Numpy Numerical Python John R Woodward Introduction Numpy
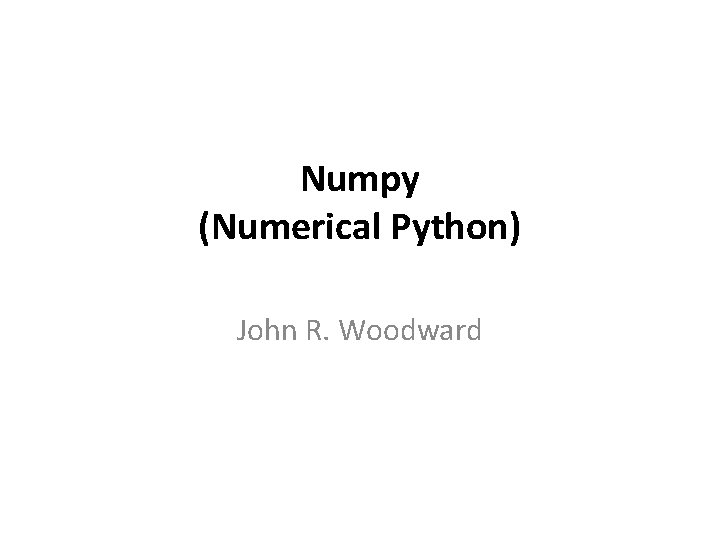
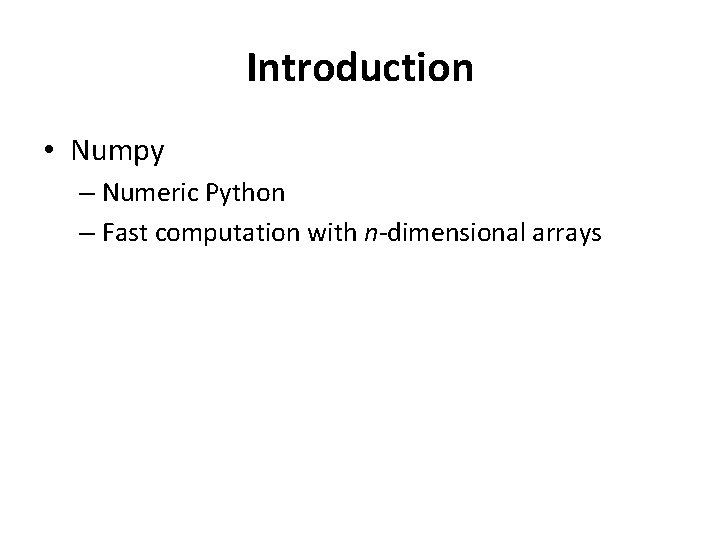
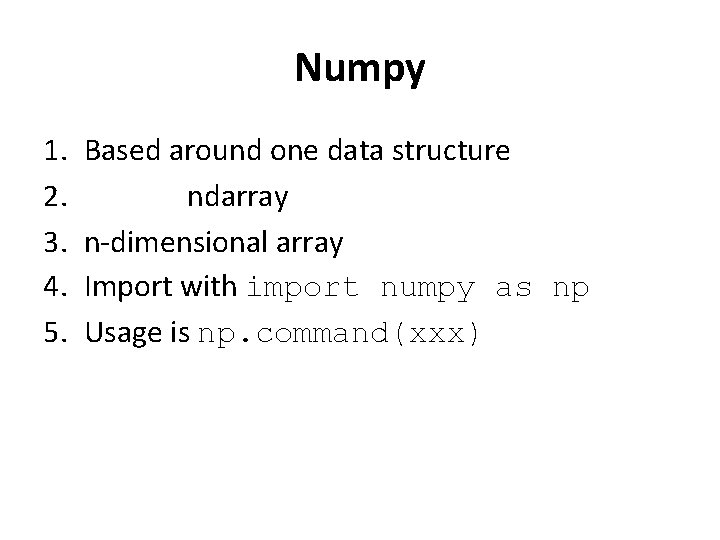
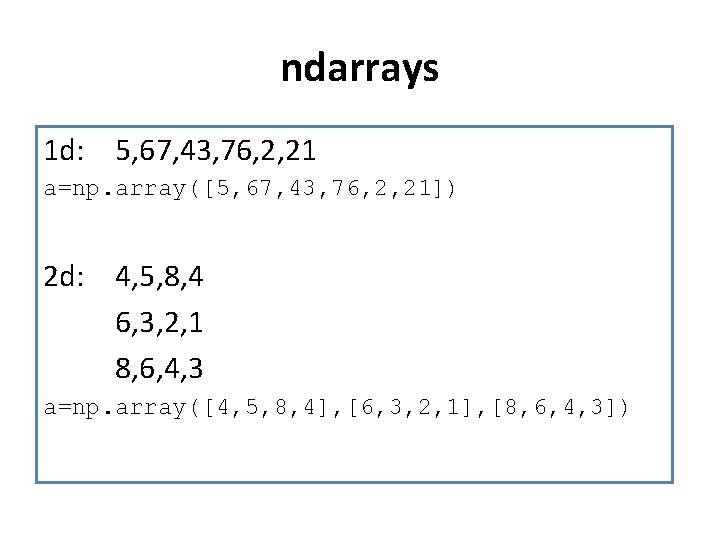
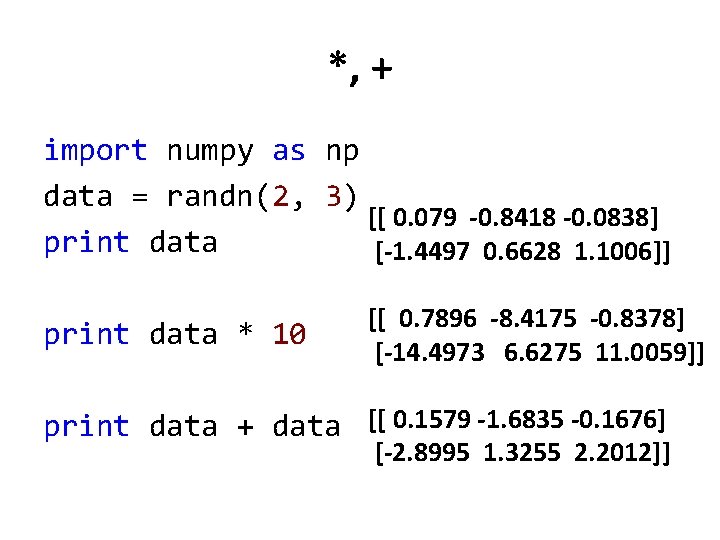
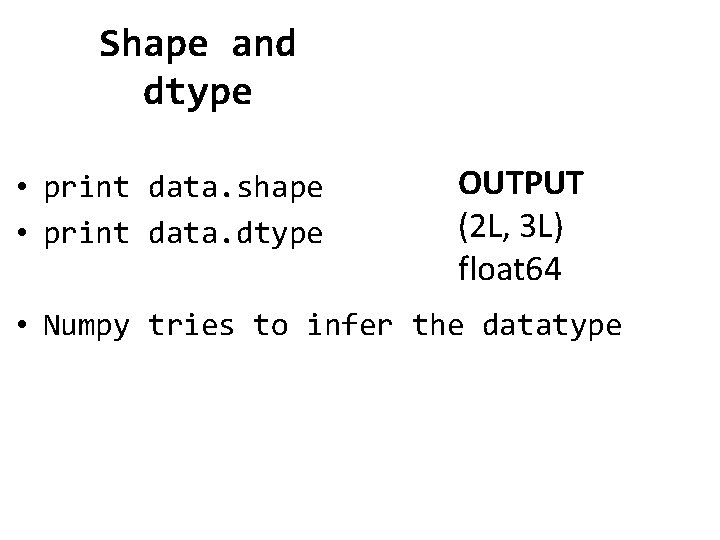
![Creating ndarrays • data 1 = [6, 7. 5, 8, 0, 1] • arr Creating ndarrays • data 1 = [6, 7. 5, 8, 0, 1] • arr](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-7.jpg)
![Multidimensional arrays data 2 = [[1, 2, 3, 4], [5, 6, 7, 8]] arr Multidimensional arrays data 2 = [[1, 2, 3, 4], [5, 6, 7, 8]] arr](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-8.jpg)
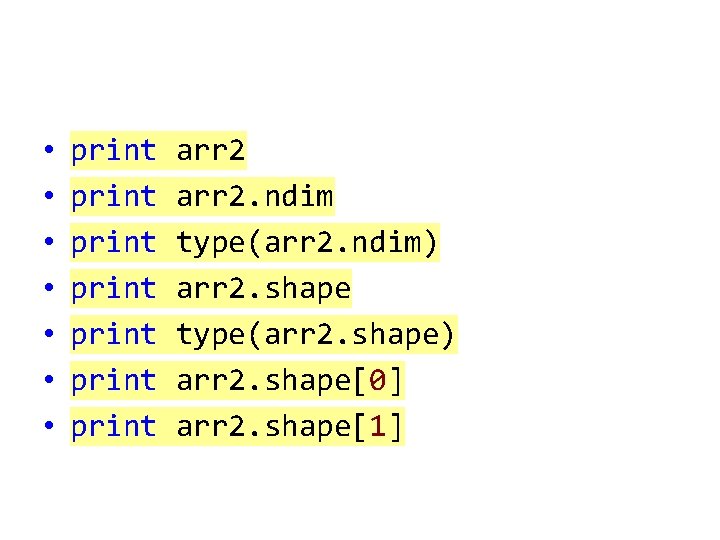
![• • print print OUTPUT [[1 2 3 4] arr 2 [5 6 • • print print OUTPUT [[1 2 3 4] arr 2 [5 6](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-10.jpg)
![3 d array • • • data 2 = [[[1]]] arr 2 = np. 3 d array • • • data 2 = [[[1]]] arr 2 = np.](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-11.jpg)
![3 d array • • • data 2 = [[[1]]] arr 2 = np. 3 d array • • • data 2 = [[[1]]] arr 2 = np.](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-12.jpg)
![More making arrays • np. zeros(10) [ 0. 0. 0. ] [[ 0. 0. More making arrays • np. zeros(10) [ 0. 0. 0. ] [[ 0. 0.](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-13.jpg)
