mutex n n counter pthreadmutext countmutex PTHREADMUTEXINITIALIZER pthreadmutexlock
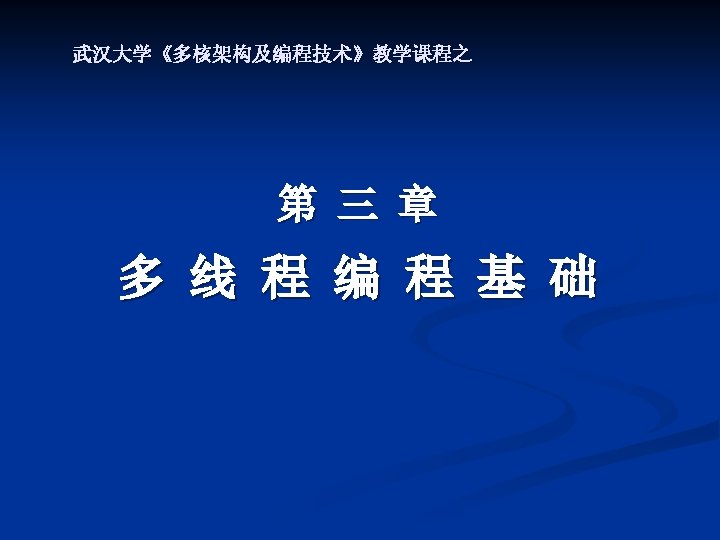
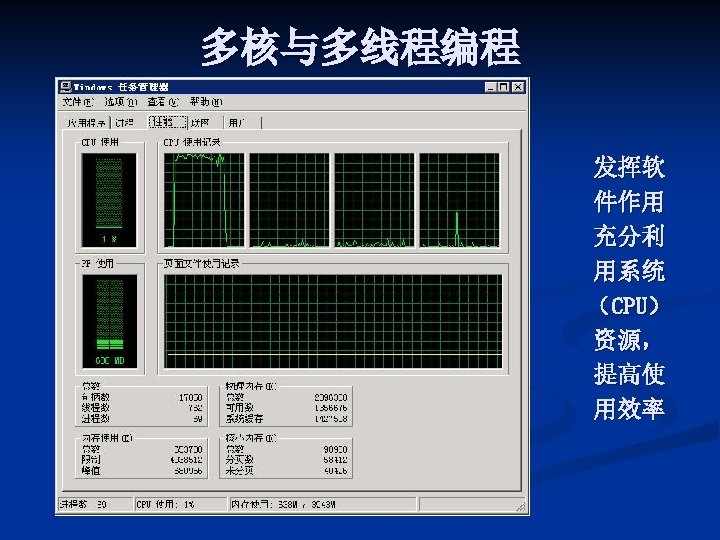
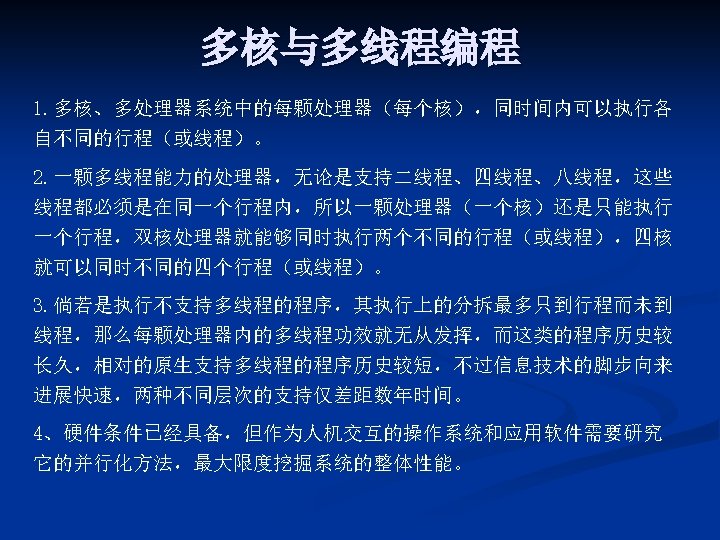
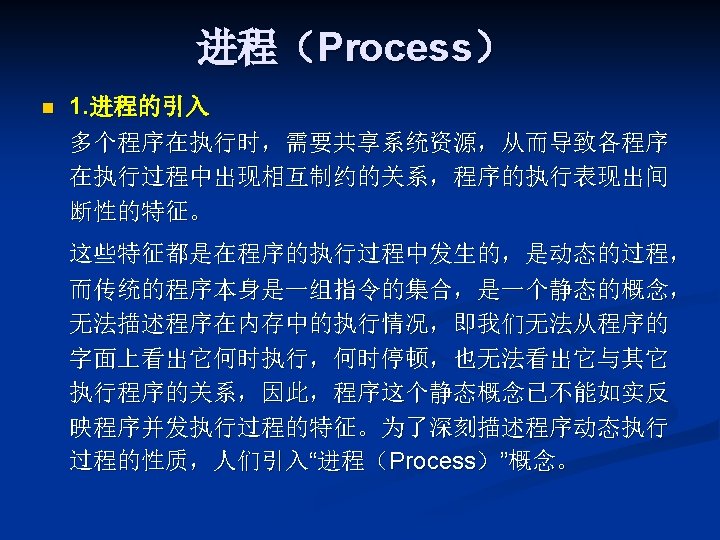
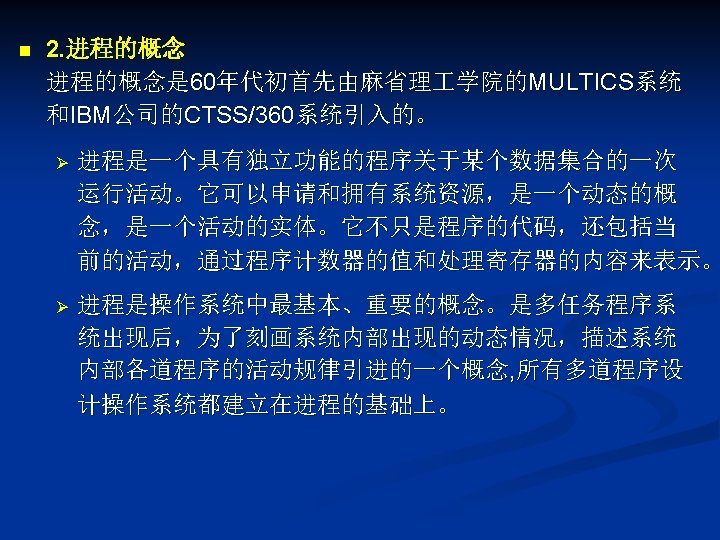
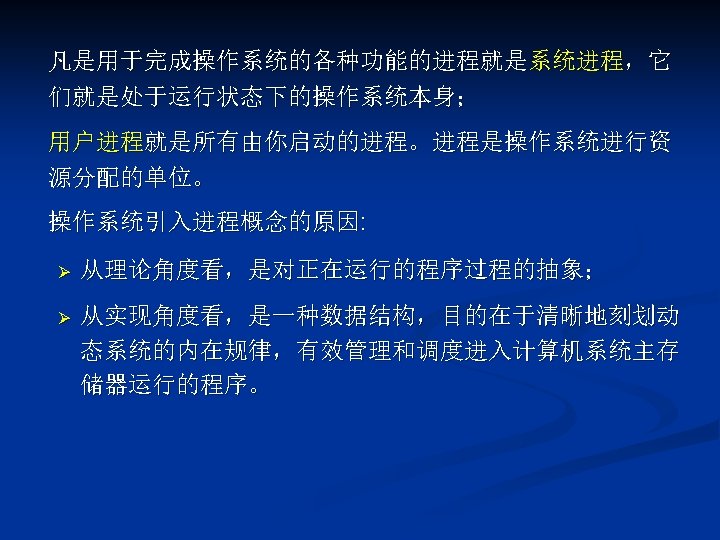
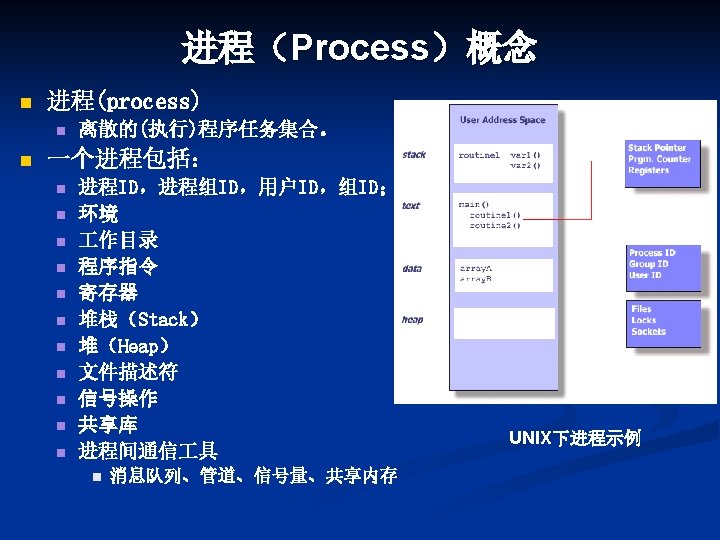
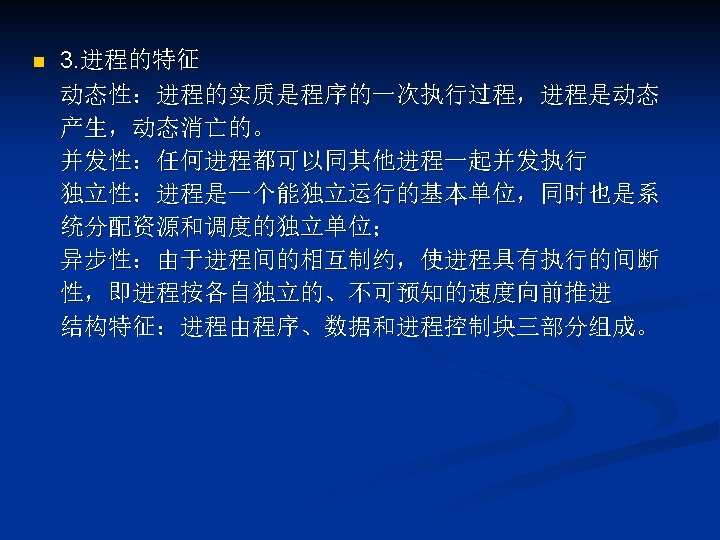
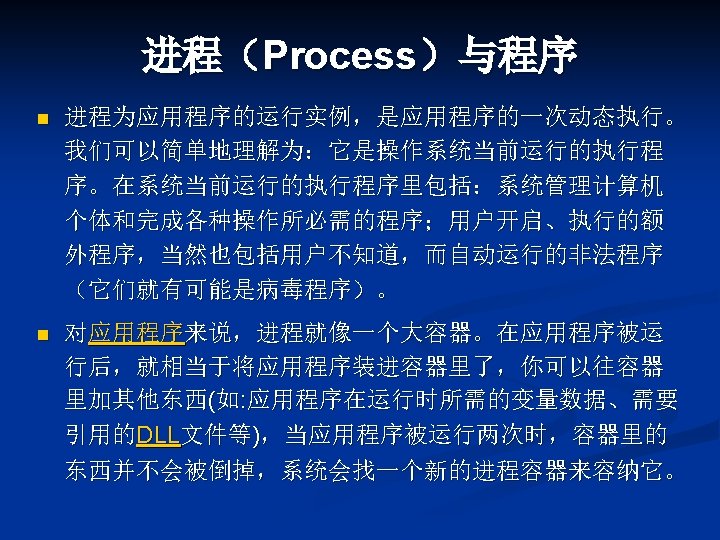
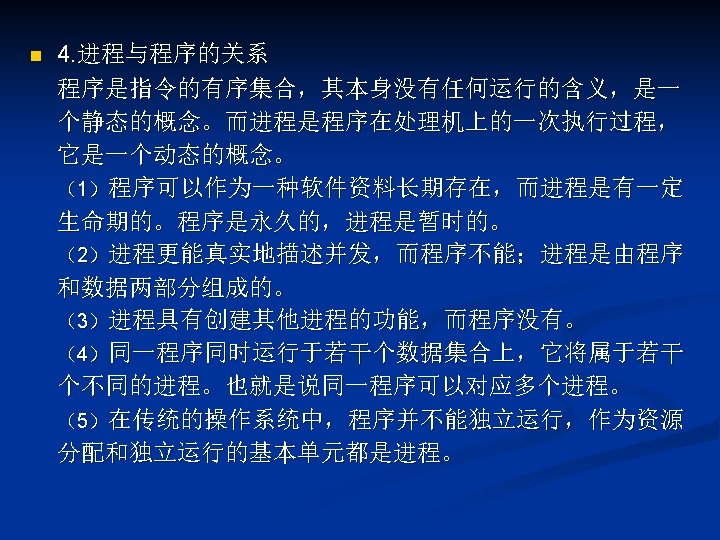
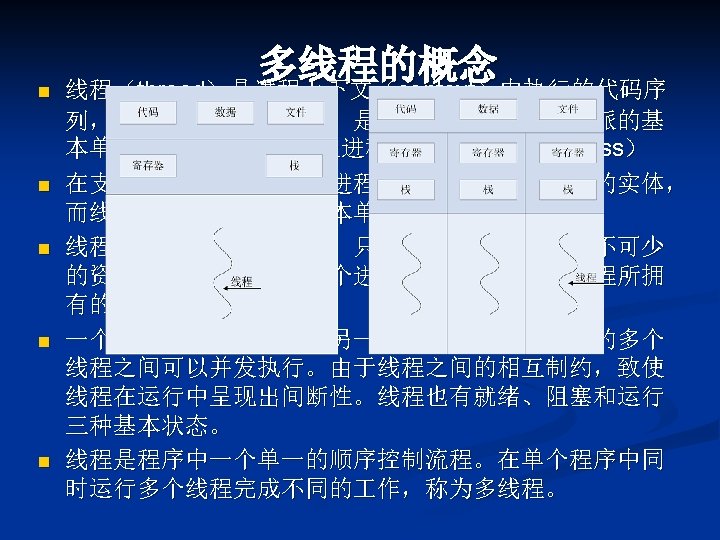
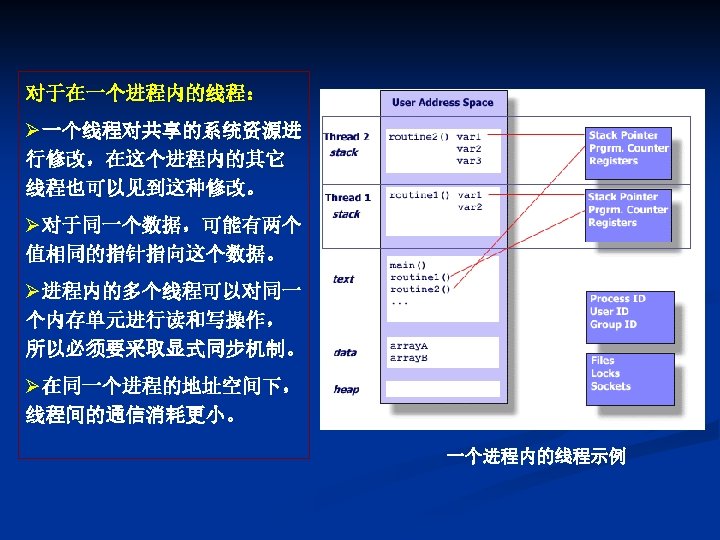
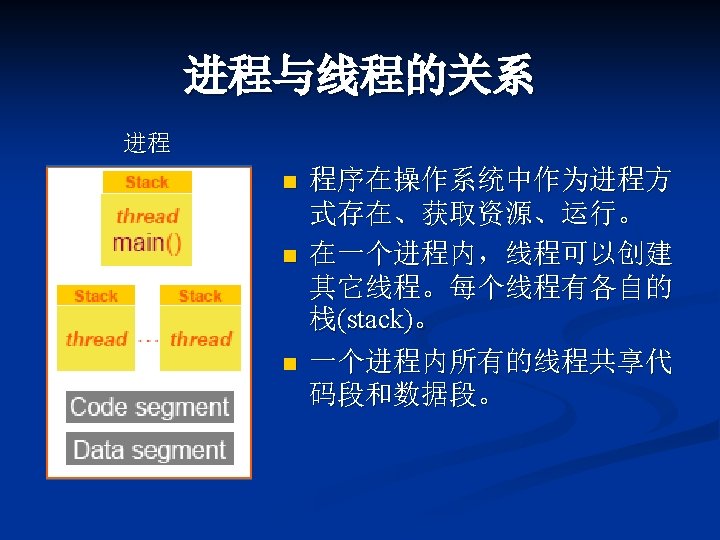
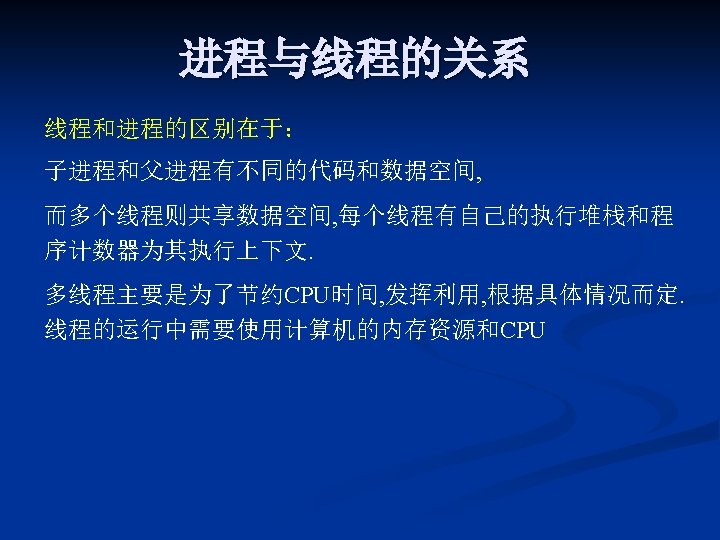
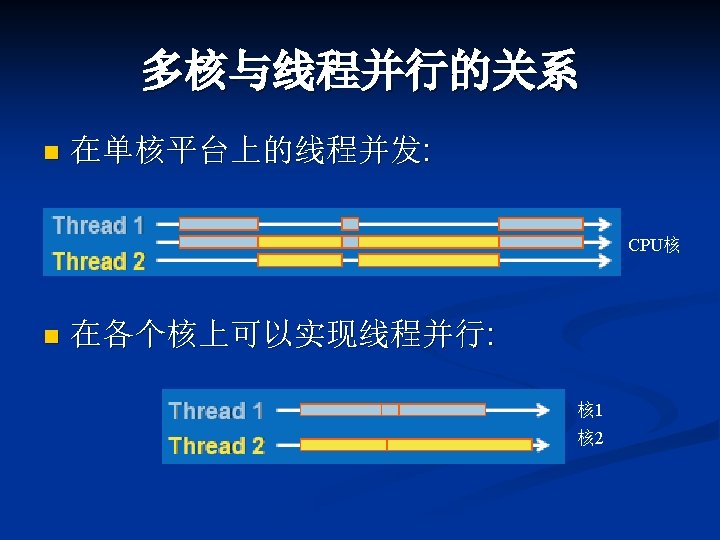
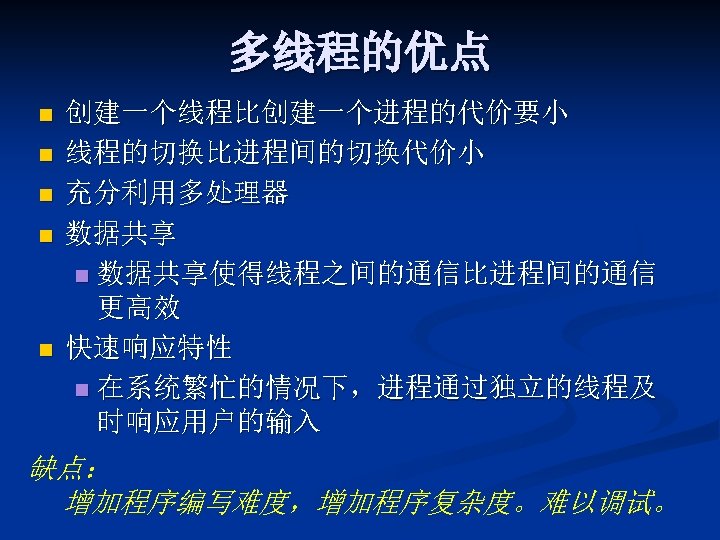
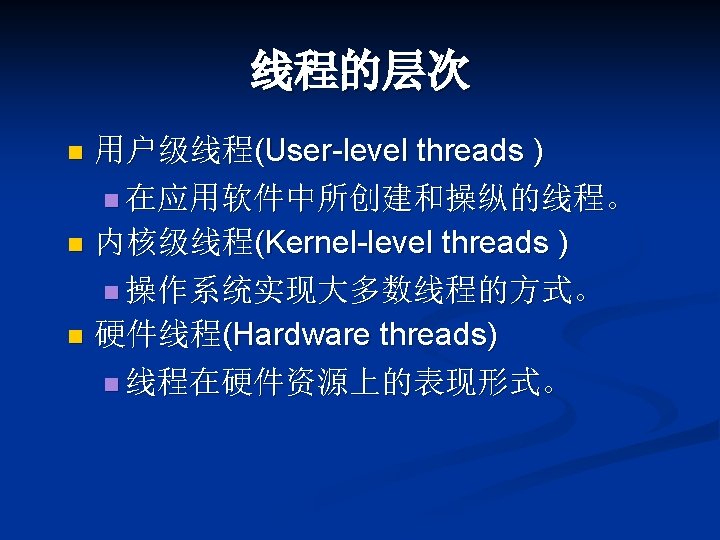
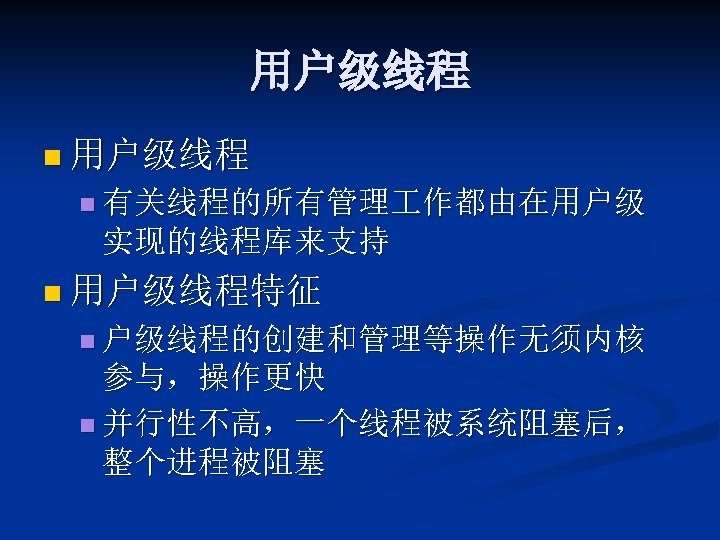
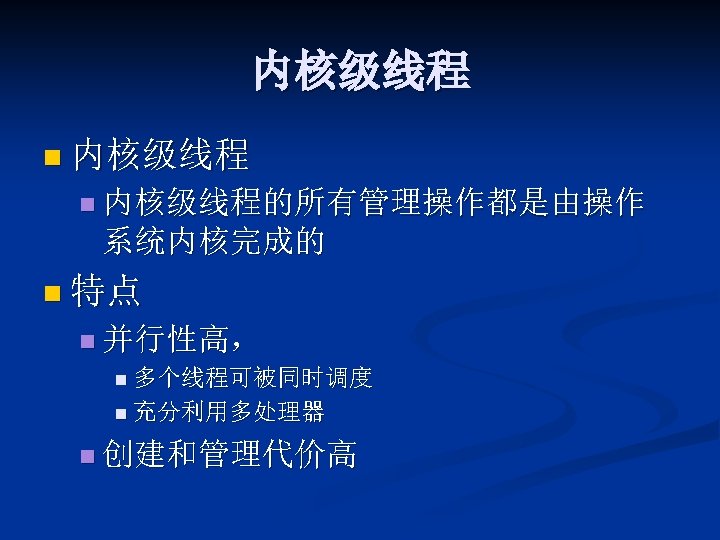
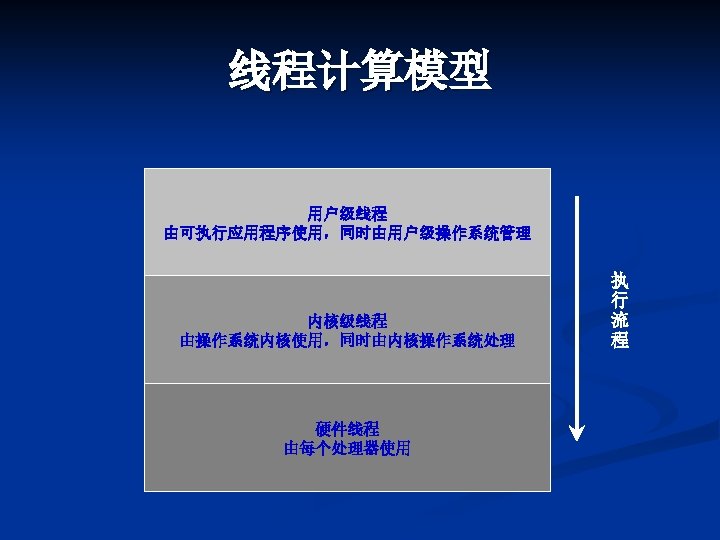
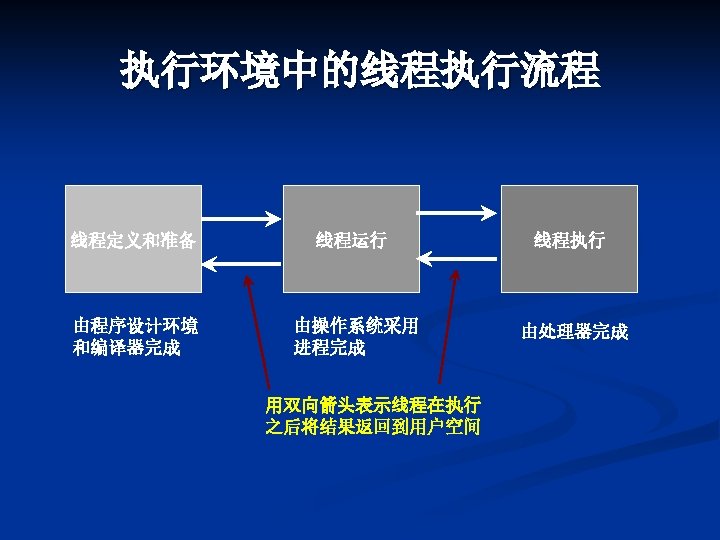
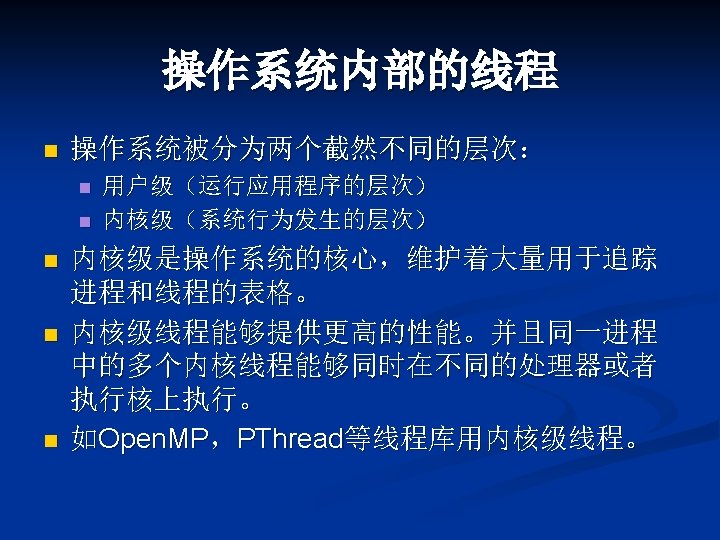
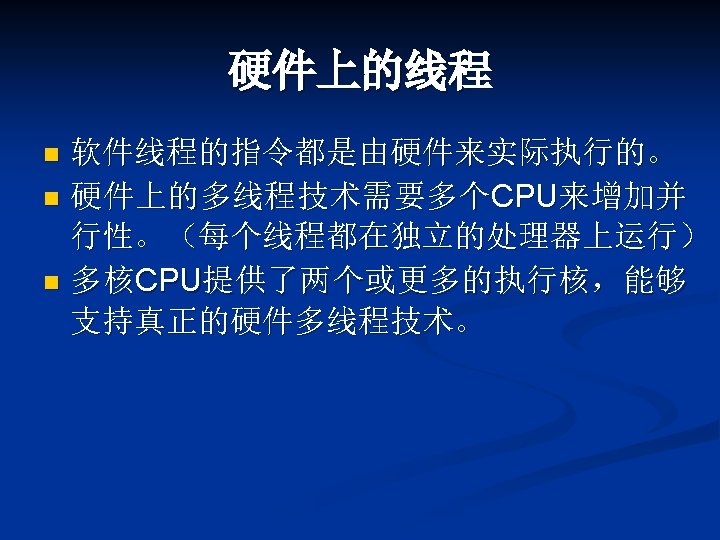
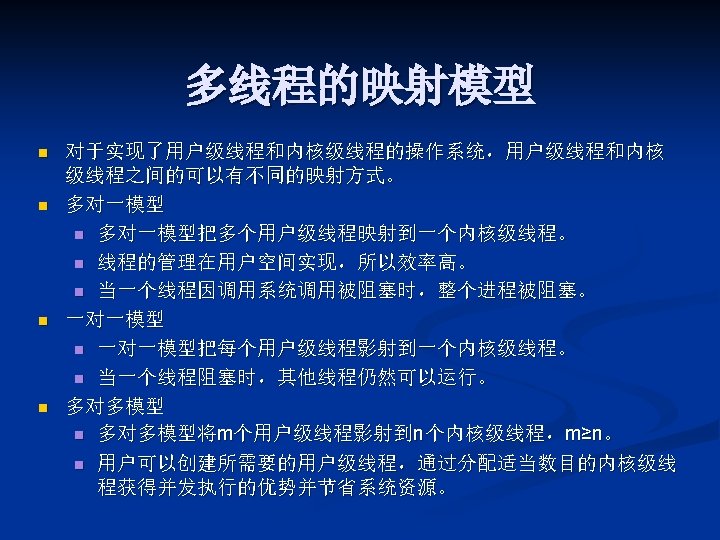
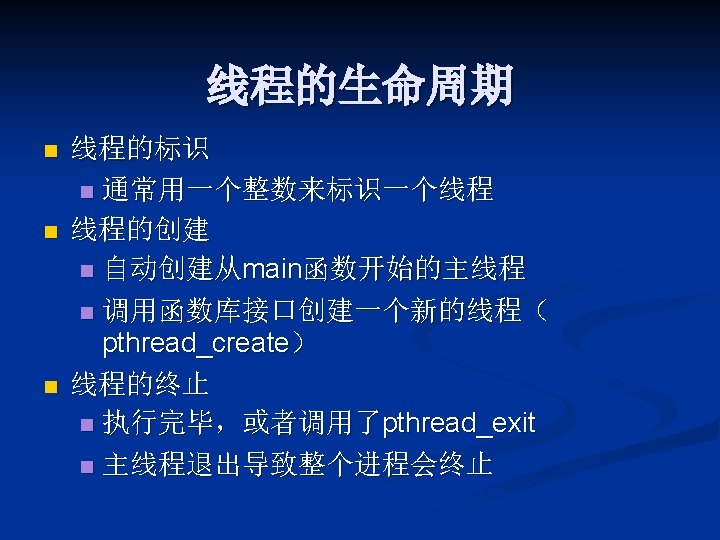
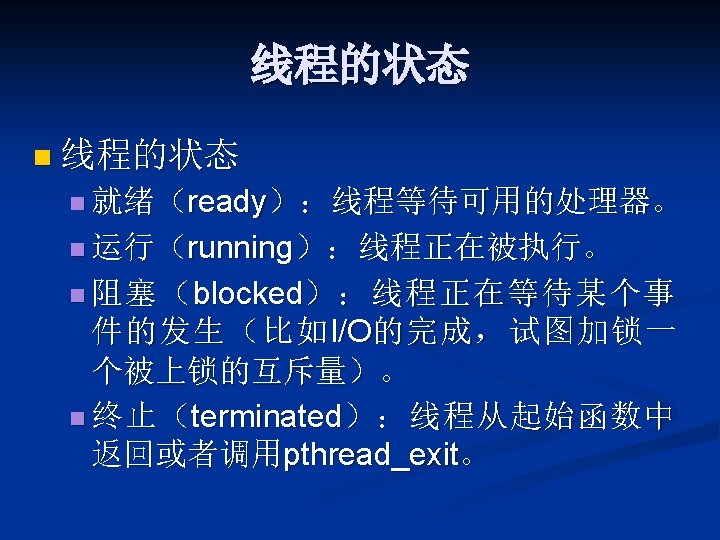
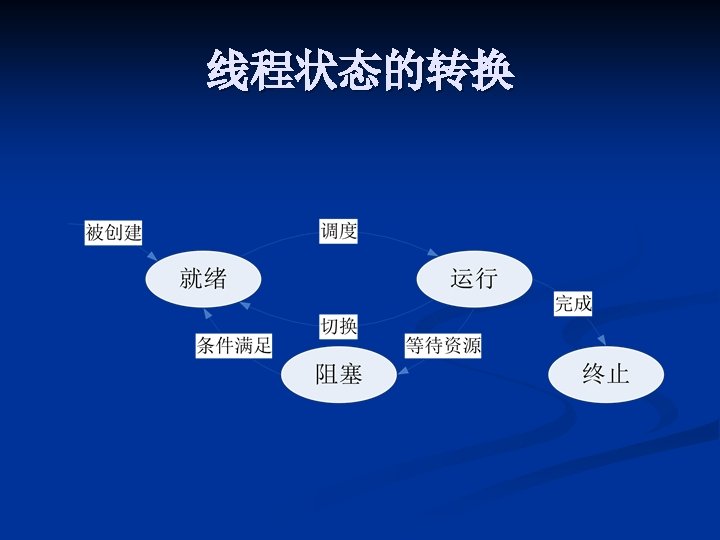
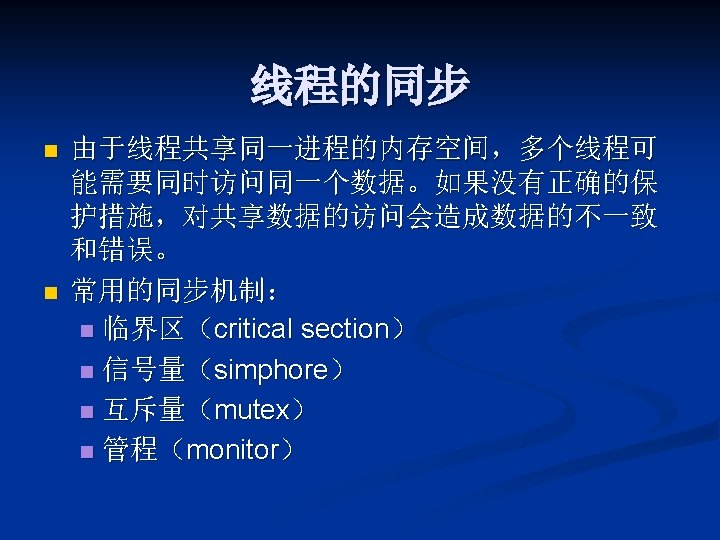
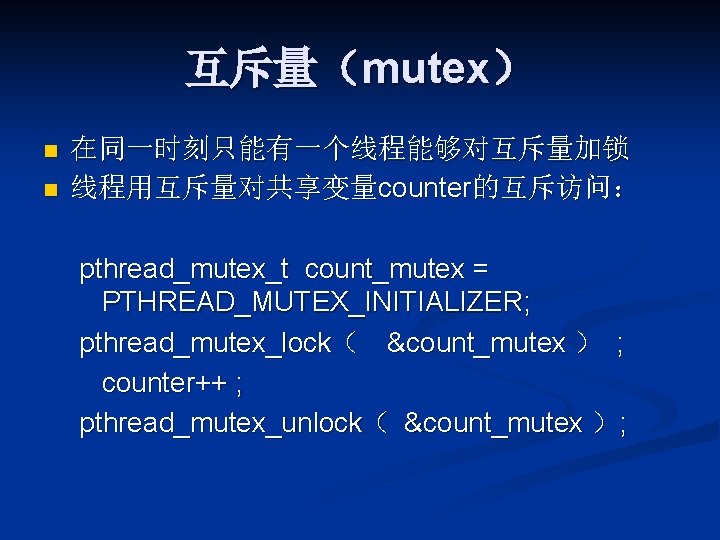
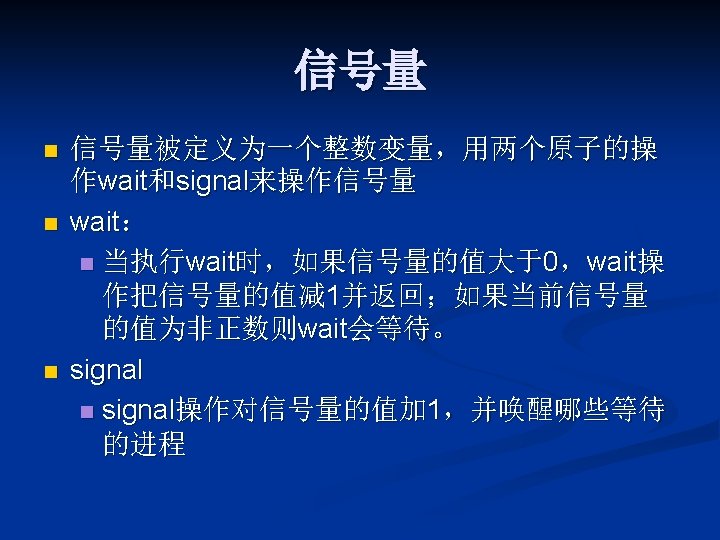
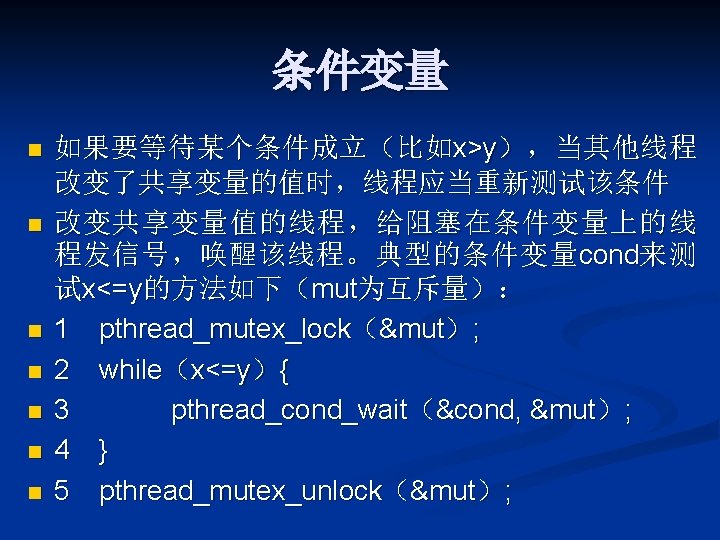
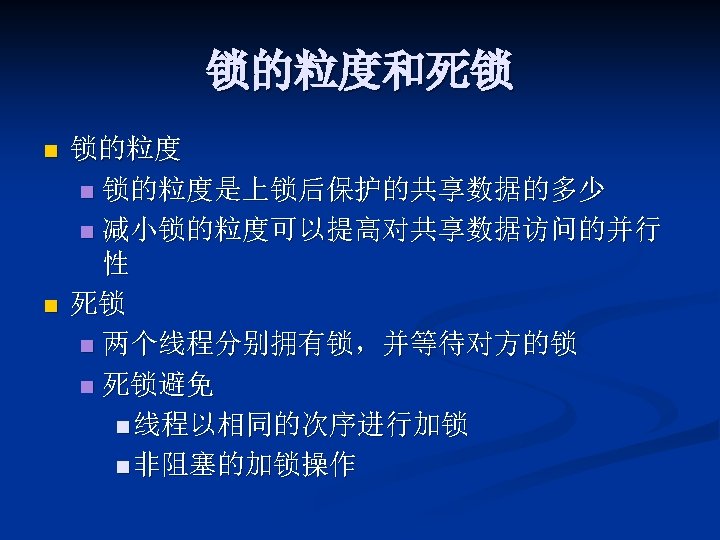
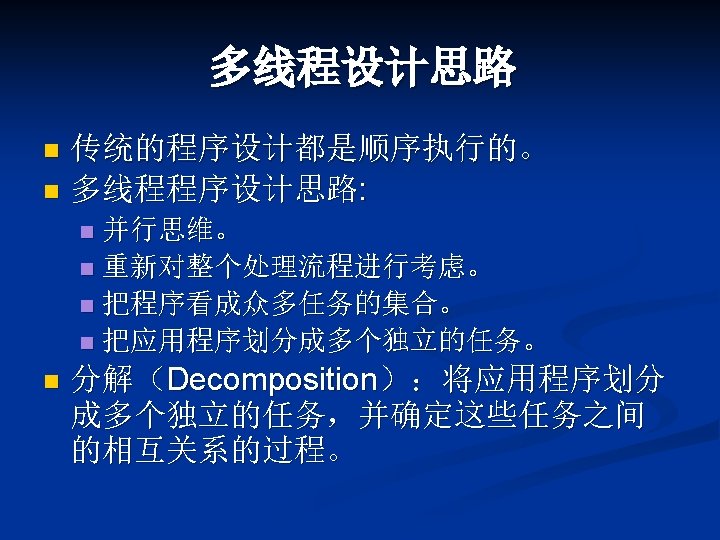
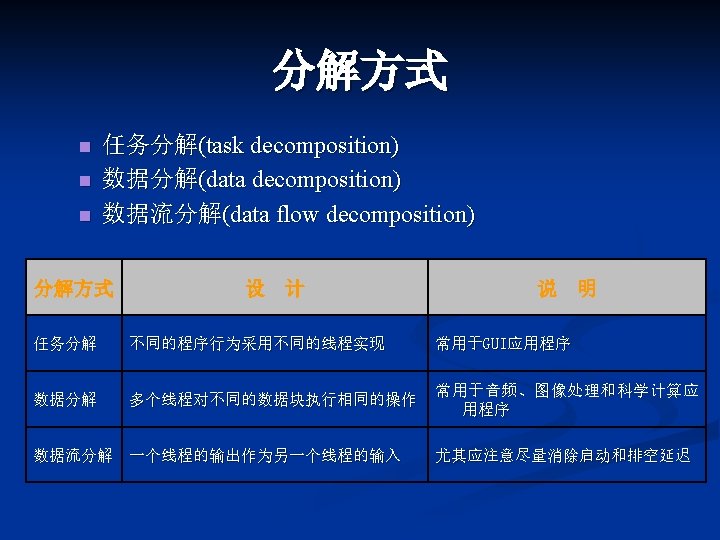
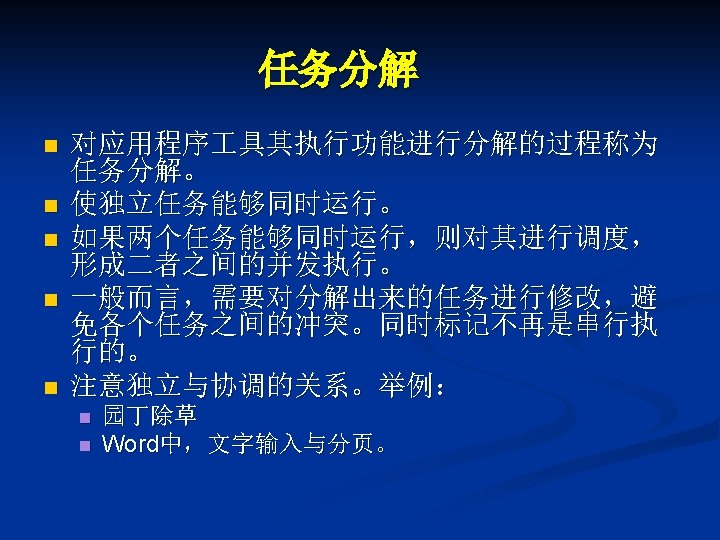
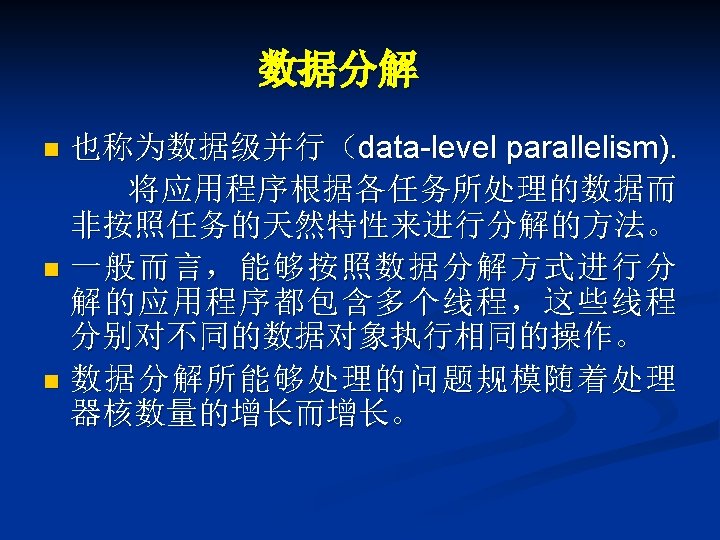
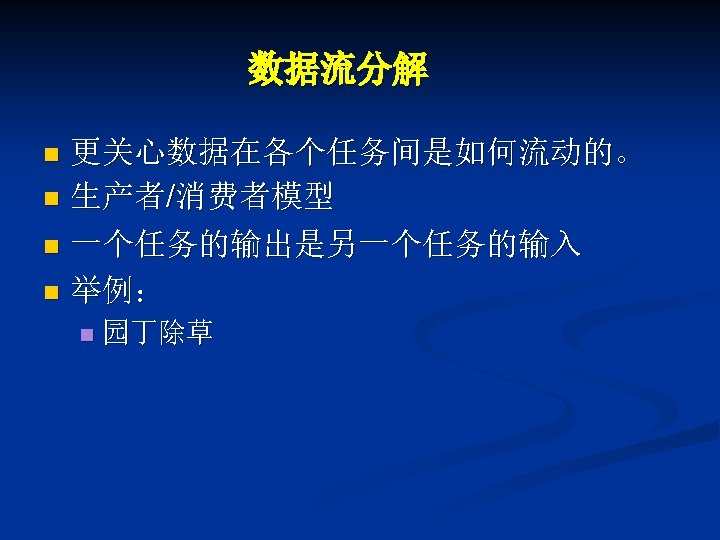
