ModelViewController Pattern Model Represents enterprise data and the
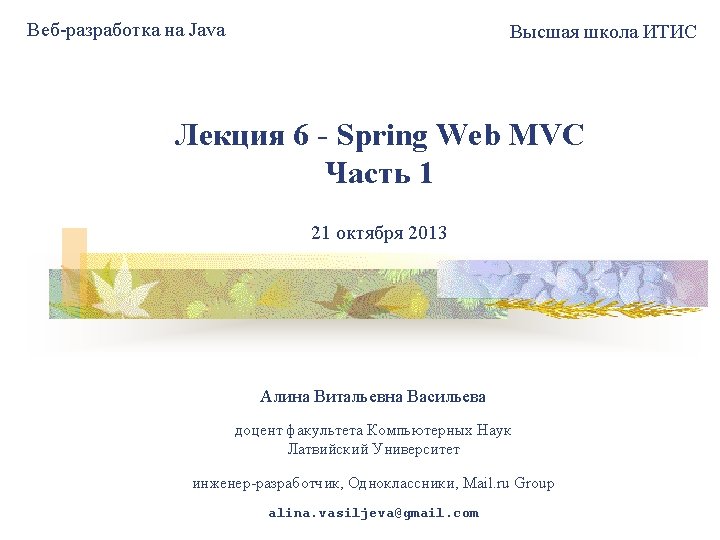
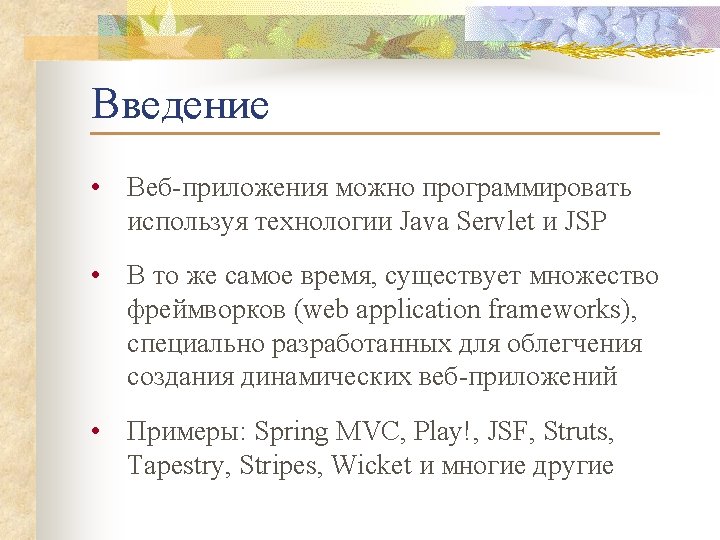
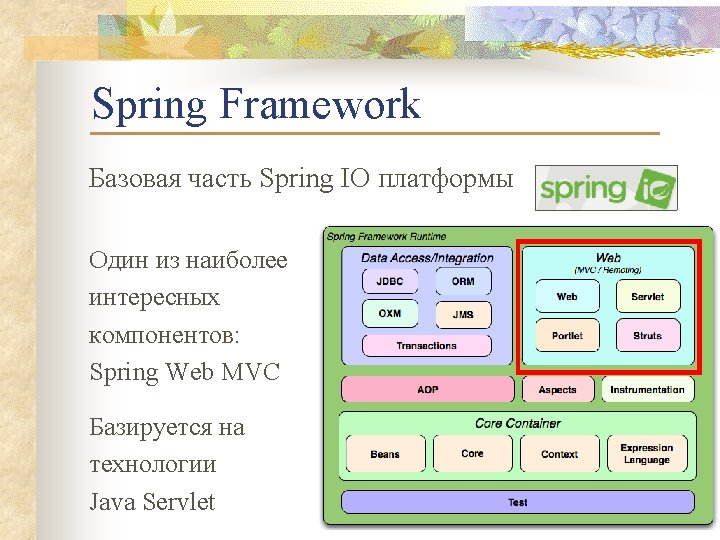
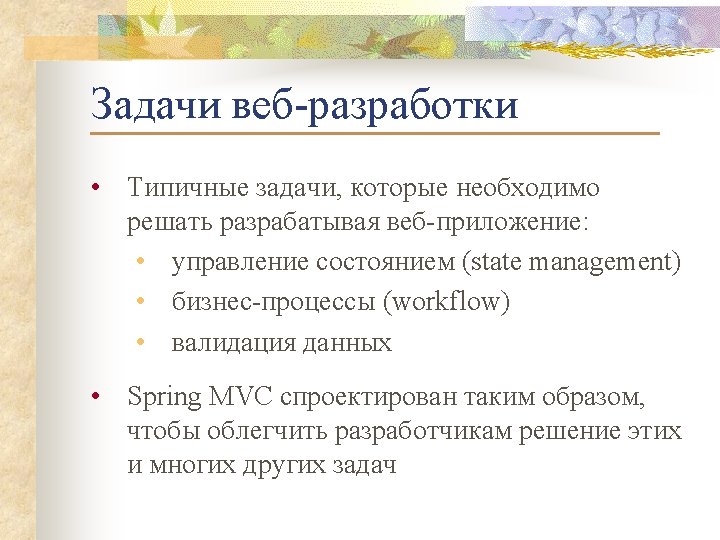
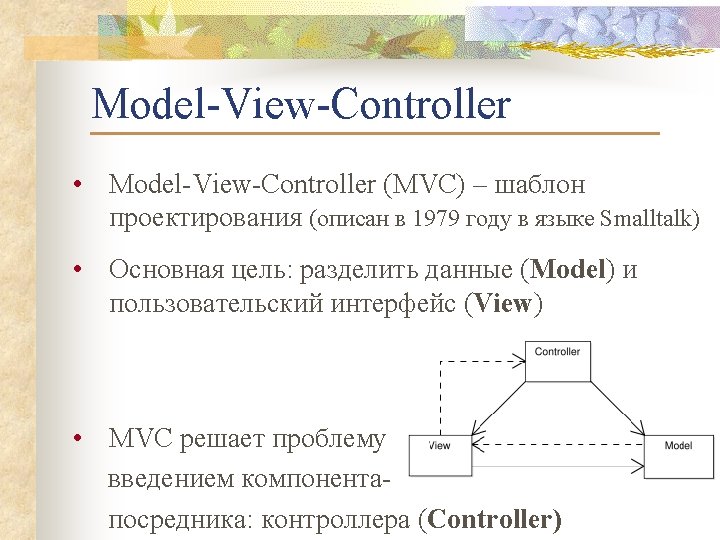
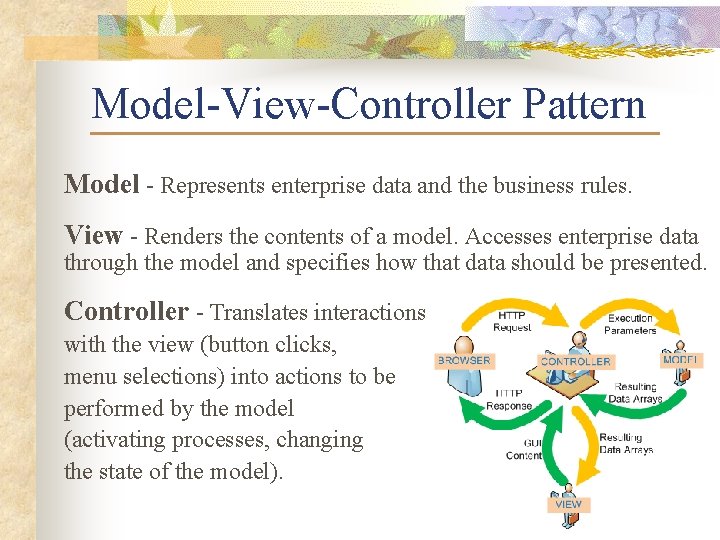
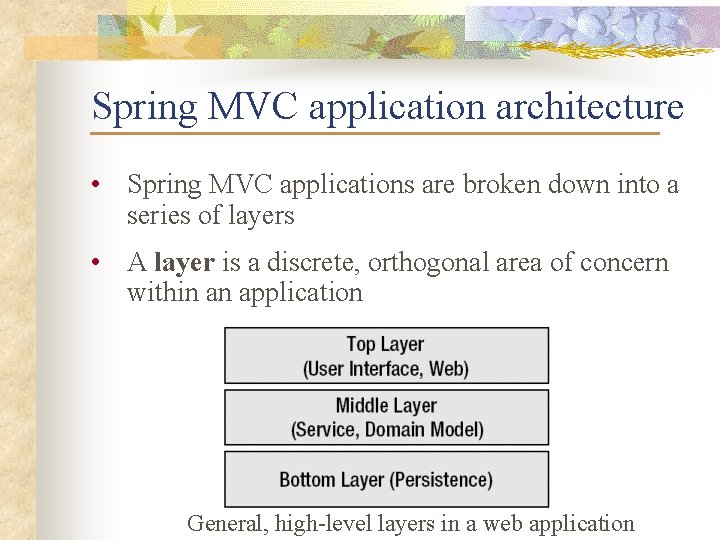
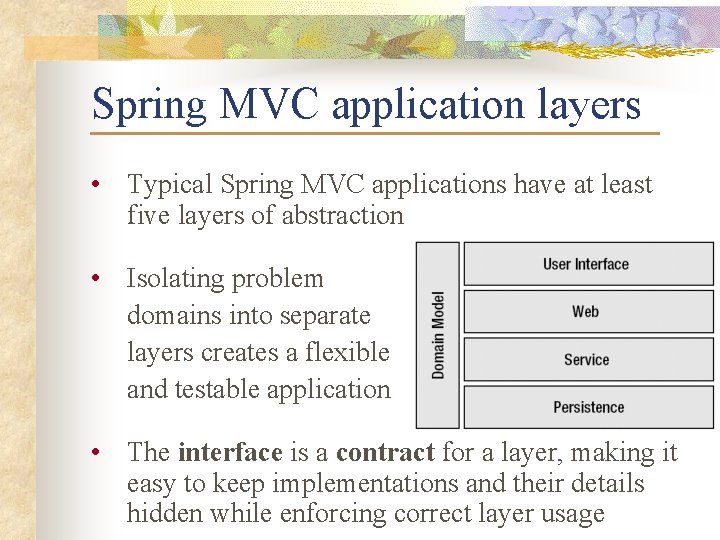
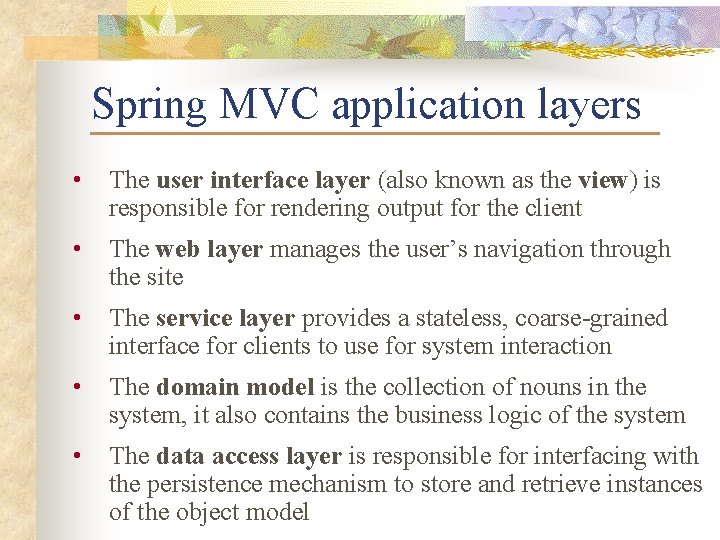
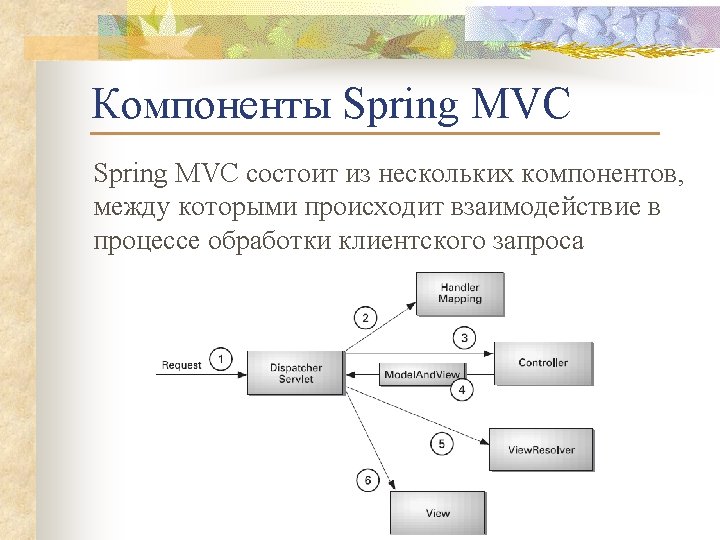
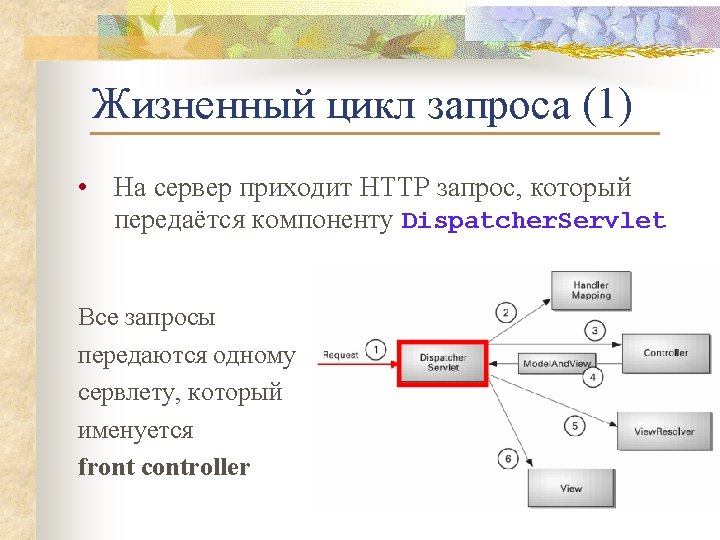
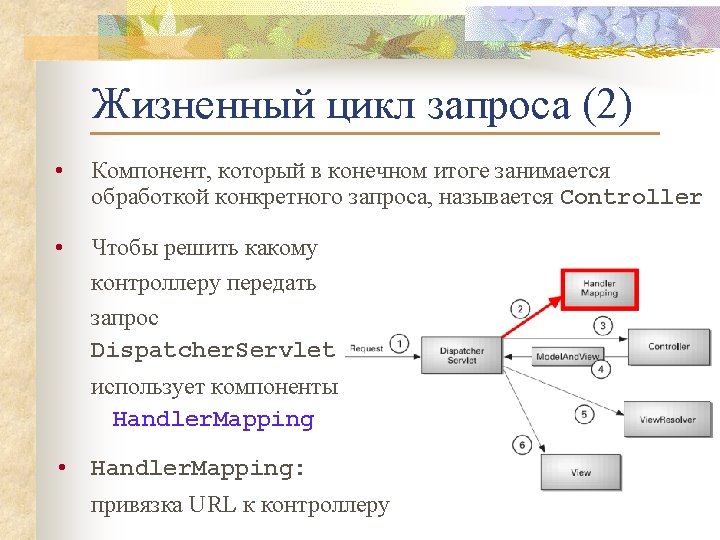
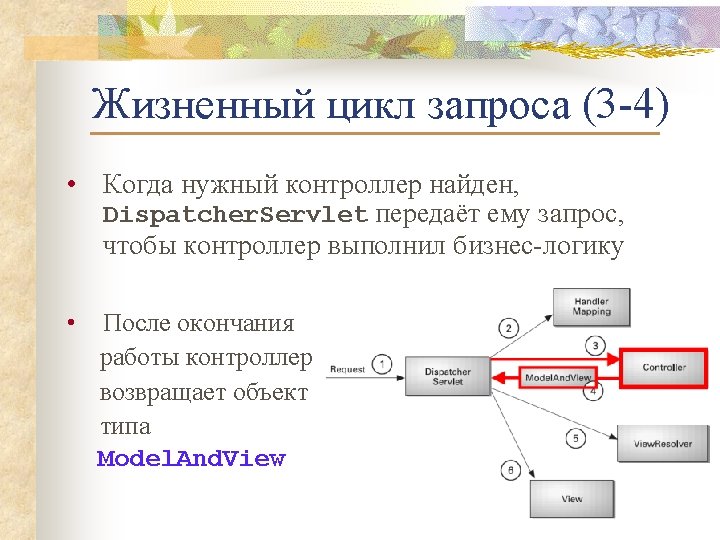
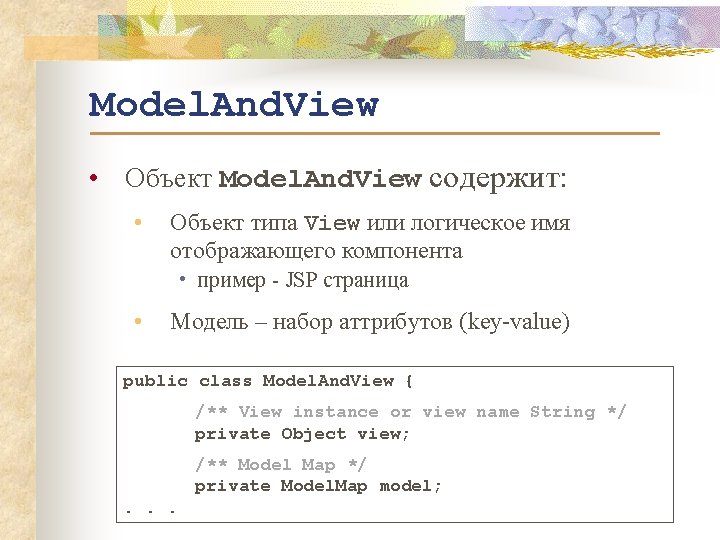
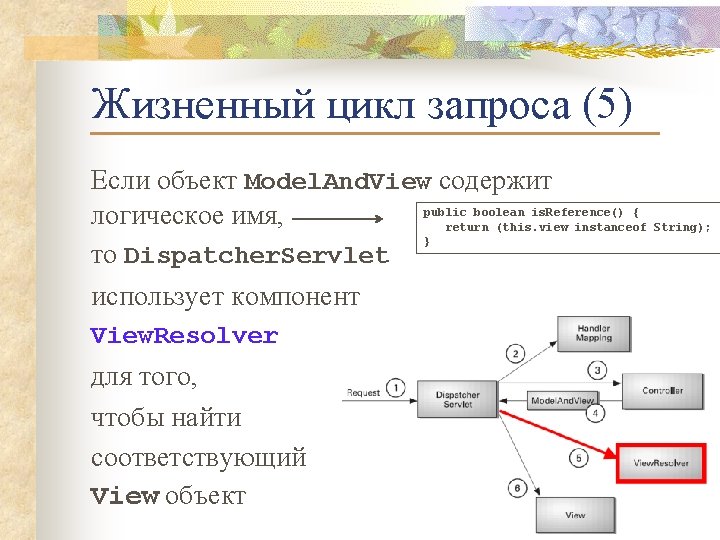
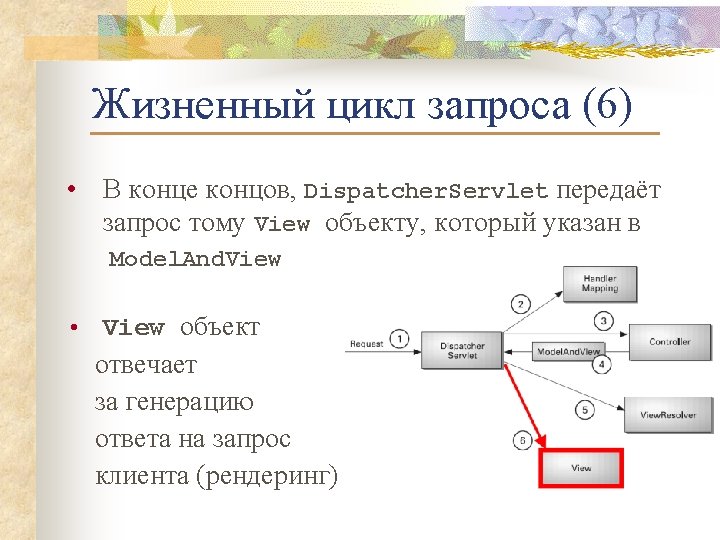
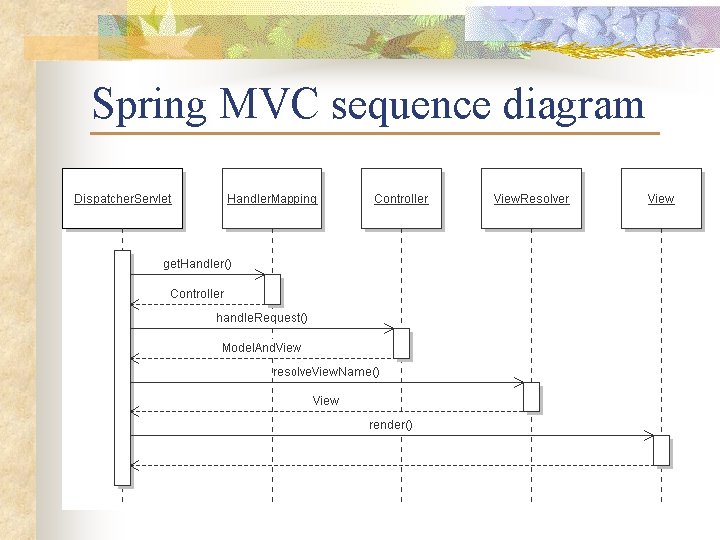
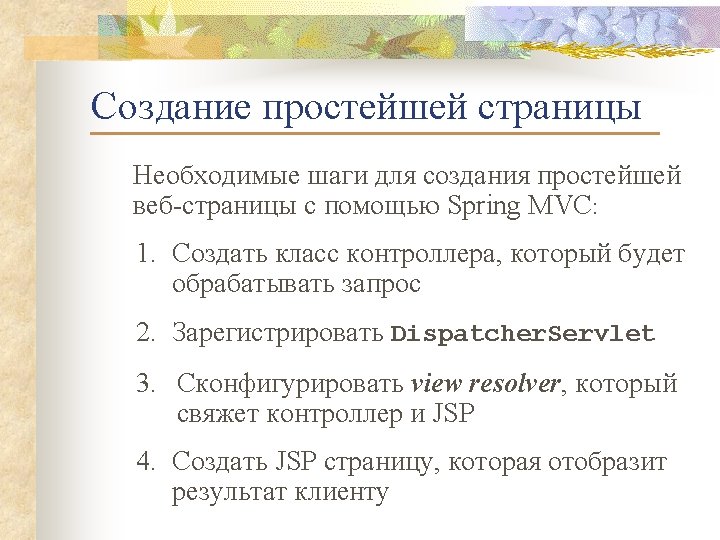
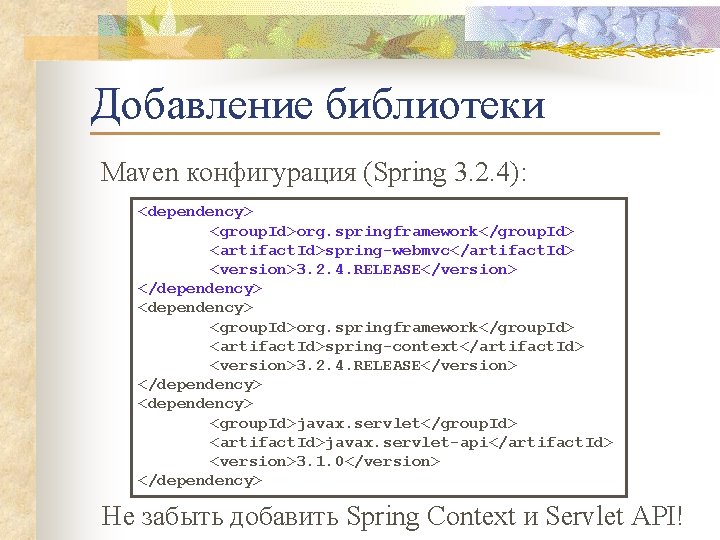
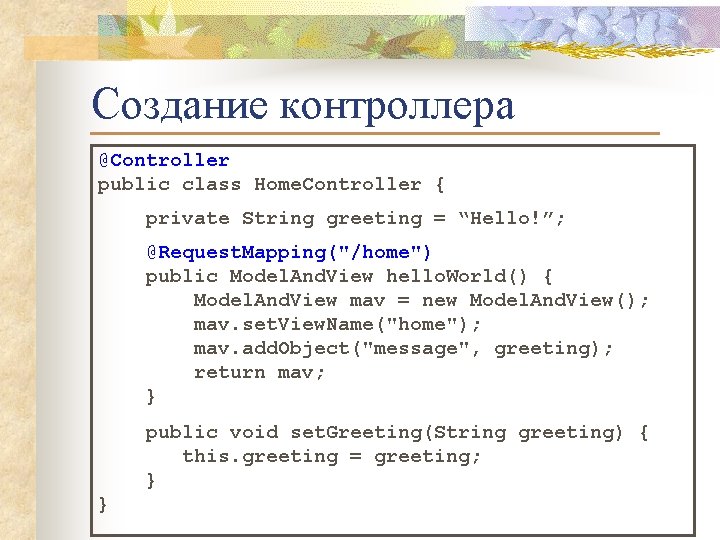
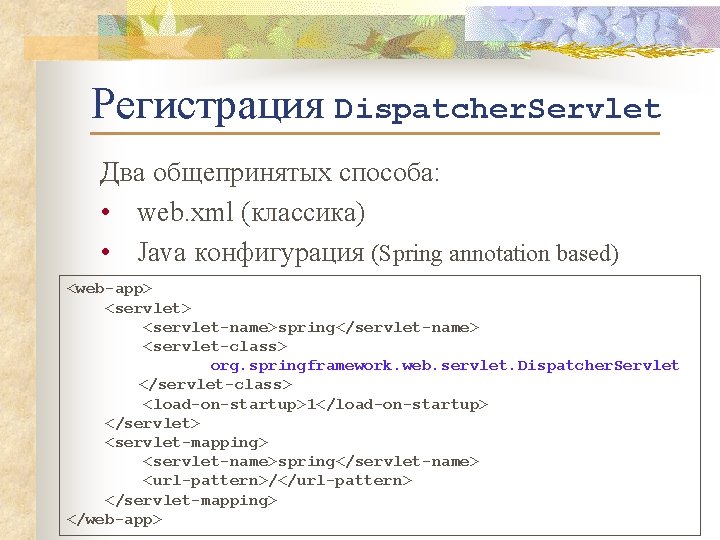
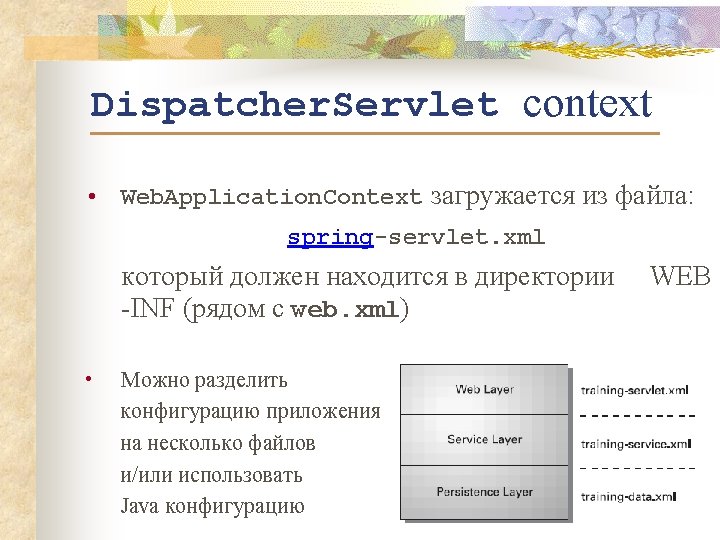
