MT 311 Oct 2006 Java Application Development Tutorial
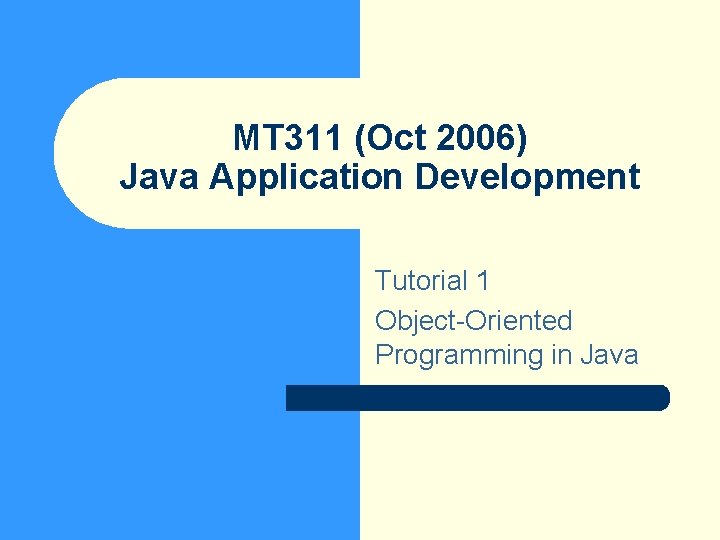
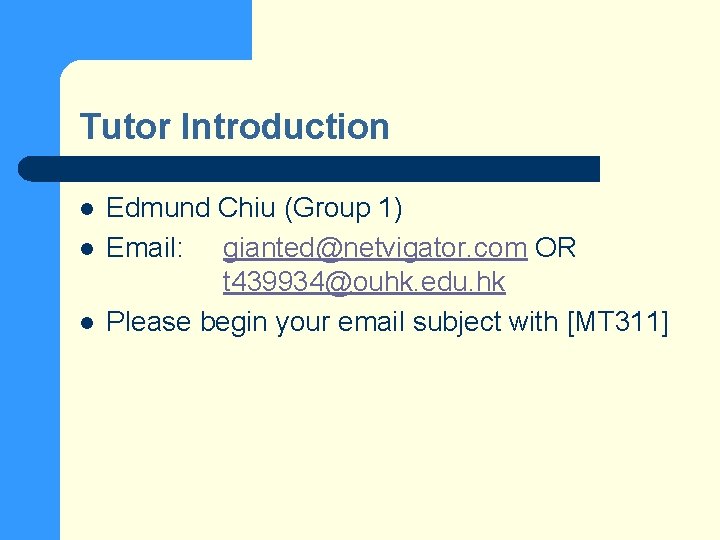
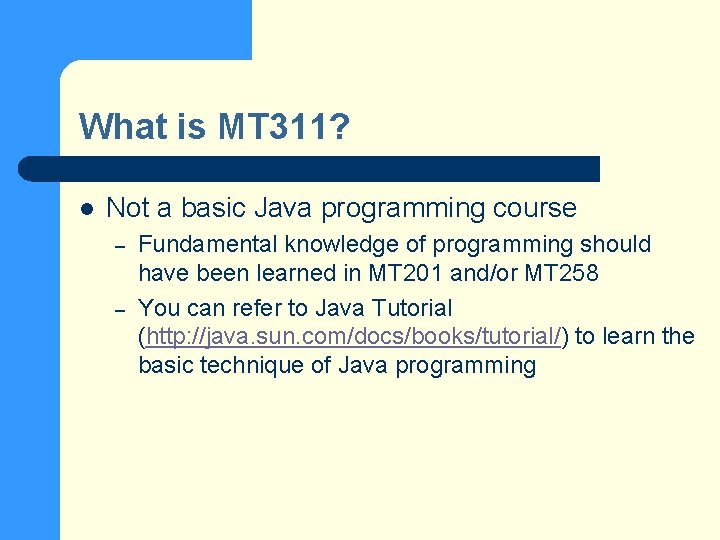
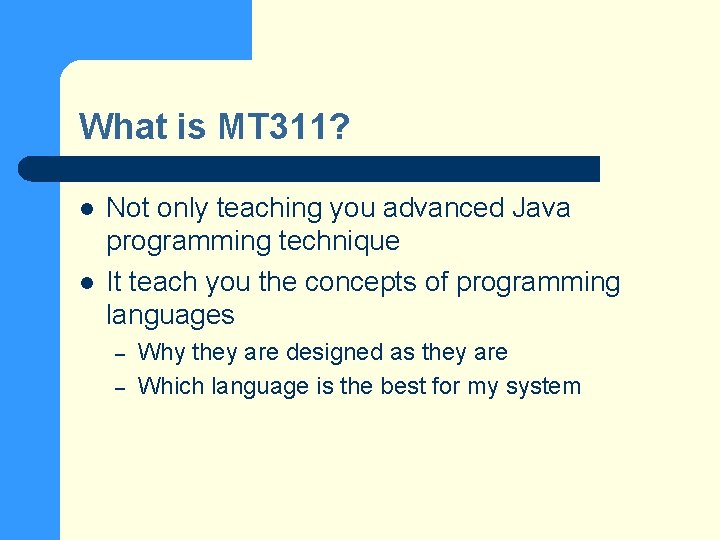
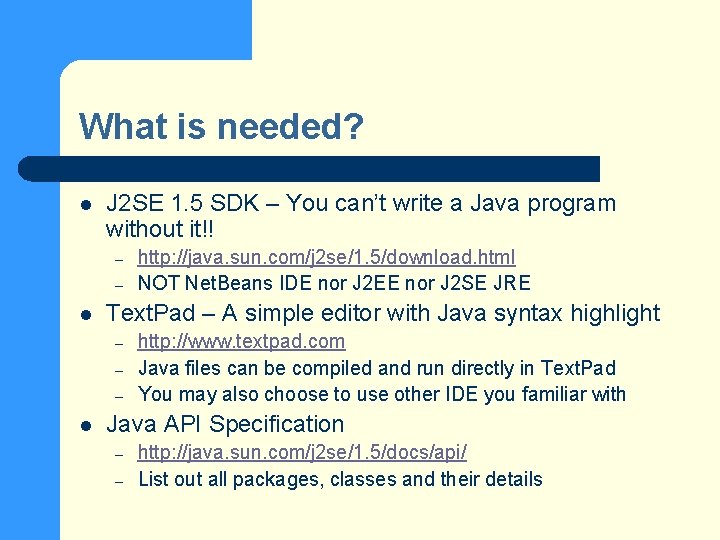
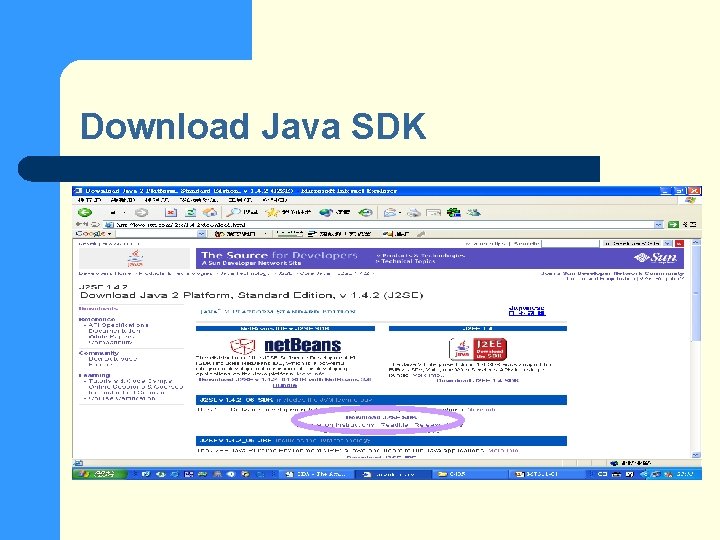
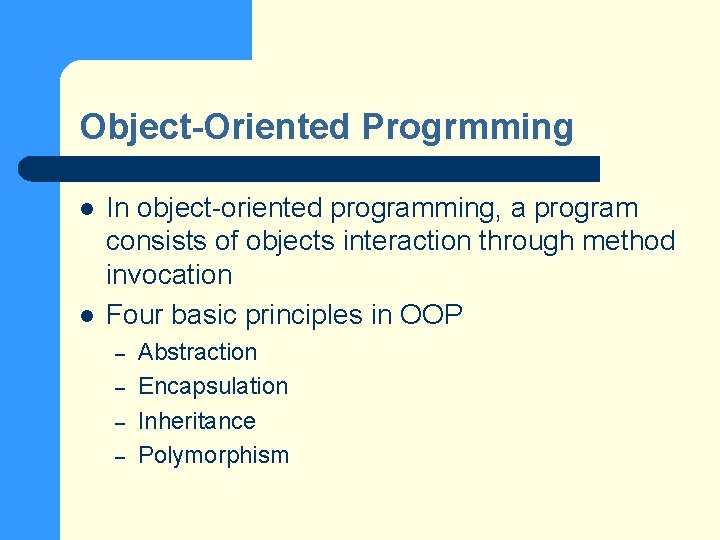
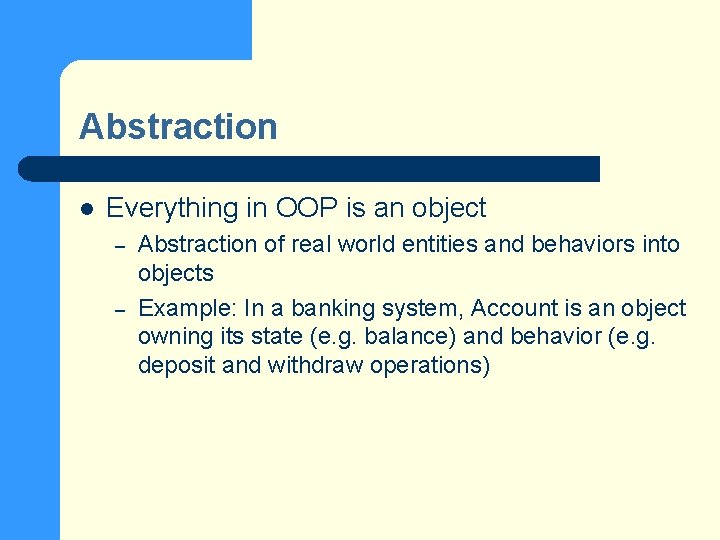
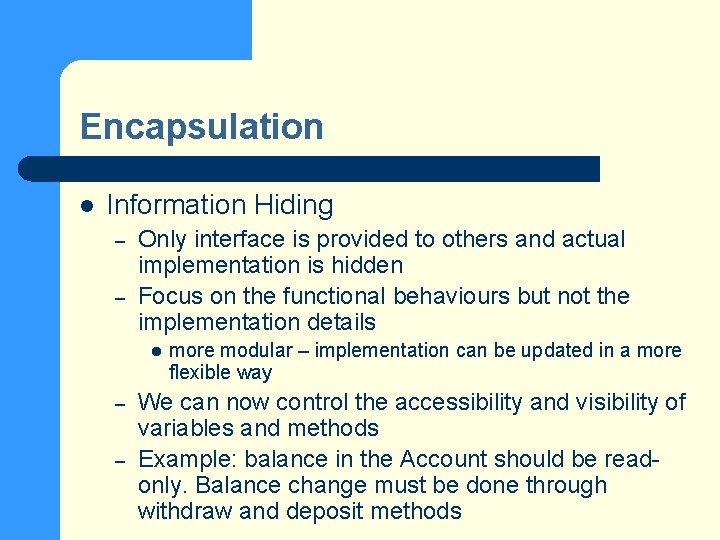
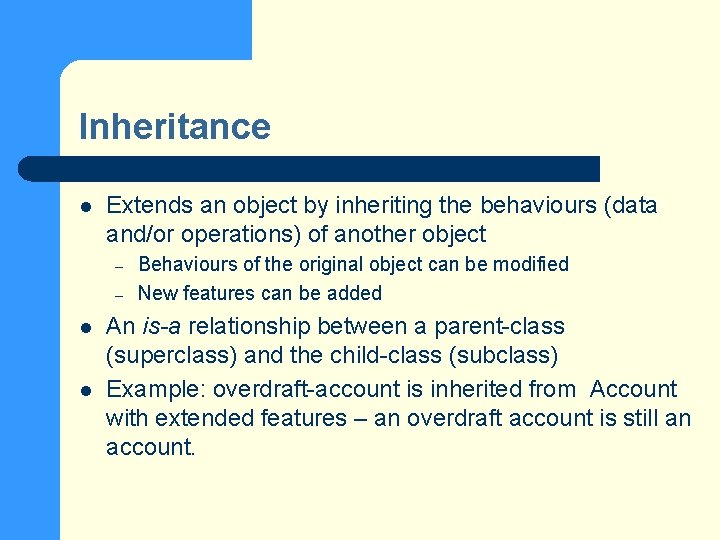
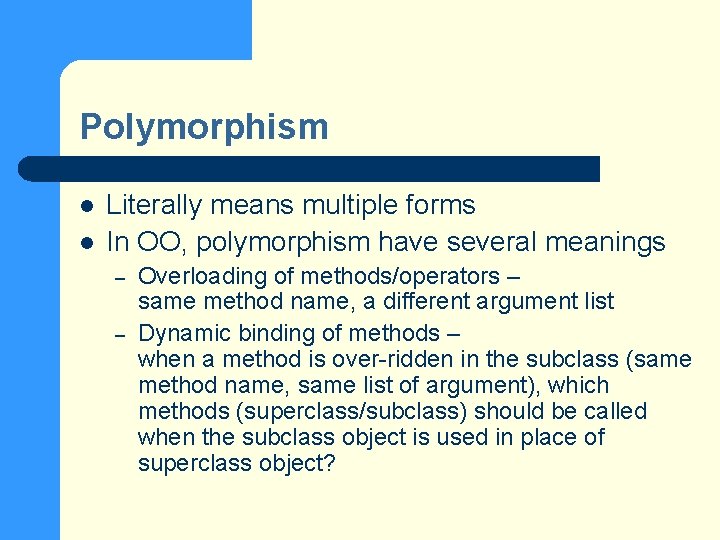
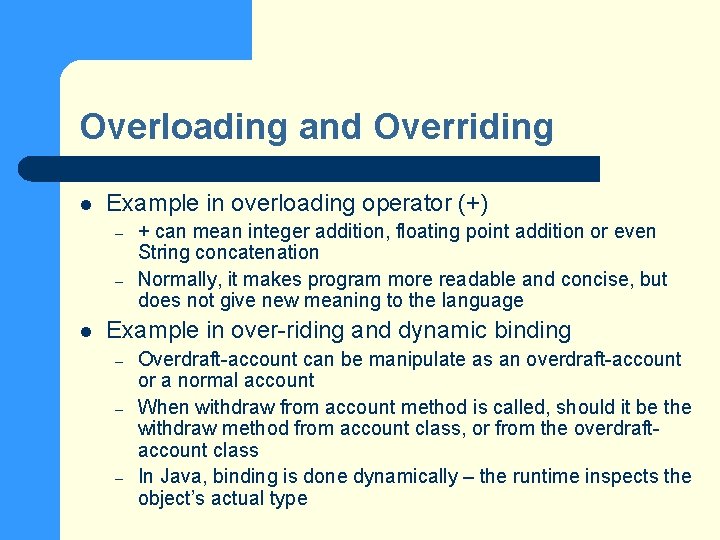
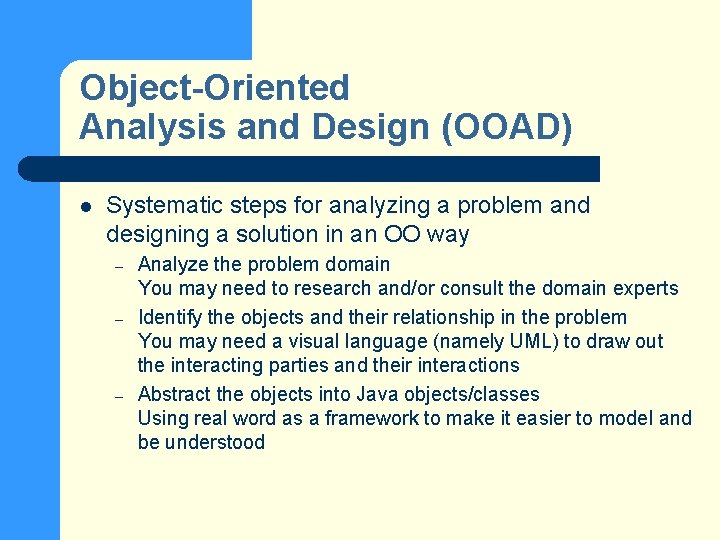
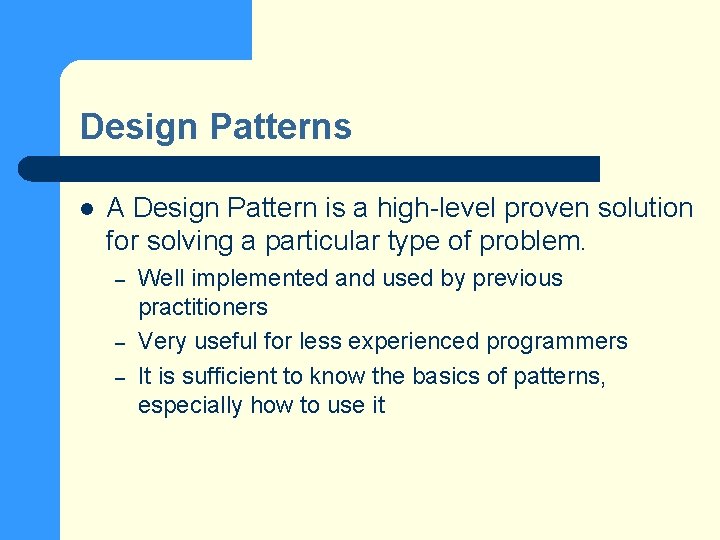
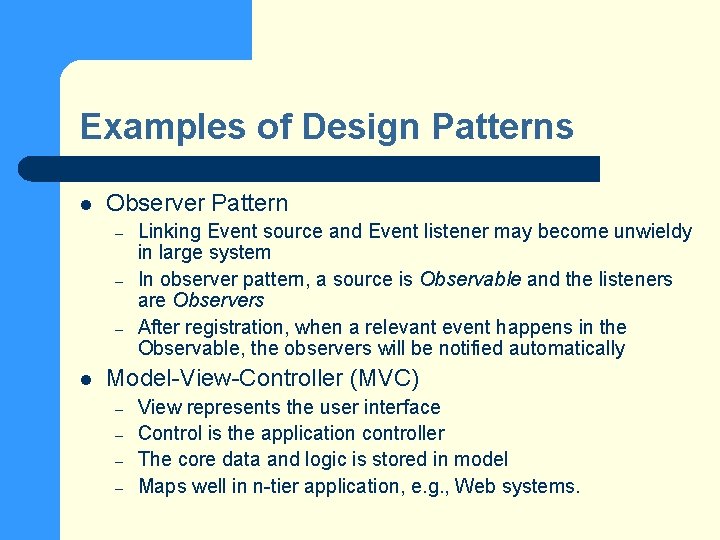
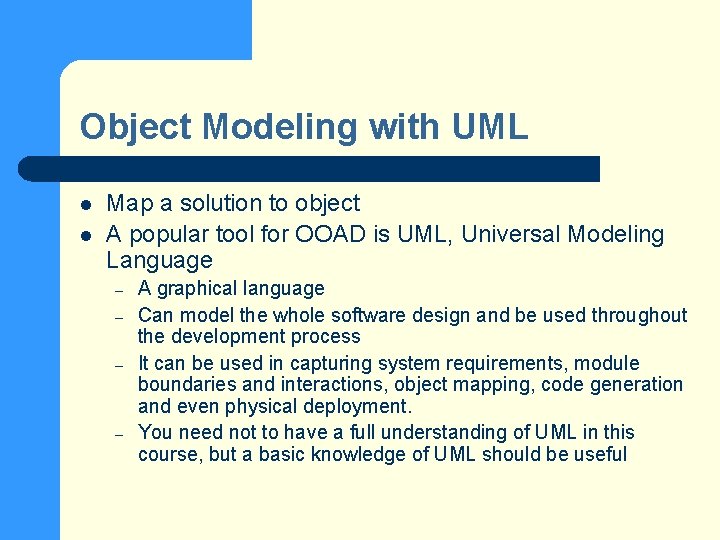
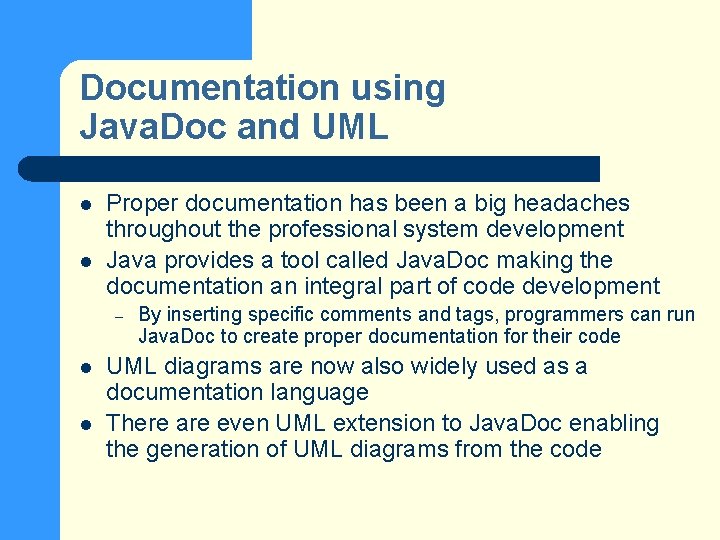
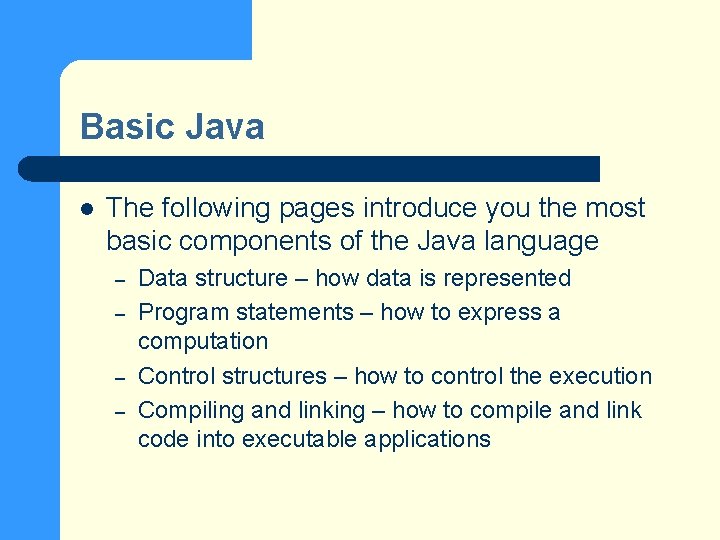
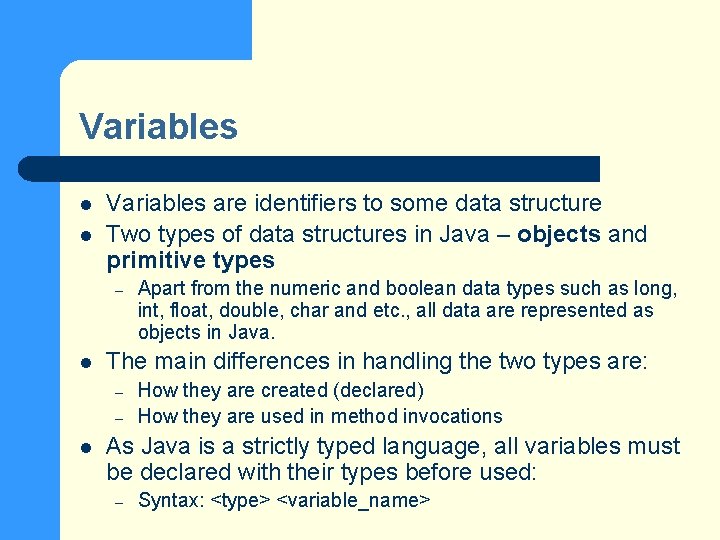
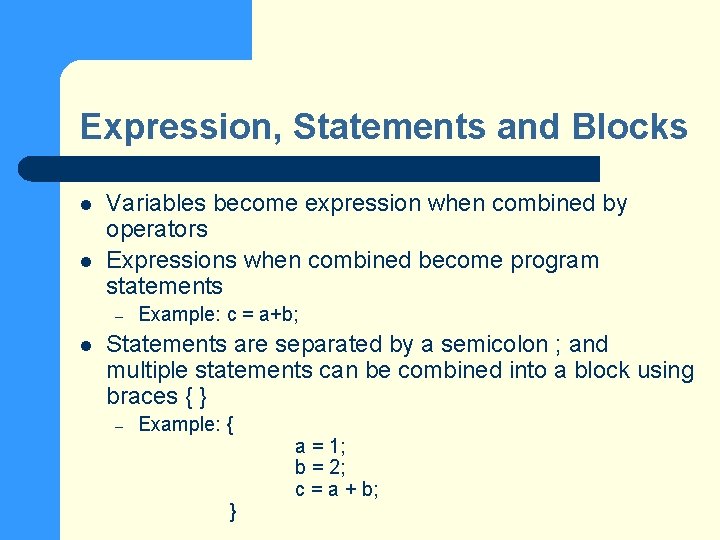
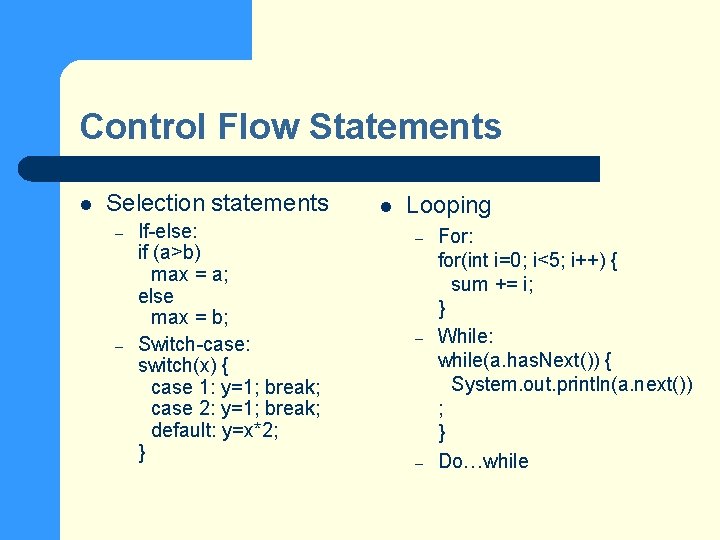
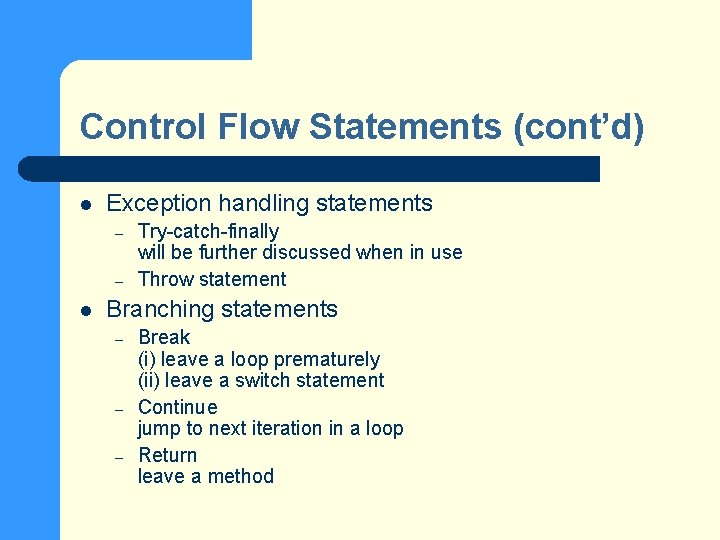
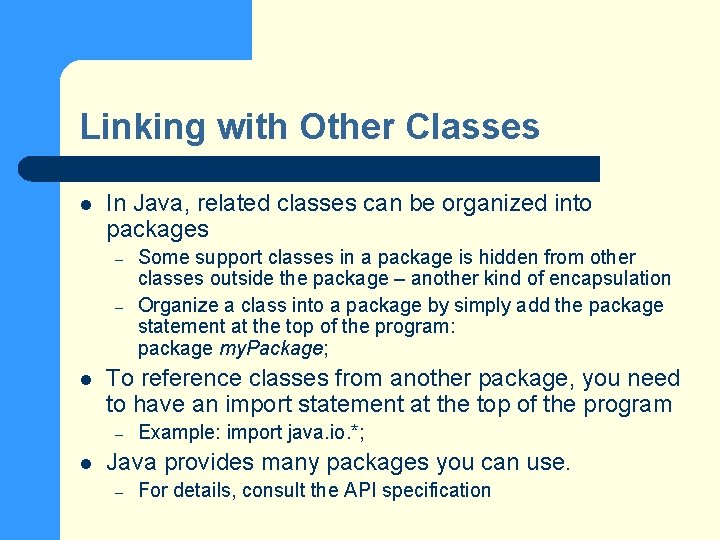
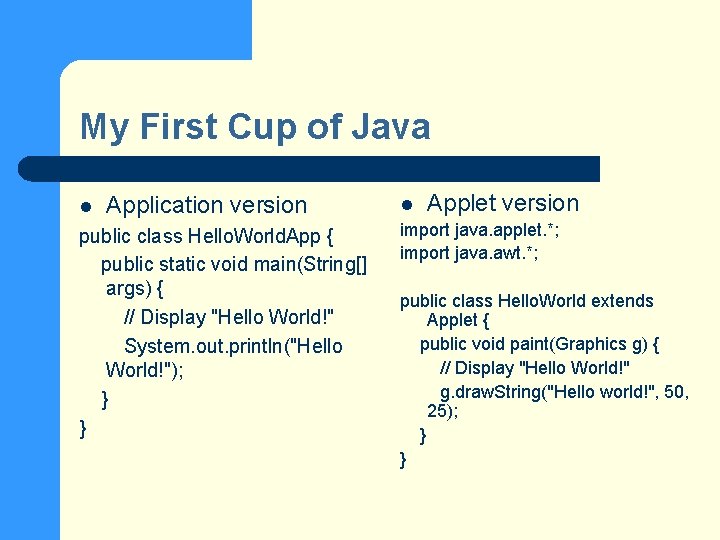
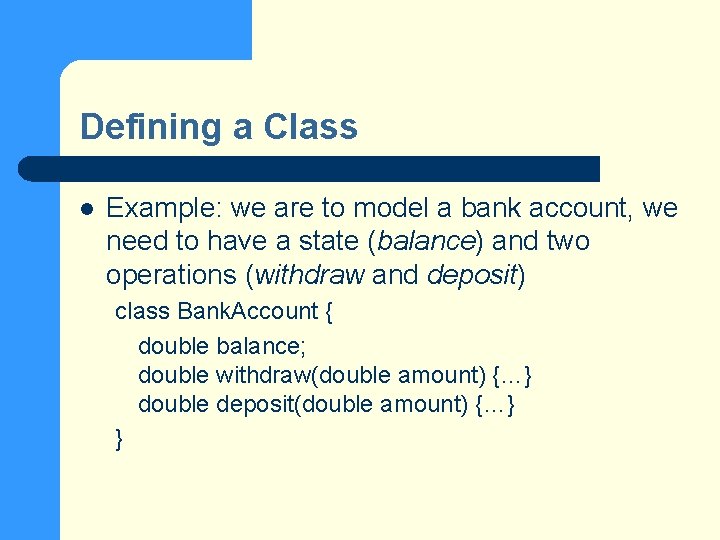
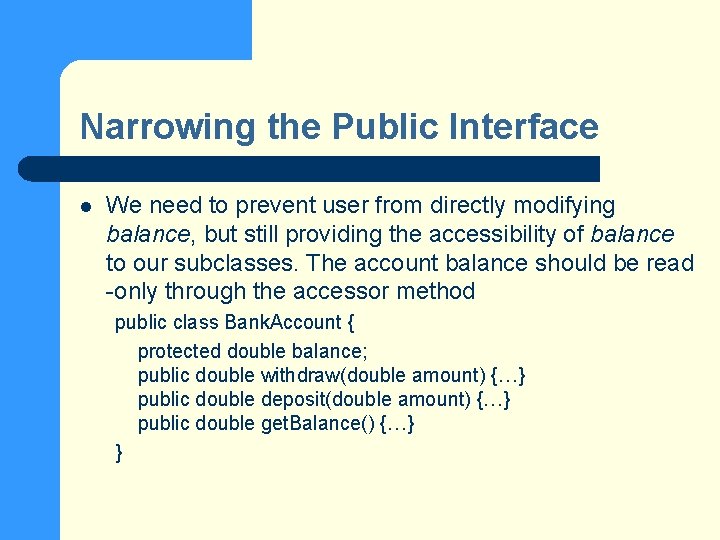
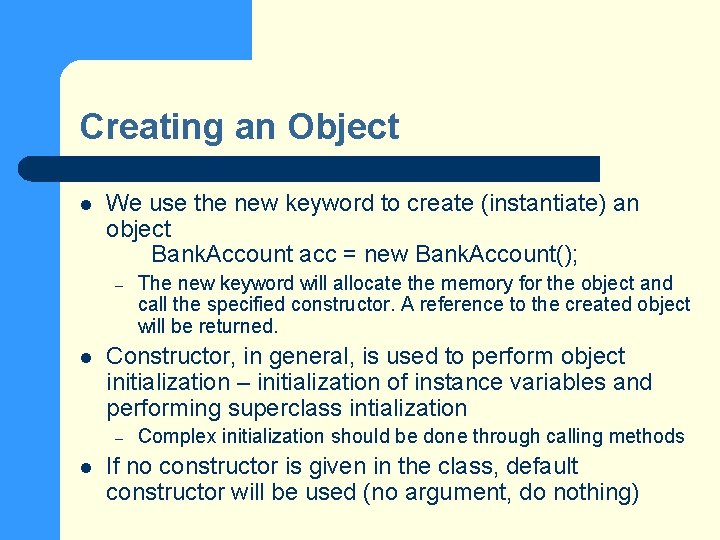
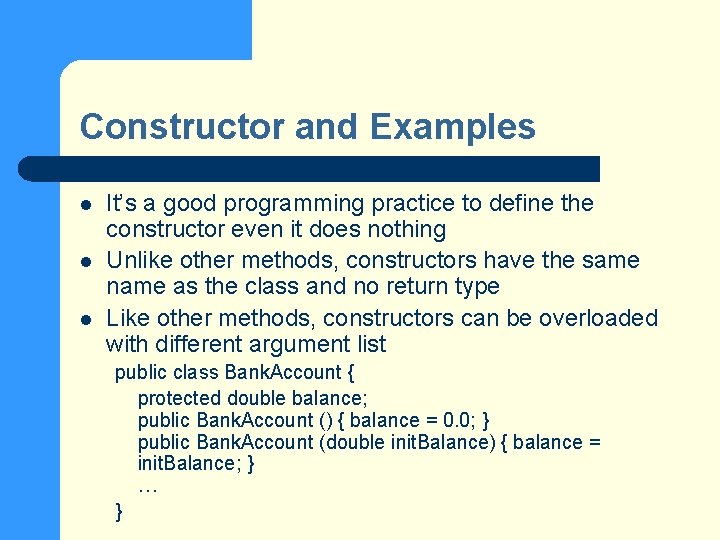
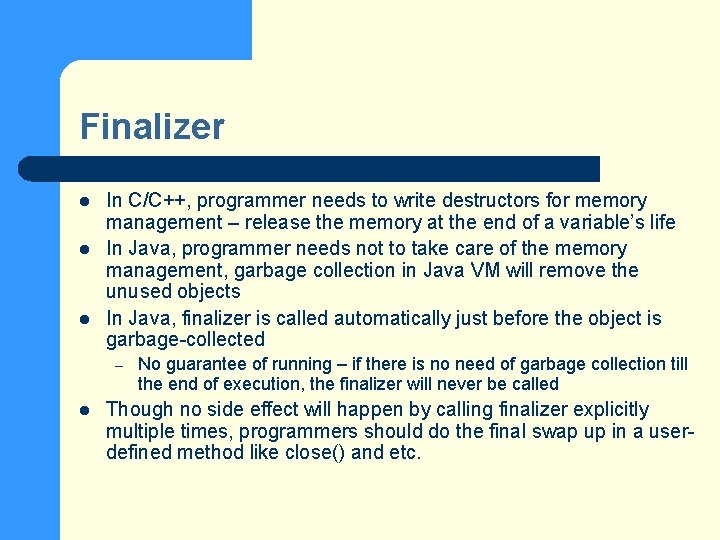
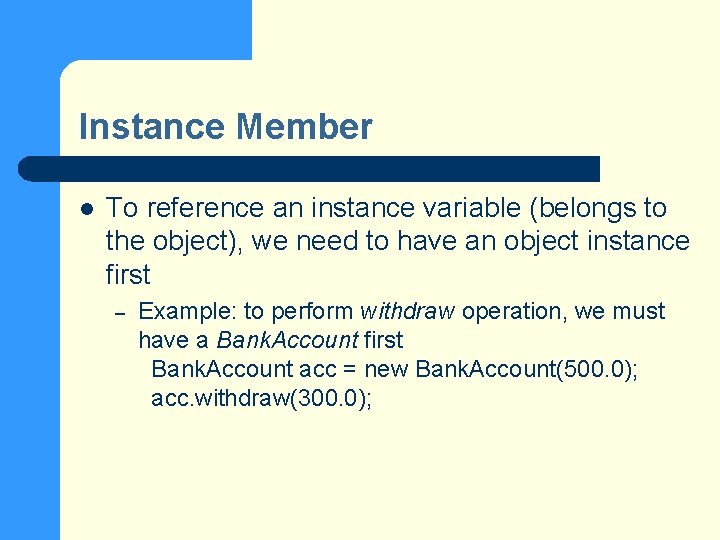
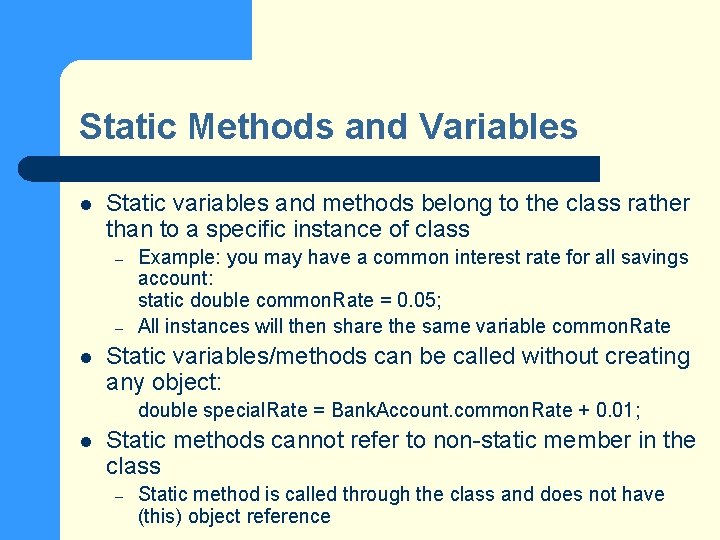
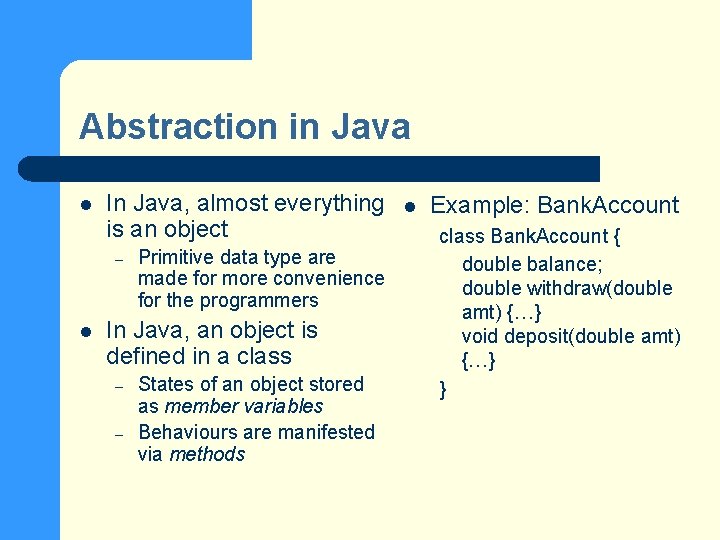
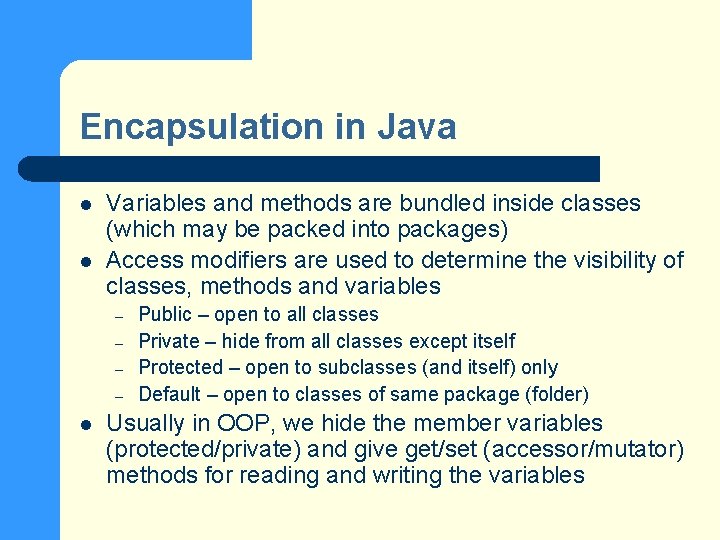
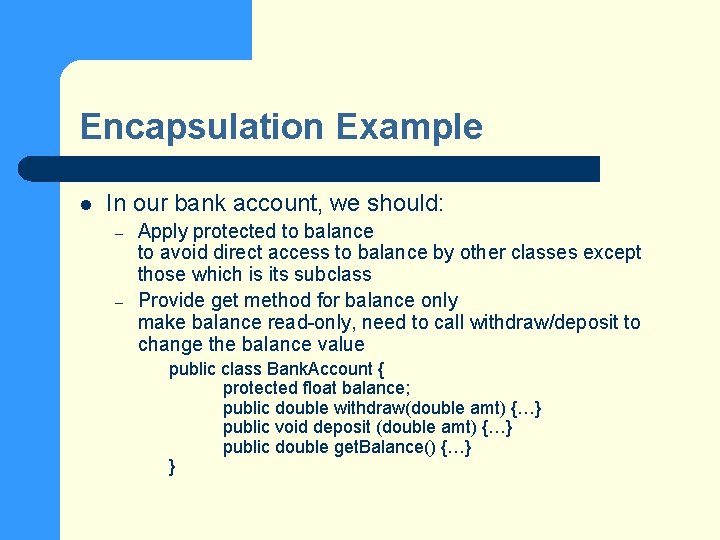
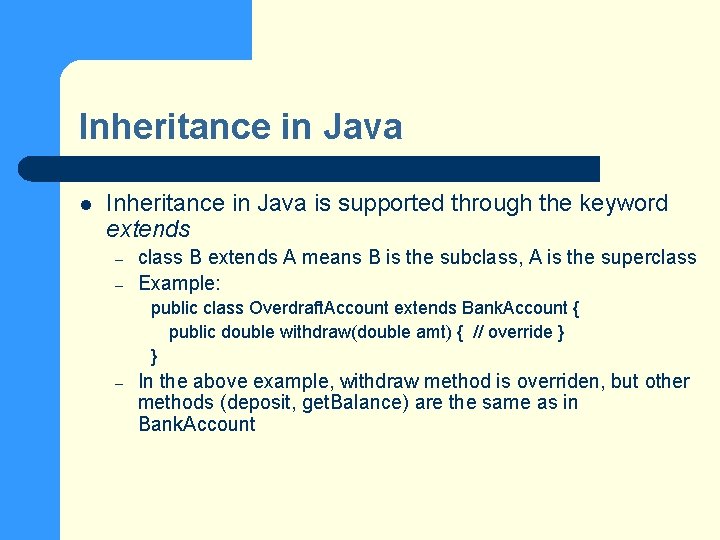
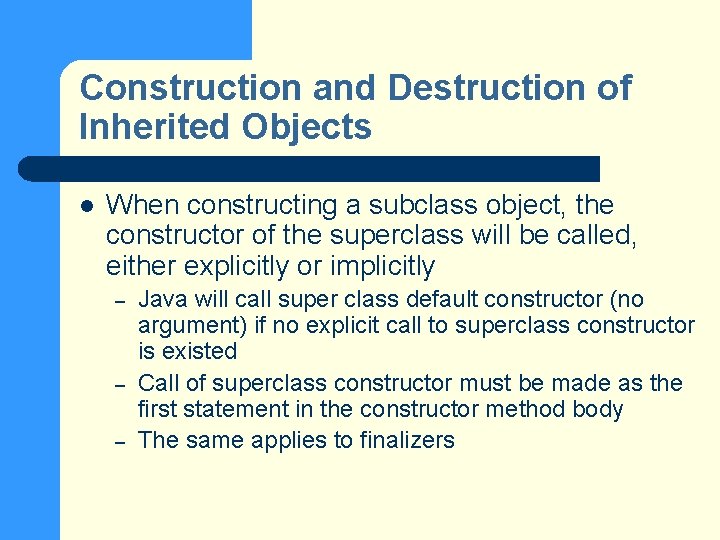
