Linked Lists 2004 Goodrich Tamassia Linked Lists 1
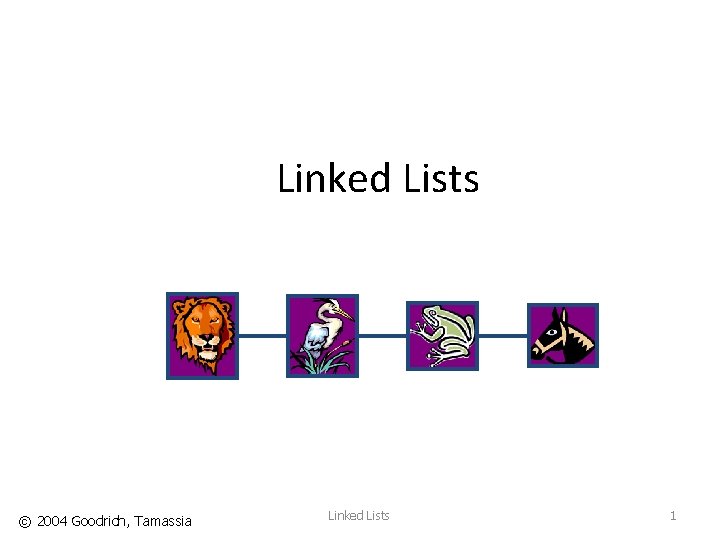
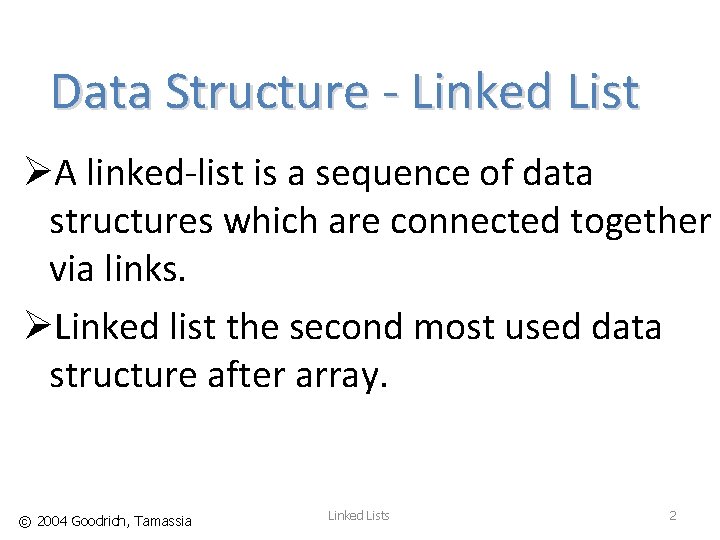
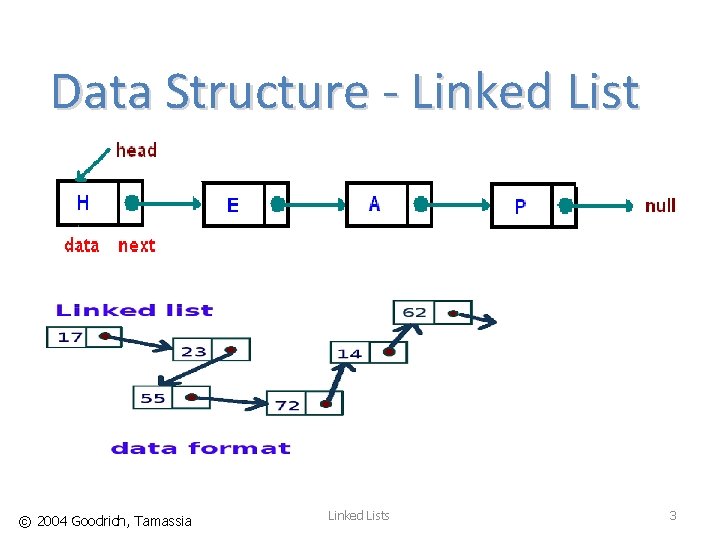
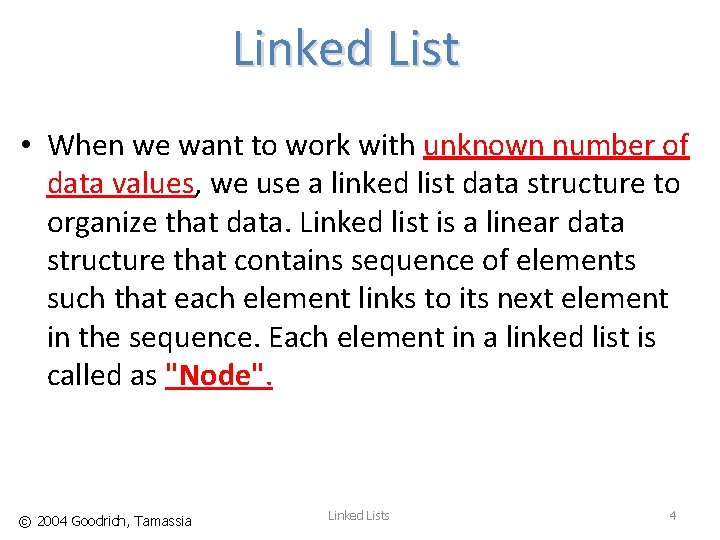
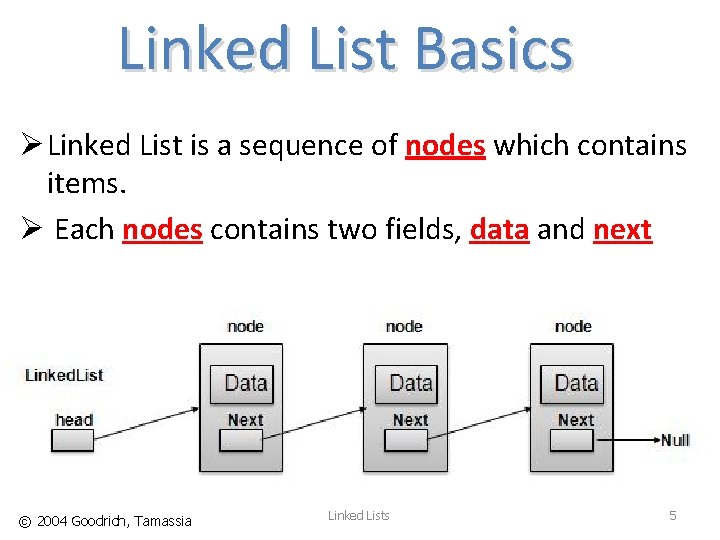
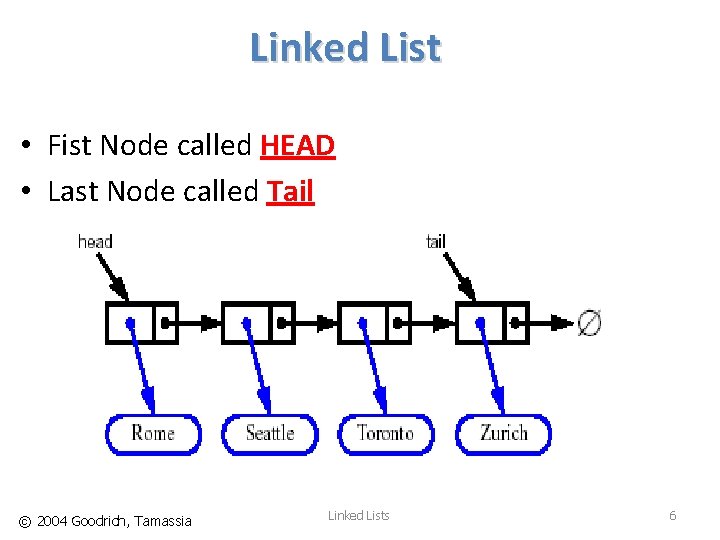
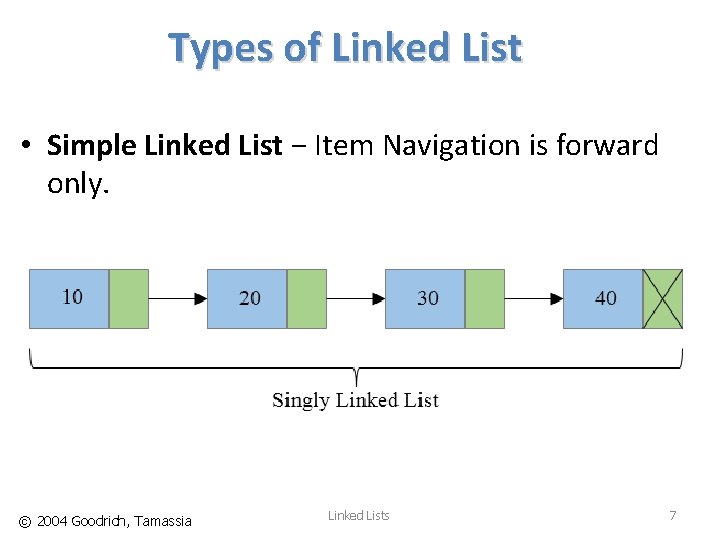
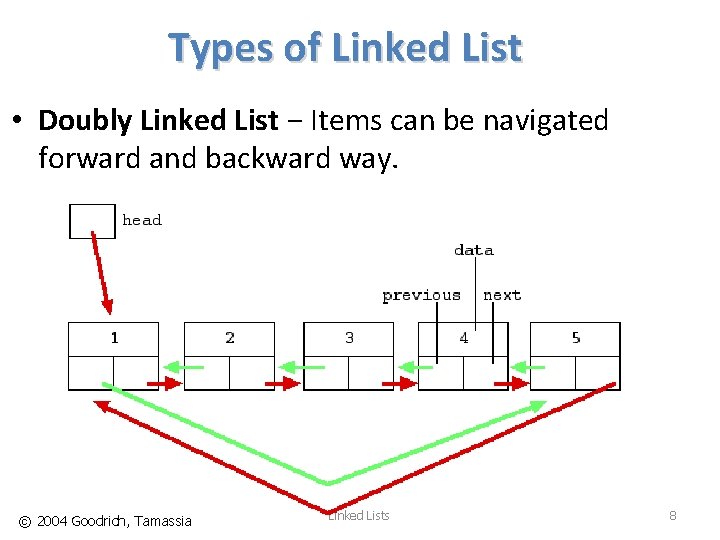
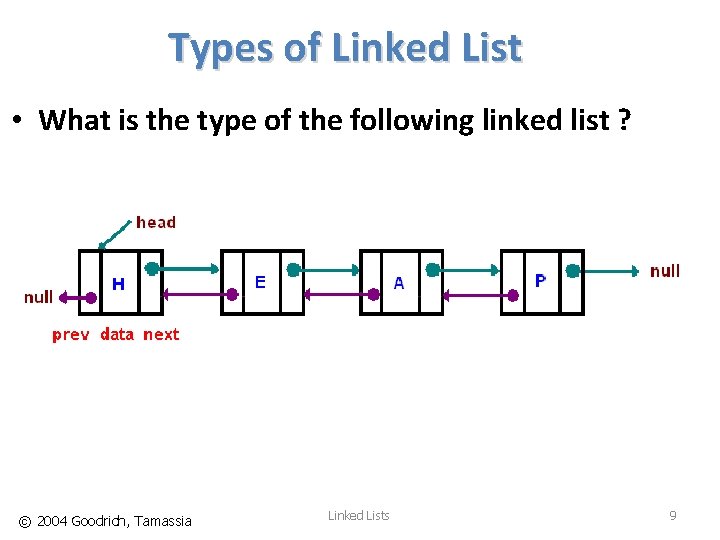
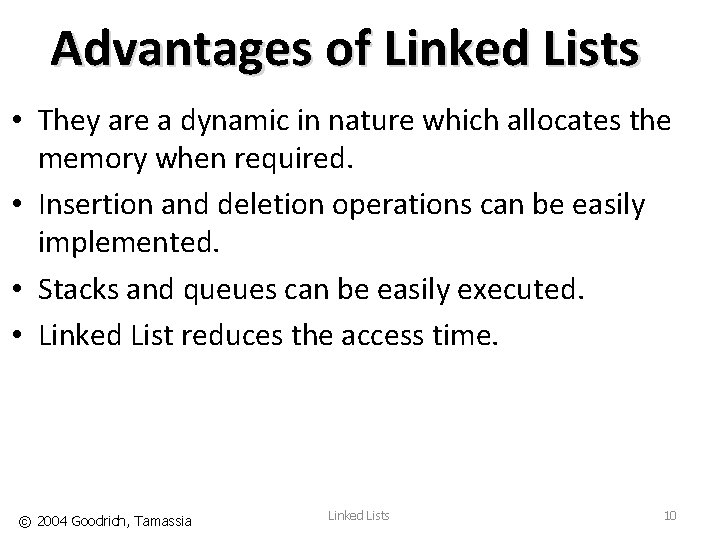
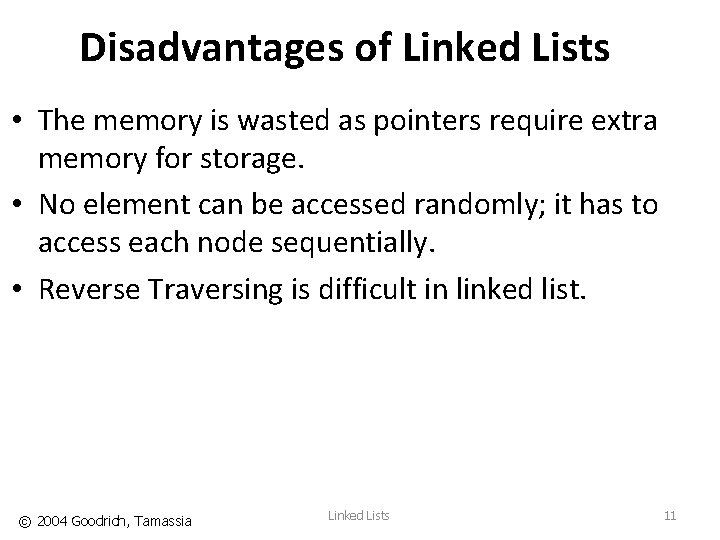
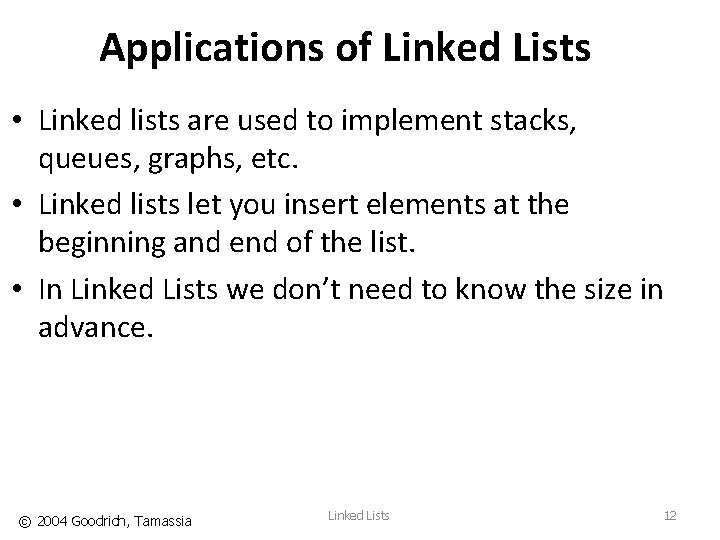
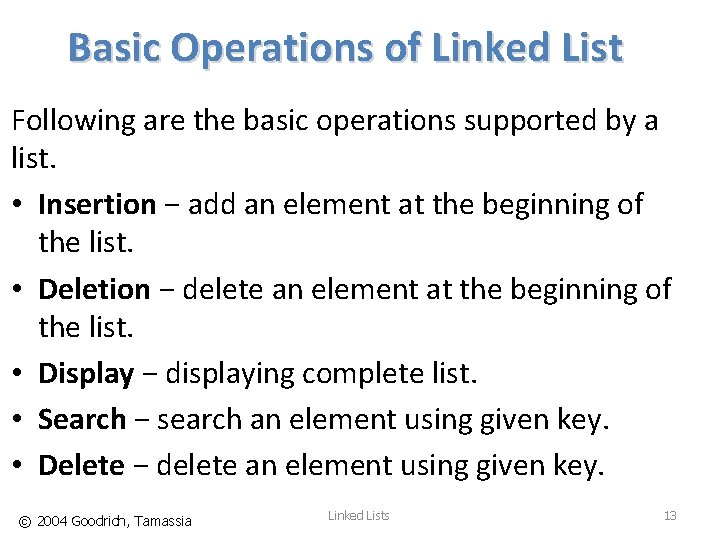
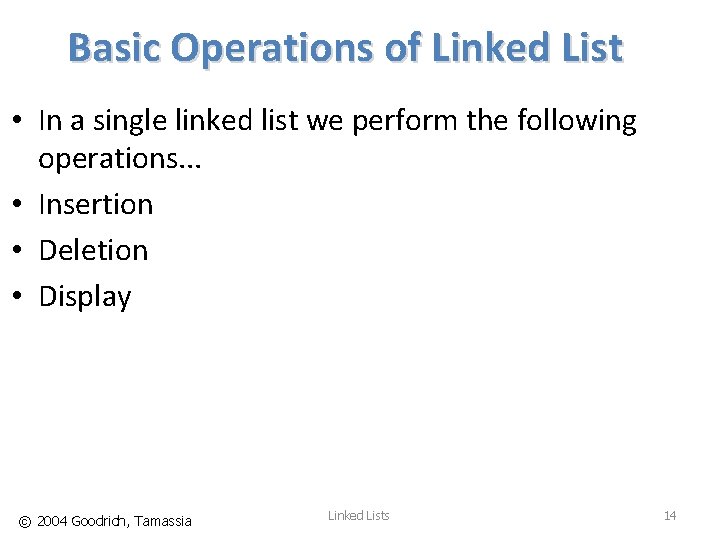
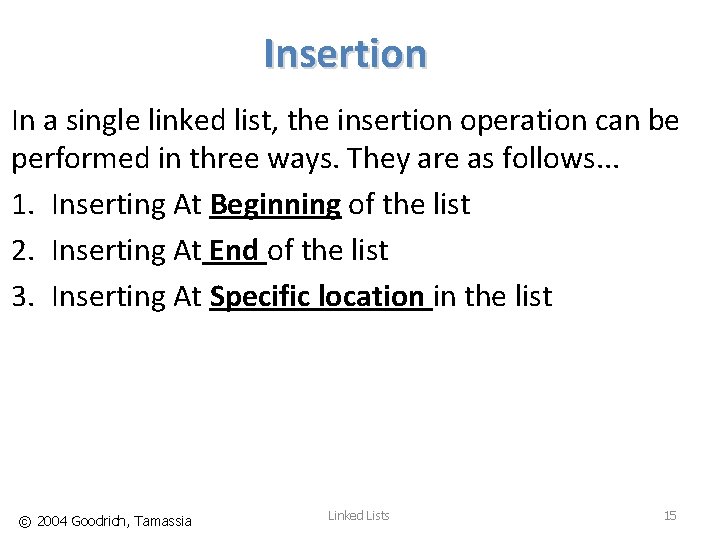
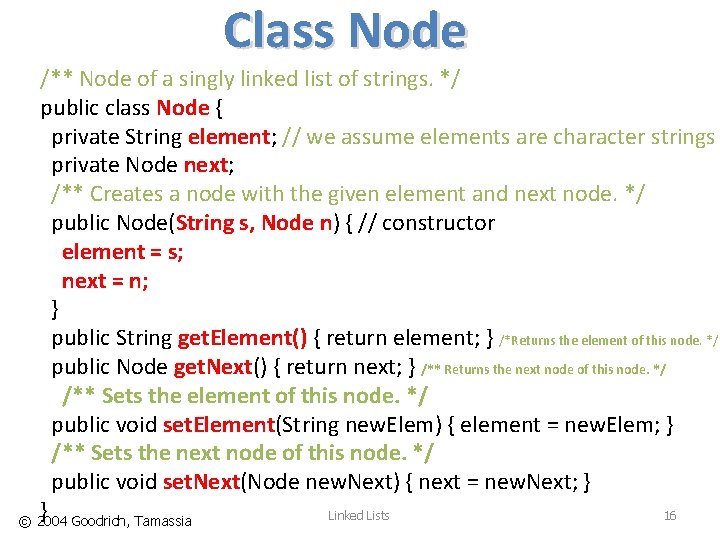
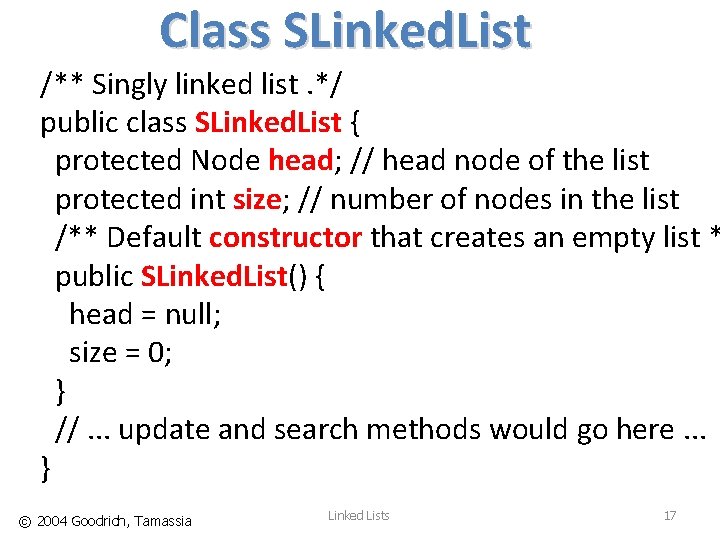
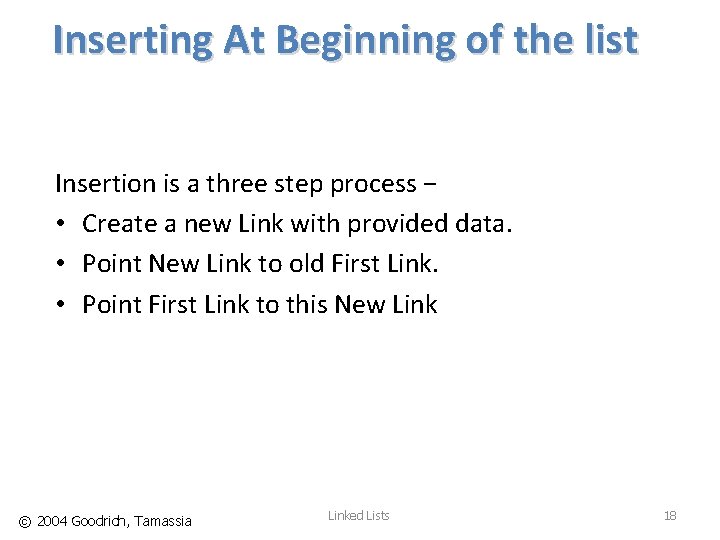
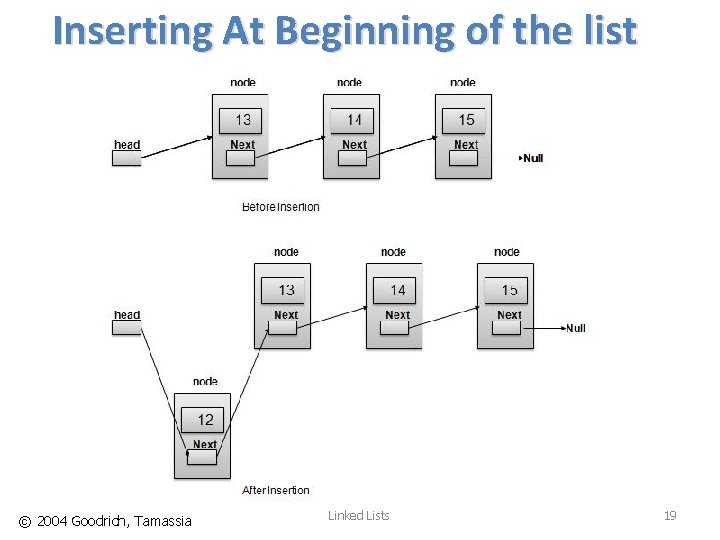
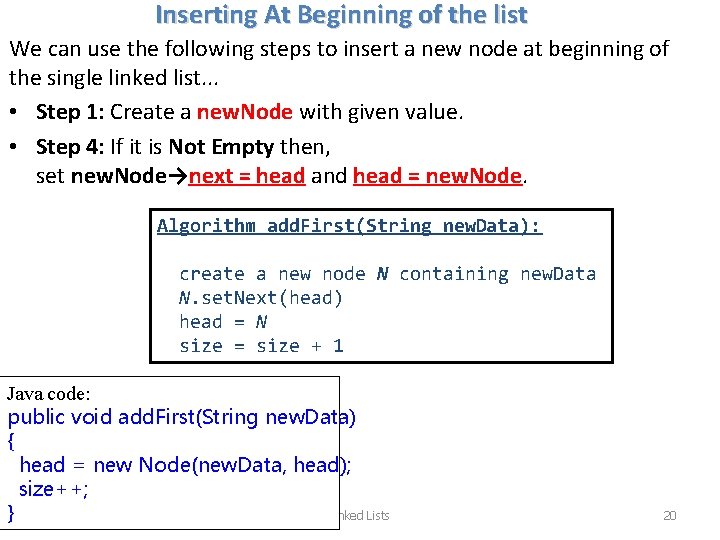
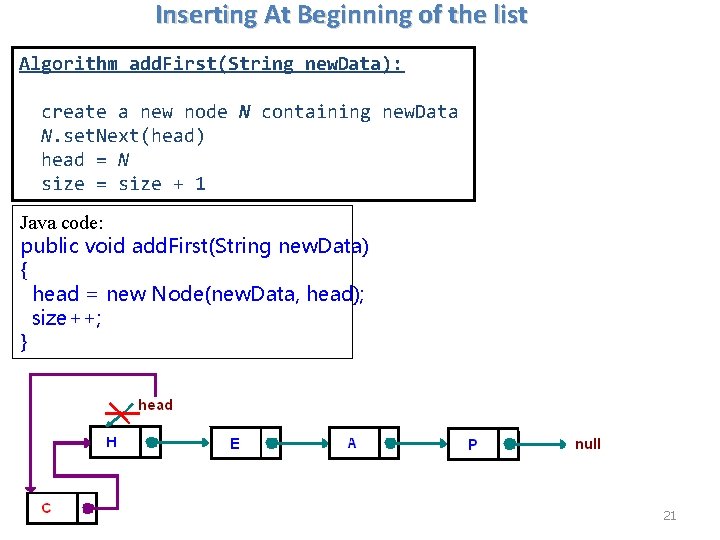
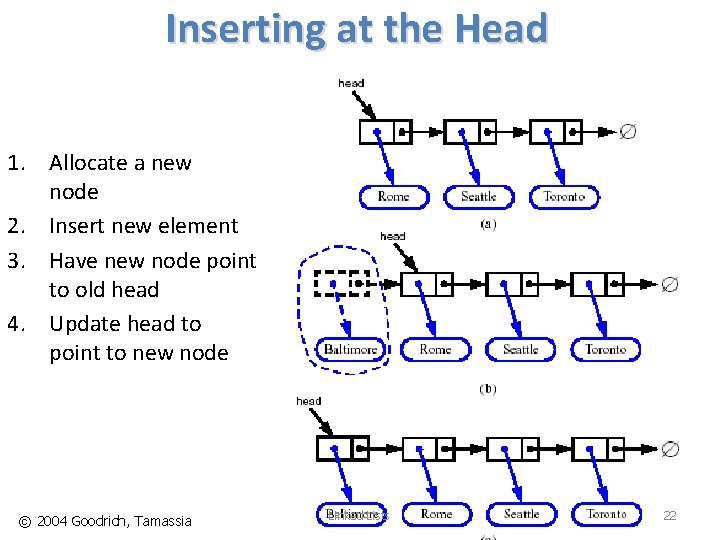
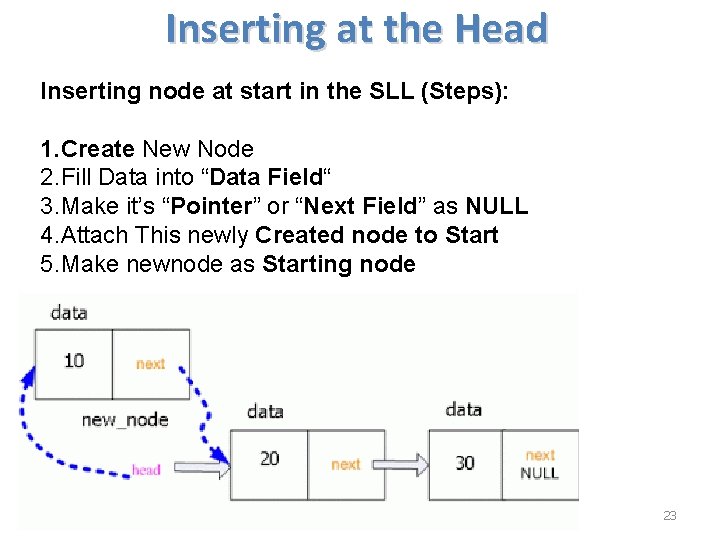
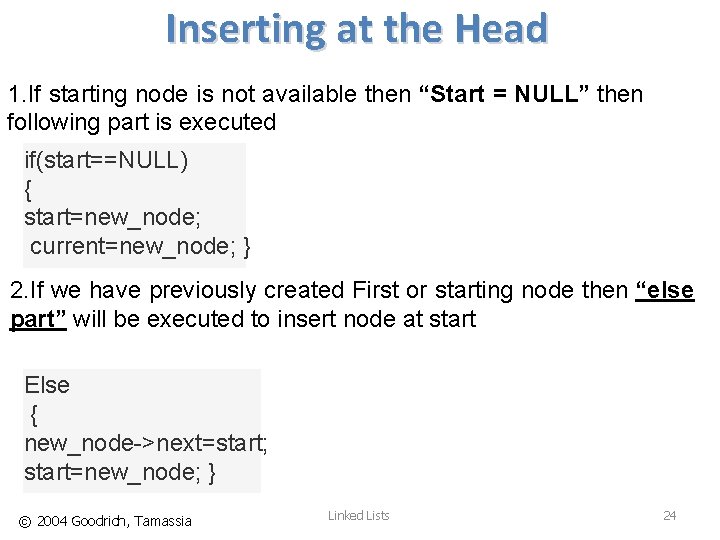
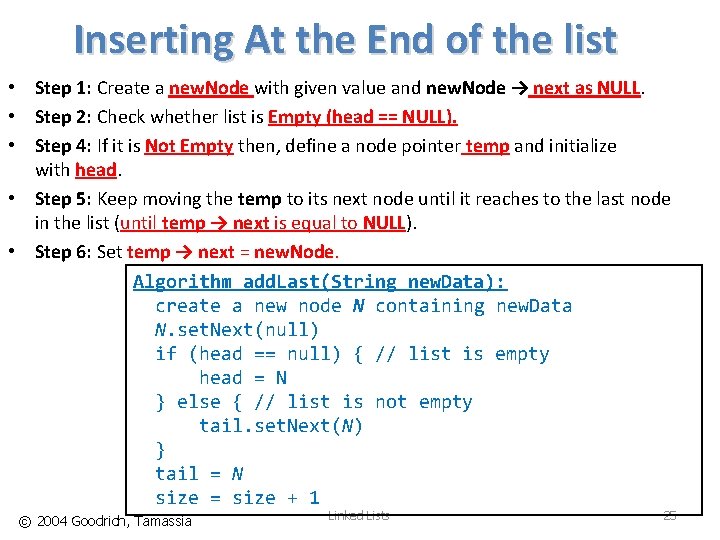
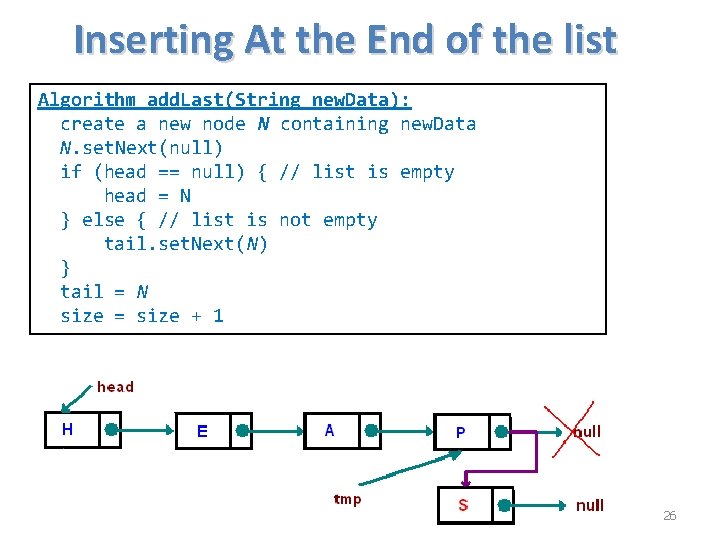
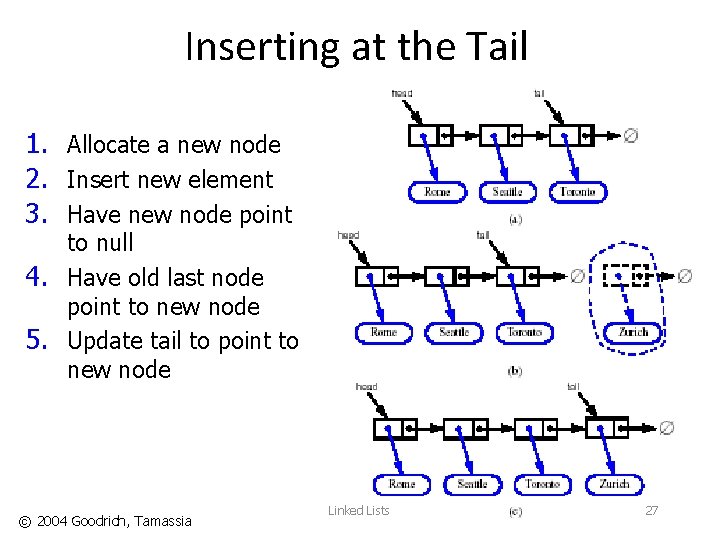
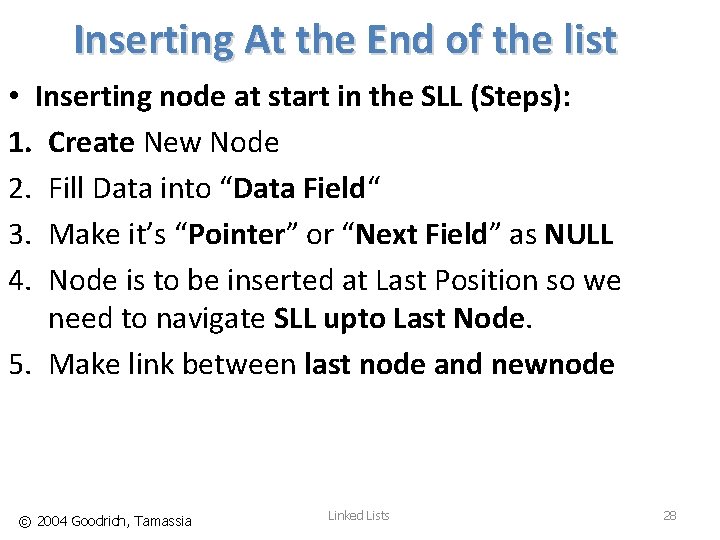
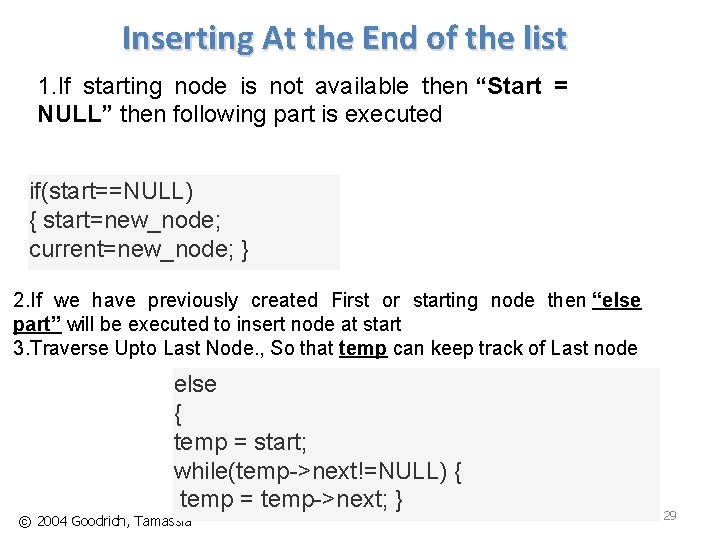
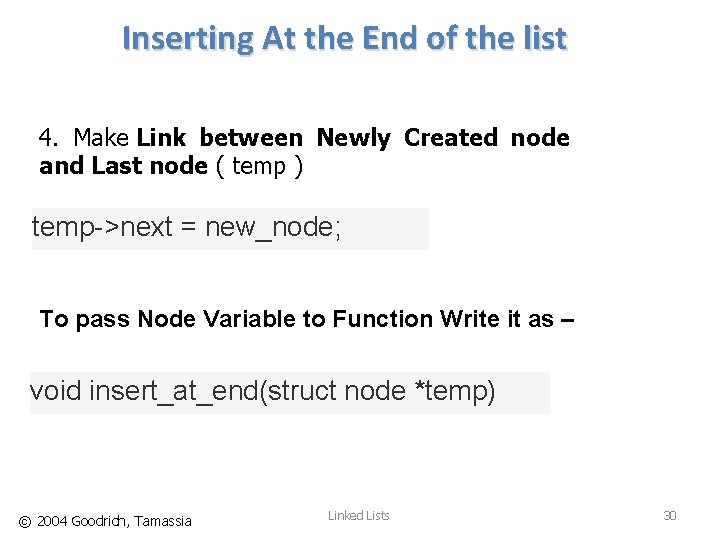
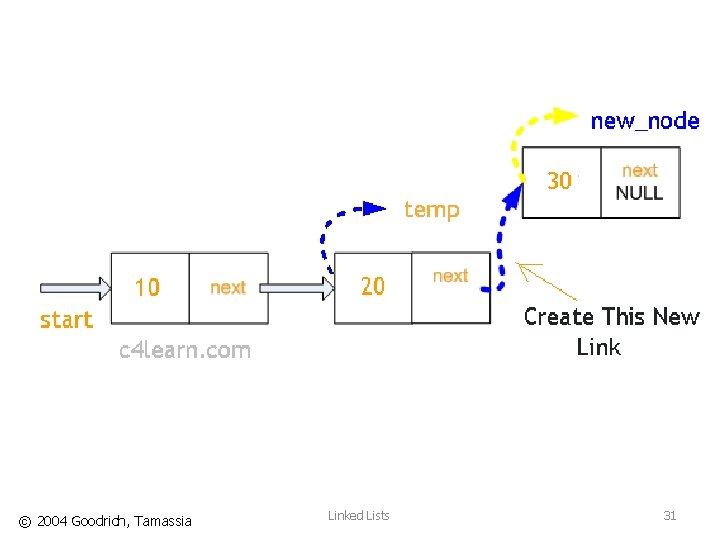
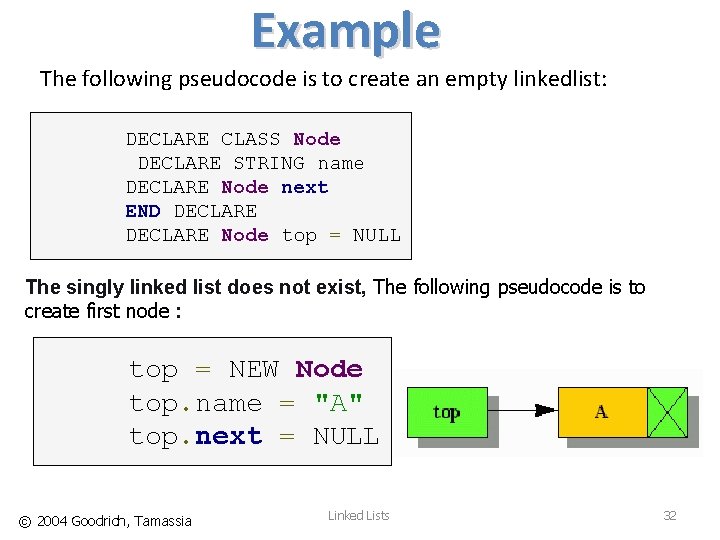
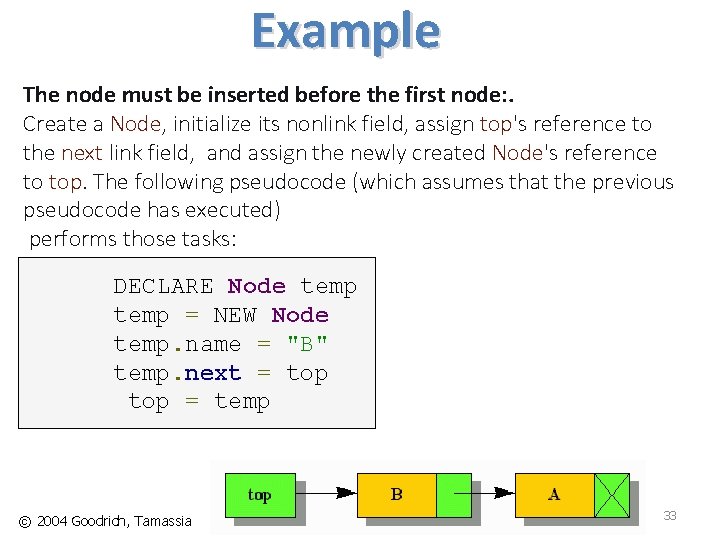
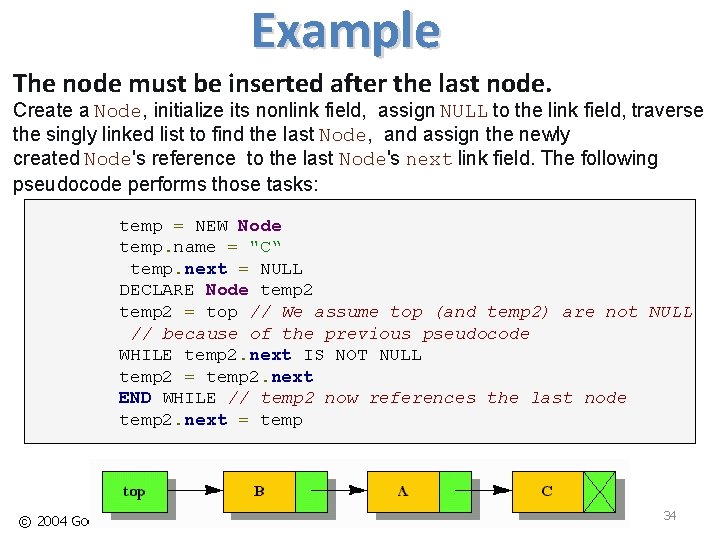
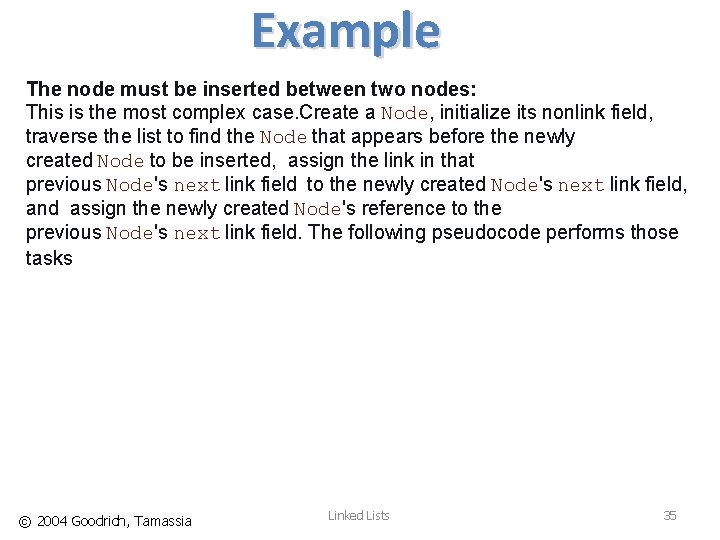
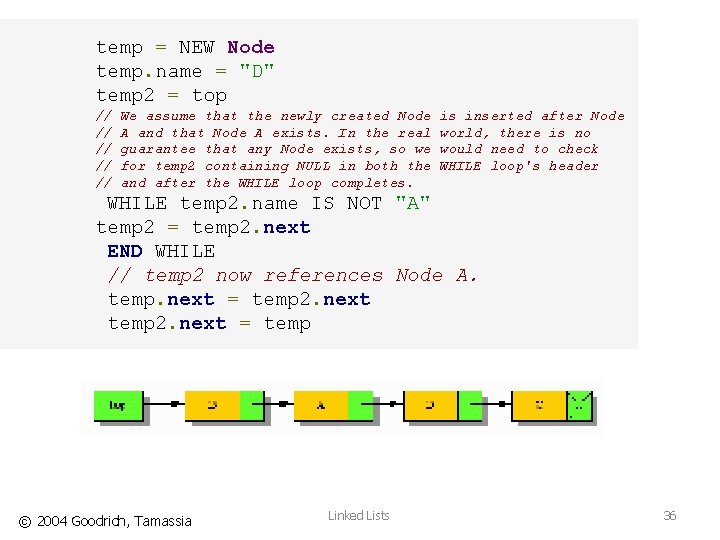
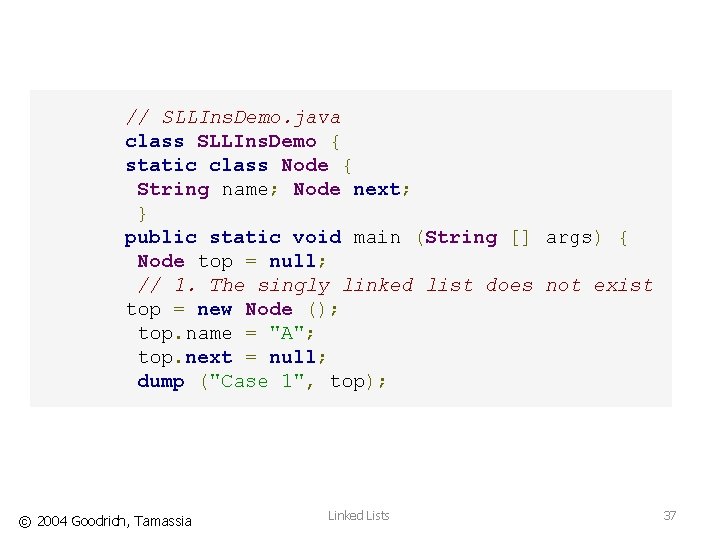
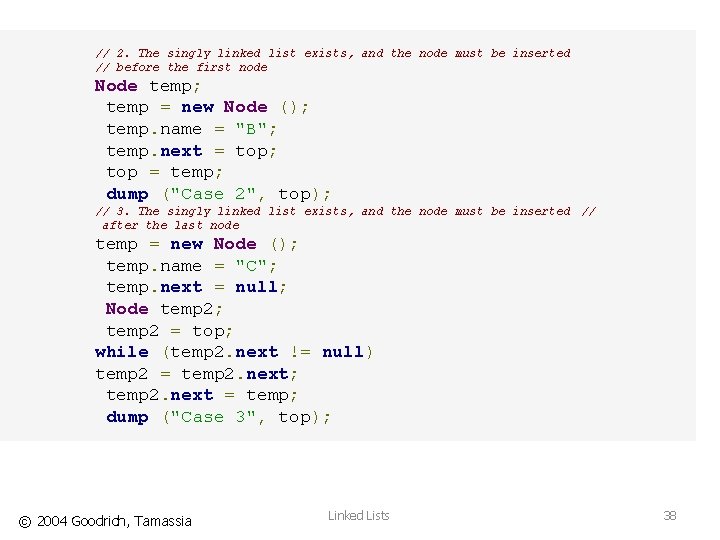
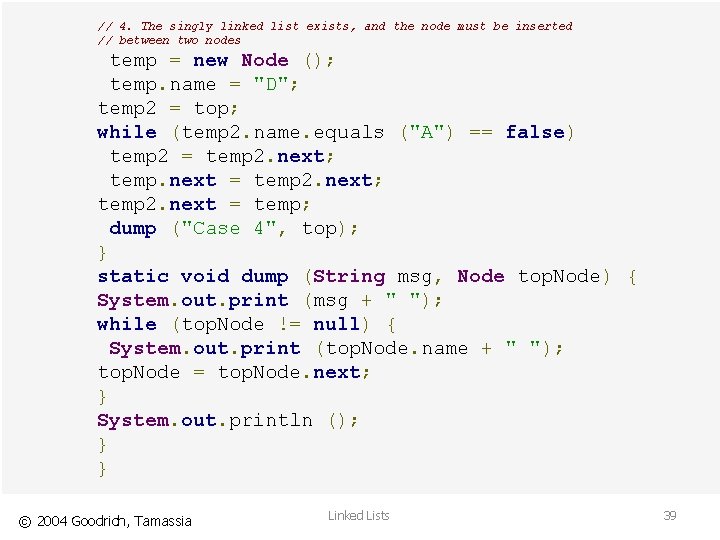
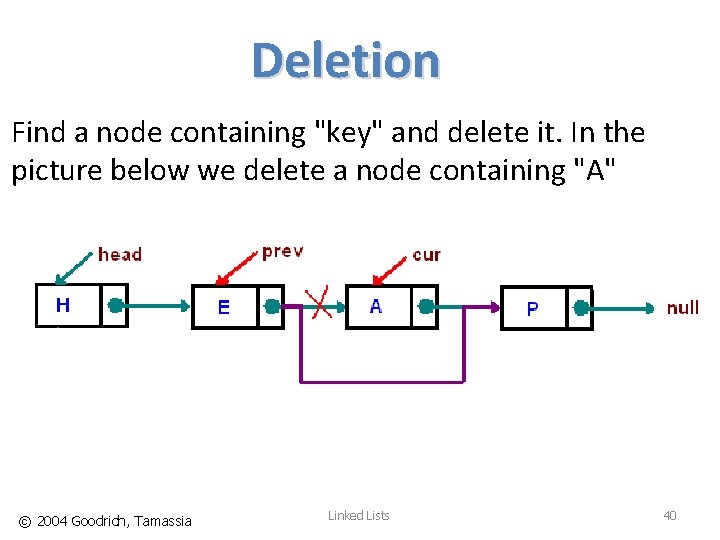
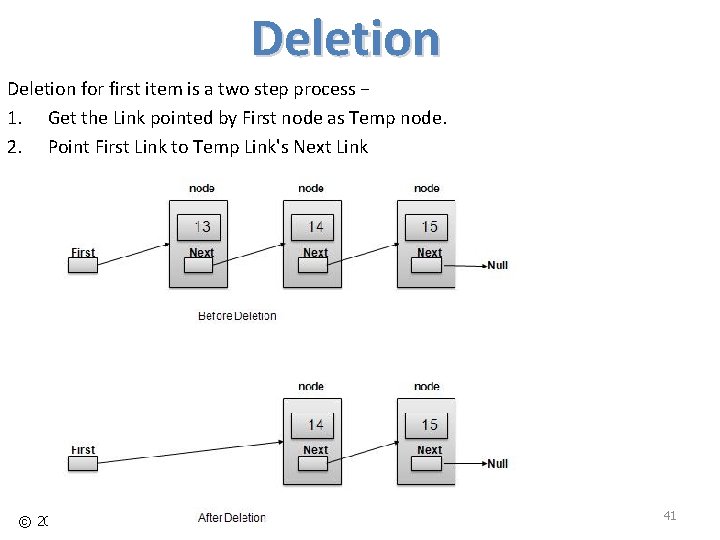
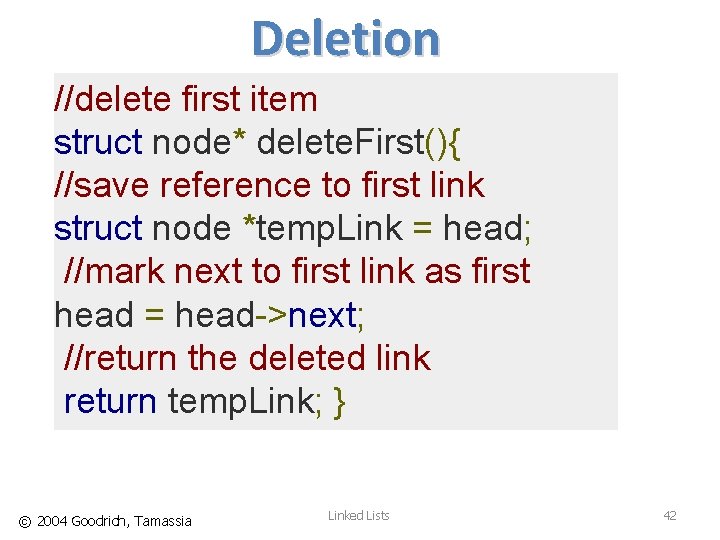
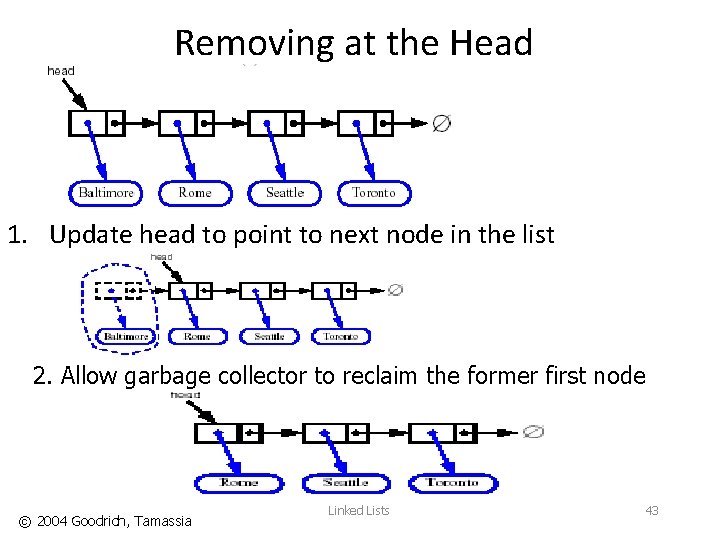
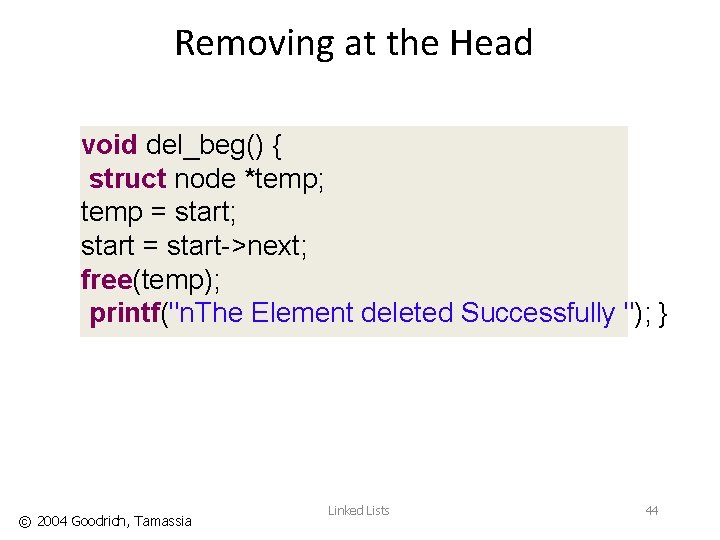
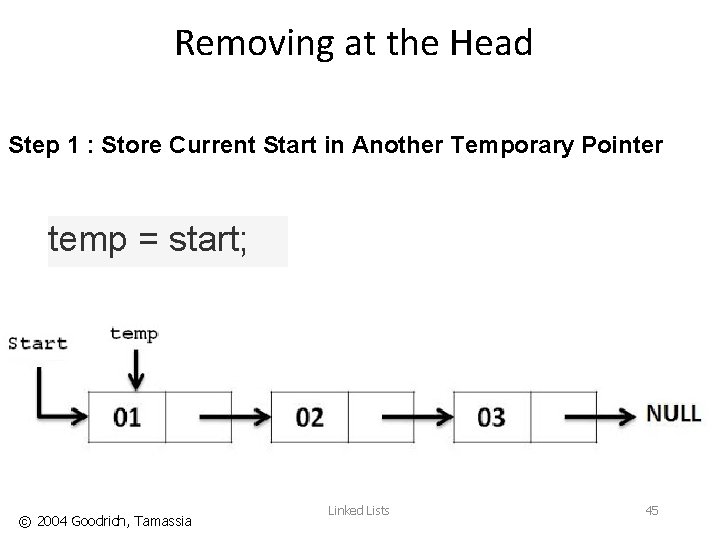
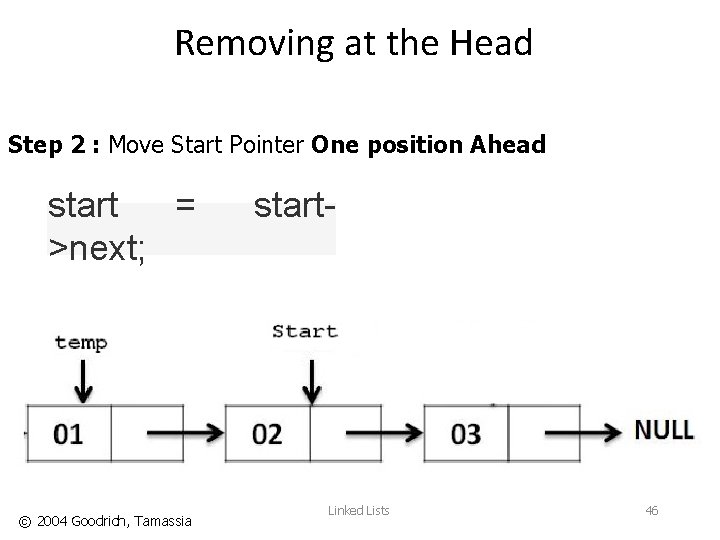
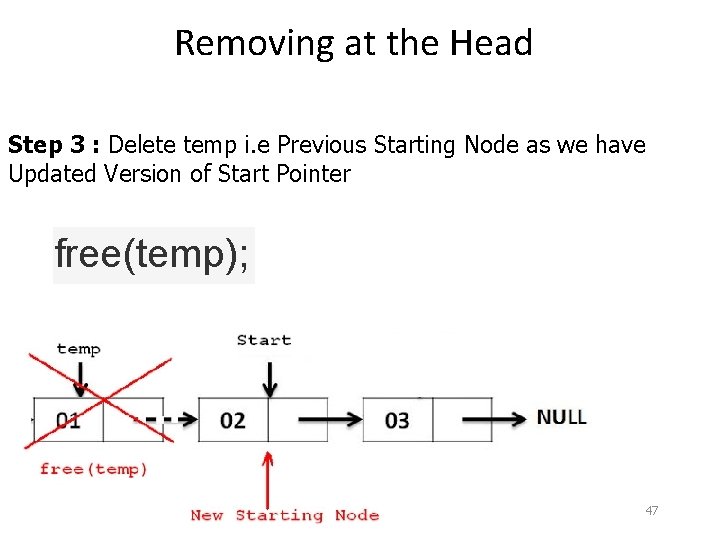
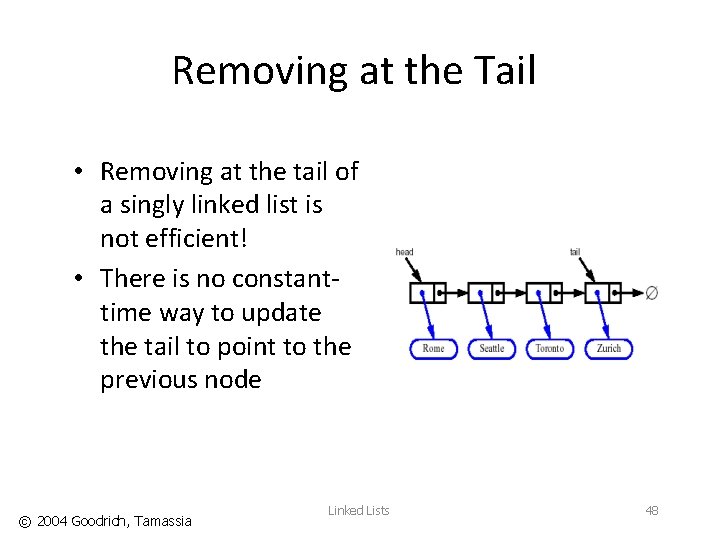
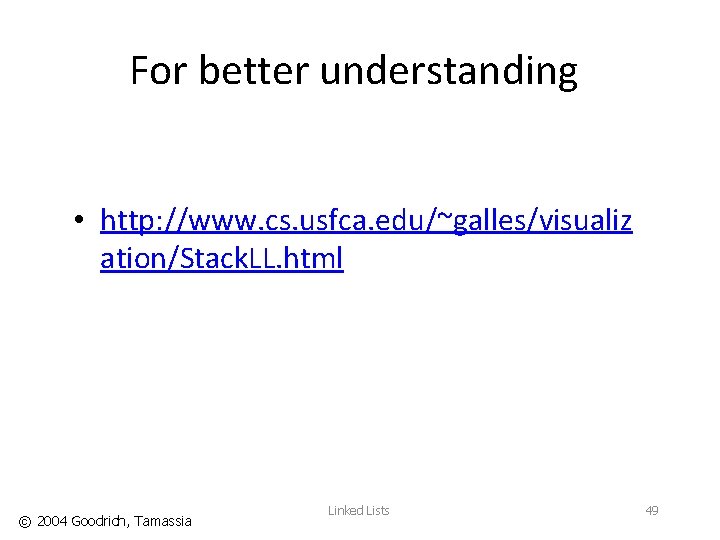
- Slides: 49
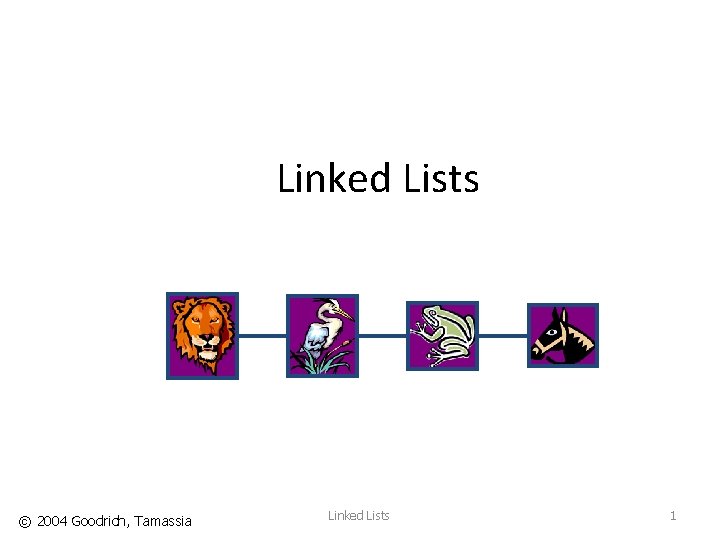
Linked Lists © 2004 Goodrich, Tamassia Linked Lists 1
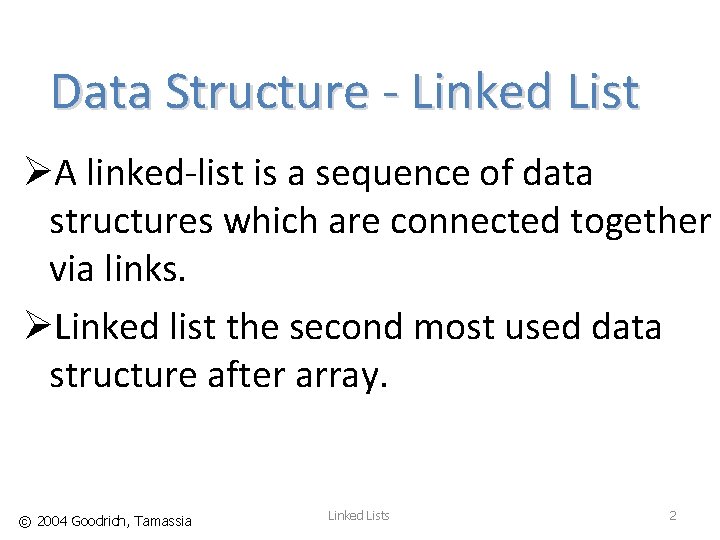
