Looping and Repetition SE1010 Dr Mark L Hornick
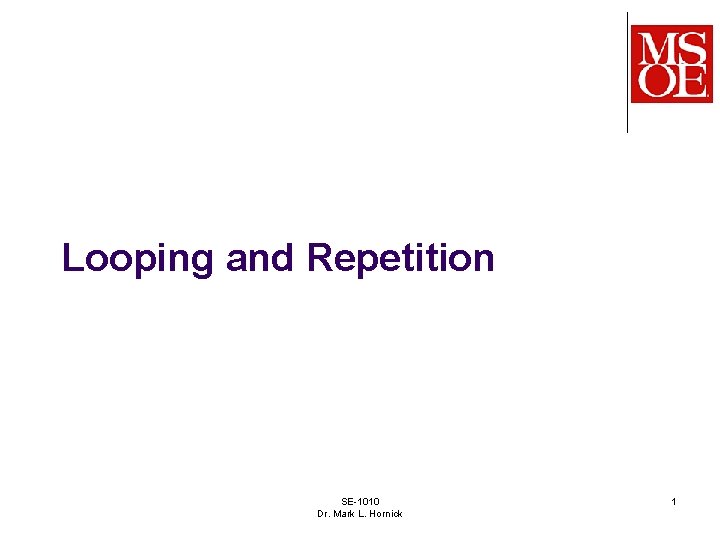
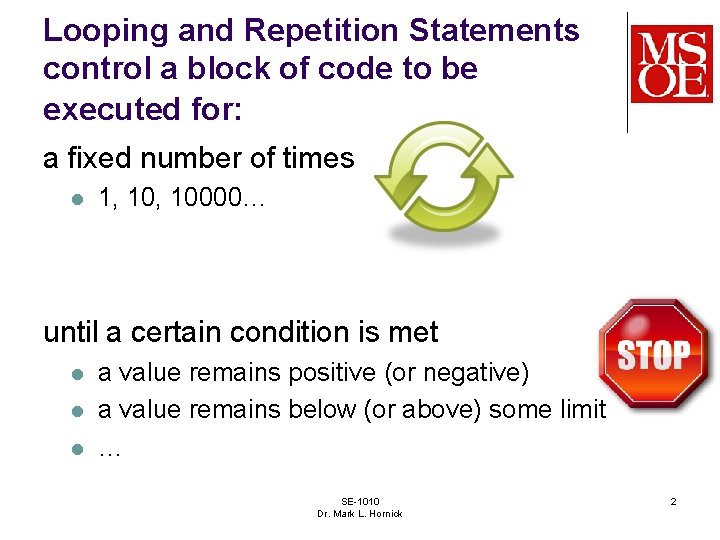
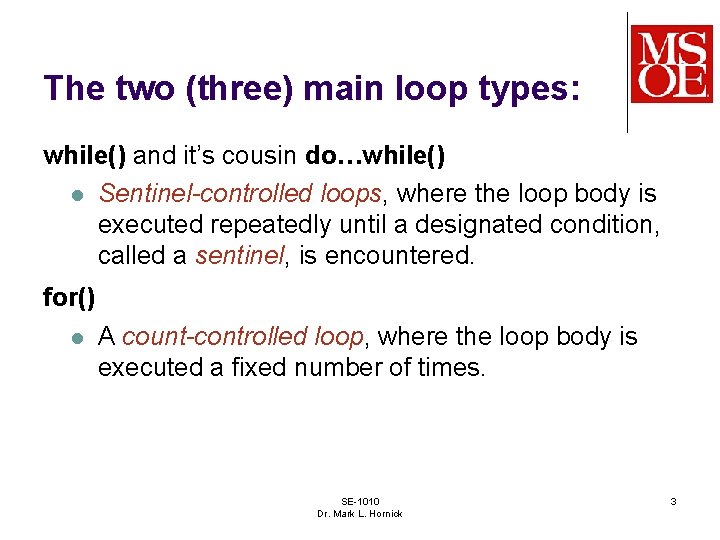
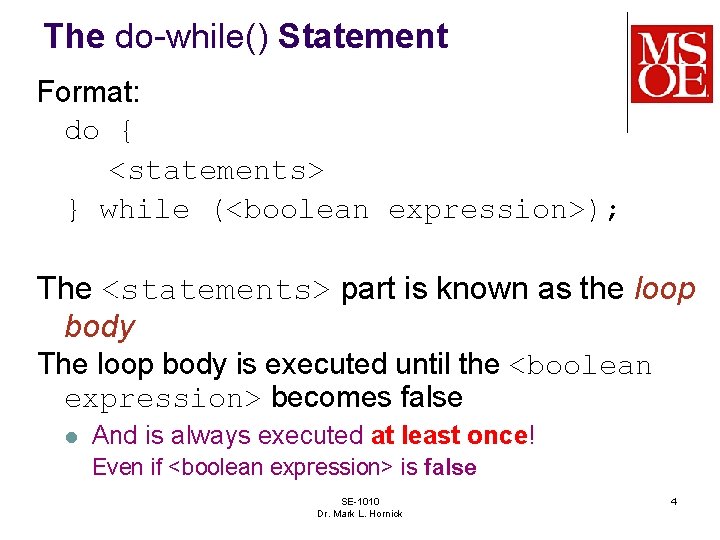
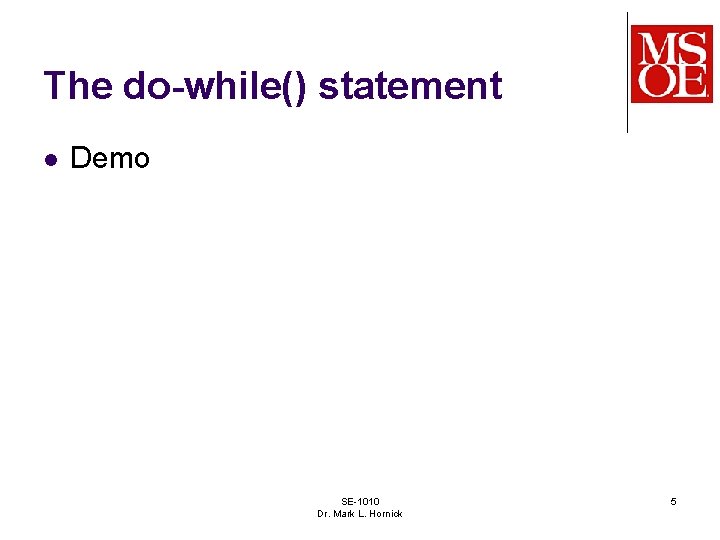
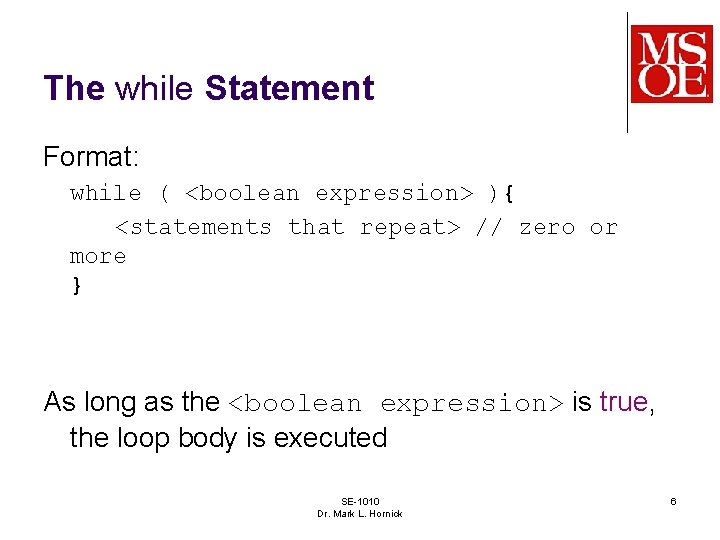
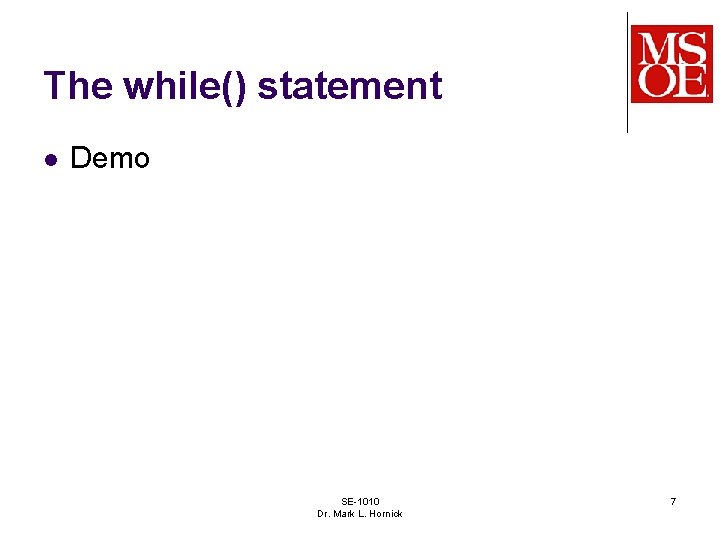
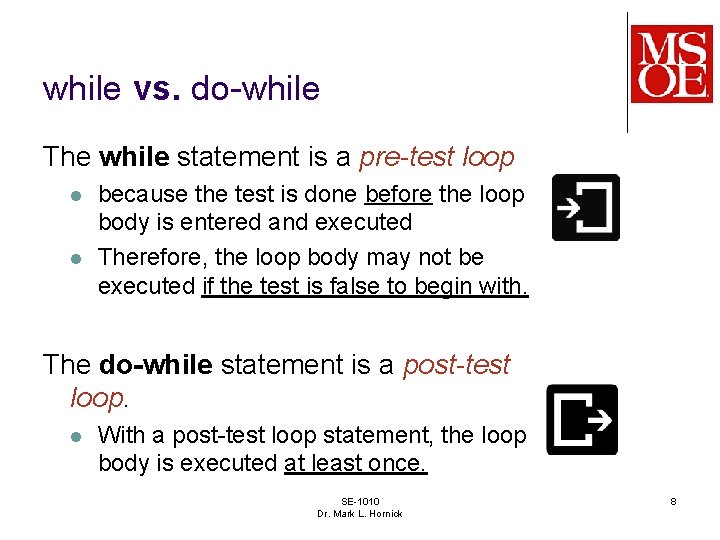
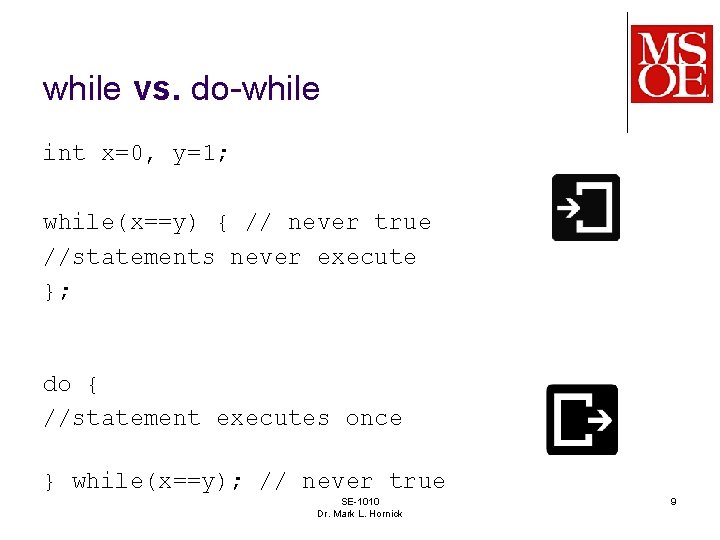
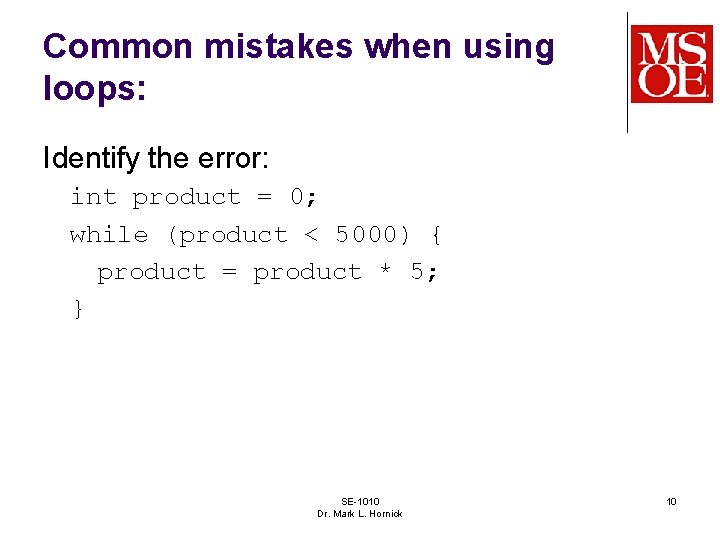
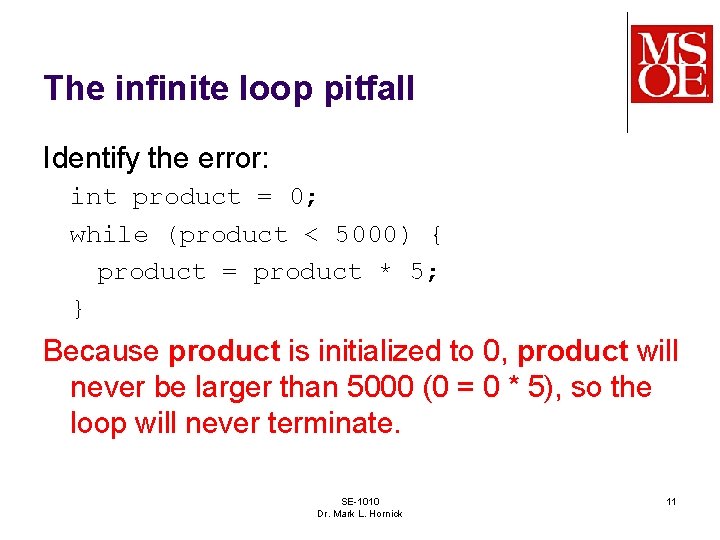
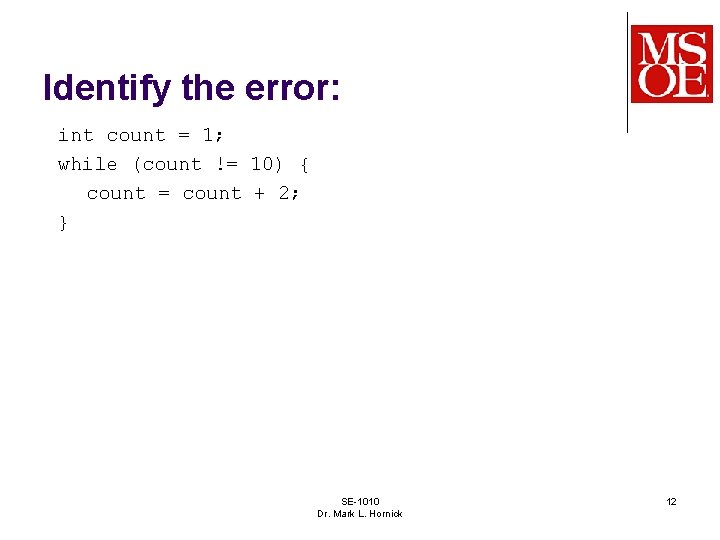
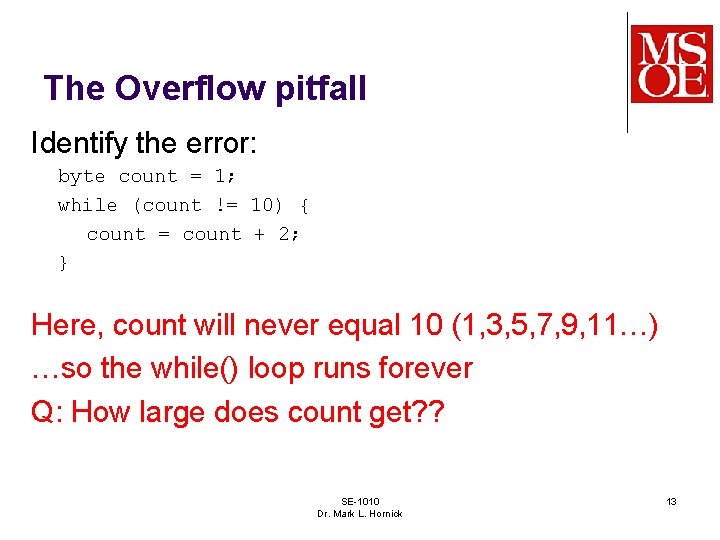
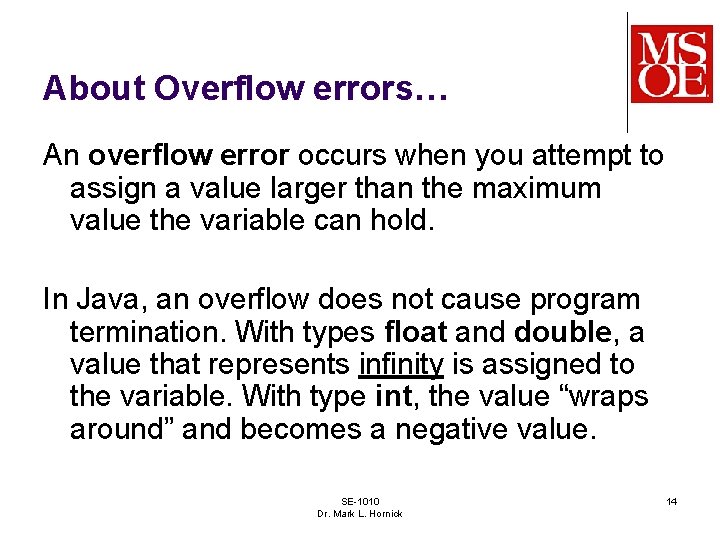
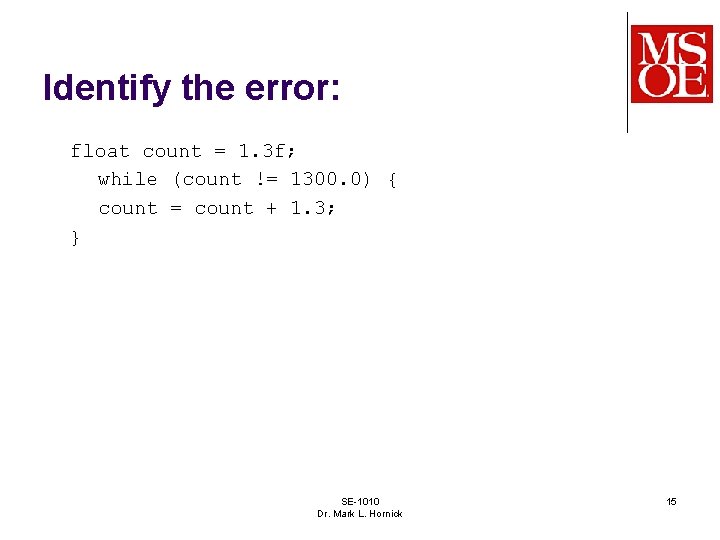
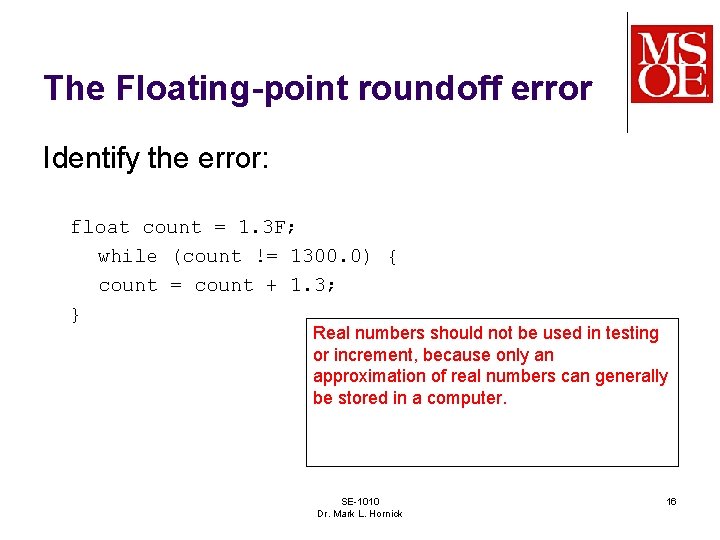
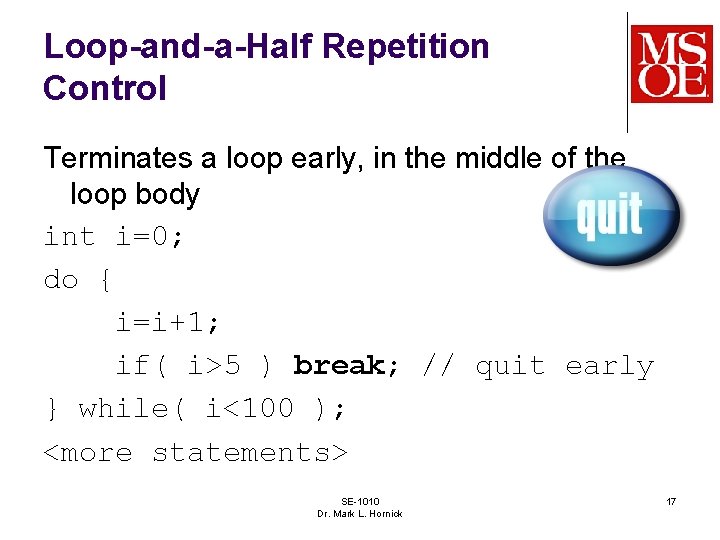
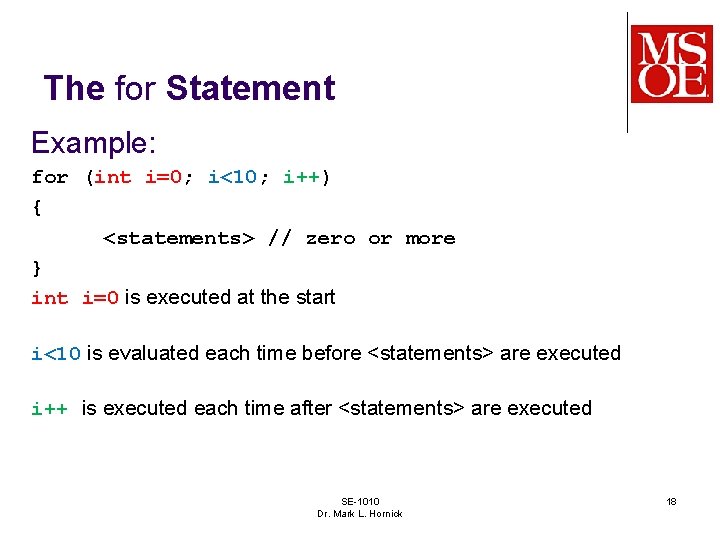
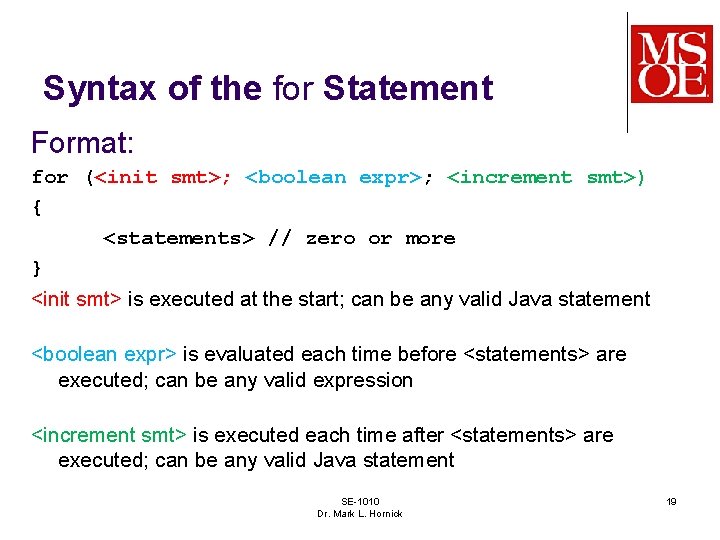
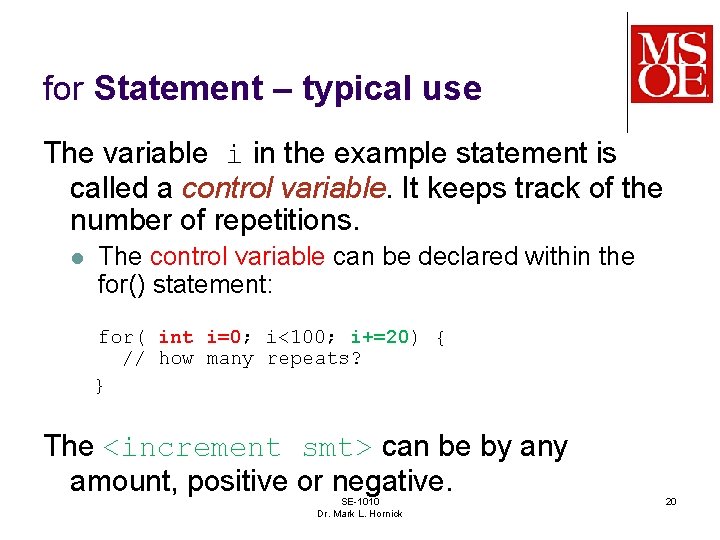
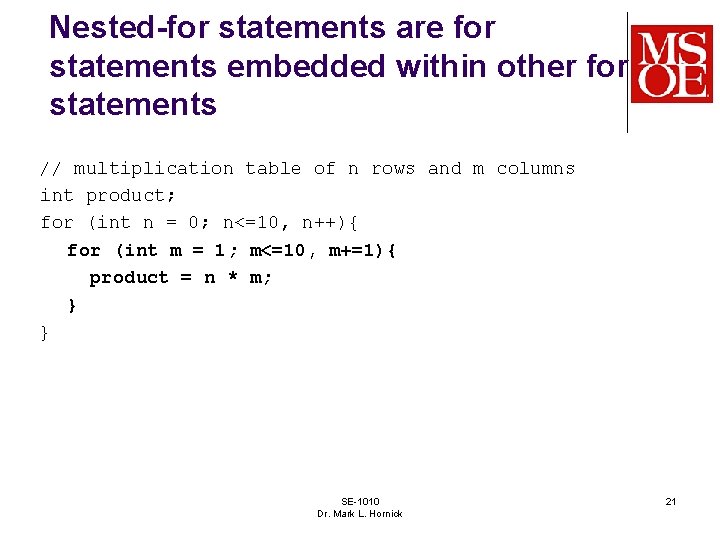
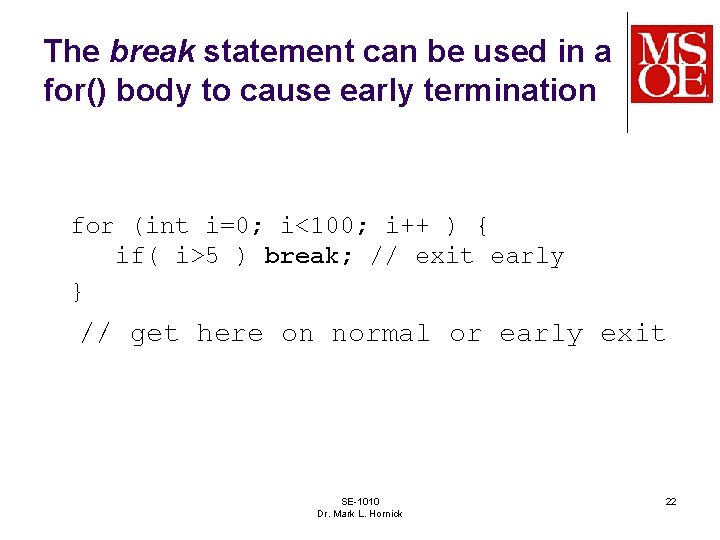