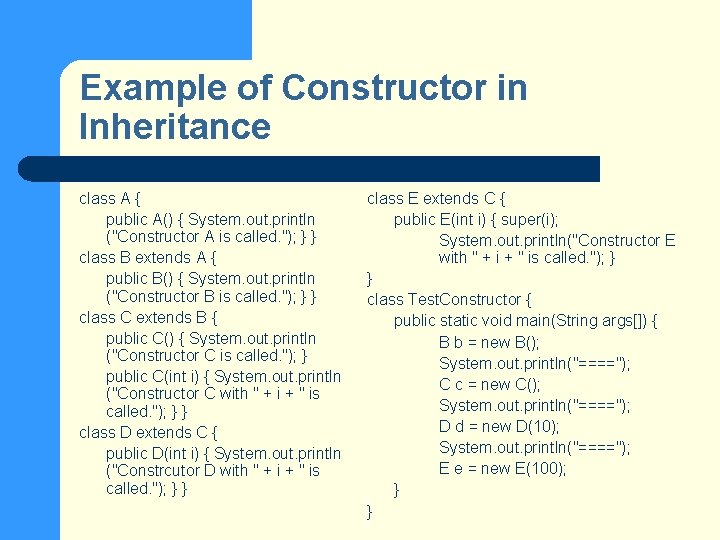
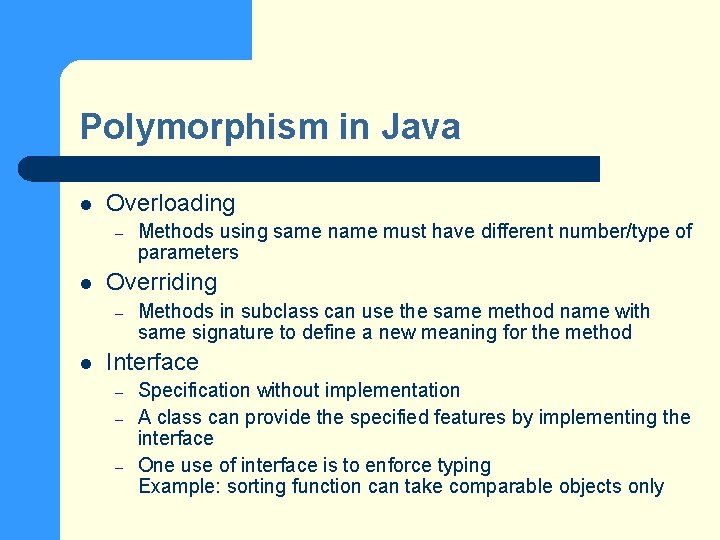
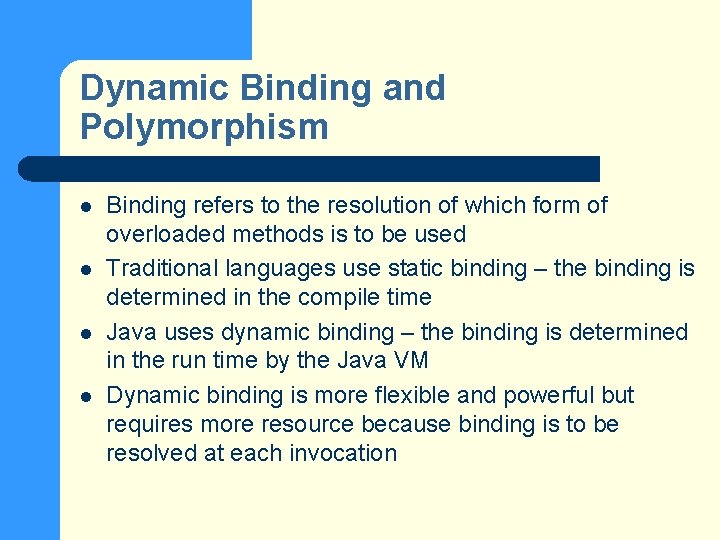
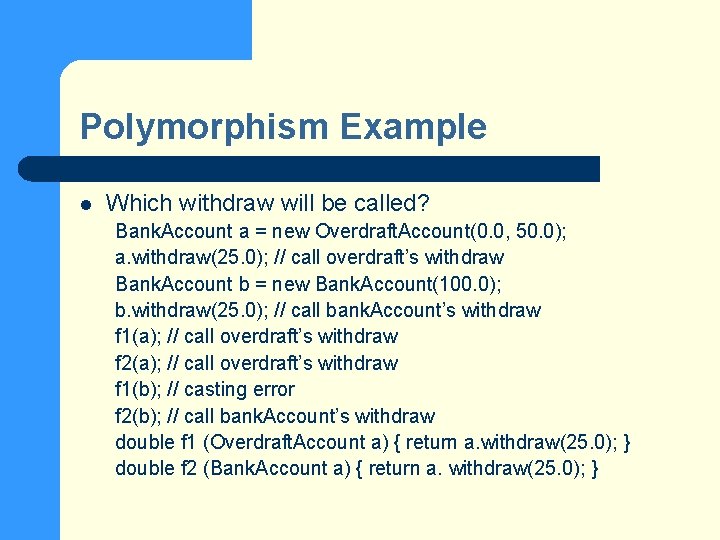
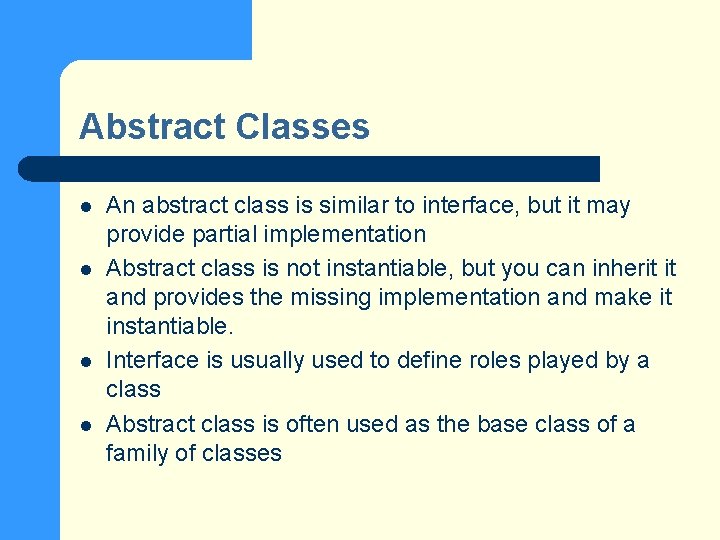
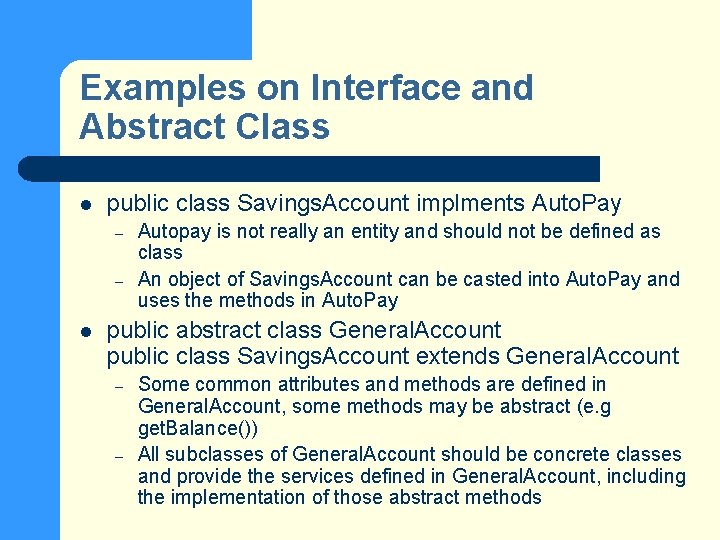
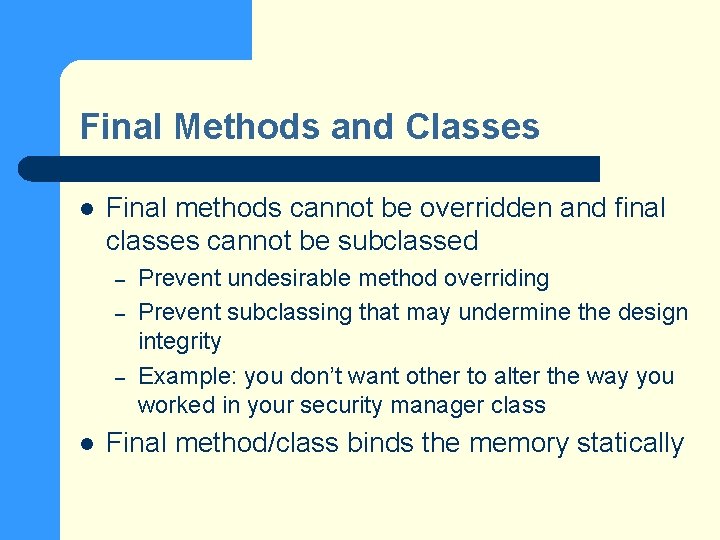
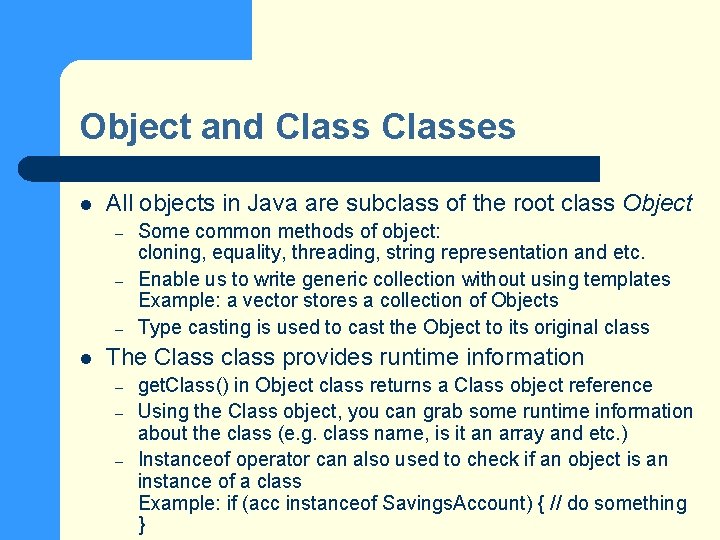
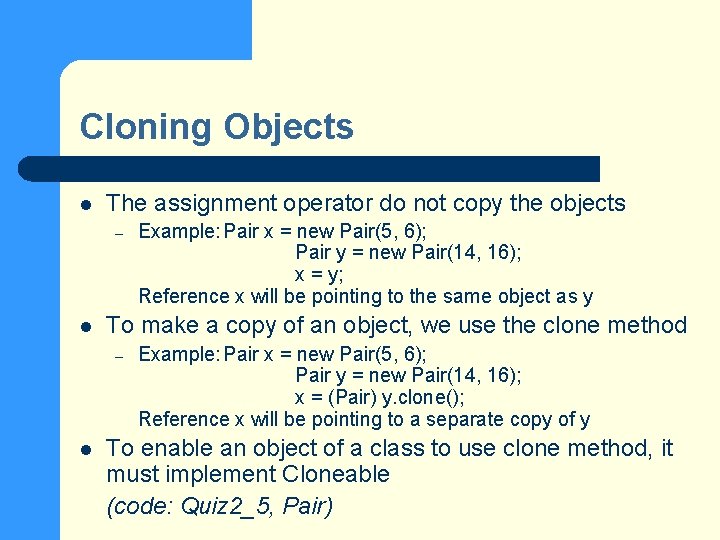
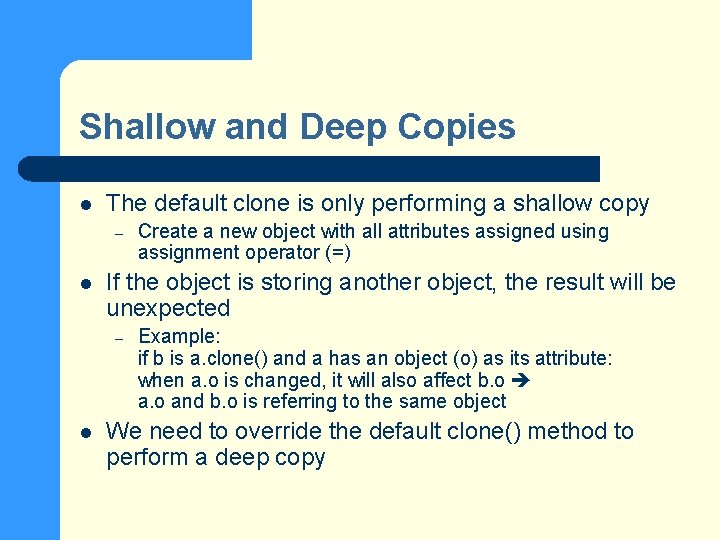
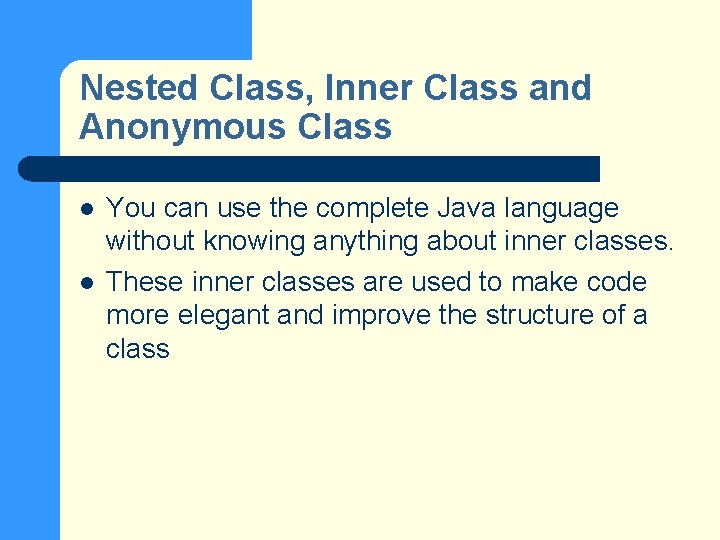
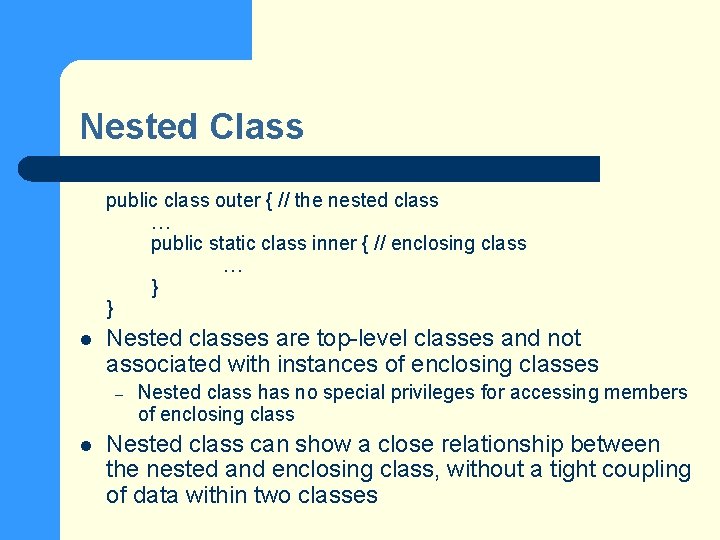
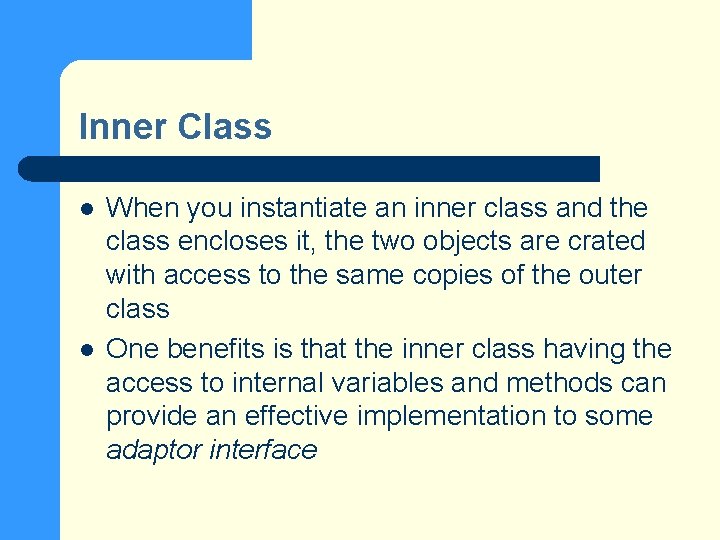
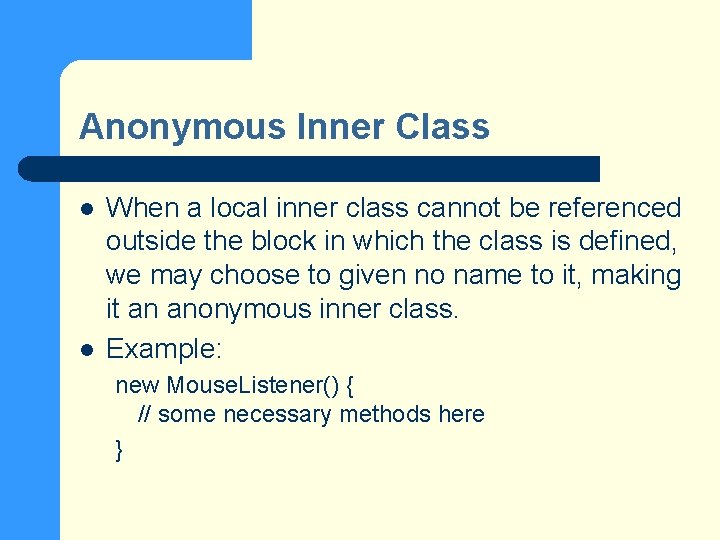
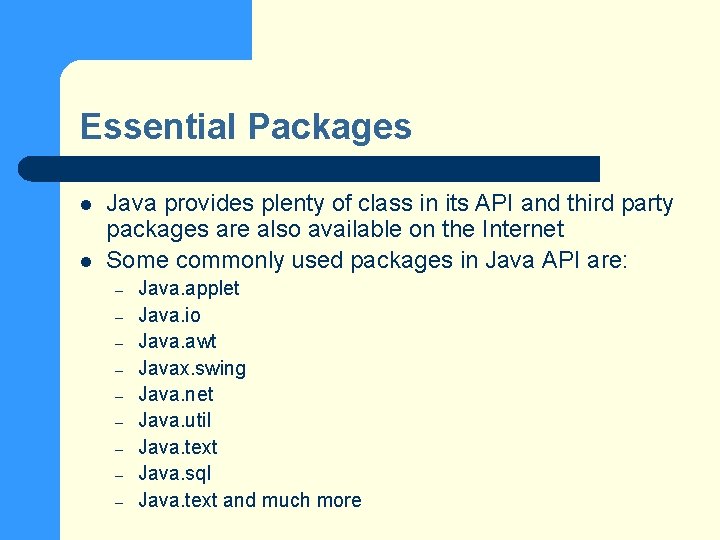
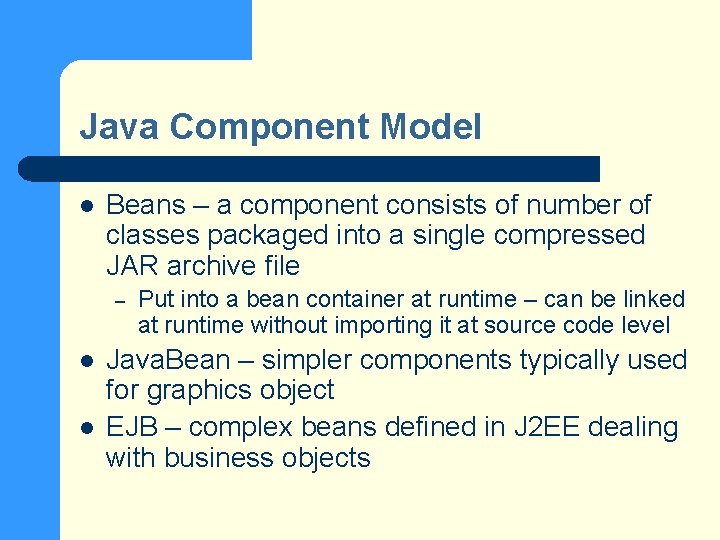
- Slides: 52
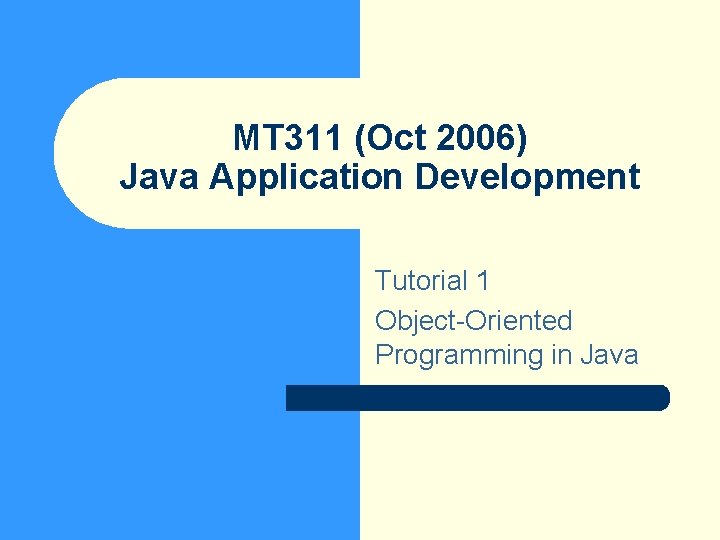
MT 311 (Oct 2006) Java Application Development Tutorial 1 Object-Oriented Programming in Java
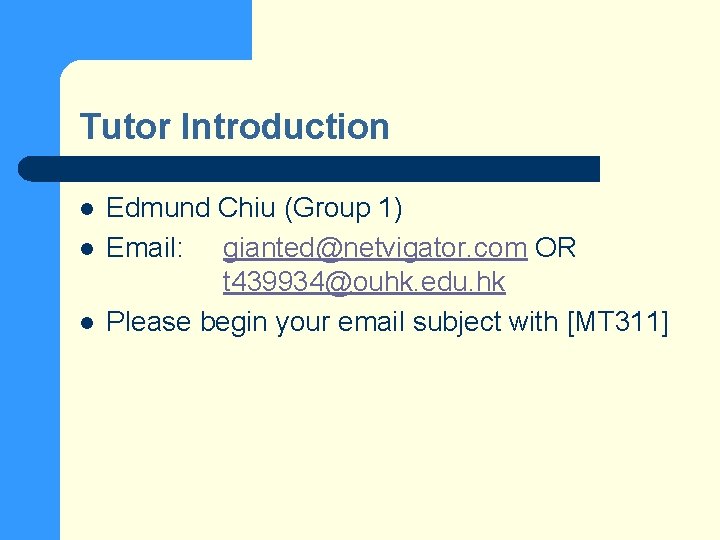
Tutor Introduction l l l Edmund Chiu (Group 1) Email: gianted@netvigator. com OR t 439934@ouhk. edu. hk Please begin your email subject with [MT 311]
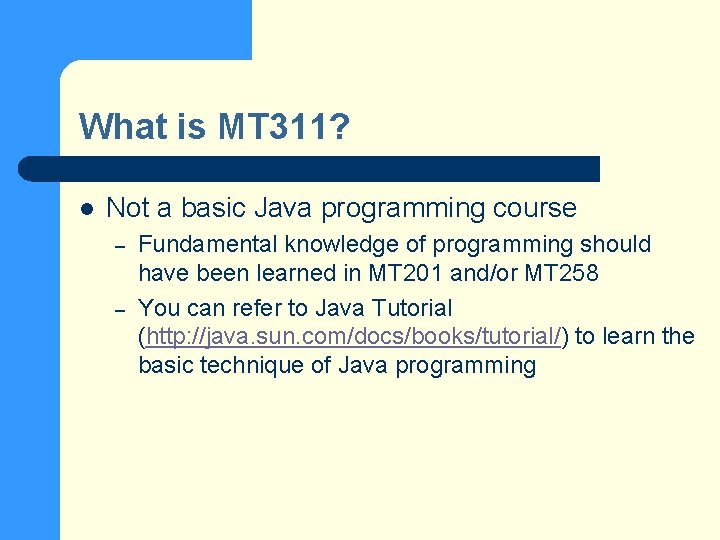
What is MT 311? l Not a basic Java programming course – – Fundamental knowledge of programming should have been learned in MT 201 and/or MT 258 You can refer to Java Tutorial (http: //java. sun. com/docs/books/tutorial/) to learn the basic technique of Java programming
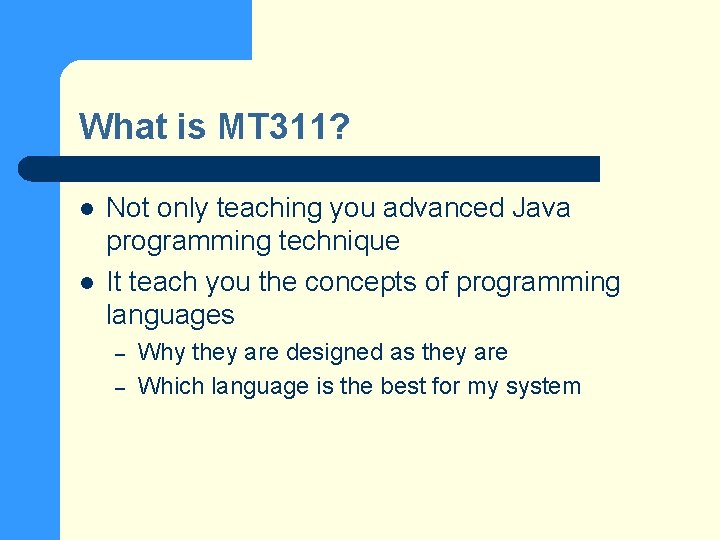
What is MT 311? l l Not only teaching you advanced Java programming technique It teach you the concepts of programming languages – – Why they are designed as they are Which language is the best for my system