Data Structure - Linked List ØA linked-list is a sequence of data structures which are connected together via links. ØLinked list the second most used data structure after array. © 2004 Goodrich, Tamassia Linked Lists 2
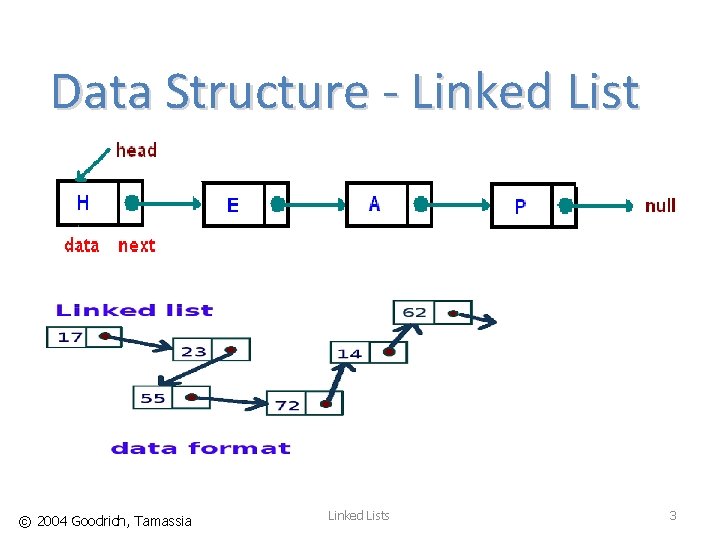
Data Structure - Linked List © 2004 Goodrich, Tamassia Linked Lists 3
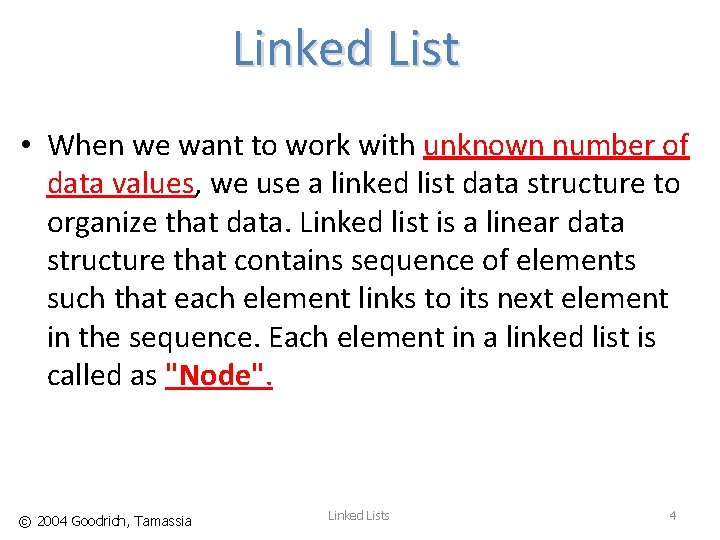
Linked List • When we want to work with unknown number of data values, we use a linked list data structure to organize that data. Linked list is a linear data structure that contains sequence of elements such that each element links to its next element in the sequence. Each element in a linked list is called as "Node". © 2004 Goodrich, Tamassia Linked Lists 4
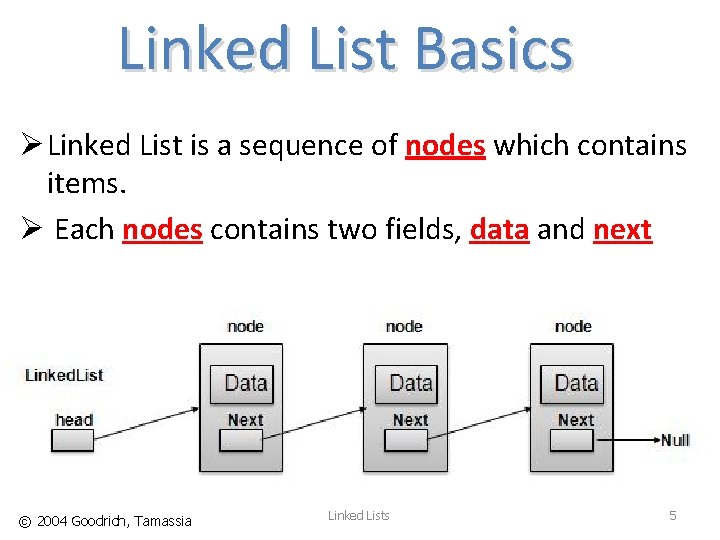
Linked List Basics Ø Linked List is a sequence of nodes which contains items. Ø Each nodes contains two fields, data and next © 2004 Goodrich, Tamassia Linked Lists 5
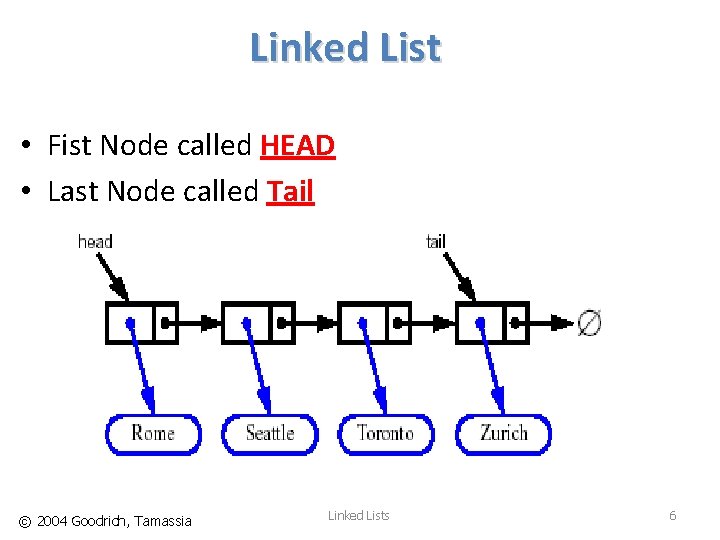
Linked List • Fist Node called HEAD • Last Node called Tail © 2004 Goodrich, Tamassia Linked Lists 6
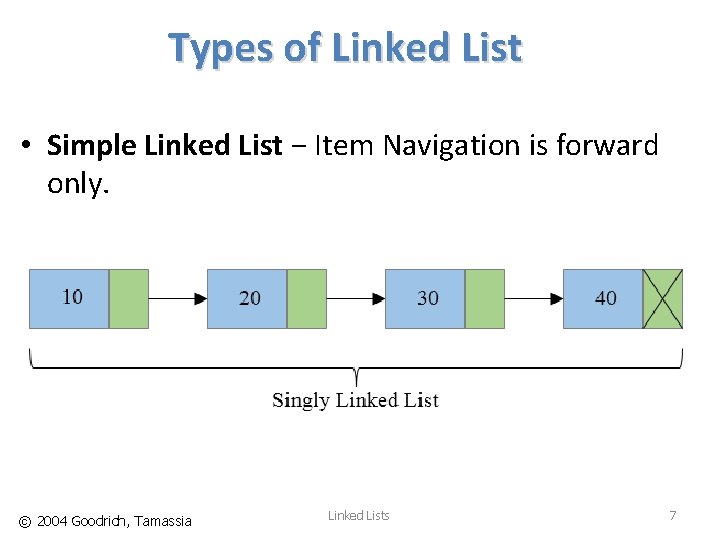
Types of Linked List • Simple Linked List − Item Navigation is forward only. © 2004 Goodrich, Tamassia Linked Lists 7
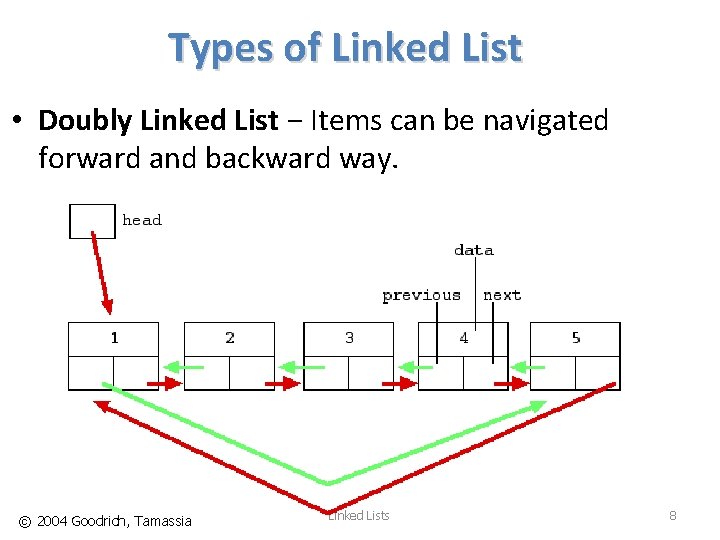
Types of Linked List • Doubly Linked List − Items can be navigated forward and backward way. © 2004 Goodrich, Tamassia Linked Lists 8
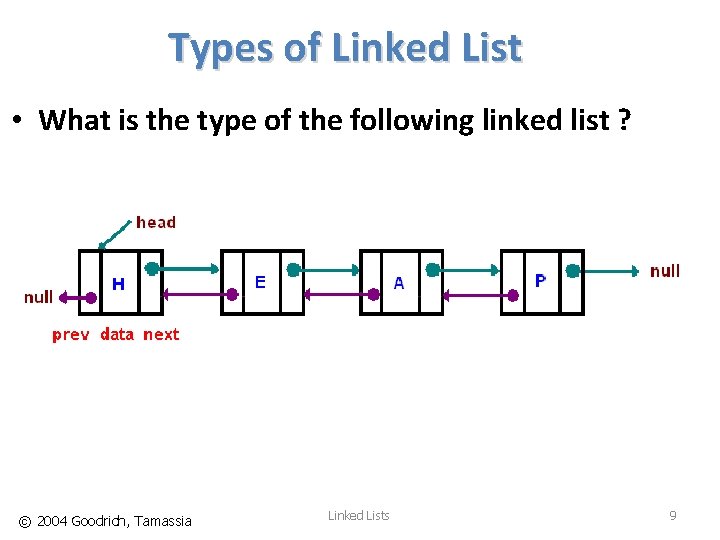
Types of Linked List • What is the type of the following linked list ? © 2004 Goodrich, Tamassia Linked Lists 9
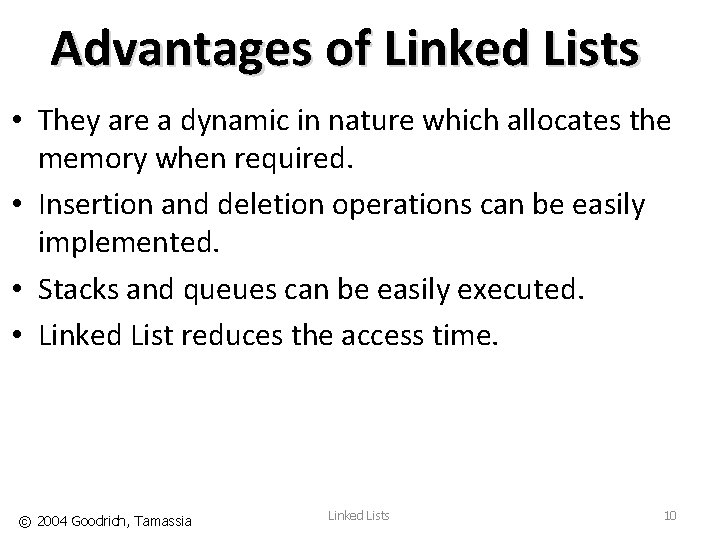
Advantages of Linked Lists • They are a dynamic in nature which allocates the memory when required. • Insertion and deletion operations can be easily implemented. • Stacks and queues can be easily executed. • Linked List reduces the access time. © 2004 Goodrich, Tamassia Linked Lists 10
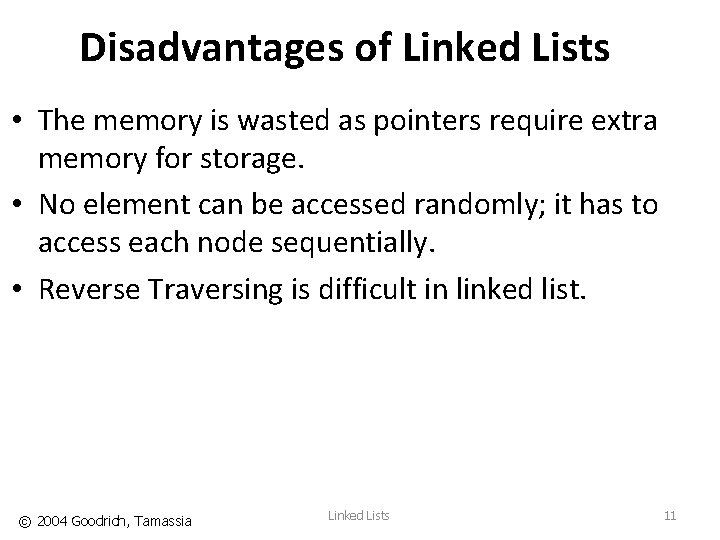
Disadvantages of Linked Lists • The memory is wasted as pointers require extra memory for storage. • No element can be accessed randomly; it has to access each node sequentially. • Reverse Traversing is difficult in linked list. © 2004 Goodrich, Tamassia Linked Lists 11
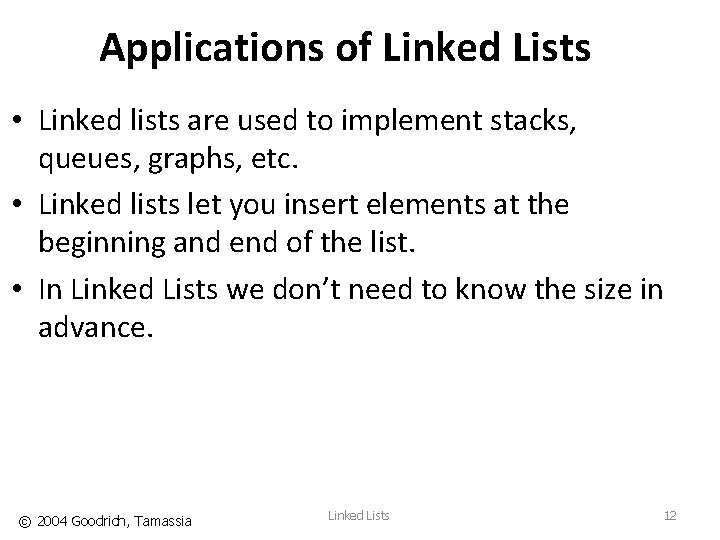
