Module 11 Struct Type in C Thanawin Rakthanmanon
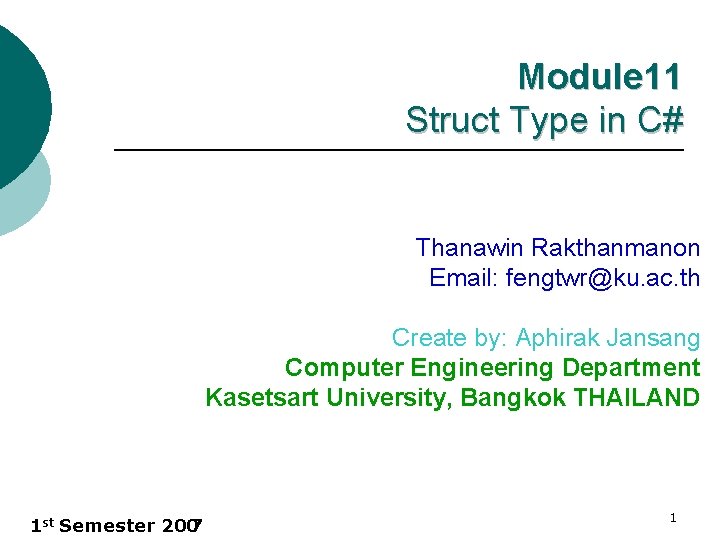
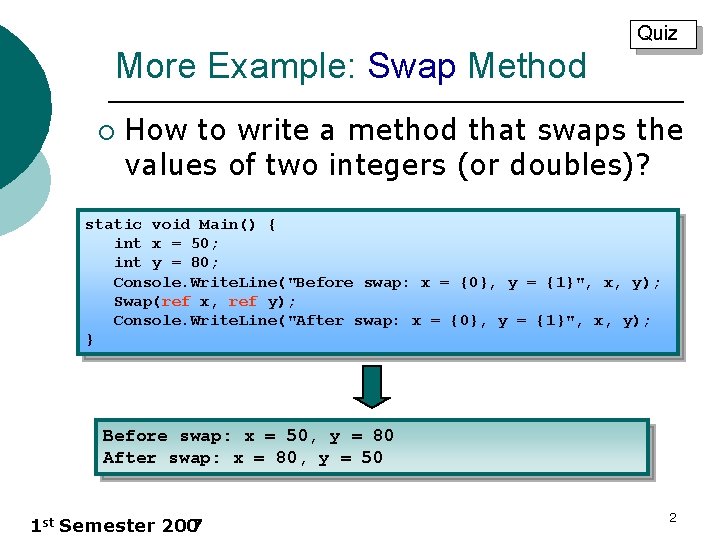
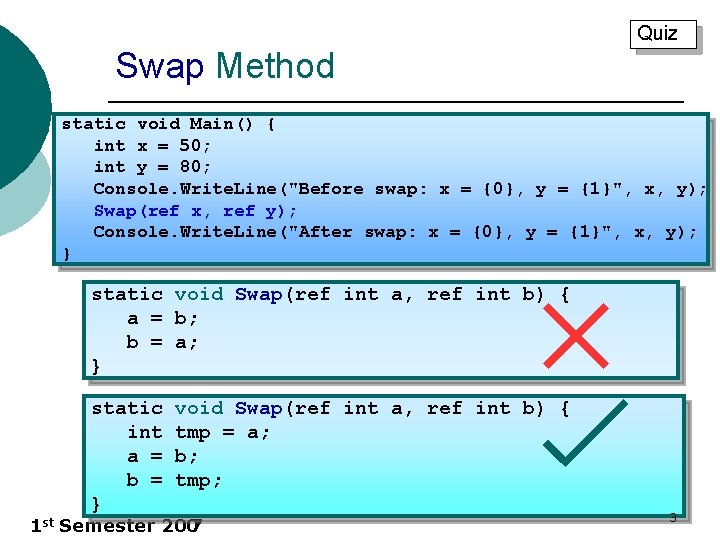
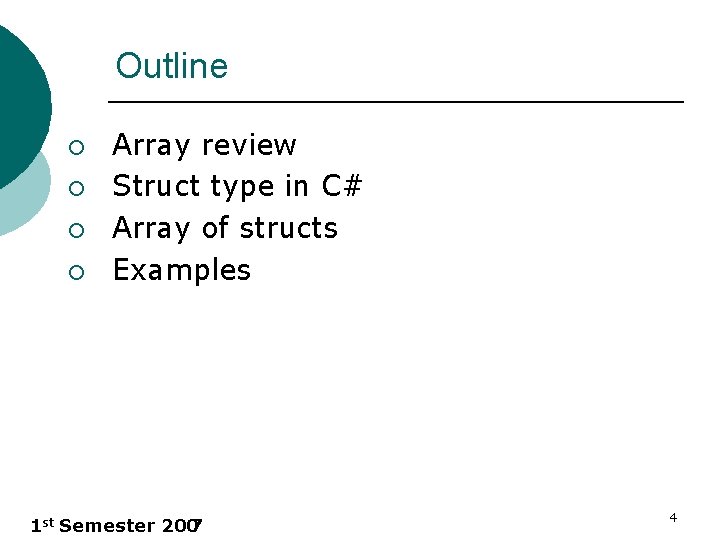
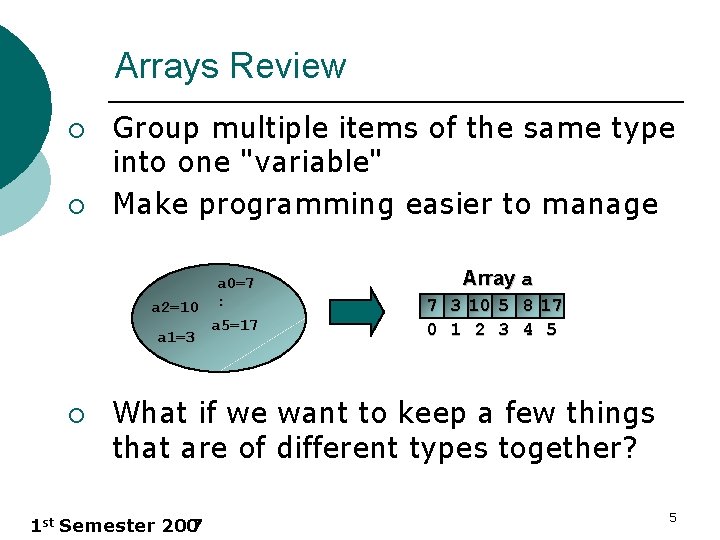
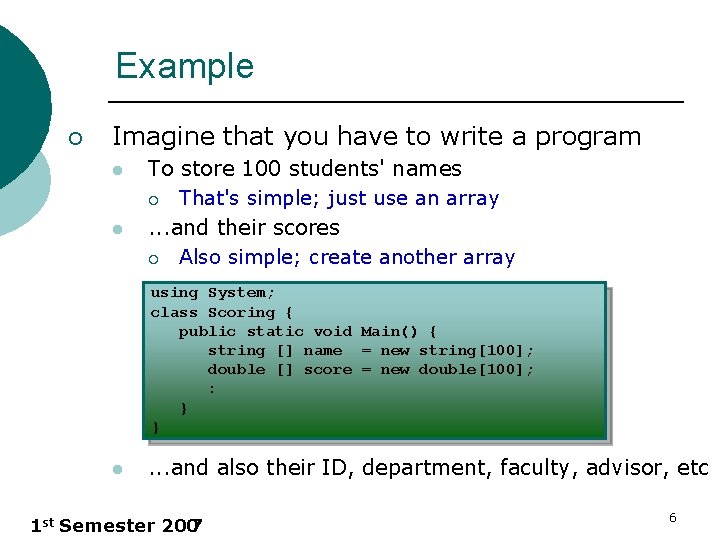
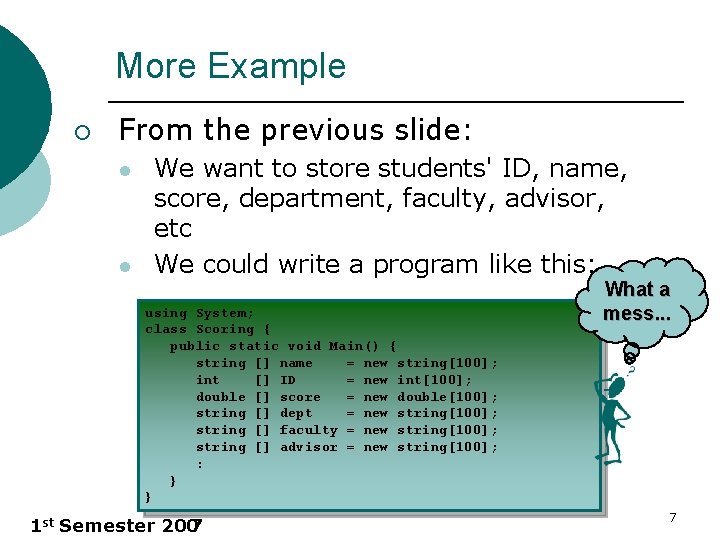
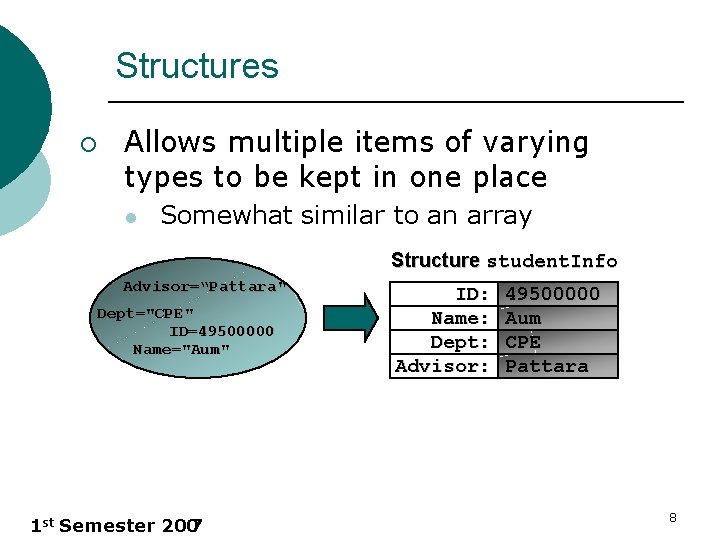
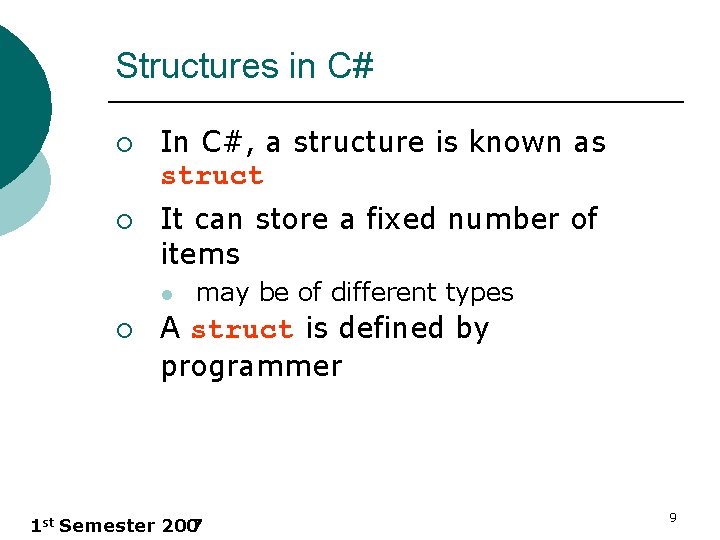
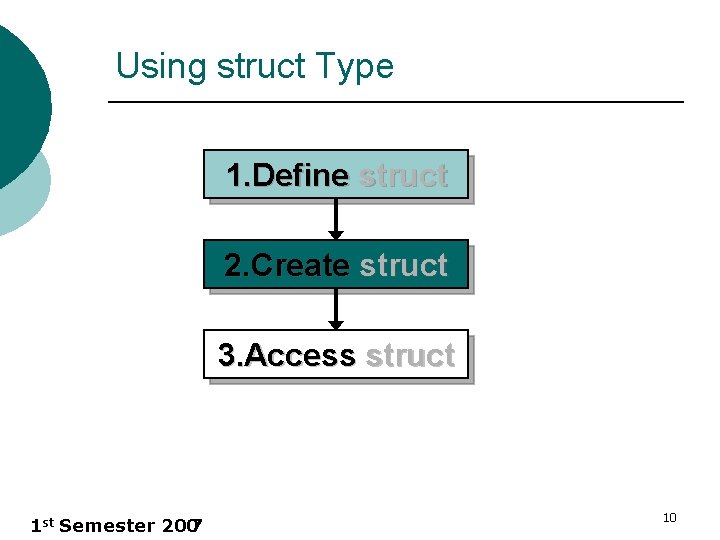
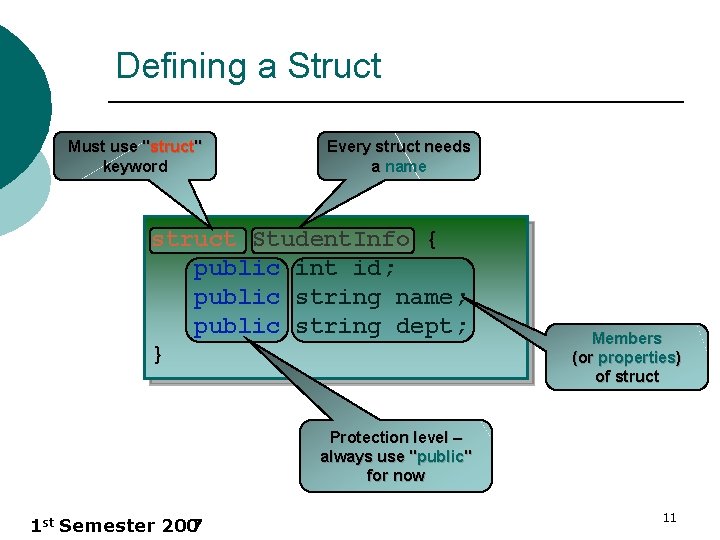
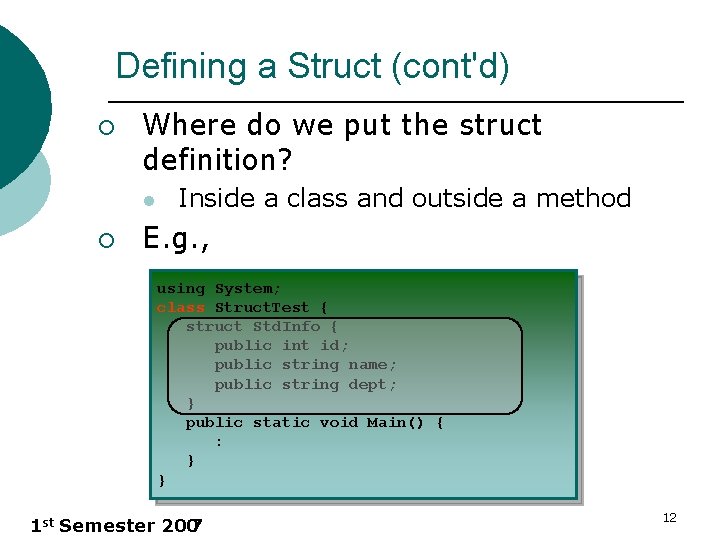
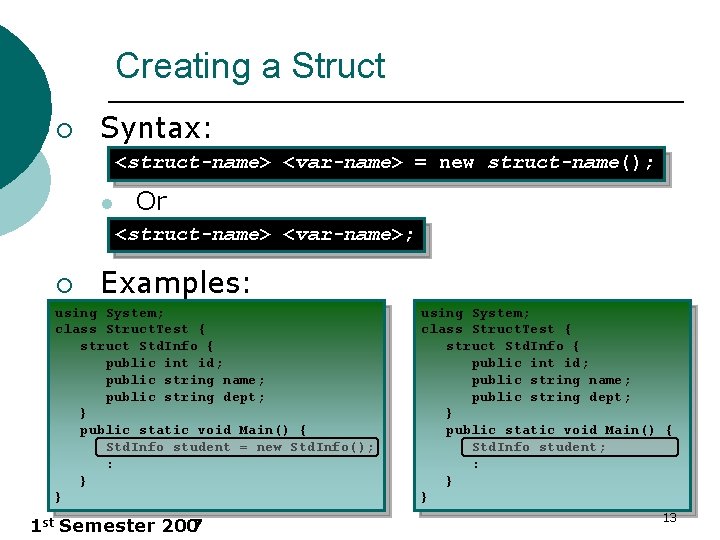
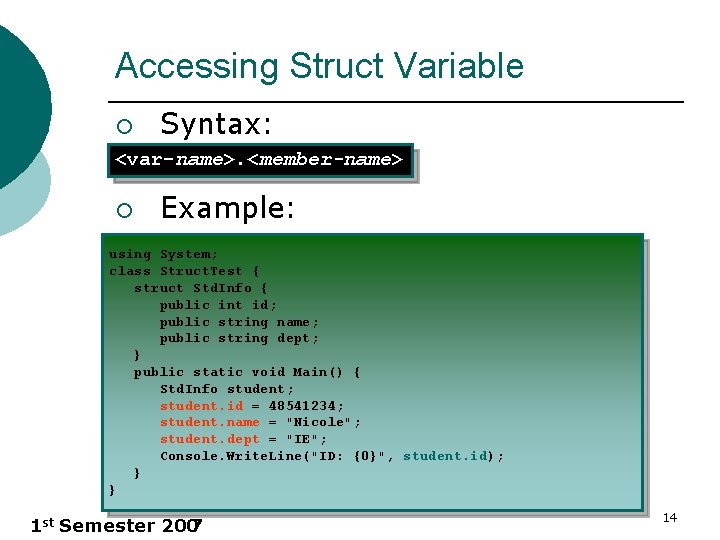
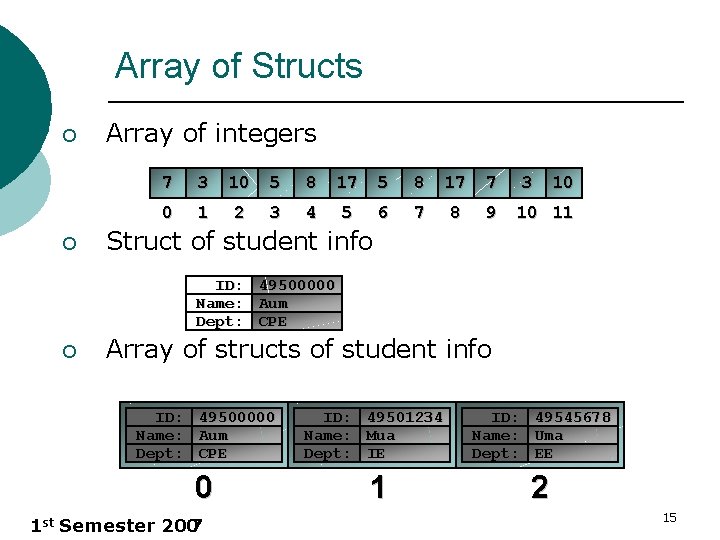
![Creating an Array of Structs ¡ Syntax: <struct-name> [] <array-name> = new <struct-name>[<size>]; ¡ Creating an Array of Structs ¡ Syntax: <struct-name> [] <array-name> = new <struct-name>[<size>]; ¡](https://slidetodoc.com/presentation_image/84178b685e86090e4cb67ab0168a702e/image-16.jpg)
![Accessing Array of Structs ¡ Syntax: <array-name>[<index>]. <member> ¡ Example: l Set Student#5's name Accessing Array of Structs ¡ Syntax: <array-name>[<index>]. <member> ¡ Example: l Set Student#5's name](https://slidetodoc.com/presentation_image/84178b685e86090e4cb67ab0168a702e/image-17.jpg)
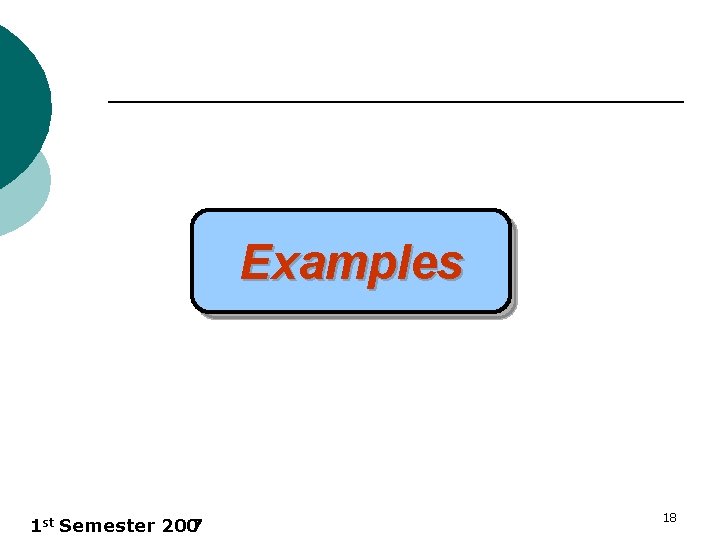
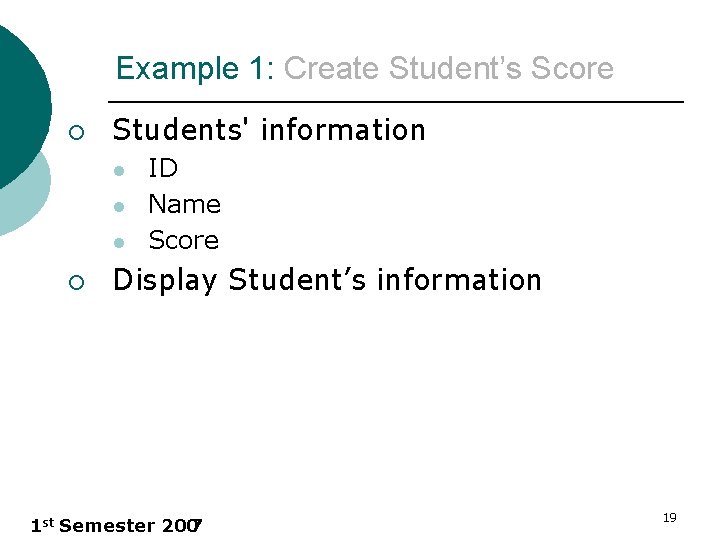
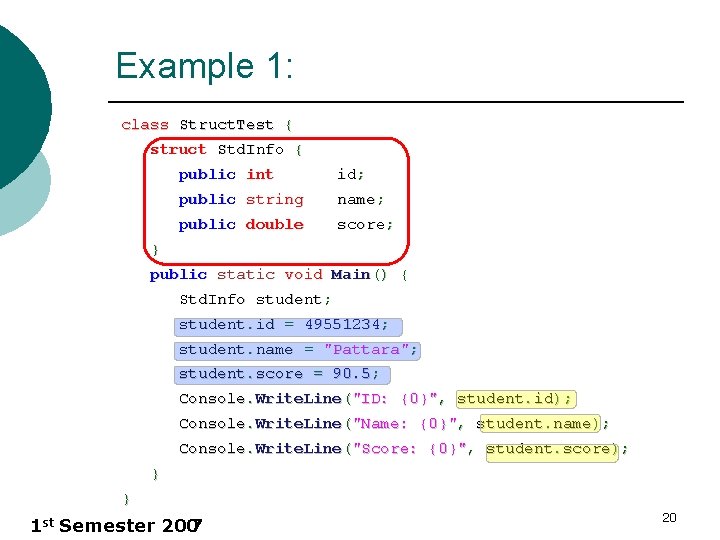
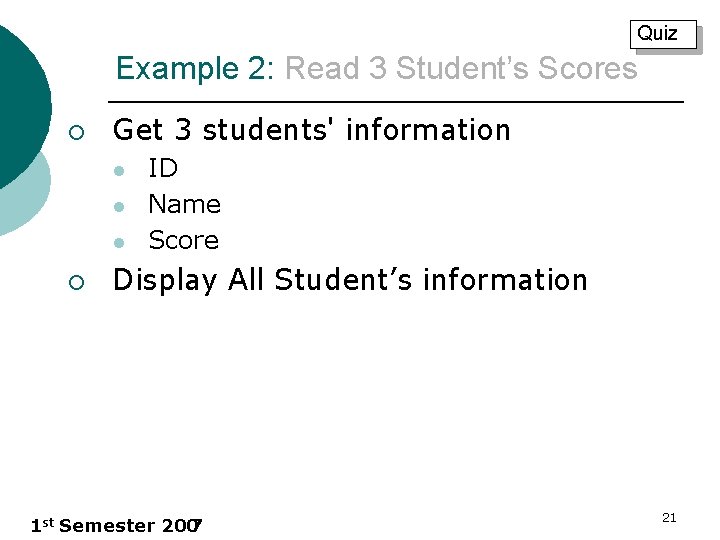