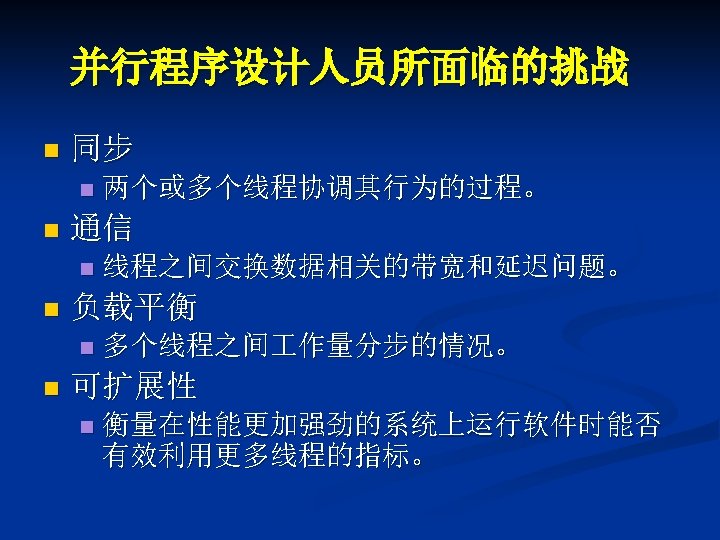
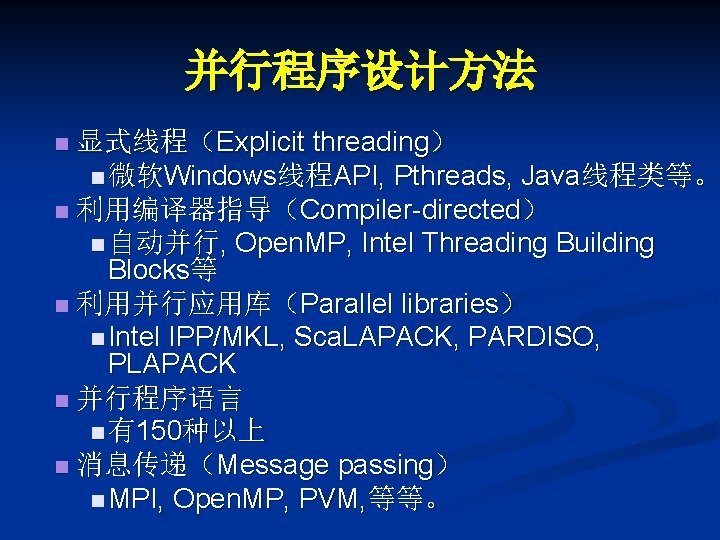
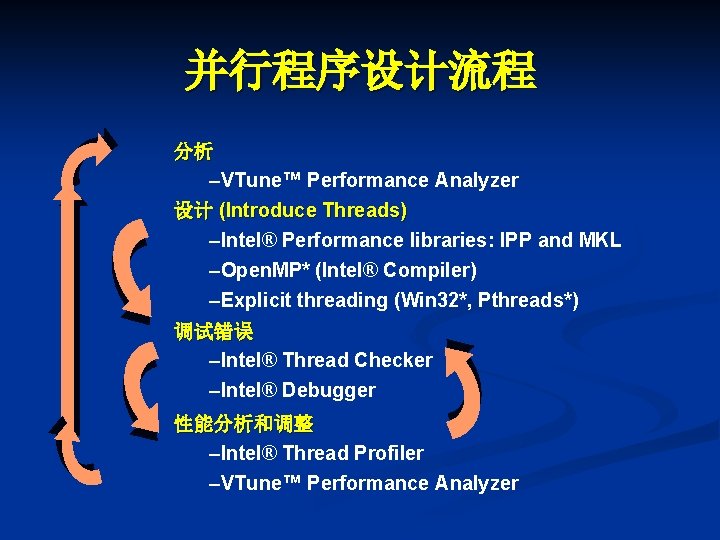
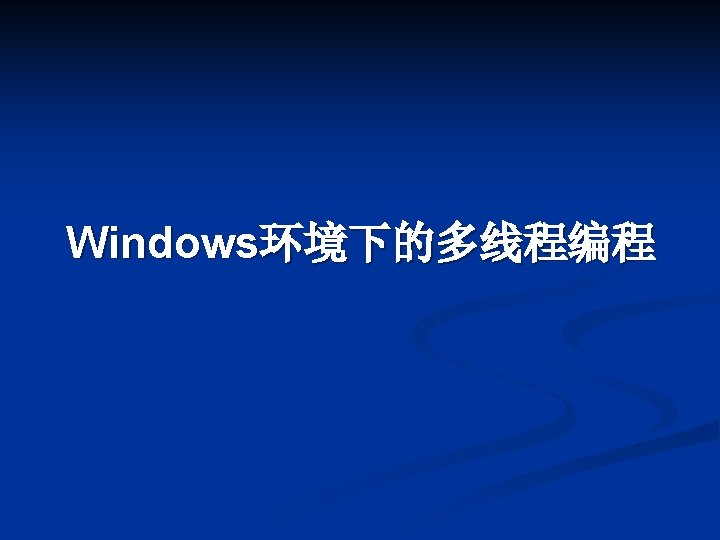
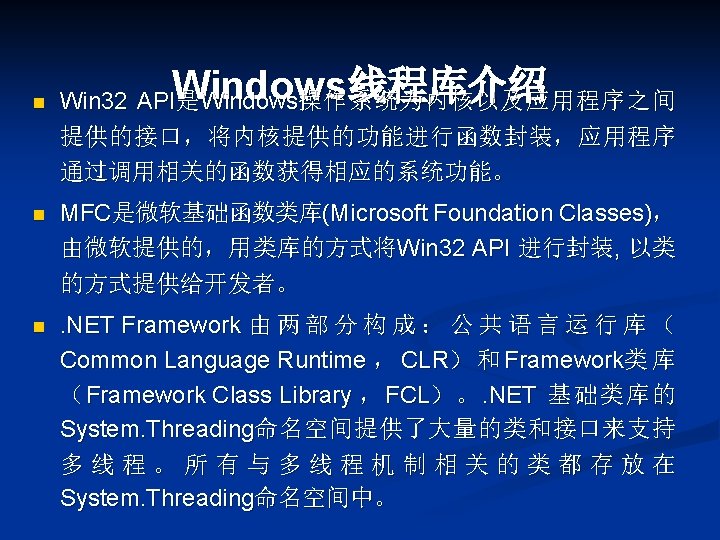
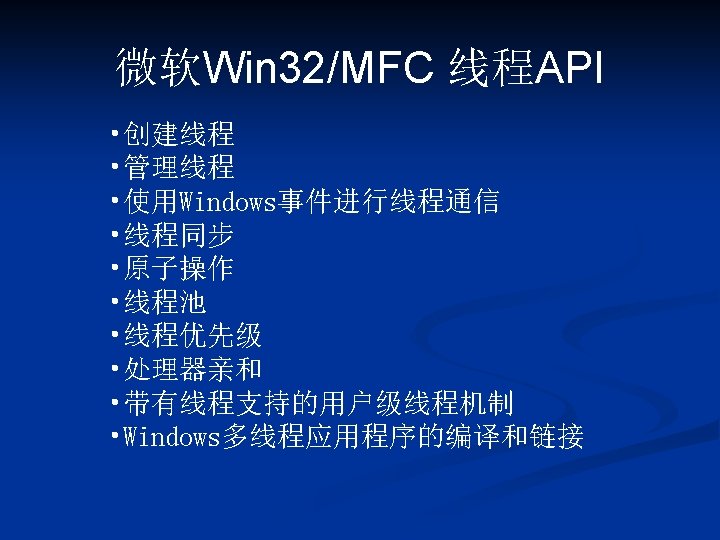
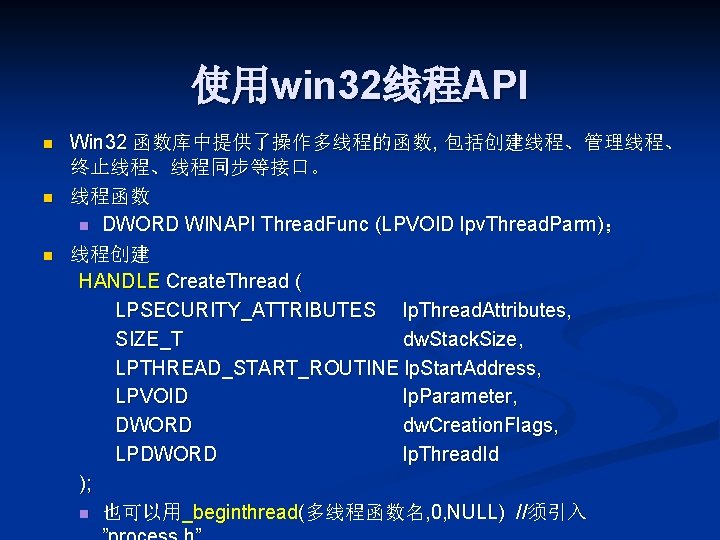
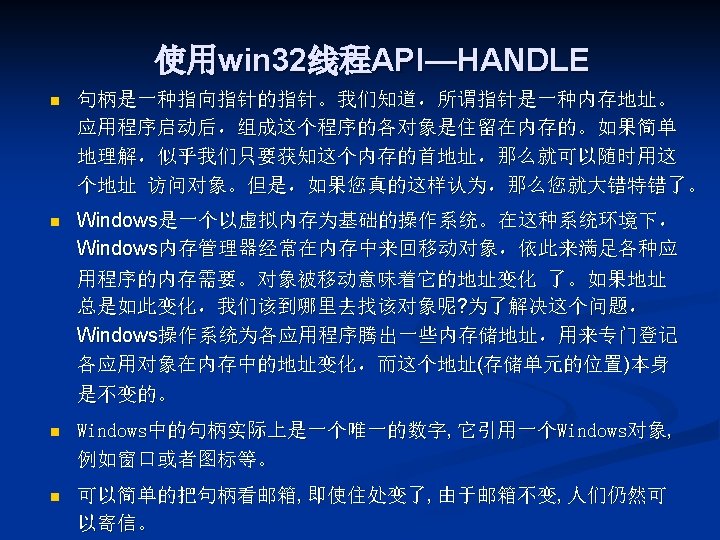
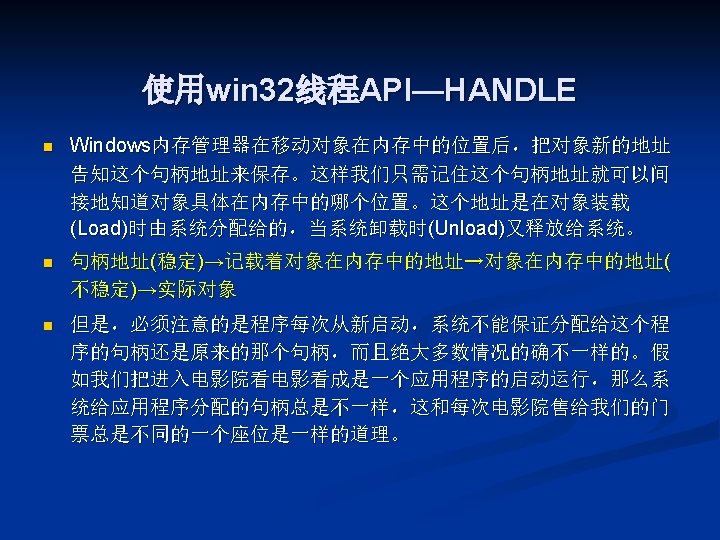
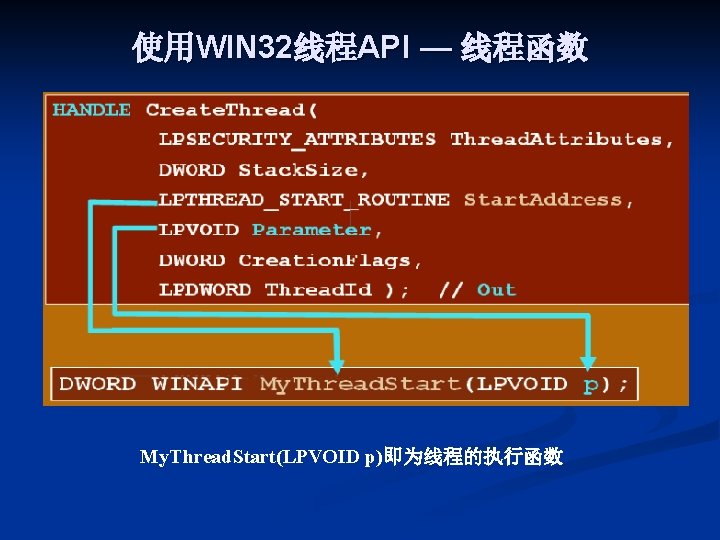
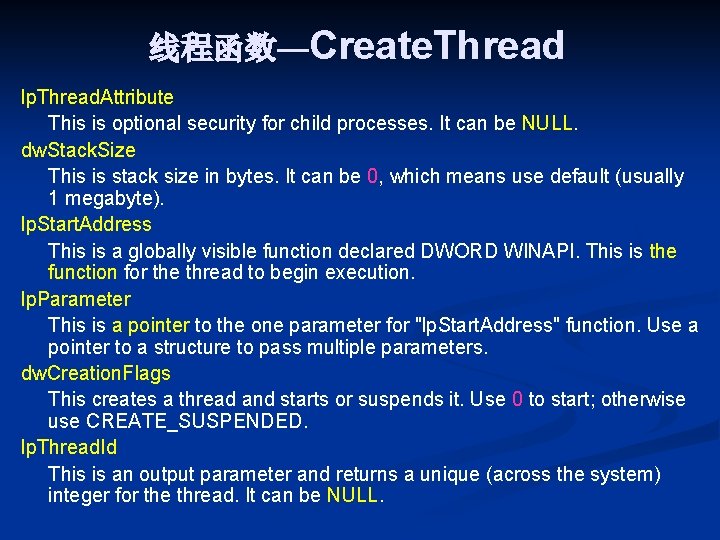
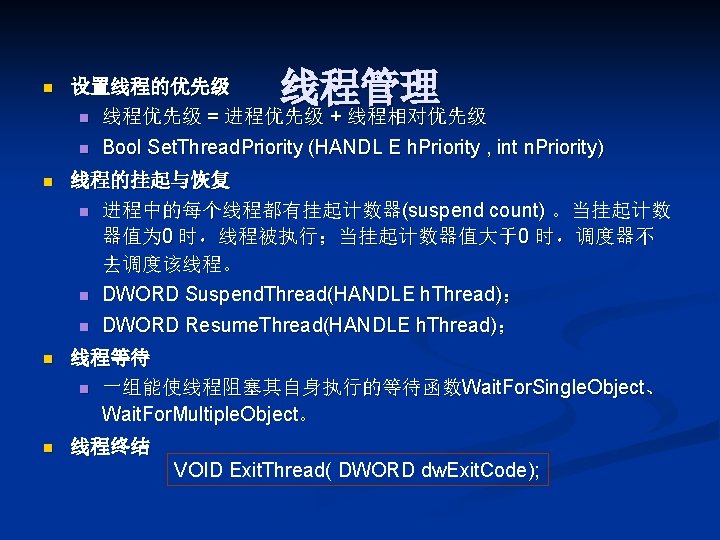
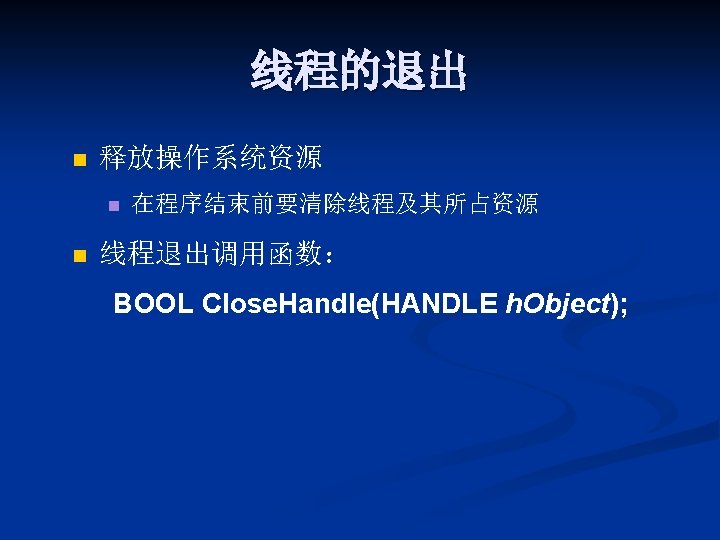
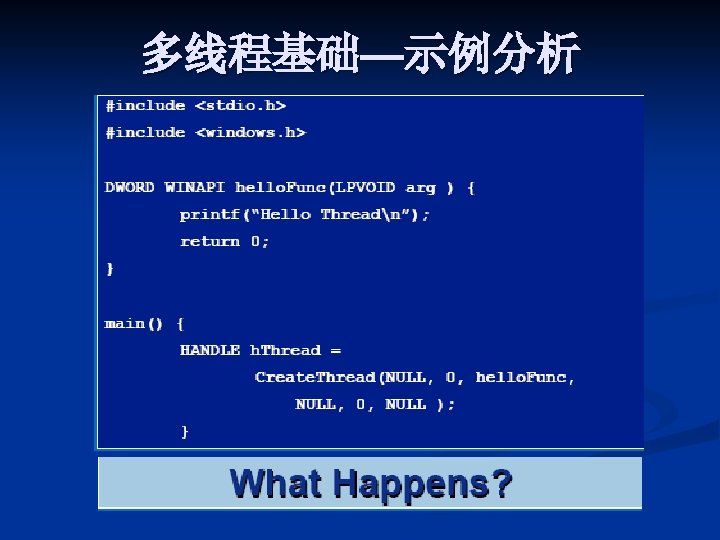
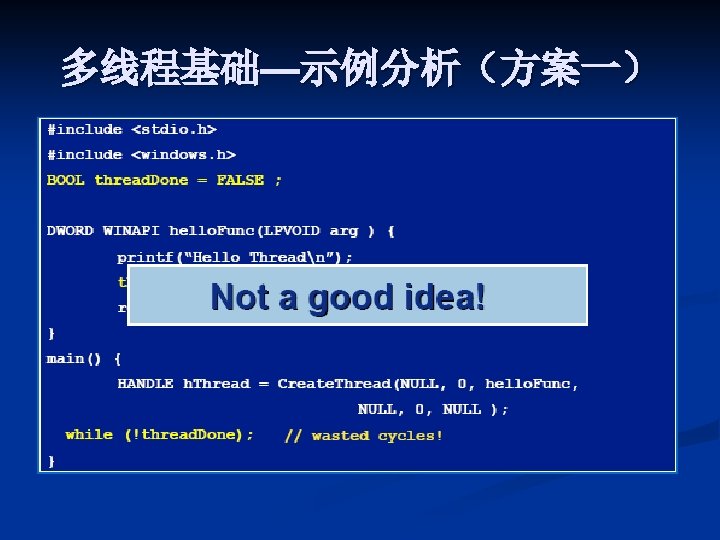
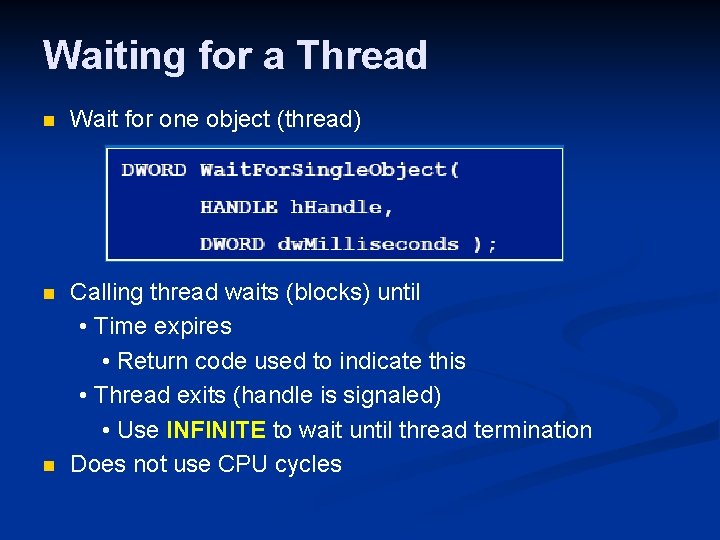
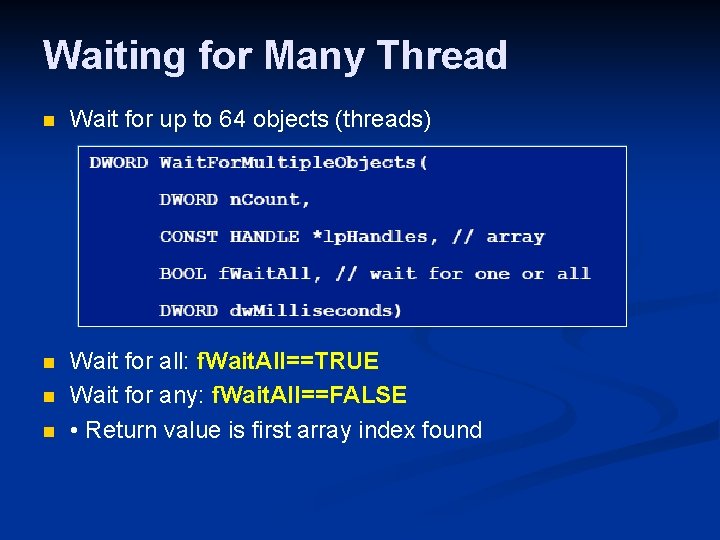
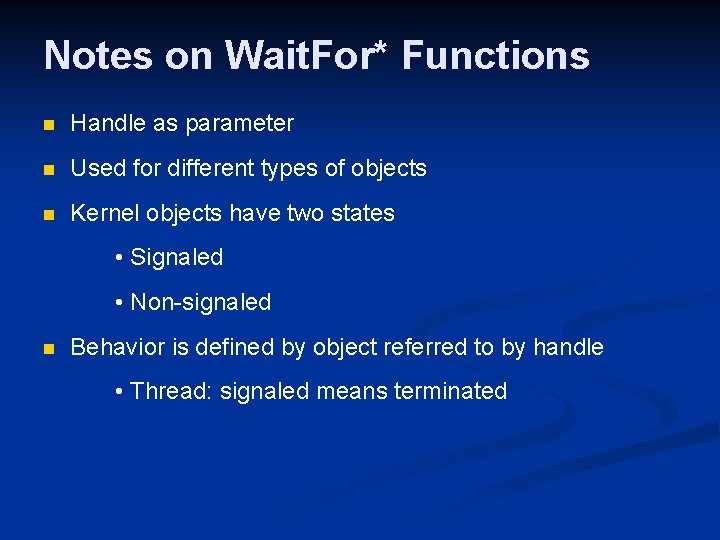
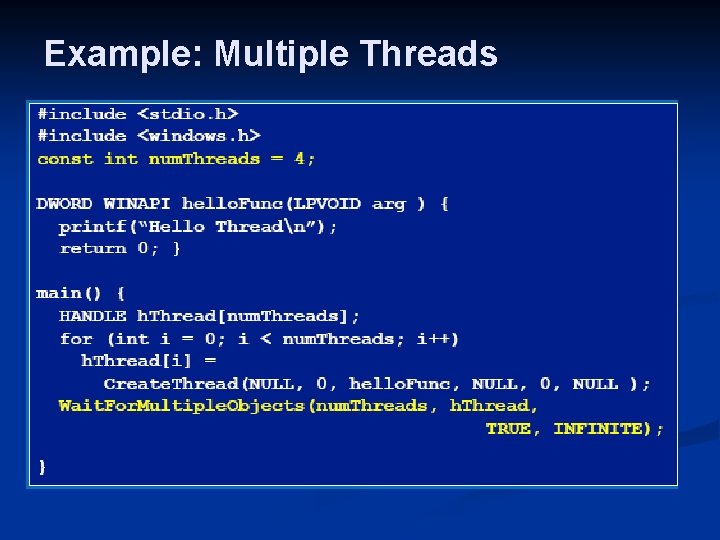
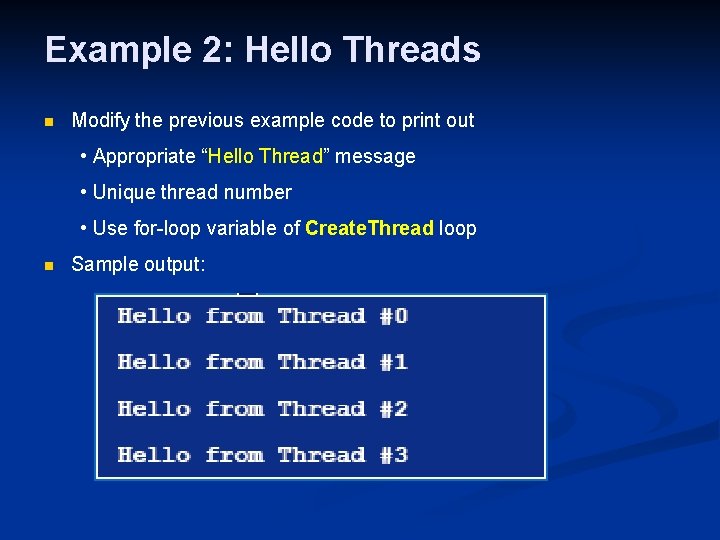
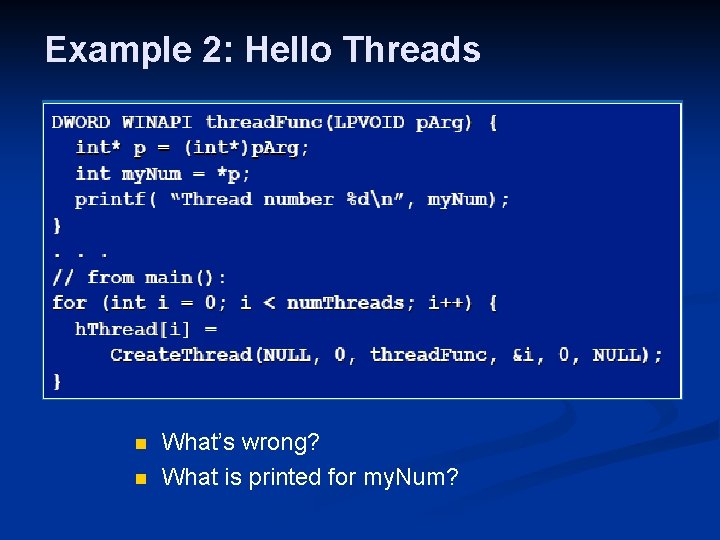
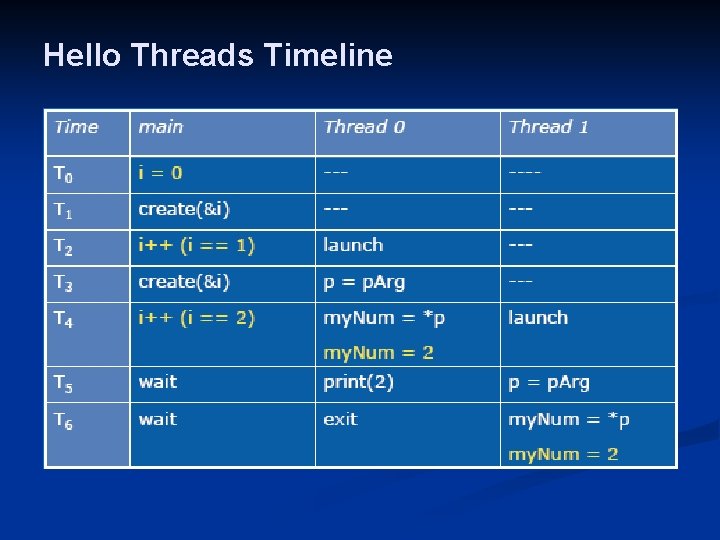
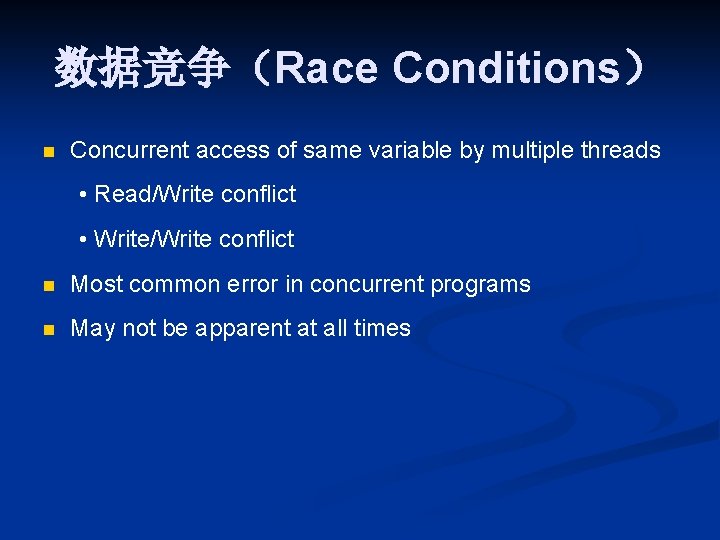
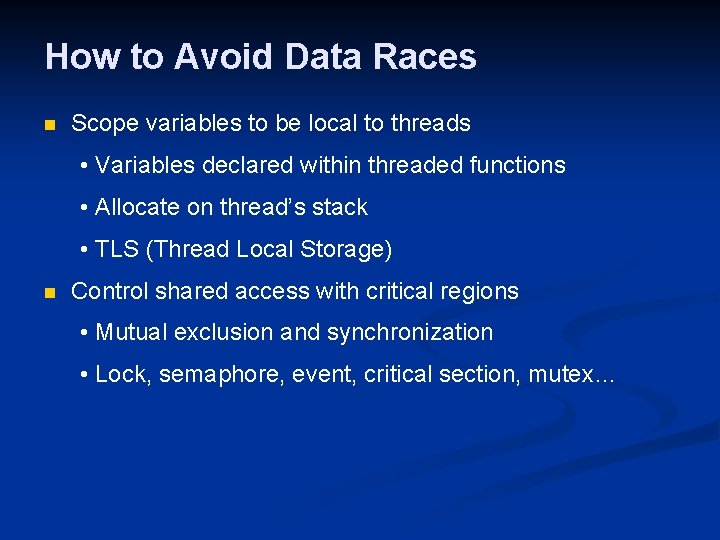
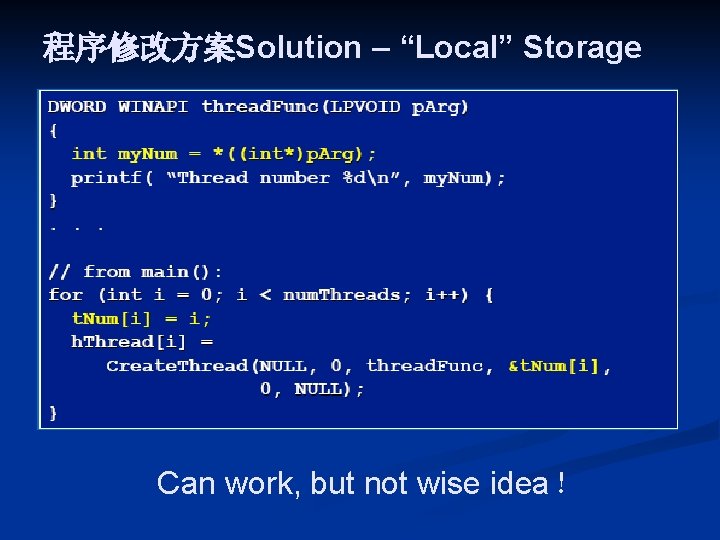
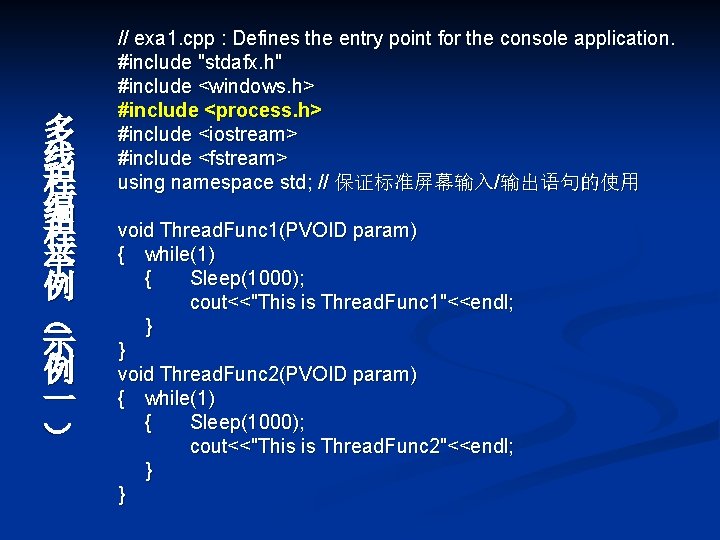
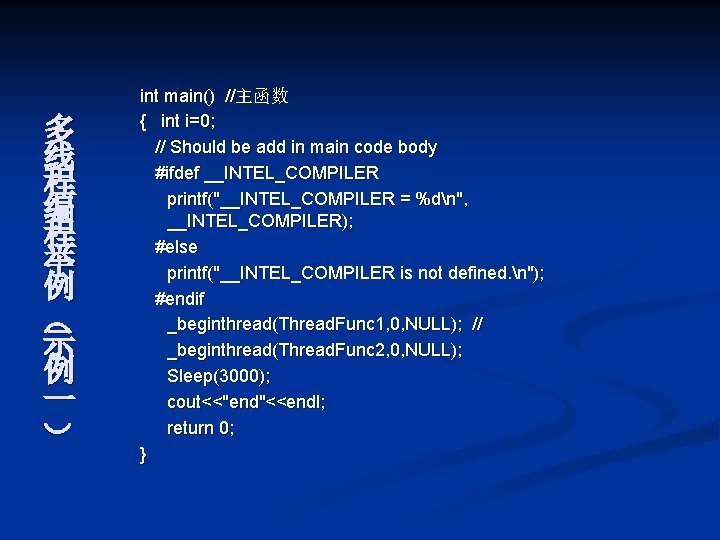
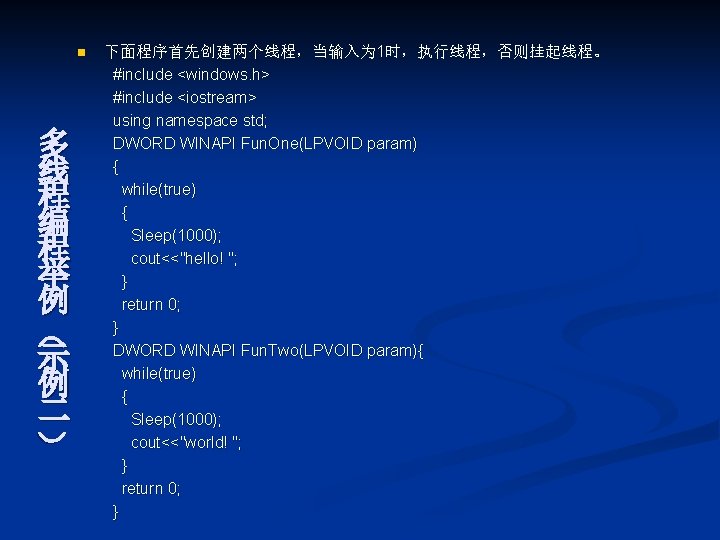
![多线程编程举例(示例二) int main(int argc, char* argv[]) { int input=0; HANDLE hand 1= Create. Thread 多线程编程举例(示例二) int main(int argc, char* argv[]) { int input=0; HANDLE hand 1= Create. Thread](https://slidetodoc.com/presentation_image/deebd894239ba8723b431a008c5d2b8e/image-66.jpg)
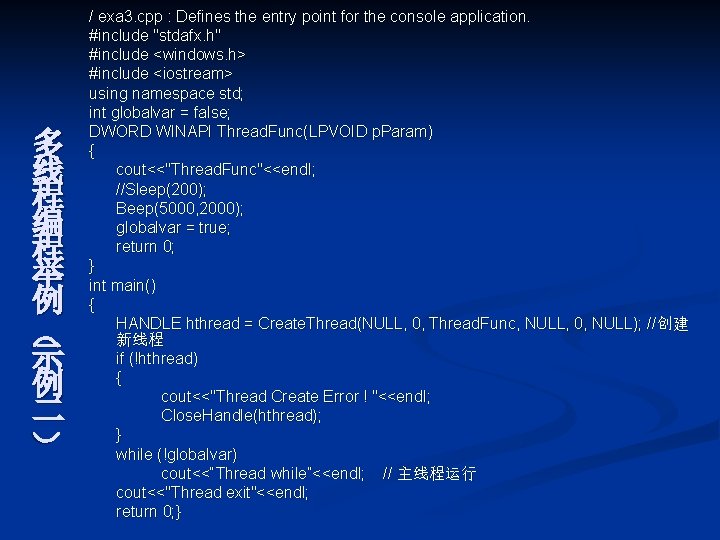
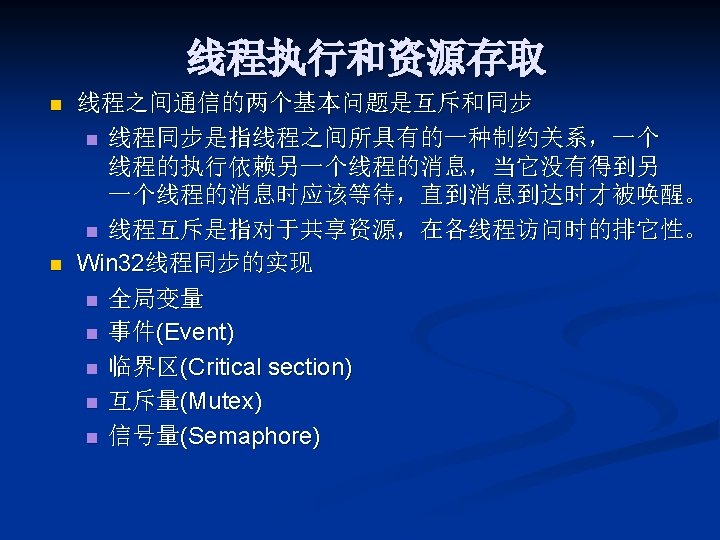
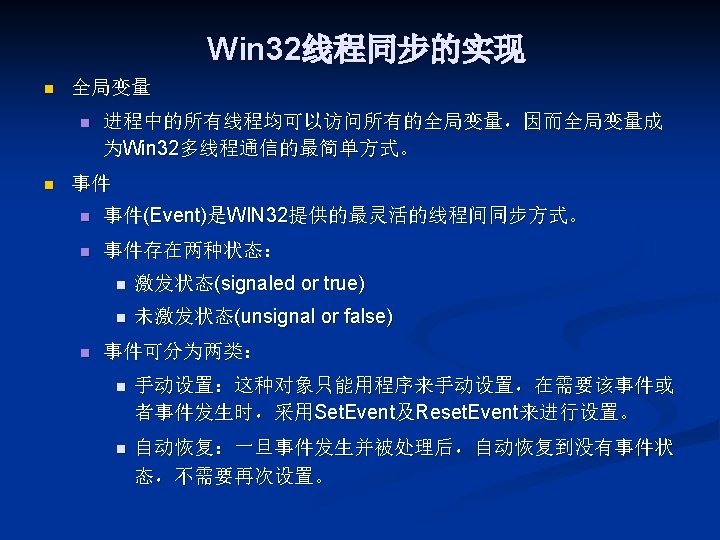
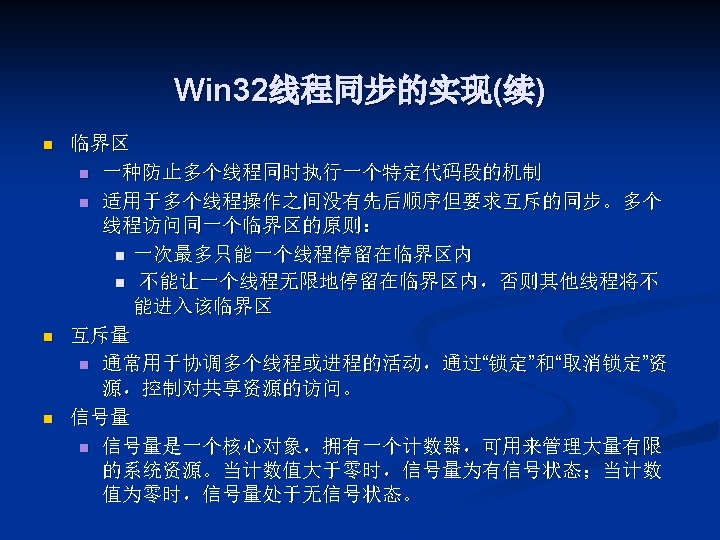
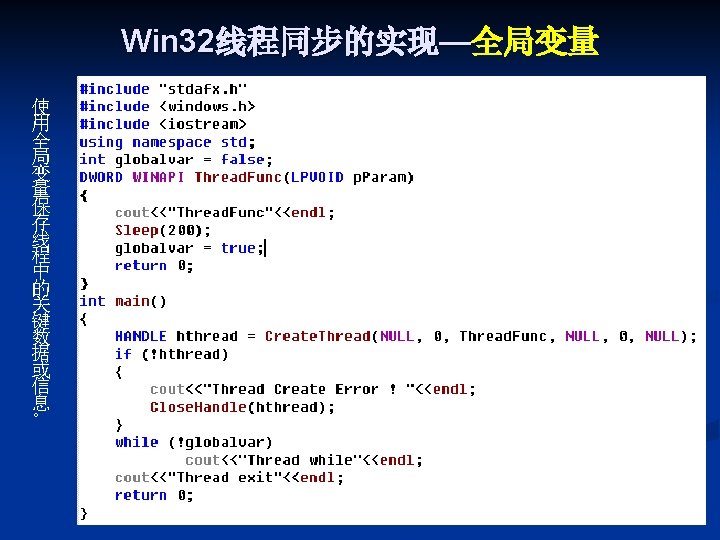
