LOOPING while for I 0 I 5 I
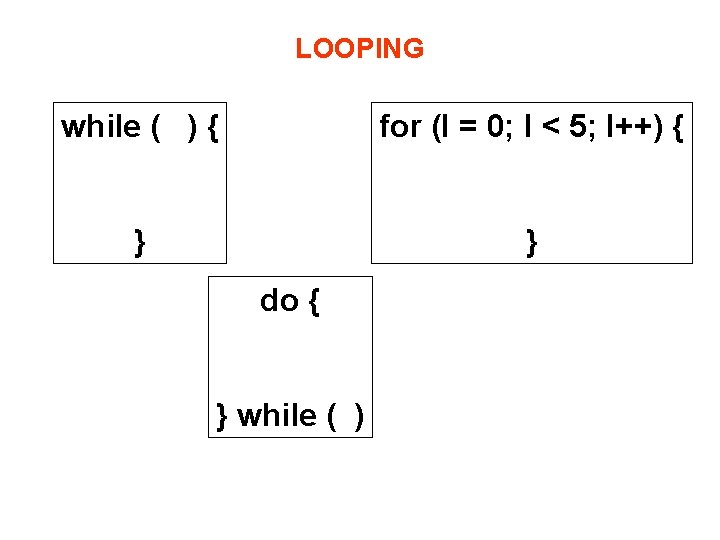
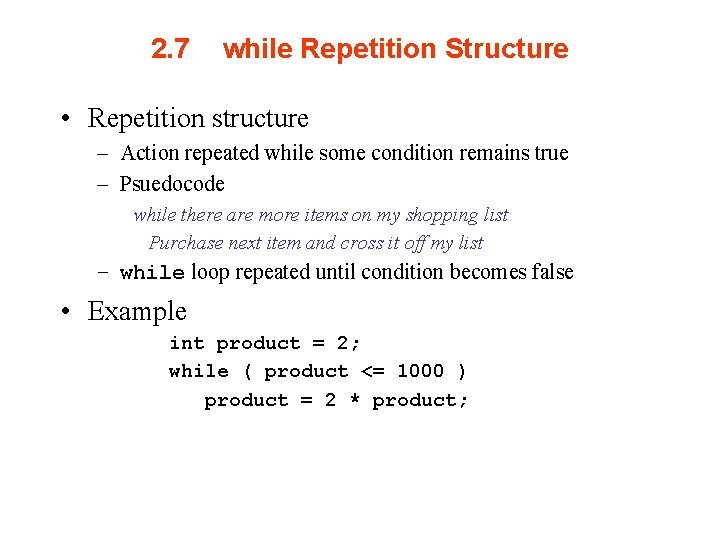
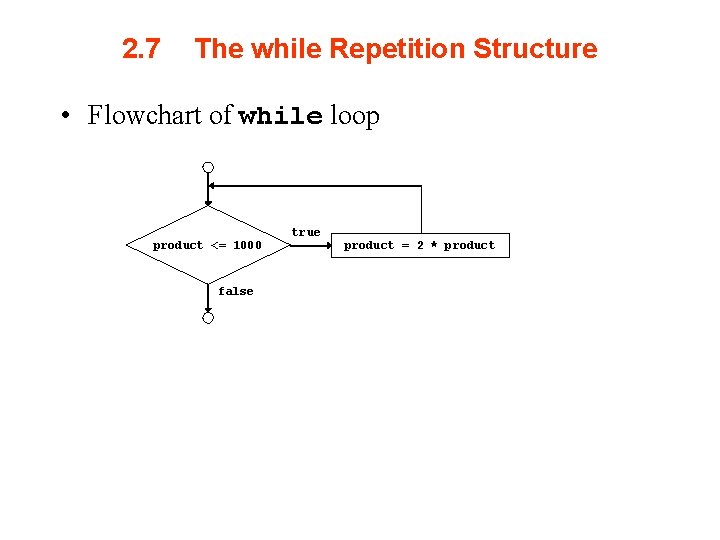
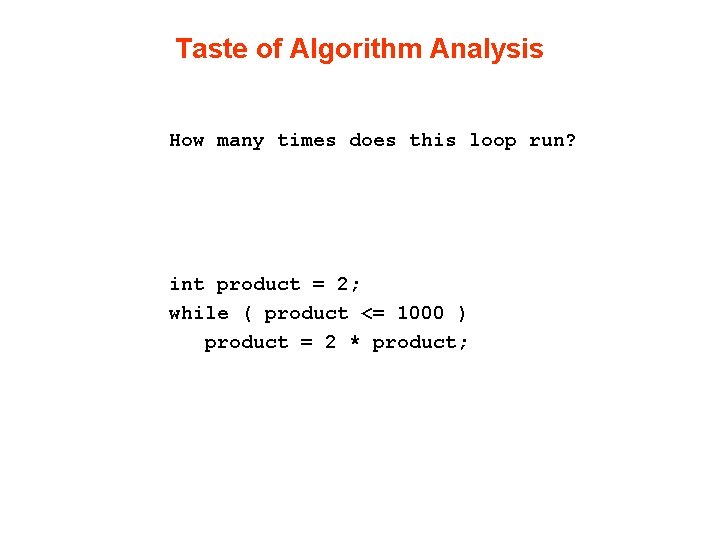
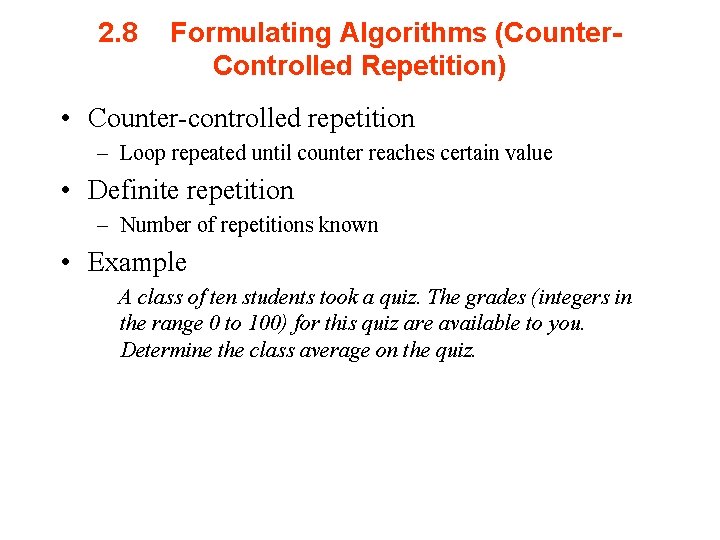
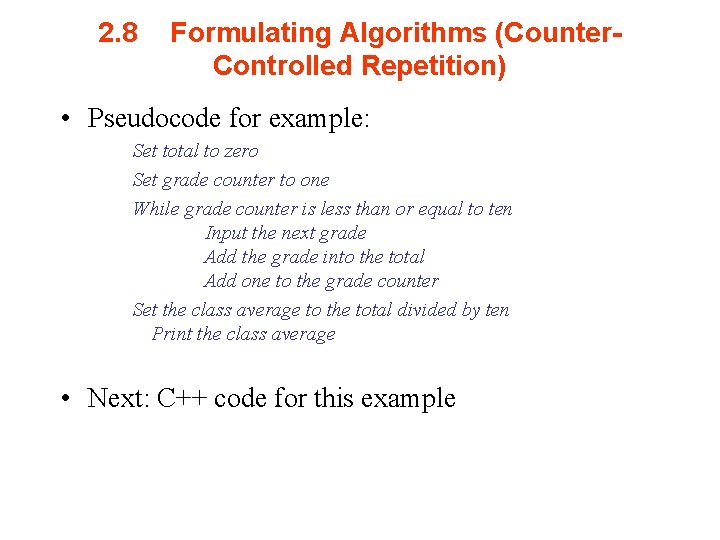
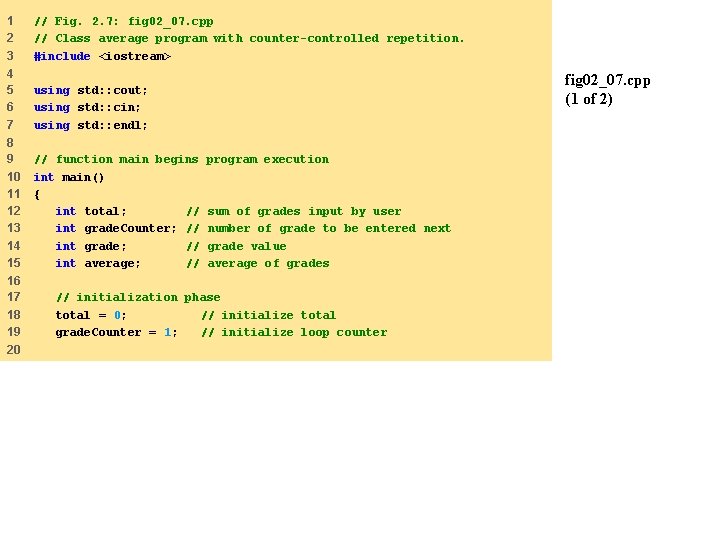
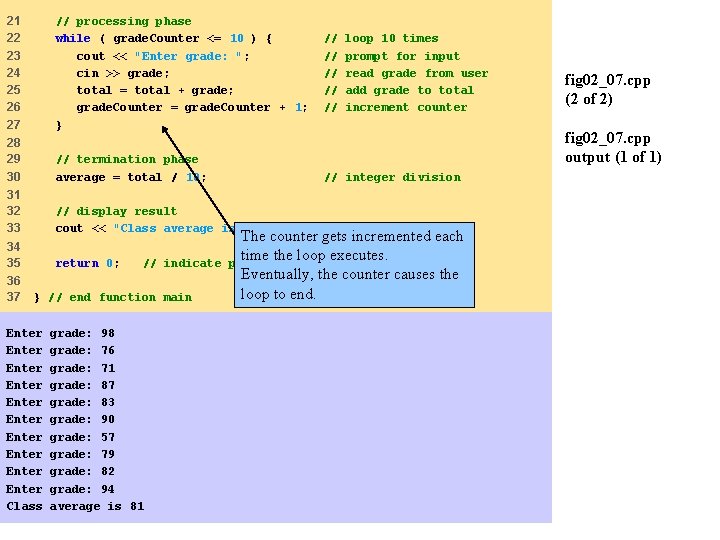
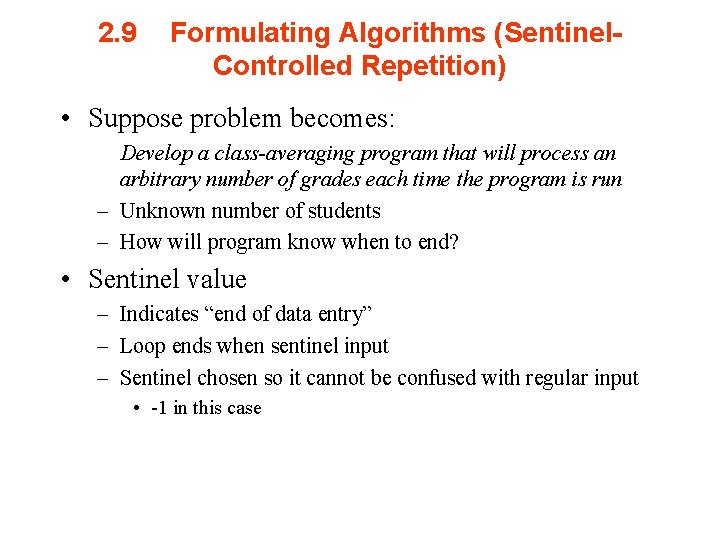
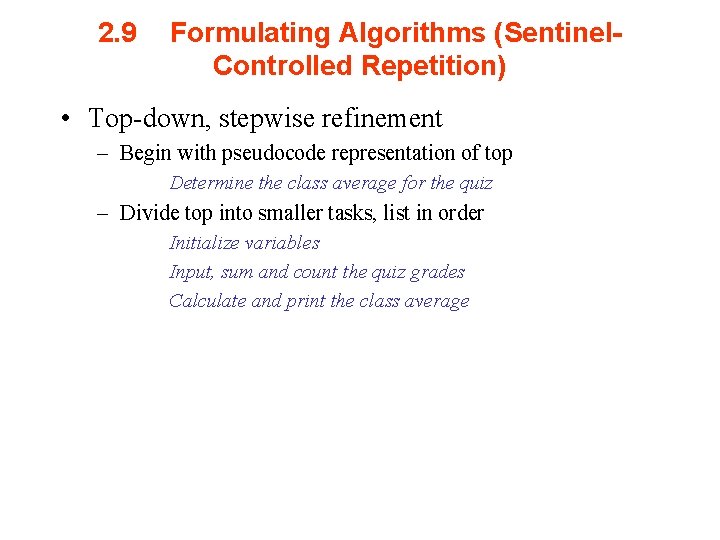
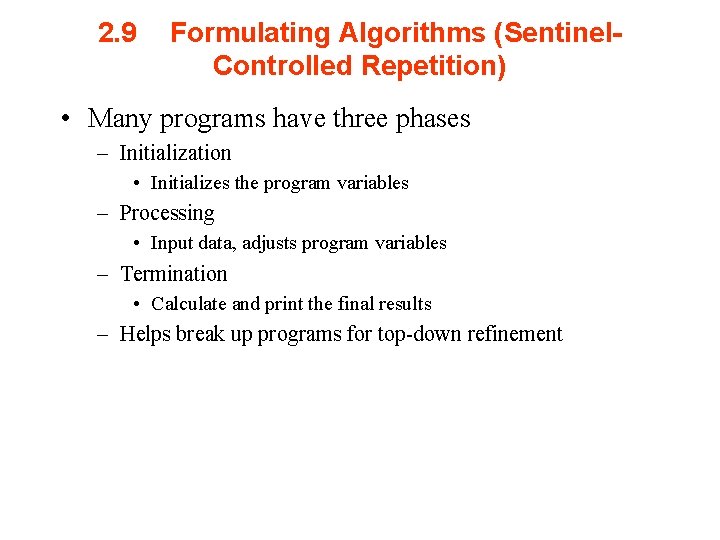
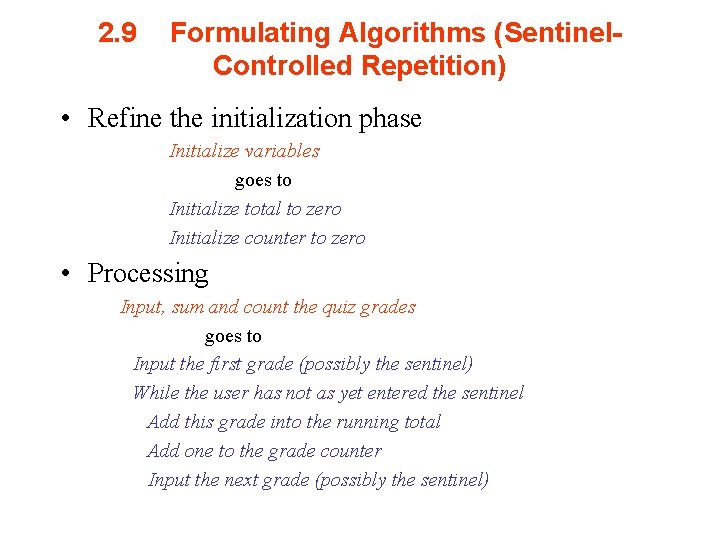
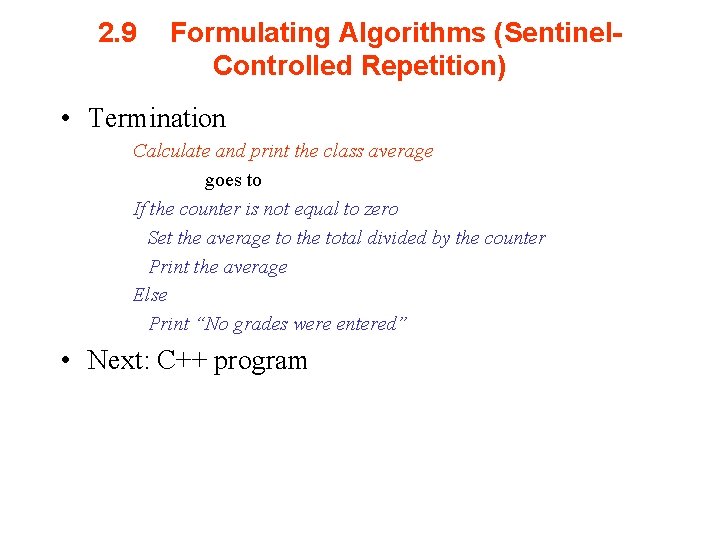
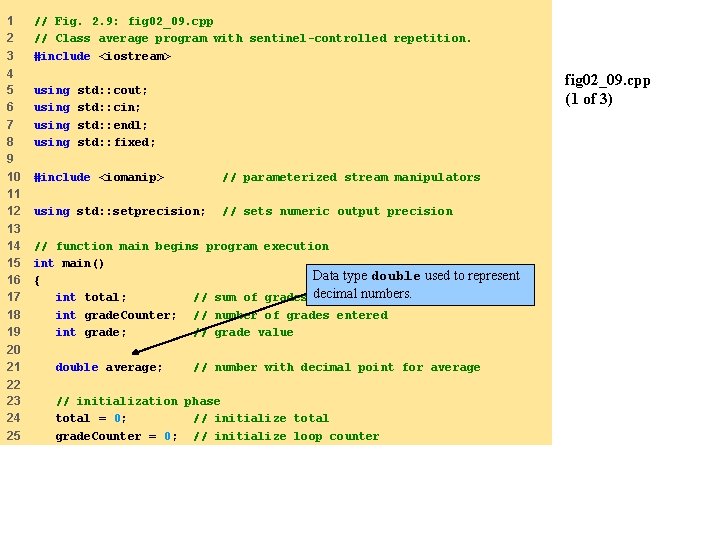
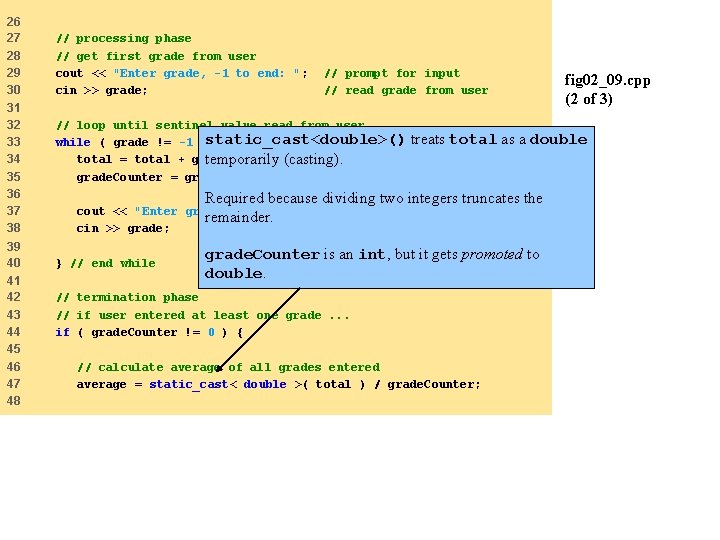
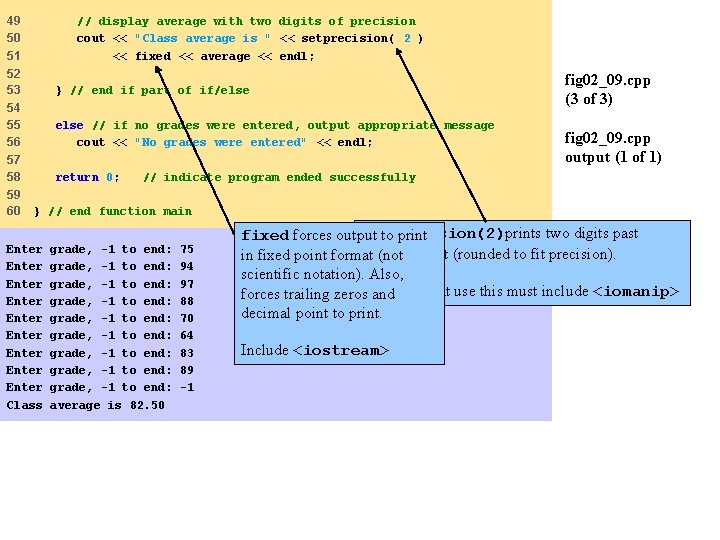
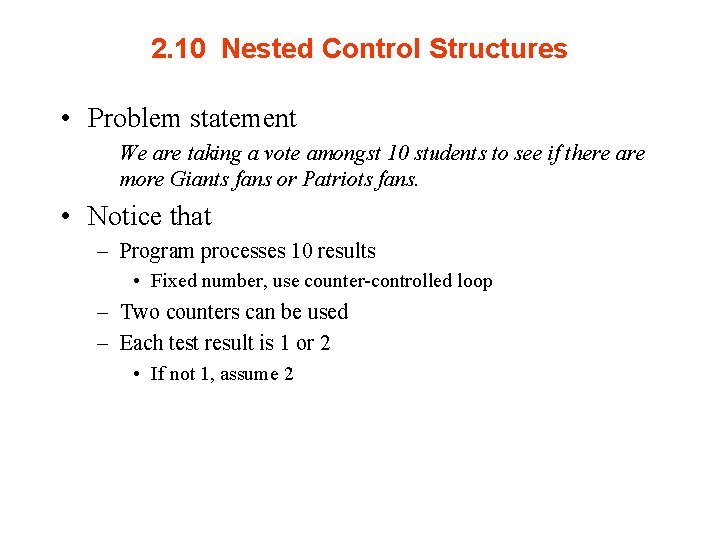
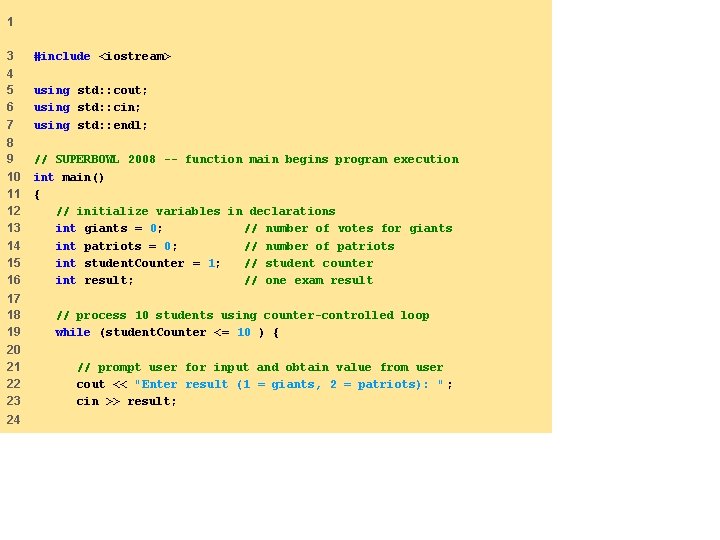
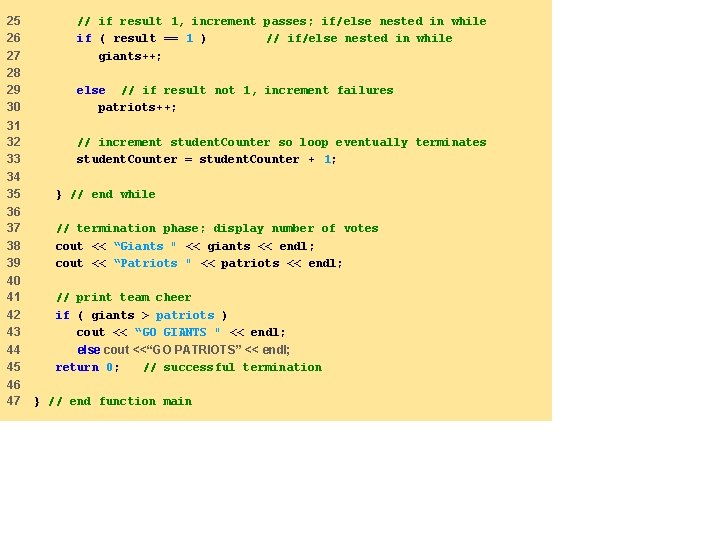
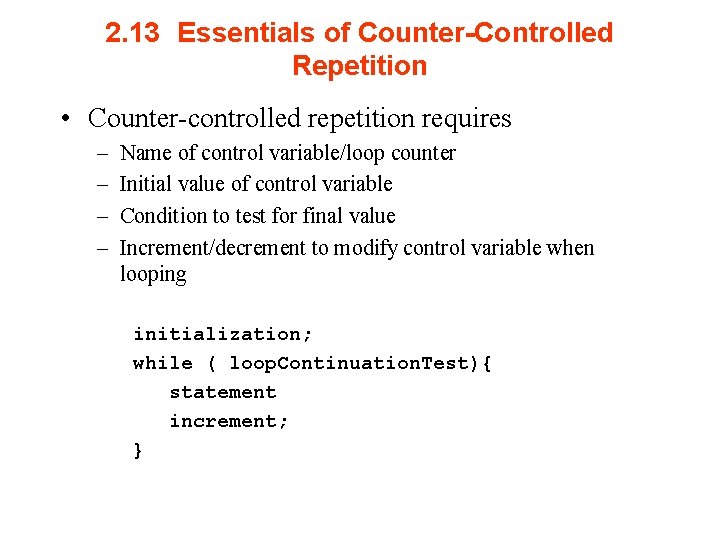
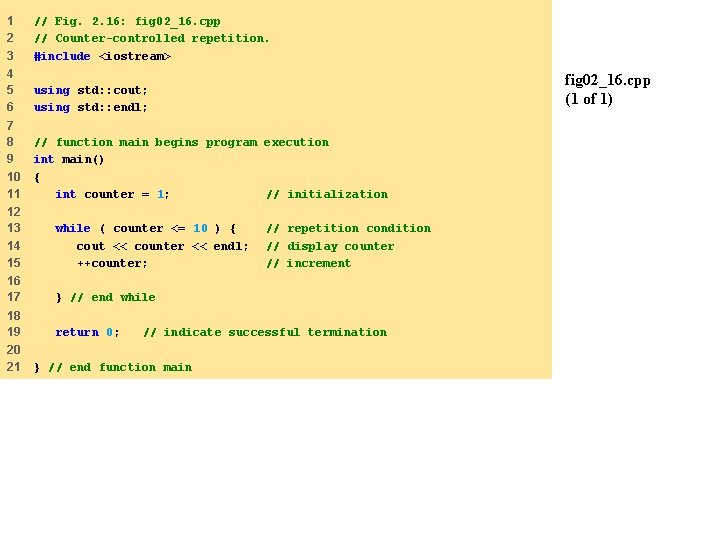
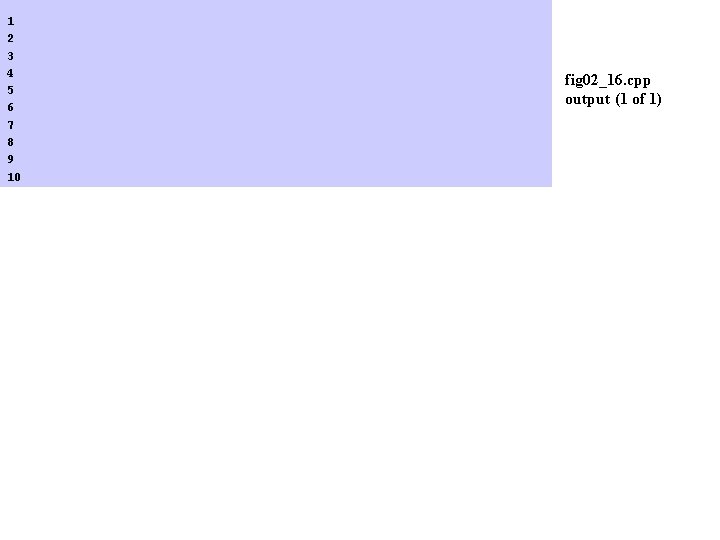
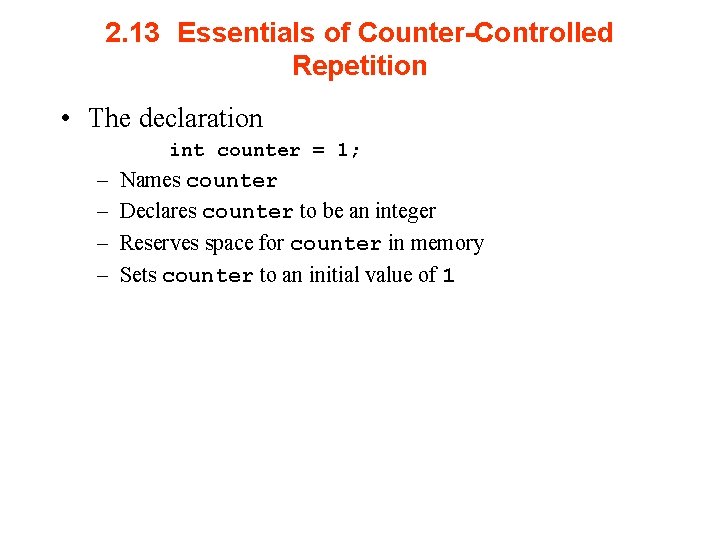
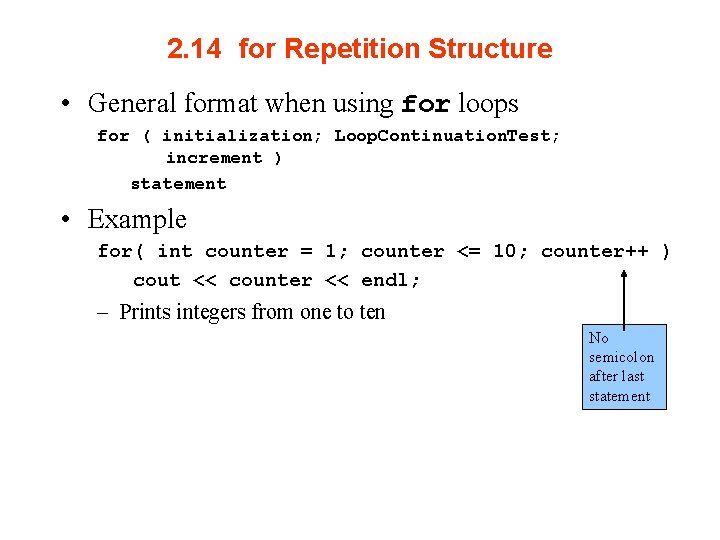
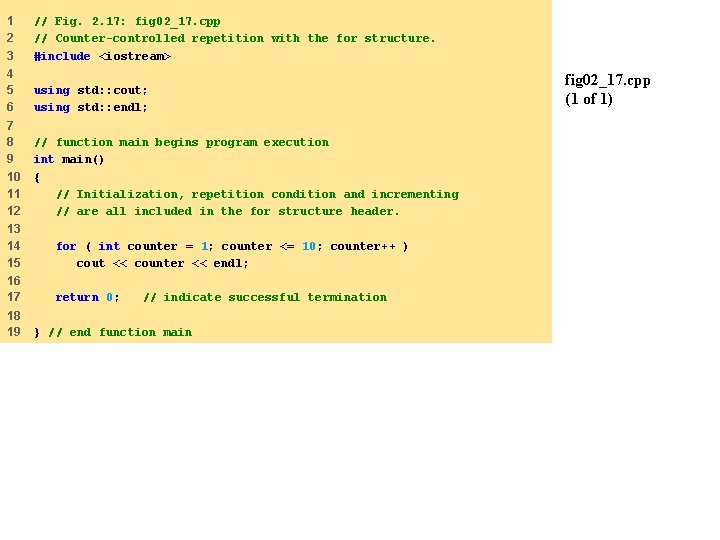
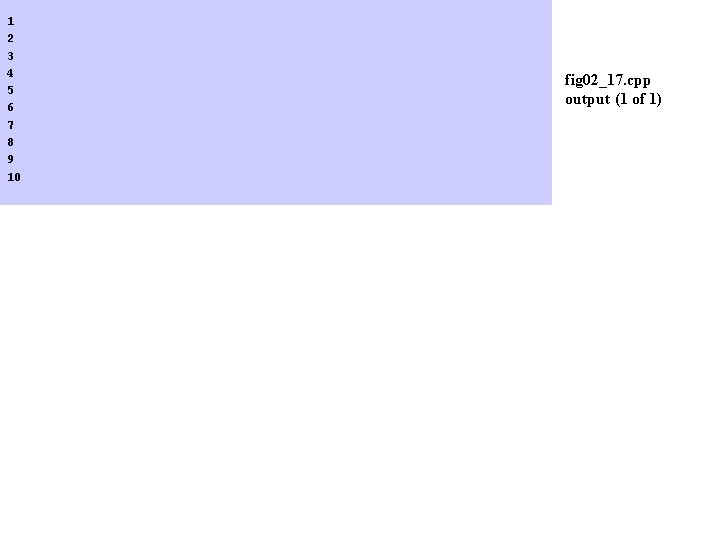
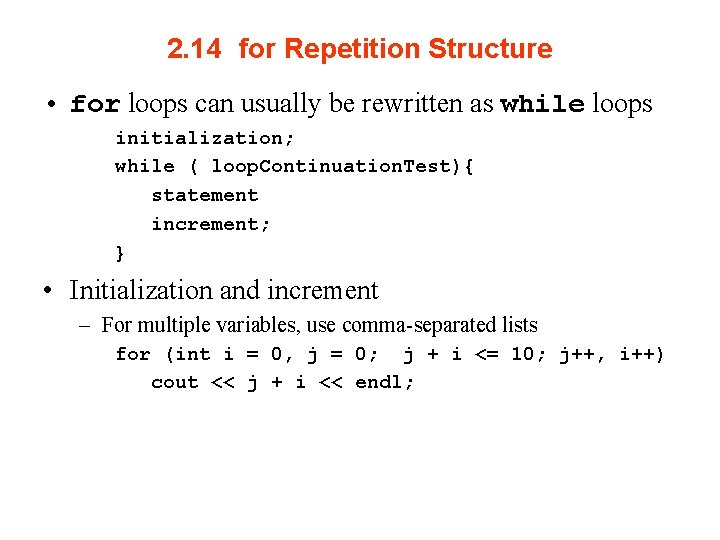
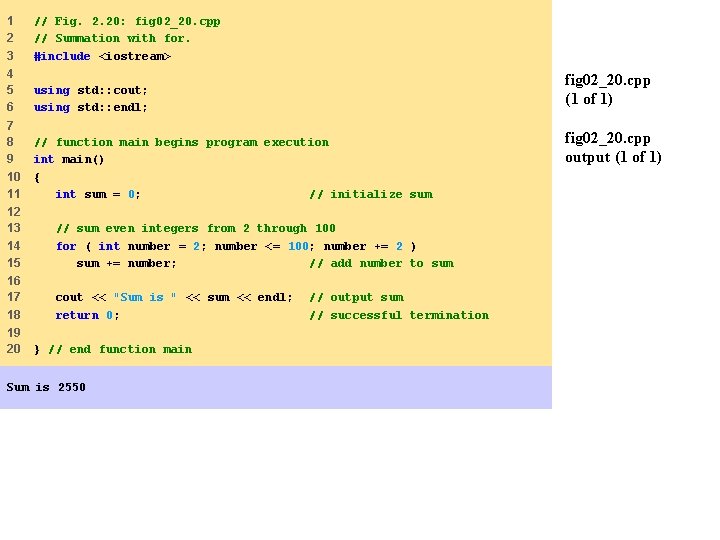
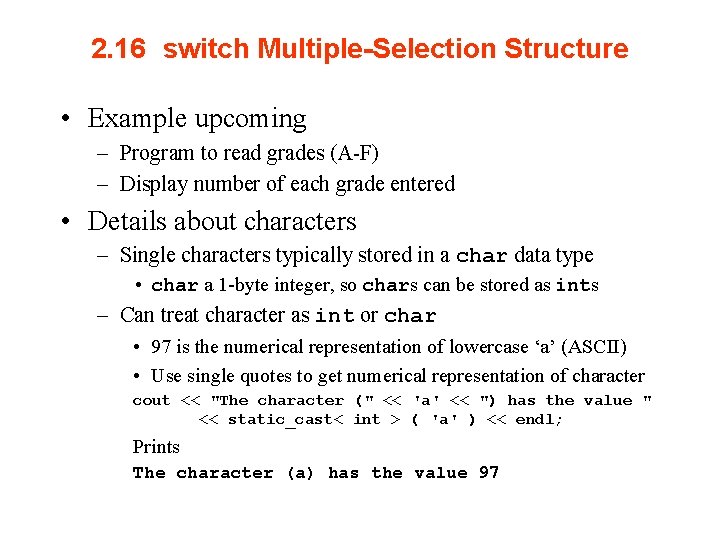
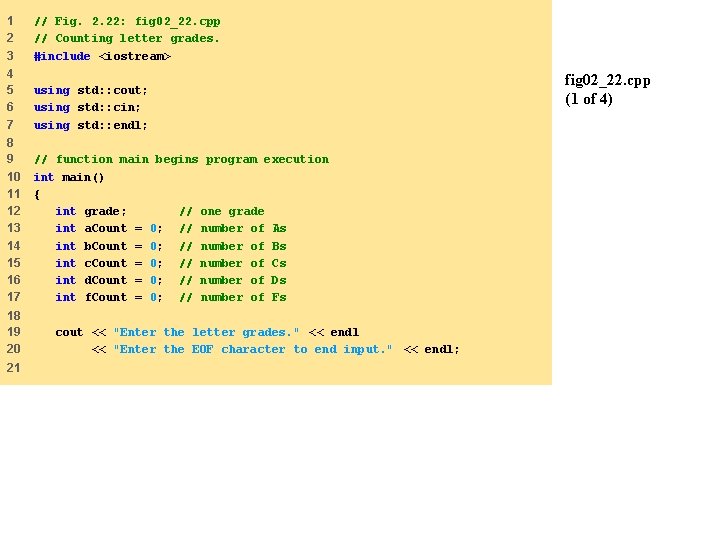
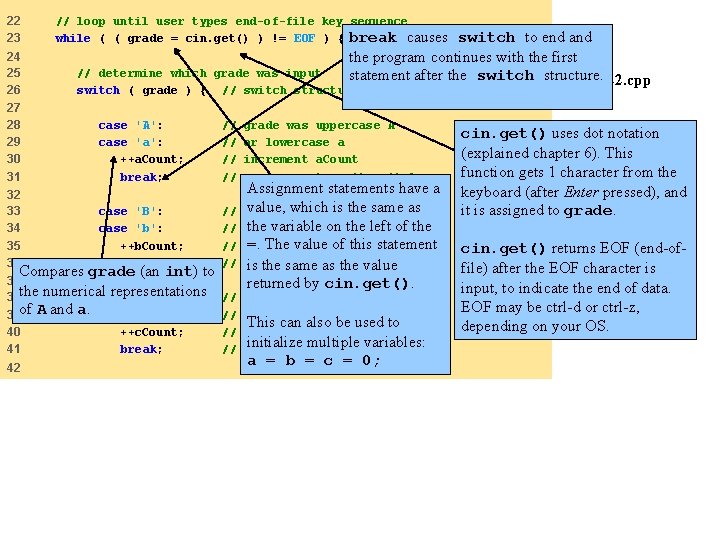
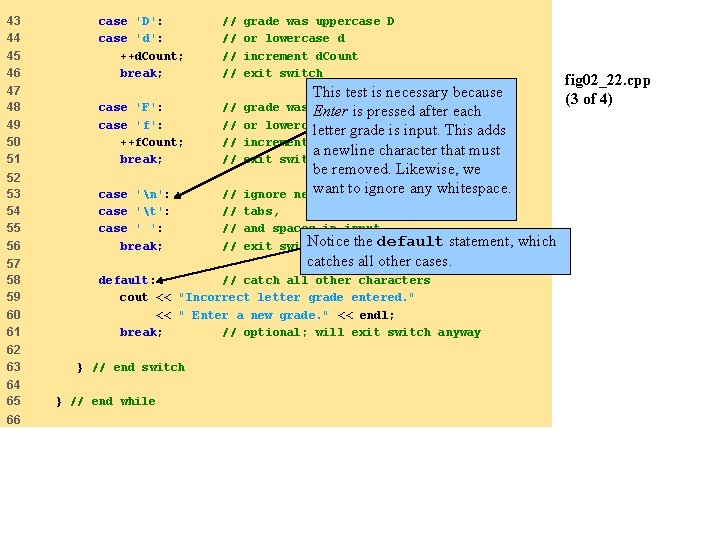
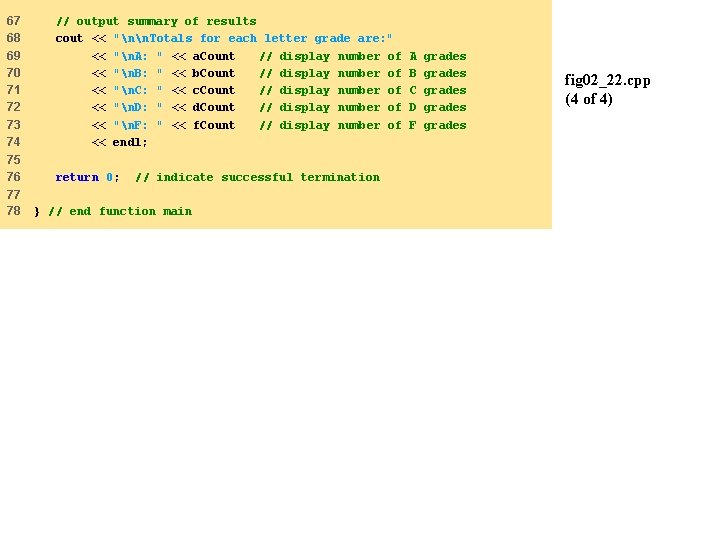
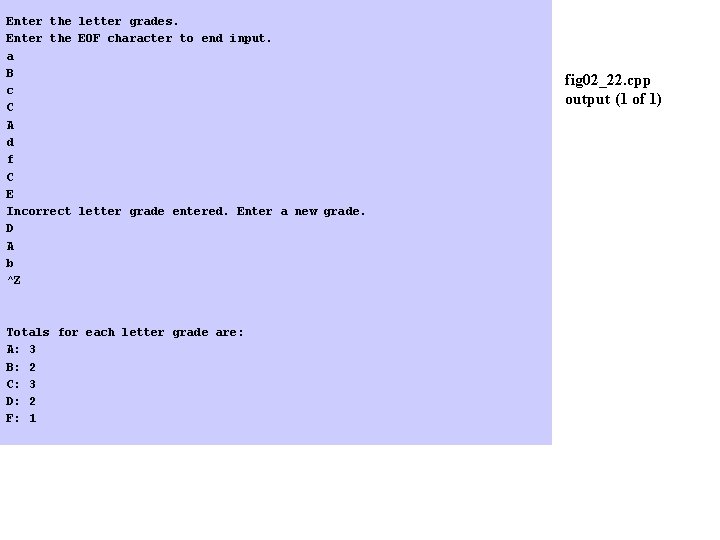
