Logging What is logging n Logging is producing
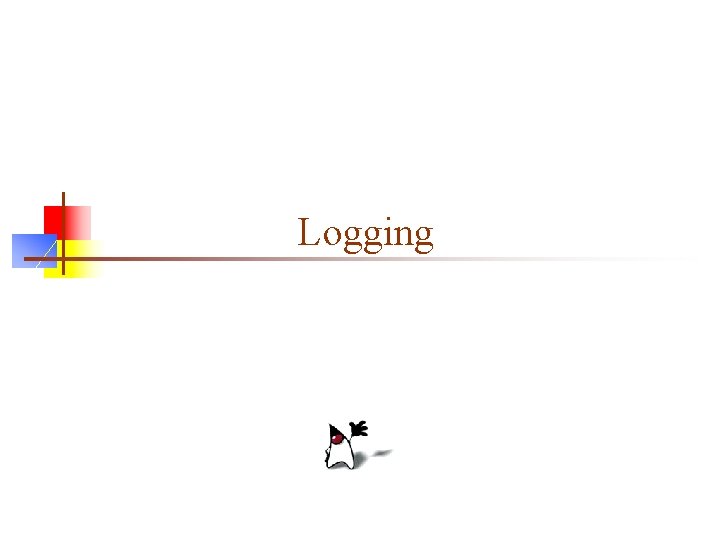
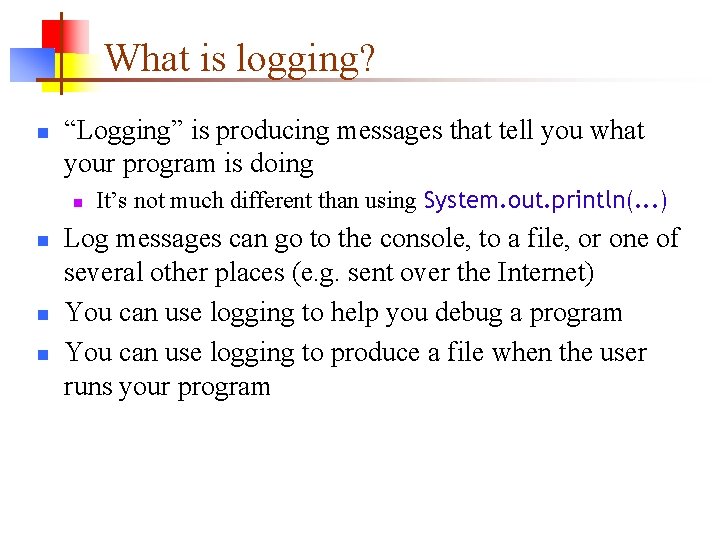
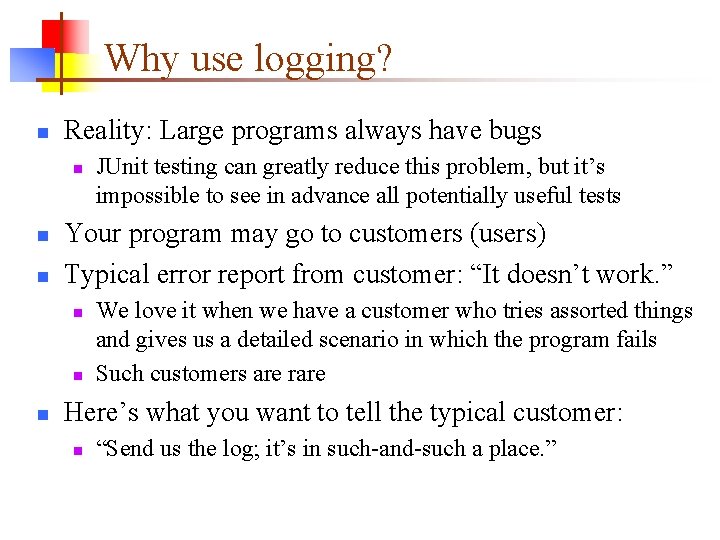
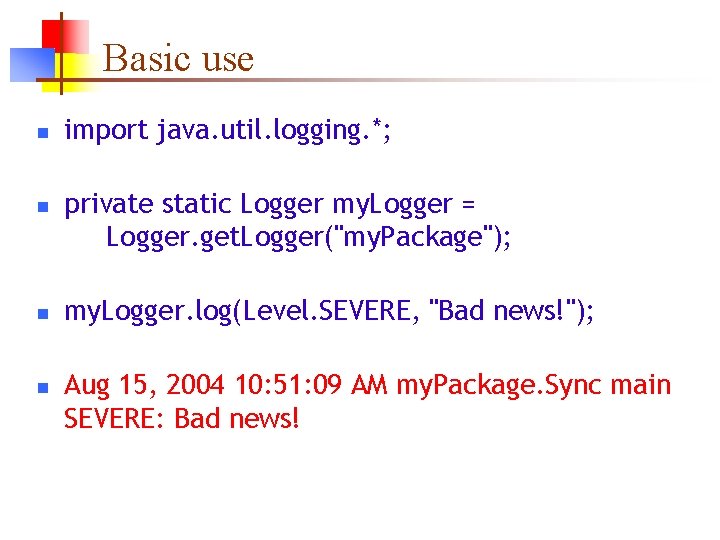
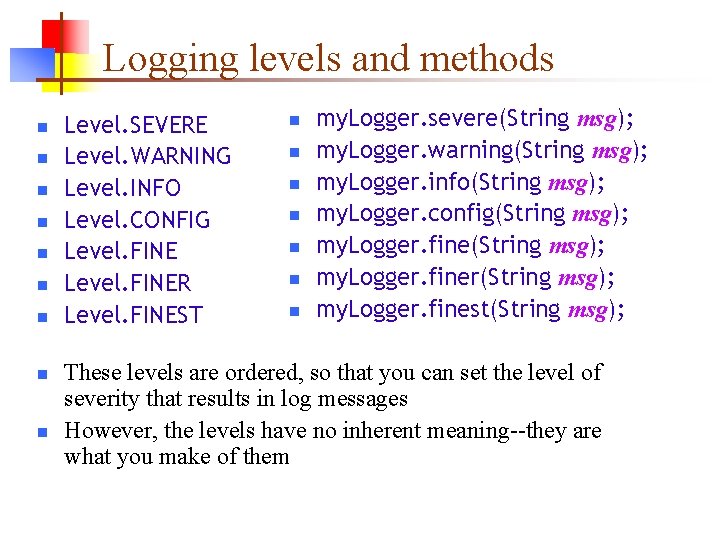
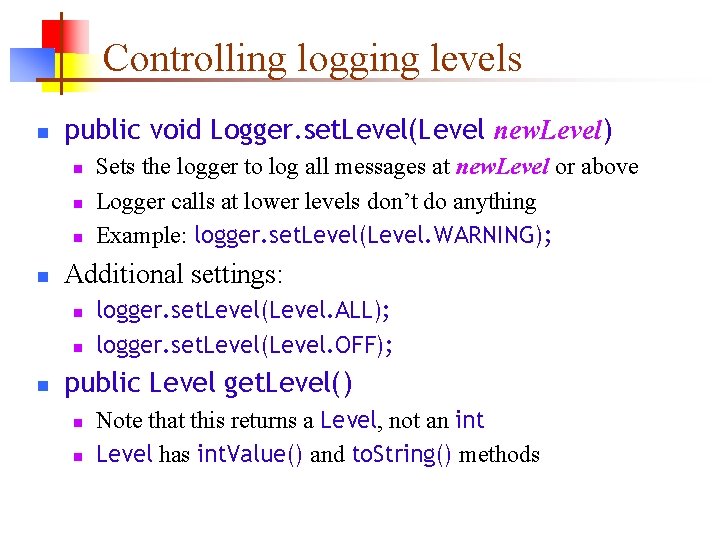
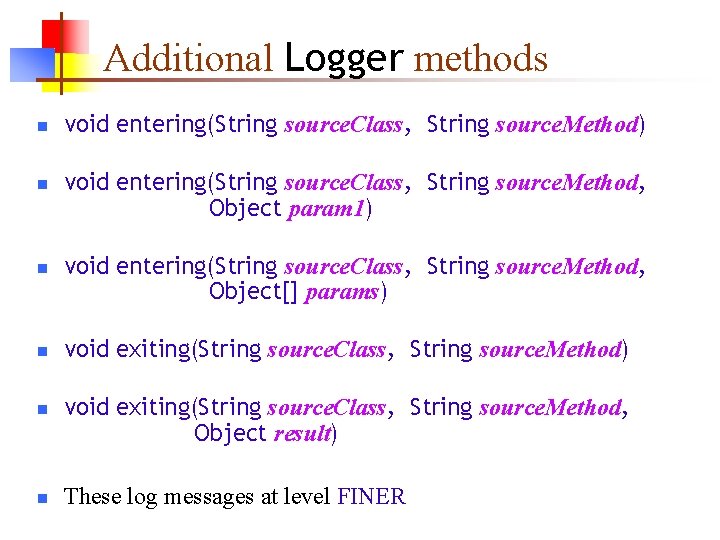
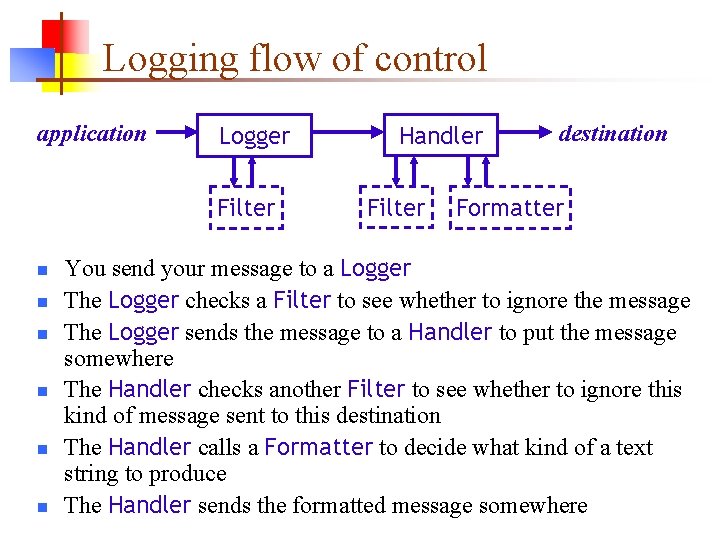
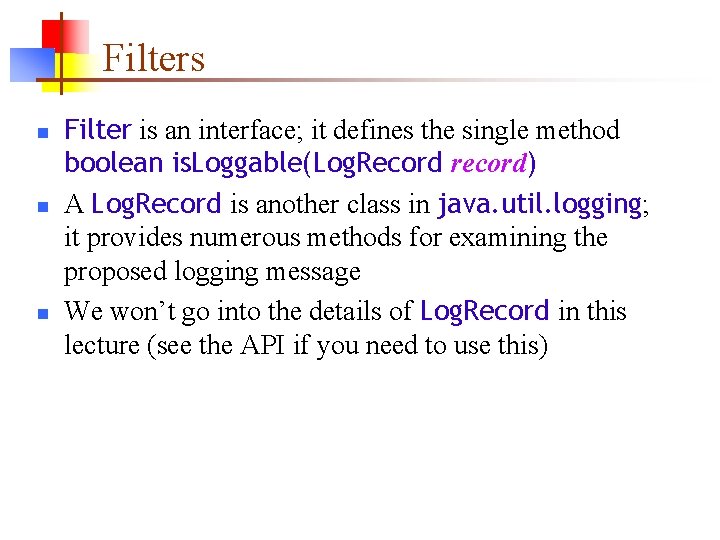
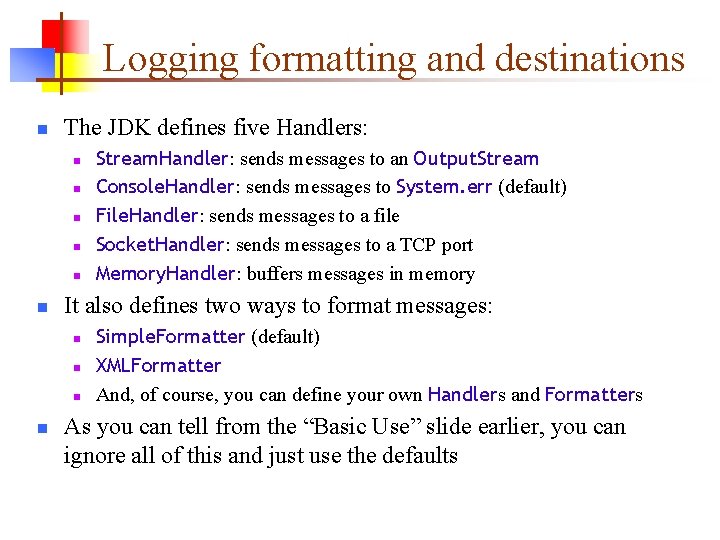
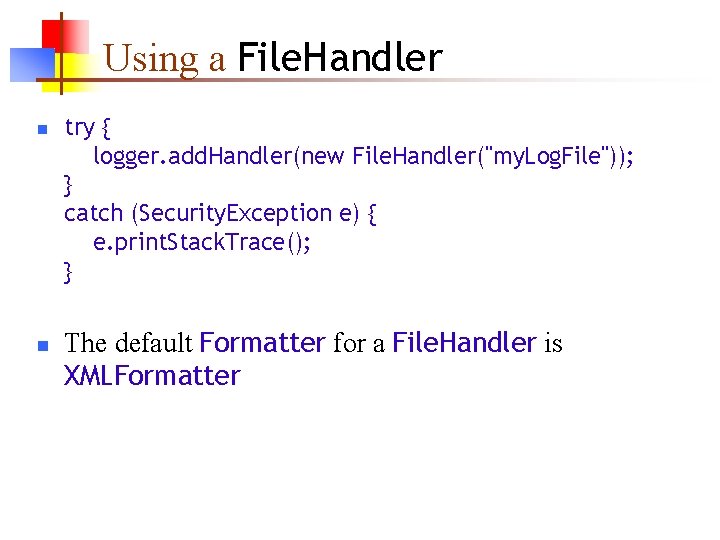
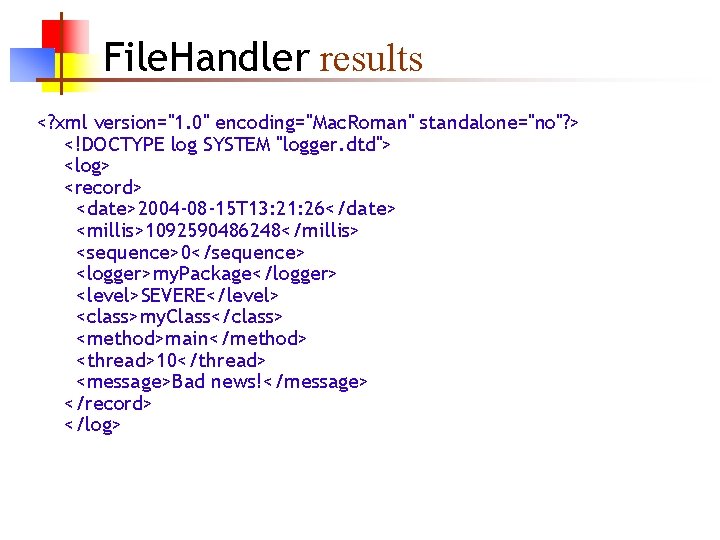
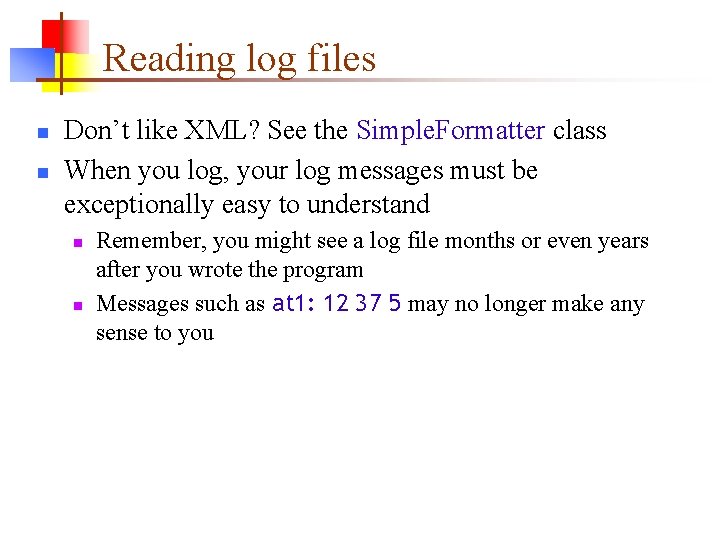
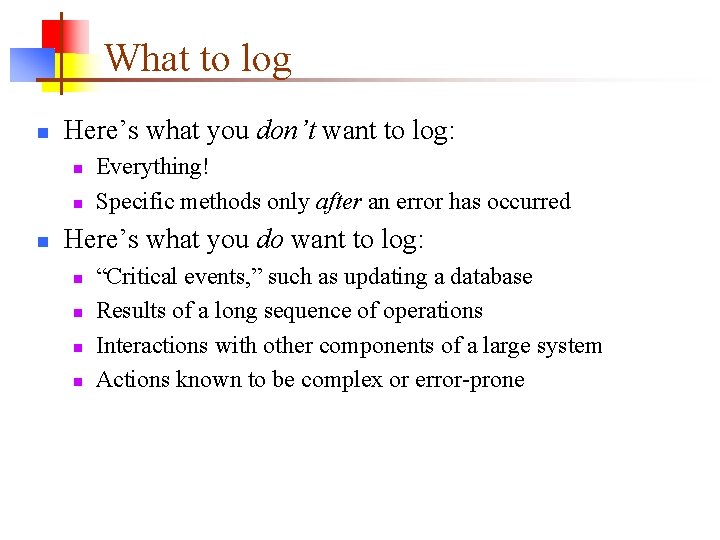
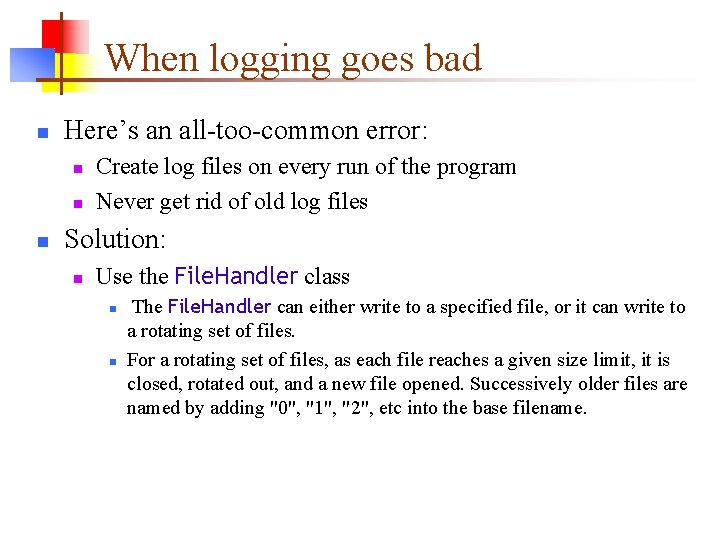
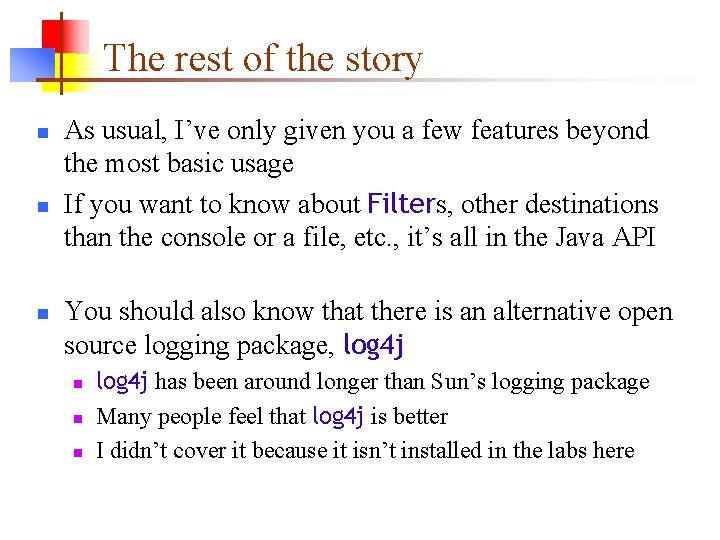
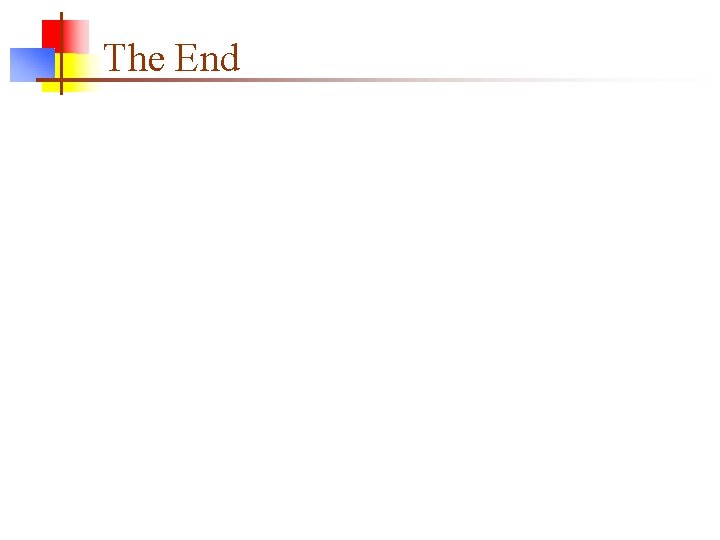
- Slides: 17
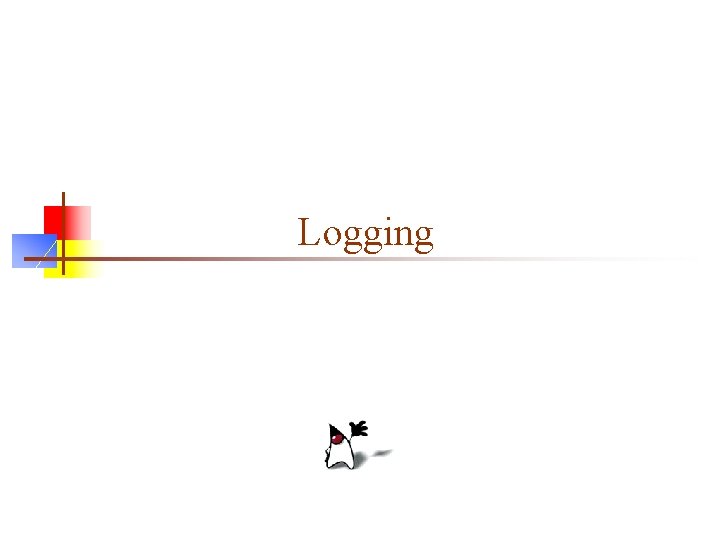
Logging
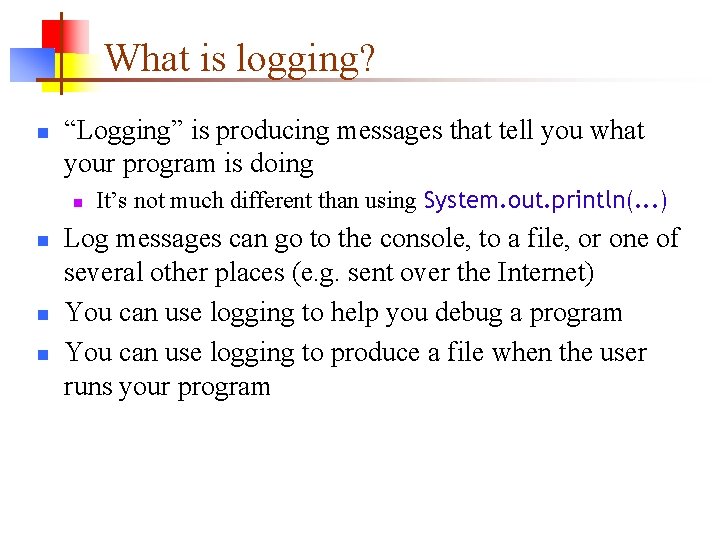
