More on Programs and Processes Jeff Chase Duke
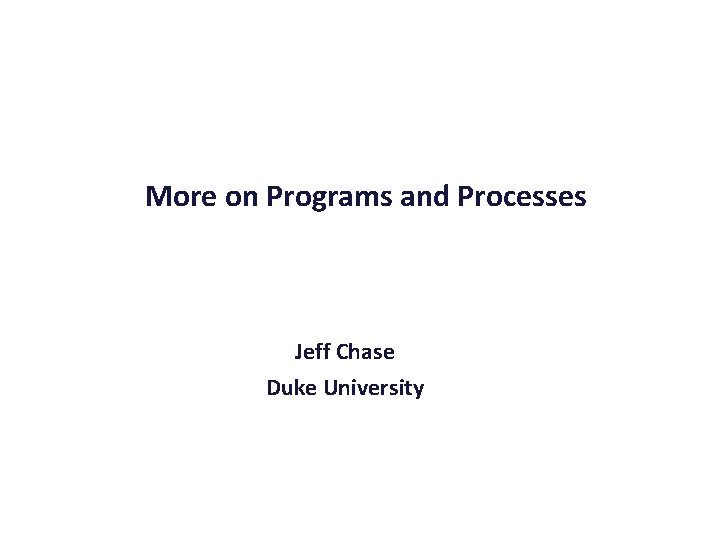
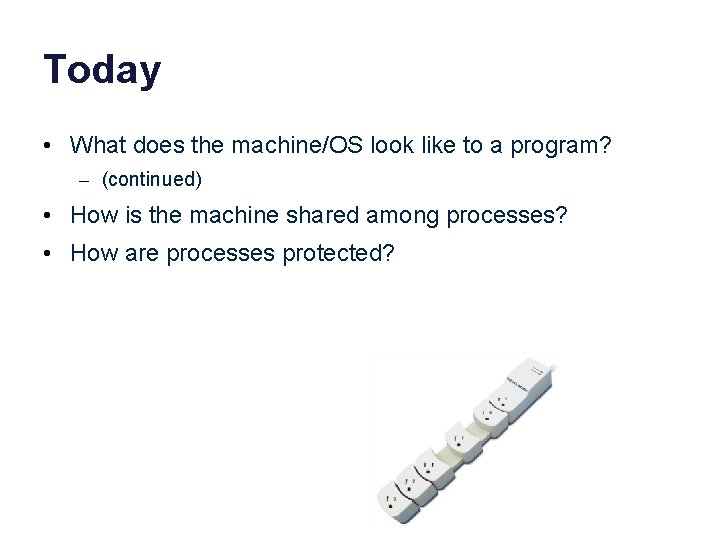
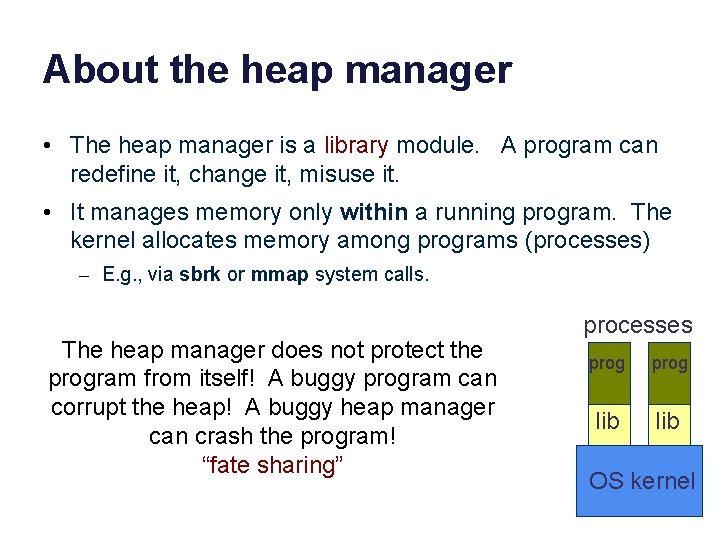
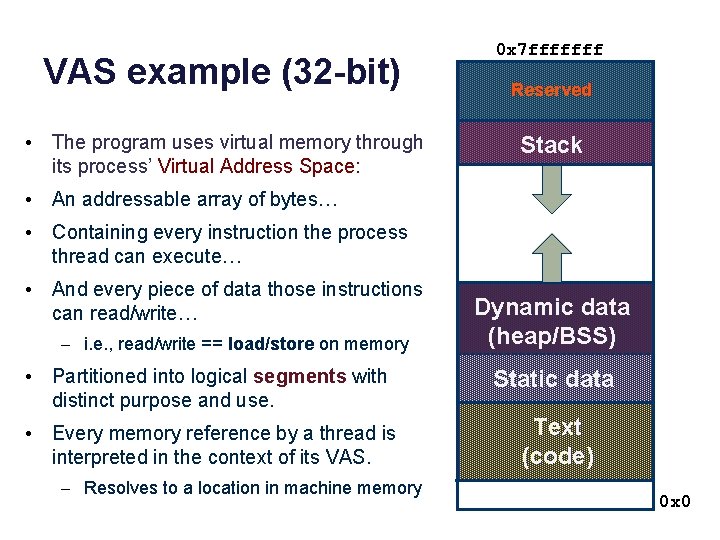
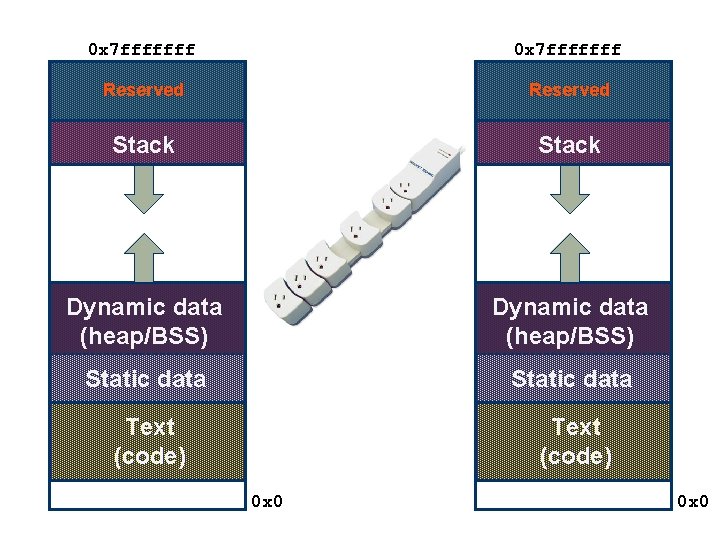
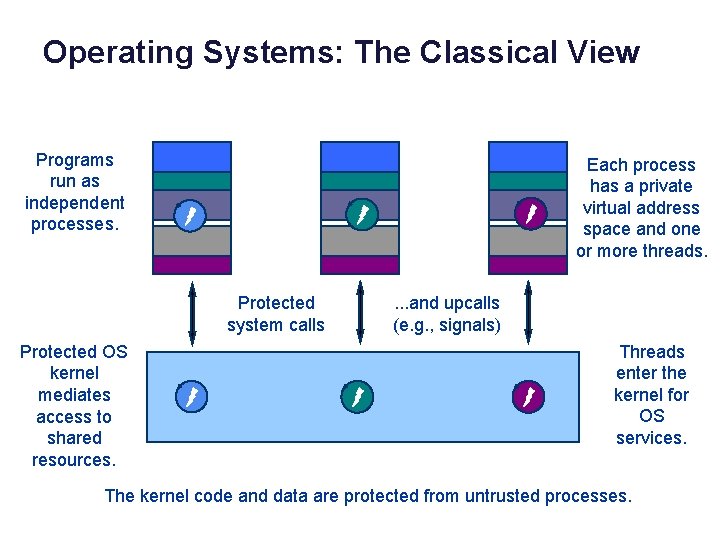
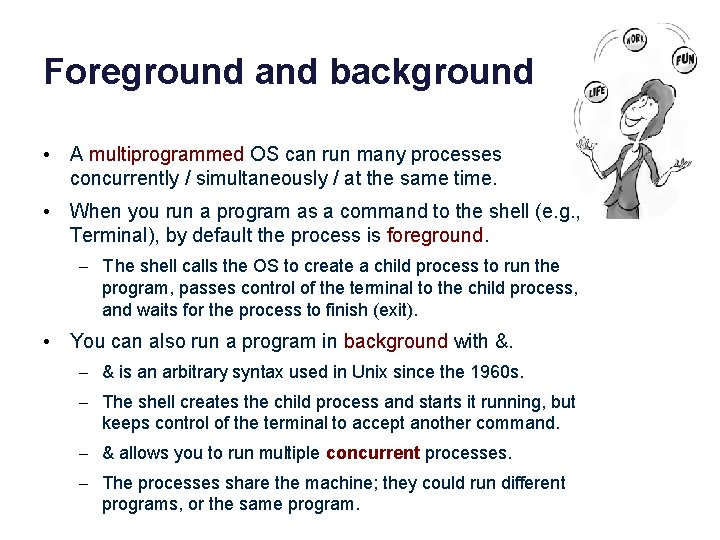
![cpu. c (OSTEP) int main(int argc, char *argv[]) { if (argc != 2) { cpu. c (OSTEP) int main(int argc, char *argv[]) { if (argc != 2) {](https://slidetodoc.com/presentation_image_h2/95d3c582669b46e5a353726b787b7c84/image-8.jpg)
![mem. c (OSTEP) data p int main(int argc, char *argv[]) { int *p; p mem. c (OSTEP) data p int main(int argc, char *argv[]) { int *p; p](https://slidetodoc.com/presentation_image_h2/95d3c582669b46e5a353726b787b7c84/image-9.jpg)
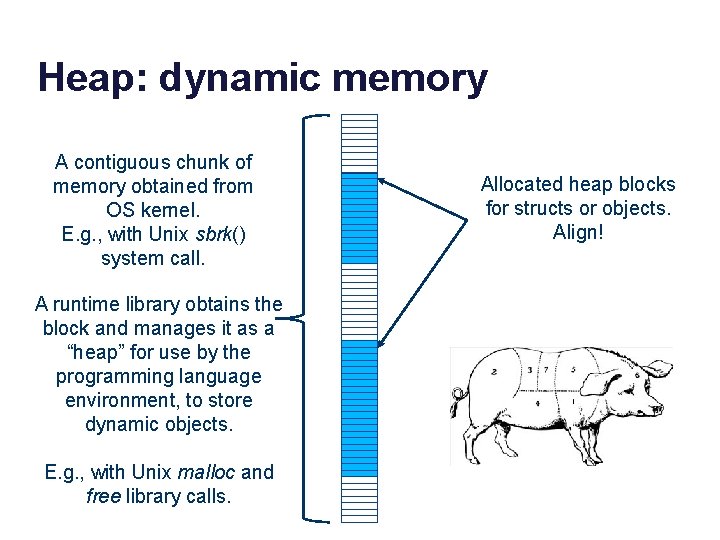
