Module 8 Delegates and Events Overview n Delegates
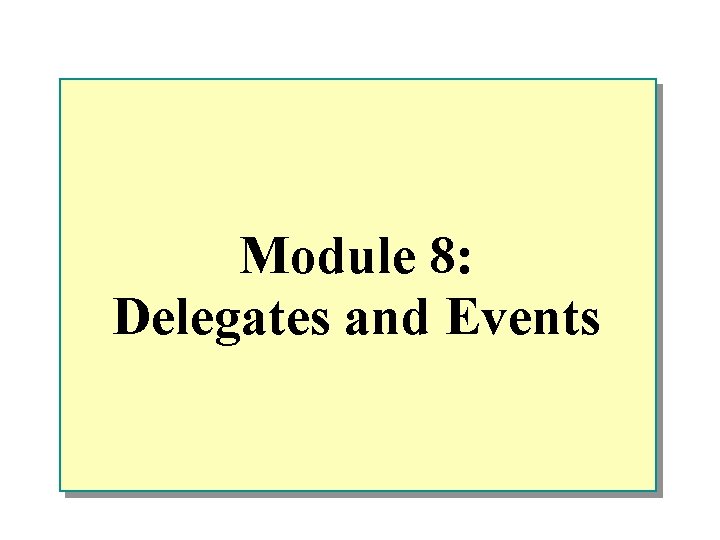
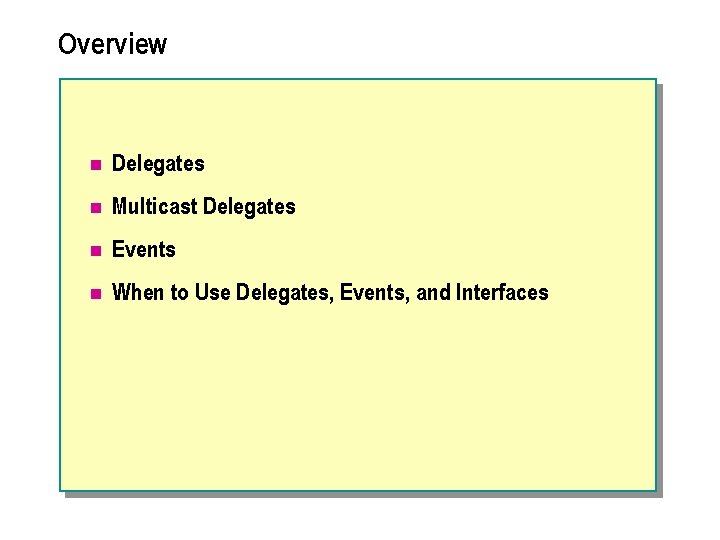
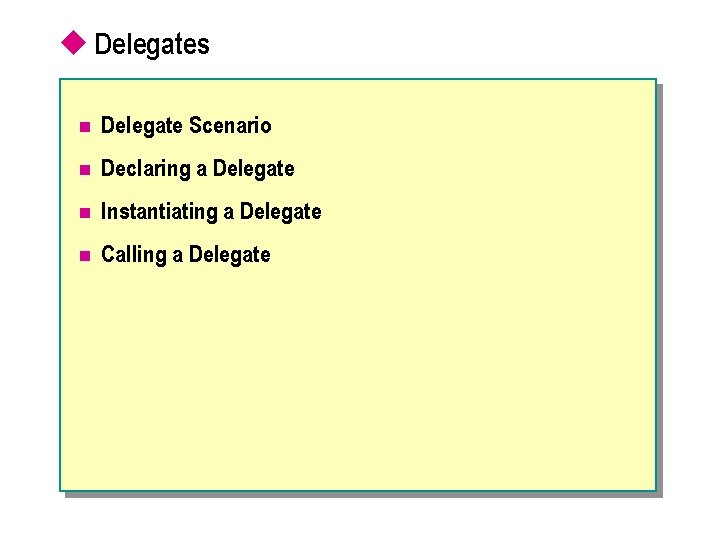
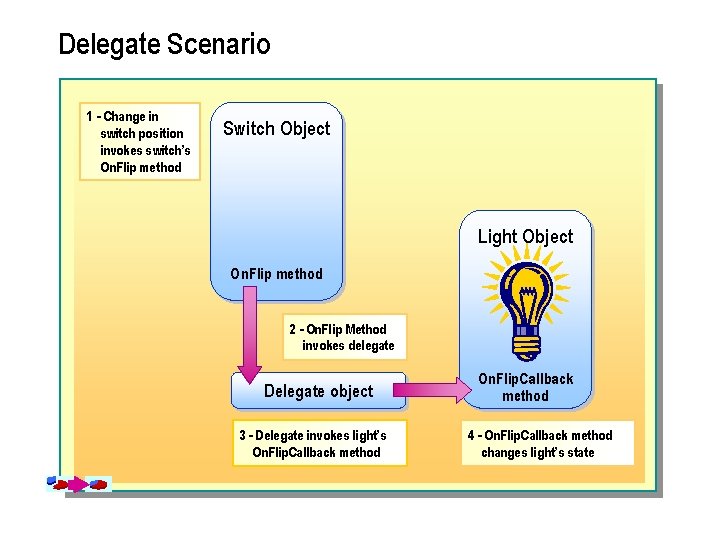
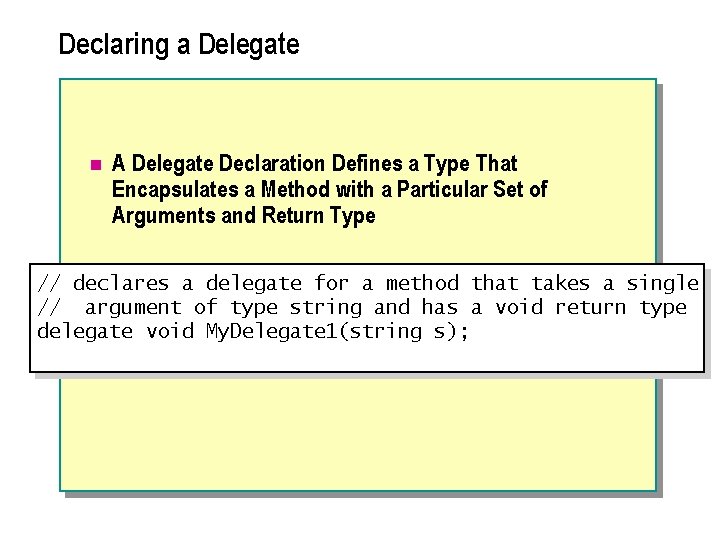
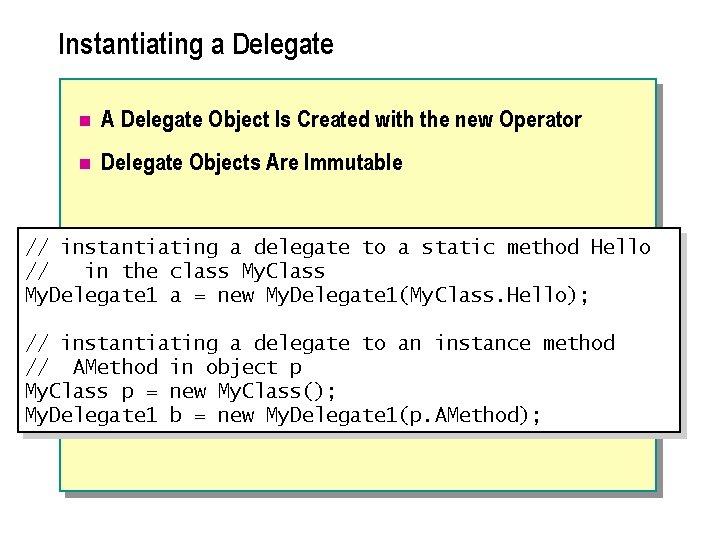
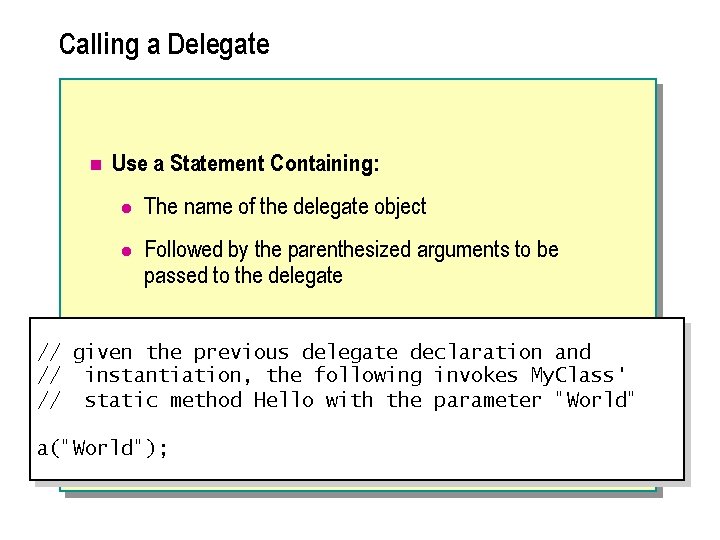
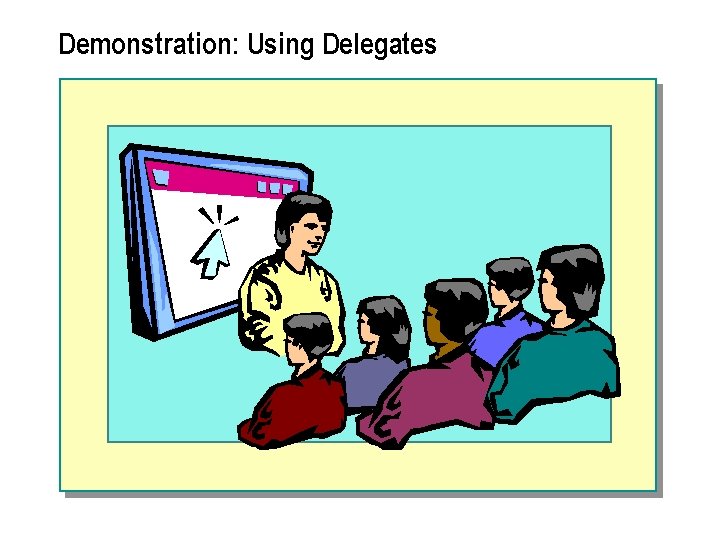
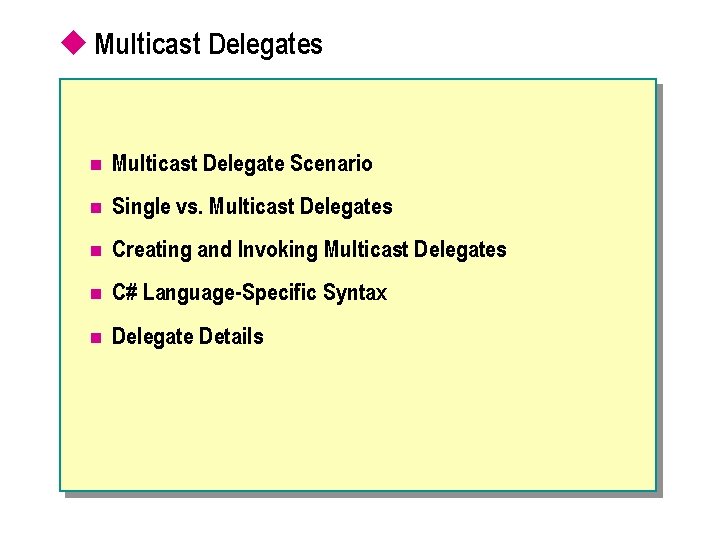
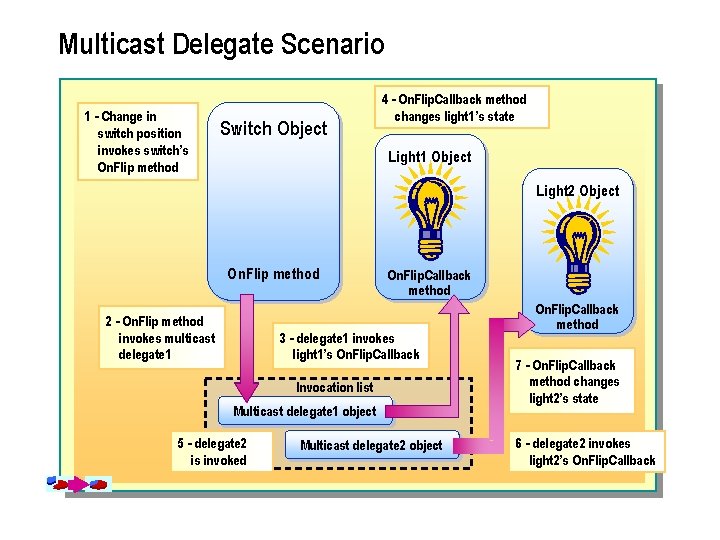
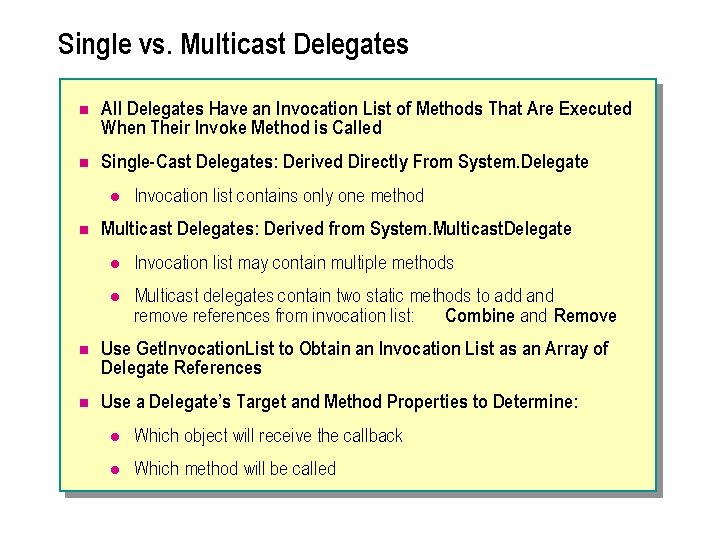
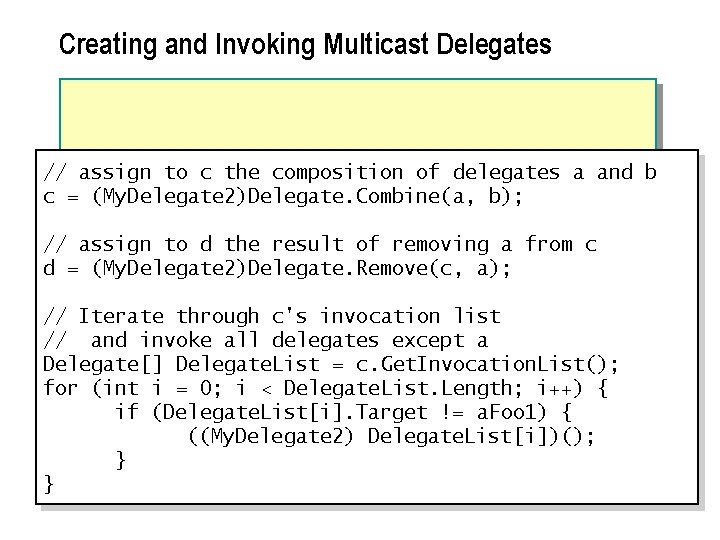
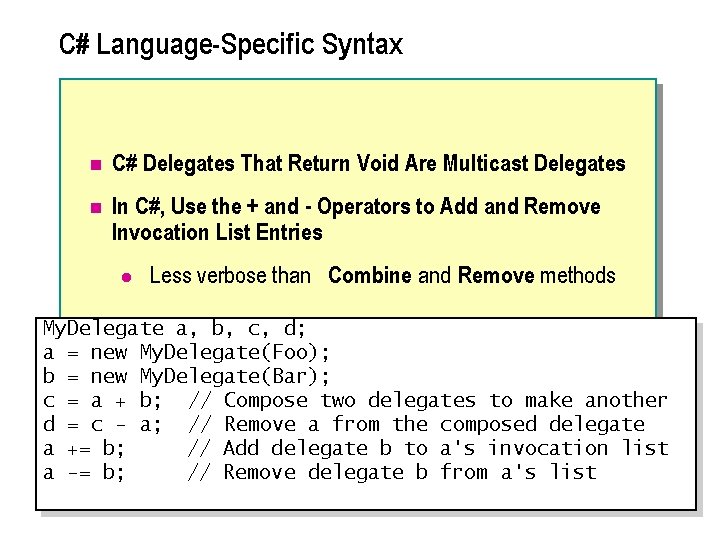
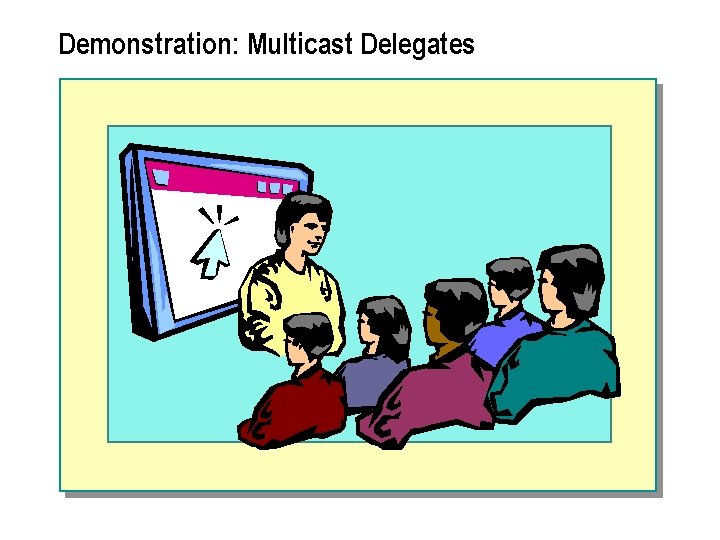
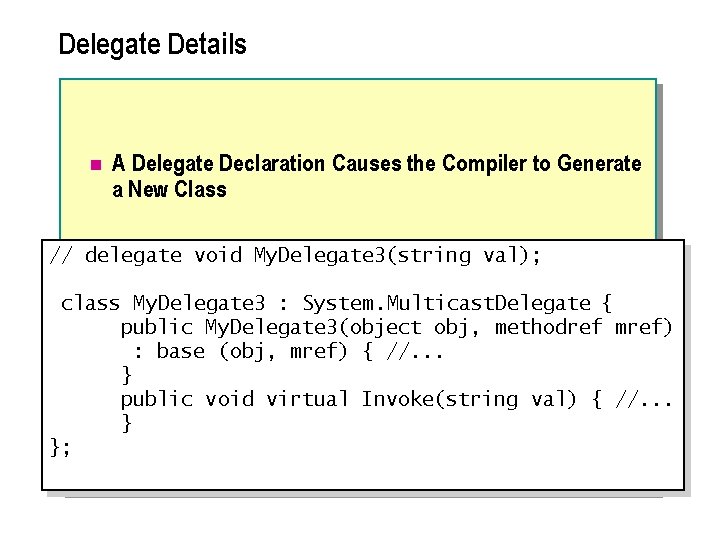
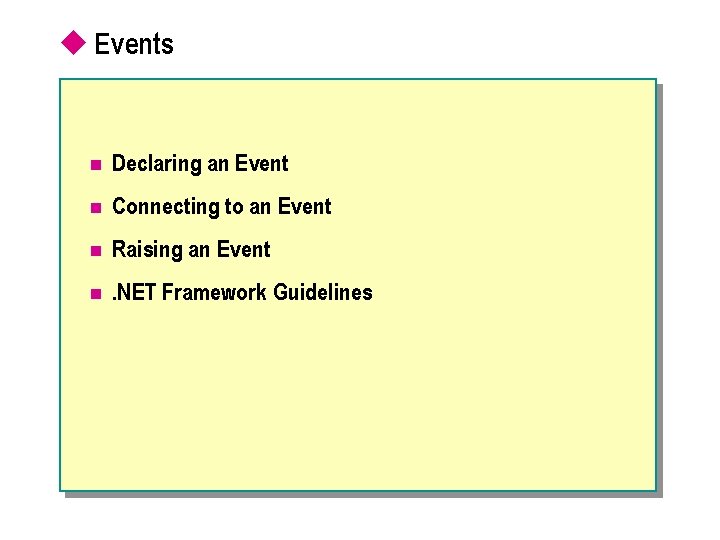
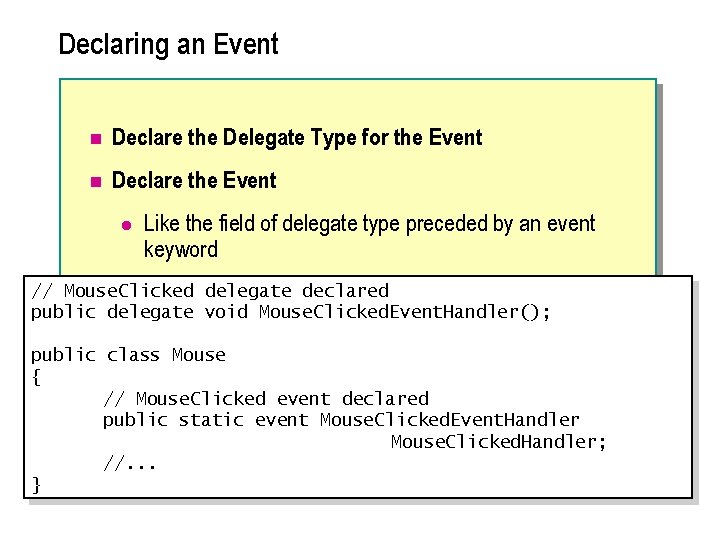
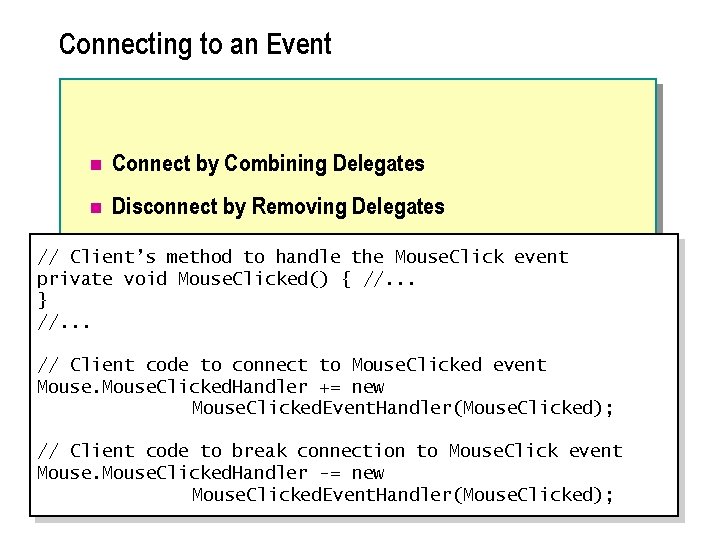
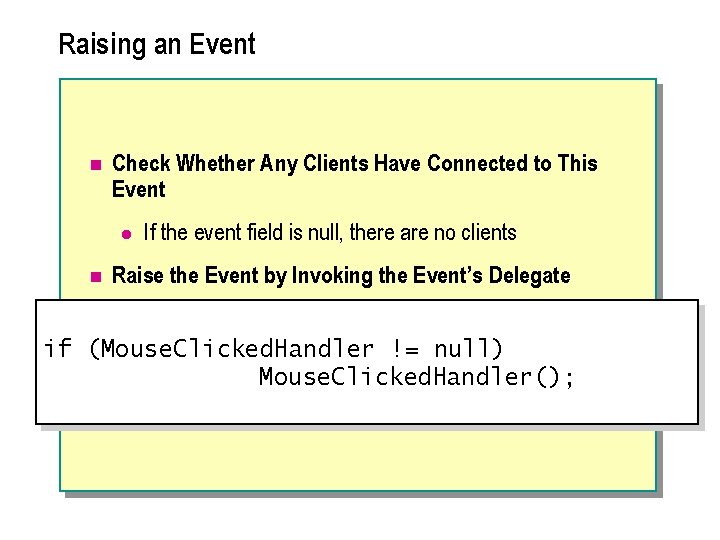
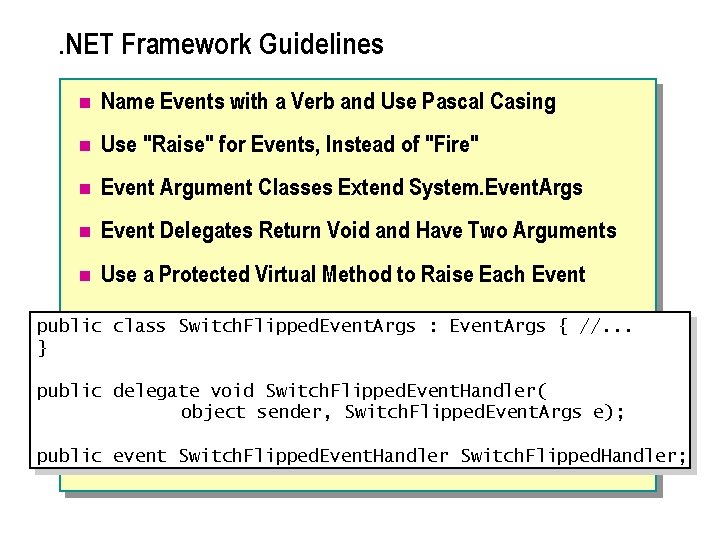
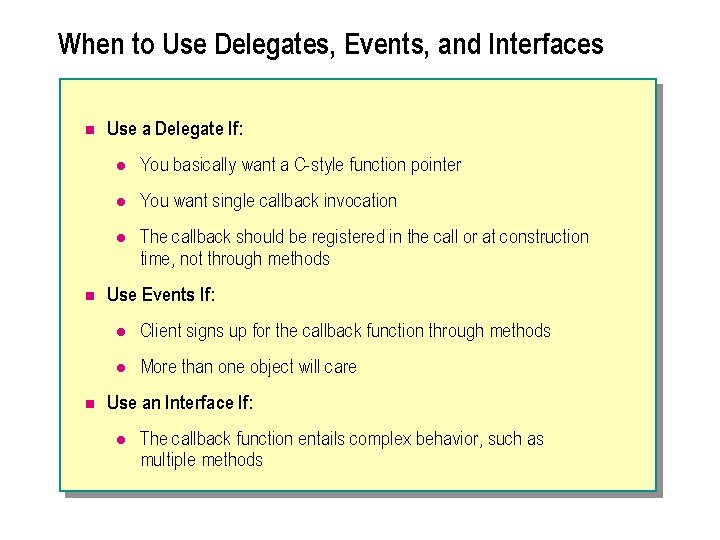
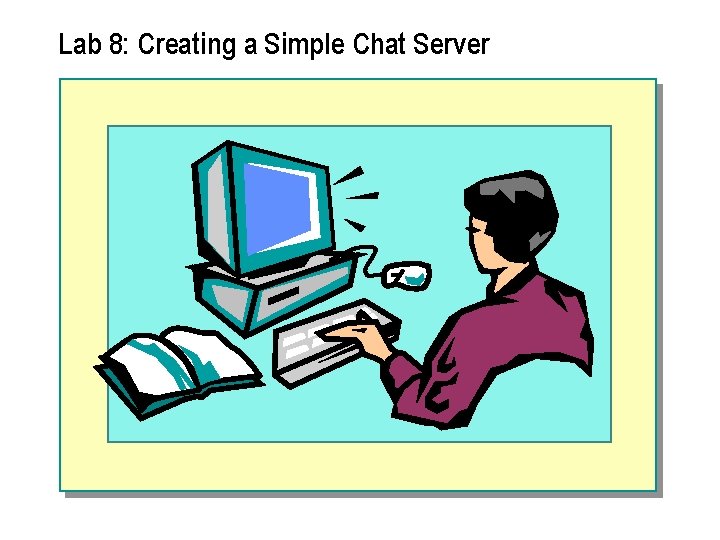
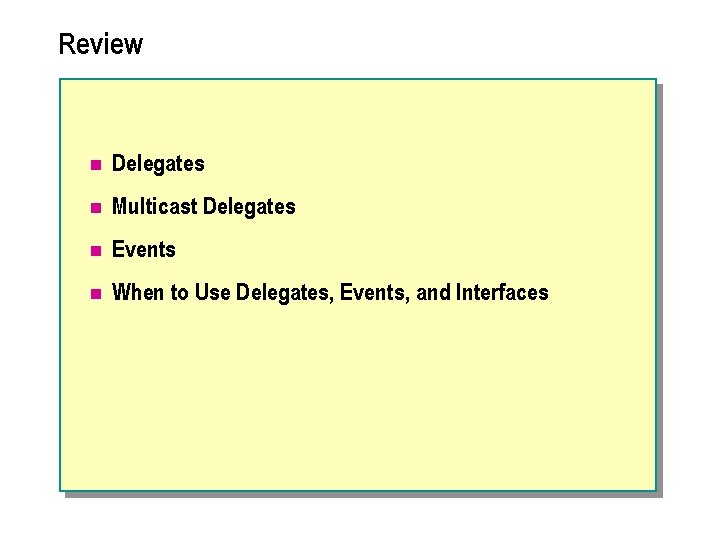
- Slides: 23
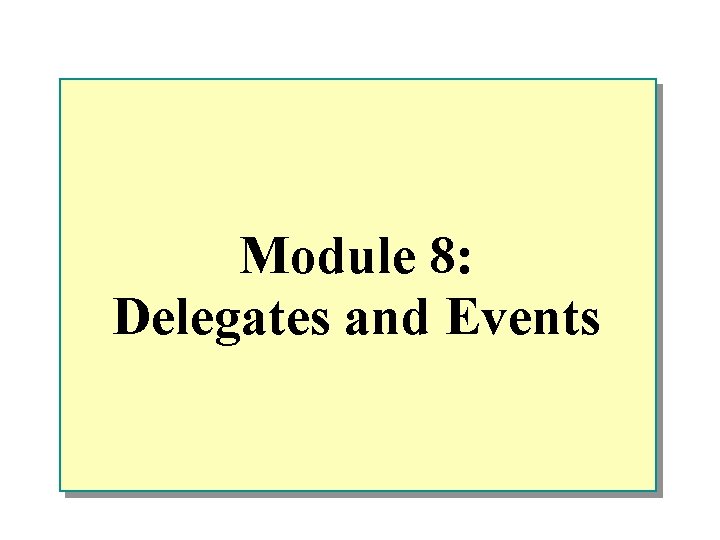
Module 8: Delegates and Events