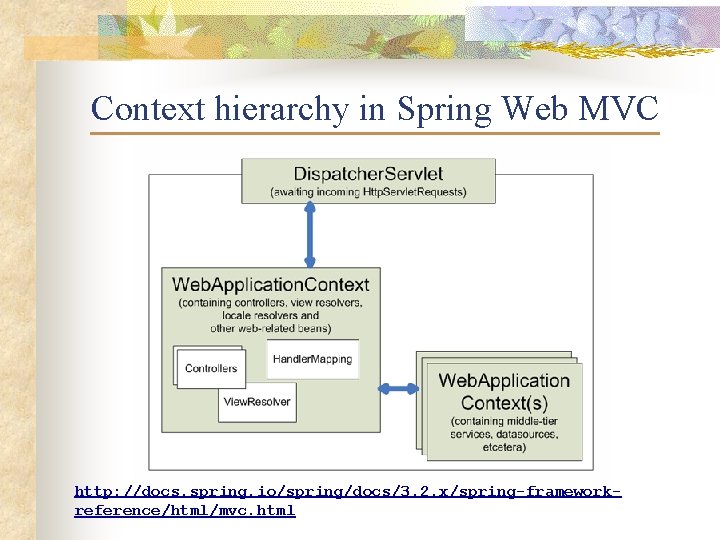
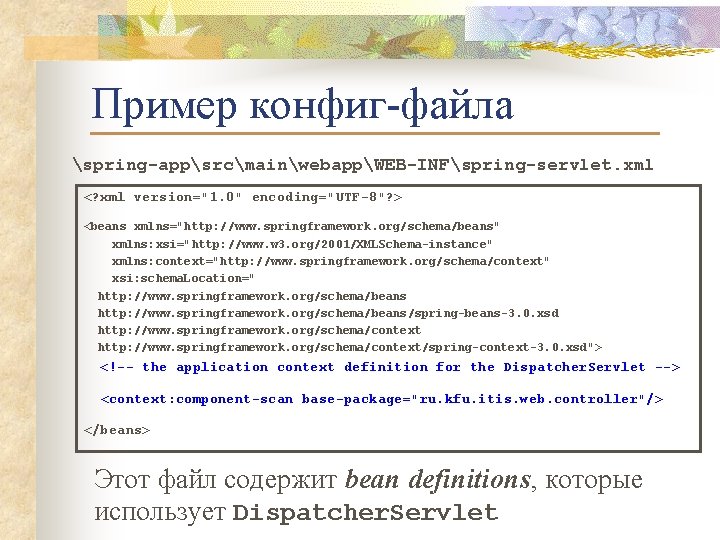
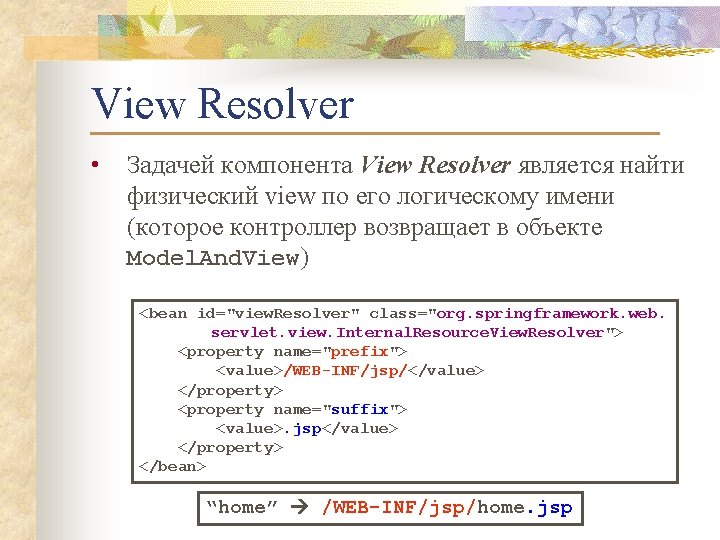
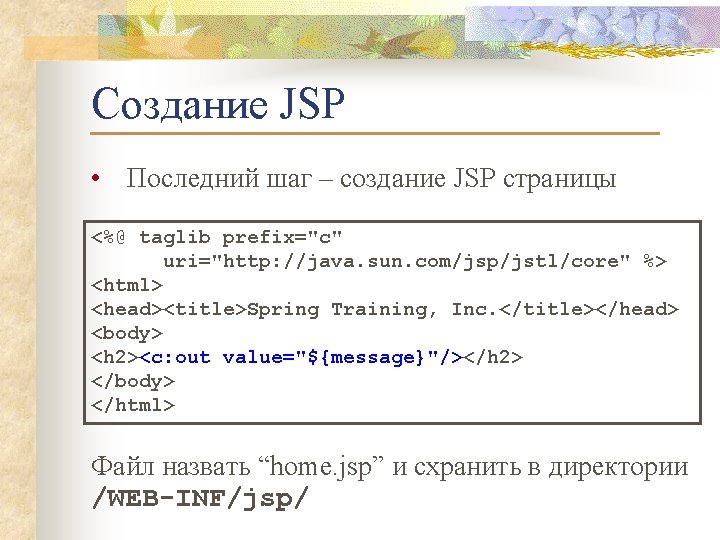
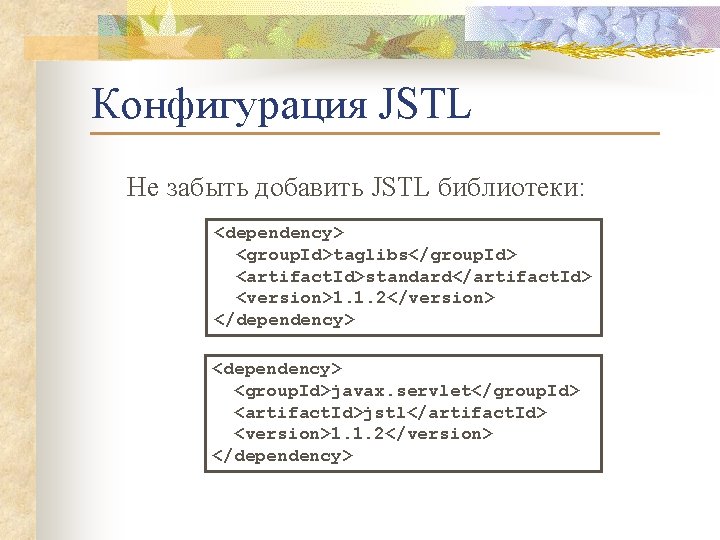
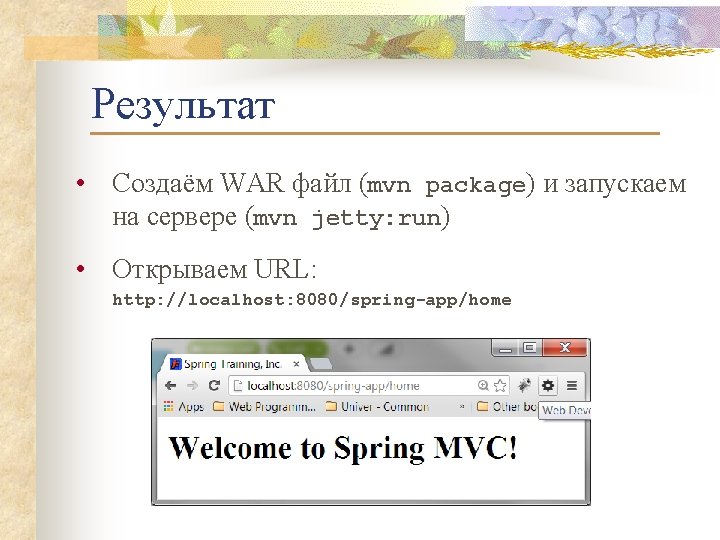
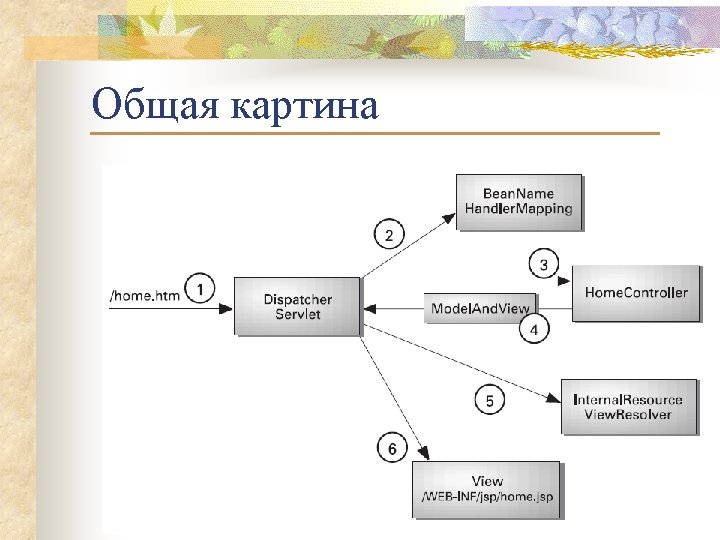
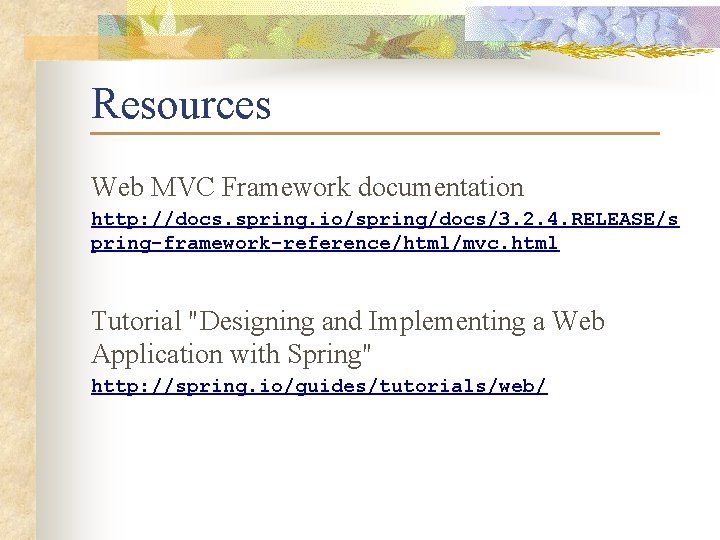
- Slides: 30
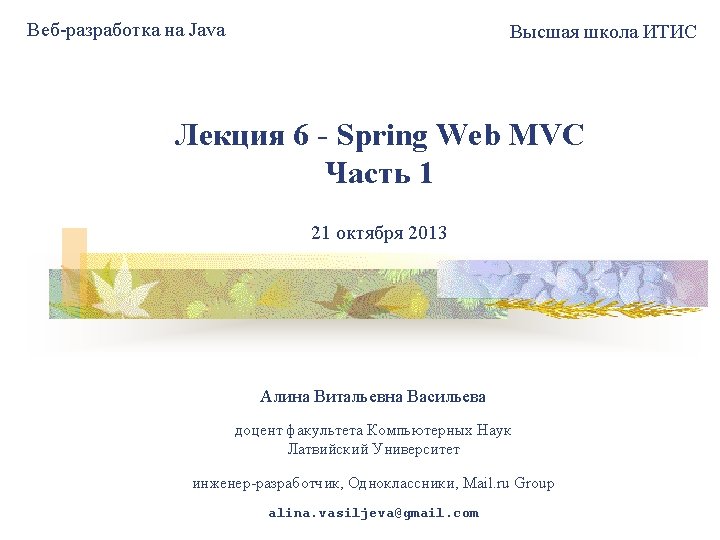
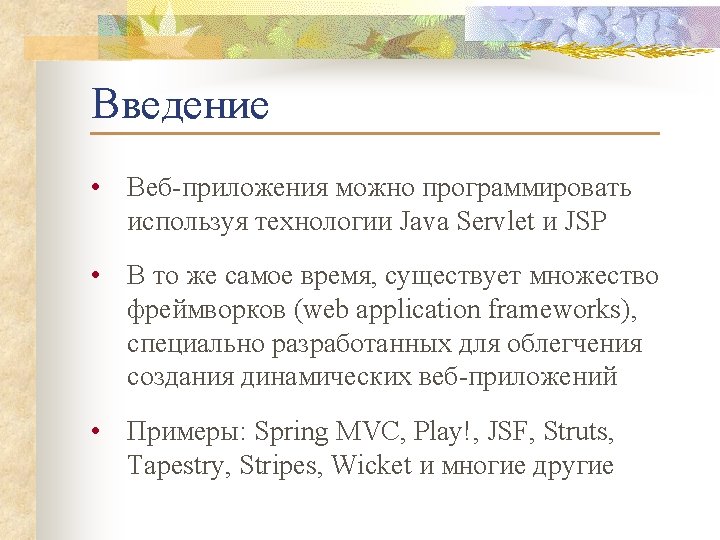
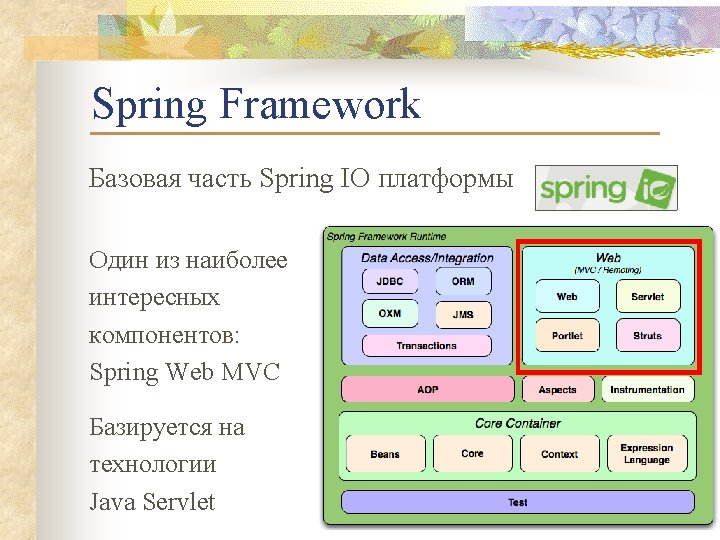
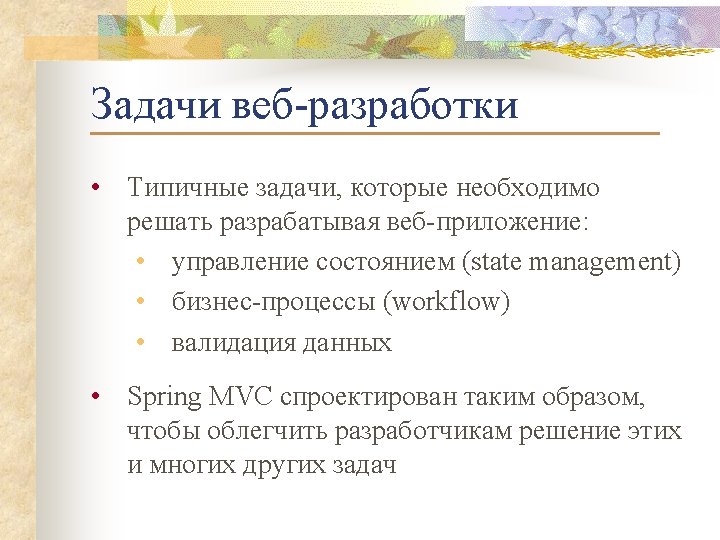
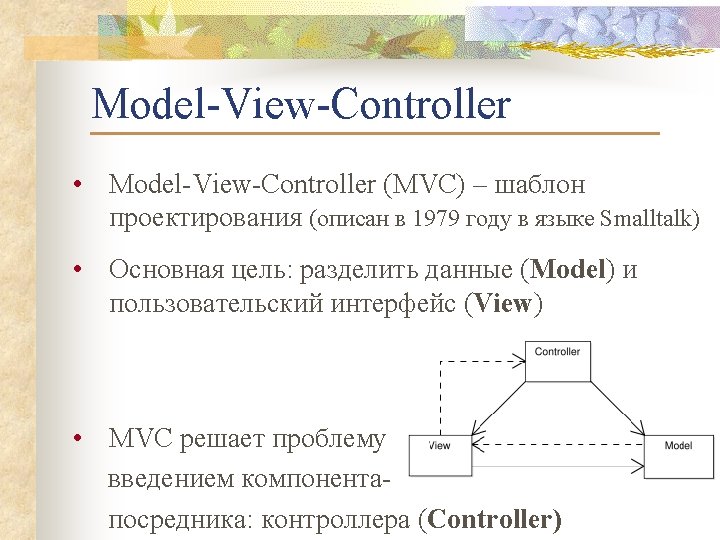
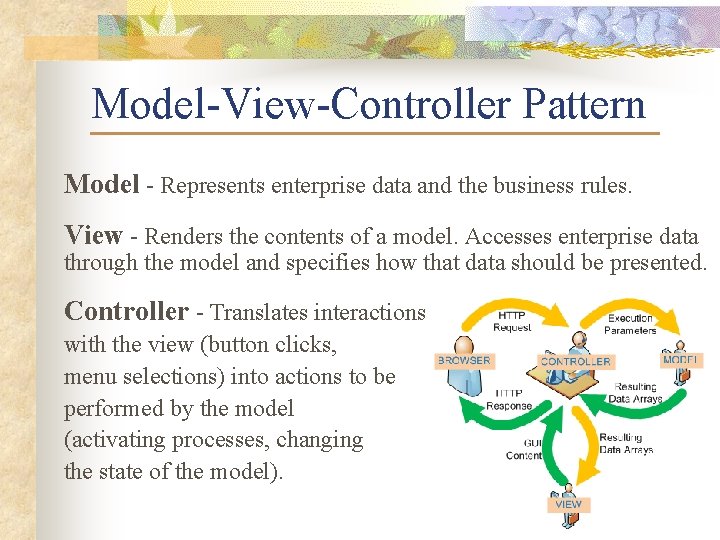
Model-View-Controller Pattern Model - Represents enterprise data and the business rules. View - Renders the contents of a model. Accesses enterprise data through the model and specifies how that data should be presented. Controller - Translates interactions with the view (button clicks, menu selections) into actions to be performed by the model (activating processes, changing the state of the model).