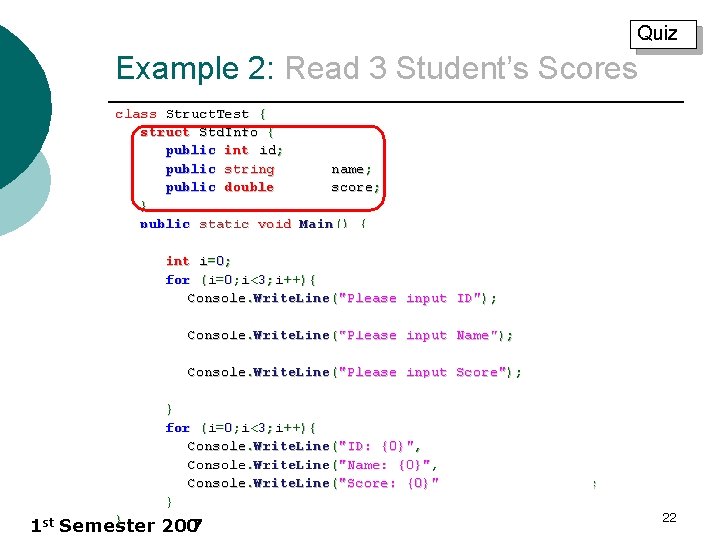
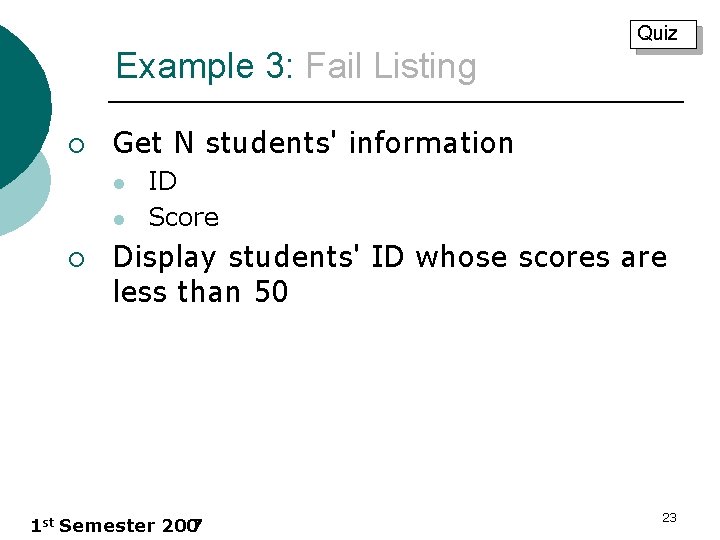
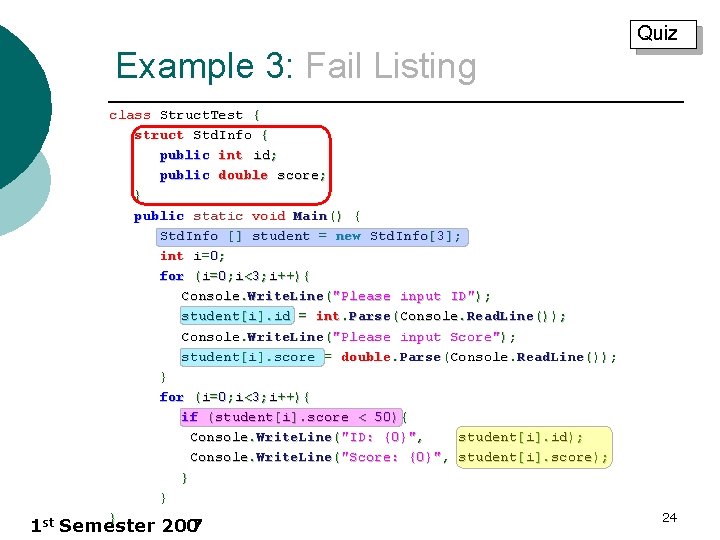
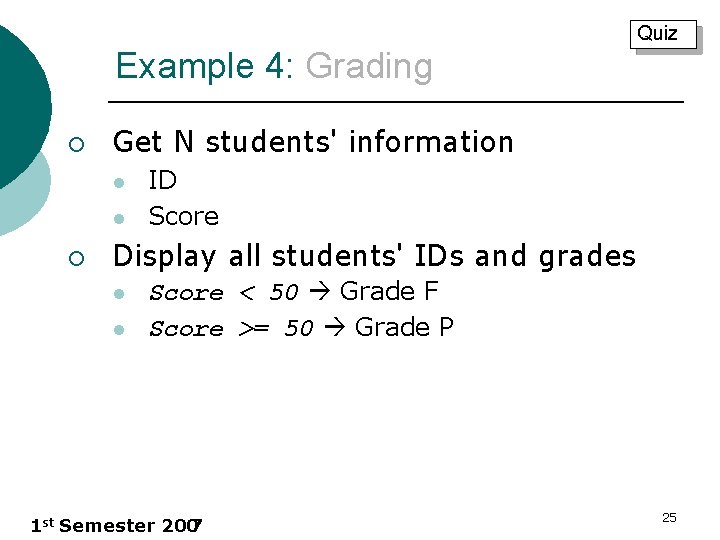
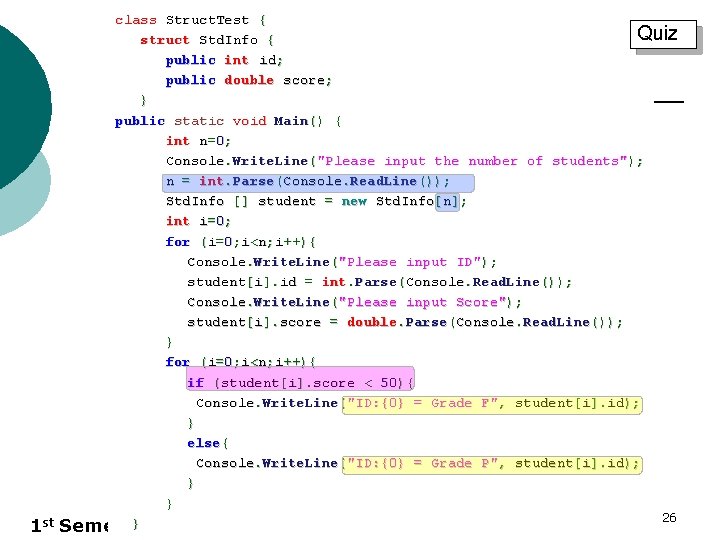
- Slides: 26
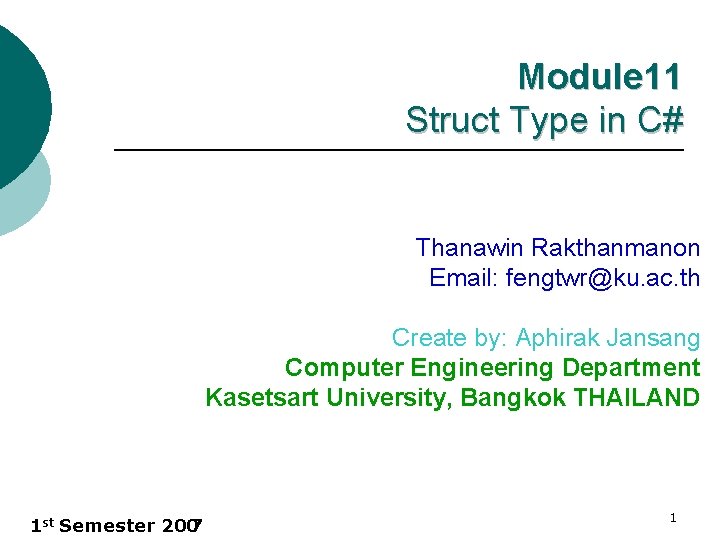
Module 11 Struct Type in C# Thanawin Rakthanmanon Email: fengtwr@ku. ac. th Create by: Aphirak Jansang Computer Engineering Department Kasetsart University, Bangkok THAILAND 1 st Semester 200 7 1
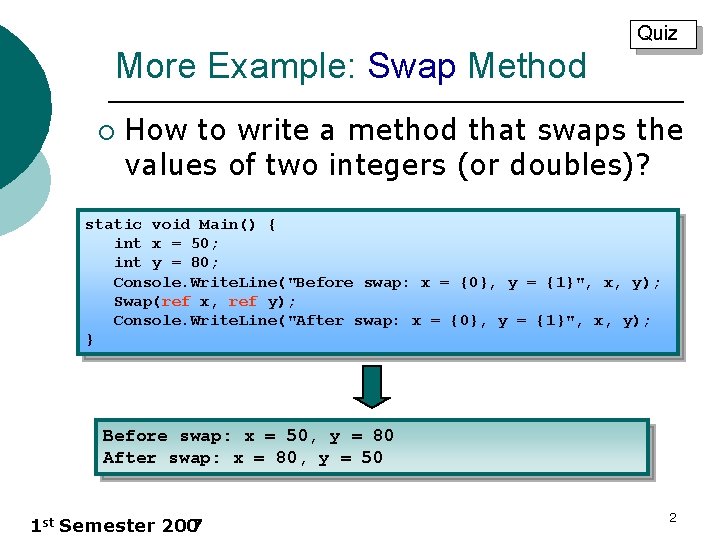
Quiz More Example: Swap Method ¡ How to write a method that swaps the values of two integers (or doubles)? static void Main() { int x = 50; int y = 80; Console. Write. Line("Before swap: x = {0}, y = {1}", x, y); Swap(ref x, ref y); Console. Write. Line("After swap: x = {0}, y = {1}", x, y); } Before swap: x = 50, y = 80 After swap: x = 80, y = 50 1 st Semester 200 7 2
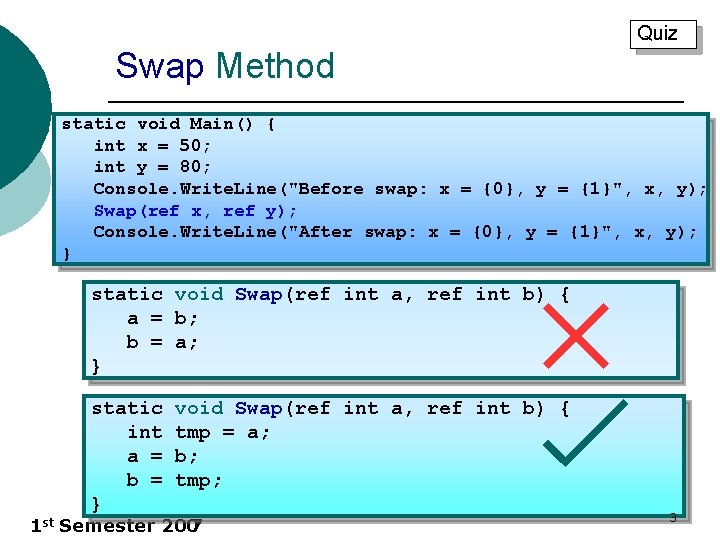
Quiz Swap Method static void Main() { int x = 50; int y = 80; Console. Write. Line("Before swap: x = {0}, y = {1}", x, y); Swap(ref x, ref y); Console. Write. Line("After swap: x = {0}, y = {1}", x, y); } static void Swap(ref int a, ref int b) { a = b; b = a; } static int a = b = } void Swap(ref int a, ref int b) { tmp = a; b; tmp; 1 st Semester 200 7 3
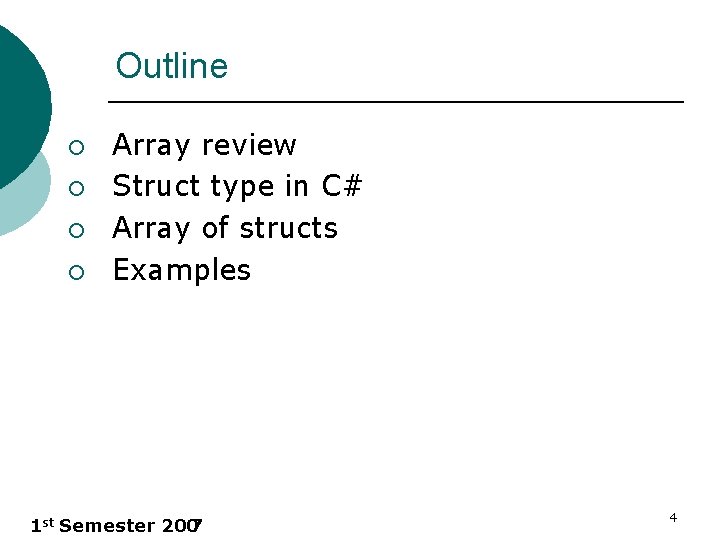
Outline ¡ ¡ Array review Struct type in C# Array of structs Examples 1 st Semester 200 7 4
