Linked list Linked list A collection of nodes
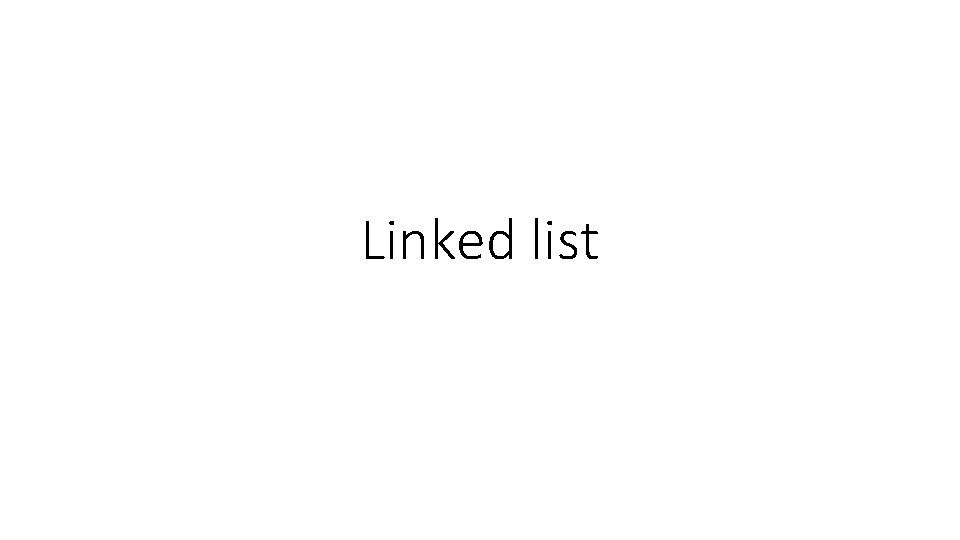
