MachineLevel Programming 6 Structured Data Topics n Structs
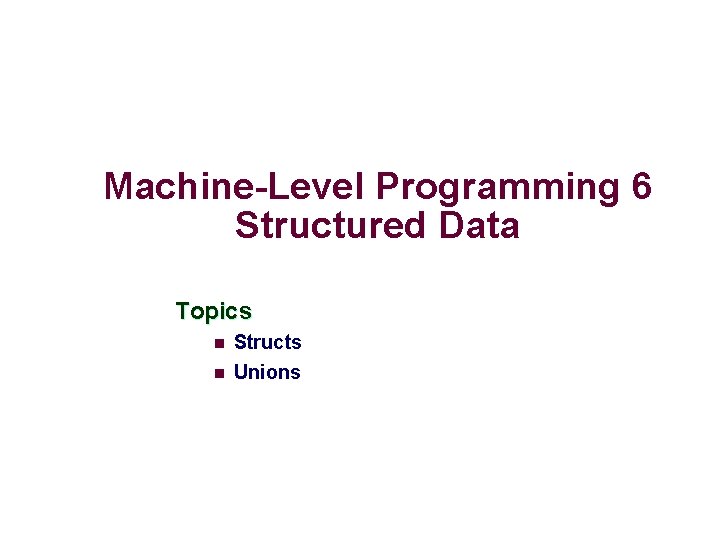
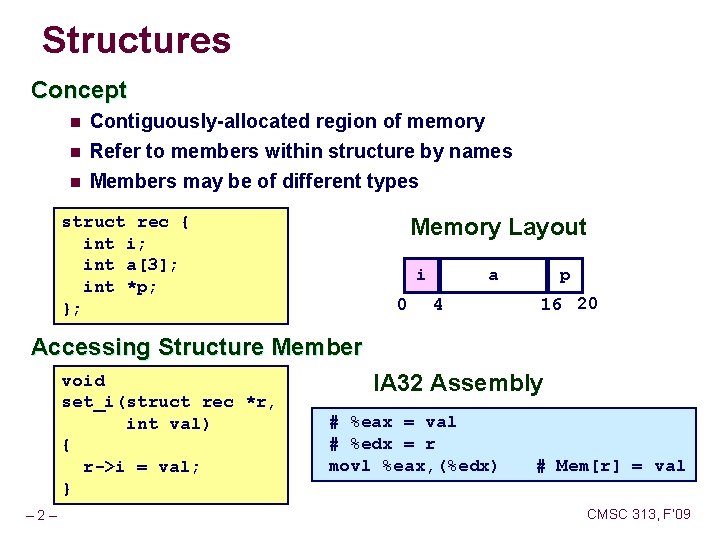
![Generating Pointer to Struct. Member r struct rec { int i; int a[3]; int Generating Pointer to Struct. Member r struct rec { int i; int a[3]; int](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-3.jpg)
![Structure Referencing (Cont. ) C Code i struct rec { int i; int a[3]; Structure Referencing (Cont. ) C Code i struct rec { int i; int a[3];](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-4.jpg)
![The sizeof( ) a struct rec { int i; int a[3]; int *p; struct The sizeof( ) a struct rec { int i; int a[3]; int *p; struct](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-5.jpg)
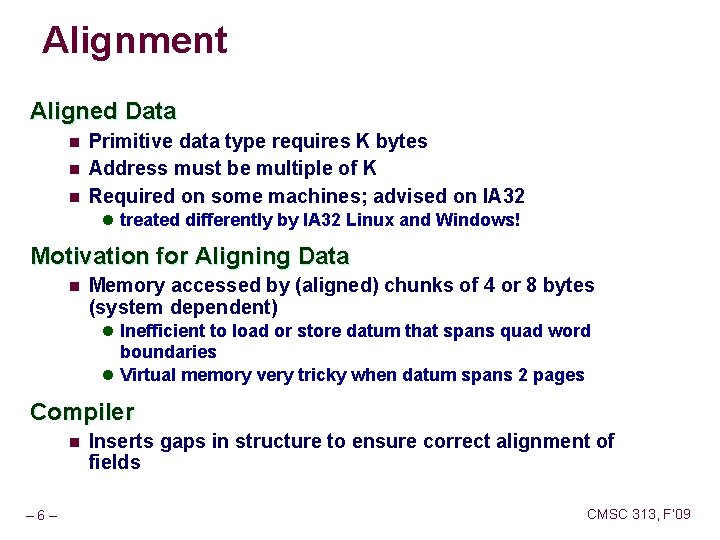
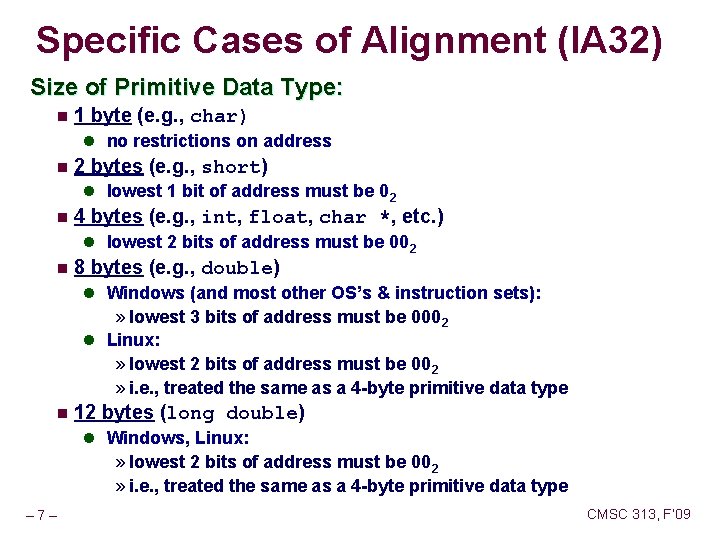
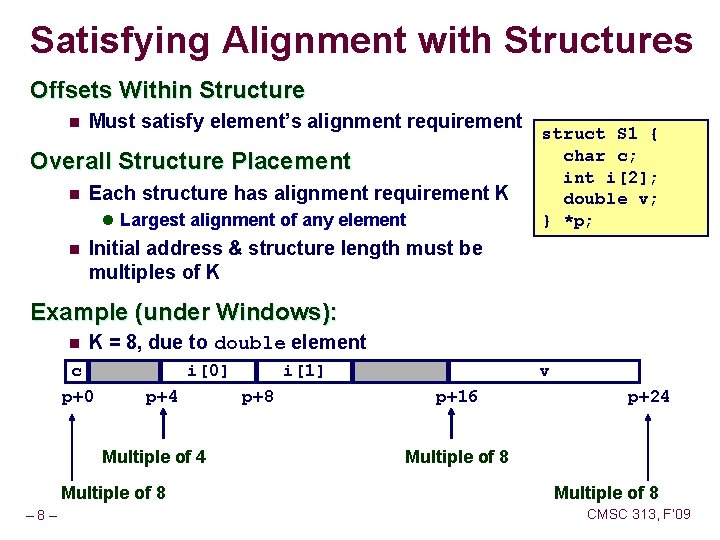
![Different Alignment Conventions struct S 1 { char c; int i[2]; double v; } Different Alignment Conventions struct S 1 { char c; int i[2]; double v; }](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-9.jpg)
![Overall Alignment Requirement struct S 2 { double x; int i[2]; char c; } Overall Alignment Requirement struct S 2 { double x; int i[2]; char c; }](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-10.jpg)
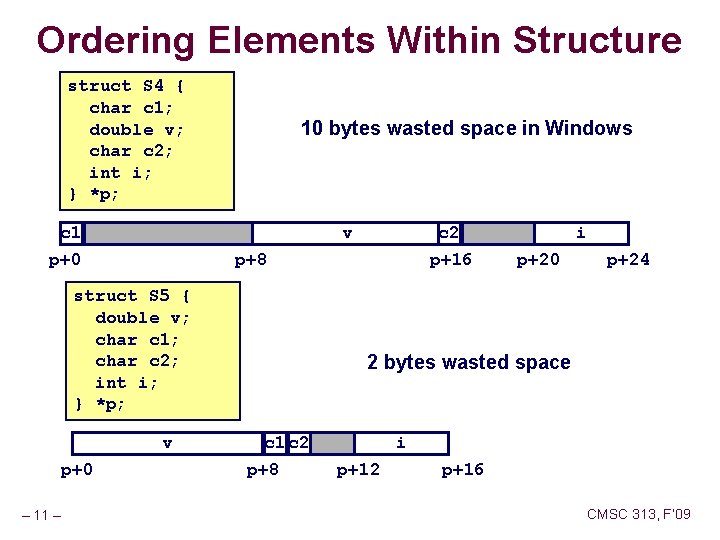
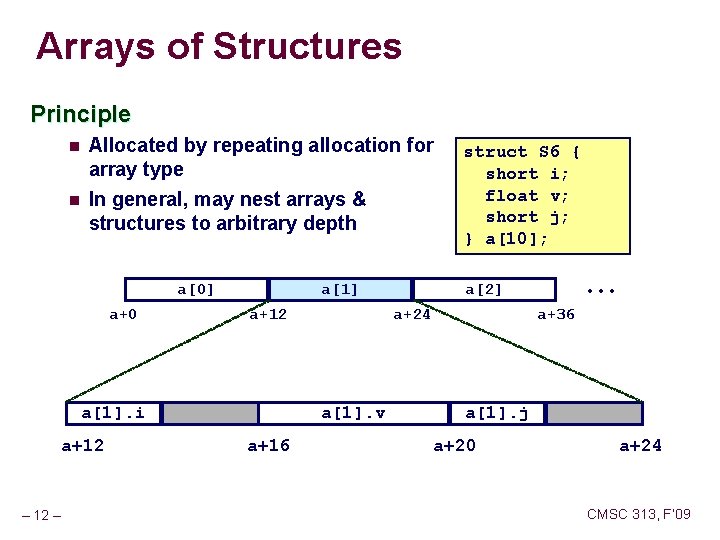
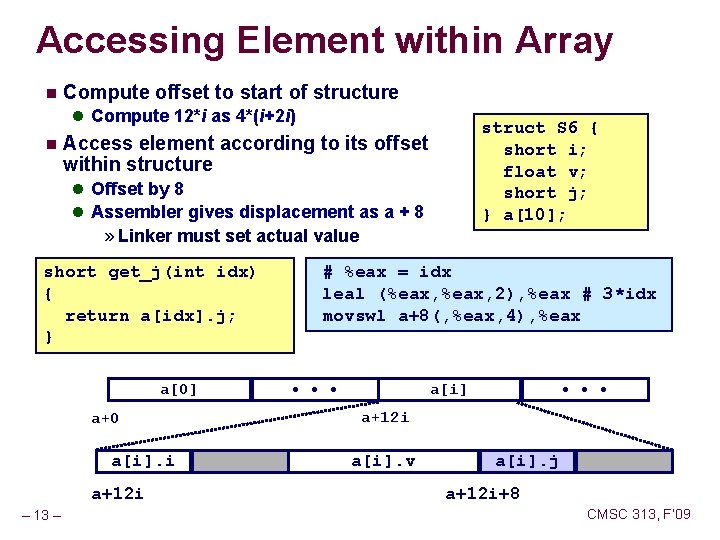
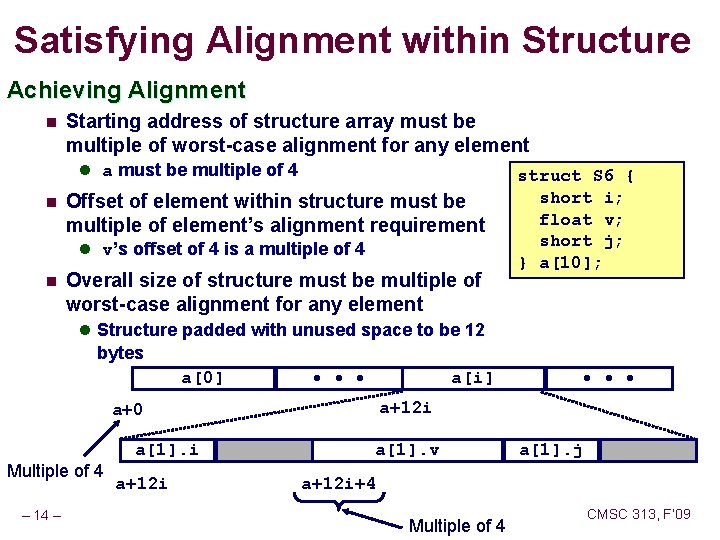
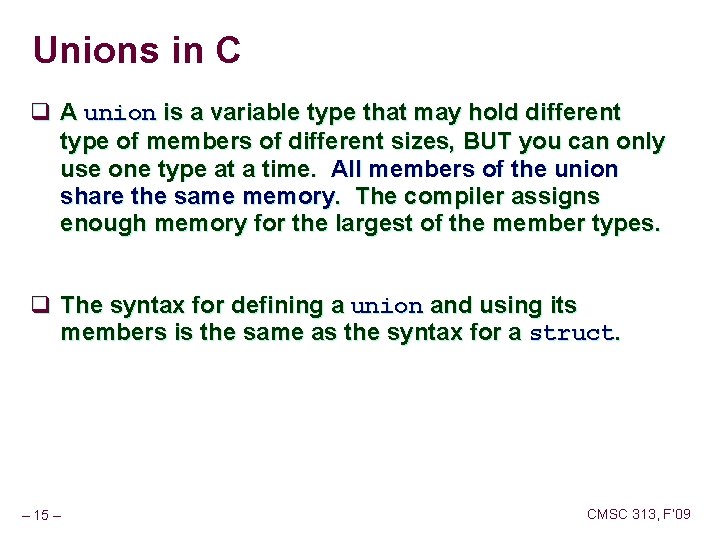
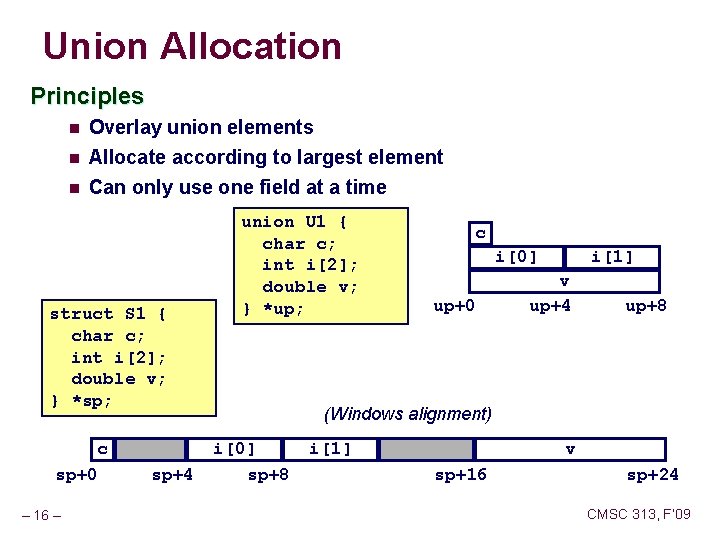
