Object Oriented Programming Lecture 4 Flow Control Mustafa
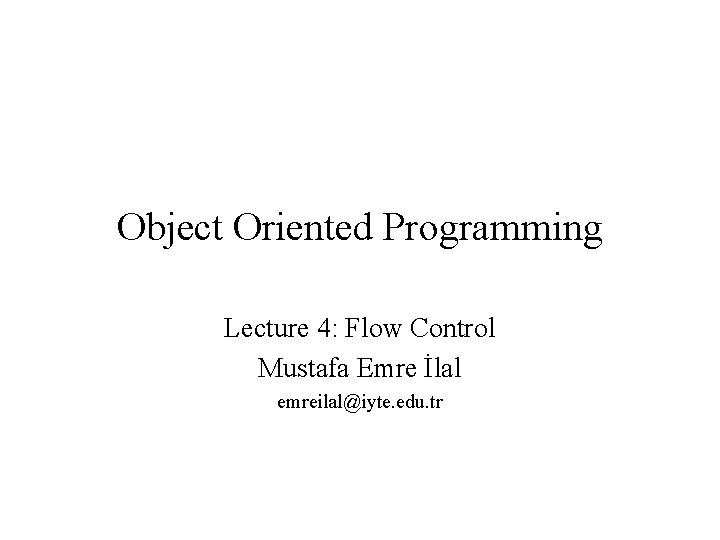
Object Oriented Programming Lecture 4: Flow Control Mustafa Emre İlal emreilal@iyte. edu. tr
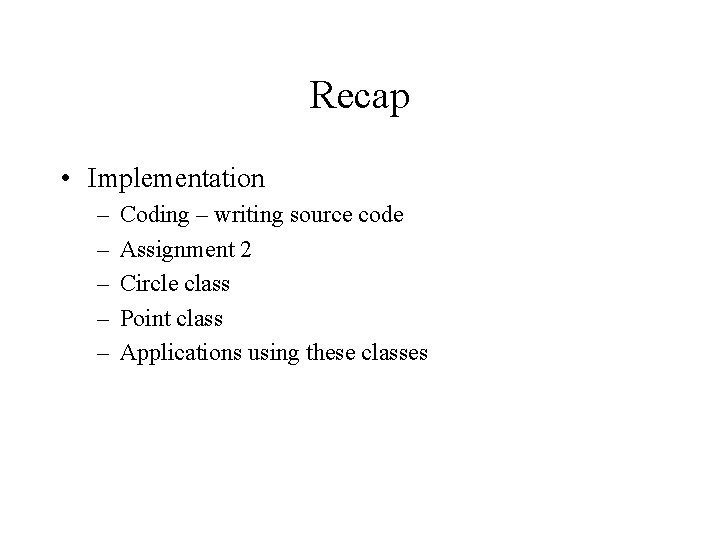
Recap • Implementation – – – Coding – writing source code Assignment 2 Circle class Point class Applications using these classes
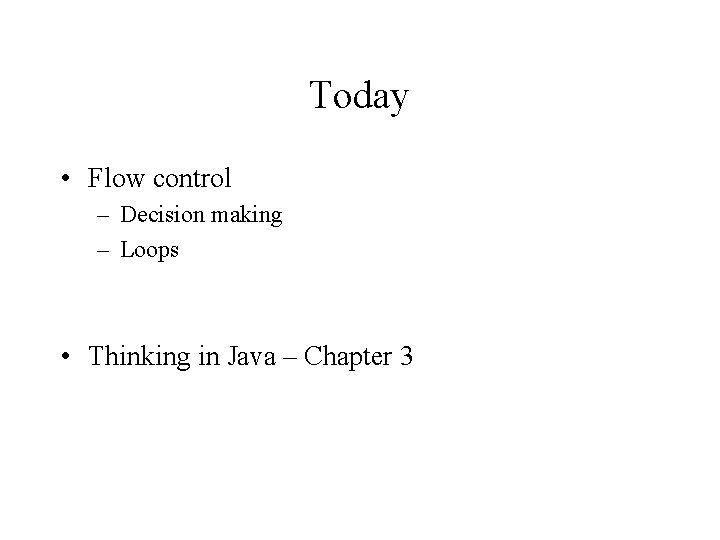
Today • Flow control – Decision making – Loops • Thinking in Java – Chapter 3
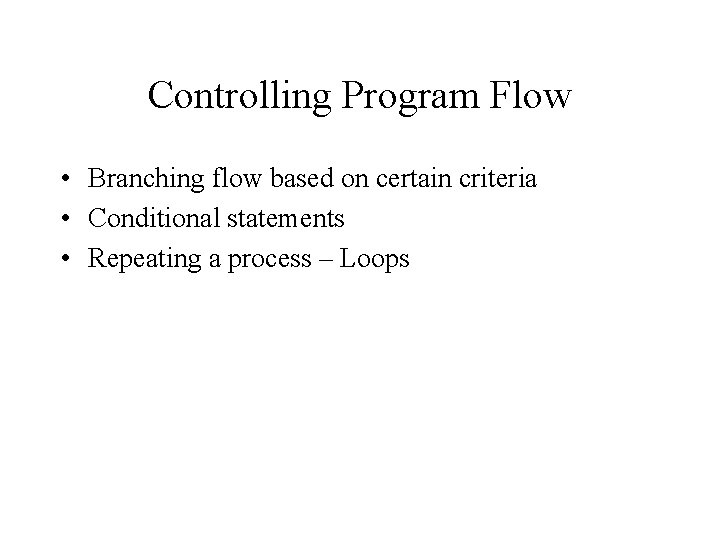
Controlling Program Flow • Branching flow based on certain criteria • Conditional statements • Repeating a process – Loops
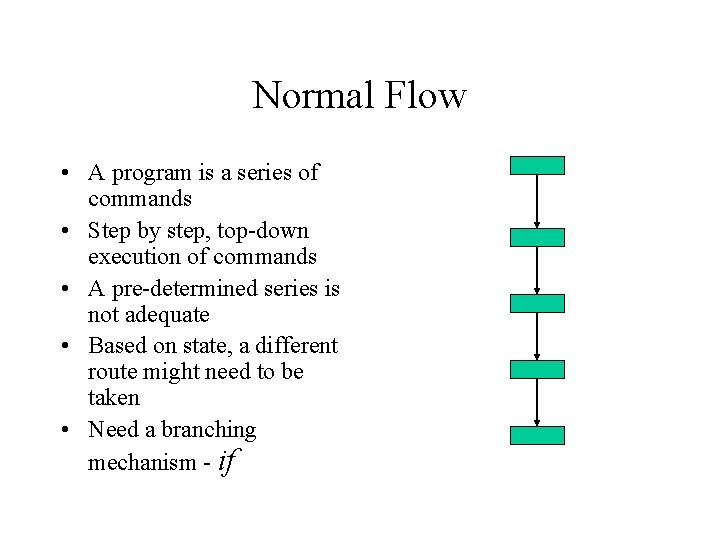
