Loops For While and Do While Loop structure
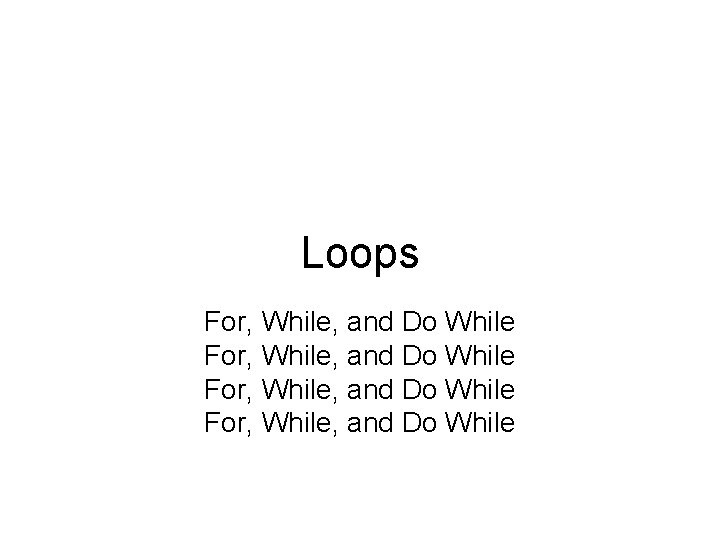
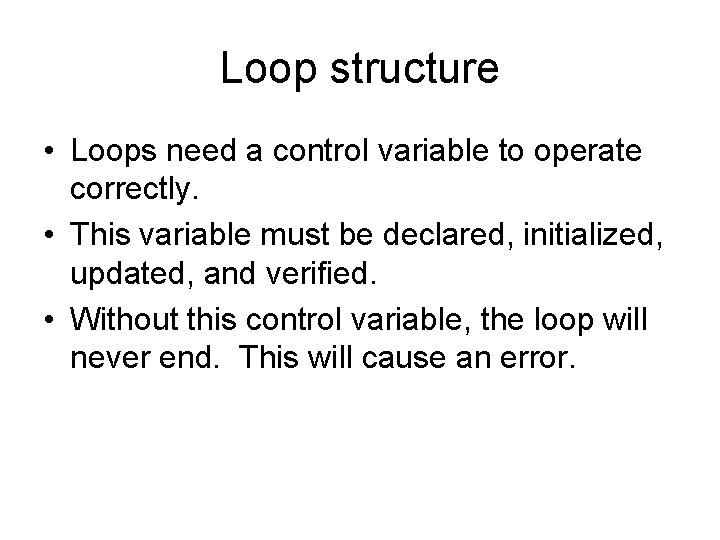
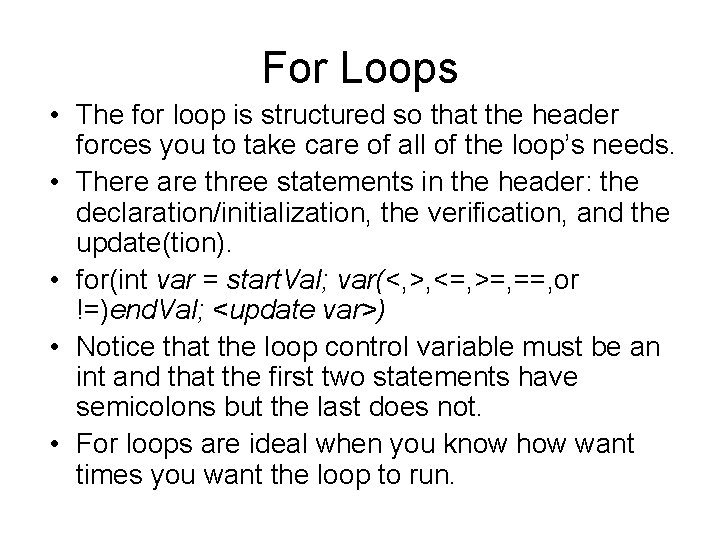
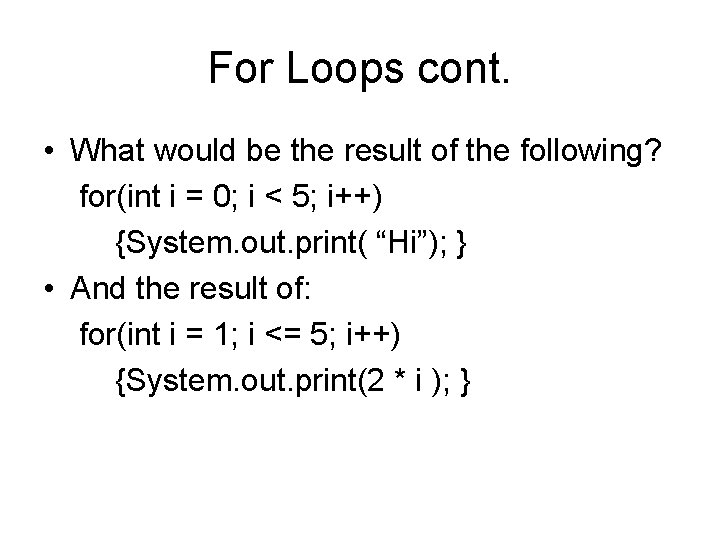
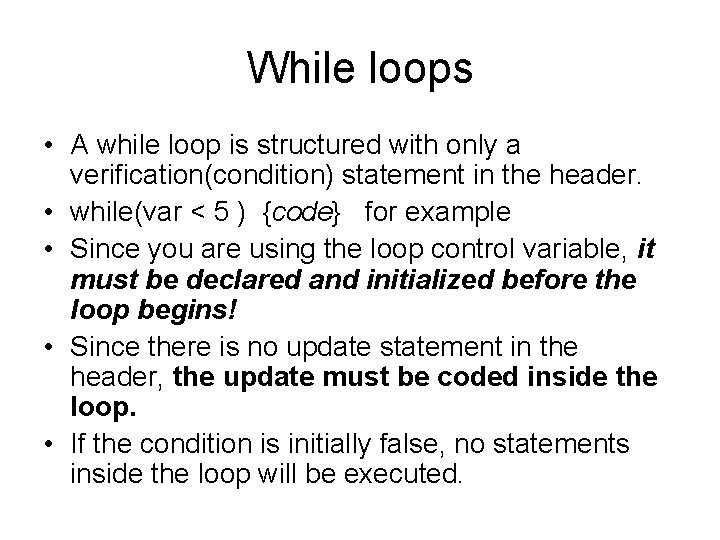
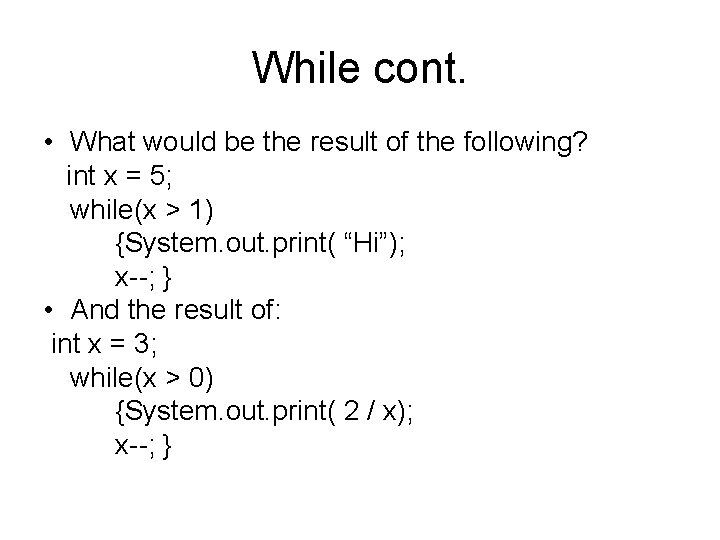