What is logging? n “Logging” is producing messages that tell you what your program is doing n n It’s not much different than using System. out. println(. . . ) Log messages can go to the console, to a file, or one of several other places (e. g. sent over the Internet) You can use logging to help you debug a program You can use logging to produce a file when the user runs your program
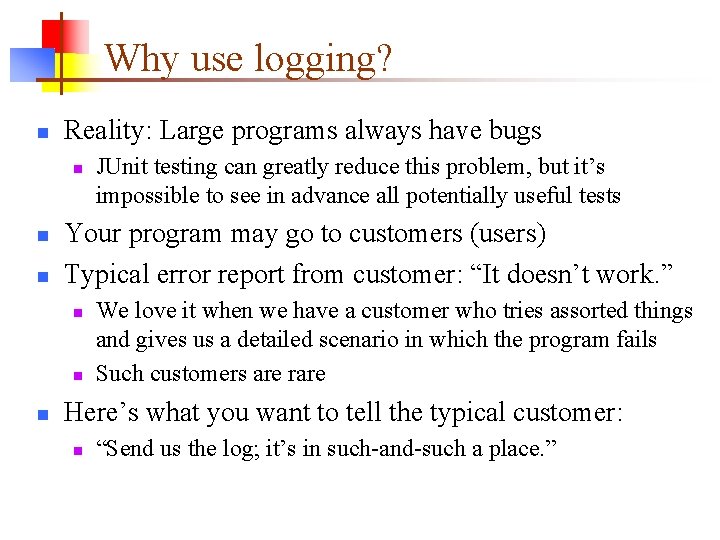
Why use logging? n Reality: Large programs always have bugs n n n Your program may go to customers (users) Typical error report from customer: “It doesn’t work. ” n n n JUnit testing can greatly reduce this problem, but it’s impossible to see in advance all potentially useful tests We love it when we have a customer who tries assorted things and gives us a detailed scenario in which the program fails Such customers are rare Here’s what you want to tell the typical customer: n “Send us the log; it’s in such-and-such a place. ”
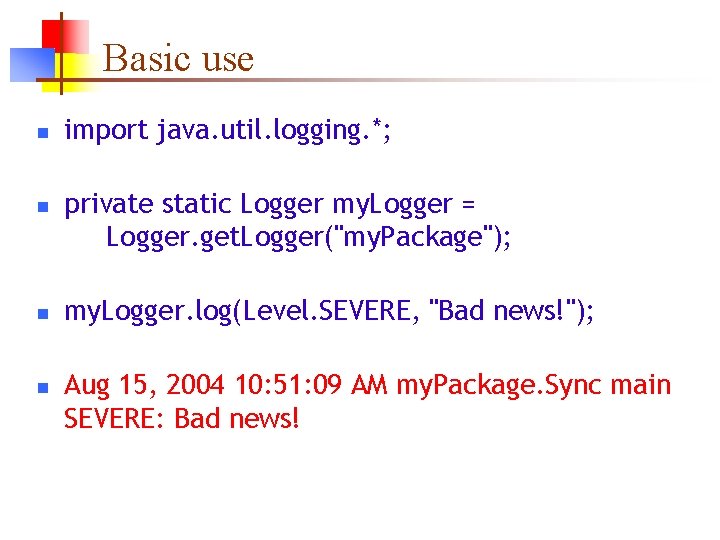