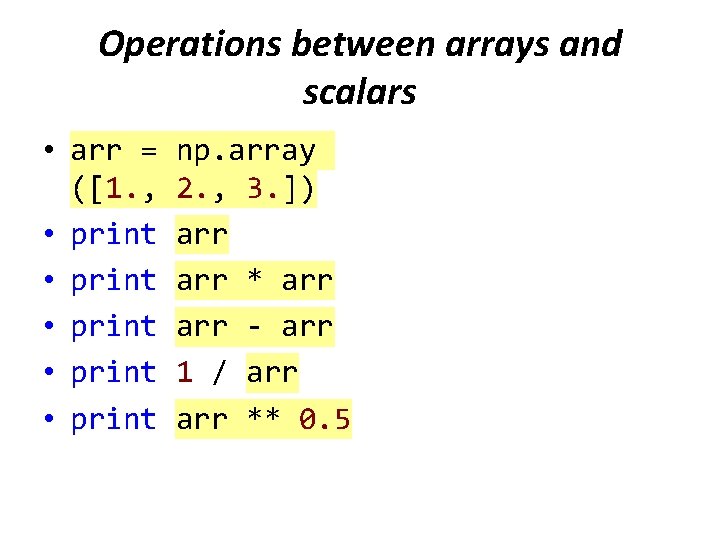
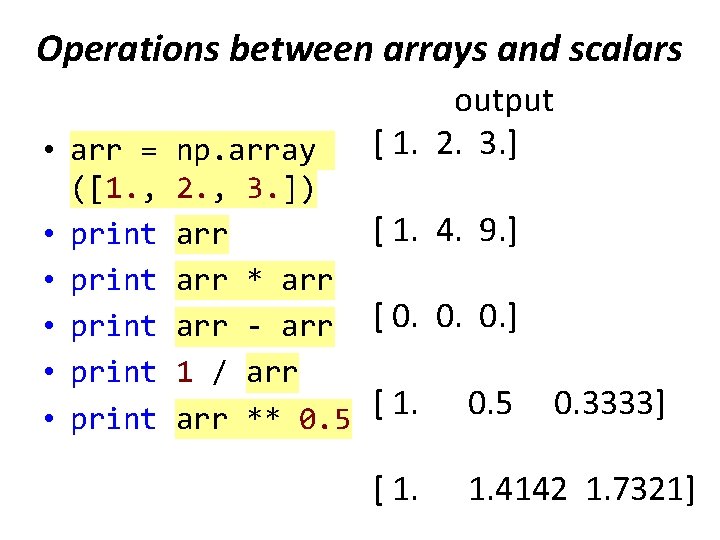
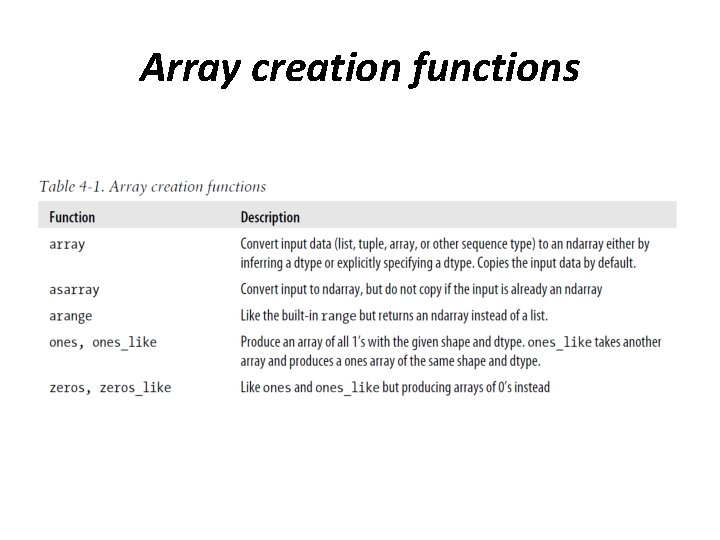
![• a=np. array([True], dtype=np. int 64) • print a. dtype • a=np. array([True], • a=np. array([True], dtype=np. int 64) • print a. dtype • a=np. array([True],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-17.jpg)
![• a=np. array([True], dtype=np. int 64) • print a. dtype • a=np. array([True], • a=np. array([True], dtype=np. int 64) • print a. dtype • a=np. array([True],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-18.jpg)
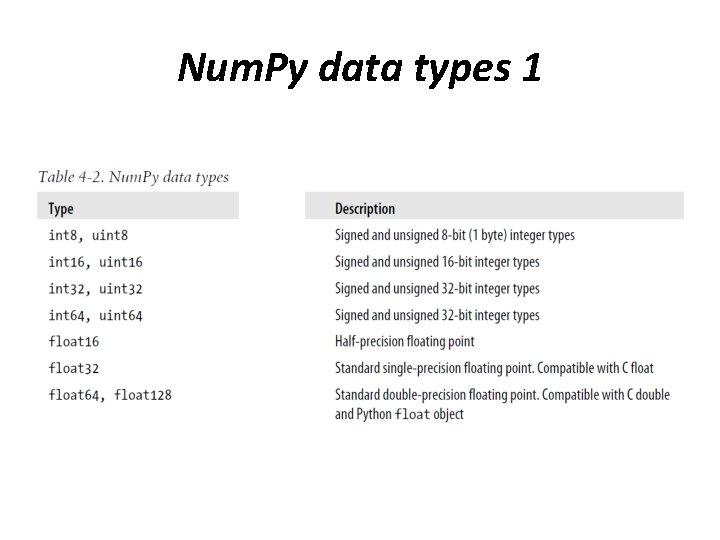
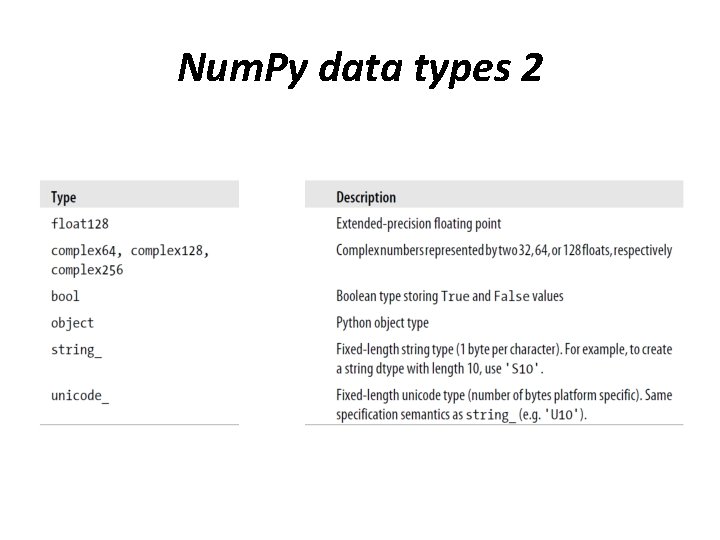
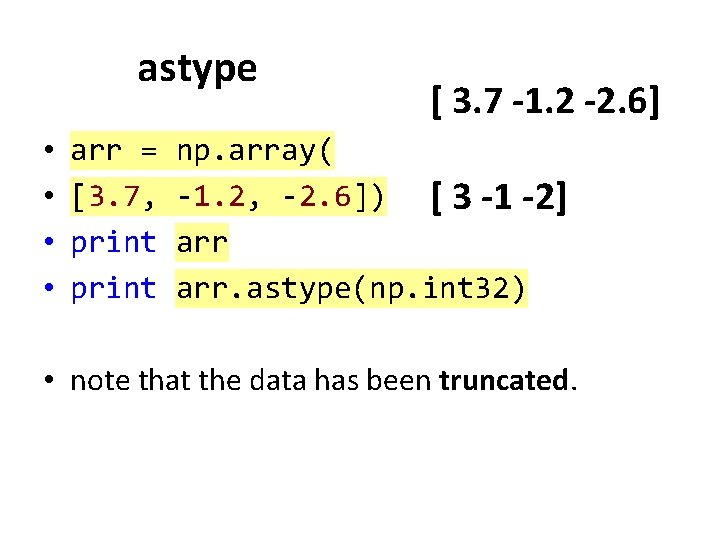
![astype - string to float • numeric_strings = np. array(['1. 25', '-9. 6', '42'], astype - string to float • numeric_strings = np. array(['1. 25', '-9. 6', '42'],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-22.jpg)
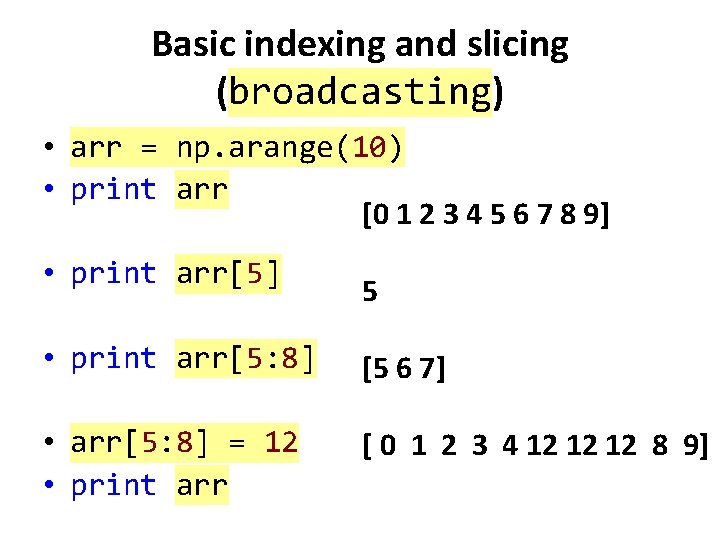
![The original array has changed • • • arr_slice = arr[5: 8] arr_slice[1] = The original array has changed • • • arr_slice = arr[5: 8] arr_slice[1] =](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-24.jpg)
![2 d array • • arr 2 d = np. array ([[1, 2, 3], 2 d array • • arr 2 d = np. array ([[1, 2, 3],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-25.jpg)