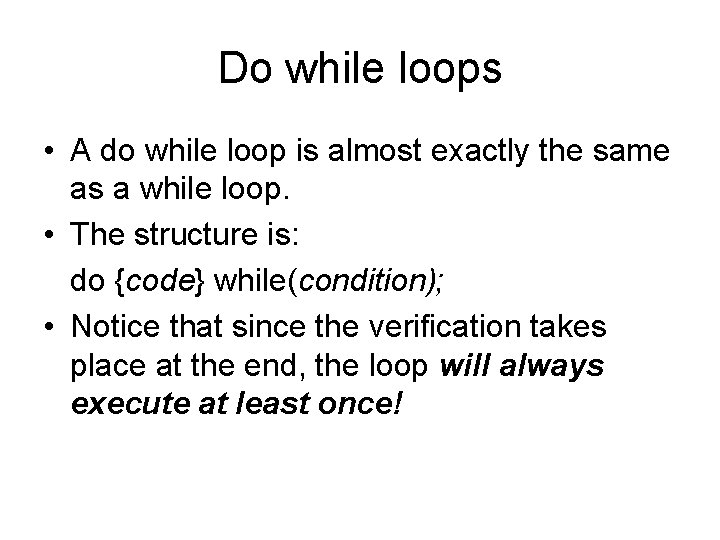
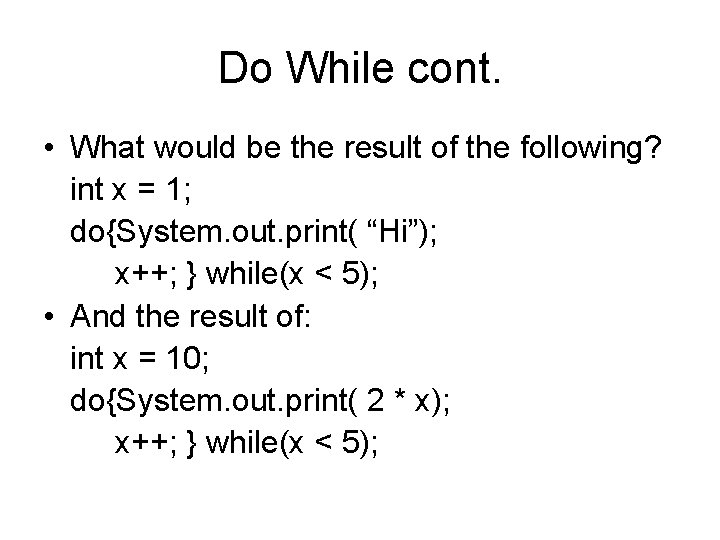
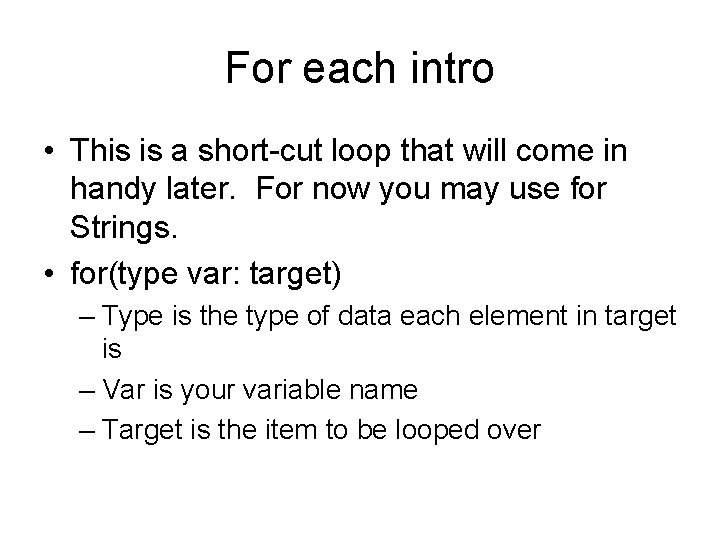
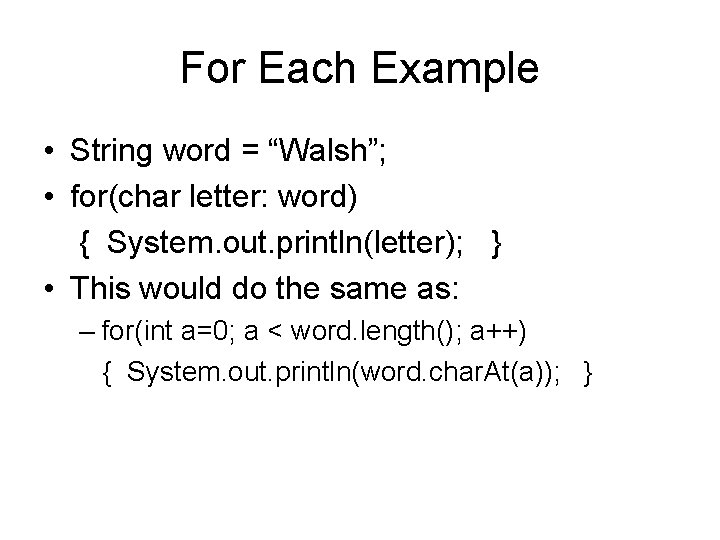
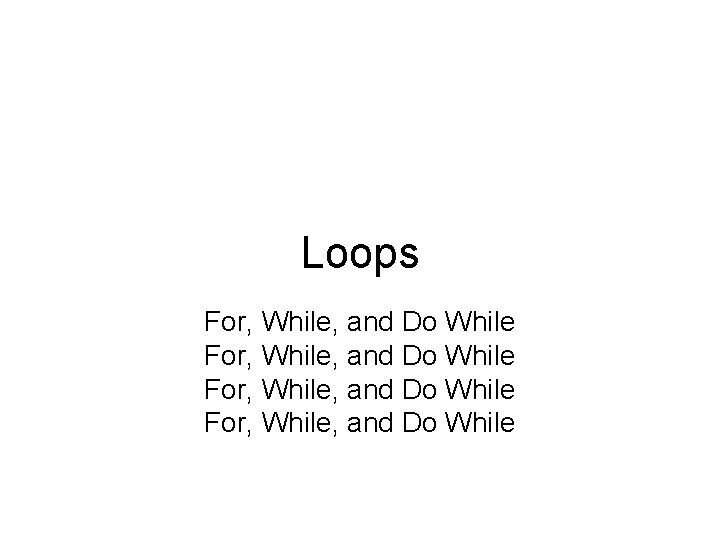
- Slides: 11
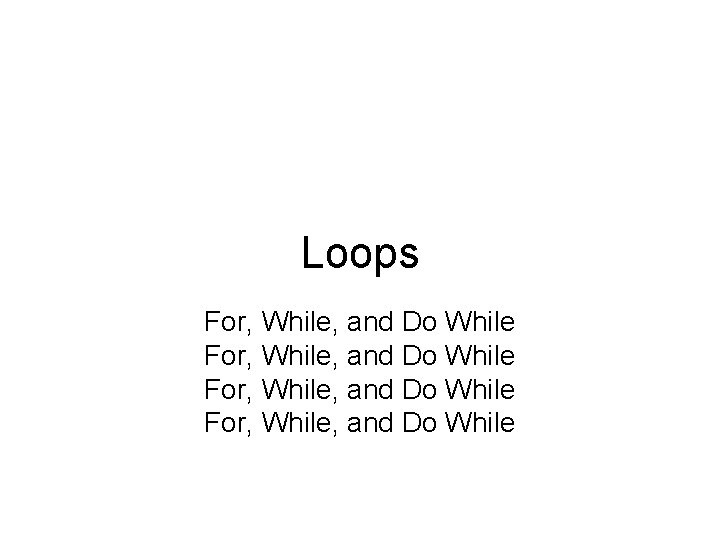
