Network programming network programming java net clientserver programming
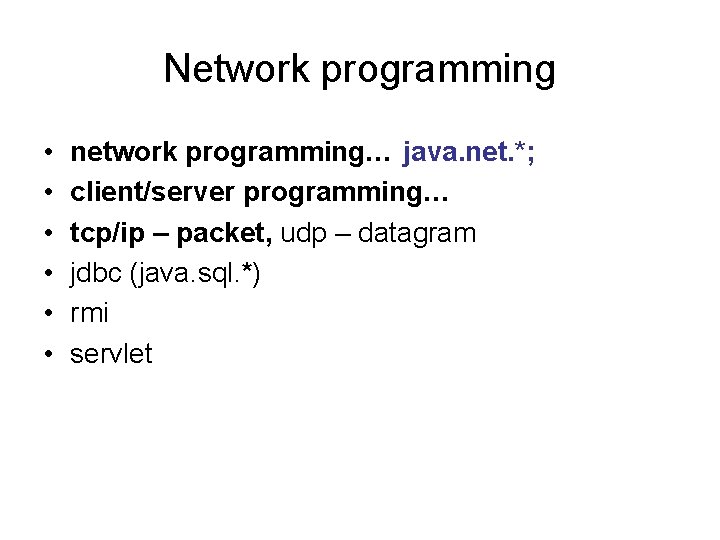
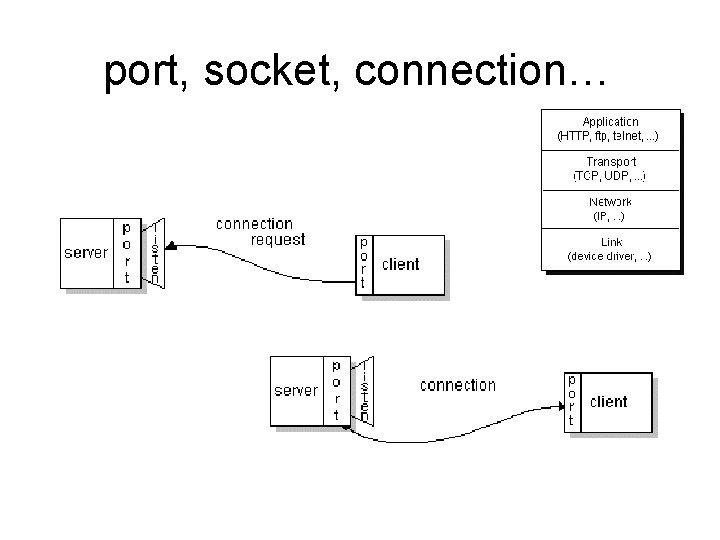
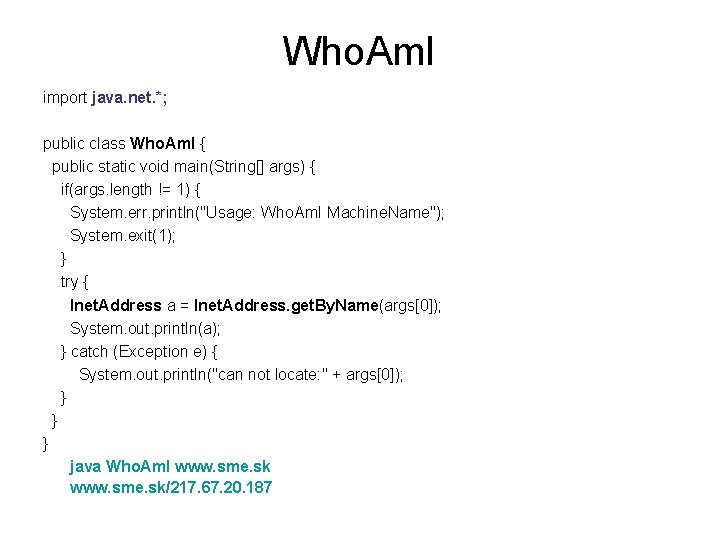
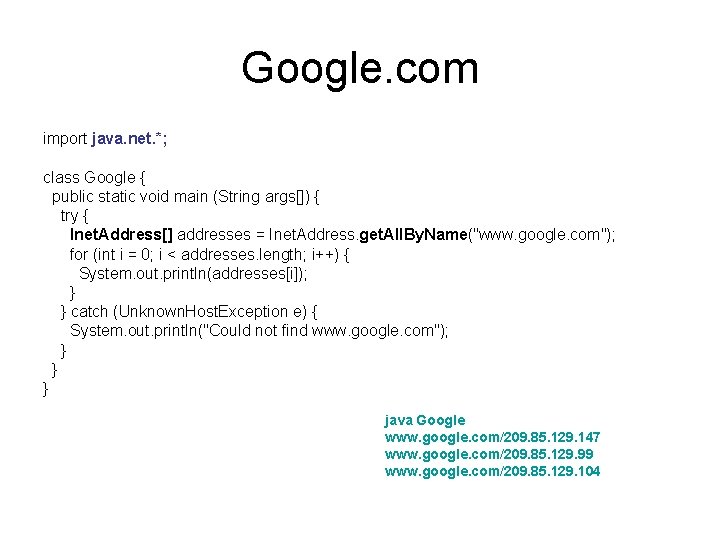
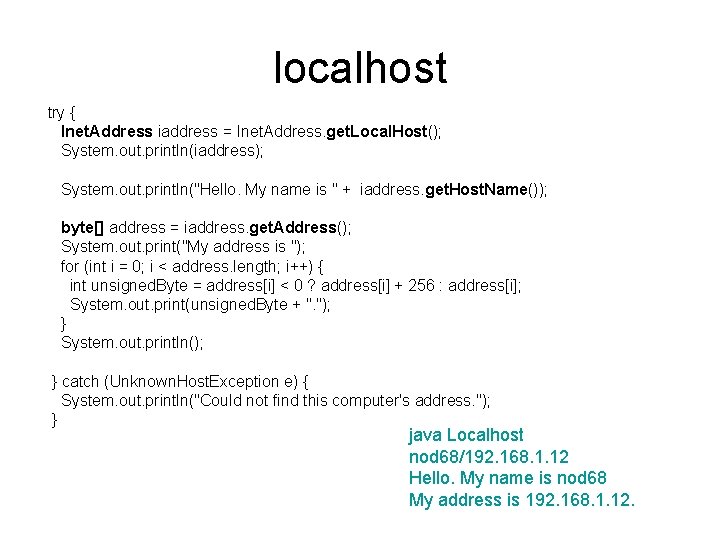
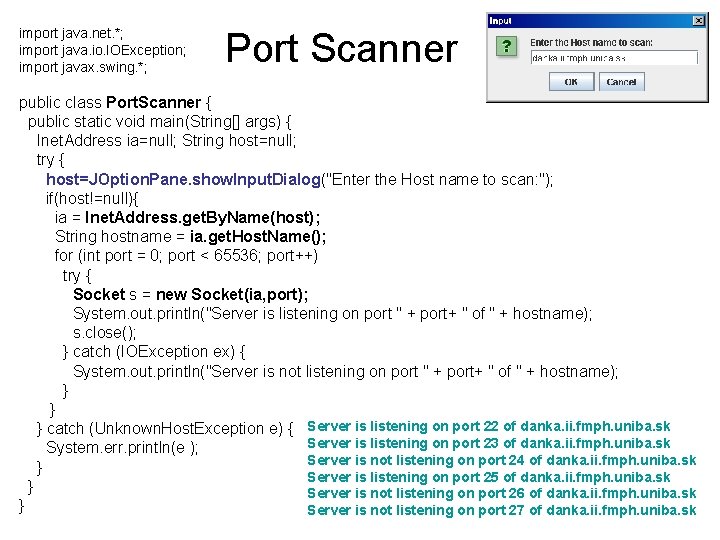
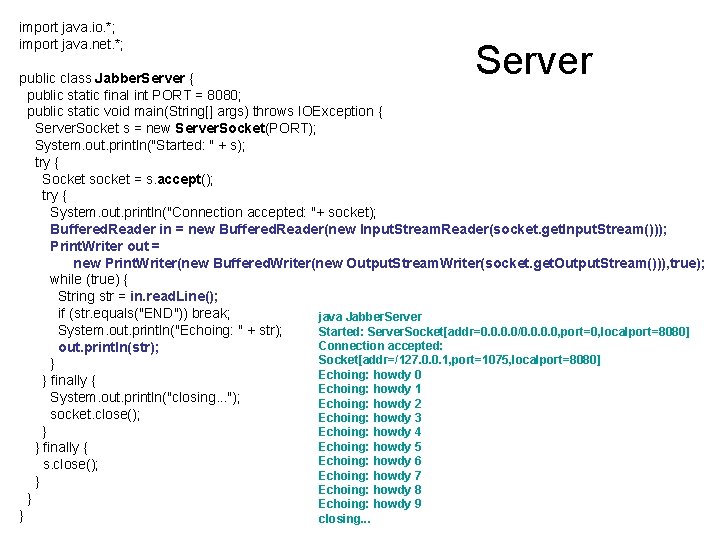
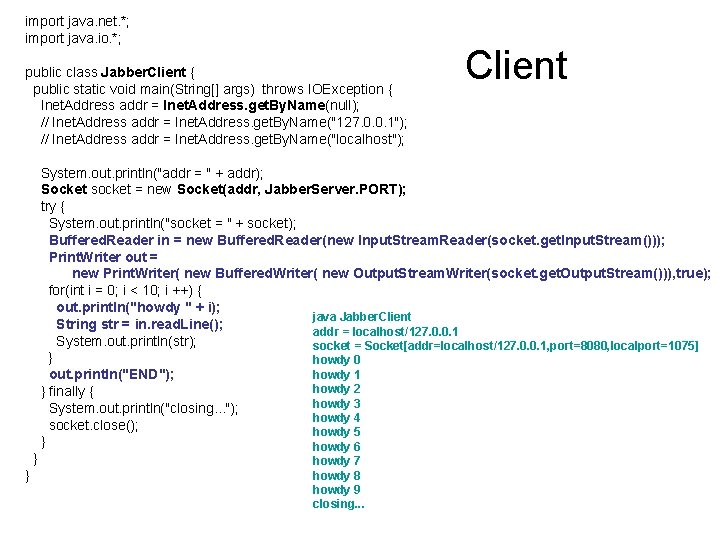
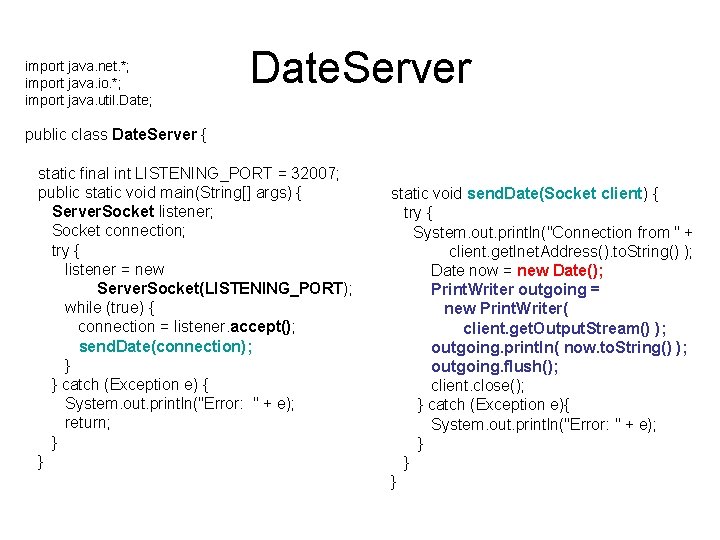
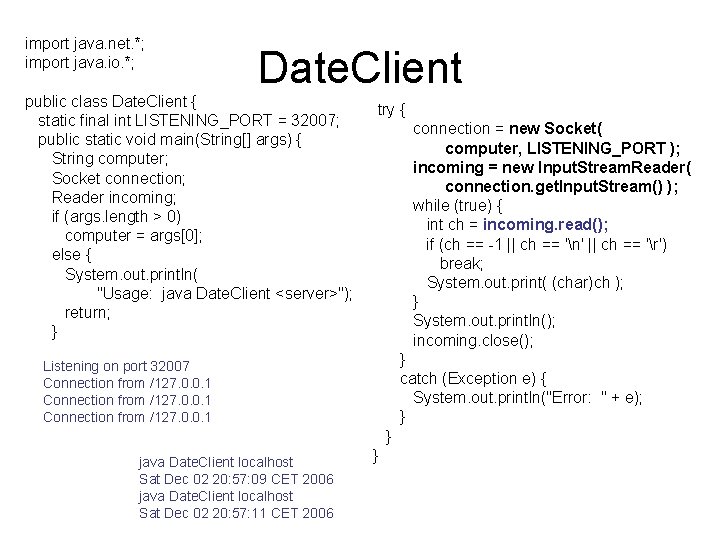
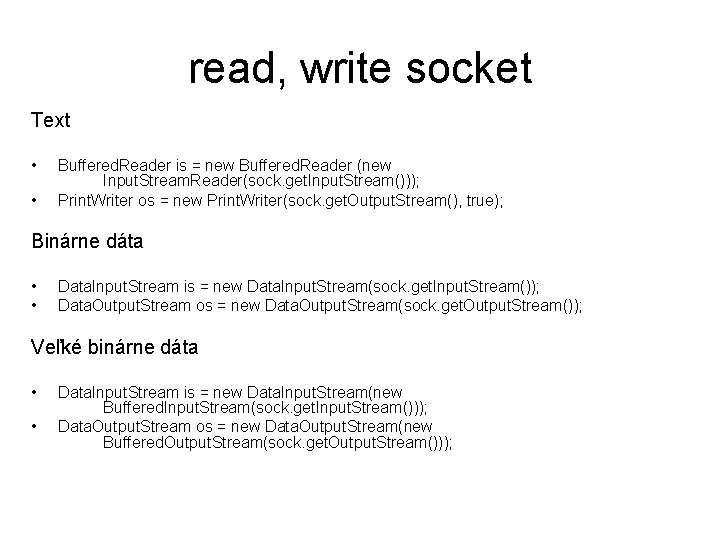
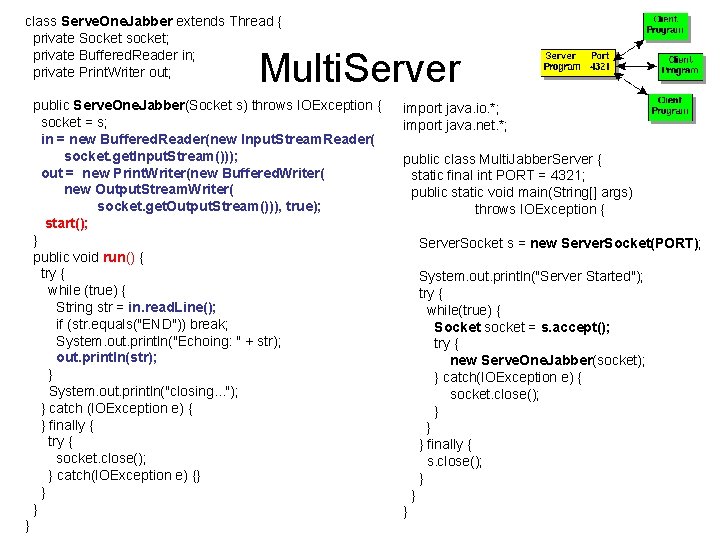
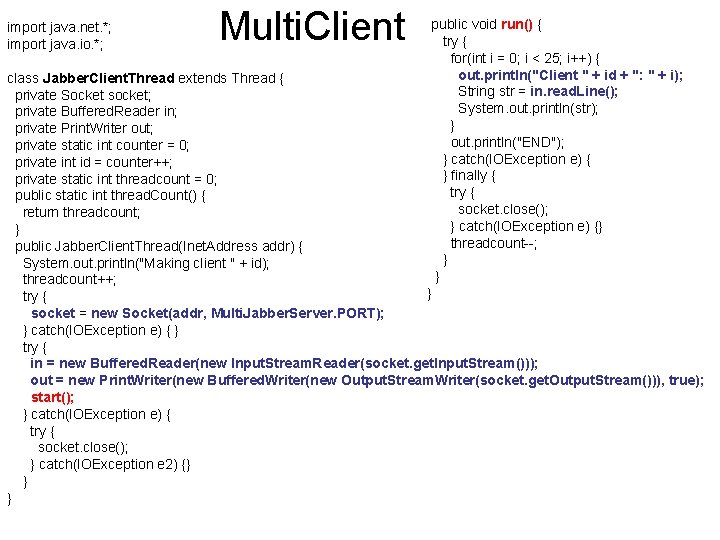
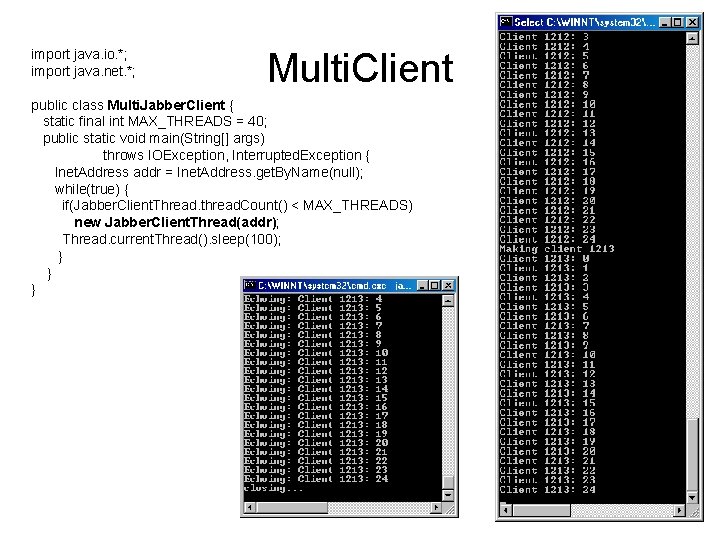
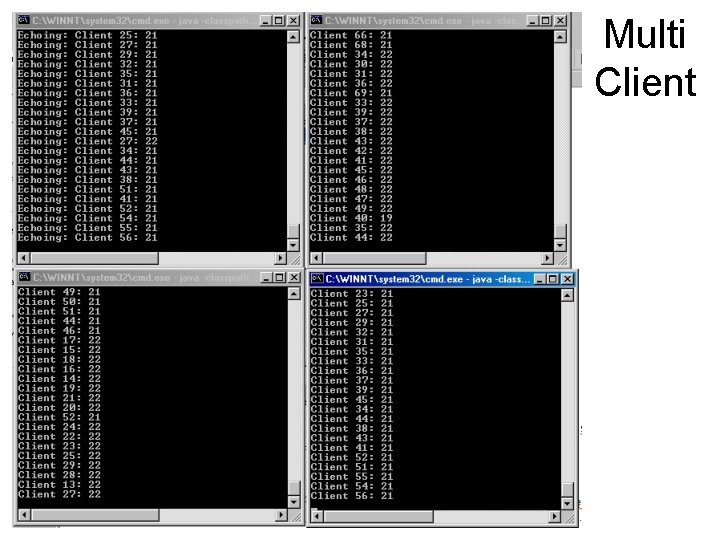
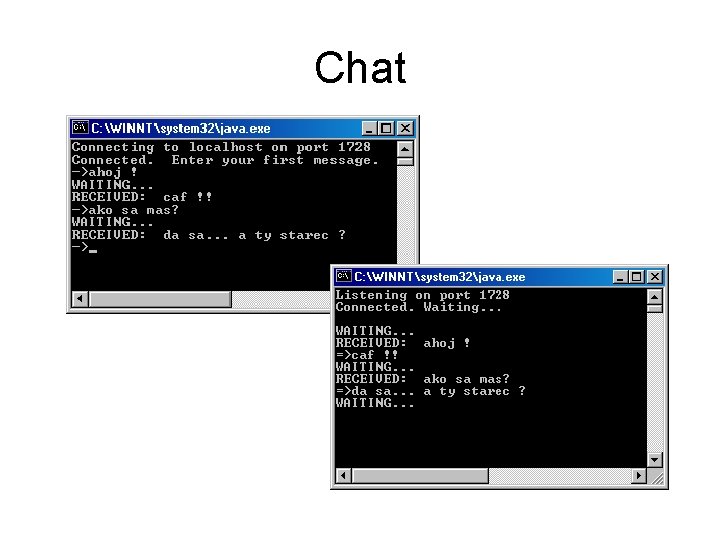
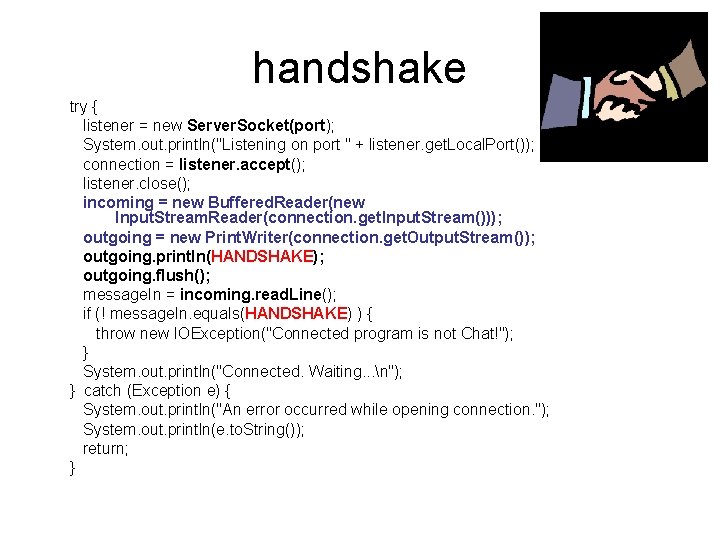
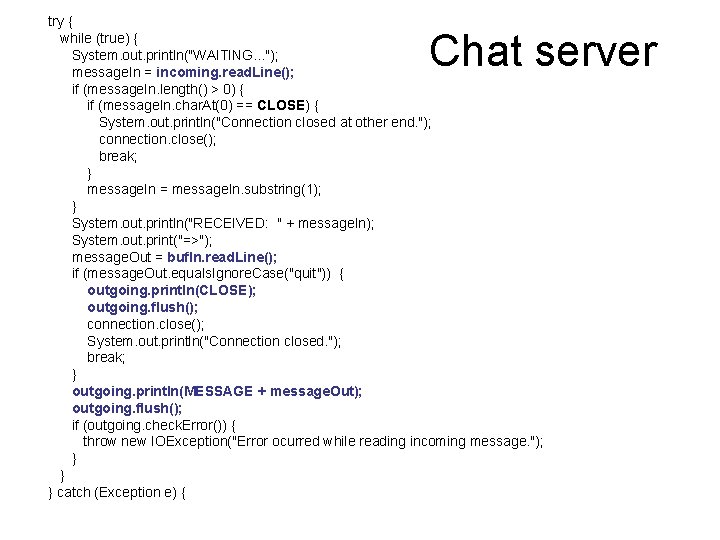
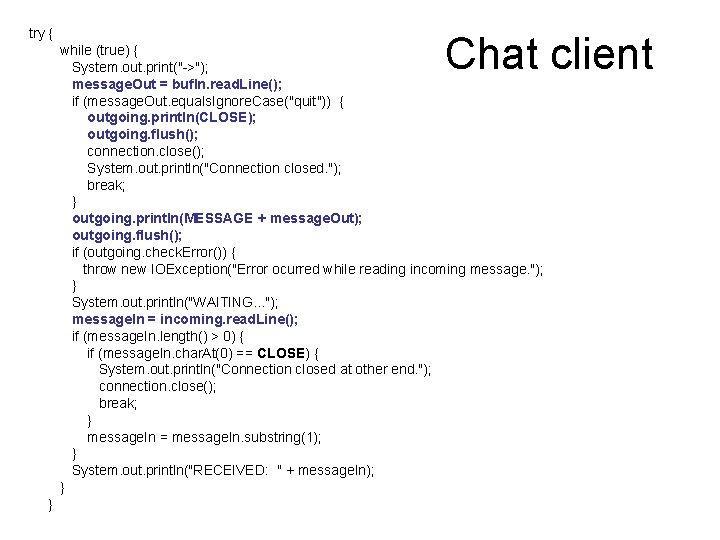
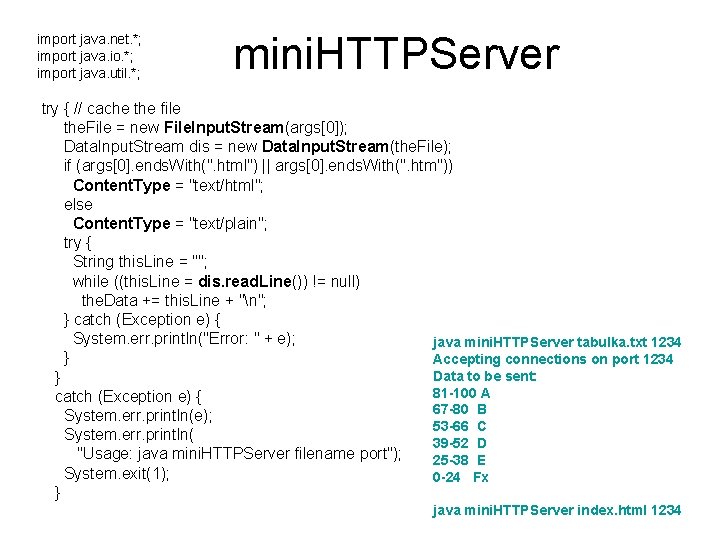
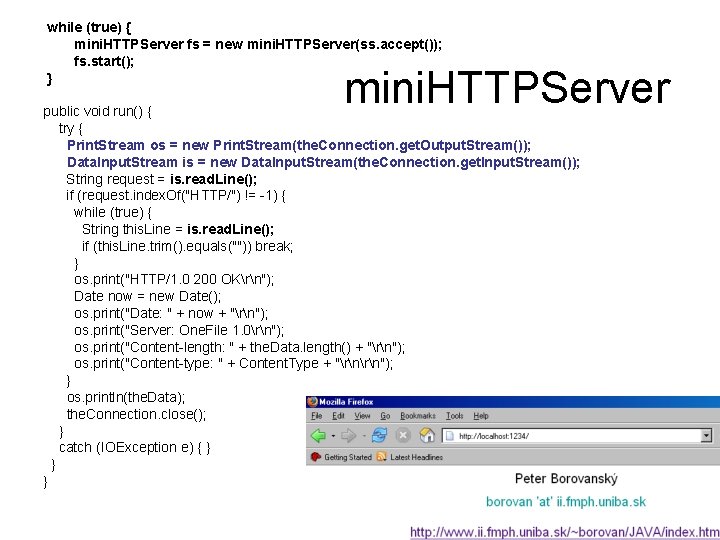
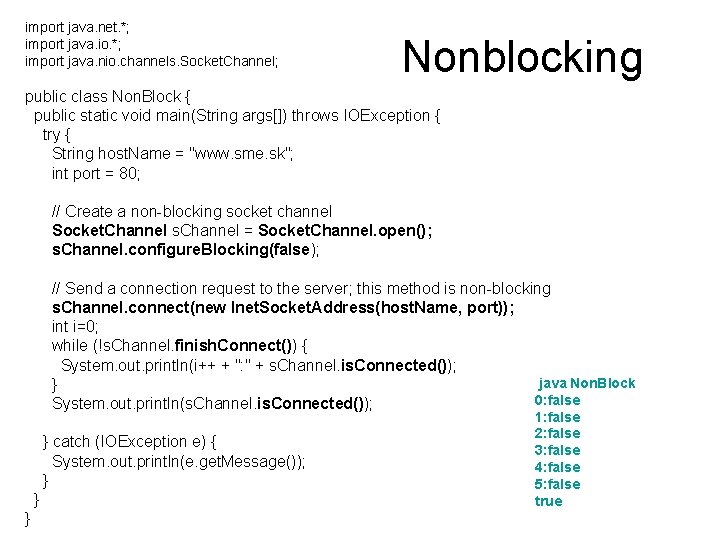
![import java. sql. *; jdbc public class Lookup { public static void main(String[] args) import java. sql. *; jdbc public class Lookup { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/28daed8a257cec7d8113d93f07d69040/image-23.jpg)
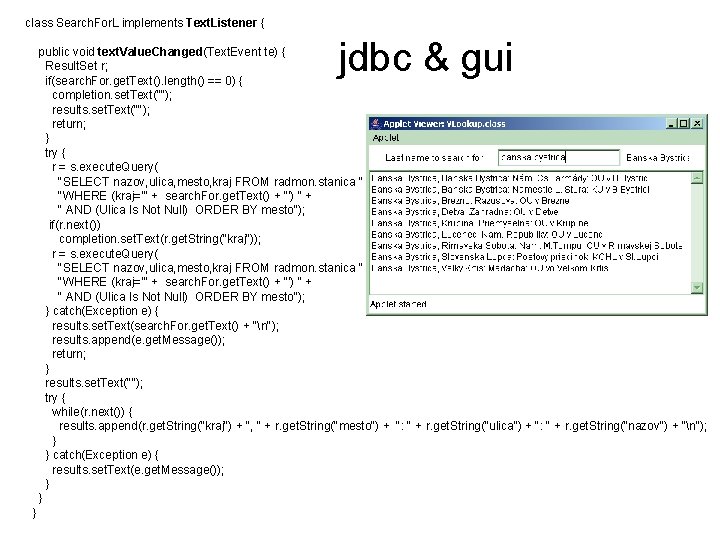
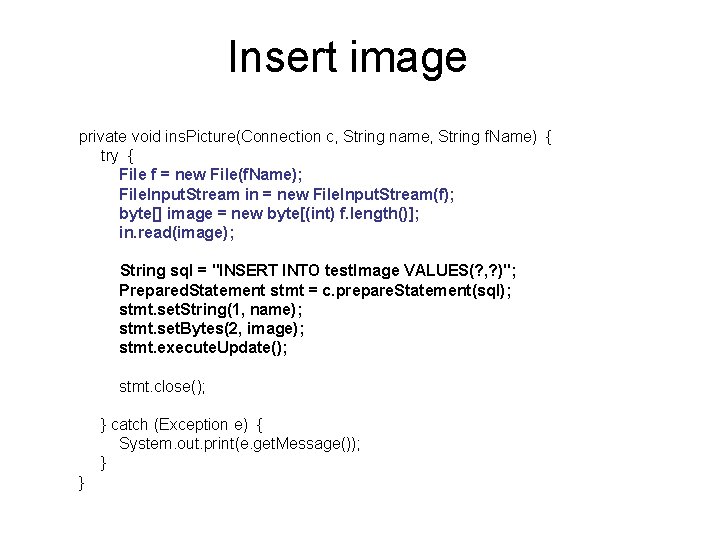
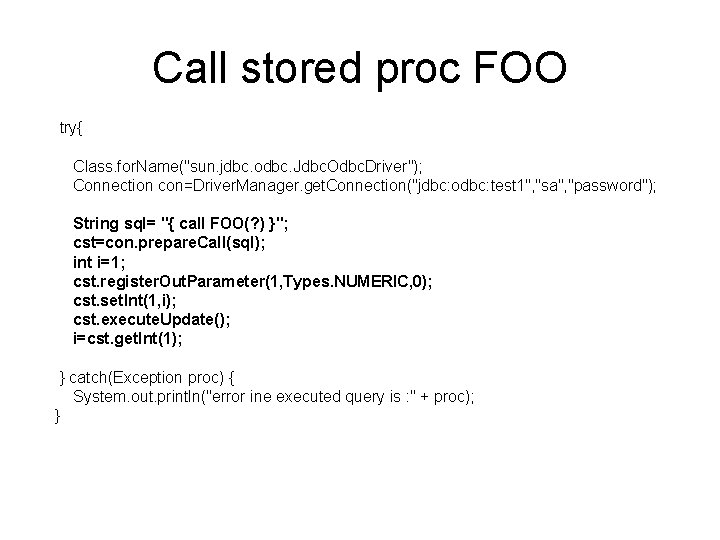
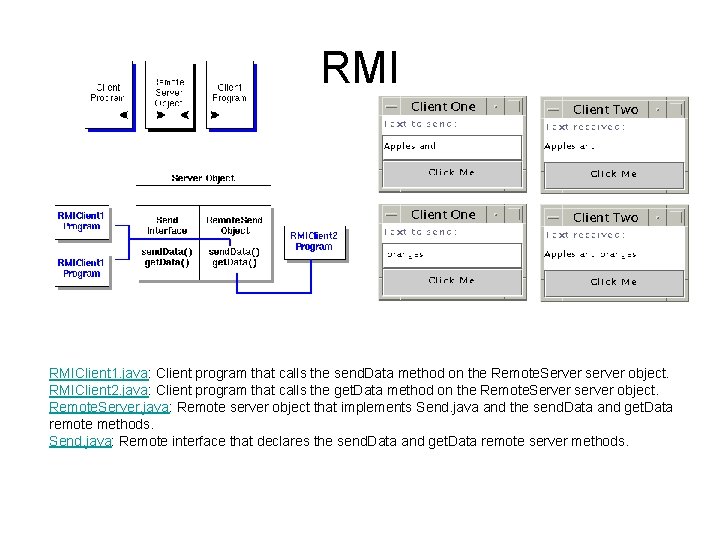
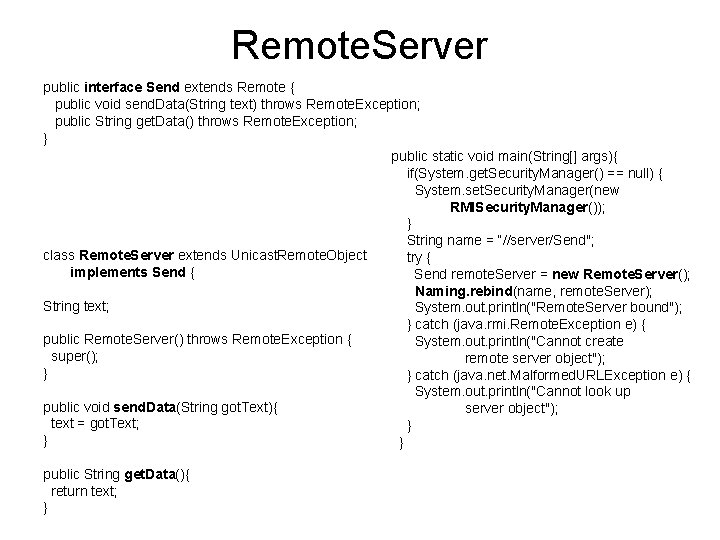
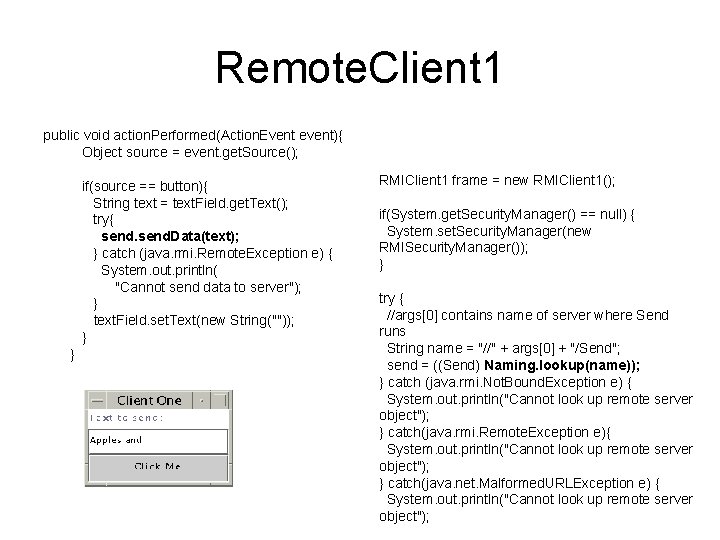