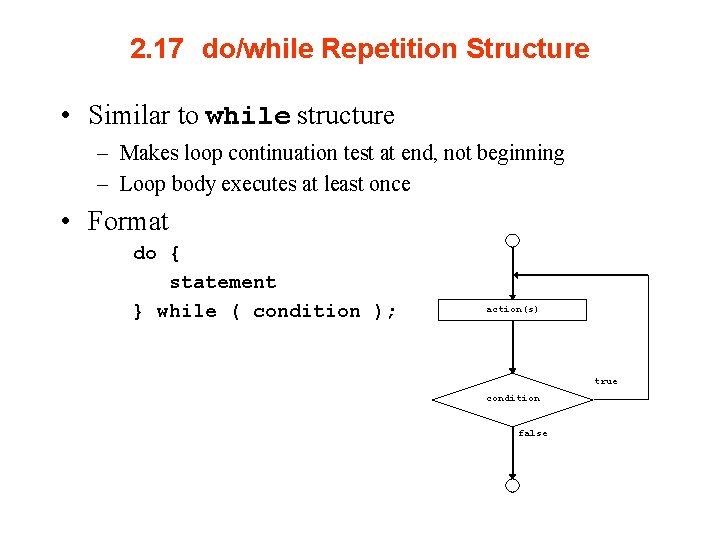
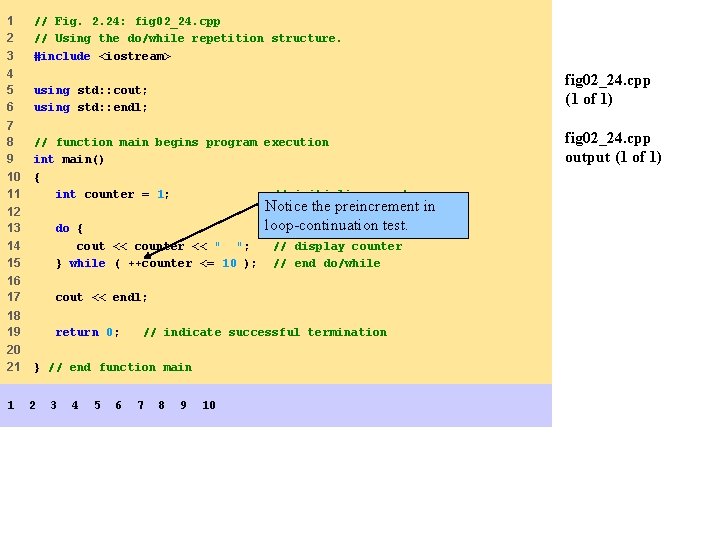
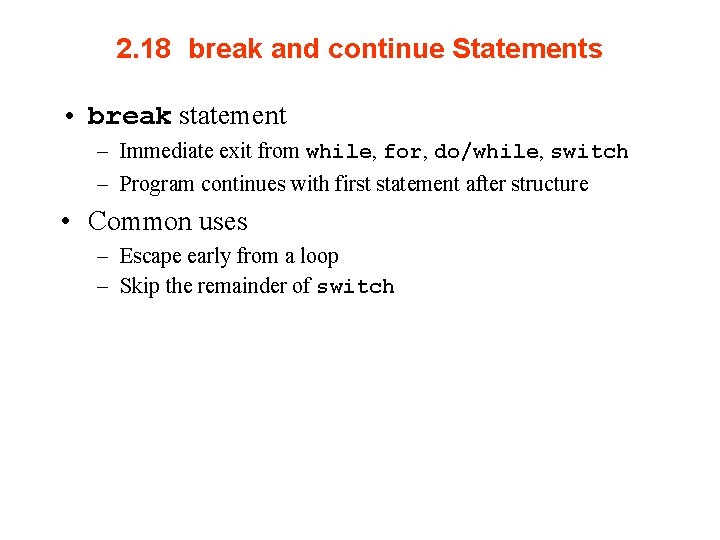
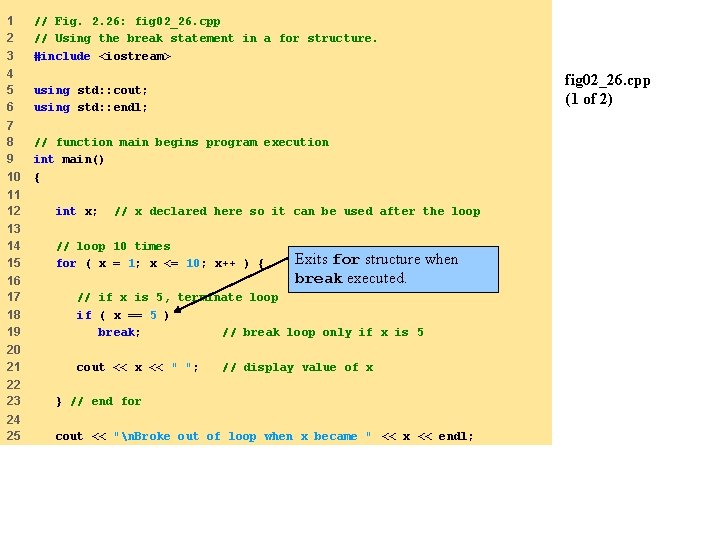
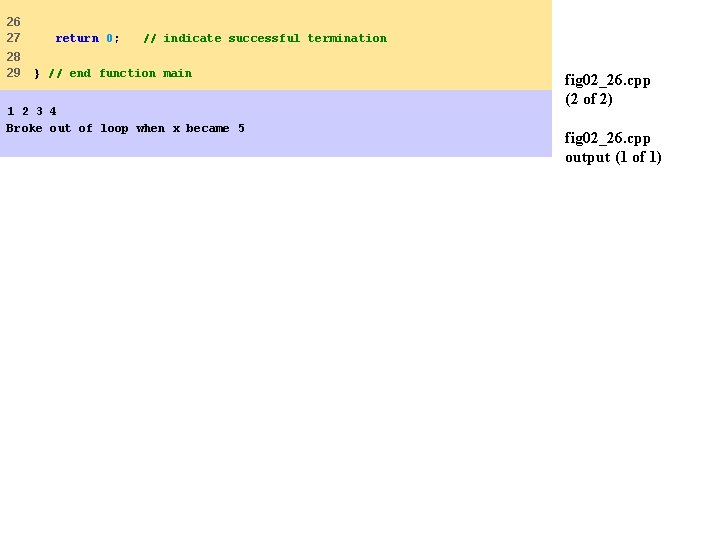
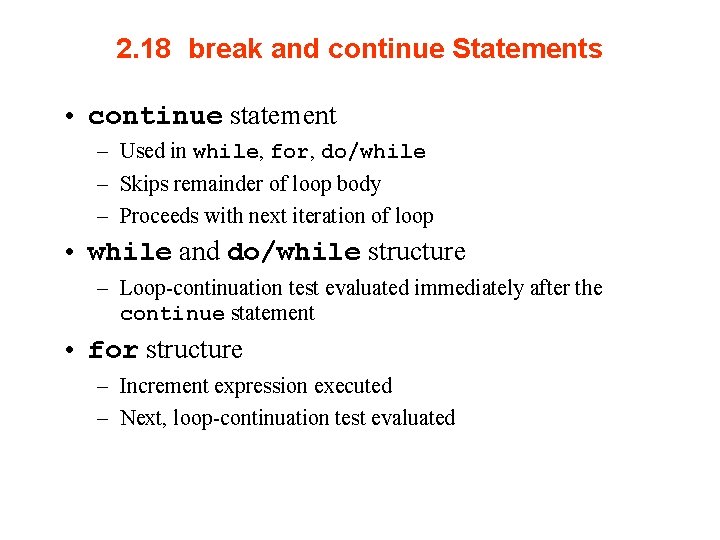
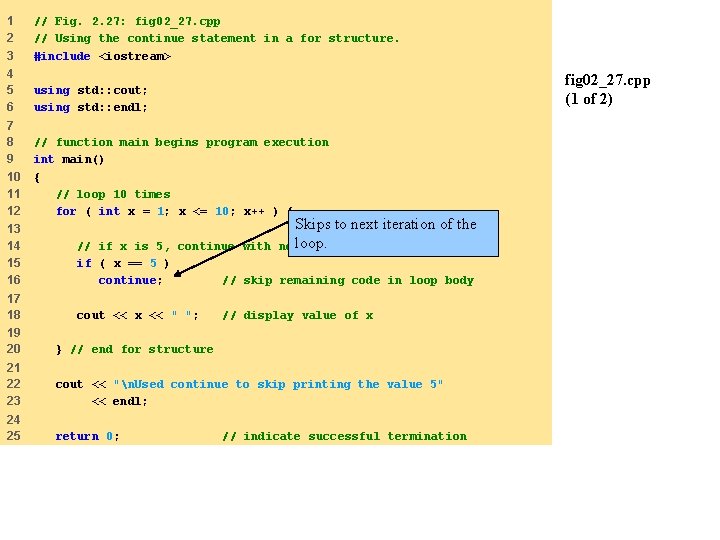
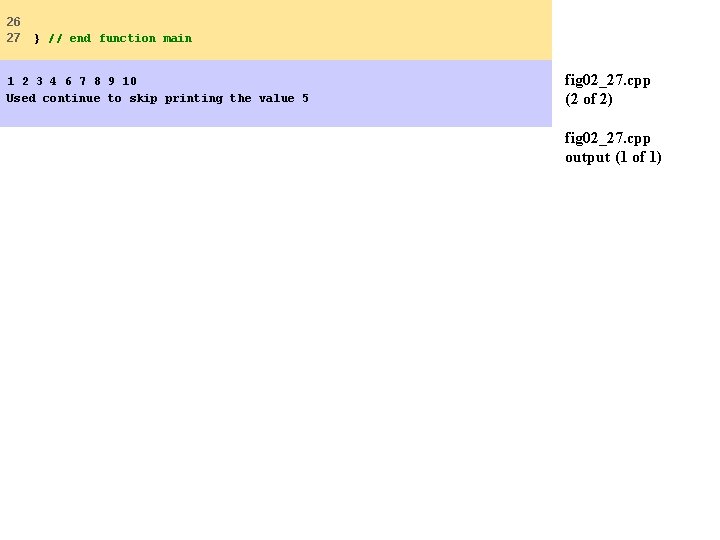
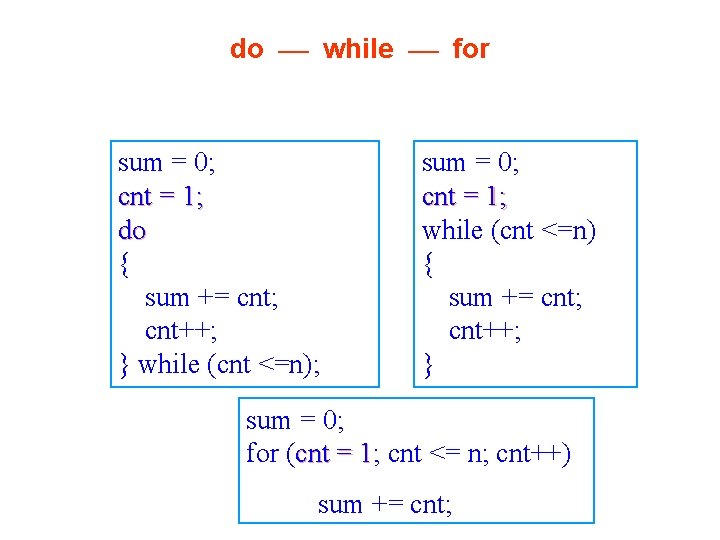
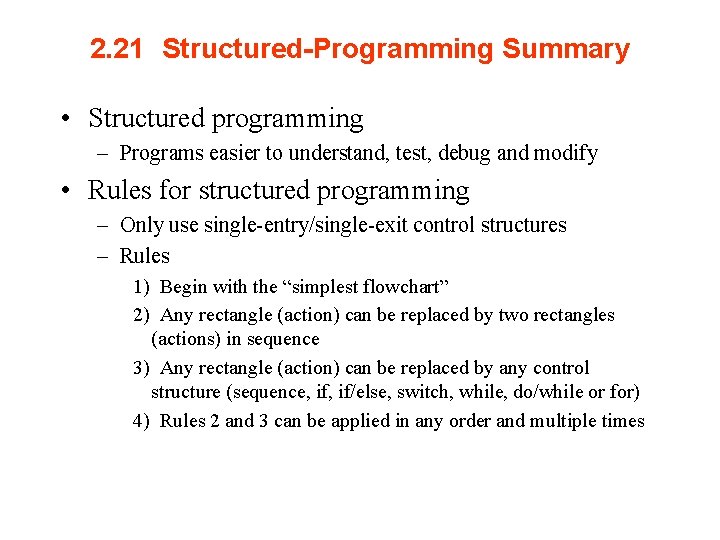
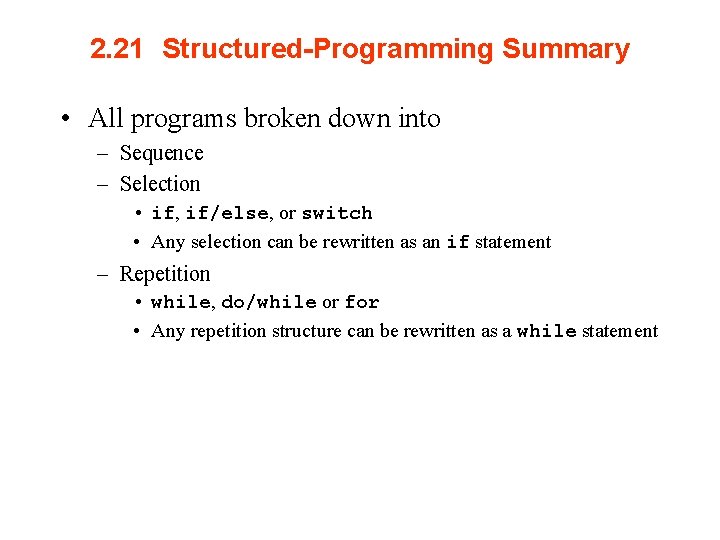
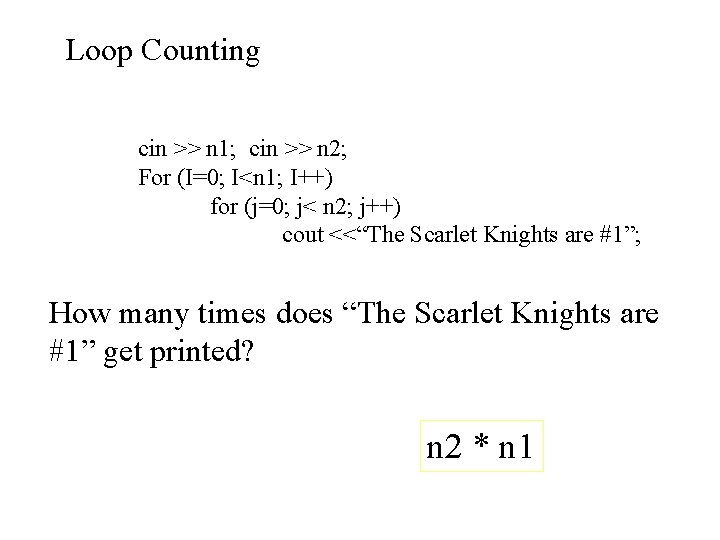
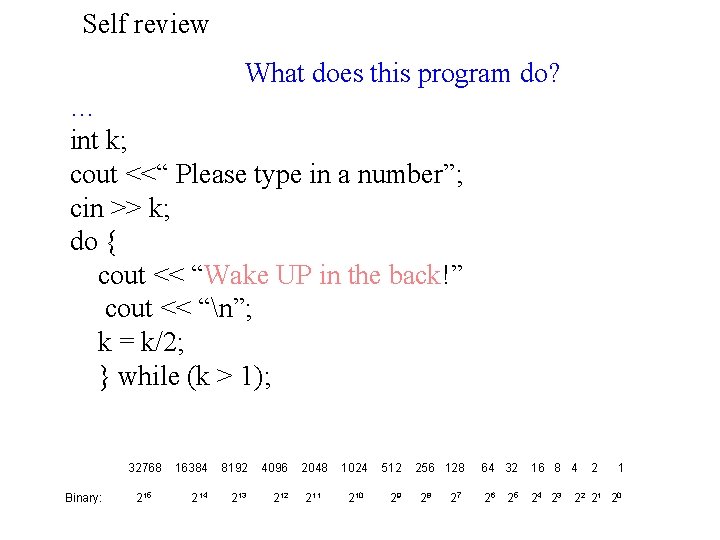
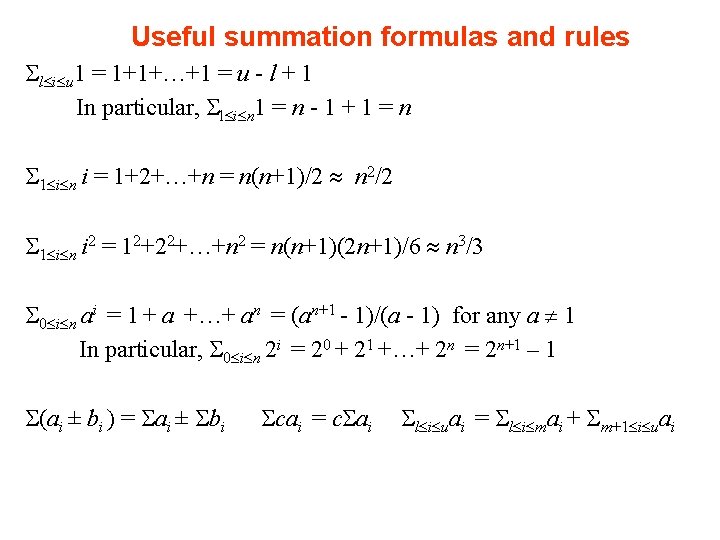
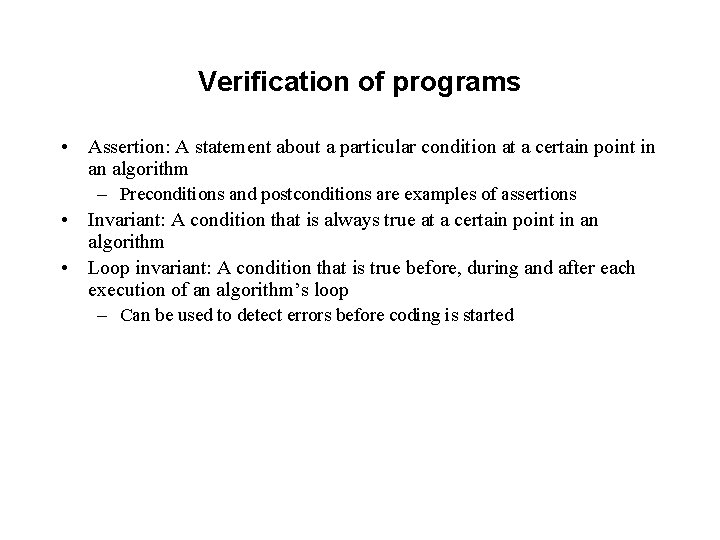
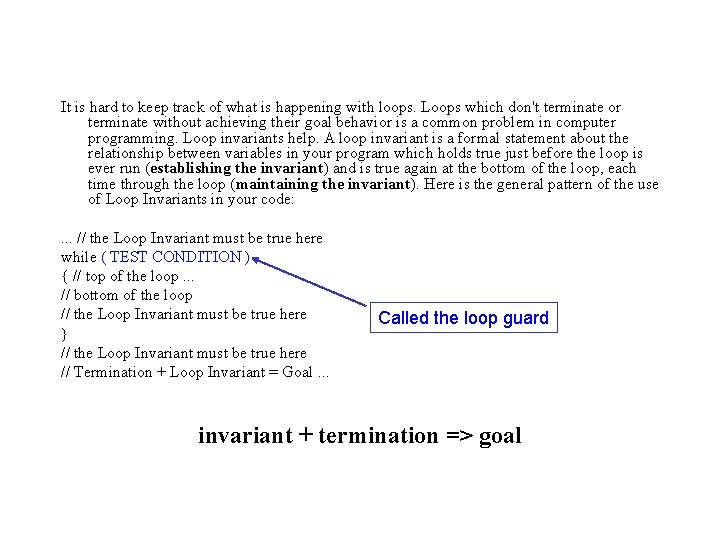
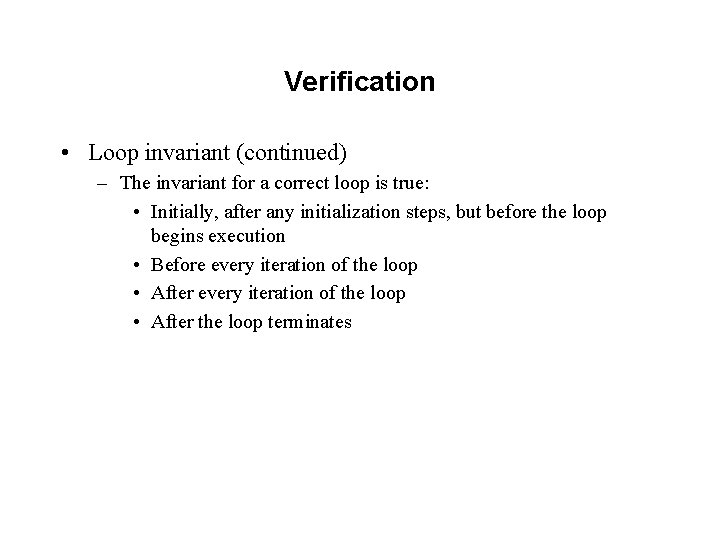
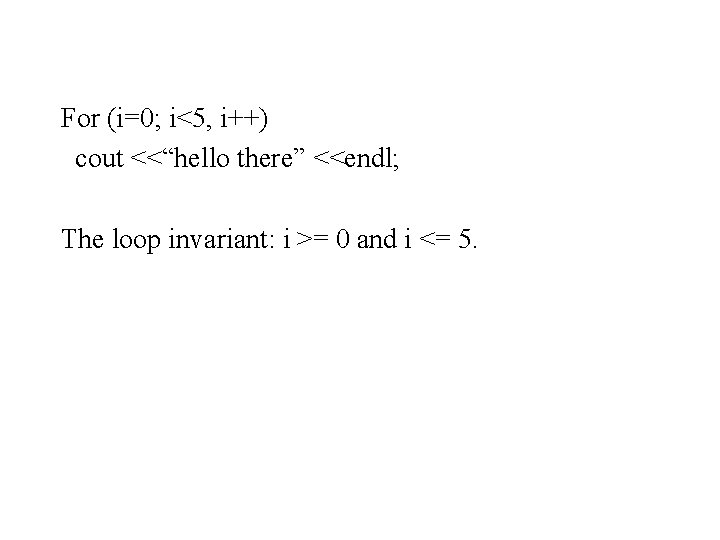
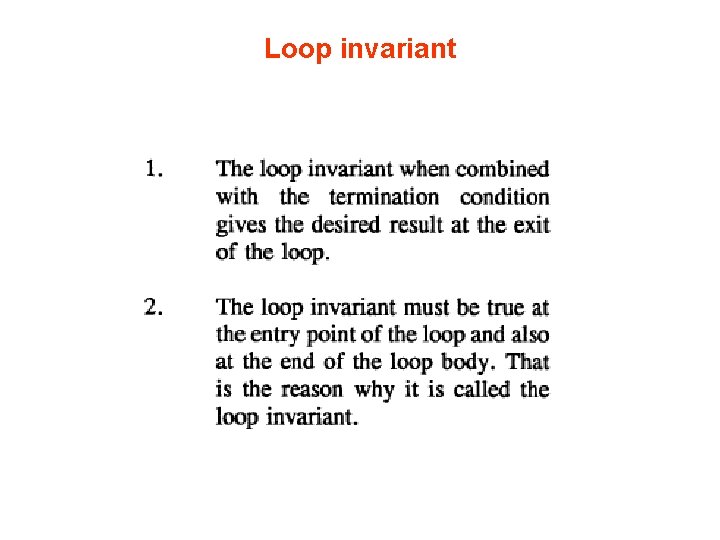
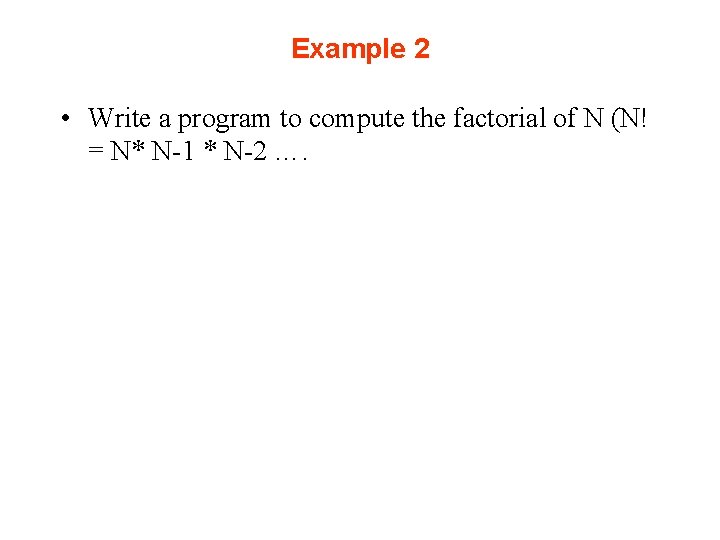
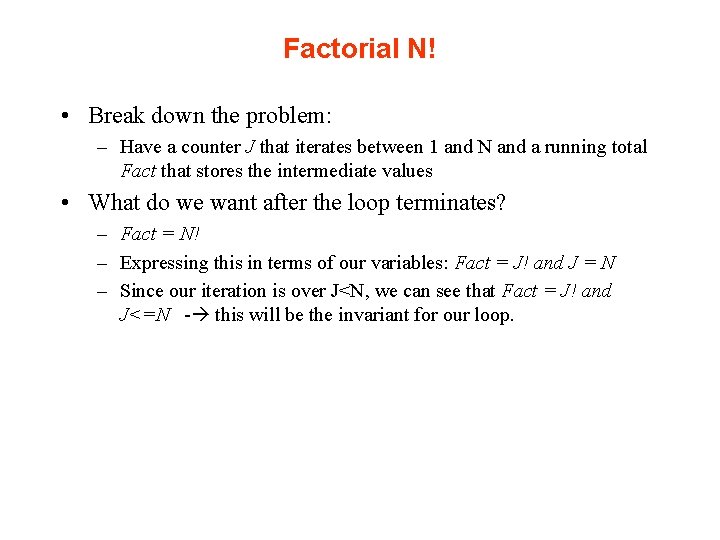
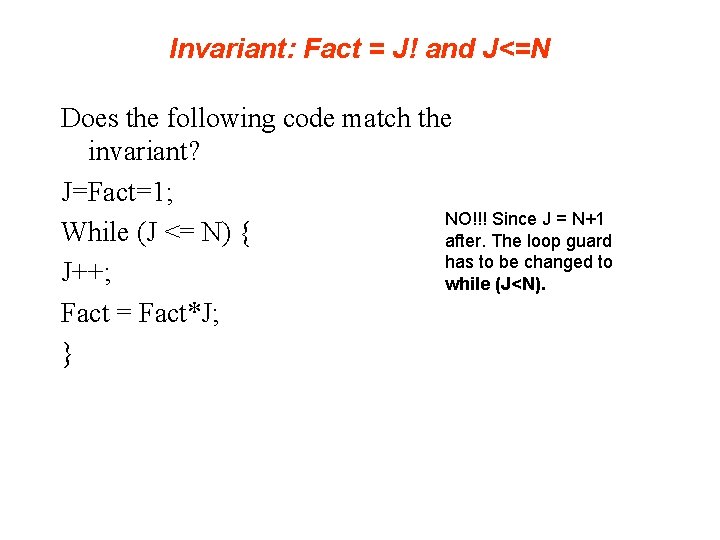
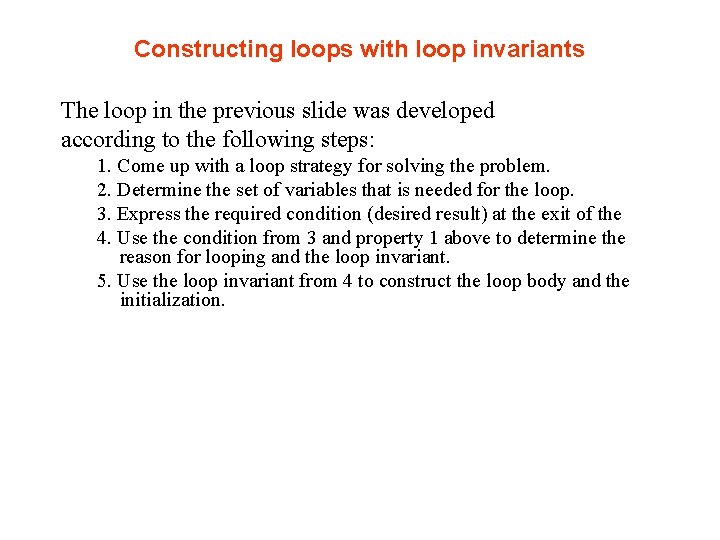
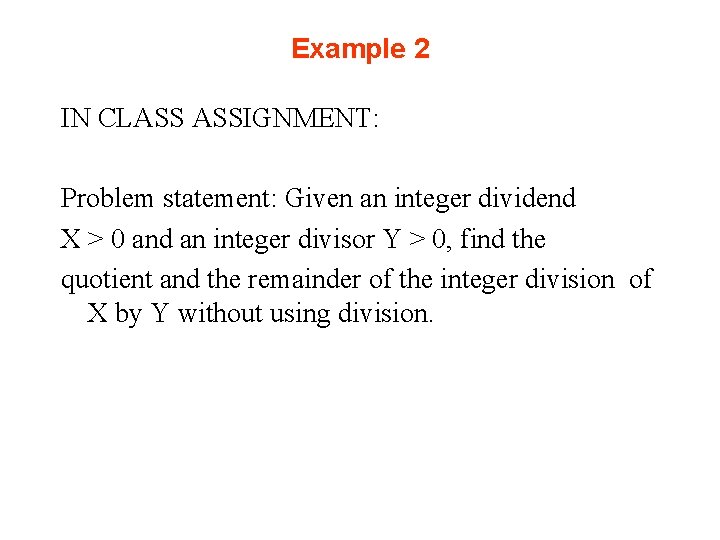
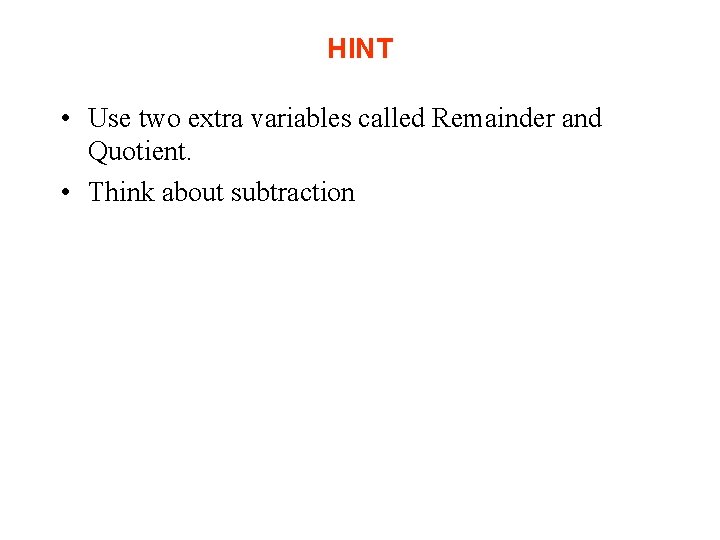
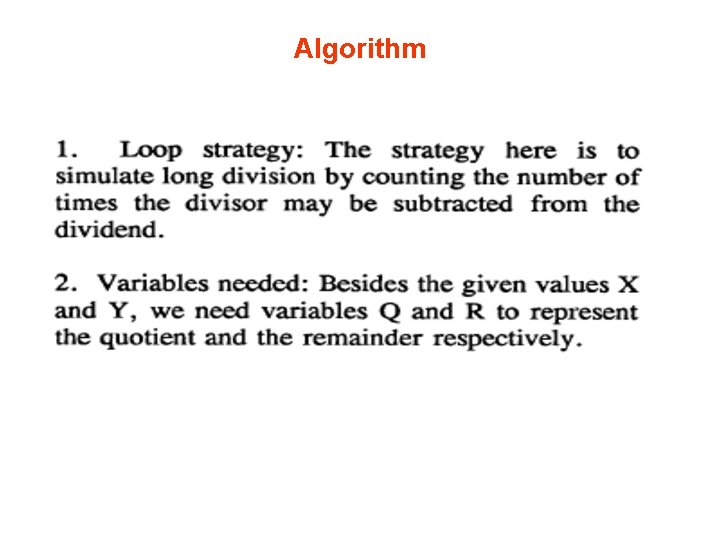
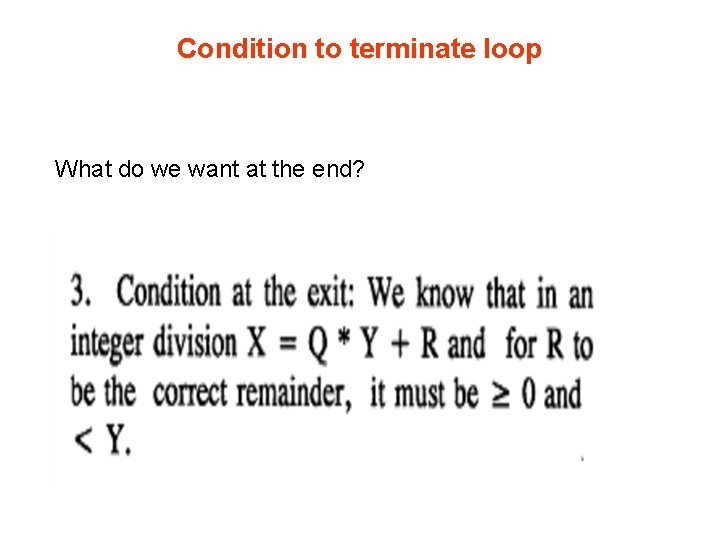
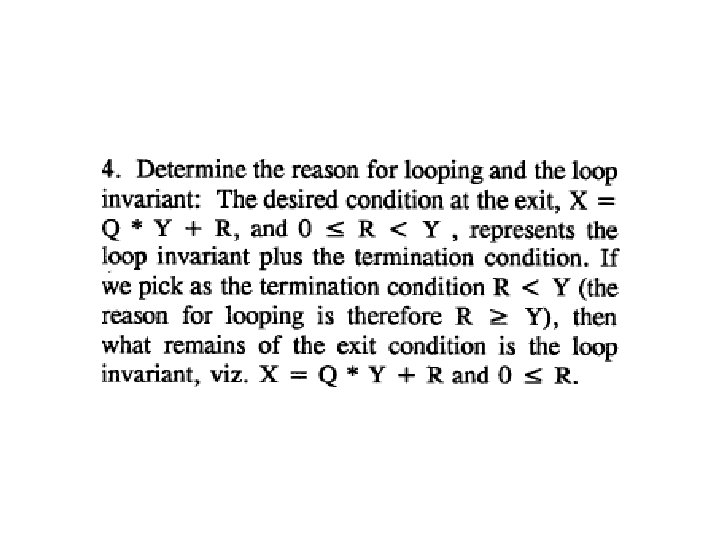
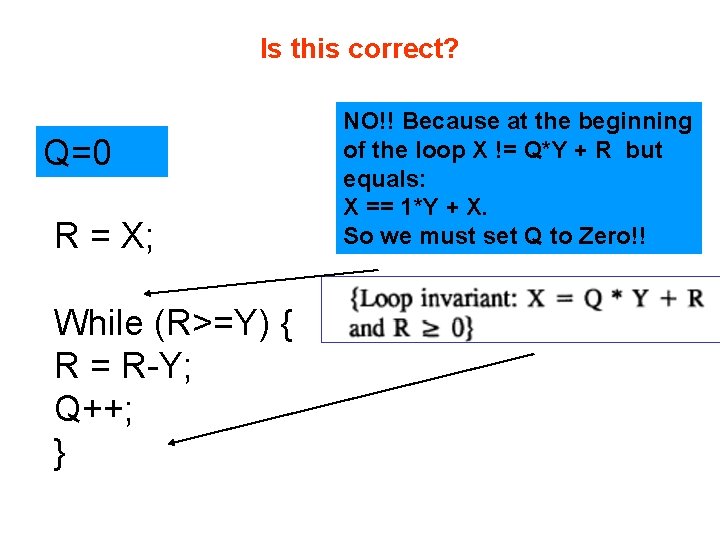
- Slides: 63
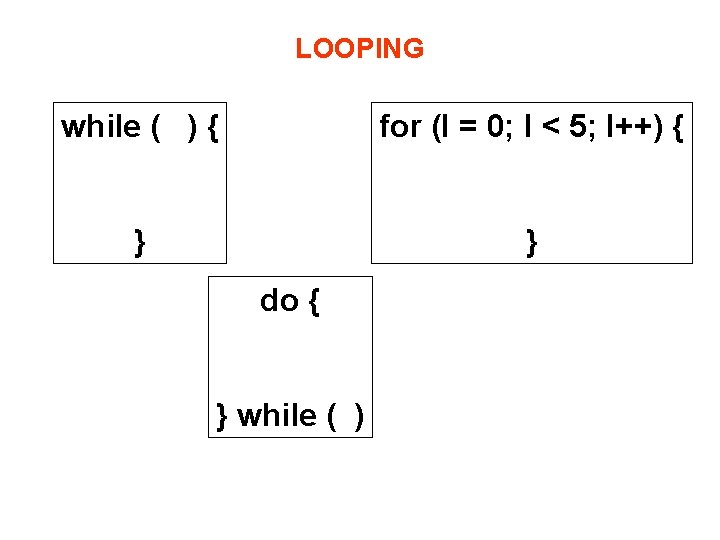
LOOPING while ( ) { for (I = 0; I < 5; I++) { } } do { } while ( )
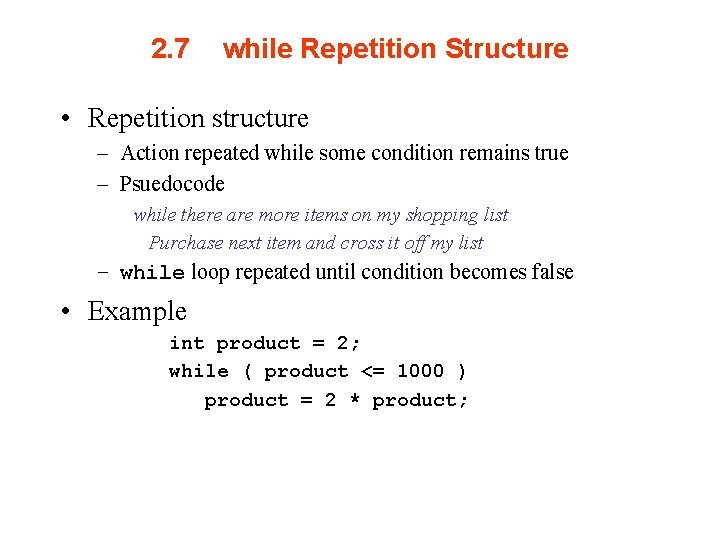
