Lists The List ADT List ADT A list
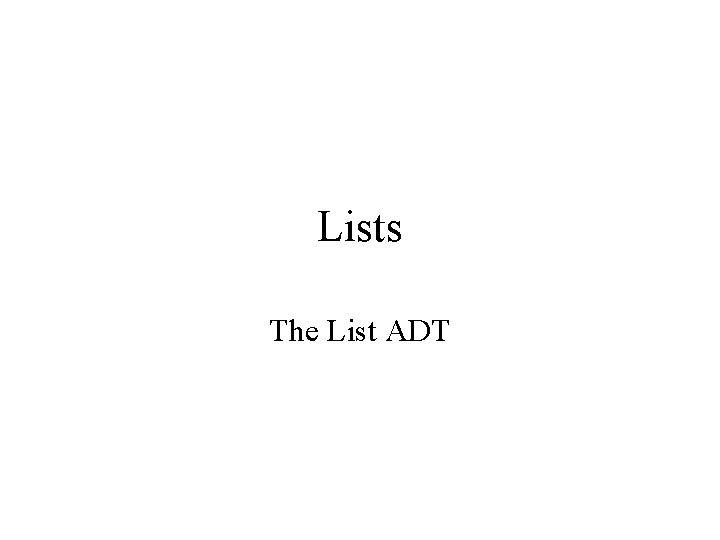
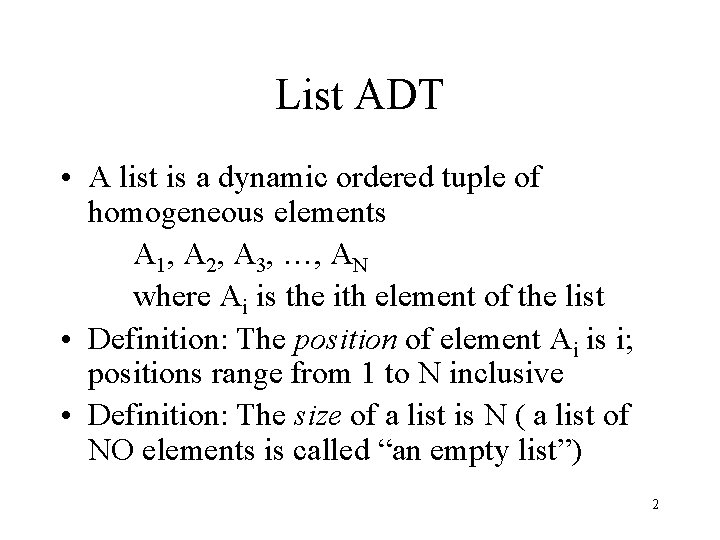
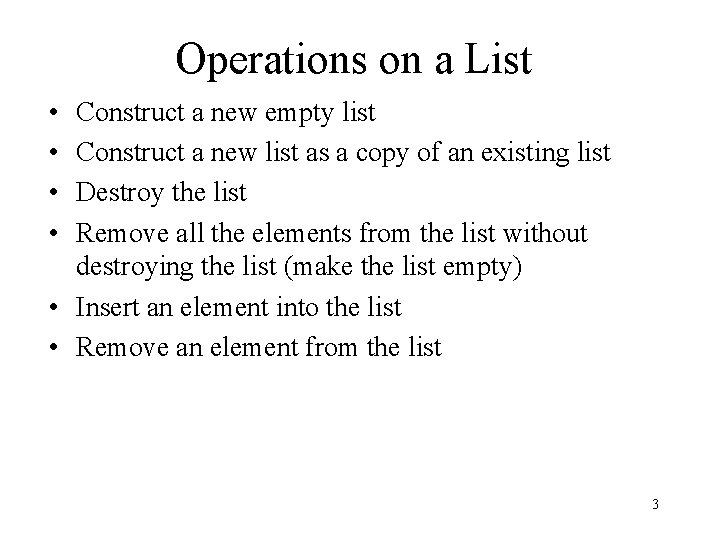
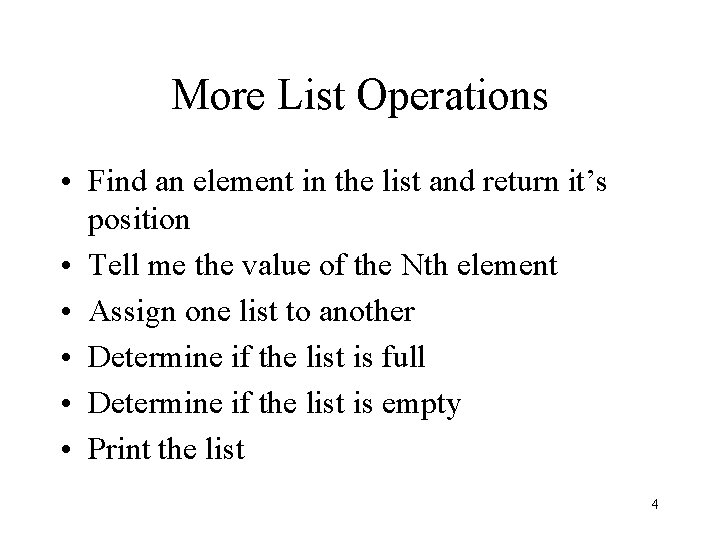
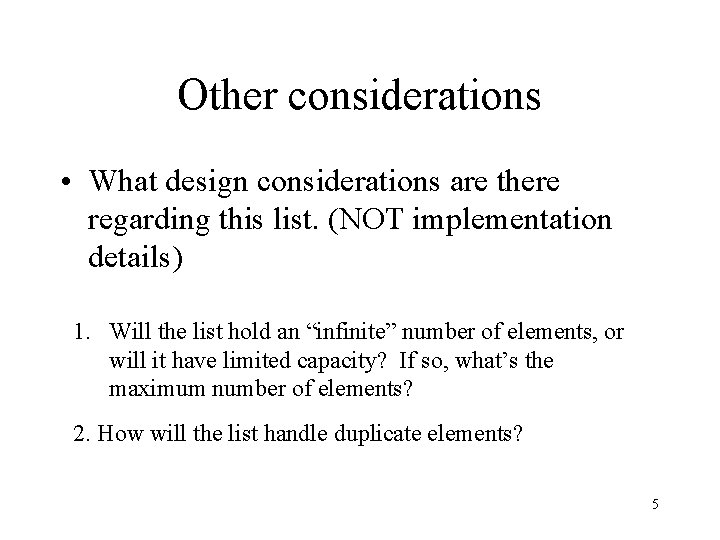
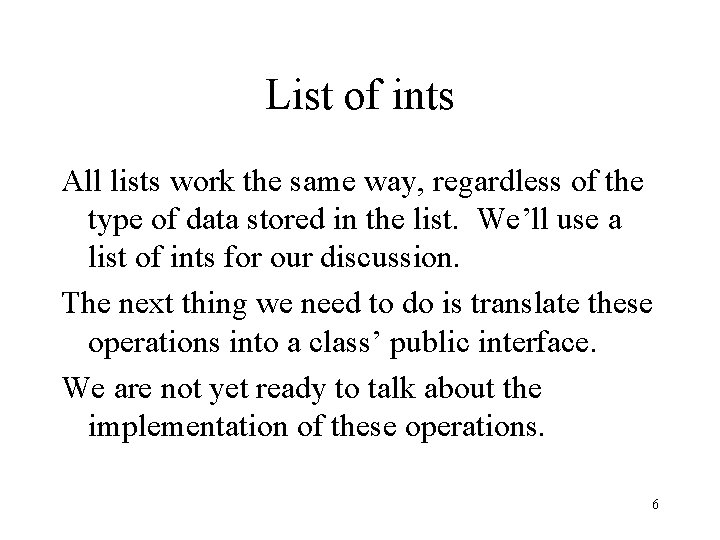
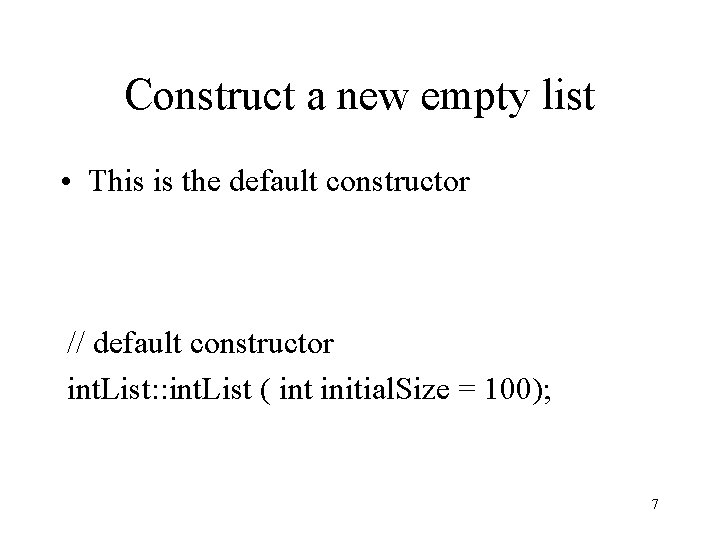
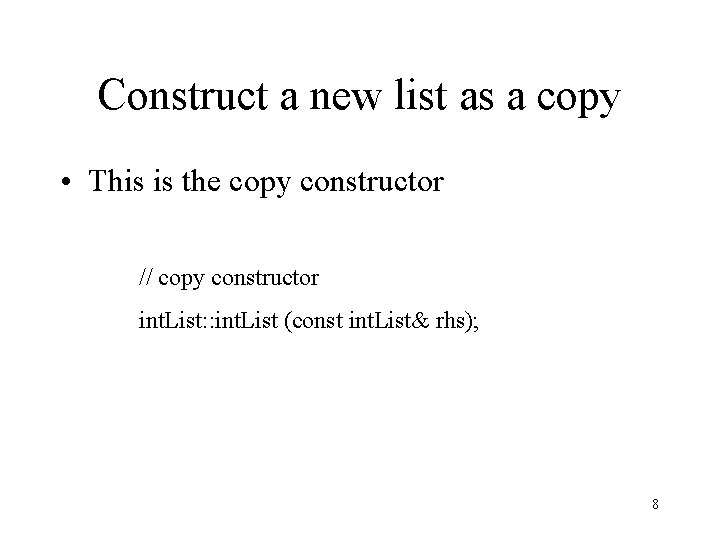
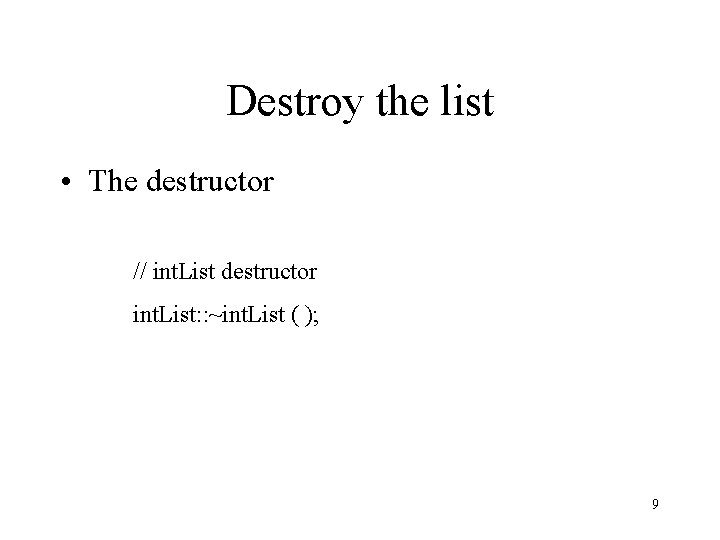
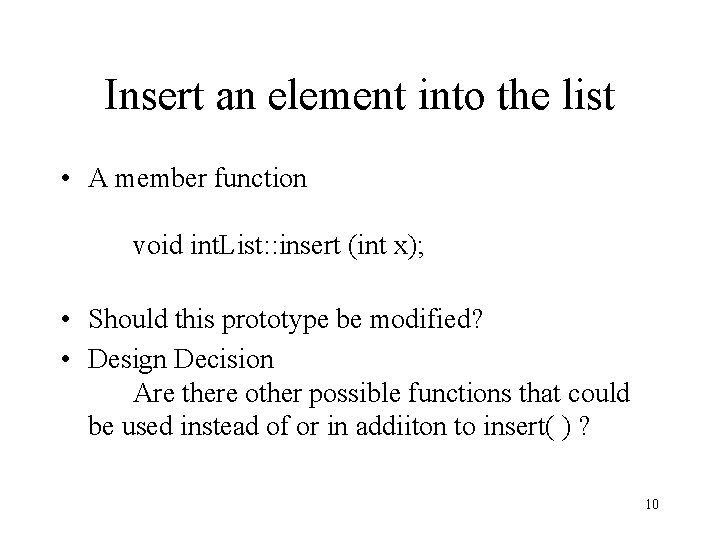
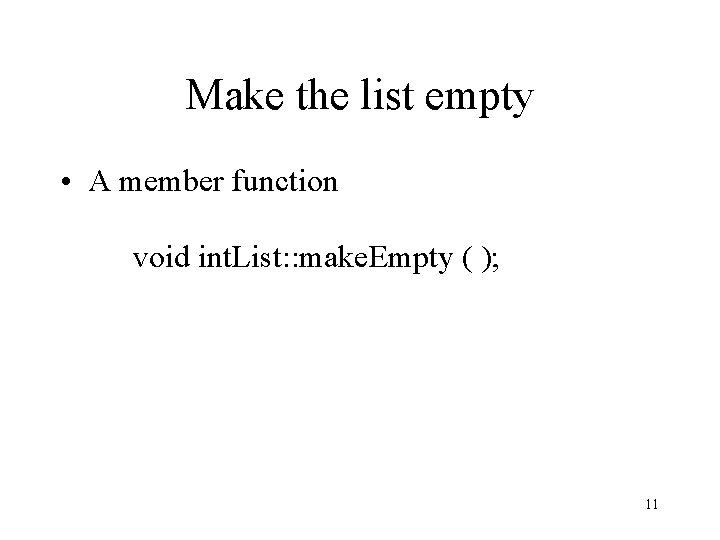
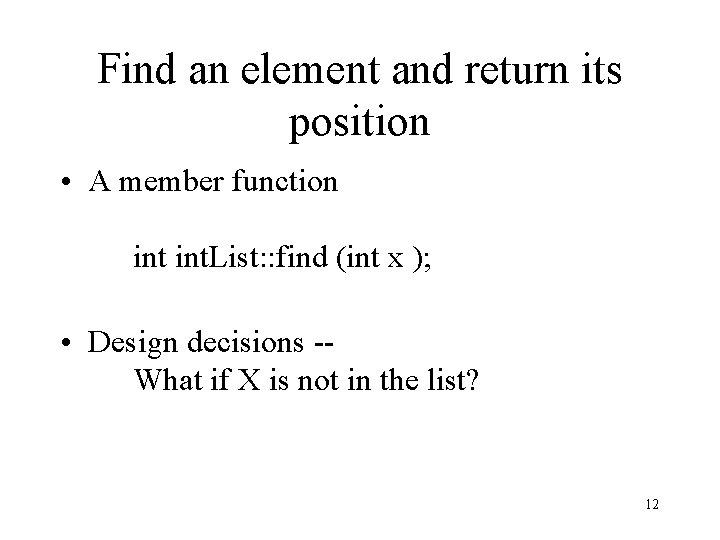
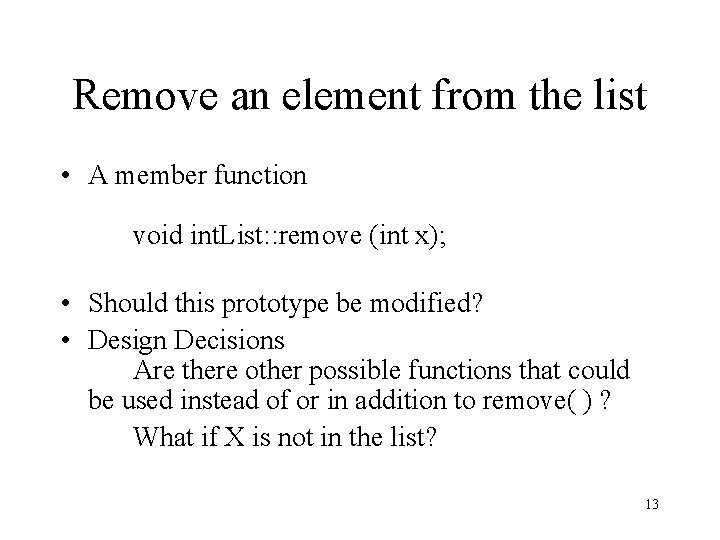
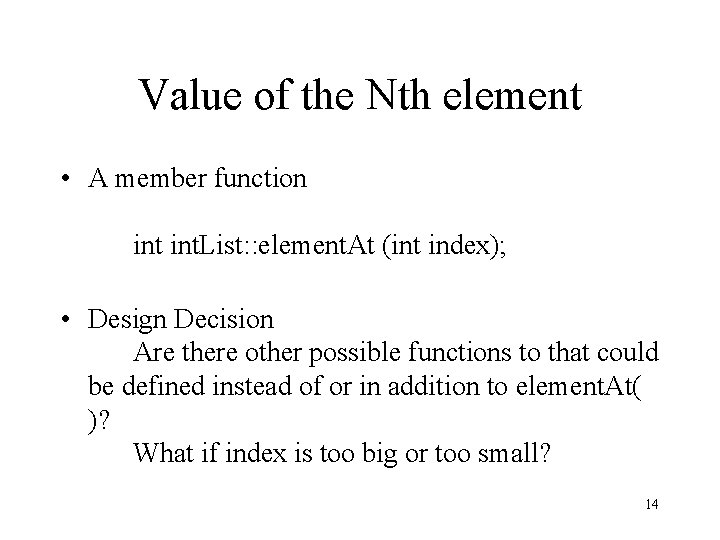
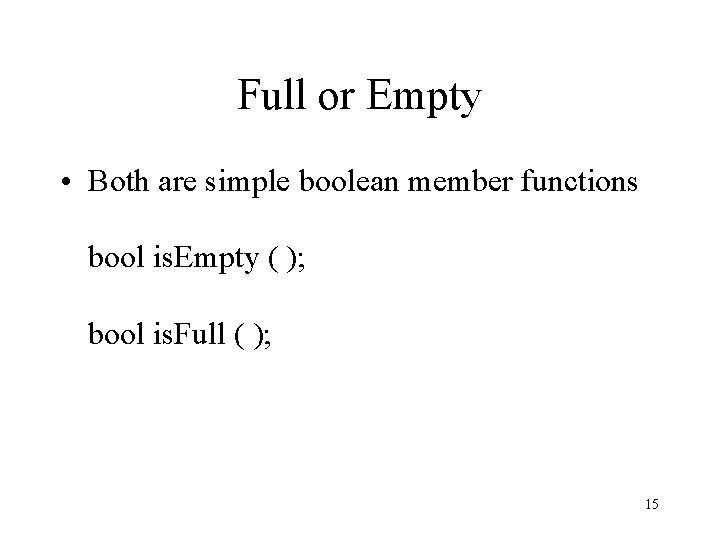
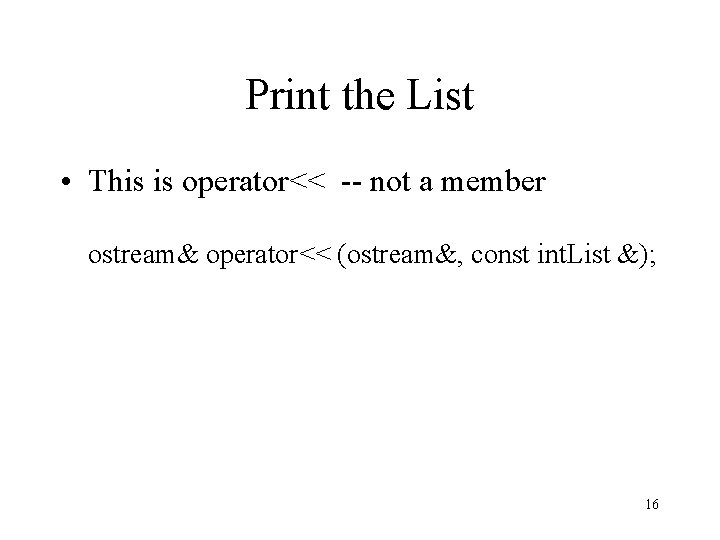
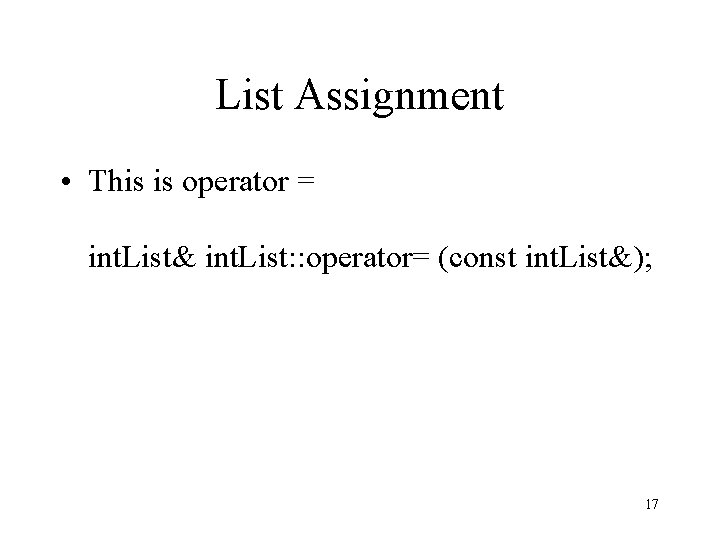
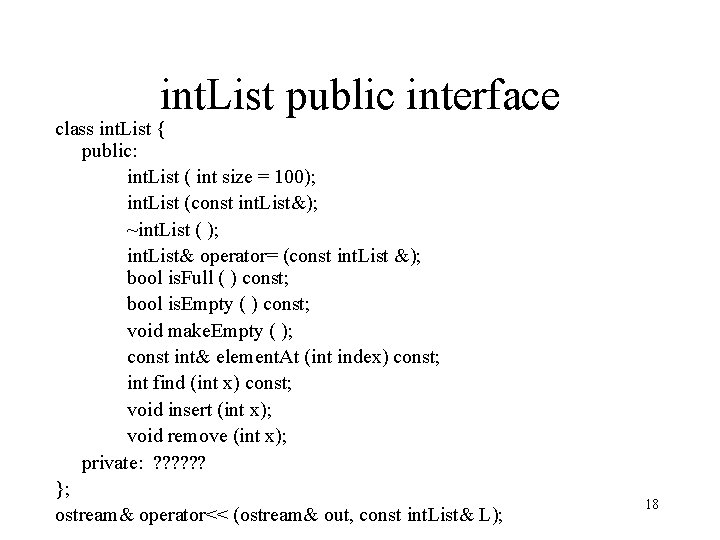
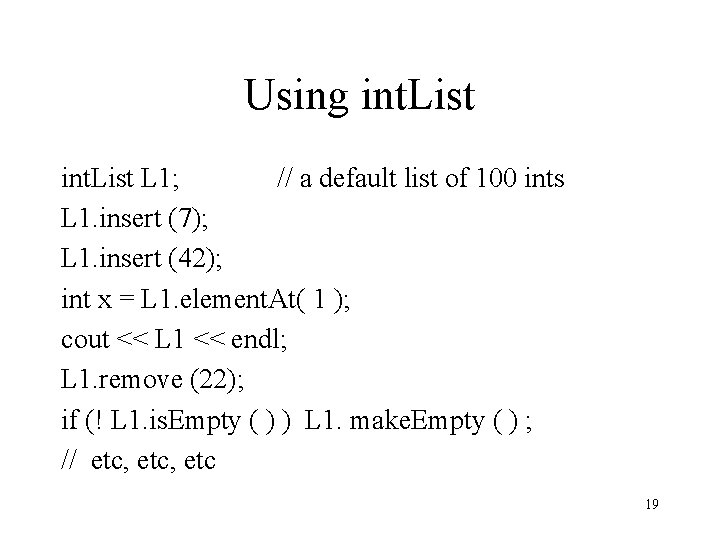
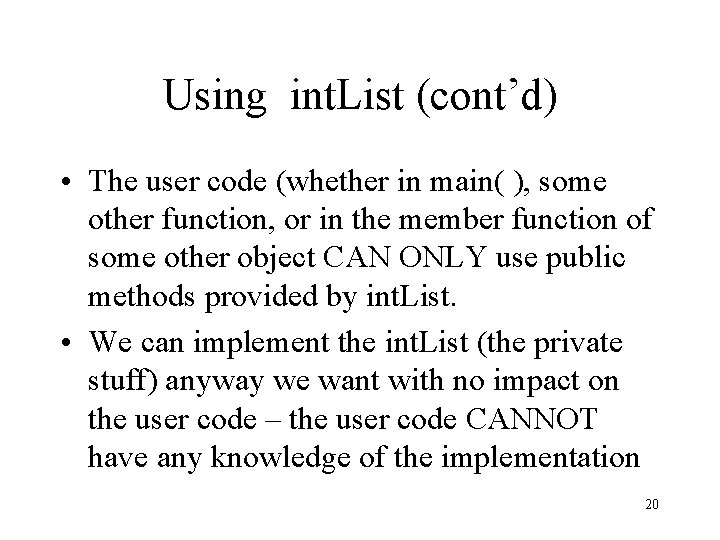
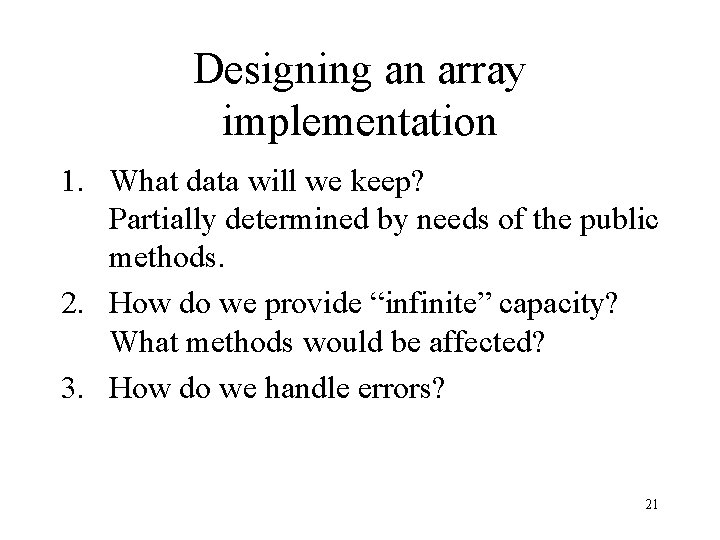
