Memory Management Int List List header new Int
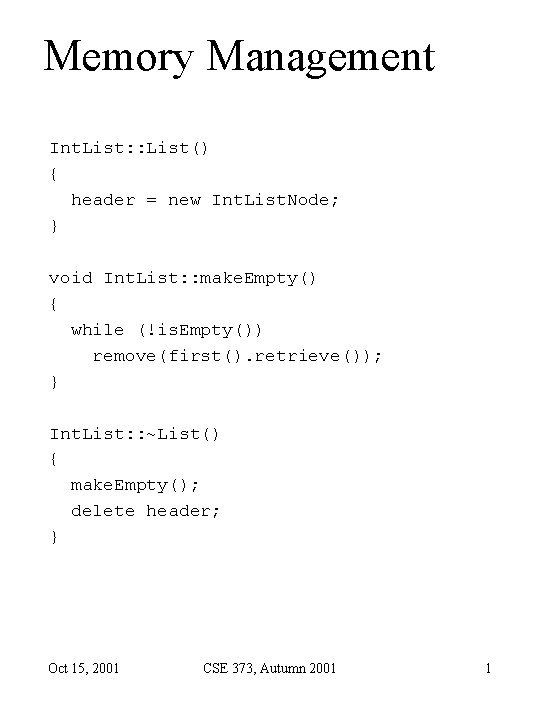
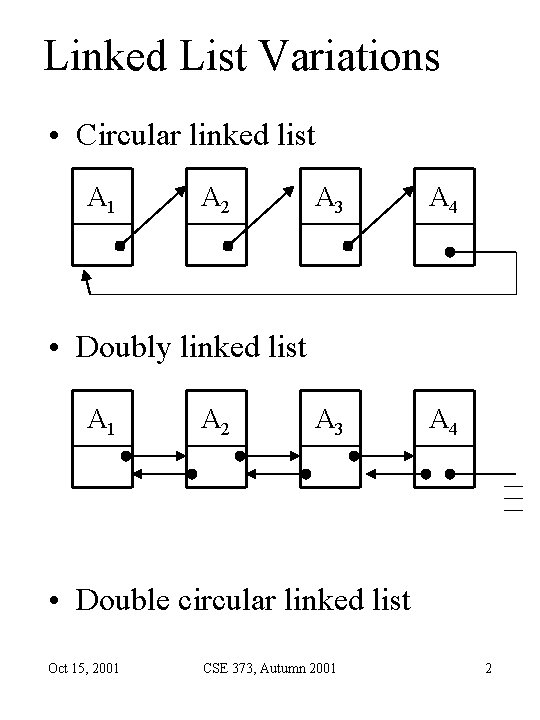
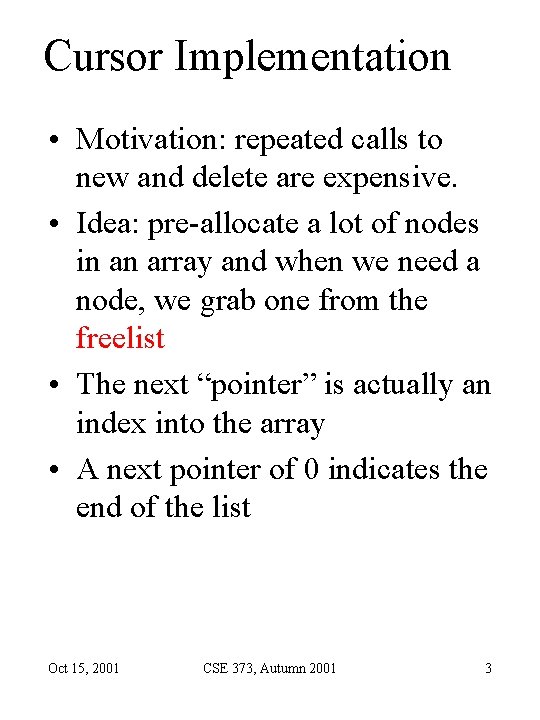
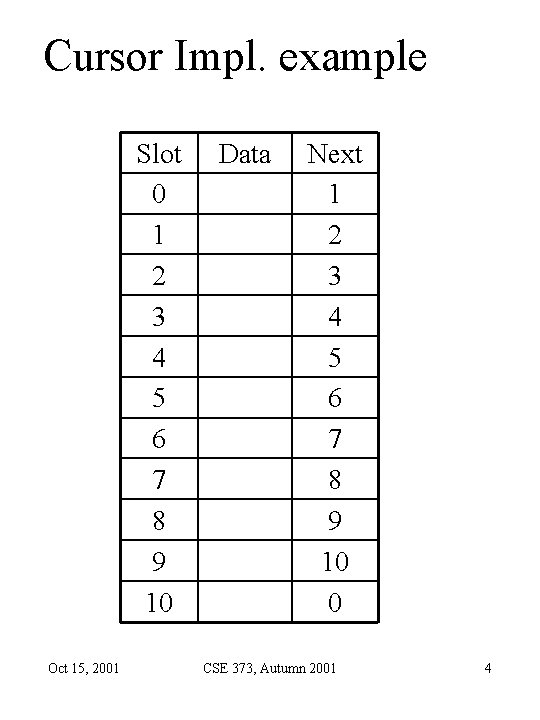
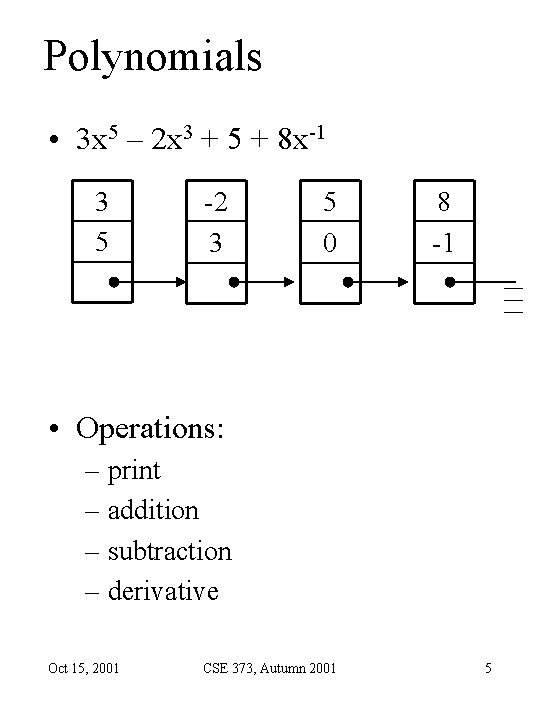
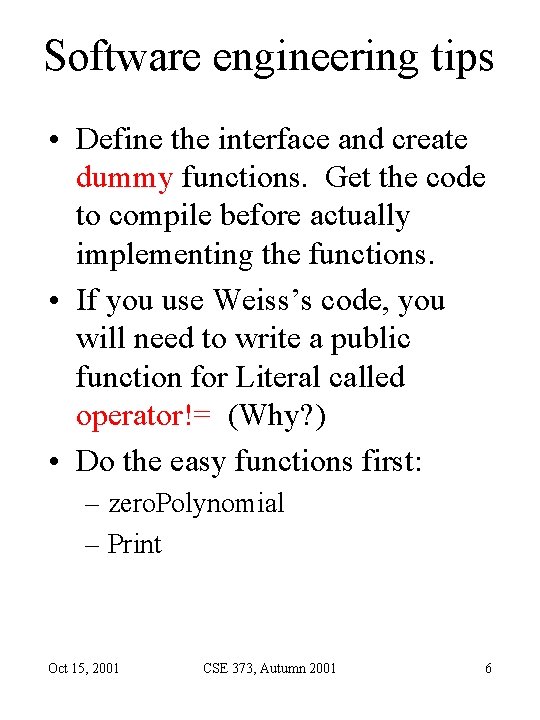
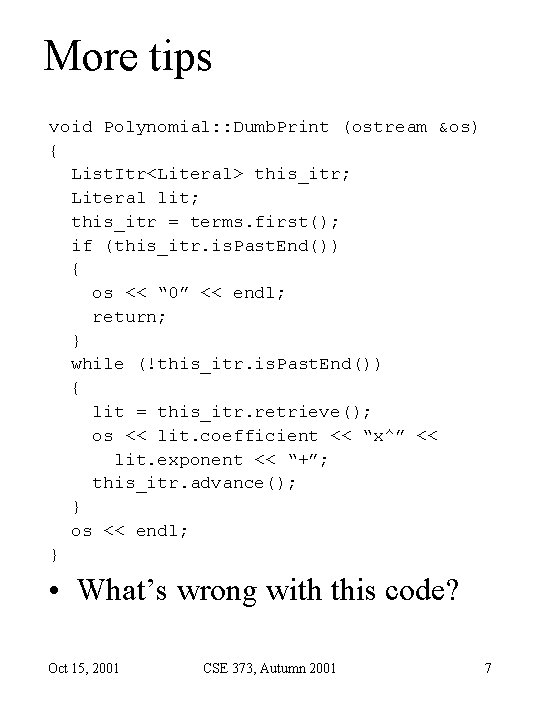
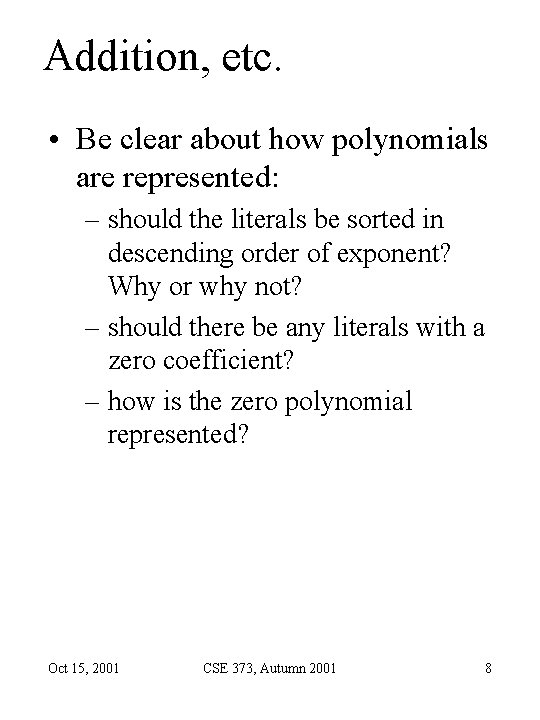
- Slides: 8
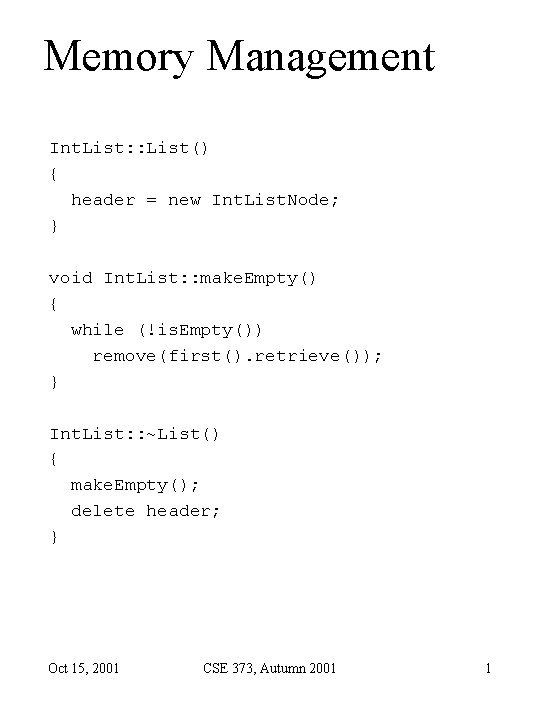