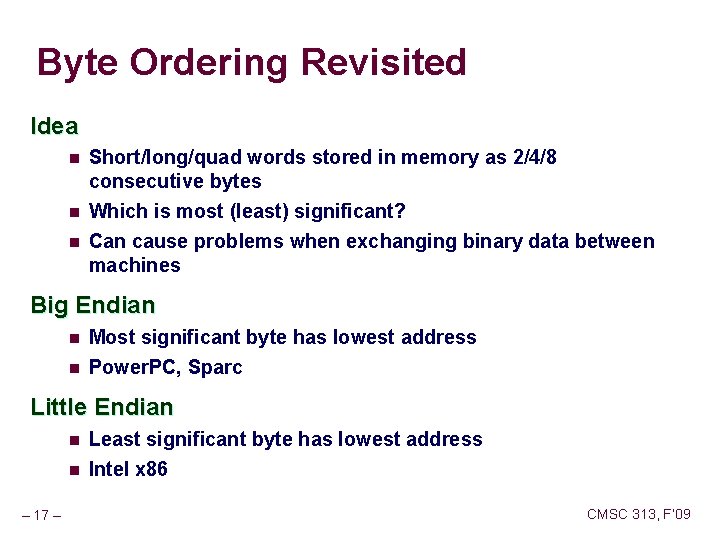
![Byte Ordering Example union { unsigned } dw; char c[8]; short s[4]; int i[2]; Byte Ordering Example union { unsigned } dw; char c[8]; short s[4]; int i[2];](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-18.jpg)
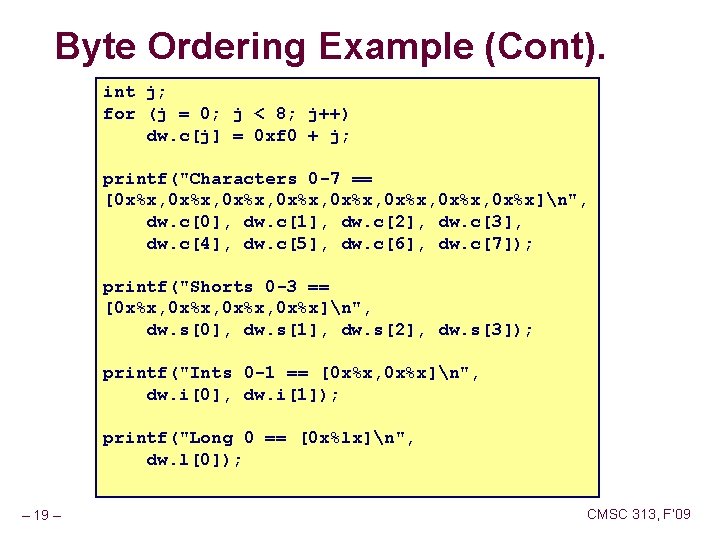
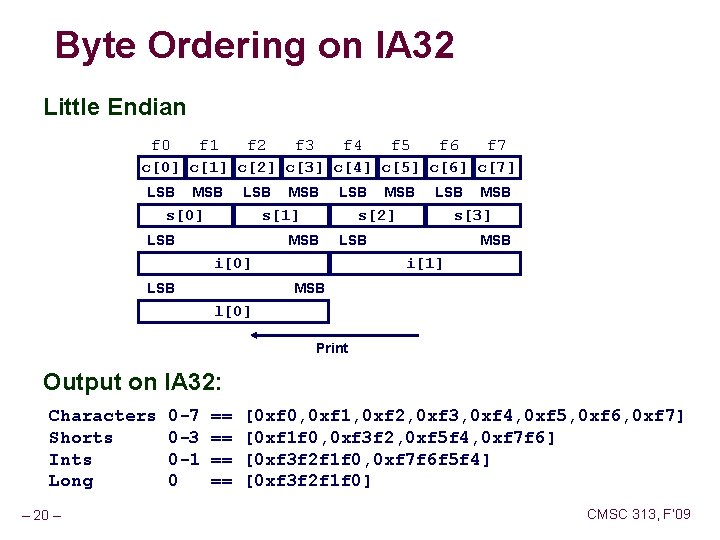
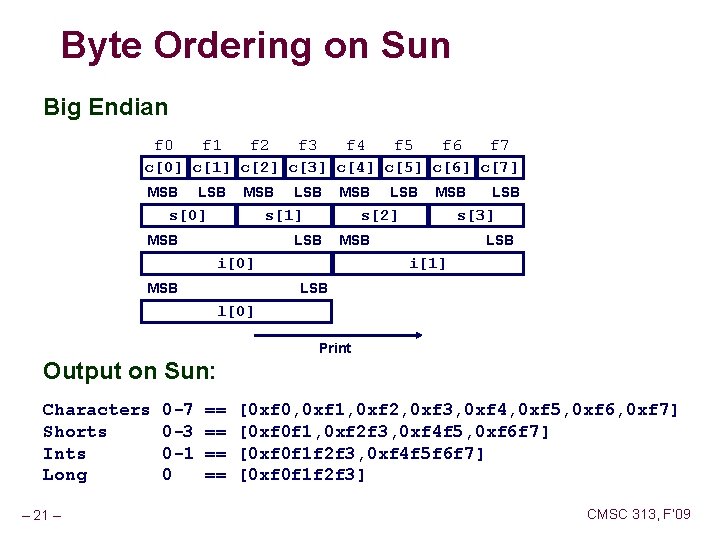
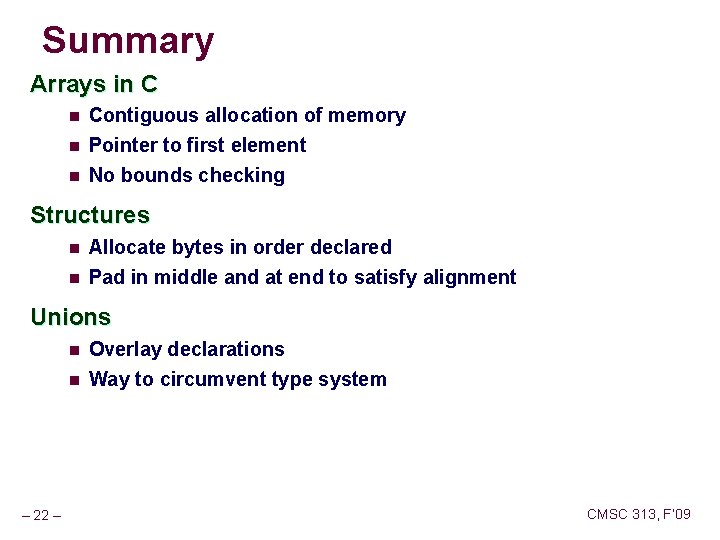
- Slides: 22
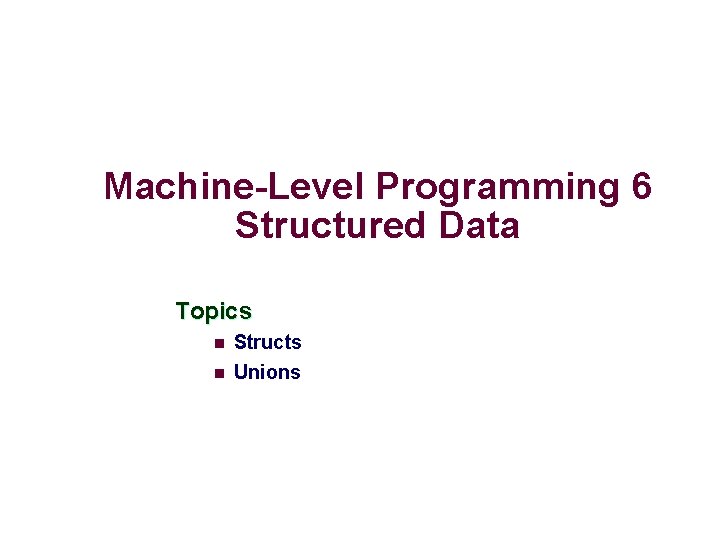
Machine-Level Programming 6 Structured Data Topics n Structs n Unions
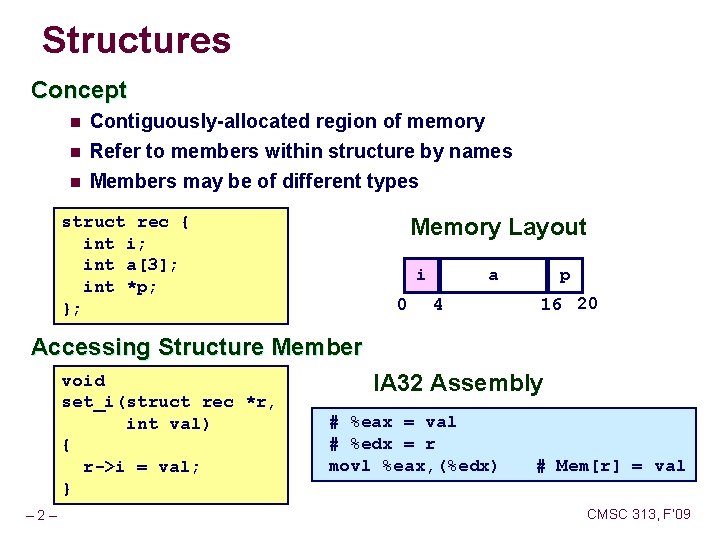
Structures Concept n Contiguously-allocated region of memory n Refer to members within structure by names Members may be of different types n struct rec { int i; int a[3]; int *p; }; Memory Layout i 0 a 4 p 16 20 Accessing Structure Member void set_i(struct rec *r, int val) { r->i = val; } – 2– IA 32 Assembly # %eax = val # %edx = r movl %eax, (%edx) # Mem[r] = val CMSC 313, F’ 09
![Generating Pointer to Struct Member r struct rec int i int a3 int Generating Pointer to Struct. Member r struct rec { int i; int a[3]; int](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-3.jpg)
Generating Pointer to Struct. Member r struct rec { int i; int a[3]; int *p; }; Generating Pointer to Array Element n Offset of each structure member determined at compile time i 0 a p 4 16 r + 4*idx int * find_a (struct rec *r, int idx) { return &r->a[idx]; } # %ecx = idx # %edx = r leal 0(, %ecx, 4), %eax # 4*idx leal 4(%eax, %edx), %eax # r+4*idx+4 – 3– CMSC 313, F’ 09