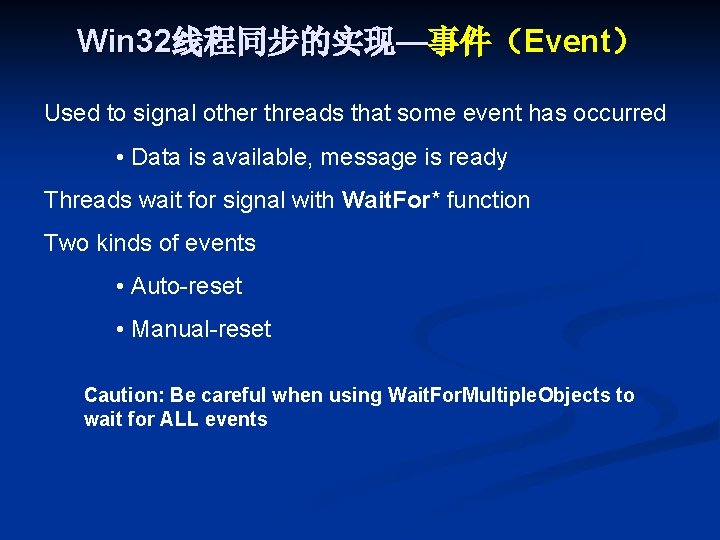
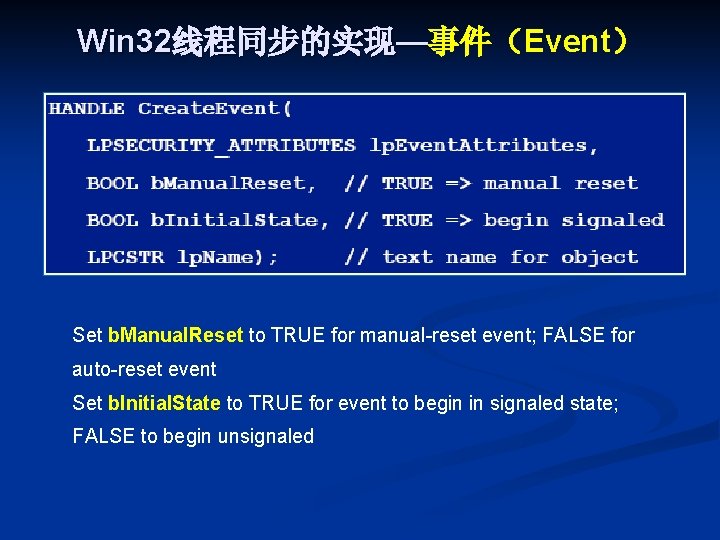
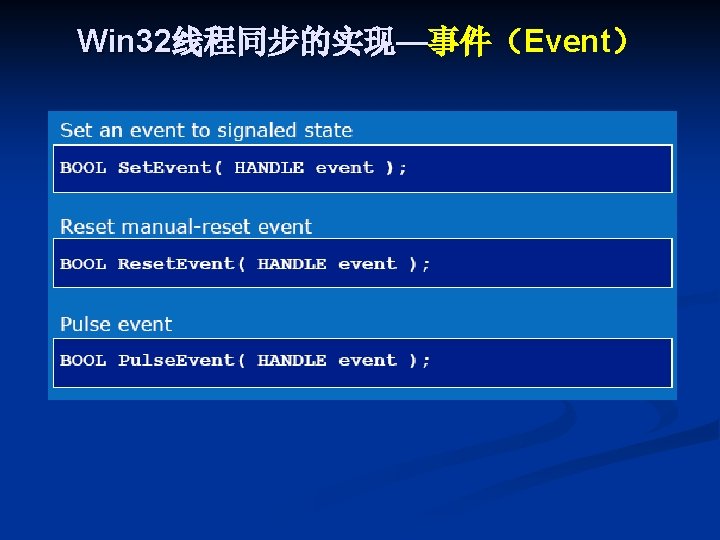
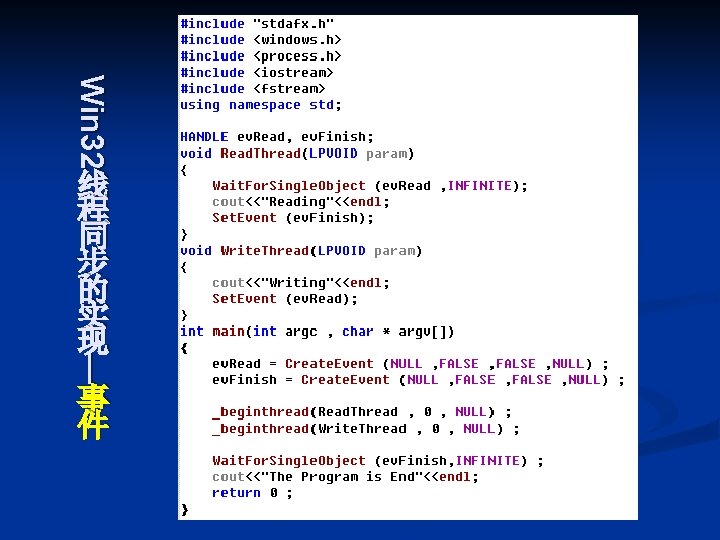
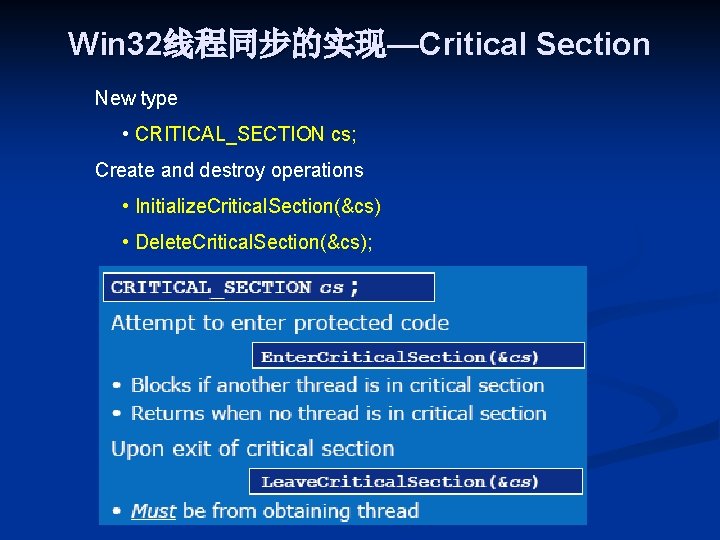
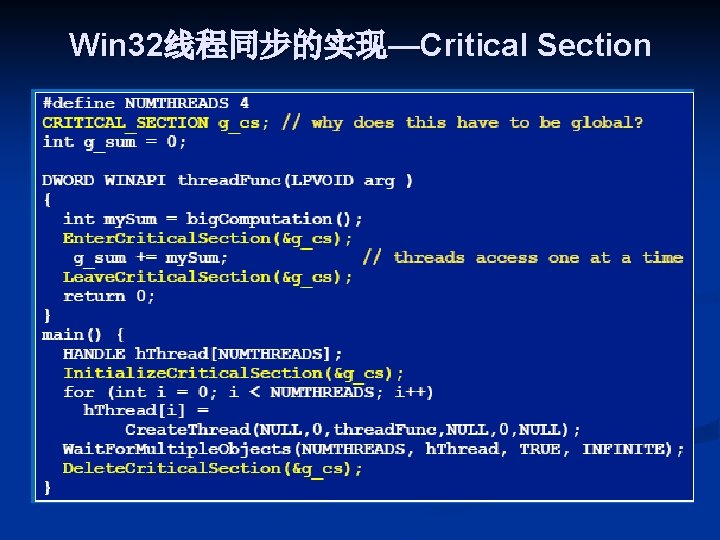
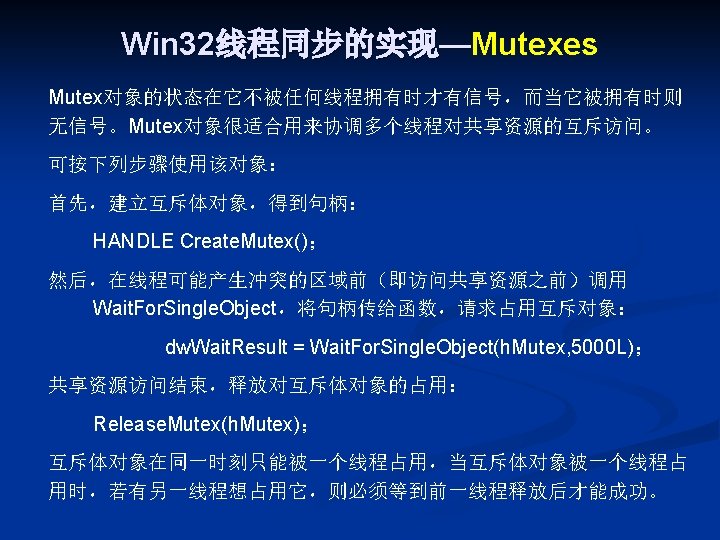
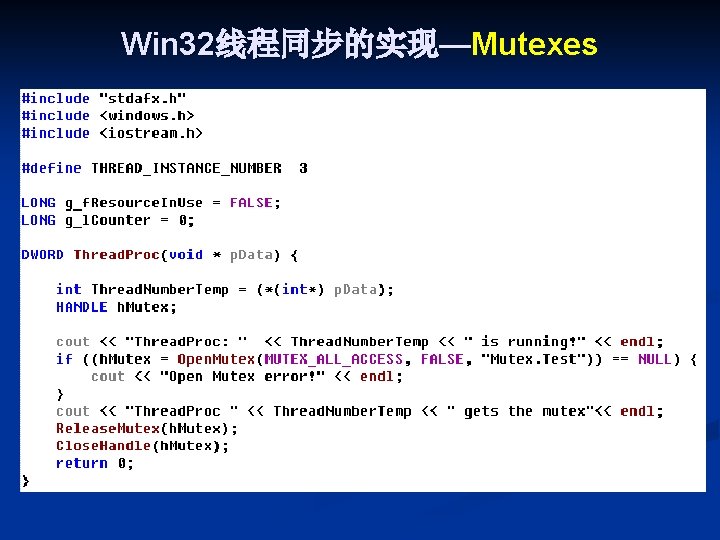
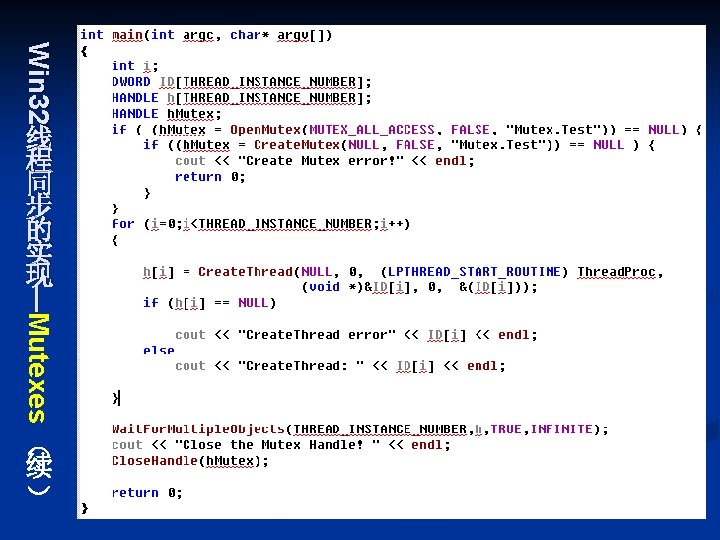
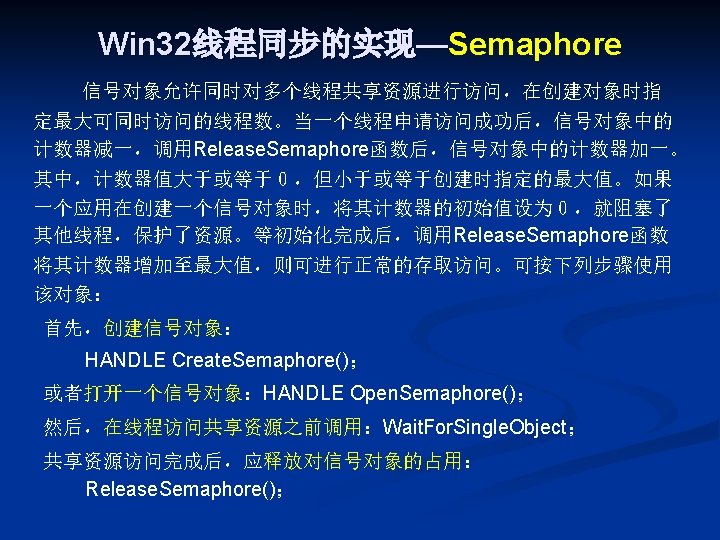
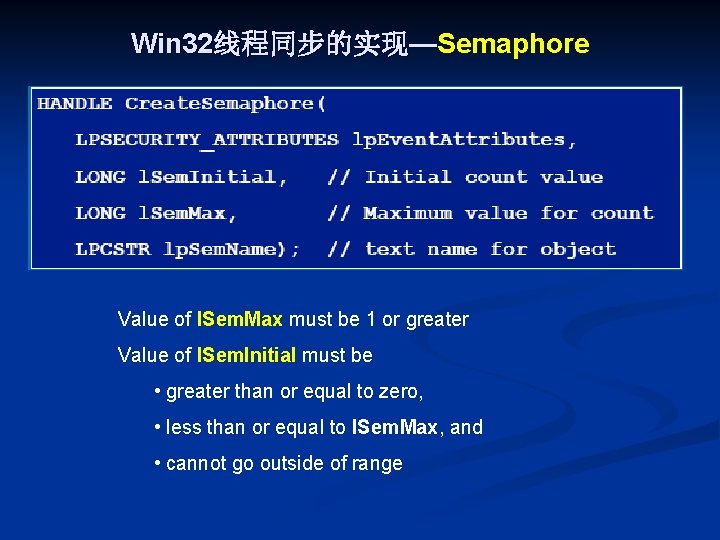
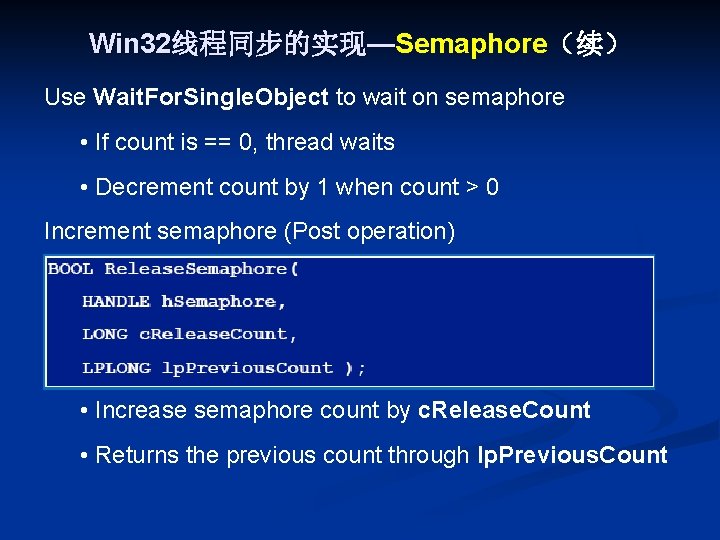
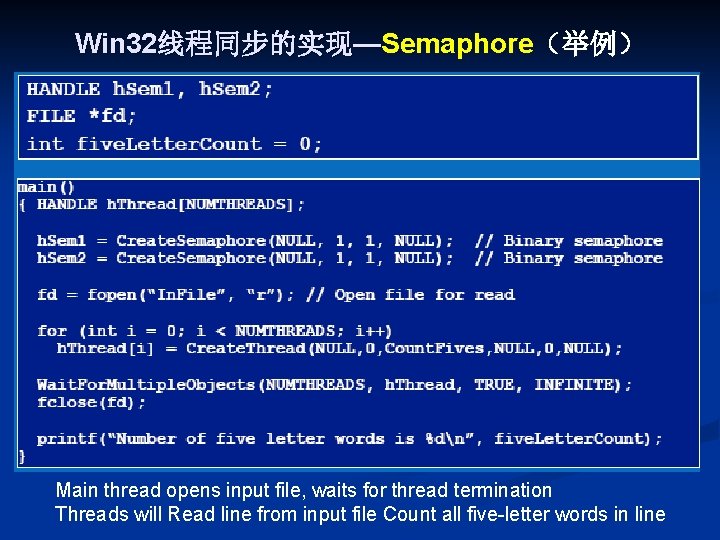
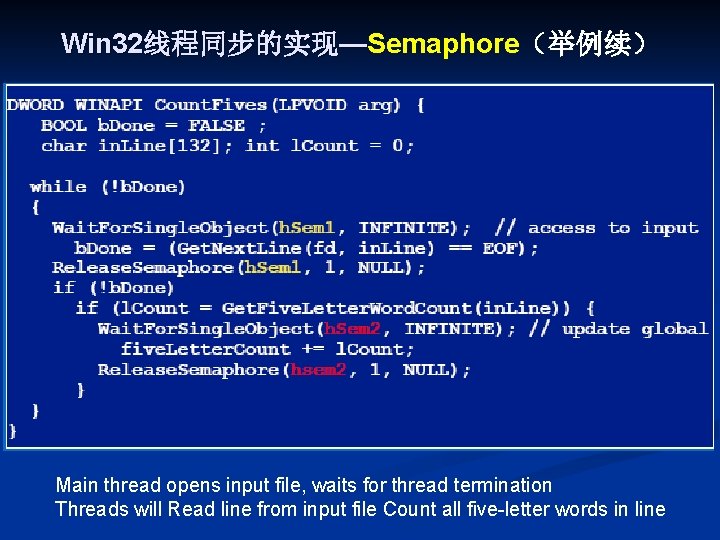
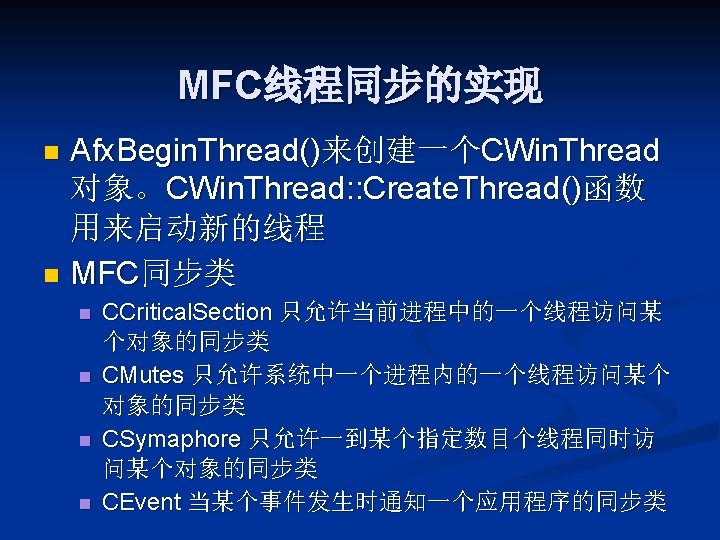
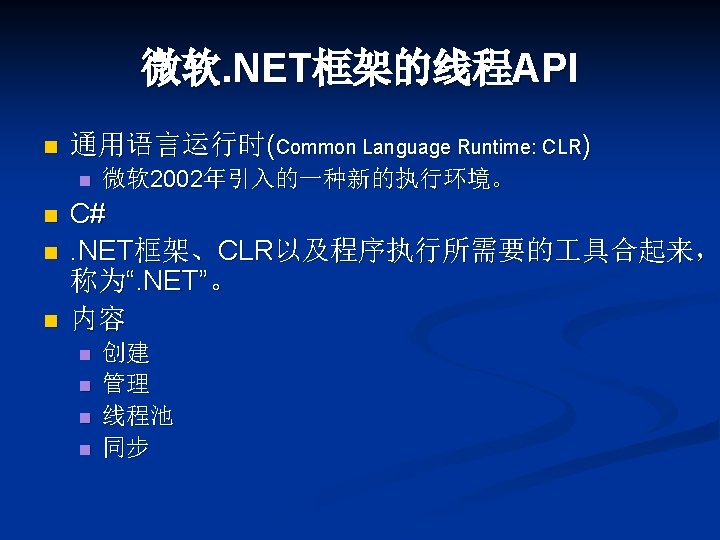
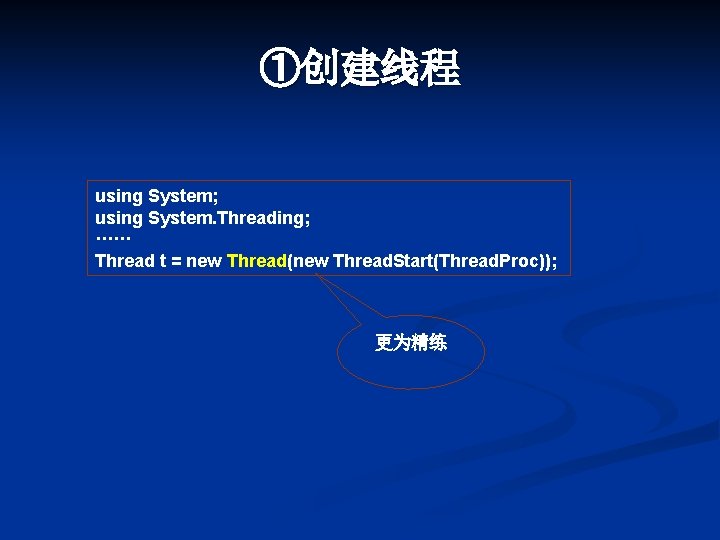
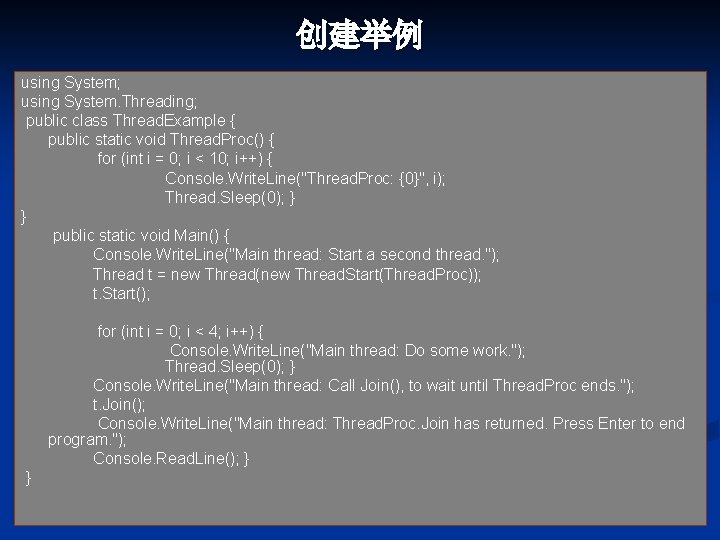
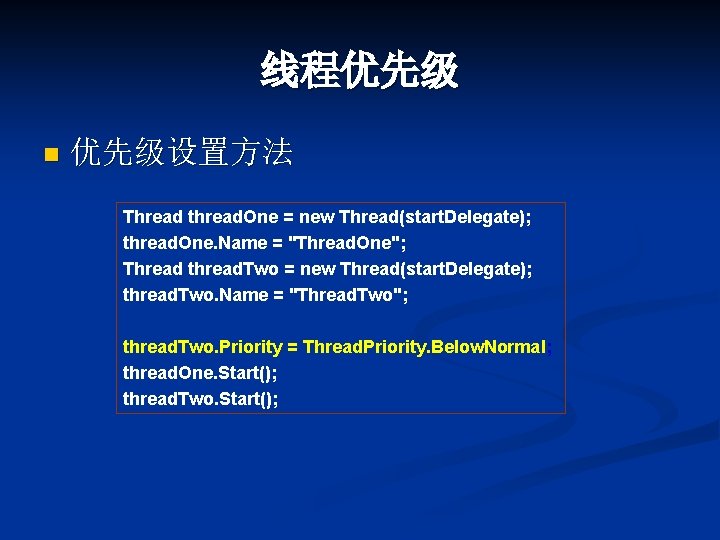
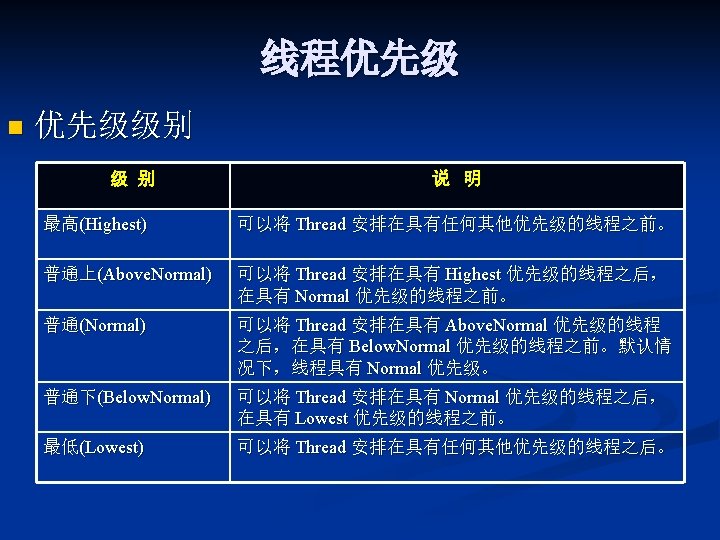
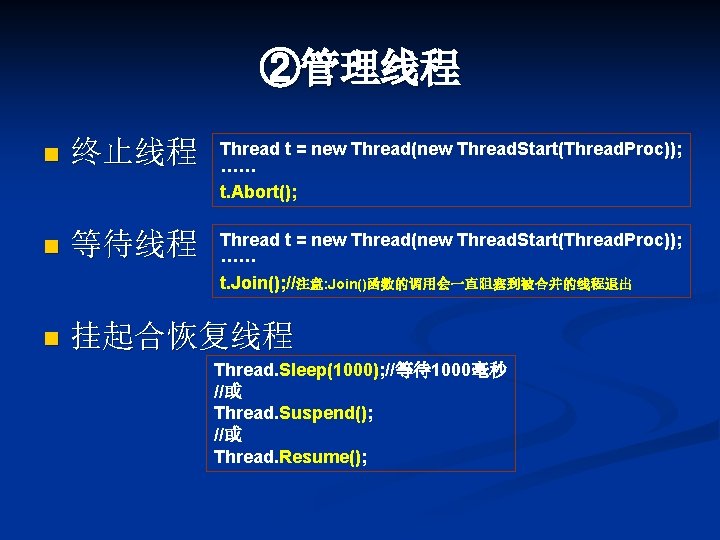
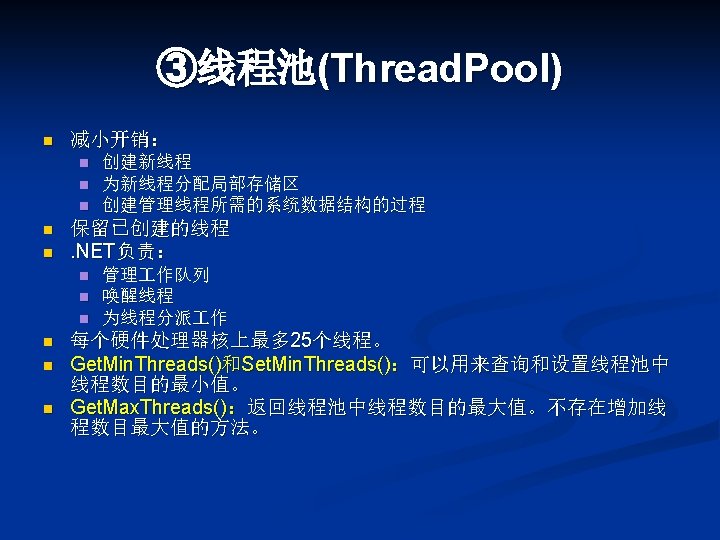
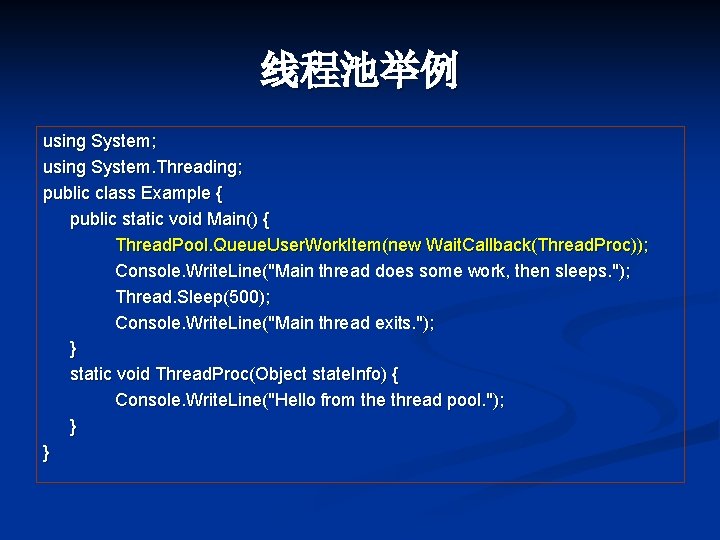
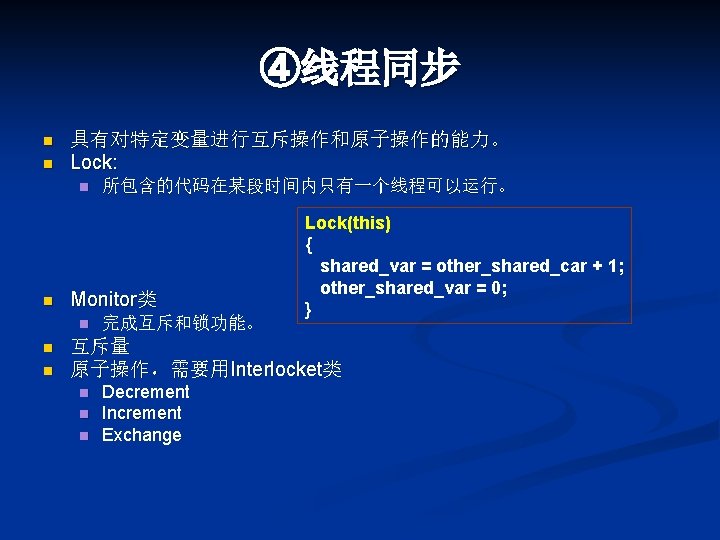
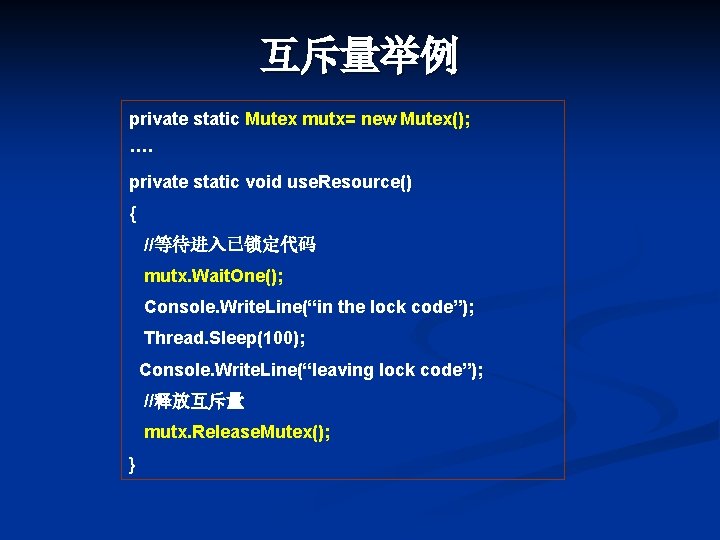
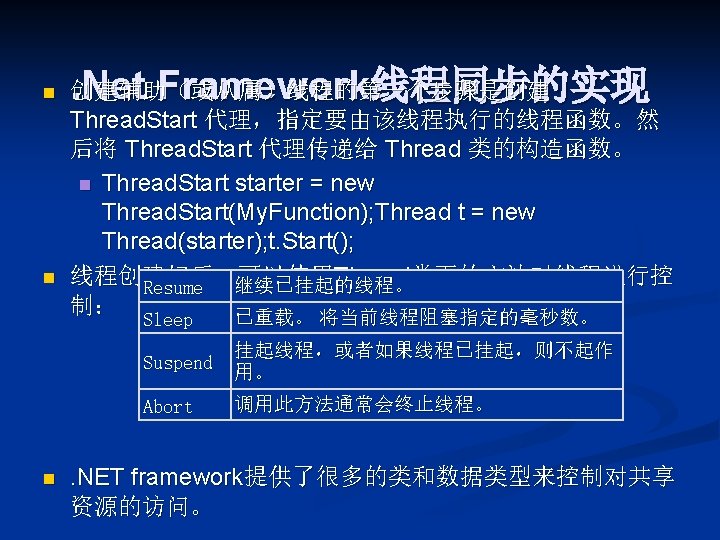
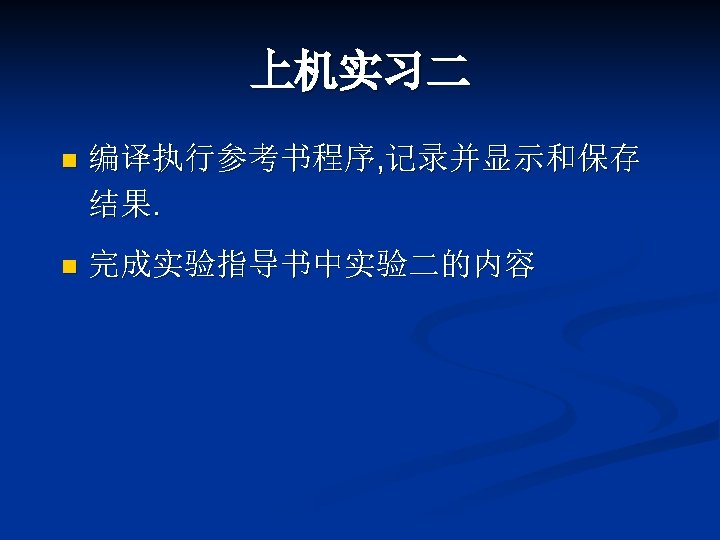
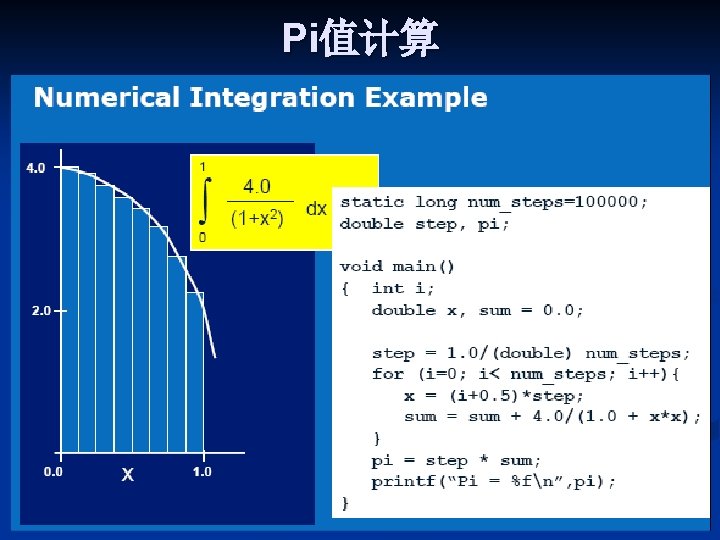
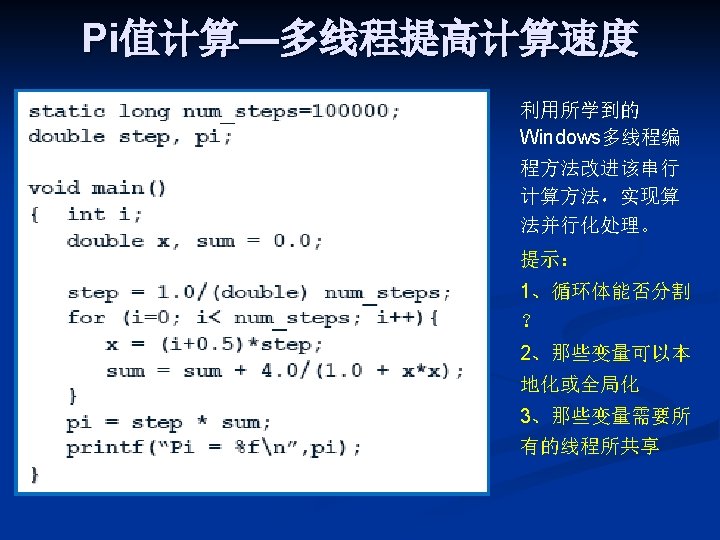
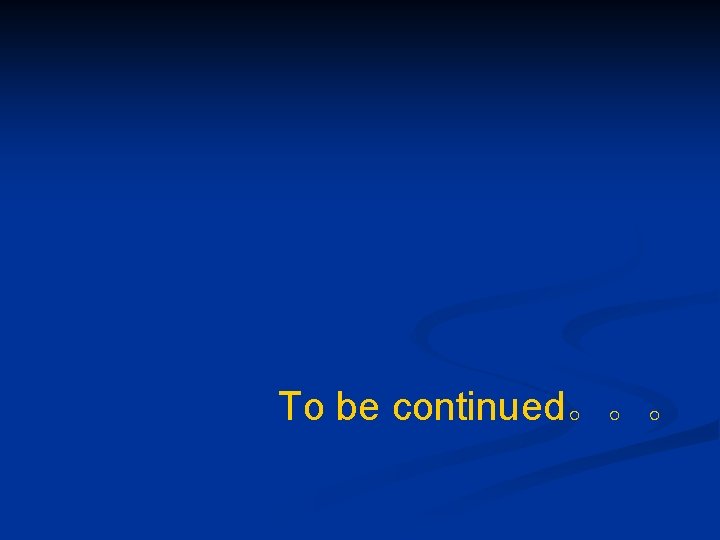
- Slides: 101
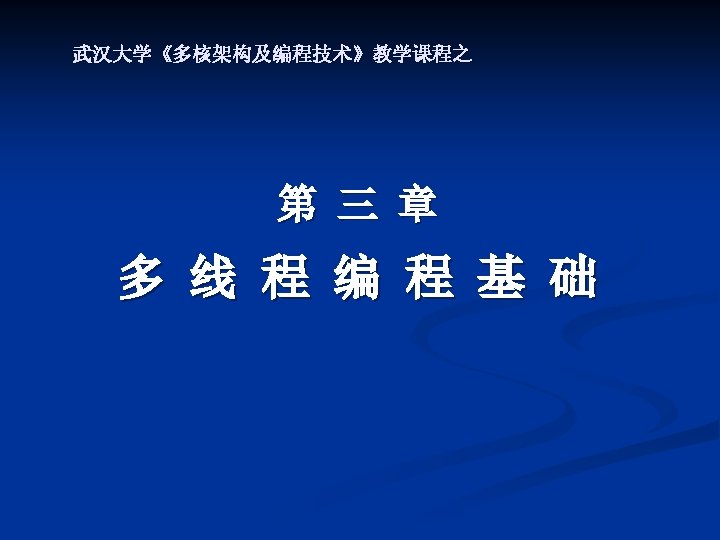
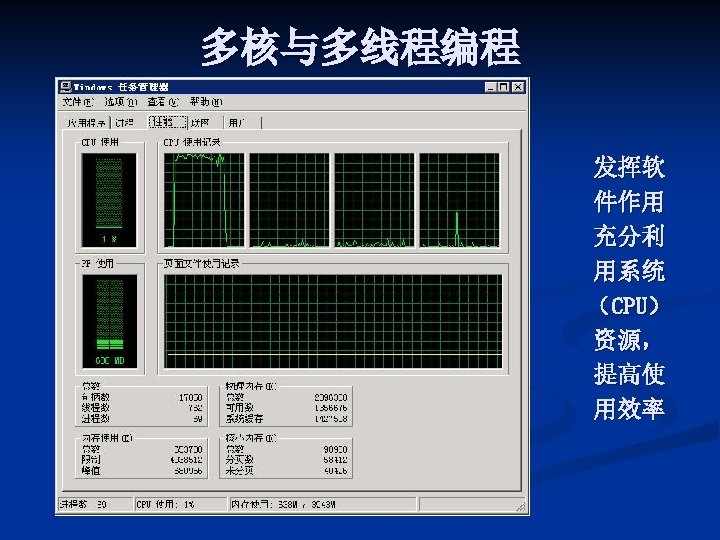
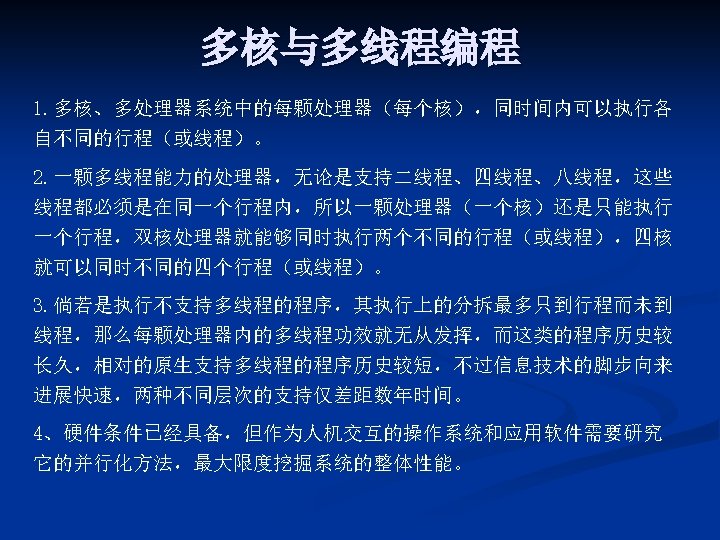
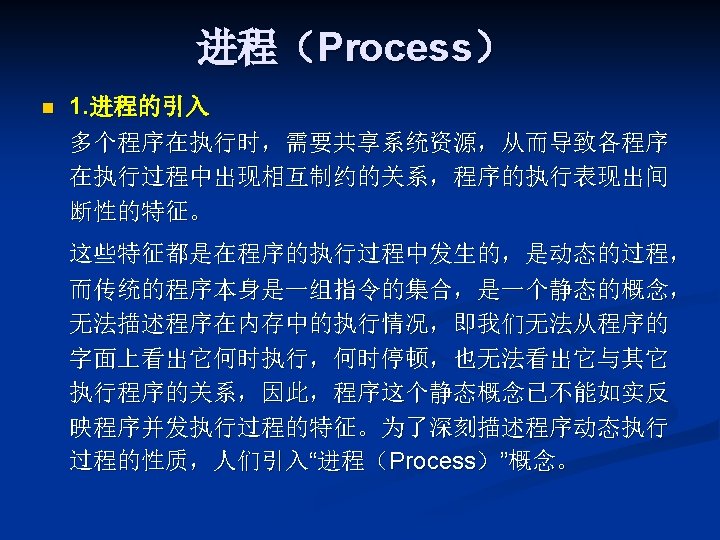
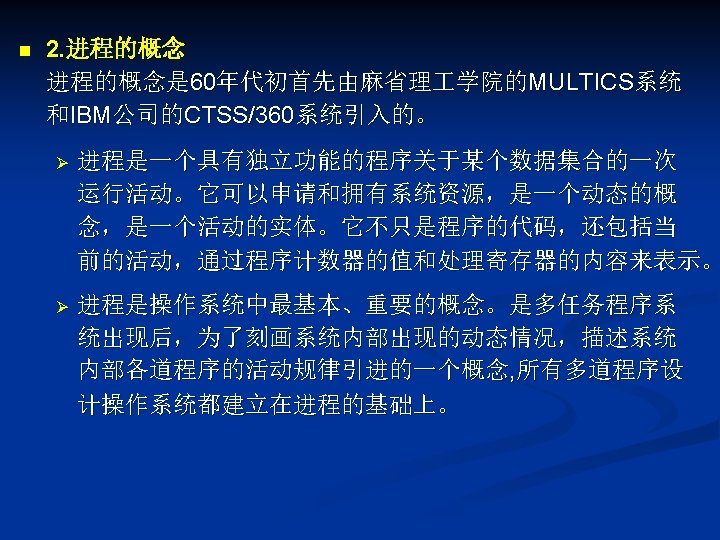
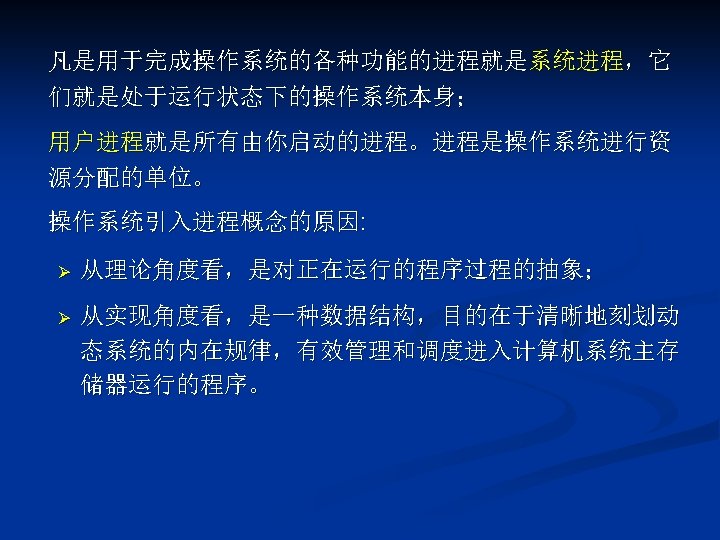
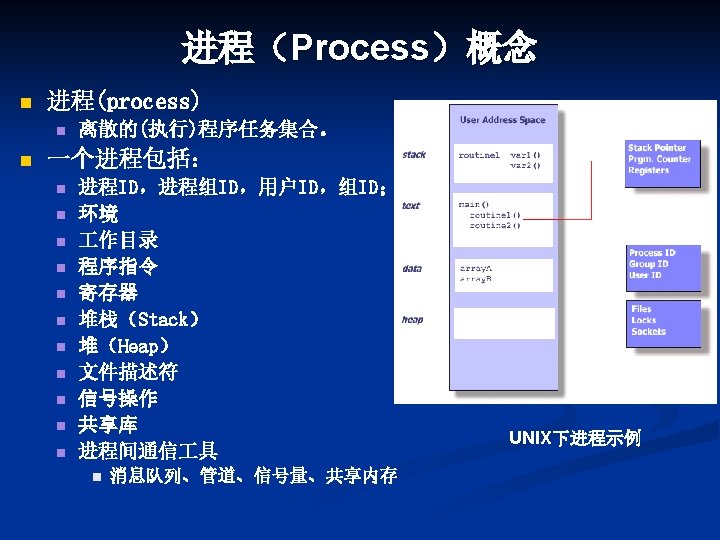
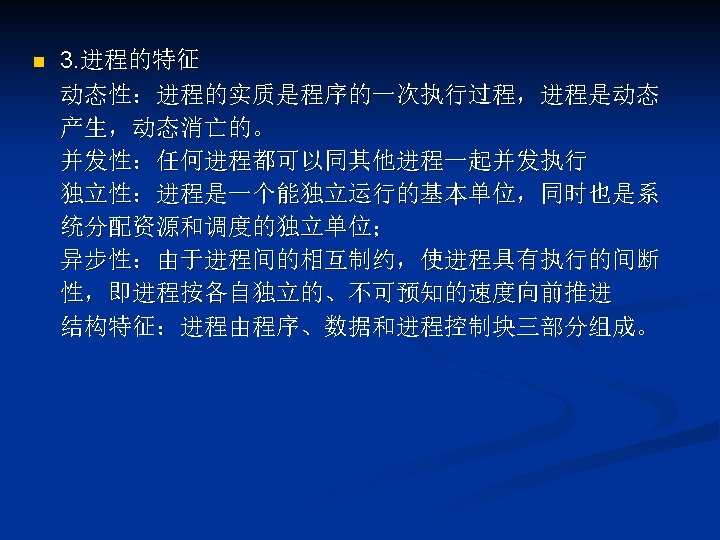
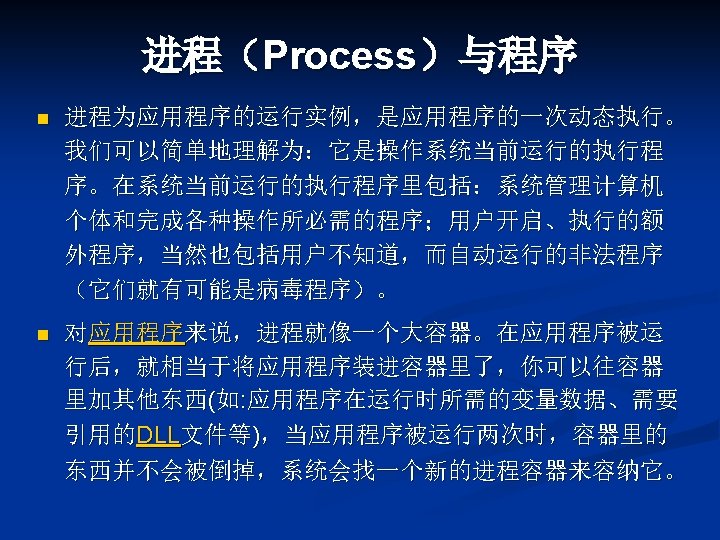
