Node js Internet course Dr Solange Karsenty Hadassah
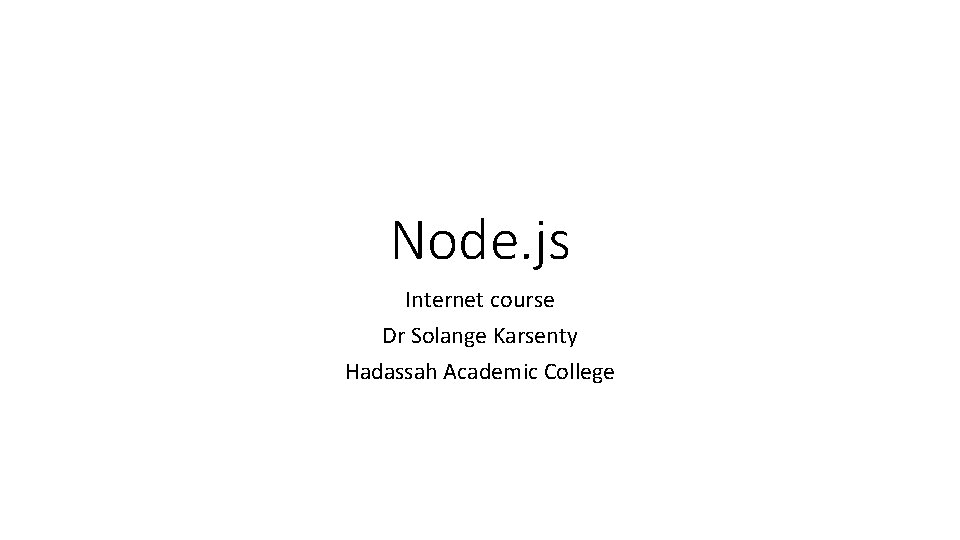
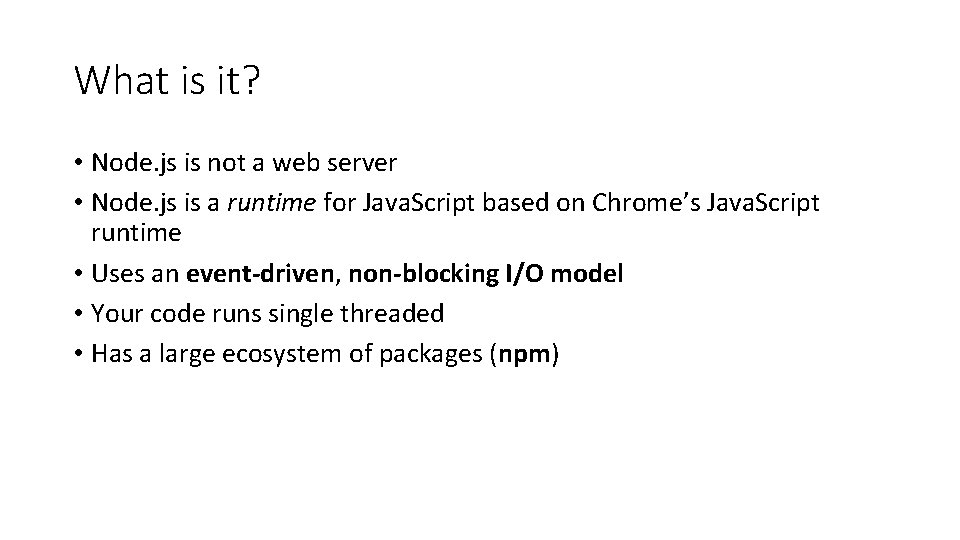
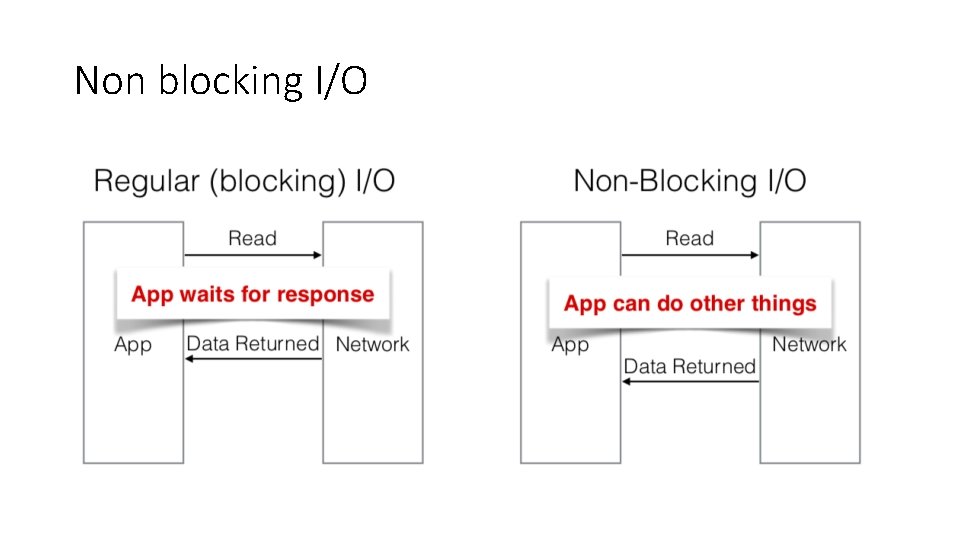
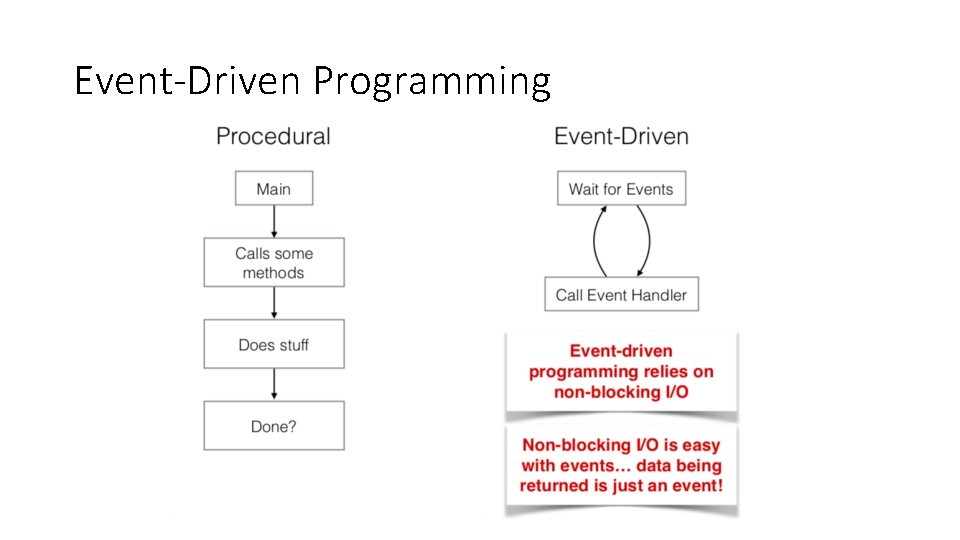
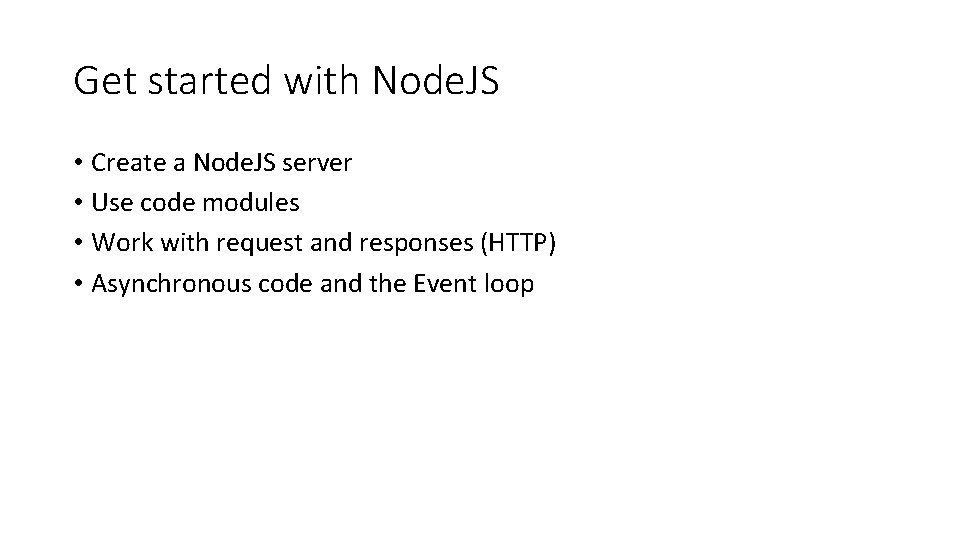
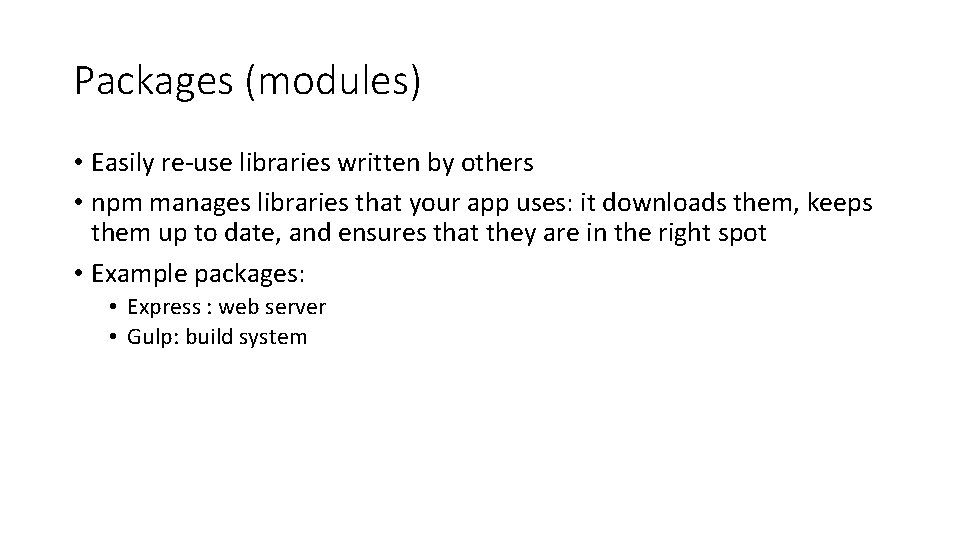
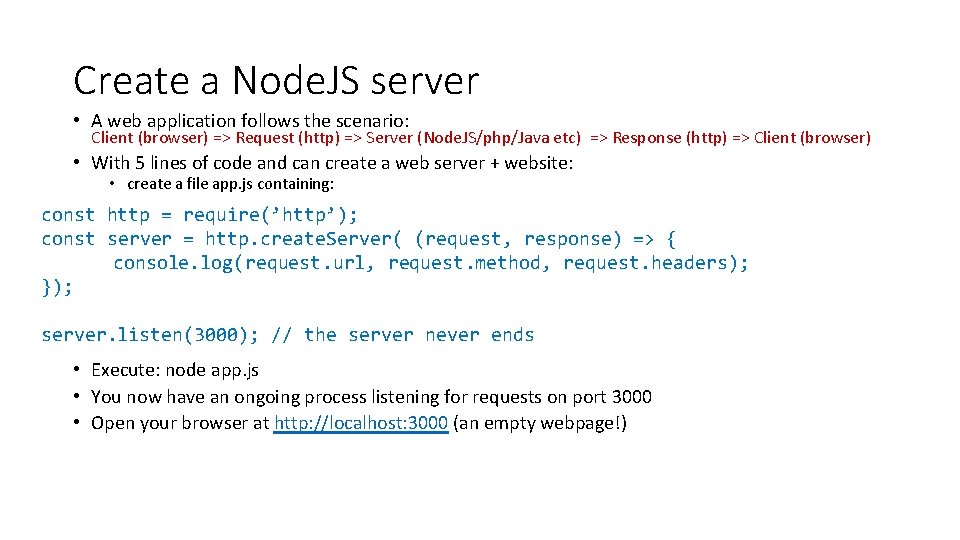
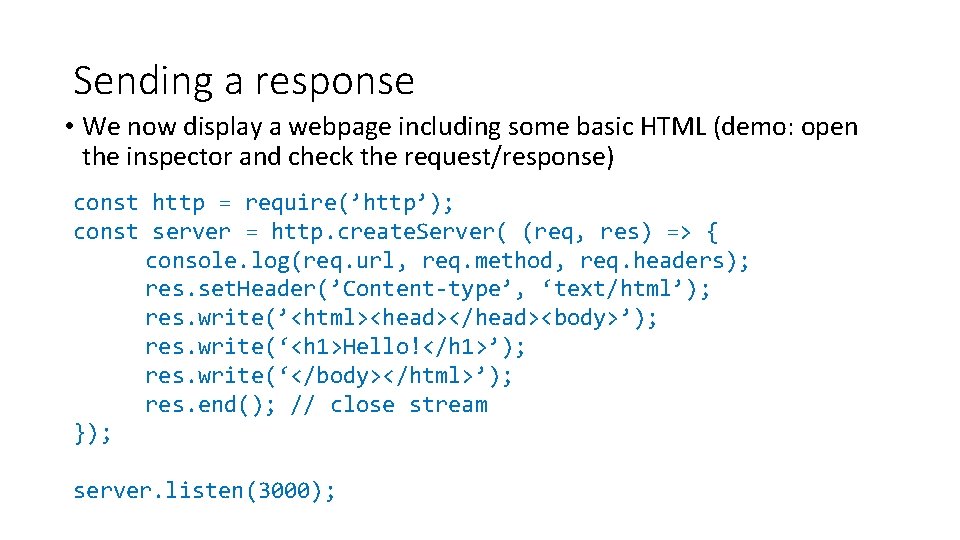
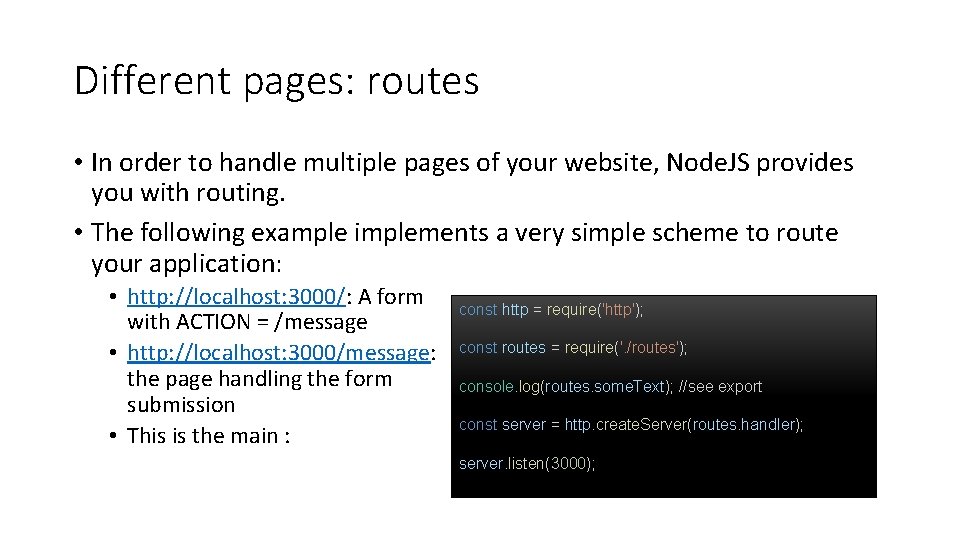
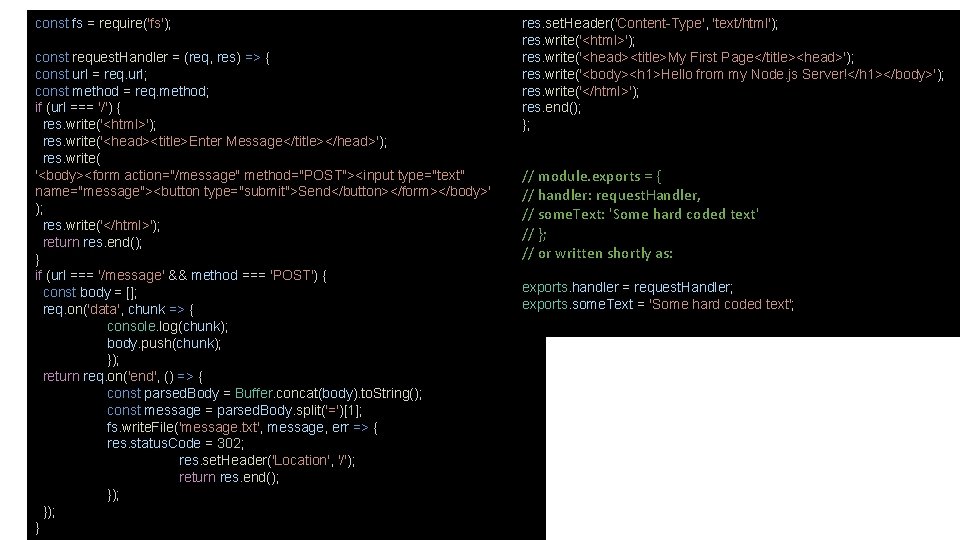
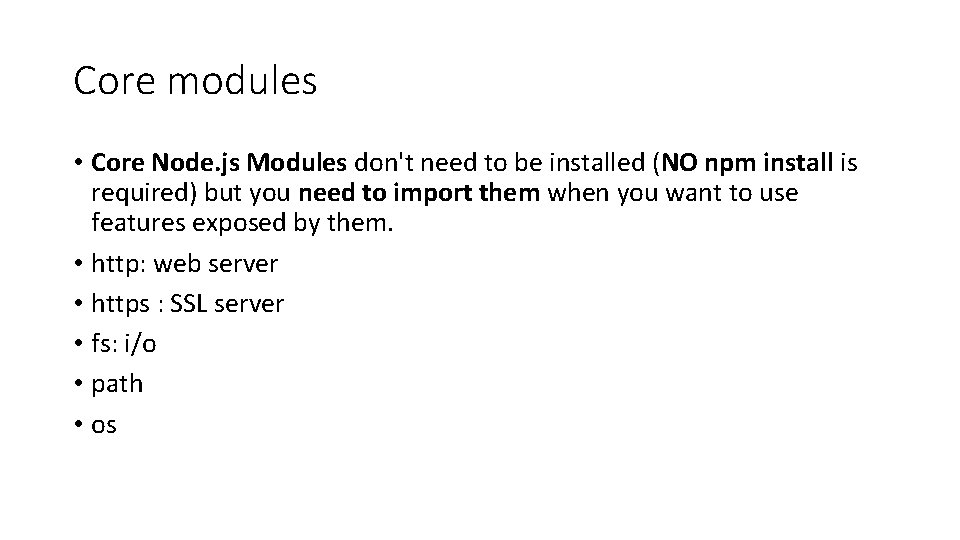
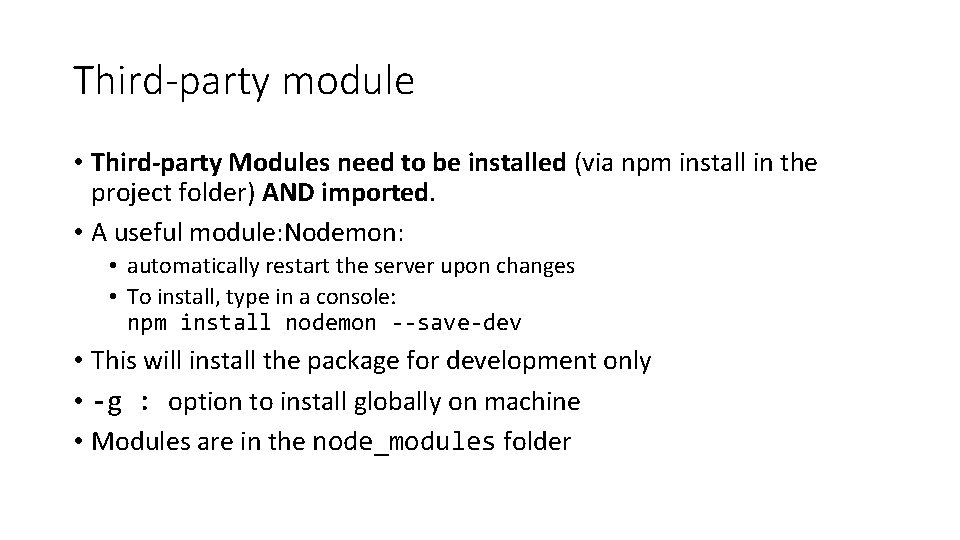
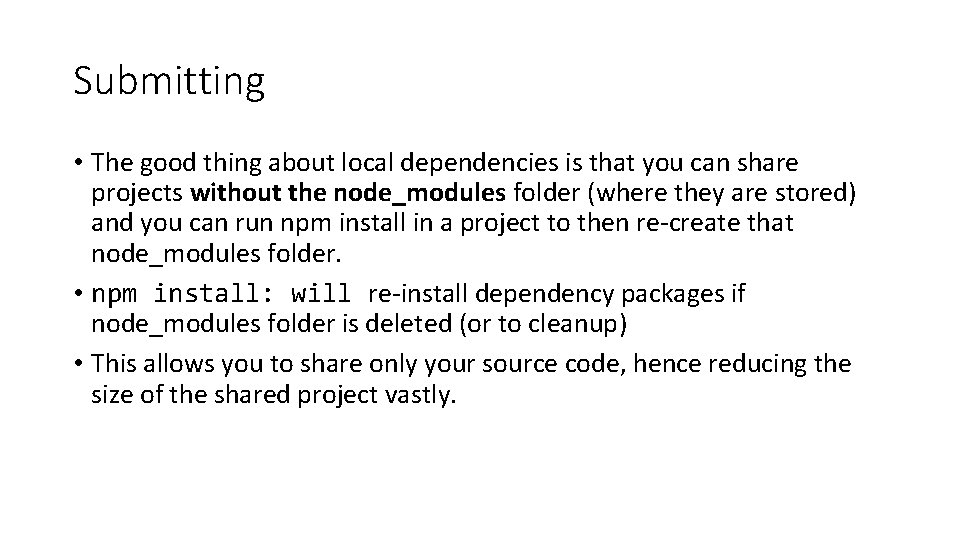
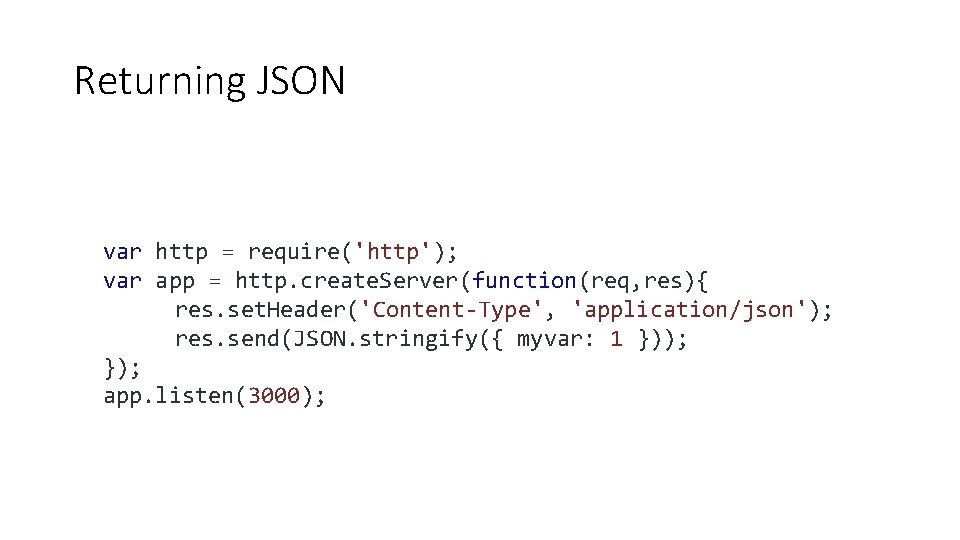
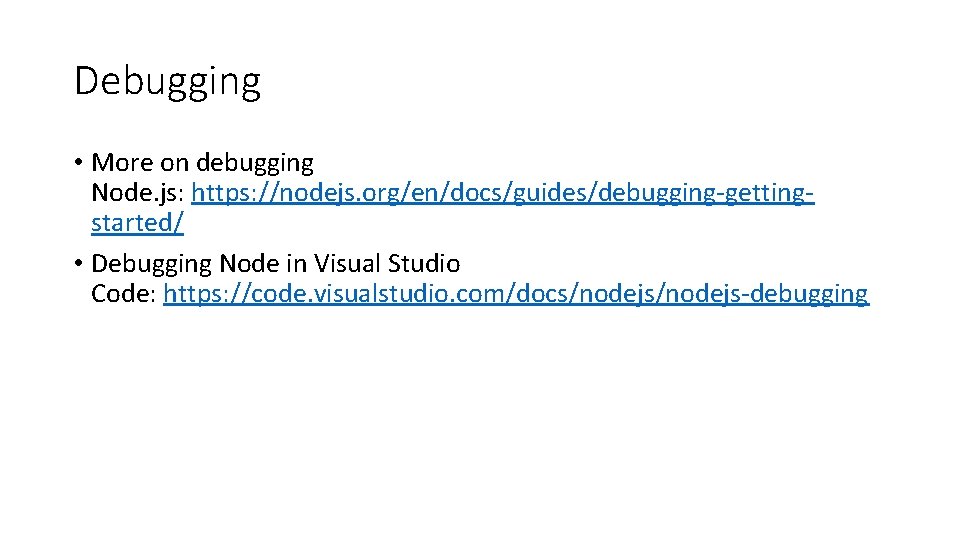
- Slides: 15
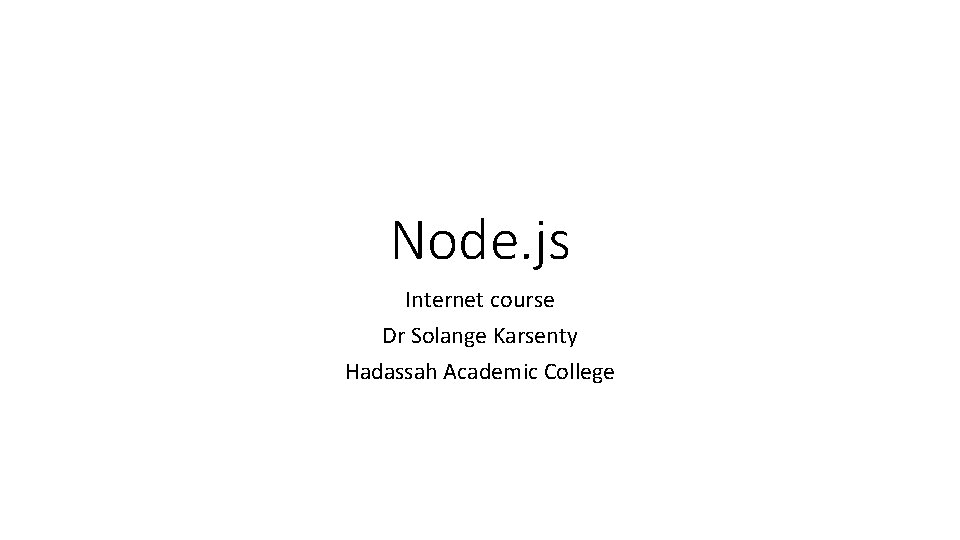
Node. js Internet course Dr Solange Karsenty Hadassah Academic College
