LIST PROCESSING 1 Self Referential Structures 14 struct
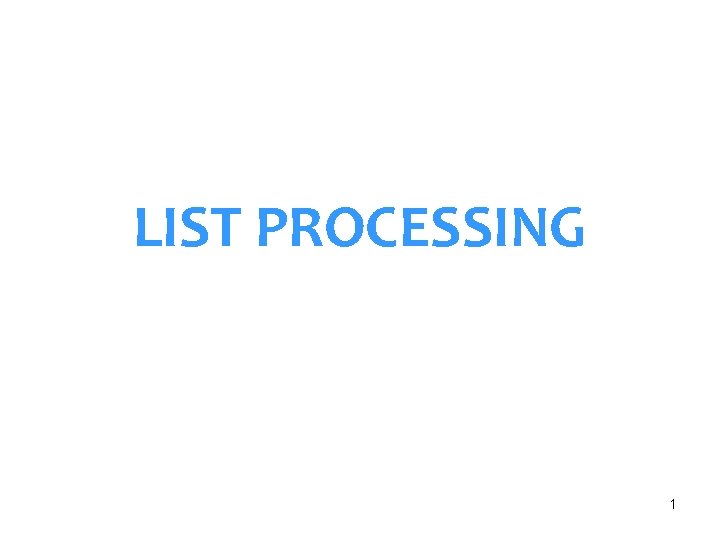
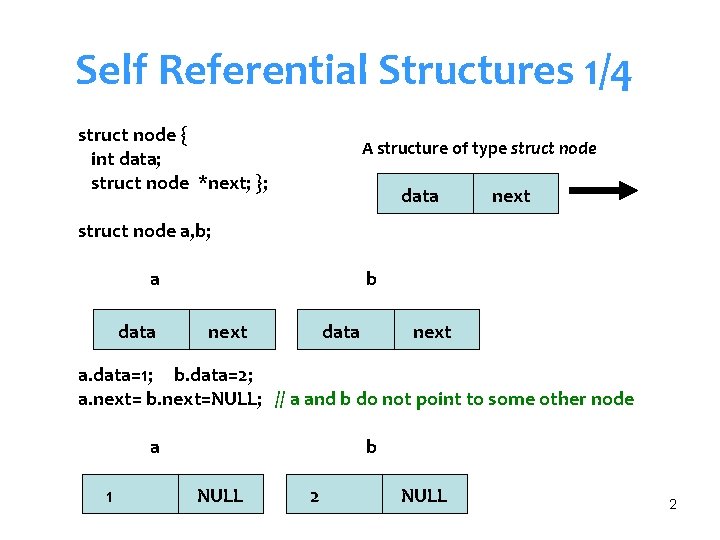
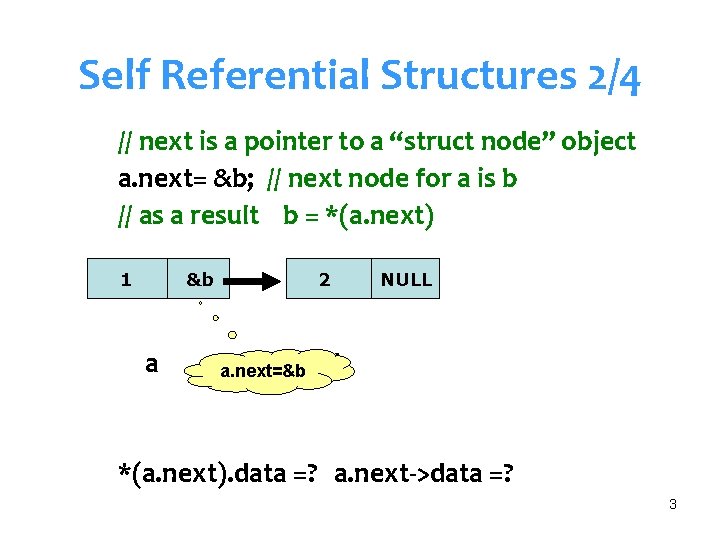
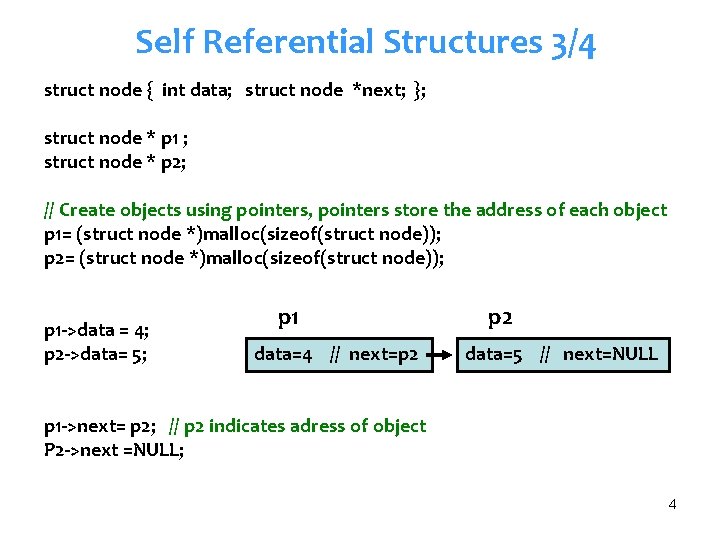
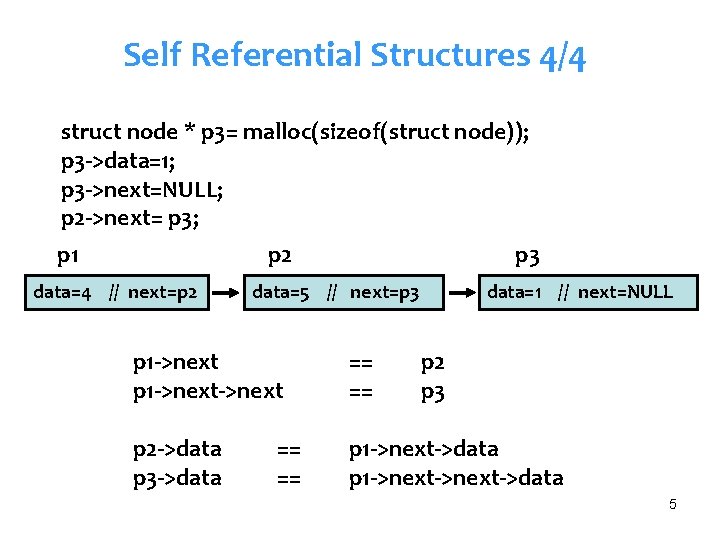
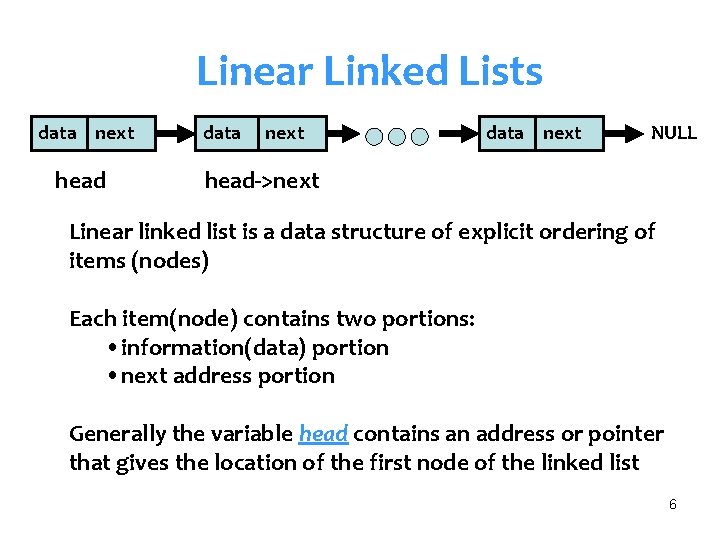
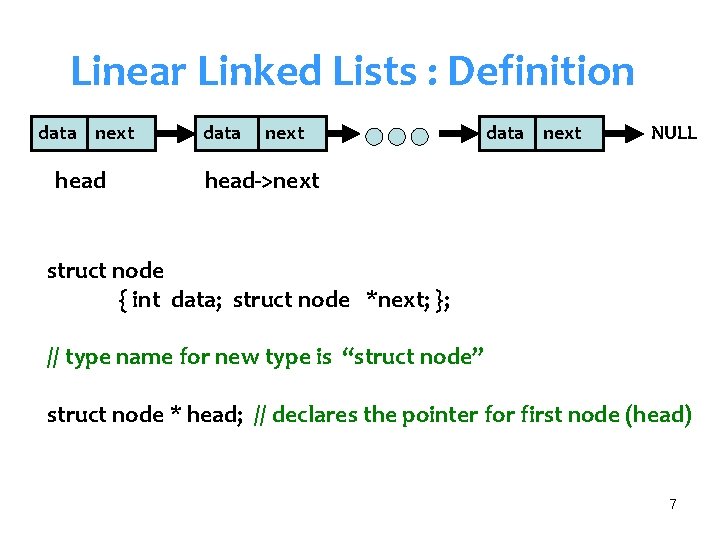
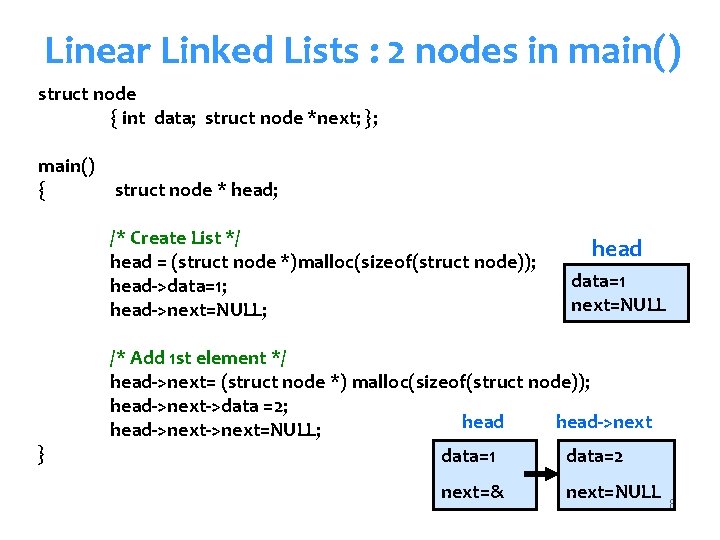
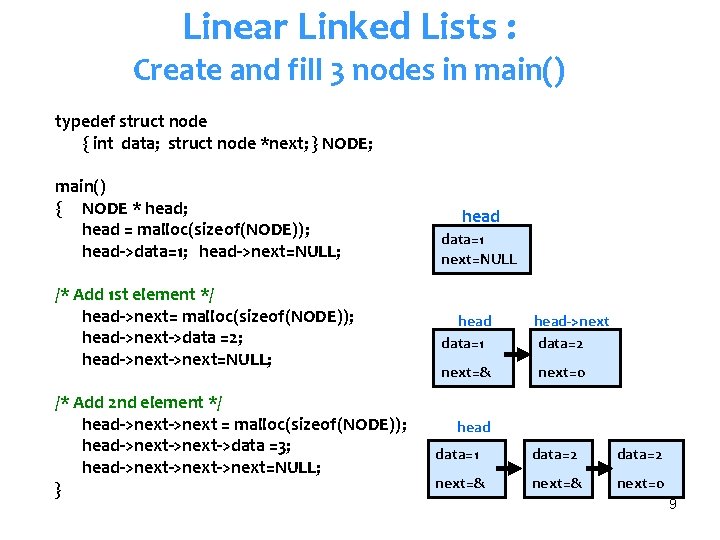
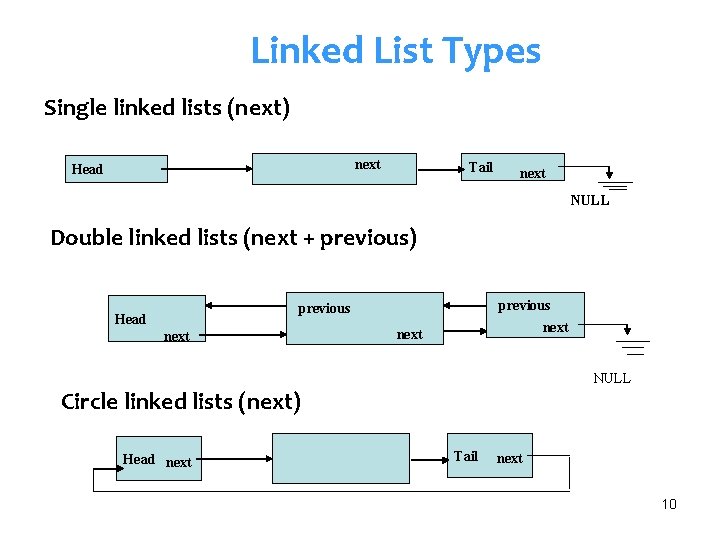
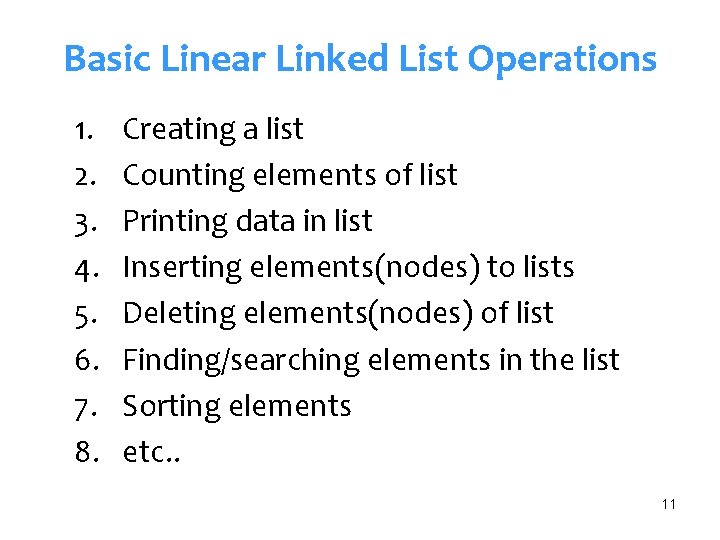
![CREATING LINEAR LINKED LIST An integer array (x[4]) will be used to create a CREATING LINEAR LINKED LIST An integer array (x[4]) will be used to create a](https://slidetodoc.com/presentation_image_h/d29d592ab00a360a0e5f9252f2f2245d/image-12.jpg)
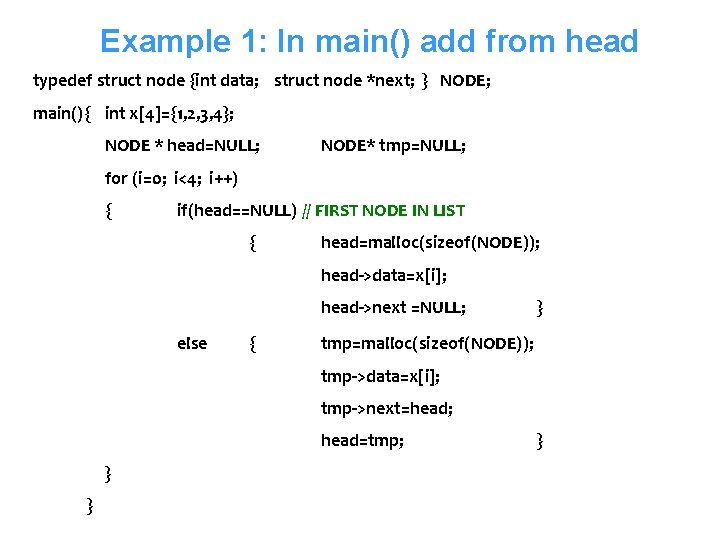
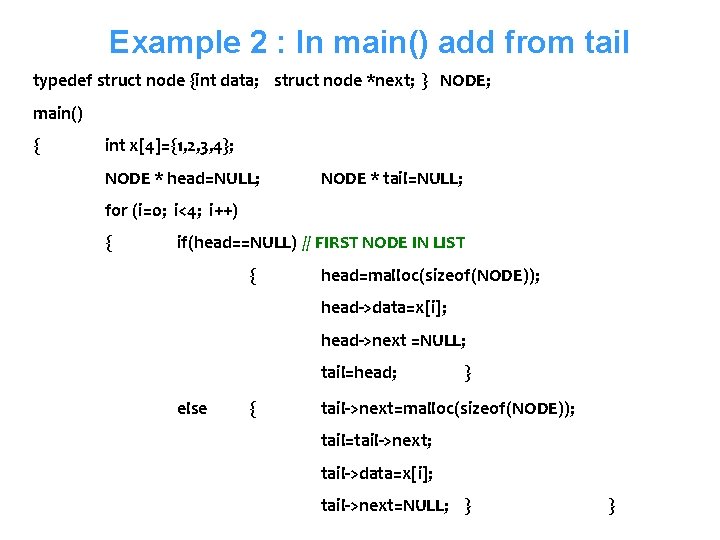
![Example 3 : In function ( Using Iteration) NODE * create_ite (int x[] , Example 3 : In function ( Using Iteration) NODE * create_ite (int x[] ,](https://slidetodoc.com/presentation_image_h/d29d592ab00a360a0e5f9252f2f2245d/image-15.jpg)
![Example 4 : In function (Using Recursion) NODE * create _rec(int x[], int size) Example 4 : In function (Using Recursion) NODE * create _rec(int x[], int size)](https://slidetodoc.com/presentation_image_h/d29d592ab00a360a0e5f9252f2f2245d/image-16.jpg)
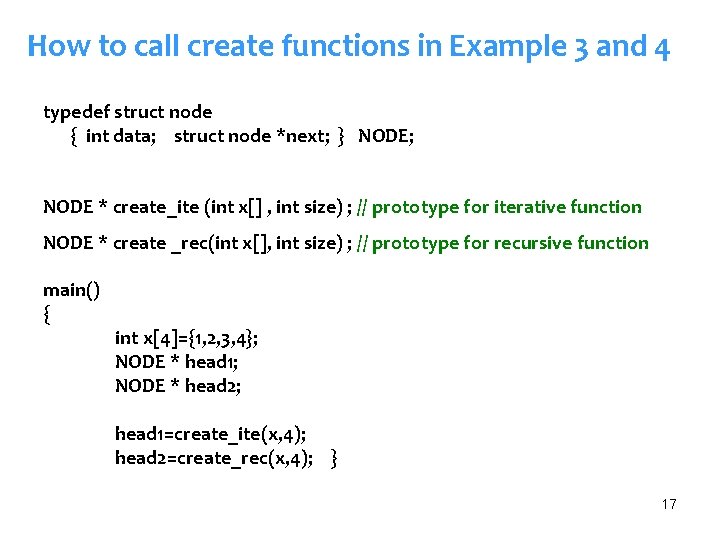
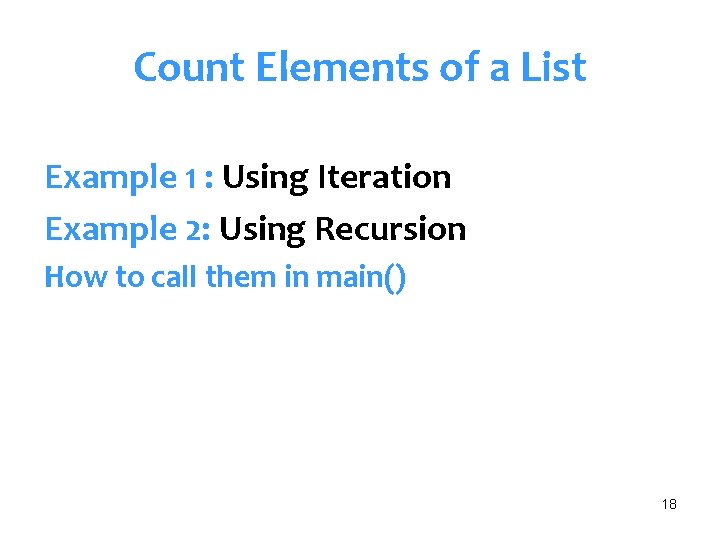