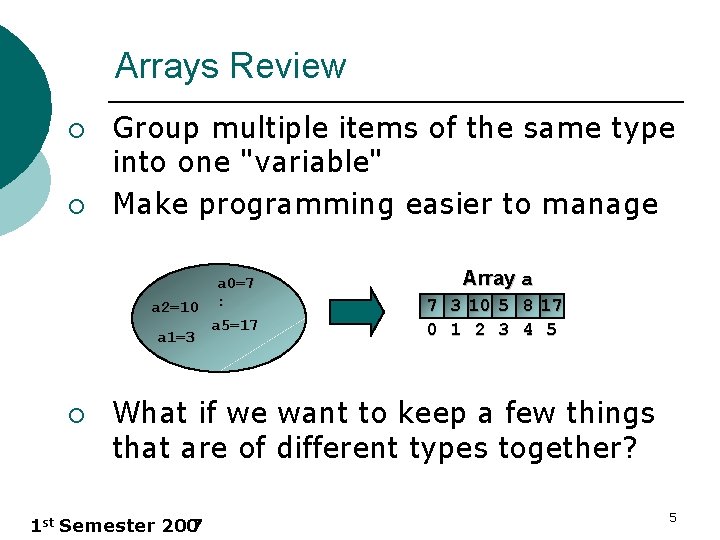
Arrays Review ¡ ¡ Group multiple items of the same type into one "variable" Make programming easier to manage a 0=7 a 2=10 : a 5=17 a 1=3 ¡ Array a 7 3 10 5 8 17 0 1 2 3 4 5 What if we want to keep a few things that are of different types together? 1 st Semester 200 7 5
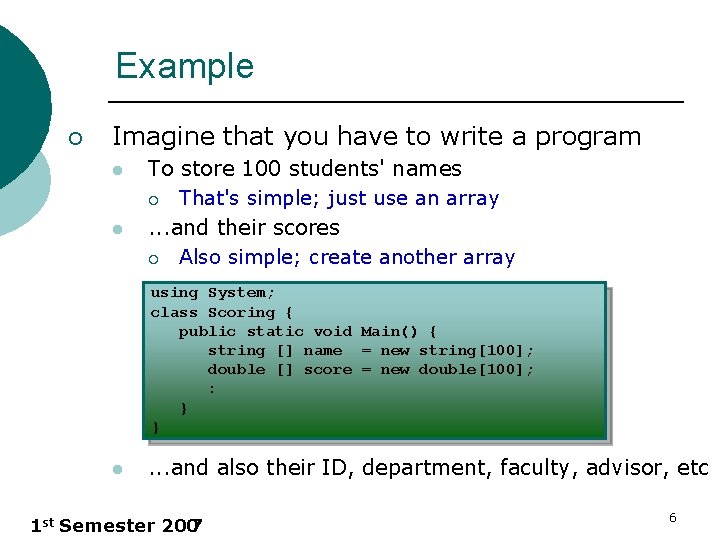
Example ¡ Imagine that you have to write a program l l To store 100 students' names ¡ That's simple; just use an array. . . and their scores ¡ Also simple; create another array using System; class Scoring { public static void Main() { string [] name = new string[100]; double [] score = new double[100]; : } } l . . . and also their ID, department, faculty, advisor, etc 1 st Semester 200 7 6
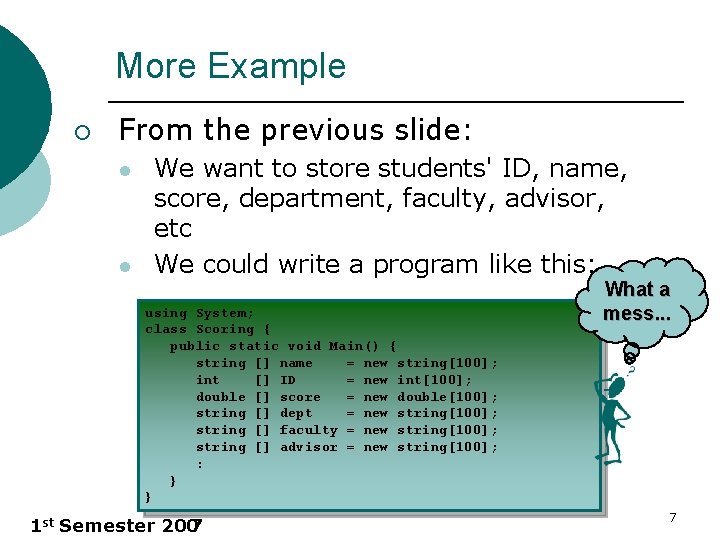
More Example ¡ From the previous slide: l l We want to store students' ID, name, score, department, faculty, advisor, etc We could write a program like this: using System; class Scoring { public static void Main() { string [] name = new string[100]; int [] ID = new int[100]; double [] score = new double[100]; string [] dept = new string[100]; string [] faculty = new string[100]; string [] advisor = new string[100]; : } } 1 st Semester 200 7 What a mess. . . 7
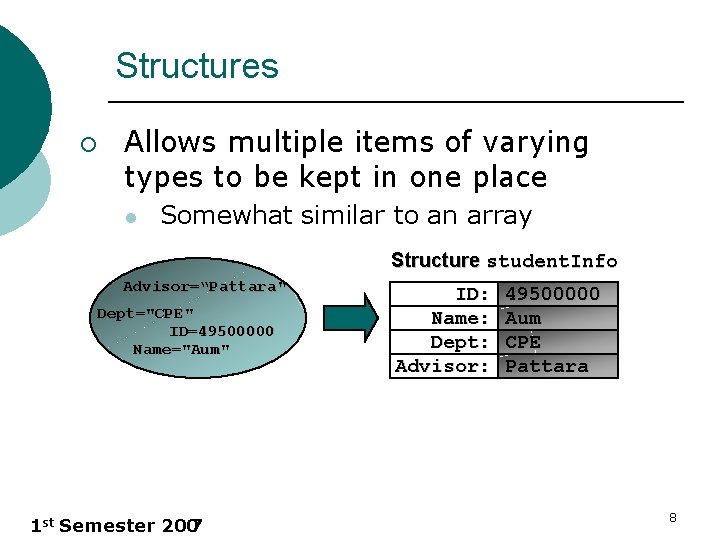