Applications of Linked Lists • Linked lists are used to implement stacks, queues, graphs, etc. • Linked lists let you insert elements at the beginning and end of the list. • In Linked Lists we don’t need to know the size in advance. © 2004 Goodrich, Tamassia Linked Lists 12
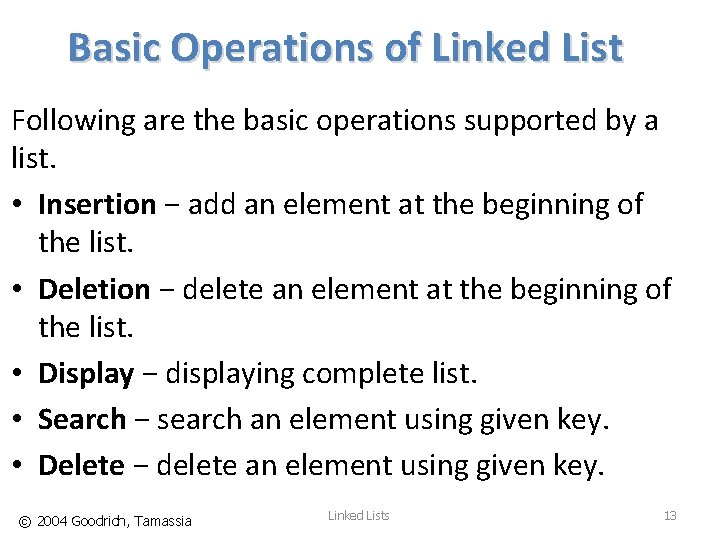
Basic Operations of Linked List Following are the basic operations supported by a list. • Insertion − add an element at the beginning of the list. • Deletion − delete an element at the beginning of the list. • Display − displaying complete list. • Search − search an element using given key. • Delete − delete an element using given key. © 2004 Goodrich, Tamassia Linked Lists 13
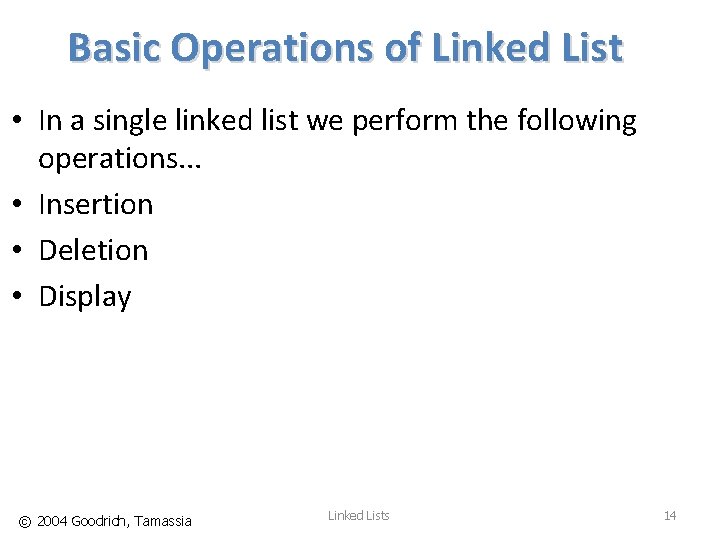
Basic Operations of Linked List • In a single linked list we perform the following operations. . . • Insertion • Deletion • Display © 2004 Goodrich, Tamassia Linked Lists 14
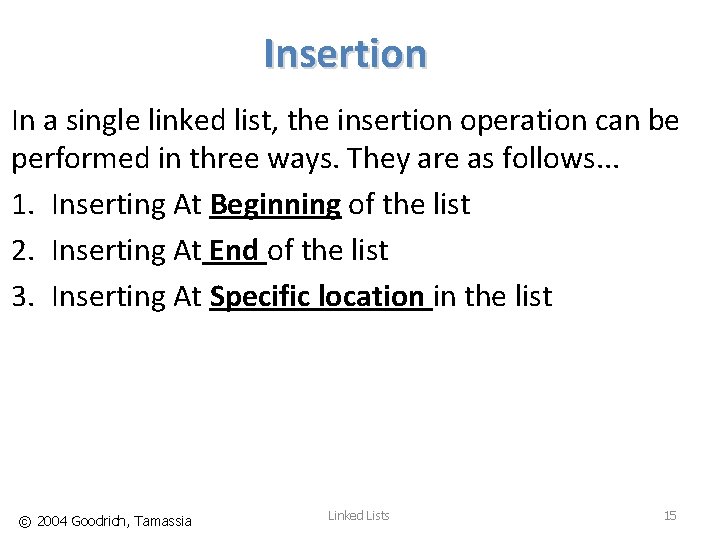
Insertion In a single linked list, the insertion operation can be performed in three ways. They are as follows. . . 1. Inserting At Beginning of the list 2. Inserting At End of the list 3. Inserting At Specific location in the list © 2004 Goodrich, Tamassia Linked Lists 15
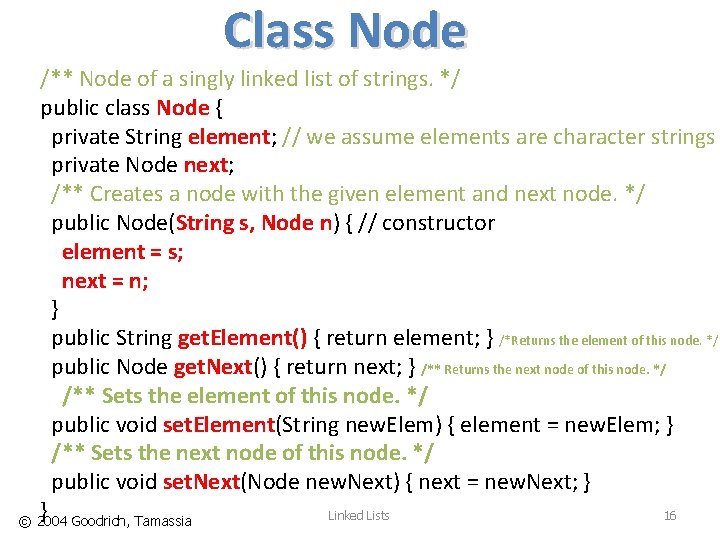
Class Node /** Node of a singly linked list of strings. */ public class Node { private String element; // we assume elements are character strings private Node next; /** Creates a node with the given element and next node. */ public Node(String s, Node n) { // constructor element = s; next = n; } public String get. Element() { return element; } /*Returns the element of this node. */ public Node get. Next() { return next; } /** Returns the next node of this node. */ /** Sets the element of this node. */ public void set. Element(String new. Elem) { element = new. Elem; } /** Sets the next node of this node. */ public void set. Next(Node new. Next) { next = new. Next; } } Linked Lists 16 © 2004 Goodrich, Tamassia
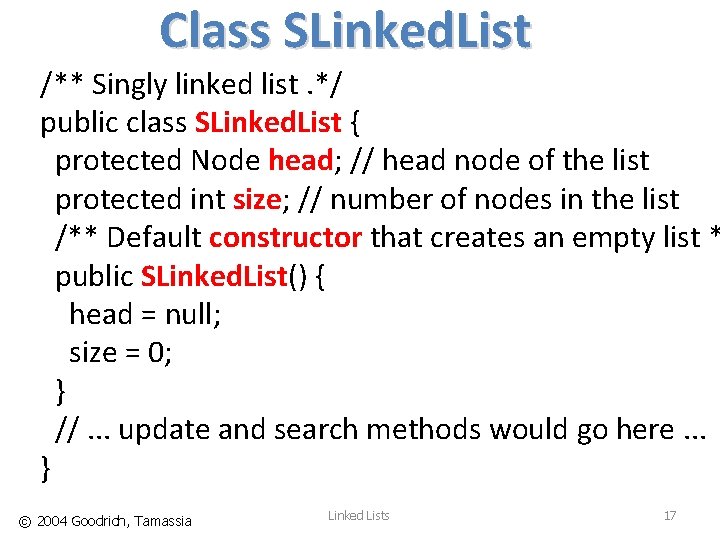
Class SLinked. List /** Singly linked list. */ public class SLinked. List { protected Node head; // head node of the list protected int size; // number of nodes in the list /** Default constructor that creates an empty list * public SLinked. List() { head = null; size = 0; } //. . . update and search methods would go here. . . } © 2004 Goodrich, Tamassia Linked Lists 17