Basic use n n import java. util. logging. *; private static Logger my. Logger = Logger. get. Logger("my. Package"); my. Logger. log(Level. SEVERE, "Bad news!"); Aug 15, 2004 10: 51: 09 AM my. Package. Sync main SEVERE: Bad news!
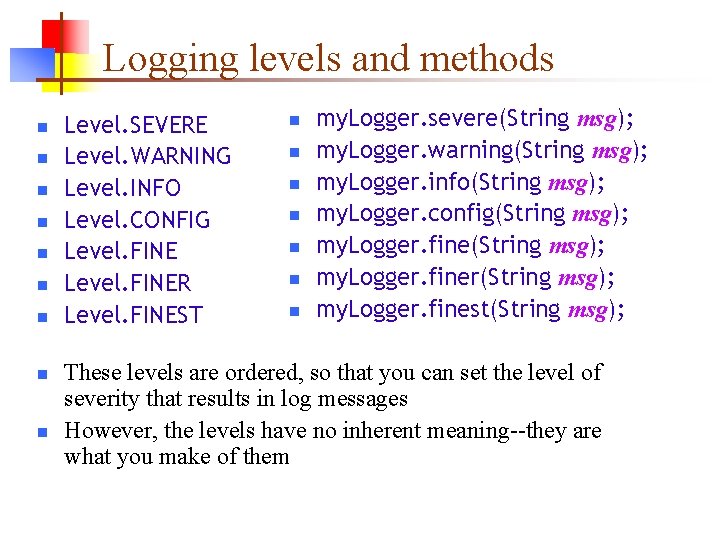
Logging levels and methods n n n n n Level. SEVERE Level. WARNING Level. INFO Level. CONFIG Level. FINER Level. FINEST n n n n my. Logger. severe(String msg); my. Logger. warning(String msg); my. Logger. info(String msg); my. Logger. config(String msg); my. Logger. finer(String msg); my. Logger. finest(String msg); These levels are ordered, so that you can set the level of severity that results in log messages However, the levels have no inherent meaning--they are what you make of them
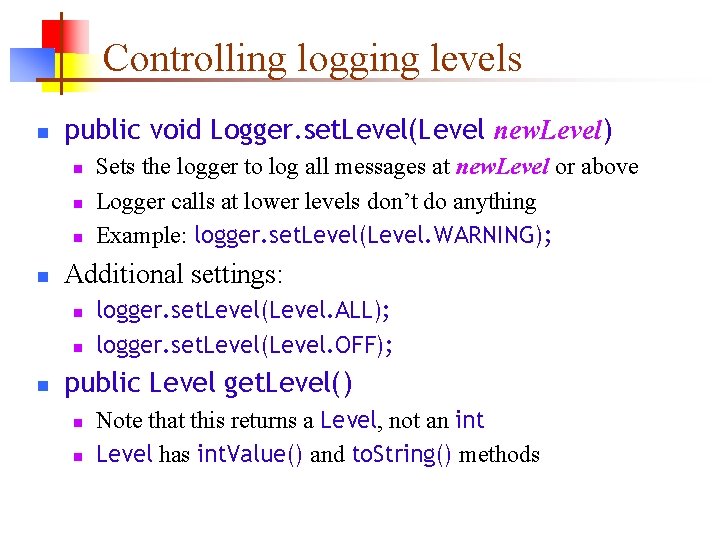
Controlling logging levels n public void Logger. set. Level(Level new. Level) n n Additional settings: n n n Sets the logger to log all messages at new. Level or