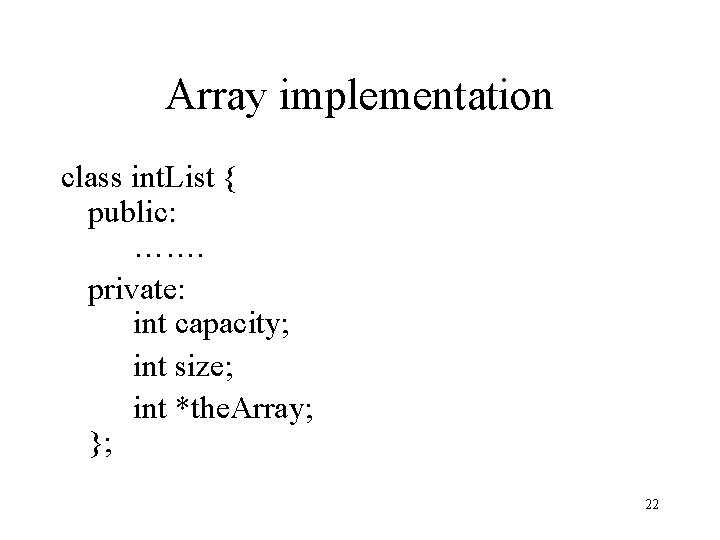
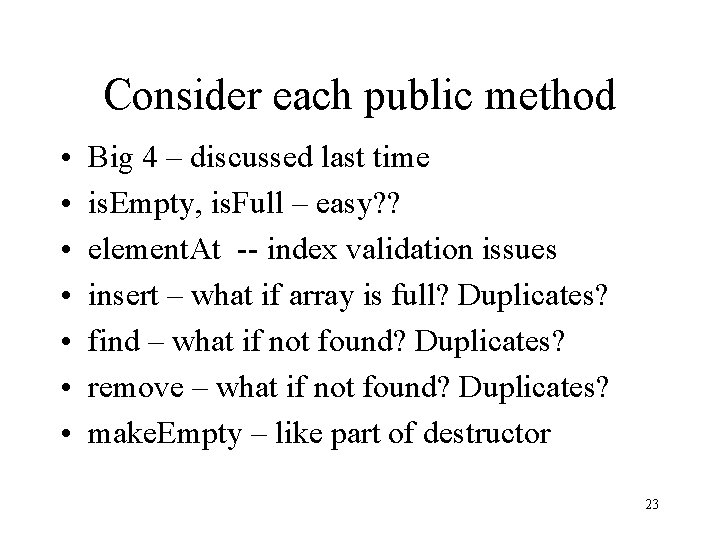
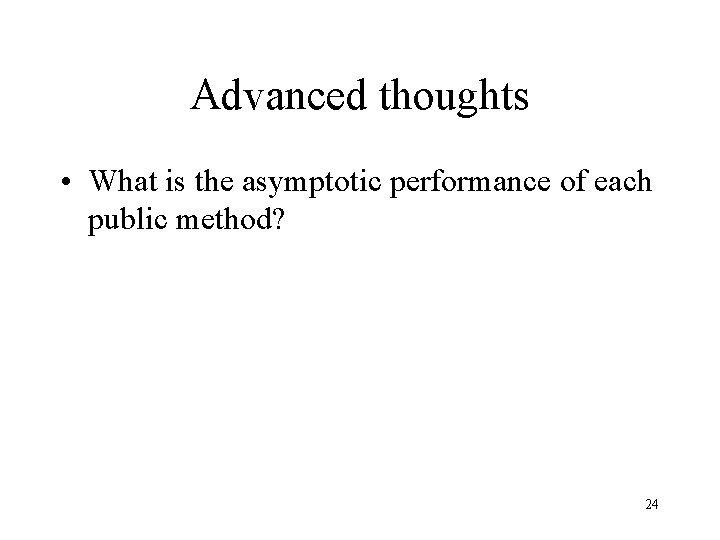
- Slides: 24
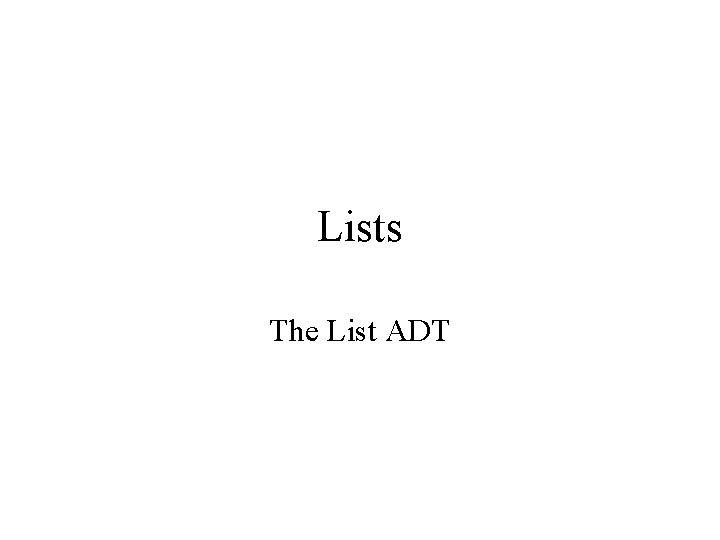
Lists The List ADT
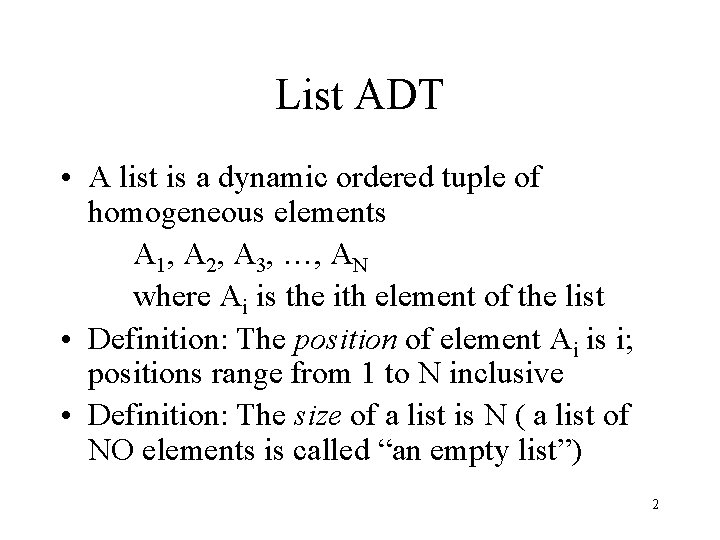
List ADT • A list is a dynamic ordered tuple of homogeneous elements A 1, A 2, A 3, …, AN where Ai is the ith element of the list • Definition: The position of element Ai is i; positions range from 1 to N inclusive • Definition: The size of a list is N ( a list of NO elements is called “an empty list”) 2
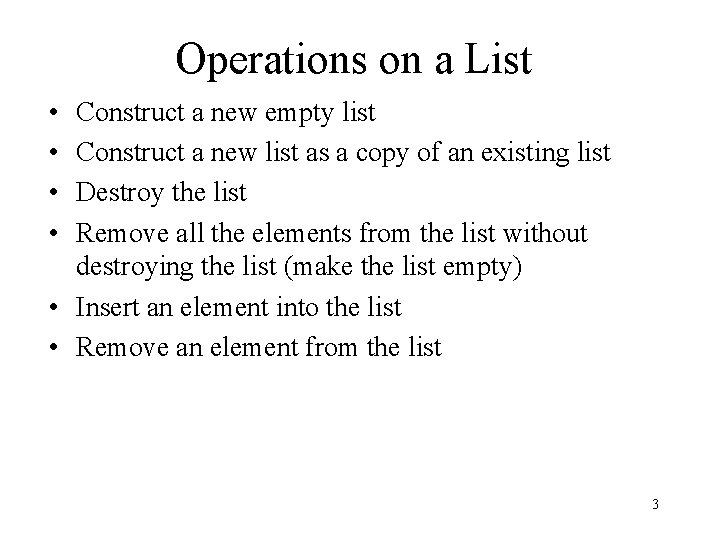
Operations on a List • • Construct a new empty list Construct a new list as a copy of an existing list Destroy the list Remove all the elements from the list without destroying the list (make the list empty) • Insert an element into the list • Remove an element from the list 3
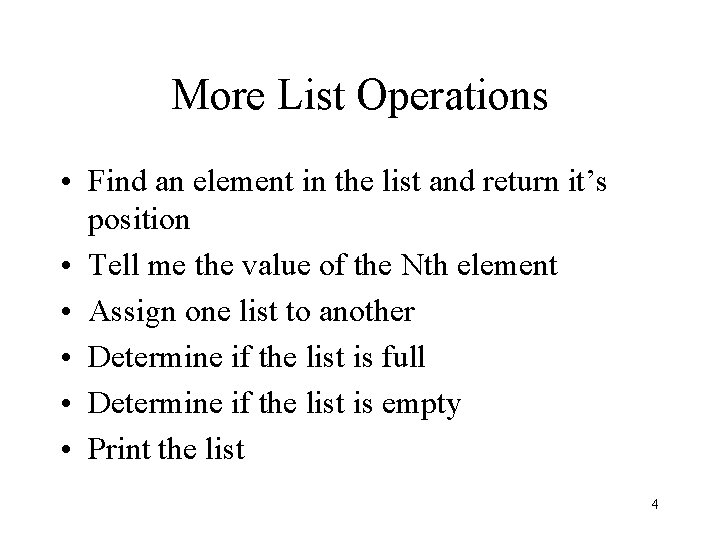