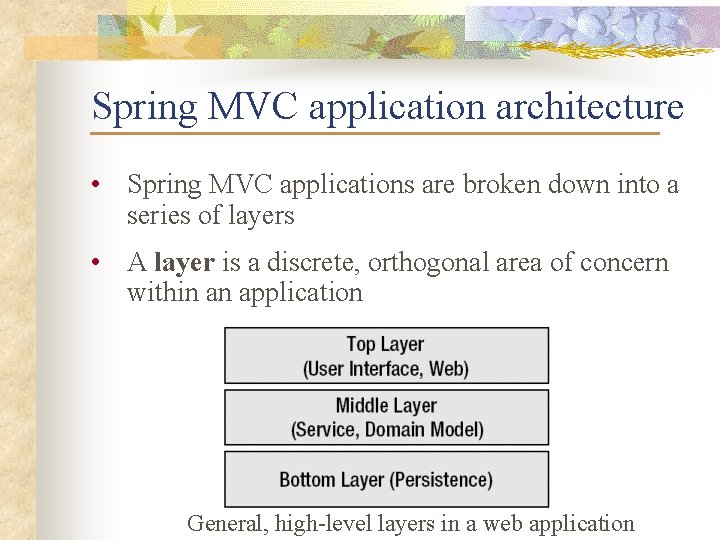
Spring MVC application architecture • Spring MVC applications are broken down into a series of layers • A layer is a discrete, orthogonal area of concern within an application General, high-level layers in a web application
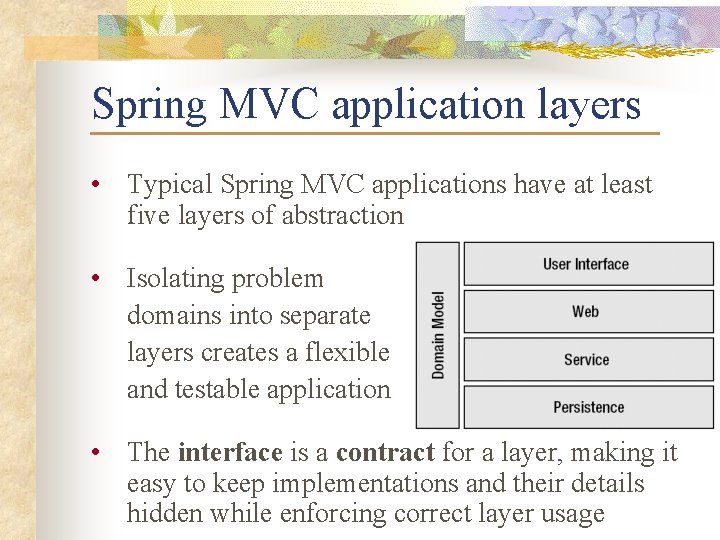
Spring MVC application layers • Typical Spring MVC applications have at least five layers of abstraction • Isolating problem domains into separate layers creates a flexible and testable application • The interface is a contract for a layer, making it easy to keep implementations and their details hidden while enforcing correct layer usage
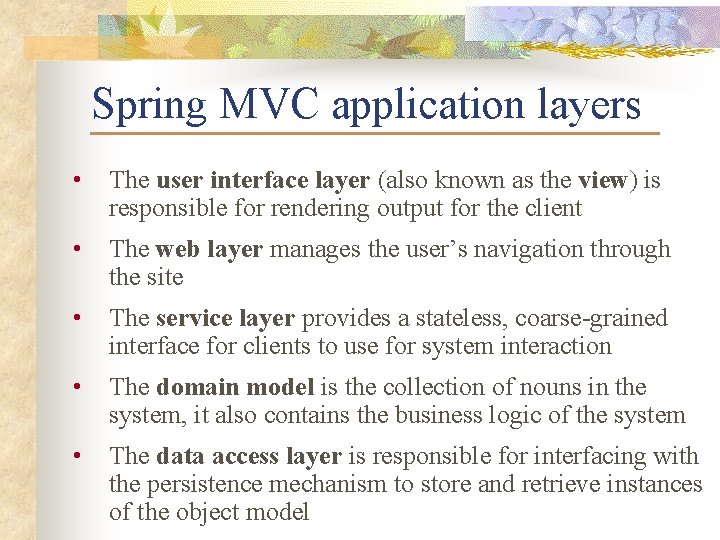
Spring MVC application layers • The user interface layer (also known as the view) is responsible for rendering output for the client • The web layer manages the user’s navigation through the site • The service layer provides a stateless, coarse-grained interface