Structures ¡ Allows multiple items of varying types to be kept in one place l Somewhat similar to an array Structure student. Info Advisor=“Pattara" Dept="CPE" ID=49500000 Name="Aum" 1 st Semester 200 7 ID: Name: Dept: Advisor: 49500000 Aum CPE Pattara 8
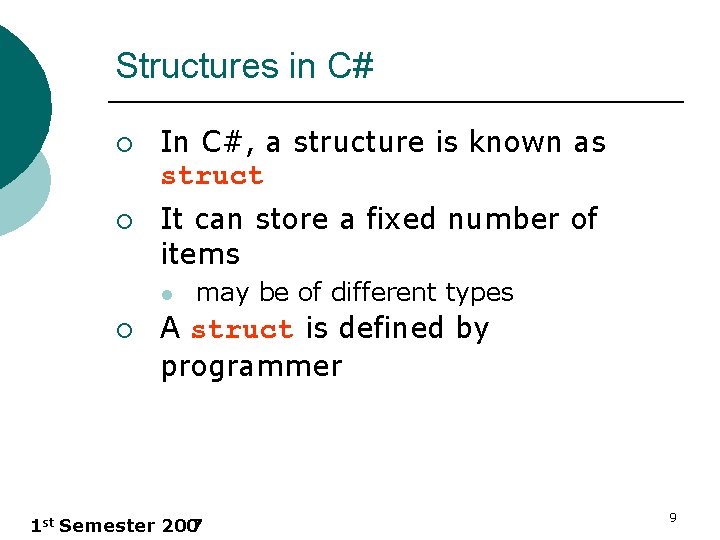
Structures in C# ¡ ¡ In C#, a structure is known as struct It can store a fixed number of items l ¡ may be of different types A struct is defined by programmer 1 st Semester 200 7 9
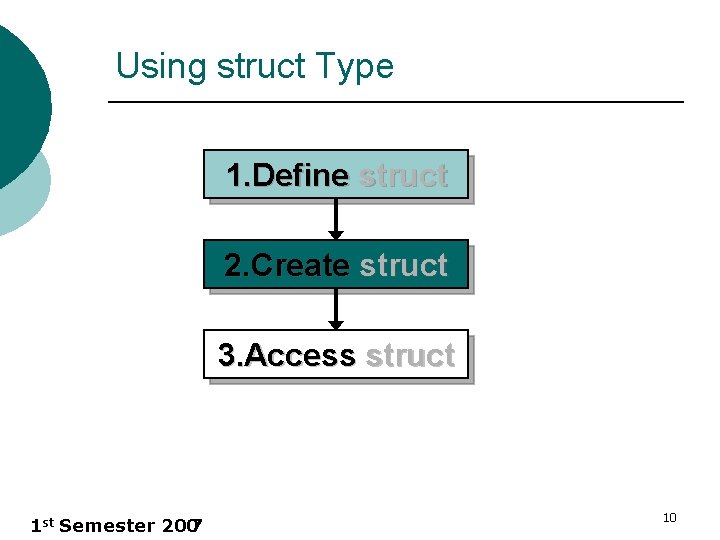
Using struct Type 1. Define struct 2. Create struct 3. Access struct 1 st Semester 200 7 10
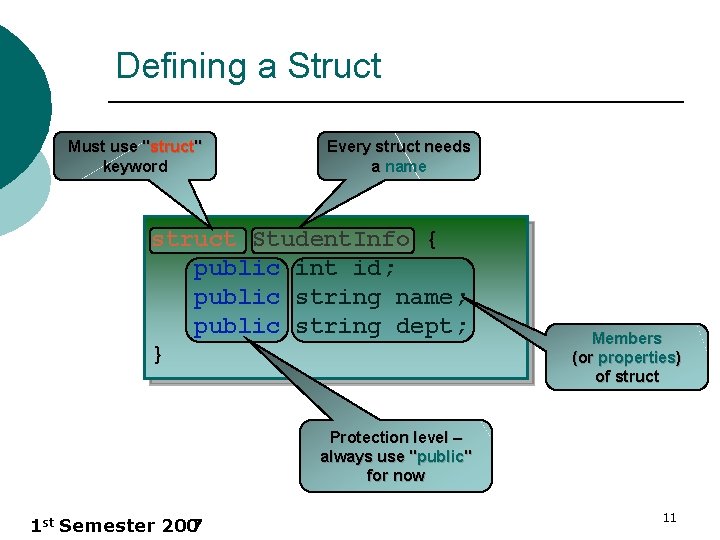
Defining a Struct Must use "struct" keyword Every struct needs a name struct Student. Info { public int id; public string name; public string dept; } Members (or properties) of struct Protection level – always use "public" for now 1 st Semester 200 7 11
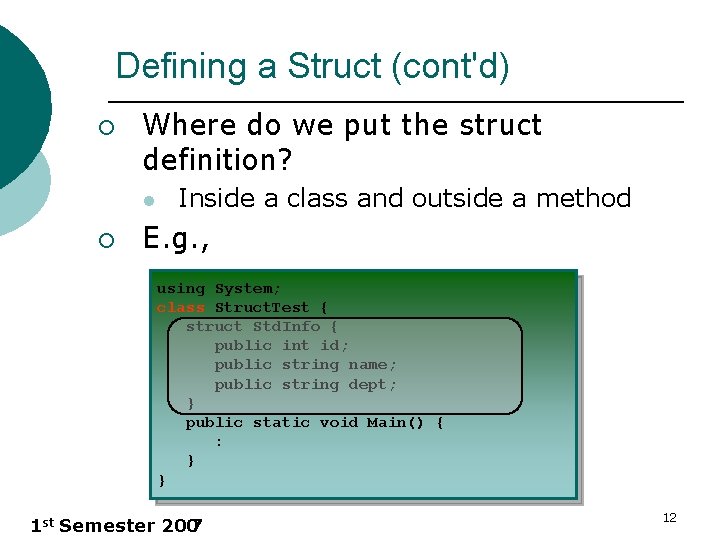
Defining a Struct (cont'd) ¡ Where do we put the struct definition? l ¡ Inside a class and outside a method E. g. , using System; class Struct. Test { struct Std. Info { public int id; public string name; public string dept; } public static void Main() { : } } 1 st Semester 200 7 12
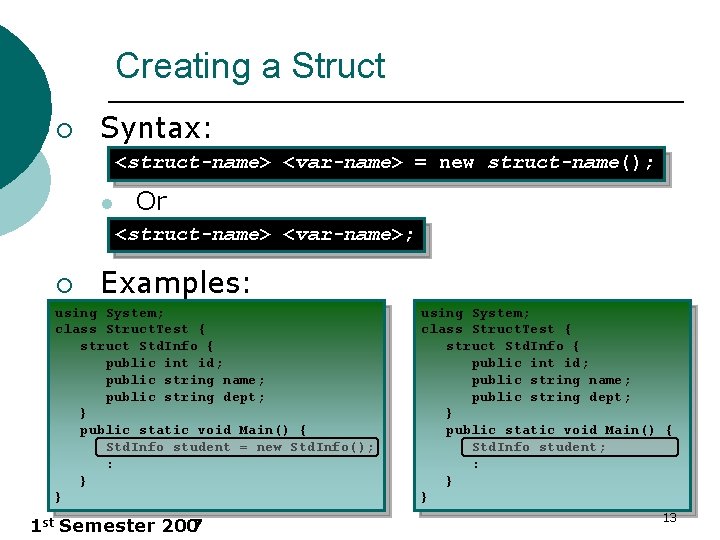
Creating a Struct ¡ Syntax: <struct-name> <var-name> = new struct-name(); l Or <struct-name> <var-name>; ¡ Examples: using System; class Struct. Test { struct Std. Info { public int id; public string name; public string dept; } public