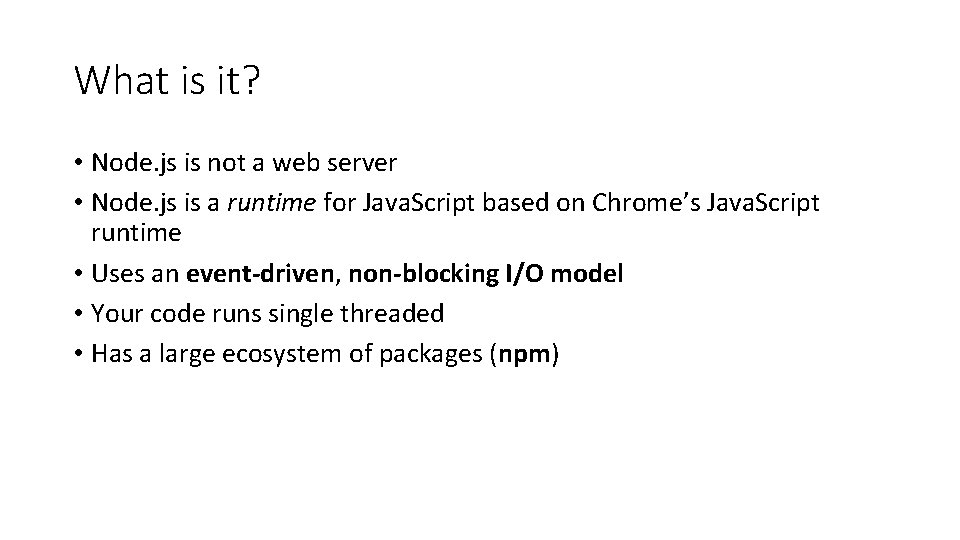
What is it? • Node. js is not a web server • Node. js is a runtime for Java. Script based on Chrome’s Java. Script runtime • Uses an event-driven, non-blocking I/O model • Your code runs single threaded • Has a large ecosystem of packages (npm)
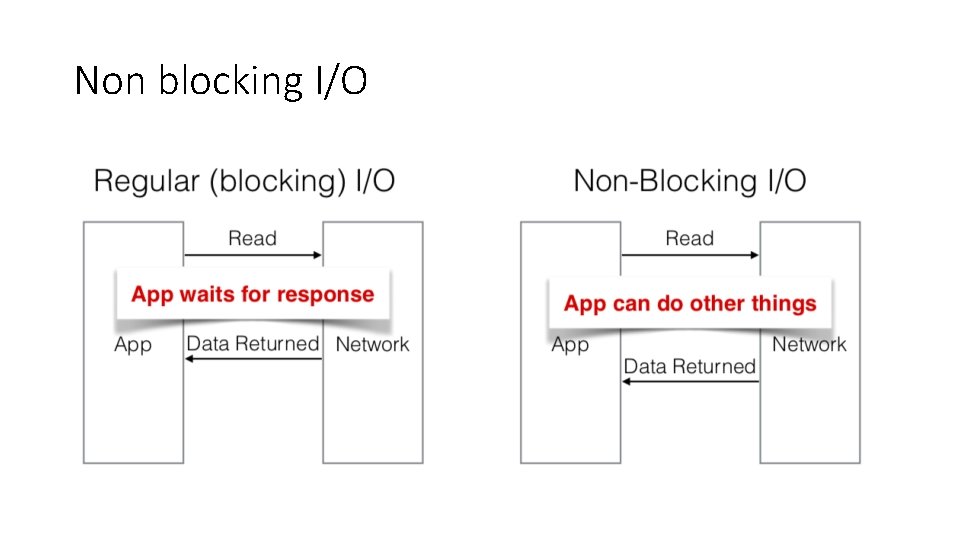
Non blocking I/O
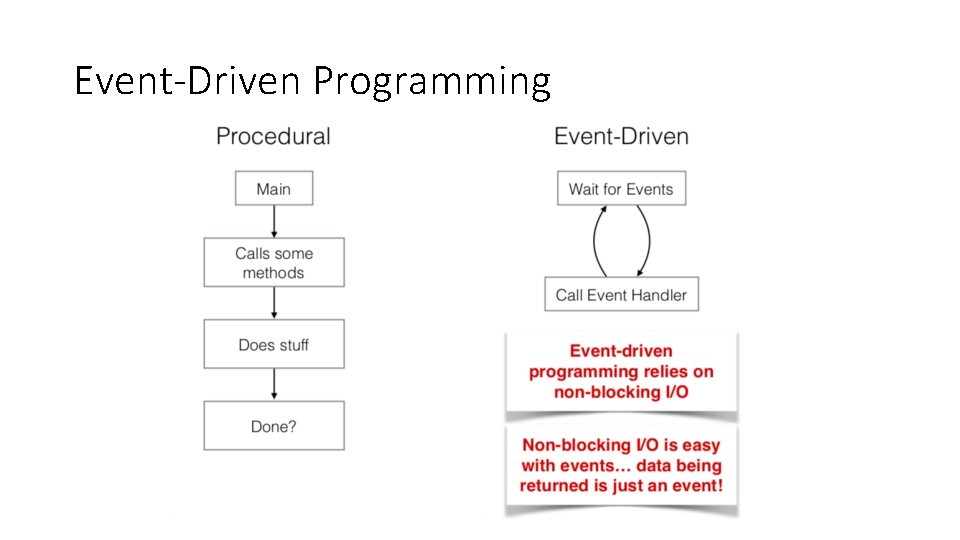
Event-Driven Programming
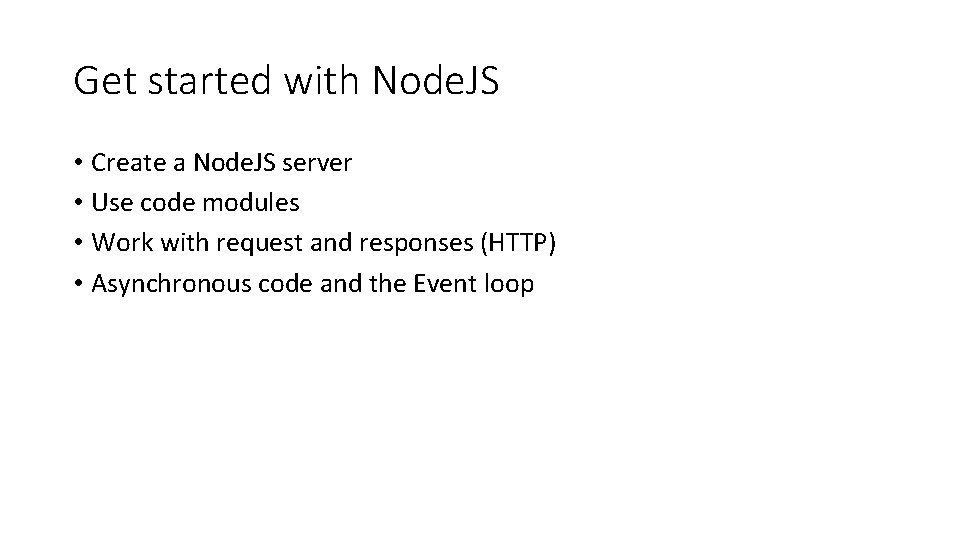
Get started with Node. JS • Create a Node. JS server • Use code modules • Work with request and responses (HTTP) • Asynchronous code and the Event loop
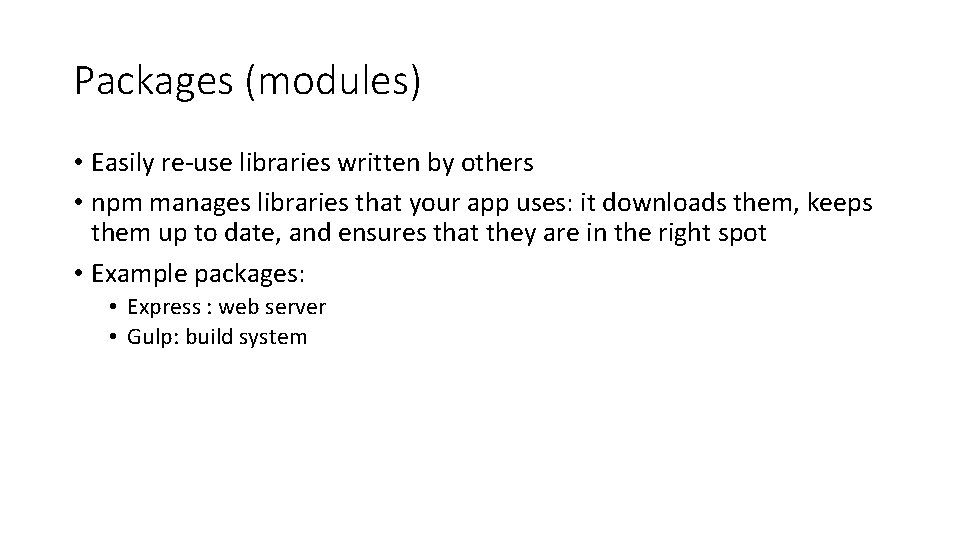
Packages (modules) • Easily re-use libraries written by others • npm manages libraries that your app uses: it downloads them, keeps them up to date, and ensures that they are in the right spot • Example packages: • Express : web server • Gulp: build system