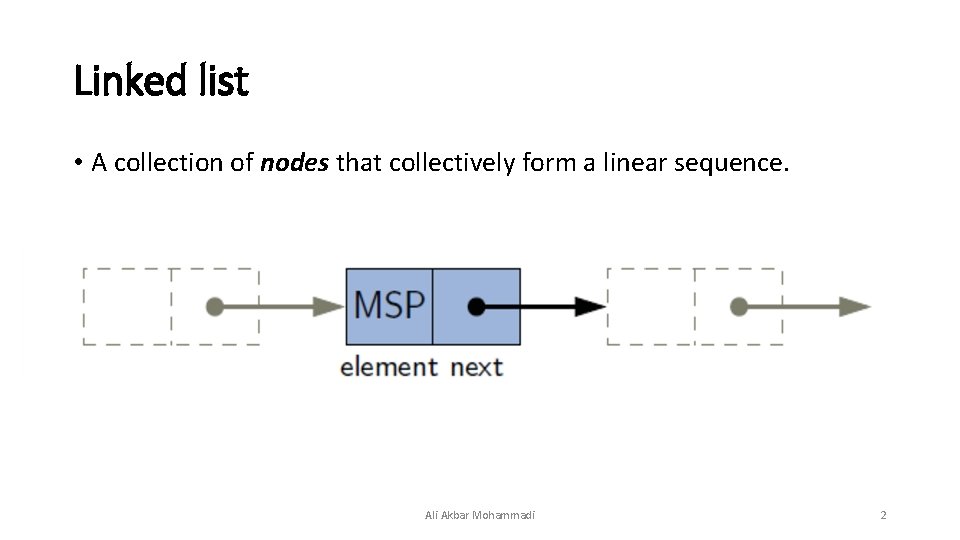
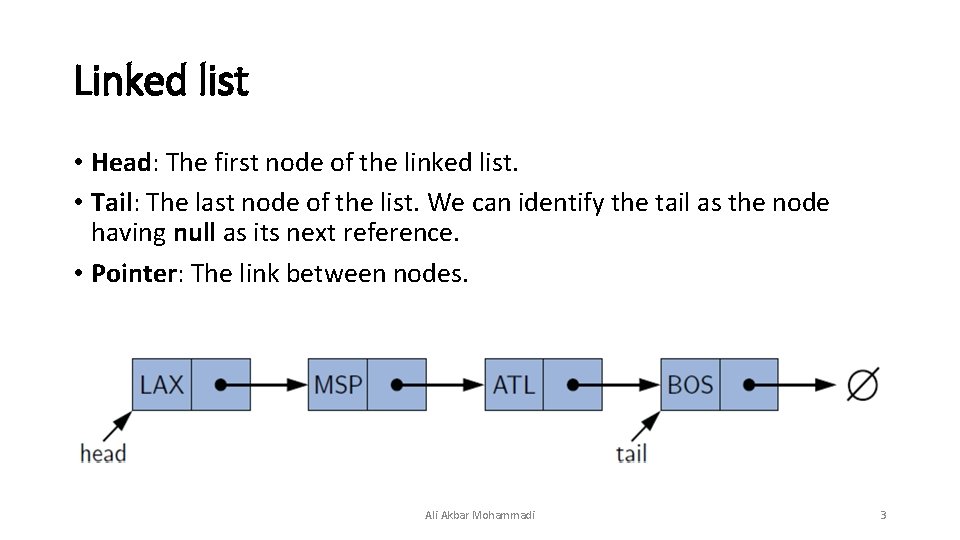
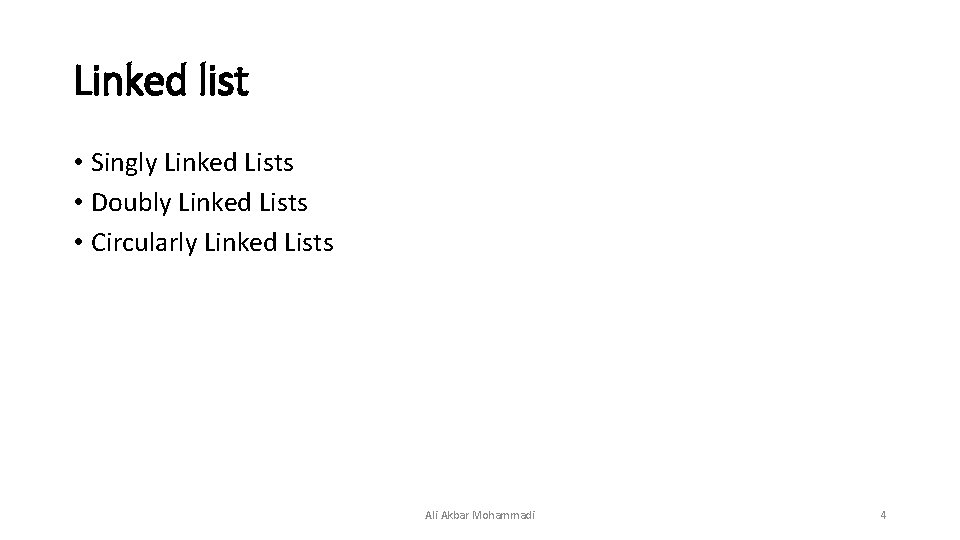