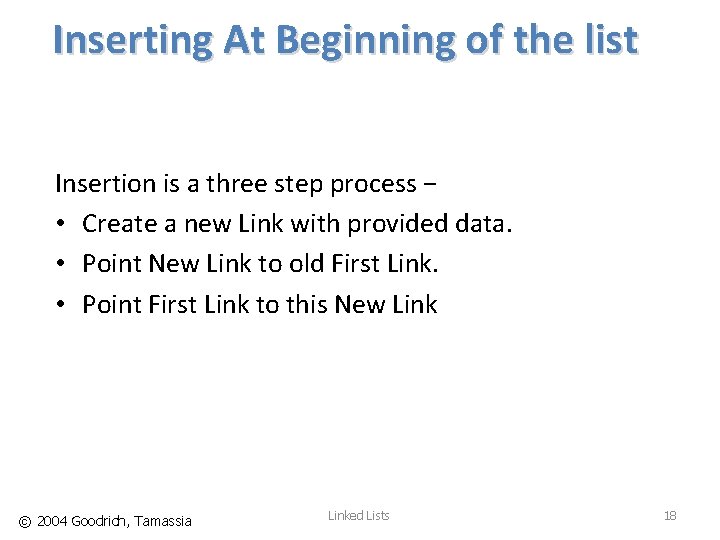
Inserting At Beginning of the list Insertion is a three step process − • Create a new Link with provided data. • Point New Link to old First Link. • Point First Link to this New Link © 2004 Goodrich, Tamassia Linked Lists 18
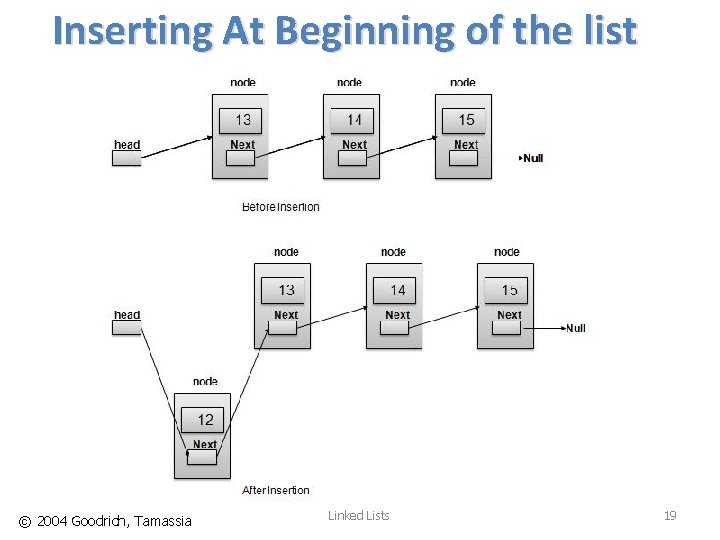
Inserting At Beginning of the list © 2004 Goodrich, Tamassia Linked Lists 19
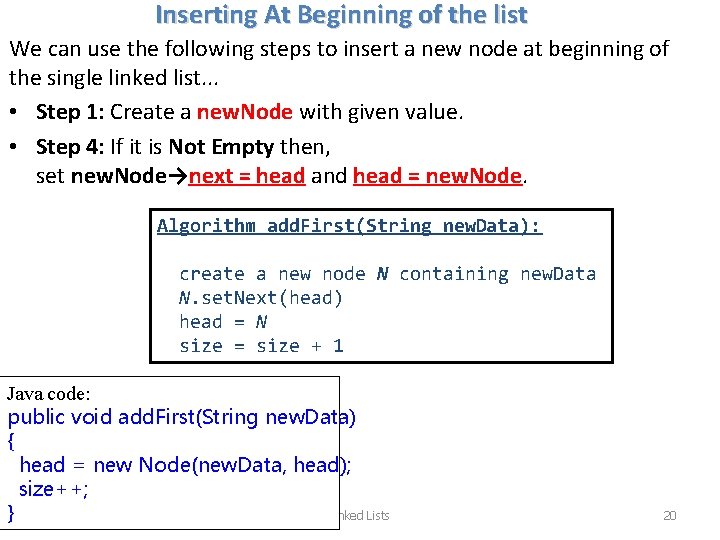
Inserting At Beginning of the list We can use the following steps to insert a new node at beginning of the single linked list. . . • Step 1: Create a new. Node with given value. • Step 4: If it is Not Empty then, set new. Node→next = head and head = new. Node. Algorithm add. First(String new. Data): create a new node N containing new. Data N. set. Next(head) head = N size = size + 1 Java code: public void add. First(String new. Data) { head = new Node(new. Data, head); size++; Linked Lists } © 2004 Goodrich, Tamassia 20
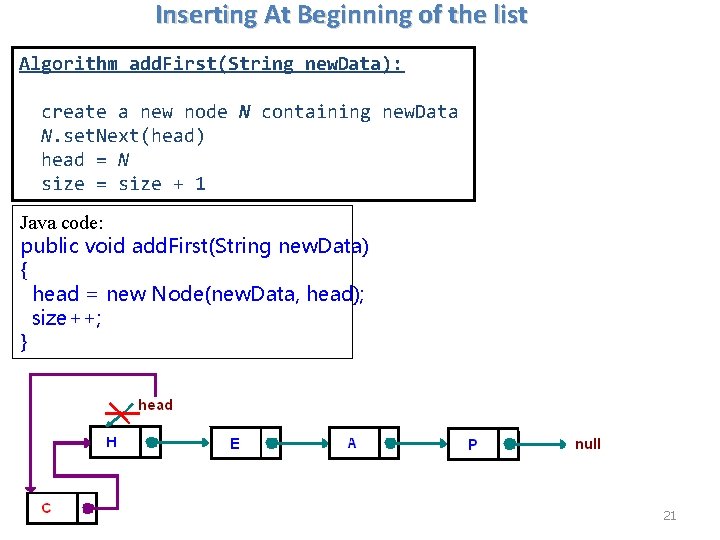
Inserting At Beginning of the list Algorithm add. First(String new. Data): create a new node N containing new. Data N. set. Next(head) head = N size = size + 1 Java code: public void add. First(String new. Data) { head = new Node(new. Data, head); size++; } © 2004 Goodrich, Tamassia Linked Lists 21
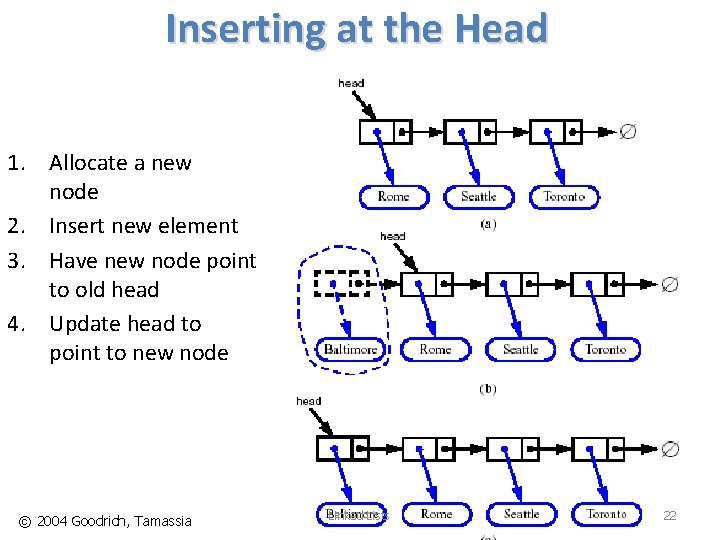
Inserting at the Head 1. Allocate a new node 2. Insert new element 3. Have new node point to old head 4. Update head to point to new node © 2004 Goodrich, Tamassia Linked Lists 22
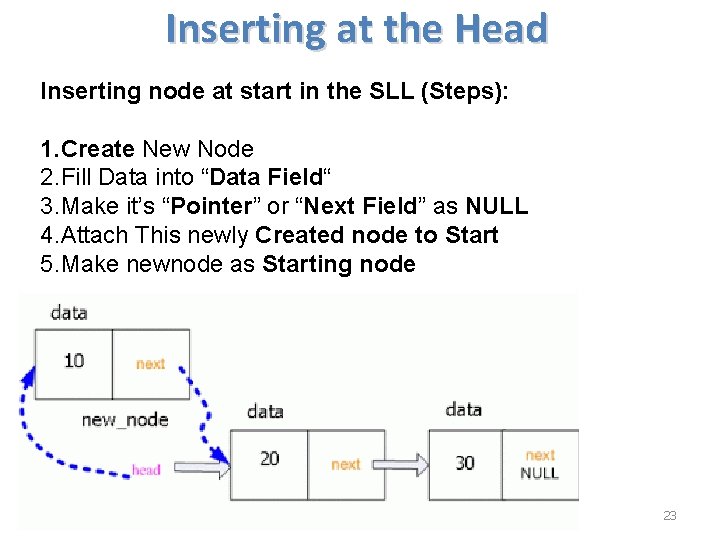
Inserting at the Head Inserting node at start in the SLL (Steps): 1. Create New Node 2. Fill Data into “Data Field“ 3. Make it’s “Pointer” or “Next Field” as NULL 4. Attach This newly Created node to Start 5. Make newnode as Starting node © 2004 Goodrich, Tamassia Linked Lists 23
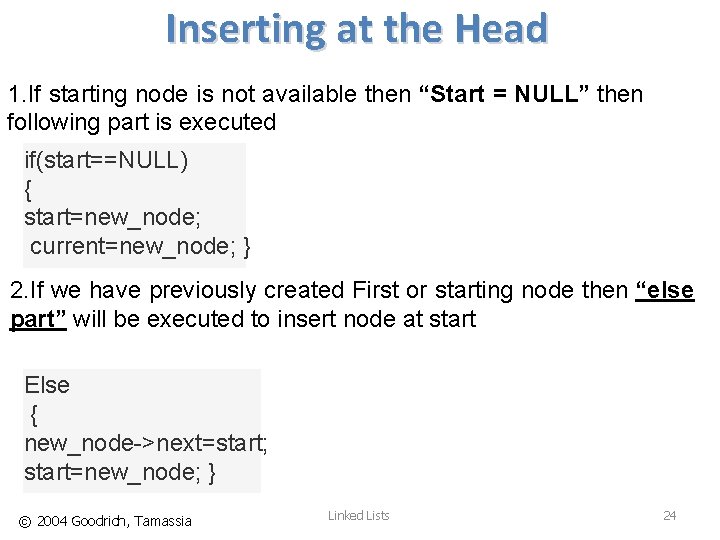
Inserting at the Head 1. If starting node is not available then “Start = NULL” then following part is executed if(start==NULL) { start=new_node; current=new_node; } 2. If we have previously created First or starting node then “else part” will be executed to insert node at start Else { new_node->next=start; start=new_node; } © 2004 Goodrich, Tamassia Linked Lists 24