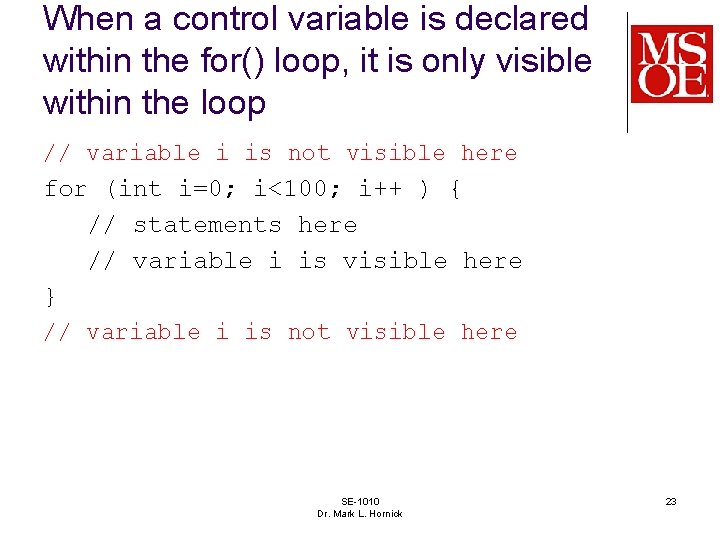
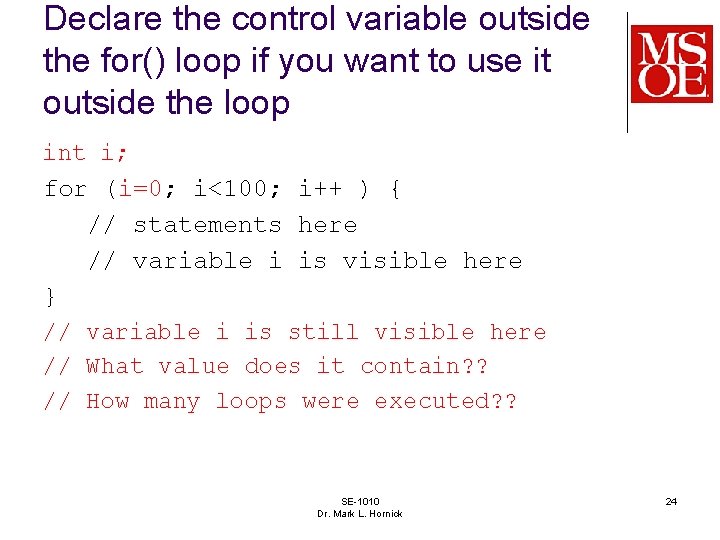
- Slides: 24
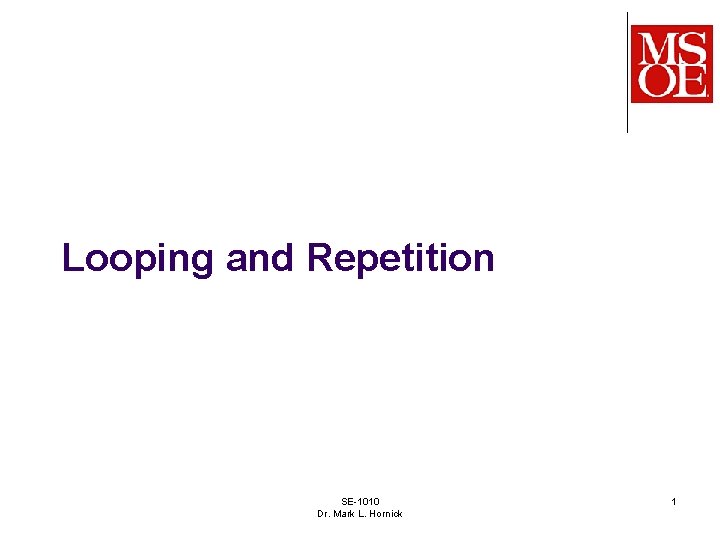
Looping and Repetition SE-1010 Dr. Mark L. Hornick 1
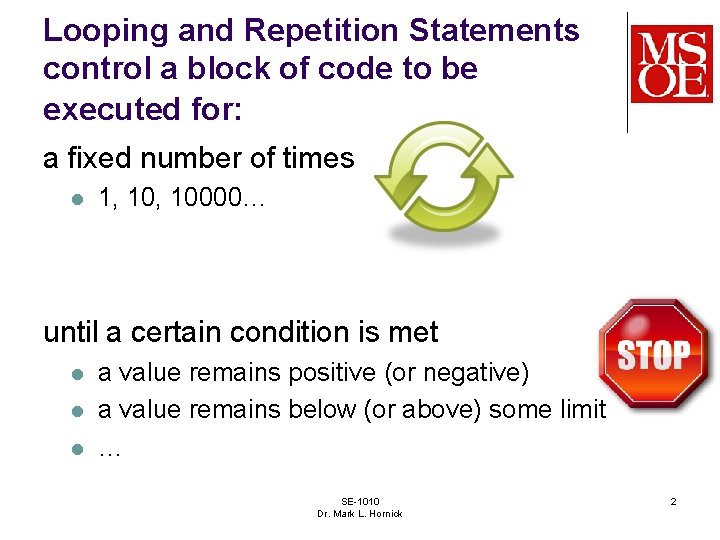
Looping and Repetition Statements control a block of code to be executed for: a fixed number of times l 1, 10000… until a certain condition is met l l l a value remains positive (or negative) a value remains below (or above) some limit … SE-1010 Dr. Mark L. Hornick 2
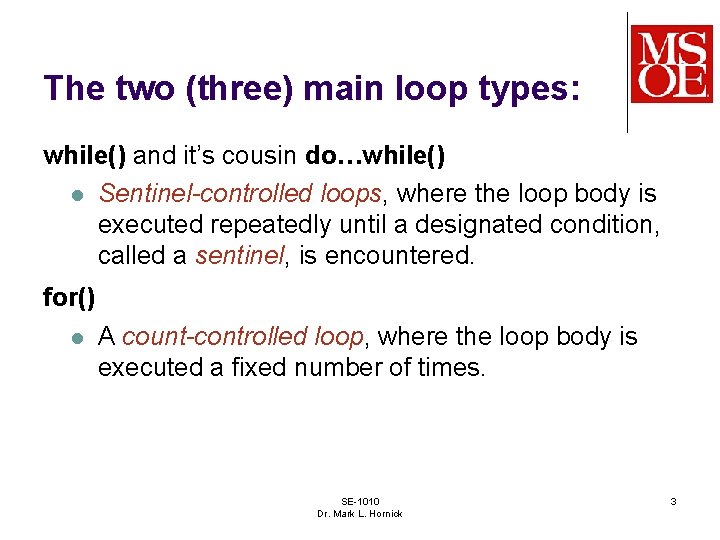
The two (three) main loop types: while() and it’s cousin do…while() l Sentinel-controlled loops, where the loop body is executed repeatedly until a designated condition, called a sentinel, is encountered. for() l A count-controlled loop, where the loop body is executed a fixed number of times. SE-1010 Dr. Mark L. Hornick 3
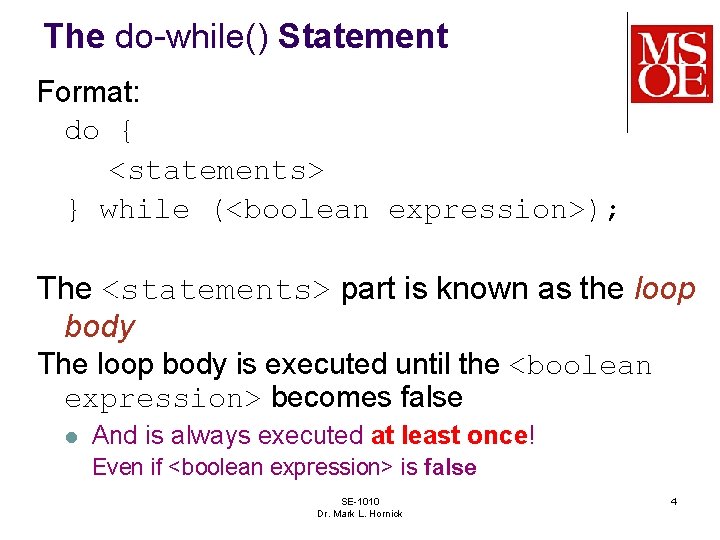