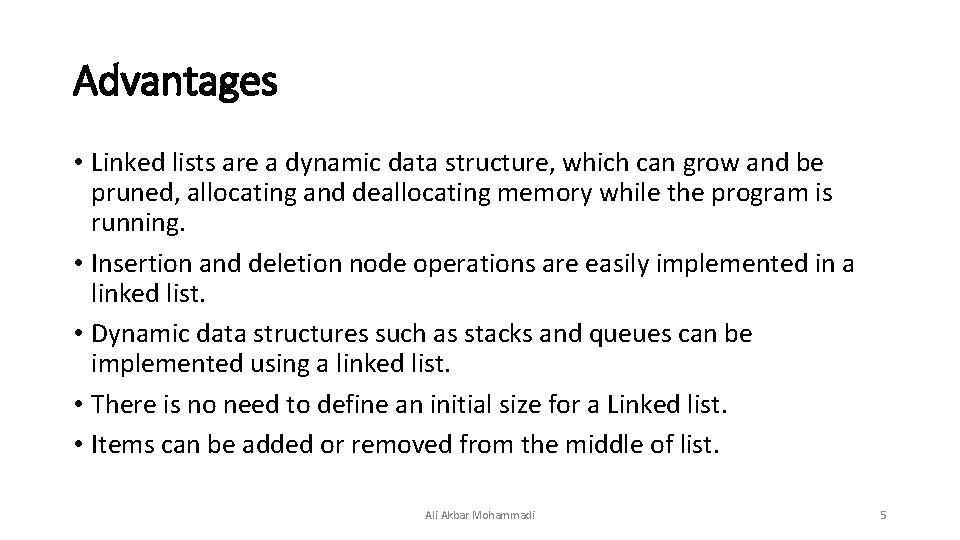
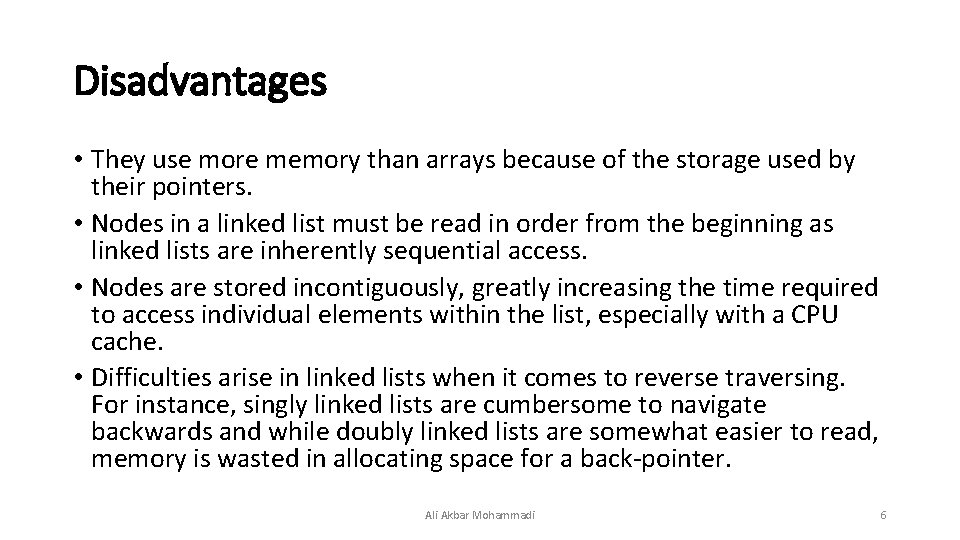