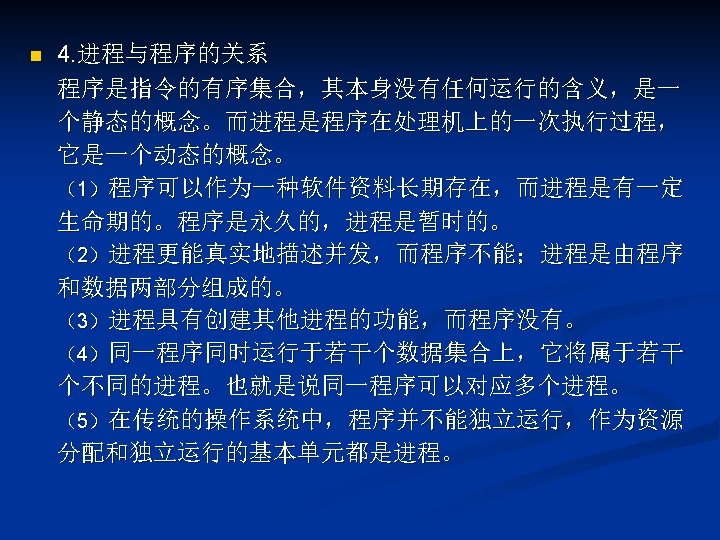
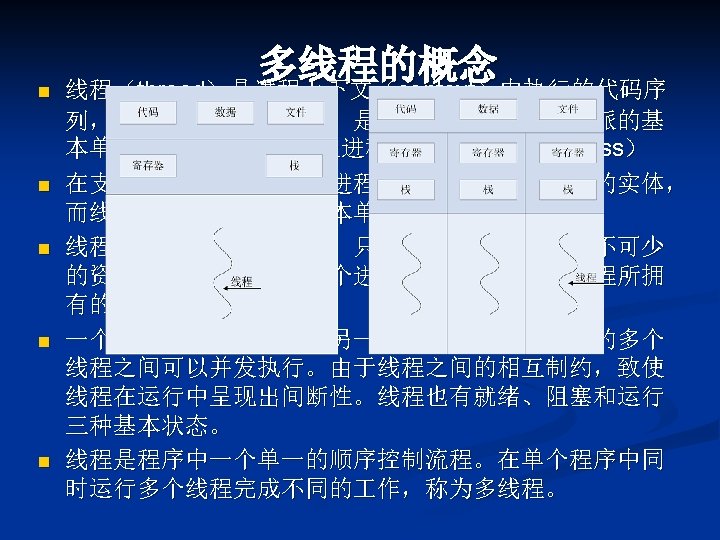
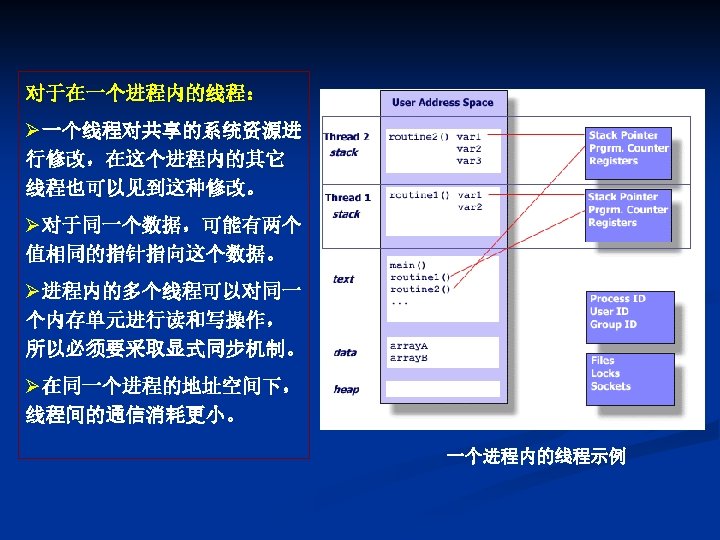
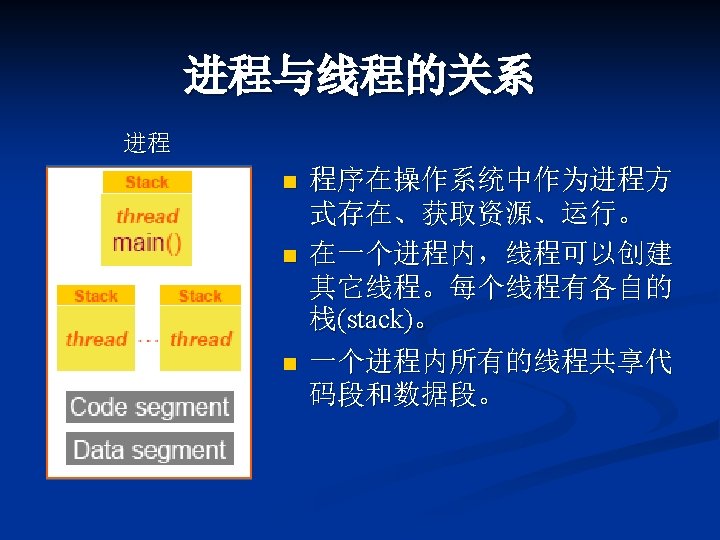
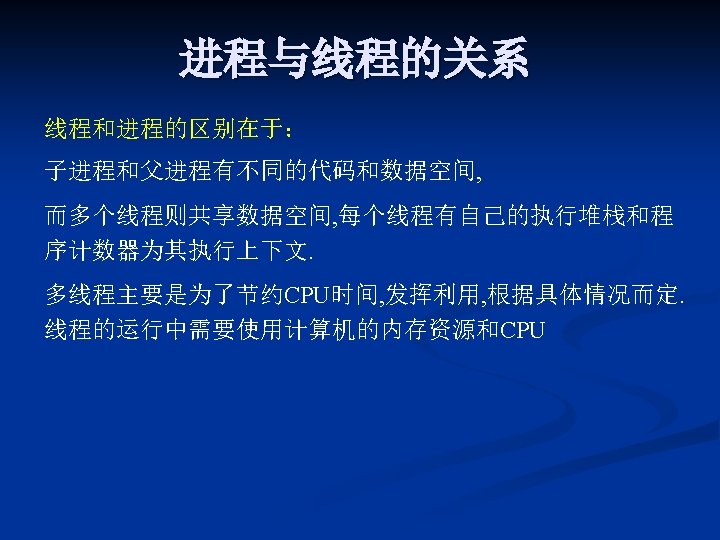
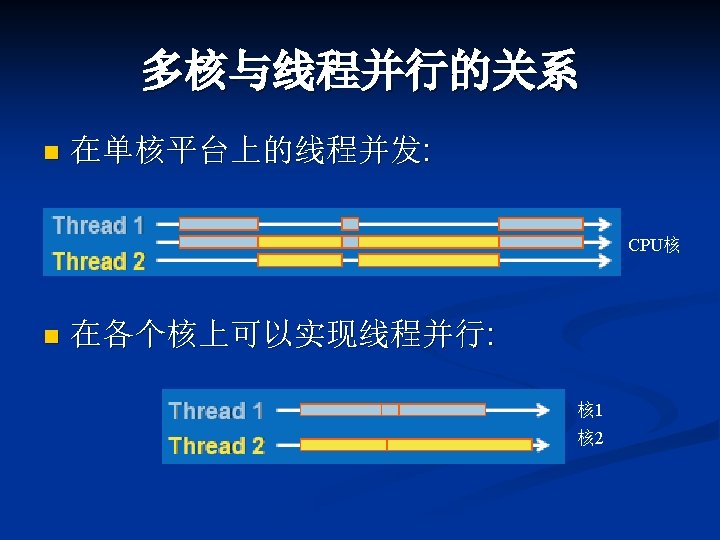
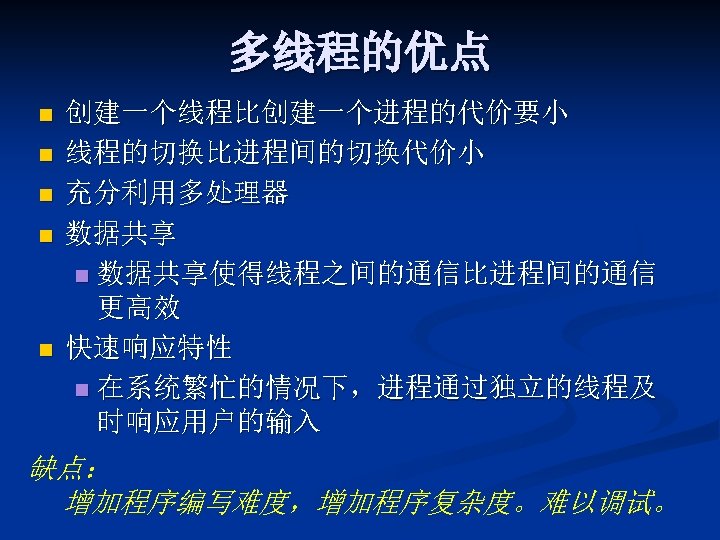
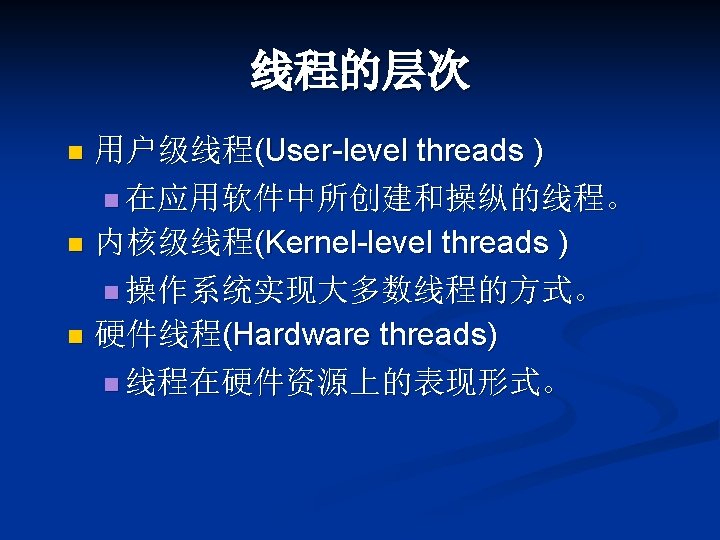
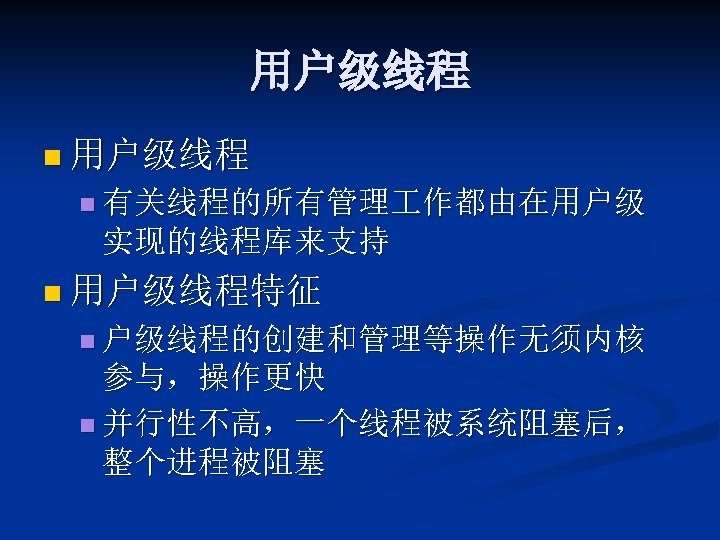
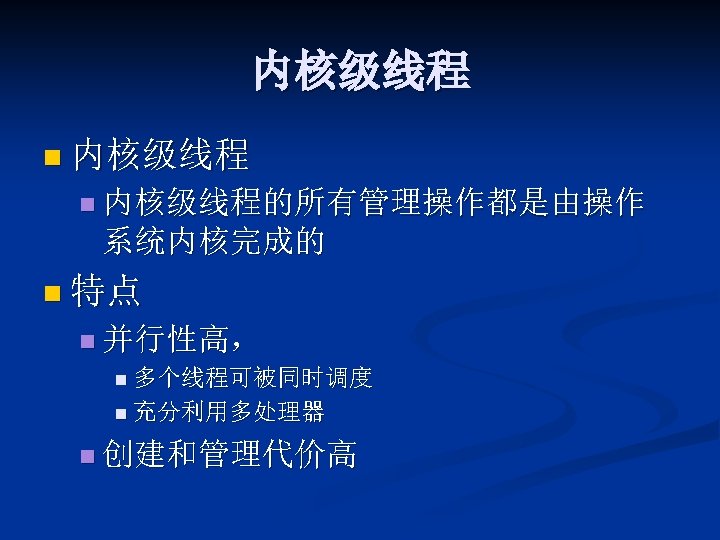
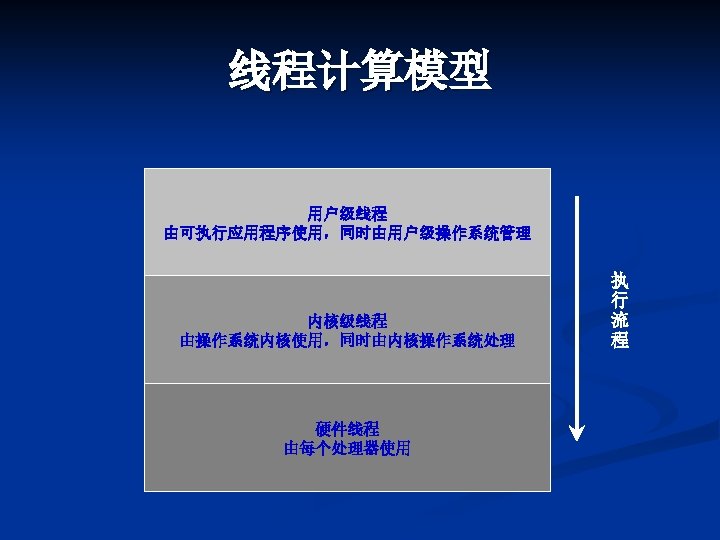
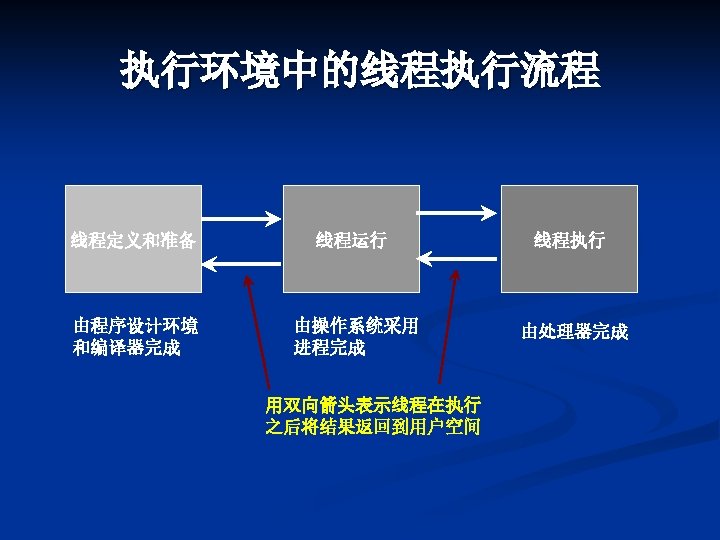
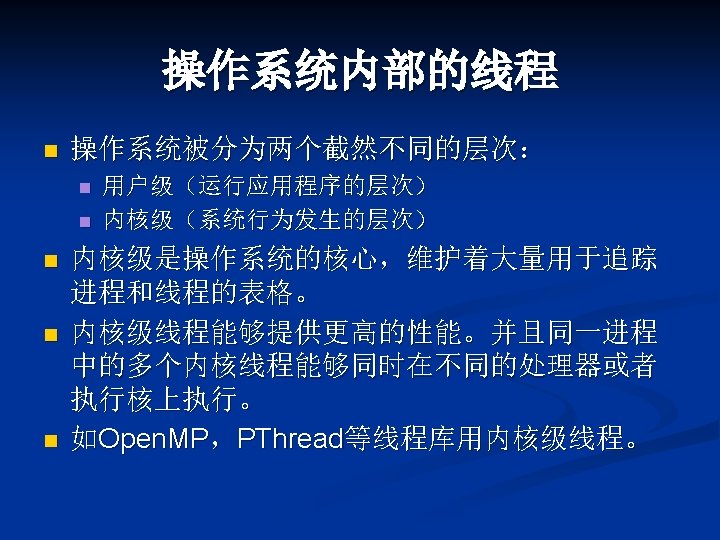
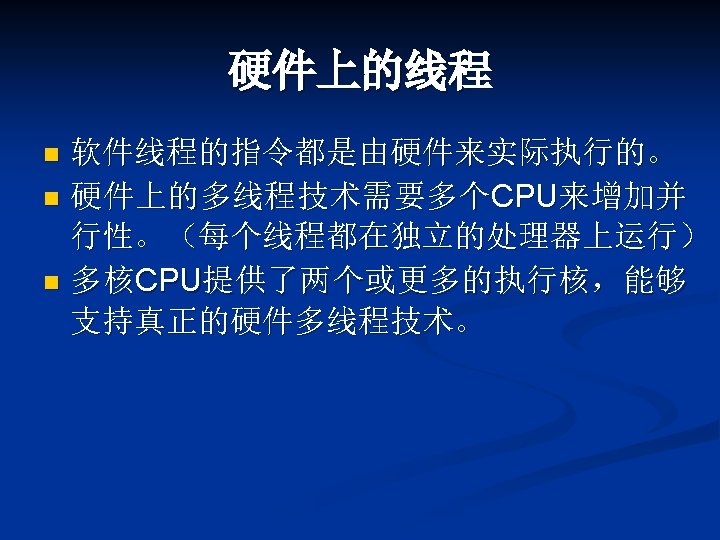
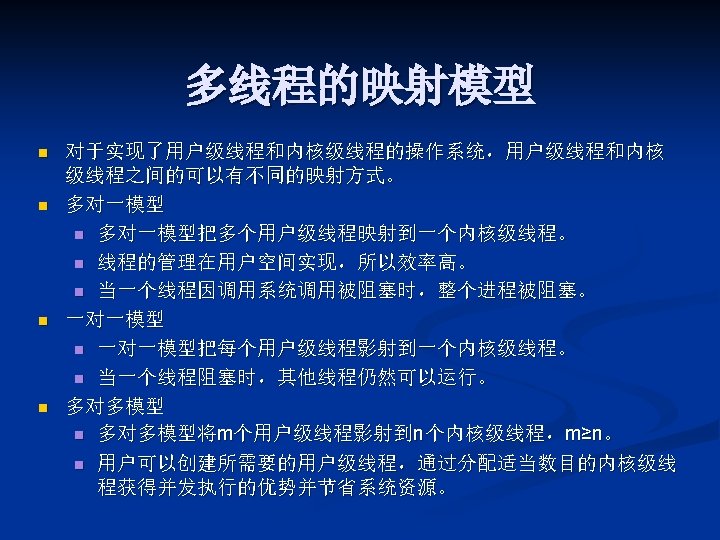
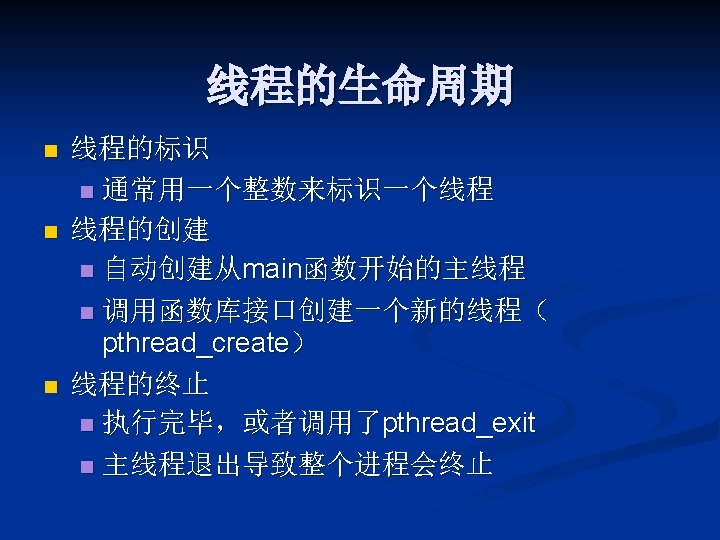
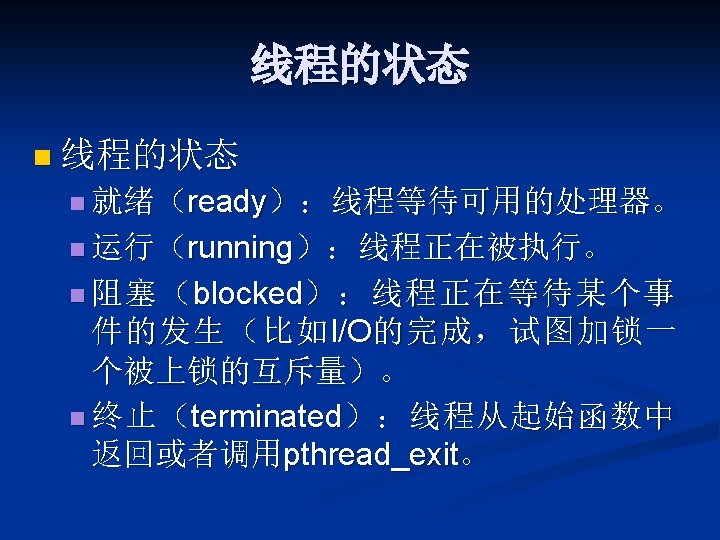
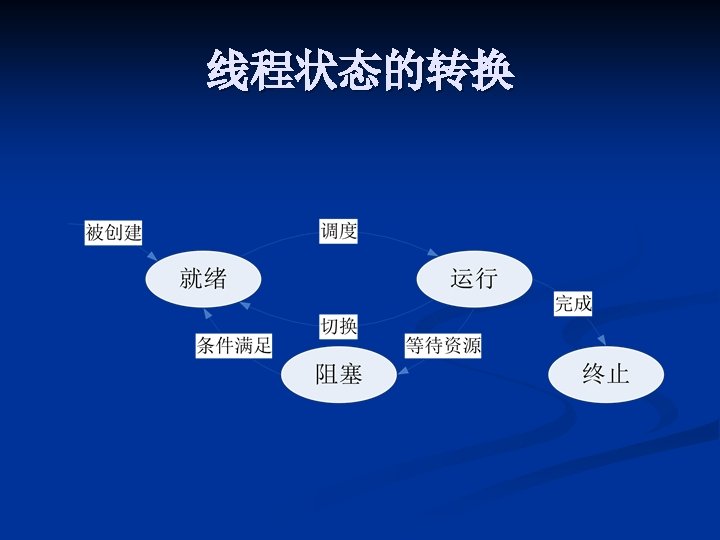
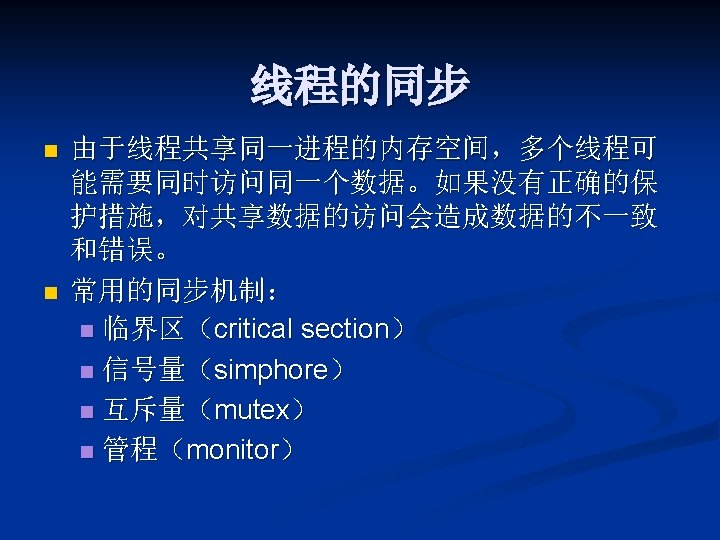
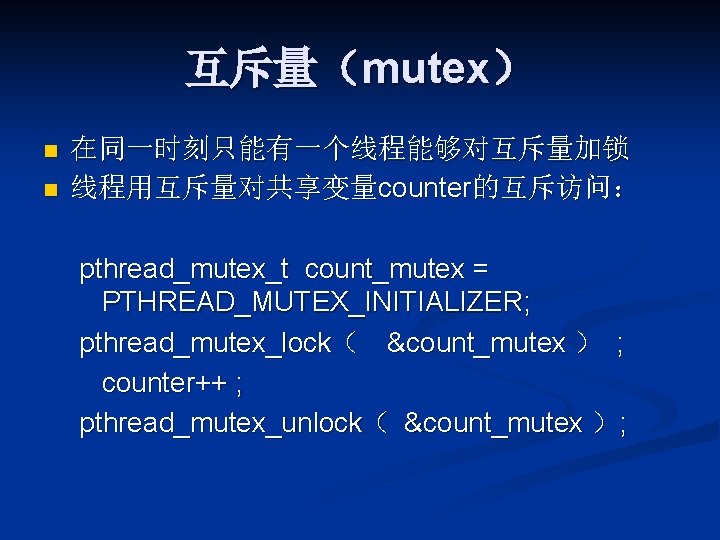
互斥量(mutex) n n 在同一时刻只能有一个线程能够对互斥量加锁 线程用互斥量对共享变量counter的互斥访问: pthread_mutex_t count_mutex = PTHREAD_MUTEX_INITIALIZER; pthread_mutex_lock( &count_mutex ) ; counter++ ; pthread_mutex_unlock( &count_mutex );
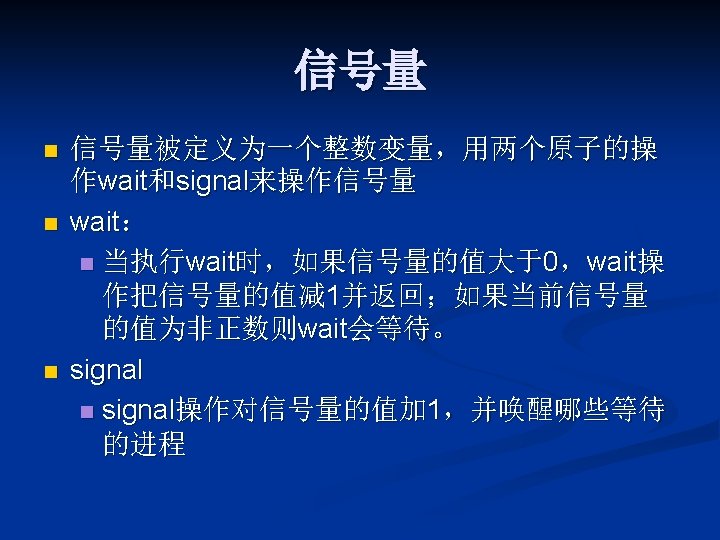
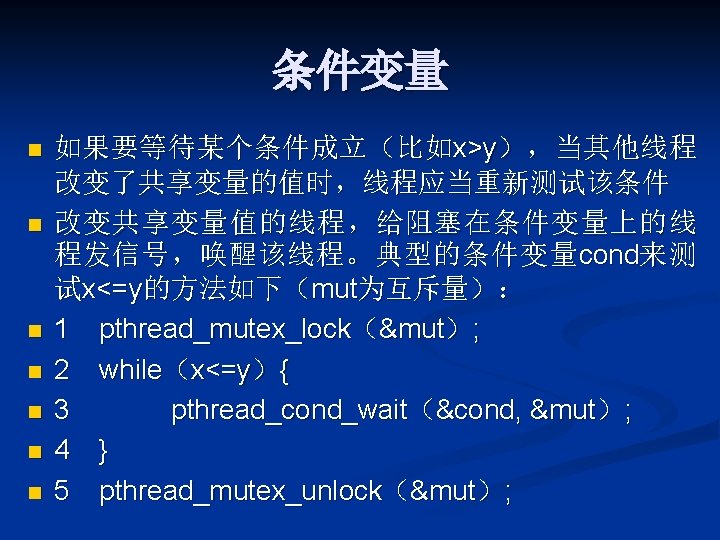
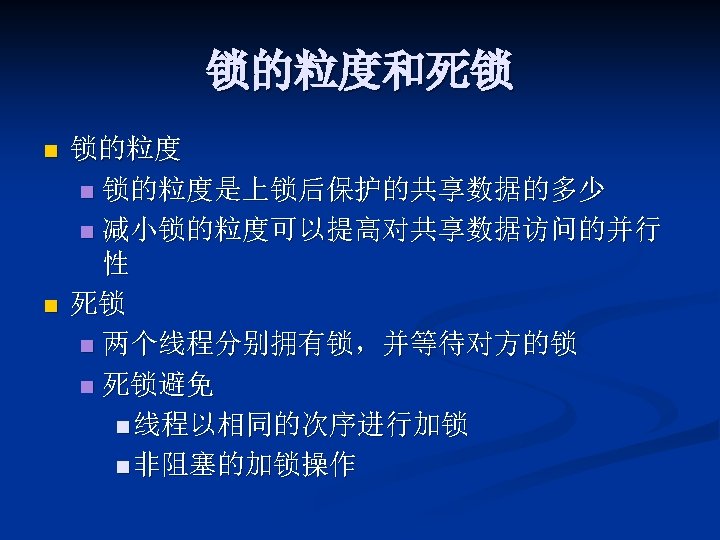
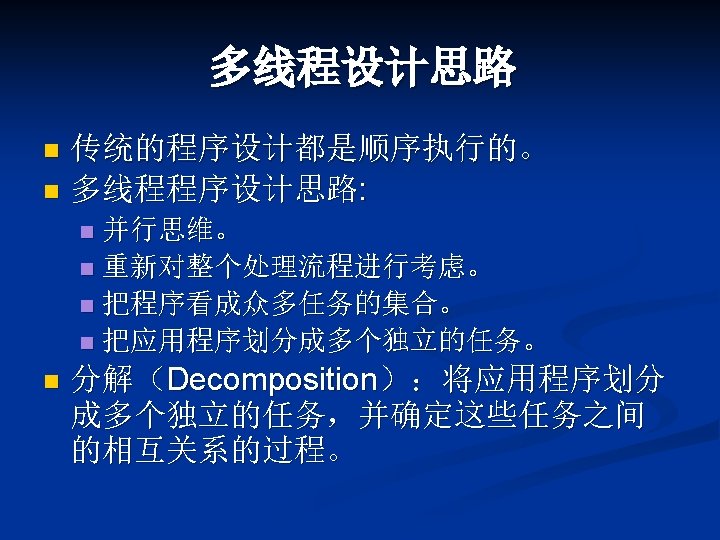
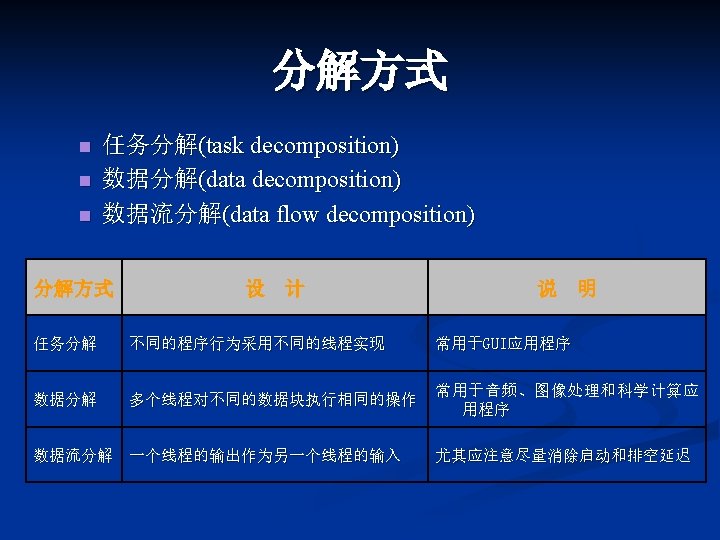
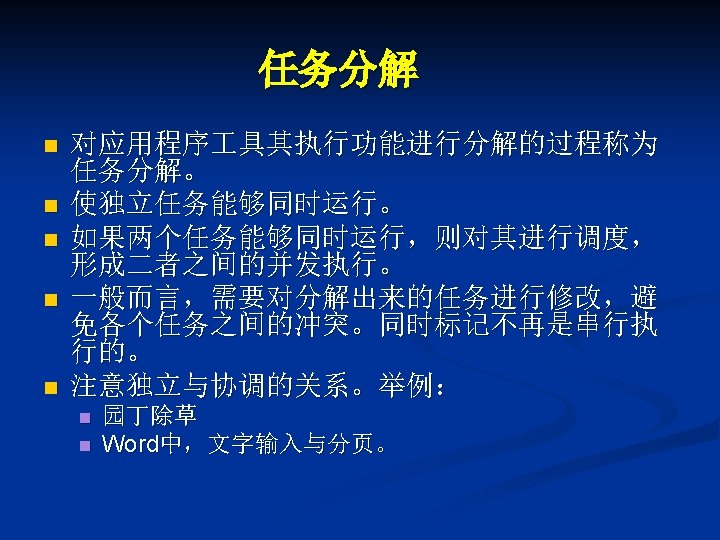
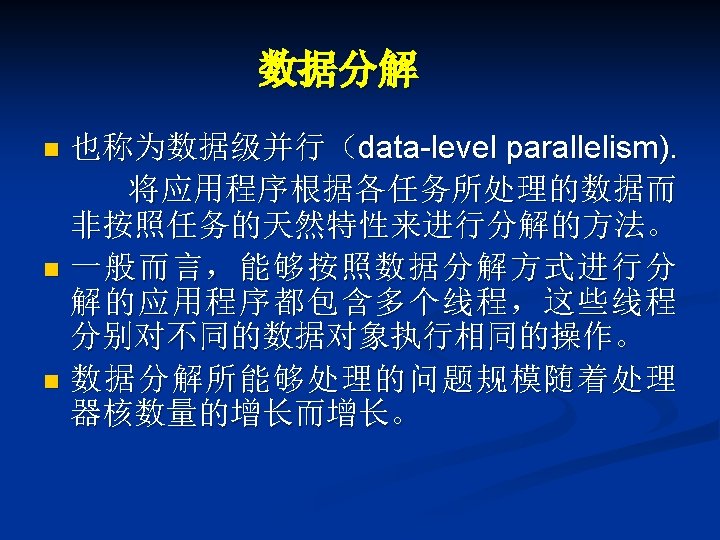
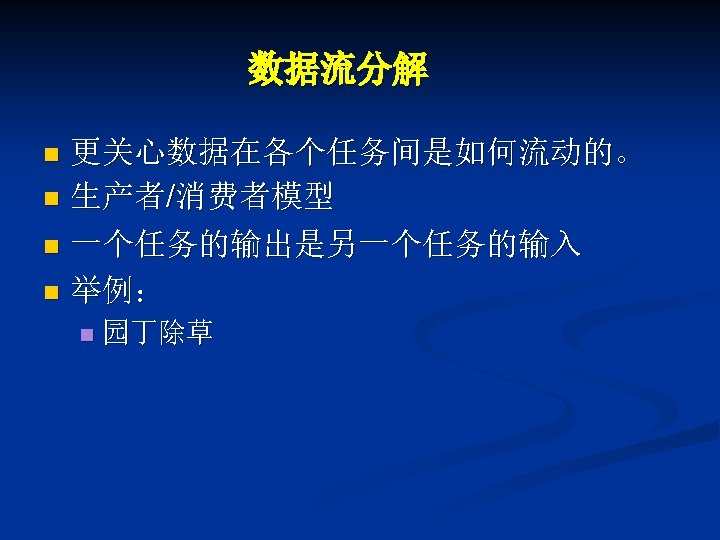
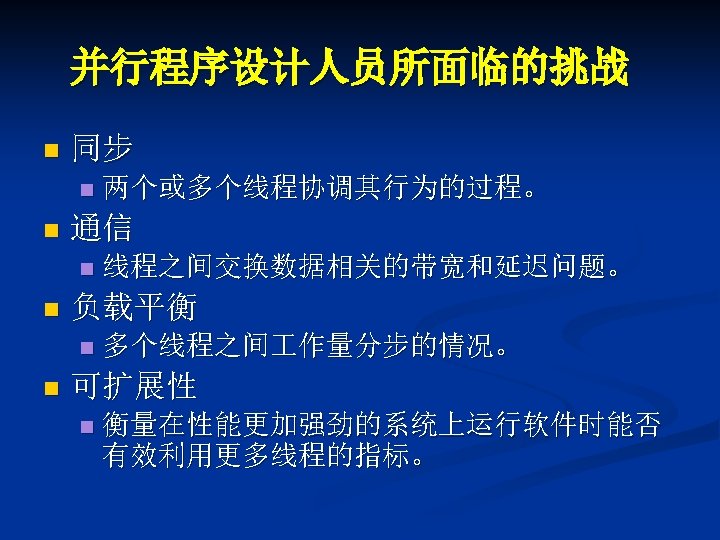
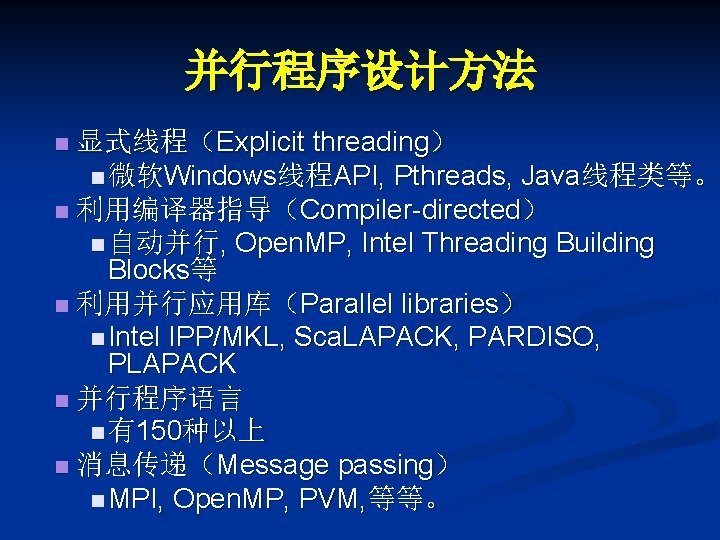
并行程序设计方法 显式线程(Explicit threading) n 微软Windows线程API, Pthreads, Java线程类等。 n 利用编译器指导(Compiler-directed) n 自动并行, Open. MP, Intel Threading Building Blocks等 n 利用并行应用库(Parallel libraries) n Intel IPP/MKL, Sca. LAPACK, PARDISO, PLAPACK n 并行程序语言 n 有150种以上 n 消息传递(Message passing) n MPI, Open. MP, PVM, 等等。 n