2. 7 while Repetition Structure • Repetition structure – Action repeated while some condition remains true – Psuedocode while there are more items on my shopping list Purchase next item and cross it off my list – while loop repeated until condition becomes false • Example int product = 2; while ( product <= 1000 ) product = 2 * product;
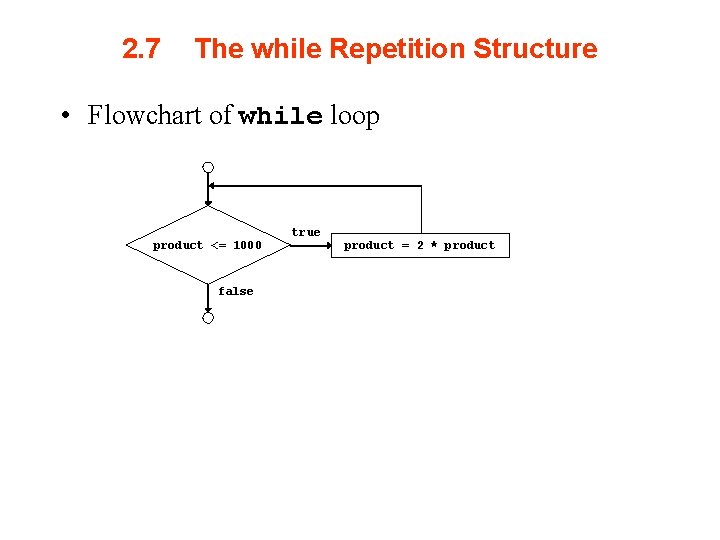
2. 7 The while Repetition Structure • Flowchart of while loop product <= 1000 false true product = 2 * product
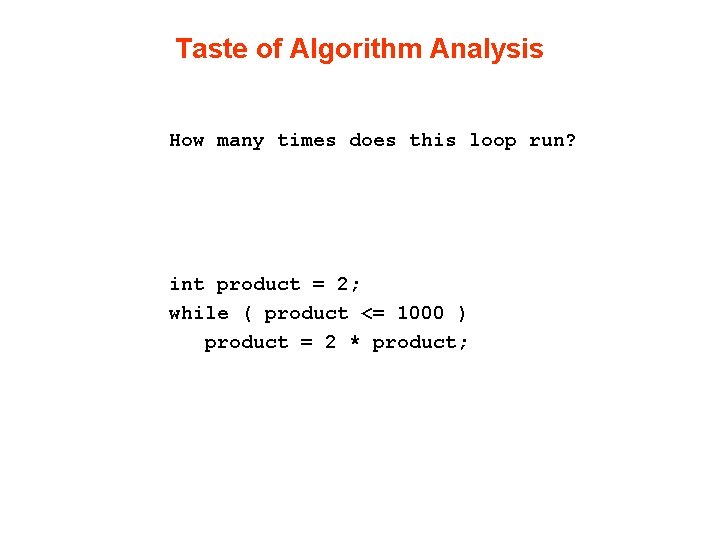
Taste of Algorithm Analysis How many times does this loop run? int product = 2; while ( product <= 1000 ) product = 2 * product;
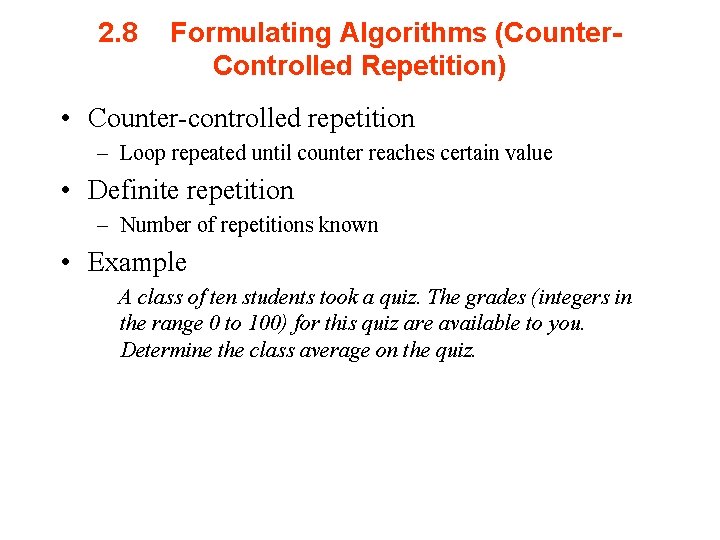
2. 8 Formulating Algorithms (Counter. Controlled Repetition) • Counter-controlled repetition – Loop repeated until counter reaches certain value • Definite repetition – Number of repetitions known • Example A class of ten students took a quiz. The grades (integers in the range 0 to 100) for this quiz are available to you. Determine the class average on the quiz.
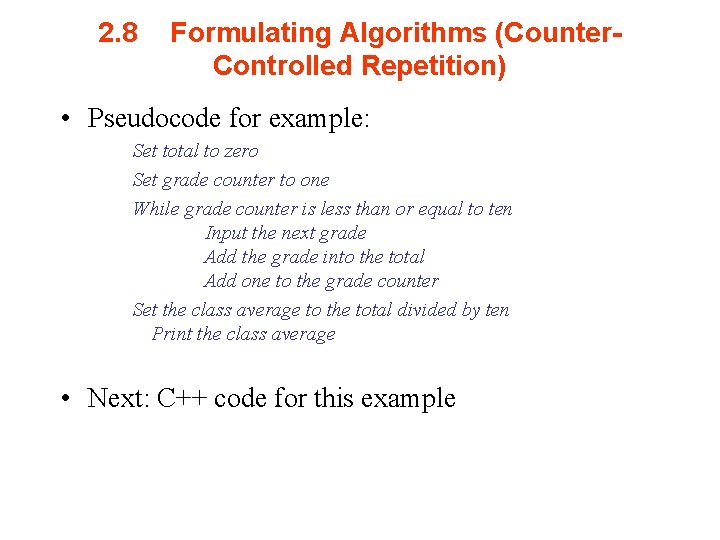
2. 8 Formulating Algorithms (Counter. Controlled Repetition) • Pseudocode for example: Set total to zero Set grade counter to one While grade counter is less than or equal to ten Input the next grade Add the grade into the total Add one to the grade counter Set the class average to the total divided by ten Print the class average • Next: C++ code for this example