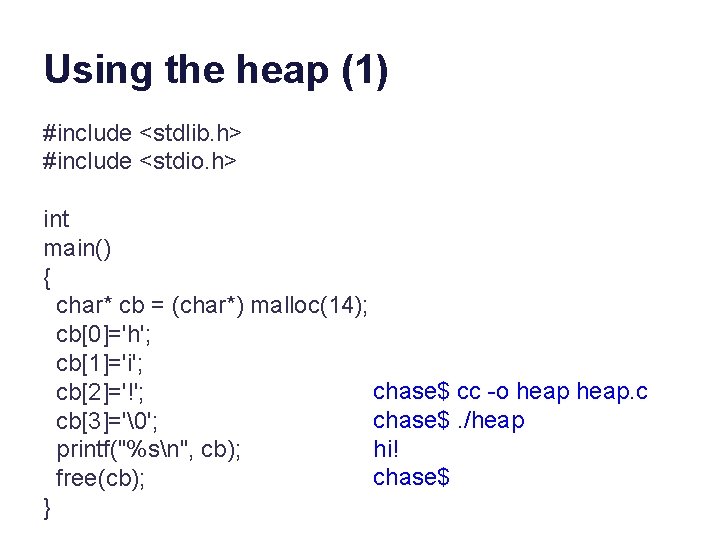
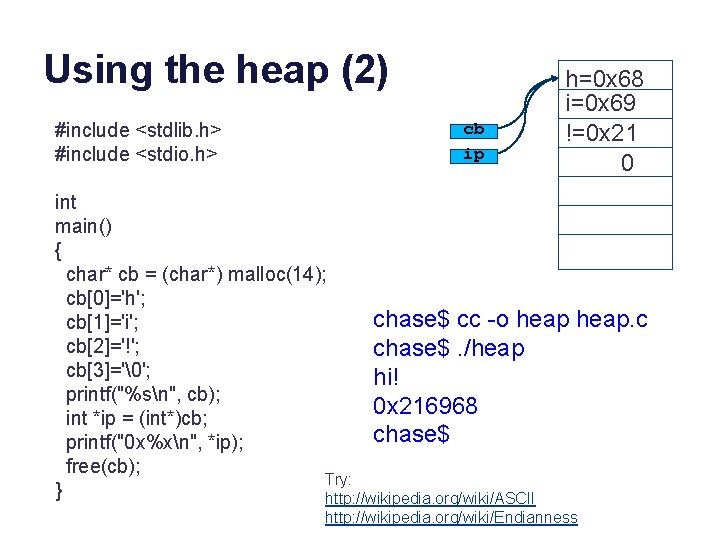
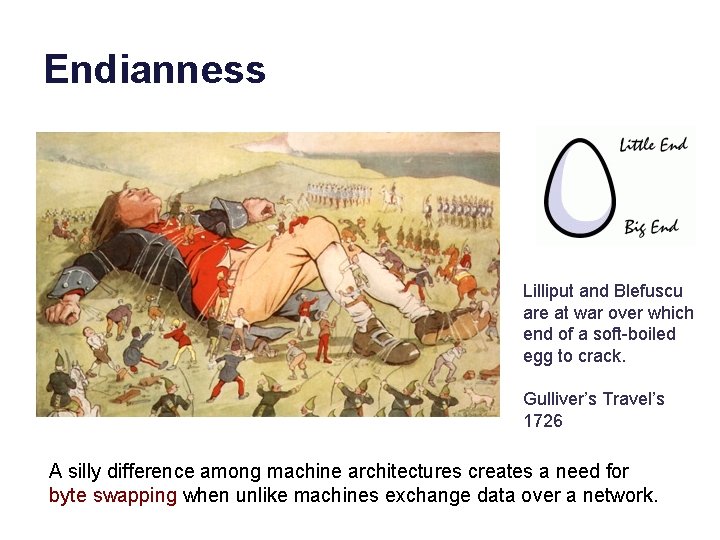
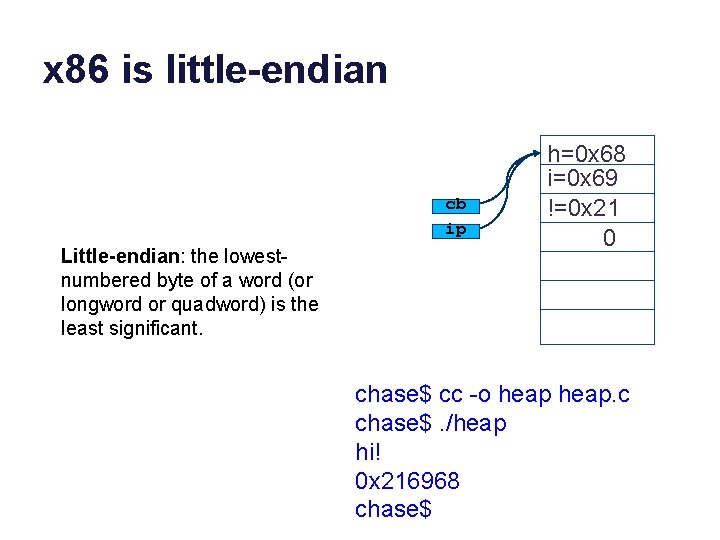
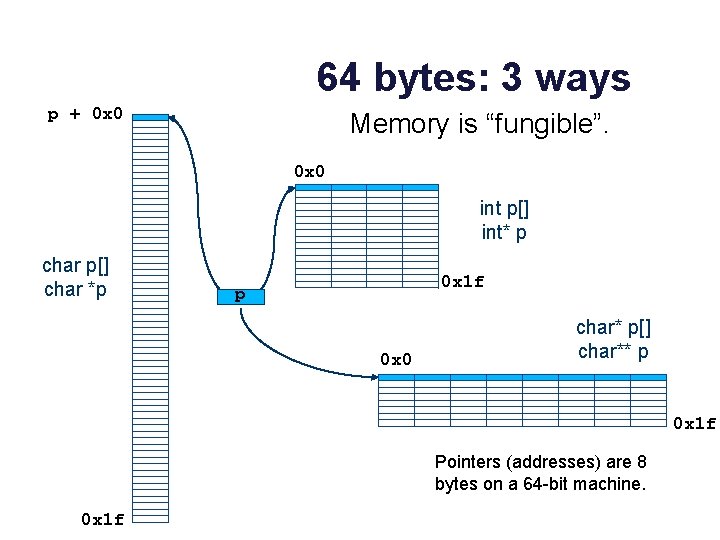
![Alignment p + 0 x 0 X char p[] char *p int p[] int* Alignment p + 0 x 0 X char p[] char *p int p[] int*](https://slidetodoc.com/presentation_image_h2/95d3c582669b46e5a353726b787b7c84/image-16.jpg)
