Memory Management How memory is used to hold
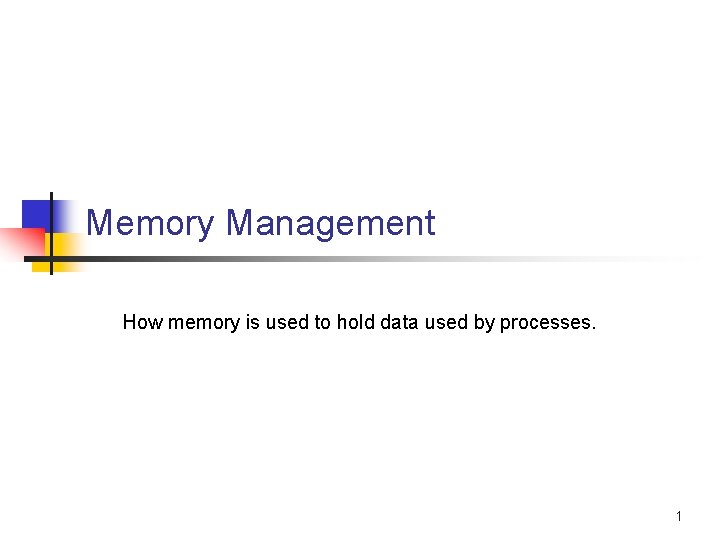
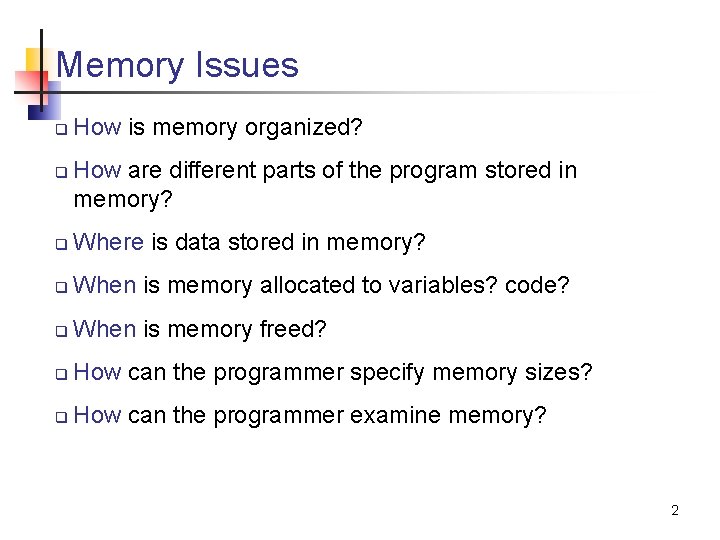
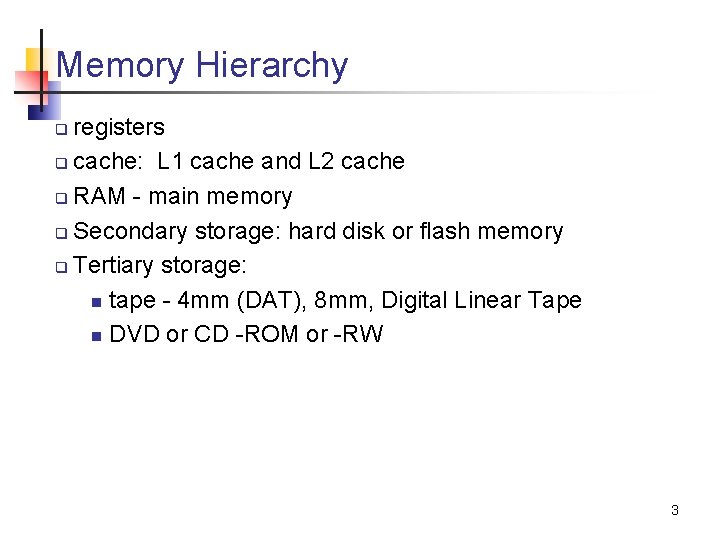
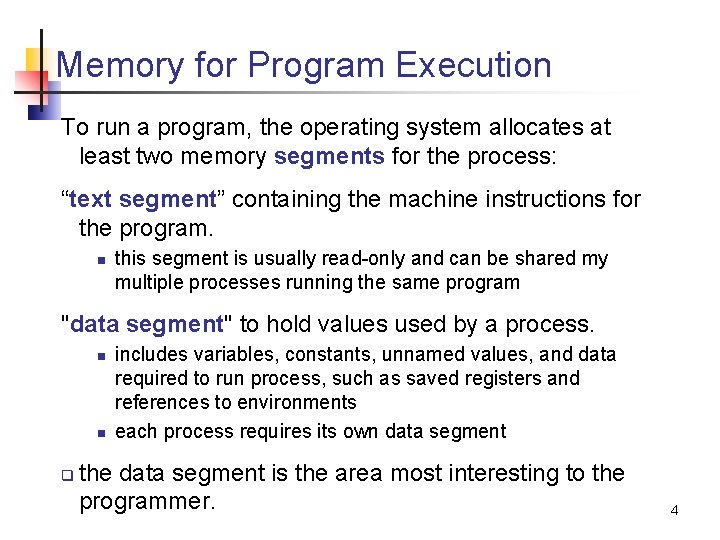
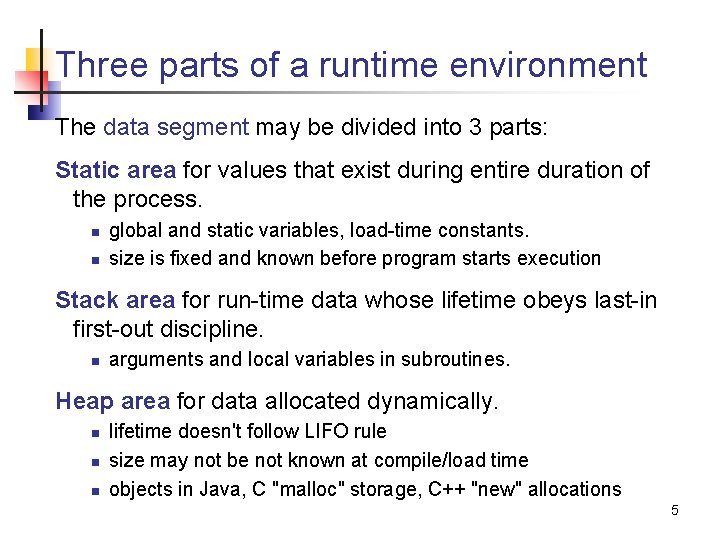
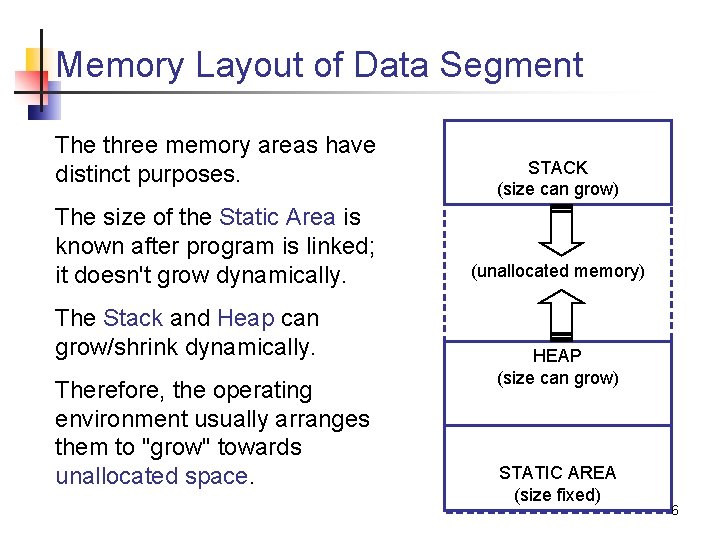
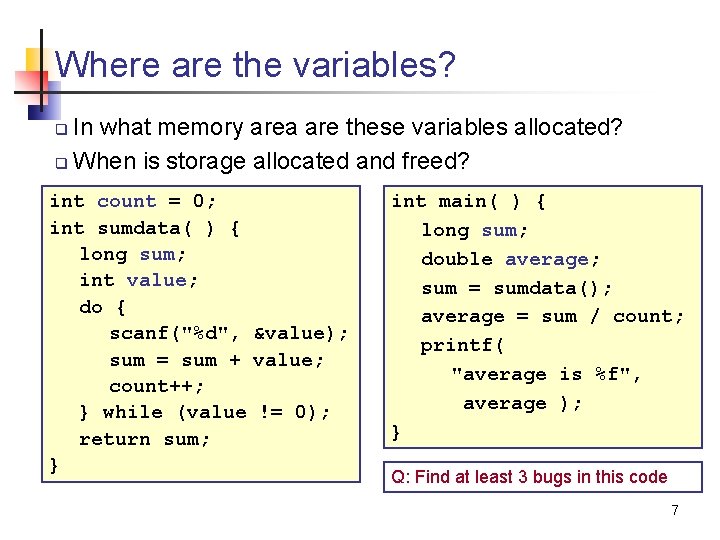
![Memory Usage stack space for "main" stack space for "sumdata" sum average [temporaries] [return Memory Usage stack space for "main" stack space for "sumdata" sum average [temporaries] [return](https://slidetodoc.com/presentation_image/352373c12ab72a7deecc528a8ff0b3b7/image-8.jpg)
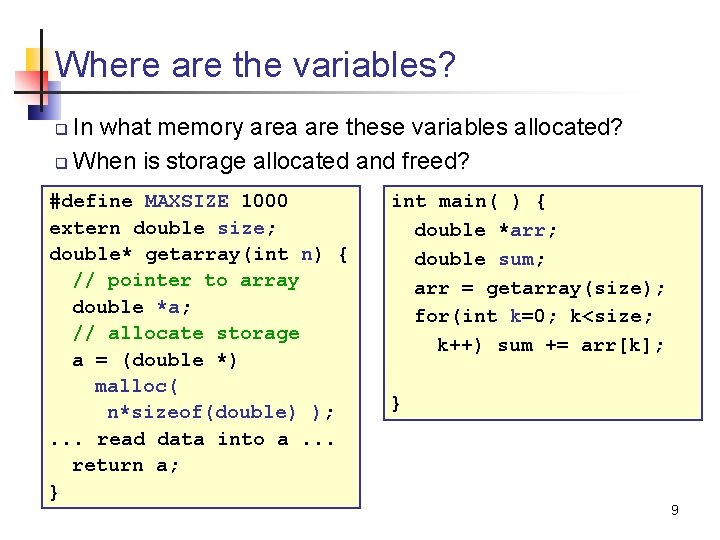
![Memory Usage arr sum k n [return addr and value] a stack space for Memory Usage arr sum k n [return addr and value] a stack space for](https://slidetodoc.com/presentation_image/352373c12ab72a7deecc528a8ff0b3b7/image-10.jpg)
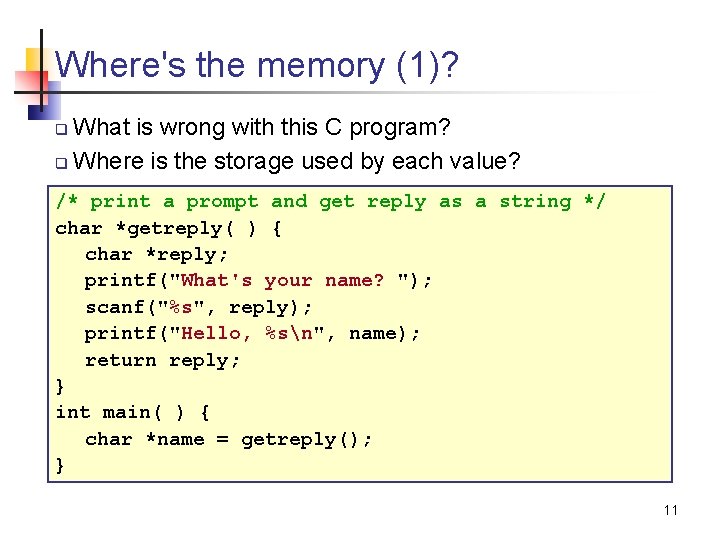
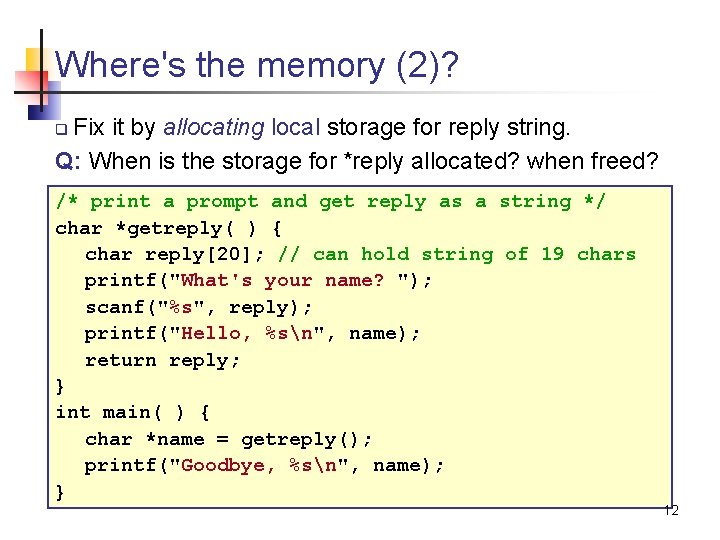
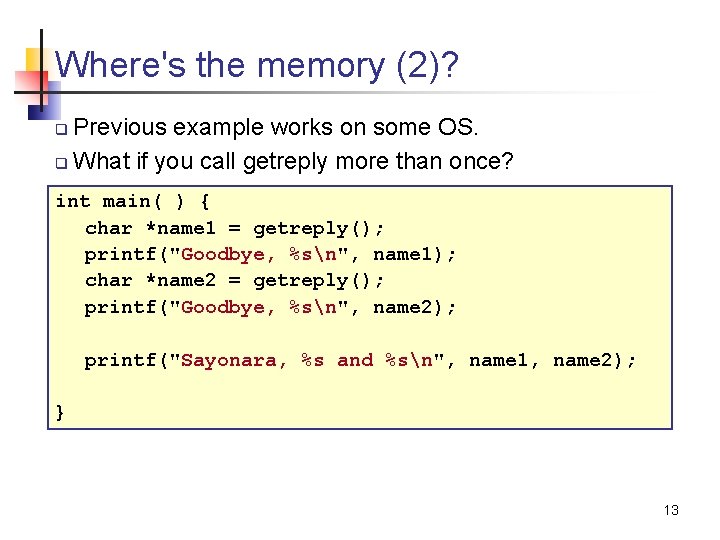
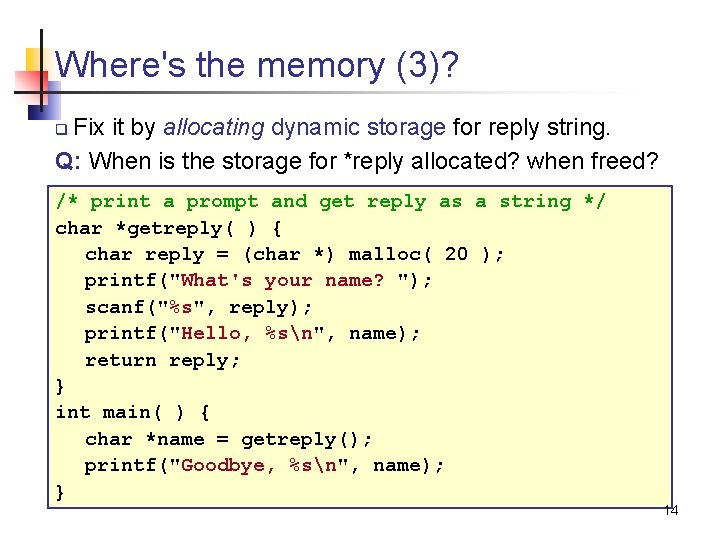
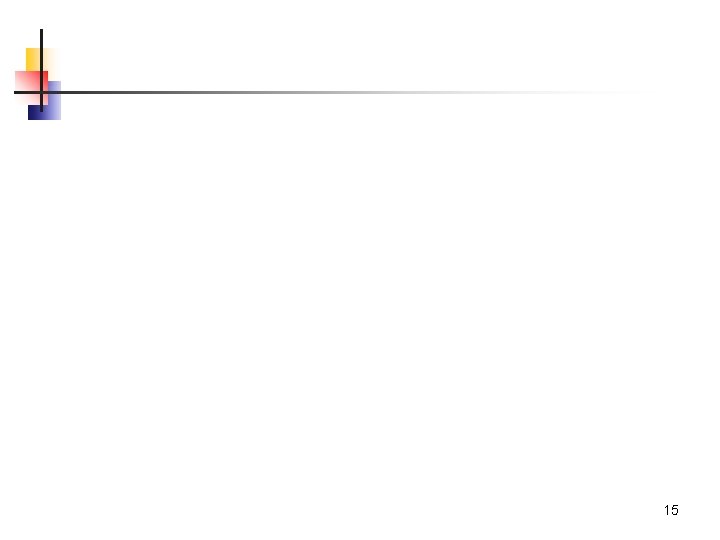
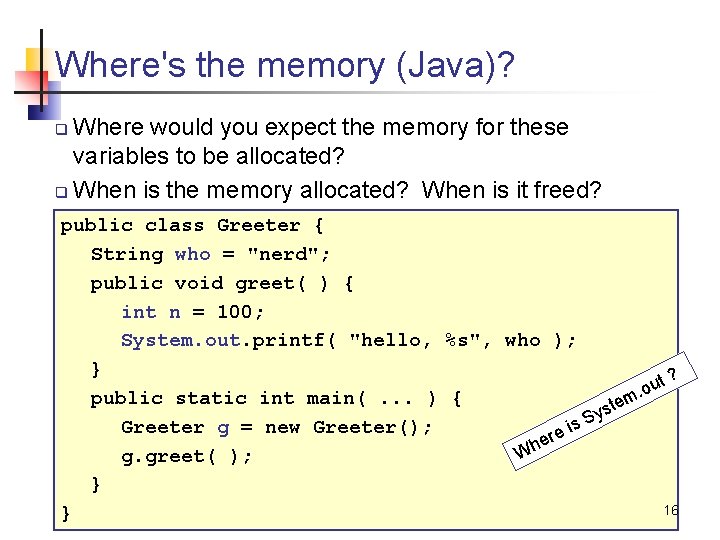
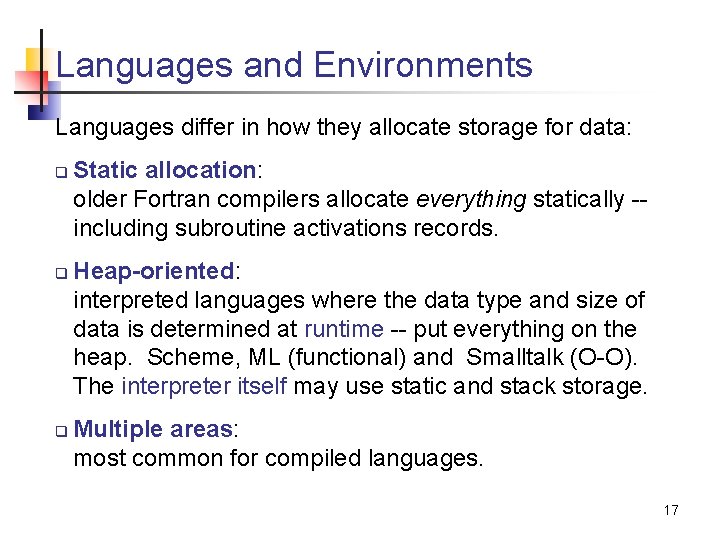
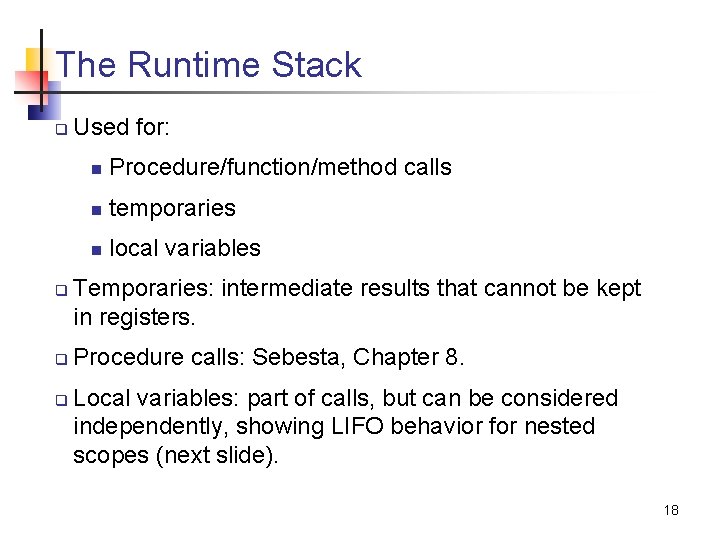
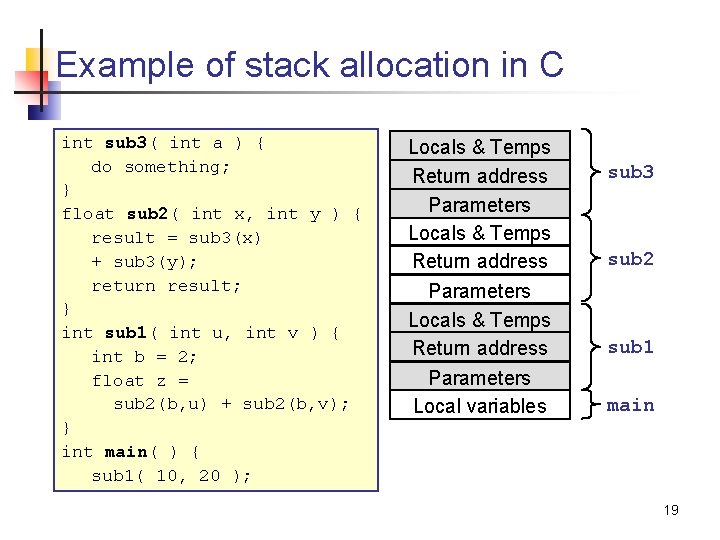
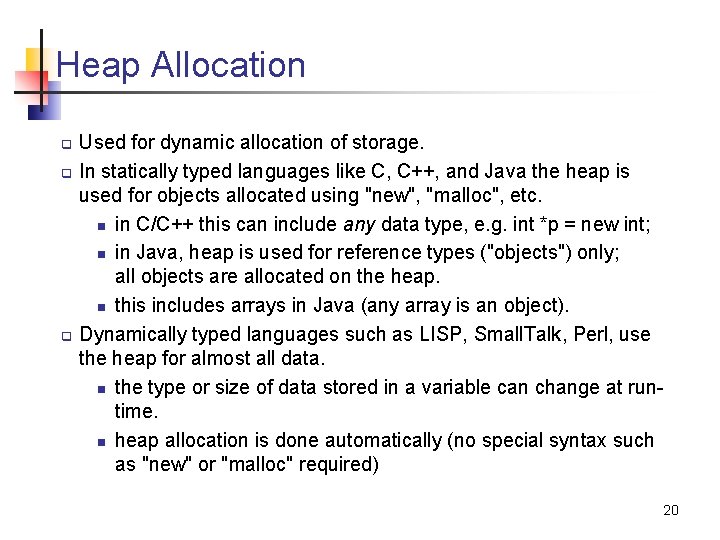
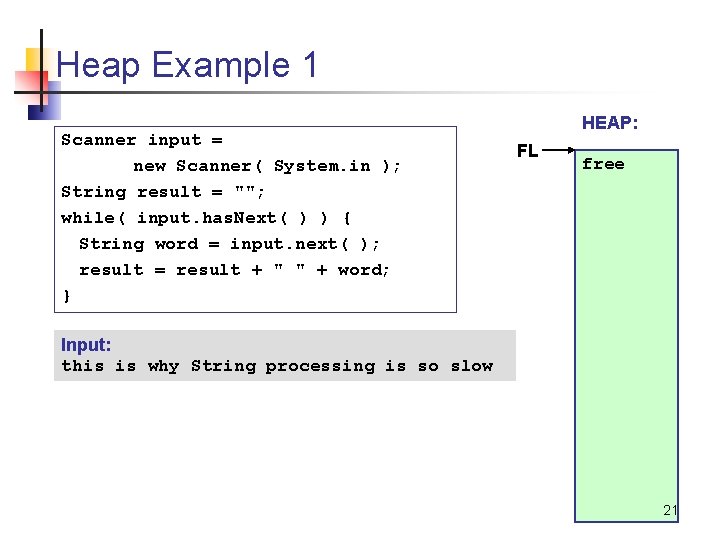
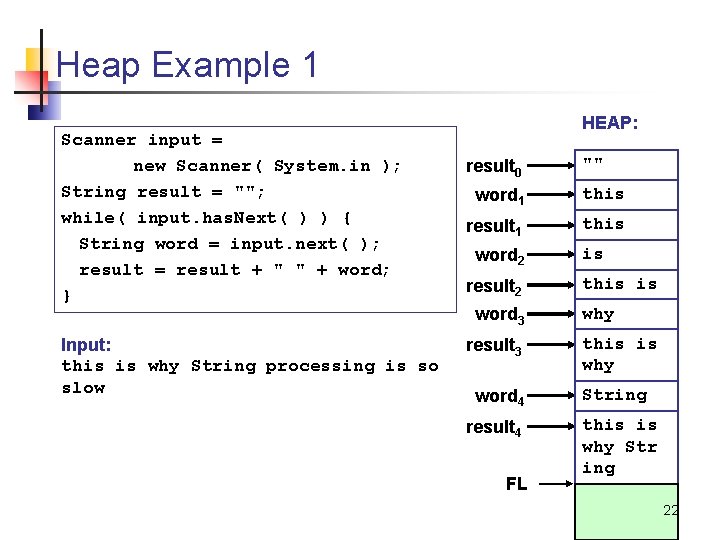
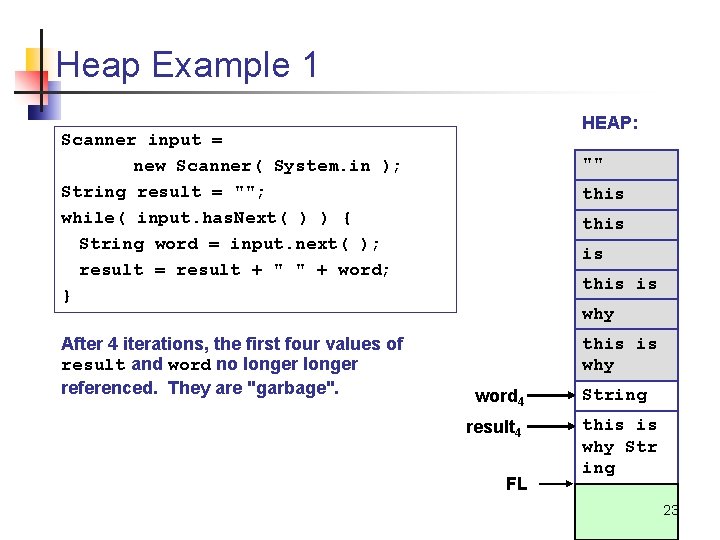
![Heap Example 2 Scanner input = new Scanner( in ); String [ ] r Heap Example 2 Scanner input = new Scanner( in ); String [ ] r](https://slidetodoc.com/presentation_image/352373c12ab72a7deecc528a8ff0b3b7/image-24.jpg)
![Heap Example 2 Scanner input = new Scanner( in ); String [ ] r Heap Example 2 Scanner input = new Scanner( in ); String [ ] r](https://slidetodoc.com/presentation_image/352373c12ab72a7deecc528a8ff0b3b7/image-25.jpg)
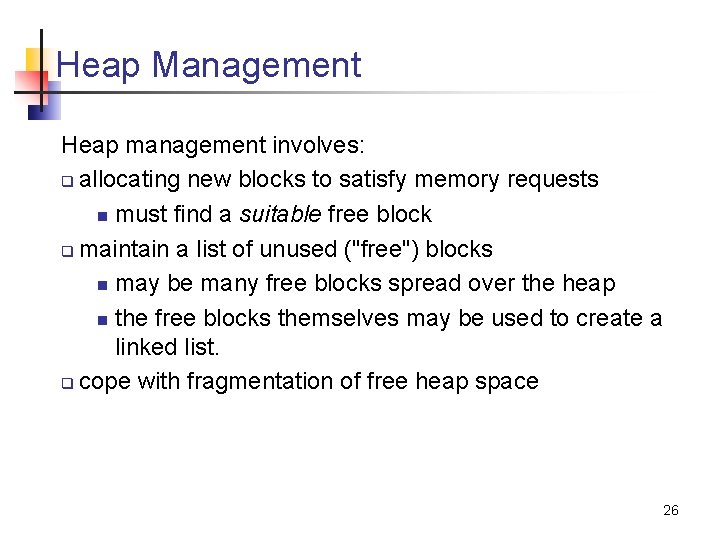
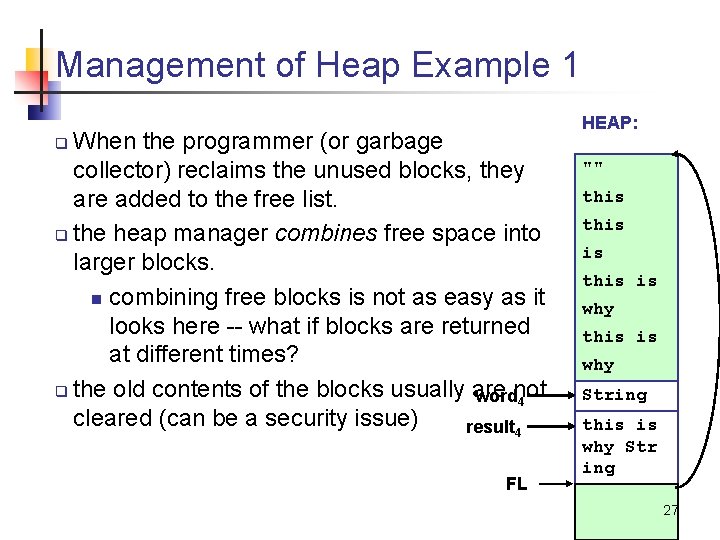
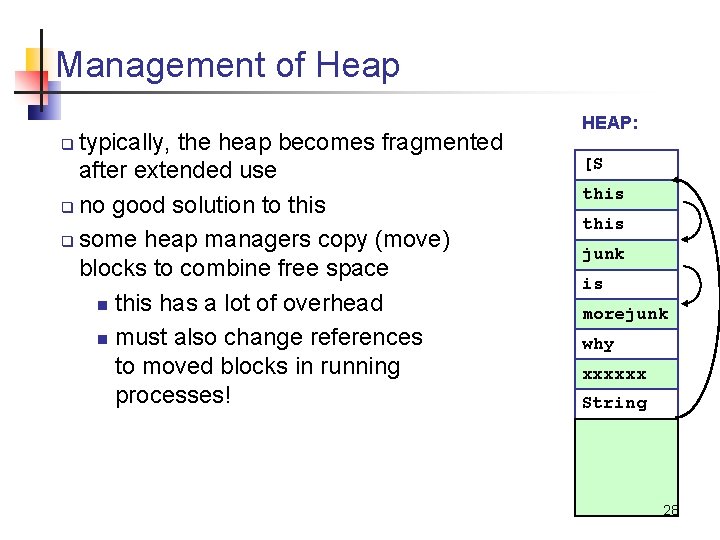
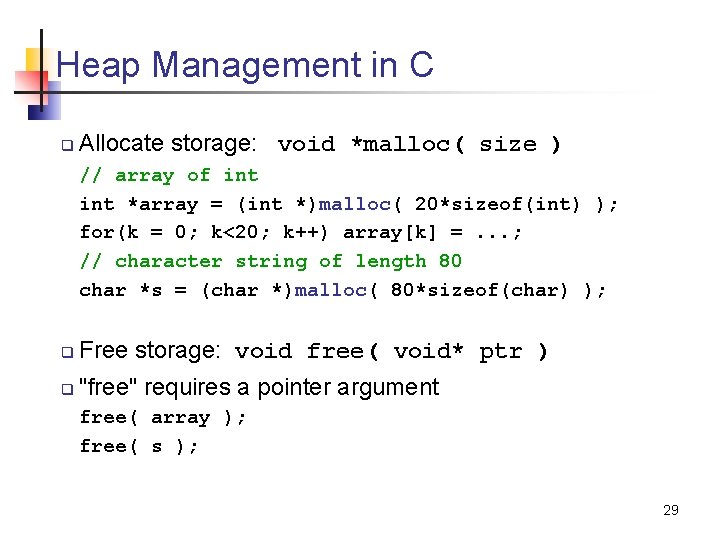
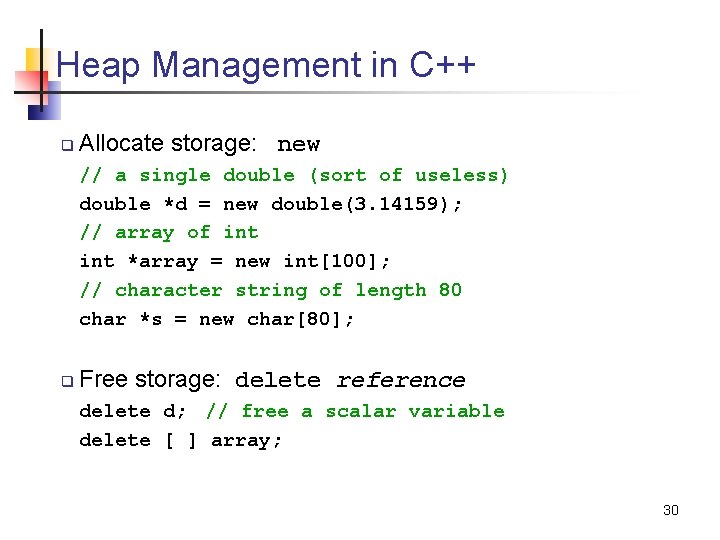
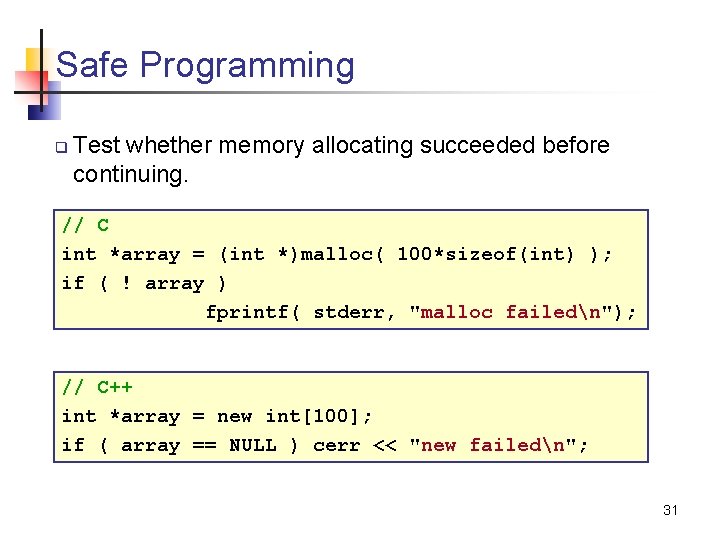
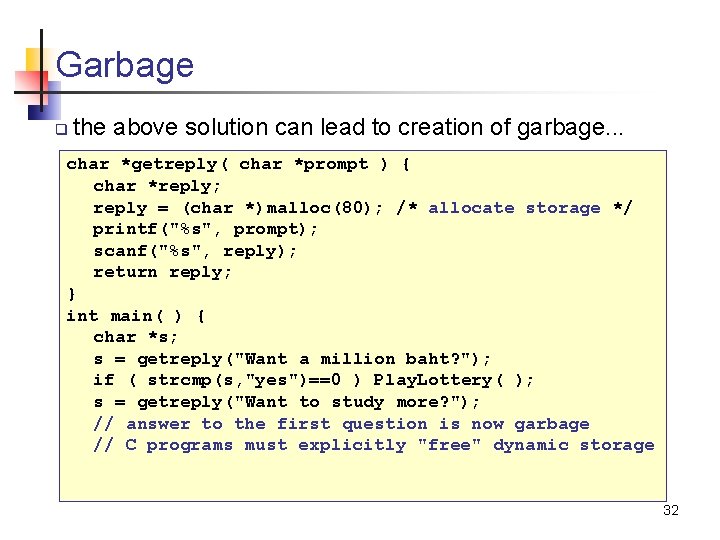
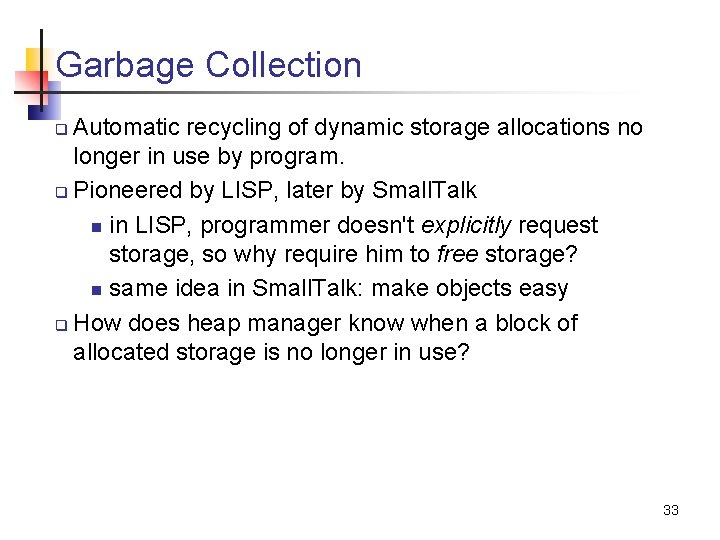
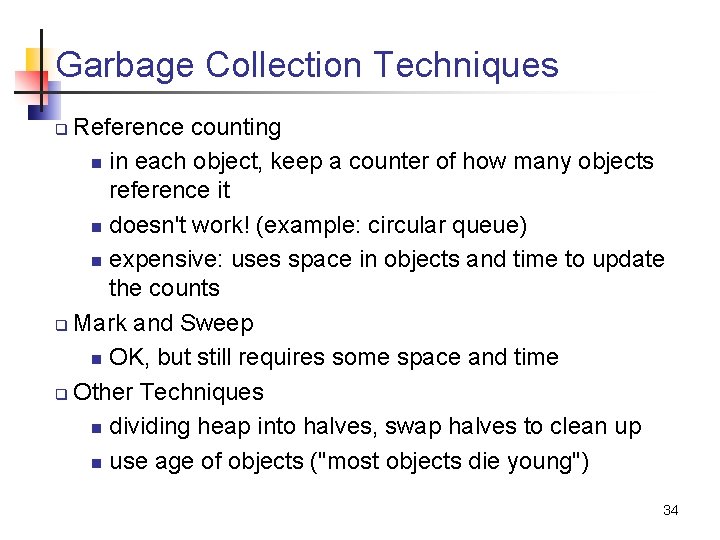
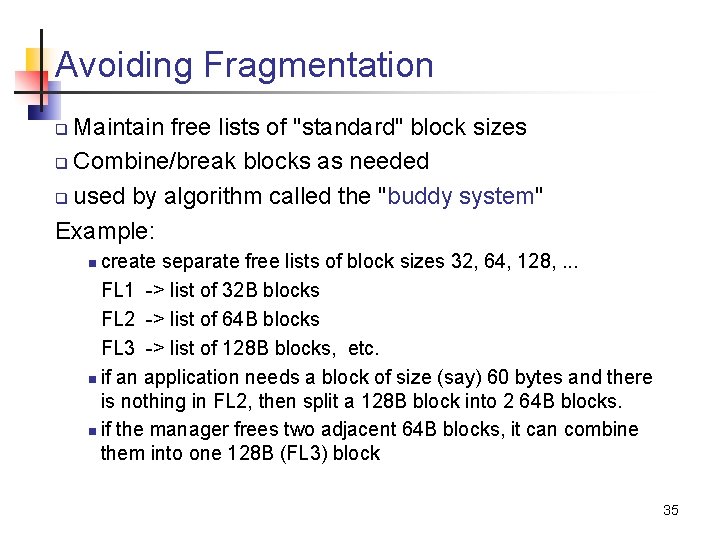
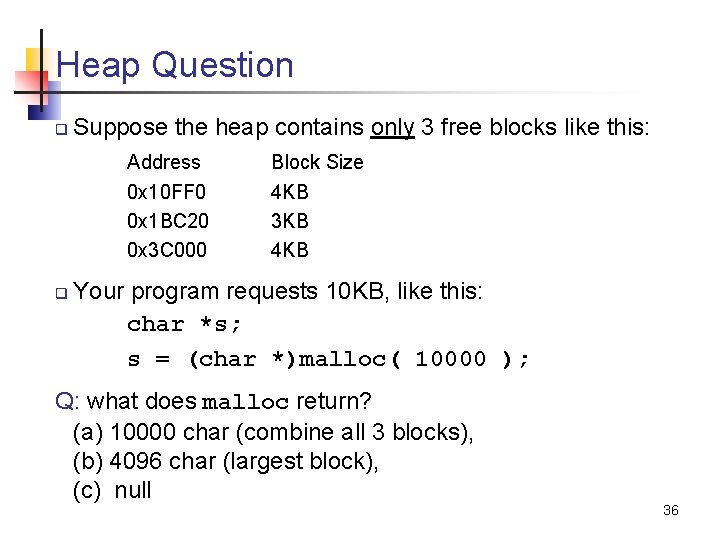
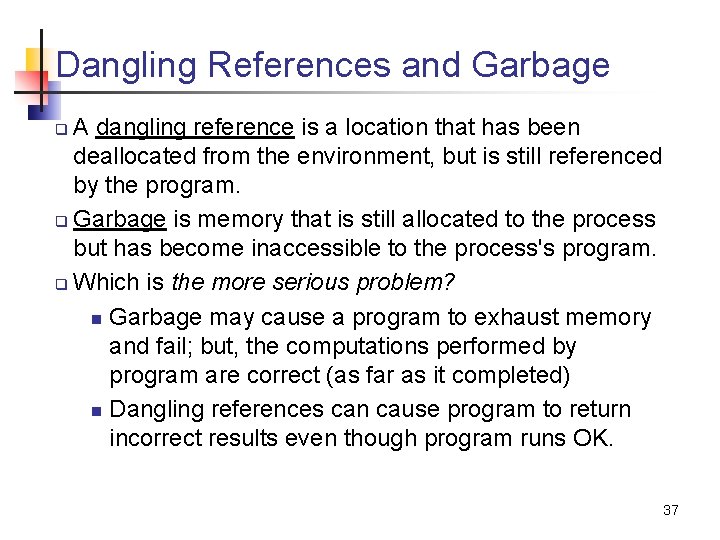
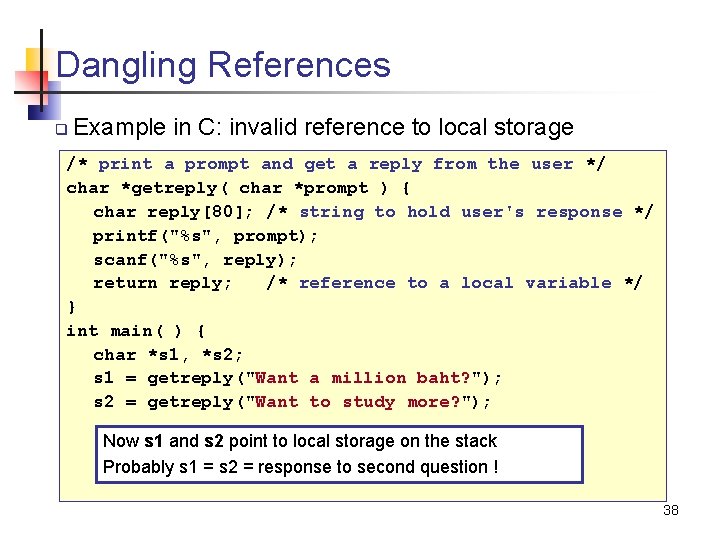
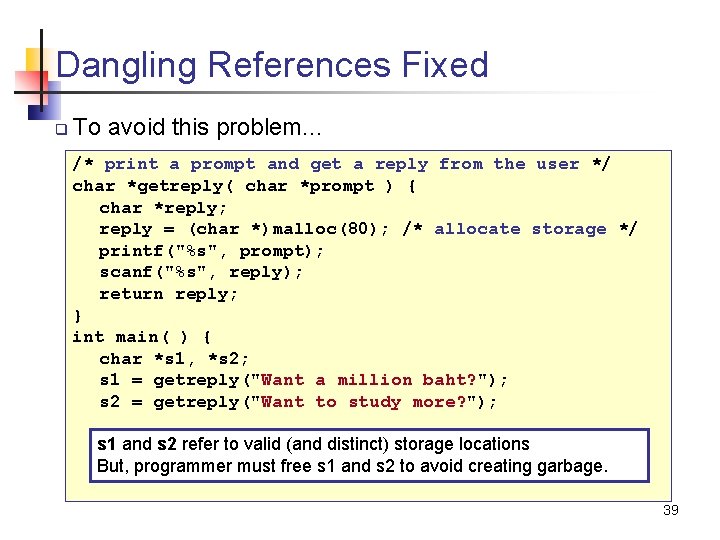
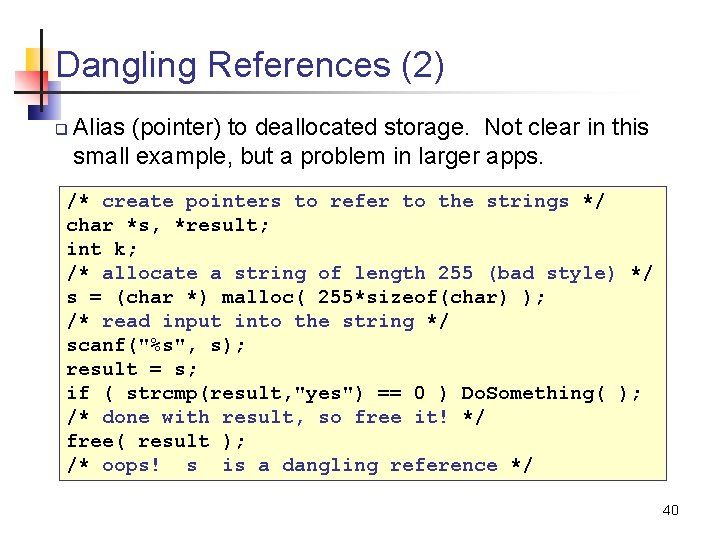
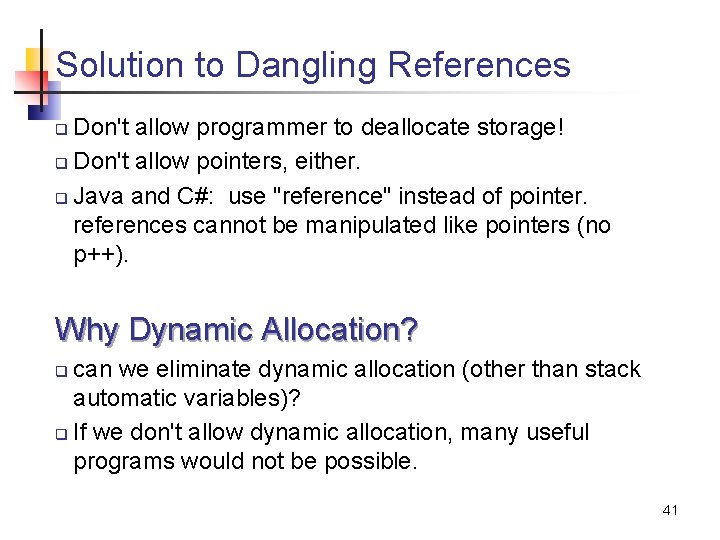
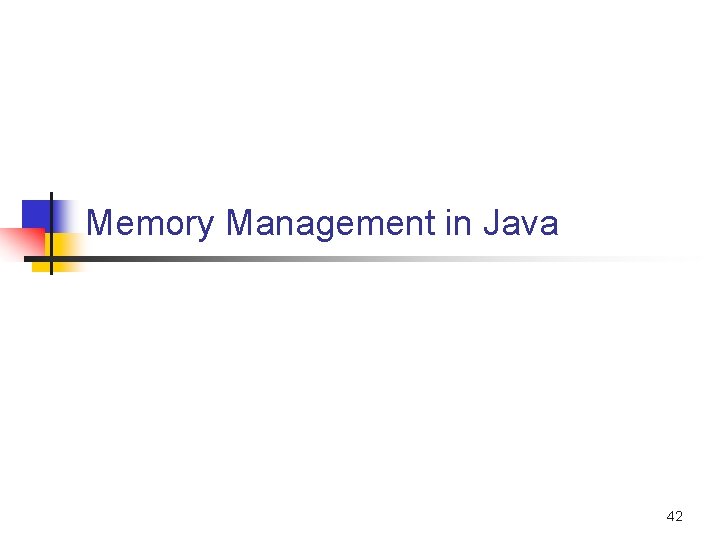
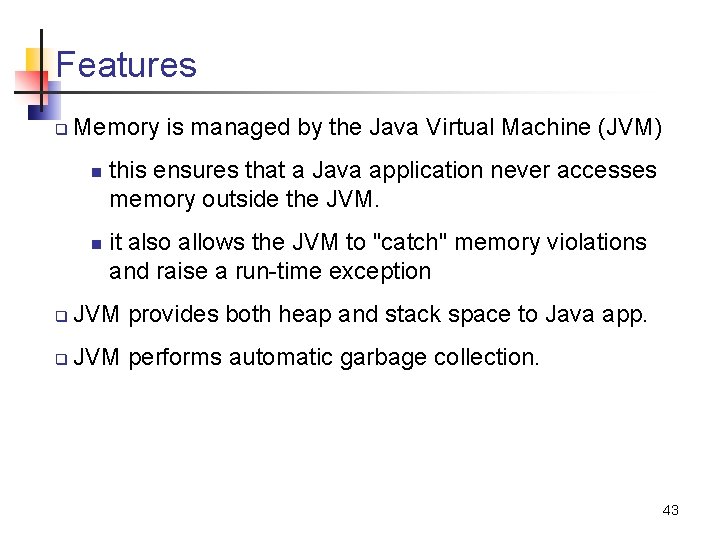
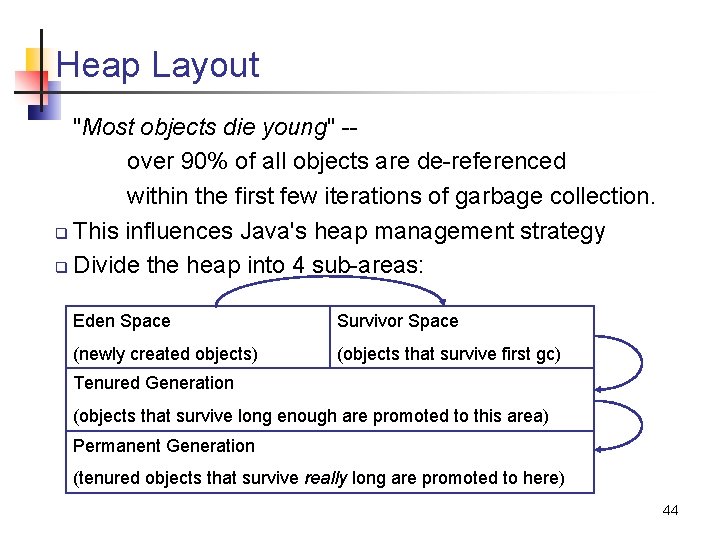
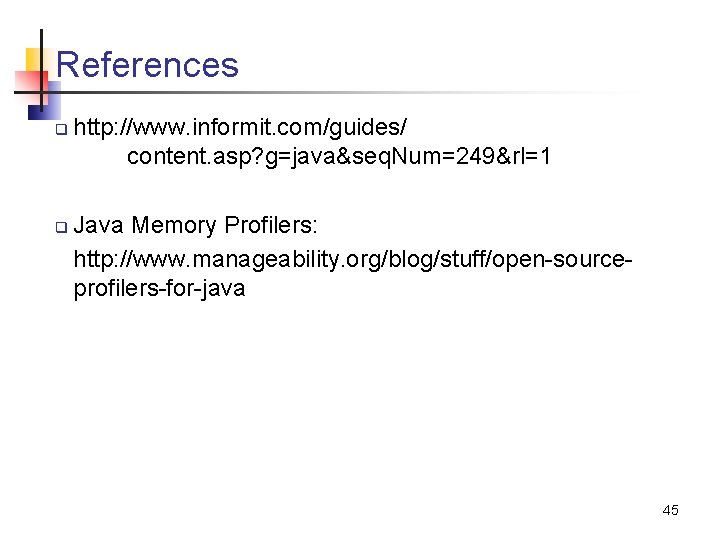
- Slides: 45