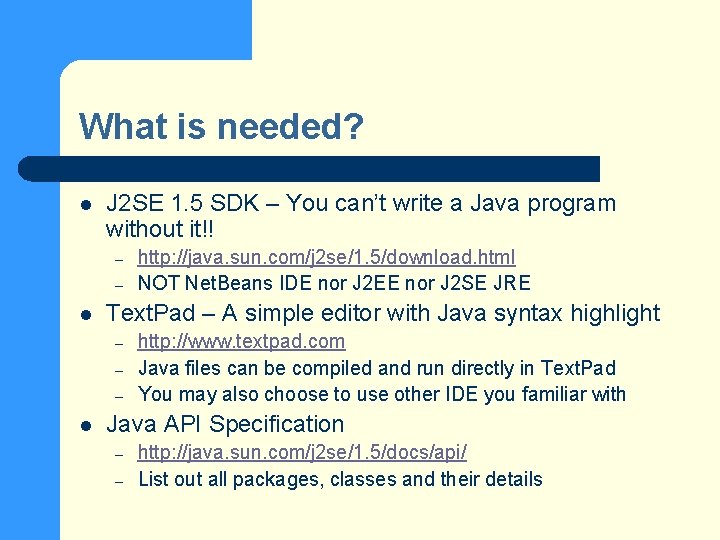
What is needed? l J 2 SE 1. 5 SDK – You can’t write a Java program without it!! – – l Text. Pad – A simple editor with Java syntax highlight – – – l http: //java. sun. com/j 2 se/1. 5/download. html NOT Net. Beans IDE nor J 2 EE nor J 2 SE JRE http: //www. textpad. com Java files can be compiled and run directly in Text. Pad You may also choose to use other IDE you familiar with Java API Specification – – http: //java. sun. com/j 2 se/1. 5/docs/api/ List out all packages, classes and their details
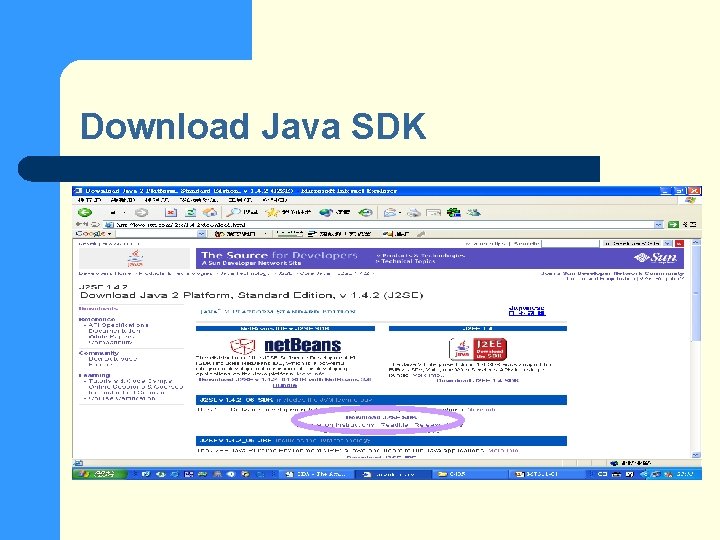
Download Java SDK
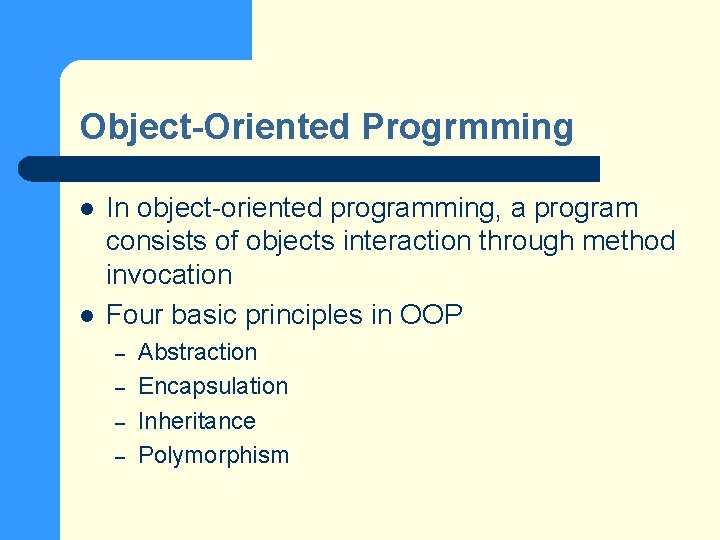
Object-Oriented Progrmming l l In object-oriented programming, a program consists of objects interaction through method invocation Four basic principles in OOP – – Abstraction Encapsulation Inheritance Polymorphism
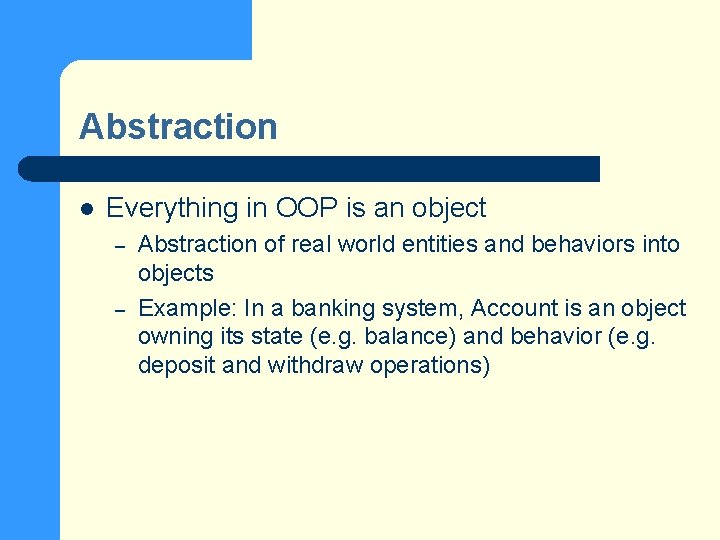
Abstraction l Everything in OOP is an object – – Abstraction of real world entities and behaviors into objects Example: In a banking system, Account is an object owning its state (e. g. balance) and behavior (e. g. deposit and withdraw operations)
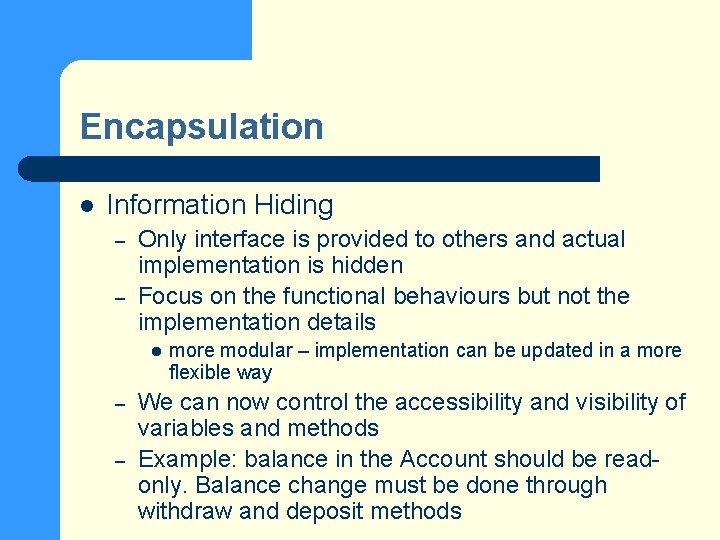
Encapsulation l Information Hiding – – Only interface is provided to others and actual implementation is hidden Focus on the functional behaviours but not the implementation details l – – more modular – implementation can be updated in a more flexible way We can now control the accessibility and visibility of variables and methods Example: balance in the Account should be readonly. Balance change must be done through withdraw and deposit methods