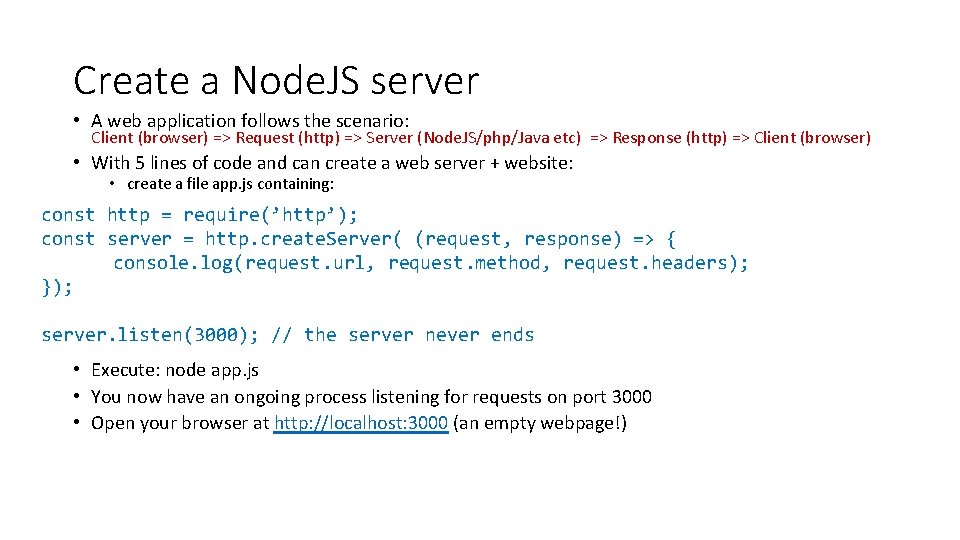
Create a Node. JS server • A web application follows the scenario: Client (browser) => Request (http) => Server (Node. JS/php/Java etc) => Response (http) => Client (browser) • With 5 lines of code and can create a web server + website: • create a file app. js containing: const http = require(’http’); const server = http. create. Server( (request, response) => { console. log(request. url, request. method, request. headers); }); server. listen(3000); // the server never ends • Execute: node app. js • You now have an ongoing process listening for requests on port 3000 • Open your browser at http: //localhost: 3000 (an empty webpage!)
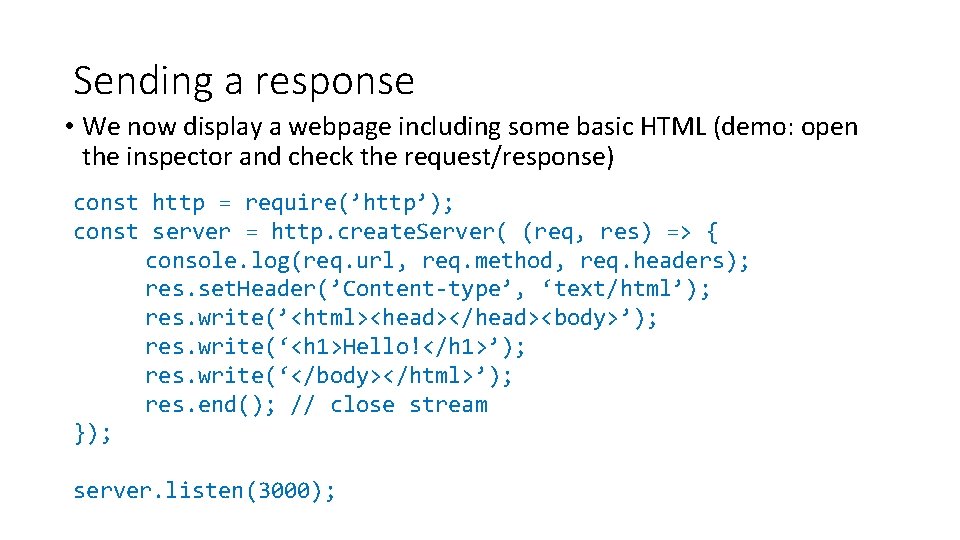