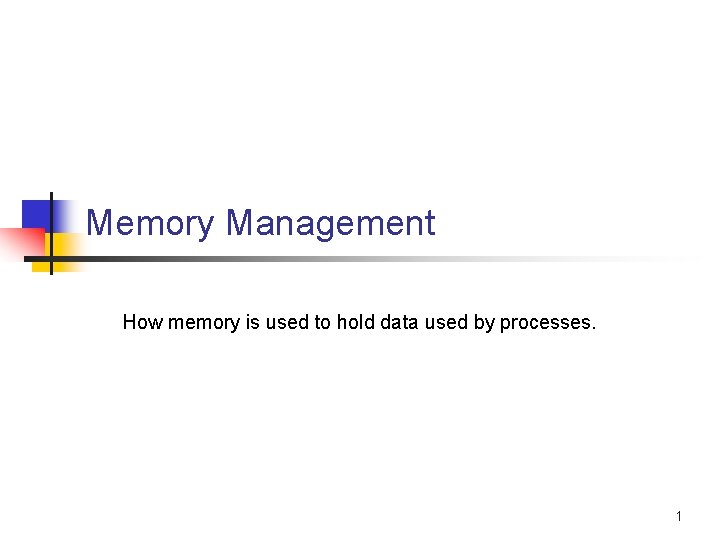
Memory Management How memory is used to hold data used by processes. 1
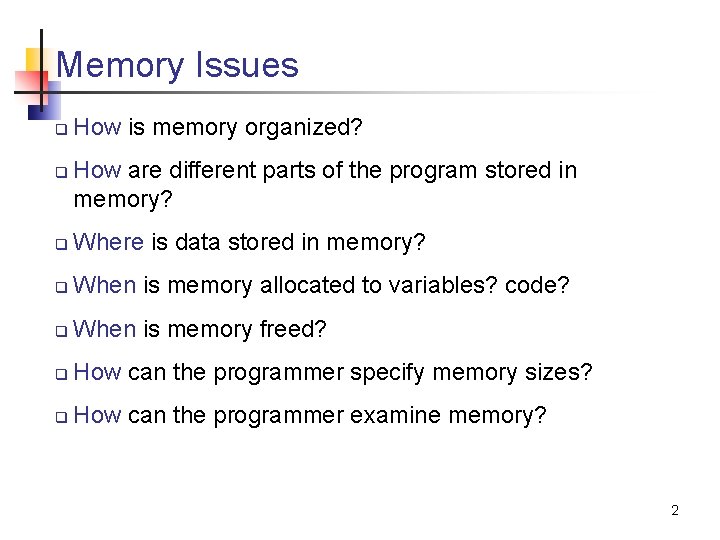
Memory Issues q q How is memory organized? How are different parts of the program stored in memory? q Where is data stored in memory? q When is memory allocated to variables? code? q When is memory freed? q How can the programmer specify memory sizes? q How can the programmer examine memory? 2
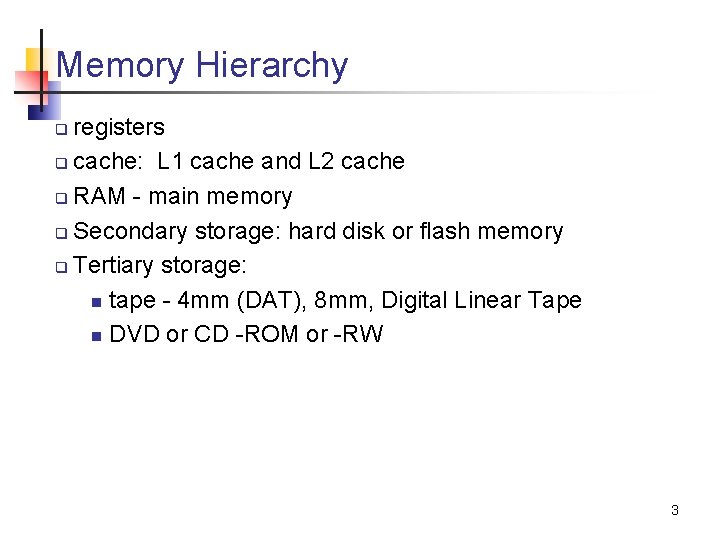
Memory Hierarchy registers q cache: L 1 cache and L 2 cache q RAM - main memory q Secondary storage: hard disk or flash memory q Tertiary storage: n tape - 4 mm (DAT), 8 mm, Digital Linear Tape n DVD or CD -ROM or -RW q 3
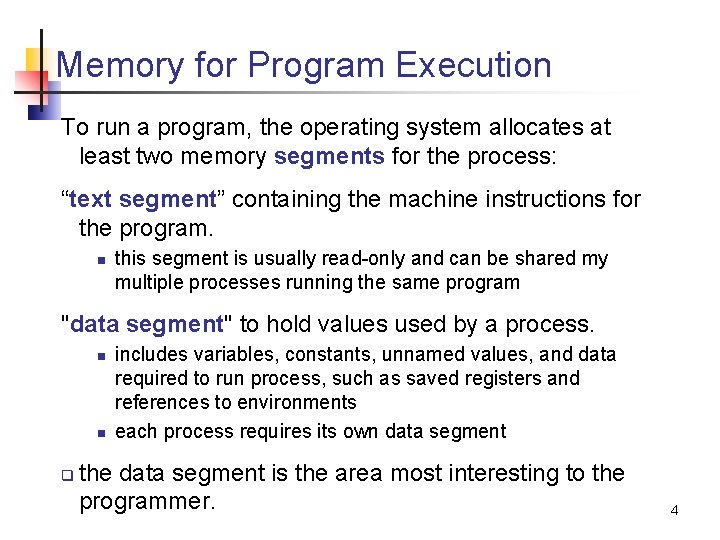
Memory for Program Execution To run a program, the operating system allocates at least two memory segments for the process: “text segment” containing the machine instructions for the program. n this segment is usually read-only and can be shared my multiple processes running the same program "data segment" to hold values used by a process. n n q includes variables, constants, unnamed values, and data required to run process, such as saved registers and references to environments each process requires its own data segment the data segment is the area most interesting to the programmer. 4
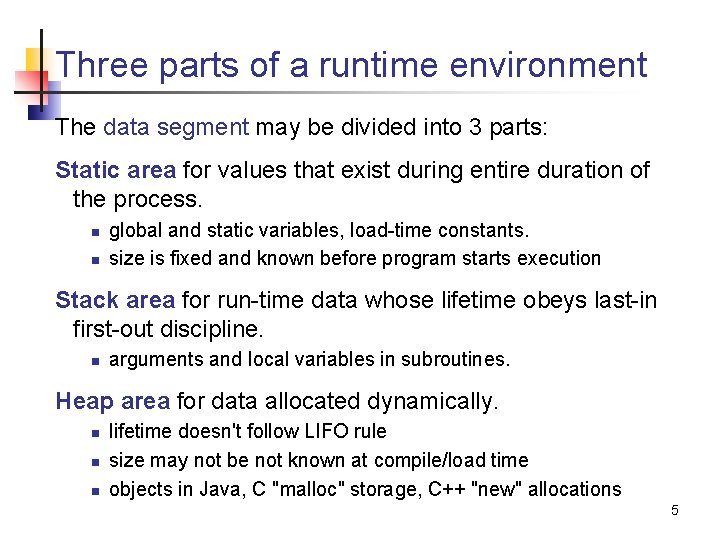
Three parts of a runtime environment The data segment may be divided into 3 parts: Static area for values that exist during entire duration of the process. n n global and static variables, load-time constants. size is fixed and known before program starts execution Stack area for run-time data whose lifetime obeys last-in first-out discipline. n arguments and local variables in subroutines. Heap area for data allocated dynamically. n n n lifetime doesn't follow LIFO rule size may not be not known at compile/load time objects in Java, C "malloc" storage, C++ "new" allocations 5
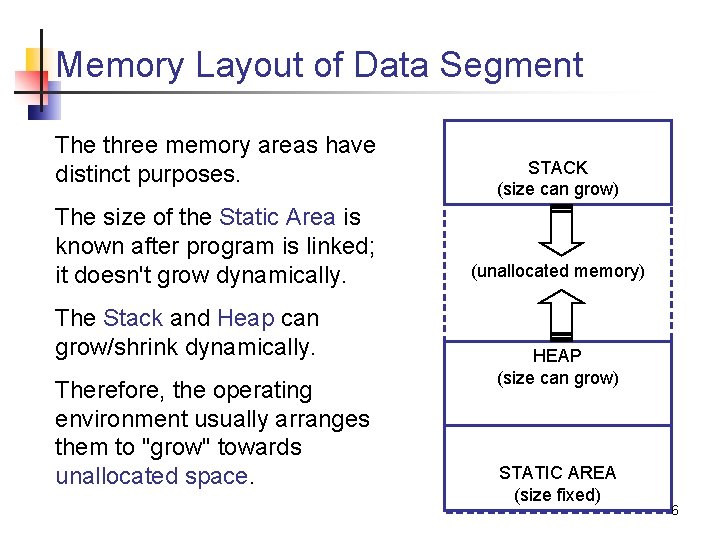
Memory Layout of Data Segment The three memory areas have distinct purposes. The size of the Static Area is known after program is linked; it doesn't grow dynamically. The Stack and Heap can grow/shrink dynamically. Therefore, the operating environment usually arranges them to "grow" towards unallocated space. STACK (size can grow) (unallocated memory) HEAP (size can grow) STATIC AREA (size fixed) 6
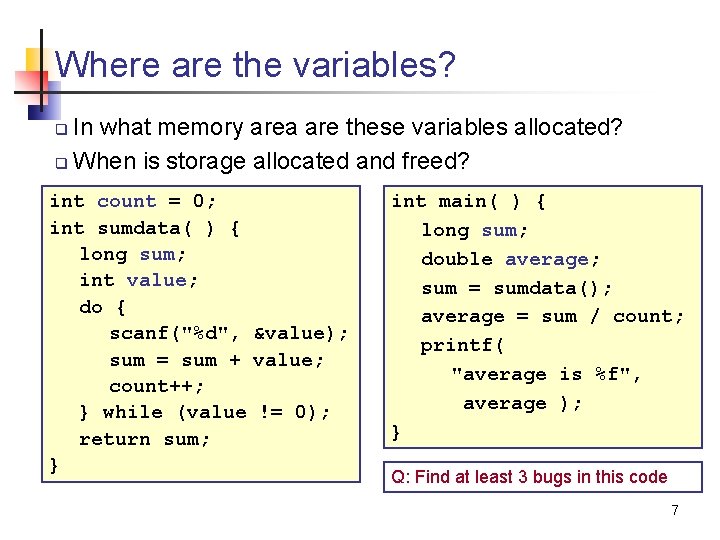
Where are the variables? In what memory area are these variables allocated? q When is storage allocated and freed? q int count = 0; int sumdata( ) { long sum; int value; do { scanf("%d", &value); sum = sum + value; count++; } while (value != 0); return sum; } int main( ) { long sum; double average; sum = sumdata(); average = sum / count; printf( "average is %f", average ); } Q: Find at least 3 bugs in this code 7