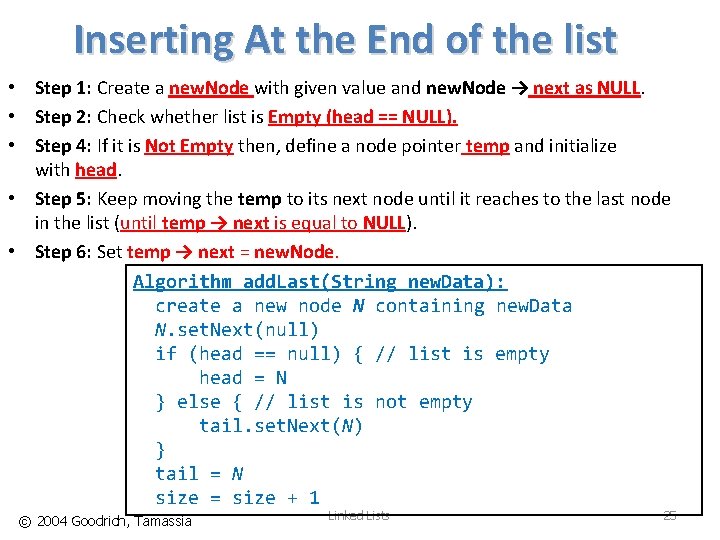
Inserting At the End of the list • Step 1: Create a new. Node with given value and new. Node → next as NULL. • Step 2: Check whether list is Empty (head == NULL). • Step 4: If it is Not Empty then, define a node pointer temp and initialize with head. • Step 5: Keep moving the temp to its next node until it reaches to the last node in the list (until temp → next is equal to NULL). • Step 6: Set temp → next = new. Node. Algorithm add. Last(String new. Data): create a new node N containing new. Data N. set. Next(null) if (head == null) { // list is empty head = N } else { // list is not empty tail. set. Next(N) } tail = N size = size + 1 © 2004 Goodrich, Tamassia Linked Lists 25
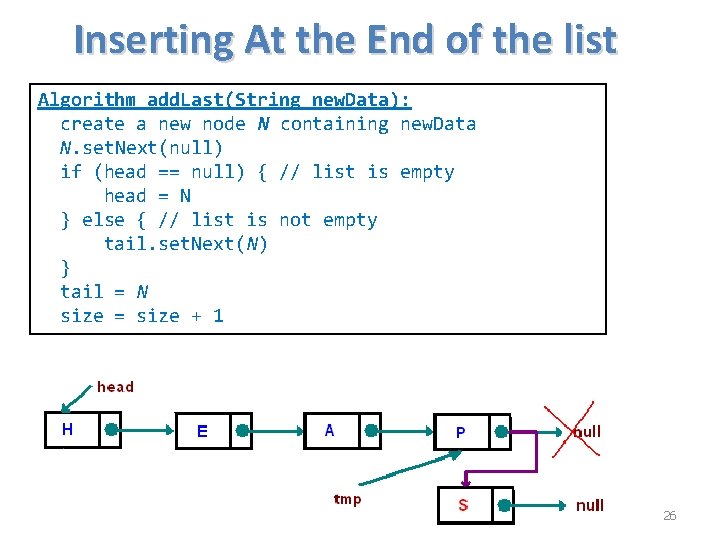
Inserting At the End of the list Algorithm add. Last(String new. Data): create a new node N containing new. Data N. set. Next(null) if (head == null) { // list is empty head = N } else { // list is not empty tail. set. Next(N) } tail = N size = size + 1 © 2004 Goodrich, Tamassia Linked Lists 26
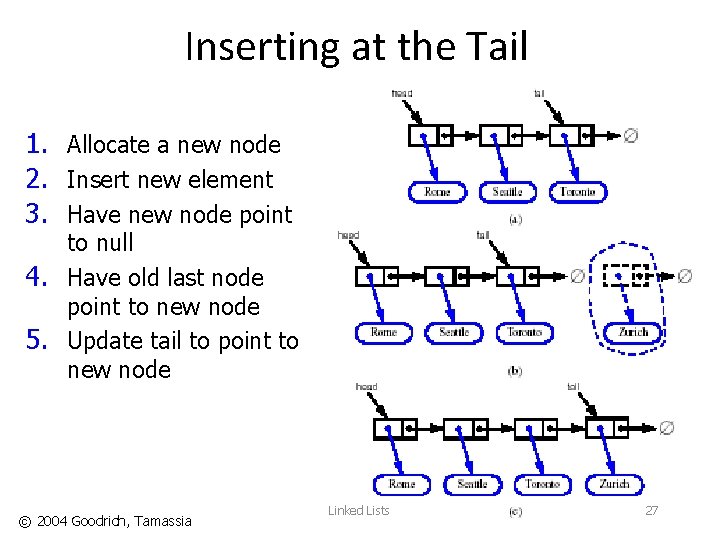
Inserting at the Tail 1. Allocate a new node 2. Insert new element 3. Have new node point to null 4. Have old last node point to new node 5. Update tail to point to new node © 2004 Goodrich, Tamassia Linked Lists 27
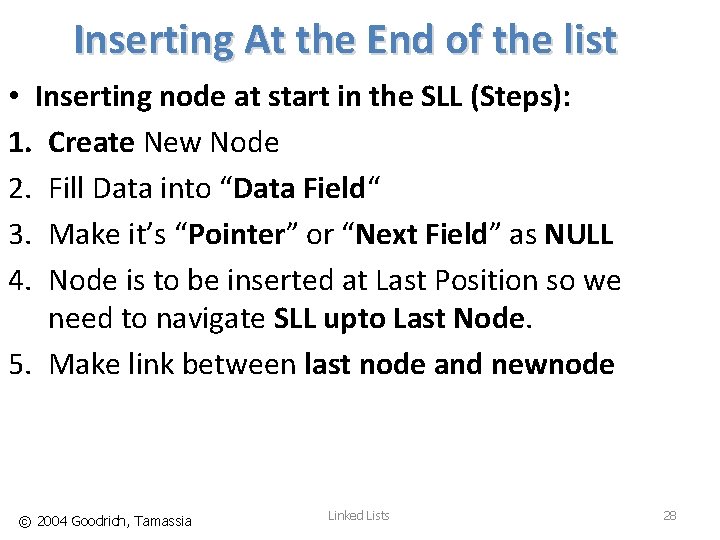
Inserting At the End of the list • Inserting node at start in the SLL (Steps): 1. Create New Node 2. Fill Data into “Data Field“ 3. Make it’s “Pointer” or “Next Field” as NULL 4. Node is to be inserted at Last Position so we need to navigate SLL upto Last Node. 5. Make link between last node and newnode © 2004 Goodrich, Tamassia Linked Lists 28
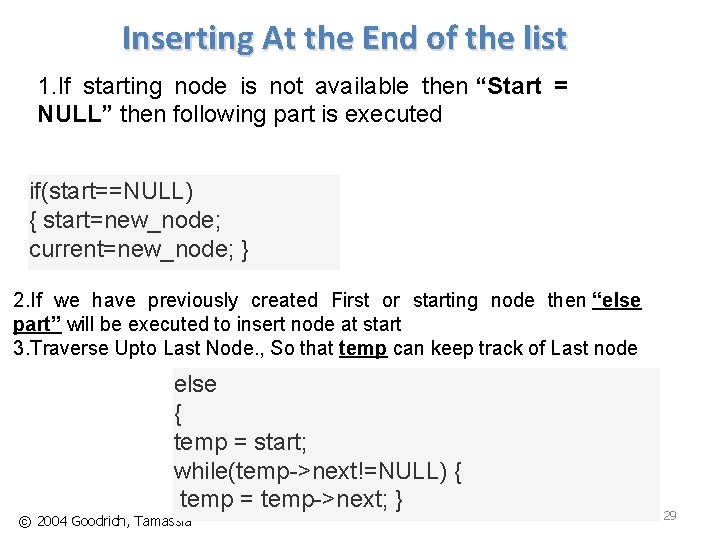
Inserting At the End of the list 1. If starting node is not available then “Start = NULL” then following part is executed if(start==NULL) { start=new_node; current=new_node; } 2. If we have previously created First or starting node then “else part” will be executed to insert node at start 3. Traverse Upto Last Node. , So that temp can keep track of Last node else { temp = start; while(temp->next!=NULL) { temp = temp->next; } © 2004 Goodrich, Tamassia Linked Lists 29