Loops For, While, and Do While
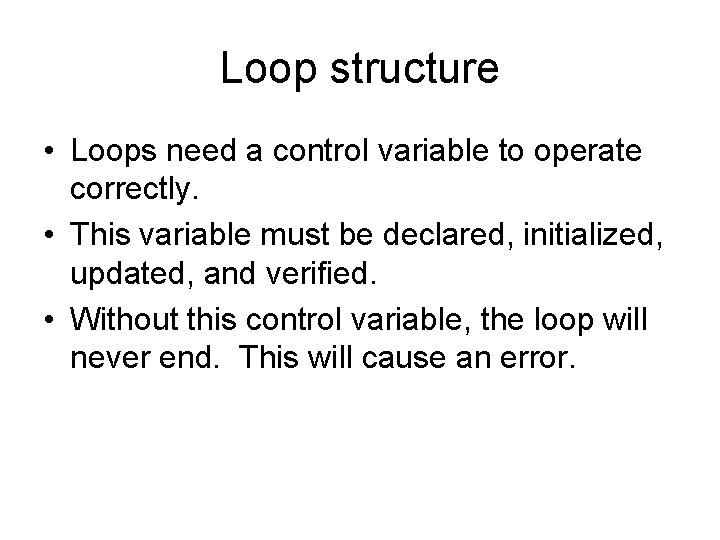
Loop structure • Loops need a control variable to operate correctly. • This variable must be declared, initialized, updated, and verified. • Without this control variable, the loop will never end. This will cause an error.