![Memory Usage stack space for main stack space for sumdata sum average temporaries return Memory Usage stack space for "main" stack space for "sumdata" sum average [temporaries] [return](https://slidetodoc.com/presentation_image/352373c12ab72a7deecc528a8ff0b3b7/image-8.jpg)
Memory Usage stack space for "main" stack space for "sumdata" sum average [temporaries] [return addr and value] sum value (unallocated memory) HEAP (size can grow) STATIC AREA count 8
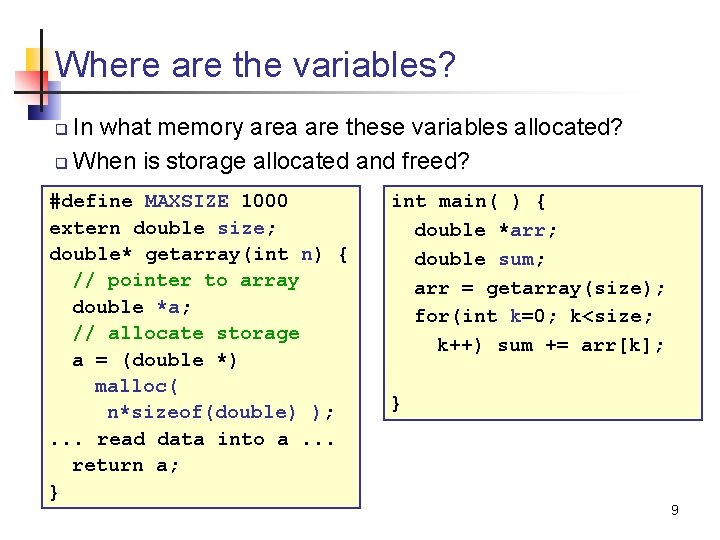
Where are the variables? In what memory area are these variables allocated? q When is storage allocated and freed? q #define MAXSIZE 1000 extern double size; double* getarray(int n) { // pointer to array double *a; // allocate storage a = (double *) malloc( n*sizeof(double) ); . . . read data into a. . . return a; } int main( ) { double *arr; double sum; arr = getarray(size); for(int k=0; k<size; k++) sum += arr[k]; } 9
![Memory Usage arr sum k n return addr and value a stack space for Memory Usage arr sum k n [return addr and value] a stack space for](https://slidetodoc.com/presentation_image/352373c12ab72a7deecc528a8ff0b3b7/image-10.jpg)
Memory Usage arr sum k n [return addr and value] a stack space for "main" stack space for "getarray" (unallocated memory) value points to HEAP STATIC AREA *a 1000*sizeof(double) size (allocated by another part of the program) 10
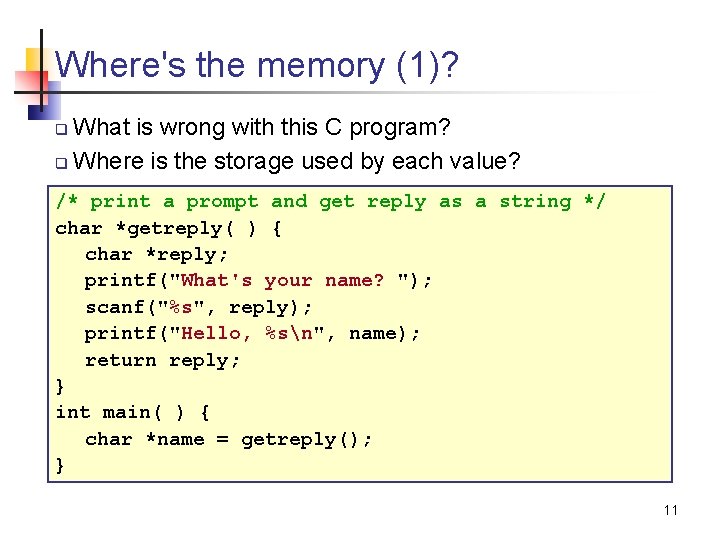
Where's the memory (1)? What is wrong with this C program? q Where is the storage used by each value? q /* print a prompt and get reply as a string */ char *getreply( ) { char *reply; printf("What's your name? "); scanf("%s", reply); printf("Hello, %sn", name); return reply; } int main( ) { char *name = getreply(); } 11
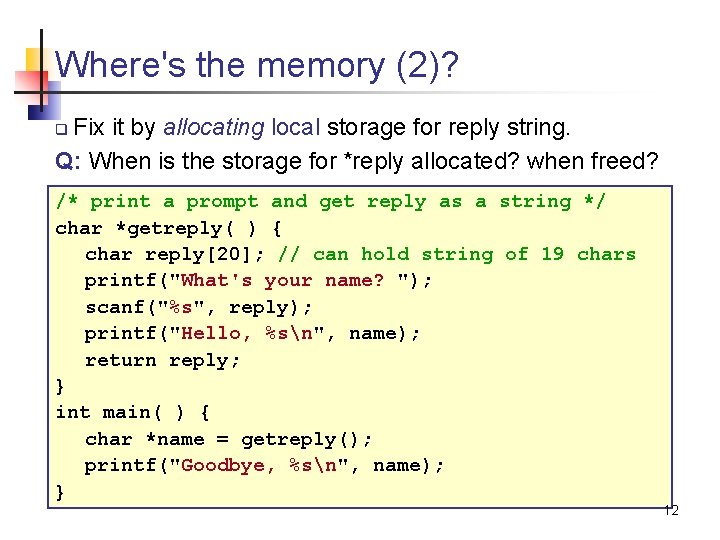
Where's the memory (2)? Fix it by allocating local storage for reply string. Q: When is the storage for *reply allocated? when freed? q /* print a prompt and get reply as a string */ char *getreply( ) { char reply[20]; // can hold string of 19 chars printf("What's your name? "); scanf("%s", reply); printf("Hello, %sn", name); return reply; } int main( ) { char *name = getreply(); printf("Goodbye, %sn", name); } 12
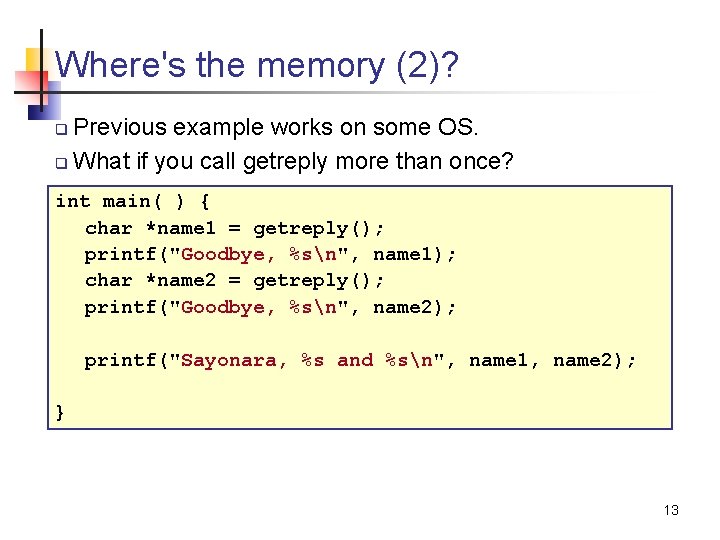
Where's the memory (2)? Previous example works on some OS. q What if you call getreply more than once? q int main( ) { char *name 1 = getreply(); printf("Goodbye, %sn", name 1); char *name 2 = getreply(); printf("Goodbye, %sn", name 2); printf("Sayonara, %s and %sn", name 1, name 2); } 13