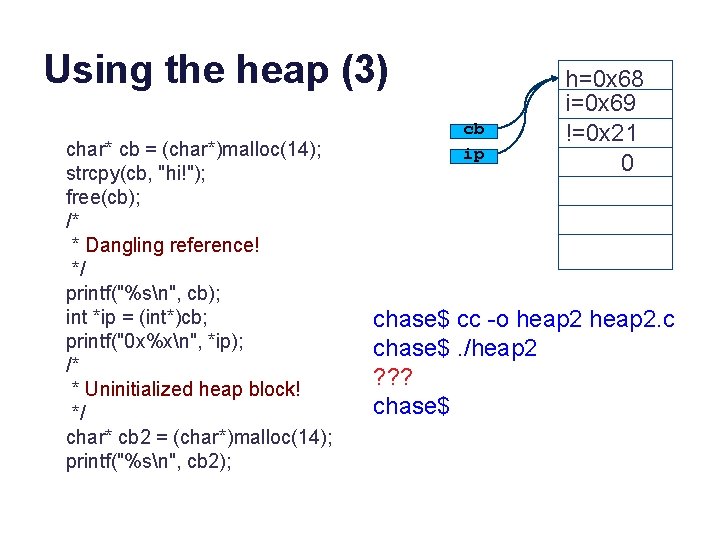
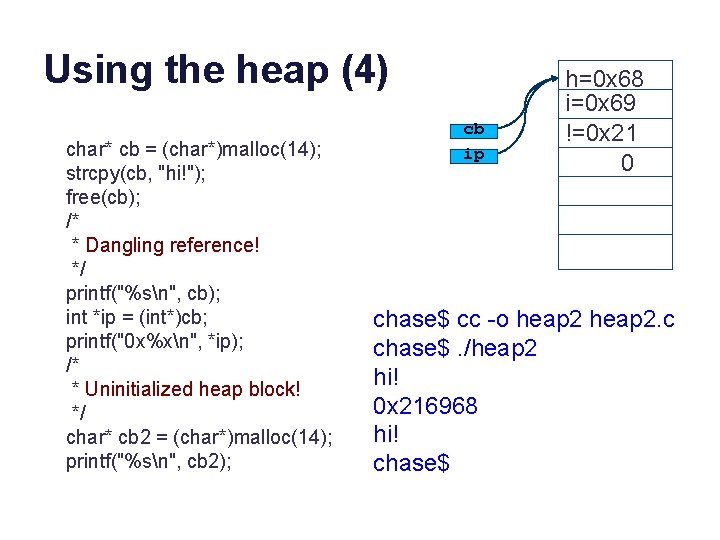
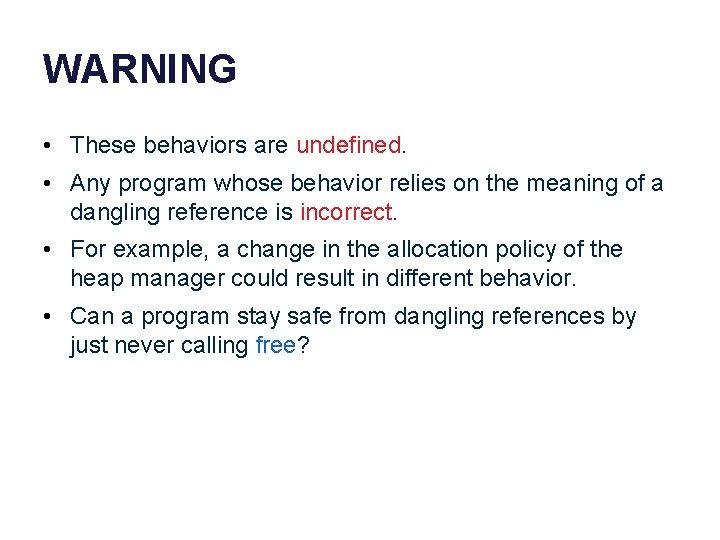
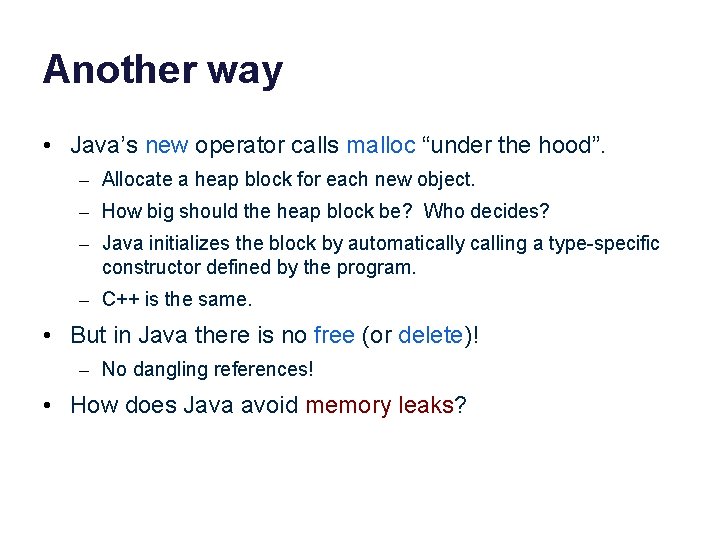
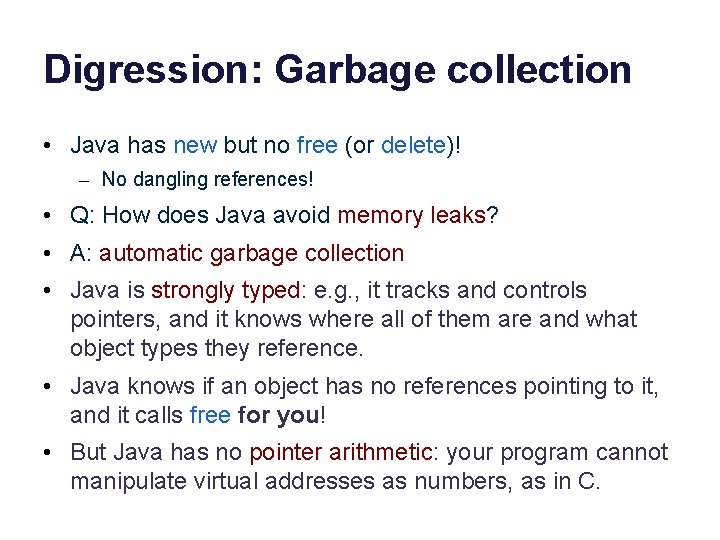
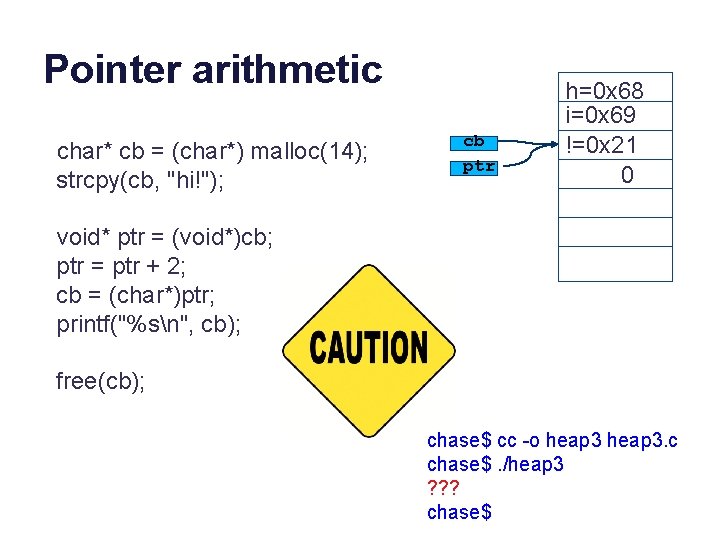
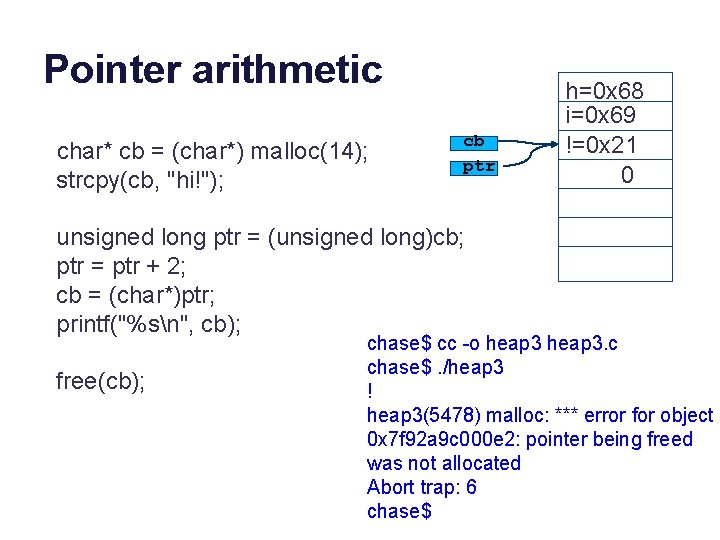
![Simple I/O: args and printf #include <stdio. h> int main(int argc, char* argv[]) { Simple I/O: args and printf #include <stdio. h> int main(int argc, char* argv[]) {](https://slidetodoc.com/presentation_image_h2/95d3c582669b46e5a353726b787b7c84/image-24.jpg)
![Environment variables #include <stdio. h> #include <stdlib. h> int main(int argc, char* argv[], char* Environment variables #include <stdio. h> #include <stdlib. h> int main(int argc, char* argv[], char*](https://slidetodoc.com/presentation_image_h2/95d3c582669b46e5a353726b787b7c84/image-25.jpg)
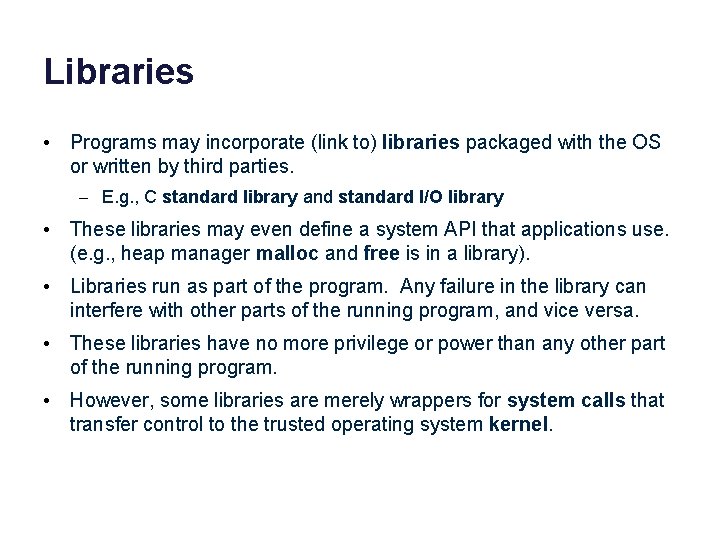
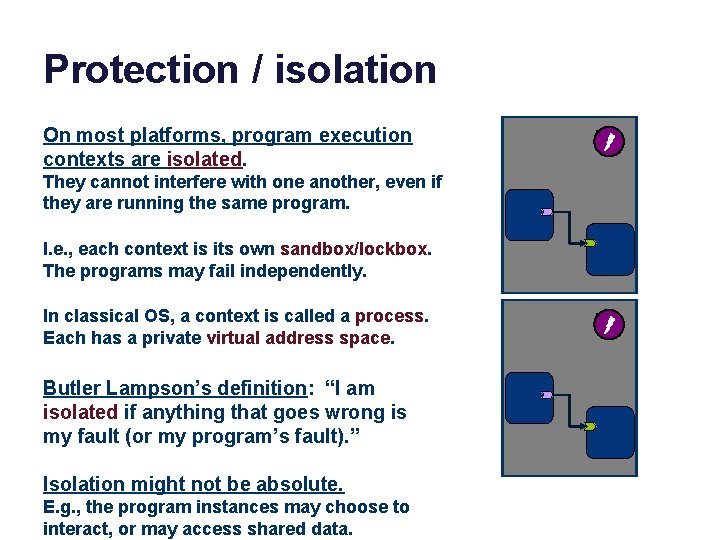
- Slides: 27
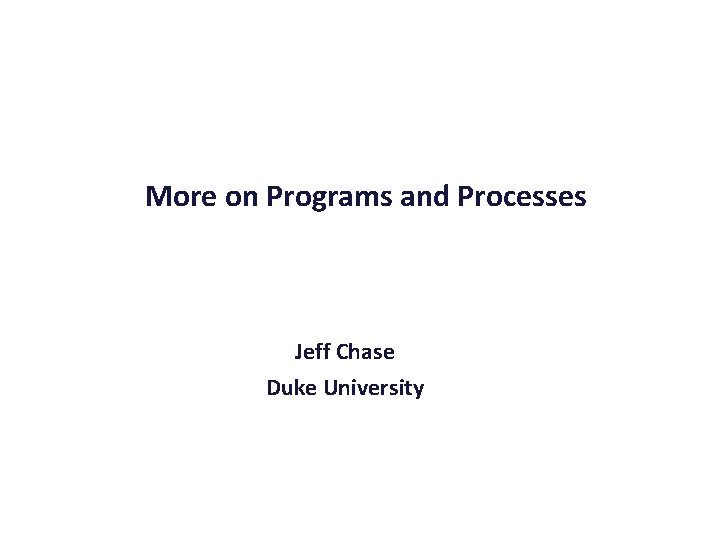
More on Programs and Processes Jeff Chase Duke University
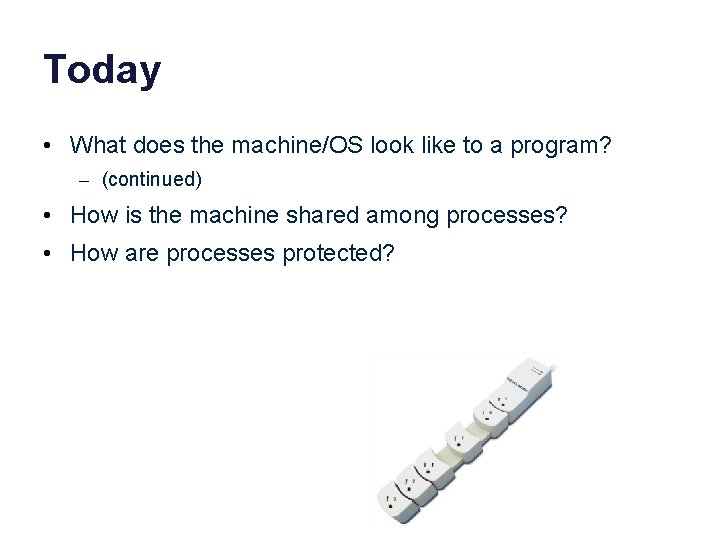
Today • What does the machine/OS look like to a program? – (continued) • How is the machine shared among processes? • How are processes protected?