Normal Flow • A program is a series of commands • Step by step, top-down execution of commands • A pre-determined series is not adequate • Based on state, a different route might need to be taken • Need a branching mechanism - if
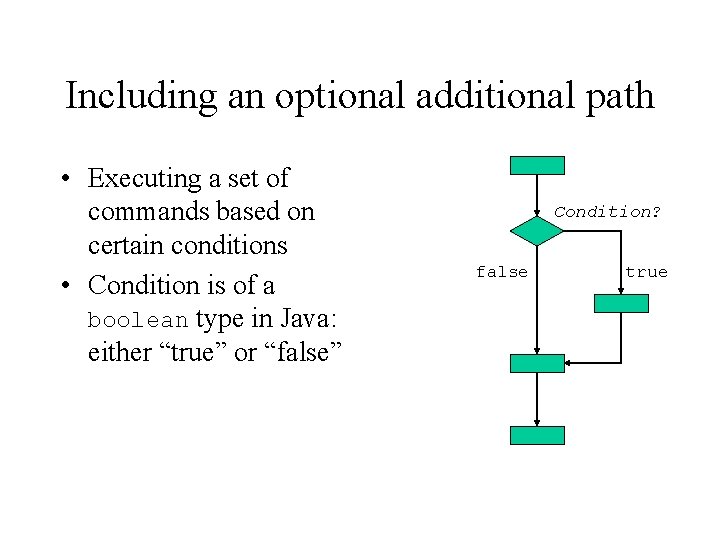
Including an optional additional path • Executing a set of commands based on certain conditions • Condition is of a boolean type in Java: either “true” or “false” Condition? false true
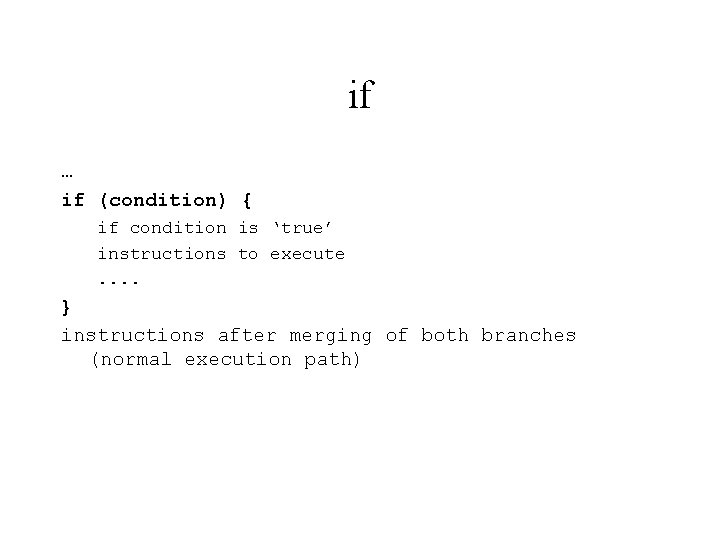
if … if (condition) { if condition is ‘true’ instructions to execute. . } instructions after merging of both branches (normal execution path)
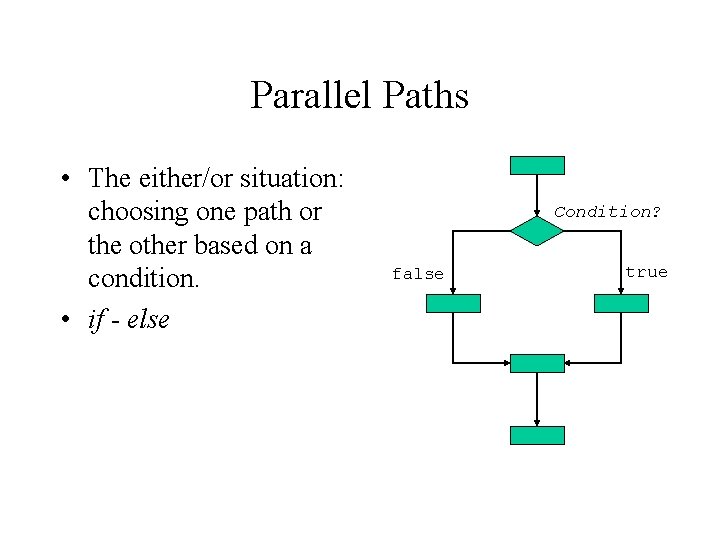
Parallel Paths • The either/or situation: choosing one path or the other based on a condition. • if - else Condition? false true
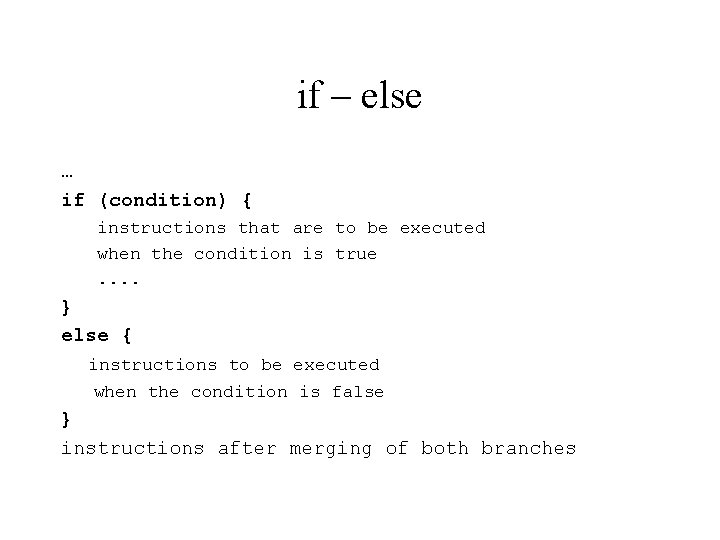