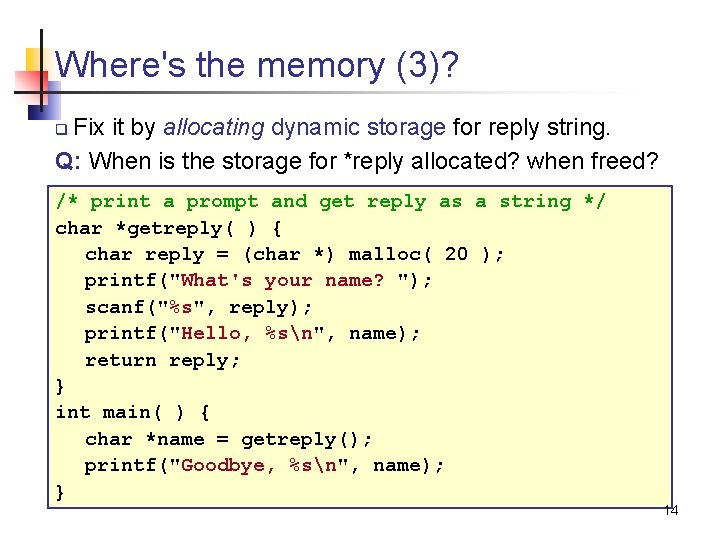
Where's the memory (3)? Fix it by allocating dynamic storage for reply string. Q: When is the storage for *reply allocated? when freed? q /* print a prompt and get reply as a string */ char *getreply( ) { char reply = (char *) malloc( 20 ); printf("What's your name? "); scanf("%s", reply); printf("Hello, %sn", name); return reply; } int main( ) { char *name = getreply(); printf("Goodbye, %sn", name); } 14
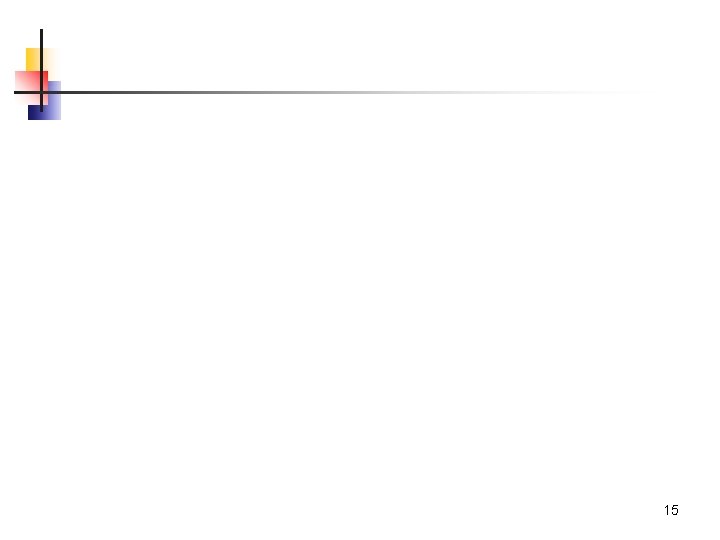
15
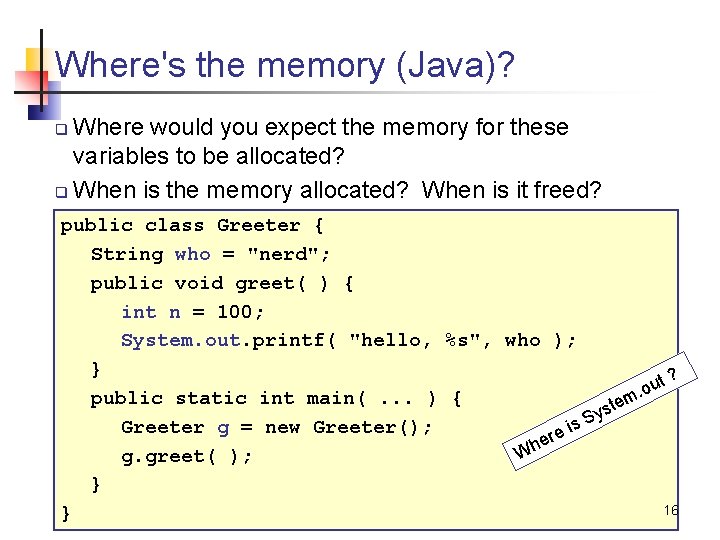
Where's the memory (Java)? Where would you expect the memory for these variables to be allocated? q When is the memory allocated? When is it freed? q public class Greeter { String who = "nerd"; public void greet( ) { int n = 100; System. out. printf( "hello, %s", who ); } t? u o public static int main(. . . ) { m. e t ys S Greeter g = new Greeter(); is e r he W g. greet( ); } 16 }
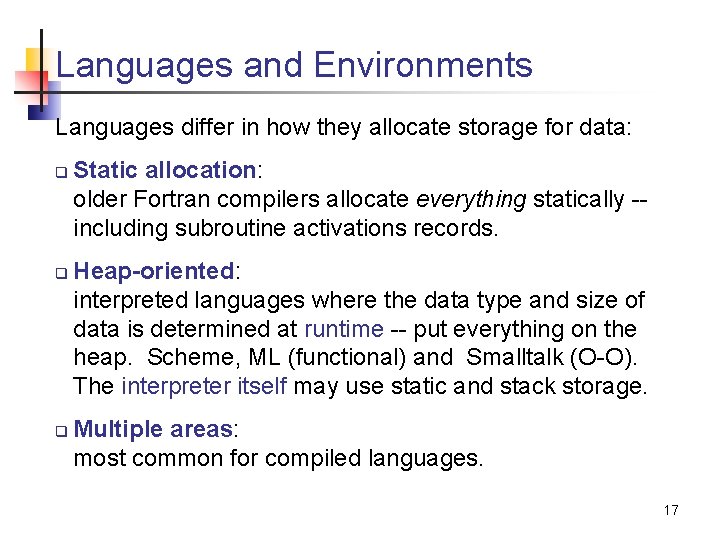
Languages and Environments Languages differ in how they allocate storage for data: q q q Static allocation: older Fortran compilers allocate everything statically -including subroutine activations records. Heap-oriented: interpreted languages where the data type and size of data is determined at runtime -- put everything on the heap. Scheme, ML (functional) and Smalltalk (O-O). The interpreter itself may use static and stack storage. Multiple areas: most common for compiled languages. 17
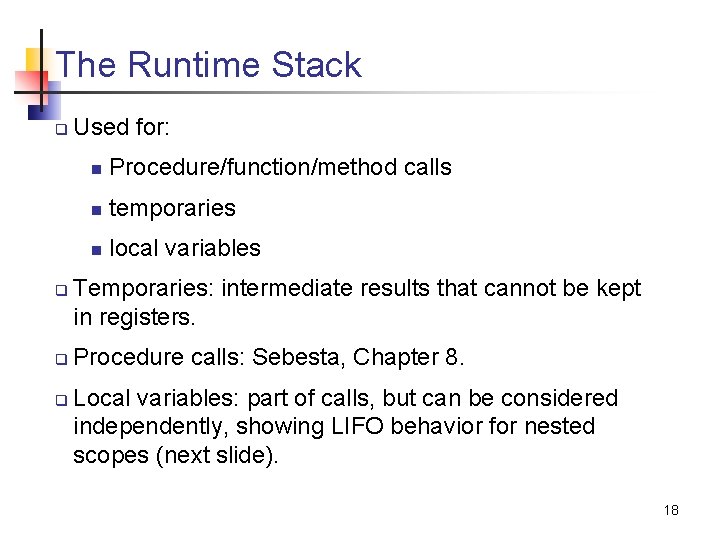
The Runtime Stack q q Used for: n Procedure/function/method calls n temporaries n local variables Temporaries: intermediate results that cannot be kept in registers. Procedure calls: Sebesta, Chapter 8. Local variables: part of calls, but can be considered independently, showing LIFO behavior for nested scopes (next slide). 18
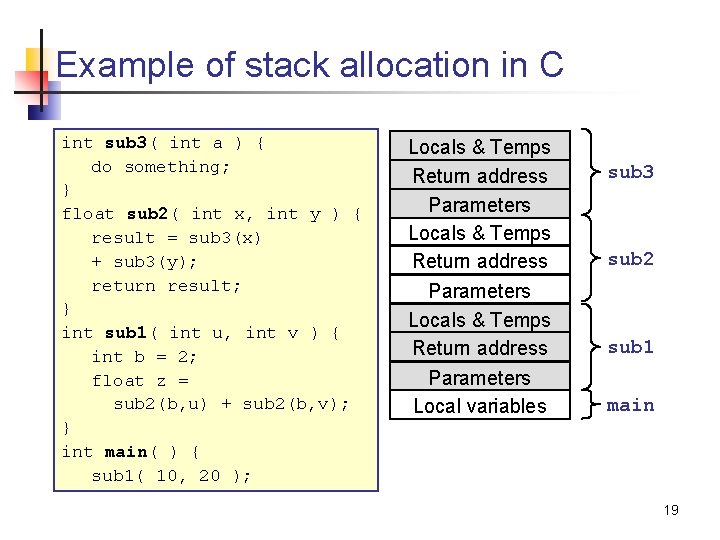
Example of stack allocation in C int sub 3( int a ) { do something; } float sub 2( int x, int y ) { result = sub 3(x) + sub 3(y); return result; } int sub 1( int u, int v ) { int b = 2; float z = sub 2(b, u) + sub 2(b, v); } int main( ) { sub 1( 10, 20 ); Locals & Temps Return address Parameters Local variables sub 3 sub 2 sub 1 main 19
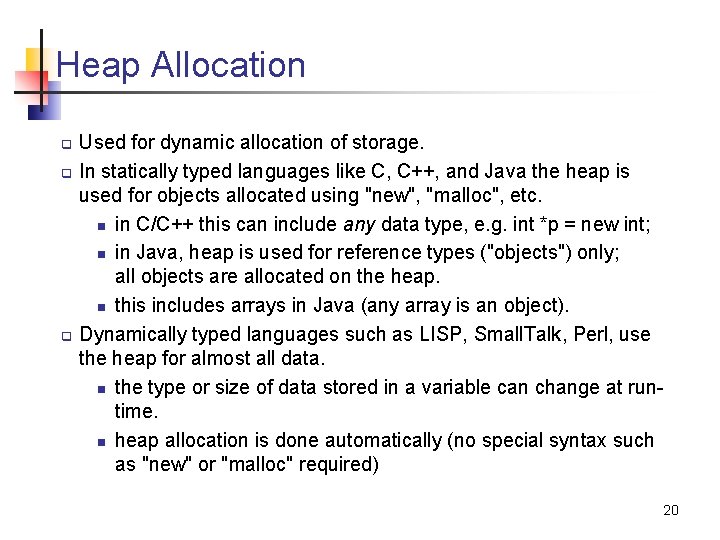
Heap Allocation q q q Used for dynamic allocation of storage. In statically typed languages like C, C++, and Java the heap is used for objects allocated using "new", "malloc", etc. n in C/C++ this can include any data type, e. g. int *p = new int; n in Java, heap is used for reference types ("objects") only; all objects are allocated on the heap. n this includes arrays in Java (any array is an object). Dynamically typed languages such as LISP, Small. Talk, Perl, use the heap for almost all data. n the type or size of data stored in a variable can change at runtime. n heap allocation is done automatically (no special syntax such as "new" or "malloc" required) 20