More List Operations • Find an element in the list and return it’s position • Tell me the value of the Nth element • Assign one list to another • Determine if the list is full • Determine if the list is empty • Print the list 4
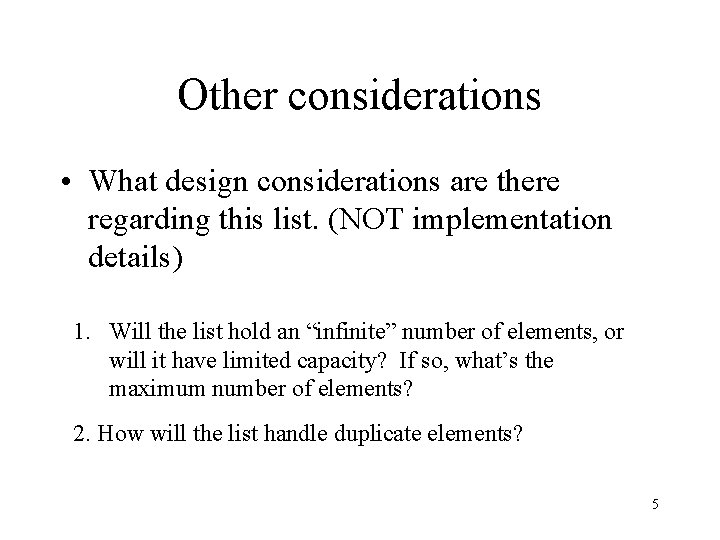
Other considerations • What design considerations are there regarding this list. (NOT implementation details) 1. Will the list hold an “infinite” number of elements, or will it have limited capacity? If so, what’s the maximum number of elements? 2. How will the list handle duplicate elements? 5
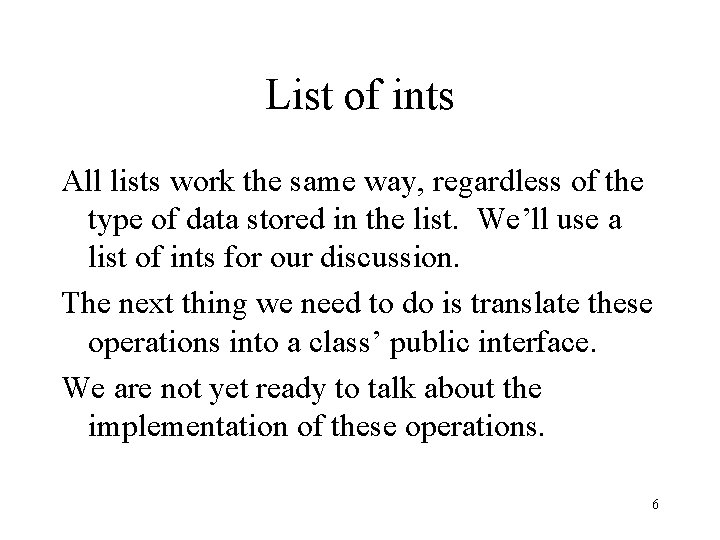
List of ints All lists work the same way, regardless of the type of data stored in the list. We’ll use a list of ints for our discussion. The next thing we need to do is translate these operations into a class’ public interface. We are not yet ready to talk about the implementation of these operations. 6
