OCR GCSE Computer Science Teaching and Learning Resources
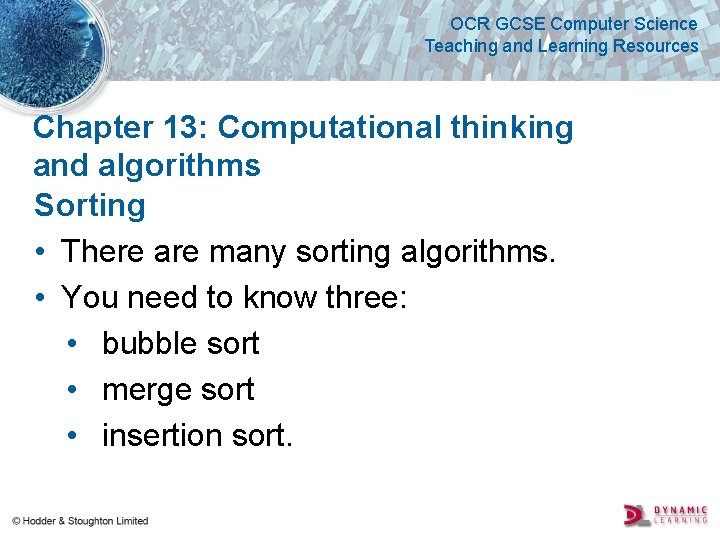
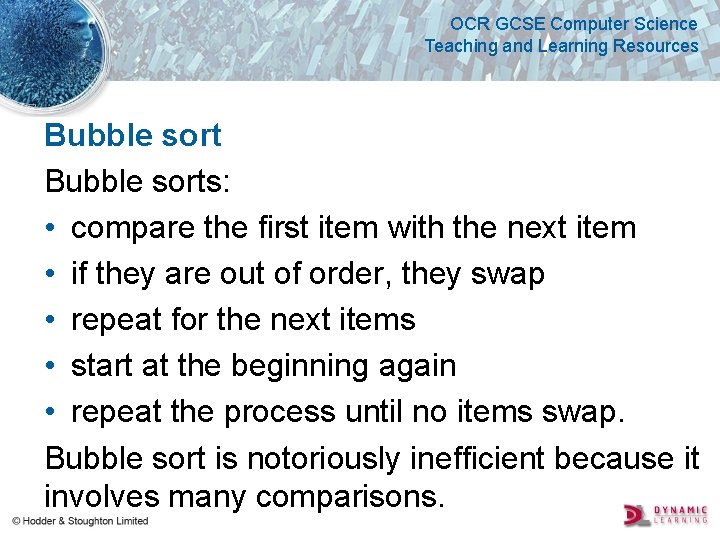
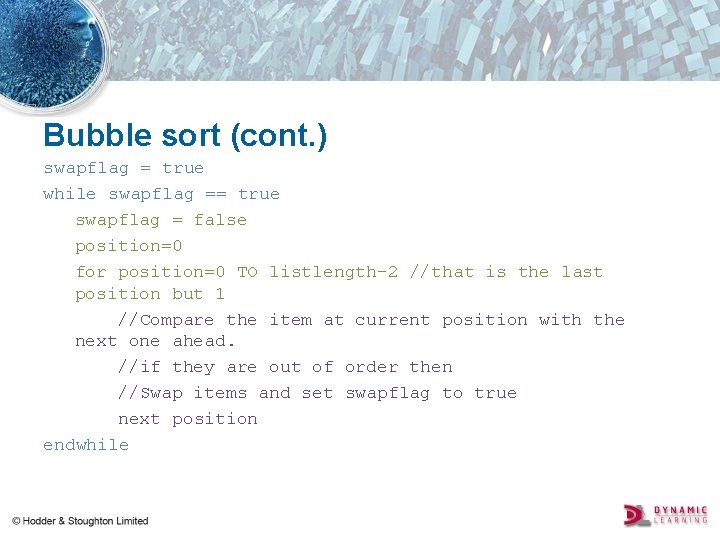
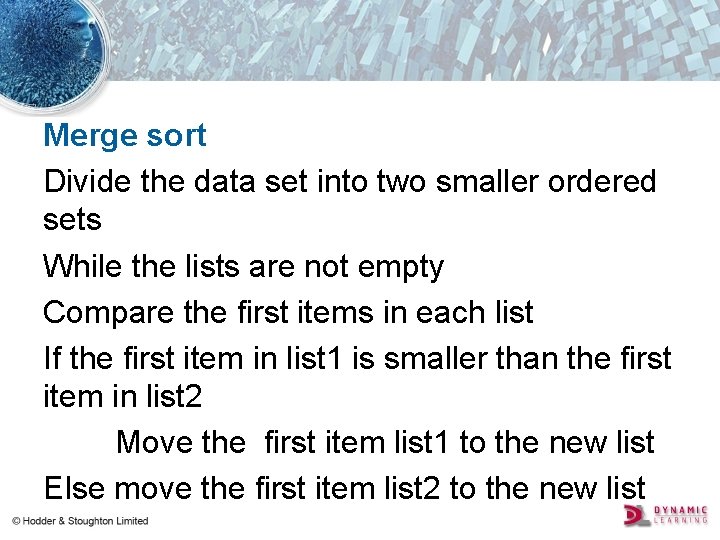
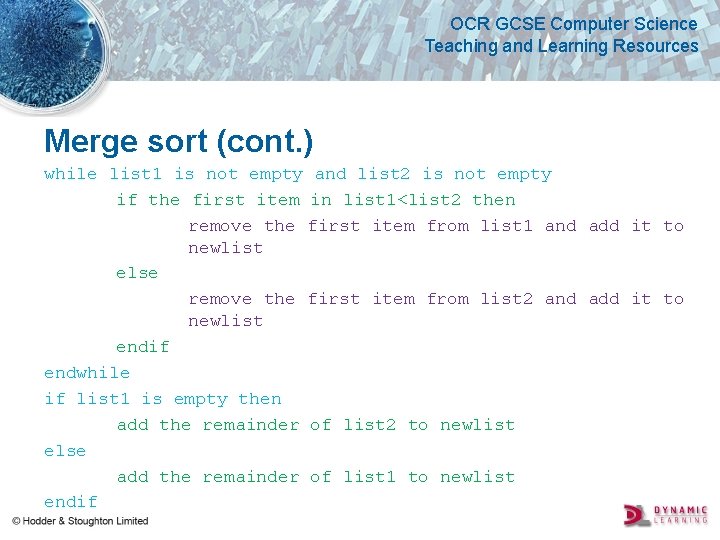
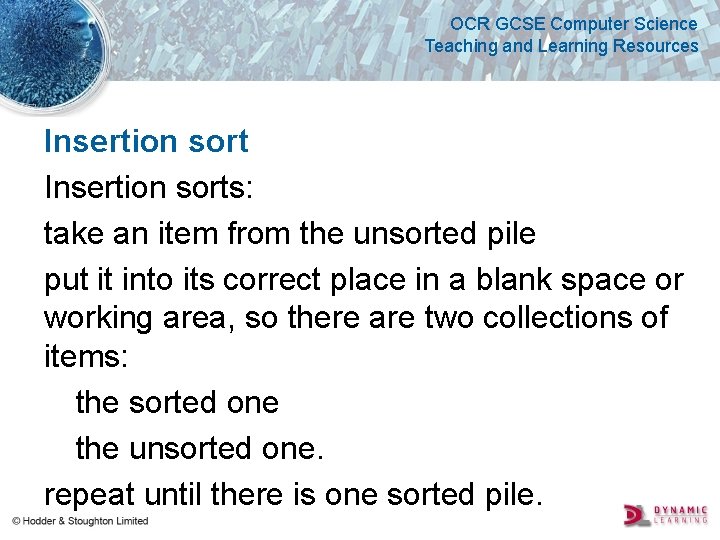
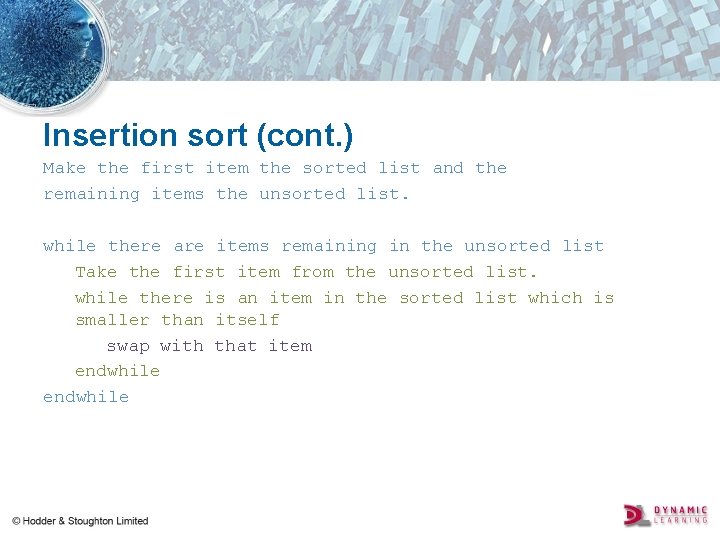