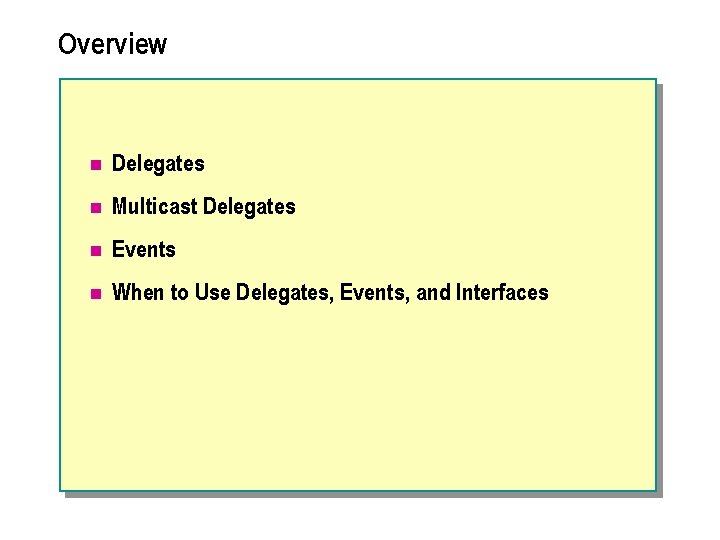
Overview n Delegates n Multicast Delegates n Events n When to Use Delegates, Events, and Interfaces
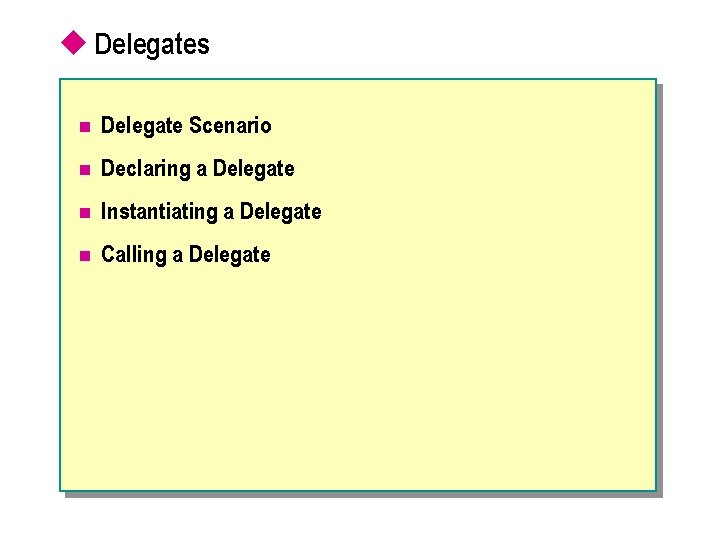
u Delegates n Delegate Scenario n Declaring a Delegate n Instantiating a Delegate n Calling a Delegate
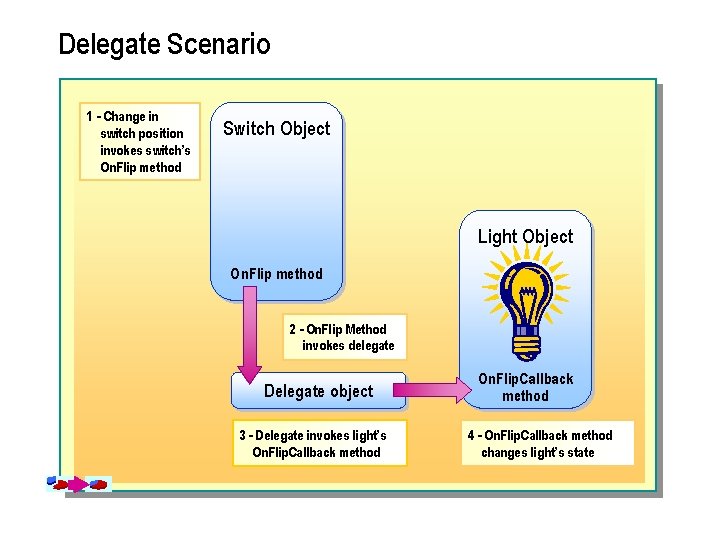
Delegate Scenario 1 - Change in switch position invokes switch’s On. Flip method Switch Object Light Object On. Flip method 2 - On. Flip Method invokes delegate Delegate object 3 - Delegate invokes light’s On. Flip. Callback method 4 - On. Flip. Callback method changes light’s state
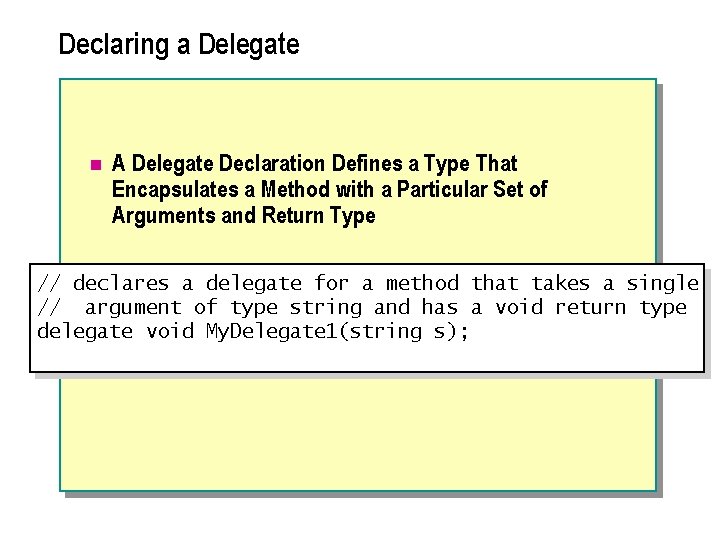
Declaring a Delegate n A Delegate Declaration Defines a Type That Encapsulates a Method with a Particular Set of Arguments and Return Type // declares a delegate for a method that takes a single // argument of type string and has a void return type delegate void My. Delegate 1(string s);
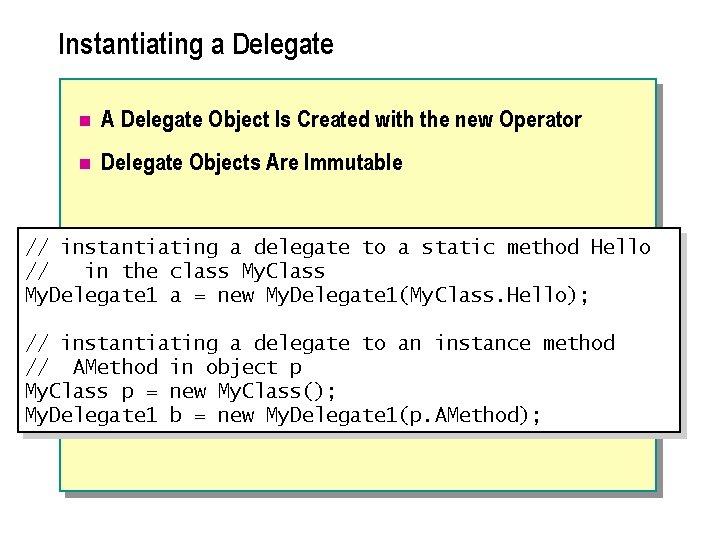
