a circular wait is: M 1+. . +Mk≥m+k. ≥m+k Note that the remaining processes’ Pk+1, . . . , Pn maximum demands are at least 1: Mi ≥ 1, k+1 ≤ i ≤ n and thus Mk+1+. . . +Mn ≥ n-k. ≥ 1, ≥ n-k The total sum of maximum demands is thus: M 1+. . . +Mn = M 1+. . . +Mk+Mk+1+. . . +Mn ≥ m+k+(n-k)=m+n It is defined that sum of all maximal needs is strictly less than m+n, m+n thus we have a contradiction.
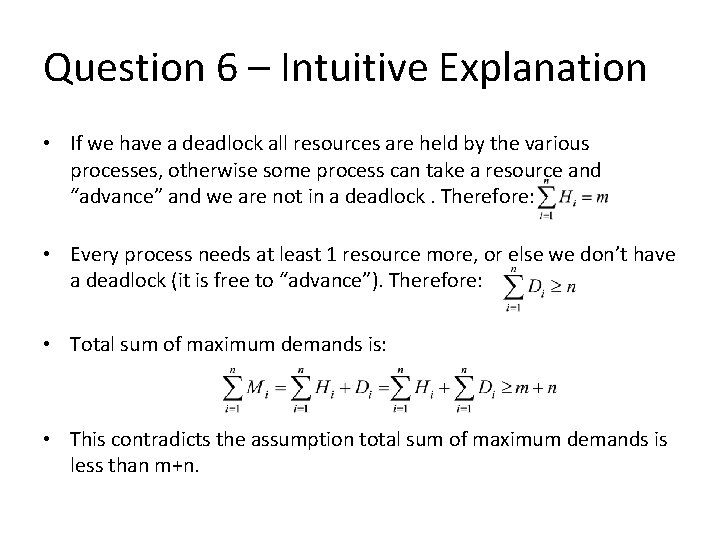
Question 6 – Intuitive Explanation • If we have a deadlock all resources are held by the various processes, otherwise some process can take a resource and “advance” and we are not in a deadlock. Therefore: • Every process needs at least 1 resource more, or else we don’t have a deadlock (it is free to “advance”). Therefore: • Total sum of maximum demands is: • This contradicts the assumption total sum of maximum demands is less than m+n.