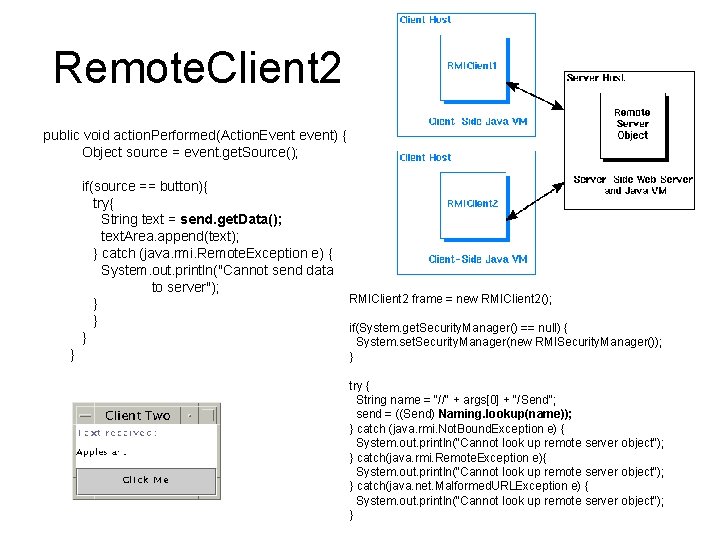
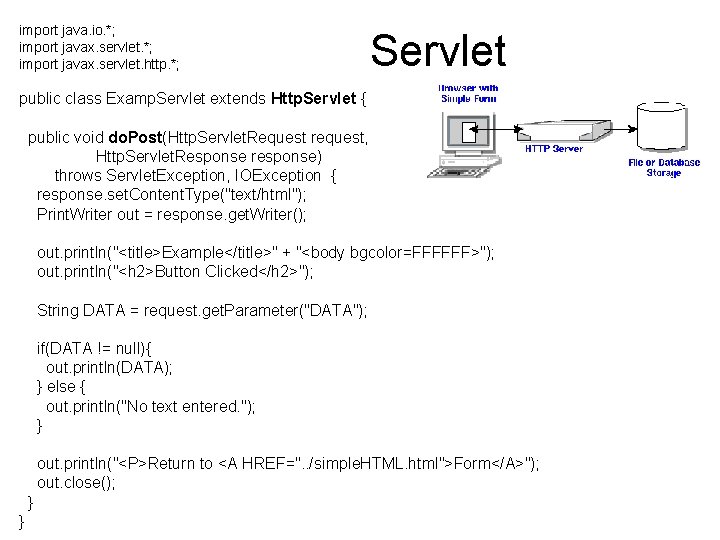
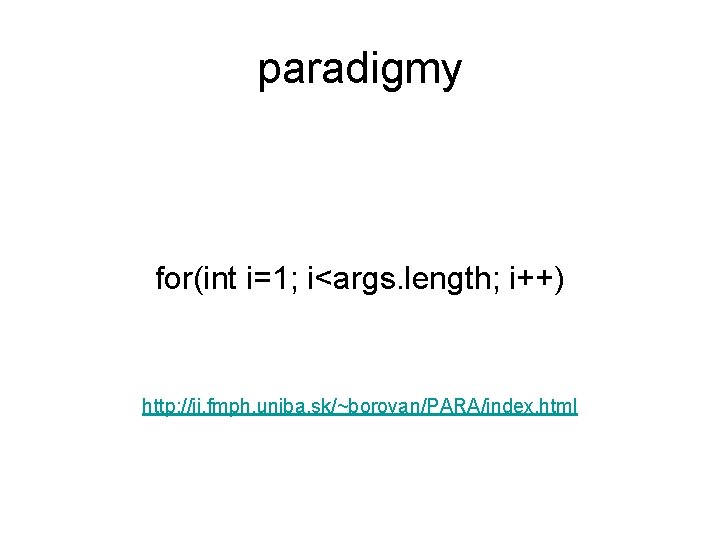
- Slides: 32
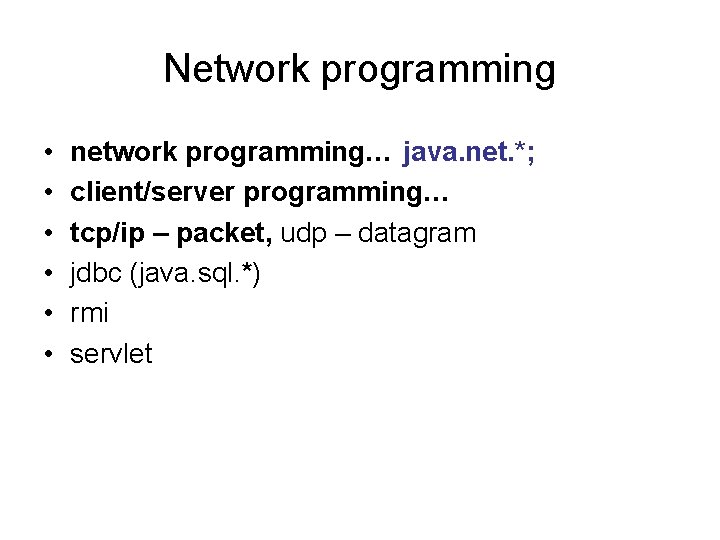
Network programming • • • network programming… java. net. *; client/server programming… tcp/ip – packet, udp – datagram jdbc (java. sql. *) rmi servlet
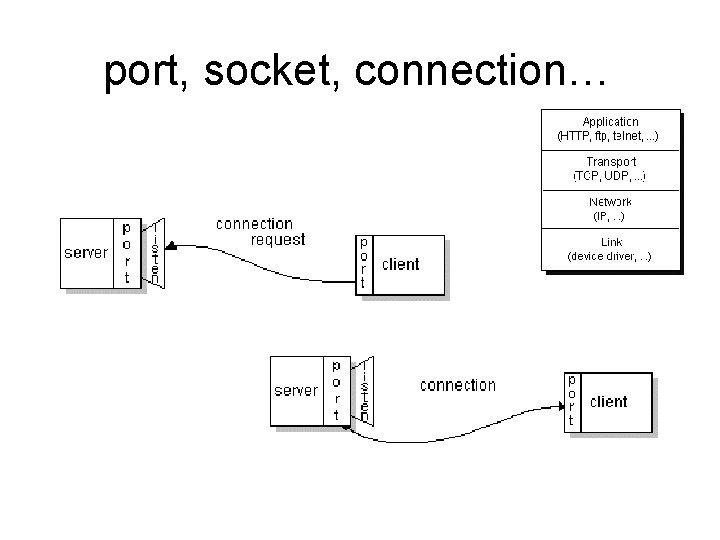
port, socket, connection…
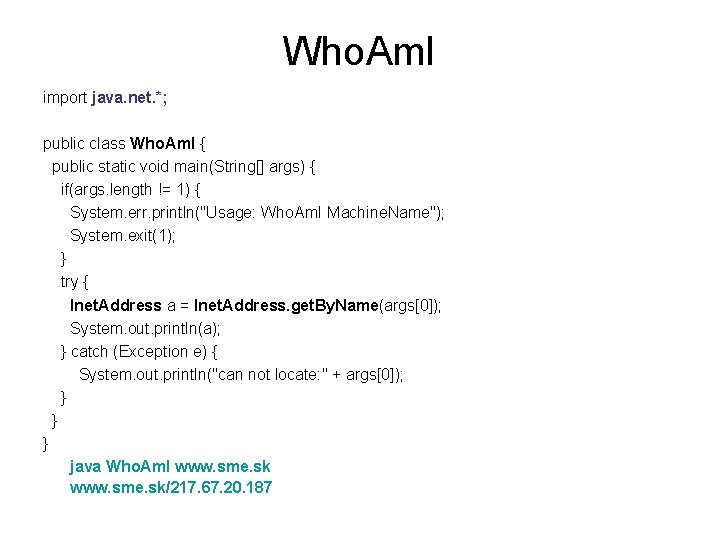
Who. Am. I import java. net. *; public class Who. Am. I { public static void main(String[] args) { if(args. length != 1) { System. err. println("Usage: Who. Am. I Machine. Name"); System. exit(1); } try { Inet. Address a = Inet. Address. get. By. Name(args[0]); System. out. println(a); } catch (Exception e) { System. out. println("can not locate: " + args[0]); } } } java Who. Am. I www. sme. sk/217. 67. 20. 187
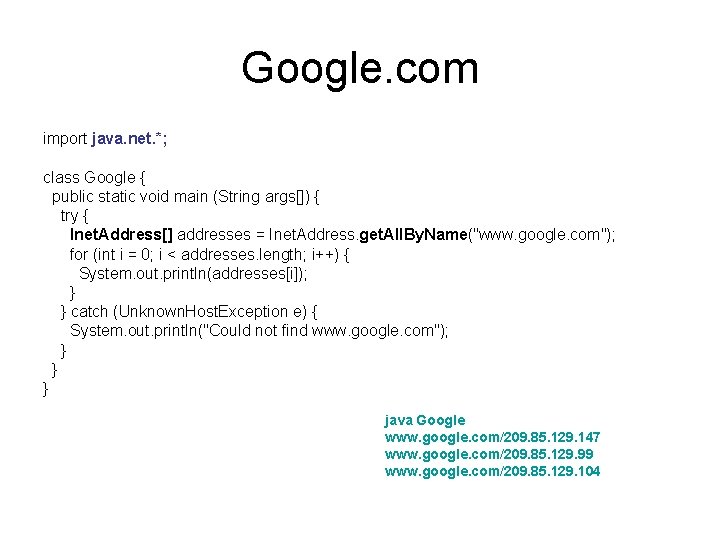
Google. com import java. net. *; class Google { public static void main (String args[]) { try { Inet. Address[] addresses = Inet. Address. get. All. By. Name("www. google. com"); for (int i = 0; i < addresses. length; i++) { System. out. println(addresses[i]); } } catch (Unknown. Host. Exception e) { System. out. println("Could not find www. google. com"); } } } java Google www. google. com/209. 85. 129. 147 www. google. com/209. 85. 129. 99 www. google. com/209. 85. 129. 104
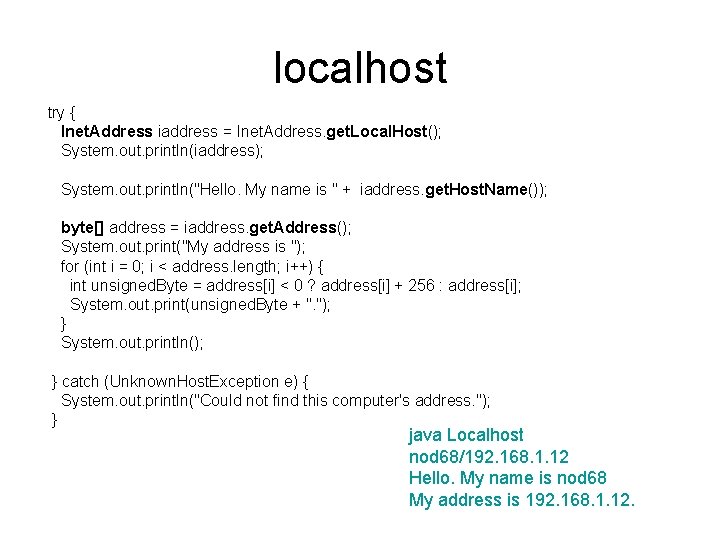