Instantiating a Delegate n A Delegate Object Is Created with the new Operator n Delegate Objects Are Immutable // instantiating a delegate to a static method Hello // in the class My. Class My. Delegate 1 a = new My. Delegate 1(My. Class. Hello); // instantiating a delegate to an instance method // AMethod in object p My. Class p = new My. Class(); My. Delegate 1 b = new My. Delegate 1(p. AMethod);
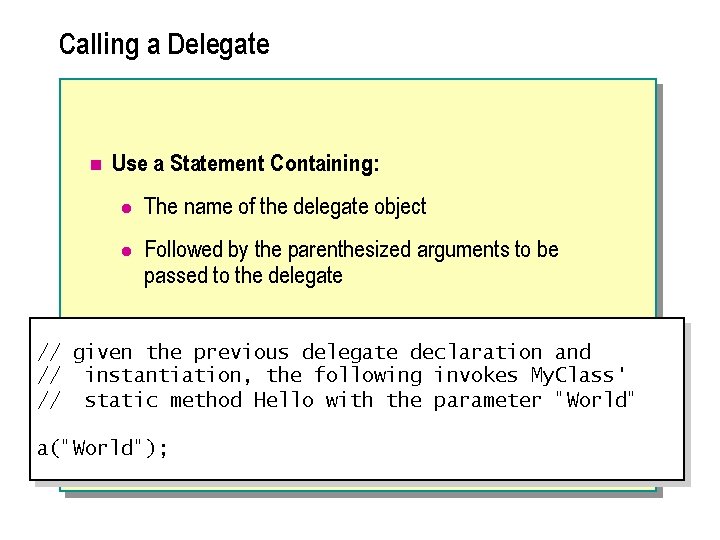
Calling a Delegate n Use a Statement Containing: l The name of the delegate object l Followed by the parenthesized arguments to be passed to the delegate // given the previous delegate declaration and // instantiation, the following invokes My. Class' // static method Hello with the parameter "World" a("World");
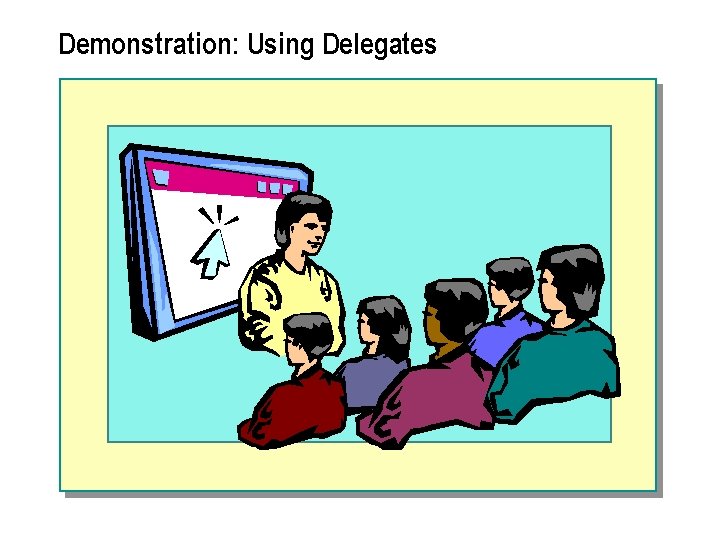
Demonstration: Using Delegates
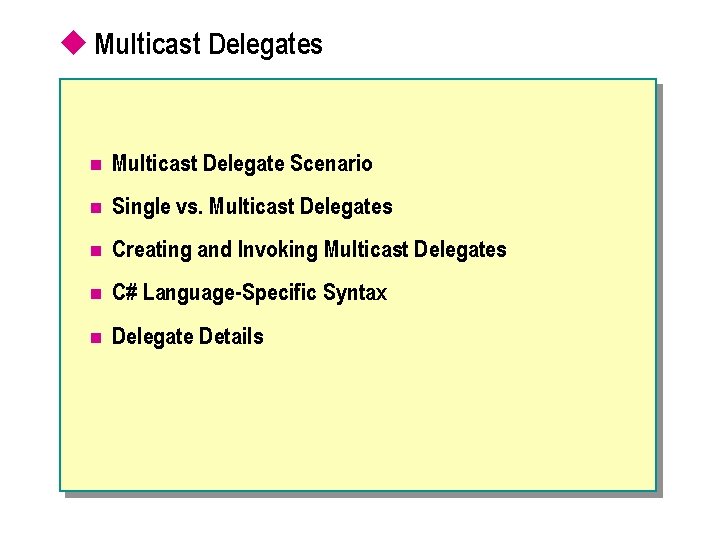
u Multicast Delegates n Multicast Delegate Scenario n Single vs. Multicast Delegates n Creating and Invoking Multicast Delegates n C# Language-Specific Syntax n Delegate Details
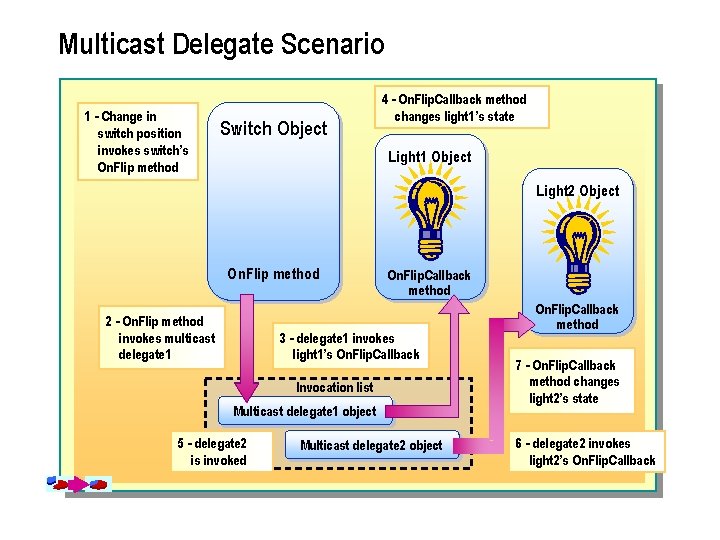