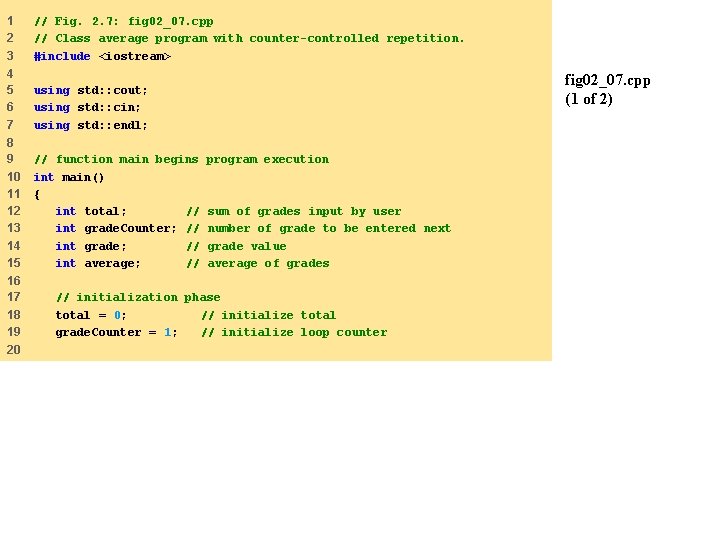
1 2 3 // Fig. 2. 7: fig 02_07. cpp // Class average program with counter-controlled repetition. #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 10 11 12 13 14 15 // function main begins int main() { int total; // int grade. Counter; // int grade; // int average; // 16 17 18 19 20 fig 02_07. cpp (1 of 2) program execution sum of grades input by user number of grade to be entered next grade value average of grades // initialization phase total = 0; // initialize total grade. Counter = 1; // initialize loop counter
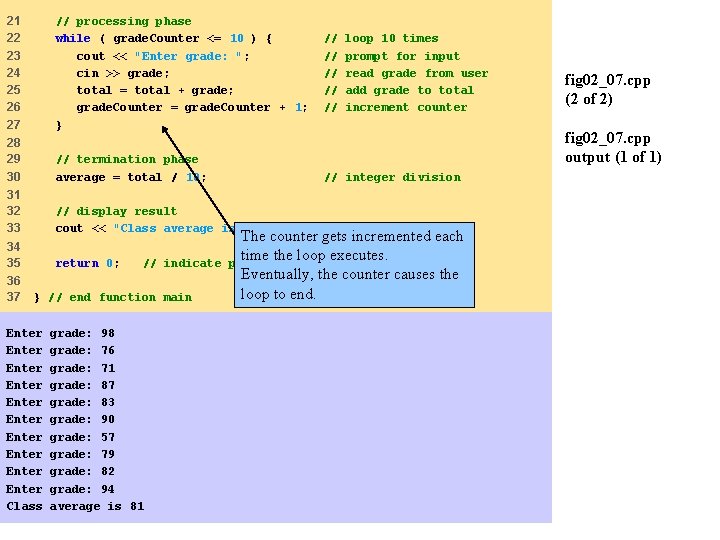
21 22 23 24 25 26 27 // processing phase while ( grade. Counter <= 10 ) { cout << "Enter grade: "; cin >> grade; total = total + grade; grade. Counter = grade. Counter + 1; } 28 29 30 // termination phase average = total / 10; 31 32 33 // display result cout << "Class average is " << average << endl; 34 35 return 0; 36 37 // indicate } // end function main Enter Enter Enter Class grade: 98 grade: 76 grade: 71 grade: 87 grade: 83 grade: 90 grade: 57 grade: 79 grade: 82 grade: 94 average is 81 // // // loop 10 times prompt for input read grade from user add grade to total increment counter fig 02_07. cpp (2 of 2) fig 02_07. cpp output (1 of 1) // integer division The counter gets incremented each time the loop executes. program ended successfully Eventually, the counter causes the loop to end.