localhost try { Inet. Address iaddress = Inet. Address. get. Local. Host(); System. out. println(iaddress); System. out. println("Hello. My name is " + iaddress. get. Host. Name()); byte[] address = iaddress. get. Address(); System. out. print("My address is "); for (int i = 0; i < address. length; i++) { int unsigned. Byte = address[i] < 0 ? address[i] + 256 : address[i]; System. out. print(unsigned. Byte + ". "); } System. out. println(); } catch (Unknown. Host. Exception e) { System. out. println("Could not find this computer's address. "); } java Localhost nod 68/192. 168. 1. 12 Hello. My name is nod 68 My address is 192. 168. 1. 12.
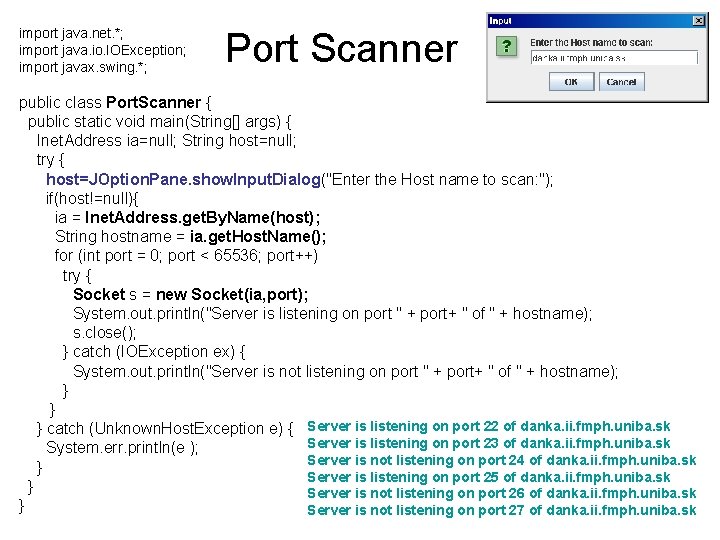
import java. net. *; import java. io. IOException; import javax. swing. *; Port Scanner public class Port. Scanner { public static void main(String[] args) { Inet. Address ia=null; String host=null; try { host=JOption. Pane. show. Input. Dialog("Enter the Host name to scan: "); if(host!=null){ ia = Inet. Address. get. By. Name(host); String hostname = ia. get. Host. Name(); for (int port = 0; port < 65536; port++) try { Socket s = new Socket(ia, port); System. out. println("Server is listening on port " + port+ " of " + hostname); s. close(); } catch (IOException ex) { System. out. println("Server is not listening on port " + port+ " of " + hostname); } } } catch (Unknown. Host. Exception e) { Server is listening on port 22 of danka. ii. fmph. uniba. sk Server is listening on port 23 of danka. ii. fmph. uniba. sk System. err. println(e ); Server is not listening on port 24 of danka. ii. fmph. uniba. sk } Server is listening on port 25 of danka. ii. fmph. uniba. sk } Server is not listening on port 26 of danka. ii. fmph. uniba. sk } Server is not listening on port 27 of danka. ii. fmph. uniba. sk
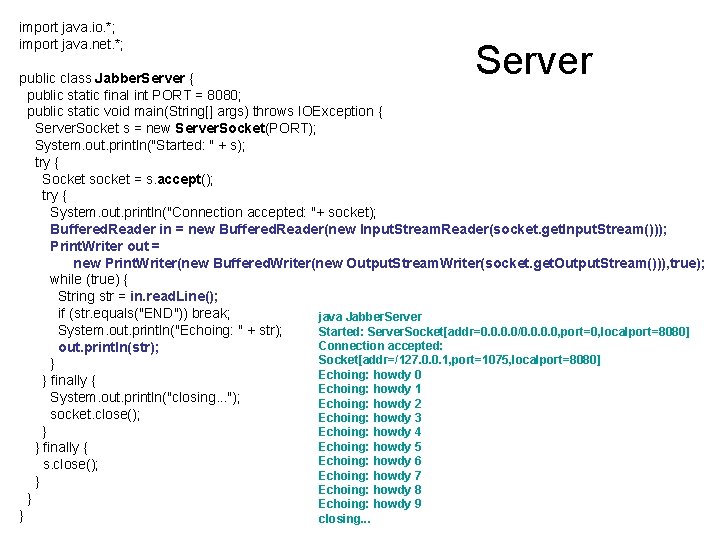
import java. io. *; import java. net. *; Server public class Jabber. Server { public static final int PORT = 8080; public static void main(String[] args) throws IOException { Server. Socket s = new Server. Socket(PORT); System. out. println("Started: " + s); try { Socket socket = s. accept(); try { System. out. println("Connection accepted: "+ socket); Buffered. Reader in = new Buffered. Reader(new Input. Stream. Reader(socket. get. Input. Stream())); Print. Writer out = new Print. Writer(new Buffered. Writer(new Output. Stream. Writer(socket. get. Output. Stream())), true); while (true) { String str = in. read. Line(); if (str. equals("END")) break; java Jabber. Server System. out. println("Echoing: " + str); Started: Server. Socket[addr=0. 0/0. 0, port=0, localport=8080] Connection accepted: out. println(str); Socket[addr=/127. 0. 0. 1, port=1075, localport=8080] } Echoing: howdy 0 } finally { Echoing: howdy 1 System. out. println("closing. . . "); Echoing: howdy 2 socket. close(); Echoing: howdy 3 } Echoing: howdy 4 Echoing: howdy 5 } finally { Echoing: howdy 6 s. close(); Echoing: howdy 7 } Echoing: howdy 8 } Echoing: howdy 9 } closing. . .