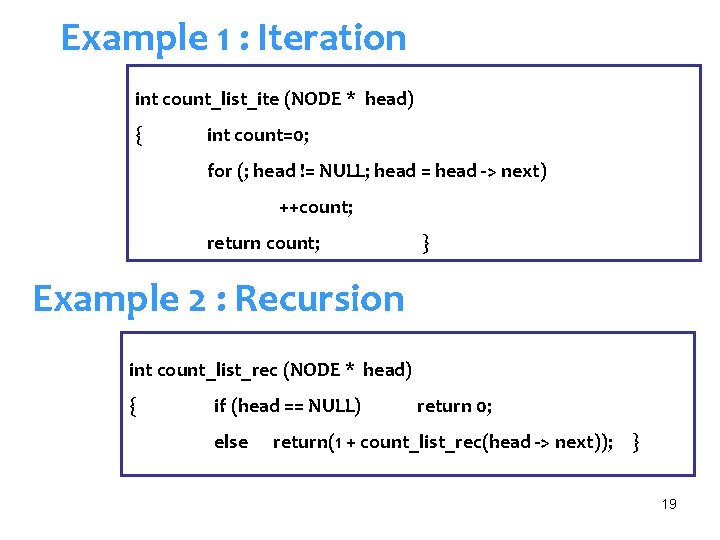
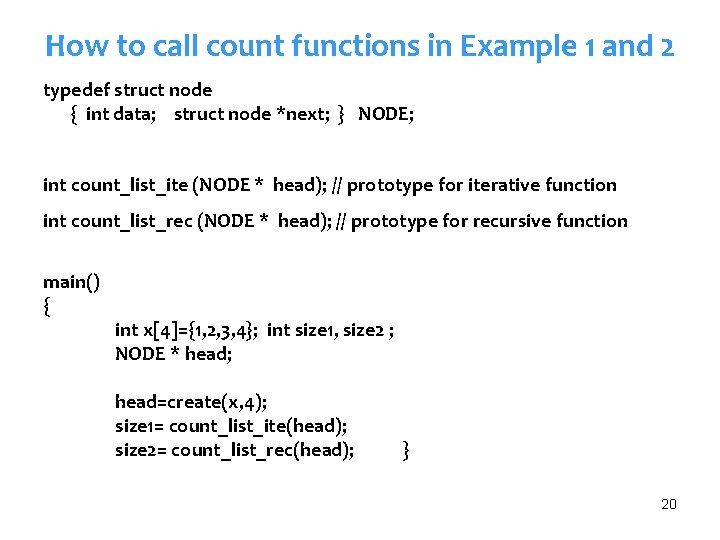
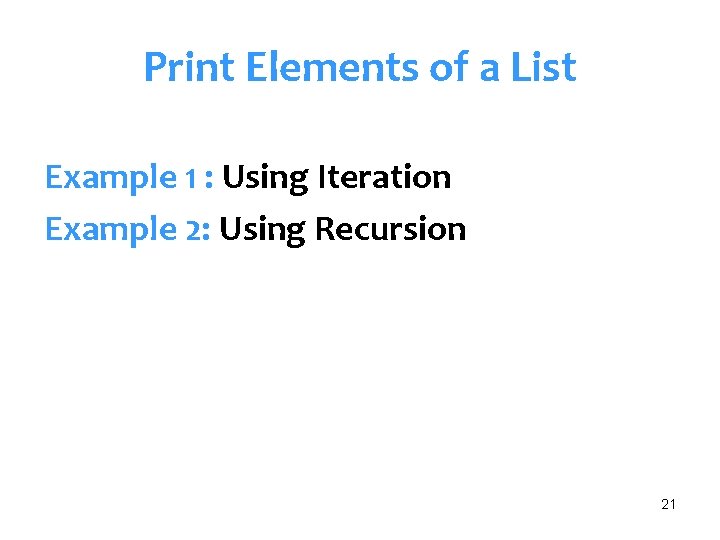
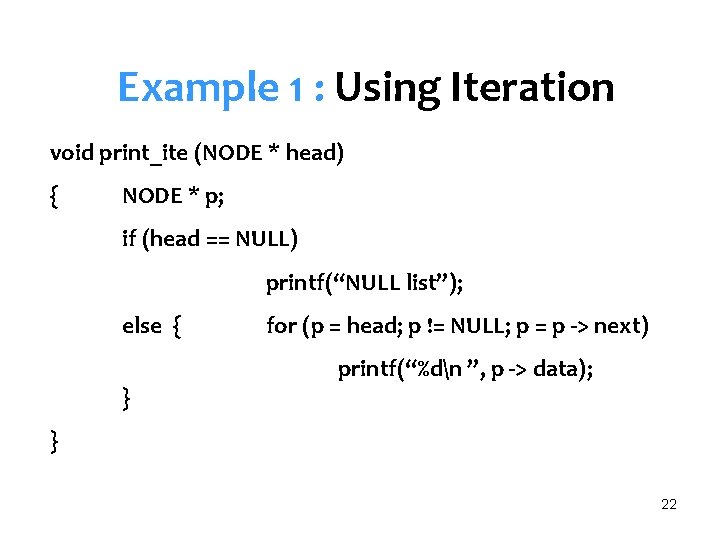
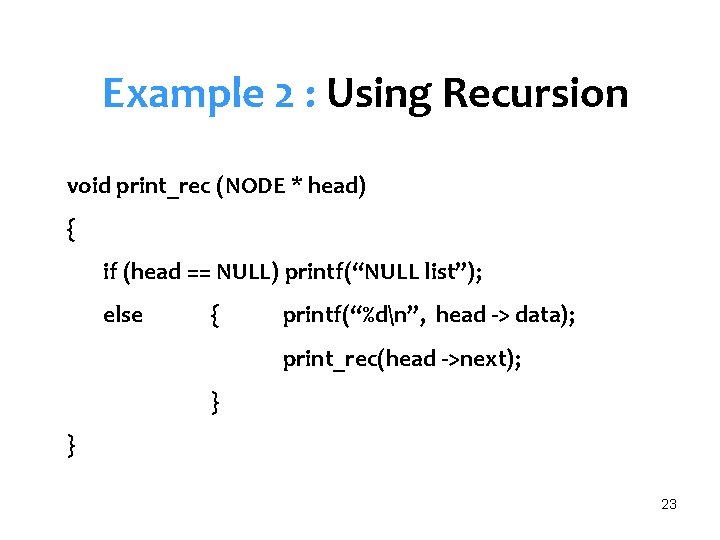
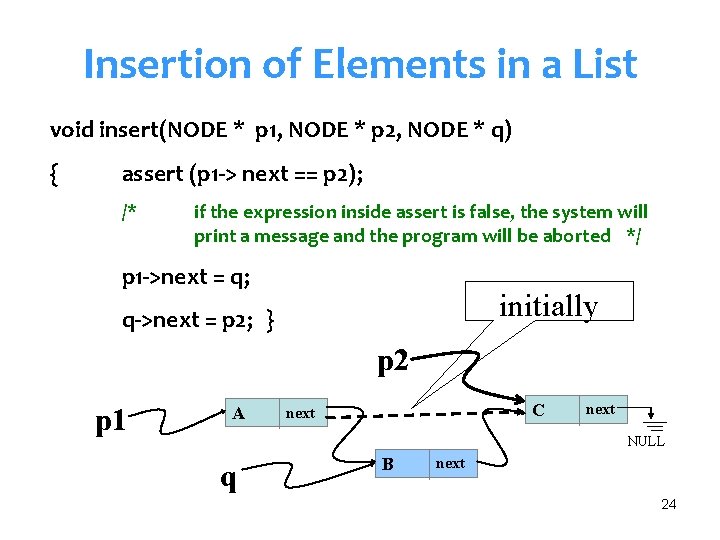
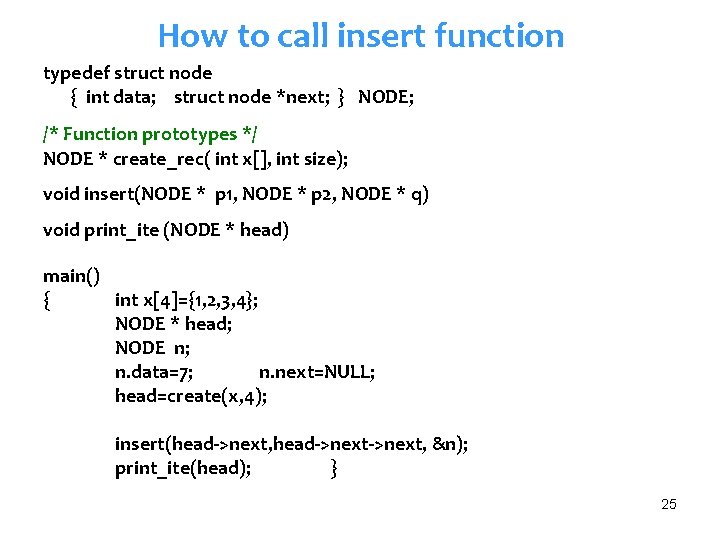
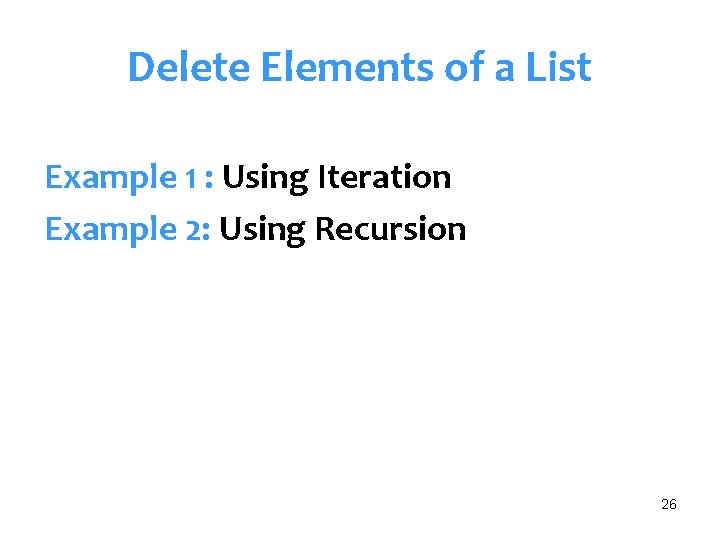
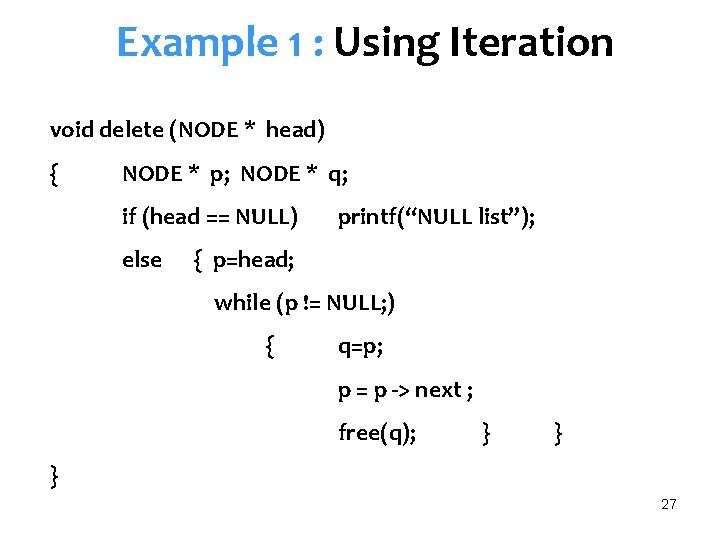
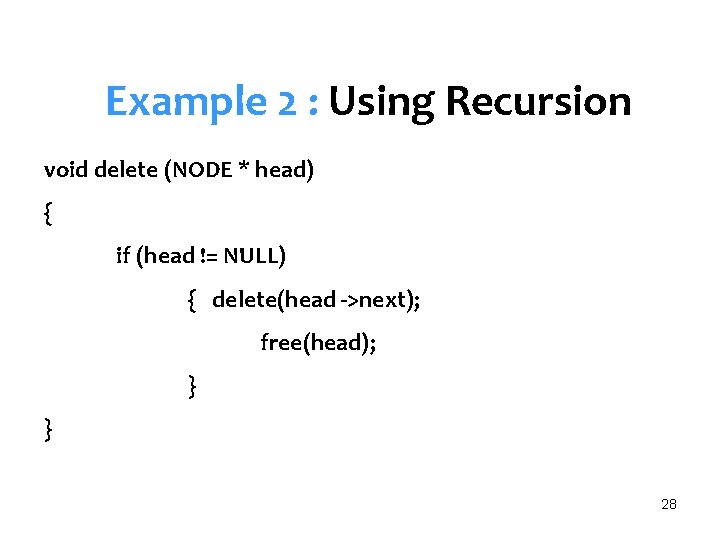
- Slides: 28
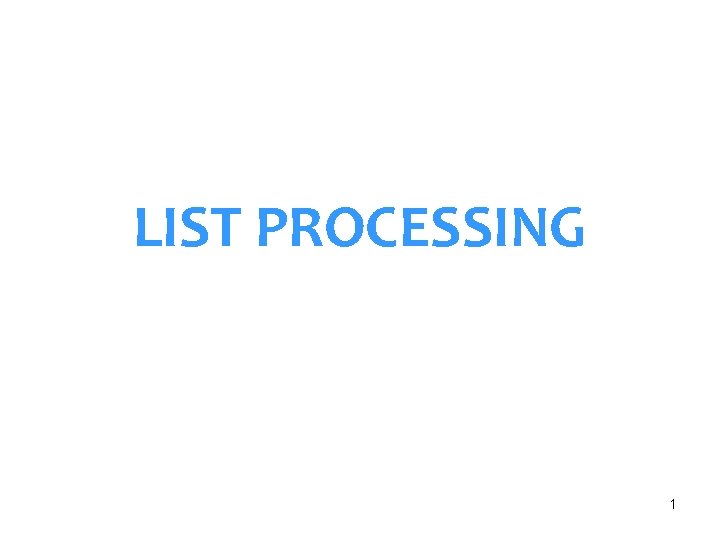
LIST PROCESSING 1
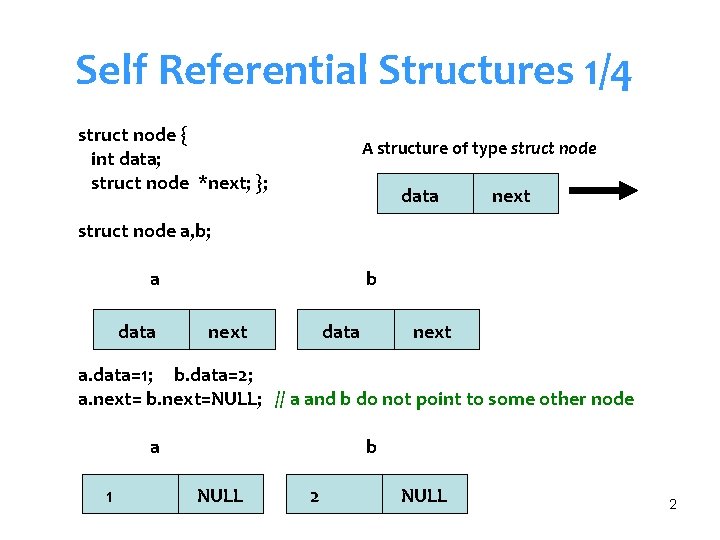
Self Referential Structures 1/4 struct node { int data; struct node *next; }; A structure of type struct node data next struct node a, b; a data b next data next a. data=1; b. data=2; a. next= b. next=NULL; // a and b do not point to some other node a 1 b NULL 2 Kumova Metin NULL Senem 2
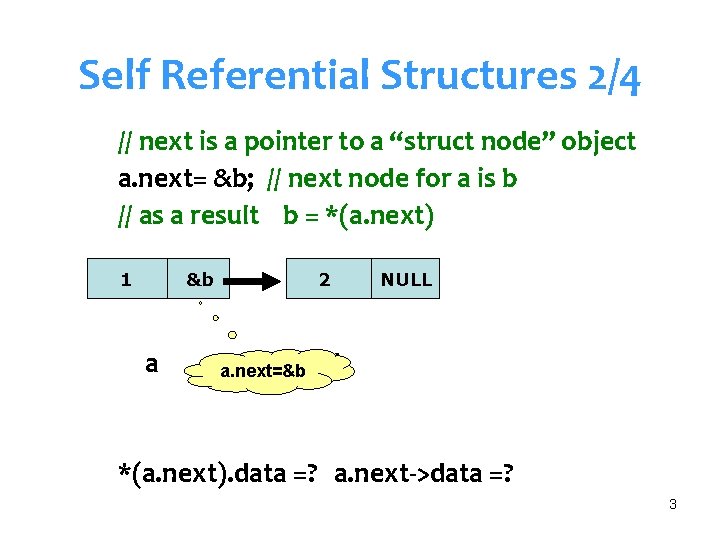
Self Referential Structures 2/4 // next is a pointer to a “struct node” object a. next= &b; // next node for a is b // as a result b = *(a. next) 1 &b a 2 a. next=&b NULL b *(a. next). data =? a. next->data =? 3
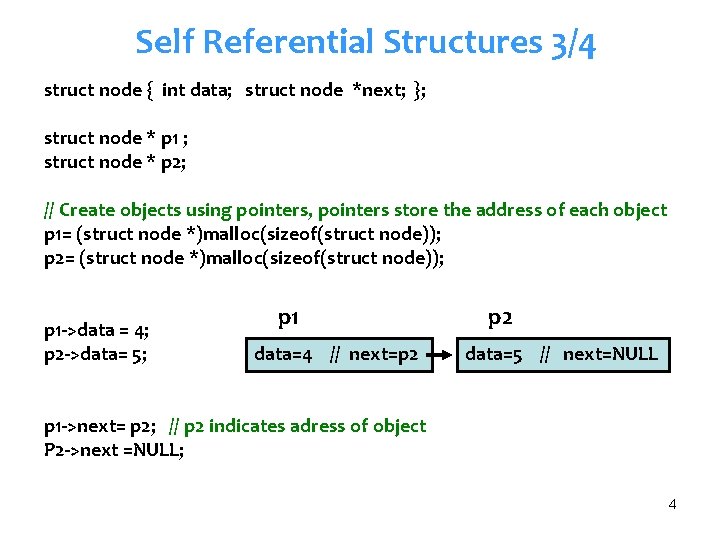
Self Referential Structures 3/4 struct node { int data; struct node *next; }; struct node * p 1 ; struct node * p 2; // Create objects using pointers, pointers store the address of each object p 1= (struct node *)malloc(sizeof(struct node)); p 2= (struct node *)malloc(sizeof(struct node)); p 1 ->data = 4; p 2 ->data= 5; p 1 data=4 // next=p 2 data=5 // next=NULL p 1 ->next= p 2; // p 2 indicates adress of object P 2 ->next =NULL; 4