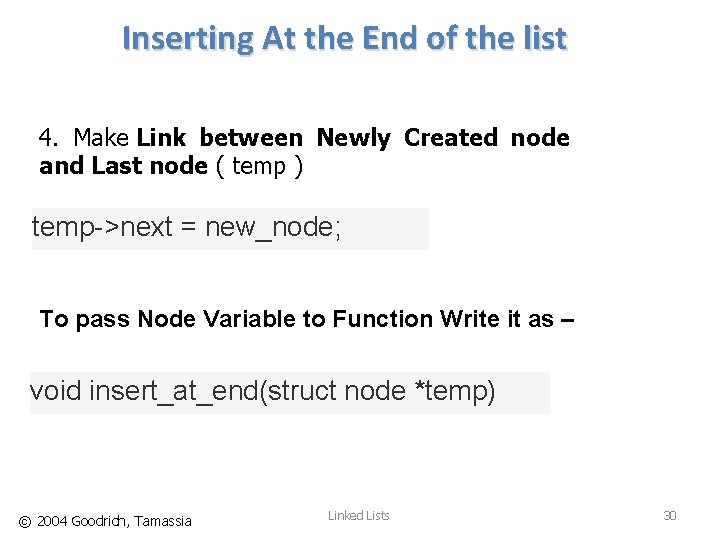
Inserting At the End of the list 4. Make Link between Newly Created node and Last node ( temp ) temp->next = new_node; To pass Node Variable to Function Write it as – void insert_at_end(struct node *temp) © 2004 Goodrich, Tamassia Linked Lists 30
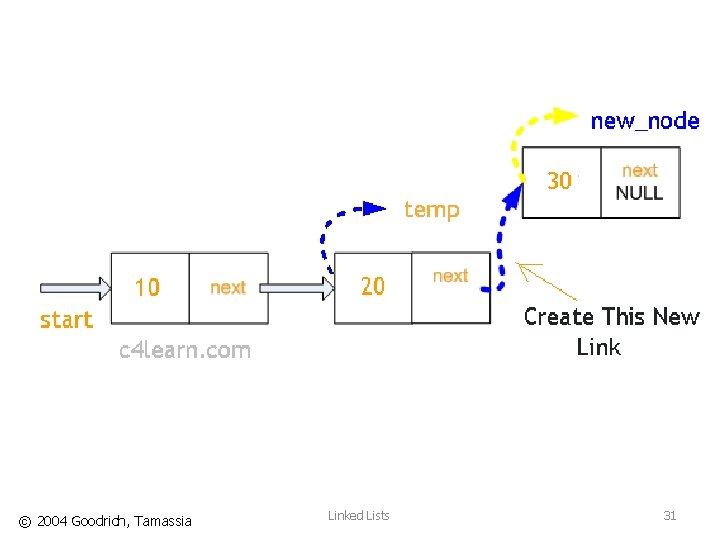
© 2004 Goodrich, Tamassia Linked Lists 31
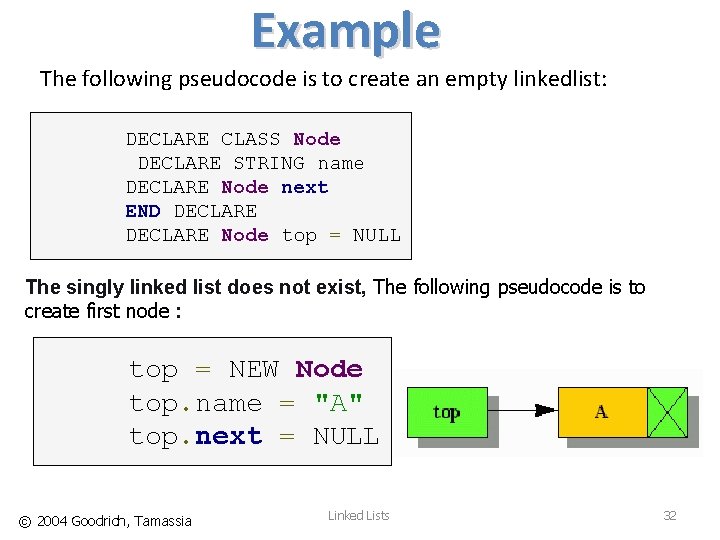
Example The following pseudocode is to create an empty linkedlist: DECLARE CLASS Node DECLARE STRING name DECLARE Node next END DECLARE Node top = NULL The singly linked list does not exist, The following pseudocode is to create first node : top = NEW Node top. name = "A" top. next = NULL © 2004 Goodrich, Tamassia Linked Lists 32
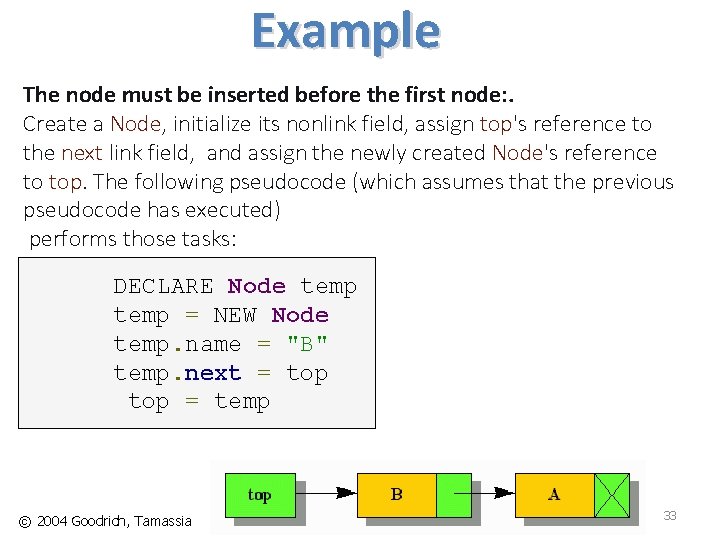
Example The node must be inserted before the first node: . Create a Node, initialize its nonlink field, assign top's reference to the next link field, and assign the newly created Node's reference to top. The following pseudocode (which assumes that the previous pseudocode has executed) performs those tasks: DECLARE Node temp = NEW Node temp. name = "B" temp. next = top = temp © 2004 Goodrich, Tamassia Linked Lists 33
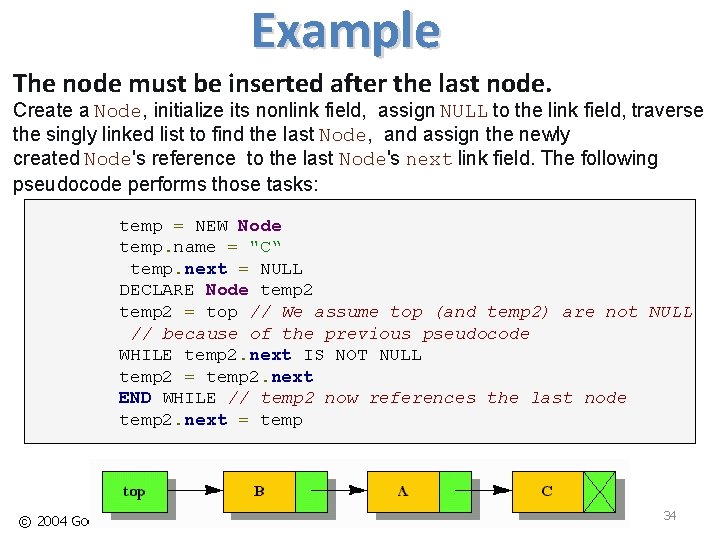
Example The node must be inserted after the last node. Create a Node, initialize its nonlink field, assign NULL to the link field, traverse the singly linked list to find the last Node, and assign the newly created Node's reference to the last Node's next link field. The following pseudocode performs those tasks: temp = NEW Node temp. name = "C“ temp. next = NULL DECLARE Node temp 2 = top // We assume top (and temp 2) are not NULL // because of the previous pseudocode WHILE temp 2. next IS NOT NULL temp 2 = temp 2. next END WHILE // temp 2 now references the last node temp 2. next = temp © 2004 Goodrich, Tamassia Linked Lists 34
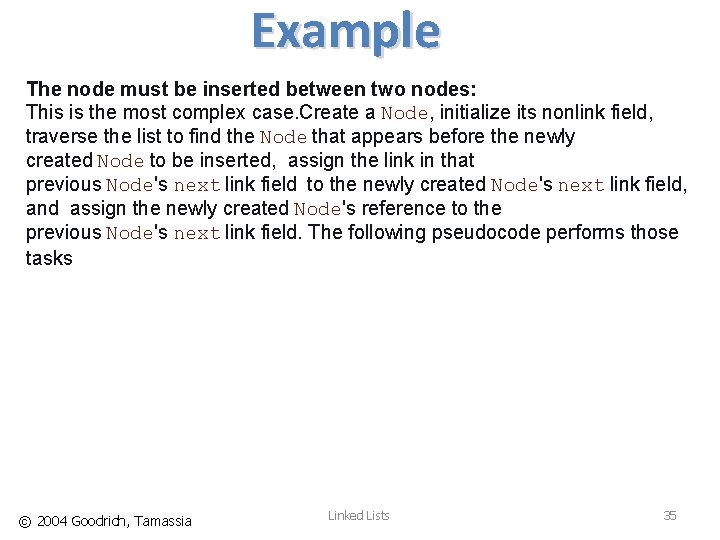