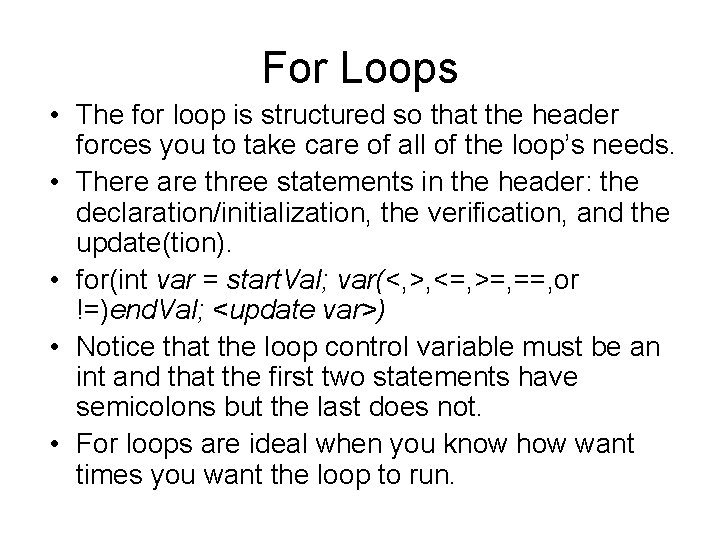
For Loops • The for loop is structured so that the header forces you to take care of all of the loop’s needs. • There are three statements in the header: the declaration/initialization, the verification, and the update(tion). • for(int var = start. Val; var(<, >, <=, >=, ==, or !=)end. Val; <update var>) • Notice that the loop control variable must be an int and that the first two statements have semicolons but the last does not. • For loops are ideal when you know how want times you want the loop to run.