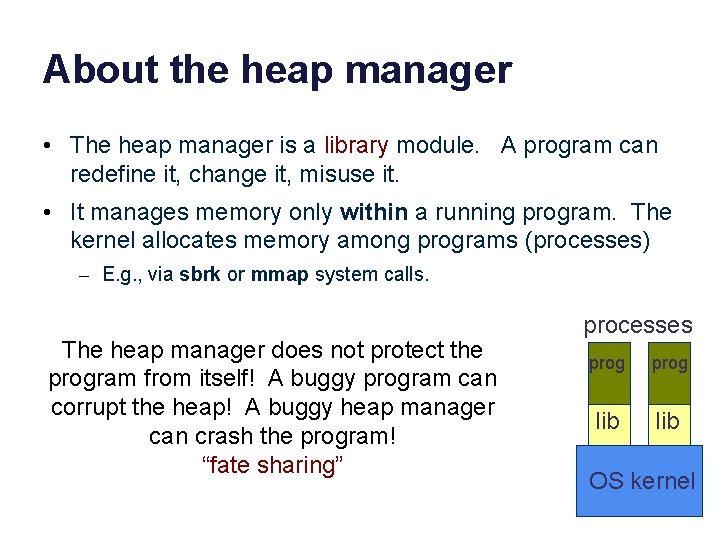
About the heap manager • The heap manager is a library module. A program can redefine it, change it, misuse it. • It manages memory only within a running program. The kernel allocates memory among programs (processes) – E. g. , via sbrk or mmap system calls. The heap manager does not protect the program from itself! A buggy program can corrupt the heap! A buggy heap manager can crash the program! “fate sharing” processes prog lib OS kernel
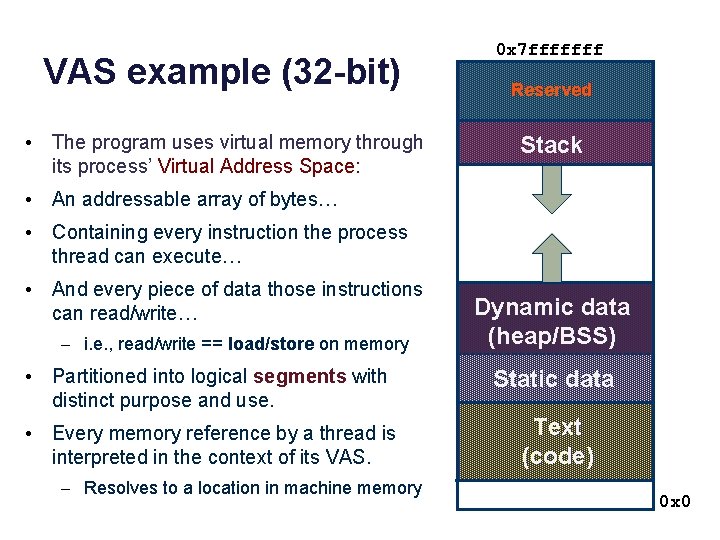
VAS example (32 -bit) • The program uses virtual memory through its process’ Virtual Address Space: 0 x 7 fffffff Reserved Stack • An addressable array of bytes… • Containing every instruction the process thread can execute… • And every piece of data those instructions can read/write… – i. e. , read/write == load/store on memory Dynamic data (heap/BSS) • Partitioned into logical segments with distinct purpose and use. Static data • Every memory reference by a thread is interpreted in the context of its VAS. Text (code) – Resolves to a location in machine memory 0 x 0
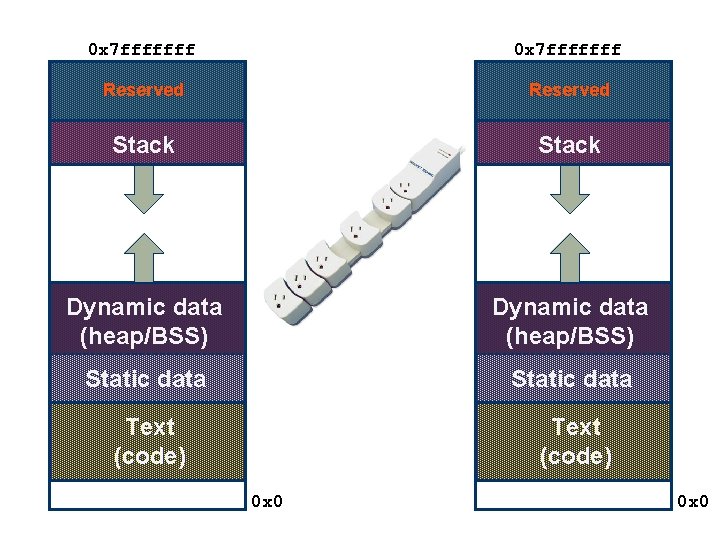
0 x 7 fffffff Reserved Stack Dynamic data (heap/BSS) Static data Text (code) 0 x 0
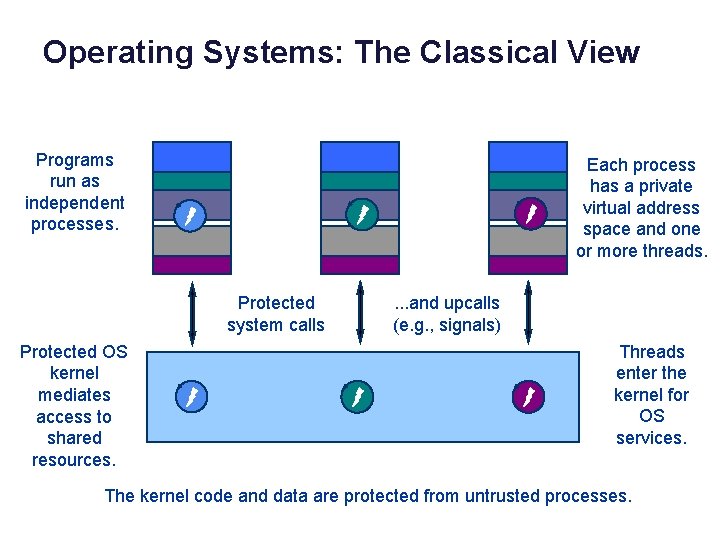
Operating Systems: The Classical View Programs run as independent processes. data Protected system calls Protected OS kernel mediates access to shared resources. Each process has a private virtual address space and one or more threads. . and upcalls (e. g. , signals) Threads enter the kernel for OS services. The kernel code and data are protected from untrusted processes.