for clients to use for system interaction • The domain model is the collection of nouns in the system, it also contains the business logic of the system • The data access layer is responsible for interfacing with the persistence mechanism to store and retrieve instances of the object model
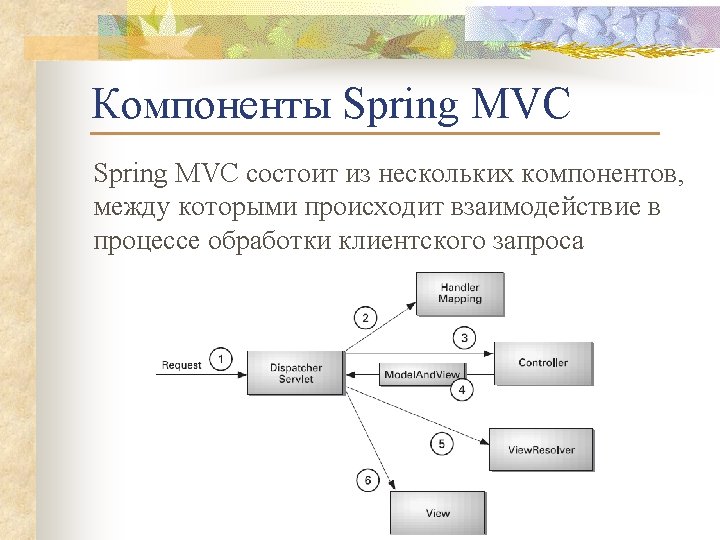
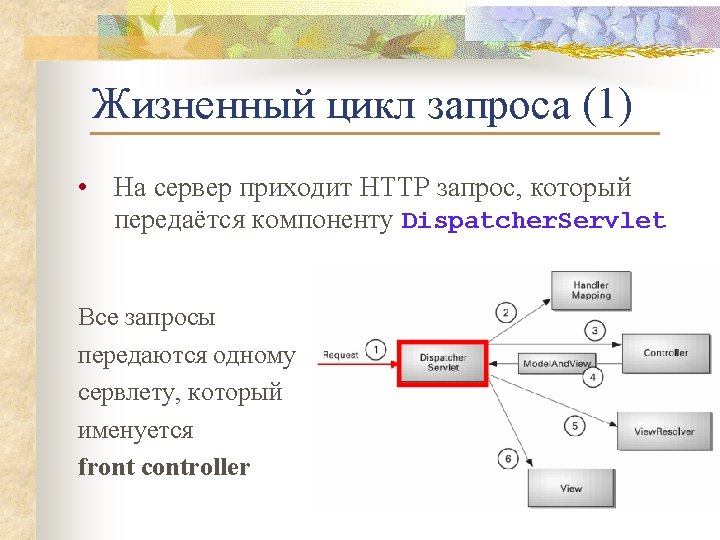
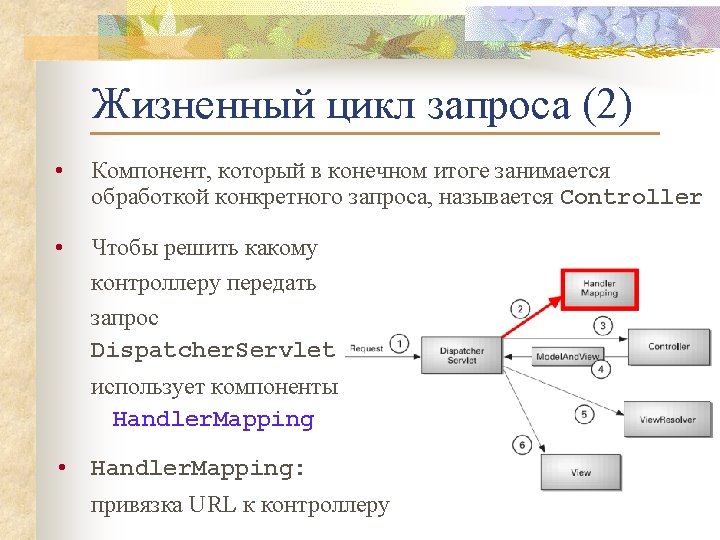
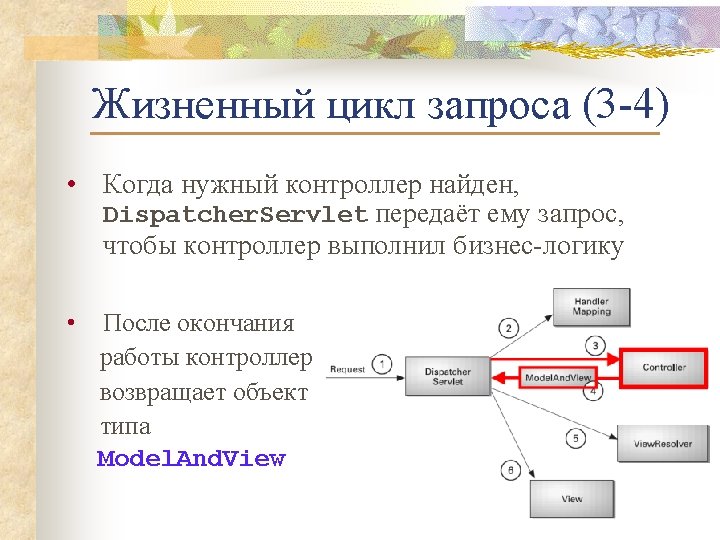
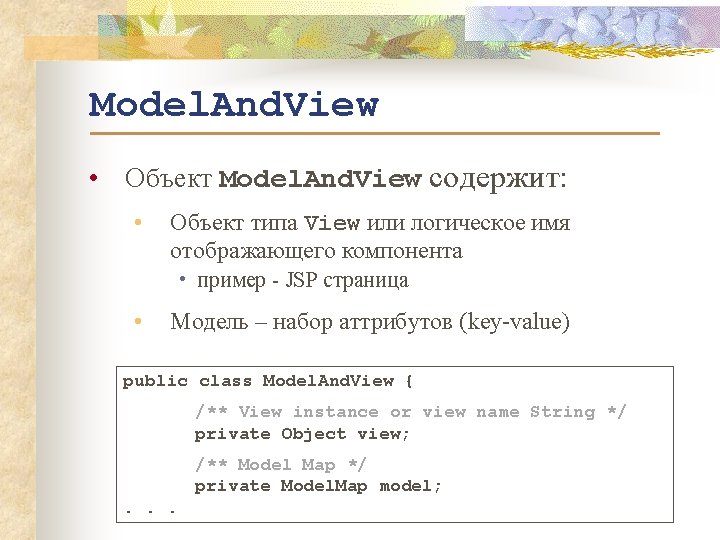
Model. And. View • Объект Model. And. View содержит: • Объект типа View или логическое имя отображающего компонента • пример - JSP страница • Модель – набор аттрибутов (key-value) public class Model. And. View { /** View instance or view name String */ private Object view; /** Model Map */ private Model. Map model; . . .
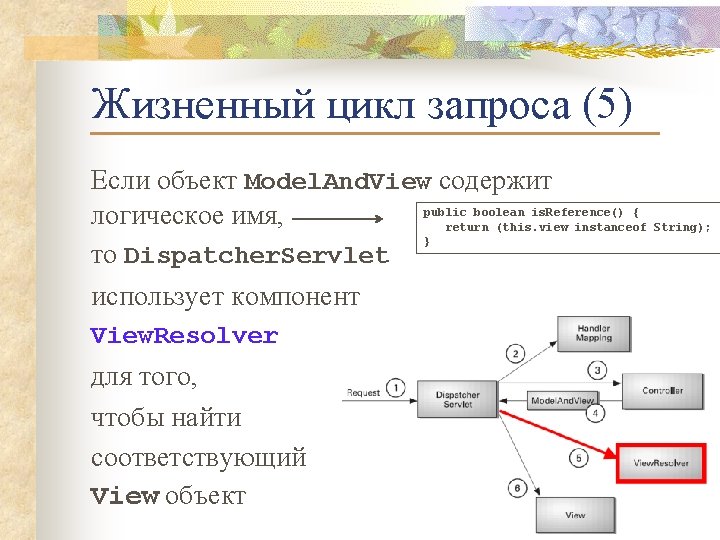
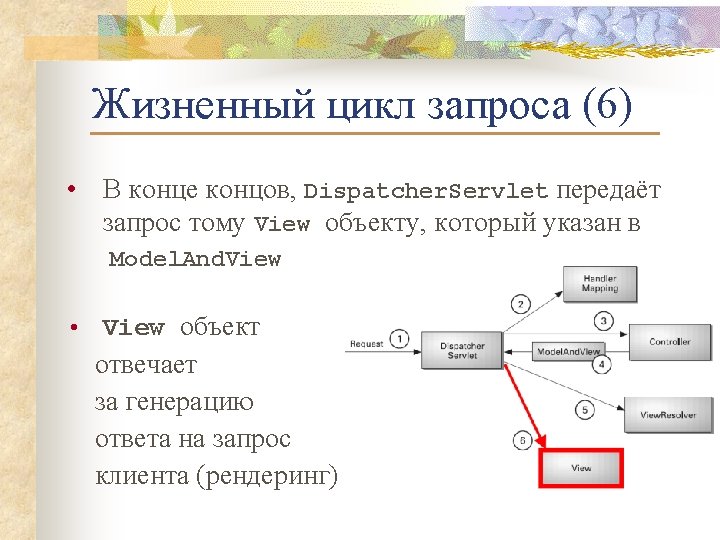
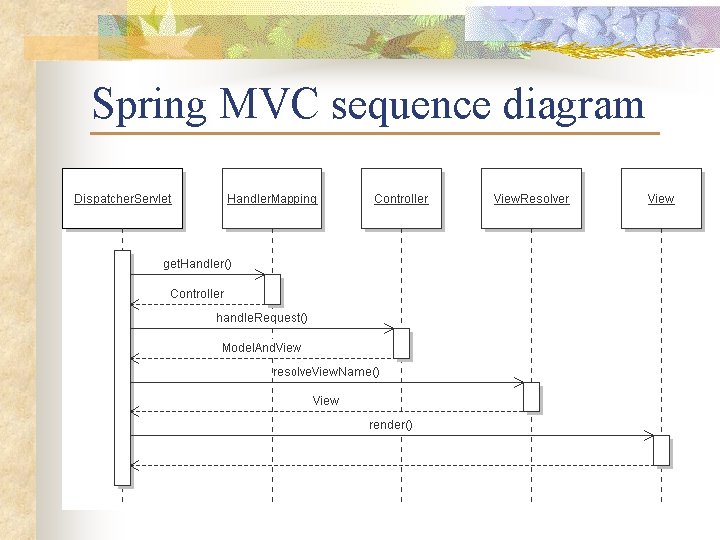
Spring MVC sequence diagram