static void Main() { Std. Info student = new Std. Info(); : } } 1 st Semester 200 7 using System; class Struct. Test { struct Std. Info { public int id; public string name; public string dept; } public static void Main() { Std. Info student; : } } 13
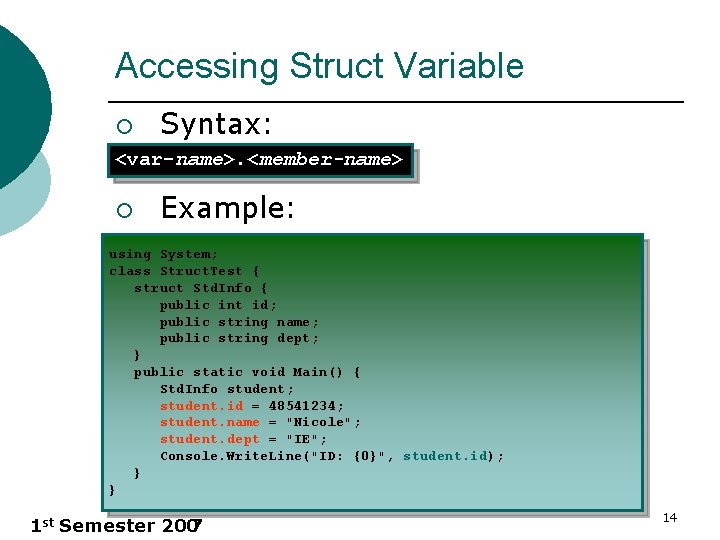
Accessing Struct Variable ¡ Syntax: <var-name>. <member-name> ¡ Example: using System; class Struct. Test { struct Std. Info { public int id; public string name; public string dept; } public static void Main() { Std. Info student; student. id = 48541234; student. name = "Nicole"; student. dept = "IE"; Console. Write. Line("ID: {0}", student. id); } } 1 st Semester 200 7 14
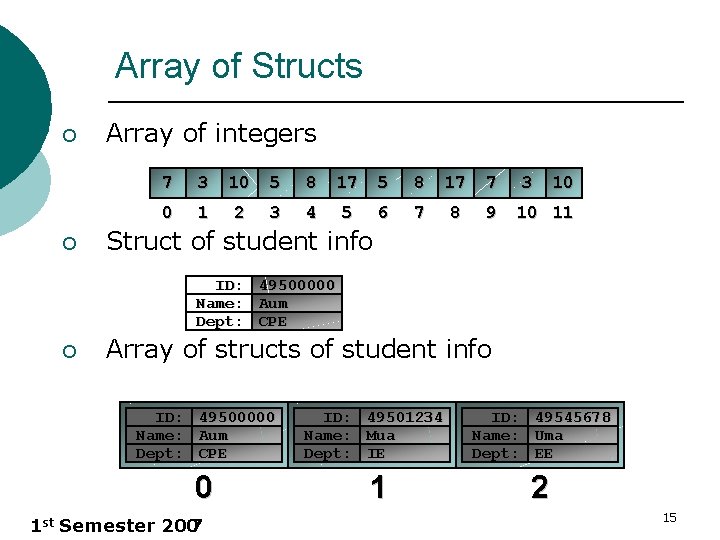
Array of Structs ¡ ¡ Array of integers 7 3 10 5 8 17 7 0 1 2 3 4 5 6 7 8 9 10 10 11 Struct of student info ID: Name: Dept: ¡ 3 49500000 Aum CPE Array of structs of student info ID: Name: Dept: 49500000 Aum CPE 0 1 st Semester 200 7 ID: Name: Dept: 49501234 Mua IE 1 ID: Name: Dept: 49545678 Uma EE 2 15
![Creating an Array of Structs Syntax structname arrayname new structnamesize Creating an Array of Structs ¡ Syntax: <struct-name> [] <array-name> = new <struct-name>[<size>]; ¡](https://slidetodoc.com/presentation_image/84178b685e86090e4cb67ab0168a702e/image-16.jpg)