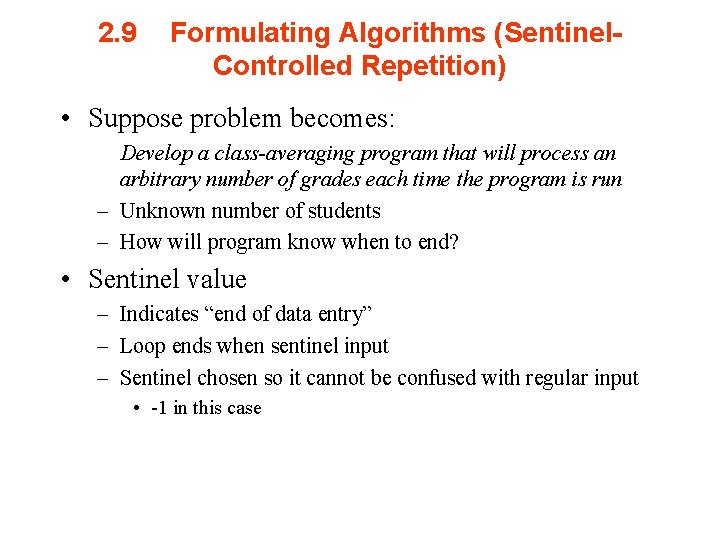
2. 9 Formulating Algorithms (Sentinel. Controlled Repetition) • Suppose problem becomes: Develop a class-averaging program that will process an arbitrary number of grades each time the program is run – Unknown number of students – How will program know when to end? • Sentinel value – Indicates “end of data entry” – Loop ends when sentinel input – Sentinel chosen so it cannot be confused with regular input • -1 in this case
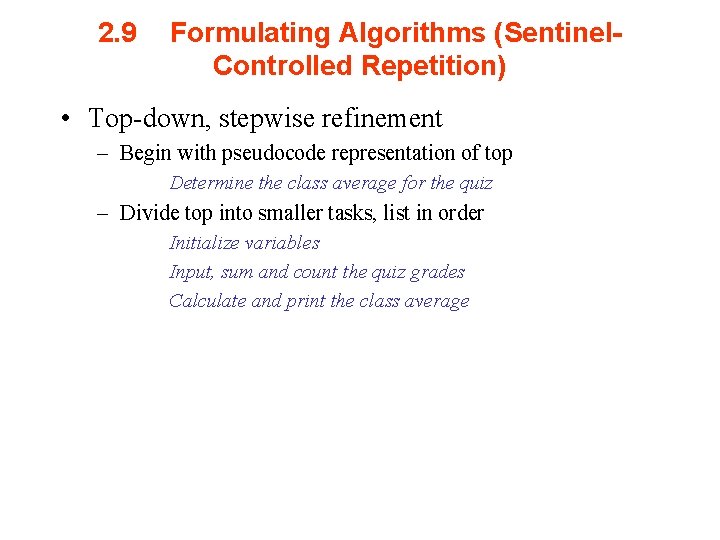
2. 9 Formulating Algorithms (Sentinel. Controlled Repetition) • Top-down, stepwise refinement – Begin with pseudocode representation of top Determine the class average for the quiz – Divide top into smaller tasks, list in order Initialize variables Input, sum and count the quiz grades Calculate and print the class average
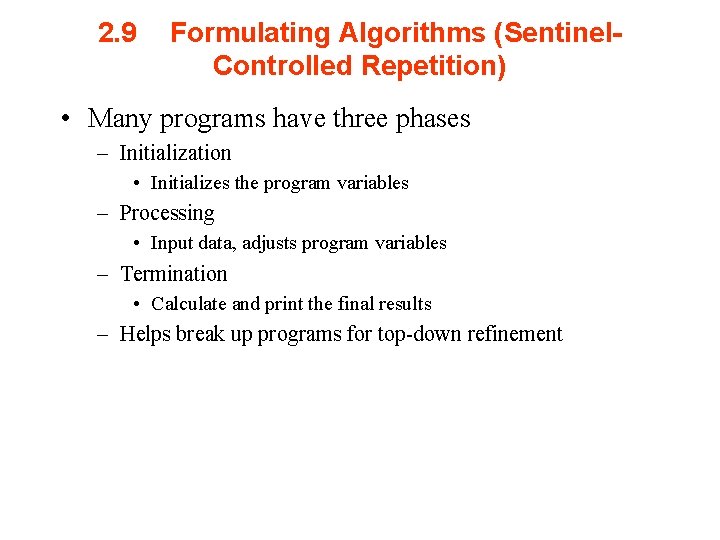
2. 9 Formulating Algorithms (Sentinel. Controlled Repetition) • Many programs have three phases – Initialization • Initializes the program variables – Processing • Input data, adjusts program variables – Termination • Calculate and print the final results – Helps break up programs for top-down refinement
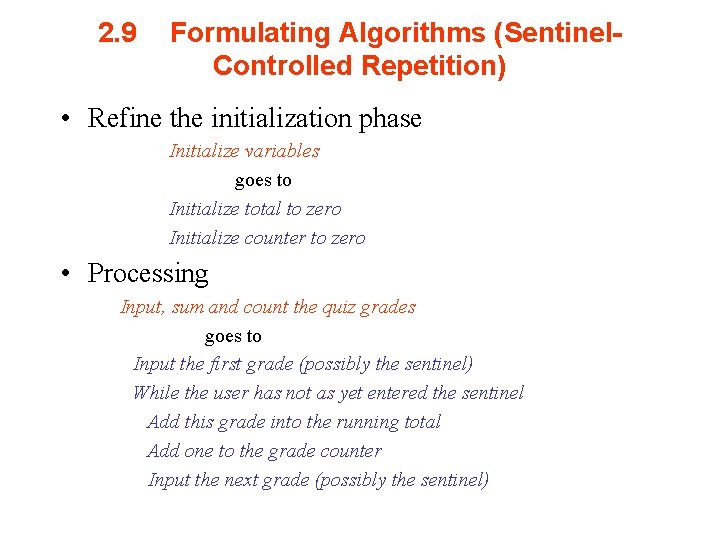
2. 9 Formulating Algorithms (Sentinel. Controlled Repetition) • Refine the initialization phase Initialize variables goes to Initialize total to zero Initialize counter to zero • Processing Input, sum and count the quiz grades goes to Input the first grade (possibly the sentinel) While the user has not as yet entered the sentinel Add this grade into the running total Add one to the grade counter Input the next grade (possibly the sentinel)
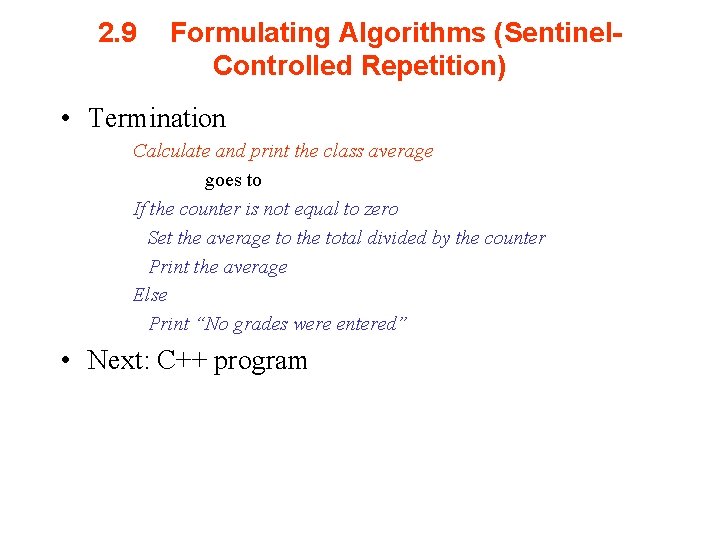
2. 9 Formulating Algorithms (Sentinel. Controlled Repetition) • Termination Calculate and print the class average goes to If the counter is not equal to zero Set the average to the total divided by the counter Print the average Else Print “No grades were entered” • Next: C++ program
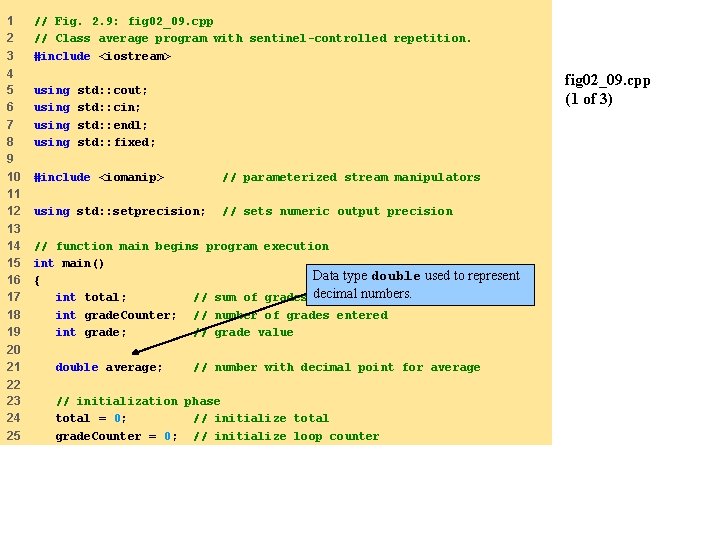
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 // Fig. 2. 9: fig 02_09. cpp // Class average program with sentinel-controlled repetition. #include <iostream> using fig 02_09. cpp (1 of 3) std: : cout; std: : cin; std: : endl; std: : fixed; #include <iomanip> // parameterized stream manipulators using std: : setprecision; // sets numeric output precision // function main begins program execution int main() Data type double used to represent { int total; // sum of grades decimal numbers. int grade. Counter; // number of grades entered int grade; // grade value 20 21 double average; 22 23 24 25 // initialization phase total = 0; // initialize total grade. Counter = 0; // initialize loop counter // number with decimal point for average
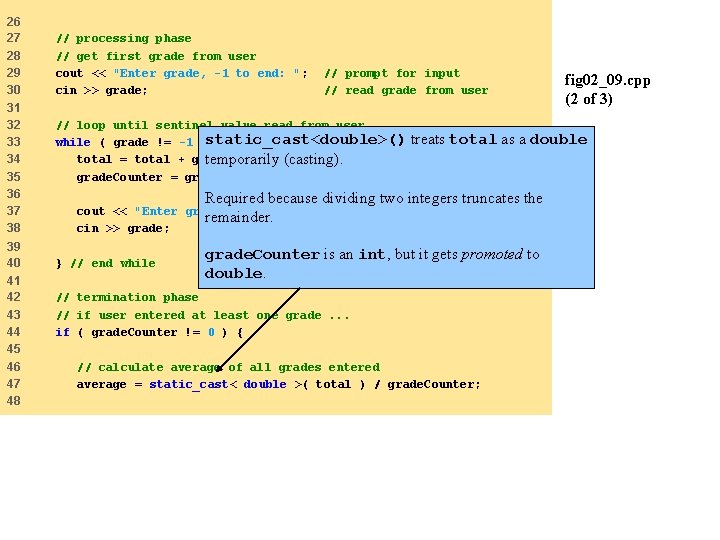
26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 // processing phase // get first grade from user cout << "Enter grade, -1 to end: " ; cin >> grade; // prompt for input // read grade from user fig 02_09. cpp (2 of 3) // loop until sentinel value read from user while ( grade != -1 )static_cast<double>() treats total as a double { total = total + grade; // add grade to total temporarily (casting). grade. Counter = grade. Counter + 1; // increment counter Required because dividing two integers truncates the cout << "Enter grade, -1 to end: " ; remainder. cin >> grade; } // end while // prompt for input // read next grade. Counter is an int, but it gets promoted to double. // termination phase // if user entered at least one grade. . . if ( grade. Counter != 0 ) { // calculate average of all grades entered average = static_cast< double >( total ) / grade. Counter;
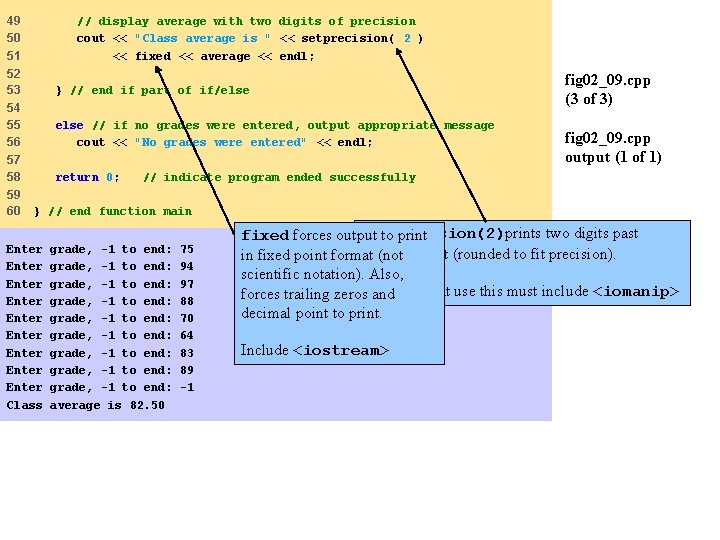
49 50 51 // display average with two digits of precision cout << "Class average is " << setprecision( 2 ) << fixed << average << endl; 52 53 } // end if part of if/else 54 55 56 else // if no grades were entered, output appropriate message cout << "No grades were entered" << endl; 57 58 return 0; 59 60 fig 02_09. cpp (3 of 3) fig 02_09. cpp output (1 of 1) // indicate program ended successfully } // end function main Enter Enter Enter Class grade, -1 to end: grade, -1 to end: grade, -1 to end: average is 82. 50 75 94 97 88 70 64 83 89 -1 setprecision(2)prints two digits past fixed forces output to print decimal point (rounded to fit precision). in fixed point format (not scientific notation). Also, Programs that use this must include <iomanip> forces trailing zeros and decimal point to print. Include <iostream>
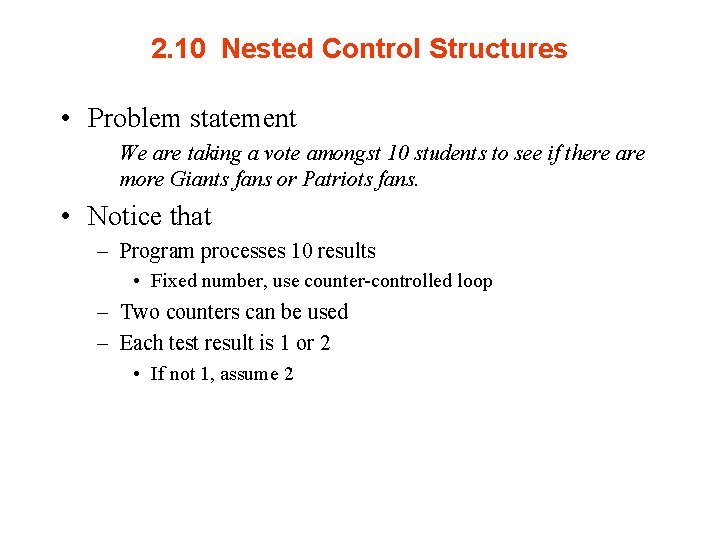
2. 10 Nested Control Structures • Problem statement We are taking a vote amongst 10 students to see if there are more Giants fans or Patriots fans. • Notice that – Program processes 10 results • Fixed number, use counter-controlled loop – Two counters can be used – Each test result is 1 or 2 • If not 1, assume 2
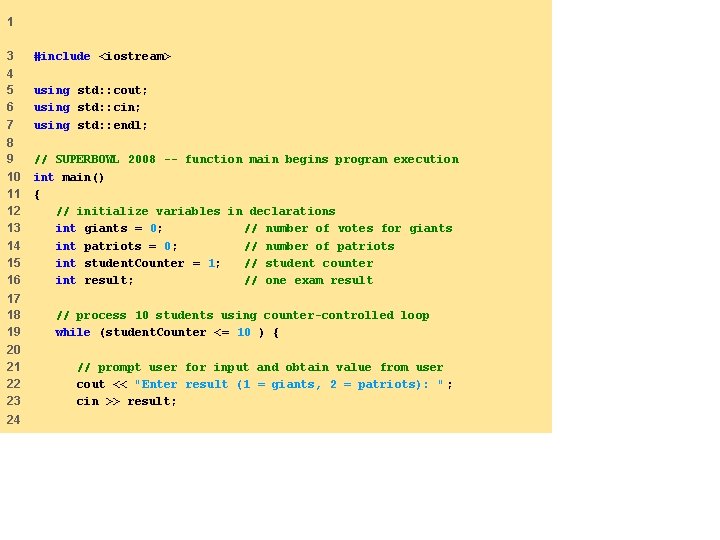
1 3 #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 10 11 12 13 14 15 16 // SUPERBOWL 2008 -- function main begins program execution int main() { // initialize variables in declarations int giants = 0; // number of votes for giants int patriots = 0; // number of patriots int student. Counter = 1; // student counter int result; // one exam result 17 18 19 20 21 22 23 24 // process 10 students using counter-controlled loop while (student. Counter <= 10 ) { // prompt user for input and obtain value from user cout << "Enter result (1 = giants, 2 = patriots): " ; cin >> result;
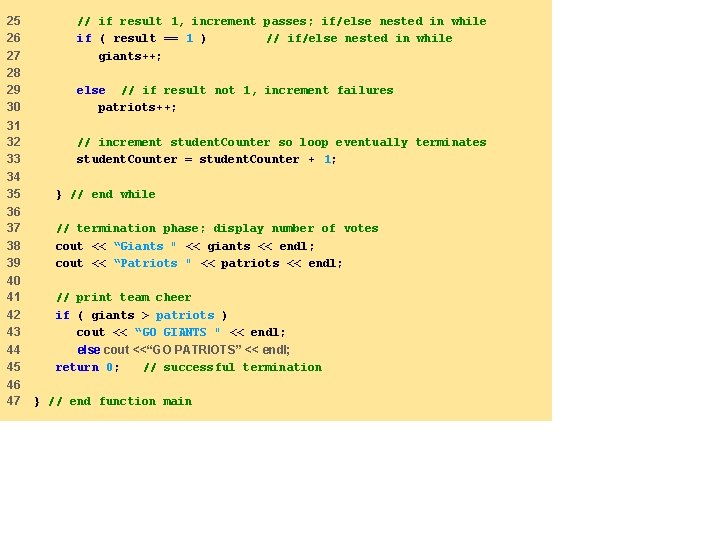
25 26 27 28 29 30 // if result 1, increment passes; if/else nested in while if ( result == 1 ) // if/else nested in while giants++; else // if result not 1, increment failures patriots++; 31 32 33 34 35 } // end while 36 37 38 39 // termination phase; display number of votes cout << “Giants " << giants << endl; cout << “Patriots " << patriots << endl; 40 41 42 43 // print team cheer if ( giants > patriots ) cout << “GO GIANTS " << endl; 44 45 else cout <<“GO PATRIOTS” << endl; return 0; // successful termination 46 47 // increment student. Counter so loop eventually terminates student. Counter = student. Counter + 1; } // end function main