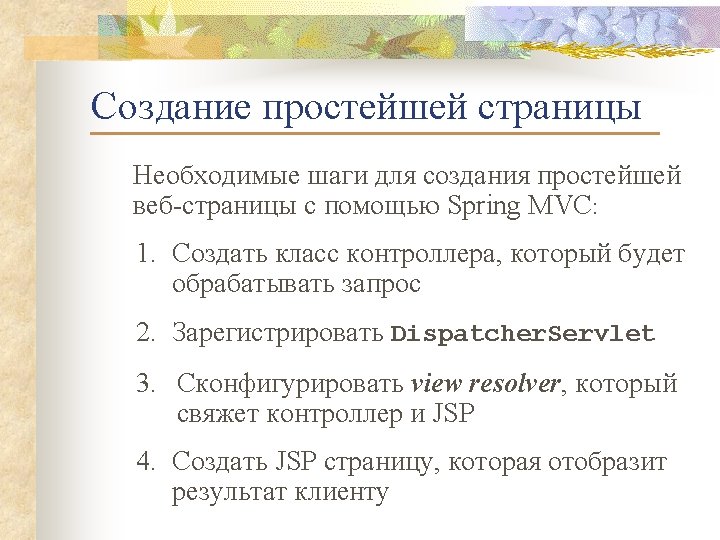
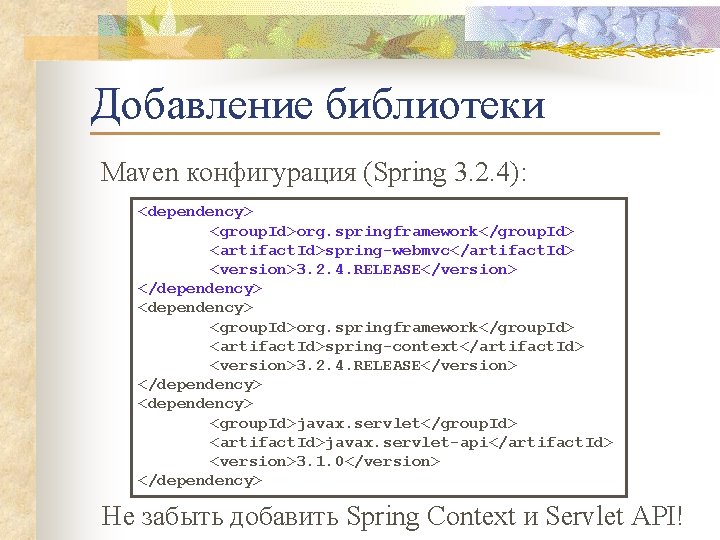
Добавление библиотеки Maven конфигурация (Spring 3. 2. 4): <dependency> <group. Id>org. springframework</group. Id> <artifact. Id>spring-webmvc</artifact. Id> <version>3. 2. 4. RELEASE</version> </dependency> <group. Id>org. springframework</group. Id> <artifact. Id>spring-context</artifact. Id> <version>3. 2. 4. RELEASE</version> </dependency> <group. Id>javax. servlet</group. Id> <artifact. Id>javax. servlet-api</artifact. Id> <version>3. 1. 0</version> </dependency> Не забыть добавить Spring Context и Servlet API!
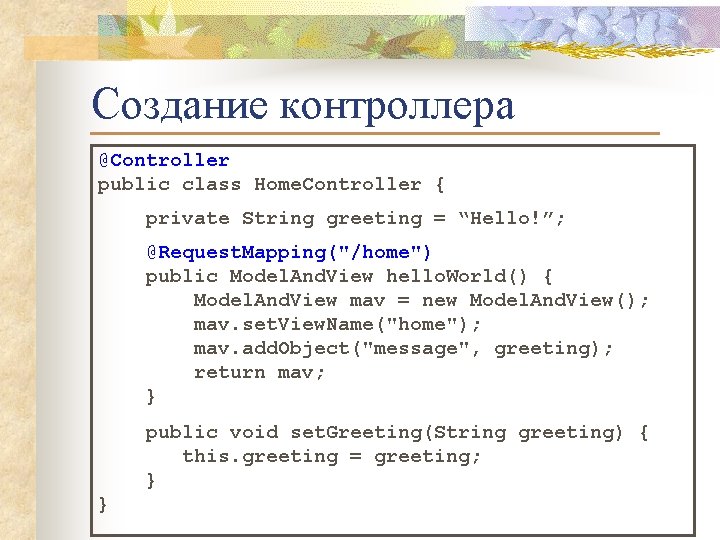
Создание контроллера @Controller public class Home. Controller { private String greeting = “Hello!”; @Request. Mapping("/home") public Model. And. View hello. World() { Model. And. View mav = new Model. And. View(); mav. set. View. Name("home"); mav. add. Object("message", greeting); return mav; } public void set. Greeting(String greeting) { this. greeting = greeting; } }