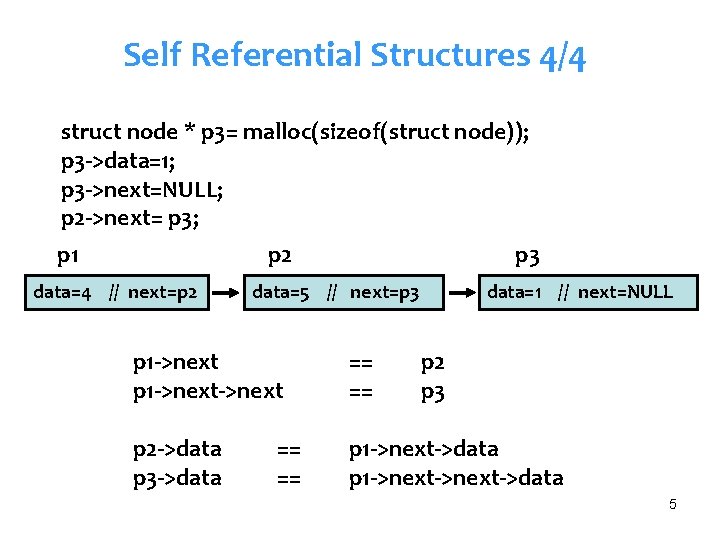
Self Referential Structures 4/4 struct node * p 3= malloc(sizeof(struct node)); p 3 ->data=1; p 3 ->next=NULL; p 2 ->next= p 3; p 1 p 2 data=4 // next=p 2 p 3 data=1 // next=NULL data=5 // next=p 3 p 1 ->next->next == == p 2 ->data p 3 ->data p 1 ->next->next->data == == p 2 p 3 5
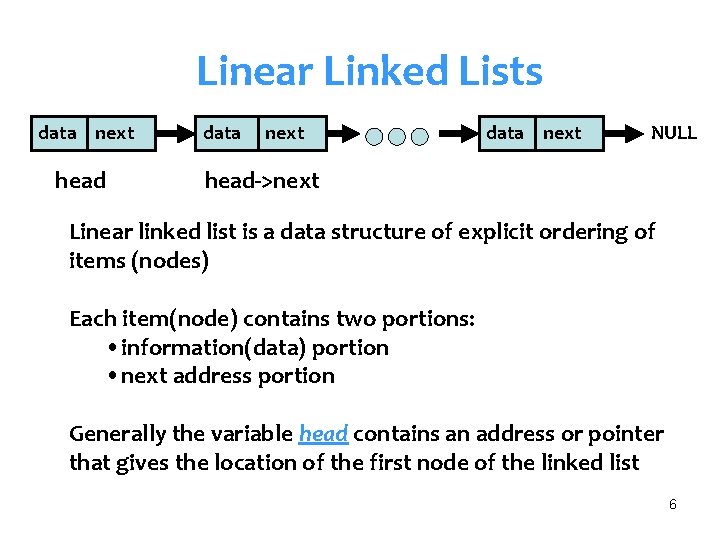
Linear Linked Lists data next head data next NULL head->next Linear linked list is a data structure of explicit ordering of items (nodes) Each item(node) contains two portions: • information(data) portion • next address portion Generally the variable head contains an address or pointer that gives the location of the first node of the linked list 6
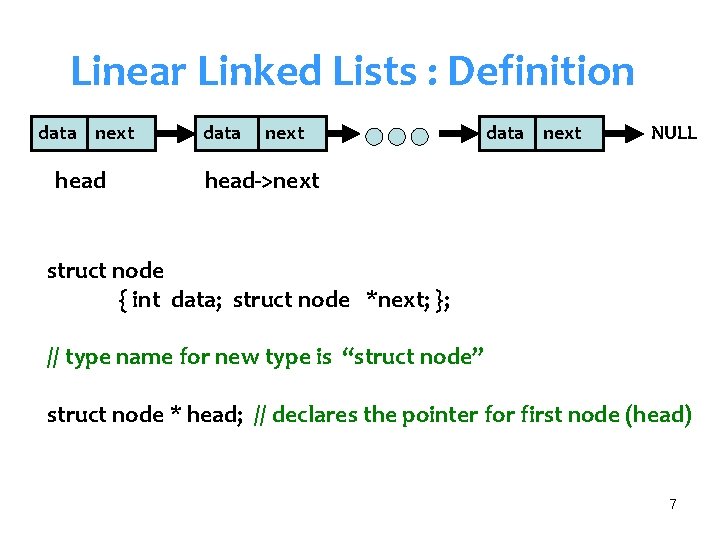
Linear Linked Lists : Definition data next head data next NULL head->next struct node { int data; struct node *next; }; // type name for new type is “struct node” struct node * head; // declares the pointer for first node (head) 7
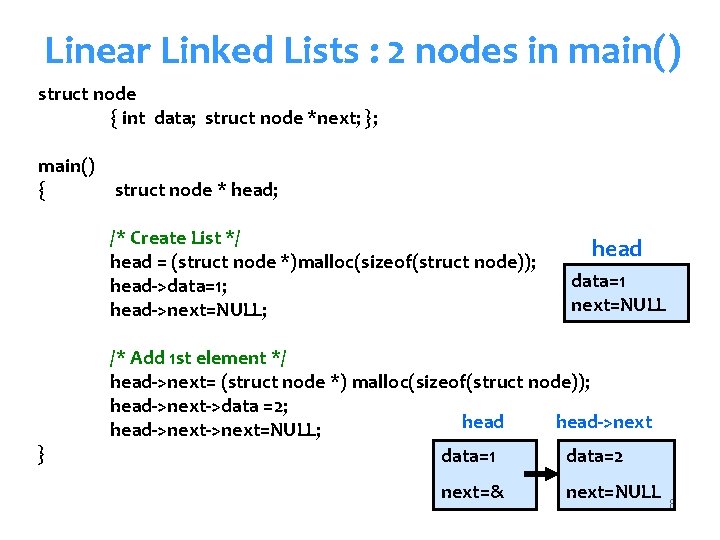
Linear Linked Lists : 2 nodes in main() struct node { int data; struct node *next; }; main() { struct node * head; /* Create List */ head = (struct node *)malloc(sizeof(struct node)); head->data=1; head->next=NULL; } head data=1 next=NULL /* Add 1 st element */ head->next= (struct node *) malloc(sizeof(struct node)); head->next->data =2; head->next=NULL; data=1 data=2 next=& next=NULL 8