if – else … if (condition) { instructions that are to be executed when the condition is true. . } else { instructions to be executed when the condition is false } instructions after merging of both branches
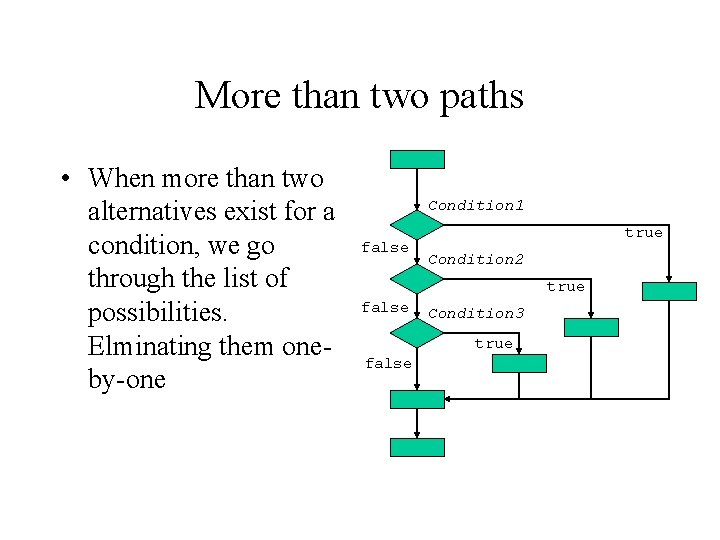
More than two paths • When more than two alternatives exist for a condition, we go through the list of possibilities. Elminating them oneby-one Condition 1 false true Condition 2 true false Condition 3 true false
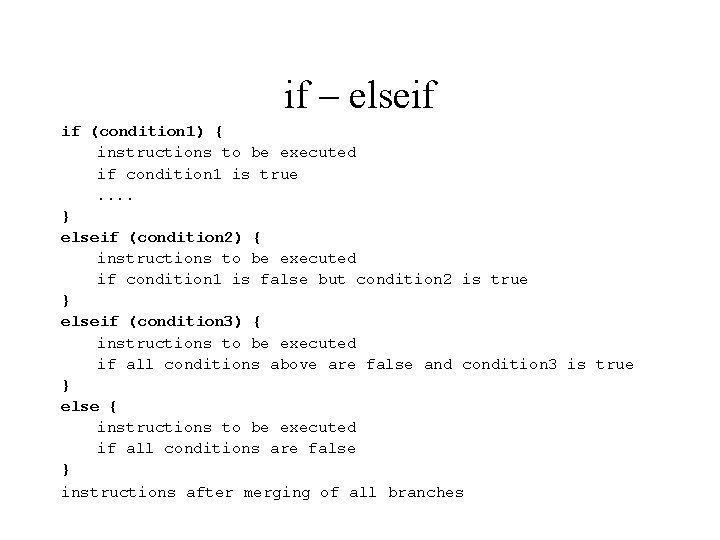
if – elseif if (condition 1) { instructions to be executed if condition 1 is true. . } elseif (condition 2) { instructions to be executed if condition 1 is false but condition 2 is true } elseif (condition 3) { instructions to be executed if all conditions above are false and condition 3 is true } else { instructions to be executed if all conditions are false } instructions after merging of all branches