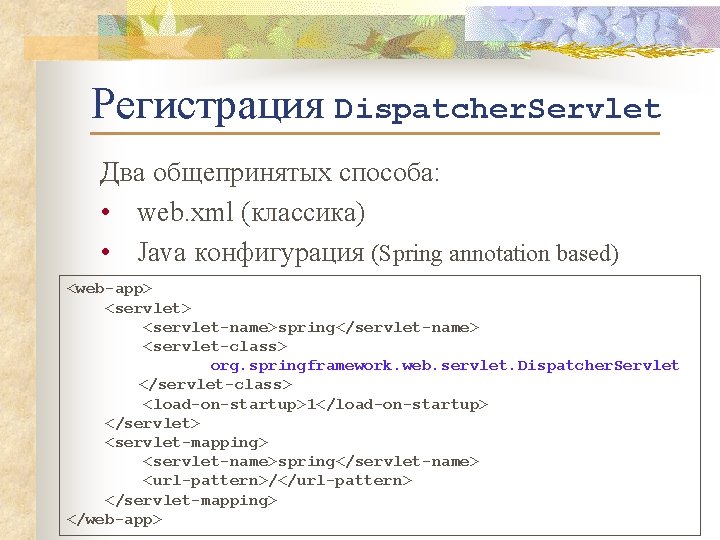
Регистрация Dispatcher. Servlet Два общепринятых способа: • web. xml (классика) • Java конфигурация (Spring annotation based) <web-app> <servlet-name>spring</servlet-name> <servlet-class> org. springframework. web. servlet. Dispatcher. Servlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>spring</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
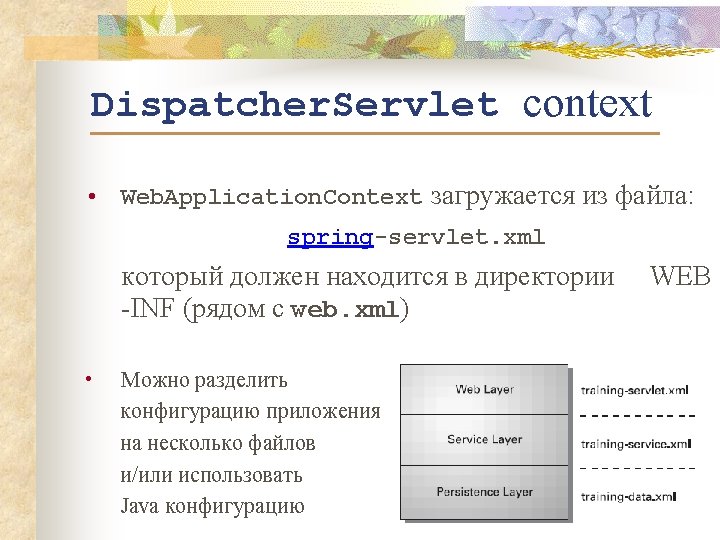
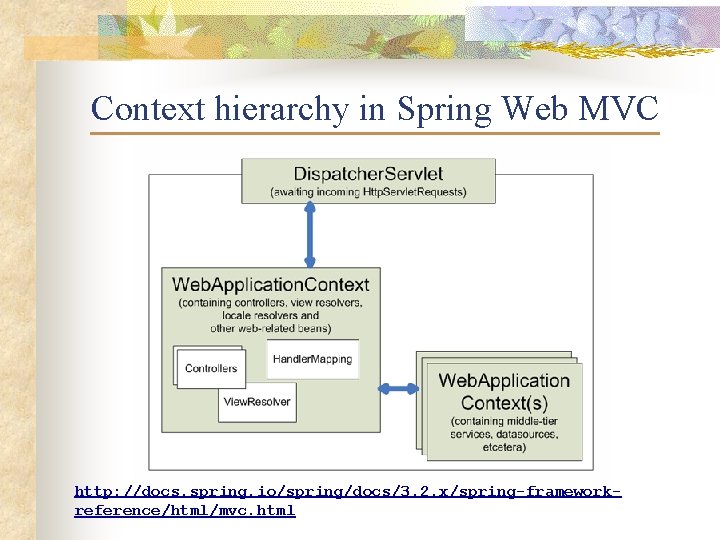
Context hierarchy in Spring Web MVC http: //docs. spring. io/spring/docs/3. 2. x/spring-frameworkreference/html/mvc. html
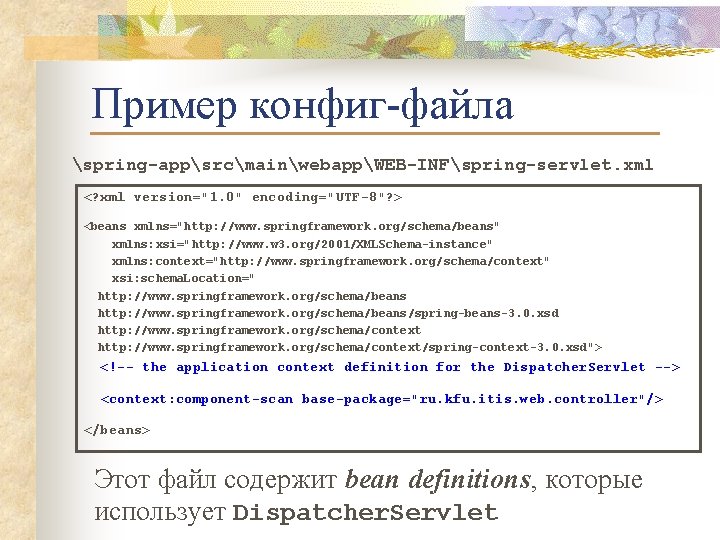
Пример конфиг-файла spring-appsrcmainwebappWEB-INFspring-servlet. xml <? xml version="1. 0" encoding="UTF-8"? > <beans xmlns="http: //www. springframework. org/schema/beans" xmlns: xsi="http: //www. w 3. org/2001/XMLSchema-instance" xmlns: context="http: //www. springframework. org/schema/context" xsi: schema. Location=" http: //www. springframework. org/schema/beans/spring-beans-3. 