Memory Management Int. List: : List() { header = new Int. List. Node; } void Int. List: : make. Empty() { while (!is. Empty()) remove(first(). retrieve()); } Int. List: : ~List() { make. Empty(); delete header; } Oct 15, 2001 CSE 373, Autumn 2001 1
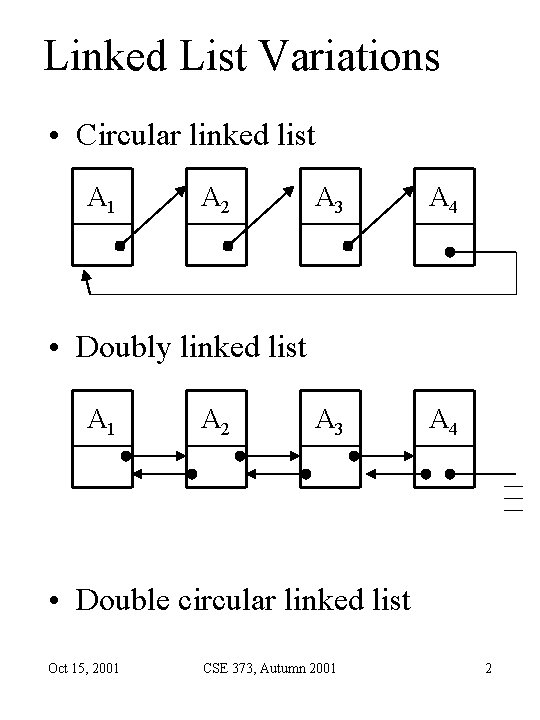
Linked List Variations • Circular linked list A 1 A 2 A 3 A 4 • Doubly linked list A 1 A 2 • Double circular linked list Oct 15, 2001 CSE 373, Autumn 2001 2
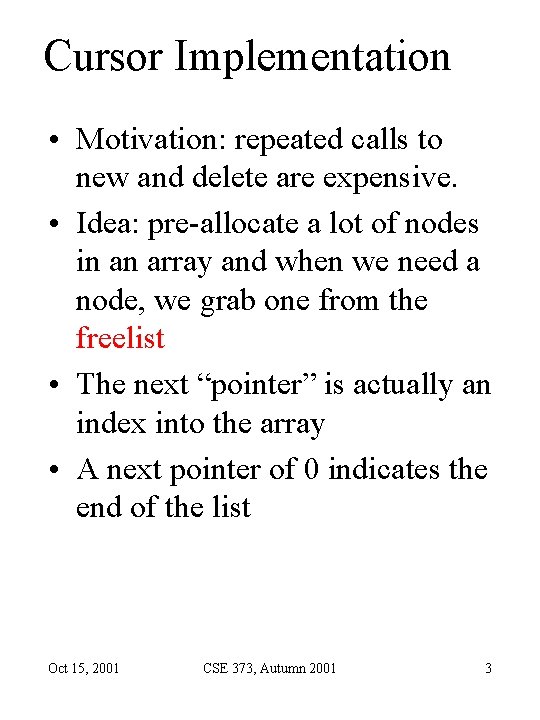