The do-while() Statement Format: do { <statements> } while (<boolean expression>); The <statements> part is known as the loop body The loop body is executed until the <boolean expression> becomes false l And is always executed at least once! Even if <boolean expression> is false SE-1010 Dr. Mark L. Hornick 4
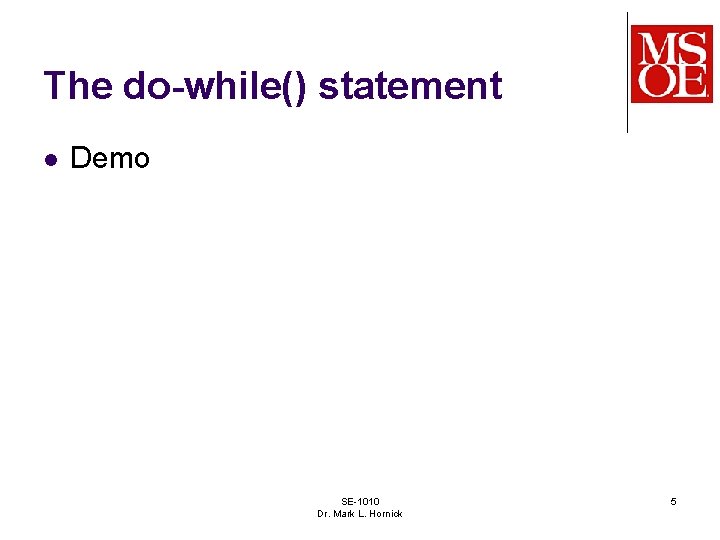
The do-while() statement l Demo SE-1010 Dr. Mark L. Hornick 5
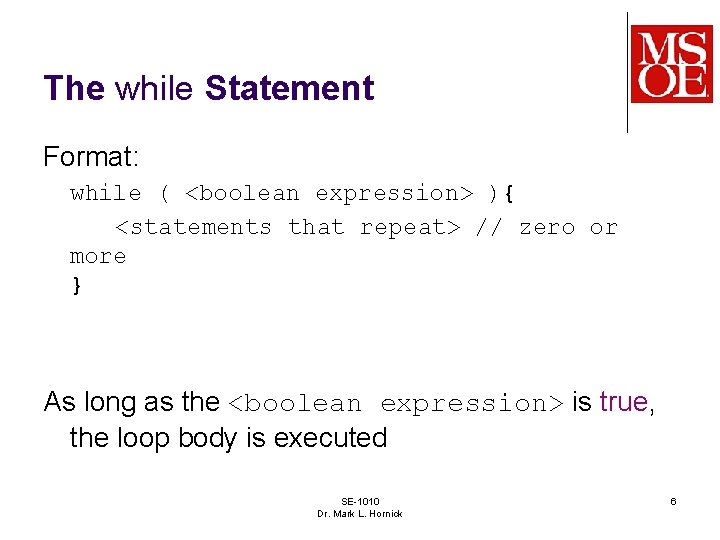
The while Statement Format: while ( <boolean expression> ){ <statements that repeat> // zero or more } As long as the <boolean expression> is true, the loop body is executed SE-1010 Dr. Mark L. Hornick 6
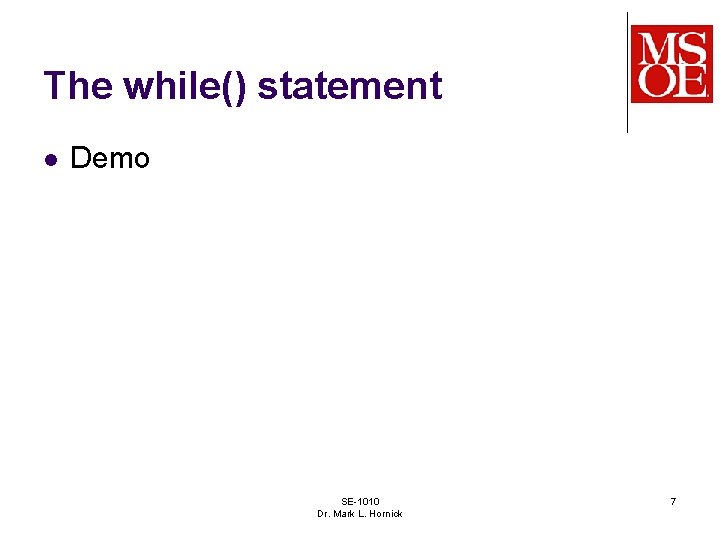
The while() statement l Demo SE-1010 Dr. Mark L. Hornick 7
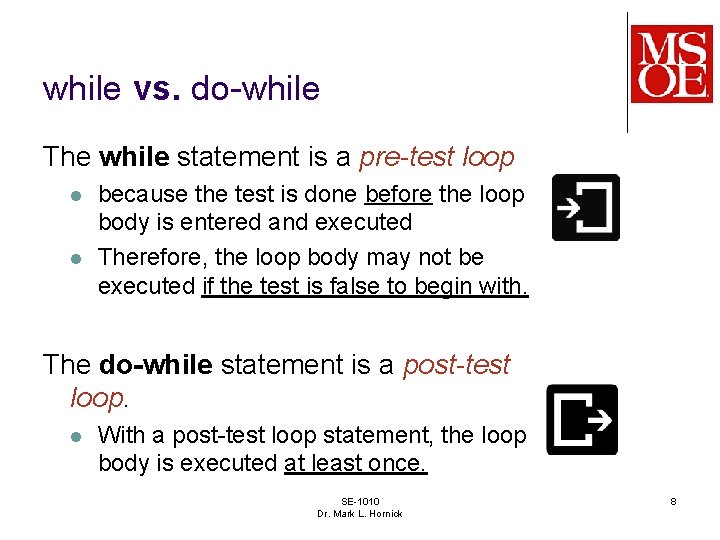
while vs. do-while The while statement is a pre-test loop l l because the test is done before the loop body is entered and executed Therefore, the loop body may not be executed if the test is false to begin with. The do-while statement is a post-test loop. l With a post-test loop statement, the loop body is executed at least once. SE-1010 Dr. Mark L. Hornick 8