0. xsd http: //www. springframework. org/schema/context/spring-context-3. 0. xsd"> <!-- the application context definition for the Dispatcher. Servlet --> <context: component-scan base-package="ru. kfu. itis. web. controller"/> </beans> Этот файл содержит bean definitions, которые использует Dispatcher. Servlet
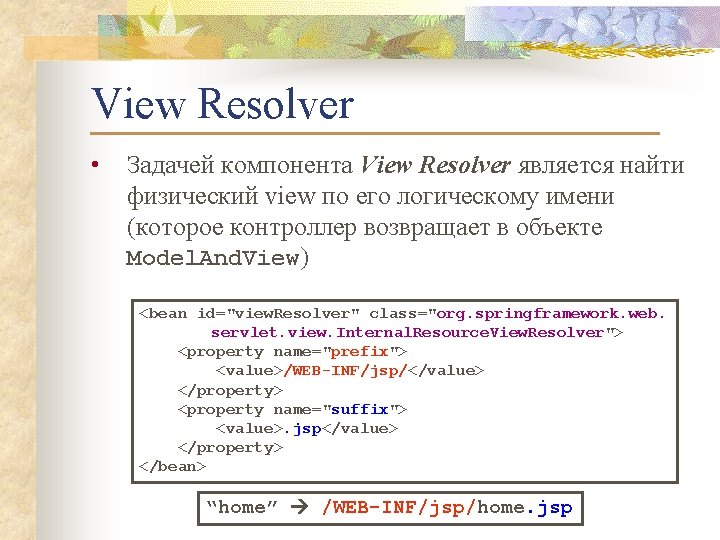
View Resolver • Задачей компонента View Resolver является найти физический view по его логическому имени (которое контроллер возвращает в объекте Model. And. View) <bean id="view. Resolver" class="org. springframework. web. servlet. view. Internal. Resource. View. Resolver"> <property name="prefix"> <value>/WEB-INF/jsp/</value> </property> <property name="suffix"> <value>. jsp</value> </property> </bean> “home” /WEB-INF/jsp/home. jsp
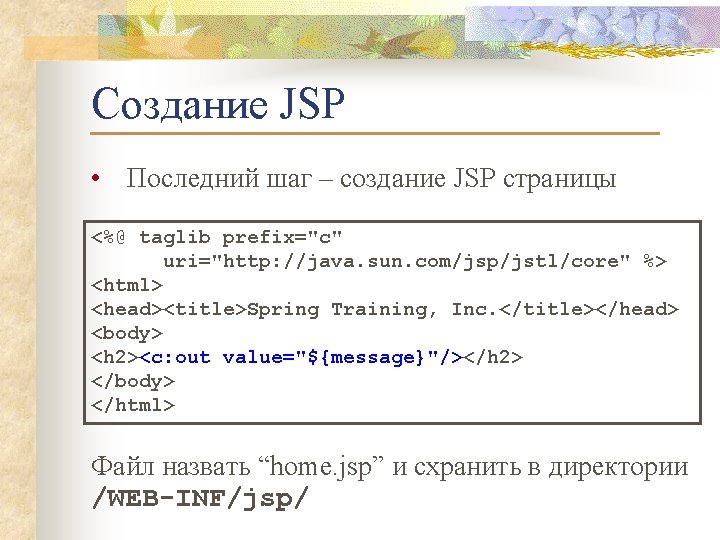