Multicast Delegate Scenario 1 - Change in switch position invokes switch’s On. Flip method Switch Object 4 - On. Flip. Callback method changes light 1’s state Light 1 Object Light 2 Object On. Flip method 2 - On. Flip method invokes multicast delegate 1 On. Flip. Callback method 3 - delegate 1 invokes light 1’s On. Flip. Callback Invocation list Multicast delegate 1 object 5 - delegate 2 is invoked Multicast delegate 2 object On. Flip. Callback method 7 - On. Flip. Callback method changes light 2’s state 6 - delegate 2 invokes light 2’s On. Flip. Callback
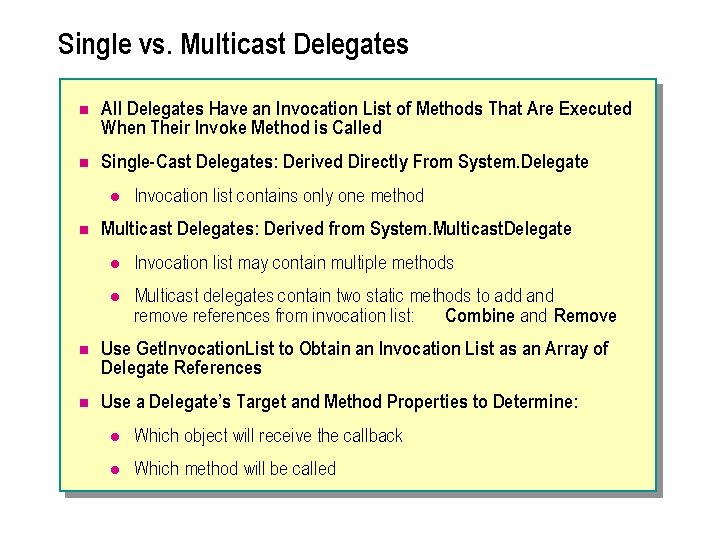
Single vs. Multicast Delegates n All Delegates Have an Invocation List of Methods That Are Executed When Their Invoke Method is Called n Single-Cast Delegates: Derived Directly From System. Delegate l n Invocation list contains only one method Multicast Delegates: Derived from System. Multicast. Delegate l Invocation list may contain multiple methods l Multicast delegates contain two static methods to add and remove references from invocation list: Combine and Remove n Use Get. Invocation. List to Obtain an Invocation List