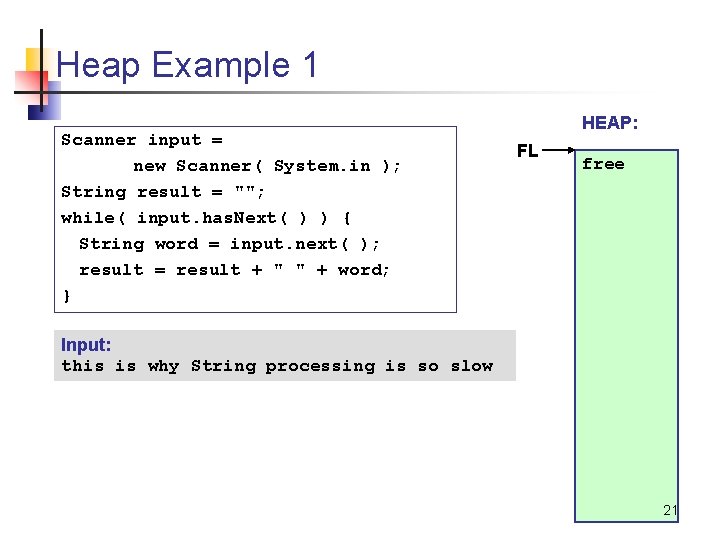
Heap Example 1 Scanner input = new Scanner( System. in ); String result = ""; while( input. has. Next( ) ) { String word = input. next( ); result = result + " " + word; } HEAP: FL free Input: this is why String processing is so slow 21
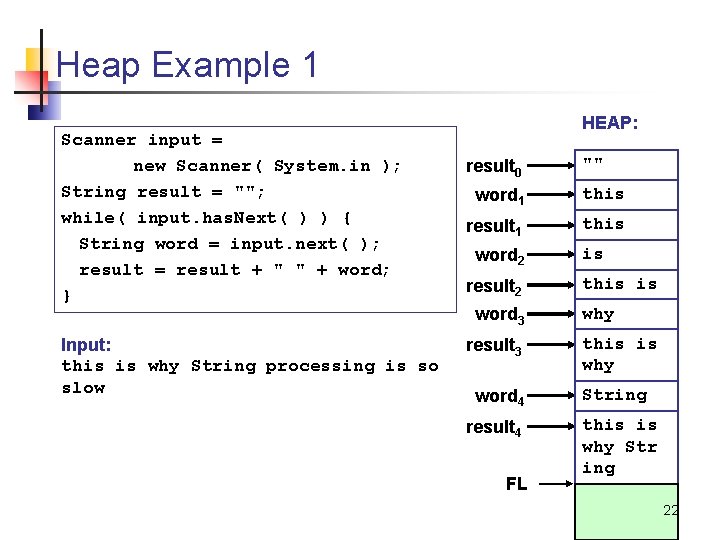
Heap Example 1 Scanner input = new Scanner( System. in ); String result = ""; while( input. has. Next( ) ) { String word = input. next( ); result = result + " " + word; } Input: this is why String processing is so slow HEAP: result 0 "" word 1 this result 1 this word 2 result 2 word 3 result 3 word 4 result 4 FL is this is why String this is why Str ing 22
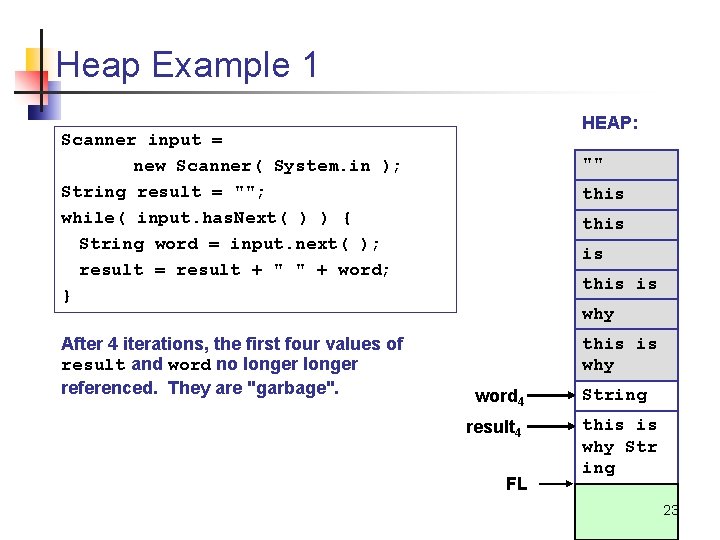
Heap Example 1 HEAP: Scanner input = new Scanner( System. in ); String result = ""; while( input. has. Next( ) ) { String word = input. next( ); result = result + " " + word; } After 4 iterations, the first four values of result and word no longer referenced. They are "garbage". "" this is why word 4 result 4 FL String this is why Str ing 23
![Heap Example 2 Scanner input new Scanner in String r Heap Example 2 Scanner input = new Scanner( in ); String [ ] r](https://slidetodoc.com/presentation_image/352373c12ab72a7deecc528a8ff0b3b7/image-24.jpg)
Heap Example 2 Scanner input = new Scanner( in ); String [ ] r = new String[10]; while( input. has. Next( ) && k < 10 ) { String word = input. next( ); r[k] = word; } HEAP: r FL [S free Input: this is why String processing is so slow 24
![Heap Example 2 Scanner input new Scanner in String r Heap Example 2 Scanner input = new Scanner( in ); String [ ] r](https://slidetodoc.com/presentation_image/352373c12ab72a7deecc528a8ff0b3b7/image-25.jpg)
Heap Example 2 Scanner input = new Scanner( in ); String [ ] r = new String[10]; while( input. has. Next( ) && k < 10 ) { String word = input. next( ); r[k] = word; } Input: this is why String processing is so slow HEAP: r [S r[0] this r[1] is r[2] why r[3] String r[4] proces. . word FL 25
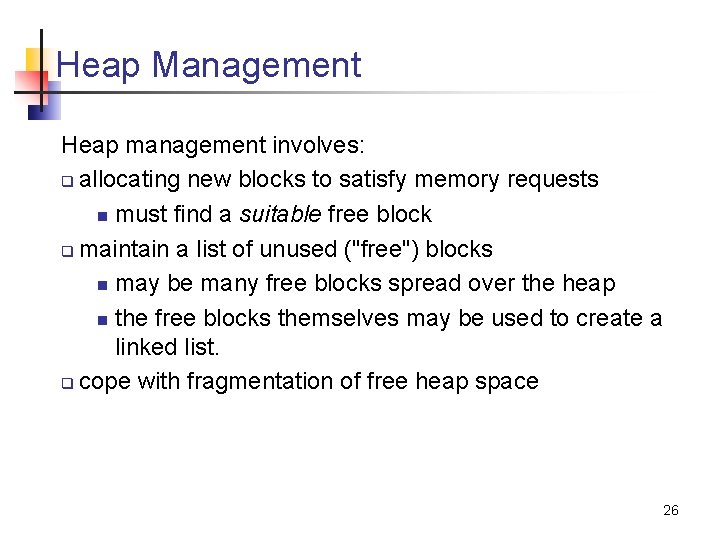
Heap Management Heap management involves: q allocating new blocks to satisfy memory requests n must find a suitable free block q maintain a list of unused ("free") blocks n may be many free blocks spread over the heap n the free blocks themselves may be used to create a linked list. q cope with fragmentation of free heap space 26
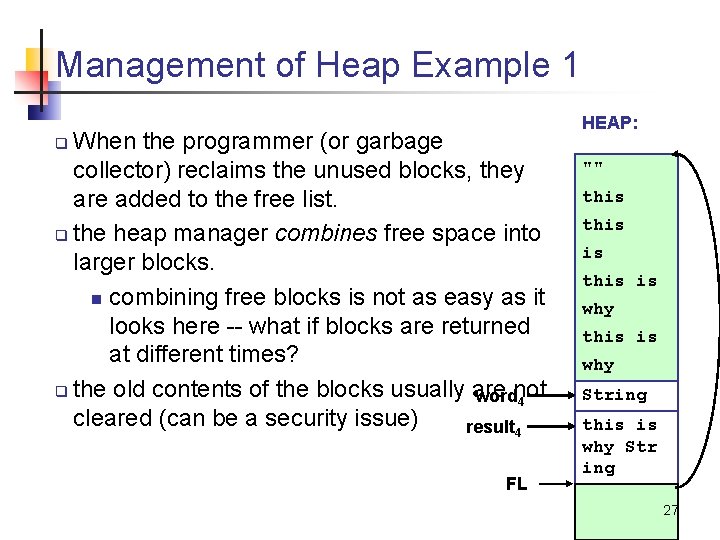