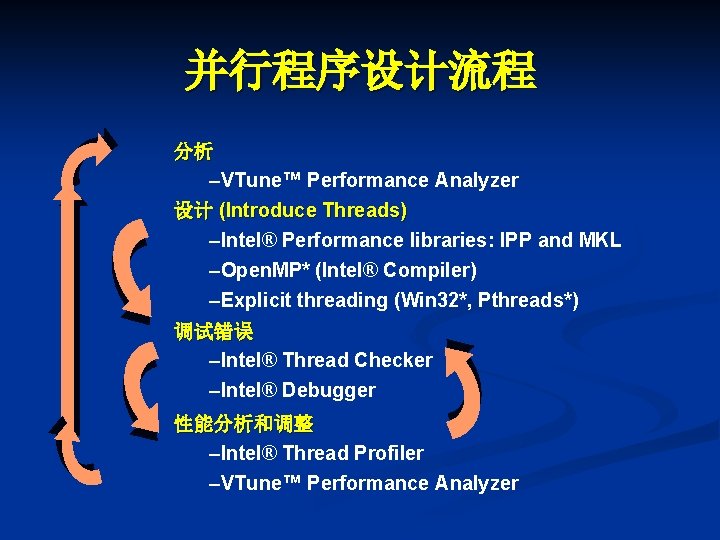
并行程序设计流程 分析 –VTune™ Performance Analyzer 设计 (Introduce Threads) –Intel® Performance libraries: IPP and MKL –Open. MP* (Intel® Compiler) –Explicit threading (Win 32*, Pthreads*) 调试错误 –Intel® Thread Checker –Intel® Debugger 性能分析和调整 –Intel® Thread Profiler –VTune™ Performance Analyzer
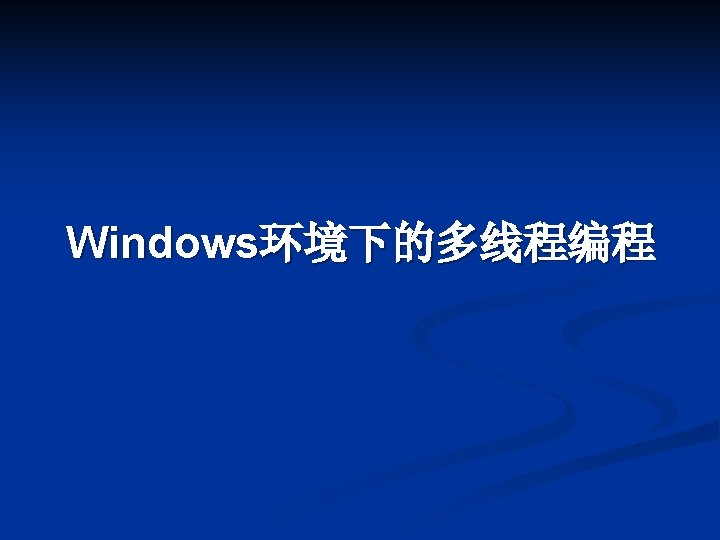
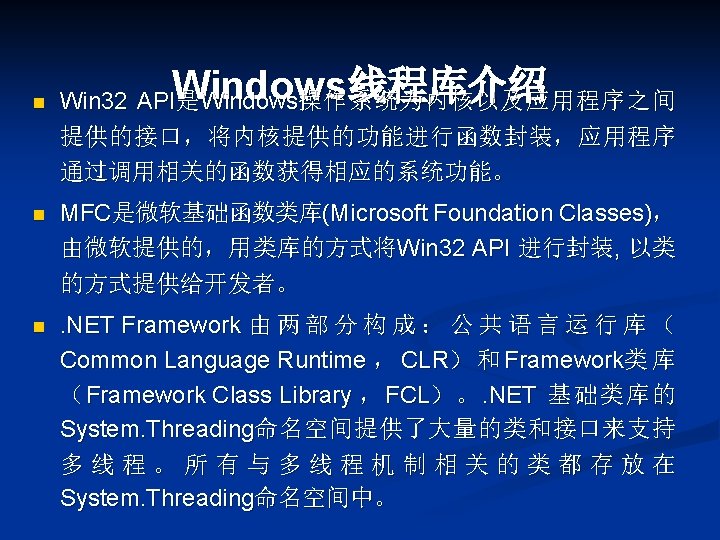
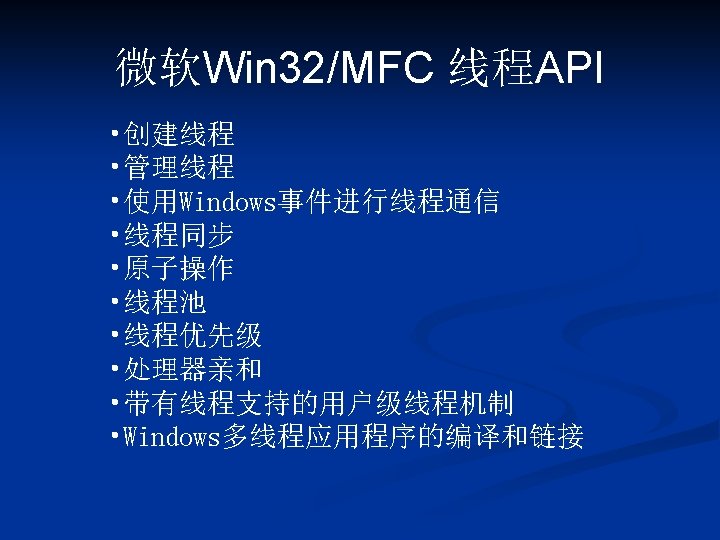
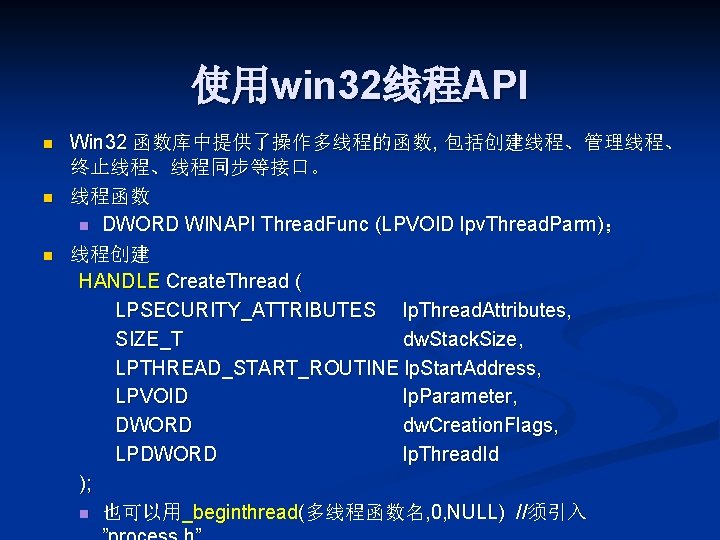
使用win 32线程API n n n Win 32 函数库中提供了操作多线程的函数, 包括创建线程、管理线程、 终止线程、线程同步等接口。 线程函数 n DWORD WINAPI Thread. Func (LPVOID lpv. Thread. Parm); 线程创建 HANDLE Create. Thread ( LPSECURITY_ATTRIBUTES lp. Thread. Attributes, SIZE_T dw. Stack. Size, LPTHREAD_START_ROUTINE lp. Start. Address, LPVOID lp. Parameter, DWORD dw. Creation. Flags, LPDWORD lp. Thread. Id ); n 也可以用_beginthread(多线程函数名, 0, NULL) //须引入
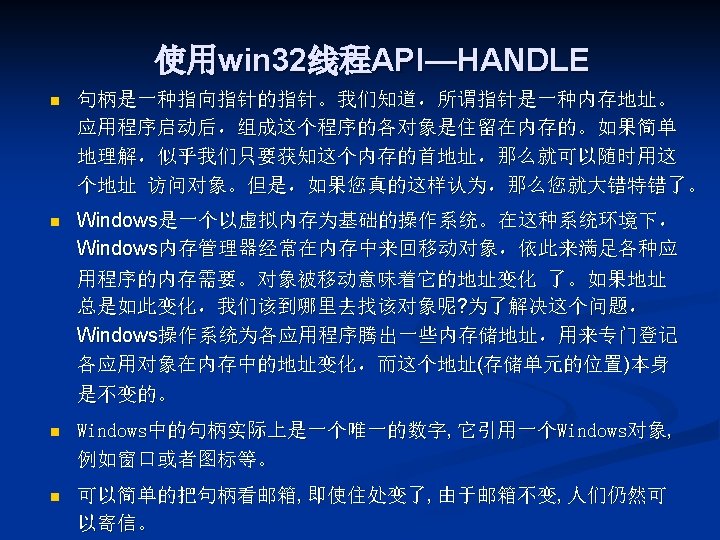
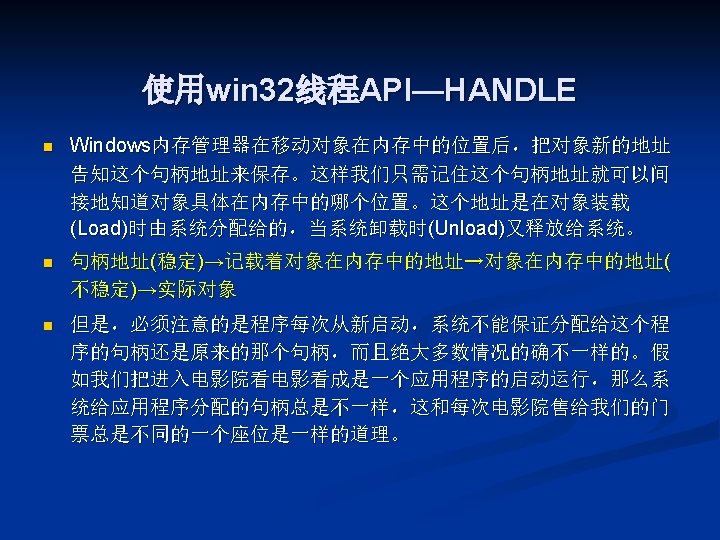
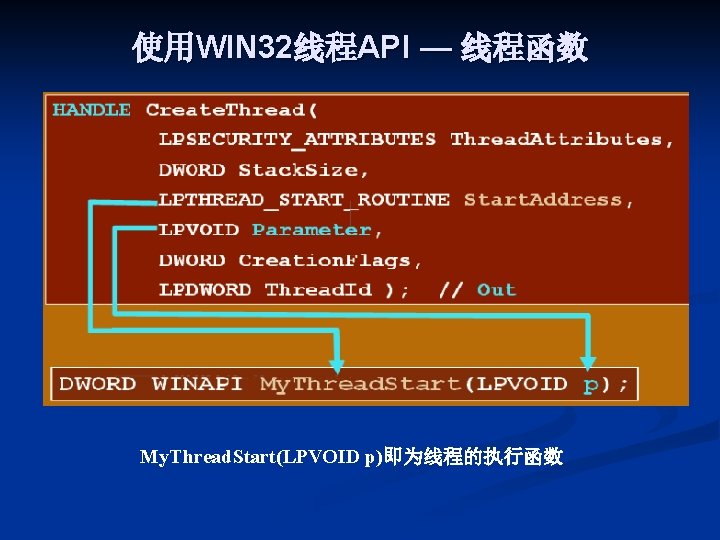
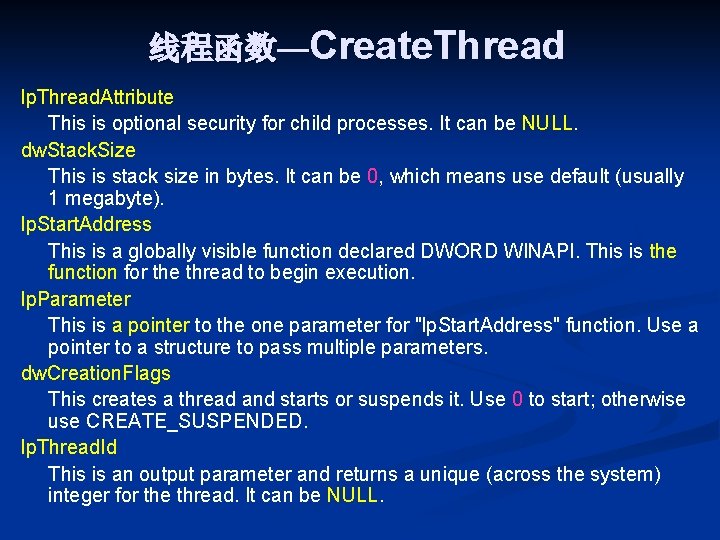
线程函数—Create. Thread lp. Thread. Attribute This is optional security for child processes. It can be NULL. dw. Stack. Size This is stack size in bytes. It can be 0, which means use default (usually 1 megabyte). lp. Start. Address This is a globally visible function declared DWORD WINAPI. This is the function for the thread to begin execution. lp. Parameter This is a pointer to the one parameter for "lp. Start. Address" function. Use a pointer to a structure to pass multiple