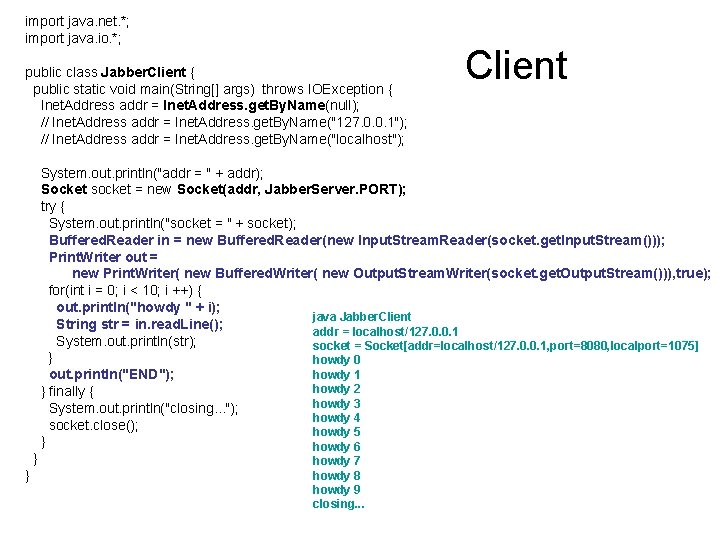
import java. net. *; import java. io. *; public class Jabber. Client { public static void main(String[] args) throws IOException { Inet. Address addr = Inet. Address. get. By. Name(null); // Inet. Address addr = Inet. Address. get. By. Name("127. 0. 0. 1"); // Inet. Address addr = Inet. Address. get. By. Name("localhost"); } } Client System. out. println("addr = " + addr); Socket socket = new Socket(addr, Jabber. Server. PORT); try { System. out. println("socket = " + socket); Buffered. Reader in = new Buffered. Reader(new Input. Stream. Reader(socket. get. Input. Stream())); Print. Writer out = new Print. Writer( new Buffered. Writer( new Output. Stream. Writer(socket. get. Output. Stream())), true); for(int i = 0; i < 10; i ++) { out. println("howdy " + i); java Jabber. Client String str = in. read. Line(); addr = localhost/127. 0. 0. 1 System. out. println(str); socket = Socket[addr=localhost/127. 0. 0. 1, port=8080, localport=1075] } howdy 0 howdy 1 out. println("END"); howdy 2 } finally { howdy 3 System. out. println("closing. . . "); howdy 4 socket. close(); howdy 5 } howdy 6 howdy 7 howdy 8 howdy 9 closing. . .
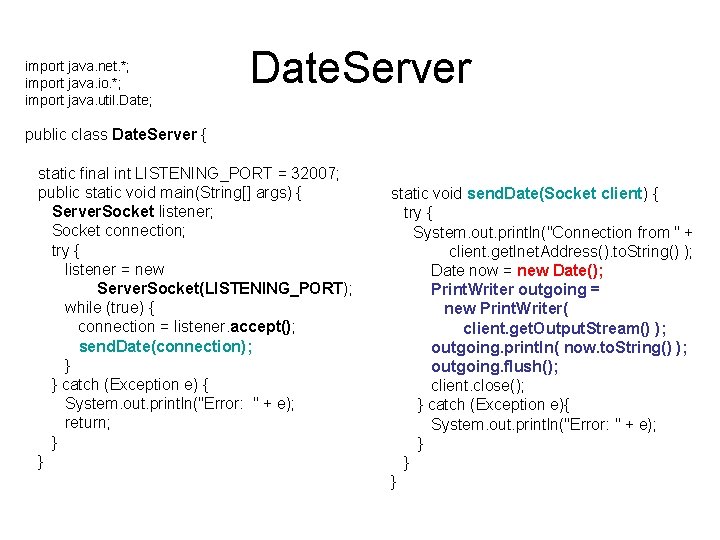
import java. net. *; import java. io. *; import java. util. Date; Date. Server public class Date. Server { static final int LISTENING_PORT = 32007; public static void main(String[] args) { Server. Socket listener; Socket connection; try { listener = new Server. Socket(LISTENING_PORT); while (true) { connection = listener. accept(); send. Date(connection); } } catch (Exception e) { System. out. println("Error: " + e); return; } } static void send. Date(Socket client) { try { System. out. println("Connection from " + client. get. Inet. Address(). to. String() ); Date now = new Date(); Print. Writer outgoing = new Print. Writer( client. get. Output. Stream() ); outgoing. println( now. to. String() ); outgoing. flush(); client. close(); } catch (Exception e){ System. out. println("Error: " + e); } } }
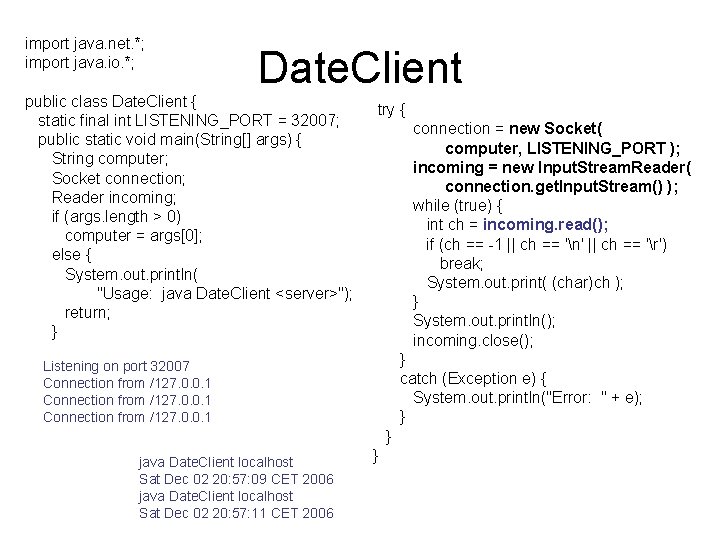
import java. net. *; import java. io. *; Date. Client public class Date. Client { static final int LISTENING_PORT = 32007; public static void main(String[] args) { String computer; Socket connection; Reader incoming; if (args. length > 0) computer = args[0]; else { System. out. println( "Usage: java Date. Client <server>"); return; } try { connection = new Socket( computer, LISTENING_PORT ); incoming = new Input. Stream. Reader( connection. get. Input. Stream() ); while (true) { int ch = incoming. read(); if (ch == -1 || ch == 'n' || ch == 'r') break; System. out. print( (char)ch ); } System. out. println(); incoming. close(); } catch (Exception e) { System. out. println("Error: " + e); } Listening on port 32007 Connection from /127. 0. 0. 1 } java Date. Client localhost Sat Dec 02 20: 57: 09 CET 2006 java Date. Client localhost Sat Dec 02 20: 57: 11 CET 2006 }
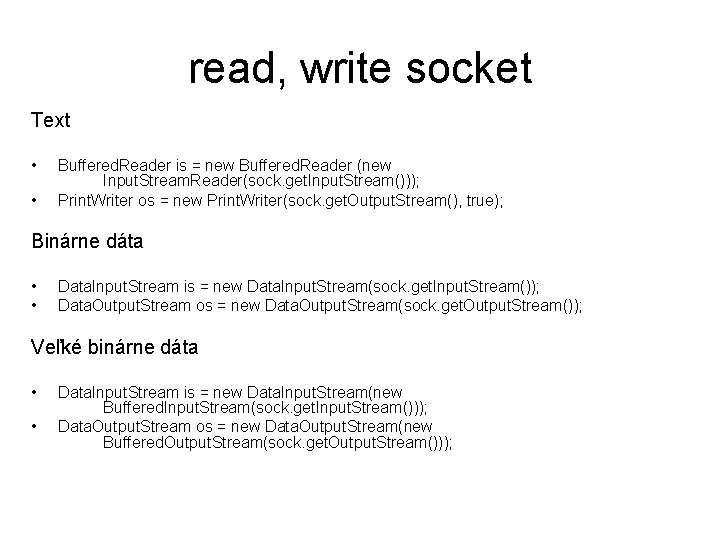
read, write socket Text • • Buffered. Reader is = new Buffered. Reader (new Input. Stream. Reader(sock. get. Input. Stream())); Print. Writer os = new Print. Writer(sock. get. Output. Stream(), true); Binárne dáta • • Data. Input. Stream is = new Data. Input. Stream(sock. get. Input. Stream()); Data. Output. Stream os = new Data. Output. Stream(sock. get. Output. Stream()); Veľké binárne dáta • • Data. Input. Stream is = new Data. Input. Stream(new Buffered. Input. Stream(sock. get. Input. Stream())); Data. Output. Stream os = new Data. Output. Stream(new Buffered. Output. Stream(sock. get. Output. Stream()));
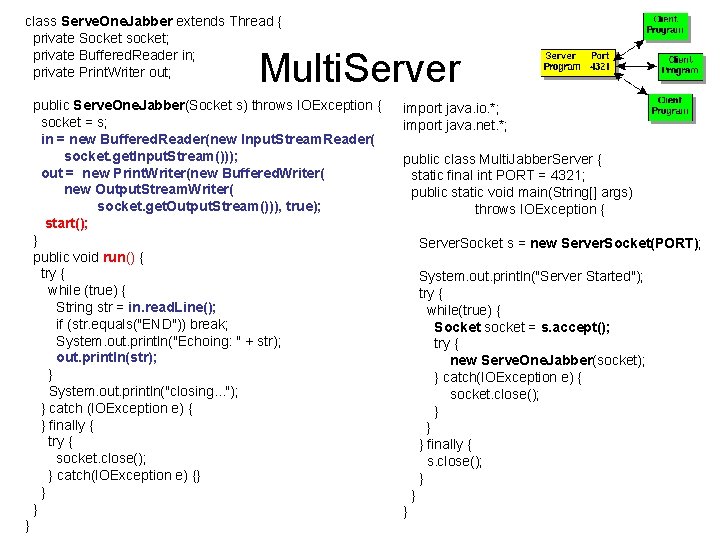
class Serve. One. Jabber extends Thread { private Socket socket; private Buffered. Reader in; private Print. Writer out; Multi. Server public Serve. One. Jabber(Socket s) throws IOException { socket = s; in = new Buffered. Reader(new Input. Stream. Reader( socket. get. Input. Stream())); out = new Print. Writer(new Buffered. Writer( new Output. Stream. Writer( socket. get. Output. Stream())), true); start(); } public void run() { try { while (true) { String str = in. read. Line(); if (str. equals("END")) break; System. out. println("Echoing: " + str); out. println(str); } System. out. println("closing. . . "); } catch (IOException e) { } finally { try { socket. close(); } catch(IOException e) {} } import java. io. *; import java. net. *; public class Multi. Jabber. Server { static final int PORT = 4321; public static void main(String[] args) throws IOException { Server. Socket s = new Server. Socket(PORT); System. out. println("Server Started"); try { while(true) { Socket socket = s. accept(); try { new Serve. One. Jabber(socket); } catch(IOException e) { socket. close(); } } } finally { s. close(); } } }
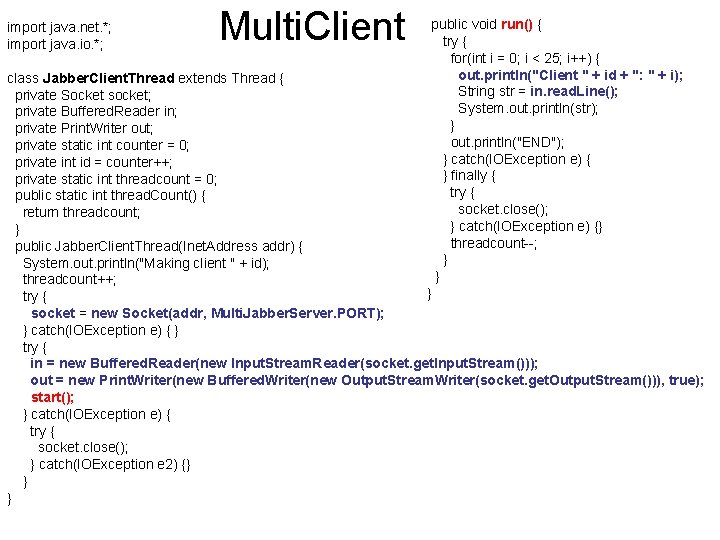