Sending a response • We now display a webpage including some basic HTML (demo: open the inspector and check the request/response) const http = require(’http’); const server = http. create. Server( (req, res) => { console. log(req. url, req. method, req. headers); res. set. Header(’Content-type’, ‘text/html’); res. write(’<html><head></head><body>’); res. write(‘<h 1>Hello!</h 1>’); res. write(‘</body></html>’); res. end(); // close stream }); server. listen(3000);
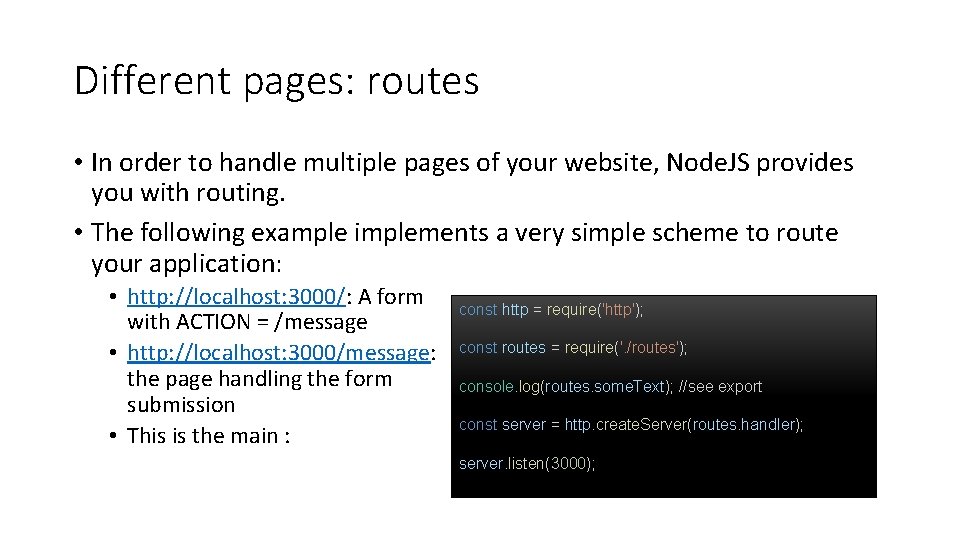
Different pages: routes • In order to handle multiple pages of your website, Node. JS provides you with routing. • The following example implements a very simple scheme to route your application: • http: //localhost: 3000/: A form with ACTION = /message • http: //localhost: 3000/message: the page handling the form submission • This is the main : const http = require('http'); const routes = require('. /routes'); console. log(routes. some. Text); //see export const server = http. create. Server(routes. handler); server. listen(3000);
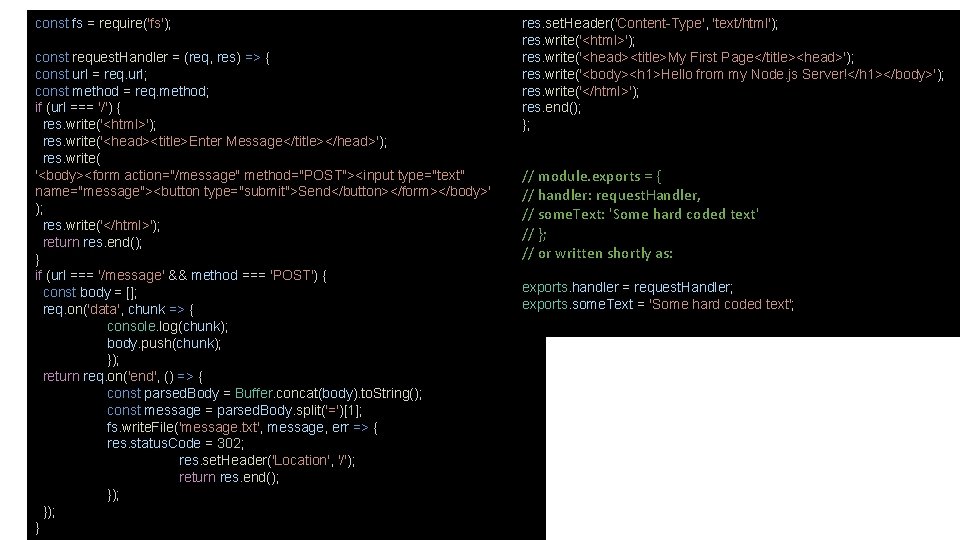
const fs = require('fs'); const request. Handler = (req, res) => { const url = req. url; const method = req. method; if (url === '/') { res. write('<html>'); res. write('<head><title>Enter Message</title></head>'); res. write( '<body><form action="/message" method="POST"><input type="text" name="message"><button type="submit">Send</button></form></body>' ); res. write('</html>'); return res. end(); } if (url === '/message' && method === 'POST') { const body = []; req. on('data', chunk => { console. log(chunk); body. push(chunk); }); return req. on('end', () => { const parsed. Body = Buffer. concat(body). to. String(); const message = parsed. Body. split('=')[1]; fs. write. File('message. txt', message, err => { res. status. Code = 302; res. set. Header('Location', '/'); return res. end(); }); } res. set. Header('Content-Type', 'text/html'); res. write('<html>'); res. write('<head><title>My First Page</title><head>'); res. write('<body><h 1>Hello from my Node. js Server!</h 1></body>'); res. write('</html>'); res. end(); }; // module. exports = { // handler: request. Handler, // some. Text: 'Some hard coded text' // }; // or written shortly as: exports. handler = request. Handler; exports. some. Text = 'Some hard coded text';
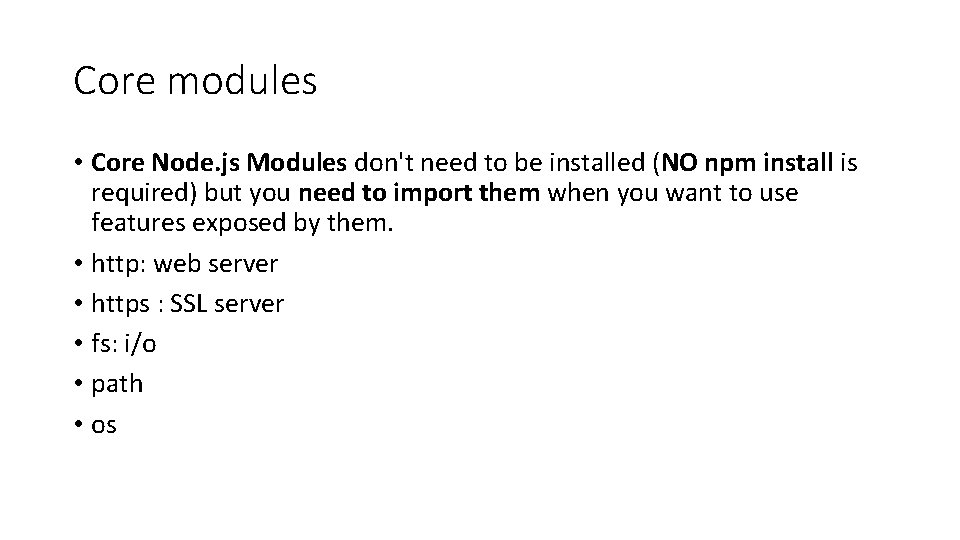