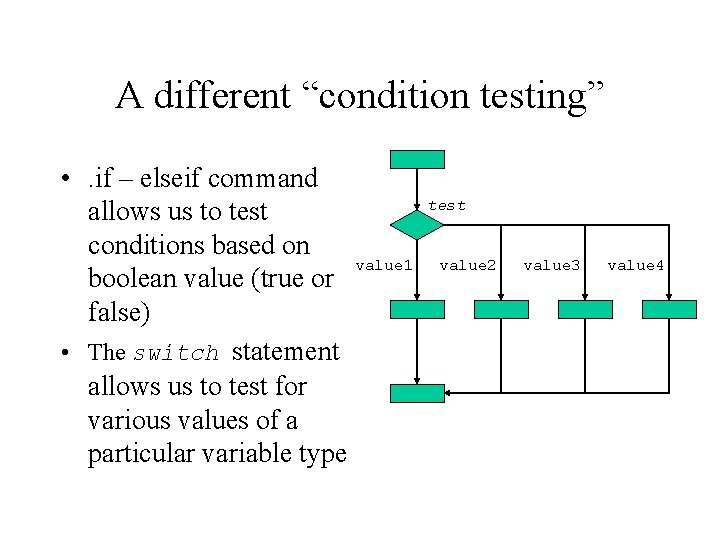
A different “condition testing” • . if – elseif command allows us to test conditions based on value 1 boolean value (true or false) • The switch statement allows us to test for various values of a particular variable type test value 2 value 3 value 4
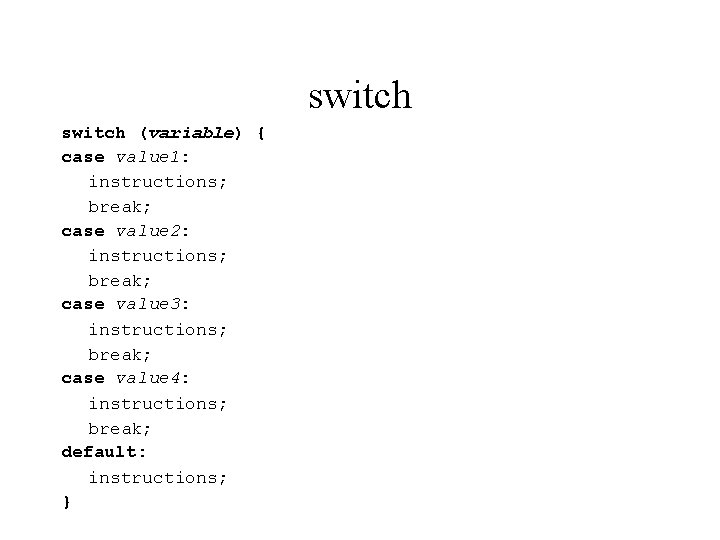
switch (variable) { case value 1: instructions; break; case value 2: instructions; break; case value 3: instructions; break; case value 4: instructions; break; default: instructions; }
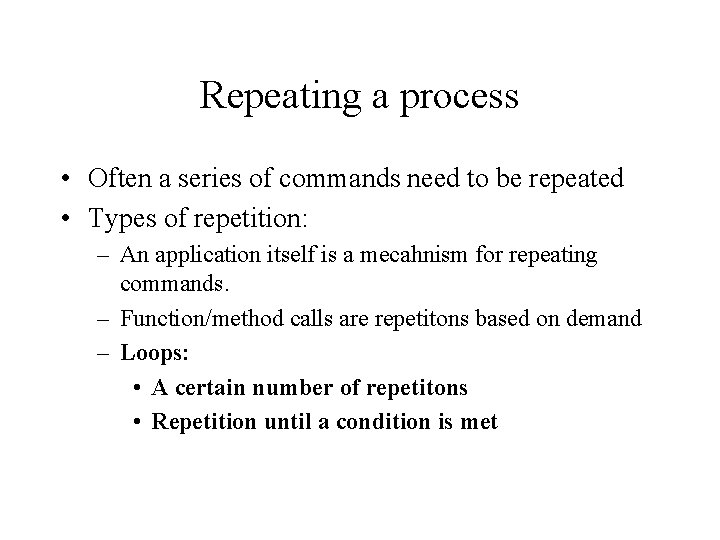
Repeating a process • Often a series of commands need to be repeated • Types of repetition: – An application itself is a mecahnism for repeating commands. – Function/method calls are repetitons based on demand – Loops: • A certain number of repetitons • Repetition until a condition is met