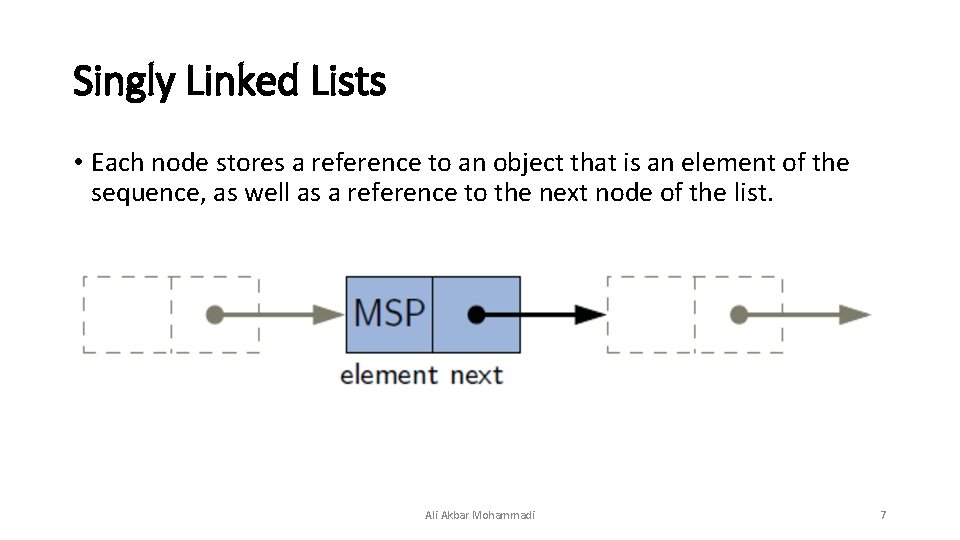
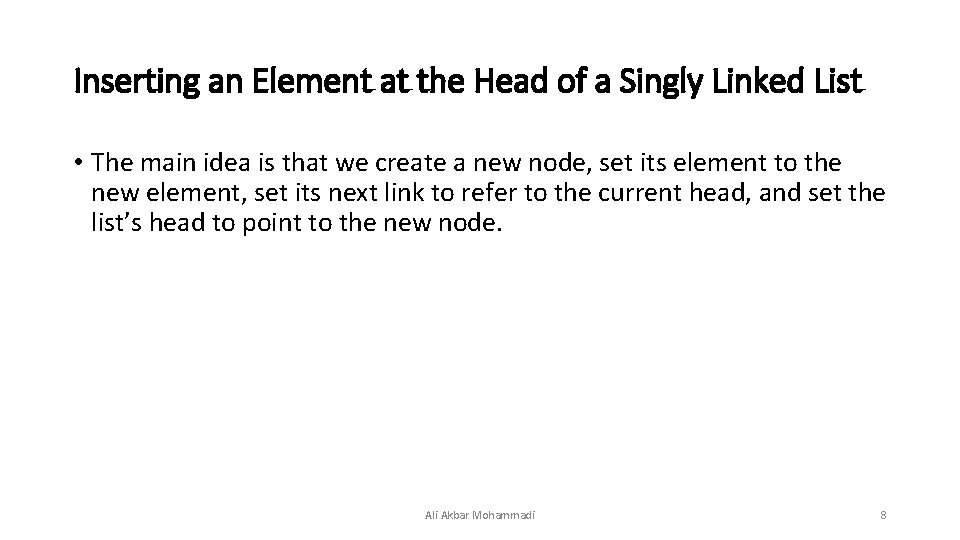
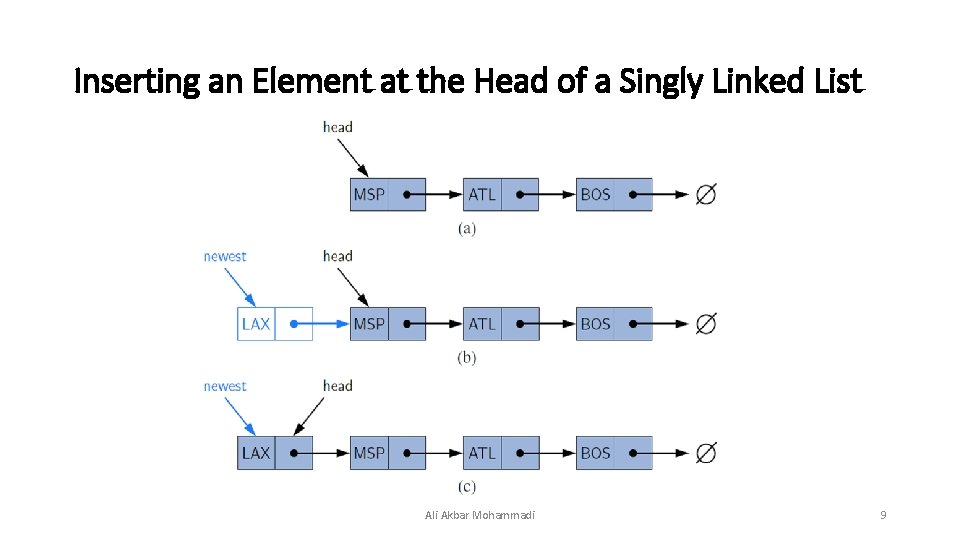