Создание JSP • Последний шаг – создание JSP страницы <%@ taglib prefix="c" uri="http: //java. sun. com/jsp/jstl/core" %> <html> <head><title>Spring Training, Inc. </title></head> <body> <h 2><c: out value="${message}"/></h 2> </body> </html> Файл назвать “home. jsp” и схранить в директории /WEB-INF/jsp/
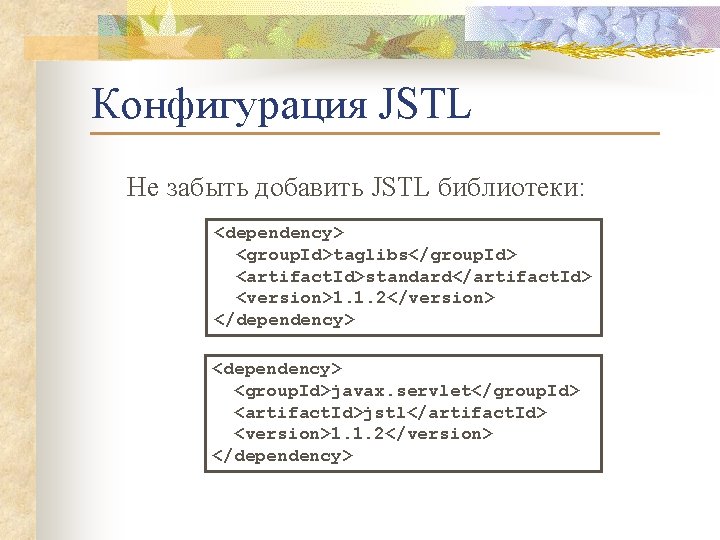
Конфигурация JSTL Не забыть добавить JSTL библиотеки: <dependency> <group. Id>taglibs</group. Id> <artifact. Id>standard</artifact. Id> <version>1. 1. 2</version> </dependency> <group. Id>javax. servlet</group. Id> <artifact. Id>jstl</artifact. Id> <version>1. 1. 2</version> </dependency>
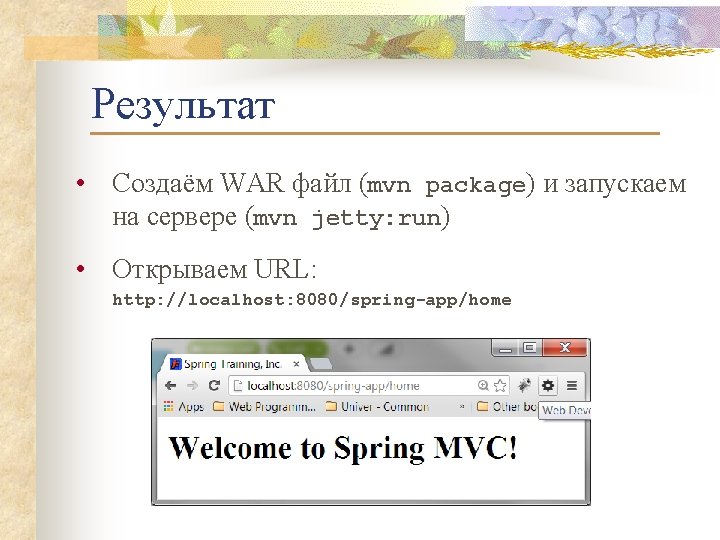
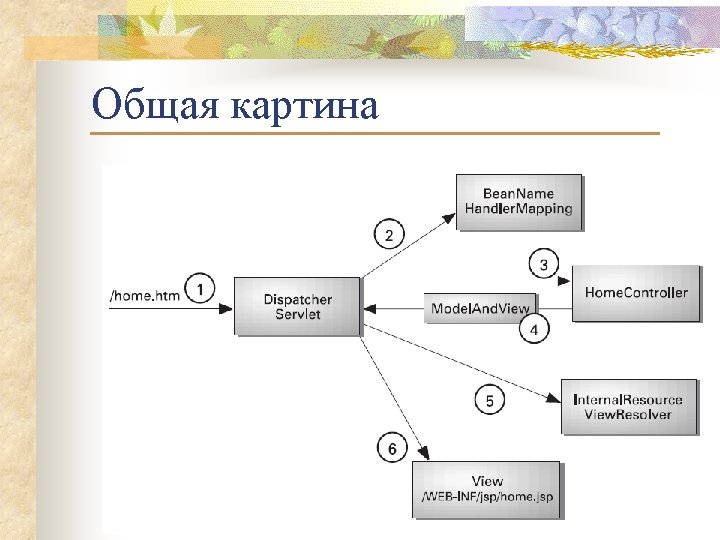
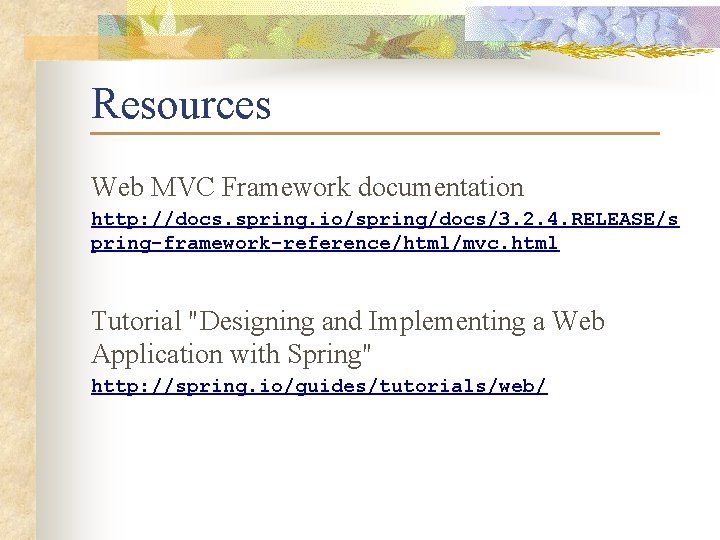
Resources Web MVC Framework documentation http: //docs. spring. io/spring/docs/3. 2. 4. RELEASE/s pring-framework-reference/html/mvc. html Tutorial "Designing and Implementing a Web Application with Spring" http: //spring. io/guides/tutorials/web/