Objects Strings String x abc String is a
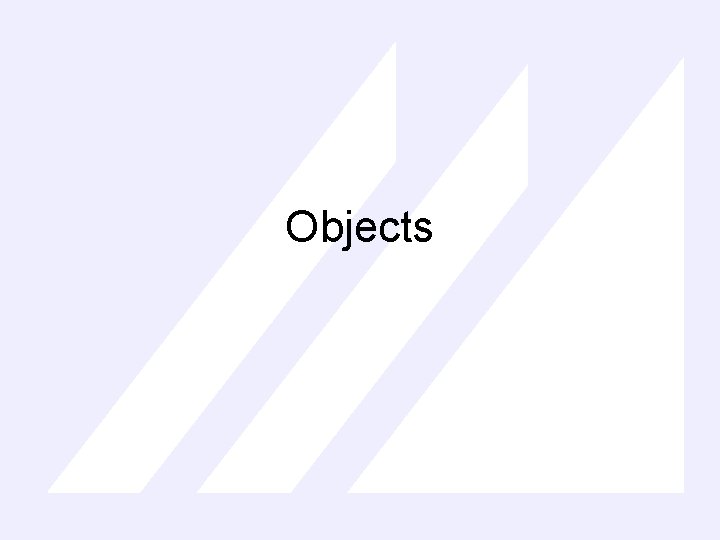
Objects
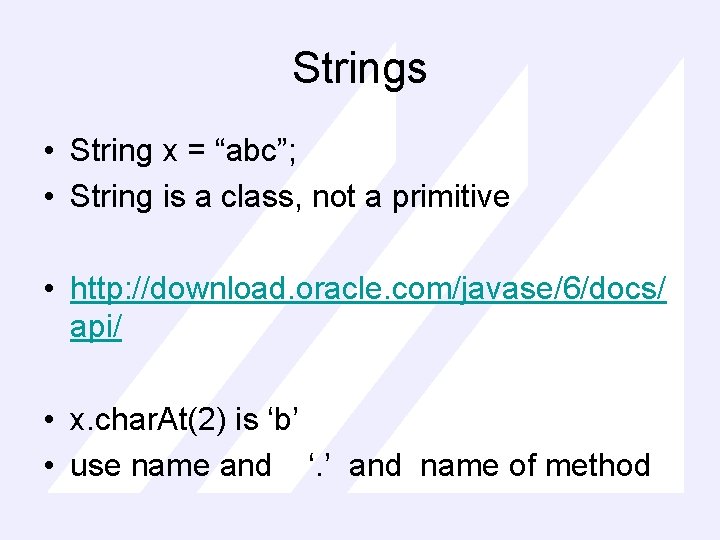
Strings • String x = “abc”; • String is a class, not a primitive • http: //download. oracle. com/javase/6/docs/ api/ • x. char. At(2) is ‘b’ • use name and ‘. ’ and name of method
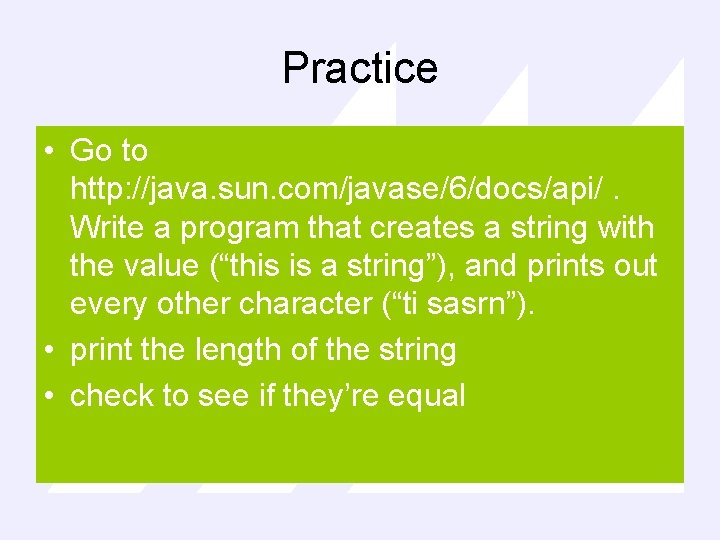
Practice • Go to http: //java. sun. com/javase/6/docs/api/. Write a program that creates a string with the value (“this is a string”), and prints out every other character (“ti sasrn”). • print the length of the string • check to see if they’re equal
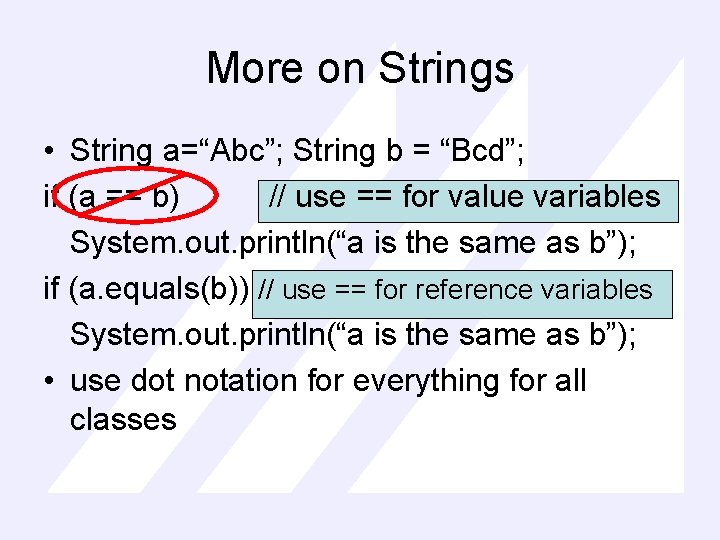