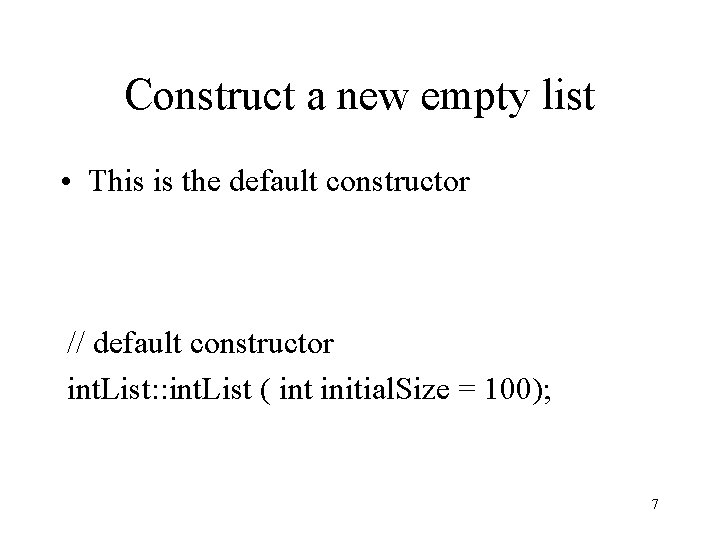
Construct a new empty list • This is the default constructor // default constructor int. List: : int. List ( int initial. Size = 100); 7
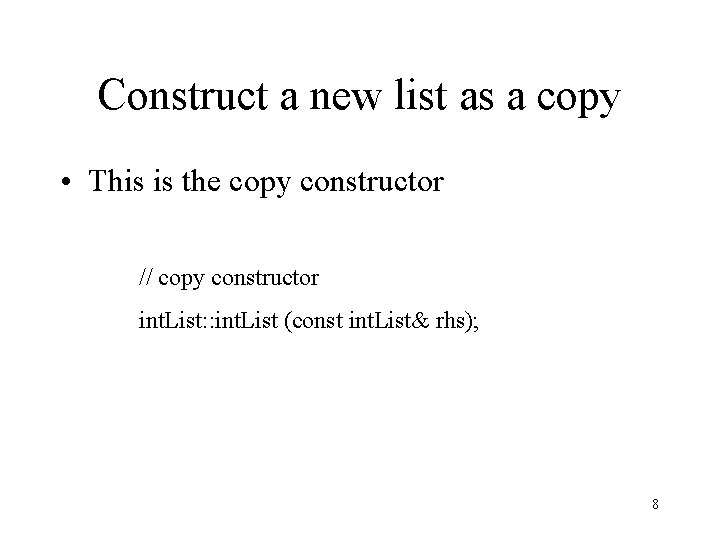
Construct a new list as a copy • This is the copy constructor // copy constructor int. List: : int. List (const int. List& rhs); 8
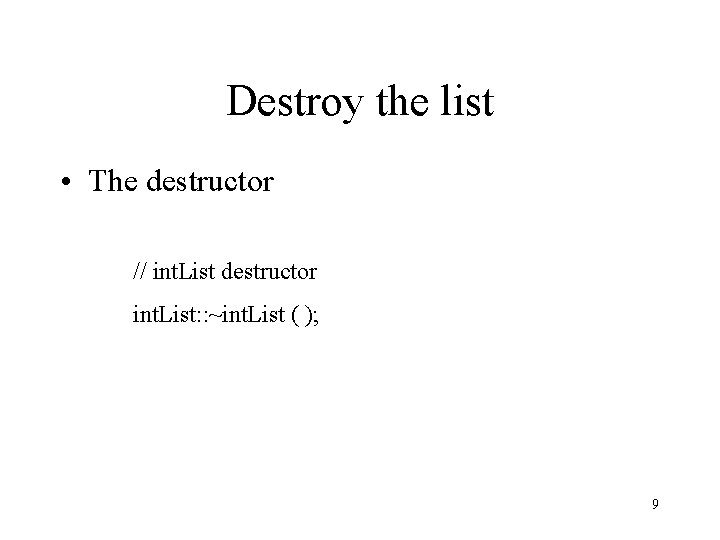
Destroy the list • The destructor // int. List destructor int. List: : ~int. List ( ); 9
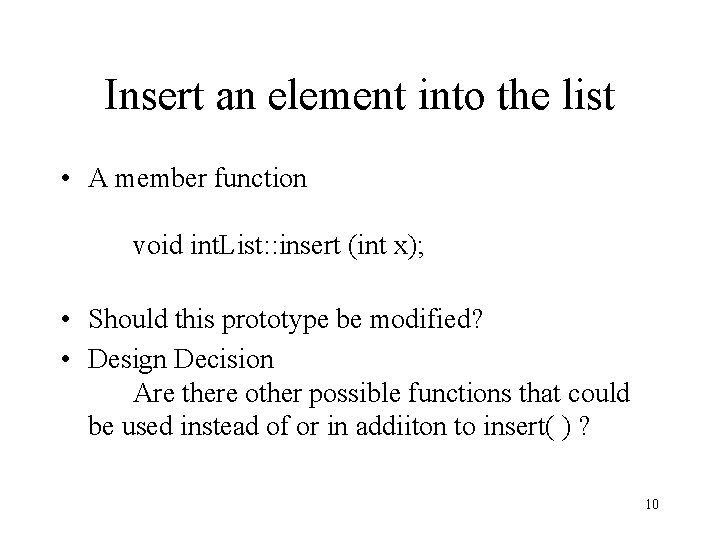
Insert an element into the list • A member function void int. List: : insert (int x); • Should this prototype be modified? • Design Decision Are there other possible functions that could be used instead of or in addiiton to insert( ) ? 10
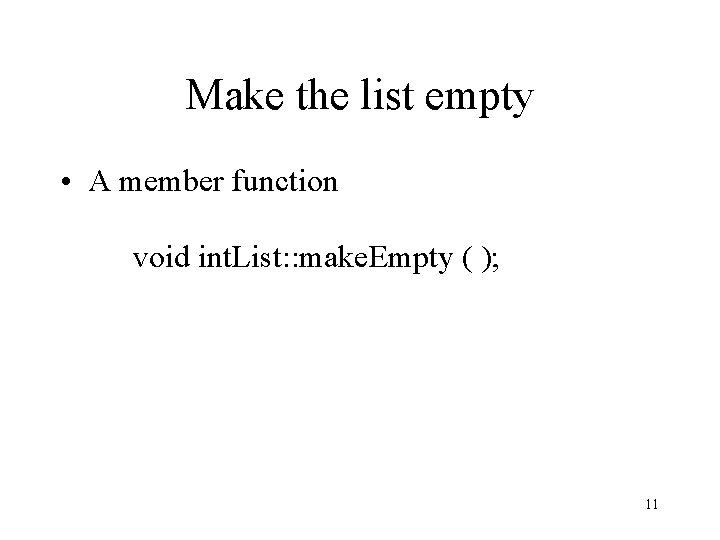
Make the list empty • A member function void int. List: : make. Empty ( ); 11
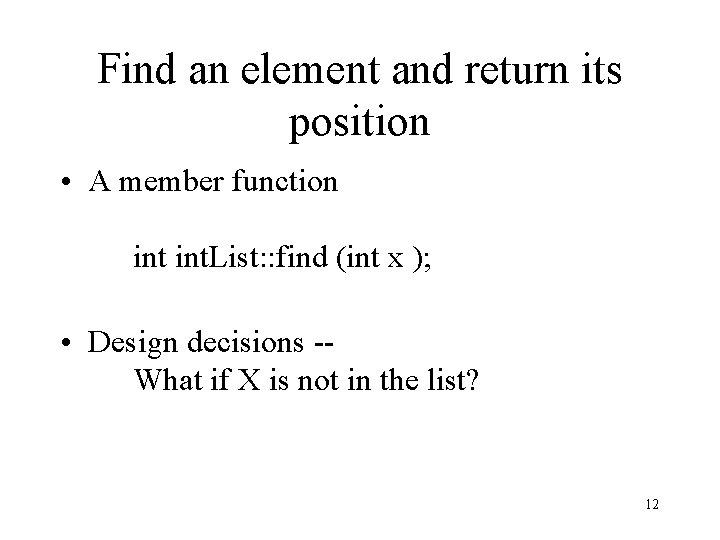