Cursor Implementation • Motivation: repeated calls to new and delete are expensive. • Idea: pre-allocate a lot of nodes in an array and when we need a node, we grab one from the freelist • The next “pointer” is actually an index into the array • A next pointer of 0 indicates the end of the list Oct 15, 2001 CSE 373, Autumn 2001 3
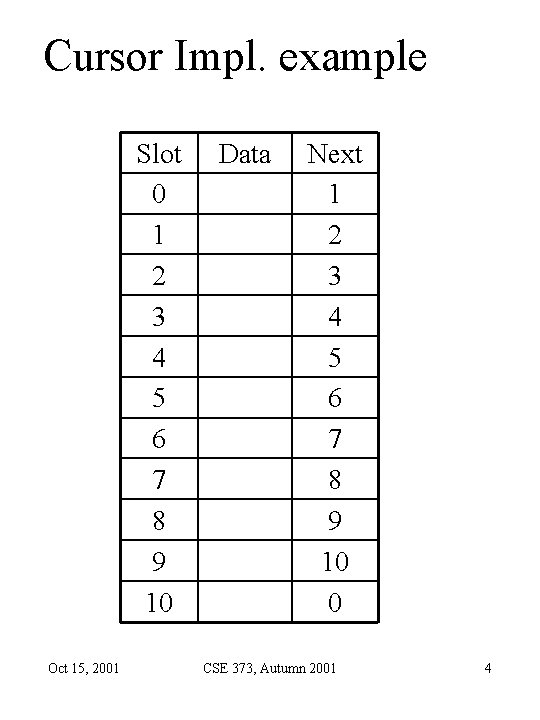
Cursor Impl. example Slot 0 1 2 3 4 5 6 7 8 9 10 Oct 15, 2001 Data Next 1 2 3 4 5 6 7 8 9 10 0 CSE 373, Autumn 2001 4