Example The node must be inserted between two nodes: This is the most complex case. Create a Node, initialize its nonlink field, traverse the list to find the Node that appears before the newly created Node to be inserted, assign the link in that previous Node's next link field to the newly created Node's next link field, and assign the newly created Node's reference to the previous Node's next link field. The following pseudocode performs those tasks © 2004 Goodrich, Tamassia Linked Lists 35
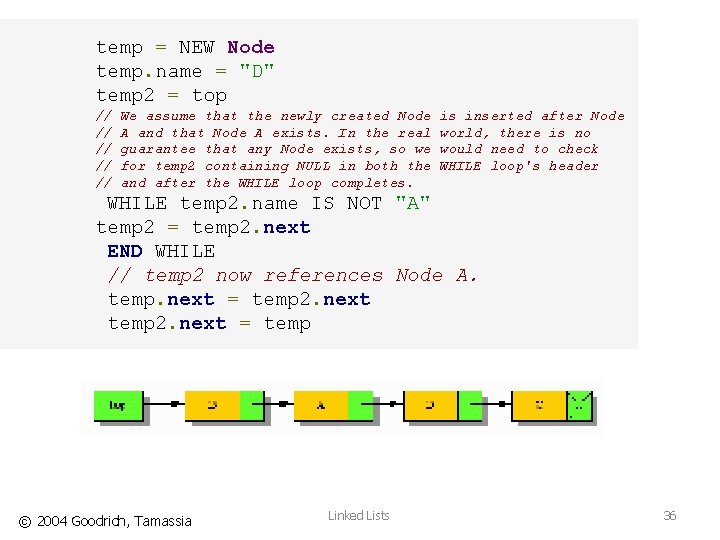
temp = NEW Node temp. name = "D" temp 2 = top // // // We assume that the newly created Node A and that Node A exists. In the real guarantee that any Node exists, so we for temp 2 containing NULL in both the and after the WHILE loop completes. is inserted after Node world, there is no would need to check WHILE loop's header WHILE temp 2. name IS NOT "A" temp 2 = temp 2. next END WHILE // temp 2 now references Node A. temp. next = temp 2. next = temp © 2004 Goodrich, Tamassia Linked Lists 36
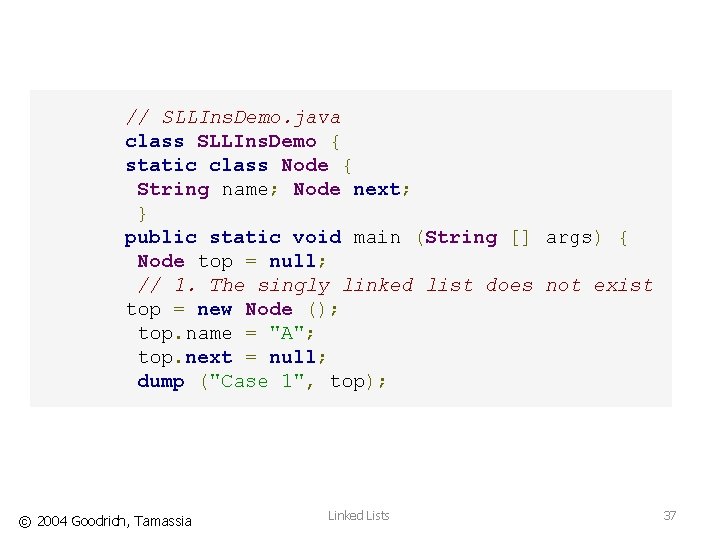
// SLLIns. Demo. java class SLLIns. Demo { static class Node { String name; Node next; } public static void main (String [] args) { Node top = null; // 1. The singly linked list does not exist top = new Node (); top. name = "A"; top. next = null; dump ("Case 1", top); © 2004 Goodrich, Tamassia Linked Lists 37
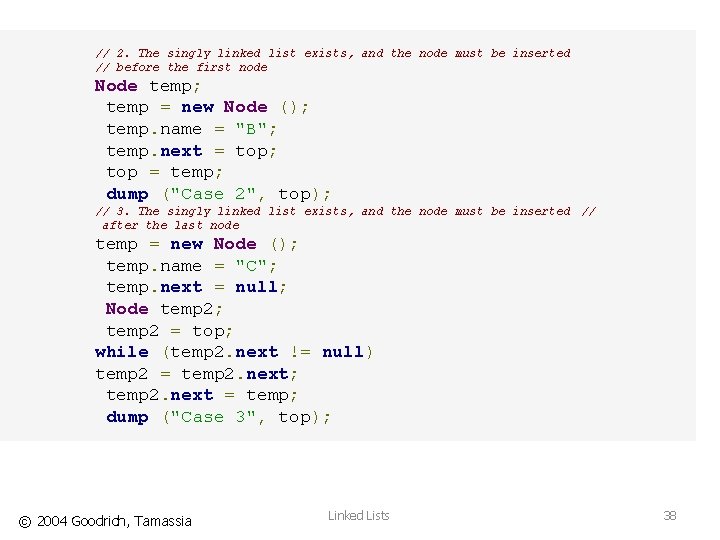
// 2. The singly linked list exists, and the node must be inserted // before the first node Node temp; temp = new Node (); temp. name = "B"; temp. next = top; top = temp; dump ("Case 2", top); // 3. The singly linked list exists, and the node must be inserted // after the last node temp = new Node (); temp. name = "C"; temp. next = null; Node temp 2; temp 2 = top; while (temp 2. next != null) temp 2 = temp 2. next; temp 2. next = temp; dump ("Case 3", top); © 2004 Goodrich, Tamassia Linked Lists 38
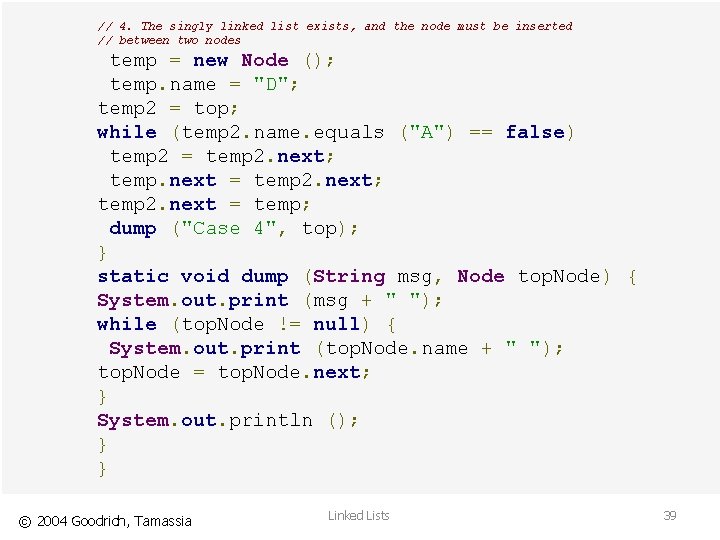
// 4. The singly linked list exists, and the node must be inserted // between two nodes temp = new Node (); temp. name = "D"; temp 2 = top; while (temp 2. name. equals ("A") == false) temp 2 = temp 2. next; temp. next = temp 2. next; temp 2. next = temp; dump ("Case 4", top); } static void dump (String msg, Node top. Node) { System. out. print (msg + " "); while (top. Node != null) { System. out. print (top. Node. name + " "); top. Node = top. Node. next; } System. out. println (); } } © 2004 Goodrich, Tamassia Linked Lists 39