Find an element and return its position • A member function int. List: : find (int x ); • Design decisions -What if X is not in the list? 12
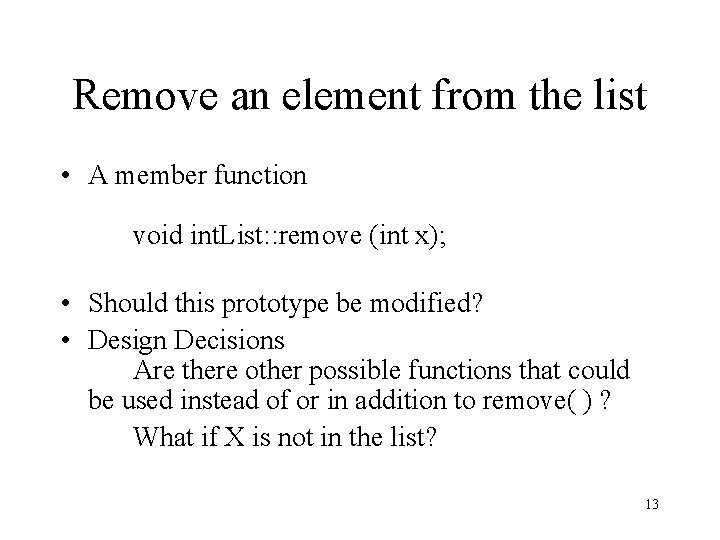
Remove an element from the list • A member function void int. List: : remove (int x); • Should this prototype be modified? • Design Decisions Are there other possible functions that could be used instead of or in addition to remove( ) ? What if X is not in the list? 13
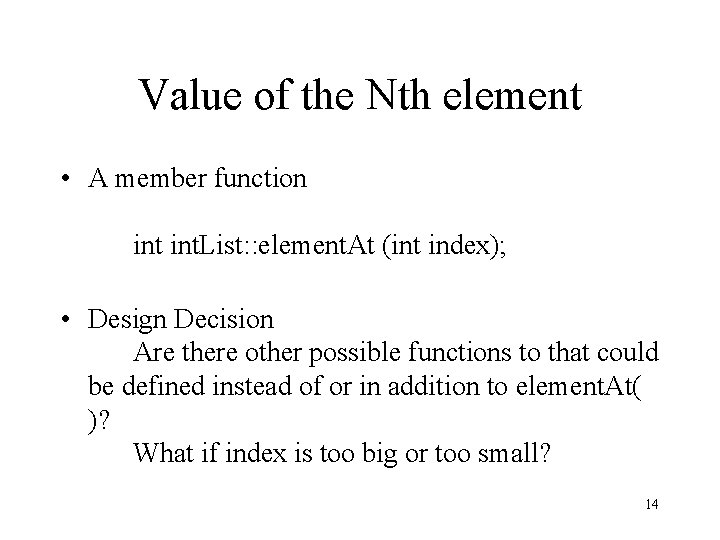
Value of the Nth element • A member function int. List: : element. At (int index); • Design Decision Are there other possible functions to that could be defined instead of or in addition to element. At( )? What if index is too big or too small? 14
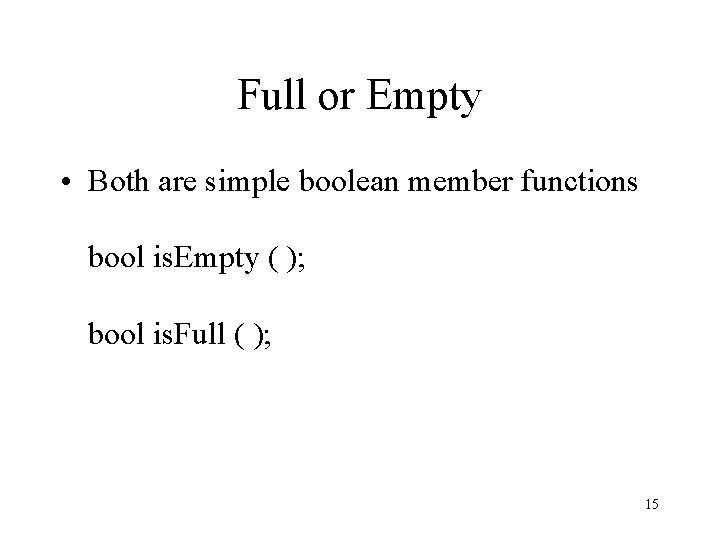
Full or Empty • Both are simple boolean member functions bool is. Empty ( ); bool is. Full ( ); 15