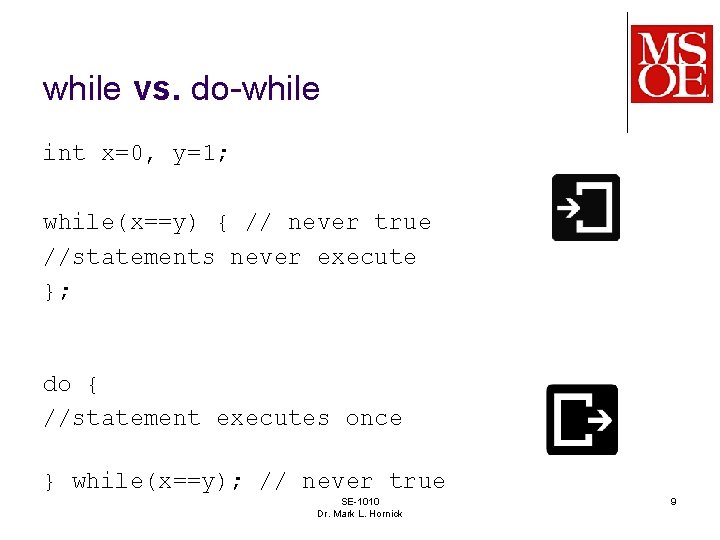
while vs. do-while int x=0, y=1; while(x==y) { // never true //statements never execute }; do { //statement executes once } while(x==y); // never true SE-1010 Dr. Mark L. Hornick 9
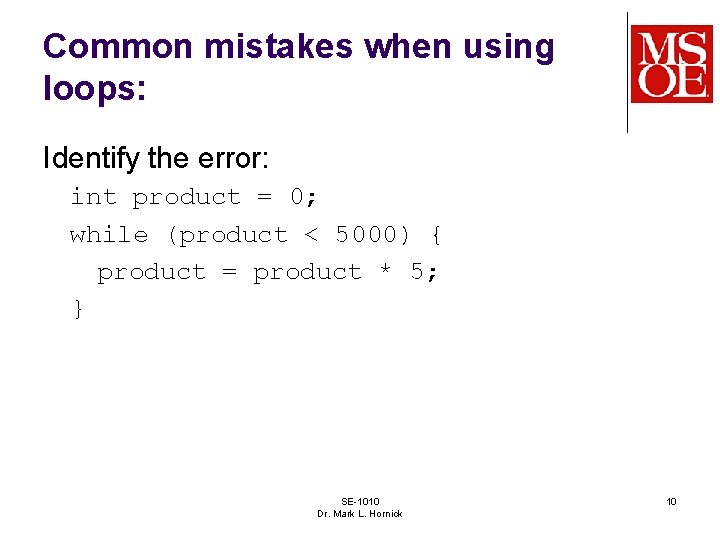
Common mistakes when using loops: Identify the error: int product = 0; while (product < 5000) { product = product * 5; } SE-1010 Dr. Mark L. Hornick 10
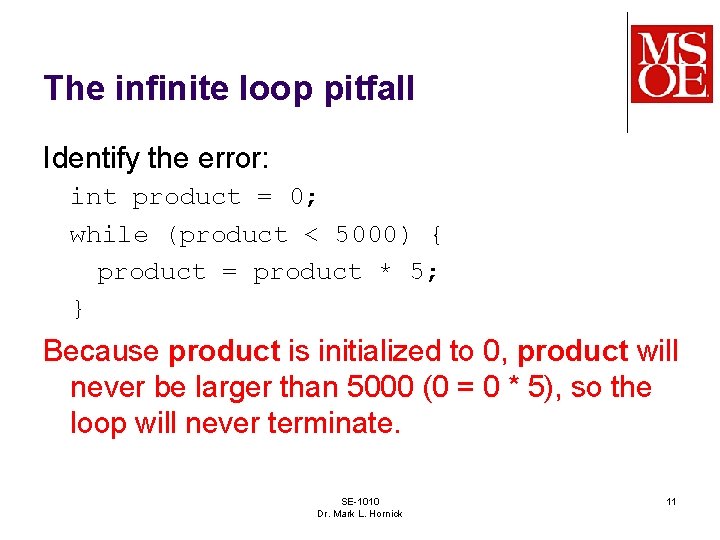
The infinite loop pitfall Identify the error: int product = 0; while (product < 5000) { product = product * 5; } Because product is initialized to 0, product will never be larger than 5000 (0 = 0 * 5), so the loop will never terminate. SE-1010 Dr. Mark L. Hornick 11
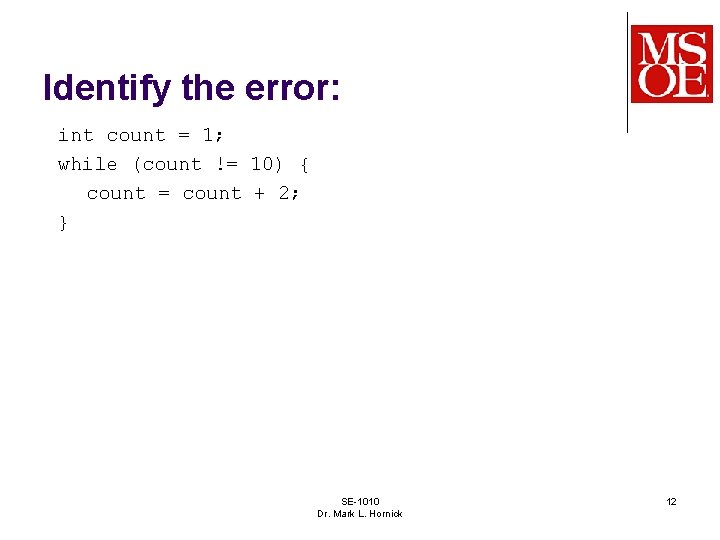
Identify the error: int count = 1; while (count != 10) { count = count + 2; } SE-1010 Dr. Mark L. Hornick 12