import java. net. *; import java. io. *; Multi. Client public void run() { try { for(int i = 0; i < 25; i++) { out. println("Client " + id + ": " + i); String str = in. read. Line(); System. out. println(str); } out. println("END"); } catch(IOException e) { } finally { try { socket. close(); } catch(IOException e) {} threadcount--; } } } class Jabber. Client. Thread extends Thread { private Socket socket; private Buffered. Reader in; private Print. Writer out; private static int counter = 0; private int id = counter++; private static int threadcount = 0; public static int thread. Count() { return threadcount; } public Jabber. Client. Thread(Inet. Address addr) { System. out. println("Making client " + id); threadcount++; try { socket = new Socket(addr, Multi. Jabber. Server. PORT); } catch(IOException e) { } try { in = new Buffered. Reader(new Input. Stream. Reader(socket. get. Input. Stream())); out = new Print. Writer(new Buffered. Writer(new Output. Stream. Writer(socket. get. Output. Stream())), true); start(); } catch(IOException e) { try { socket. close(); } catch(IOException e 2) {} } }
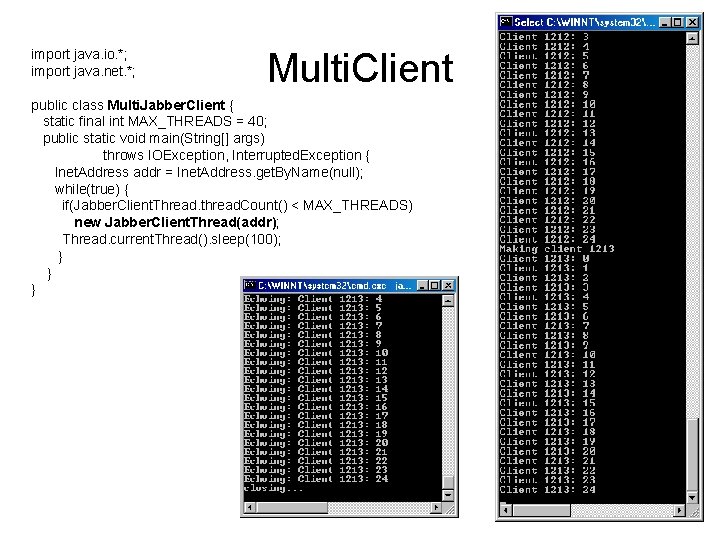
import java. io. *; import java. net. *; Multi. Client public class Multi. Jabber. Client { static final int MAX_THREADS = 40; public static void main(String[] args) throws IOException, Interrupted. Exception { Inet. Address addr = Inet. Address. get. By. Name(null); while(true) { if(Jabber. Client. Thread. thread. Count() < MAX_THREADS) new Jabber. Client. Thread(addr); Thread. current. Thread(). sleep(100); } } }
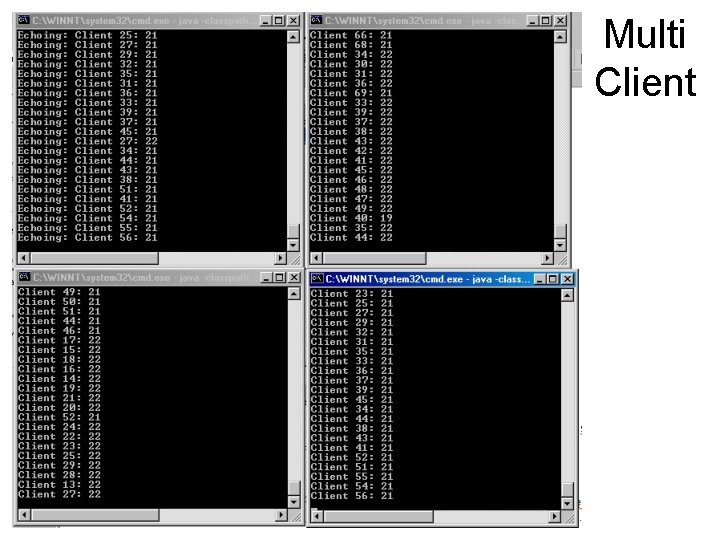
Multi Client
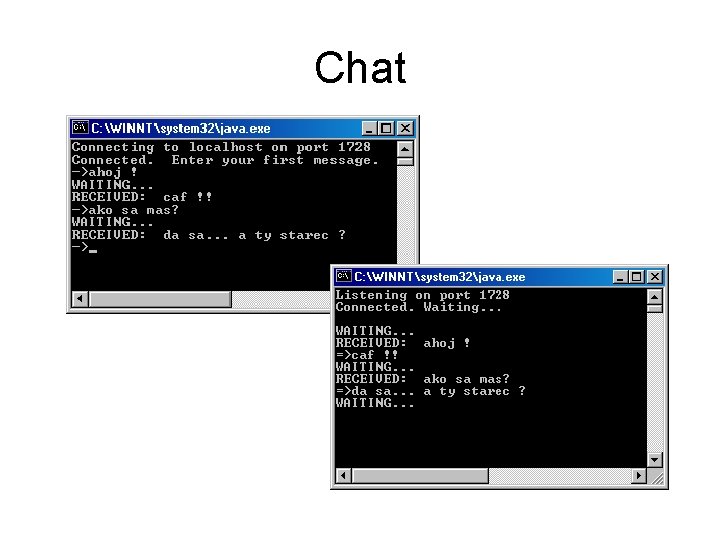
Chat
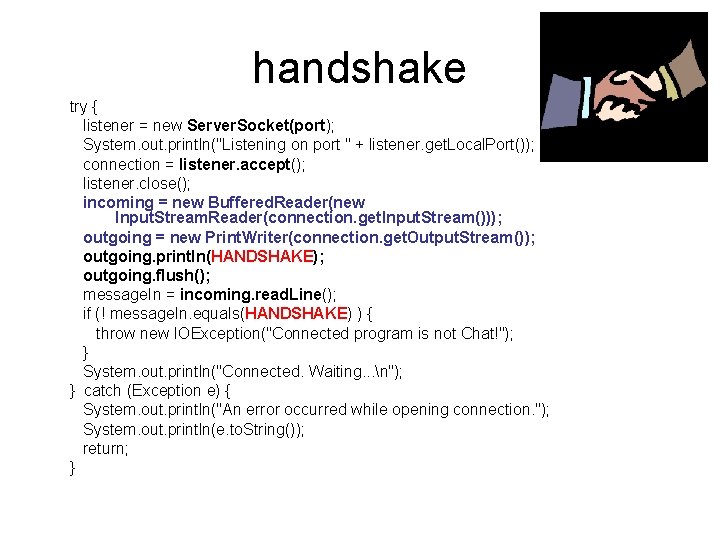
handshake try { listener = new Server. Socket(port); System. out. println("Listening on port " + listener. get. Local. Port()); connection = listener. accept(); listener. close(); incoming = new Buffered. Reader(new Input. Stream. Reader(connection. get. Input. Stream())); outgoing = new Print. Writer(connection. get. Output. Stream()); outgoing. println(HANDSHAKE); outgoing. flush(); message. In = incoming. read. Line(); if (! message. In. equals(HANDSHAKE) ) { throw new IOException("Connected program is not Chat!"); } System. out. println("Connected. Waiting. . . n"); } catch (Exception e) { System. out. println("An error occurred while opening connection. "); System. out. println(e. to. String()); return; }
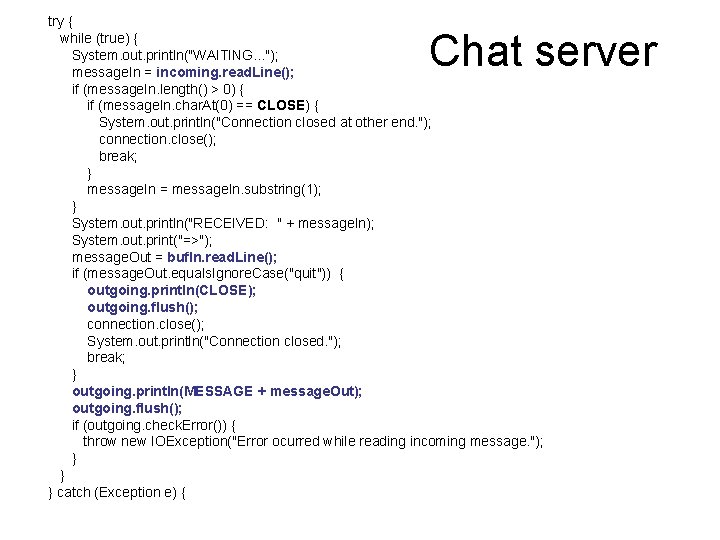
try { while (true) { System. out. println("WAITING. . . "); message. In = incoming. read. Line(); if (message. In. length() > 0) { if (message. In. char. At(0) == CLOSE) { System. out. println("Connection closed at other end. "); connection. close(); break; } message. In = message. In. substring(1); } System. out. println("RECEIVED: " + message. In); System. out. print("=>"); message. Out = buf. In. read. Line(); if (message. Out. equals. Ignore. Case("quit")) { outgoing. println(CLOSE); outgoing. flush(); connection. close(); System. out. println("Connection closed. "); break; } outgoing. println(MESSAGE + message. Out); outgoing. flush(); if (outgoing. check. Error()) { throw new IOException("Error ocurred while reading incoming message. "); } } } catch (Exception e) { Chat server
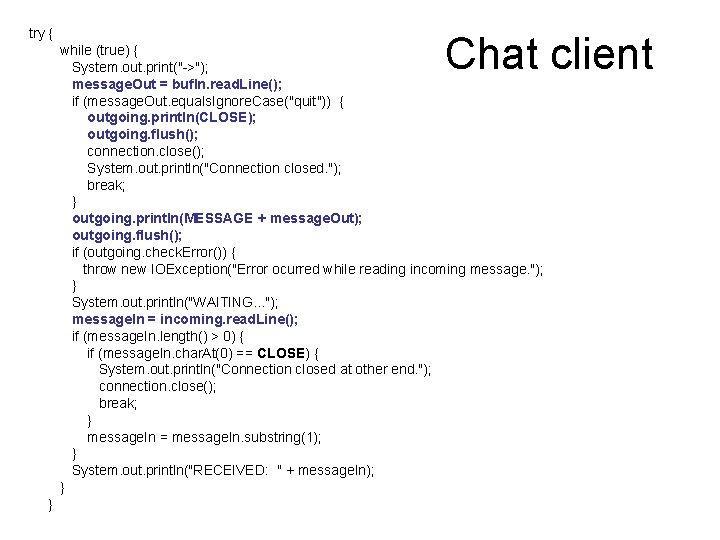
try { Chat client while (true) { System. out. print("->"); message. Out = buf. In. read. Line(); if (message. Out. equals. Ignore. Case("quit")) { outgoing. println(CLOSE); outgoing. flush(); connection. close(); System. out. println("Connection closed. "); break; } outgoing. println(MESSAGE + message. Out); outgoing. flush(); if (outgoing. check. Error()) { throw new IOException("Error ocurred while reading incoming message. "); } System. out. println("WAITING. . . "); message. In = incoming. read. Line(); if (message. In. length() > 0) { if (message. In. char. At(0) == CLOSE) { System. out. println("Connection closed at other end. "); connection. close(); break; } message. In = message. In. substring(1); } System. out. println("RECEIVED: " + message. In); } }
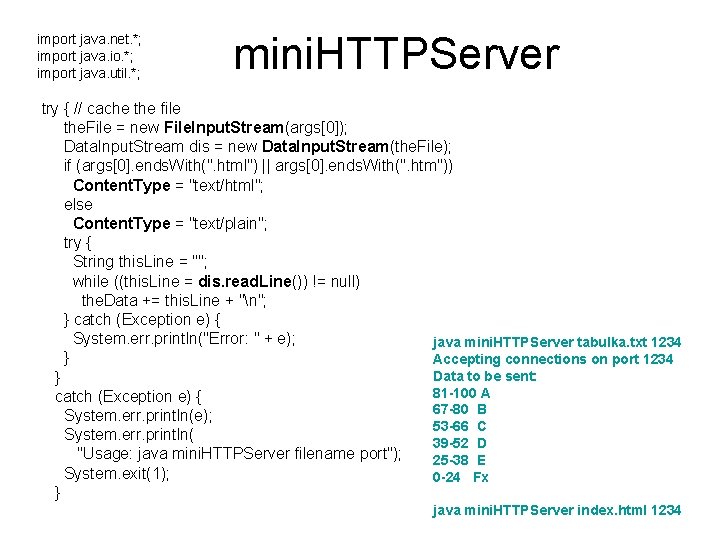
import java. net. *; import java. io. *; import java. util. *; mini. HTTPServer try { // cache the file the. File = new File. Input. Stream(args[0]); Data. Input. Stream dis = new Data. Input. Stream(the. File); if (args[0]. ends. With(". html") || args[0]. ends. With(". htm")) Content. Type = "text/html"; else Content. Type = "text/plain"; try { String this. Line = ""; while ((this. Line = dis. read. Line()) != null) the. Data += this. Line + "n"; } catch (Exception e) { System. err. println("Error: " + e); java mini. HTTPServer tabulka. txt 1234 } Accepting connections on port 1234 Data to be sent: } 81 -100 A catch (Exception e) { 67 -80 B System. err. println(e); 53 -66 C System. err. println( 39 -52 D "Usage: java mini. HTTPServer filename port"); 25 -38 E System. exit(1); 0 -24 Fx } java mini. HTTPServer index. html 1234
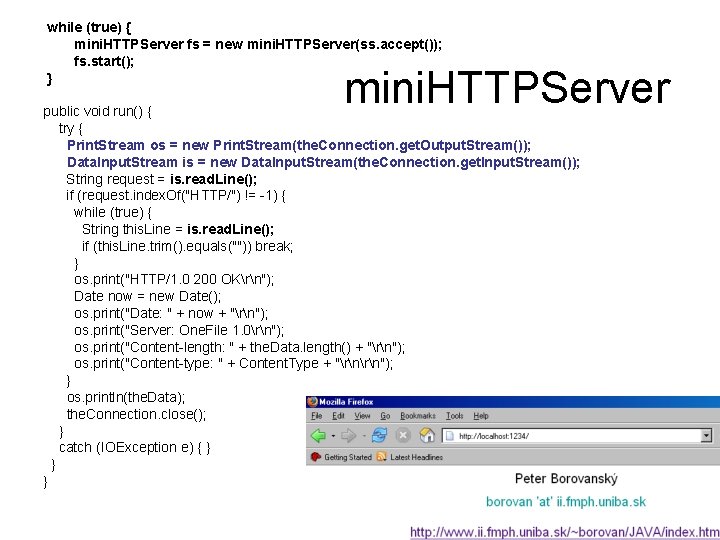
while (true) { mini. HTTPServer fs = new mini. HTTPServer(ss. accept()); fs. start(); } mini. HTTPServer public void run() { try { Print. Stream os = new Print. Stream(the. Connection. get. Output. Stream()); Data. Input. Stream is = new Data. Input. Stream(the. Connection. get. Input. Stream()); String request = is. read. Line(); if (request. index. Of("HTTP/") != -1) { while (true) { String this. Line = is. read. Line(); if (this. Line. trim(). equals("")) break; } os. print("HTTP/1. 0 200 OKrn"); Date now = new Date(); os. print("Date: " + now + "rn"); os. print("Server: One. File 1. 0rn"); os. print("Content-length: " + the. Data. length() + "rn"); os. print("Content-type: " + Content. Type + "rn"); } os. println(the. Data); the. Connection. close(); } catch (IOException e) { } } }