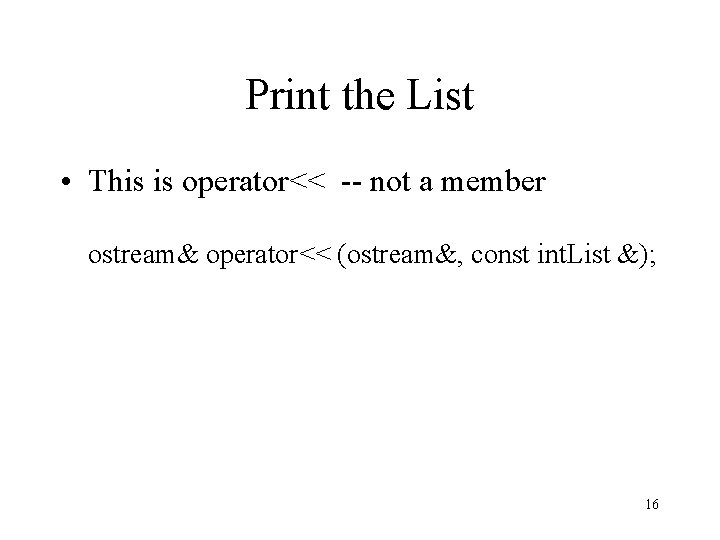
Print the List • This is operator<< -- not a member ostream& operator<< (ostream&, const int. List &); 16
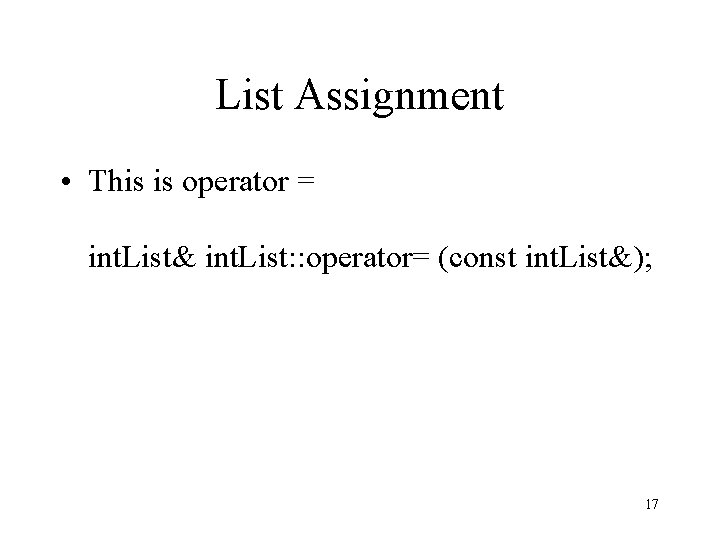
List Assignment • This is operator = int. List& int. List: : operator= (const int. List&); 17
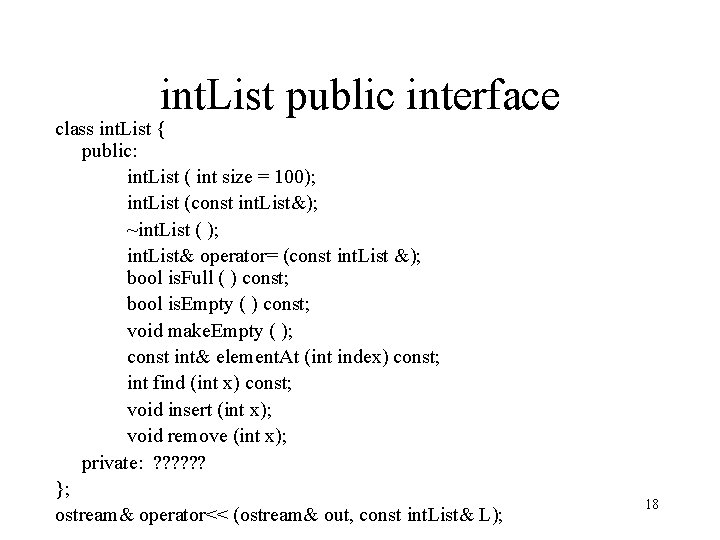
int. List public interface class int. List { public: int. List ( int size = 100); int. List (const int. List&); ~int. List ( ); int. List& operator= (const int. List &); bool is. Full ( ) const; bool is. Empty ( ) const; void make. Empty ( ); const int& element. At (int index) const; int find (int x) const; void insert (int x); void remove (int x); private: ? ? ? }; ostream& operator<< (ostream& out, const int. List& L); 18
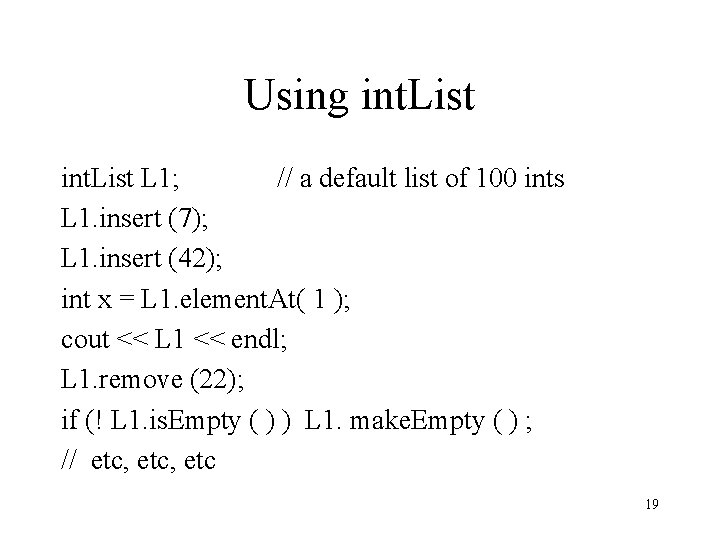
Using int. List L 1; // a default list of 100 ints L 1. insert (7); L 1. insert (42); int x = L 1. element. At( 1 ); cout << L 1 << endl; L 1. remove (22); if (! L 1. is. Empty ( ) ) L 1. make. Empty ( ) ; // etc, etc 19