Management of Heap Example 1 When the programmer (or garbage collector) reclaims the unused blocks, they are added to the free list. q the heap manager combines free space into larger blocks. n combining free blocks is not as easy as it looks here -- what if blocks are returned at different times? q the old contents of the blocks usually are wordnot 4 cleared (can be a security issue) result 4 HEAP: q FL "" this is why String this is why Str ing 27
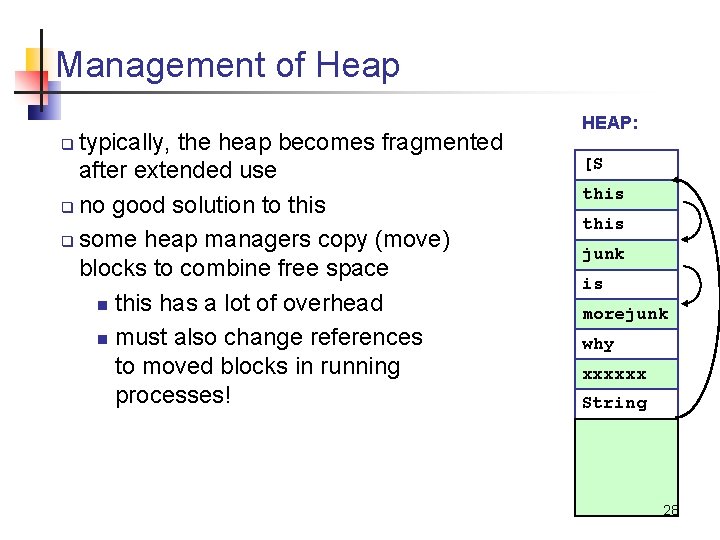
Management of Heap typically, the heap becomes fragmented after extended use q no good solution to this q some heap managers copy (move) blocks to combine free space n this has a lot of overhead n must also change references to moved blocks in running processes! HEAP: q [S this junk is morejunk why xxxxxx String 28
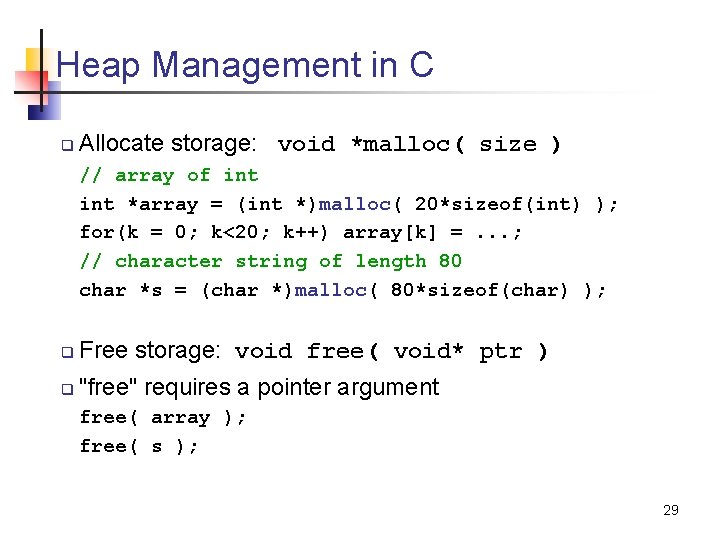
Heap Management in C q Allocate storage: void *malloc( size ) // array of int *array = (int *)malloc( 20*sizeof(int) ); for(k = 0; k<20; k++) array[k] =. . . ; // character string of length 80 char *s = (char *)malloc( 80*sizeof(char) ); q Free storage: void free( void* ptr ) q "free" requires a pointer argument free( array ); free( s ); 29
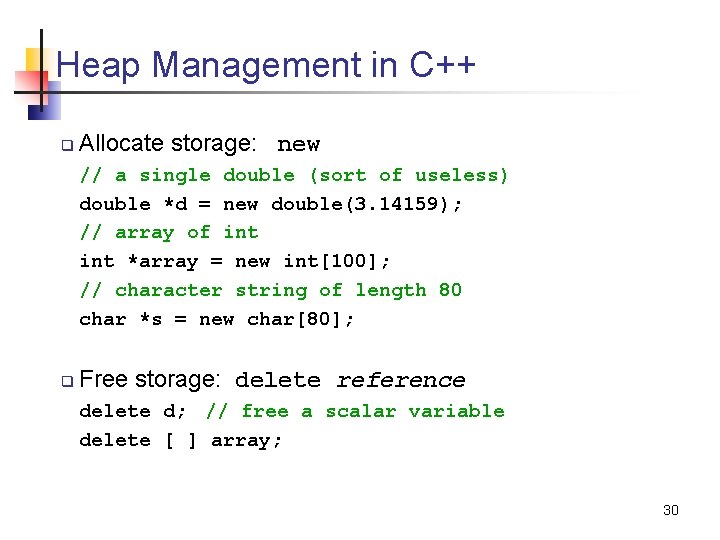
Heap Management in C++ q Allocate storage: new // a single double (sort of useless) double *d = new double(3. 14159); // array of int *array = new int[100]; // character string of length 80 char *s = new char[80]; q Free storage: delete reference delete d; // free a scalar variable delete [ ] array; 30
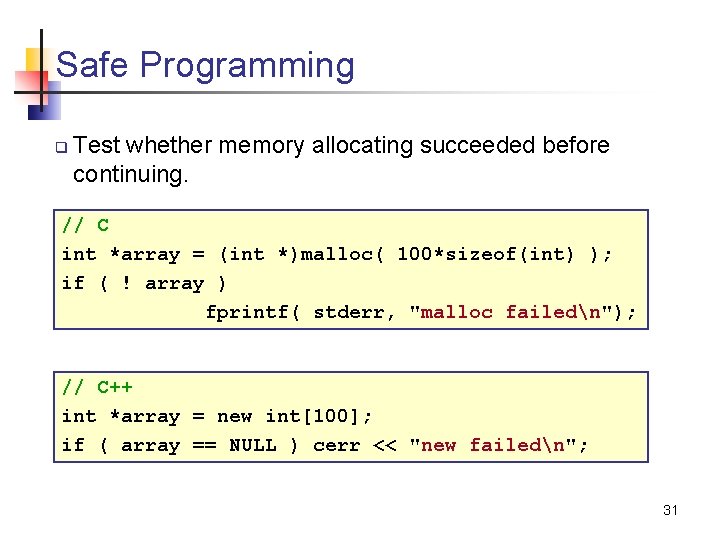
Safe Programming q Test whether memory allocating succeeded before continuing. // C int *array = (int *)malloc( 100*sizeof(int) ); if ( ! array ) fprintf( stderr, "malloc failedn"); // C++ int *array = new int[100]; if ( array == NULL ) cerr << "new failedn"; 31
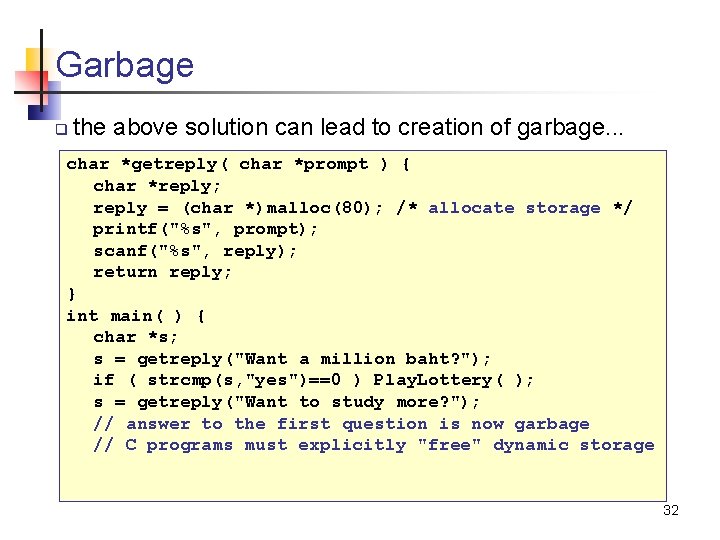
Garbage q the above solution can lead to creation of garbage. . . char *getreply( char *prompt ) { char *reply; reply = (char *)malloc(80); /* allocate storage */ printf("%s", prompt); scanf("%s", reply); return reply; } int main( ) { char *s; s = getreply("Want a million baht? "); if ( strcmp(s, "yes")==0 ) Play. Lottery( ); s = getreply("Want to study more? "); // answer to the first question is now garbage // C programs must explicitly "free" dynamic storage 32
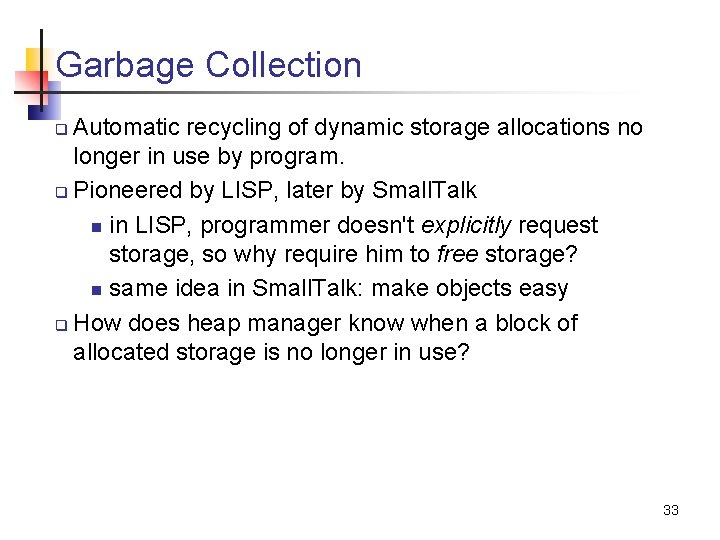
Garbage Collection Automatic recycling of dynamic storage allocations no longer in use by program. q Pioneered by LISP, later by Small. Talk n in LISP, programmer doesn't explicitly request storage, so why require him to free storage? n same idea in Small. Talk: make objects easy q How does heap manager know when a block of allocated storage is no longer in use? q 33