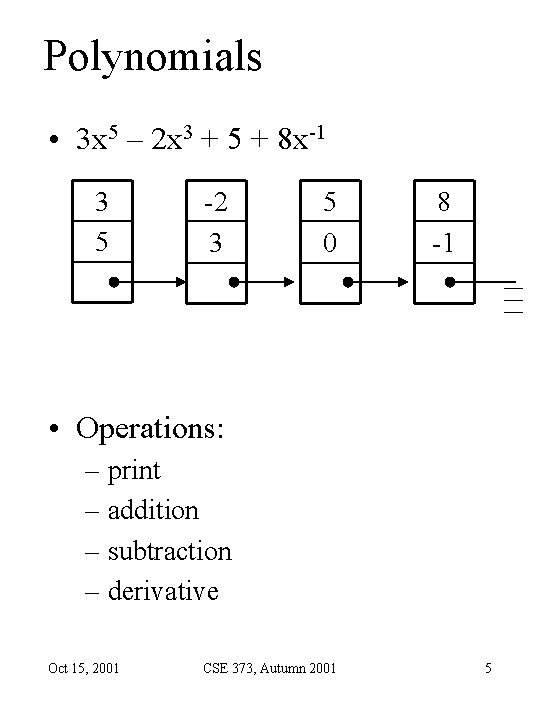
Polynomials • 3 x 5 – 2 x 3 + 5 + 8 x-1 3 5 -2 3 5 0 8 -1 • Operations: – print – addition – subtraction – derivative Oct 15, 2001 CSE 373, Autumn 2001 5
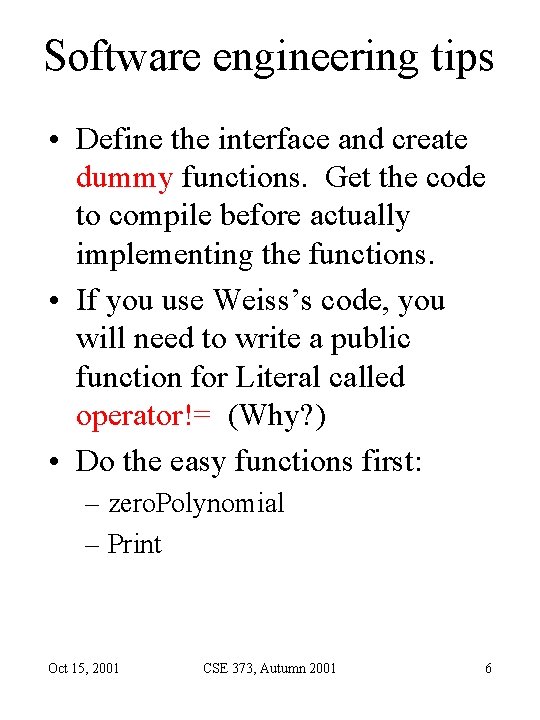
Software engineering tips • Define the interface and create dummy functions. Get the code to compile before actually implementing the functions. • If you use Weiss’s code, you will need to write a public function for Literal called operator!