above Logger calls at lower levels don’t do anything Example: logger. set. Level(Level. WARNING); logger. set. Level(Level. ALL); logger. set. Level(Level. OFF); public Level get. Level() n n Note that this returns a Level, not an int Level has int. Value() and to. String() methods
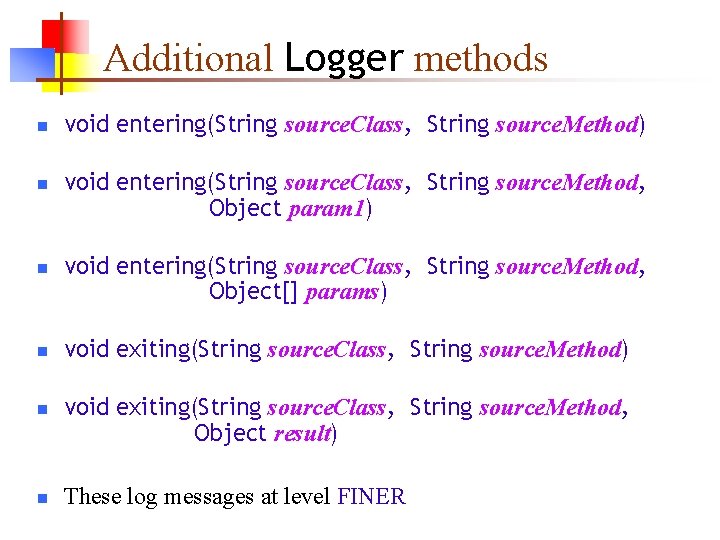
Additional Logger methods n n n void entering(String source. Class, String source. Method) void entering(String source. Class, String source. Method, Object param 1) void entering(String source. Class, String source. Method, Object[] params) void exiting(String source. Class, String source. Method, Object result) These log messages at level FINER
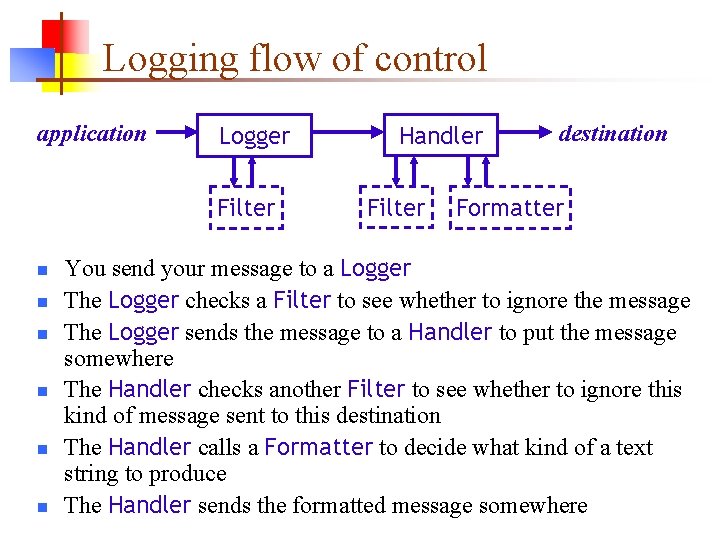
Logging flow of control application Logger Filter n n n Handler Filter destination Formatter You send your message to a Logger The Logger checks a Filter to see whether to ignore the message The Logger sends the message to a