![Structure Referencing Cont C Code i struct rec int i int a3 Structure Referencing (Cont. ) C Code i struct rec { int i; int a[3];](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-4.jpg)
Structure Referencing (Cont. ) C Code i struct rec { int i; int a[3]; int *p; }; 0 a 4 i 0 void set_p(struct rec *r) { r->p = &r->a[r->i]; } p 16 a 4 16 Element i # %edx = r movl (%edx), %ecx leal 0(, %ecx, 4), %eax leal 4(%edx, %eax), %eax movl %eax, 16(%edx) – 4– # # r->i 4*(r->i) r+4+4*(r->i) Update r->p CMSC 313, F’ 09
![The sizeof a struct rec int i int a3 int p struct The sizeof( ) a struct rec { int i; int a[3]; int *p; struct](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-5.jpg)
The sizeof( ) a struct rec { int i; int a[3]; int *p; struct S 1 { char c; int i[2]; double v; }; The size of a struct is not always what is seems. printf(“ The sizeof struct rec = %dn”, sizeof(struct rec)); printf(“ The sizeof struct S 1 = %dn”, sizeof(struct S 1)); /* output on Linux */ The sizeof struct rec = 20 The sizeof struct S 1 = 20 – 5– CMSC 313, F’ 09
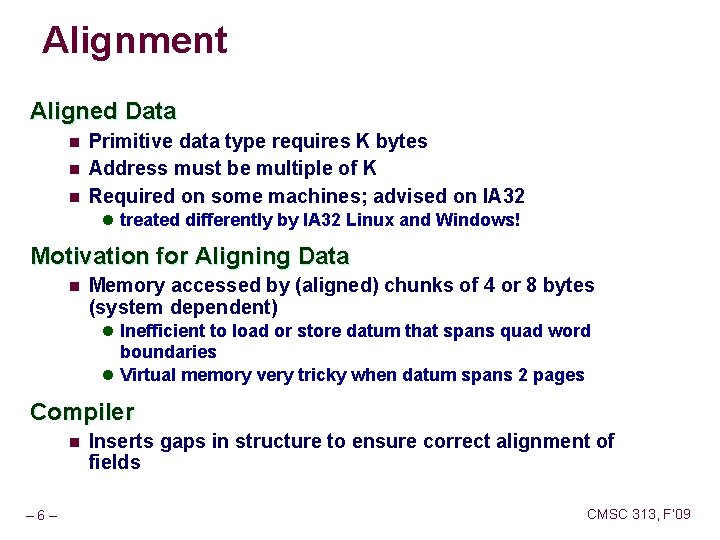
Alignment Aligned Data n n n Primitive data type requires K bytes Address must be multiple of K Required on some machines; advised on IA 32 l treated differently by IA 32 Linux and Windows! Motivation for Aligning Data n Memory accessed by (aligned) chunks of 4 or 8 bytes (system dependent) l Inefficient to load or store datum that spans quad word boundaries l Virtual memory very tricky when datum spans 2 pages Compiler n – 6– Inserts gaps in structure to ensure correct alignment of fields CMSC 313, F’ 09