- Slides: 7
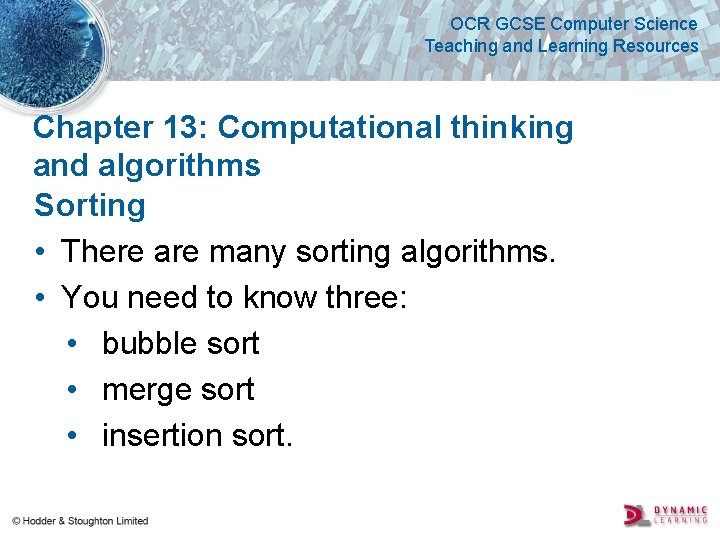
OCR GCSE Computer Science Teaching and Learning Resources Chapter 13: Computational thinking and algorithms Sorting • There are many sorting algorithms. • You need to know three: • bubble sort • merge sort • insertion sort.
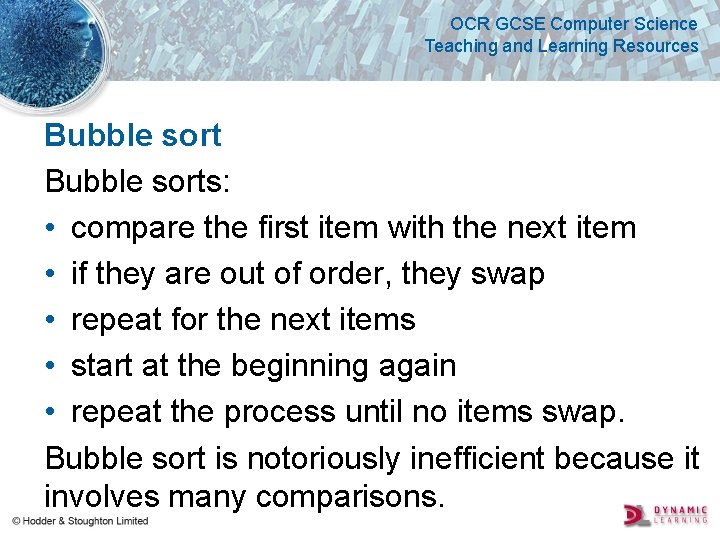
OCR GCSE Computer Science Teaching and Learning Resources Bubble sorts: • compare the first item with the next item • if they are out of order, they swap • repeat for the next items • start at the beginning again • repeat the process until no items swap. Bubble sort is notoriously inefficient because it involves many comparisons.