![3 d array • • arr 3 d = np. array ([[[1, 2, 3], 3 d array • • arr 3 d = np. array ([[[1, 2, 3],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-26.jpg)
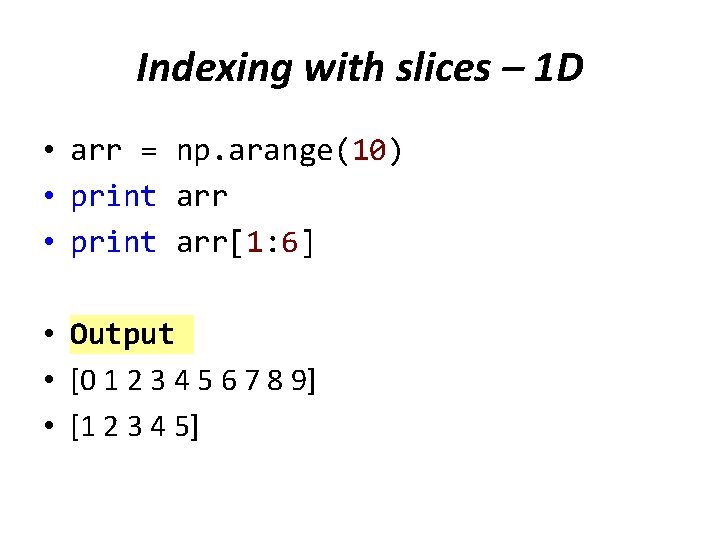
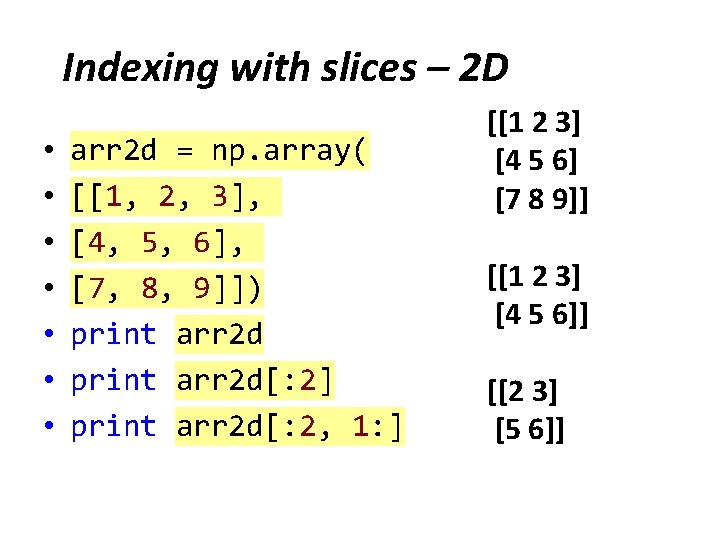
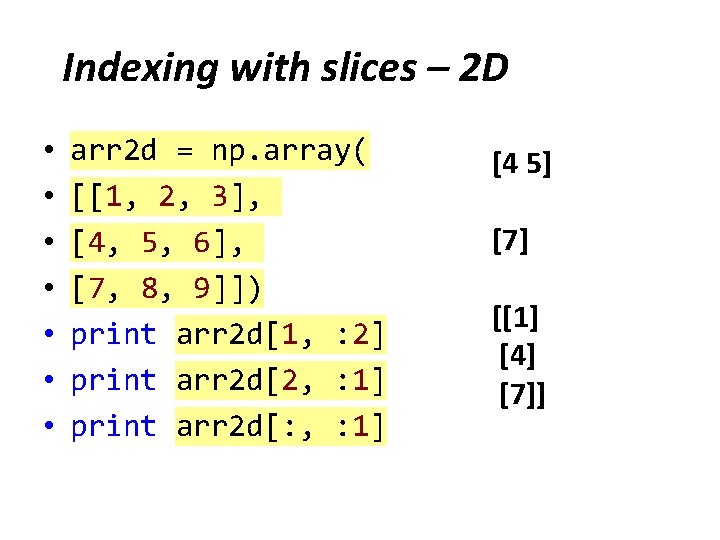
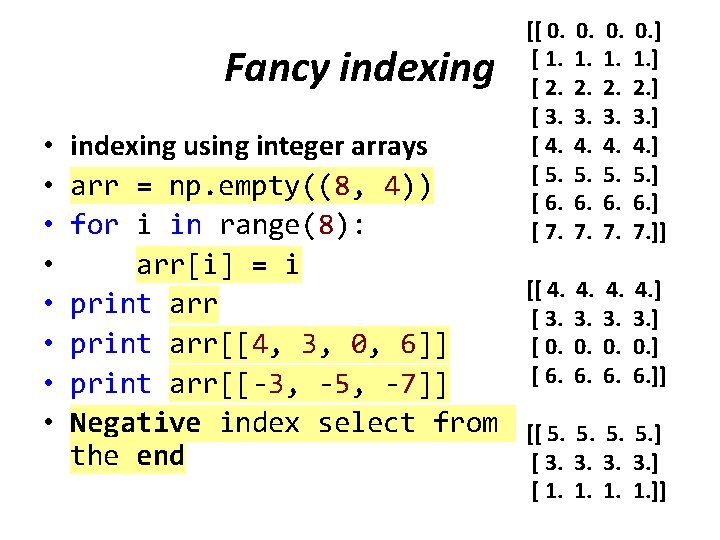
![Transposing arrays and swapping axes [[ 0 1 2 3 4] [ 5 6 Transposing arrays and swapping axes [[ 0 1 2 3 4] [ 5 6](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-31.jpg)
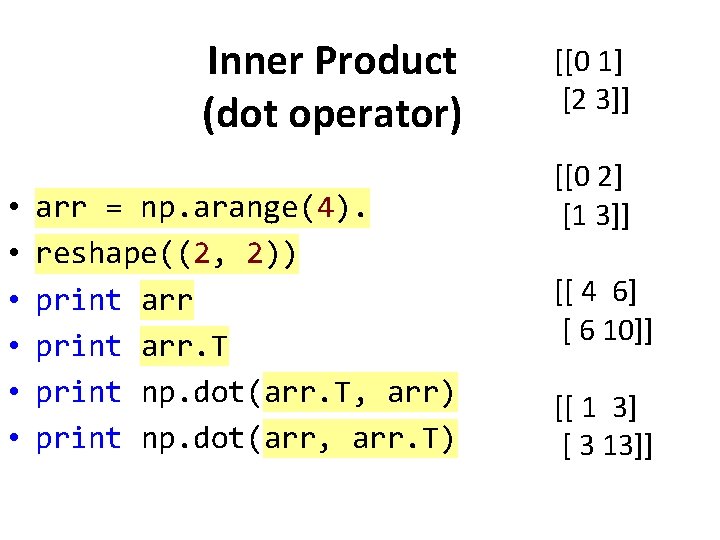
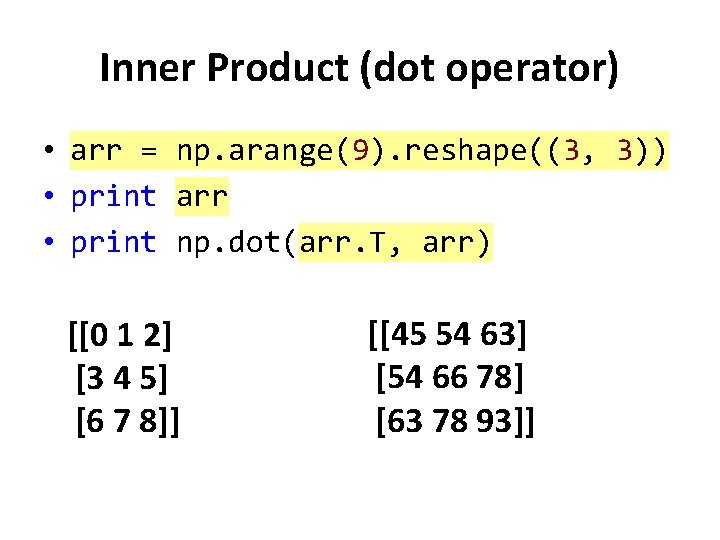
![arr*arr • arr = np. arange(9). reshape((3, 3)) • print arr*arr [[0 1 2] arr*arr • arr = np. arange(9). reshape((3, 3)) • print arr*arr [[0 1 2]](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-34.jpg)
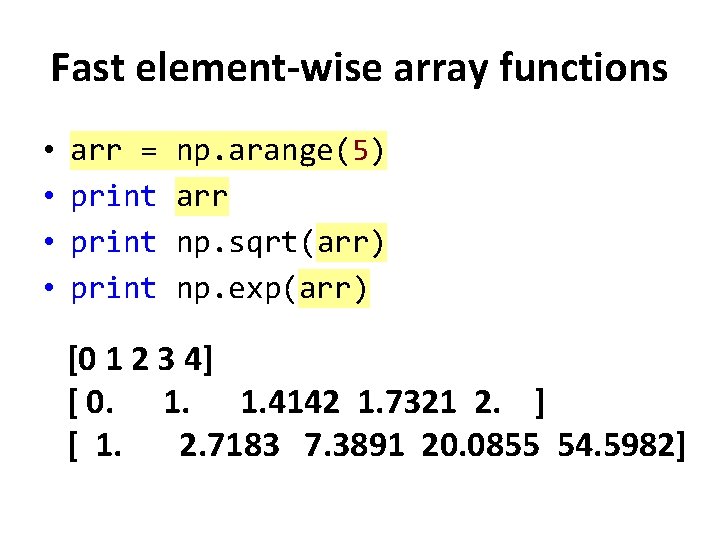
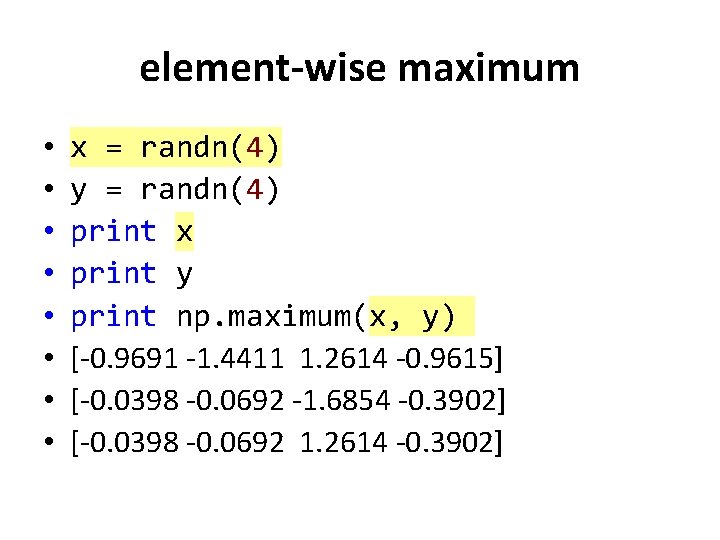
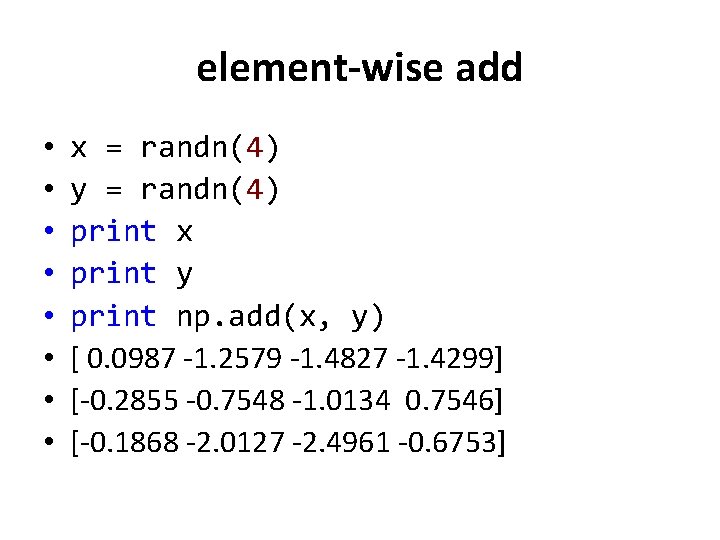
- Slides: 37
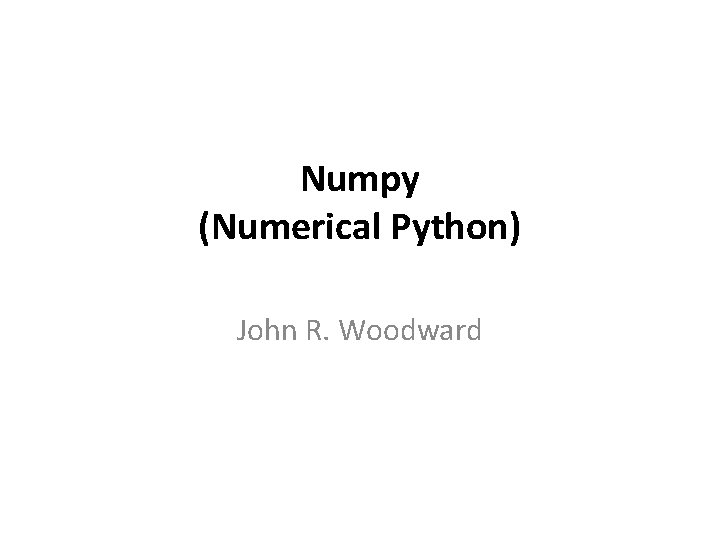
Numpy (Numerical Python) John R. Woodward
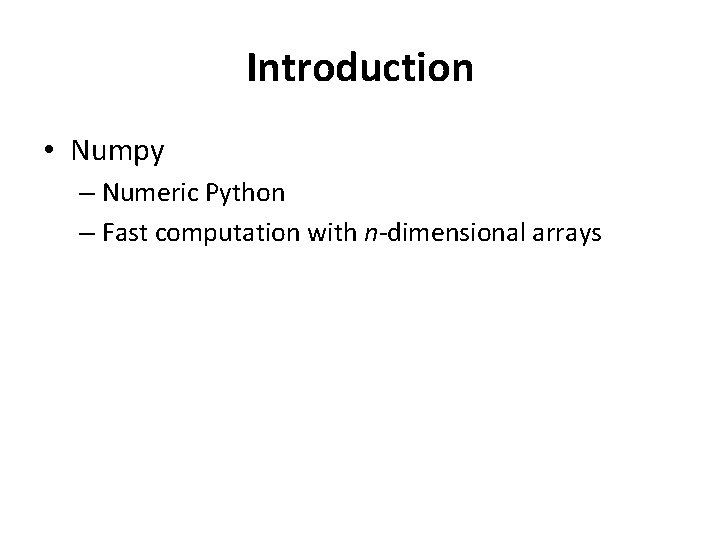
Introduction • Numpy – Numeric Python – Fast computation with n-dimensional arrays
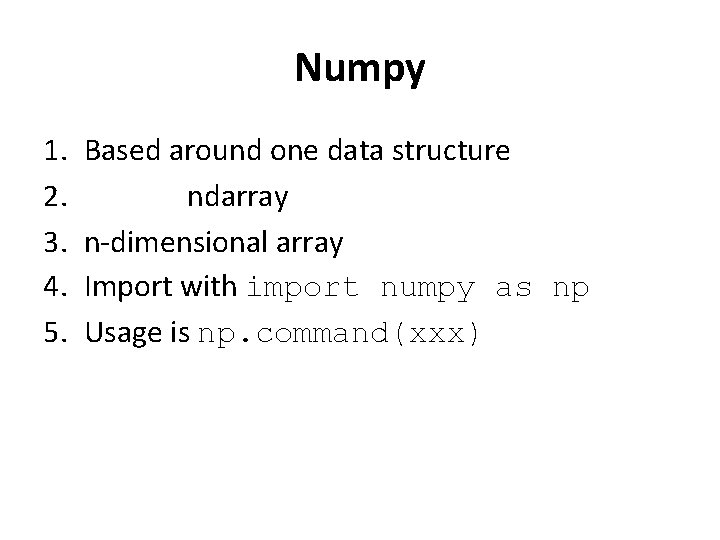
Numpy 1. 2. 3. 4. 5. Based around one data structure ndarray n-dimensional array Import with import numpy as np Usage is np. command(xxx)
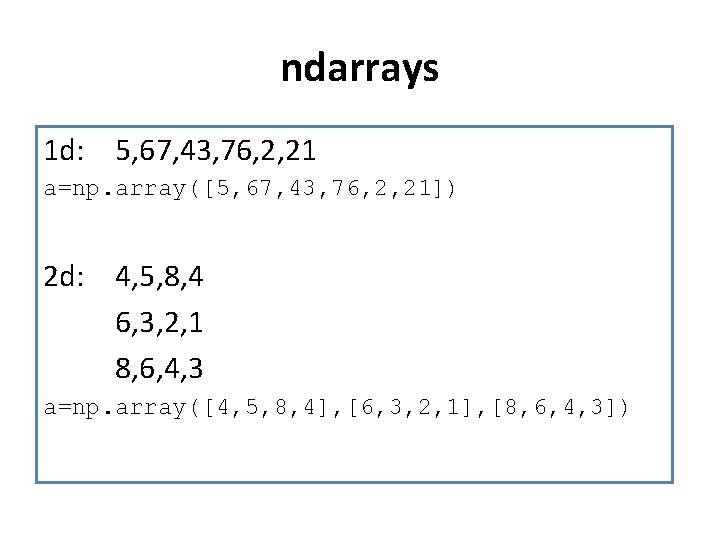