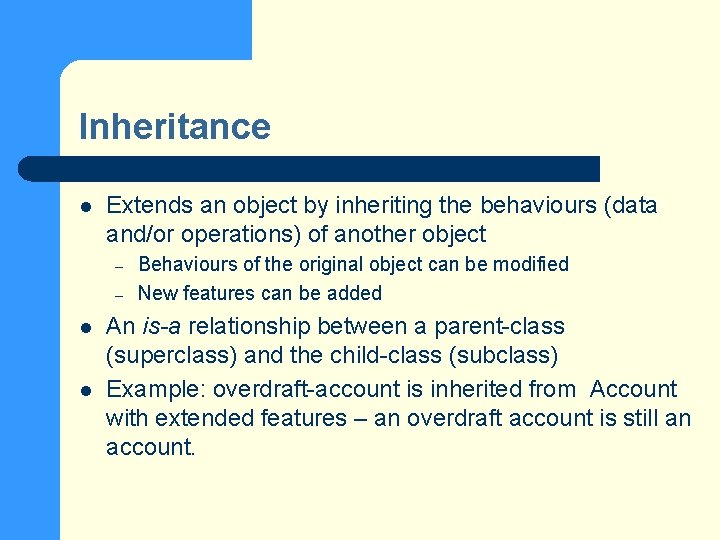
Inheritance l Extends an object by inheriting the behaviours (data and/or operations) of another object – – l l Behaviours of the original object can be modified New features can be added An is-a relationship between a parent-class (superclass) and the child-class (subclass) Example: overdraft-account is inherited from Account with extended features – an overdraft account is still an account.
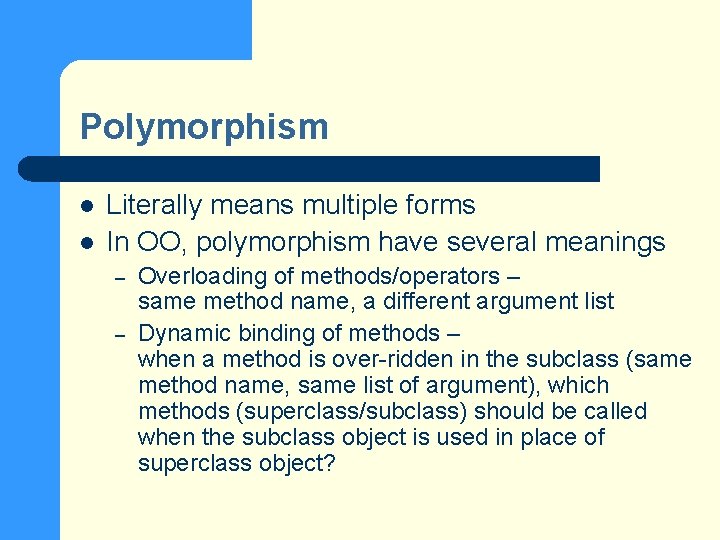
Polymorphism l l Literally means multiple forms In OO, polymorphism have several meanings – – Overloading of methods/operators – same method name, a different argument list Dynamic binding of methods – when a method is over-ridden in the subclass (same method name, same list of argument), which methods (superclass/subclass) should be called when the subclass object is used in place of superclass object?
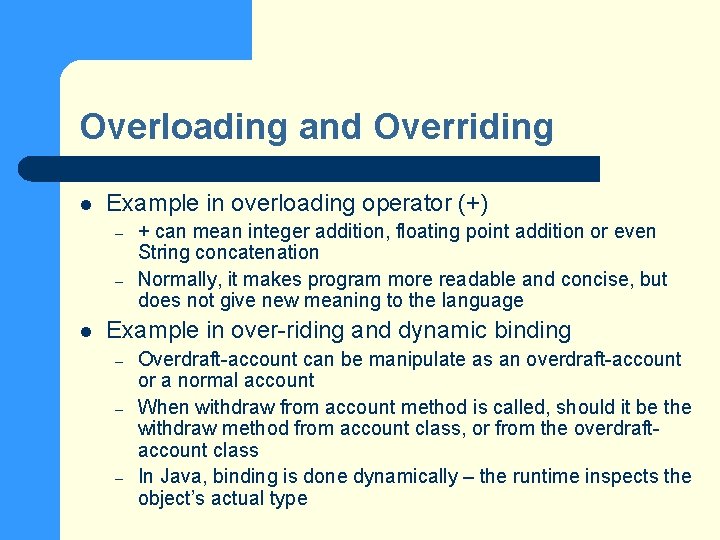
Overloading and Overriding l Example in overloading operator (+) – – l + can mean integer addition, floating point addition or even String concatenation Normally, it makes program more readable and concise, but does not give new meaning to the language Example in over-riding and dynamic binding – – – Overdraft-account can be manipulate as an overdraft-account or a normal account When withdraw from account method is called, should it be the withdraw method from account class, or from the overdraftaccount class In Java, binding is done dynamically – the runtime inspects the object’s actual type