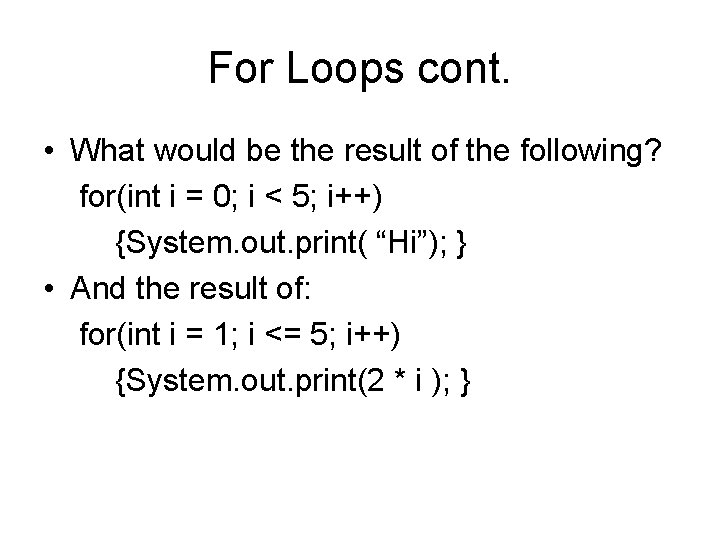
For Loops cont. • What would be the result of the following? for(int i = 0; i < 5; i++) {System. out. print( “Hi”); } • And the result of: for(int i = 1; i <= 5; i++) {System. out. print(2 * i ); }
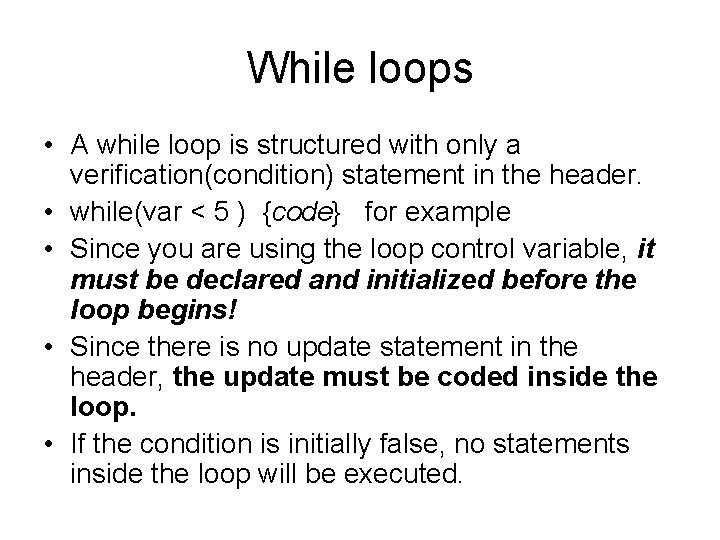
While loops • A while loop is structured with only a verification(condition) statement in the header. • while(var < 5 ) {code} for example • Since you are using the loop control variable, it must be declared and initialized before the loop begins! • Since there is no update statement in the header, the update must be coded inside the loop. • If the condition is initially false, no statements inside the loop will be executed.
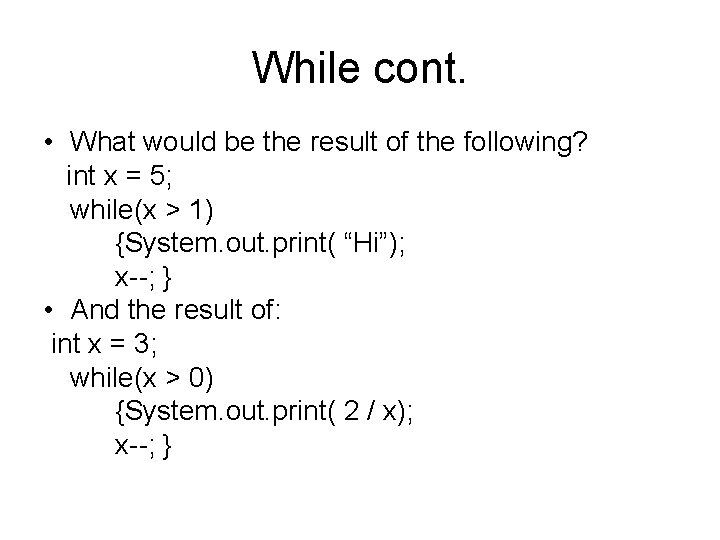