Creating an Array of Structs ¡ Syntax: <struct-name> [] <array-name> = new <struct-name>[<size>]; ¡ Example: using System; class Struct. Test { Creating an array for struct Std. Info { storing information of 50 public int id; students public string name; public string dept; } public static void Main() { Std. Info [] student = new Std. Info[50]; : } } 1 st Semester 200 7 16
![Accessing Array of Structs Syntax arraynameindex member Example l Set Student5s name Accessing Array of Structs ¡ Syntax: <array-name>[<index>]. <member> ¡ Example: l Set Student#5's name](https://slidetodoc.com/presentation_image/84178b685e86090e4cb67ab0168a702e/image-17.jpg)
Accessing Array of Structs ¡ Syntax: <array-name>[<index>]. <member> ¡ Example: l Set Student#5's name to "Pattara" student[4]. name = "Pattara"; l Display Student#3's department Console. Write. Line("Department: {0}", student[2]. dept); 1 st Semester 200 7 17
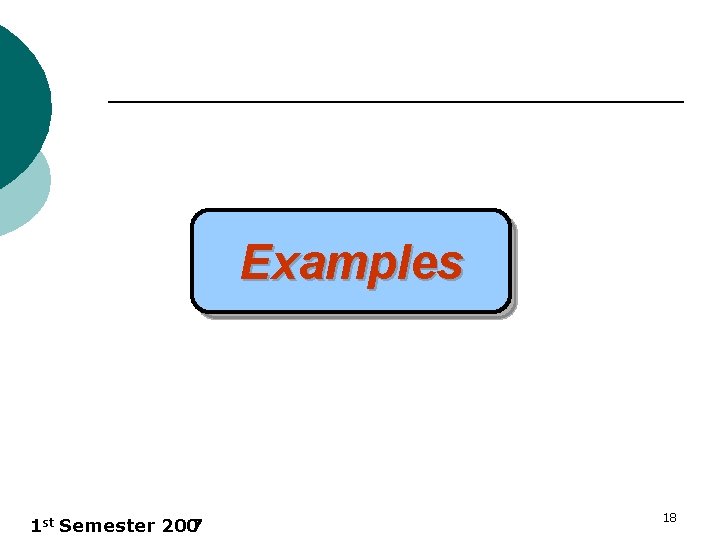
Examples 1 st Semester 200 7 18
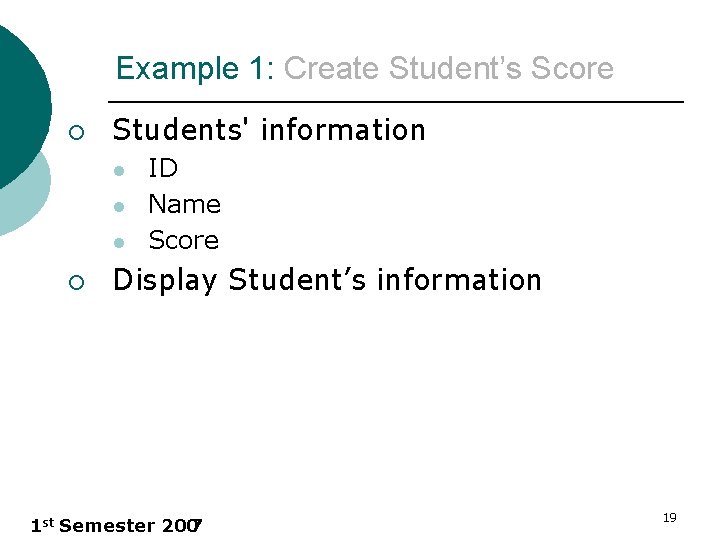
Example 1: Create Student’s Score ¡ Students' information l l l ¡ ID Name Score Display Student’s information 1 st Semester 200 7 19
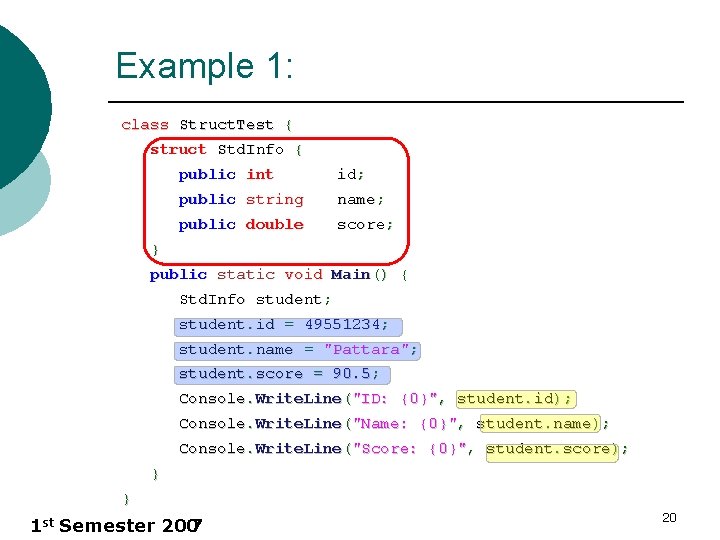
Example 1: class Struct. Test { struct Std. Info { public int id; public string name; public double score; } public static void Main() { Std. Info student; student. id = 49551234; student. name = "Pattara"; student. score = 90. 5; Console. Write. Line("ID: {0}", student. id); Console. Write. Line("Name: {0}", student. name); Console. Write. Line("Score: {0}", student. score); } } 1 st Semester 200 7 20