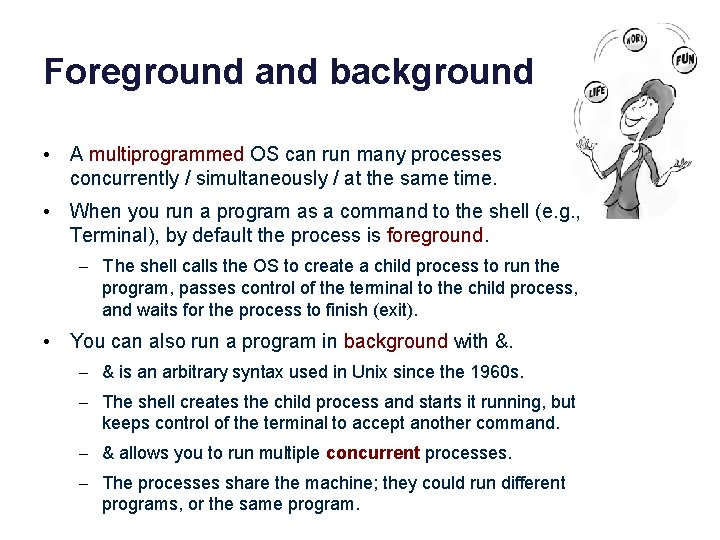
Foreground and background • A multiprogrammed OS can run many processes concurrently / simultaneously / at the same time. • When you run a program as a command to the shell (e. g. , Terminal), by default the process is foreground. – T he shell calls the OS to create a child process to run the program, passes control of the terminal to the child process, and waits for the process to finish (exit). • You can also run a program in background with &. – & is an arbitrary syntax used in Unix since the 1960 s. – The shell creates the child process and starts it running, but keeps control of the terminal to accept another command. – & allows you to run multiple concurrent processes. – The processes share the machine; they could run different programs, or the same program.
![cpu c OSTEP int mainint argc char argv if argc 2 cpu. c (OSTEP) int main(int argc, char *argv[]) { if (argc != 2) {](https://slidetodoc.com/presentation_image_h2/95d3c582669b46e5a353726b787b7c84/image-8.jpg)
cpu. c (OSTEP) int main(int argc, char *argv[]) { if (argc != 2) { fprintf(stderr, "usage: cpu <string>n"); exit(1); } char *str = argv[1]; while (1) { printf("%sn", str); Spin(1); } return 0; } time chase$ cc -o cpu. c chase$. /cpu A & chase$. /cpu B & A A B B B A B …
![mem c OSTEP data p int mainint argc char argv int p p mem. c (OSTEP) data p int main(int argc, char *argv[]) { int *p; p](https://slidetodoc.com/presentation_image_h2/95d3c582669b46e5a353726b787b7c84/image-9.jpg)
mem. c (OSTEP) data p int main(int argc, char *argv[]) { int *p; p = malloc(sizeof(int)); *p = atoi(argv[1]); while (1) { Spin(1); *p = *p + 1; printf("(pid: %d) value of p: %dn”, getpid(), *p); } } pid: 5587 pid: 5588 chase$ cc -o mem. c chase$. /mem 21 & chase$. /mem 42 & (pid: 5587) value of p: 22 (pid: 5587) value of p: 23 (pid: 5587) value of p: 24 (pid: 5588) value of p: 43 (pid: 5588) value of p: 44 (pid: 5587) value of p: 25 (pid: 5587) value of p: 26 …
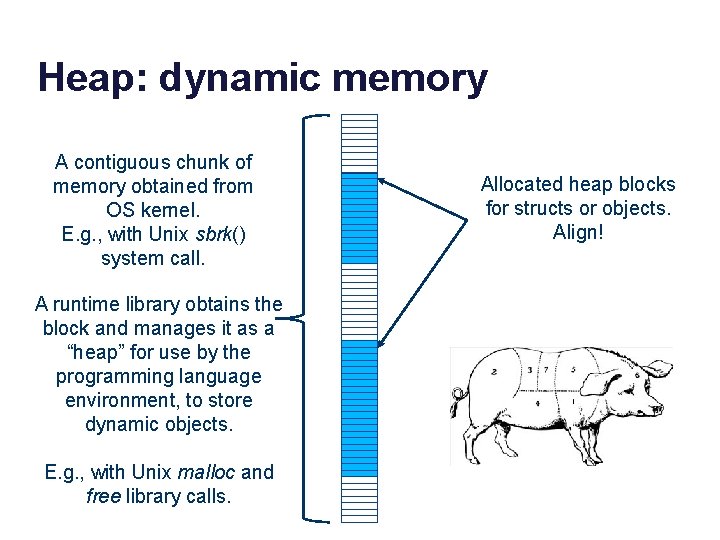
Heap: dynamic memory A contiguous chunk of memory obtained from OS kernel. E. g. , with Unix sbrk() system call. A runtime library obtains the block and manages it as a “heap” for use by the programming language environment, to store dynamic objects. E. g. , with Unix malloc and free library calls. Allocated heap blocks for structs or objects. Align!
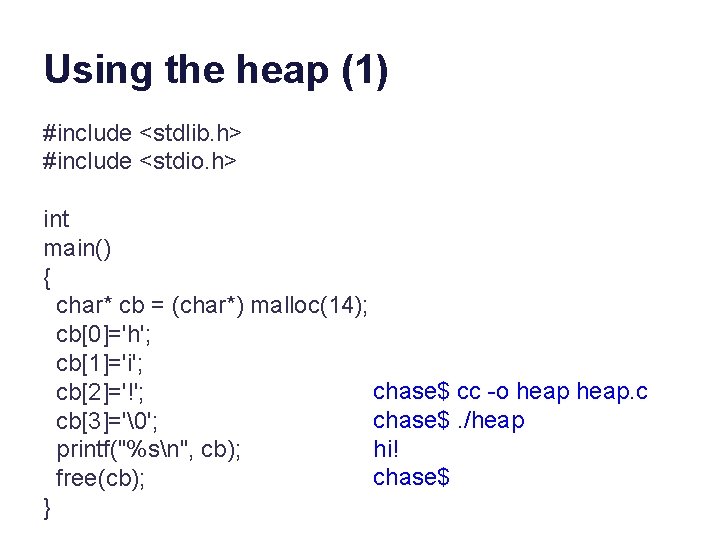
Using the heap (1) #include <stdlib. h> #include <stdio. h> int main() { char* cb = (char*) malloc(14); cb[0]='h'; cb[1]='i'; chase$ cc -o heap. c cb[2]='!'; chase$. /heap cb[3]='