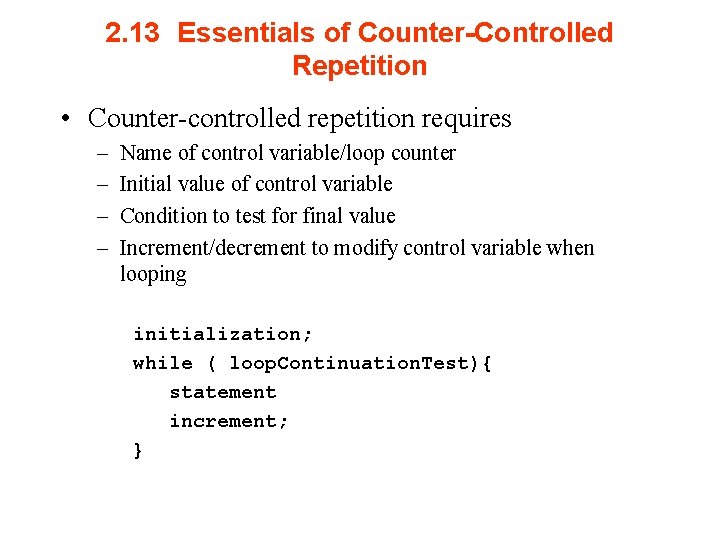
2. 13 Essentials of Counter-Controlled Repetition • Counter-controlled repetition requires – – Name of control variable/loop counter Initial value of control variable Condition to test for final value Increment/decrement to modify control variable when looping initialization; while ( loop. Continuation. Test){ statement increment; }
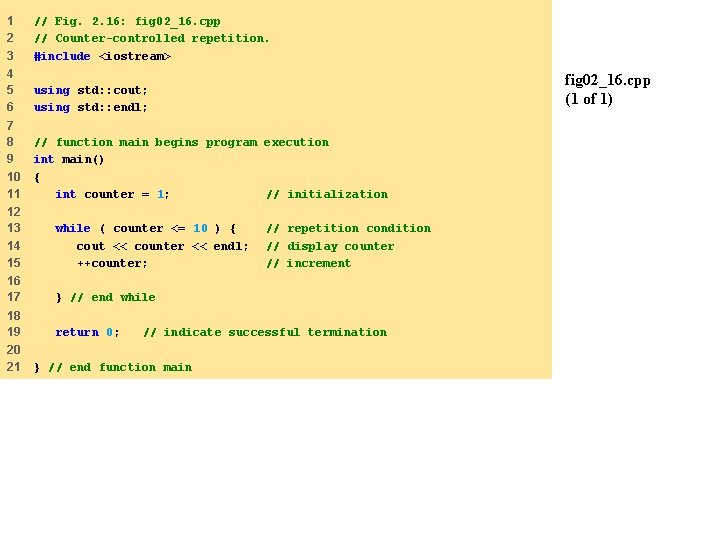
1 2 3 // Fig. 2. 16: fig 02_16. cpp // Counter-controlled repetition. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 // function main begins program execution int main() { int counter = 1; // initialization 12 13 14 15 while ( counter <= 10 ) { cout << counter << endl; ++counter; 16 17 } // end while 18 19 return 0; 20 21 fig 02_16. cpp (1 of 1) // repetition condition // display counter // increment // indicate successful termination } // end function main
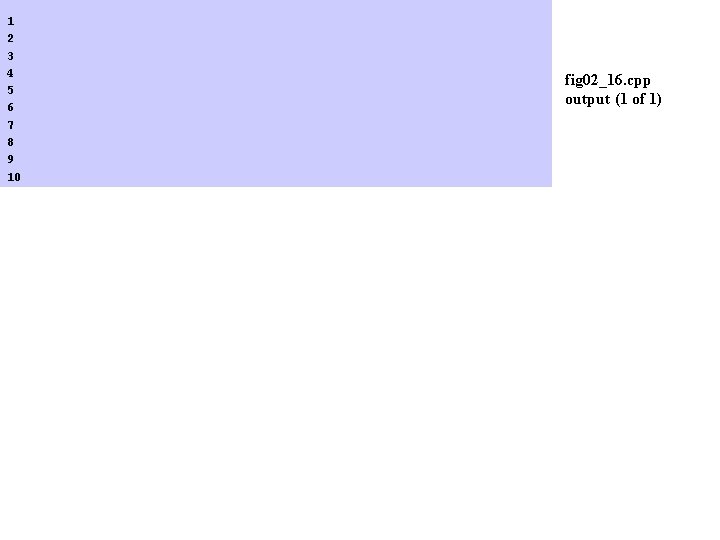
1 2 3 4 5 6 7 8 9 10 fig 02_16. cpp output (1 of 1)
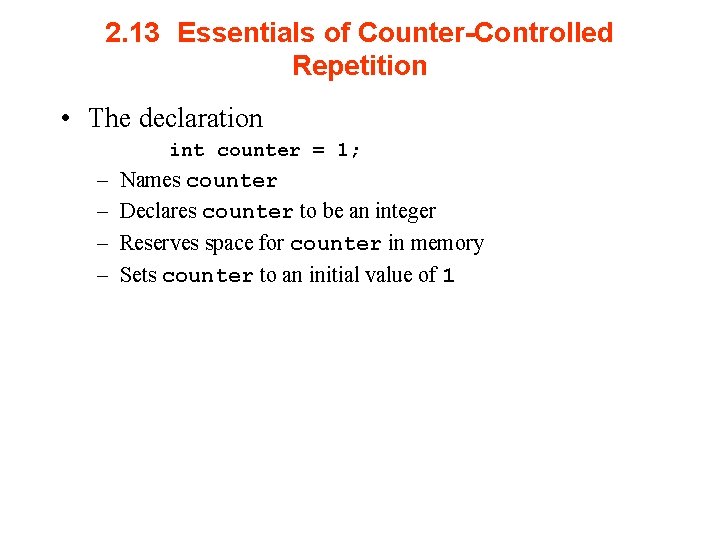
2. 13 Essentials of Counter-Controlled Repetition • The declaration int counter = 1; – – Names counter Declares counter to be an integer Reserves space for counter in memory Sets counter to an initial value of 1
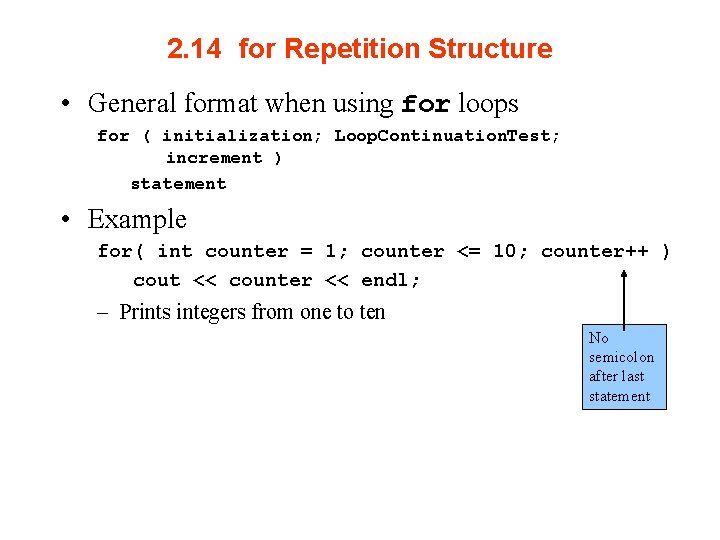
2. 14 for Repetition Structure • General format when using for loops for ( initialization; Loop. Continuation. Test; increment ) statement • Example for( int counter = 1; counter <= 10; counter++ ) cout << counter << endl; – Prints integers from one to ten No semicolon after last statement
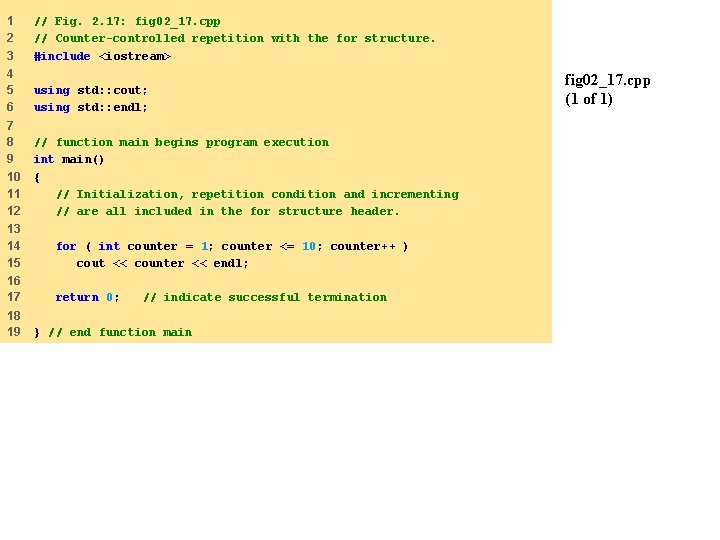
1 2 3 // Fig. 2. 17: fig 02_17. cpp // Counter-controlled repetition with the for structure. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 12 // function main begins program execution int main() { // Initialization, repetition condition and incrementing // are all included in the for structure header. 13 14 15 for ( int counter = 1; counter <= 10; counter++ ) cout << counter << endl; 16 17 return 0; 18 19 // indicate successful termination } // end function main fig 02_17. cpp (1 of 1)
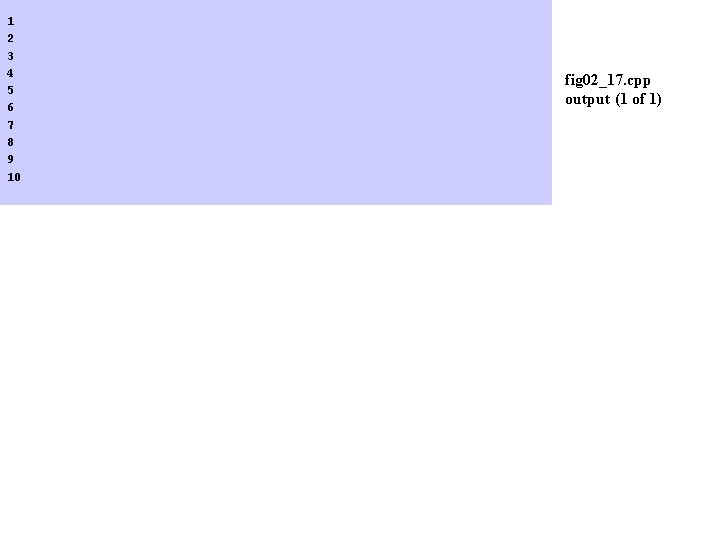
1 2 3 4 5 6 7 8 9 10 fig 02_17. cpp output (1 of 1)
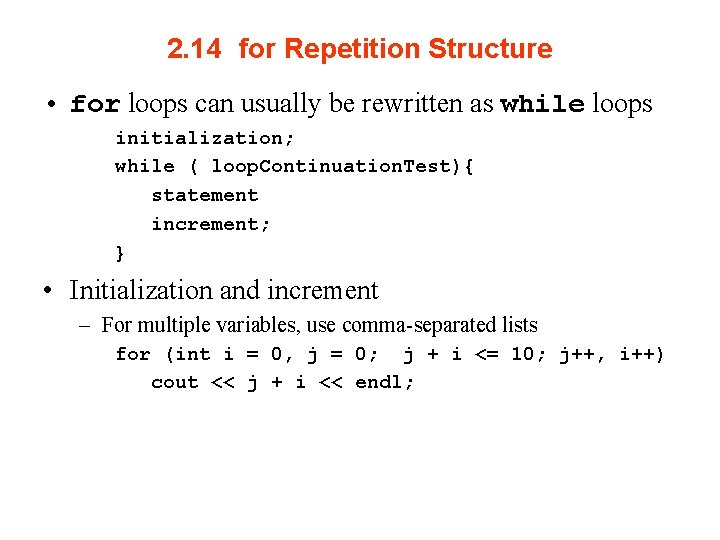
2. 14 for Repetition Structure • for loops can usually be rewritten as while loops initialization; while ( loop. Continuation. Test){ statement increment; } • Initialization and increment – For multiple variables, use comma-separated lists for (int i = 0, j = 0; j + i <= 10; j++, i++) cout << j + i << endl;
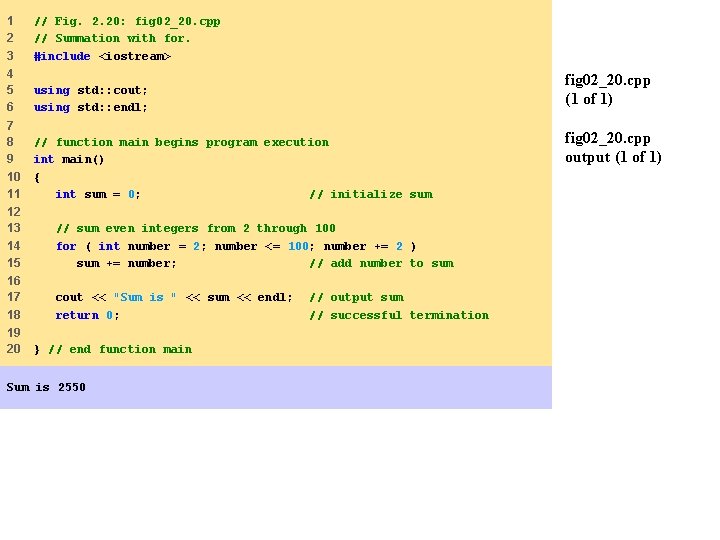
1 2 3 // Fig. 2. 20: fig 02_20. cpp // Summation with for. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 // function main begins program execution int main() { int sum = 0; // initialize sum fig 02_20. cpp (1 of 1) 12 13 14 15 // sum even integers from 2 through 100 for ( int number = 2; number <= 100; number += 2 ) sum += number; // add number to sum 16 17 18 cout << "Sum is " << sum << endl; return 0; 19 20 } // end function main Sum is 2550 // output sum // successful termination fig 02_20. cpp output (1 of 1)
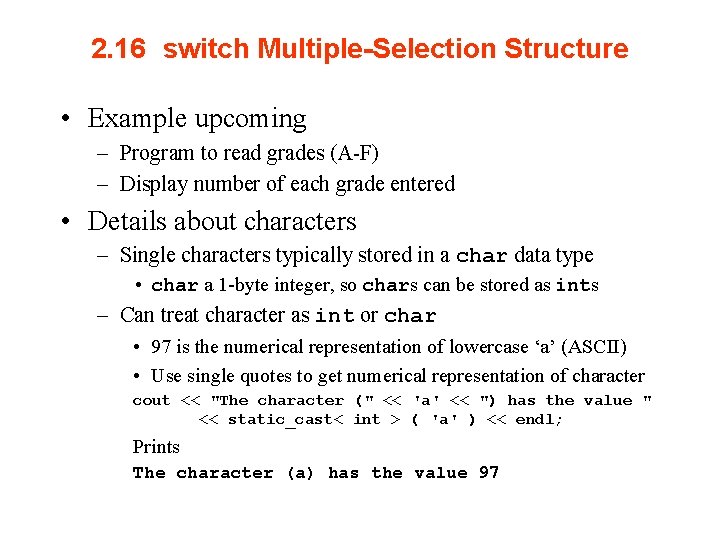
2. 16 switch Multiple-Selection Structure • Example upcoming – Program to read grades (A-F) – Display number of each grade entered • Details about characters – Single characters typically stored in a char data type • char a 1 -byte integer, so chars can be stored as ints – Can treat character as int or char • 97 is the numerical representation of lowercase ‘a’ (ASCII) • Use single quotes to get numerical representation of character cout << "The character (" << 'a' << ") has the value " << static_cast< int > ( 'a' ) << endl; Prints The character (a) has the value 97
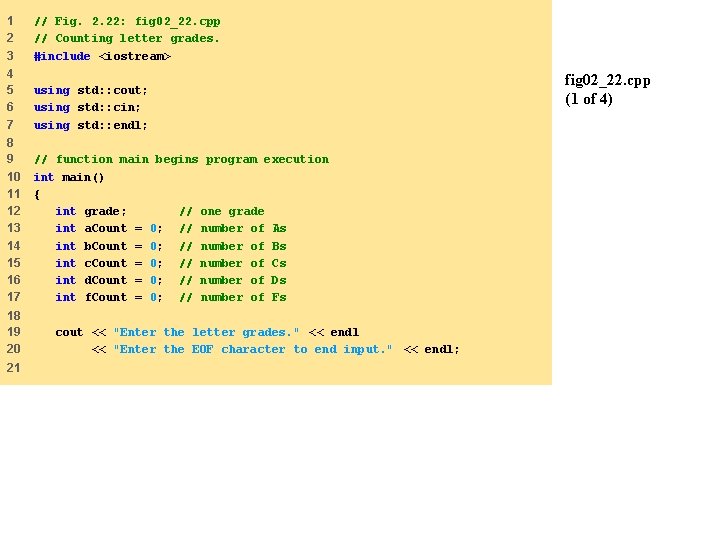
1 2 3 // Fig. 2. 22: fig 02_22. cpp // Counting letter grades. #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 10 11 12 13 14 15 16 17 // function main begins program execution int main() { int grade; // one grade int a. Count = 0; // number of As int b. Count = 0; // number of Bs int c. Count = 0; // number of Cs int d. Count = 0; // number of Ds int f. Count = 0; // number of Fs 18 19 20 21 cout << "Enter the letter grades. " << endl << "Enter the EOF character to end input. " << endl; fig 02_22. cpp (1 of 4)
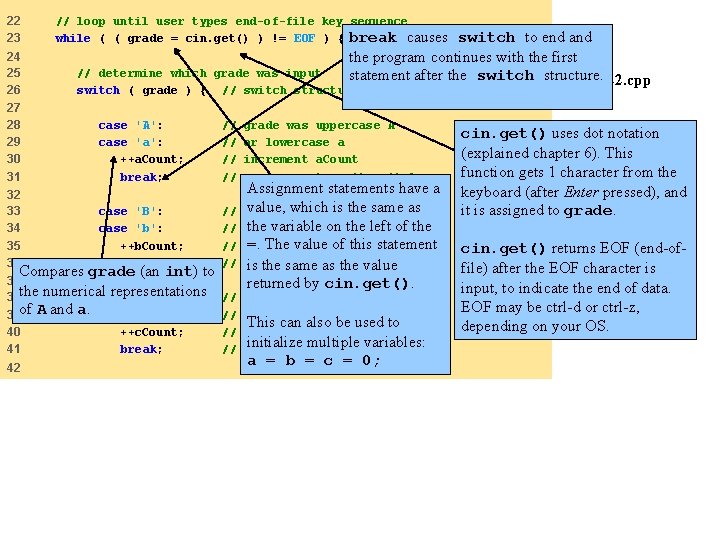
22 23 // loop until user types end-of-file key sequence while ( ( grade = cin. get() ) != EOF ) { break causes switch to end and the program continues with the first grade was input statement after the switch structure. fig 02_22. cpp // switch structure nested in while (2 of 4) 24 25 26 // determine which switch ( grade ) { 27 28 29 30 31 case 'A': case 'a': ++a. Count; break; // // 32 33 34 35 36 case 'B': case 'b': ++b. Count; break; // // Assignment statements have a value, which is the same as grade was uppercase B the variable on the left of the or lowercase b =. The value of this statement increment b. Count exit switch is the same as the value returned by cin. get(). // // grade was uppercase C or lowercase c This can also be used to increment c. Count initialize multiple variables: exit switch Compares grade (an int) to 37 38 the numerical representations case 'C': of A and a. 39 case 'c': 40 ++c. Count; 41 break; 42 grade was uppercase A or lowercase a increment a. Count necessary to exit switch a = b = c = 0; cin. get() uses dot notation (explained chapter 6). This function gets 1 character from the keyboard (after Enter pressed), and it is assigned to grade. cin. get() returns EOF (end-offile) after the EOF character is input, to indicate the end of data. EOF may be ctrl-d or ctrl-z, depending on your OS.