ndarrays 1 d: 5, 67, 43, 76, 2, 21 a=np. array([5, 67, 43, 76, 2, 21]) 2 d: 4, 5, 8, 4 6, 3, 2, 1 8, 6, 4, 3 a=np. array([4, 5, 8, 4], [6, 3, 2, 1], [8, 6, 4, 3])
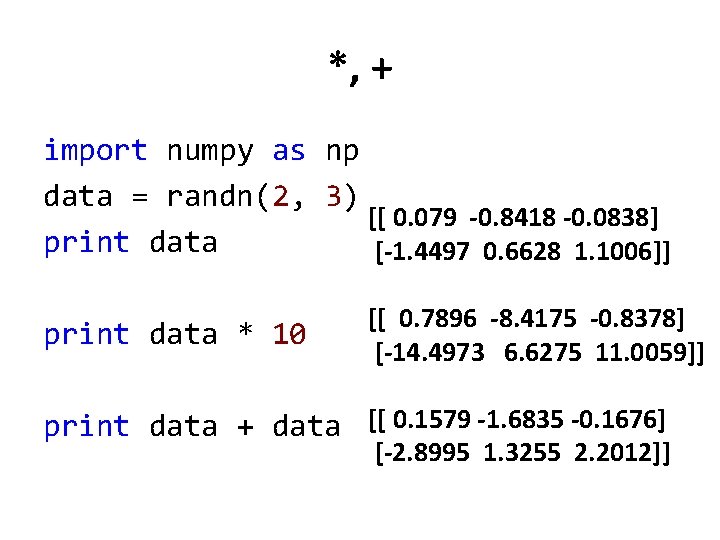
*, + import numpy as np data = randn(2, 3) [[ 0. 079 -0. 8418 -0. 0838] print data [-1. 4497 0. 6628 1. 1006]] print data * 10 [[ 0. 7896 -8. 4175 -0. 8378] [-14. 4973 6. 6275 11. 0059]] print data + data [[ 0. 1579 -1. 6835 -0. 1676] [-2. 8995 1. 3255 2. 2012]]
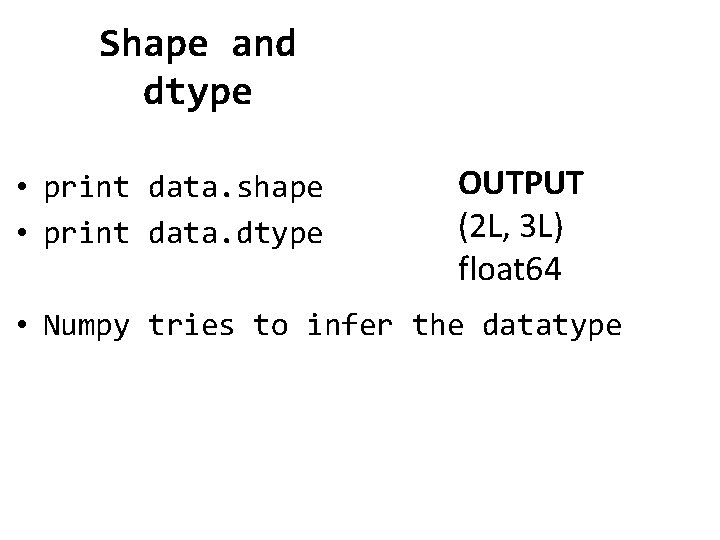
Shape and dtype • print data. shape • print data. dtype OUTPUT (2 L, 3 L) float 64 • Numpy tries to infer the datatype
![Creating ndarrays data 1 6 7 5 8 0 1 arr Creating ndarrays • data 1 = [6, 7. 5, 8, 0, 1] • arr](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-7.jpg)
Creating ndarrays • data 1 = [6, 7. 5, 8, 0, 1] • arr 1 = np. array(data 1) • print arr 1 • Output • [ 6. 7. 5 8. 0. 1. ]
![Multidimensional arrays data 2 1 2 3 4 5 6 7 8 arr Multidimensional arrays data 2 = [[1, 2, 3, 4], [5, 6, 7, 8]] arr](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-8.jpg)
Multidimensional arrays data 2 = [[1, 2, 3, 4], [5, 6, 7, 8]] arr 2 = np. array(data 2) print arr 2 OUTPUT print arr 2. ndim [[1 2 3 4] [5 6 7 8]] print arr 2. shape 2 (2 L, 4 L)
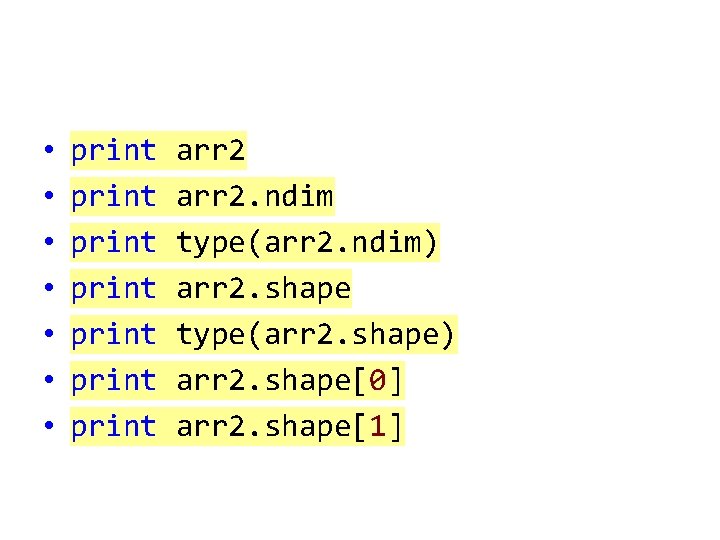