as an Array of Delegate References n Use a Delegate’s Target and Method Properties to Determine: l Which object will receive the callback l Which method will be called
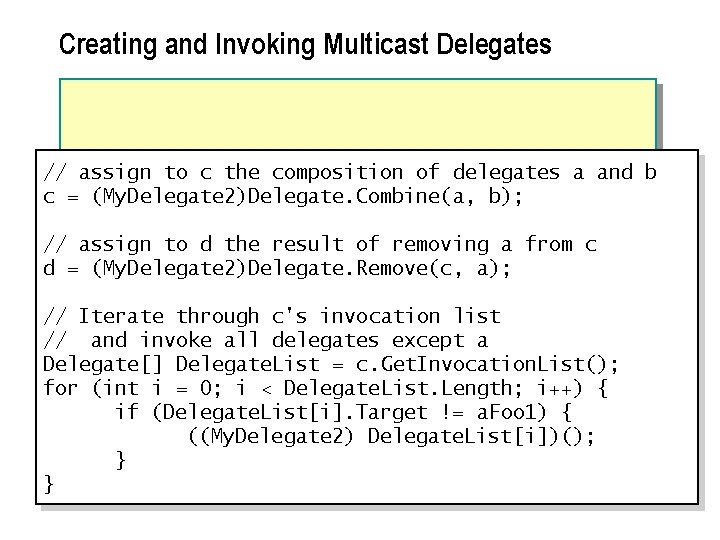
Creating and Invoking Multicast Delegates // assign to c the composition of delegates a and b c = (My. Delegate 2)Delegate. Combine(a, b); // assign to d the result of removing a from c d = (My. Delegate 2)Delegate. Remove(c, a); // Iterate through c's invocation list // and invoke all delegates except a Delegate[] Delegate. List = c. Get. Invocation. List(); for (int i = 0; i < Delegate. List. Length; i++) { if (Delegate. List[i]. Target != a. Foo 1) { ((My. Delegate 2) Delegate. List[i])(); } }
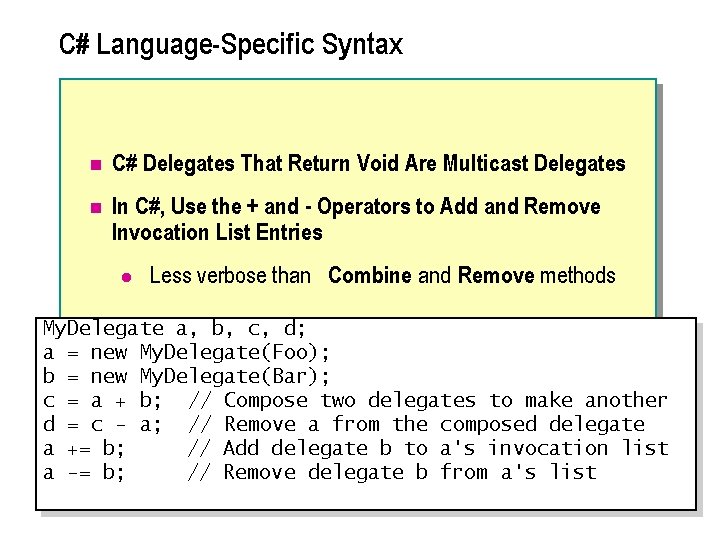
C# Language-Specific Syntax n C# Delegates That Return Void Are Multicast Delegates n In C#, Use the + and - Operators to Add and Remove Invocation List Entries l Less verbose than Combine and Remove methods My. Delegate a, b, c, d; a = new My. Delegate(Foo); b = new My. Delegate(Bar); c = a + b; // Compose two