While cont. • What would be the result of the following? int x = 5; while(x > 1) {System. out. print( “Hi”); x--; } • And the result of: int x = 3; while(x > 0) {System. out. print( 2 / x); x--; }
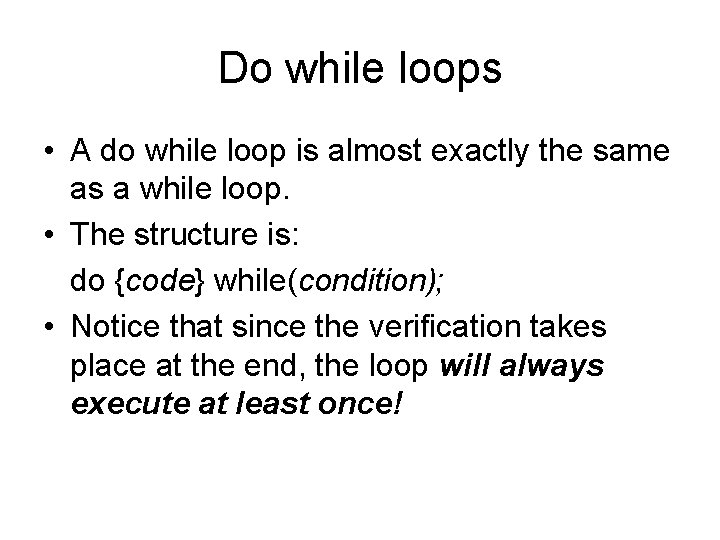
Do while loops • A do while loop is almost exactly the same as a while loop. • The structure is: do {code} while(condition); • Notice that since the verification takes place at the end, the loop will always execute at least once!
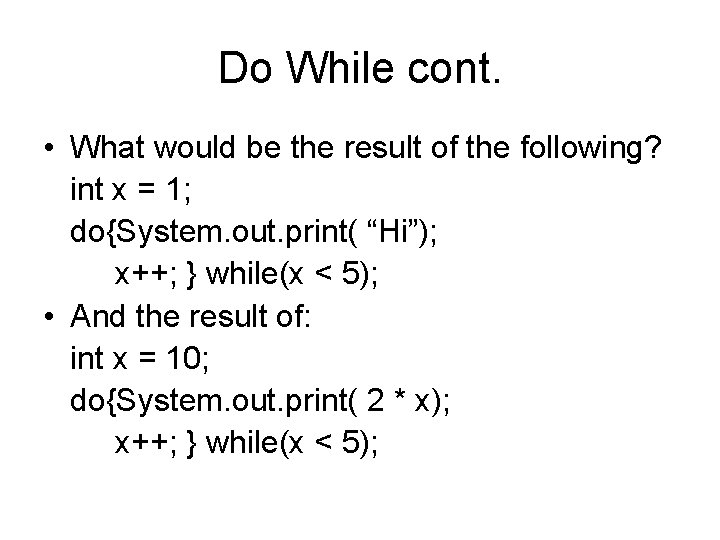
Do While cont. • What would be the result of the following? int x = 1; do{System. out. print( “Hi”); x++; } while(x < 5); • And the result of: int x = 10; do{System. out. print( 2 * x); x++; } while(x < 5);
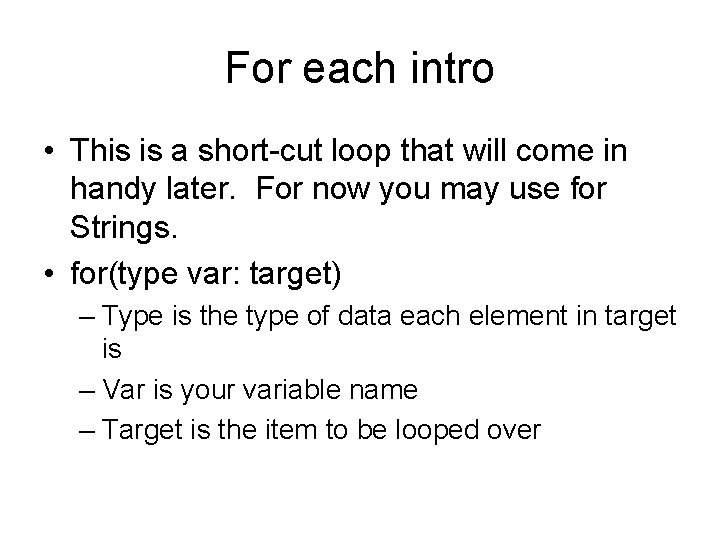
For each intro • This is a short-cut loop that will come in handy later. For now you may use for Strings. • for(type var: target) – Type is the type of data each element in target is – Var is your variable name – Target is the item to be looped over