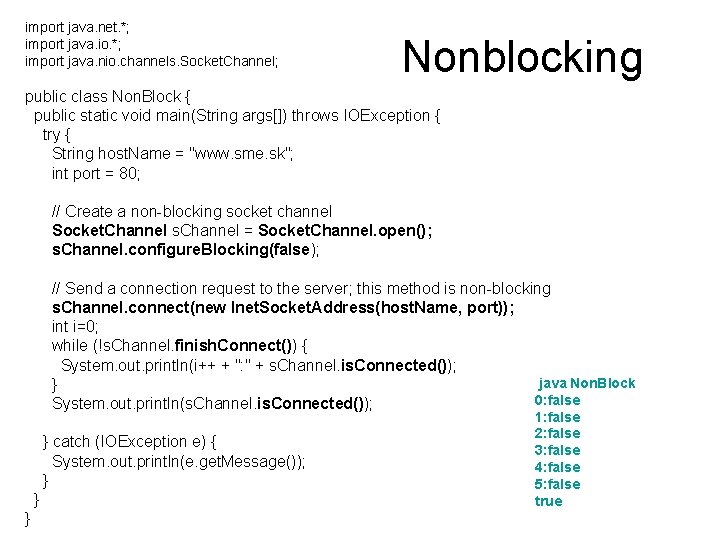
import java. net. *; import java. io. *; import java. nio. channels. Socket. Channel; Nonblocking public class Non. Block { public static void main(String args[]) throws IOException { try { String host. Name = "www. sme. sk"; int port = 80; // Create a non-blocking socket channel Socket. Channel s. Channel = Socket. Channel. open(); s. Channel. configure. Blocking(false); // Send a connection request to the server; this method is non-blocking s. Channel. connect(new Inet. Socket. Address(host. Name, port)); int i=0; while (!s. Channel. finish. Connect()) { System. out. println(i++ + ": " + s. Channel. is. Connected()); java Non. Block } 0: false System. out. println(s. Channel. is. Connected()); } catch (IOException e) { System. out. println(e. get. Message()); } } } 1: false 2: false 3: false 4: false 5: false true
![import java sql jdbc public class Lookup public static void mainString args import java. sql. *; jdbc public class Lookup { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/28daed8a257cec7d8113d93f07d69040/image-23.jpg)
import java. sql. *; jdbc public class Lookup { public static void main(String[] args) { String db. Url = "jdbc: odbc: radmon"; String user = ""; String password = ""; try { Class. for. Name("sun. jdbc. odbc. Jdbc. Odbc. Driver"); Connection c = Driver. Manager. get. Connection(db. Url, user, password); Statement s = c. create. Statement(); Result. Set r = s. execute. Query( "SELECT nazov, ulica, mesto, kraj FROM radmon. stanica " + "WHERE (kraj='" + args[0] + "') " + " AND (Ulica Is Not Null) " + "ORDER BY mesto"); while(r. next()) { System. out. println( r. get. String("kraj") + ", " + r. get. String("mesto") + ": " + r. get. String("ulica") + ": " + r. get. String("nazov") ); java Lookup "Banska Bystrica" } Banska Bystrica, Banska Bystrica: Nßm. Csl. armßdy: OU v B. Bystrici Banska Bystrica, Banska Bystrica: Namestie L. Stura: KU v B. Bystrici s. close(); Banska Bystrica, Brezno: Razusova: OU v Brezne } catch(Exception e) { Banska Bystrica, Detva: Zahradna: OU v Detve e. print. Stack. Trace(); Banska Bystrica, Krupina: Priemyselna: OU v Krupine } Banska Bystrica, Lucenec: Nam. Republiky: OU v Lucenci } Banska Bystrica, Rimavska Sobota: Nam. M. Tompu: OU v Rimavskej Sobote } Banska Bystrica, Slovenska Lupca: Postovy priecinok: KCHL v Sl. Lupci Banska Bystrica, Velky Krtis: Madacha: OU vo Velkom Krtisi
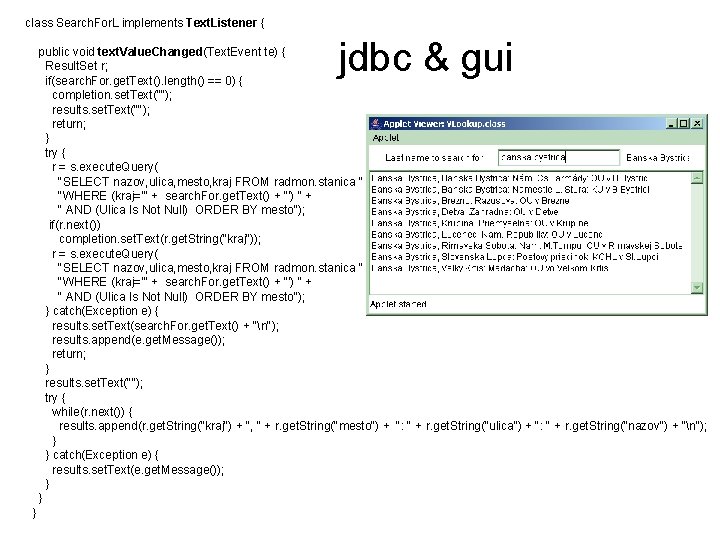
class Search. For. L implements Text. Listener { jdbc & gui public void text. Value. Changed(Text. Event te) { Result. Set r; if(search. For. get. Text(). length() == 0) { completion. set. Text(""); results. set. Text(""); return; } try { r = s. execute. Query( "SELECT nazov, ulica, mesto, kraj FROM radmon. stanica " + "WHERE (kraj='" + search. For. get. Text() + "') “ + " AND (Ulica Is Not Null) ORDER BY mesto"); if(r. next()) completion. set. Text(r. get. String("kraj")); r = s. execute. Query( "SELECT nazov, ulica, mesto, kraj FROM radmon. stanica " + "WHERE (kraj='" + search. For. get. Text() + "') “ + " AND (Ulica Is Not Null) ORDER BY mesto"); } catch(Exception e) { results. set. Text(search. For. get. Text() + "n"); results. append(e. get. Message()); return; } results. set. Text(""); try { while(r. next()) { results. append(r. get. String("kraj") + ", " + r. get. String("mesto") + ": " + r. get. String("ulica") + ": " + r. get. String("nazov") + "n"); } } catch(Exception e) { results. set. Text(e. get. Message()); } } }
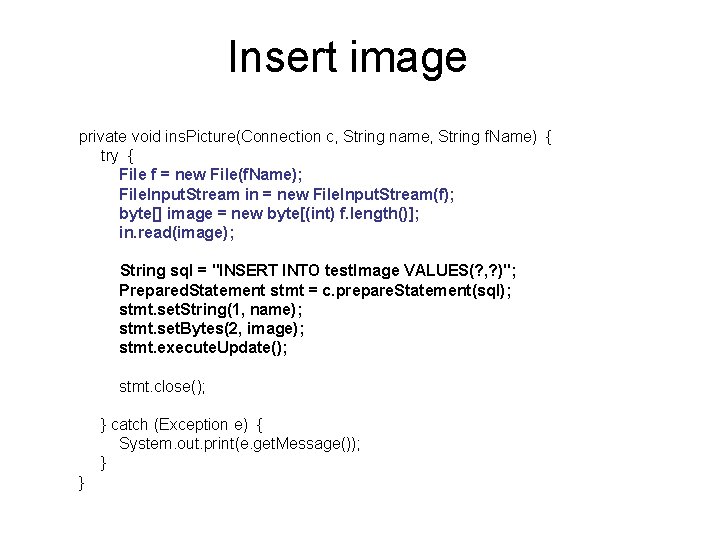
Insert image private void ins. Picture(Connection c, String name, String f. Name) { try { File f = new File(f. Name); File. Input. Stream in = new File. Input. Stream(f); byte[] image = new byte[(int) f. length()]; in. read(image); String sql = "INSERT INTO test. Image VALUES(? , ? )"; Prepared. Statement stmt = c. prepare. Statement(sql); stmt. set. String(1, name); stmt. set. Bytes(2, image); stmt. execute. Update(); stmt. close(); } catch (Exception e) { System. out. print(e. get. Message()); } }
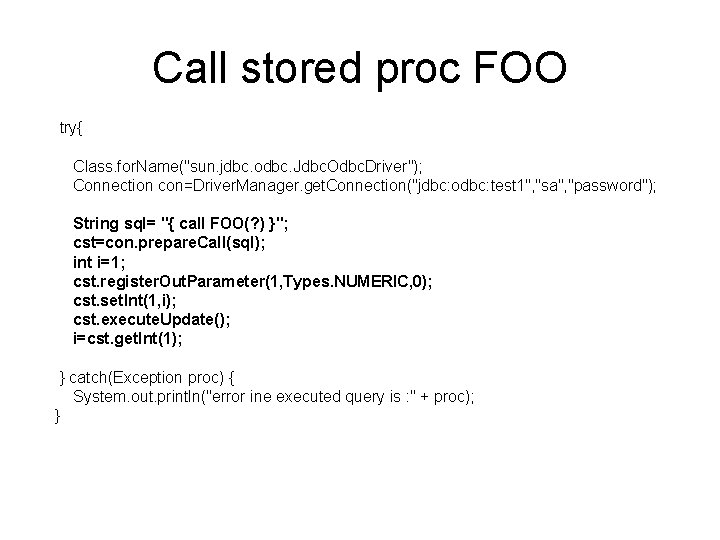
Call stored proc FOO try{ Class. for. Name("sun. jdbc. odbc. Jdbc. Odbc. Driver"); Connection con=Driver. Manager. get. Connection("jdbc: odbc: test 1", "sa", "password"); String sql= "{ call FOO(? ) }"; cst=con. prepare. Call(sql); int i=1; cst. register. Out. Parameter(1, Types. NUMERIC, 0); cst. set. Int(1, i); cst. execute. Update(); i=cst. get. Int(1); } catch(Exception proc) { System. out. println("error ine executed query is : " + proc); }
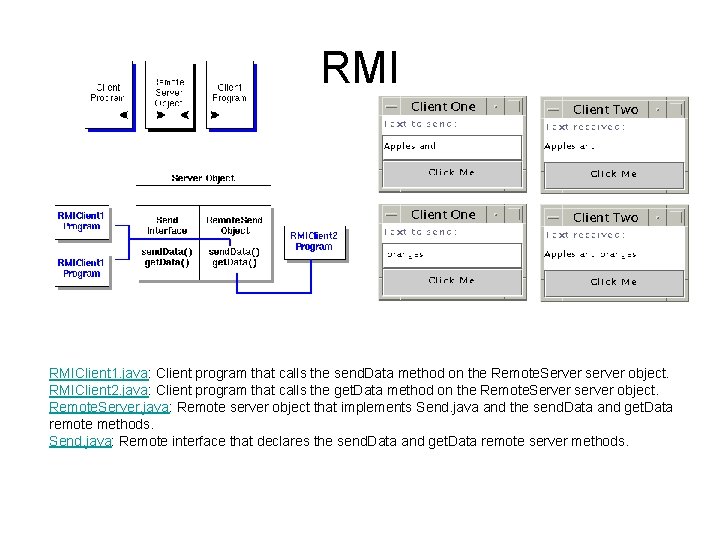
RMI RMIClient 1. java: Client program that calls the send. Data method on the Remote. Server server object. RMIClient 2. java: Client program that calls the get. Data method on the Remote. Server server object. Remote. Server. java: Remote server object that implements Send. java and the send. Data and get. Data remote methods. Send. java: Remote interface that declares the send. Data and get. Data remote server methods.