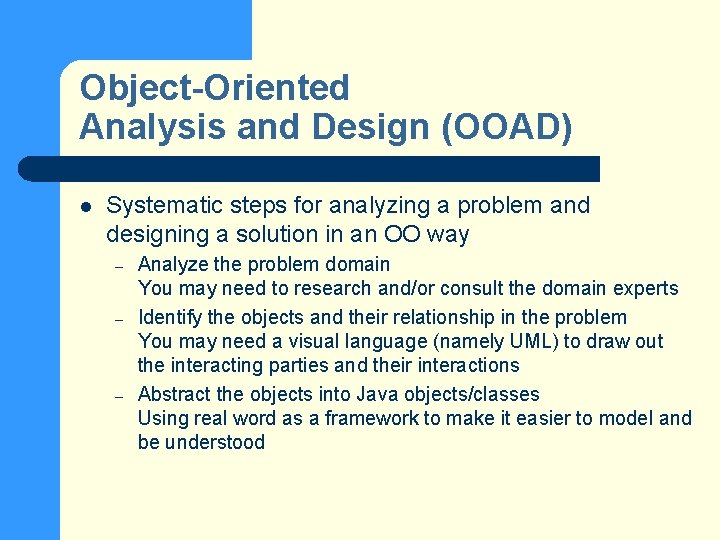
Object-Oriented Analysis and Design (OOAD) l Systematic steps for analyzing a problem and designing a solution in an OO way – – – Analyze the problem domain You may need to research and/or consult the domain experts Identify the objects and their relationship in the problem You may need a visual language (namely UML) to draw out the interacting parties and their interactions Abstract the objects into Java objects/classes Using real word as a framework to make it easier to model and be understood
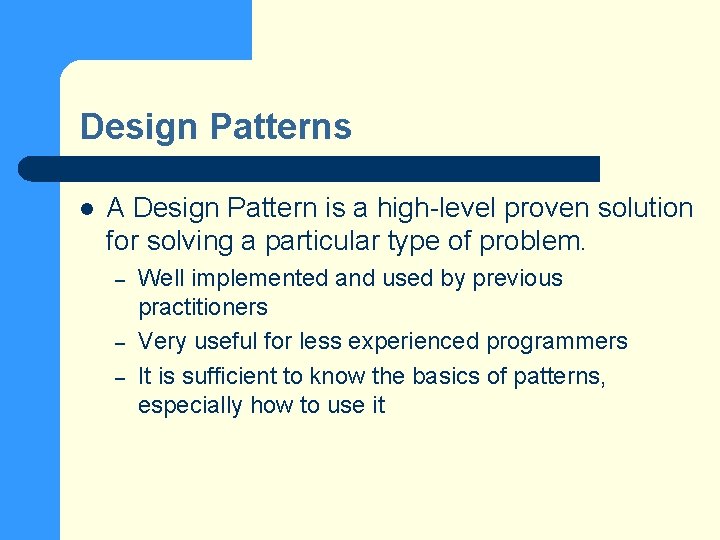
Design Patterns l A Design Pattern is a high-level proven solution for solving a particular type of problem. – – – Well implemented and used by previous practitioners Very useful for less experienced programmers It is sufficient to know the basics of patterns, especially how to use it
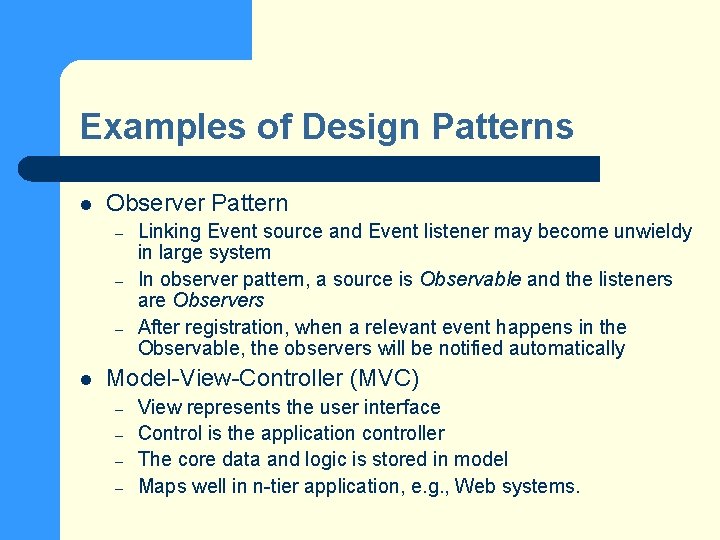
Examples of Design Patterns l Observer Pattern – – – l Linking Event source and Event listener may become unwieldy in large system In observer pattern, a source is Observable and the listeners are Observers After registration, when a relevant event happens in the Observable, the observers will be notified automatically Model-View-Controller (MVC) – – View represents the user interface Control is the application controller The core data and logic is stored in model Maps well in n-tier application, e. g. , Web systems.