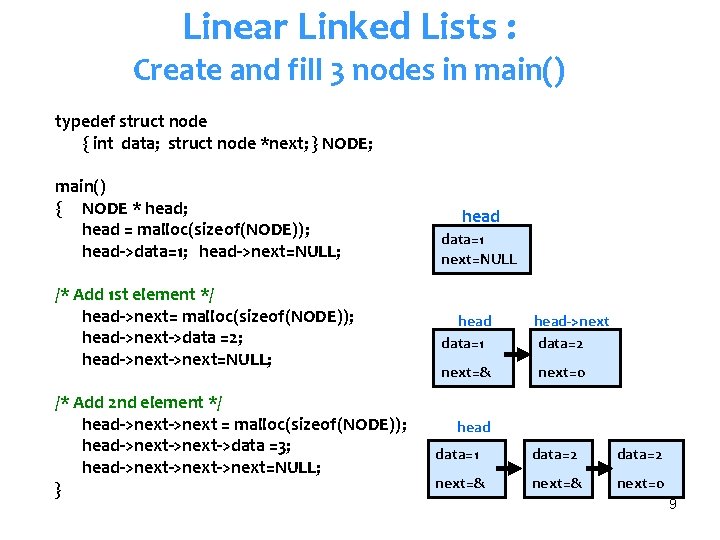
Linear Linked Lists : Create and fill 3 nodes in main() typedef struct node { int data; struct node *next; } NODE; main() { NODE * head; head = malloc(sizeof(NODE)); head->data=1; head->next=NULL; /* Add 1 st element */ head->next= malloc(sizeof(NODE)); head->next->data =2; head->next=NULL; /* Add 2 nd element */ head->next = malloc(sizeof(NODE)); head->next->data =3; head->next->next=NULL; } head data=1 next=NULL head data=1 head->next data=2 next=& next=0 head data=1 data=2 next=& next=0 9
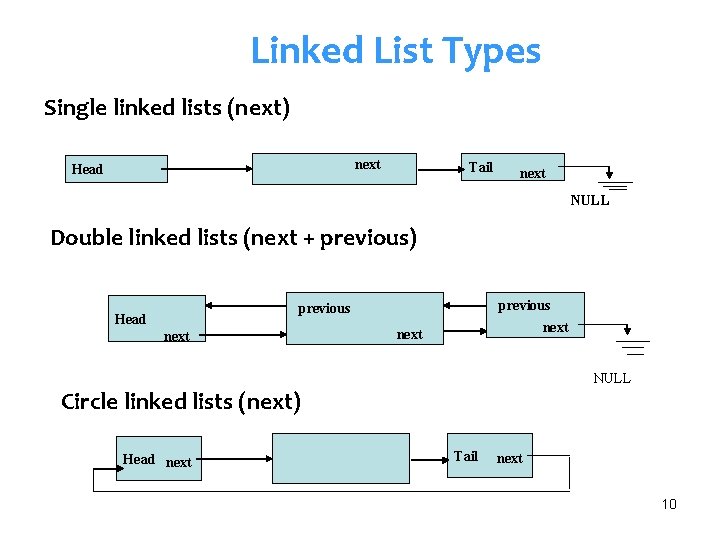
Linked List Types Single linked lists (next) next Head Tail next NULL Double linked lists (next + previous) previous Head next NULL Circle linked lists (next) Head next Tail next 10
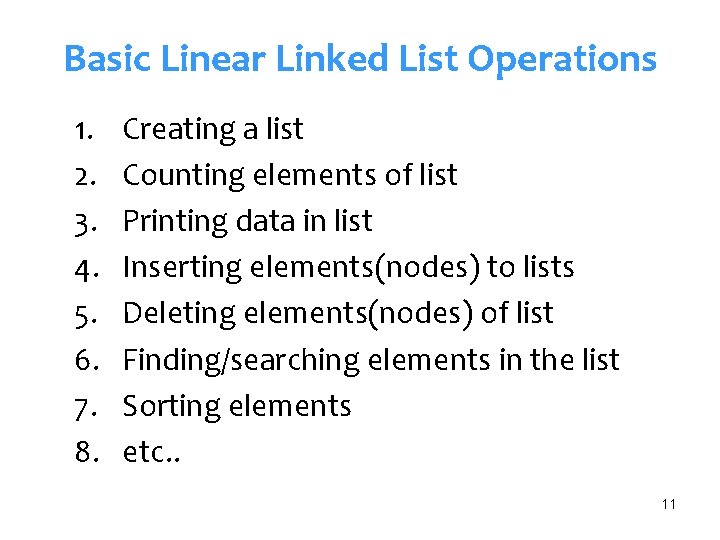
Basic Linear Linked List Operations 1. 2. 3. 4. 5. 6. 7. 8. Creating a list Counting elements of list Printing data in list Inserting elements(nodes) to lists Deleting elements(nodes) of list Finding/searching elements in the list Sorting elements etc. . 11
![CREATING LINEAR LINKED LIST An integer array x4 will be used to create a CREATING LINEAR LINKED LIST An integer array (x[4]) will be used to create a](https://slidetodoc.com/presentation_image_h/d29d592ab00a360a0e5f9252f2f2245d/image-12.jpg)