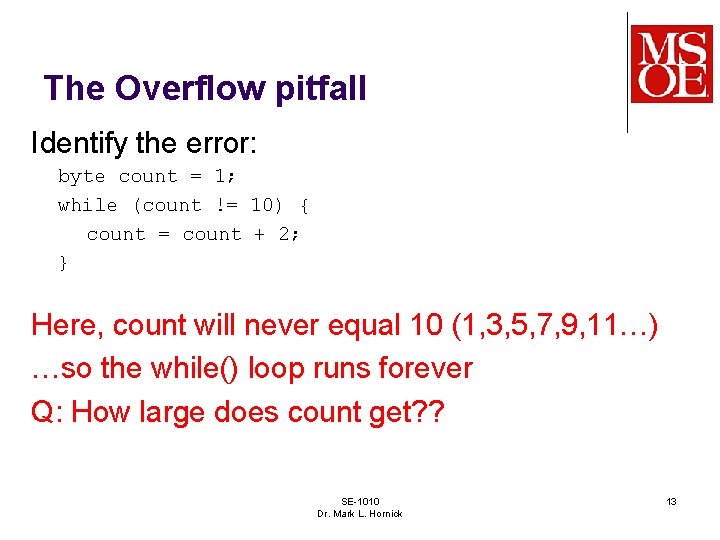
The Overflow pitfall Identify the error: byte count = 1; while (count != 10) { count = count + 2; } Here, count will never equal 10 (1, 3, 5, 7, 9, 11…) …so the while() loop runs forever Q: How large does count get? ? SE-1010 Dr. Mark L. Hornick 13
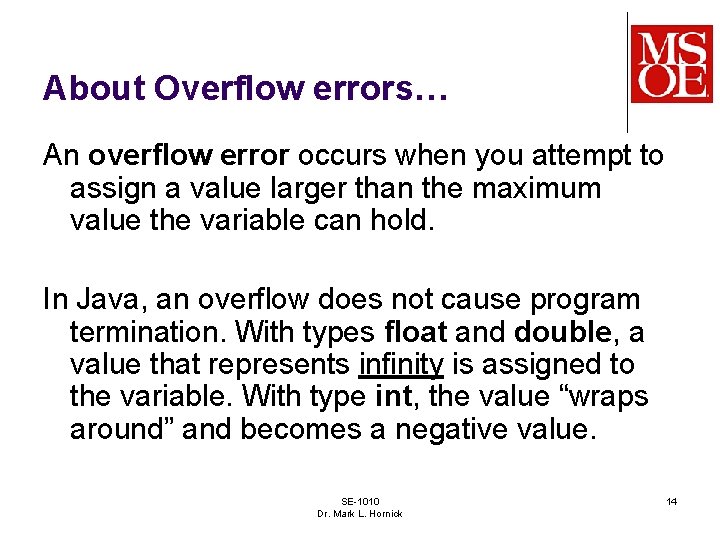
About Overflow errors… An overflow error occurs when you attempt to assign a value larger than the maximum value the variable can hold. In Java, an overflow does not cause program termination. With types float and double, a value that represents infinity is assigned to the variable. With type int, the value “wraps around” and becomes a negative value. SE-1010 Dr. Mark L. Hornick 14
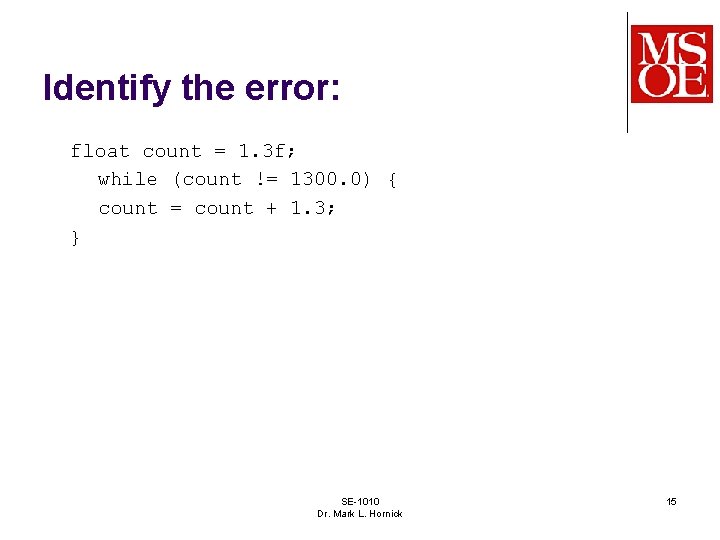
Identify the error: float count = 1. 3 f; while (count != 1300. 0) { count = count + 1. 3; } SE-1010 Dr. Mark L. Hornick 15
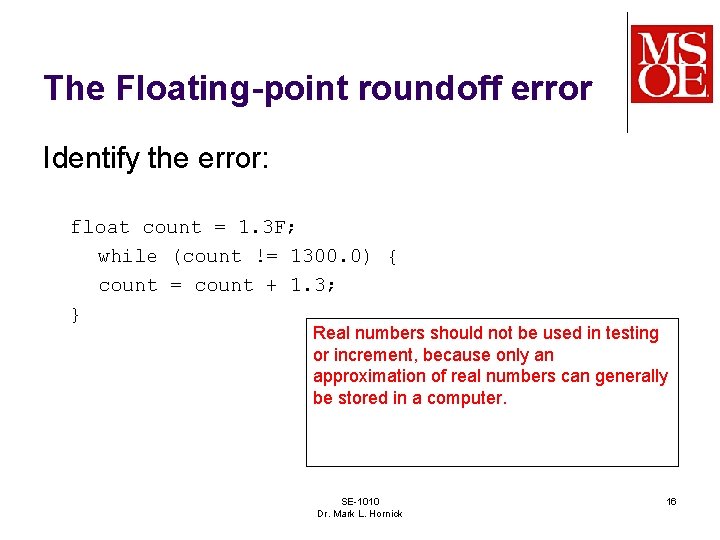