Core modules • Core Node. js Modules don't need to be installed (NO npm install is required) but you need to import them when you want to use features exposed by them. • http: web server • https : SSL server • fs: i/o • path • os
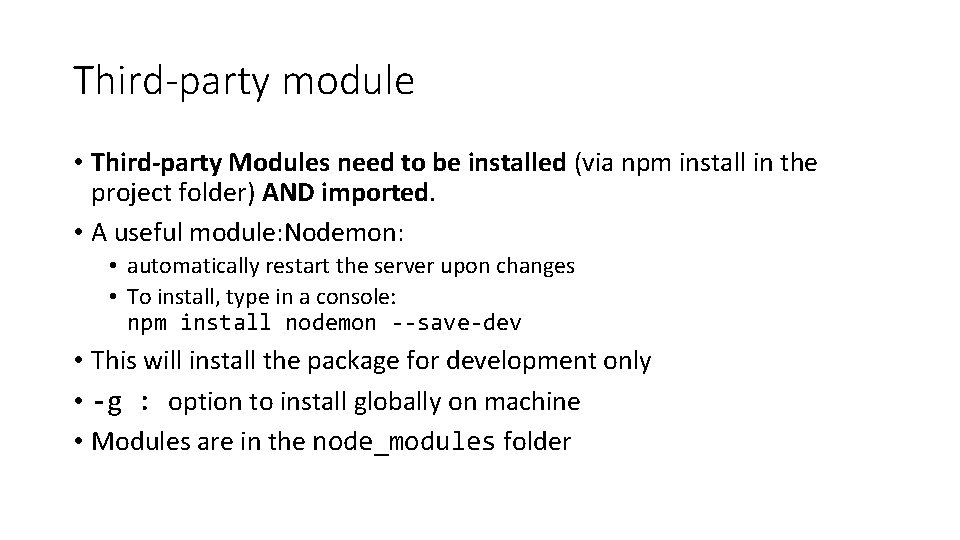
Third-party module • Third-party Modules need to be installed (via npm install in the project folder) AND imported. • A useful module: Nodemon: • automatically restart the server upon changes • To install, type in a console: npm install nodemon --save-dev • This will install the package for development only • -g : option to install globally on machine • Modules are in the node_modules folder
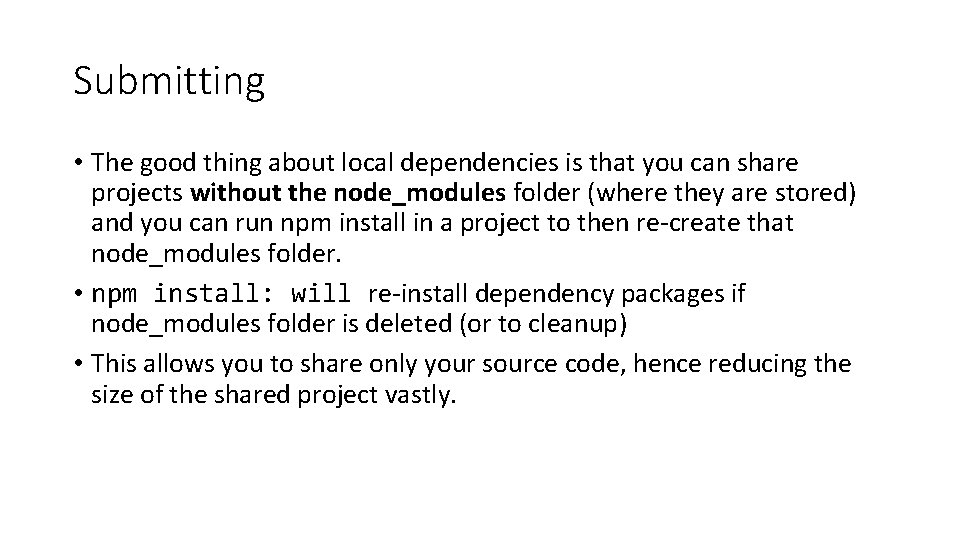