More on Strings • String a=“Abc”; String b = “Bcd”; if (a == b) // use == for value variables System. out. println(“a is the same as b”); if (a. equals(b)) // use == for reference variables System. out. println(“a is the same as b”); • use dot notation for everything for all classes
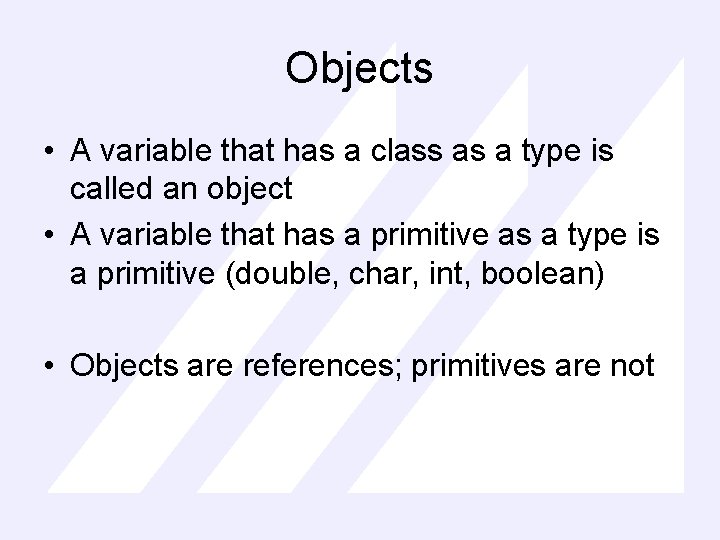
Objects • A variable that has a class as a type is called an object • A variable that has a primitive as a type is a primitive (double, char, int, boolean) • Objects are references; primitives are not
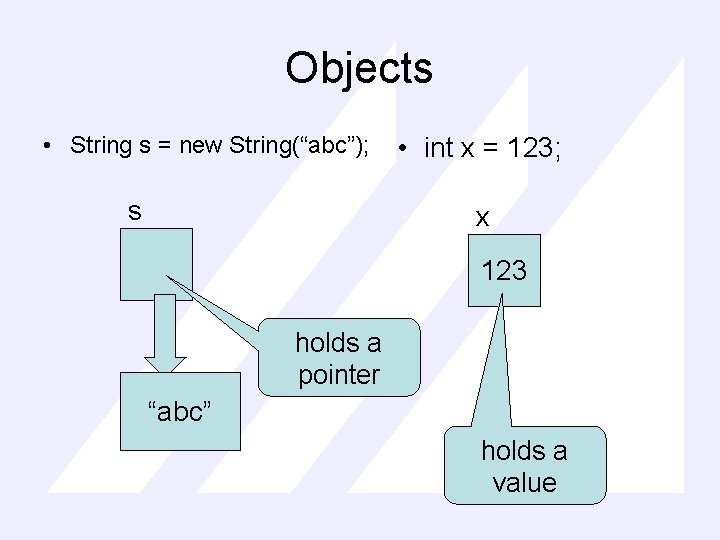
Objects • String s = new String(“abc”); s • int x = 123; x 123 holds a pointer “abc” holds a value
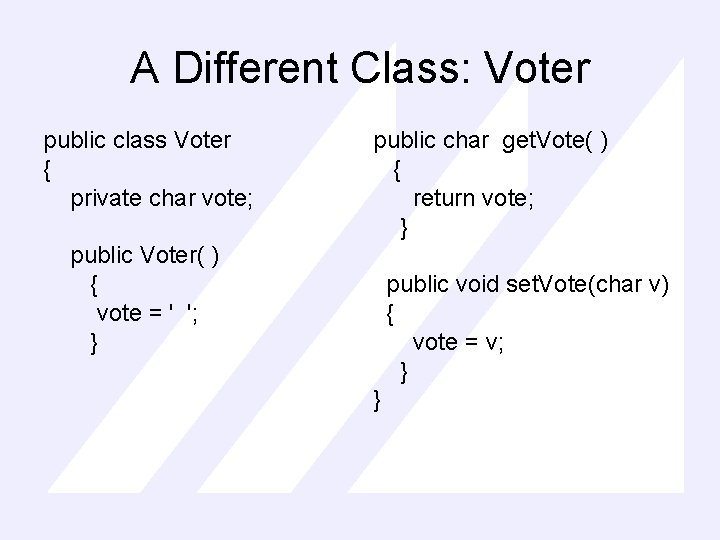
A Different Class: Voter public class Voter { private char vote; public char get. Vote( ) { return vote; } public Voter( ) { vote = ' '; } public void set. Vote(char v) { vote = v; } }