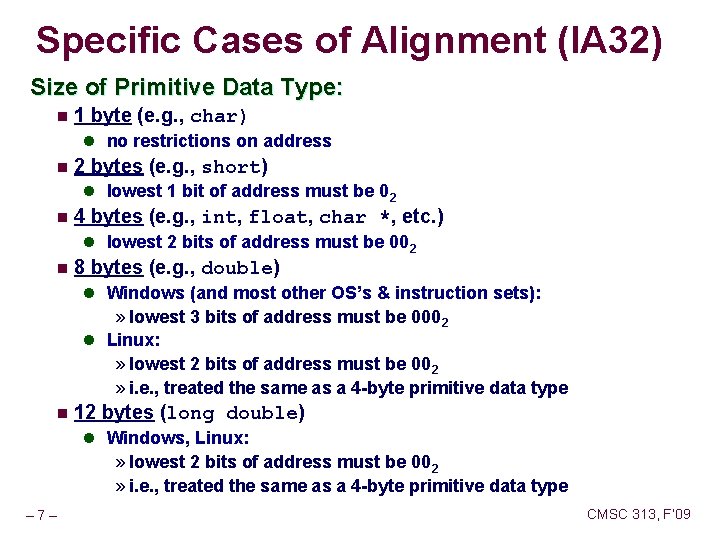
Specific Cases of Alignment (IA 32) Size of Primitive Data Type: n 1 byte (e. g. , char) l no restrictions on address n 2 bytes (e. g. , short) l lowest 1 bit of address must be 02 n 4 bytes (e. g. , int, float, char *, etc. ) l lowest 2 bits of address must be 002 n 8 bytes (e. g. , double) l Windows (and most other OS’s & instruction sets): » lowest 3 bits of address must be 0002 l Linux: » lowest 2 bits of address must be 002 » i. e. , treated the same as a 4 -byte primitive data type n 12 bytes (long double) l Windows, Linux: » lowest 2 bits of address must be 002 » i. e. , treated the same as a 4 -byte primitive data type – 7– CMSC 313, F’ 09
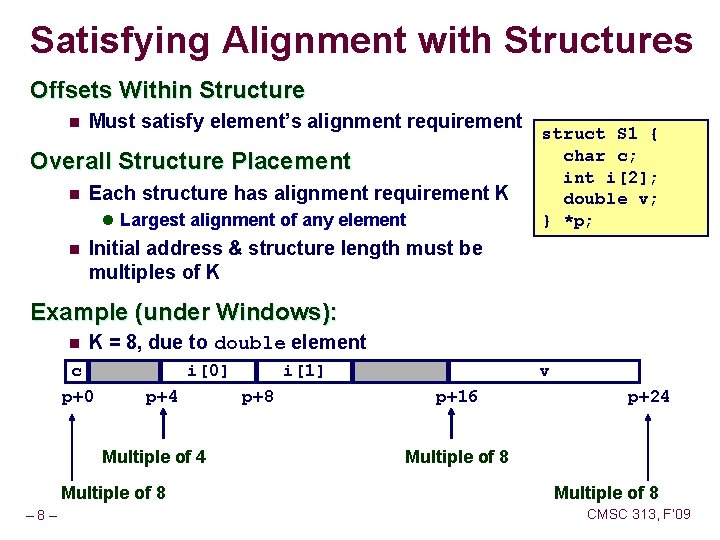
Satisfying Alignment with Structures Offsets Within Structure n Must satisfy element’s alignment requirement Overall Structure Placement n Each structure has alignment requirement K l Largest alignment of any element n struct S 1 { char c; int i[2]; double v; } *p; Initial address & structure length must be multiples of K Example (under Windows): n K = 8, due to double element c p+0 i[0] p+4 Multiple of 8 – 8– i[1] p+8 v p+16 p+24 Multiple of 8 CMSC 313, F’ 09
![Different Alignment Conventions struct S 1 char c int i2 double v Different Alignment Conventions struct S 1 { char c; int i[2]; double v; }](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-9.jpg)