Handler to put the message somewhere The Handler checks another Filter to see whether to ignore this kind of message sent to this destination The Handler calls a Formatter to decide what kind of a text string to produce The Handler sends the formatted message somewhere
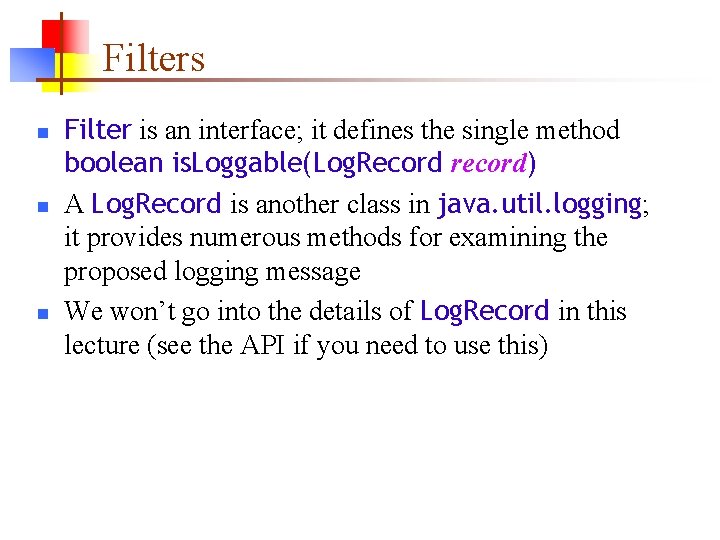
Filters n n n Filter is an interface; it defines the single method boolean is. Loggable(Log. Record record) A Log. Record is another class in java. util. logging; it provides numerous methods for examining the proposed logging message We won’t go into the details of Log. Record in this lecture (see the API if you need to use this)
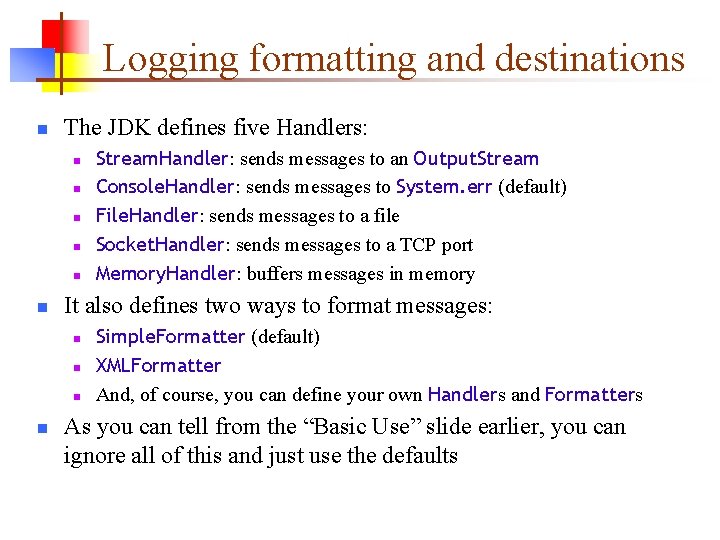
Logging formatting and destinations n The JDK defines five Handlers: n n n It also defines two ways to format messages: n n Stream. Handler: sends messages to an Output. Stream Console. Handler: sends messages to System. err (default) File. Handler: sends messages to a file Socket. Handler: sends messages to a TCP port Memory. Handler: buffers messages in memory Simple. Formatter (default) XMLFormatter And, of course, you can define your own Handlers and Formatters As you can tell from the “Basic Use” slide earlier, you can ignore all of this and just use the defaults
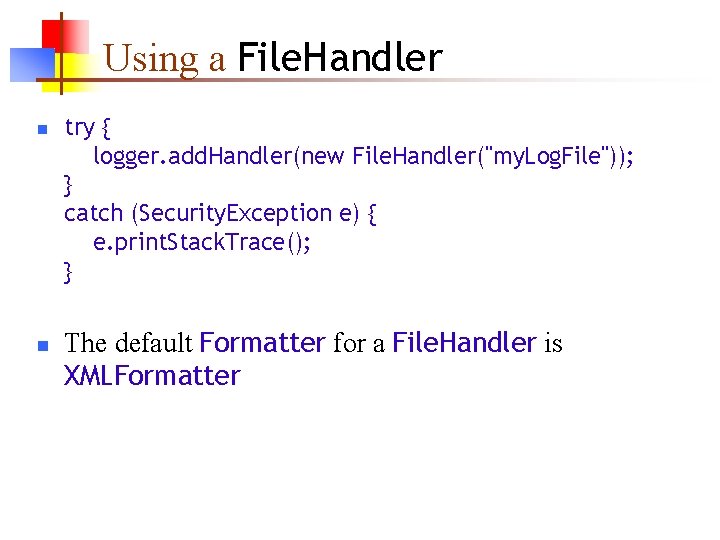
Using a File. Handler n n try { logger. add. Handler(new File. Handler("my. Log. File")); } catch (Security. Exception e) { e. print. Stack. Trace(); } The default Formatter for a File. Handler is XMLFormatter
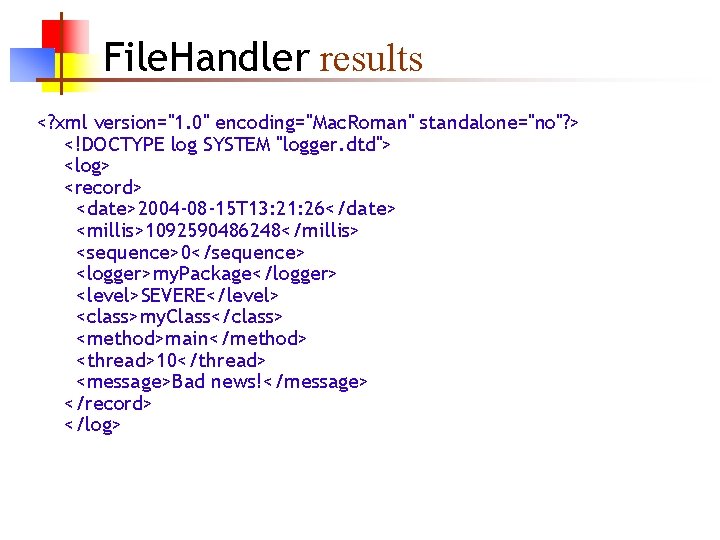
File. Handler results <? xml version="1. 0" encoding="Mac. Roman" standalone="no"? > <!DOCTYPE log SYSTEM "logger. dtd"> <log> <record> <date>2004 -08 -15 T 13: 21: 26</date> <millis>1092590486248</millis> <sequence>0</sequence> <logger>my. Package</logger> <level>SEVERE</level> <class>my. Class</class> <method>main</method> <thread>10</thread> <message>Bad news!</message> </record> </log>