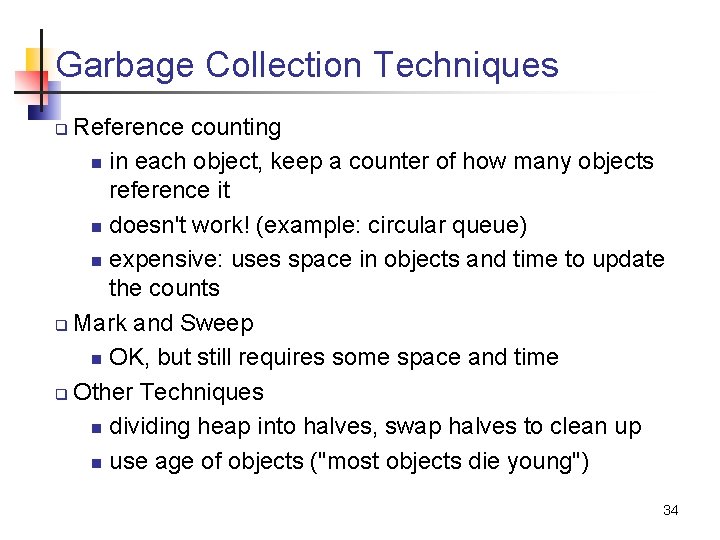
Garbage Collection Techniques Reference counting n in each object, keep a counter of how many objects reference it n doesn't work! (example: circular queue) n expensive: uses space in objects and time to update the counts q Mark and Sweep n OK, but still requires some space and time q Other Techniques n dividing heap into halves, swap halves to clean up n use age of objects ("most objects die young") q 34
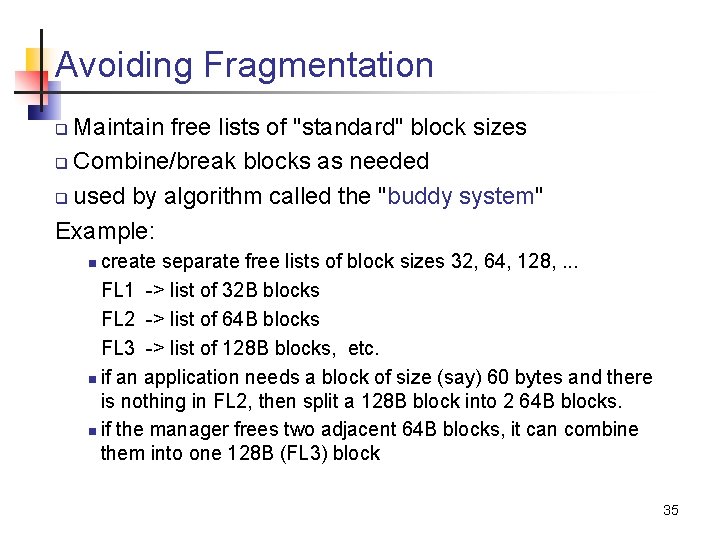
Avoiding Fragmentation Maintain free lists of "standard" block sizes q Combine/break blocks as needed q used by algorithm called the "buddy system" Example: q create separate free lists of block sizes 32, 64, 128, . . . FL 1 -> list of 32 B blocks FL 2 -> list of 64 B blocks FL 3 -> list of 128 B blocks, etc. n if an application needs a block of size (say) 60 bytes and there is nothing in FL 2, then split a 128 B block into 2 64 B blocks. n if the manager frees two adjacent 64 B blocks, it can combine them into one 128 B (FL 3) block n 35
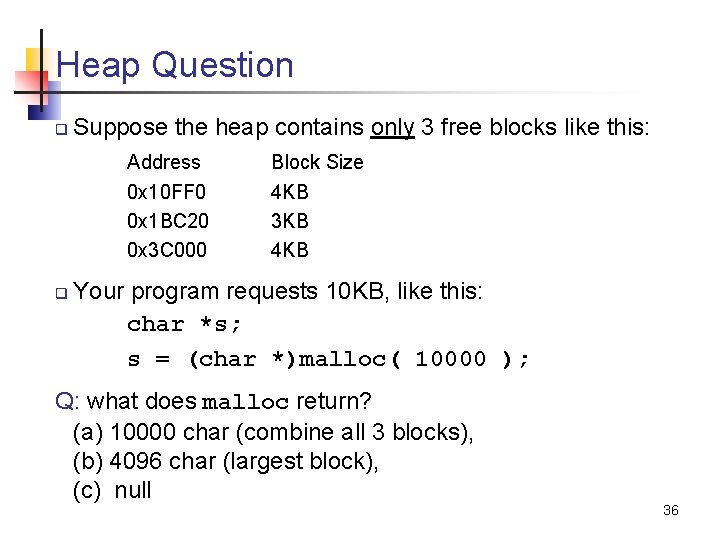
Heap Question q Suppose the heap contains only 3 free blocks like this: Address 0 x 10 FF 0 0 x 1 BC 20 0 x 3 C 000 q Block Size 4 KB 3 KB 4 KB Your program requests 10 KB, like this: char *s; s = (char *)malloc( 10000 ); Q: what does malloc return? (a) 10000 char (combine all 3 blocks), (b) 4096 char (largest block), (c) null 36
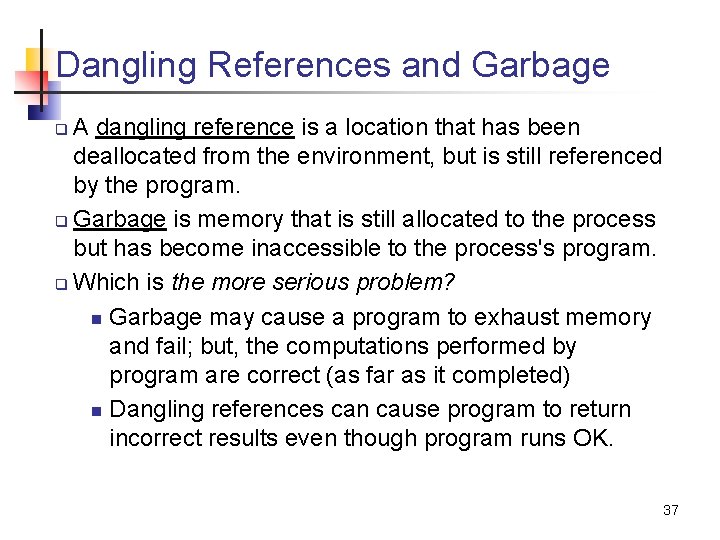
Dangling References and Garbage A dangling reference is a location that has been deallocated from the environment, but is still referenced by the program. q Garbage is memory that is still allocated to the process but has become inaccessible to the process's program. q Which is the more serious problem? n Garbage may cause a program to exhaust memory and fail; but, the computations performed by program are correct (as far as it completed) n Dangling references can cause program to return incorrect results even though program runs OK. q 37
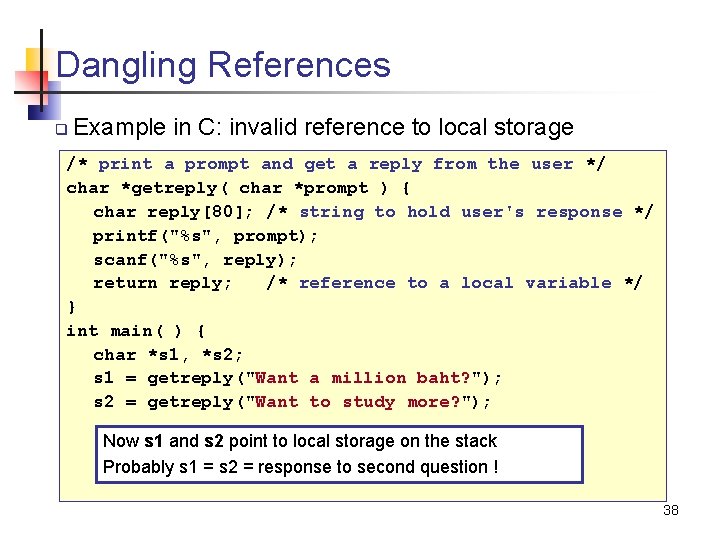
Dangling References q Example in C: invalid reference to local storage /* print a prompt and get a reply from the user */ char *getreply( char *prompt ) { char reply[80]; /* string to hold user's response */ printf("%s", prompt); scanf("%s", reply); return reply; /* reference to a local variable */ } int main( ) { char *s 1, *s 2; s 1 = getreply("Want a million baht? "); s 2 = getreply("Want to study more? "); Now s 1 and s 2 point to local storage on the stack Probably s 1 = s 2 = response to second question ! 38
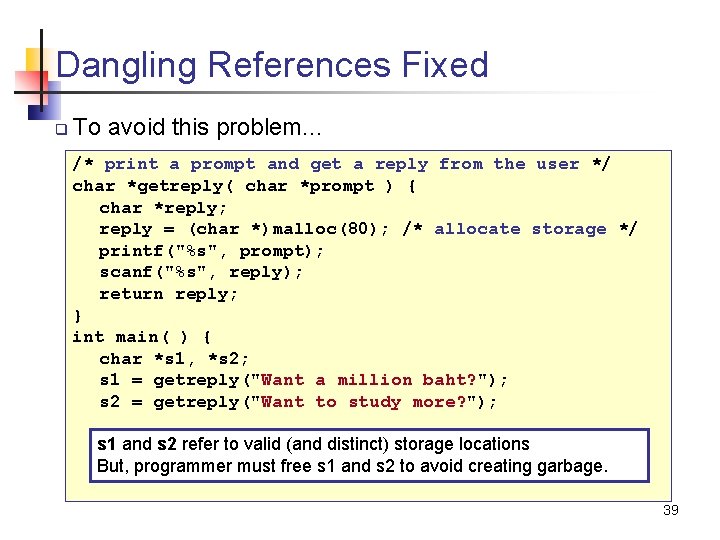