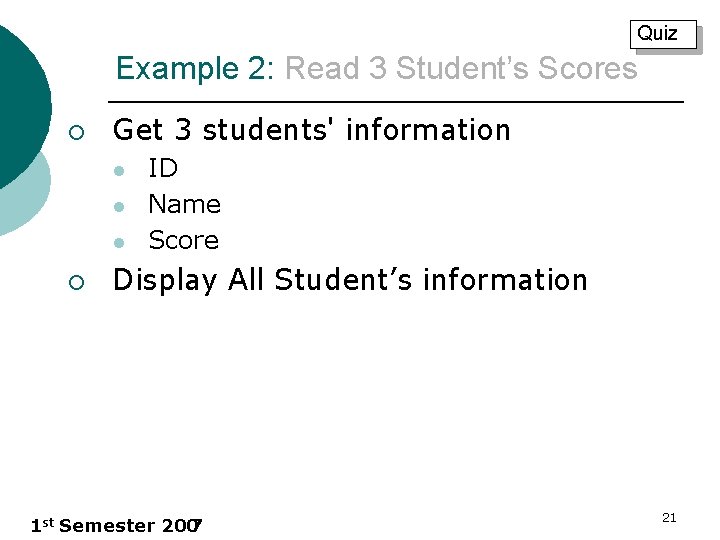
Quiz Example 2: Read 3 Student’s Scores ¡ Get 3 students' information l l l ¡ ID Name Score Display All Student’s information 1 st Semester 200 7 21
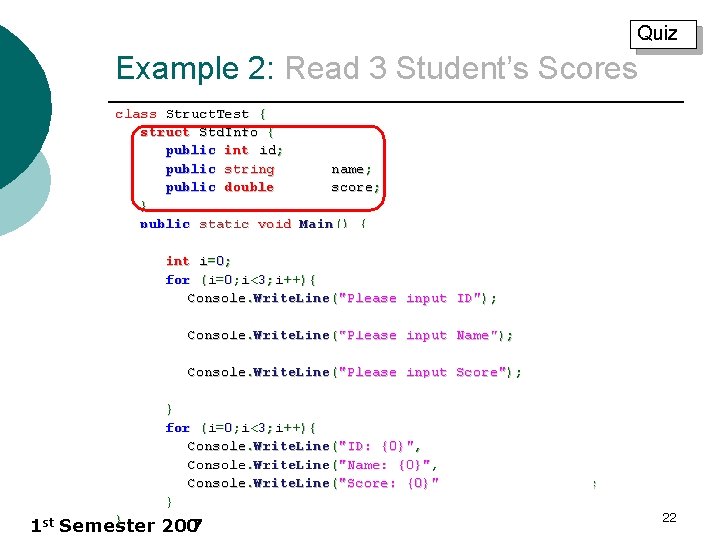
Quiz Example 2: Read 3 Student’s Scores class Struct. Test { struct Std. Info { public int id; public string name; public double score; } public static void Main() { Std. Info [] student = new Std. Info[3]; int i=0; for (i=0; i<3; i++){ Console. Write. Line("Please input ID"); student[i]. id = int. Parse(Console. Read. Line()); Console. Write. Line("Please input Name"); student[i]. name = Console. Read. Line(); Console. Write. Line("Please input Score"); student[i]. score = double. Parse(Console. Read. Line()); } for (i=0; i<3; i++){ Console. Write. Line("ID: {0}", student[i]. id); Console. Write. Line("Name: {0}", student[i]. name); Console. Write. Line("Score: {0}", student[i]. score); } } 1 st Semester 200 7 22
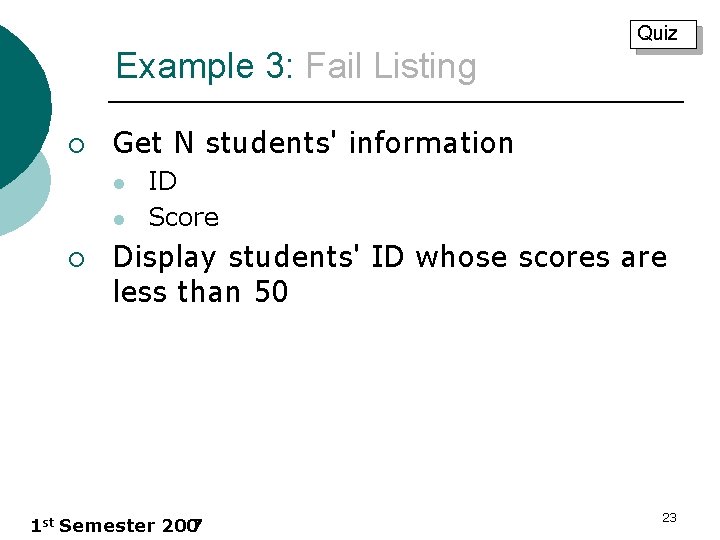
Quiz Example 3: Fail Listing ¡ Get N students' information l l ¡ ID Score Display students' ID whose scores are less than 50 1 st Semester 200 7 23
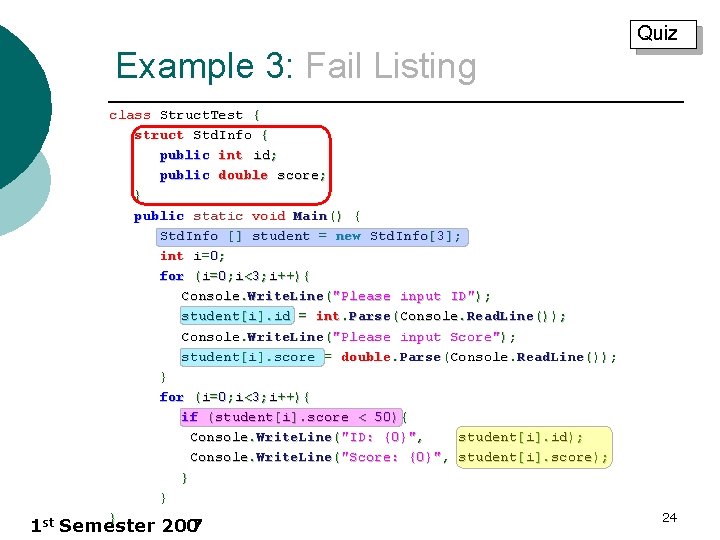