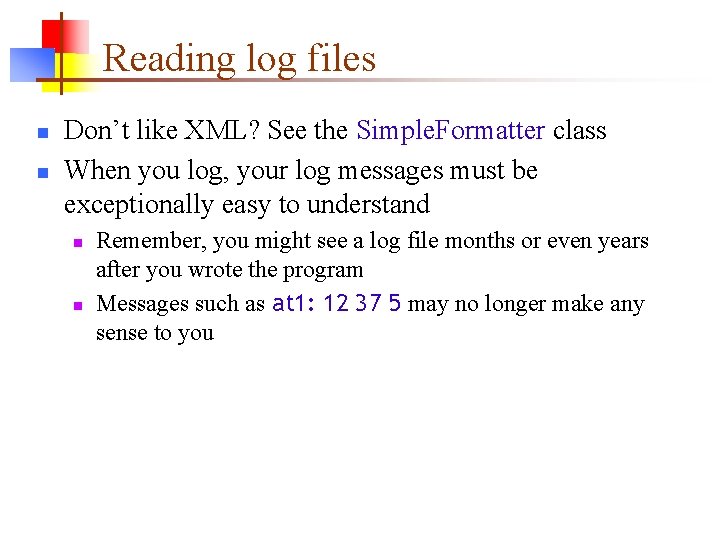
Reading log files n n Don’t like XML? See the Simple. Formatter class When you log, your log messages must be exceptionally easy to understand n n Remember, you might see a log file months or even years after you wrote the program Messages such as at 1: 12 37 5 may no longer make any sense to you
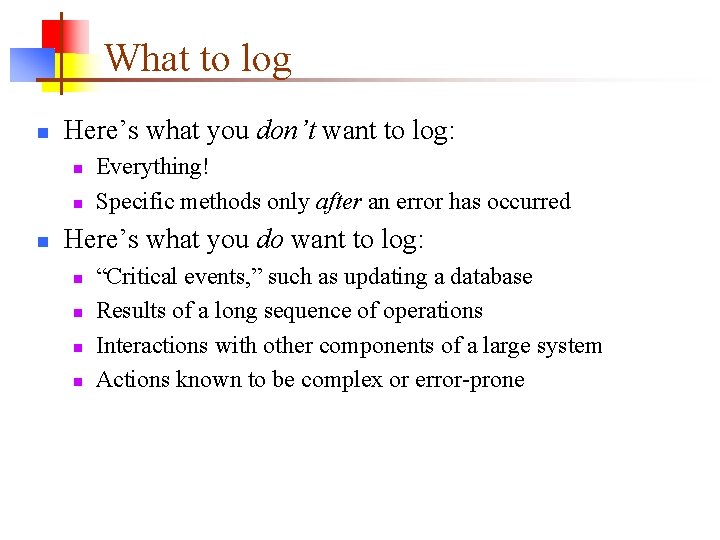
What to log n Here’s what you don’t want to log: n n n Everything! Specific methods only after an error has occurred Here’s what you do want to log: n n “Critical events, ” such as updating a database Results of a long sequence of operations Interactions with other components of a large system Actions known to be complex or error-prone
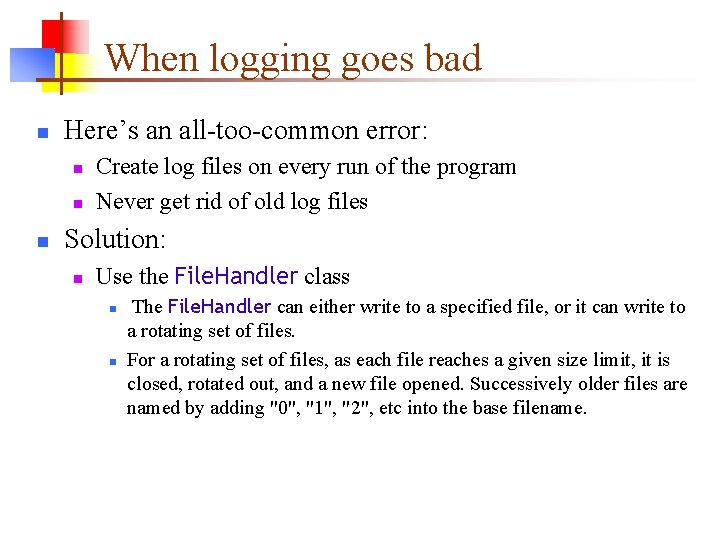