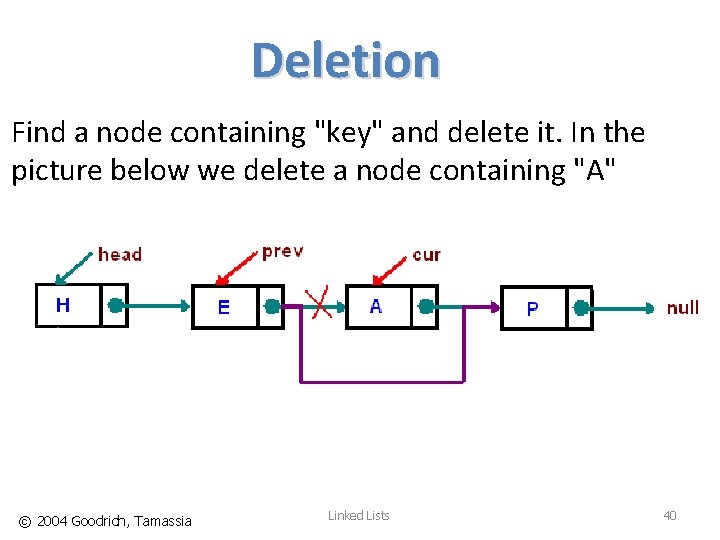
Deletion Find a node containing "key" and delete it. In the picture below we delete a node containing "A" © 2004 Goodrich, Tamassia Linked Lists 40
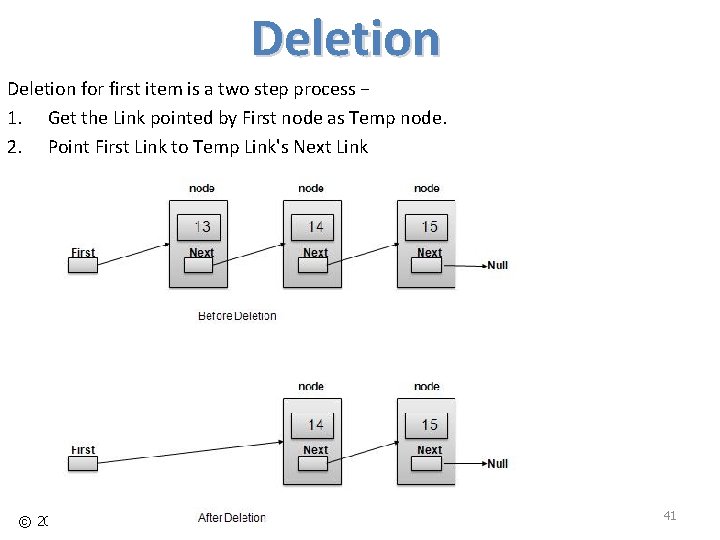
Deletion for first item is a two step process − 1. Get the Link pointed by First node as Temp node. 2. Point First Link to Temp Link's Next Link © 2004 Goodrich, Tamassia Linked Lists 41
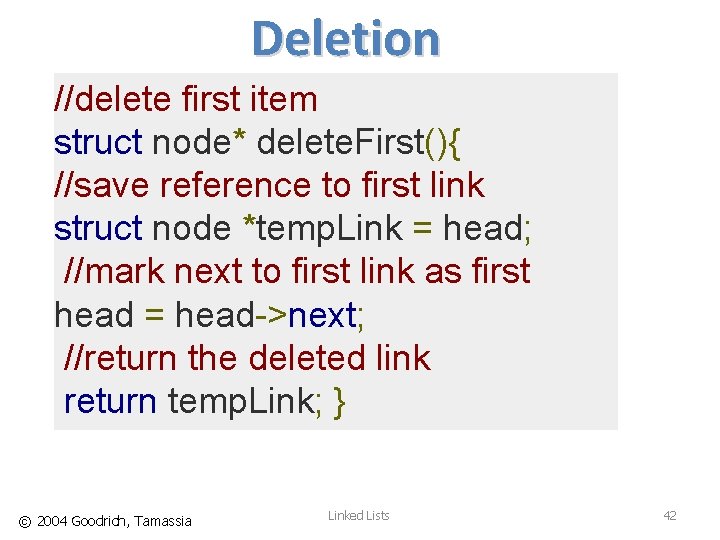
Deletion //delete first item struct node* delete. First(){ //save reference to first link struct node *temp. Link = head; //mark next to first link as first head = head->next; //return the deleted link return temp. Link; } © 2004 Goodrich, Tamassia Linked Lists 42
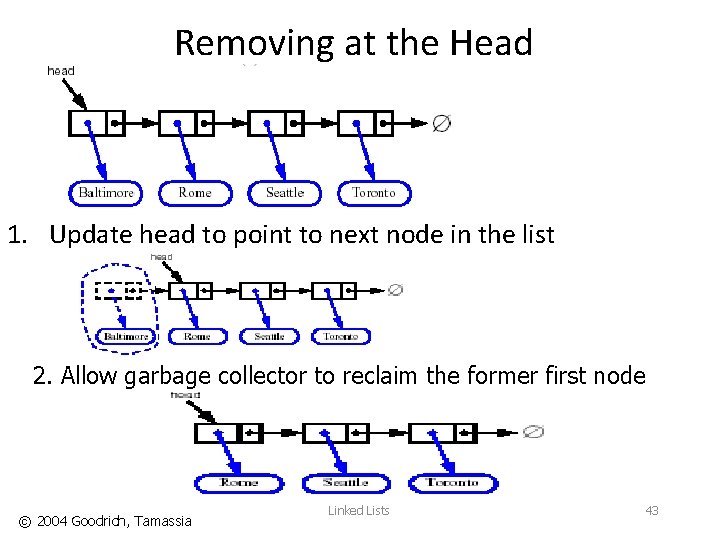
Removing at the Head 1. Update head to point to next node in the list 2. Allow garbage collector to reclaim the former first node © 2004 Goodrich, Tamassia Linked Lists 43
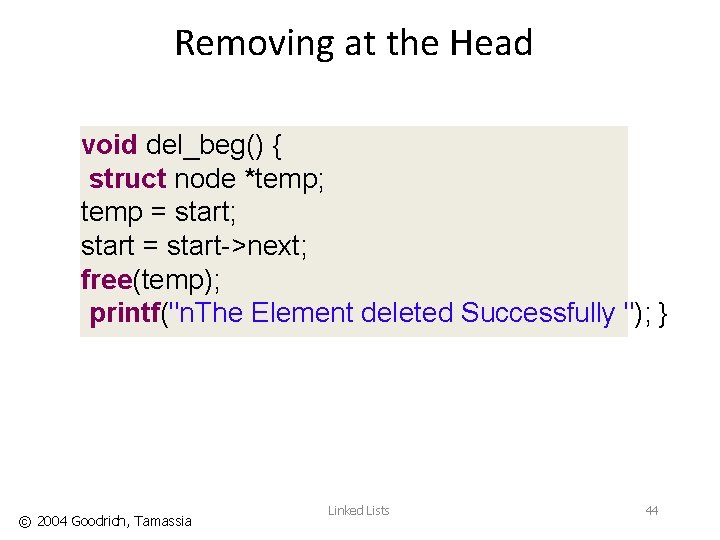
Removing at the Head void del_beg() { struct node *temp; temp = start; start = start->next; free(temp); printf("n. The Element deleted Successfully "); } © 2004 Goodrich, Tamassia Linked Lists 44
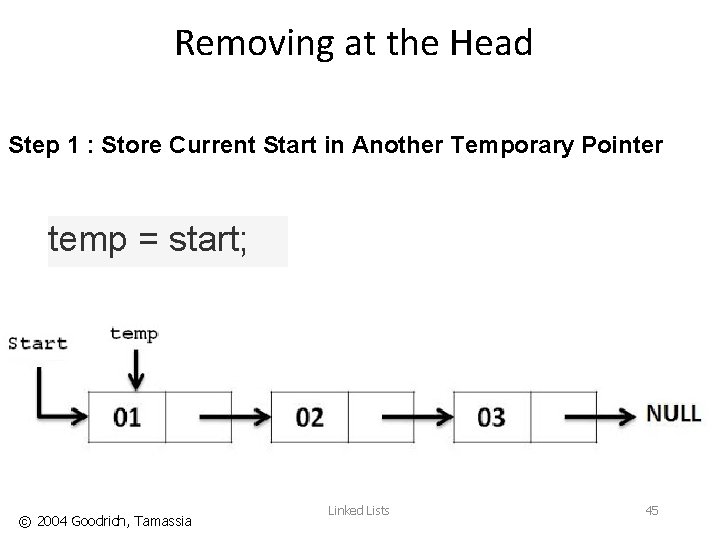
Removing at the Head Step 1 : Store Current Start in Another Temporary Pointer temp = start; © 2004 Goodrich, Tamassia Linked Lists 45
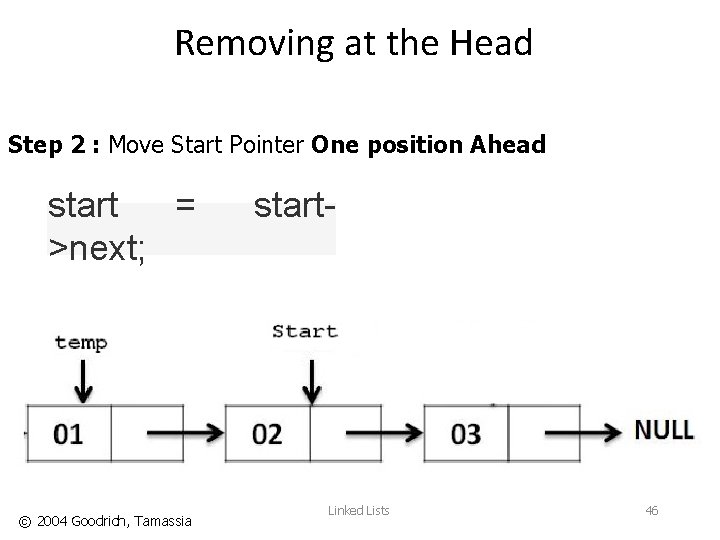
Removing at the Head Step 2 : Move Start Pointer One position Ahead start = >next; © 2004 Goodrich, Tamassia start- Linked Lists 46
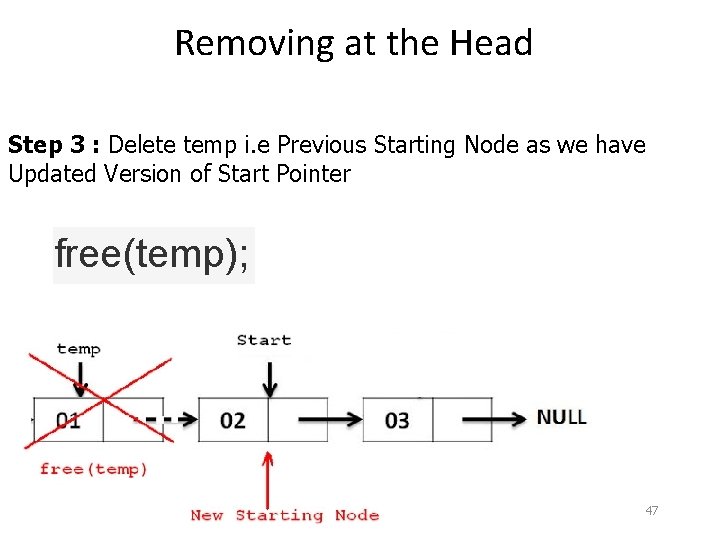
Removing at the Head Step 3 : Delete temp i. e Previous Starting Node as we have Updated Version of Start Pointer free(temp); © 2004 Goodrich, Tamassia Linked Lists 47
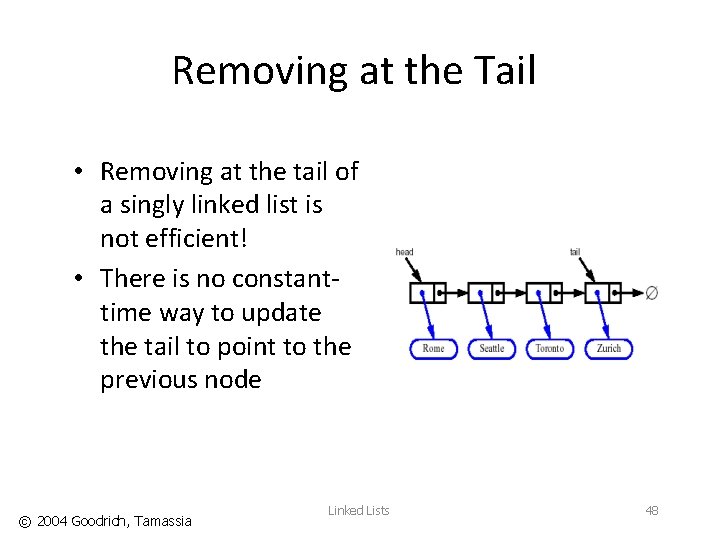
Removing at the Tail • Removing at the tail of a singly linked list is not efficient! • There is no constanttime way to update the tail to point to the previous node © 2004 Goodrich, Tamassia Linked Lists 48
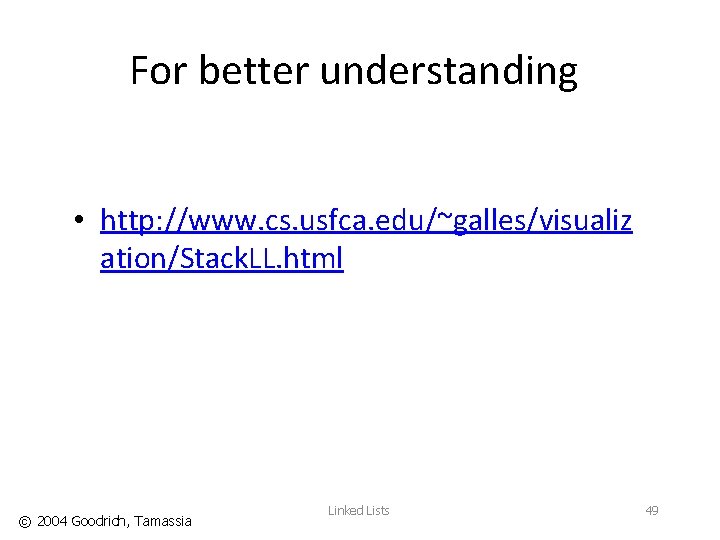
For better understanding • http: //www. cs. usfca. edu/~galles/visualiz ation/Stack. LL. html © 2004 Goodrich, Tamassia Linked Lists 49