Different Alignment Conventions struct S 1 { char c; int i[2]; double v; } *p; IA 32 Windows: n K = 8, due to double element c p+0 i[0] p+4 i[1] v p+8 p+16 Multiple of 4 Multiple of 8 p+24 Multiple of 8 IA 32 Linux n K = 4; double treated like a 4 -byte data type c p+0 – 9– i[0] p+4 Multiple of 4 i[1] p+8 v p+12 Multiple of 4 p+20 Multiple of 4 CMSC 313, F’ 09
![Overall Alignment Requirement struct S 2 double x int i2 char c Overall Alignment Requirement struct S 2 { double x; int i[2]; char c; }](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-10.jpg)
Overall Alignment Requirement struct S 2 { double x; int i[2]; char c; } *p; p must be multiple of: 8 for IA 32 Windows 4 for IA 32 Linux x i[0] p+0 p+8 struct S 3 { float x[2]; int i[2]; char c; } *p; x[0] p+0 – 10 – p+12 c p+16 Windows: p+24 Linux: p+20 p must be multiple of 4 (all cases) x[1] p+4 i[1] i[0] p+8 i[1] p+12 c p+16 p+20 CMSC 313, F’ 09
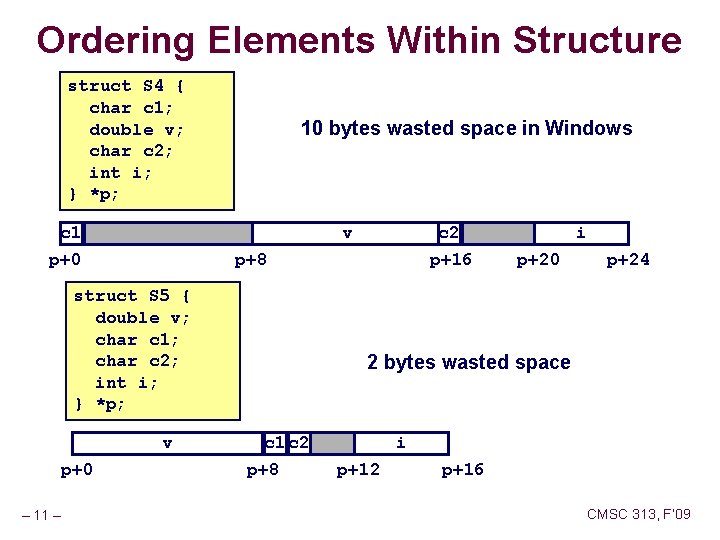
Ordering Elements Within Structure struct S 4 { char c 1; double v; char c 2; int i; } *p; 10 bytes wasted space in Windows c 1 v p+0 p+8 struct S 5 { double v; char c 1; char c 2; int i; } *p; v p+0 – 11 – c 2 p+16 i p+20 p+24 2 bytes wasted space c 1 c 2 p+8 i p+12 p+16 CMSC 313, F’ 09
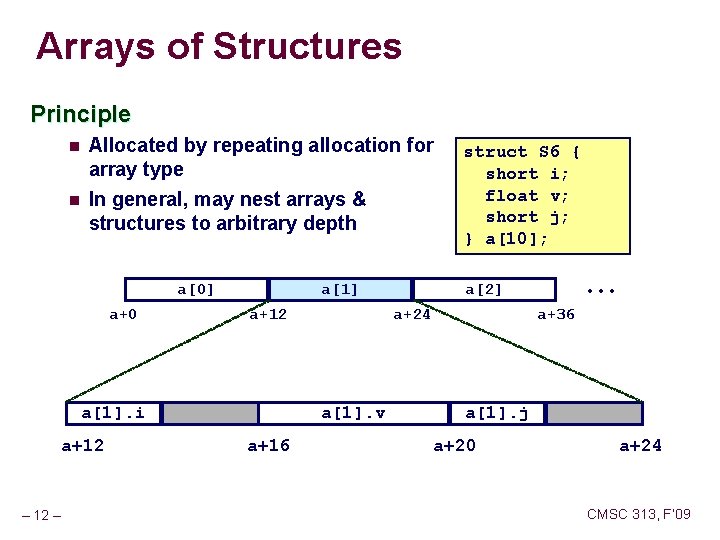
Arrays of Structures Principle n n Allocated by repeating allocation for array type In general, may nest arrays & structures to arbitrary depth a[0] a+0 a[1] a+12 a[1]. i a+12 – • • • a[2] a+24 a[1]. v a+16 struct S 6 { short i; float v; short j; } a[10]; a+36 a[1]. j a+20 a+24 CMSC 313, F’ 09