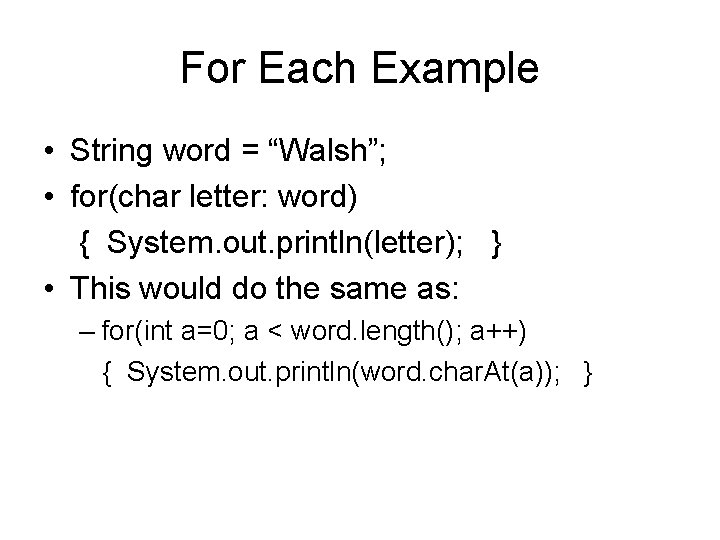
For Each Example • String word = “Walsh”; • for(char letter: word) { System. out. println(letter); } • This would do the same as: – for(int a=0; a < word. length(); a++) { System. out. println(word. char. At(a)); }
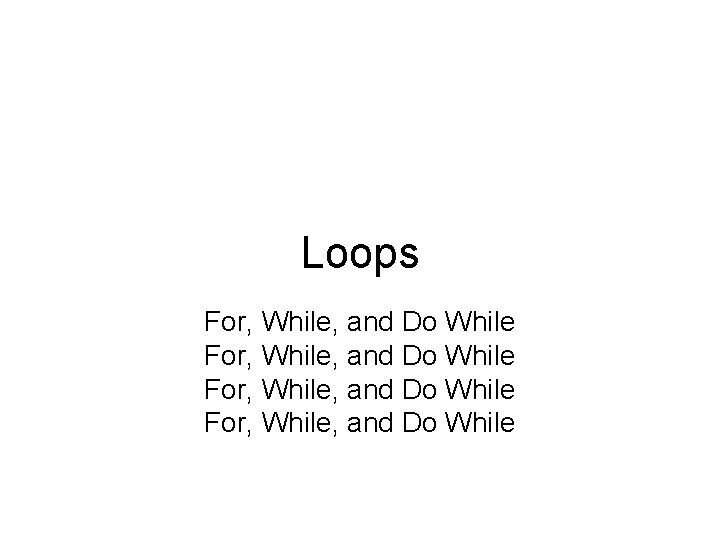
Loops For, While, and Do While
Perbedaan for while do while
While loops and if-else structures
Matlab while loop example
For break matlab
Control structure
Kompor lpg termasuk open loop atau close loop
Fifth gear loop the loop
Open loop vs closed loop in cars
Manakah yang lebih baik open loop atau close loop system
Accidental fingerprint
Multi loop pid controller regolatore pid multi loop
What are control structures in c++