Quiz Example 3: Fail Listing class Struct. Test { struct Std. Info { public int id; public double score; } public static void Main() { Std. Info [] student = new Std. Info[3]; int i=0; for (i=0; i<3; i++){ Console. Write. Line("Please input ID"); student[i]. id = int. Parse(Console. Read. Line()); Console. Write. Line("Please input Score"); student[i]. score = double. Parse(Console. Read. Line()); } for (i=0; i<3; i++){ if (student[i]. score < 50){ Console. Write. Line("ID: {0}", student[i]. id); Console. Write. Line("Score: {0}", student[i]. score); } } } 1 st Semester 200 7 24
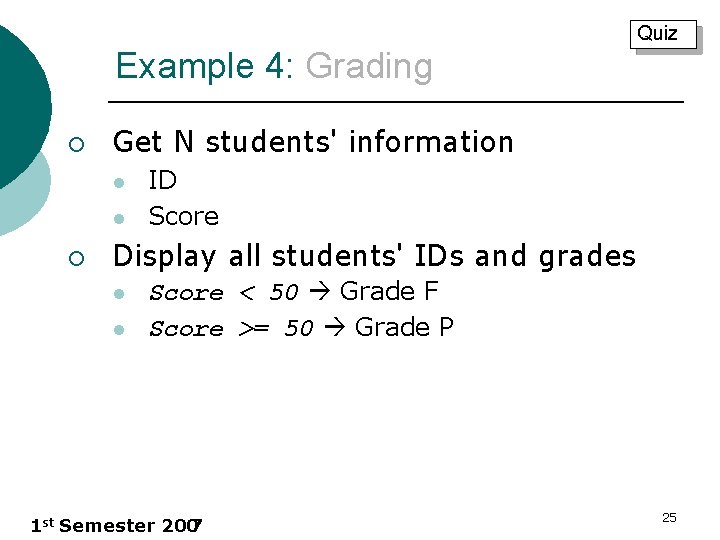
Quiz Example 4: Grading ¡ Get N students' information l l ¡ ID Score Display all students' IDs and grades l l Score < 50 Grade F Score >= 50 Grade P 1 st Semester 200 7 25
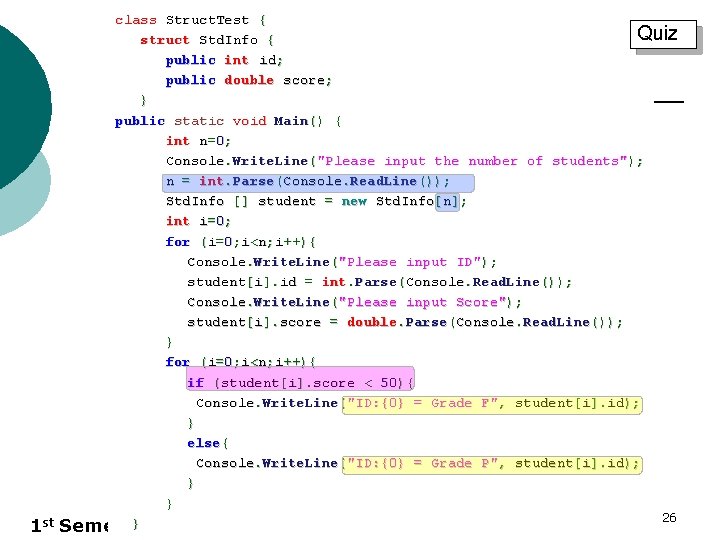
class Struct. Test { Quiz struct Std. Info { public int id; public double score; } public static void Main() { int n=0; Console. Write. Line("Please input the number of students"); n = int. Parse(Console. Read. Line()); Std. Info [] student = new Std. Info[n]; int i=0; for (i=0; i<n; i++){ Console. Write. Line("Please input ID"); student[i]. id = int. Parse(Console. Read. Line()); Console. Write. Line("Please input Score"); student[i]. score = double. Parse(Console. Read. Line()); } for (i=0; i<n; i++){ if (student[i]. score < 50){ Console. Write. Line("ID: {0} = Grade F", student[i]. id); } else{ Console. Write. Line("ID: {0} = Grade P", student[i]. id); } } 26 } 200 1 st Semester 7