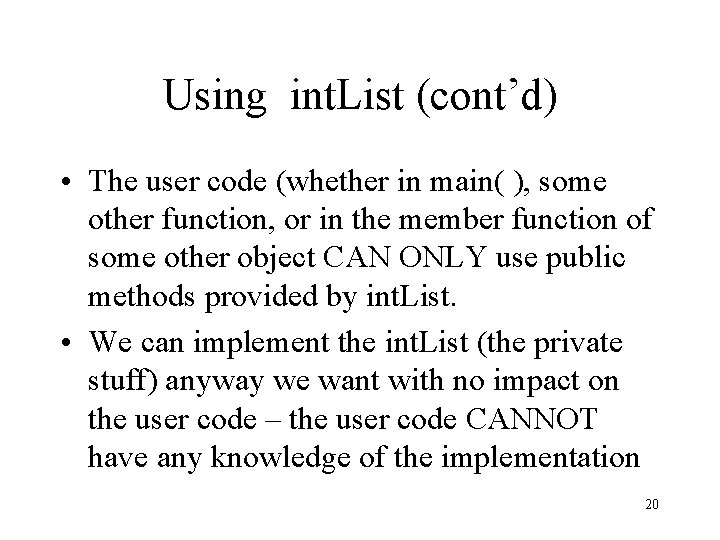
Using int. List (cont’d) • The user code (whether in main( ), some other function, or in the member function of some other object CAN ONLY use public methods provided by int. List. • We can implement the int. List (the private stuff) anyway we want with no impact on the user code – the user code CANNOT have any knowledge of the implementation 20
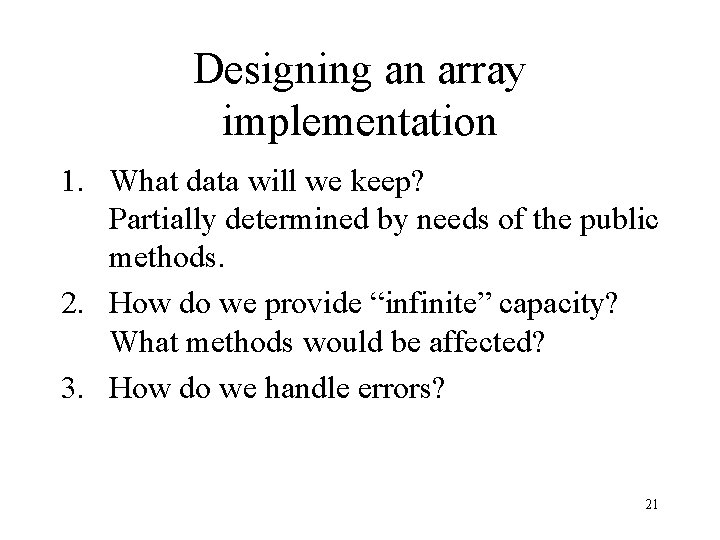
Designing an array implementation 1. What data will we keep? Partially determined by needs of the public methods. 2. How do we provide “infinite” capacity? What methods would be affected? 3. How do we handle errors? 21
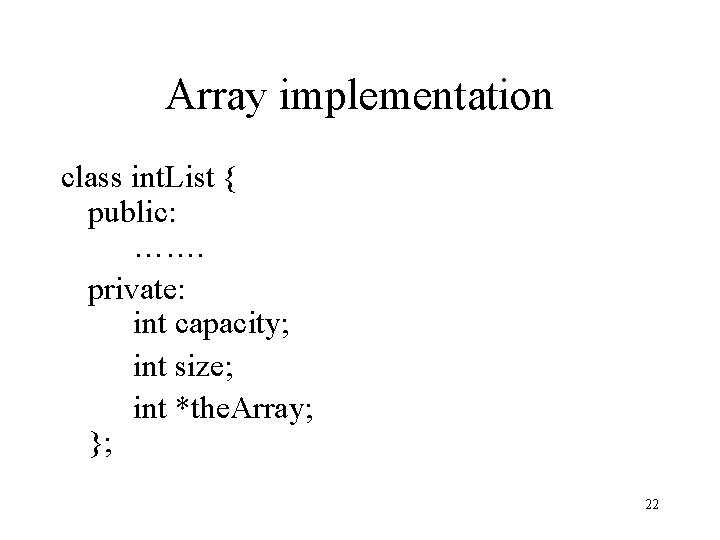
Array implementation class int. List { public: ……. private: int capacity; int size; int *the. Array; }; 22