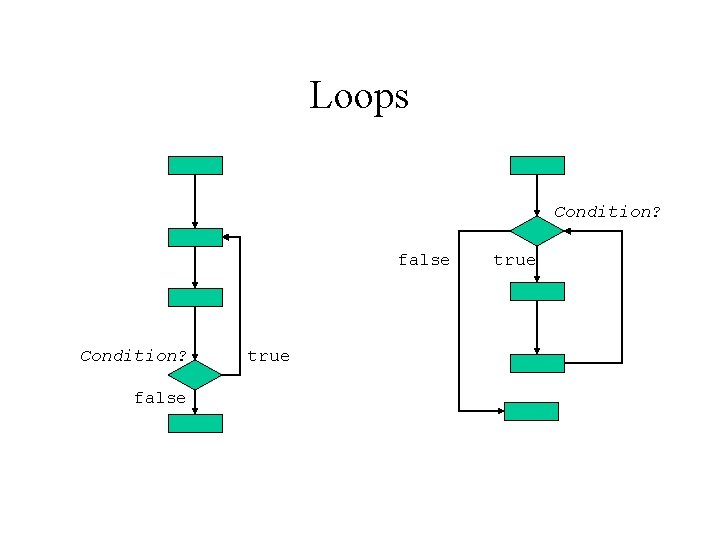
Loops Condition? false true
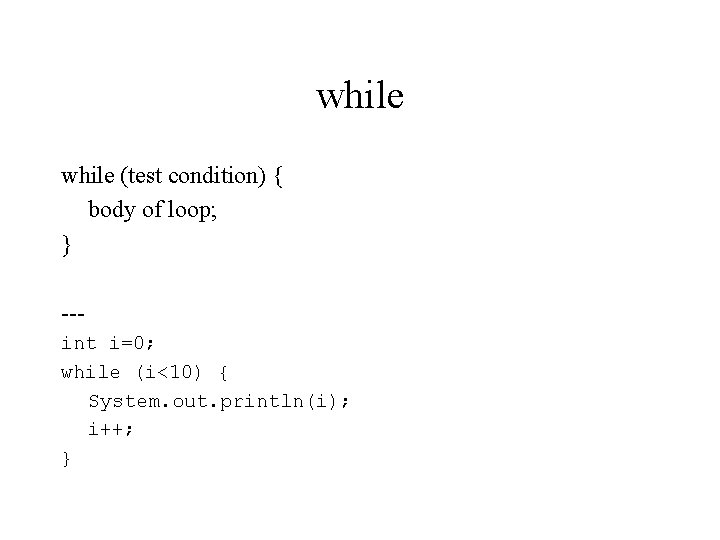
while (test condition) { body of loop; } --int i=0; while (i<10) { System. out. println(i); i++; }
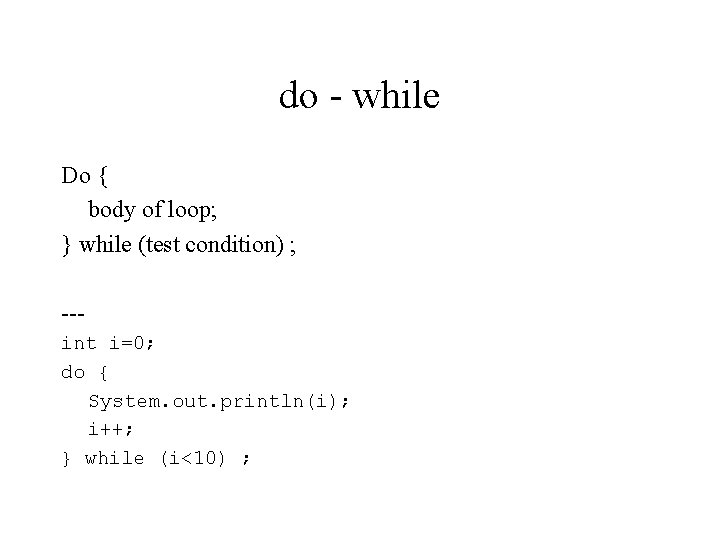
do - while Do { body of loop; } while (test condition) ; --int i=0; do { System. out. println(i); i++; } while (i<10) ;
![for (initialization[s]; test condition; counter update[s]) { body of loop; } ----for (int i=0; for (initialization[s]; test condition; counter update[s]) { body of loop; } ----for (int i=0;](http://slidetodoc.com/presentation_image/f8b033f54c9bcded3da159881aa694c8/image-18.jpg)