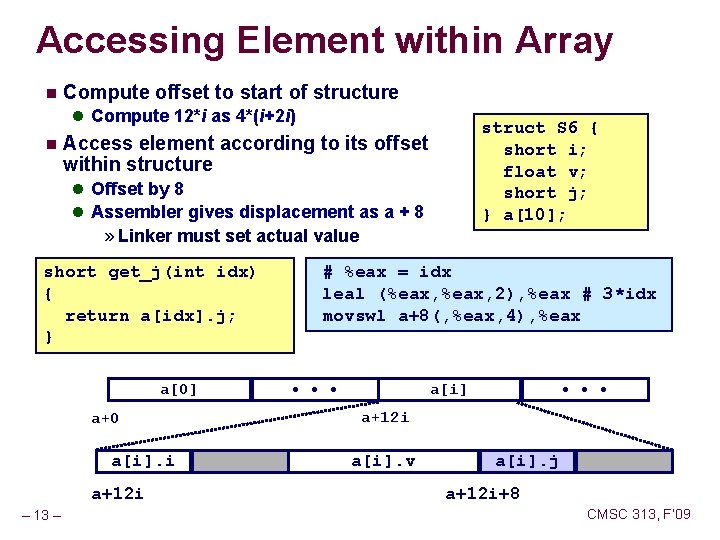
Accessing Element within Array n Compute offset to start of structure l Compute 12*i as 4*(i+2 i) n struct S 6 { short i; float v; short j; } a[10]; Access element according to its offset within structure l Offset by 8 l Assembler gives displacement as a + 8 » Linker must set actual value short get_j(int idx) { return a[idx]. j; } a[0] a+0 a[i]. i a+12 i – 13 – # %eax = idx leal (%eax, 2), %eax # 3*idx movswl a+8(, %eax, 4), %eax • • • a[i] • • • a+12 i a[i]. v a[i]. j a+12 i+8 CMSC 313, F’ 09
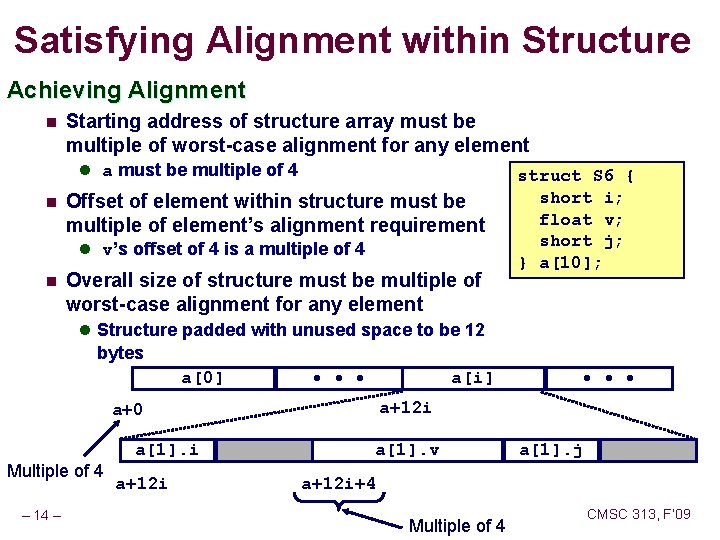
Satisfying Alignment within Structure Achieving Alignment n Starting address of structure array must be multiple of worst-case alignment for any element l a must be multiple of 4 n Offset of element within structure must be multiple of element’s alignment requirement l v’s offset of 4 is a multiple of 4 n Overall size of structure must be multiple of worst-case alignment for any element struct S 6 { short i; float v; short j; } a[10]; l Structure padded with unused space to be 12 bytes a[0] • • • a[i] a+12 i a+0 Multiple of 4 – 14 – a[1]. i a+12 i • • • a[1]. v a[1]. j a+12 i+4 Multiple of 4 CMSC 313, F’ 09
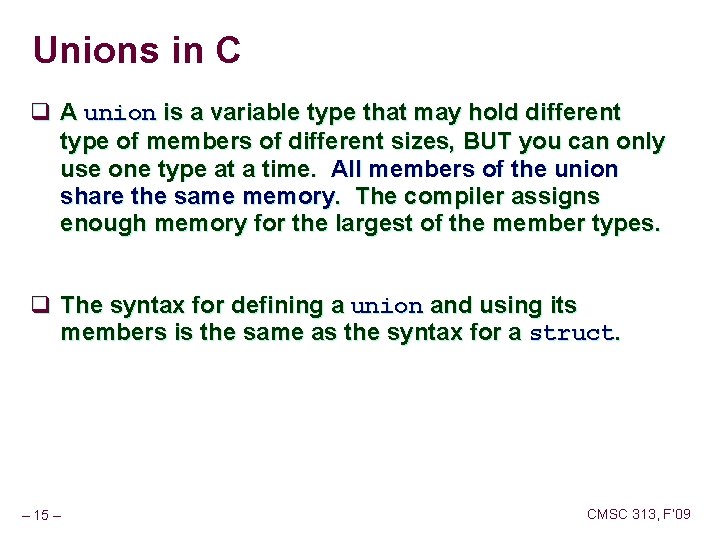
Unions in C q A union is a variable type that may hold different type of members of different sizes, BUT you can only use one type at a time. All members of the union share the same memory. The compiler assigns enough memory for the largest of the member types. q The syntax for defining a union and using its members is the same as the syntax for a struct. – 15 – CMSC 313, F’ 09