• • print print arr 2. ndim type(arr 2. ndim) arr 2. shape type(arr 2. shape) arr 2. shape[0] arr 2. shape[1]
![print print OUTPUT 1 2 3 4 arr 2 5 6 • • print print OUTPUT [[1 2 3 4] arr 2 [5 6](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-10.jpg)
• • print print OUTPUT [[1 2 3 4] arr 2 [5 6 7 8]] arr 2. ndim 2 type(arr 2. ndim) <type 'int'> arr 2. shape (2 L, 4 L) type(arr 2. shape) <type 'tuple'> arr 2. shape[0] 2 arr 2. shape[1] 4
![3 d array data 2 1 arr 2 np 3 d array • • • data 2 = [[[1]]] arr 2 = np.](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-11.jpg)
3 d array • • • data 2 = [[[1]]] arr 2 = np. array(data 2) print arr 2. ndim print arr 2. shape
![3 d array data 2 1 arr 2 np 3 d array • • • data 2 = [[[1]]] arr 2 = np.](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-12.jpg)
3 d array • • • data 2 = [[[1]]] arr 2 = np. array(data 2) print arr 2. ndim print arr 2. shape OUTPUT [[[1]]] 3 (1 L, 1 L)
![More making arrays np zeros10 0 0 0 0 0 More making arrays • np. zeros(10) [ 0. 0. 0. ] [[ 0. 0.](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-13.jpg)
More making arrays • np. zeros(10) [ 0. 0. 0. ] [[ 0. 0. 0. ] • np. zeros((3, 6)) [ 0. 0. 0. ]] • np. empty((2, 3, 2)) [[[ 0. 0. ] [ 0. 0. ]]]
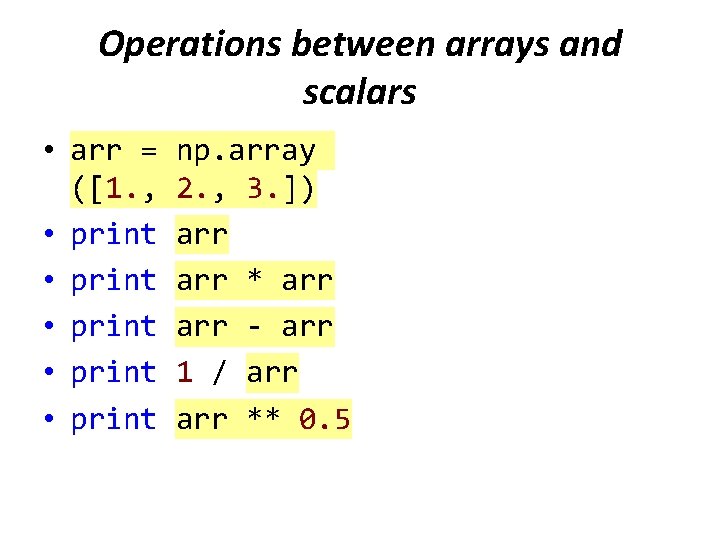
Operations between arrays and scalars • arr = ([1. , • print • print np. array 2. , 3. ]) arr * arr - arr 1 / arr ** 0. 5