delegates to make another d = c - a; // Remove a from the composed delegate a += b; // Add delegate b to a's invocation list a -= b; // Remove delegate b from a's list
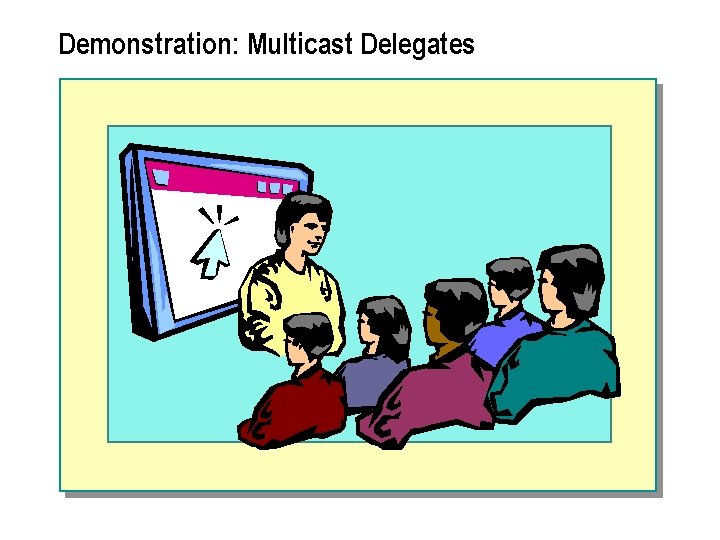
Demonstration: Multicast Delegates
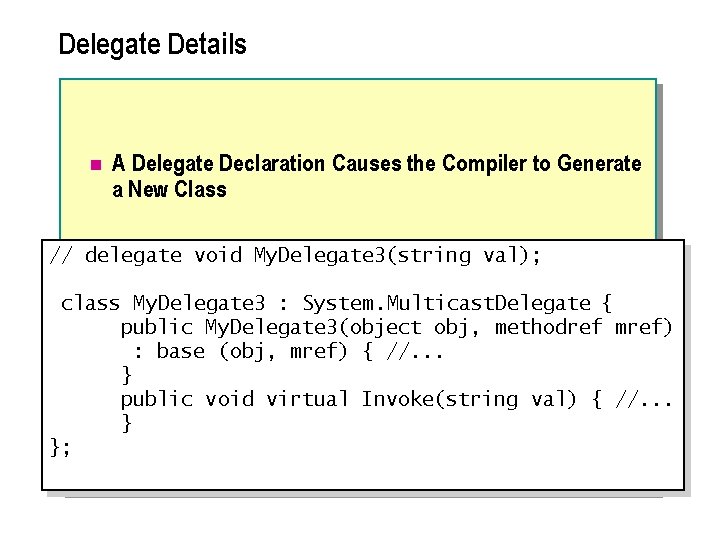
Delegate Details n A Delegate Declaration Causes the Compiler to Generate a New Class // delegate void My. Delegate 3(string val); class My. Delegate 3 : System. Multicast. Delegate { public My. Delegate 3(object obj, methodref mref) : base (obj, mref) { //. . . } public void virtual Invoke(string val) { //. . . } };
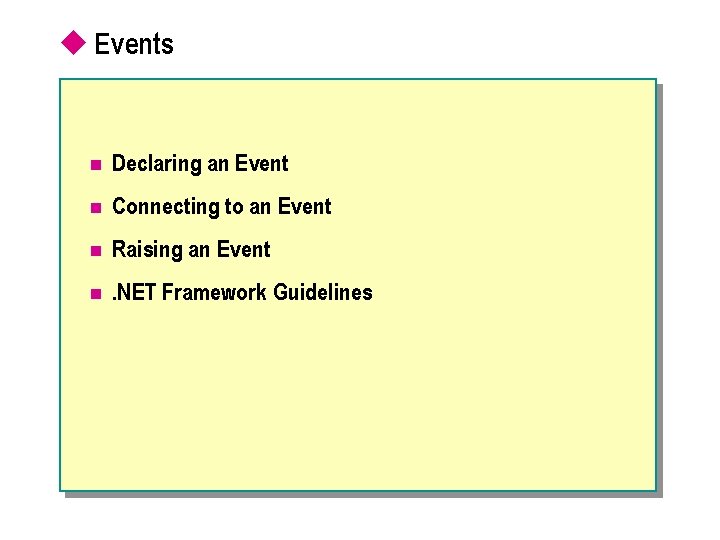
u Events n Declaring an Event n Connecting to an Event n Raising an Event n . NET Framework Guidelines
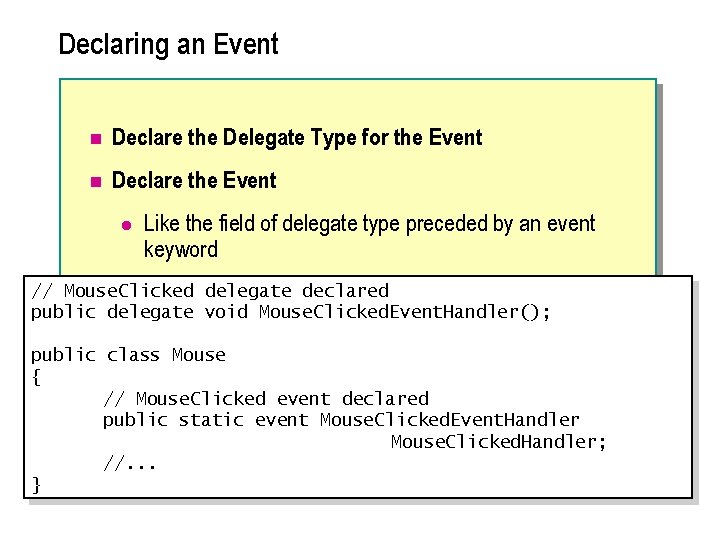
Declaring an Event n Declare the Delegate Type for the Event n Declare the Event l Like the field of delegate type preceded by an event keyword // Mouse. Clicked delegate declared public delegate void Mouse. Clicked. Event. Handler(); public class Mouse { // Mouse. Clicked event declared public static event Mouse. Clicked. Event. Handler Mouse. Clicked. Handler; //. . . }