parameters. dw. Creation. Flags This creates a thread and starts or suspends it. Use 0 to start; otherwise use CREATE_SUSPENDED. lp. Thread. Id This is an output parameter and returns a unique (across the system) integer for the thread. It can be NULL.
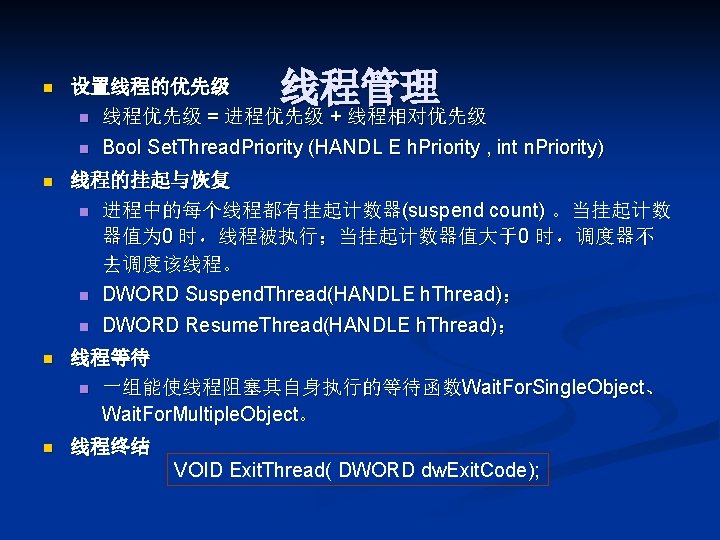
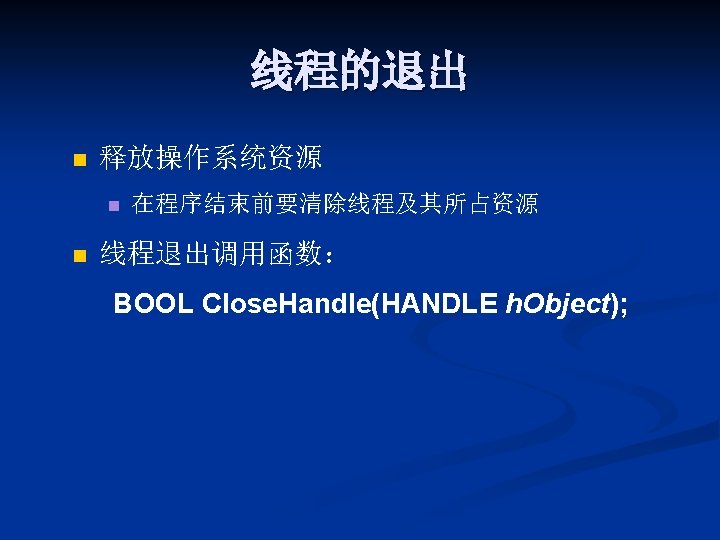
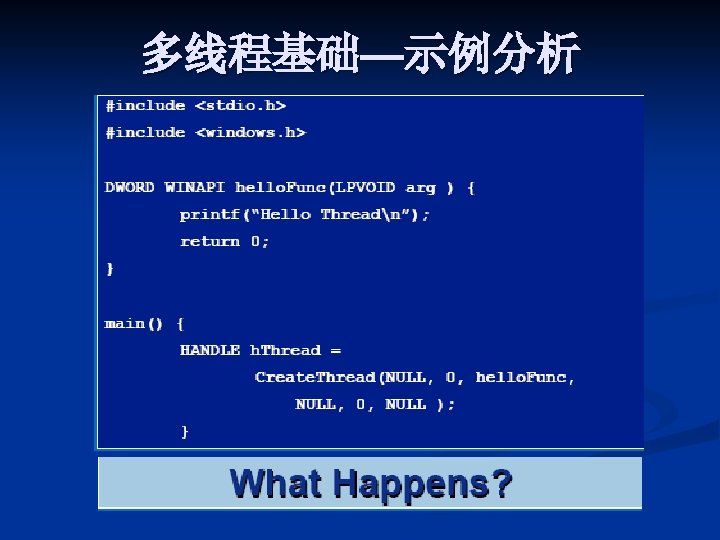
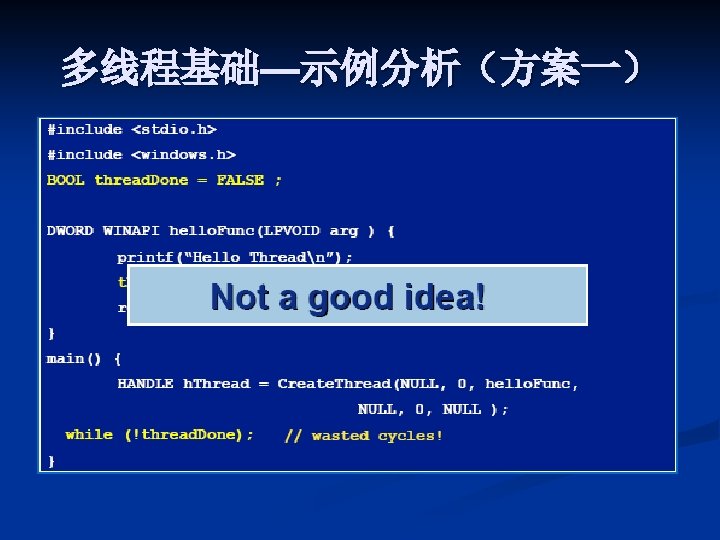
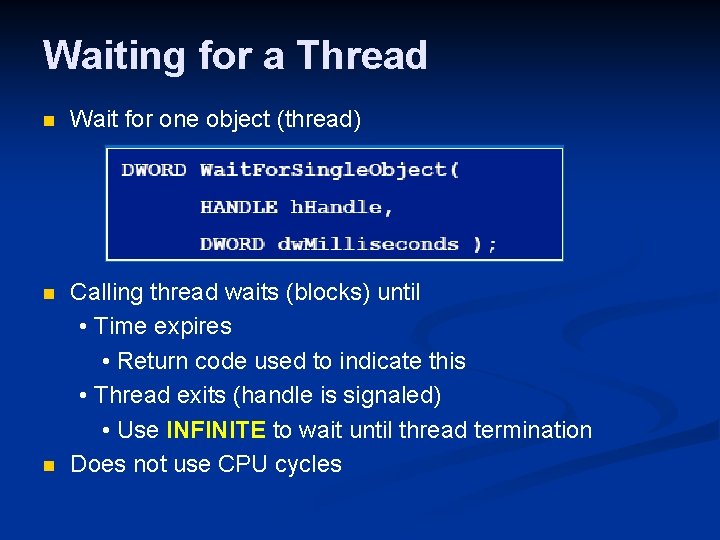
Waiting for a Thread n Wait for one object (thread) n Calling thread waits (blocks) until • Time expires • Return code used to indicate this • Thread exits (handle is signaled) • Use INFINITE to wait until thread termination Does not use CPU cycles n
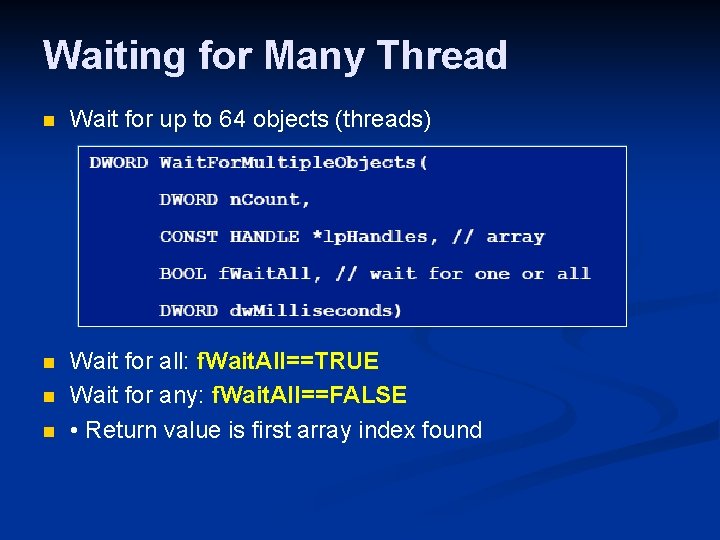
Waiting for Many Thread n Wait for up to 64 objects (threads) n Wait for all: f. Wait. All==TRUE Wait for any: f. Wait. All==FALSE • Return value is first array index found n n
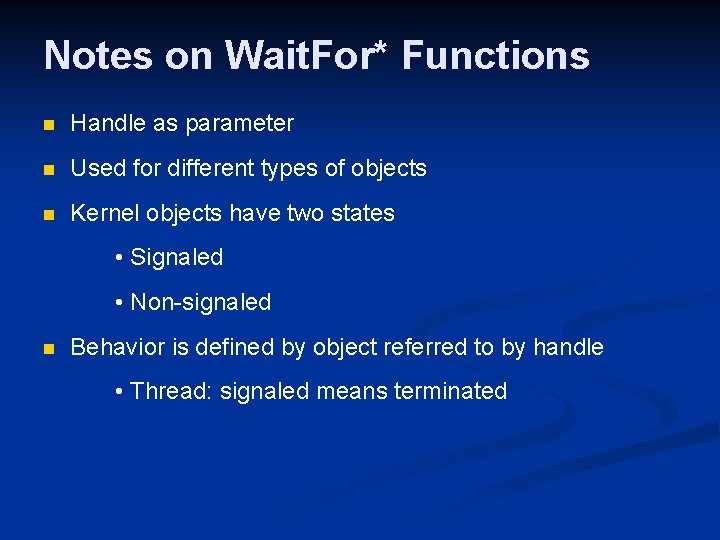
Notes on Wait. For* Functions n Handle as parameter n Used for different types of objects n Kernel objects have two states • Signaled • Non-signaled n Behavior is defined by object referred to by handle • Thread: signaled means terminated
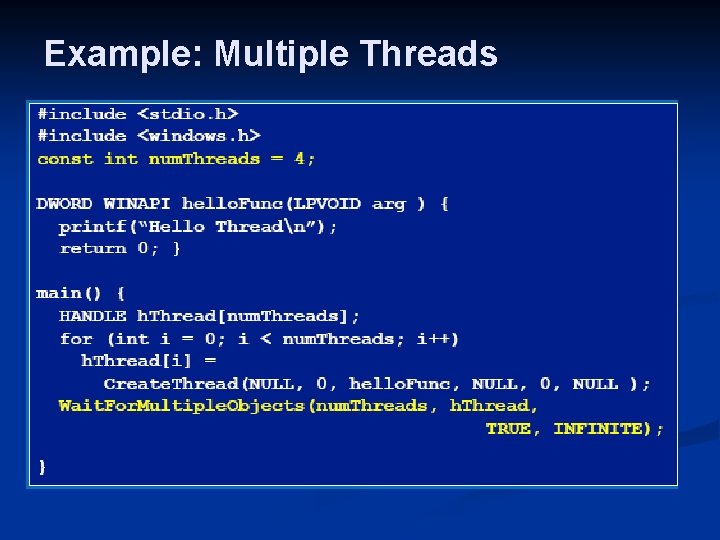
Example: Multiple Threads
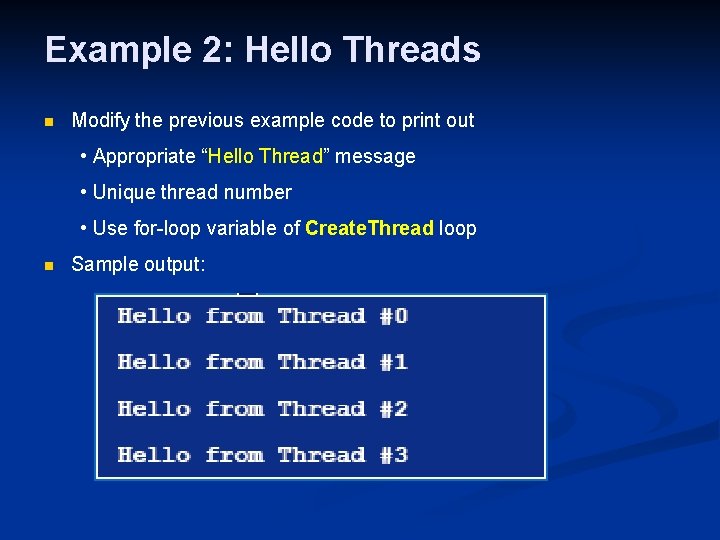
Example 2: Hello Threads n Modify the previous example code to print out • Appropriate “Hello Thread” message • Unique thread number • Use for-loop variable of Create. Thread loop n Sample output:
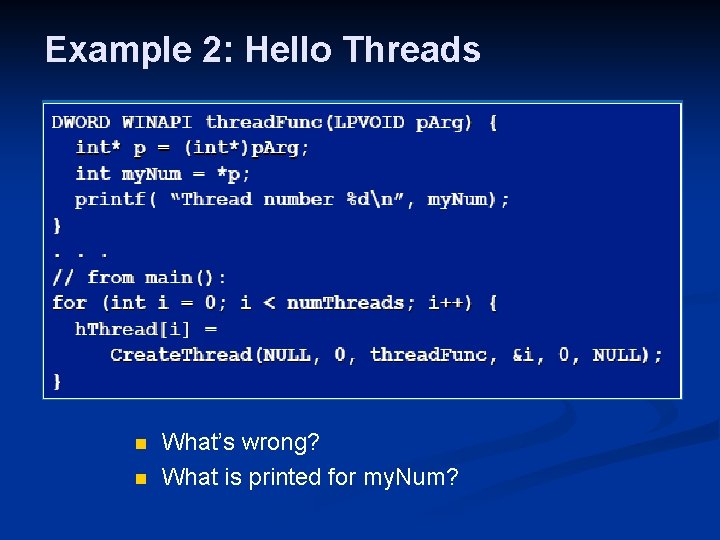
Example 2: Hello Threads n n What’s wrong? What is printed for my. Num?