for (initialization[s]; test condition; counter update[s]) { body of loop; } ----for (int i=0; i<10; i++) { System. out. println(i); }
![enhanced for (initialization[s] : iterable collection or array) { body of loop; } ----for enhanced for (initialization[s] : iterable collection or array) { body of loop; } ----for](http://slidetodoc.com/presentation_image/f8b033f54c9bcded3da159881aa694c8/image-19.jpg)
enhanced for (initialization[s] : iterable collection or array) { body of loop; } ----for (int i : an_array[]) { System. out. println(i); }
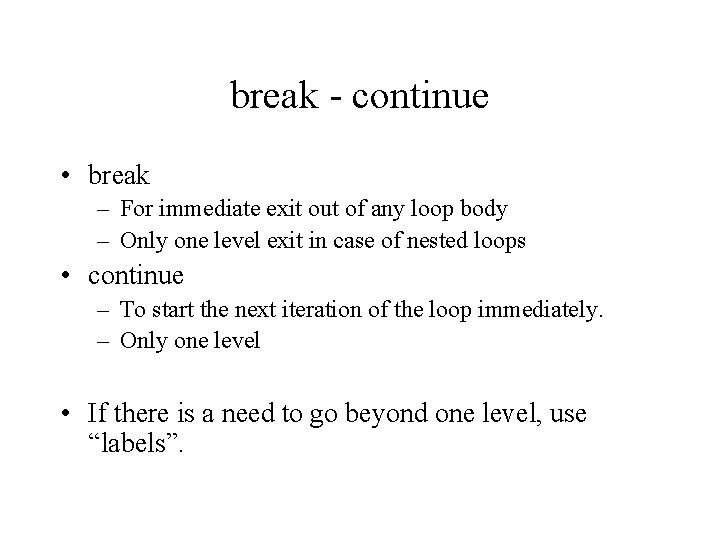
break - continue • break – For immediate exit out of any loop body – Only one level exit in case of nested loops • continue – To start the next iteration of the loop immediately. – Only one level • If there is a need to go beyond one level, use “labels”.