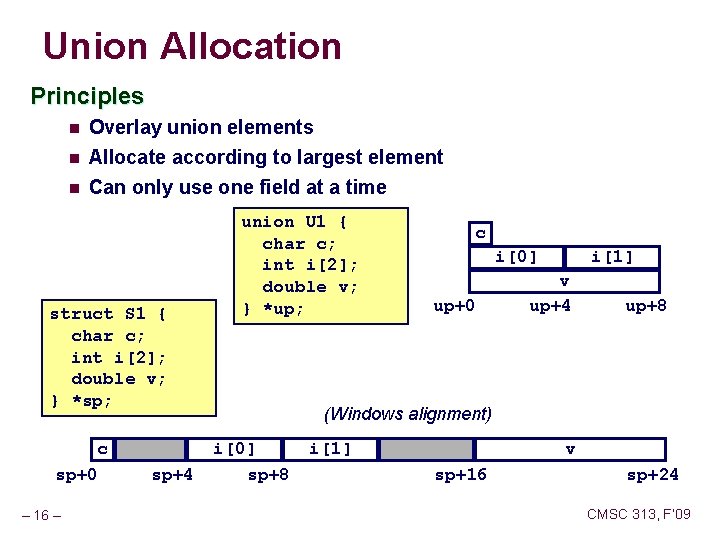
Union Allocation Principles n Overlay union elements n Allocate according to largest element Can only use one field at a time n struct S 1 { char c; int i[2]; double v; } *sp; c sp+0 – 16 – sp+4 union U 1 { char c; int i[2]; double v; } *up; c i[0] up+0 i[1] v up+4 up+8 (Windows alignment) i[0] sp+8 i[1] v sp+16 sp+24 CMSC 313, F’ 09
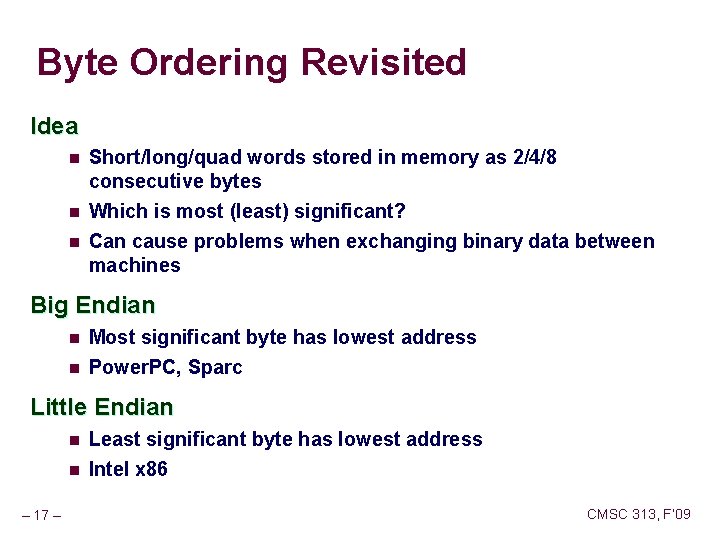
Byte Ordering Revisited Idea n n n Short/long/quad words stored in memory as 2/4/8 consecutive bytes Which is most (least) significant? Can cause problems when exchanging binary data between machines Big Endian n n Most significant byte has lowest address Power. PC, Sparc Little Endian n n – 17 – Least significant byte has lowest address Intel x 86 CMSC 313, F’ 09
![Byte Ordering Example union unsigned dw char c8 short s4 int i2 Byte Ordering Example union { unsigned } dw; char c[8]; short s[4]; int i[2];](https://slidetodoc.com/presentation_image_h2/10d9a2b7530bae33530681ca4e631f5b/image-18.jpg)
Byte Ordering Example union { unsigned } dw; char c[8]; short s[4]; int i[2]; long l[1]; c[0] c[1] c[2] c[3] c[4] c[5] c[6] c[7] s[0] s[1] s[2] s[3] i[0] i[1] l[0] – 18 – CMSC 313, F’ 09
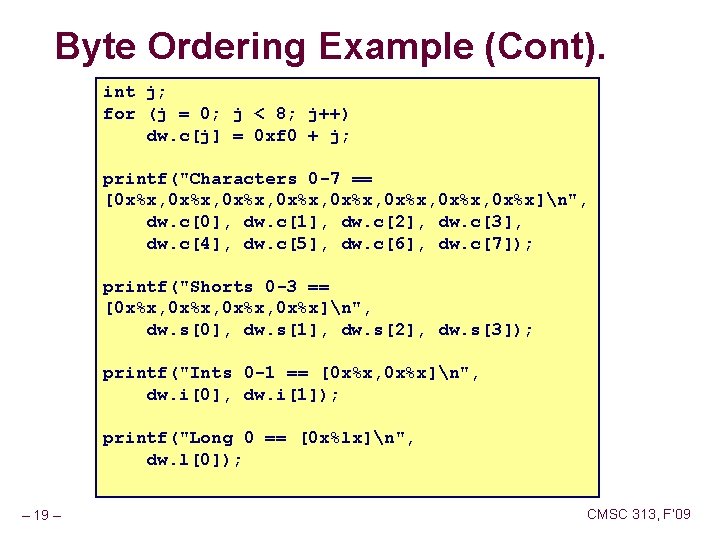