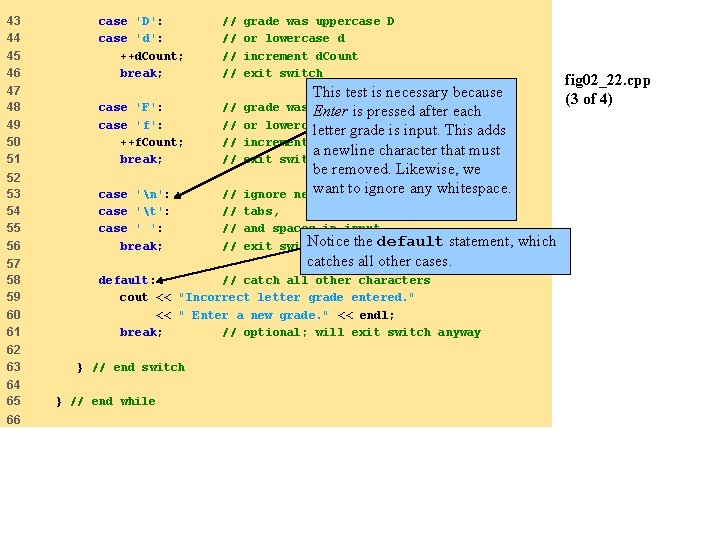
43 44 45 46 case 'D': case 'd': ++d. Count; break; // // grade was uppercase D or lowercase d increment d. Count exit switch This test is necessary because grade was Enter is pressed after each uppercase F or lowercase f letter grade is input. This adds increment f. Count a newline character that must exit switch be removed. Likewise, we want to ignore any whitespace. ignore newlines, 47 48 49 50 51 case 'F': case 'f': ++f. Count; break; // // 52 53 54 55 56 case 'n': case 't': case ' ': break; // // tabs, // and spaces in input Notice the default statement, which // exit switch 57 58 59 60 61 default: // catch all other characters cout << "Incorrect letter grade entered. " << " Enter a new grade. " << endl; break; // optional; will exit switch anyway 62 63 64 65 66 } // end switch } // end while catches all other cases. fig 02_22. cpp (3 of 4)
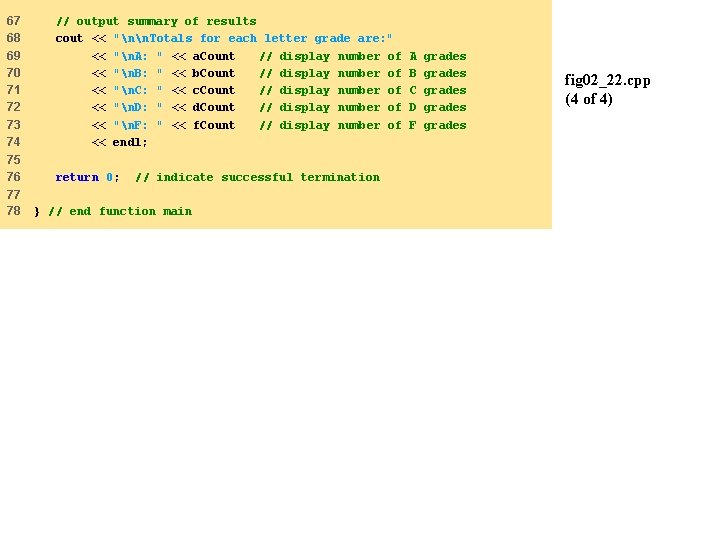
67 68 69 70 71 72 73 74 // output summary of results cout << "nn. Totals for each letter grade are: " << "n. A: " << a. Count // display number of << "n. B: " << b. Count // display number of << "n. C: " << c. Count // display number of << "n. D: " << d. Count // display number of << "n. F: " << f. Count // display number of << endl; 75 76 return 0; 77 78 // indicate successful termination } // end function main A B C D F grades grades fig 02_22. cpp (4 of 4)
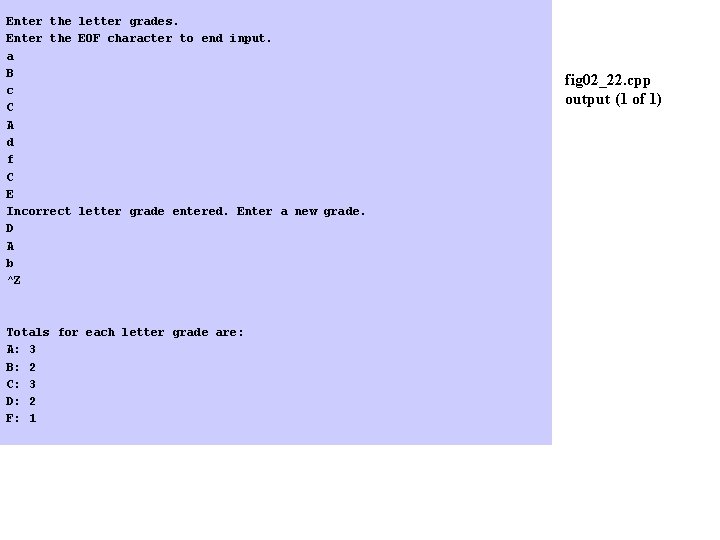
Enter the letter grades. Enter the EOF character to end input. a B c C A d f C E Incorrect letter grade entered. Enter a new grade. D A b ^Z Totals for each letter grade are: A: 3 B: 2 C: 3 D: 2 F: 1 fig 02_22. cpp output (1 of 1)
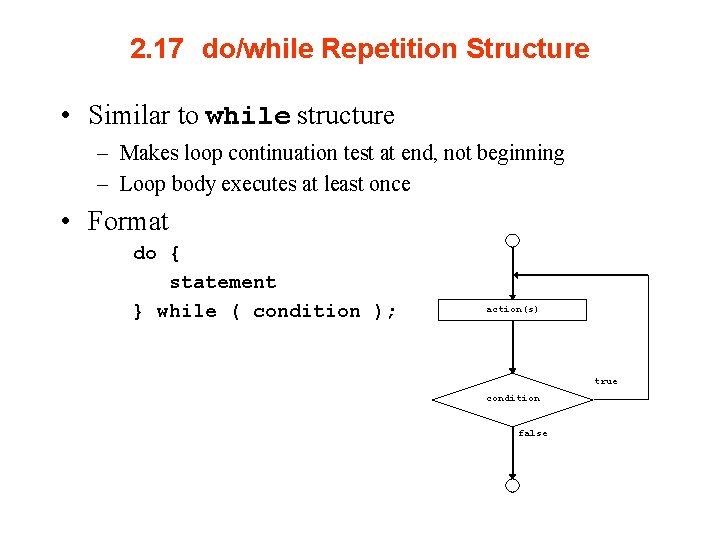
2. 17 do/while Repetition Structure • Similar to while structure – Makes loop continuation test at end, not beginning – Loop body executes at least once • Format do { statement } while ( condition ); action(s) true condition false
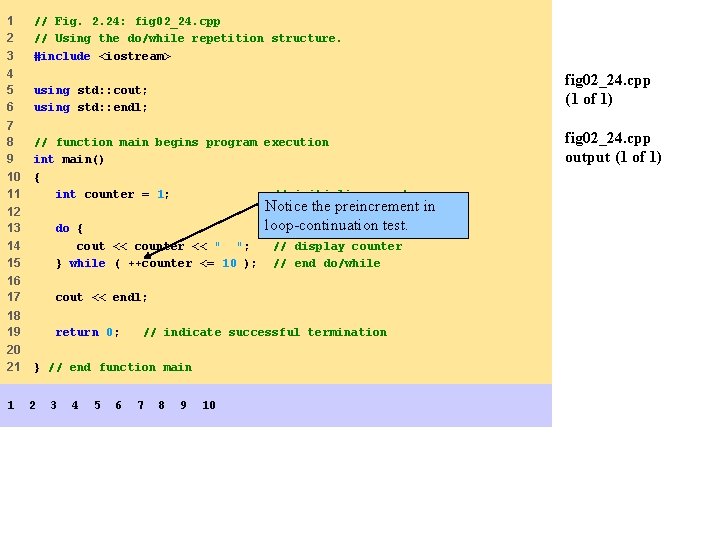
1 2 3 // Fig. 2. 24: fig 02_24. cpp // Using the do/while repetition structure. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 // function main begins program execution int main() { int counter = 1; // initialize counter fig 02_24. cpp (1 of 1) 12 13 14 15 do { cout << counter << " "; } while ( ++counter <= 10 ); 16 17 cout << endl; 18 19 return 0; 20 21 1 3 4 5 6 // display counter // end do/while // indicate successful termination } // end function main 2 Notice the preincrement in loop-continuation test. 7 8 9 10 fig 02_24. cpp output (1 of 1)
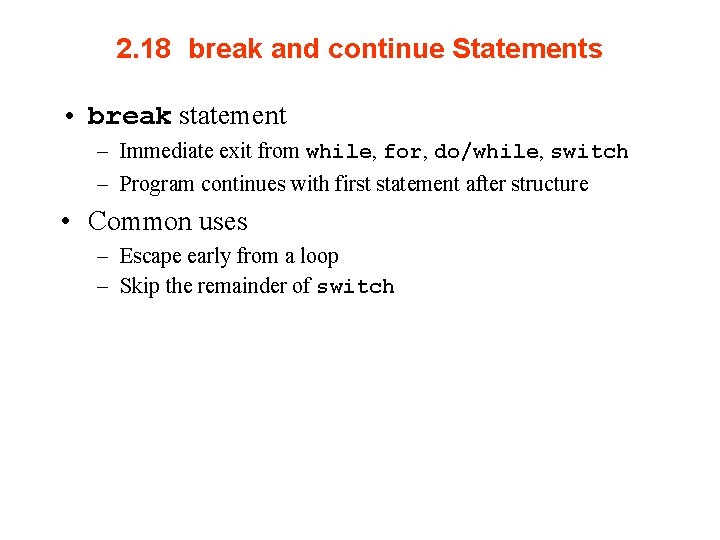
2. 18 break and continue Statements • break statement – Immediate exit from while, for, do/while, switch – Program continues with first statement after structure • Common uses – Escape early from a loop – Skip the remainder of switch
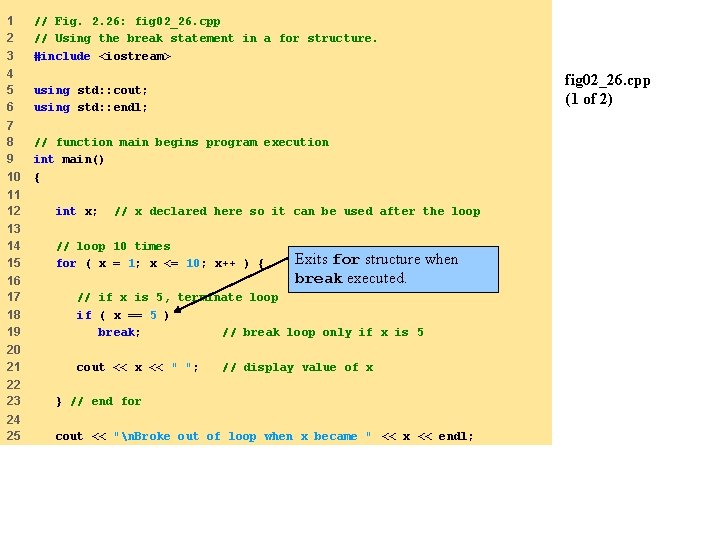
1 2 3 // Fig. 2. 26: fig 02_26. cpp // Using the break statement in a for structure. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 // function main begins program execution int main() { fig 02_26. cpp (1 of 2) 11 12 int x; 13 14 15 // loop 10 times for ( x = 1; x <= 10; x++ ) { // x declared here so it can be used after the loop Exits for structure when break executed. 16 17 18 19 // if x is 5, terminate loop if ( x == 5 ) break; // break loop only if x is 5 20 21 cout << x << " "; // display value of x 22 23 } // end for 24 25 cout << "n. Broke out of loop when x became " << x << endl;
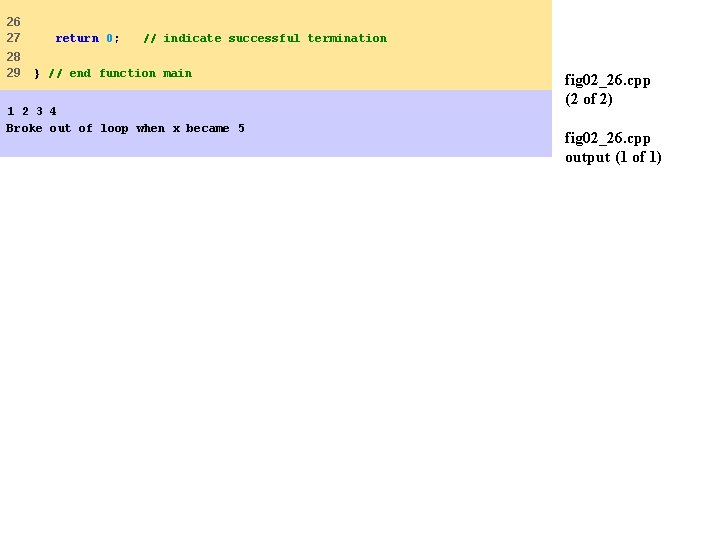
26 27 28 29 return 0; // indicate successful termination } // end function main 1 2 3 4 Broke out of loop when x became 5 fig 02_26. cpp (2 of 2) fig 02_26. cpp output (1 of 1)
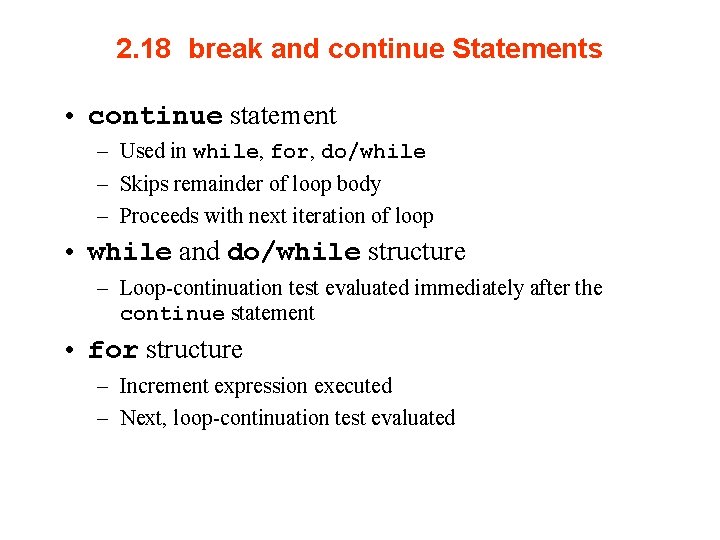
2. 18 break and continue Statements • continue statement – Used in while, for, do/while – Skips remainder of loop body – Proceeds with next iteration of loop • while and do/while structure – Loop-continuation test evaluated immediately after the continue statement • for structure – Increment expression executed – Next, loop-continuation test evaluated
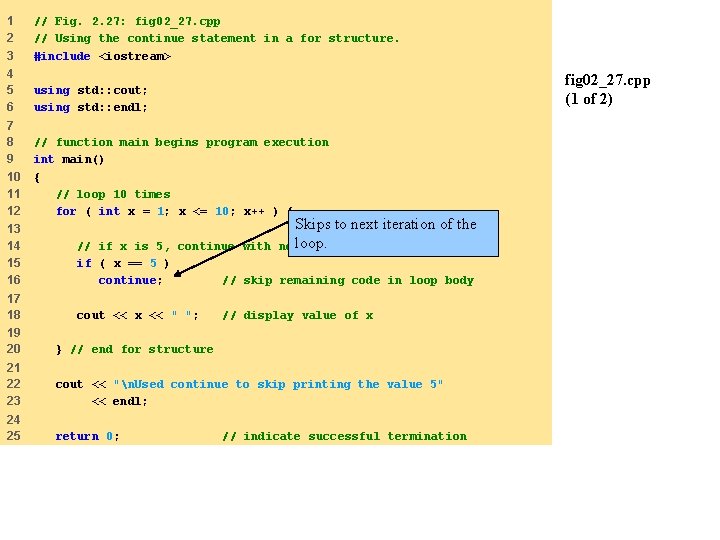
1 2 3 // Fig. 2. 27: fig 02_27. cpp // Using the continue statement in a for structure. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 12 // function main begins program execution int main() { // loop 10 times for ( int x = 1; x <= 10; x++ ) { fig 02_27. cpp (1 of 2) Skips to next iteration of the loop. next iteration of loop 13 14 15 16 // if x is 5, continue with if ( x == 5 ) continue; // skip remaining code in loop body 17 18 cout << x << " "; // display value of x 19 20 } // end for structure 21 22 23 cout << "n. Used continue to skip printing the value 5" << endl; 24 25 return 0; // indicate successful termination
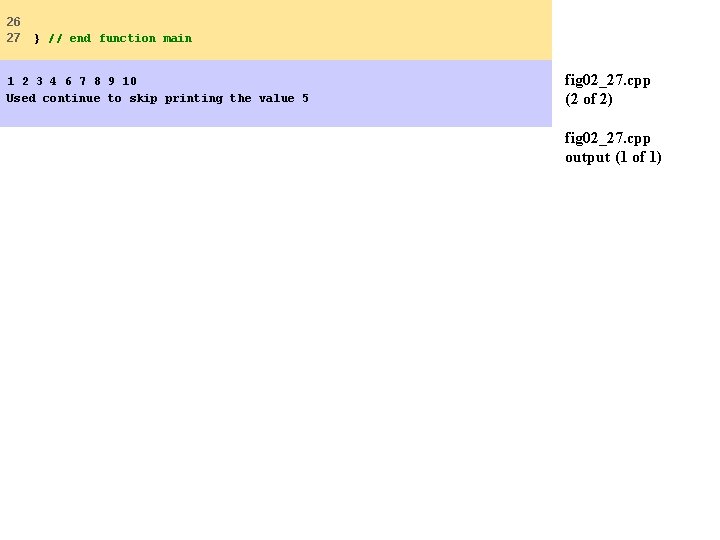
26 27 } // end function main 1 2 3 4 6 7 8 9 10 Used continue to skip printing the value 5 fig 02_27. cpp (2 of 2) fig 02_27. cpp output (1 of 1)
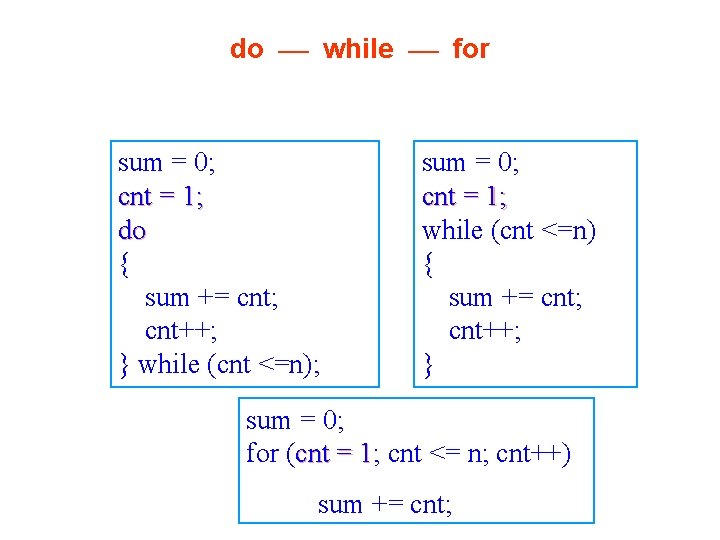
do ¾ while ¾ for sum = 0; cnt = 1; do { sum += cnt; cnt++; } while (cnt <=n); sum = 0; cnt = 1; while (cnt <=n) { sum += cnt; cnt++; } sum = 0; for (cnt = 1; cnt <= n; cnt++) cnt = 1 sum += cnt;
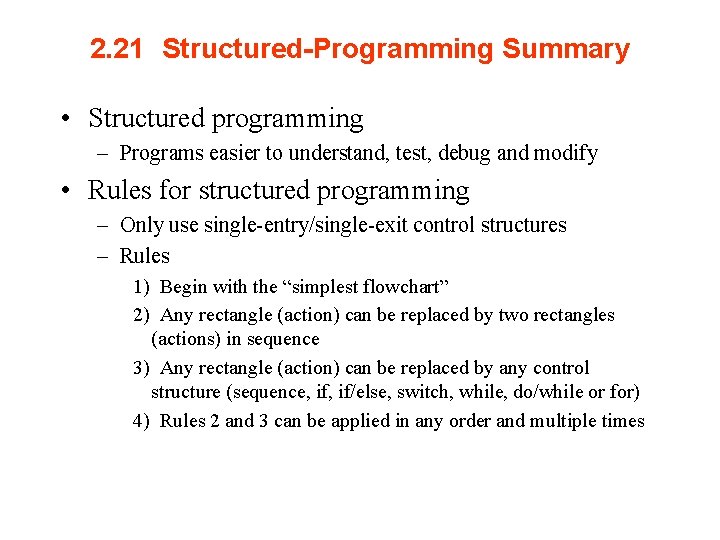