CREATING LINEAR LINKED LIST An integer array (x[4]) will be used to create a list. Example 1 : In main() add from head Example 2 : In main() add from tail Example 3 : In function ( Using Iteration) Example 4 : In function ( Using Recursion) How to call functions in Example 3 and 4 12
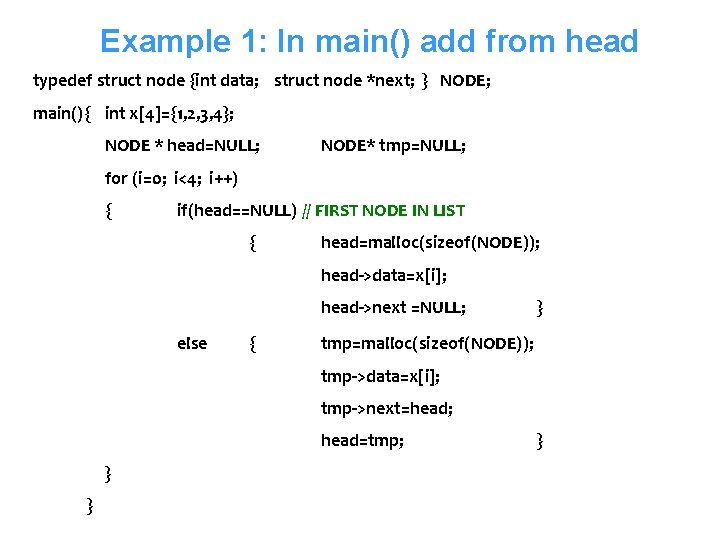
Example 1: In main() add from head typedef struct node {int data; struct node *next; } NODE; main(){ int x[4]={1, 2, 3, 4}; NODE * head=NULL; NODE* tmp=NULL; for (i=0; i<4; i++) { if(head==NULL) // FIRST NODE IN LIST { head=malloc(sizeof(NODE)); head->data=x[i]; head->next =NULL; else { } tmp=malloc(sizeof(NODE)); tmp->data=x[i]; tmp->next=head; head=tmp; } } } Senem Kumova Metin 13
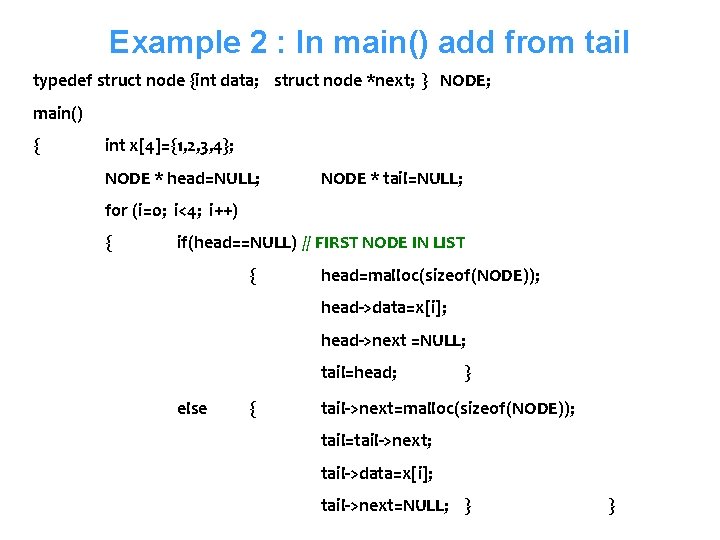
Example 2 : In main() add from tail typedef struct node {int data; struct node *next; } NODE; main() { int x[4]={1, 2, 3, 4}; NODE * head=NULL; NODE * tail=NULL; for (i=0; i<4; i++) { if(head==NULL) // FIRST NODE IN LIST { head=malloc(sizeof(NODE)); head->data=x[i]; head->next =NULL; tail=head; else { } tail->next=malloc(sizeof(NODE)); tail=tail->next; tail->data=x[i]; Senem Kumova Metin tail->next=NULL; } } 14
![Example 3 In function Using Iteration NODE createite int x Example 3 : In function ( Using Iteration) NODE * create_ite (int x[] ,](https://slidetodoc.com/presentation_image_h/d29d592ab00a360a0e5f9252f2f2245d/image-15.jpg)