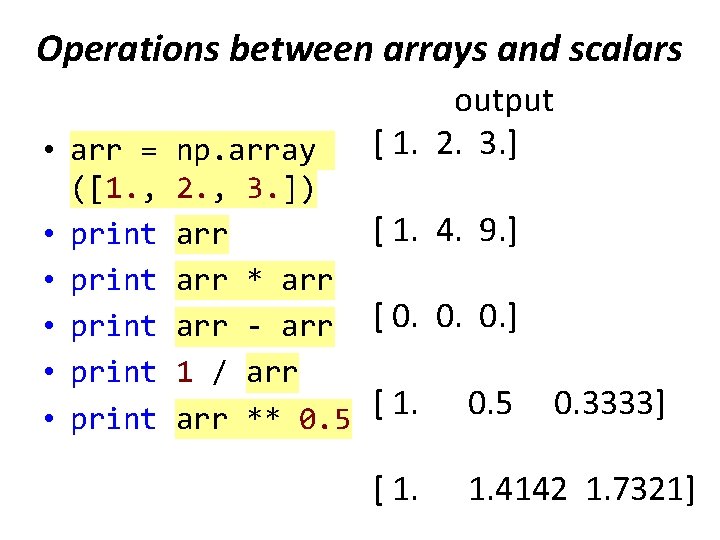
Operations between arrays and scalars • arr = ([1. , • print • print output [ 1. 2. 3. ] np. array 2. , 3. ]) [ 1. 4. 9. ] arr * arr - arr [ 0. 0. 0. ] 1 / arr [ 1. 0. 5 arr ** 0. 5 [ 1. 0. 3333] 1. 4142 1. 7321]
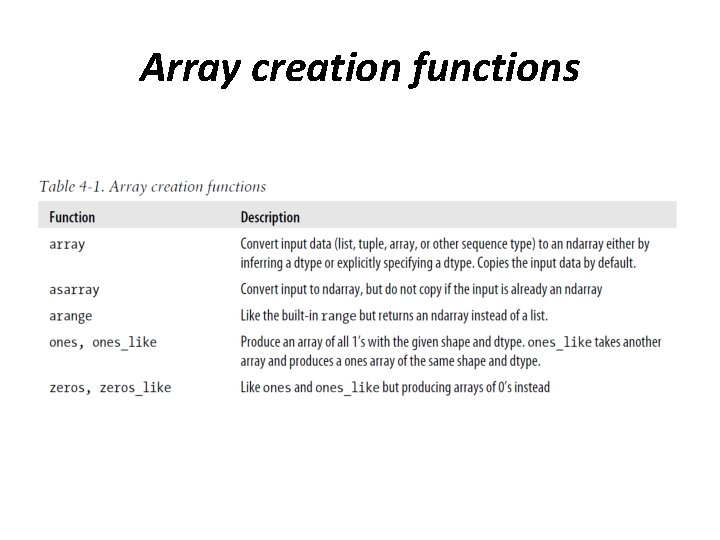
Array creation functions
![anp arrayTrue dtypenp int 64 print a dtype anp arrayTrue • a=np. array([True], dtype=np. int 64) • print a. dtype • a=np. array([True],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-17.jpg)
• a=np. array([True], dtype=np. int 64) • print a. dtype • a=np. array([True], dtype=np. bool) • print a. dtype
![anp arrayTrue dtypenp int 64 print a dtype anp arrayTrue • a=np. array([True], dtype=np. int 64) • print a. dtype • a=np. array([True],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-18.jpg)
• a=np. array([True], dtype=np. int 64) • print a. dtype • a=np. array([True], dtype=np. bool) • print a. dtype [1] Int 64 [ True] bool
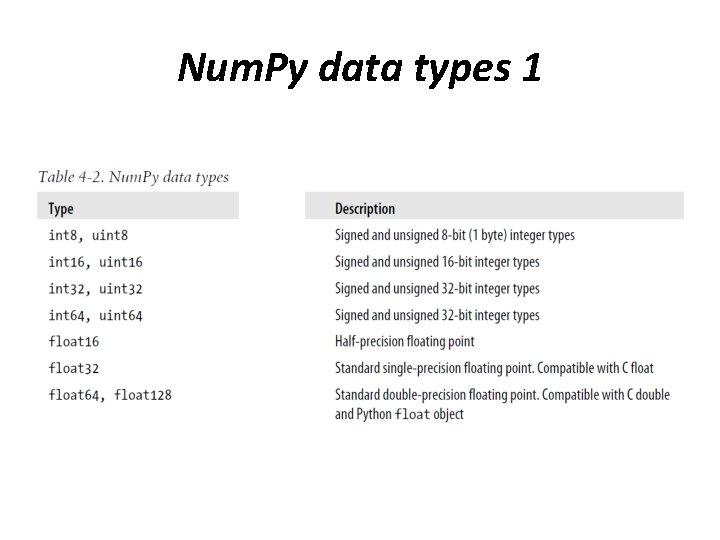
Num. Py data types 1
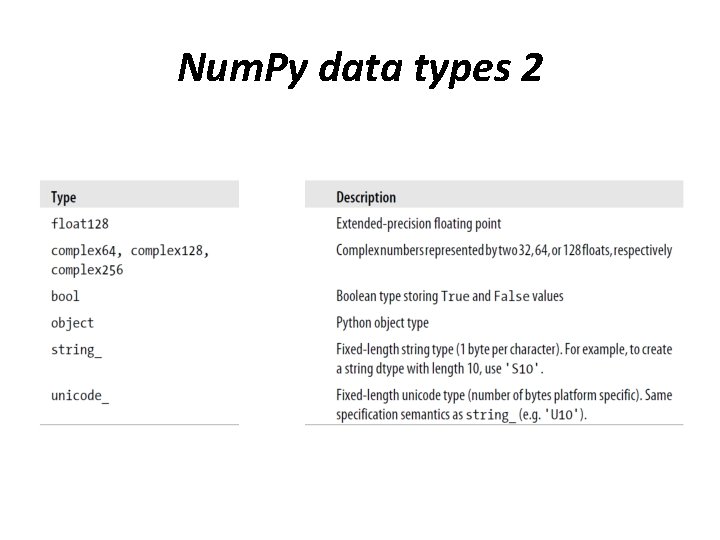
Num. Py data types 2
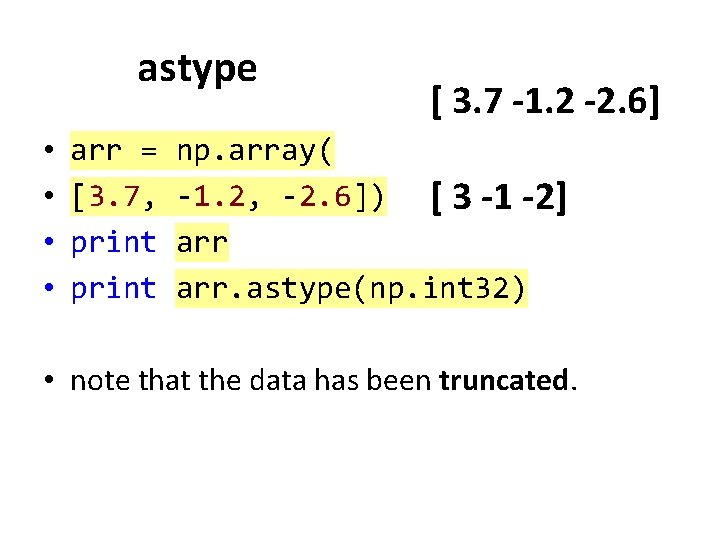
astype • • arr = [3. 7, print [ 3. 7 -1. 2 -2. 6] np. array( -1. 2, -2. 6]) [ 3 -1 -2] arr. astype(np. int 32) • note that the data has been truncated.