Byte Ordering Example (Cont). int j; for (j = 0; j < 8; j++) dw. c[j] = 0 xf 0 + j; printf("Characters 0 -7 == [0 x%x, 0 x%x, 0 x%x]n", dw. c[0], dw. c[1], dw. c[2], dw. c[3], dw. c[4], dw. c[5], dw. c[6], dw. c[7]); printf("Shorts 0 -3 == [0 x%x, 0 x%x]n", dw. s[0], dw. s[1], dw. s[2], dw. s[3]); printf("Ints 0 -1 == [0 x%x, 0 x%x]n", dw. i[0], dw. i[1]); printf("Long 0 == [0 x%lx]n", dw. l[0]); – 19 – CMSC 313, F’ 09
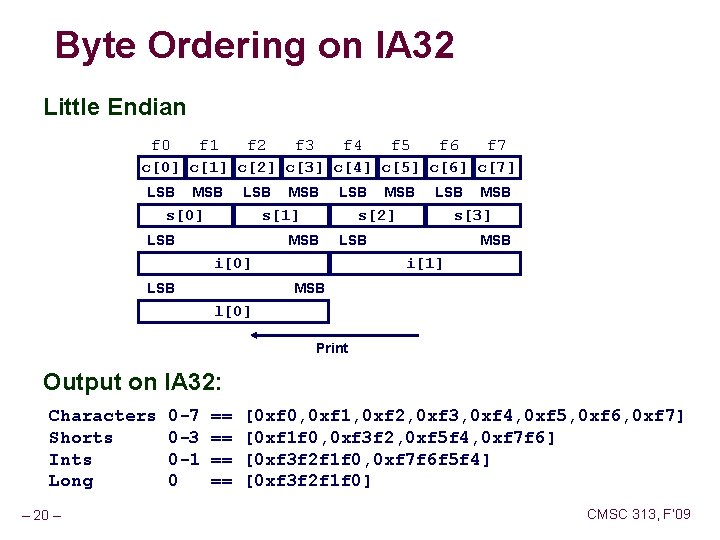
Byte Ordering on IA 32 Little Endian f 0 f 1 f 2 f 3 f 4 f 5 f 6 f 7 c[0] c[1] c[2] c[3] c[4] c[5] c[6] c[7] LSB MSB LSB s[0] MSB LSB s[1] LSB s[2] MSB s[3] LSB i[0] LSB MSB i[1] MSB l[0] Print Output on IA 32: Characters Shorts Ints Long – 20 – 0 -7 0 -3 0 -1 0 == == [0 xf 0, 0 xf 1, 0 xf 2, 0 xf 3, 0 xf 4, 0 xf 5, 0 xf 6, 0 xf 7] [0 xf 1 f 0, 0 xf 3 f 2, 0 xf 5 f 4, 0 xf 7 f 6] [0 xf 3 f 2 f 1 f 0, 0 xf 7 f 6 f 5 f 4] [0 xf 3 f 2 f 1 f 0] CMSC 313, F’ 09
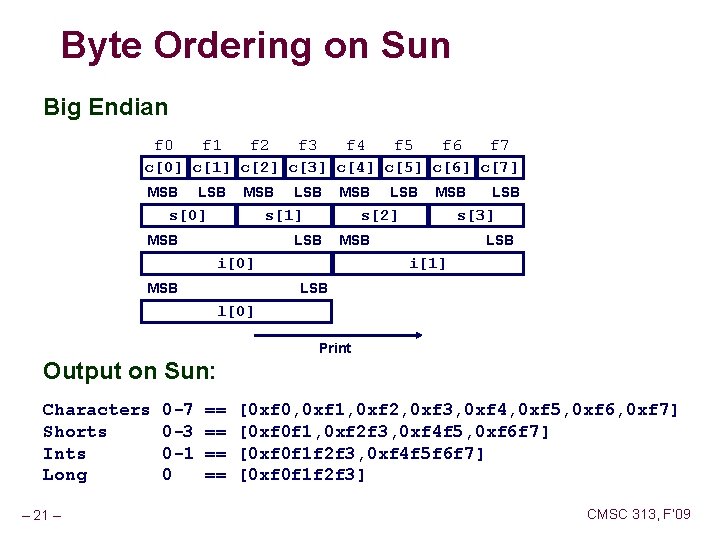
Byte Ordering on Sun Big Endian f 0 f 1 f 2 f 3 f 4 f 5 f 6 f 7 c[0] c[1] c[2] c[3] c[4] c[5] c[6] c[7] MSB LSB MSB s[0] LSB MSB s[1] MSB s[2] LSB s[3] MSB i[0] MSB LSB i[1] LSB l[0] Print Output on Sun: Characters Shorts Ints Long – 21 – 0 -7 0 -3 0 -1 0 == == [0 xf 0, 0 xf 1, 0 xf 2, 0 xf 3, 0 xf 4, 0 xf 5, 0 xf 6, 0 xf 7] [0 xf 0 f 1, 0 xf 2 f 3, 0 xf 4 f 5, 0 xf 6 f 7] [0 xf 0 f 1 f 2 f 3, 0 xf 4 f 5 f 6 f 7] [0 xf 0 f 1 f 2 f 3] CMSC 313, F’ 09
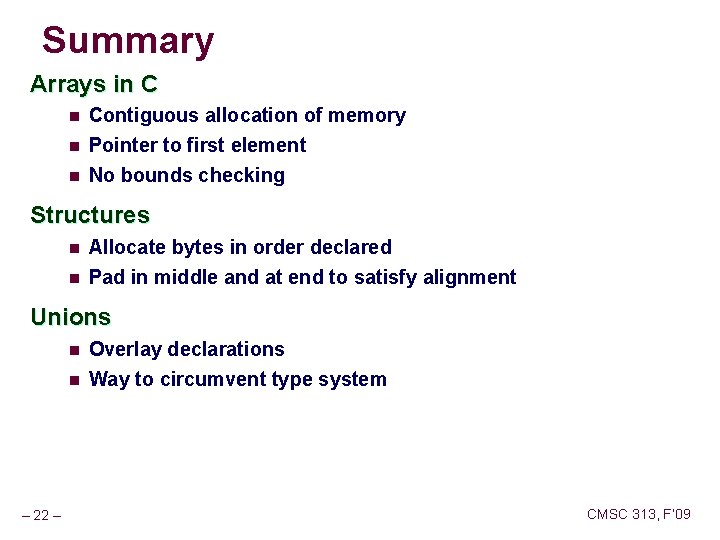
Summary Arrays in C n Contiguous allocation of memory n Pointer to first element No bounds checking n Structures n n Allocate bytes in order declared Pad in middle and at end to satisfy alignment Unions n n – 22 – Overlay declarations Way to circumvent type system CMSC 313, F’ 09