Example 3 : In function ( Using Iteration) NODE * create_ite (int x[] , int size) { NODE * head = NULL; NODE * tail =NULL; int i; if(size!=0) { head = malloc(sizeof(NODE)); head -> data = x[0]; tail = head; for (i = 1; i<size; ++i) { /* add to tail */ tail -> next = malloc(sizeof(NODE)); tail = tail -> next; tail -> data = x[i]; } tail -> next = NULL; /* end of list */ } return head; } Senem Kumova Metin 15
![Example 4 In function Using Recursion NODE create recint x int size Example 4 : In function (Using Recursion) NODE * create _rec(int x[], int size)](https://slidetodoc.com/presentation_image_h/d29d592ab00a360a0e5f9252f2f2245d/image-16.jpg)
Example 4 : In function (Using Recursion) NODE * create _rec(int x[], int size) { NODE * head; if (size==0 ) /* base case */ return NULL; else { /* method */ head = malloc(sizeof(NODE)); head -> data = x[0]; head -> next = create_rec(x + 1 , size-1); return head; } } 16
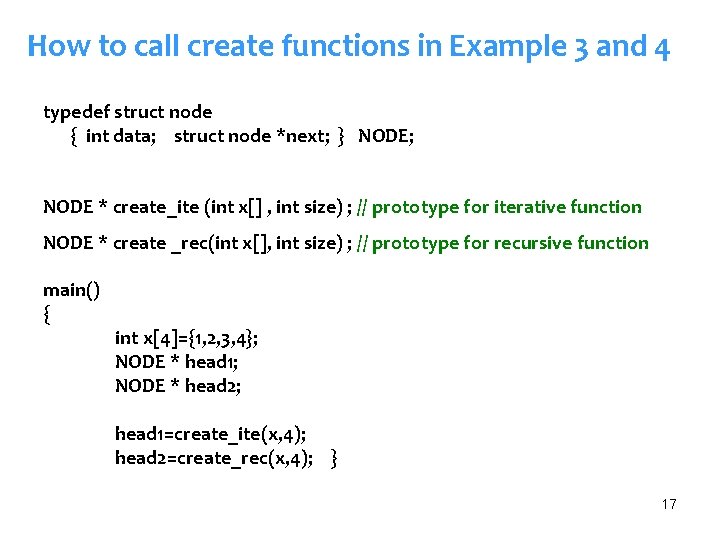
How to call create functions in Example 3 and 4 typedef struct node { int data; struct node *next; } NODE; NODE * create_ite (int x[] , int size) ; // prototype for iterative function NODE * create _rec(int x[], int size) ; // prototype for recursive function main() { int x[4]={1, 2, 3, 4}; NODE * head 1; NODE * head 2; head 1=create_ite(x, 4); head 2=create_rec(x, 4); } 17