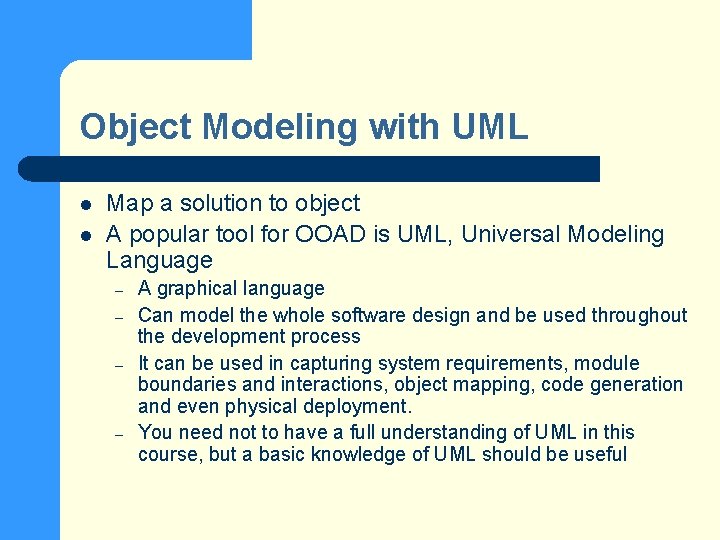
Object Modeling with UML l l Map a solution to object A popular tool for OOAD is UML, Universal Modeling Language – – A graphical language Can model the whole software design and be used throughout the development process It can be used in capturing system requirements, module boundaries and interactions, object mapping, code generation and even physical deployment. You need not to have a full understanding of UML in this course, but a basic knowledge of UML should be useful
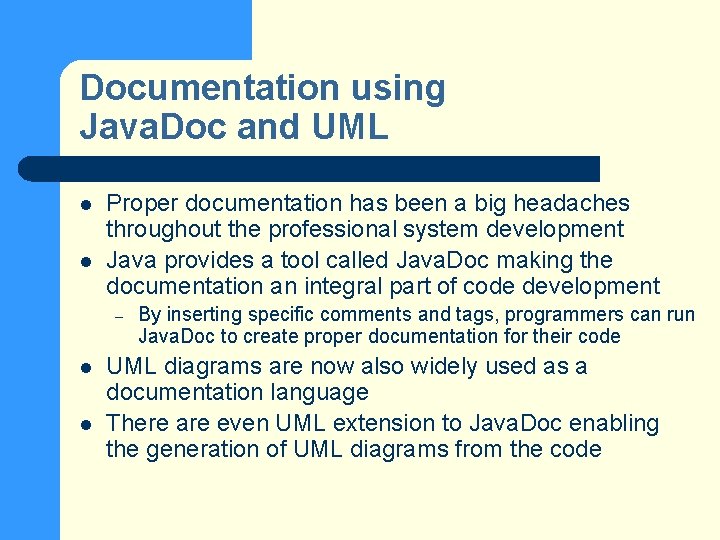
Documentation using Java. Doc and UML l l Proper documentation has been a big headaches throughout the professional system development Java provides a tool called Java. Doc making the documentation an integral part of code development – l l By inserting specific comments and tags, programmers can run Java. Doc to create proper documentation for their code UML diagrams are now also widely used as a documentation language There are even UML extension to Java. Doc enabling the generation of UML diagrams from the code
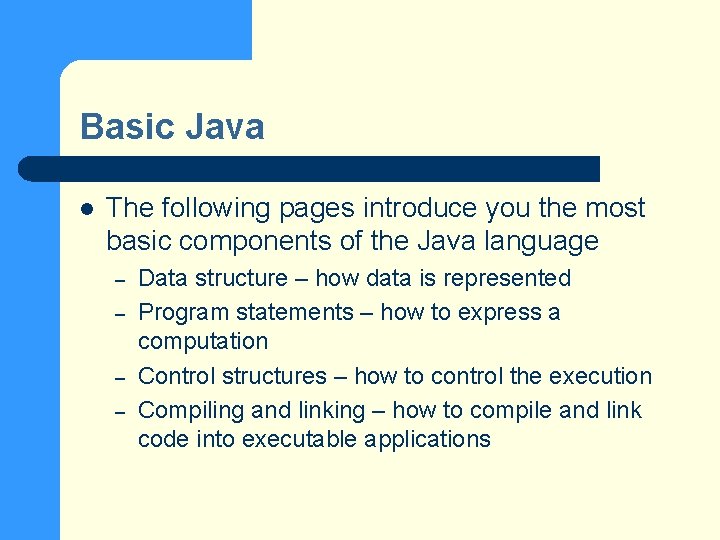
Basic Java l The following pages introduce you the most basic components of the Java language – – Data structure – how data is represented Program statements – how to express a computation Control structures – how to control the execution Compiling and linking – how to compile and link code into executable applications
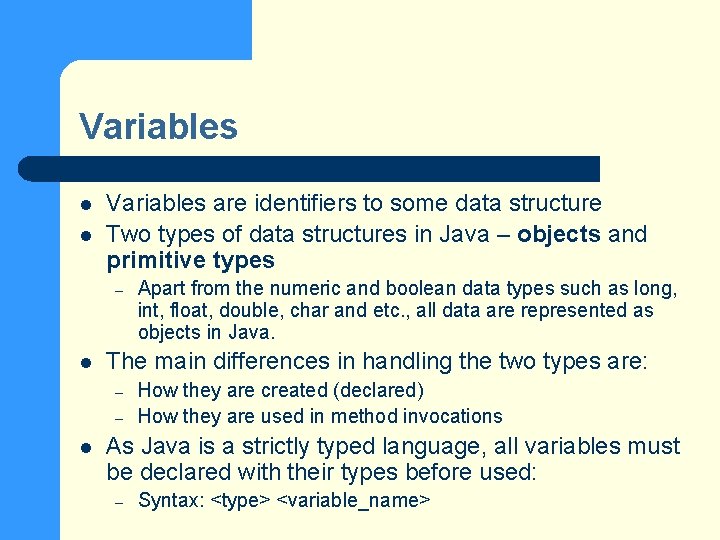
Variables l l Variables are identifiers to some data structure Two types of data structures in Java – objects and primitive types – l The main differences in handling the two types are: – – l Apart from the numeric and boolean data types such as long, int, float, double, char and etc. , all data are represented as objects in Java. How they are created (declared) How they are used in method invocations As Java is a strictly typed language, all variables must be declared with their types before used: – Syntax: <type> <variable_name>
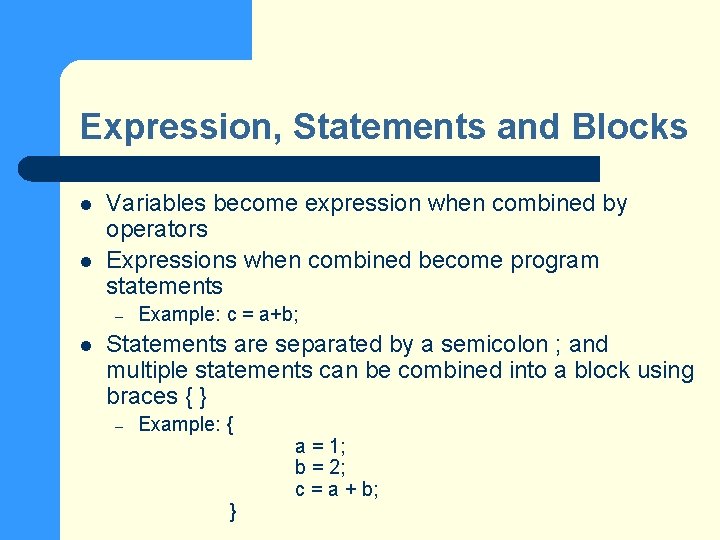
Expression, Statements and Blocks l l Variables become expression when combined by operators Expressions when combined become program statements – l Example: c = a+b; Statements are separated by a semicolon ; and multiple statements can be combined into a block using braces { } – Example: { } a = 1; b = 2; c = a + b;
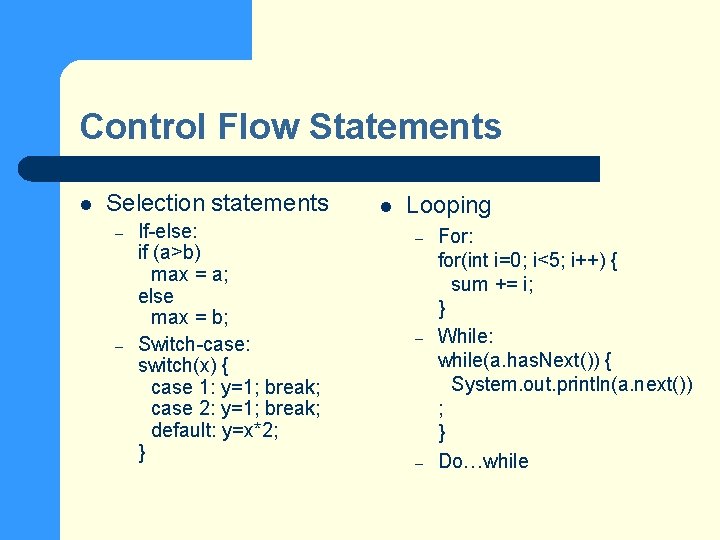
Control Flow Statements l Selection statements – – If-else: if (a>b) max = a; else max = b; Switch-case: switch(x) { case 1: y=1; break; case 2: y=1; break; default: y=x*2; } l Looping – – – For: for(int i=0; i<5; i++) { sum += i; } While: while(a. has. Next()) { System. out. println(a. next()) ; } Do…while
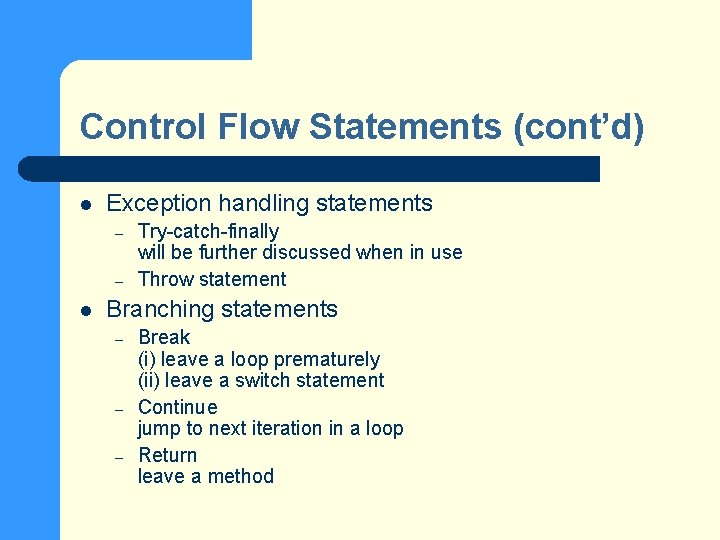
Control Flow Statements (cont’d) l Exception handling statements – – l Try-catch-finally will be further discussed when in use Throw statement Branching statements – – – Break (i) leave a loop prematurely (ii) leave a switch statement Continue jump to next iteration in a loop Return leave a method
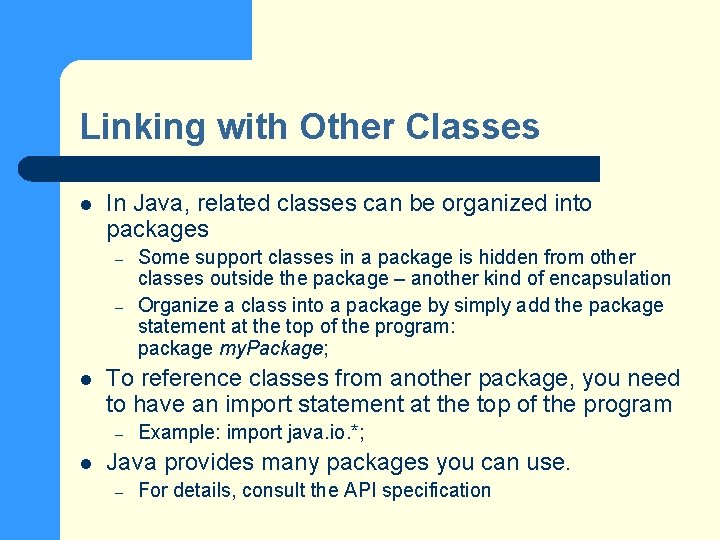
Linking with Other Classes l In Java, related classes can be organized into packages – – l To reference classes from another package, you need to have an import statement at the top of the program – l Some support classes in a package is hidden from other classes outside the package – another kind of encapsulation Organize a class into a package by simply add the package statement at the top of the program: package my. Package; Example: import java. io. *; Java provides many packages you can use. – For details, consult the API specification
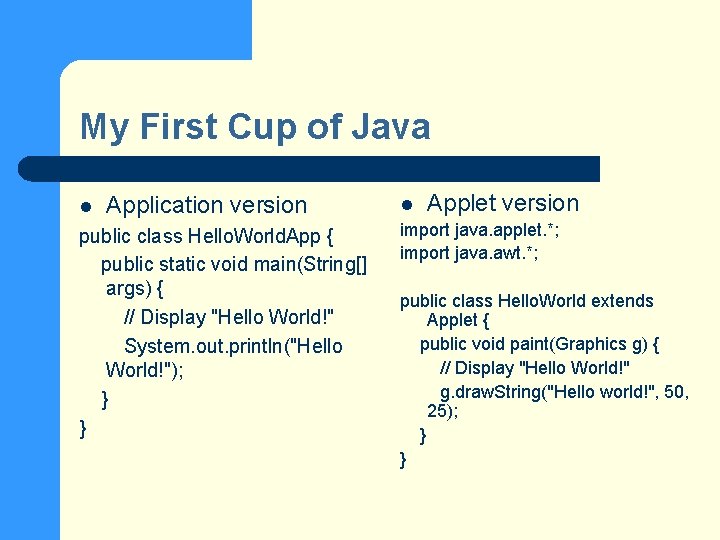
My First Cup of Java l Application version public class Hello. World. App { public static void main(String[] args) { // Display "Hello World!" System. out. println("Hello World!"); } } l Applet version import java. applet. *; import java. awt. *; public class Hello. World extends Applet { public void paint(Graphics g) { // Display "Hello World!" g. draw. String("Hello world!", 50, 25); } }
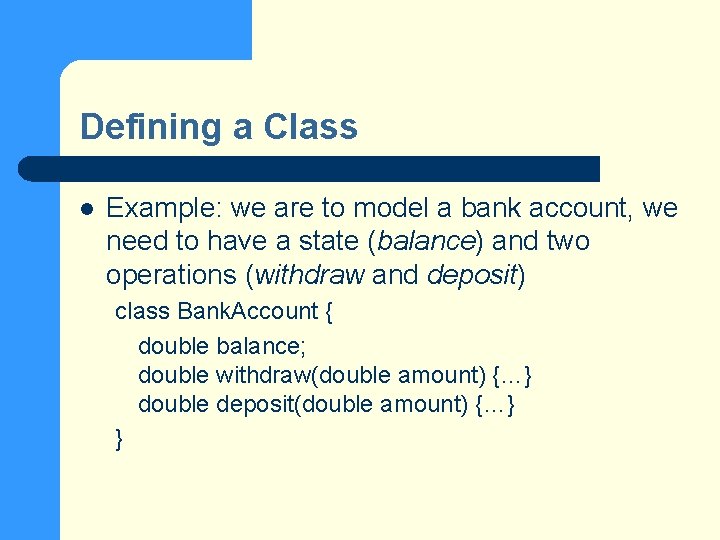
Defining a Class l Example: we are to model a bank account, we need to have a state (balance) and two operations (withdraw and deposit) class Bank. Account { double balance; double withdraw(double amount) {…} double deposit(double amount) {…} }
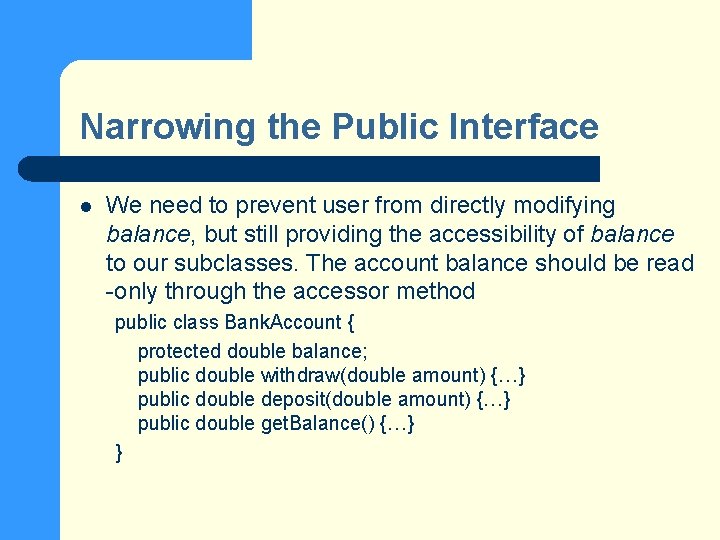
Narrowing the Public Interface l We need to prevent user from directly modifying balance, but still providing the accessibility of balance to our subclasses. The account balance should be read -only through the accessor method public class Bank. Account { protected double balance; public double withdraw(double amount) {…} public double deposit(double amount) {…} public double get. Balance() {…} }
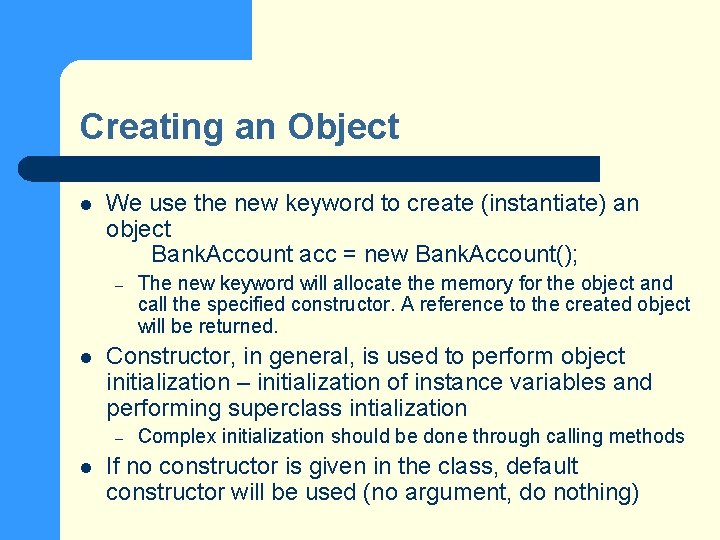
Creating an Object l We use the new keyword to create (instantiate) an object Bank. Account acc = new Bank. Account(); – l Constructor, in general, is used to perform object initialization – initialization of instance variables and performing superclass intialization – l The new keyword will allocate the memory for the object and call the specified constructor. A reference to the created object will be returned. Complex initialization should be done through calling methods If no constructor is given in the class, default constructor will be used (no argument, do nothing)
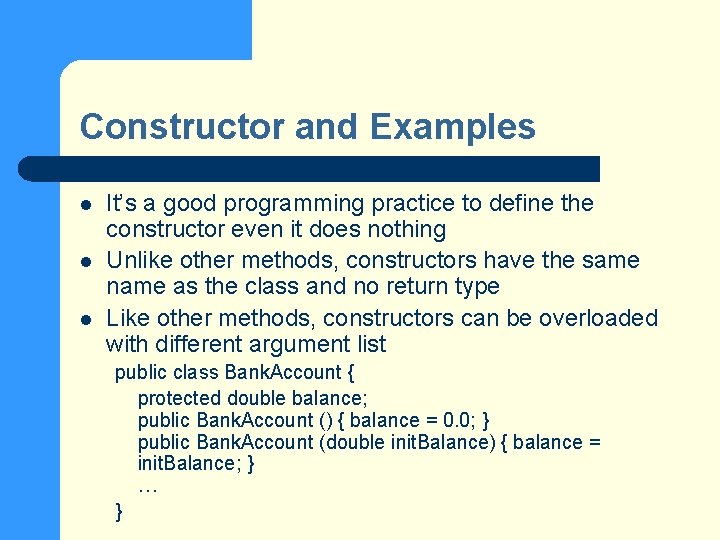
Constructor and Examples l l l It’s a good programming practice to define the constructor even it does nothing Unlike other methods, constructors have the same name as the class and no return type Like other methods, constructors can be overloaded with different argument list public class Bank. Account { protected double balance; public Bank. Account () { balance = 0. 0; } public Bank. Account (double init. Balance) { balance = init. Balance; } … }
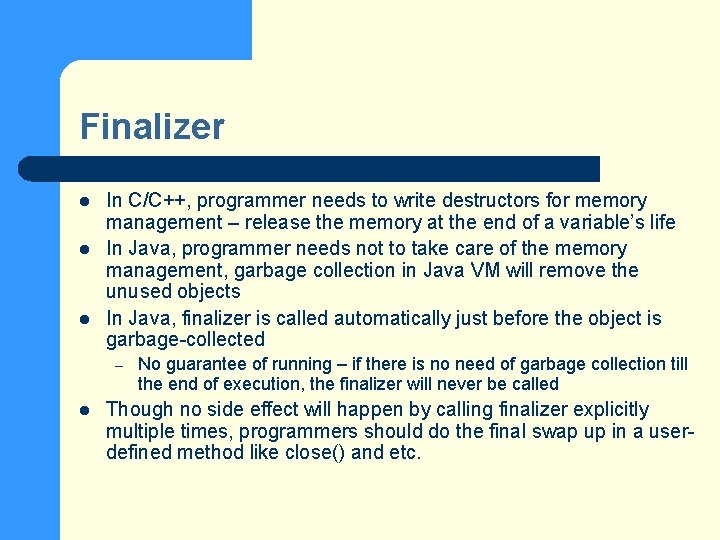
Finalizer l l l In C/C++, programmer needs to write destructors for memory management – release the memory at the end of a variable’s life In Java, programmer needs not to take care of the memory management, garbage collection in Java VM will remove the unused objects In Java, finalizer is called automatically just before the object is garbage-collected – l No guarantee of running – if there is no need of garbage collection till the end of execution, the finalizer will never be called Though no side effect will happen by calling finalizer explicitly multiple times, programmers should do the final swap up in a userdefined method like close() and etc.