![astype string to float numericstrings np array1 25 9 6 42 astype - string to float • numeric_strings = np. array(['1. 25', '-9. 6', '42'],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-22.jpg)
astype - string to float • numeric_strings = np. array(['1. 25', '-9. 6', '42'], dtype=np. string_) • print numeric_strings. astype(float) ['1. 25' '-9. 6' '42'] [ 1. 25 -9. 6 42. ]
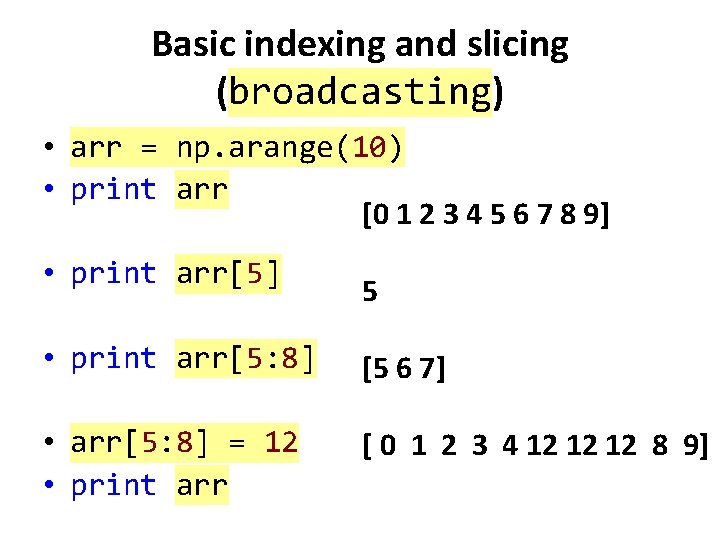
Basic indexing and slicing (broadcasting) • arr = np. arange(10) • print arr [0 1 2 3 4 5 6 7 8 9] • print arr[5] 5 • print arr[5: 8] [5 6 7] • arr[5: 8] = 12 • print arr [ 0 1 2 3 4 12 12 12 8 9]
![The original array has changed arrslice arr5 8 arrslice1 The original array has changed • • • arr_slice = arr[5: 8] arr_slice[1] =](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-24.jpg)
The original array has changed • • • arr_slice = arr[5: 8] arr_slice[1] = 12345 print arr_slice[: ] = 64 print arr [ 0 1 2 3 4 12 12345 12 [ 0 1 2 3 4 64 64 64 8 9]
![2 d array arr 2 d np array 1 2 3 2 d array • • arr 2 d = np. array ([[1, 2, 3],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-25.jpg)
2 d array • • arr 2 d = np. array ([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) print arr 2 d[2] print arr 2 d[0][2] print arr 2 d[0, 2] (last two are same) [7 8 9] 3 3
![3 d array arr 3 d np array 1 2 3 3 d array • • arr 3 d = np. array ([[[1, 2, 3],](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-26.jpg)
3 d array • • arr 3 d = np. array ([[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]]) print arr 3 d[0] print arr 3 d[1] [[[ 1 2 3] [ 4 5 6]] [[ 7 8 9] [10 11 12]]] [[1 2 3] [4 5 6]] [[ 7 8 9] [10 11 12]]
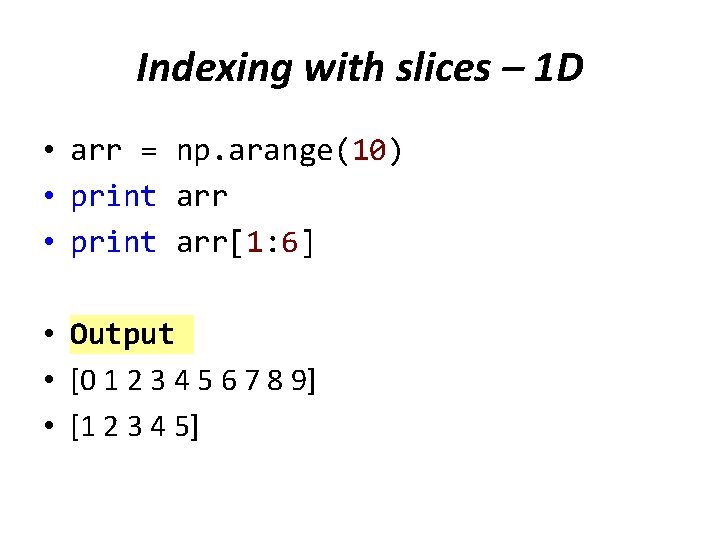
Indexing with slices – 1 D • arr = np. arange(10) • print arr[1: 6] • Output • [0 1 2 3 4 5 6 7 8 9] • [1 2 3 4 5]
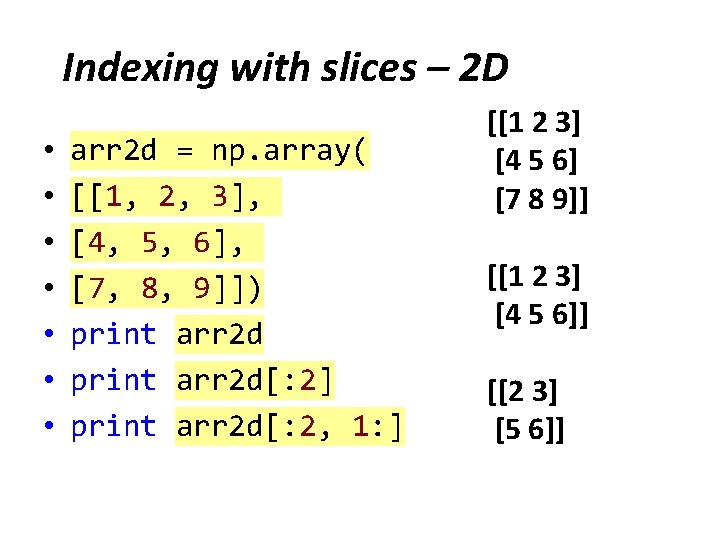
Indexing with slices – 2 D • • arr 2 d = np. array( [[1, 2, 3], [4, 5, 6], [7, 8, 9]]) print arr 2 d[: 2] print arr 2 d[: 2, 1: ] [[1 2 3] [4 5 6] [7 8 9]] [[1 2 3] [4 5 6]] [[2 3] [5 6]]
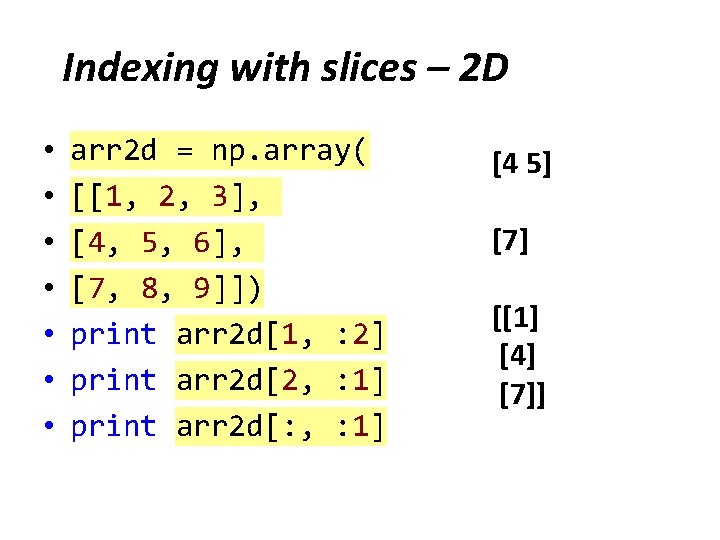
Indexing with slices – 2 D • • arr 2 d = np. array( [[1, 2, 3], [4, 5, 6], [7, 8, 9]]) print arr 2 d[1, : 2] print arr 2 d[2, : 1] print arr 2 d[: , : 1] [4 5] [7] [[1] [4] [7]]
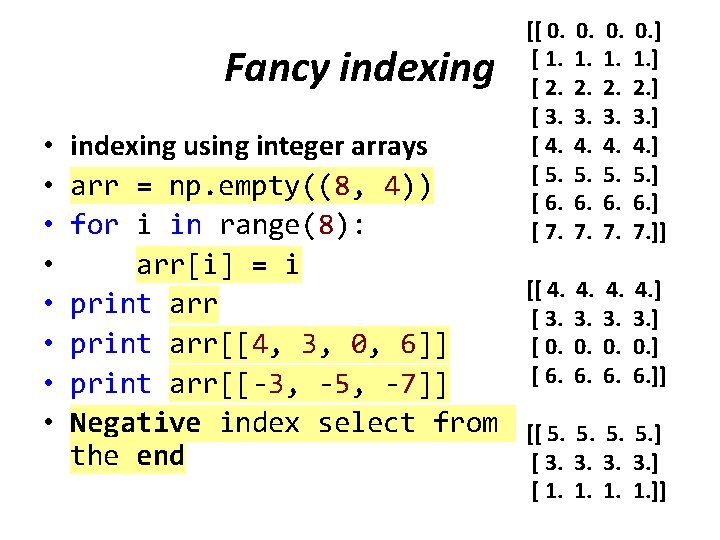