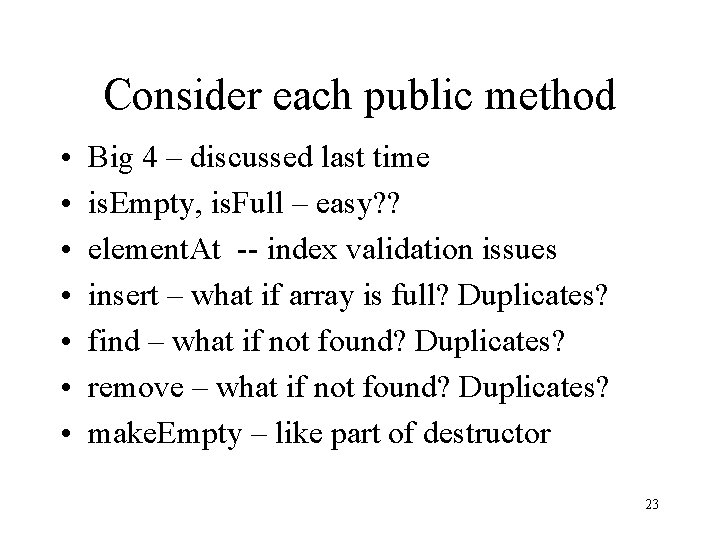
Consider each public method • • Big 4 – discussed last time is. Empty, is. Full – easy? ? element. At -- index validation issues insert – what if array is full? Duplicates? find – what if not found? Duplicates? remove – what if not found? Duplicates? make. Empty – like part of destructor 23
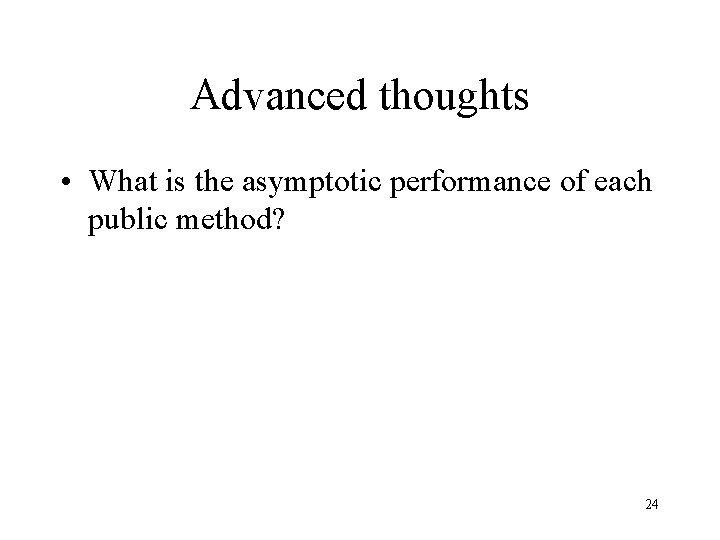
Advanced thoughts • What is the asymptotic performance of each public method? 24
Lists in prolog
Concatinates
Adt linked list
Polynomial addition in data structure
List adt
Array based implementation of list adt
List adt
List adt
List adt
List adt
Java list adt
List adt java
List adt
Who wrote this
Frwords
Swot analysis between nike and adidas
Wish lists year
No nonsense spelling strategies
Cons in lisp
Political wish lists
Parallel structure in lists
Words with double.letters
Blockly c
Wish lists year
Political lists new