The Floating-point roundoff error Identify the error: float count = 1. 3 F; while (count != 1300. 0) { count = count + 1. 3; } Real numbers should not be used in testing or increment, because only an approximation of real numbers can generally be stored in a computer. SE-1010 Dr. Mark L. Hornick 16
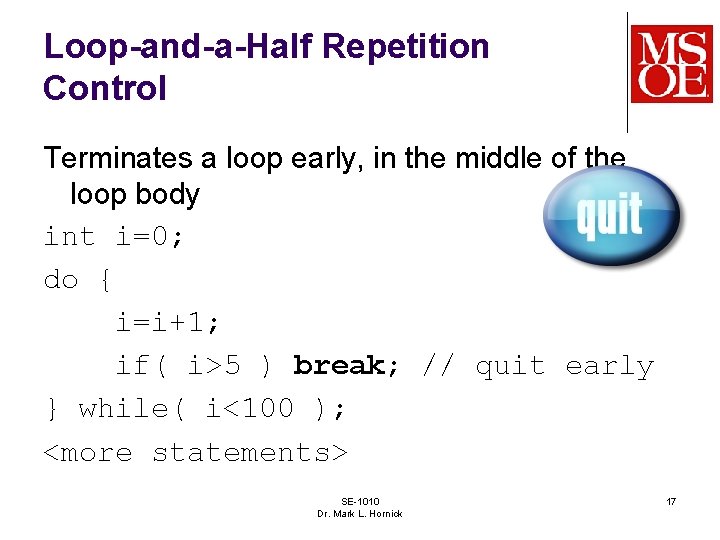
Loop-and-a-Half Repetition Control Terminates a loop early, in the middle of the loop body int i=0; do { i=i+1; if( i>5 ) break; // quit early } while( i<100 ); <more statements> SE-1010 Dr. Mark L. Hornick 17
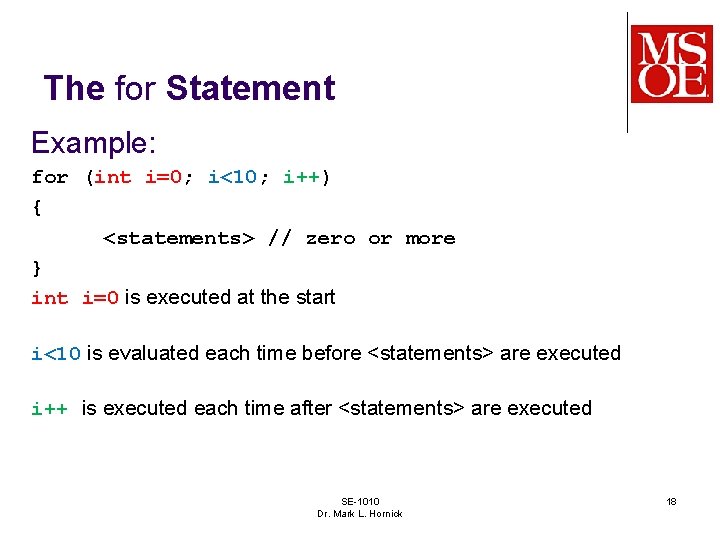
The for Statement Example: for (int i=0; i<10; i++) { <statements> // zero or more } int i=0 is executed at the start i<10 is evaluated each time before <statements> are executed i++ is executed each time after <statements> are executed SE-1010 Dr. Mark L. Hornick 18
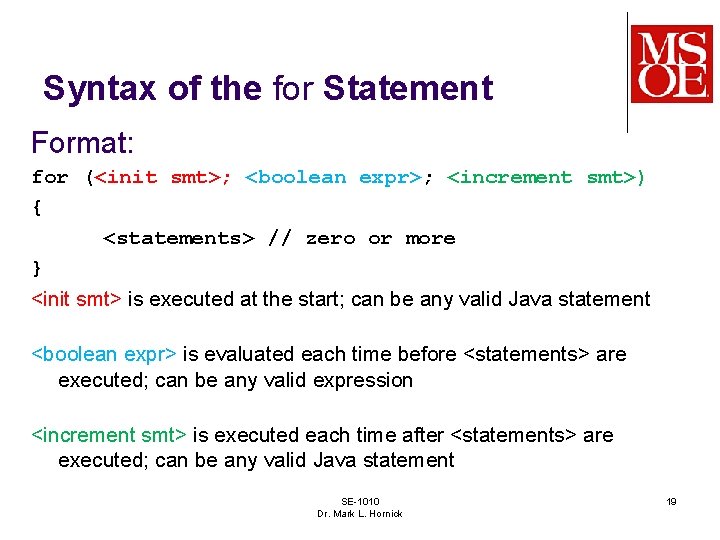