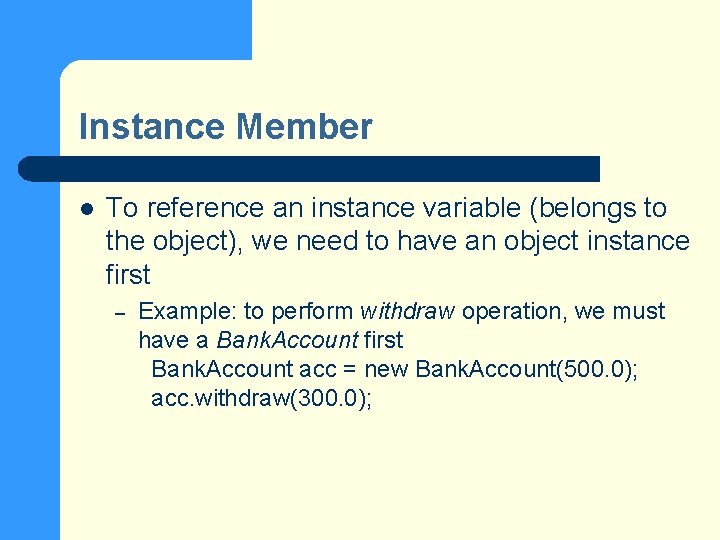
Instance Member l To reference an instance variable (belongs to the object), we need to have an object instance first – Example: to perform withdraw operation, we must have a Bank. Account first Bank. Account acc = new Bank. Account(500. 0); acc. withdraw(300. 0);
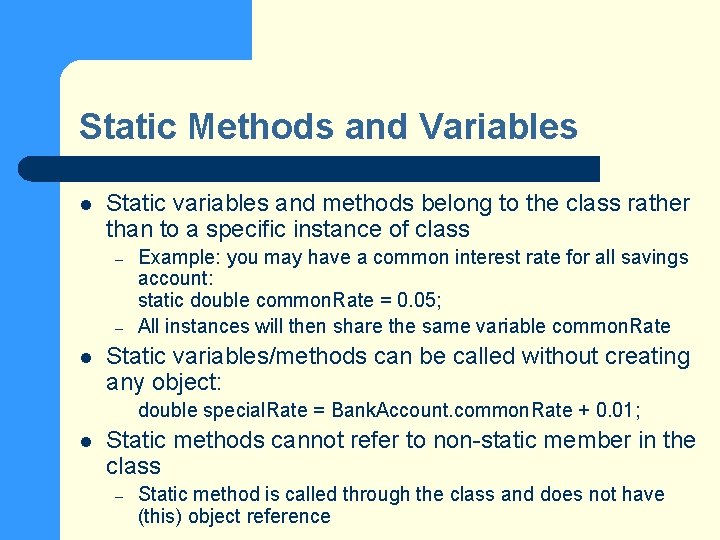
Static Methods and Variables l Static variables and methods belong to the class rather than to a specific instance of class – – l Example: you may have a common interest rate for all savings account: static double common. Rate = 0. 05; All instances will then share the same variable common. Rate Static variables/methods can be called without creating any object: double special. Rate = Bank. Account. common. Rate + 0. 01; l Static methods cannot refer to non-static member in the class – Static method is called through the class and does not have (this) object reference
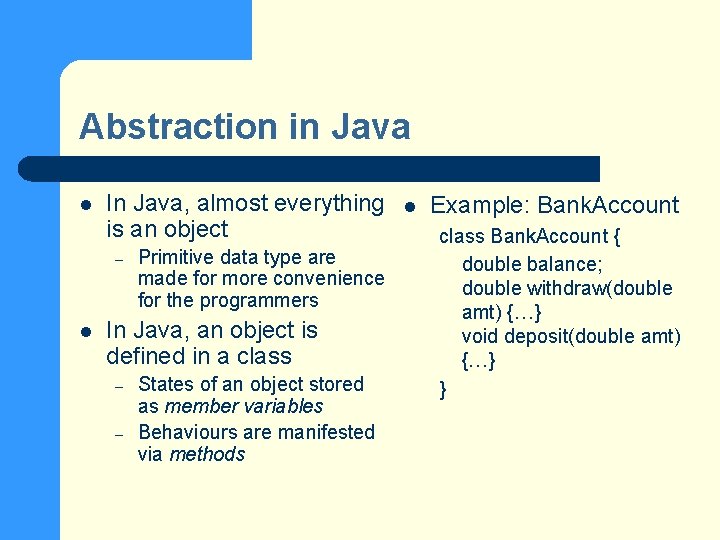
Abstraction in Java l In Java, almost everything is an object – l Primitive data type are made for more convenience for the programmers In Java, an object is defined in a class – – States of an object stored as member variables Behaviours are manifested via methods l Example: Bank. Account class Bank. Account { double balance; double withdraw(double amt) {…} void deposit(double amt) {…} }
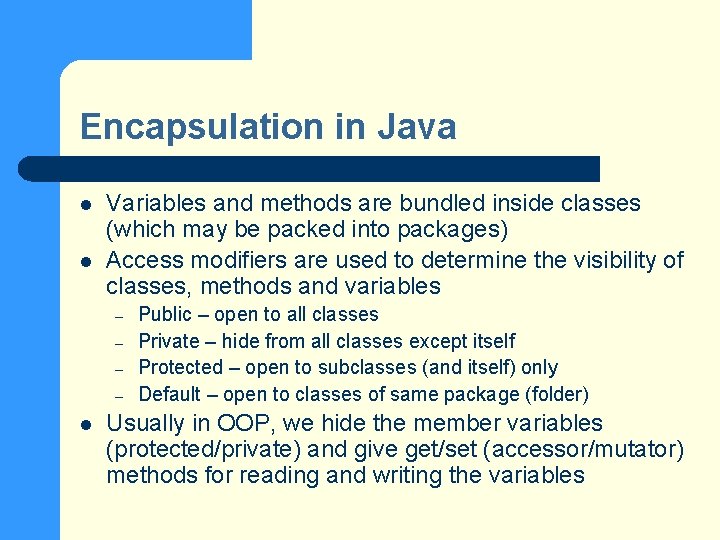
Encapsulation in Java l l Variables and methods are bundled inside classes (which may be packed into packages) Access modifiers are used to determine the visibility of classes, methods and variables – – l Public – open to all classes Private – hide from all classes except itself Protected – open to subclasses (and itself) only Default – open to classes of same package (folder) Usually in OOP, we hide the member variables (protected/private) and give get/set (accessor/mutator) methods for reading and writing the variables
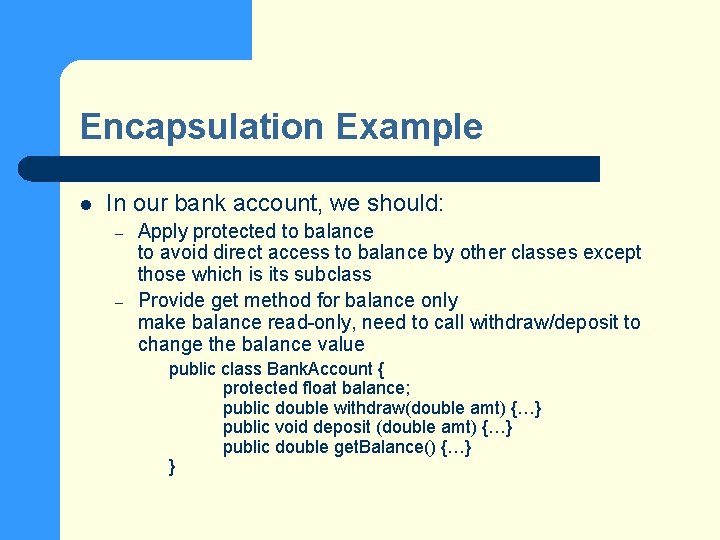
Encapsulation Example l In our bank account, we should: – – Apply protected to balance to avoid direct access to balance by other classes except those which is its subclass Provide get method for balance only make balance read-only, need to call withdraw/deposit to change the balance value public class Bank. Account { protected float balance; public double withdraw(double amt) {…} public void deposit (double amt) {…} public double get. Balance() {…} }
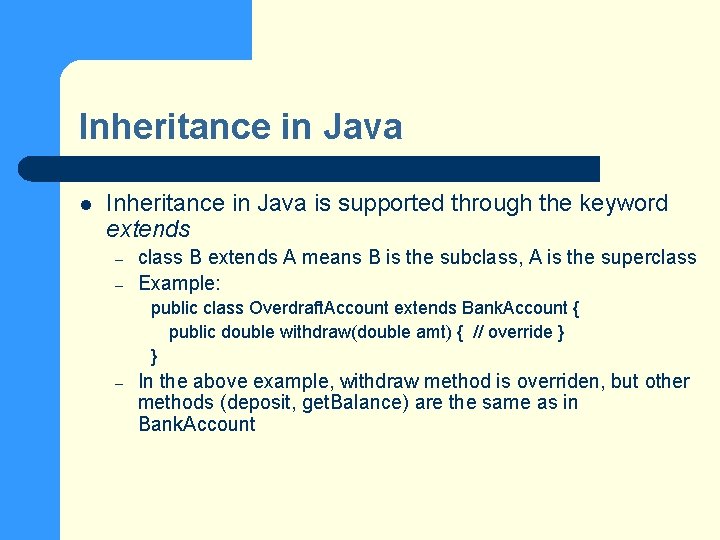
Inheritance in Java l Inheritance in Java is supported through the keyword extends – – class B extends A means B is the subclass, A is the superclass Example: public class Overdraft. Account extends Bank. Account { public double withdraw(double amt) { // override } } – In the above example, withdraw method is overriden, but other methods (deposit, get. Balance) are the same as in Bank. Account
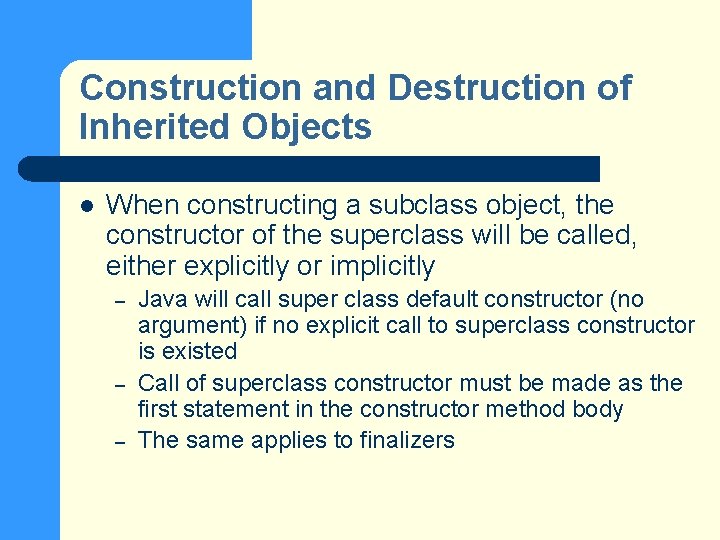
Construction and Destruction of Inherited Objects l When constructing a subclass object, the constructor of the superclass will be called, either explicitly or implicitly – – – Java will call super class default constructor (no argument) if no explicit call to superclass constructor is existed Call of superclass constructor must be made as the first statement in the constructor method body The same applies to finalizers
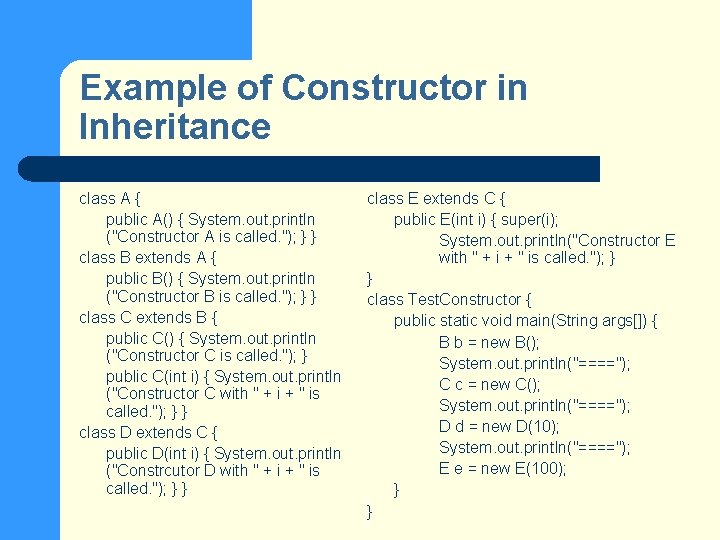
Example of Constructor in Inheritance class A { public A() { System. out. println ("Constructor A is called. "); } } class B extends A { public B() { System. out. println ("Constructor B is called. "); } } class C extends B { public C() { System. out. println ("Constructor C is called. "); } public C(int i) { System. out. println ("Constructor C with " + i + " is called. "); } } class D extends C { public D(int i) { System. out. println ("Constrcutor D with " + i + " is called. "); } } class E extends C { public E(int i) { super(i); System. out. println("Constructor E with " + i + " is called. "); } } class Test. Constructor { public static void main(String args[]) { B b = new B(); System. out. println("===="); C c = new C(); System. out. println("===="); D d = new D(10); System. out. println("===="); E e = new E(100); } }
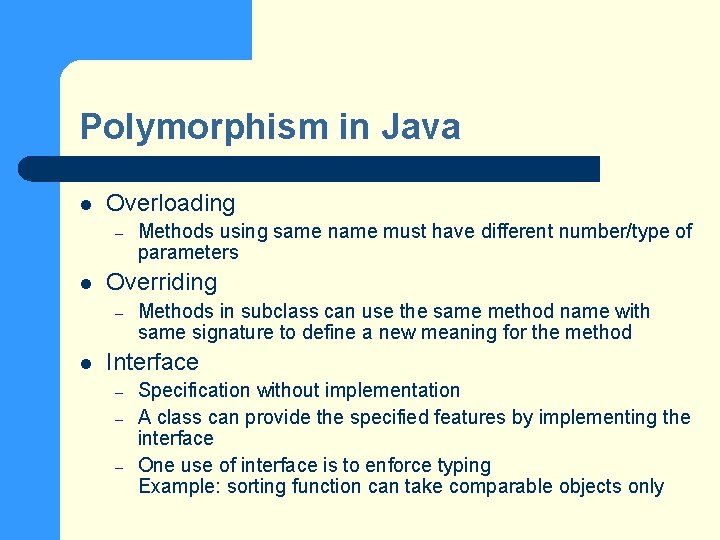
Polymorphism in Java l Overloading – l Overriding – l Methods using same name must have different number/type of parameters Methods in subclass can use the same method name with same signature to define a new meaning for the method Interface – – – Specification without implementation A class can provide the specified features by implementing the interface One use of interface is to enforce typing Example: sorting function can take comparable objects only
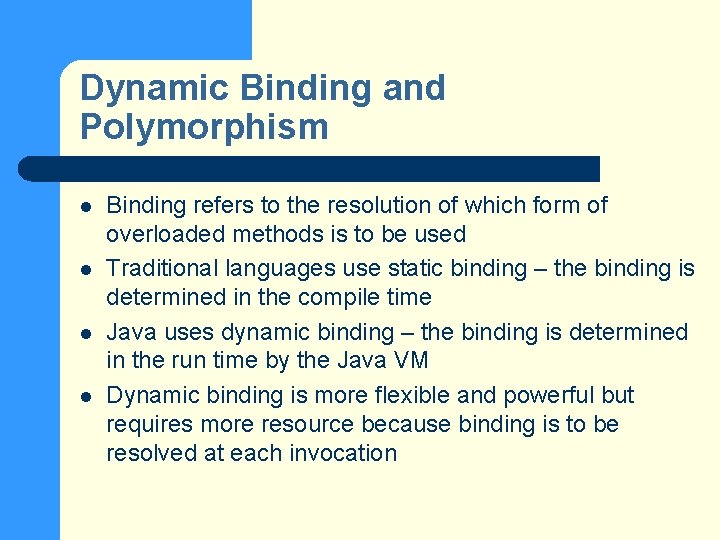
Dynamic Binding and Polymorphism l l Binding refers to the resolution of which form of overloaded methods is to be used Traditional languages use static binding – the binding is determined in the compile time Java uses dynamic binding – the binding is determined in the run time by the Java VM Dynamic binding is more flexible and powerful but requires more resource because binding is to be resolved at each invocation
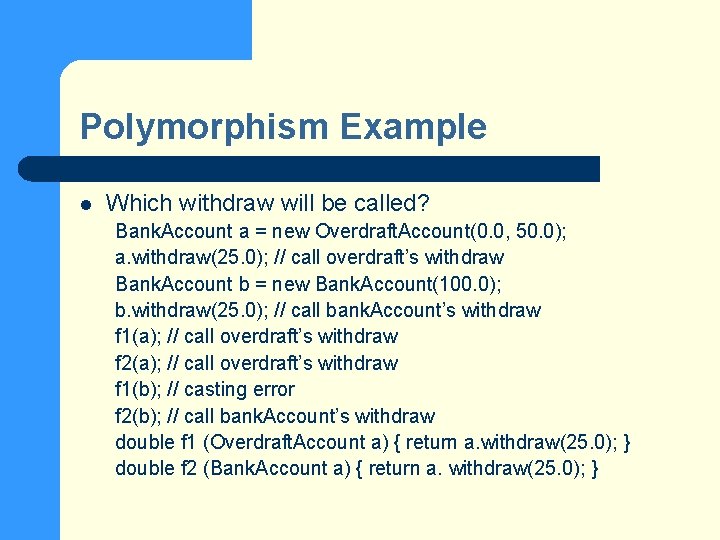
Polymorphism Example l Which withdraw will be called? Bank. Account a = new Overdraft. Account(0. 0, 50. 0); a. withdraw(25. 0); // call overdraft’s withdraw Bank. Account b = new Bank. Account(100. 0); b. withdraw(25. 0); // call bank. Account’s withdraw f 1(a); // call overdraft’s withdraw f 2(a); // call overdraft’s withdraw f 1(b); // casting error f 2(b); // call bank. Account’s withdraw double f 1 (Overdraft. Account a) { return a. withdraw(25. 0); } double f 2 (Bank. Account a) { return a. withdraw(25. 0); }