When logging goes bad n Here’s an all-too-common error: n n n Create log files on every run of the program Never get rid of old log files Solution: n Use the File. Handler class n n The File. Handler can either write to a specified file, or it can write to a rotating set of files. For a rotating set of files, as each file reaches a given size limit, it is closed, rotated out, and a new file opened. Successively older files are named by adding "0", "1", "2", etc into the base filename.
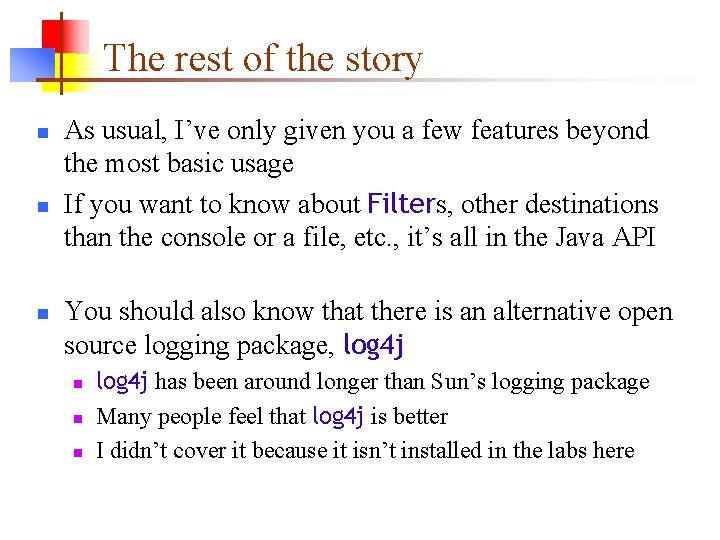
The rest of the story n n n As usual, I’ve only given you a few features beyond the most basic usage If you want to know about Filters, other destinations than the console or a file, etc. , it’s all in the Java API You should also know that there is an alternative open source logging package, log 4 j n n n log 4 j has been around longer than Sun’s logging package Many people feel that log 4 j is better I didn’t cover it because it isn’t installed in the labs here
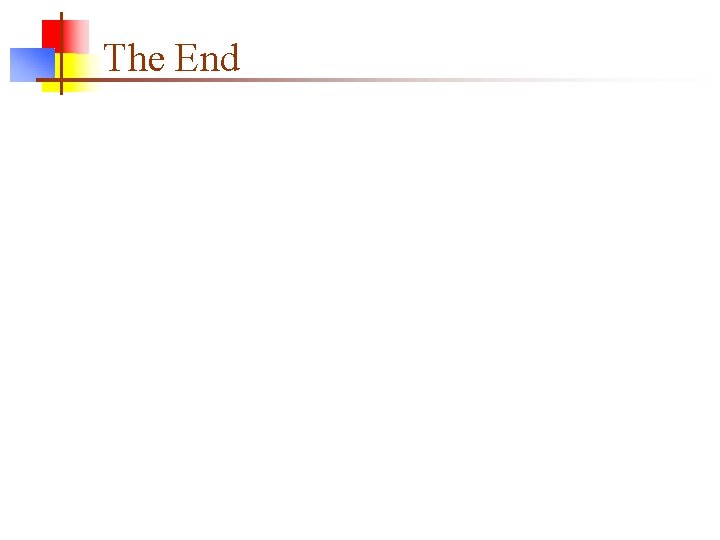
The End