![public static void main(String args[]) { Voter v 1 = new Voter( ); Voter public static void main(String args[]) { Voter v 1 = new Voter( ); Voter](http://slidetodoc.com/presentation_image_h/a753b4d55533551f0c8d1596f983d881/image-8.jpg)
public static void main(String args[]) { Voter v 1 = new Voter( ); Voter v 2 = new Voter( ); Voter v 3 = new Voter( ); v 1. set. Vote(‘r’); v 2. set. Vote(‘o’); v 3. set. Vote(‘d’); if (v 2. get. Vote( ) == ‘r’) System. out. println(“V 2 is voting Republican”); } }
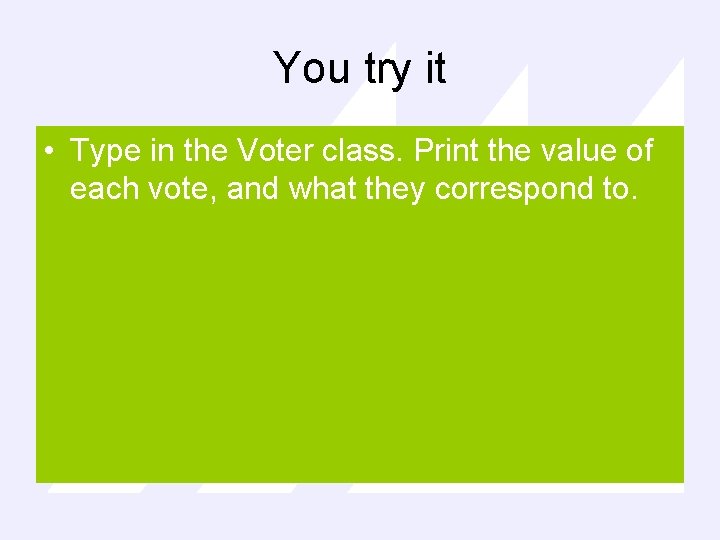
You try it • Type in the Voter class. Print the value of each vote, and what they correspond to.