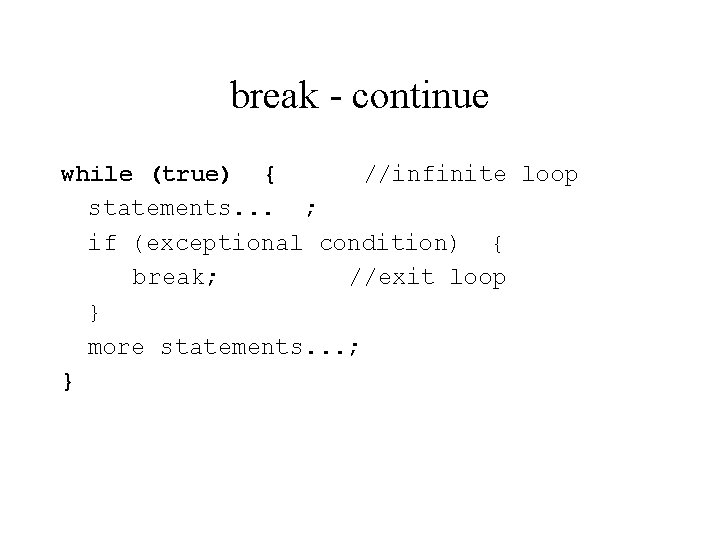
break - continue while (true) { //infinite loop statements. . . ; if (exceptional condition) { break; //exit loop } more statements. . . ; }
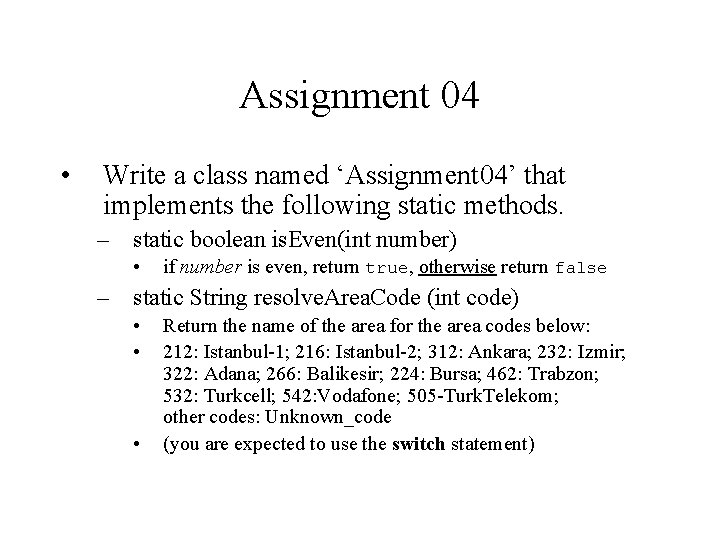
Assignment 04 • Write a class named ‘Assignment 04’ that implements the following static methods. – static boolean is. Even(int number) • if number is even, return true, otherwise return false – static String resolve. Area. Code (int code) • • • Return the name of the area for the area codes below: 212: Istanbul-1; 216: Istanbul-2; 312: Ankara; 232: Izmir; 322: Adana; 266: Balikesir; 224: Bursa; 462: Trabzon; 532: Turkcell; 542: Vodafone; 505 -Turk. Telekom; other codes: Unknown_code (you are expected to use the switch statement)
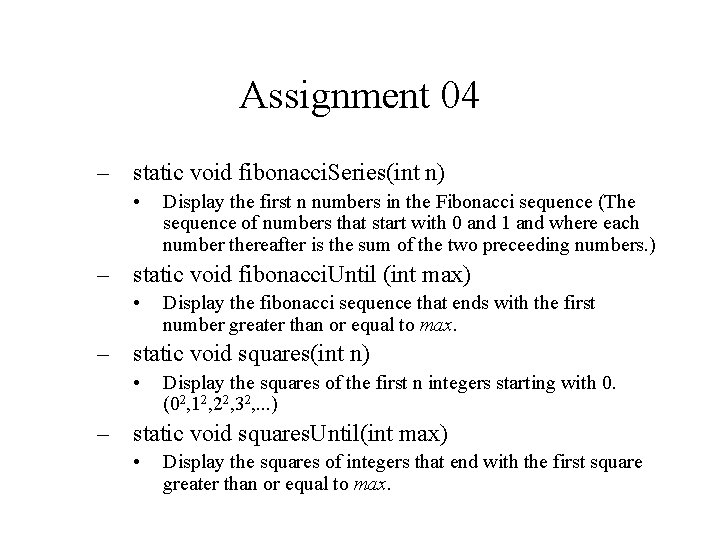
Assignment 04 – static void fibonacci. Series(int n) • Display the first n numbers in the Fibonacci sequence (The sequence of numbers that start with 0 and 1 and where each number thereafter is the sum of the two preceeding numbers. ) – static void fibonacci. Until (int max) • Display the fibonacci sequence that ends with the first number greater than or equal to max. – static void squares(int n) • Display the squares of the first n integers starting with 0. (02, 12, 22, 32, . . . ) – static void squares. Until(int max) • Display the squares of integers that end with the first square greater than or equal to max.