2. 21 Structured-Programming Summary • Structured programming – Programs easier to understand, test, debug and modify • Rules for structured programming – Only use single-entry/single-exit control structures – Rules 1) Begin with the “simplest flowchart” 2) Any rectangle (action) can be replaced by two rectangles (actions) in sequence 3) Any rectangle (action) can be replaced by any control structure (sequence, if/else, switch, while, do/while or for) 4) Rules 2 and 3 can be applied in any order and multiple times
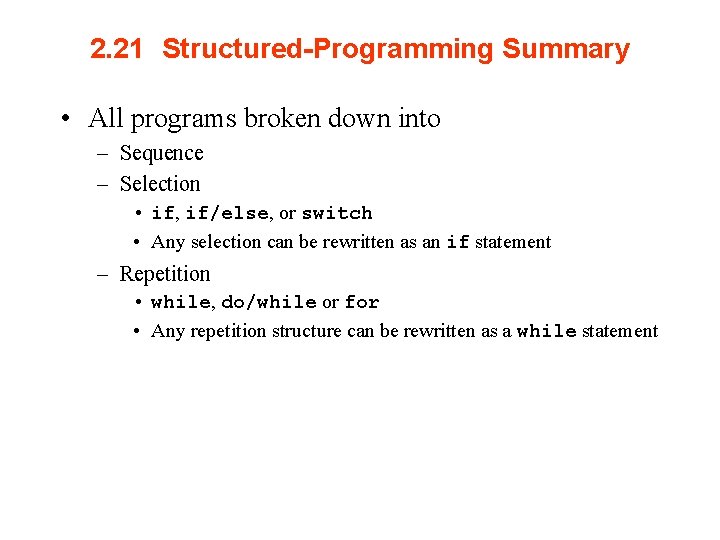
2. 21 Structured-Programming Summary • All programs broken down into – Sequence – Selection • if, if/else, or switch • Any selection can be rewritten as an if statement – Repetition • while, do/while or for • Any repetition structure can be rewritten as a while statement
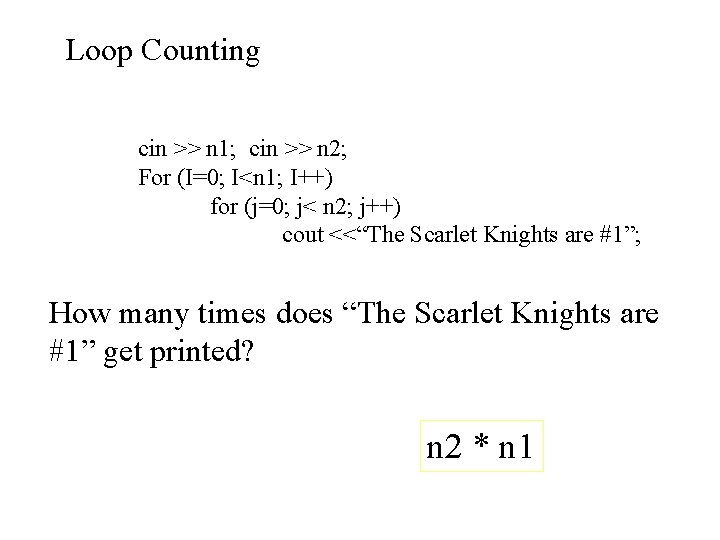
Loop Counting cin >> n 1; cin >> n 2; For (I=0; I<n 1; I++) for (j=0; j< n 2; j++) cout <<“The Scarlet Knights are #1”; How many times does “The Scarlet Knights are #1” get printed? n 2 * n 1
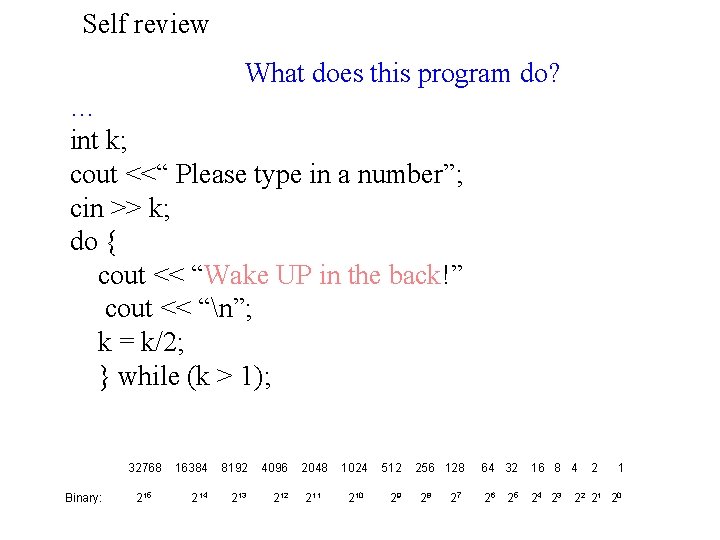
Self review What does this program do? … int k; cout <<“ Please type in a number”; cin >> k; do { cout << “Wake UP in the back!” cout << “n”; k = k/2; } while (k > 1); 32768 16384 8192 4096 2048 1024 512 256 128 64 32 16 8 4 2 1 Binary: 2 15 2 14 213 2 12 211 2 10 2 9 28 27 26 25 24 23 22 21 20
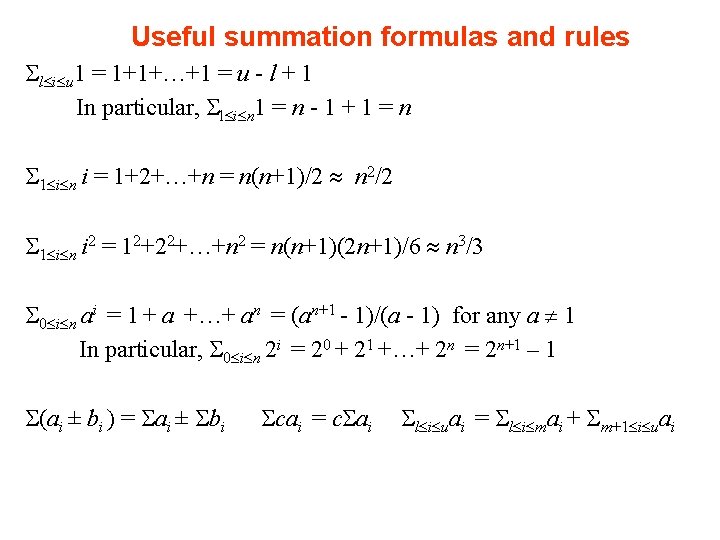
Useful summation formulas and rules l i u 1 = 1+1+…+1 = u - l + 1 In particular, l i n 1 = n - 1 + 1 = n 1 i n i = 1+2+…+n = n(n+1)/2 n 2/2 1 i n i 2 = 12+22+…+n 2 = n(n+1)(2 n+1)/6 n 3/3 0 i n ai = 1 + a +…+ an = (an+1 - 1)/(a - 1) for any a 1 In particular, 0 i n 2 i = 20 + 21 +…+ 2 n = 2 n+1 – 1 (ai ± bi ) = ai ± bi cai = c ai l i uai = l i mai + m+1 i uai
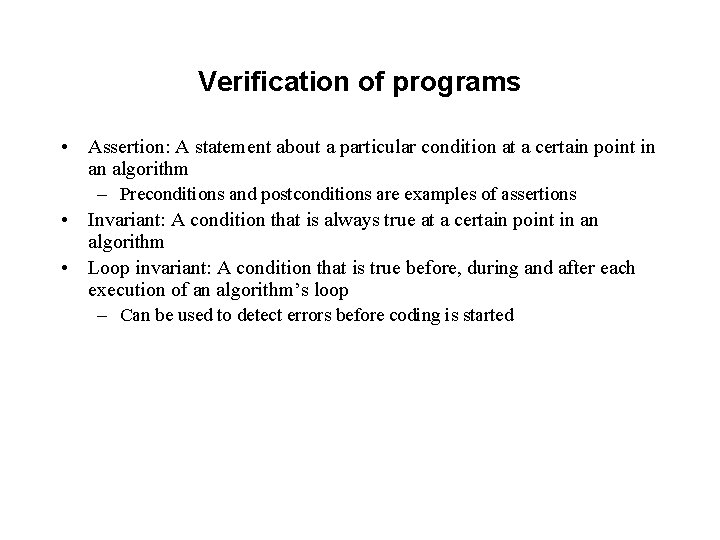
Verification of programs • Assertion: A statement about a particular condition at a certain point in an algorithm – Preconditions and postconditions are examples of assertions • Invariant: A condition that is always true at a certain point in an algorithm • Loop invariant: A condition that is true before, during and after each execution of an algorithm’s loop – Can be used to detect errors before coding is started
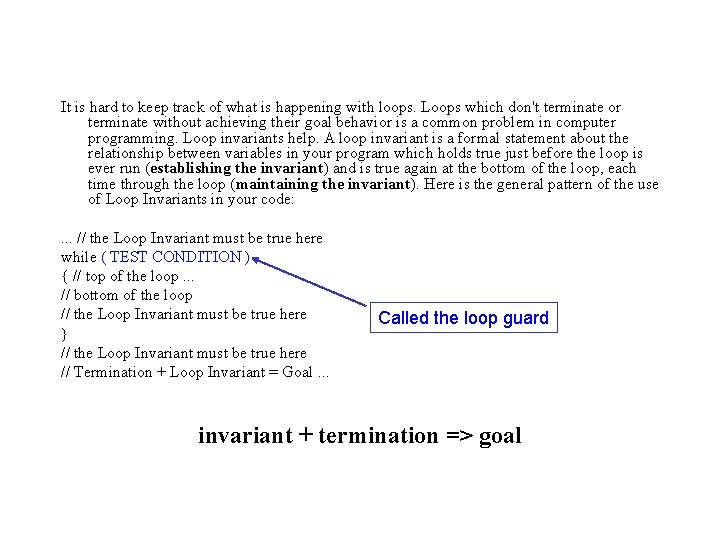
It is hard to keep track of what is happening with loops. Loops which don't terminate or terminate without achieving their goal behavior is a common problem in computer programming. Loop invariants help. A loop invariant is a formal statement about the relationship between variables in your program which holds true just before the loop is ever run (establishing the invariant) and is true again at the bottom of the loop, each time through the loop (maintaining the invariant). Here is the general pattern of the use of Loop Invariants in your code: . . . // the Loop Invariant must be true here while ( TEST CONDITION ) { // top of the loop. . . // bottom of the loop // the Loop Invariant must be true here } // the Loop Invariant must be true here // Termination + Loop Invariant = Goal. . . Called the loop guard invariant + termination => goal
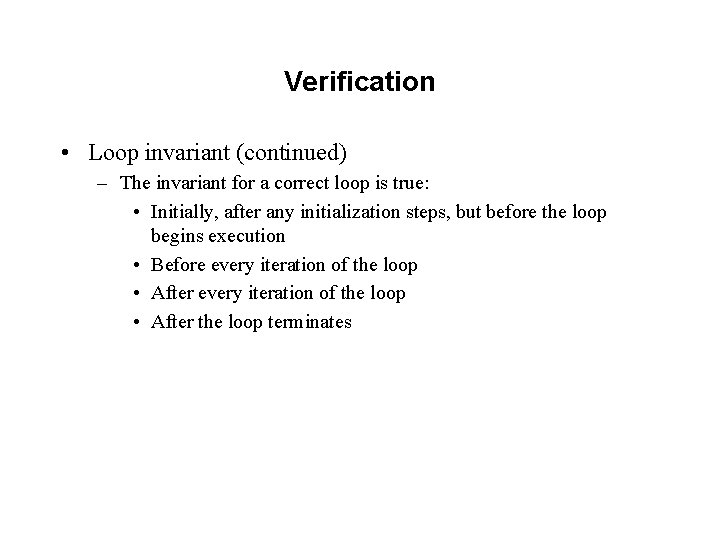
Verification • Loop invariant (continued) – The invariant for a correct loop is true: • Initially, after any initialization steps, but before the loop begins execution • Before every iteration of the loop • After the loop terminates
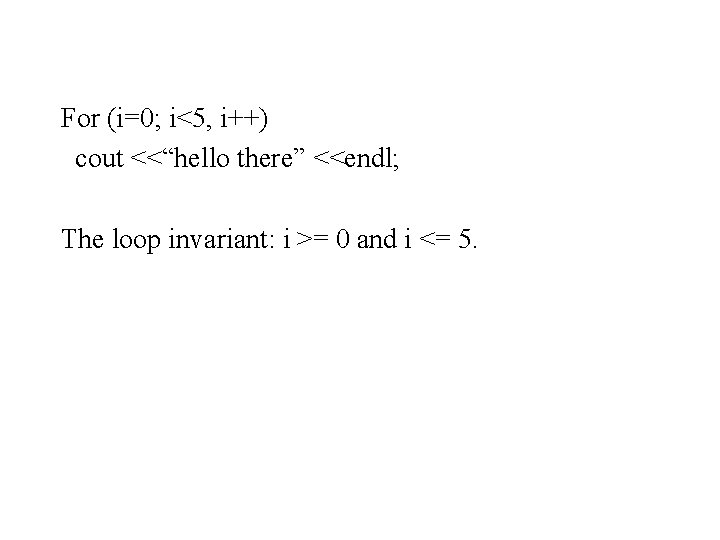
For (i=0; i<5, i++) cout <<“hello there” <<endl; The loop invariant: i >= 0 and i <= 5.
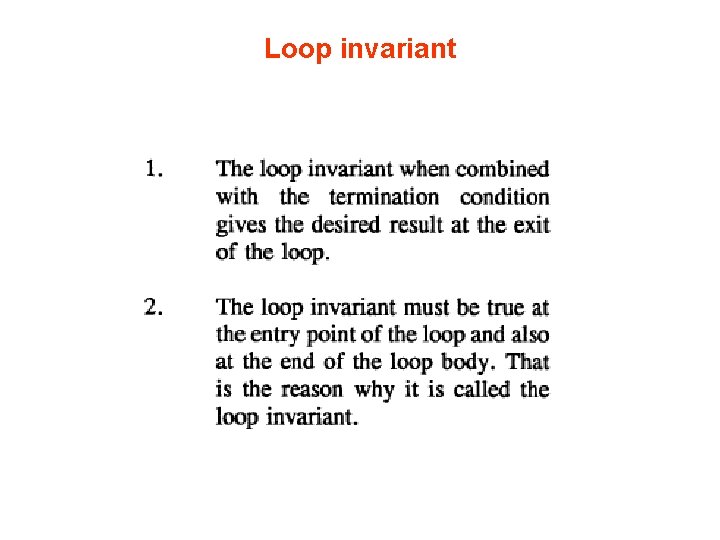
Loop invariant
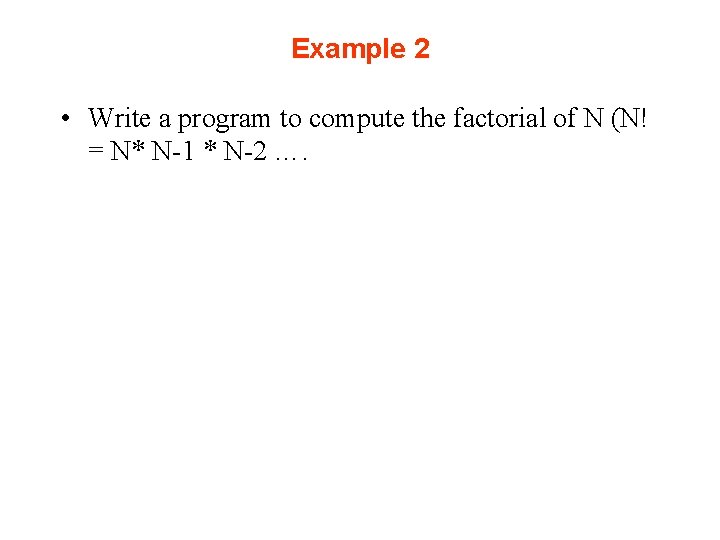
Example 2 • Write a program to compute the factorial of N (N! = N* N-1 * N-2 ….
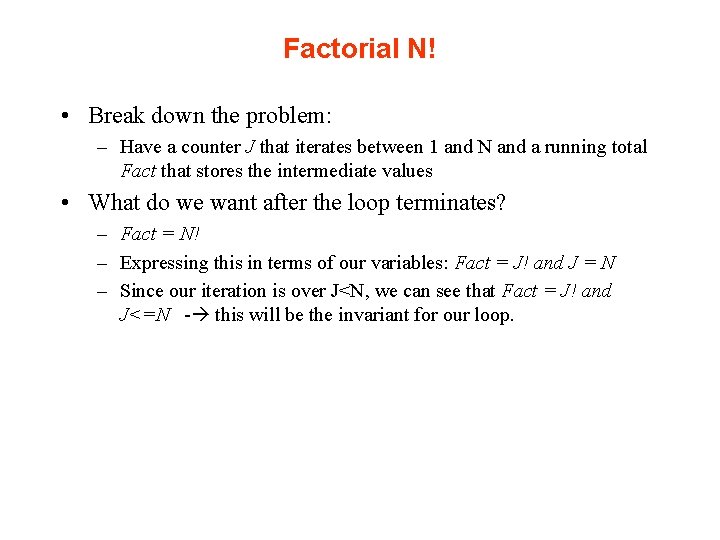
Factorial N! • Break down the problem: – Have a counter J that iterates between 1 and N and a running total Fact that stores the intermediate values • What do we want after the loop terminates? – Fact = N! – Expressing this in terms of our variables: Fact = J! and J = N – Since our iteration is over J<N, we can see that Fact = J! and J<=N - this will be the invariant for our loop.
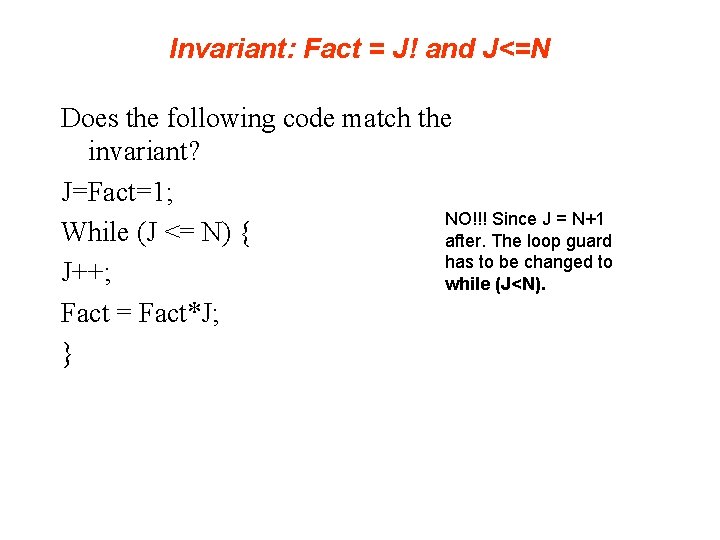
Invariant: Fact = J! and J<=N Does the following code match the invariant? J=Fact=1; NO!!! Since J = N+1 While (J <= N) { after. The loop guard has to be changed to J++; while (J<N). Fact = Fact*J; }
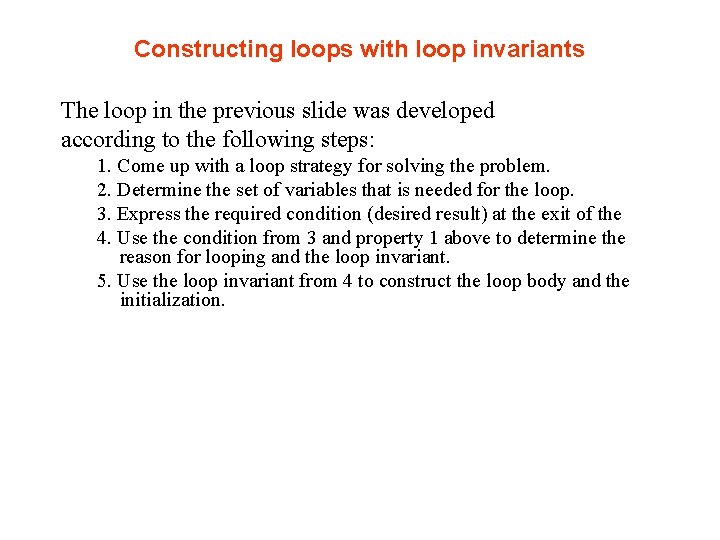
Constructing loops with loop invariants The loop in the previous slide was developed according to the following steps: 1. Come up with a loop strategy for solving the problem. 2. Determine the set of variables that is needed for the loop. 3. Express the required condition (desired result) at the exit of the 4. Use the condition from 3 and property 1 above to determine the reason for looping and the loop invariant. 5. Use the loop invariant from 4 to construct the loop body and the initialization.
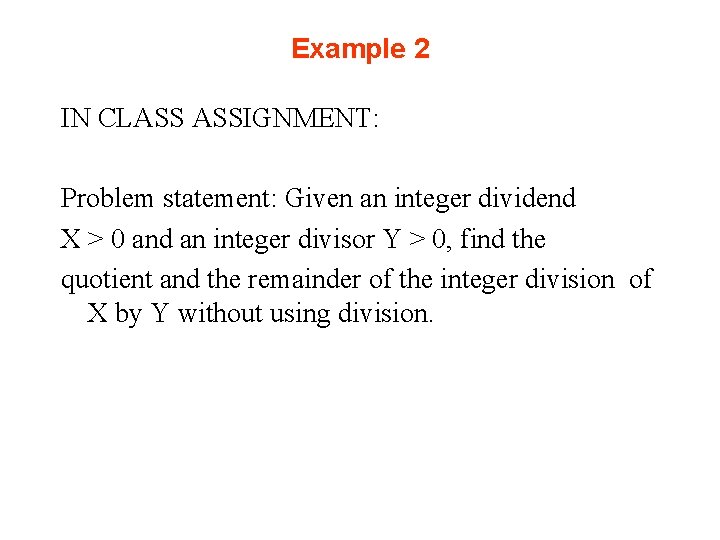
Example 2 IN CLASS ASSIGNMENT: Problem statement: Given an integer dividend X > 0 and an integer divisor Y > 0, find the quotient and the remainder of the integer division of X by Y without using division.
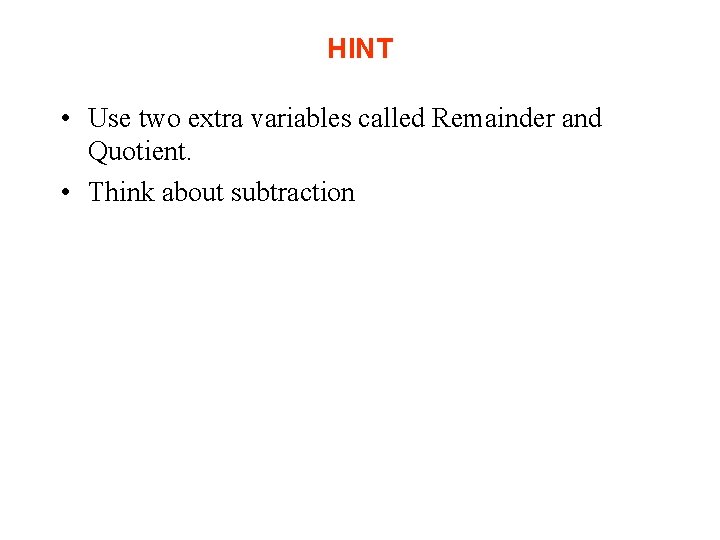
HINT • Use two extra variables called Remainder and Quotient. • Think about subtraction
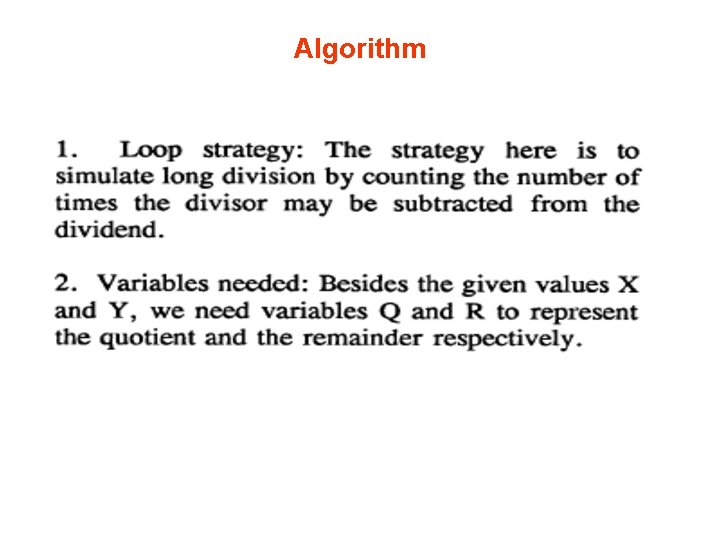
Algorithm
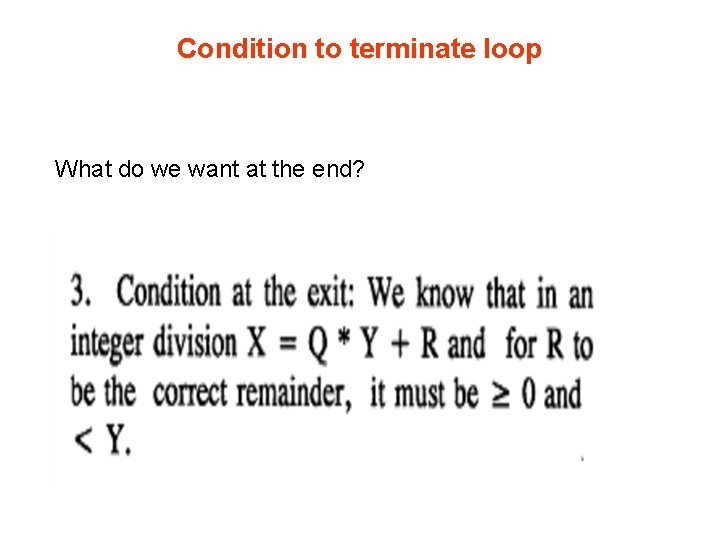
Condition to terminate loop What do we want at the end?
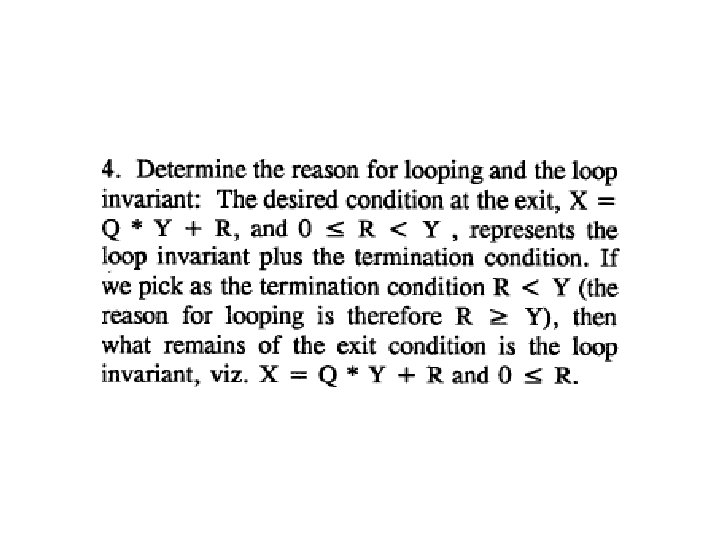
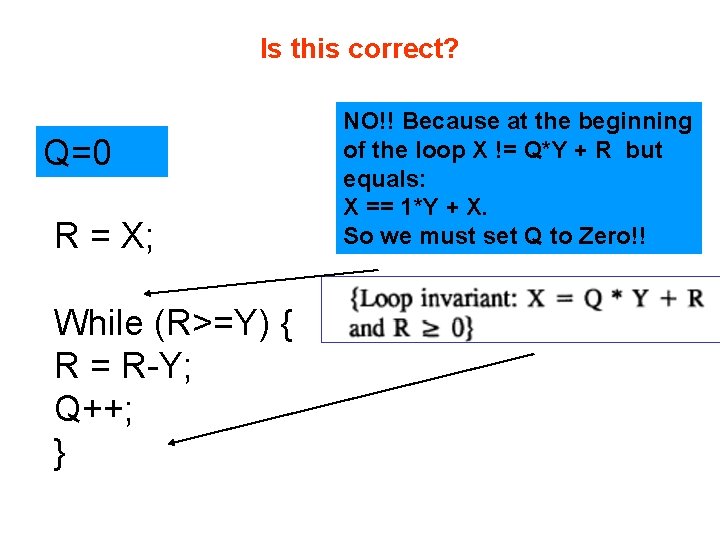
Is this correct? Q=1; Q=0 R = X; While (R>=Y) { R = R-Y; Q++; } NO!! Because at the beginning of the loop X != Q*Y + R but equals: X == 1*Y + X. So we must set Q to Zero!!