Fancy indexing • • indexing using integer arrays arr = np. empty((8, 4)) for i in range(8): arr[i] = i print arr[[4, 3, 0, 6]] print arr[[-3, -5, -7]] Negative index select from the end [[ 0. [ 1. [ 2. [ 3. [ 4. [ 5. [ 6. [ 7. 0. 1. 2. 3. 4. 5. 6. 7. 0. ] 1. ] 2. ] 3. ] 4. ] 5. ] 6. ] 7. ]] [[ 4. [ 3. [ 0. [ 6. 4. 3. 0. 6. 4. ] 3. ] 0. ] 6. ]] [[ 5. 5. ] [ 3. 3. ] [ 1. 1. ]]
![Transposing arrays and swapping axes 0 1 2 3 4 5 6 Transposing arrays and swapping axes [[ 0 1 2 3 4] [ 5 6](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-31.jpg)
Transposing arrays and swapping axes [[ 0 1 2 3 4] [ 5 6 7 8 9] [10 11 12 13 14]] • arr = np. arange(15). resh (3 L, 5 L) ape((3, 5)) • print arr [[ 0 5 10] [ 1 6 11] • print arr. shape [ 2 7 12] • print arr. T [ 3 8 13] • print arr. T. shape [ 4 9 14]]
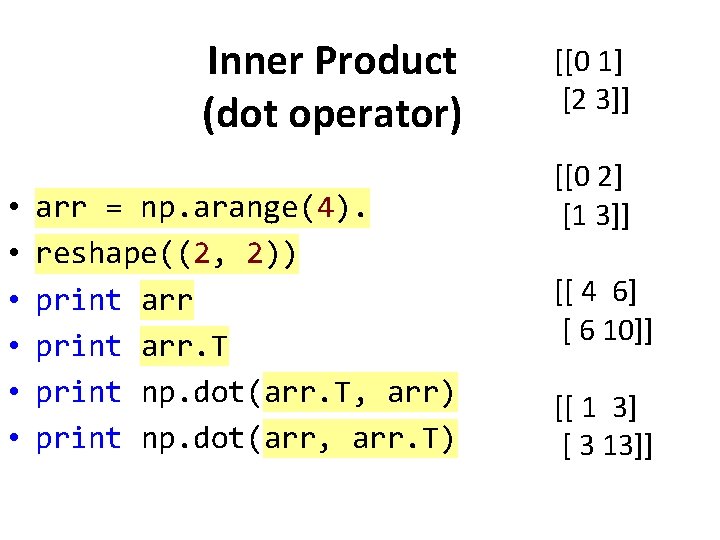
Inner Product (dot operator) • • • arr = np. arange(4). reshape((2, 2)) print arr. T print np. dot(arr. T, arr) print np. dot(arr, arr. T) [[0 1] [2 3]] [[0 2] [1 3]] [[ 4 6] [ 6 10]] [[ 1 3] [ 3 13]]
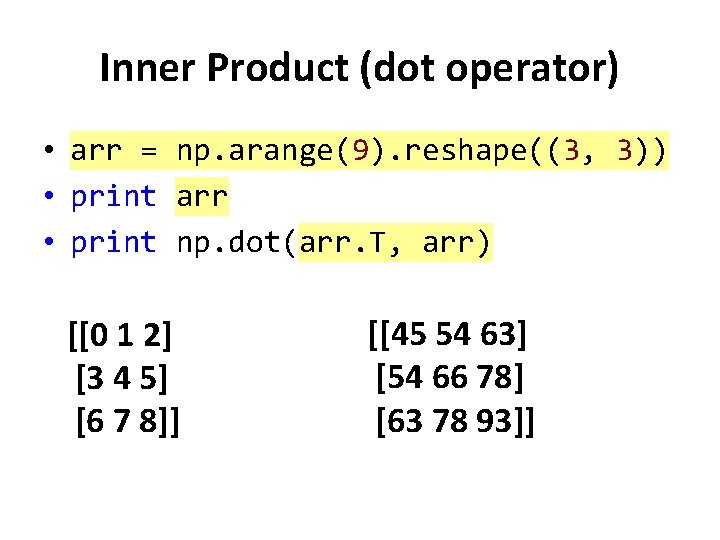
Inner Product (dot operator) • arr = np. arange(9). reshape((3, 3)) • print arr • print np. dot(arr. T, arr) [[0 1 2] [3 4 5] [6 7 8]] [[45 54 63] [54 66 78] [63 78 93]]
![arrarr arr np arange9 reshape3 3 print arrarr 0 1 2 arr*arr • arr = np. arange(9). reshape((3, 3)) • print arr*arr [[0 1 2]](https://slidetodoc.com/presentation_image_h2/7c55f055144e54fe16fa66661674cf12/image-34.jpg)
arr*arr • arr = np. arange(9). reshape((3, 3)) • print arr*arr [[0 1 2] [3 4 5] [6 7 8]] [[ 0 1 4] [ 9 16 25] [36 49 64]]
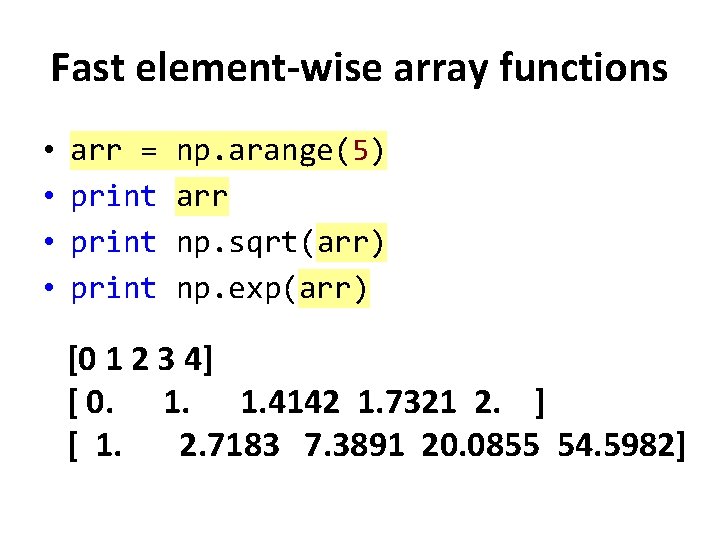
Fast element-wise array functions • • arr = print np. arange(5) arr np. sqrt(arr) np. exp(arr) [0 1 2 3 4] [ 0. 1. 1. 4142 1. 7321 2. ] [ 1. 2. 7183 7. 3891 20. 0855 54. 5982]
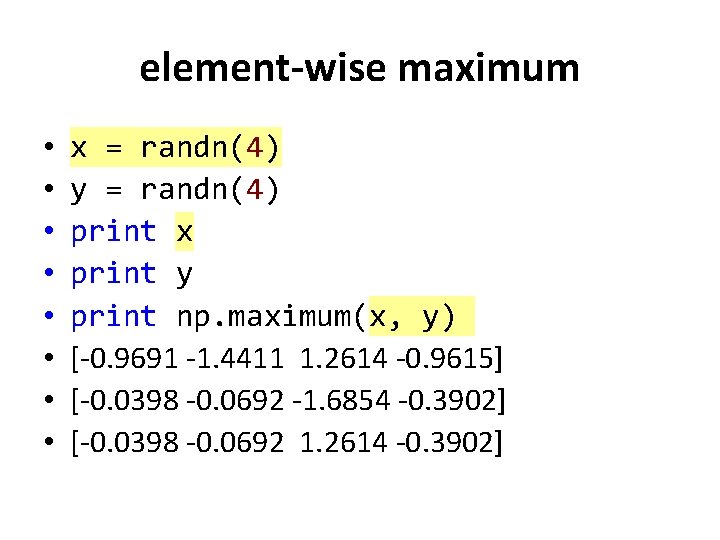
element-wise maximum • • x = randn(4) y = randn(4) print x print y print np. maximum(x, y) [-0. 9691 -1. 4411 1. 2614 -0. 9615] [-0. 0398 -0. 0692 -1. 6854 -0. 3902] [-0. 0398 -0. 0692 1. 2614 -0. 3902]
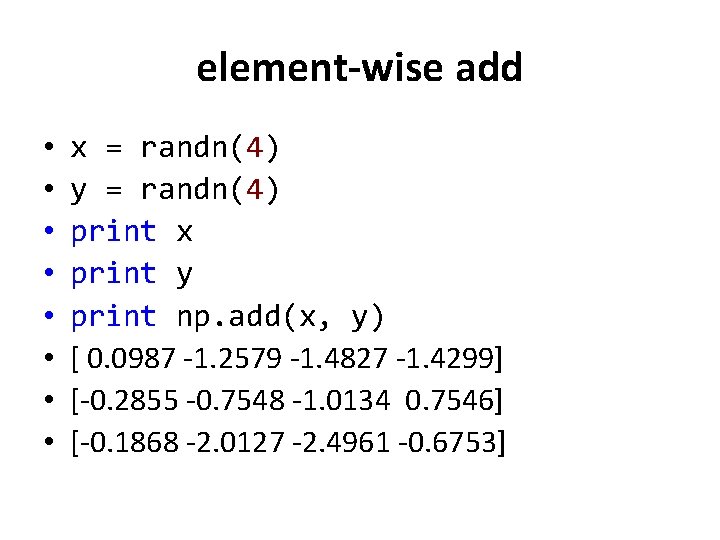
element-wise add • • x = randn(4) y = randn(4) print x print y print np. add(x, y) [ 0. 0987 -1. 2579 -1. 4827 -1. 4299] [-0. 2855 -0. 7548 -1. 0134 0. 7546] [-0. 1868 -2. 0127 -2. 4961 -0. 6753]