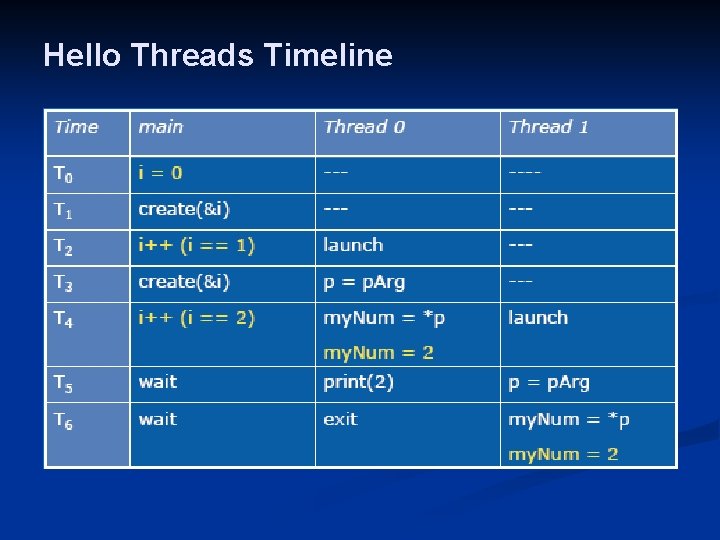
Hello Threads Timeline
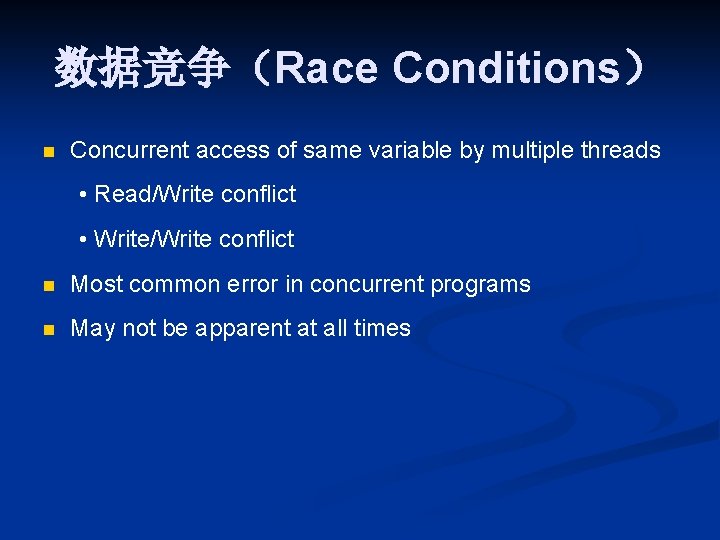
数据竞争(Race Conditions) n Concurrent access of same variable by multiple threads • Read/Write conflict • Write/Write conflict n Most common error in concurrent programs n May not be apparent at all times
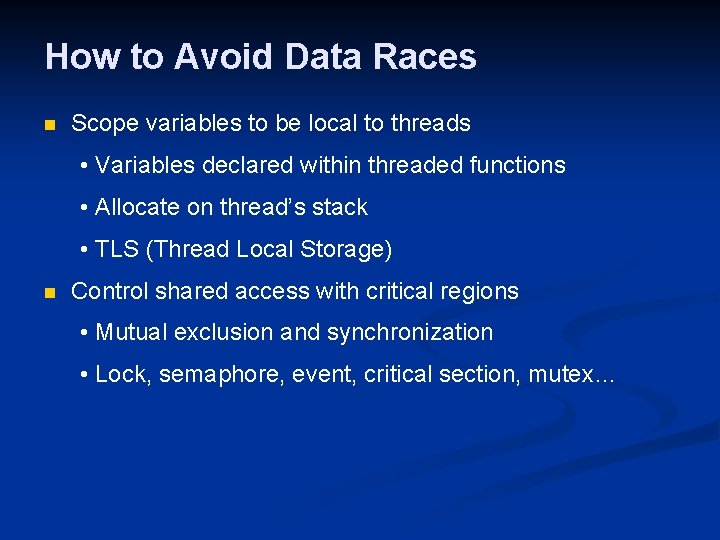
How to Avoid Data Races n Scope variables to be local to threads • Variables declared within threaded functions • Allocate on thread’s stack • TLS (Thread Local Storage) n Control shared access with critical regions • Mutual exclusion and synchronization • Lock, semaphore, event, critical section, mutex…
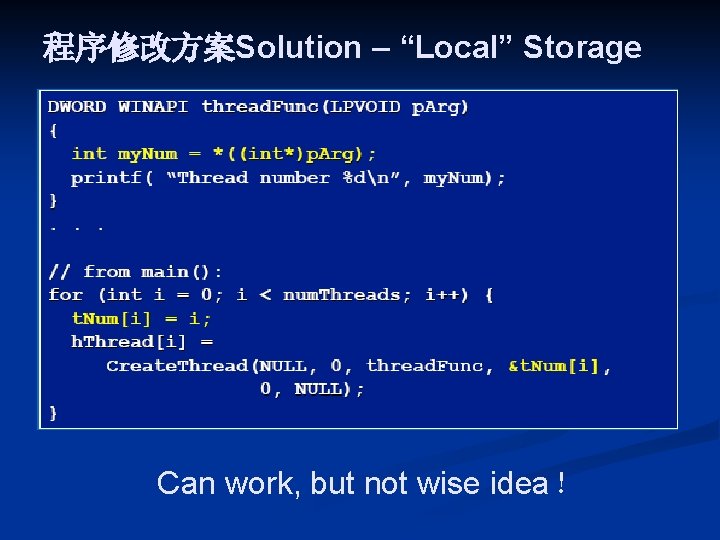
程序修改方案Solution – “Local” Storage Can work, but not wise idea!
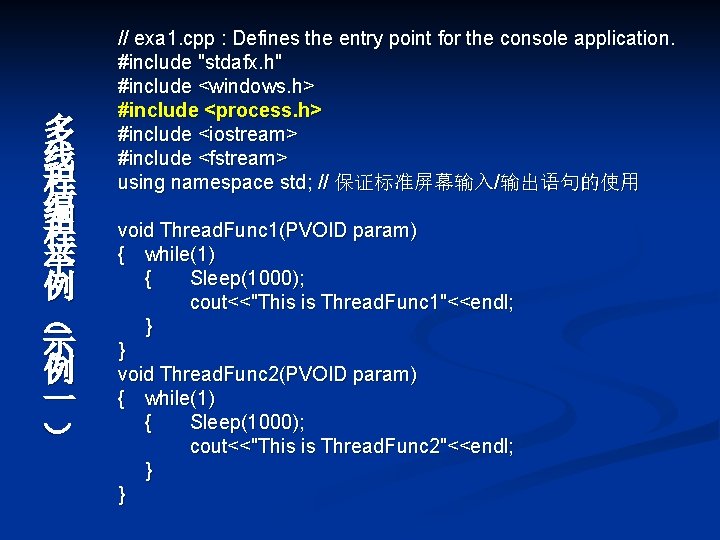
多 线 程 编 程 举 例 ( 示 例 一 // exa 1. cpp : Defines the entry point for the console application. #include "stdafx. h" #include <windows. h> #include <process. h> #include <iostream> #include <fstream> using namespace std; // 保证标准屏幕输入/输出语句的使用 ) void Thread. Func 1(PVOID param) { while(1) { Sleep(1000); cout<<"This is Thread. Func 1"<<endl; } } void Thread. Func 2(PVOID param) { while(1) { Sleep(1000); cout<<"This is Thread. Func 2"<<endl; } }
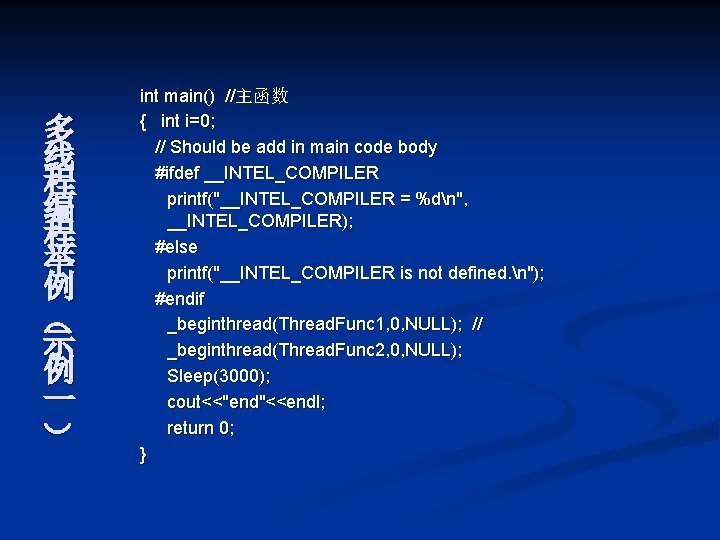
多 线 程 编 程 举 例 ( 示 例 一 ) int main() //主函数 { int i=0; // Should be add in main code body #ifdef __INTEL_COMPILER printf("__INTEL_COMPILER = %dn", __INTEL_COMPILER); #else printf("__INTEL_COMPILER is not defined. n"); #endif _beginthread(Thread. Func 1, 0, NULL); // _beginthread(Thread. Func 2, 0, NULL); Sleep(3000); cout<<"end"<<endl; return 0; }
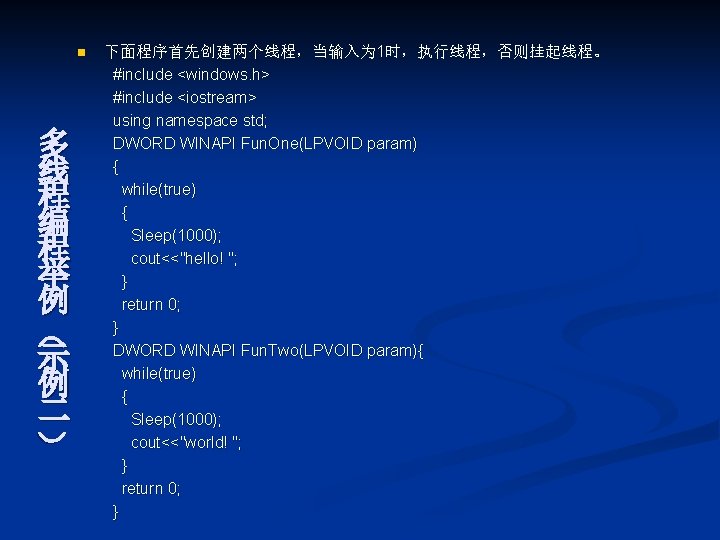
n 多 线 程 编 程 举 例 ( 示 例 二 ) 下面程序首先创建两个线程,当输入为 1时,执行线程,否则挂起线程。 #include <windows. h> #include <iostream> using namespace std; DWORD WINAPI Fun. One(LPVOID param) { while(true) { Sleep(1000); cout<<"hello! "; } return 0; } DWORD WINAPI Fun. Two(LPVOID param){ while(true) { Sleep(1000); cout<<"world! "; } return 0; }
![多线程编程举例示例二 int mainint argc char argv int input0 HANDLE hand 1 Create Thread 多线程编程举例(示例二) int main(int argc, char* argv[]) { int input=0; HANDLE hand 1= Create. Thread](https://slidetodoc.com/presentation_image/deebd894239ba8723b431a008c5d2b8e/image-66.jpg)
多线程编程举例(示例二) int main(int argc, char* argv[]) { int input=0; HANDLE hand 1= Create. Thread (NULL, 0, Fun. One, (void*)&input, CREATE_SUSPENDED, NULL); HANDLE hand 2= Create. Thread (NULL, 0, Fun. Two, (void*)&input, CREATE_SUSPENDED, NULL); while(true){ cin>>input; if(input==1) { Resume. Thread(hand 1); Resume. Thread(hand 2); } if(input==0) { Suspend. Thread(hand 1); Suspend. Thread(hand 2); } if(input==-1) { Exit. Thread(hand 1); Exit. Thread(hand 2); } }; Terminate. Thread(hand 1, 1); Terminate. Thread(hand 2, 1); return 0; }
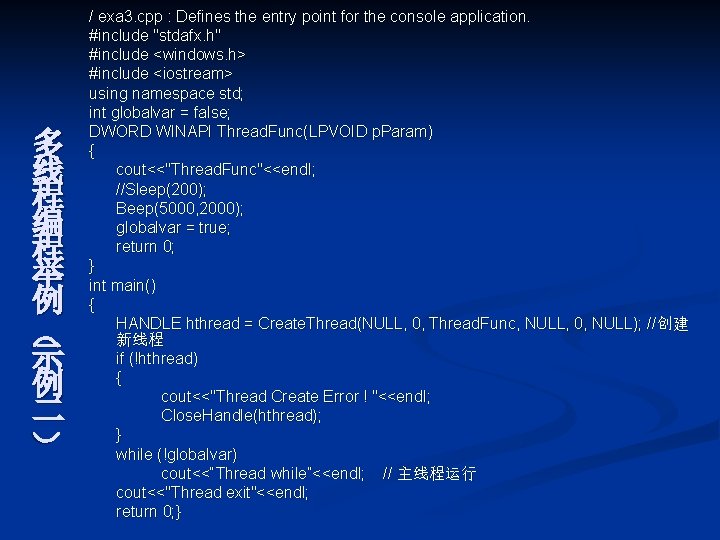
多 线 程 编 程 举 例 ( 示 例 二 ) / exa 3. cpp : Defines the entry point for the console application. #include "stdafx. h" #include <windows. h> #include <iostream> using namespace std; int globalvar = false; DWORD WINAPI Thread. Func(LPVOID p. Param) { cout<<"Thread. Func"<<endl; //Sleep(200); Beep(5000, 2000); globalvar = true; return 0; } int main() { HANDLE hthread = Create. Thread(NULL, 0, Thread. Func, NULL, 0, NULL); //创建 新线程 if (!hthread) { cout<<"Thread Create Error ! "<<endl; Close. Handle(hthread); } while (!globalvar) cout<<“Thread while”<<endl; // 主线程运行 cout<<"Thread exit"<<endl; return 0; }
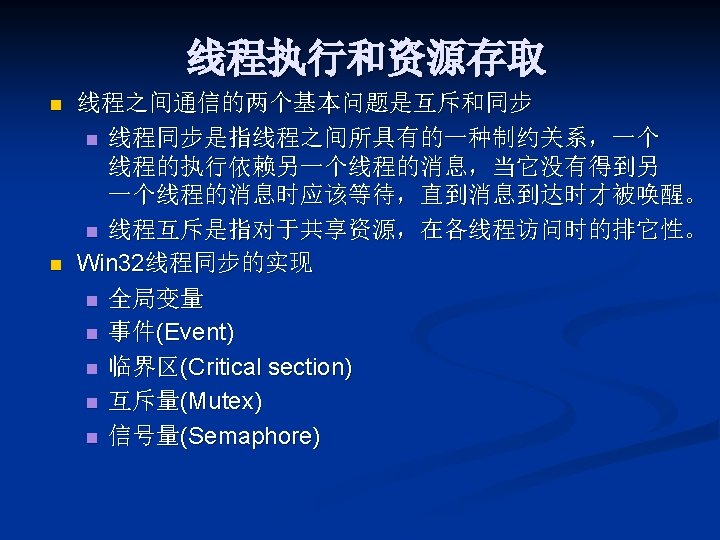
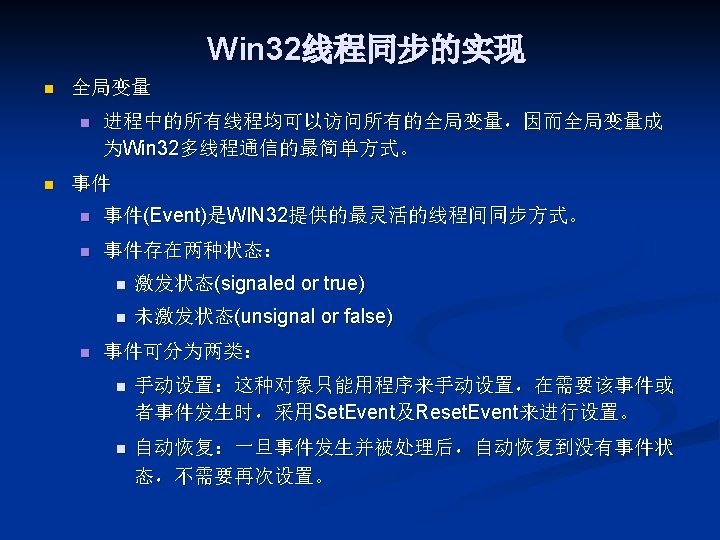
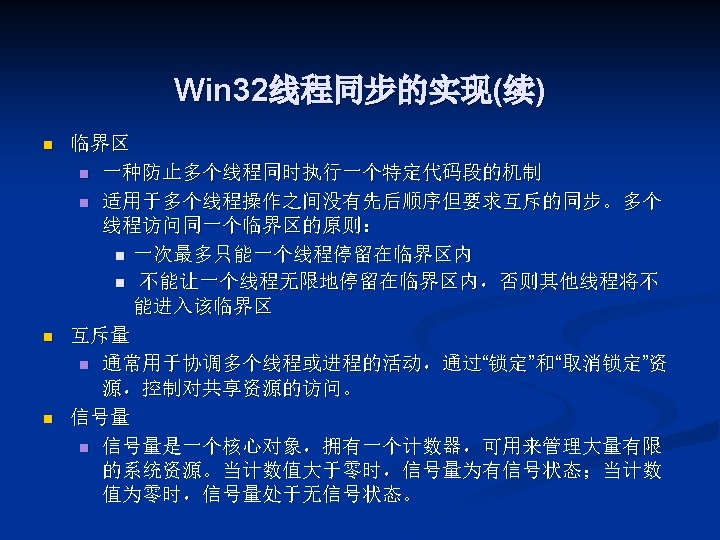
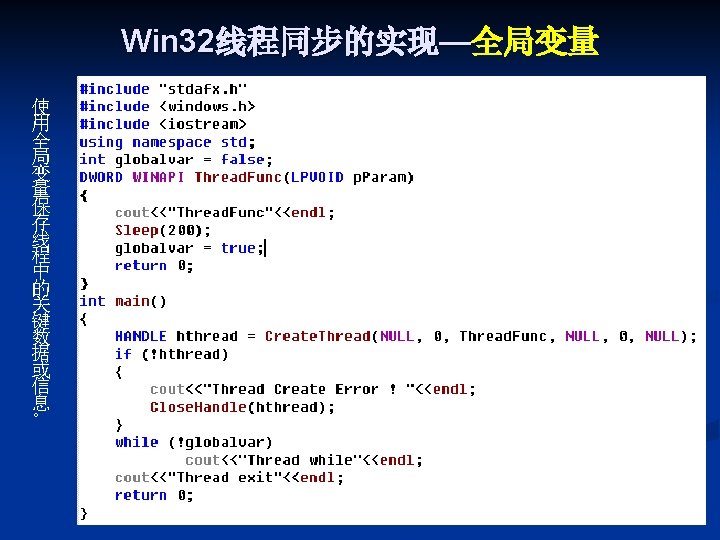
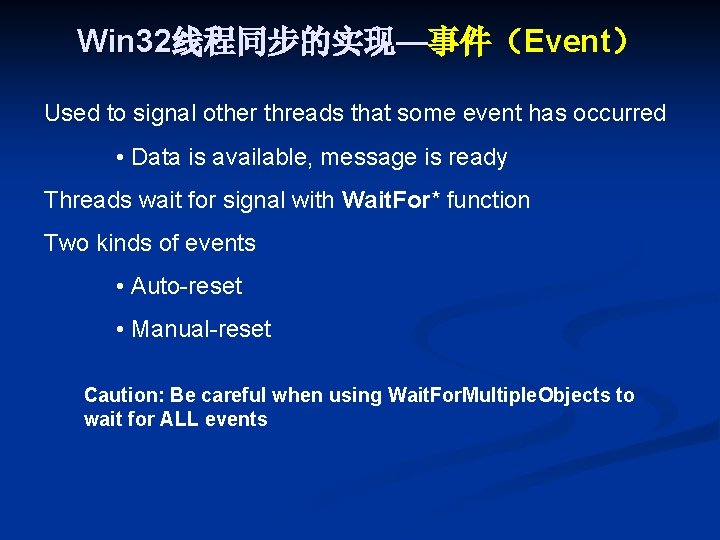
Win 32线程同步的实现—事件(Event) Used to signal other threads that some event has occurred • Data is available, message is ready Threads wait for signal with Wait. For* function Two kinds of events • Auto-reset • Manual-reset Caution: Be careful when using Wait. For. Multiple. Objects to wait for ALL events
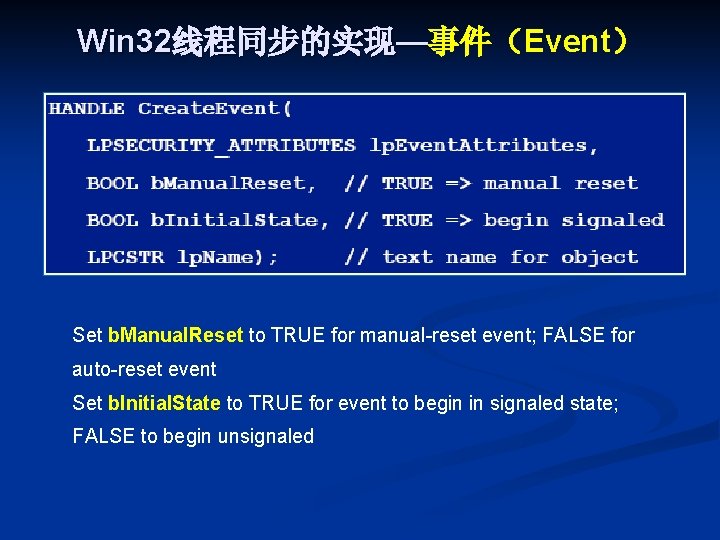
Win 32线程同步的实现—事件(Event) Set b. Manual. Reset to TRUE for manual-reset event; FALSE for auto-reset event Set b. Initial. State to TRUE for event to begin in signaled state; FALSE to begin unsignaled
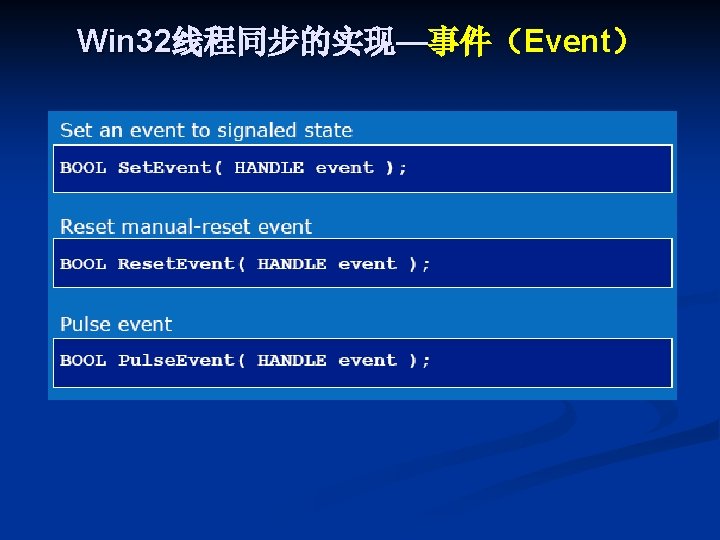