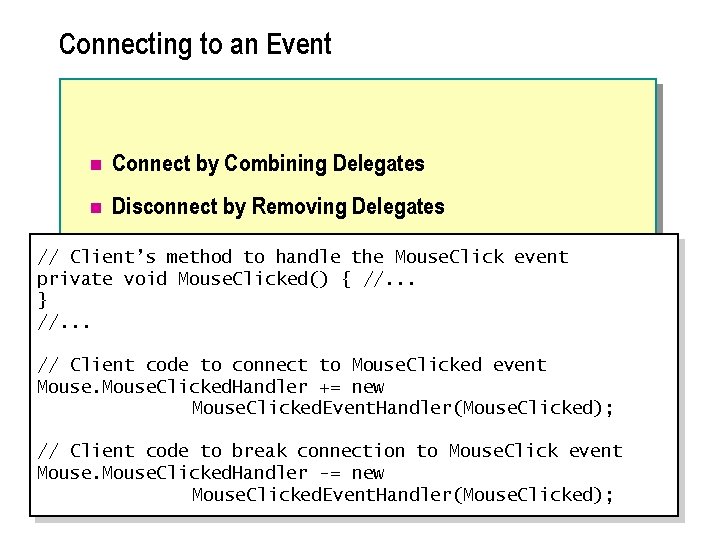
Connecting to an Event n Connect by Combining Delegates n Disconnect by Removing Delegates // Client’s method to handle the Mouse. Click event private void Mouse. Clicked() { //. . . } //. . . // Client code to connect to Mouse. Clicked event Mouse. Clicked. Handler += new Mouse. Clicked. Event. Handler(Mouse. Clicked); // Client code to break connection to Mouse. Click event Mouse. Clicked. Handler -= new Mouse. Clicked. Event. Handler(Mouse. Clicked);
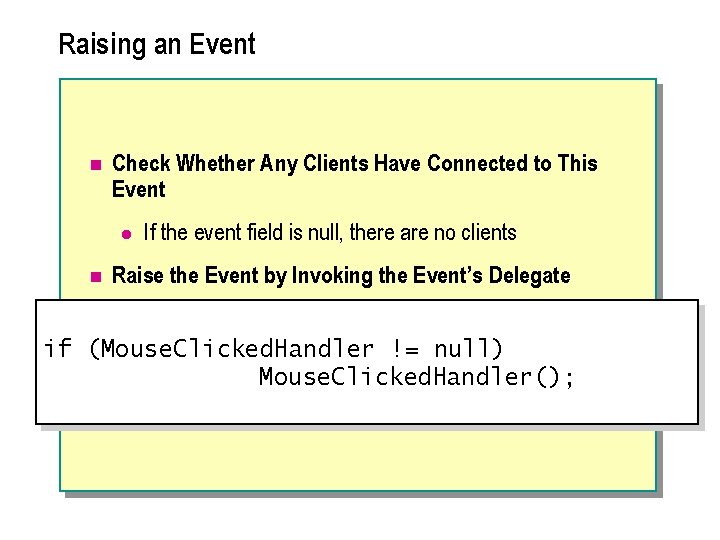
Raising an Event n Check Whether Any Clients Have Connected to This Event l n If the event field is null, there are no clients Raise the Event by Invoking the Event’s Delegate if (Mouse. Clicked. Handler != null) Mouse. Clicked. Handler();
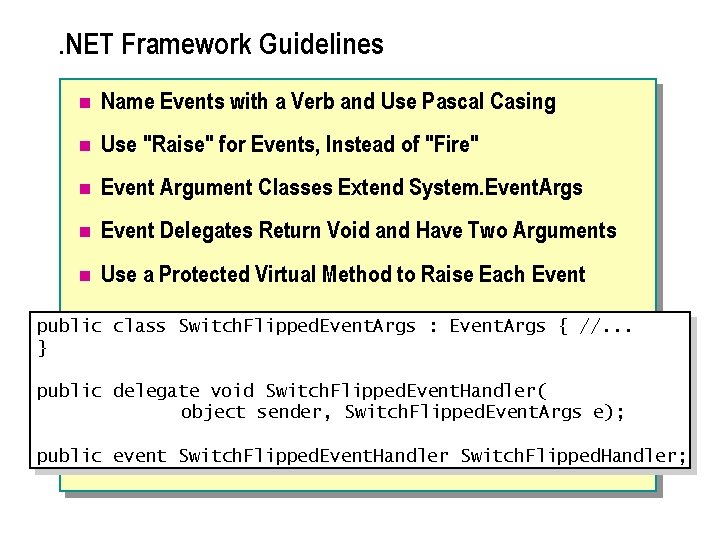