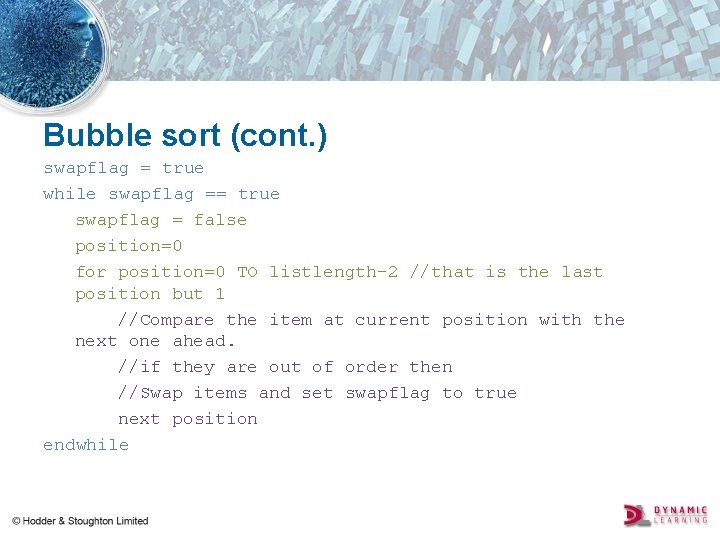
Bubble sort (cont. ) swapflag = true while swapflag == true swapflag = false position=0 for position=0 TO listlength− 2 //that is the last position but 1 //Compare the item at current position with the next one ahead. //if they are out of order then //Swap items and set swapflag to true next position endwhile
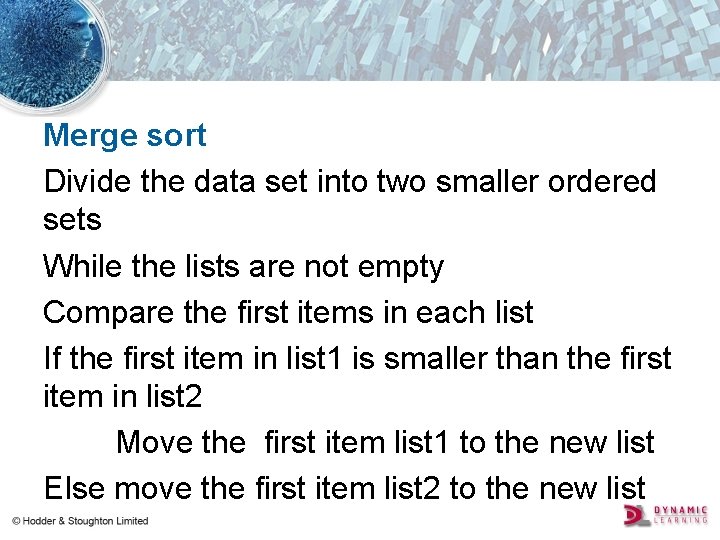
Merge sort Divide the data set into two smaller ordered sets While the lists are not empty Compare the first items in each list If the first item in list 1 is smaller than the first item in list 2 Move the first item list 1 to the new list Else move the first item list 2 to the new list
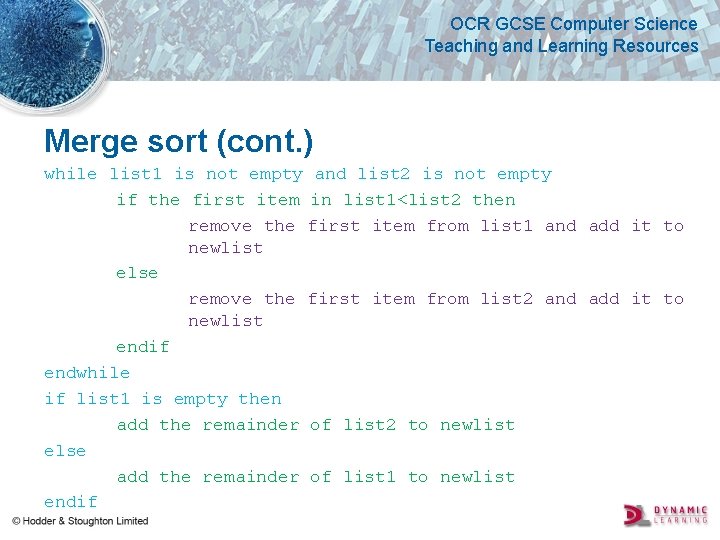
OCR GCSE Computer Science Teaching and Learning Resources Merge sort (cont. ) while list 1 is not empty and list 2 is not empty if the first item in list 1<list 2 then remove the