= (Why? ) • Do the easy functions first: – zero. Polynomial – Print Oct 15, 2001 CSE 373, Autumn 2001 6
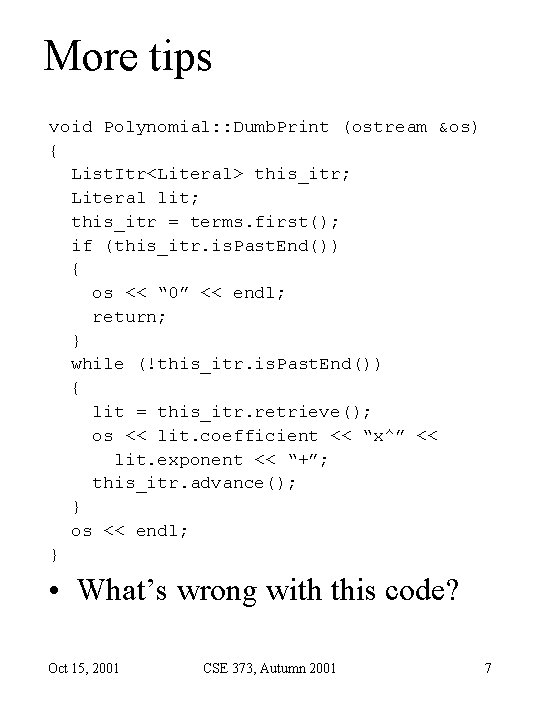
More tips void Polynomial: : Dumb. Print (ostream &os) { List. Itr<Literal> this_itr; Literal lit; this_itr = terms. first(); if (this_itr. is. Past. End()) { os << “ 0” << endl; return; } while (!this_itr. is. Past. End()) { lit = this_itr. retrieve(); os << lit. coefficient << “x^” << lit. exponent << “+”; this_itr. advance(); } os << endl; } • What’s wrong with this code? Oct 15, 2001 CSE 373, Autumn 2001 7
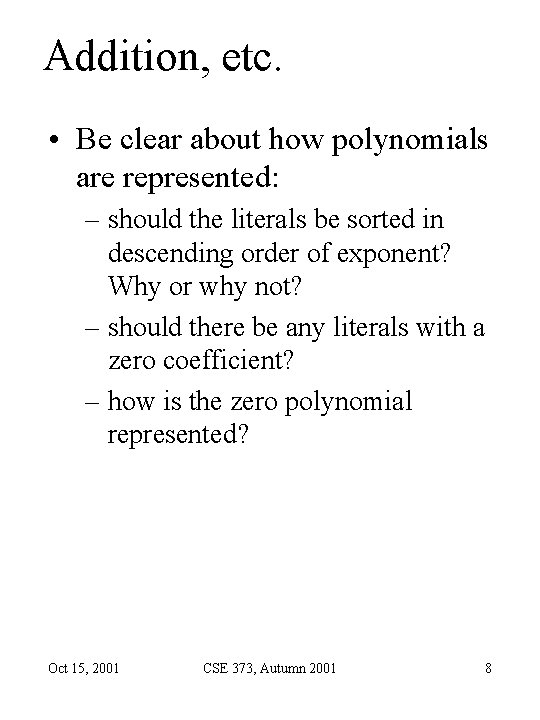
Addition, etc. • Be clear about how polynomials are represented: – should the literals be sorted in descending order of exponent? Why or why not? – should there be any literals with a zero coefficient? – how is the zero polynomial represented? Oct 15, 2001 CSE 373, Autumn 2001 8