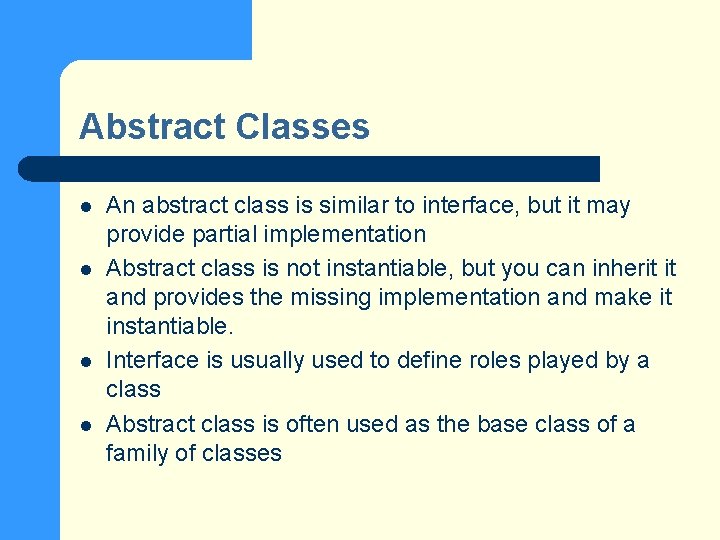
Abstract Classes l l An abstract class is similar to interface, but it may provide partial implementation Abstract class is not instantiable, but you can inherit it and provides the missing implementation and make it instantiable. Interface is usually used to define roles played by a class Abstract class is often used as the base class of a family of classes
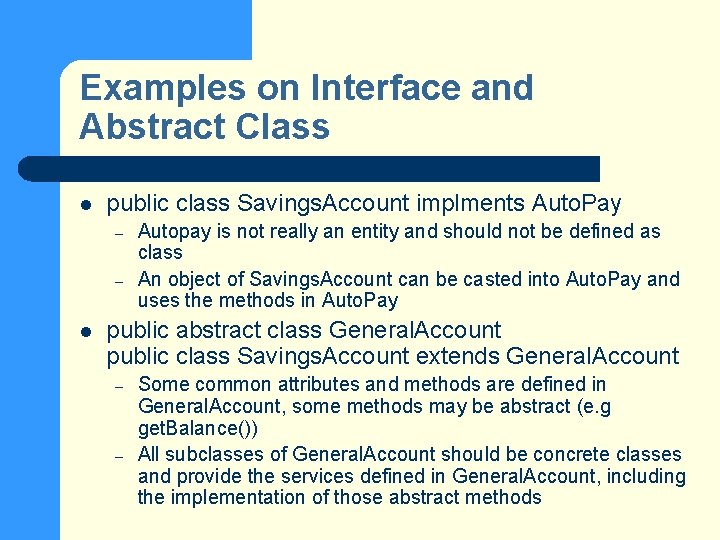
Examples on Interface and Abstract Class l public class Savings. Account implments Auto. Pay – – l Autopay is not really an entity and should not be defined as class An object of Savings. Account can be casted into Auto. Pay and uses the methods in Auto. Pay public abstract class General. Account public class Savings. Account extends General. Account – – Some common attributes and methods are defined in General. Account, some methods may be abstract (e. g get. Balance()) All subclasses of General. Account should be concrete classes and provide the services defined in General. Account, including the implementation of those abstract methods
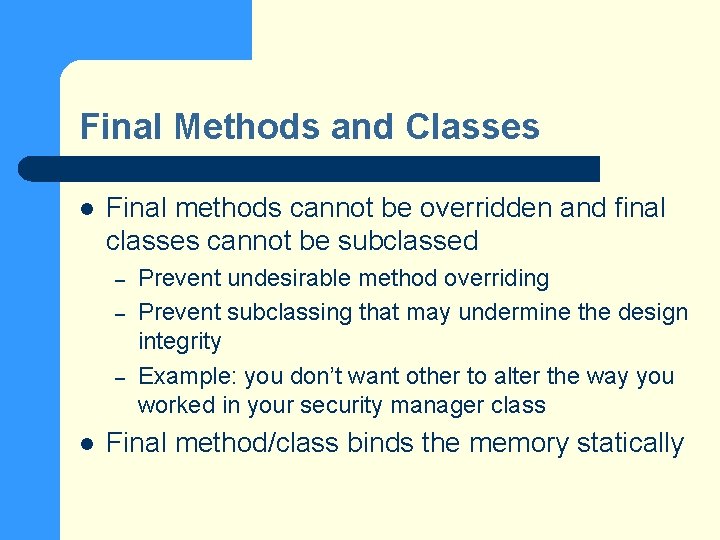
Final Methods and Classes l Final methods cannot be overridden and final classes cannot be subclassed – – – l Prevent undesirable method overriding Prevent subclassing that may undermine the design integrity Example: you don’t want other to alter the way you worked in your security manager class Final method/class binds the memory statically
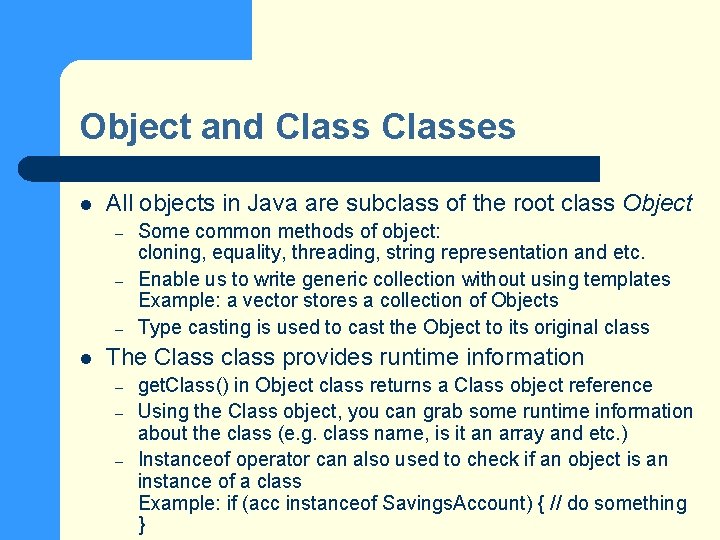
Object and Classes l All objects in Java are subclass of the root class Object – – – l Some common methods of object: cloning, equality, threading, string representation and etc. Enable us to write generic collection without using templates Example: a vector stores a collection of Objects Type casting is used to cast the Object to its original class The Class class provides runtime information – – – get. Class() in Object class returns a Class object reference Using the Class object, you can grab some runtime information about the class (e. g. class name, is it an array and etc. ) Instanceof operator can also used to check if an object is an instance of a class Example: if (acc instanceof Savings. Account) { // do something }
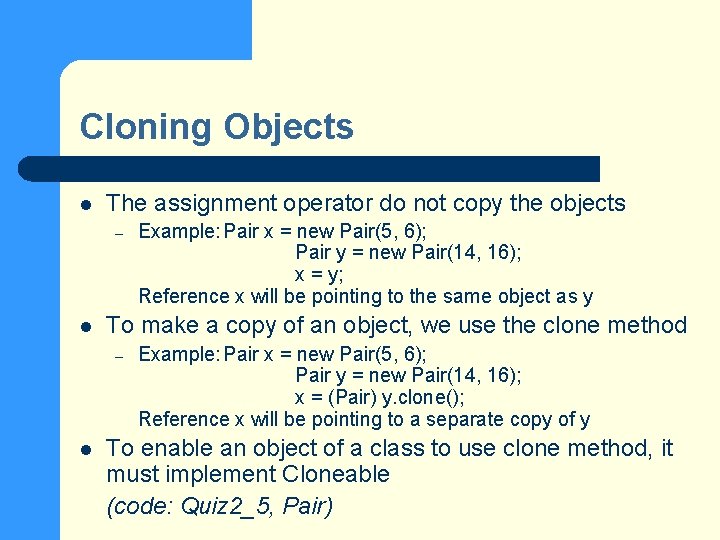
Cloning Objects l The assignment operator do not copy the objects – l To make a copy of an object, we use the clone method – l Example: Pair x = new Pair(5, 6); Pair y = new Pair(14, 16); x = y; Reference x will be pointing to the same object as y Example: Pair x = new Pair(5, 6); Pair y = new Pair(14, 16); x = (Pair) y. clone(); Reference x will be pointing to a separate copy of y To enable an object of a class to use clone method, it must implement Cloneable (code: Quiz 2_5, Pair)
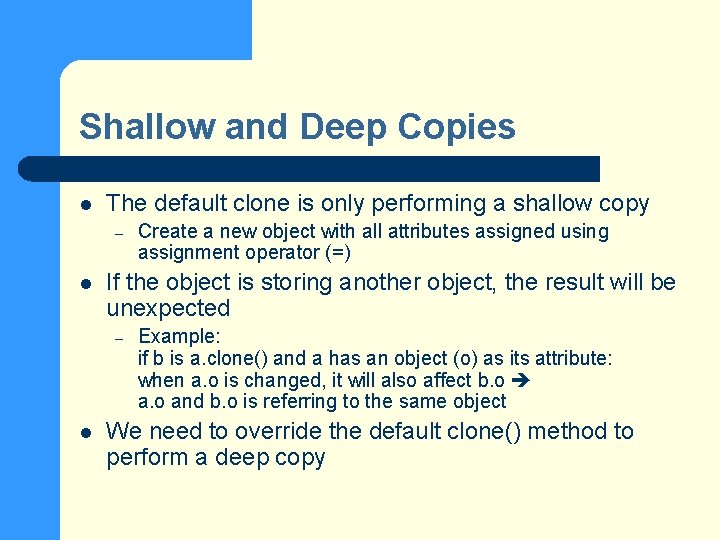
Shallow and Deep Copies l The default clone is only performing a shallow copy – l If the object is storing another object, the result will be unexpected – l Create a new object with all attributes assigned using assignment operator (=) Example: if b is a. clone() and a has an object (o) as its attribute: when a. o is changed, it will also affect b. o a. o and b. o is referring to the same object We need to override the default clone() method to perform a deep copy
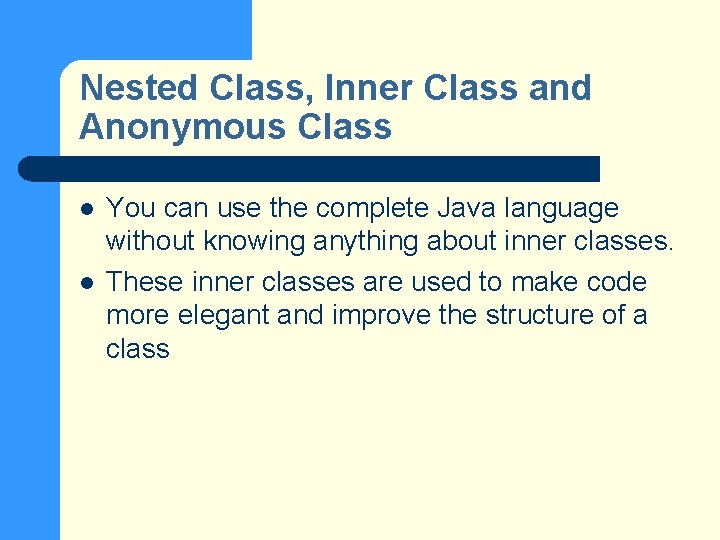
Nested Class, Inner Class and Anonymous Class l l You can use the complete Java language without knowing anything about inner classes. These inner classes are used to make code more elegant and improve the structure of a class
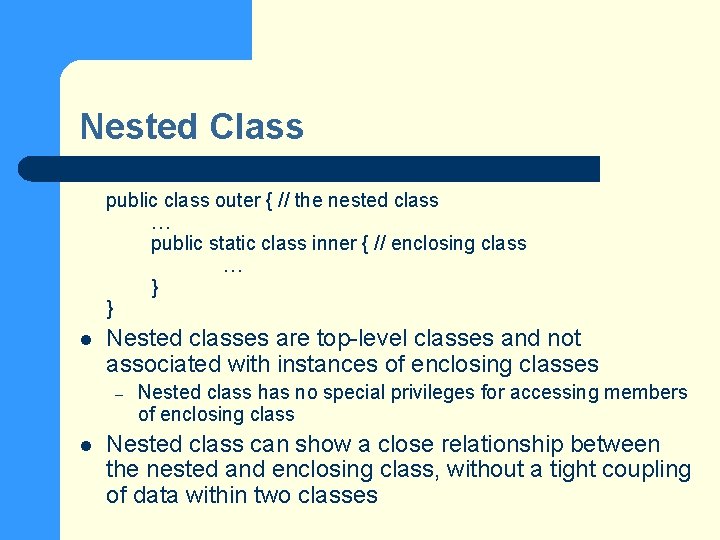
Nested Class public class outer { // the nested class … public static class inner { // enclosing class … } } l Nested classes are top-level classes and not associated with instances of enclosing classes – l Nested class has no special privileges for accessing members of enclosing class Nested class can show a close relationship between the nested and enclosing class, without a tight coupling of data within two classes
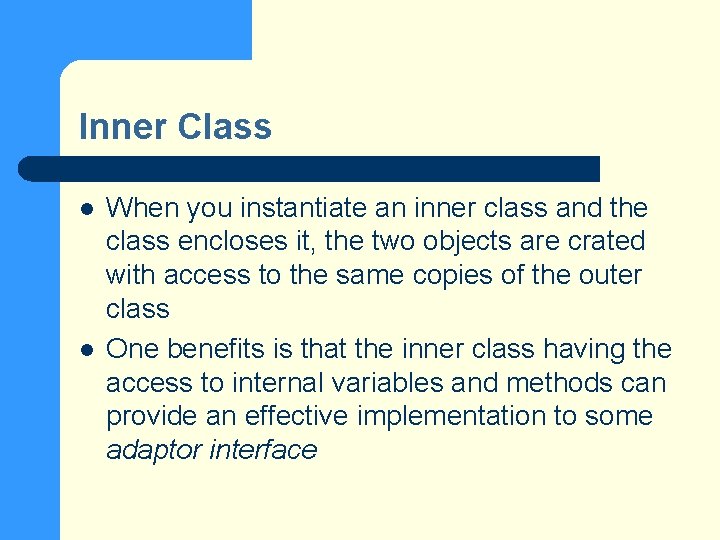
Inner Class l l When you instantiate an inner class and the class encloses it, the two objects are crated with access to the same copies of the outer class One benefits is that the inner class having the access to internal variables and methods can provide an effective implementation to some adaptor interface
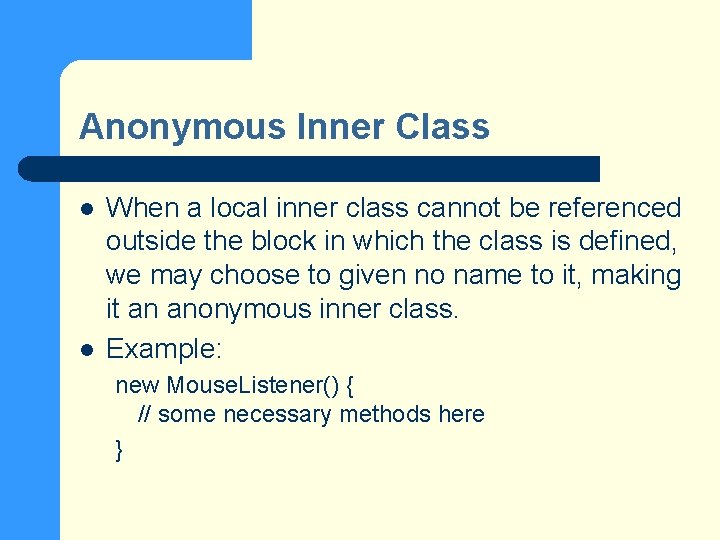
Anonymous Inner Class l l When a local inner class cannot be referenced outside the block in which the class is defined, we may choose to given no name to it, making it an anonymous inner class. Example: new Mouse. Listener() { // some necessary methods here }
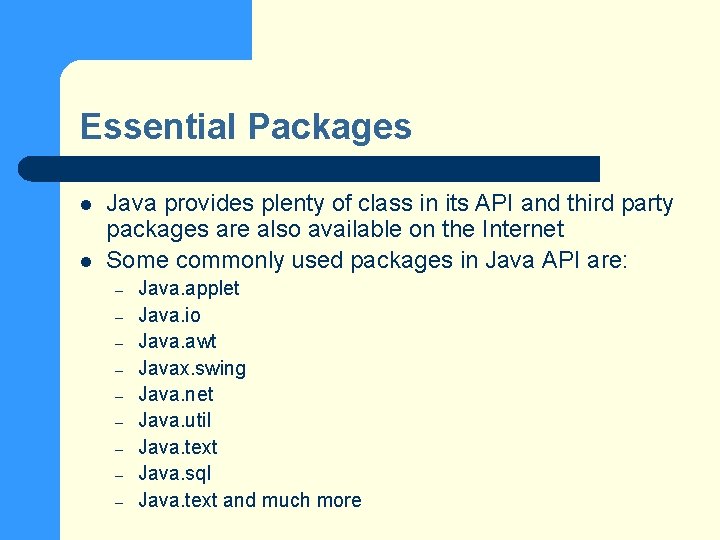
Essential Packages l l Java provides plenty of class in its API and third party packages are also available on the Internet Some commonly used packages in Java API are: – – – – – Java. applet Java. io Java. awt Javax. swing Java. net Java. util Java. text Java. sql Java. text and much more
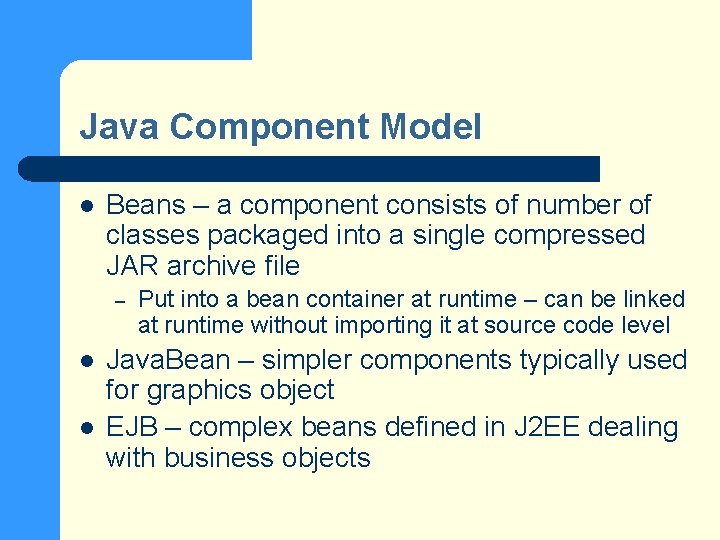
Java Component Model l Beans – a component consists of number of classes packaged into a single compressed JAR archive file – l l Put into a bean container at runtime – can be linked at runtime without importing it at source code level Java. Bean – simpler components typically used for graphics object EJB – complex beans defined in J 2 EE dealing with business objects