Syntax of the for Statement Format: for (<init smt>; <boolean expr>; <increment smt>) { <statements> // zero or more } <init smt> is executed at the start; can be any valid Java statement <boolean expr> is evaluated each time before <statements> are executed; can be any valid expression <increment smt> is executed each time after <statements> are executed; can be any valid Java statement SE-1010 Dr. Mark L. Hornick 19
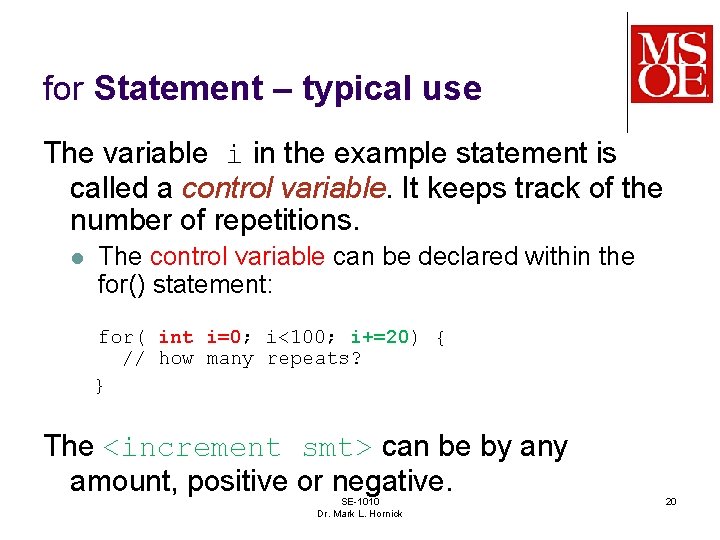
for Statement – typical use The variable i in the example statement is called a control variable. It keeps track of the number of repetitions. l The control variable can be declared within the for() statement: for( int i=0; i<100; i+=20) { // how many repeats? } The <increment smt> can be by any amount, positive or negative. SE-1010 Dr. Mark L. Hornick 20
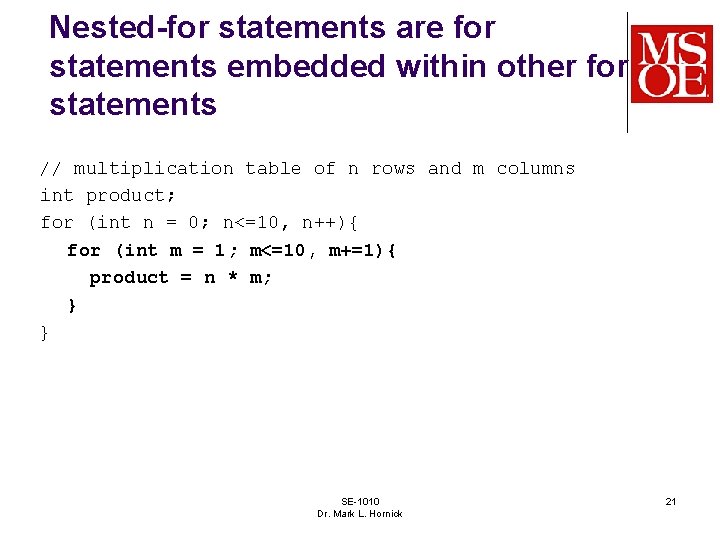
Nested-for statements are for statements embedded within other for statements // multiplication table of n rows and m columns int product; for (int n = 0; n<=10, n++){ for (int m = 1; m<=10, m+=1){ product = n * m; } } SE-1010 Dr. Mark L. Hornick 21