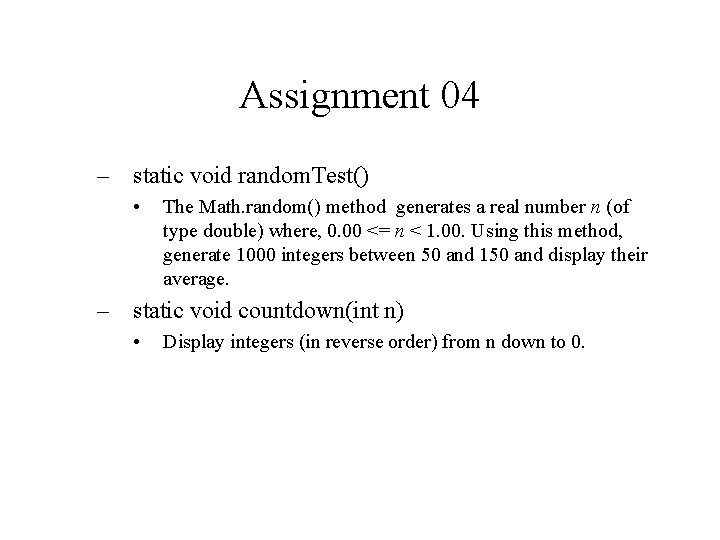
Assignment 04 – static void random. Test() • The Math. random() method generates a real number n (of type double) where, 0. 00 <= n < 1. 00. Using this method, generate 1000 integers between 50 and 150 and display their average. – static void countdown(int n) • Display integers (in reverse order) from n down to 0.
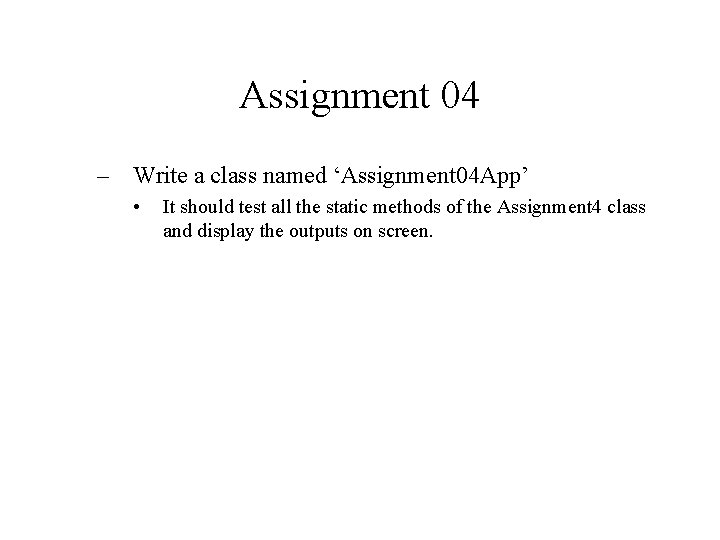
Assignment 04 – Write a class named ‘Assignment 04 App’ • It should test all the static methods of the Assignment 4 class and display the outputs on screen.
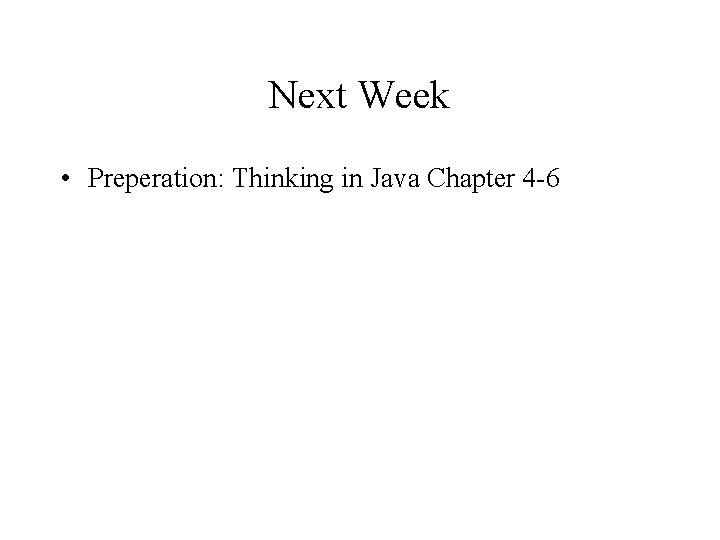
Next Week • Preperation: Thinking in Java Chapter 4 -6
- Slides: 26