. NET Framework Guidelines n Name Events with a Verb and Use Pascal Casing n Use "Raise" for Events, Instead of "Fire" n Event Argument Classes Extend System. Event. Args n Event Delegates Return Void and Have Two Arguments n Use a Protected Virtual Method to Raise Each Event public class Switch. Flipped. Event. Args : Event. Args { //. . . } public delegate void Switch. Flipped. Event. Handler( object sender, Switch. Flipped. Event. Args e); public event Switch. Flipped. Event. Handler Switch. Flipped. Handler;
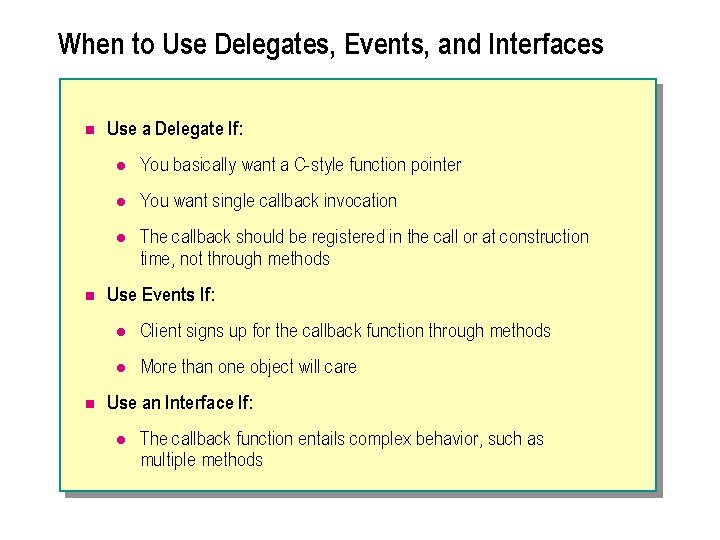
When to Use Delegates, Events, and Interfaces n n n Use a Delegate If: l You basically want a C-style function pointer l You want single callback invocation l The callback should be registered in the call or at construction time, not through methods Use Events If: l Client signs up for the callback function through methods l More than one object will care Use an Interface If: l The callback function entails complex behavior, such as multiple methods
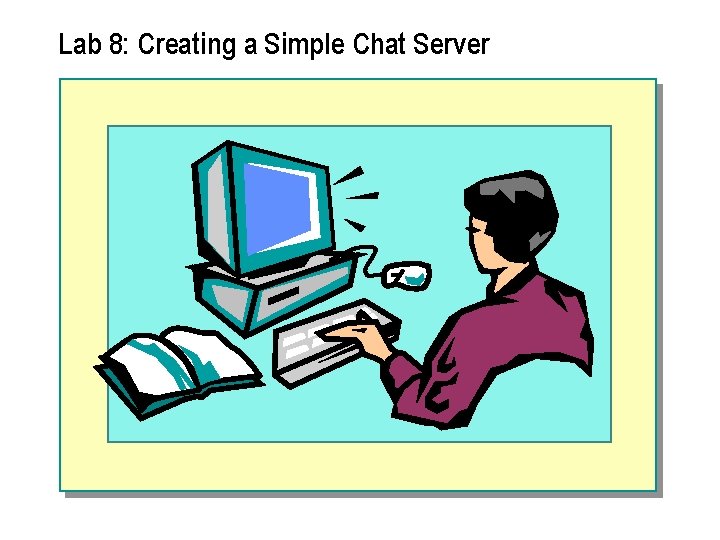
Lab 8: Creating a Simple Chat Server
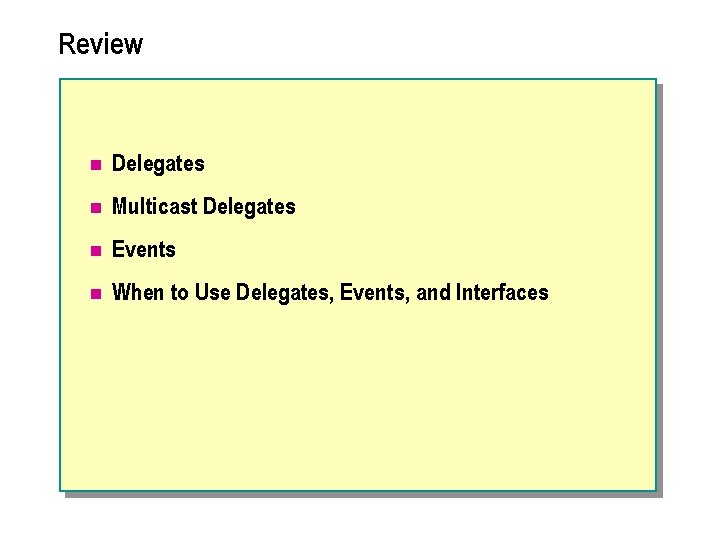
Review n Delegates n Multicast Delegates n Events n When to Use Delegates, Events, and Interfaces