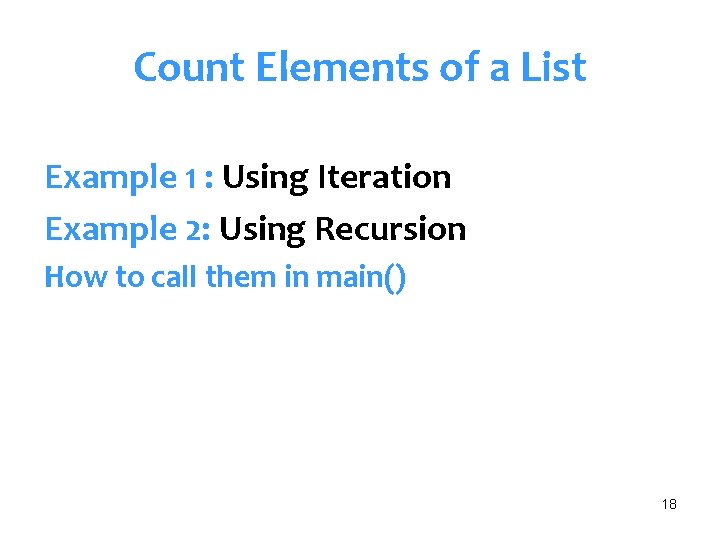
Count Elements of a List Example 1 : Using Iteration Example 2: Using Recursion How to call them in main() 18
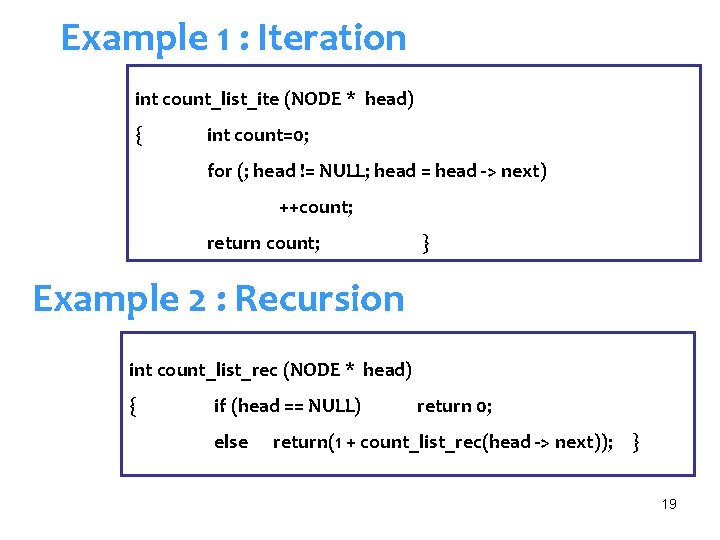
Example 1 : Iteration int count_list_ite (NODE * head) { int count=0; for (; head != NULL; head = head -> next) ++count; return count; } Example 2 : Recursion int count_list_rec (NODE * head) { if (head == NULL) else return 0; return(1 + count_list_rec(head -> next)); } 19
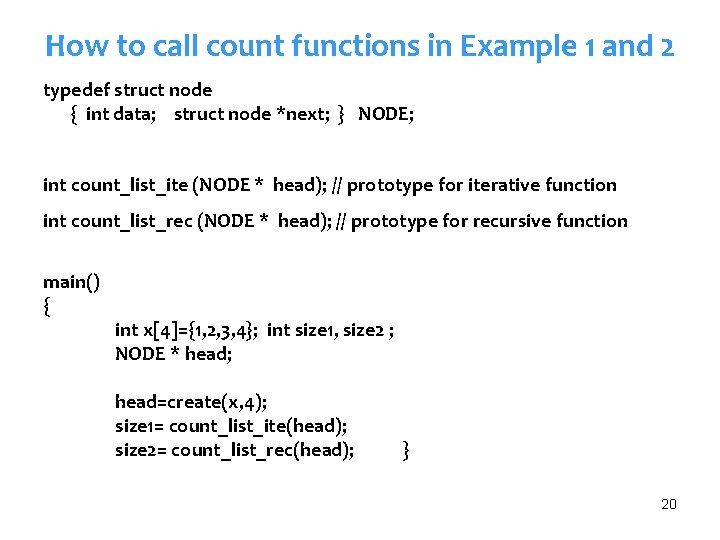
How to call count functions in Example 1 and 2 typedef struct node { int data; struct node *next; } NODE; int count_list_ite (NODE * head); // prototype for iterative function int count_list_rec (NODE * head); // prototype for recursive function main() { int x[4]={1, 2, 3, 4}; int size 1, size 2 ; NODE * head; head=create(x, 4); size 1= count_list_ite(head); size 2= count_list_rec(head); } 20
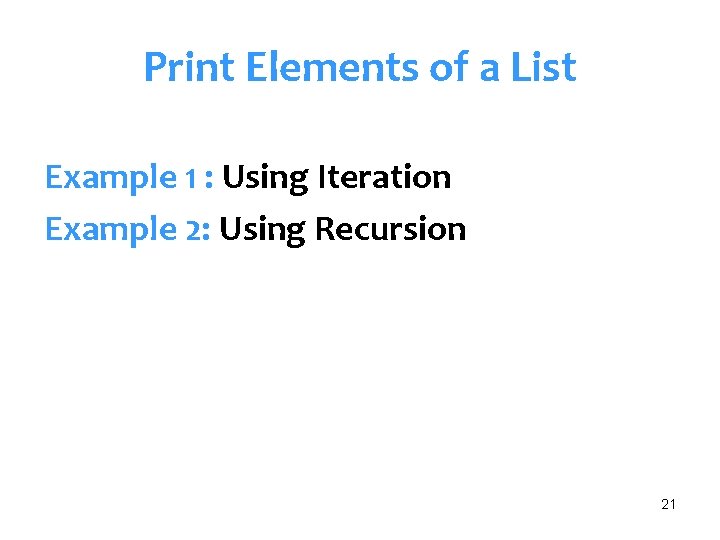
Print Elements of a List Example 1 : Using Iteration Example 2: Using Recursion 21
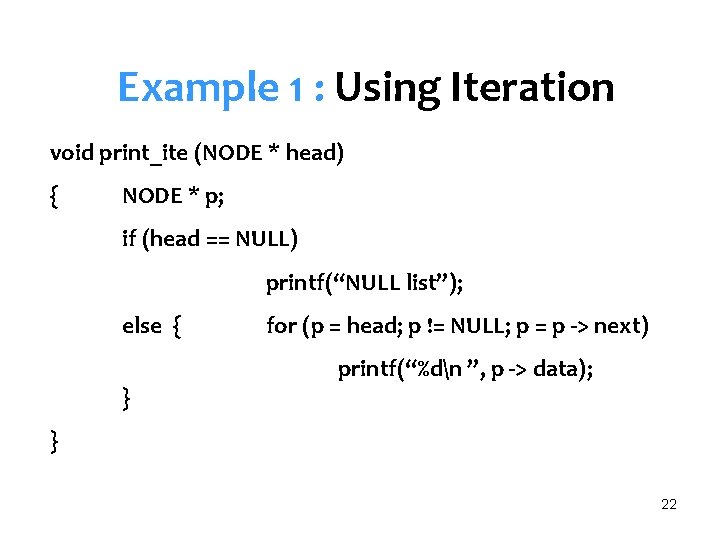
Example 1 : Using Iteration void print_ite (NODE * head) { NODE * p; if (head == NULL) printf(“NULL list”); else { } for (p = head; p != NULL; p = p -> next) printf(“%dn ”, p -> data); } 22
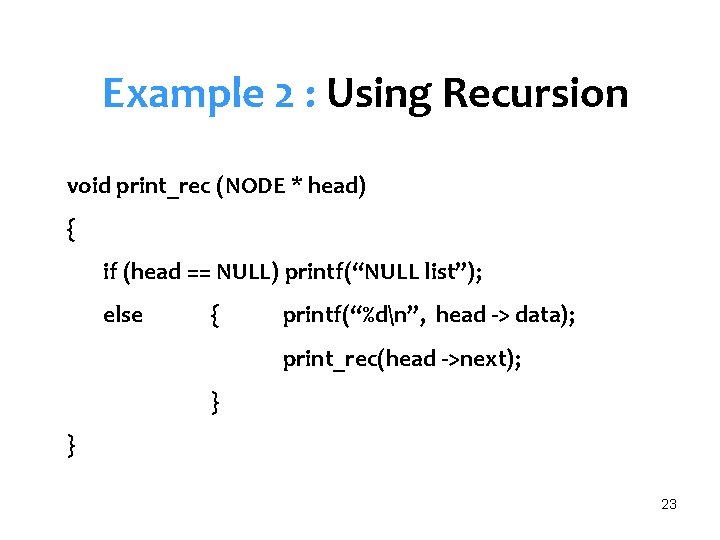