first item from list 1 and add it to newlist else remove the first item from list 2 and add it to newlist endif endwhile if list 1 is empty then add the remainder of list 2 to newlist else add the remainder of list 1 to newlist endif
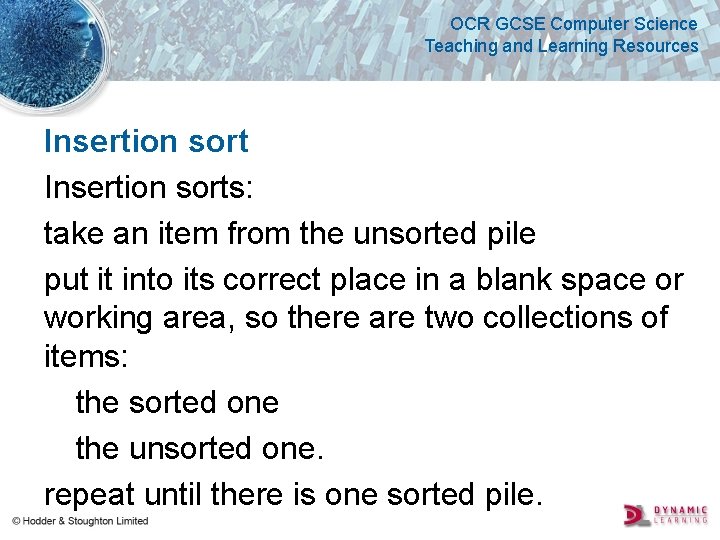
OCR GCSE Computer Science Teaching and Learning Resources Insertion sorts: take an item from the unsorted pile put it into its correct place in a blank space or working area, so there are two collections of items: the sorted one the unsorted one. repeat until there is one sorted pile.
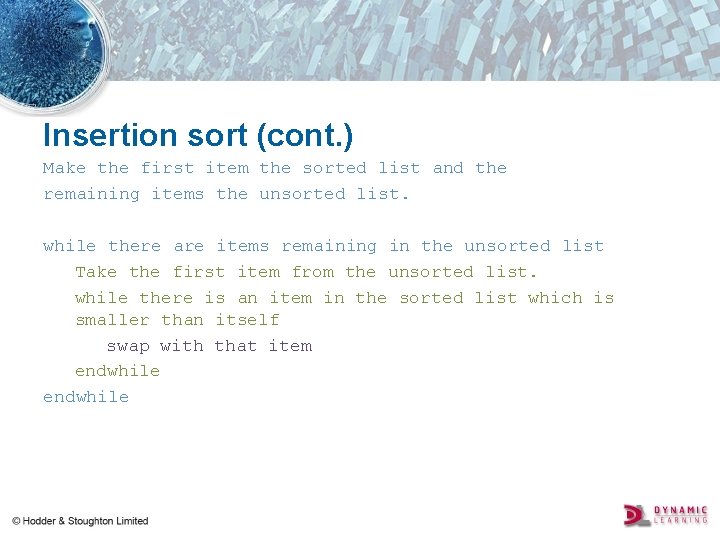
Insertion sort (cont. ) Make the first item the sorted list and the remaining items the unsorted list. while there are items remaining in the unsorted list Take the first item from the unsorted list. while there is an item in the sorted list which is smaller than itself swap with that item endwhile