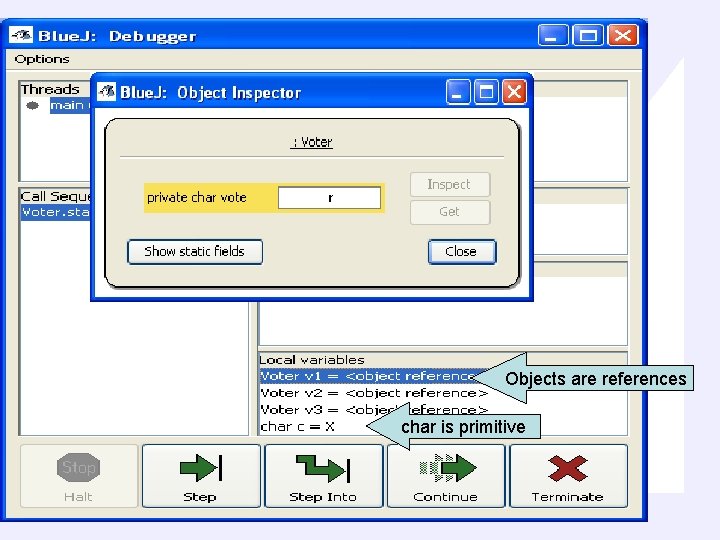
Objects are references char is primitive
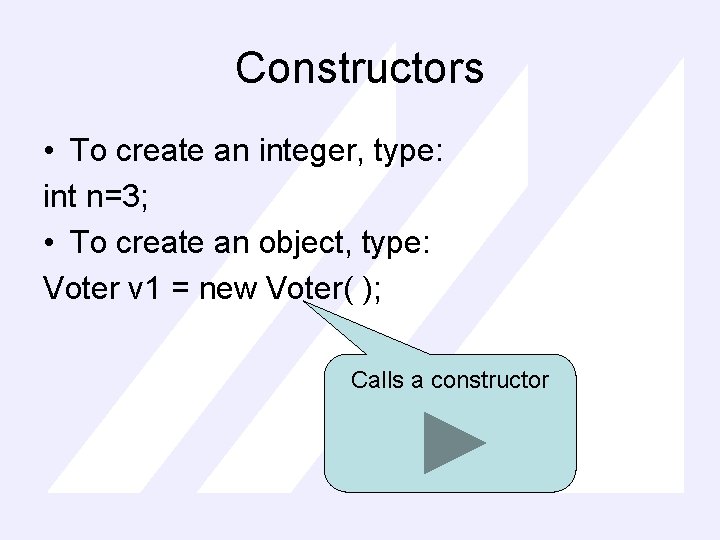
Constructors • To create an integer, type: int n=3; • To create an object, type: Voter v 1 = new Voter( ); Calls a constructor
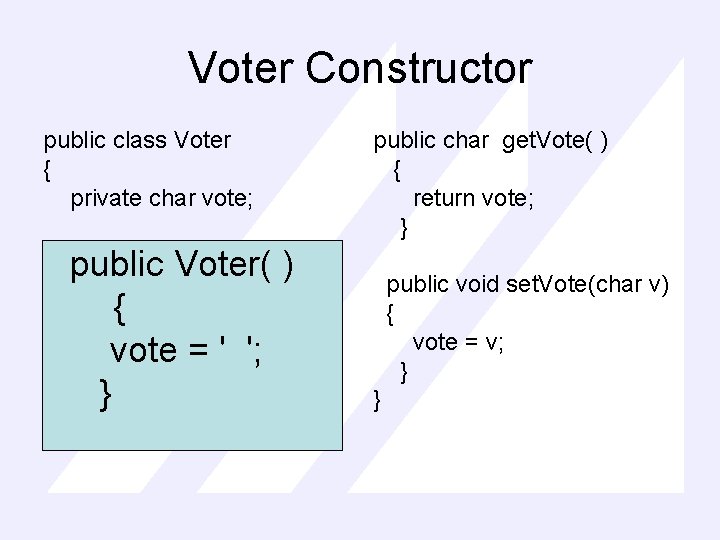
Voter Constructor public class Voter { private char vote; public Voter( ) { vote = ' '; } public char get. Vote( ) { return vote; } public void set. Vote(char v) { vote = v; } }
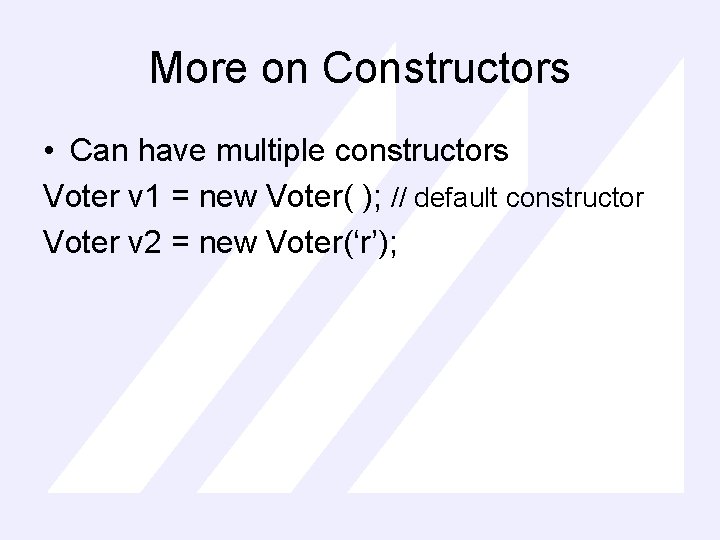
More on Constructors • Can have multiple constructors Voter v 1 = new Voter( ); // default constructor Voter v 2 = new Voter(‘r’);
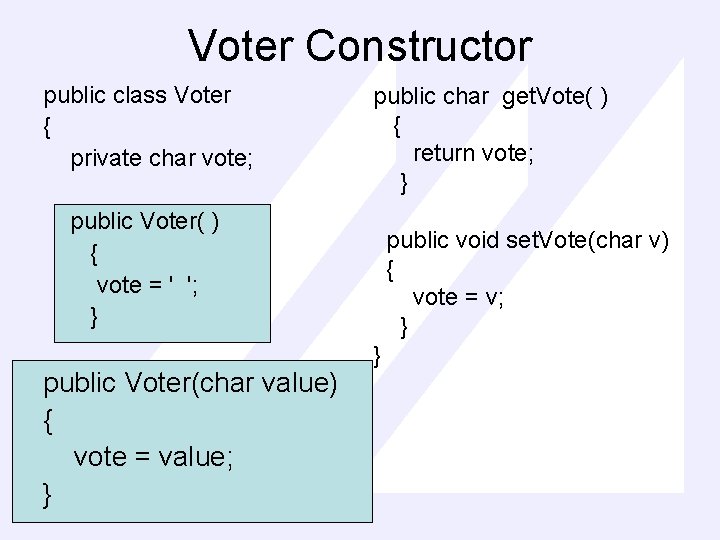
Voter Constructor public class Voter { private char vote; public char get. Vote( ) { return vote; } public Voter( ) { vote = ' '; } public Voter(char value) { vote = value; } public void set. Vote(char v) { vote = v; } }