Example 2 : Using Recursion void print_rec (NODE * head) { if (head == NULL) printf(“NULL list”); else { printf(“%dn”, head -> data); print_rec(head ->next); } } 23
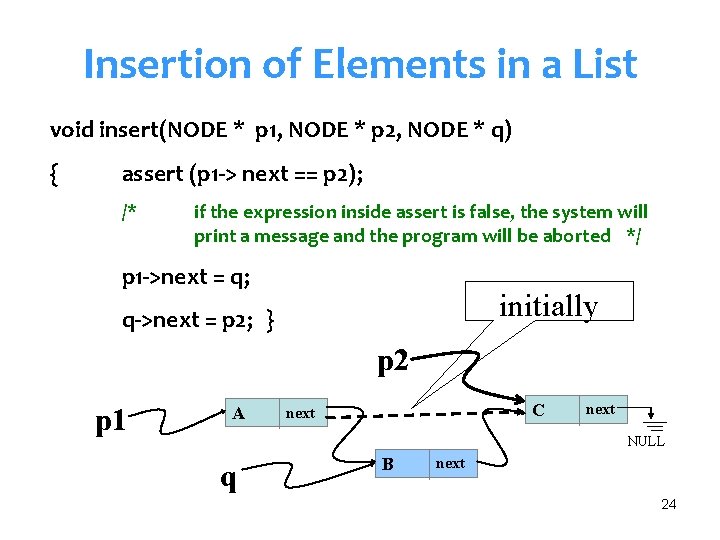
Insertion of Elements in a List void insert(NODE * p 1, NODE * p 2, NODE * q) { assert (p 1 -> next == p 2); /* if the expression inside assert is false, the system will print a message and the program will be aborted */ p 1 ->next = q; initially q->next = p 2; } p 2 p 1 A C next NULL q B next 24
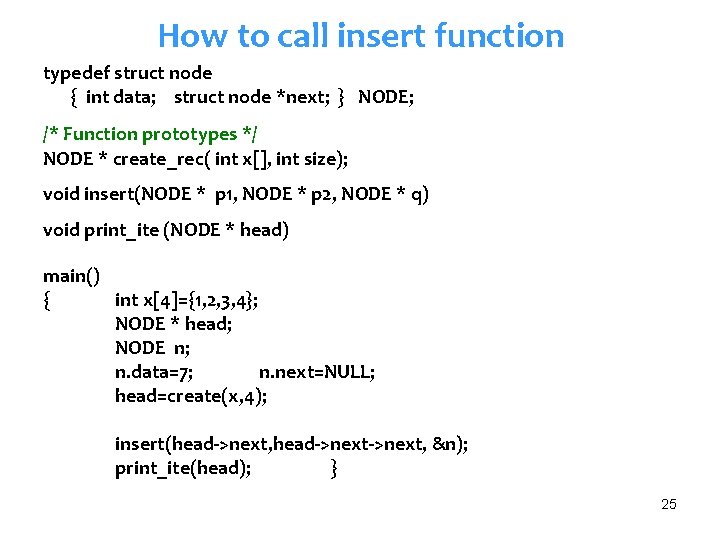
How to call insert function typedef struct node { int data; struct node *next; } NODE; /* Function prototypes */ NODE * create_rec( int x[], int size); void insert(NODE * p 1, NODE * p 2, NODE * q) void print_ite (NODE * head) main() { int x[4]={1, 2, 3, 4}; NODE * head; NODE n; n. data=7; n. next=NULL; head=create(x, 4); insert(head->next, &n); print_ite(head); } 25
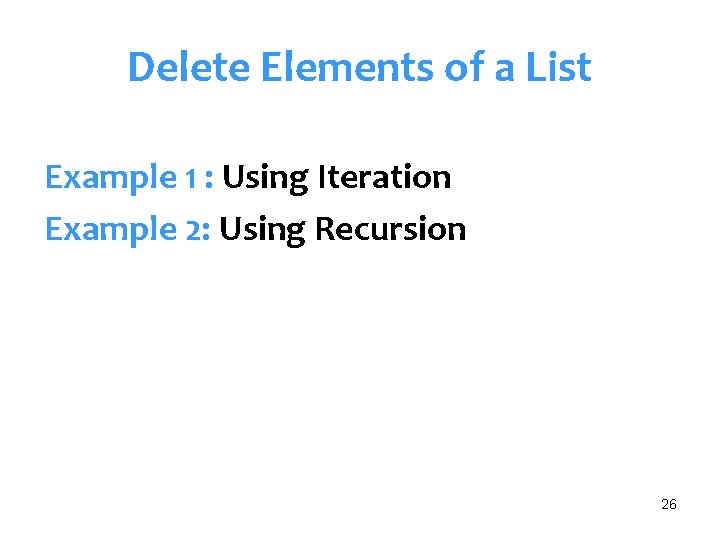
Delete Elements of a List Example 1 : Using Iteration Example 2: Using Recursion 26
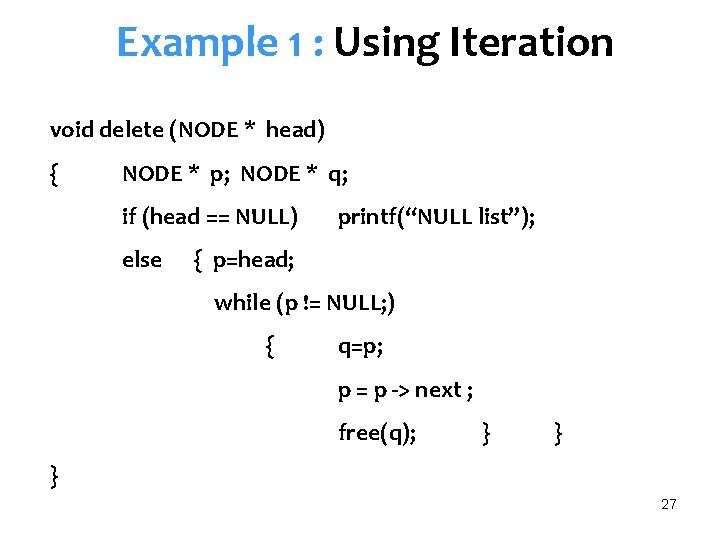
Example 1 : Using Iteration void delete (NODE * head) { NODE * p; NODE * q; if (head == NULL) else printf(“NULL list”); { p=head; while (p != NULL; ) { q=p; p = p -> next ; free(q); } } } 27
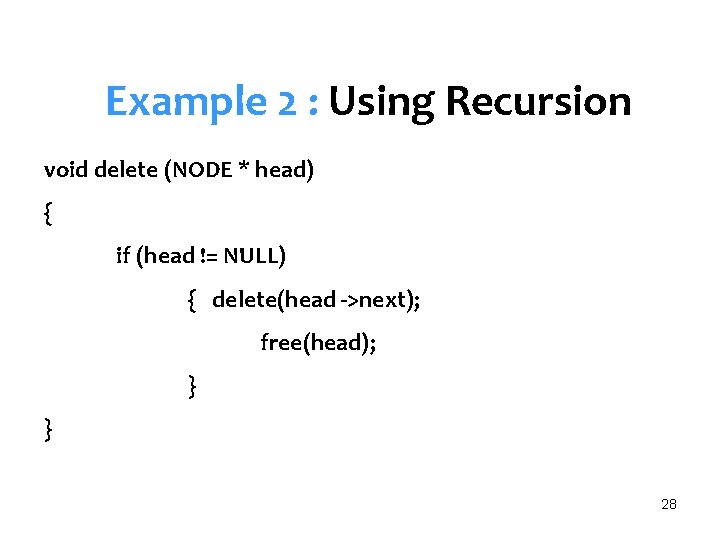
Example 2 : Using Recursion void delete (NODE * head) { if (head != NULL) { delete(head ->next); free(head); } } 28