Dangling References Fixed q To avoid this problem. . . /* print a prompt and get a reply from the user */ char *getreply( char *prompt ) { char *reply; reply = (char *)malloc(80); /* allocate storage */ printf("%s", prompt); scanf("%s", reply); return reply; } int main( ) { char *s 1, *s 2; s 1 = getreply("Want a million baht? "); s 2 = getreply("Want to study more? "); s 1 and s 2 refer to valid (and distinct) storage locations But, programmer must free s 1 and s 2 to avoid creating garbage. 39
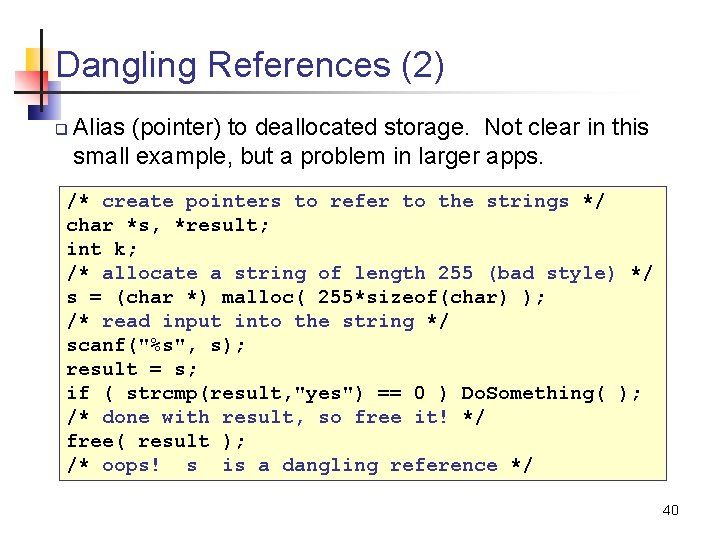
Dangling References (2) q Alias (pointer) to deallocated storage. Not clear in this small example, but a problem in larger apps. /* create pointers to refer to the strings */ char *s, *result; int k; /* allocate a string of length 255 (bad style) */ s = (char *) malloc( 255*sizeof(char) ); /* read input into the string */ scanf("%s", s); result = s; if ( strcmp(result, "yes") == 0 ) Do. Something( ); /* done with result, so free it! */ free( result ); /* oops! s is a dangling reference */ 40
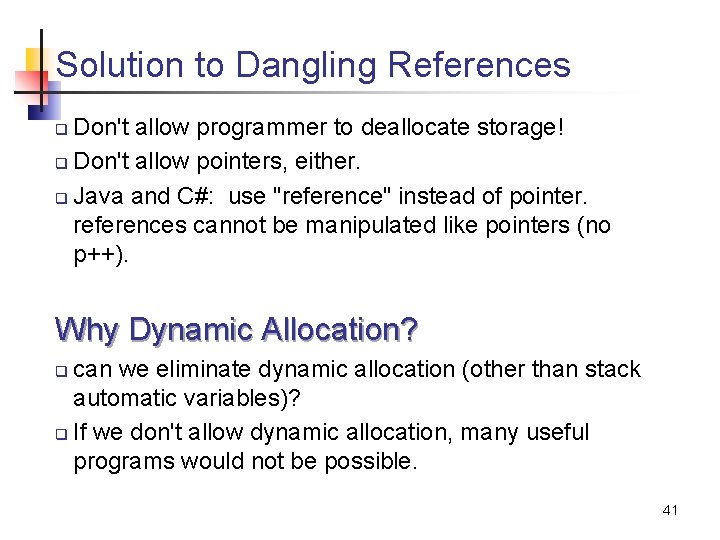
Solution to Dangling References Don't allow programmer to deallocate storage! q Don't allow pointers, either. q Java and C#: use "reference" instead of pointer. references cannot be manipulated like pointers (no p++). q Why Dynamic Allocation? can we eliminate dynamic allocation (other than stack automatic variables)? q If we don't allow dynamic allocation, many useful programs would not be possible. q 41
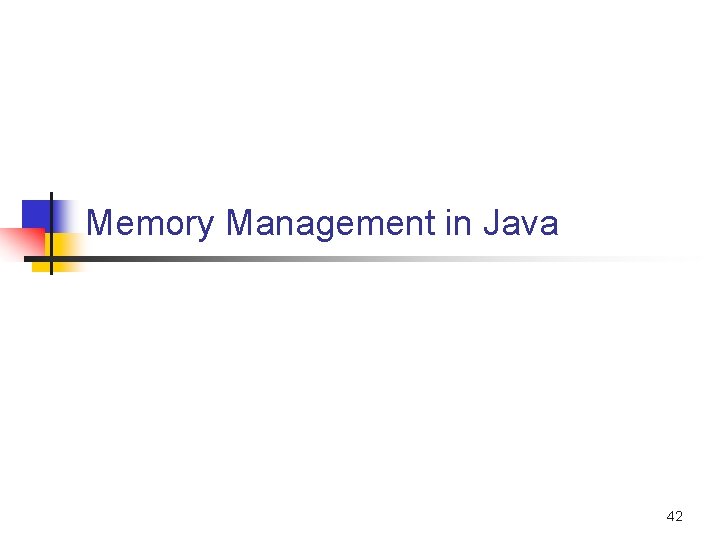
Memory Management in Java 42
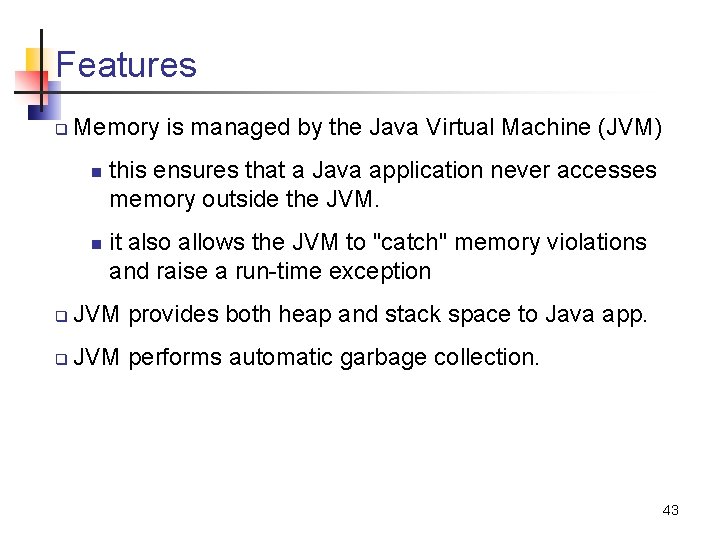
Features q Memory is managed by the Java Virtual Machine (JVM) n n this ensures that a Java application never accesses memory outside the JVM. it also allows the JVM to "catch" memory violations and raise a run-time exception q JVM provides both heap and stack space to Java app. q JVM performs automatic garbage collection. 43
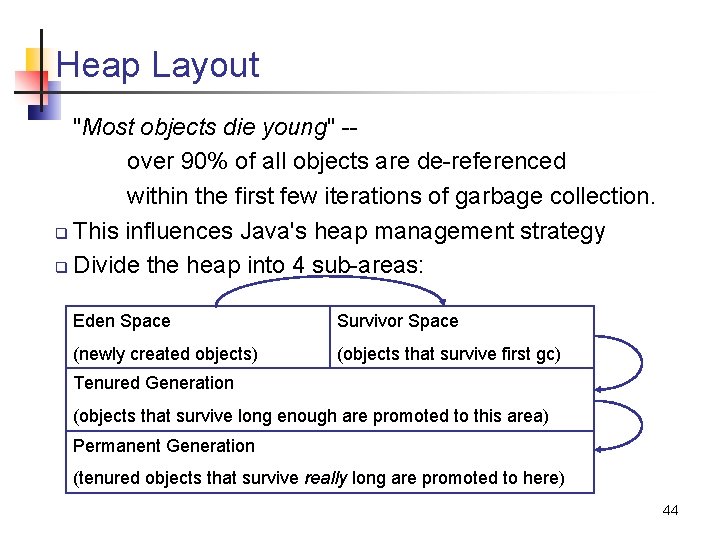
Heap Layout "Most objects die young" -over 90% of all objects are de-referenced within the first few iterations of garbage collection. q This influences Java's heap management strategy q Divide the heap into 4 sub-areas: Eden Space Survivor Space (newly created objects) (objects that survive first gc) Tenured Generation (objects that survive long enough are promoted to this area) Permanent Generation (tenured objects that survive really long are promoted to here) 44
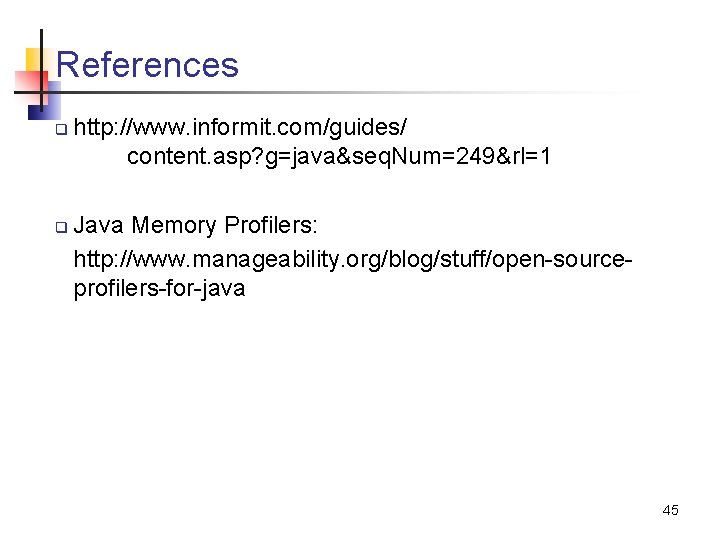
References q q http: //www. informit. com/guides/ content. asp? g=java&seq. Num=249&rl=1 Java Memory Profilers: http: //www. manageability. org/blog/stuff/open-sourceprofilers-for-java 45