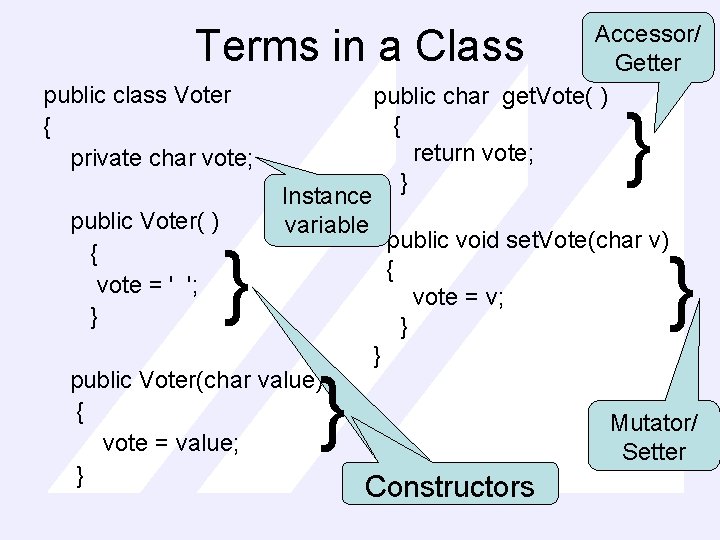
Accessor/ Getter public char get. Vote( ) { return vote; } Terms in a Class public class Voter { private char vote; public Voter( ) { vote = ' '; } } Instance variable } public void set. Vote(char v) { vote = v; } } } public Voter(char value) { vote = value; } } Mutator/ Setter Constructors
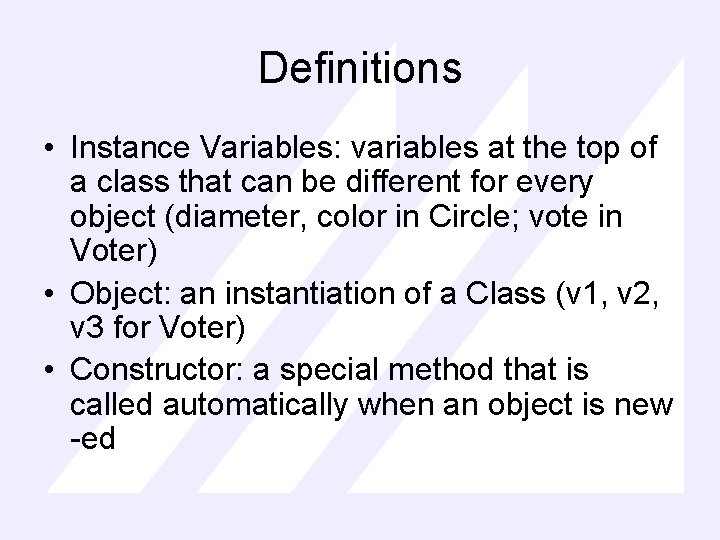
Definitions • Instance Variables: variables at the top of a class that can be different for every object (diameter, color in Circle; vote in Voter) • Object: an instantiation of a Class (v 1, v 2, v 3 for Voter) • Constructor: a special method that is called automatically when an object is new -ed
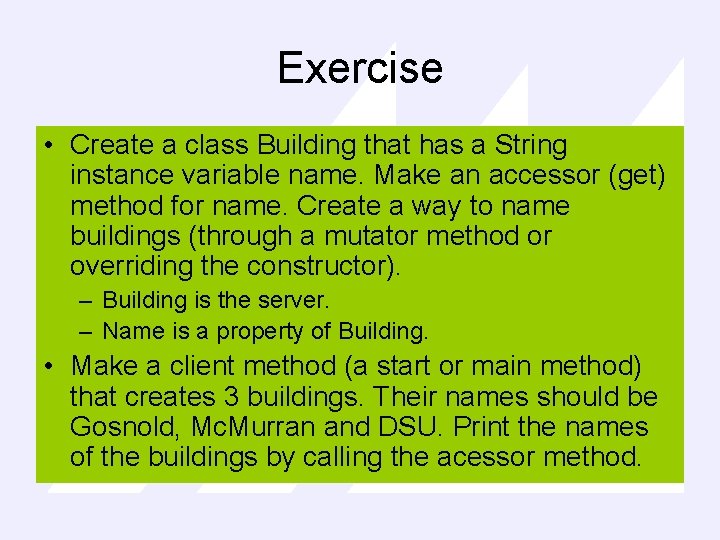
Exercise • Create a class Building that has a String instance variable name. Make an accessor (get) method for name. Create a way to name buildings (through a mutator method or overriding