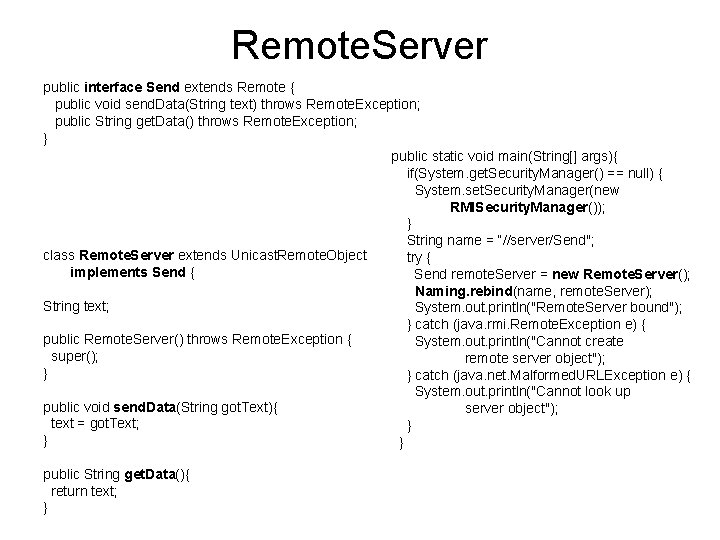
Remote. Server public interface Send extends Remote { public void send. Data(String text) throws Remote. Exception; public String get. Data() throws Remote. Exception; } public static void main(String[] args){ if(System. get. Security. Manager() == null) { System. set. Security. Manager(new RMISecurity. Manager()); } String name = “//server/Send"; class Remote. Server extends Unicast. Remote. Object try { implements Send { Send remote. Server = new Remote. Server(); Naming. rebind(name, remote. Server); String text; System. out. println("Remote. Server bound"); } catch (java. rmi. Remote. Exception e) { public Remote. Server() throws Remote. Exception { System. out. println("Cannot create super(); remote server object"); } } catch (java. net. Malformed. URLException e) { System. out. println("Cannot look up public void send. Data(String got. Text){ server object"); text = got. Text; } } } public String get. Data(){ return text; }
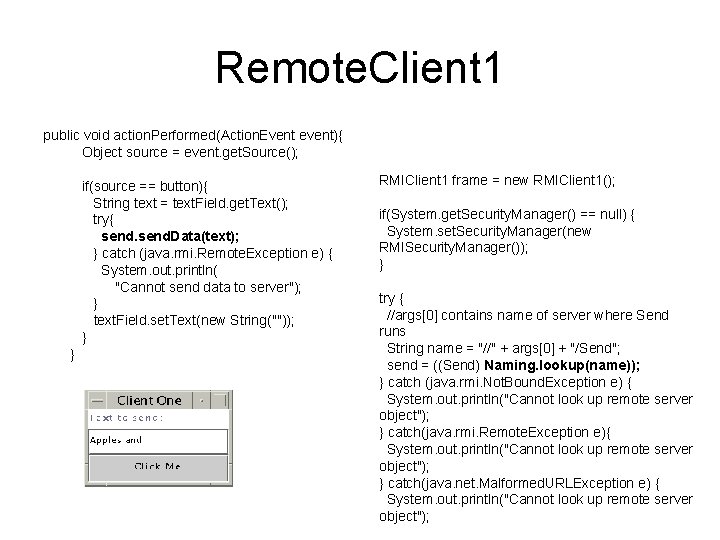
Remote. Client 1 public void action. Performed(Action. Event event){ Object source = event. get. Source(); if(source == button){ String text = text. Field. get. Text(); try{ send. Data(text); } catch (java. rmi. Remote. Exception e) { System. out. println( "Cannot send data to server"); } text. Field. set. Text(new String("")); } } RMIClient 1 frame = new RMIClient 1(); if(System. get. Security. Manager() == null) { System. set. Security. Manager(new RMISecurity. Manager()); } try { //args[0] contains name of server where Send runs String name = "//" + args[0] + "/Send"; send = ((Send) Naming. lookup(name)); } catch (java. rmi. Not. Bound. Exception e) { System. out. println("Cannot look up remote server object"); } catch(java. rmi. Remote. Exception e){ System. out. println("Cannot look up remote server object"); } catch(java. net. Malformed. URLException e) { System. out. println("Cannot look up remote server object");
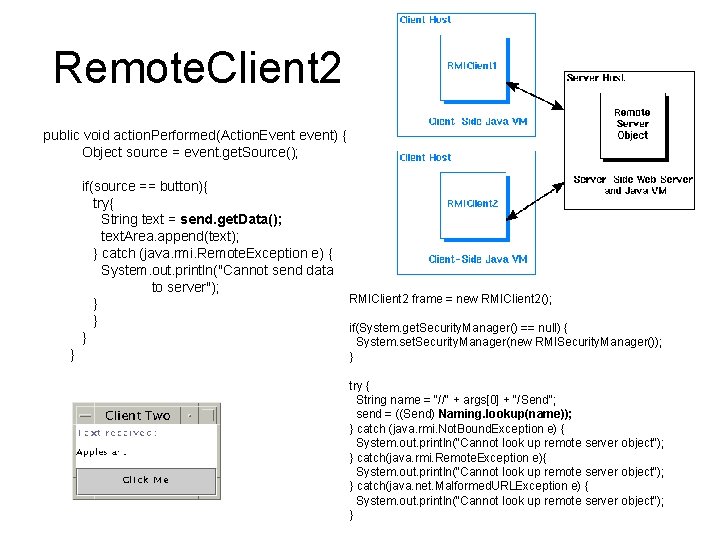
Remote. Client 2 public void action. Performed(Action. Event event) { Object source = event. get. Source(); if(source == button){ try{ String text = send. get. Data(); text. Area. append(text); } catch (java. rmi. Remote. Exception e) { System. out. println("Cannot send data to server"); } } RMIClient 2 frame = new RMIClient 2(); if(System. get. Security. Manager() == null) { System. set. Security. Manager(new RMISecurity. Manager()); } try { String name = "//" + args[0] + "/Send"; send = ((Send) Naming. lookup(name)); } catch (java. rmi. Not. Bound. Exception e) { System. out. println("Cannot look up remote server object"); } catch(java. rmi. Remote. Exception e){ System. out. println("Cannot look up remote server object"); } catch(java. net. Malformed. URLException e) { System. out. println("Cannot look up remote server object"); }
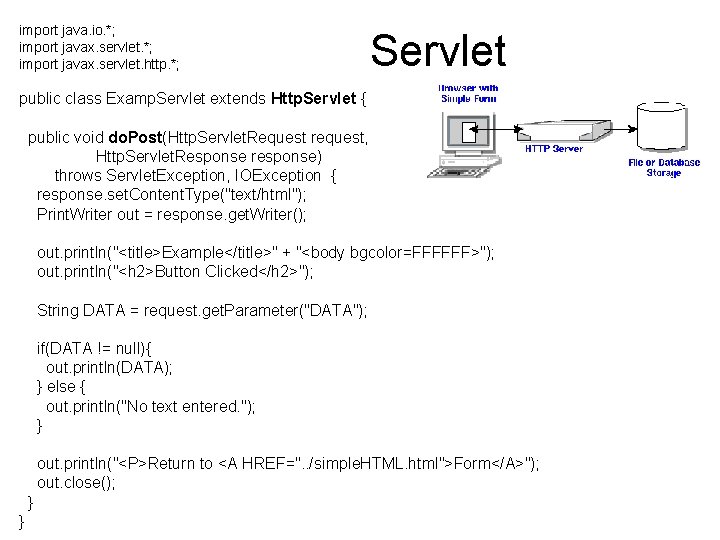
import java. io. *; import javax. servlet. http. *; Servlet public class Examp. Servlet extends Http. Servlet { public void do. Post(Http. Servlet. Request request, Http. Servlet. Response response) throws Servlet. Exception, IOException { response. set. Content. Type("text/html"); Print. Writer out = response. get. Writer(); out. println("<title>Example</title>" + "<body bgcolor=FFFFFF>"); out. println("<h 2>Button Clicked</h 2>"); String DATA = request. get. Parameter("DATA"); if(DATA != null){ out. println(DATA); } else { out. println("No text entered. "); } out. println("<P>Return to <A HREF=". . /simple. HTML. html">Form</A>"); out. close(); } }
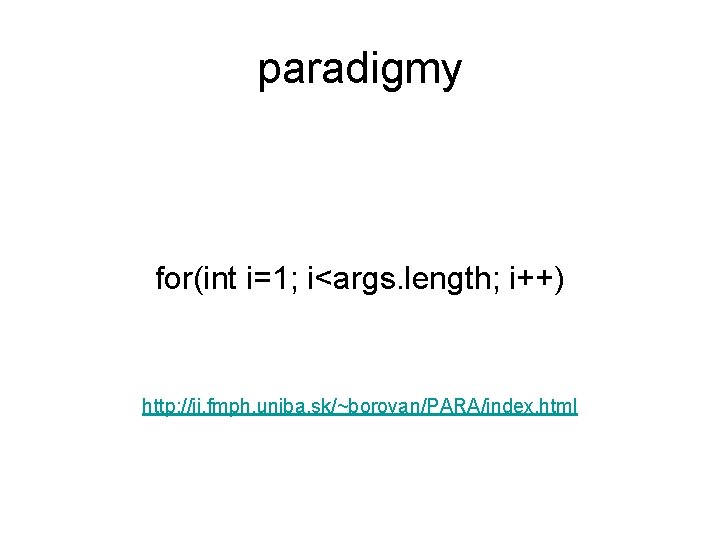
paradigmy for(int i=1; i<args. length; i++) http: //ii. fmph. uniba. sk/~borovan/PARA/index. html