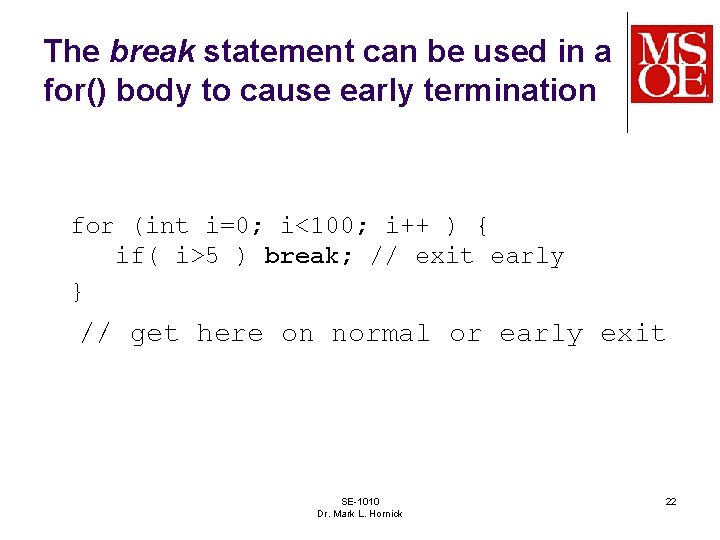
The break statement can be used in a for() body to cause early termination for (int i=0; i<100; i++ ) { if( i>5 ) break; // exit early } // get here on normal or early exit SE-1010 Dr. Mark L. Hornick 22
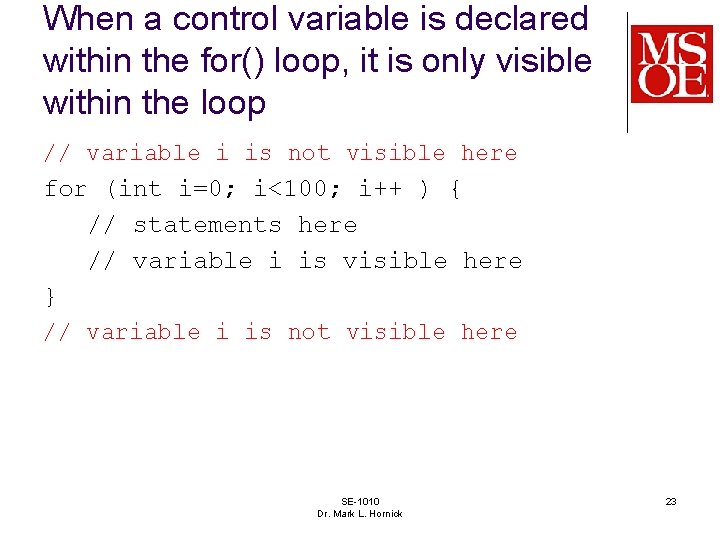
When a control variable is declared within the for() loop, it is only visible within the loop // variable i is not visible here for (int i=0; i<100; i++ ) { // statements here // variable i is visible here } // variable i is not visible here SE-1010 Dr. Mark L. Hornick 23
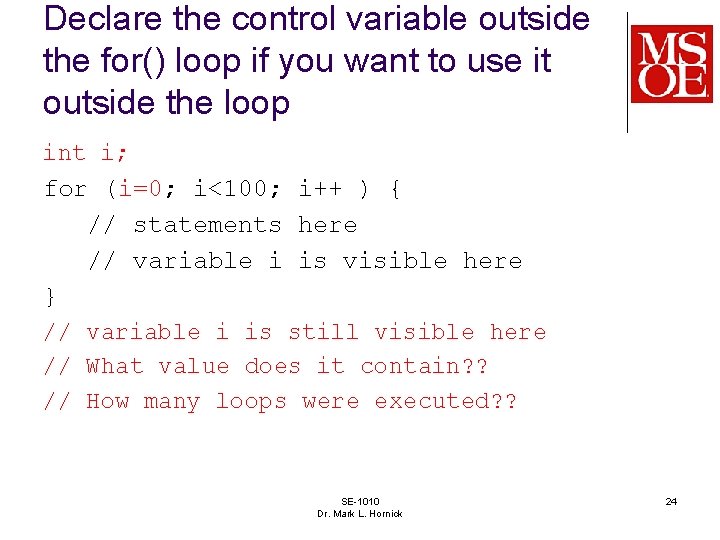
Declare the control variable outside the for() loop if you want to use it outside the loop int i; for (i=0; i<100; i++ ) { // statements here // variable i is visible here } // variable i is still visible here // What value does it contain? ? // How many loops were executed? ? SE-1010 Dr. Mark L. Hornick 24