the constructor). – Building is the server. – Name is a property of Building. • Make a client method (a start or main method) that creates 3 buildings. Their names should be Gosnold, Mc. Murran and DSU. Print the names of the buildings by calling the acessor method.
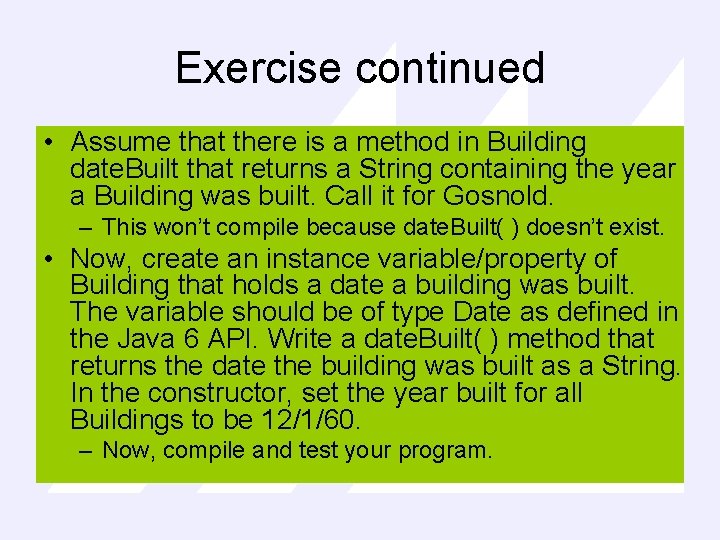
Exercise continued • Assume that there is a method in Building date. Built that returns a String containing the year a Building was built. Call it for Gosnold. – This won’t compile because date. Built( ) doesn’t exist. • Now, create an instance variable/property of Building that holds a date a building was built. The variable should be of type Date as defined in the Java 6 API. Write a date. Built( ) method that returns the date the building was built as a String. In the constructor, set the year built for all Buildings to be 12/1/60. – Now, compile and test your program.
- Slides: 18