Submitting • The good thing about local dependencies is that you can share projects without the node_modules folder (where they are stored) and you can run npm install in a project to then re-create that node_modules folder. • npm install: will re-install dependency packages if node_modules folder is deleted (or to cleanup) • This allows you to share only your source code, hence reducing the size of the shared project vastly.
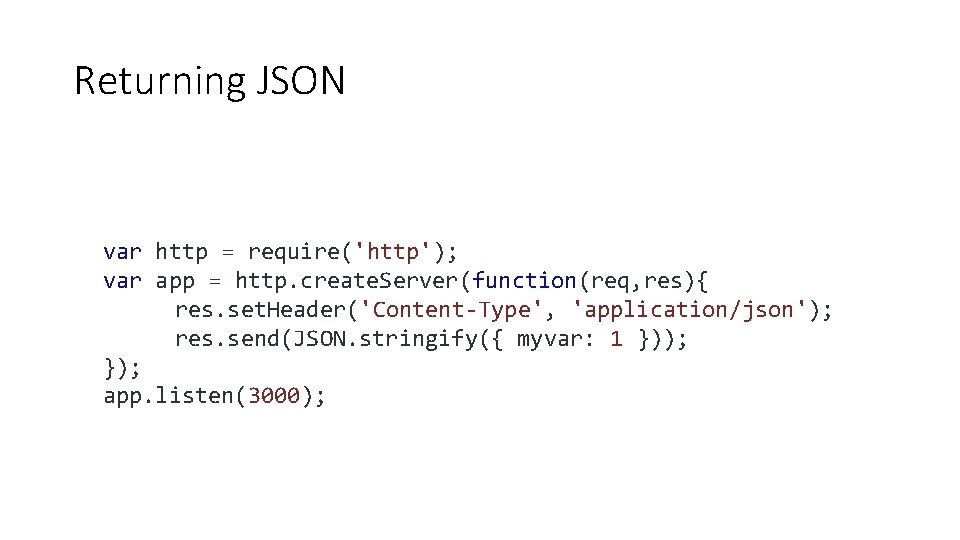
Returning JSON var http = require('http'); var app = http. create. Server(function(req, res){ res. set. Header('Content-Type', 'application/json'); res. send(JSON. stringify({ myvar: 1 })); }); app. listen(3000);
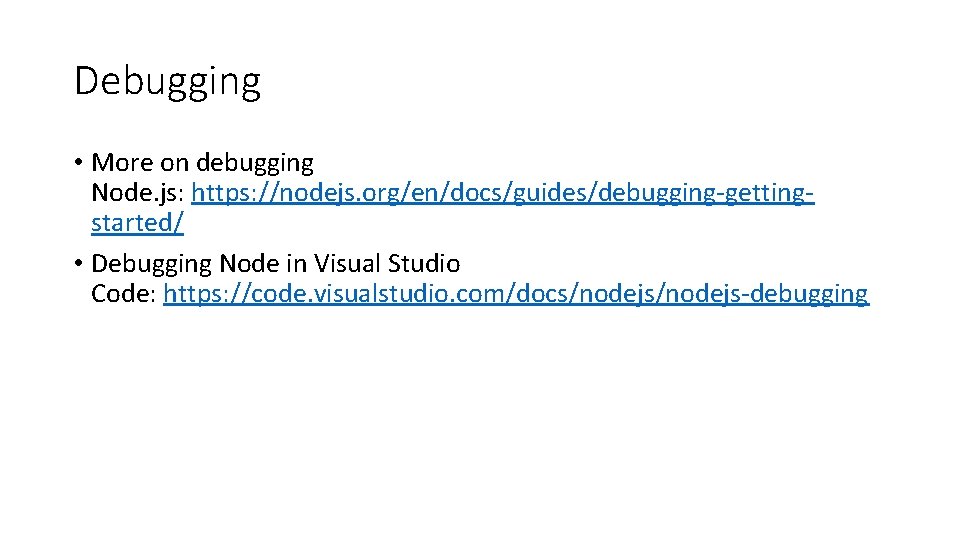
Debugging • More on debugging Node. js: https: //nodejs. org/en/docs/guides/debugging-gettingstarted/ • Debugging Node in Visual Studio Code: https: //code. visualstudio. com/docs/nodejs-debugging