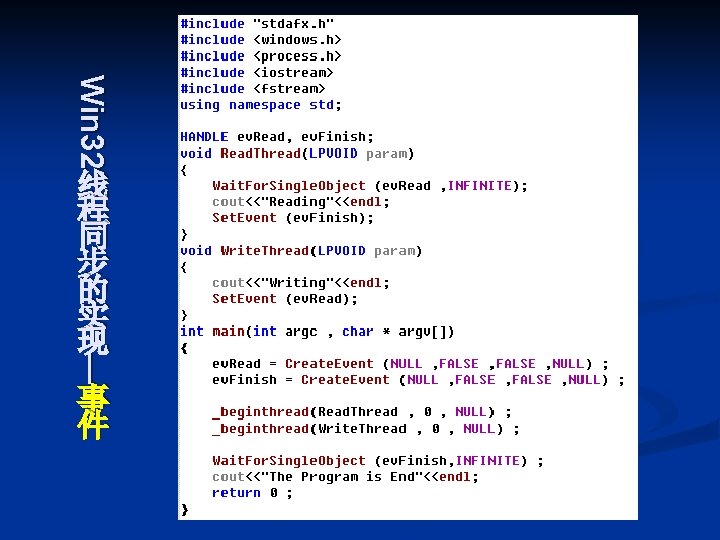
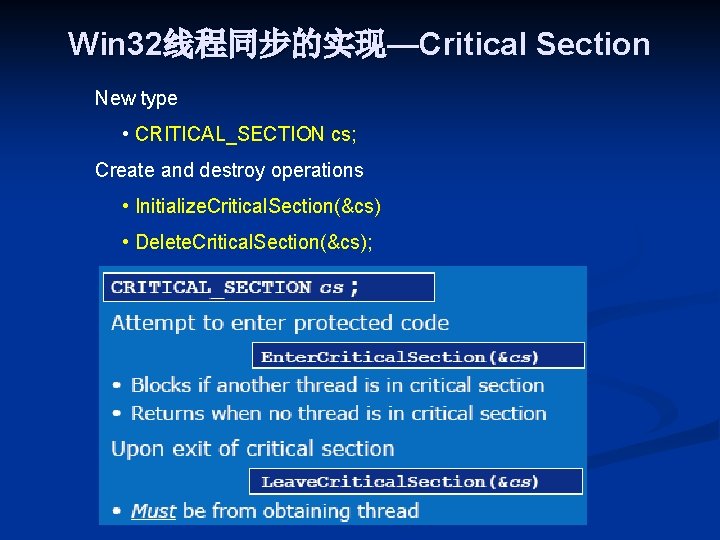
Win 32线程同步的实现—Critical Section New type • CRITICAL_SECTION cs; Create and destroy operations • Initialize. Critical. Section(&cs) • Delete. Critical. Section(&cs);
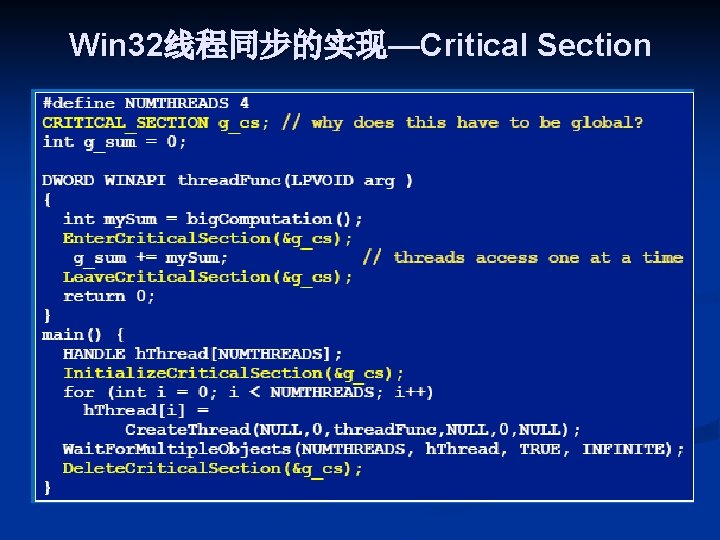
Win 32线程同步的实现—Critical Section
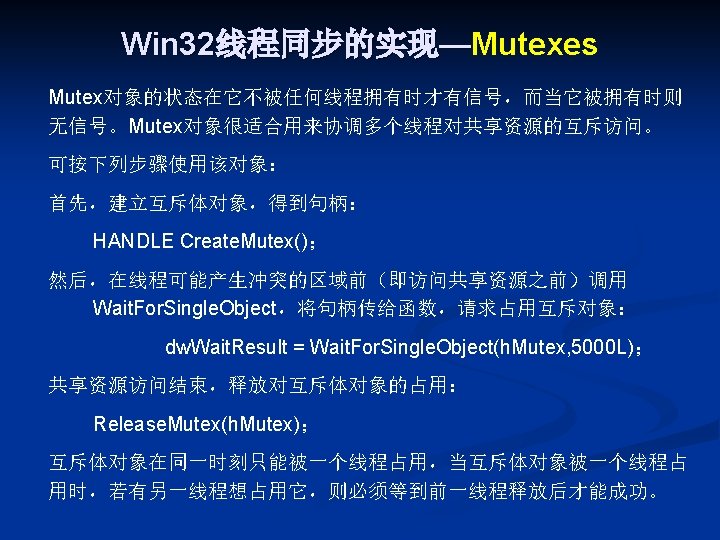
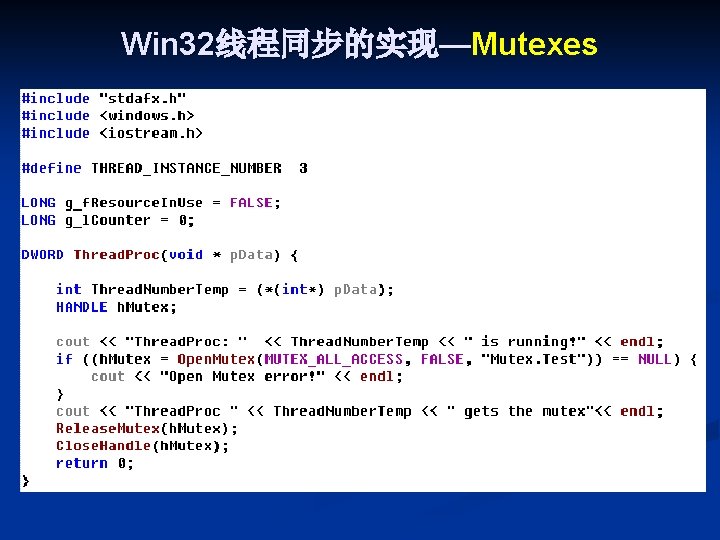
Win 32线程同步的实现—Mutexes
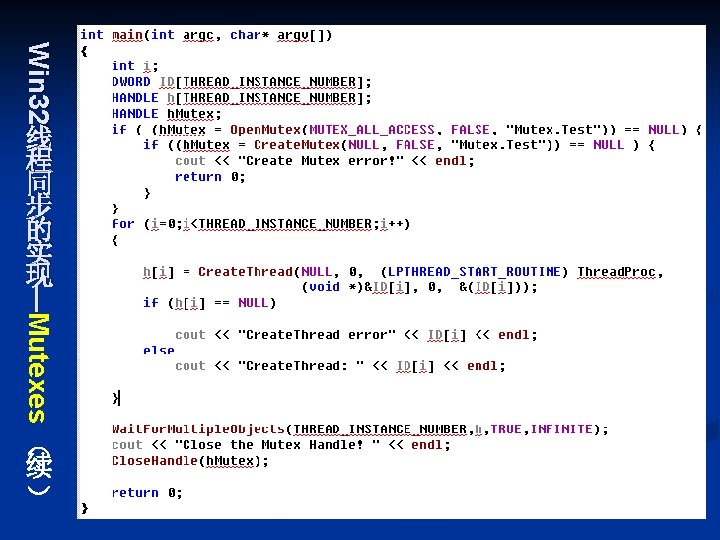
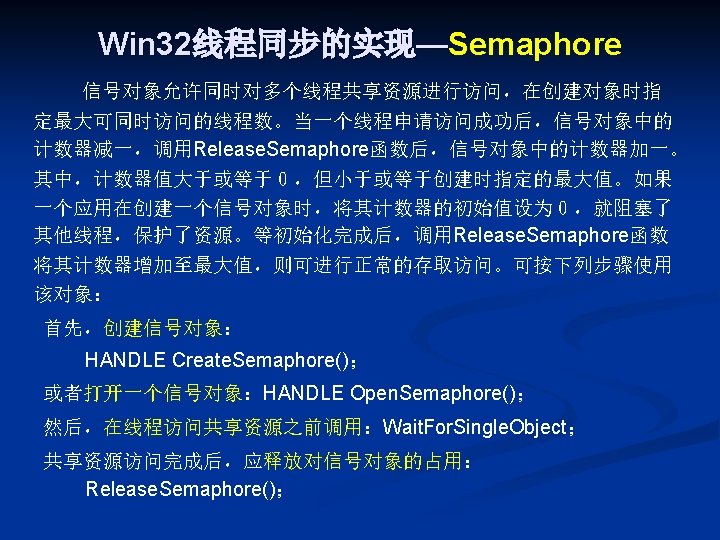
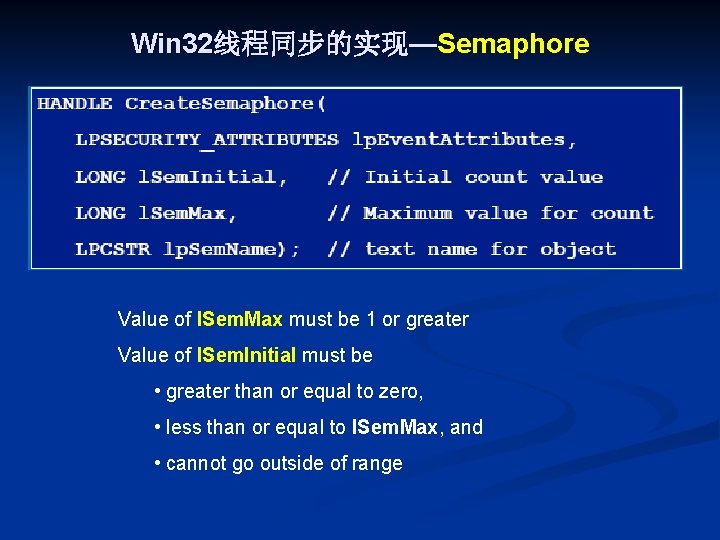
Win 32线程同步的实现—Semaphore Value of l. Sem. Max must be 1 or greater Value of l. Sem. Initial must be • greater than or equal to zero, • less than or equal to l. Sem. Max, and • cannot go outside of range
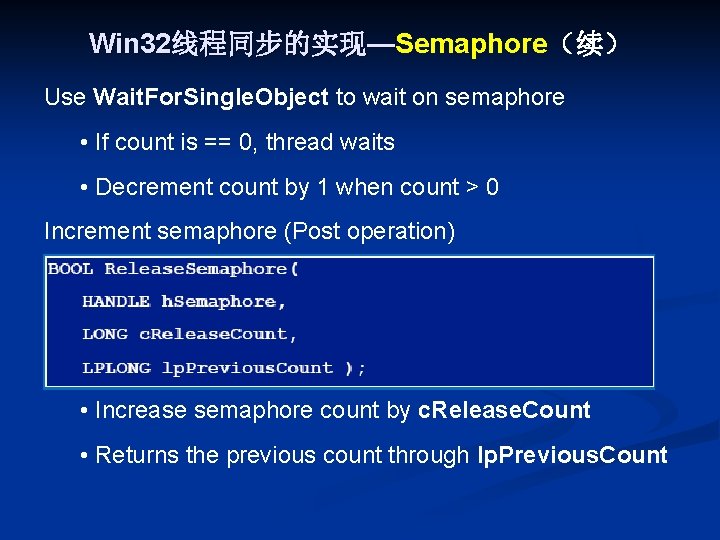
Win 32线程同步的实现—Semaphore(续) Use Wait. For. Single. Object to wait on semaphore • If count is == 0, thread waits • Decrement count by 1 when count > 0 Increment semaphore (Post operation) • Increase semaphore count by c. Release. Count • Returns the previous count through lp. Previous. Count
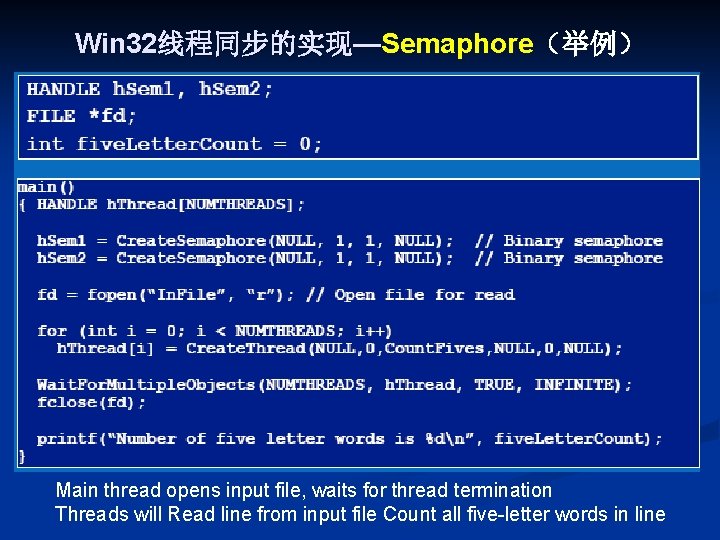
Win 32线程同步的实现—Semaphore(举例) Main thread opens input file, waits for thread termination Threads will Read line from input file Count all five-letter words in line
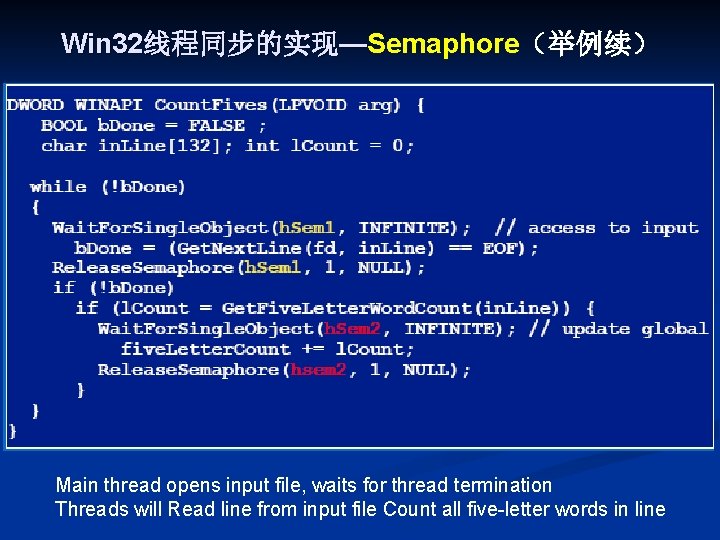
Win 32线程同步的实现—Semaphore(举例续) Main thread opens input file, waits for thread termination Threads will Read line from input file Count all five-letter words in line
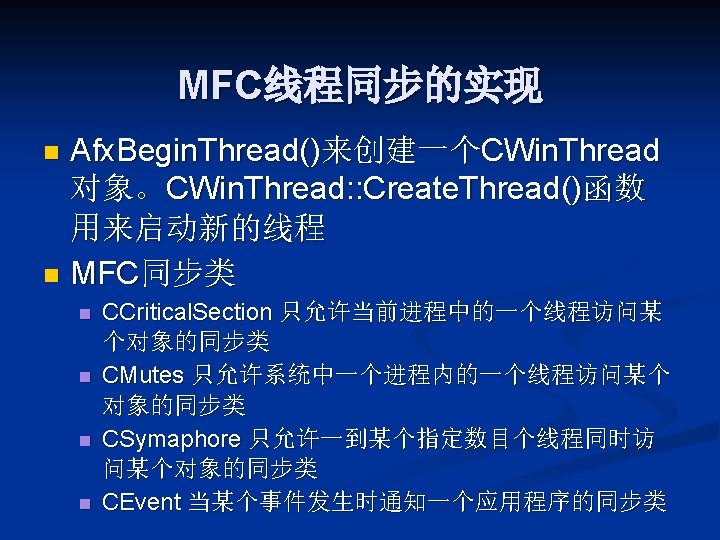
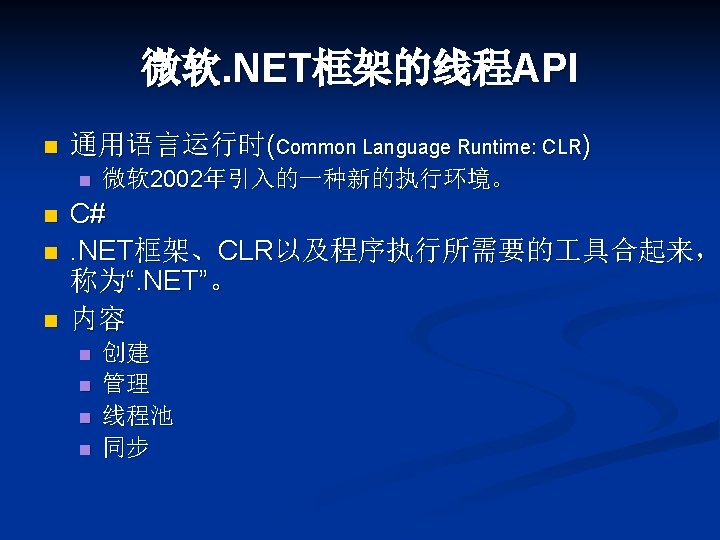
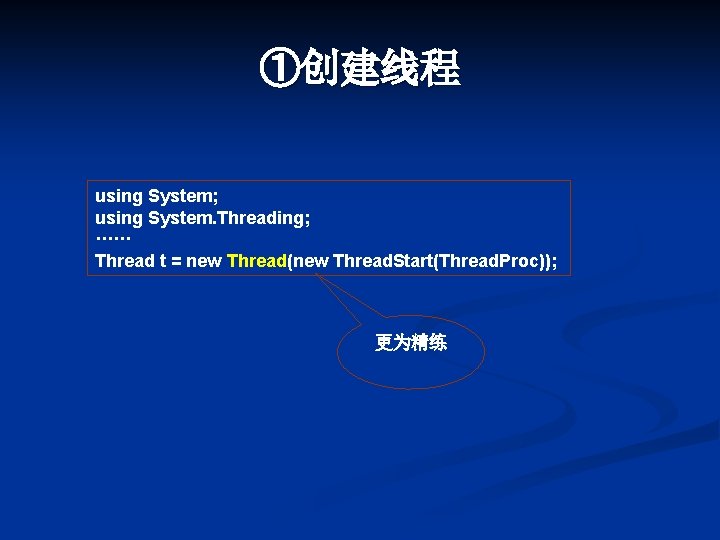
①创建线程 using System; using System. Threading; ······ Thread t = new Thread(new Thread. Start(Thread. Proc)); 更为精练
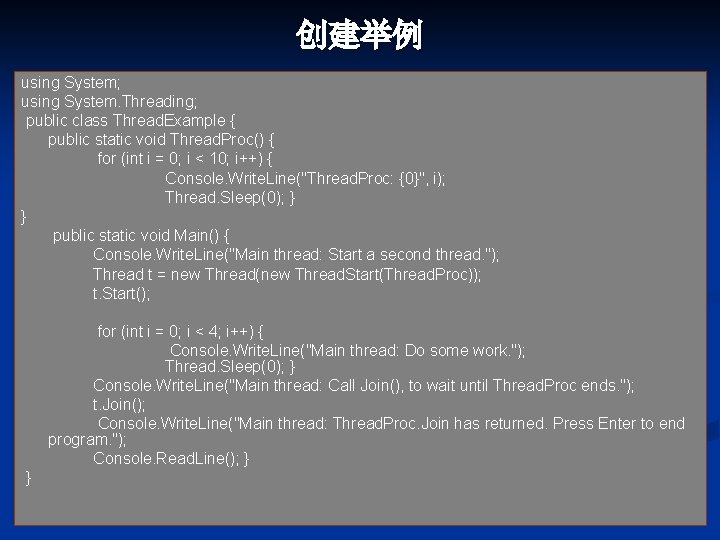
创建举例 using System; using System. Threading; public class Thread. Example { public static void Thread. Proc() { for (int i = 0; i < 10; i++) { Console. Write. Line("Thread. Proc: {0}", i); Thread. Sleep(0); } } public static void Main() { Console. Write. Line("Main thread: Start a second thread. "); Thread t = new Thread(new Thread. Start(Thread. Proc)); t. Start(); for (int i = 0; i < 4; i++) { Console. Write. Line("Main thread: Do some work. "); Thread. Sleep(0); } Console. Write. Line("Main thread: Call Join(), to wait until Thread. Proc ends. "); t. Join(); Console. Write. Line("Main thread: Thread. Proc. Join has returned. Press Enter to end program. "); Console. Read. Line(); } }
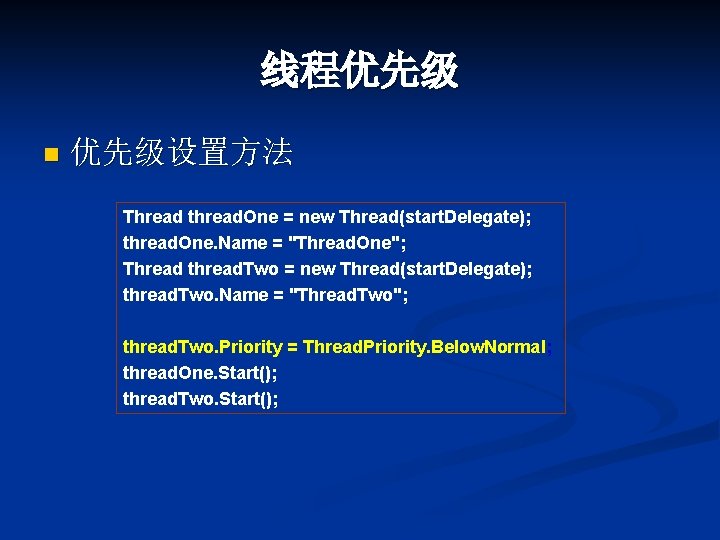
线程优先级 n 优先级设置方法 Thread thread. One = new Thread(start. Delegate); thread. One. Name = "Thread. One"; Thread thread. Two = new Thread(start. Delegate); thread. Two. Name = "Thread. Two"; thread. Two. Priority = Thread. Priority. Below. Normal; thread. One. Start(); thread. Two. Start();
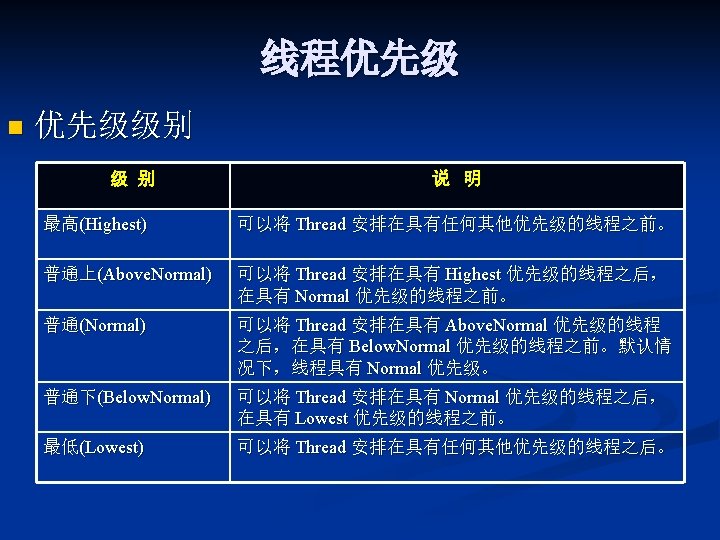
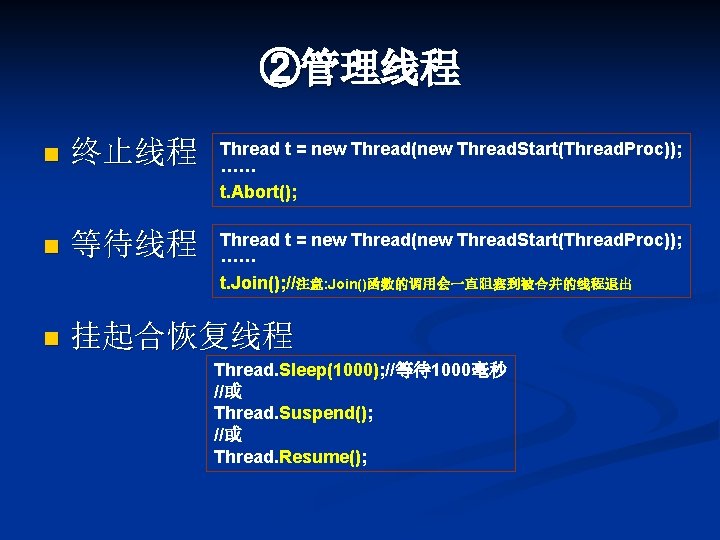
②管理线程 n 终止线程 Thread t = new Thread(new Thread. Start(Thread. Proc)); ······ t. Abort(); n 等待线程 Thread t = new Thread(new Thread. Start(Thread. Proc)); ······ t. Join(); //注意: Join()函数的调用会一直阻塞到被合并的线程退出 n 挂起合恢复线程 Thread. Sleep(1000); //等待1000毫秒 //或 Thread. Suspend(); //或 Thread. Resume();
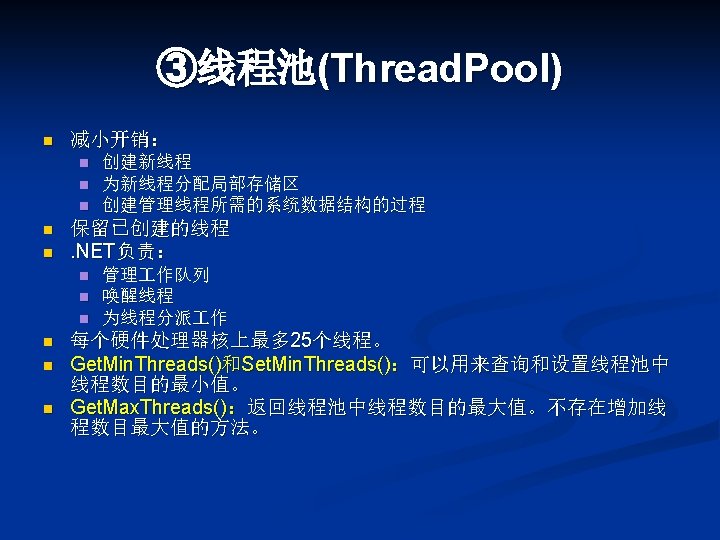
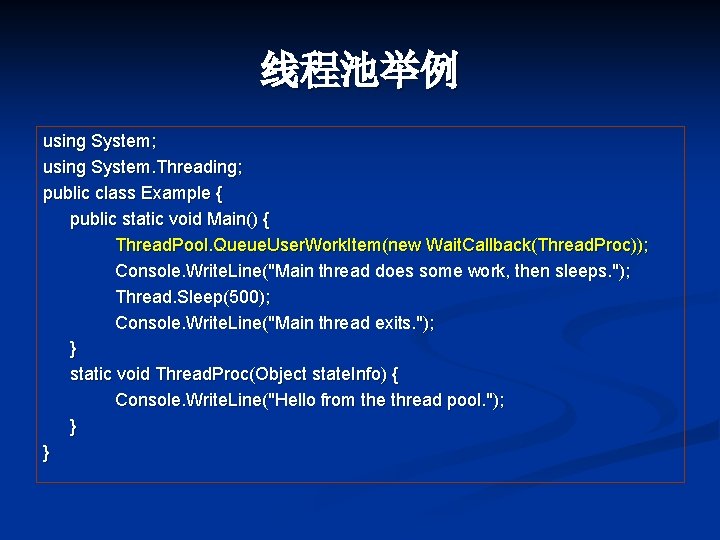
线程池举例 using System; using System. Threading; public class Example { public static void Main() { Thread. Pool. Queue. User. Work. Item(new Wait. Callback(Thread. Proc)); Console. Write. Line("Main thread does some work, then sleeps. "); Thread. Sleep(500); Console. Write. Line("Main thread exits. "); } static void Thread. Proc(Object state. Info) { Console. Write. Line("Hello from the thread pool. "); } }
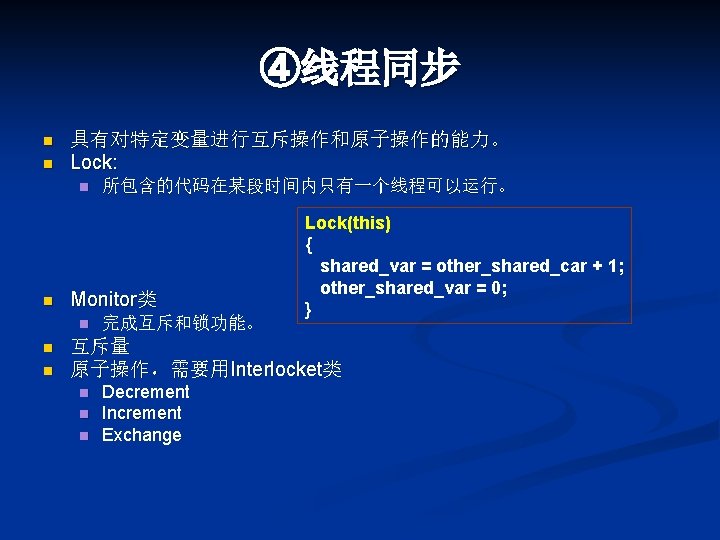
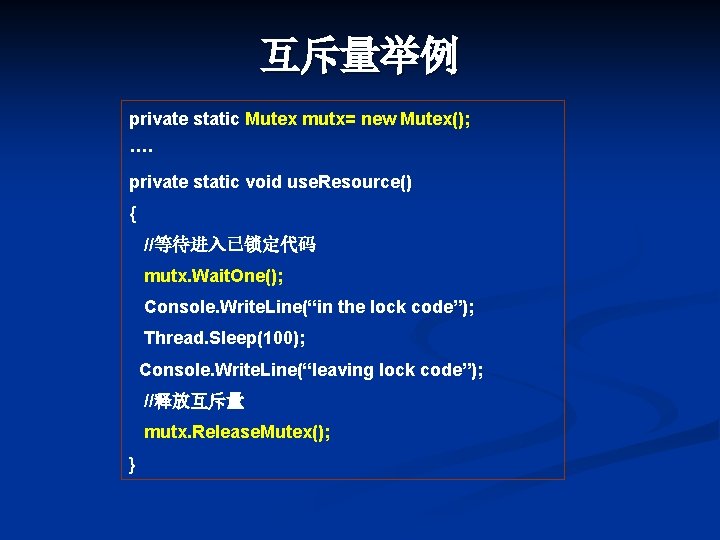
互斥量举例 private static Mutex mutx= new Mutex(); ···· private static void use. Resource() { //等待进入已锁定代码 mutx. Wait. One(); Console. Write. Line(“in the lock code”); Thread. Sleep(100); Console. Write. Line(“leaving lock code”); //释放互斥量 mutx. Release. Mutex(); }
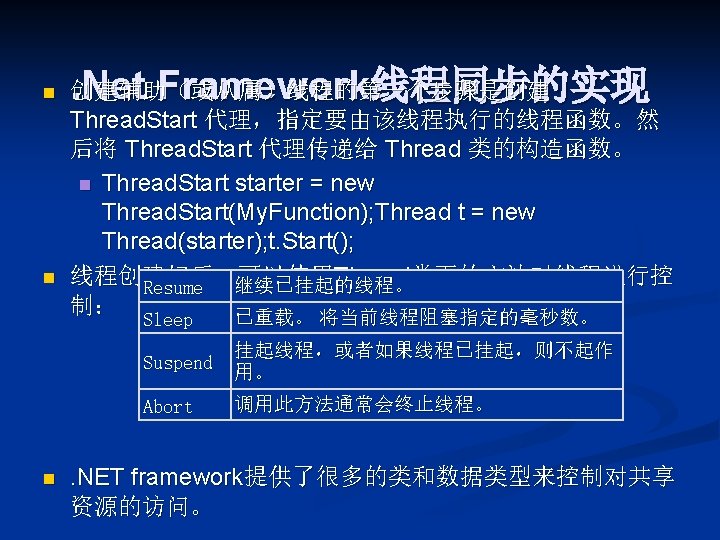
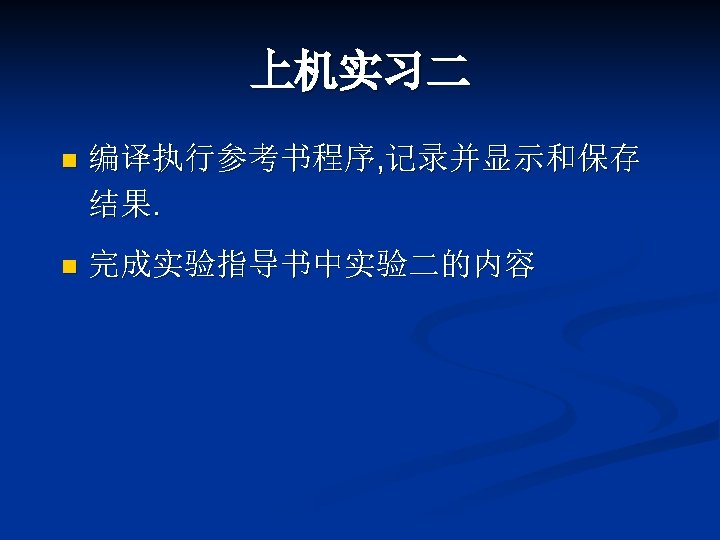
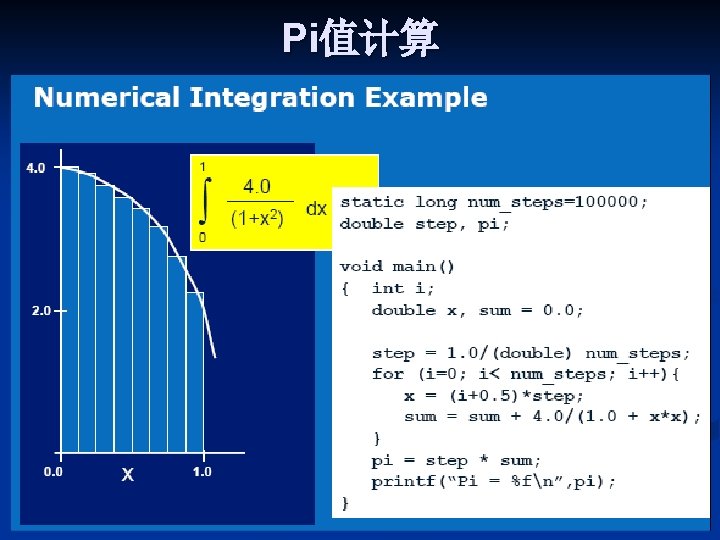
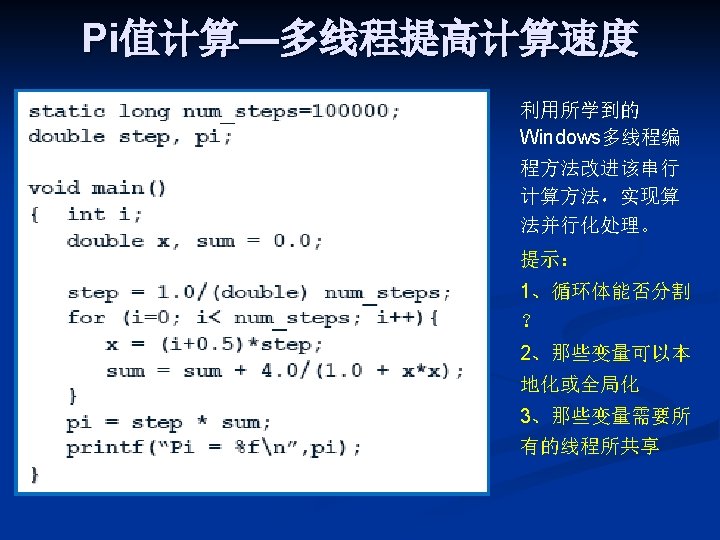
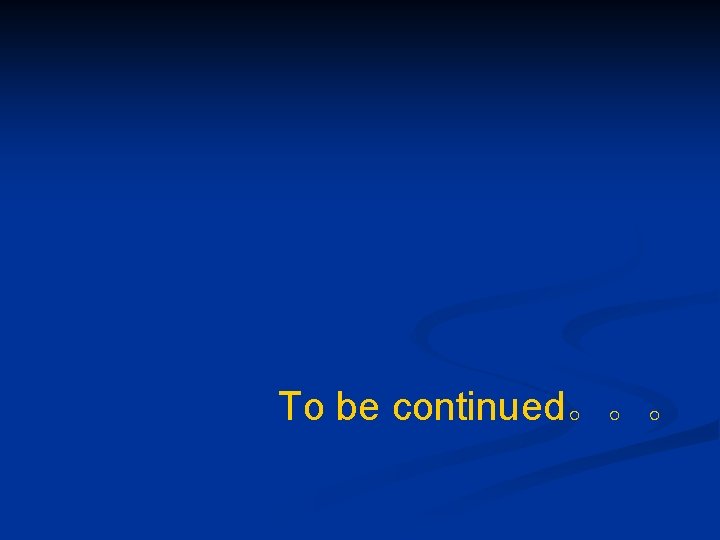
To be continued。。。