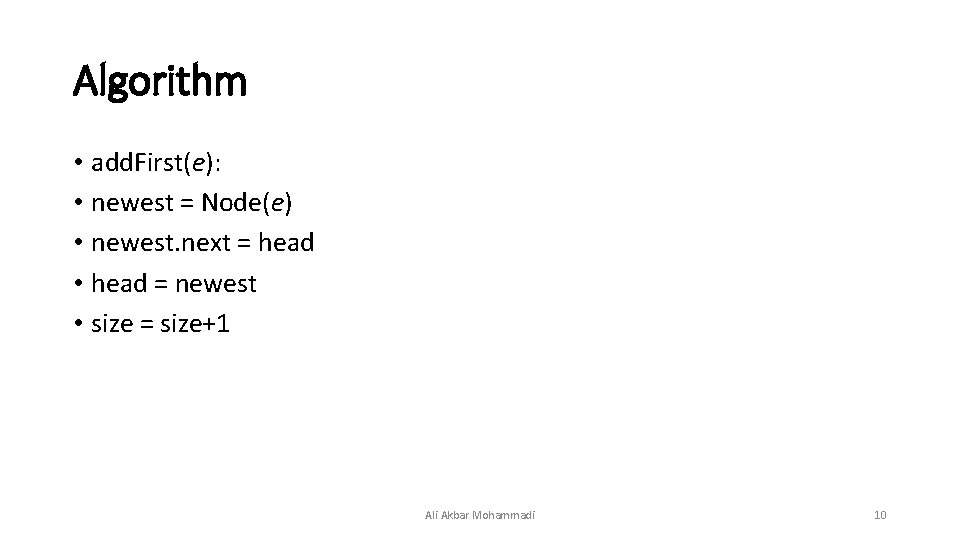
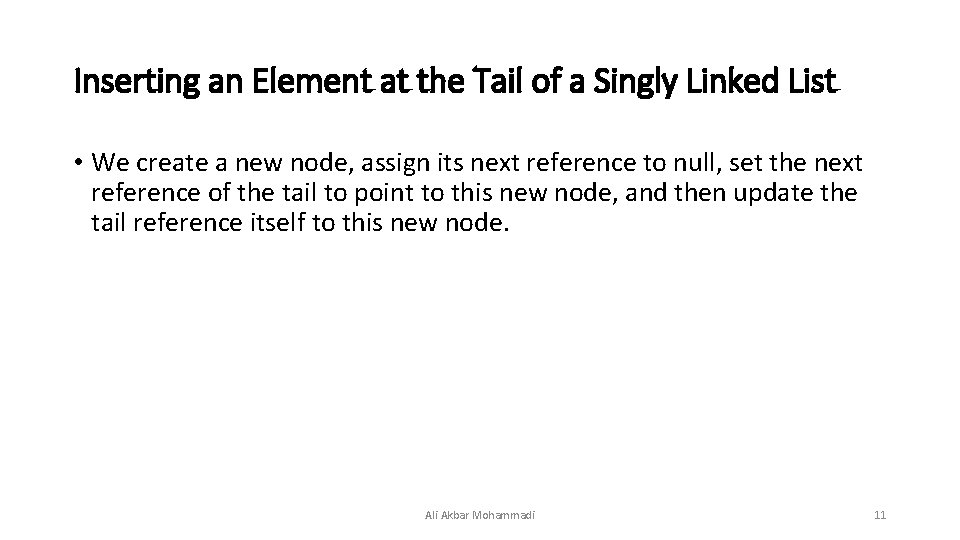
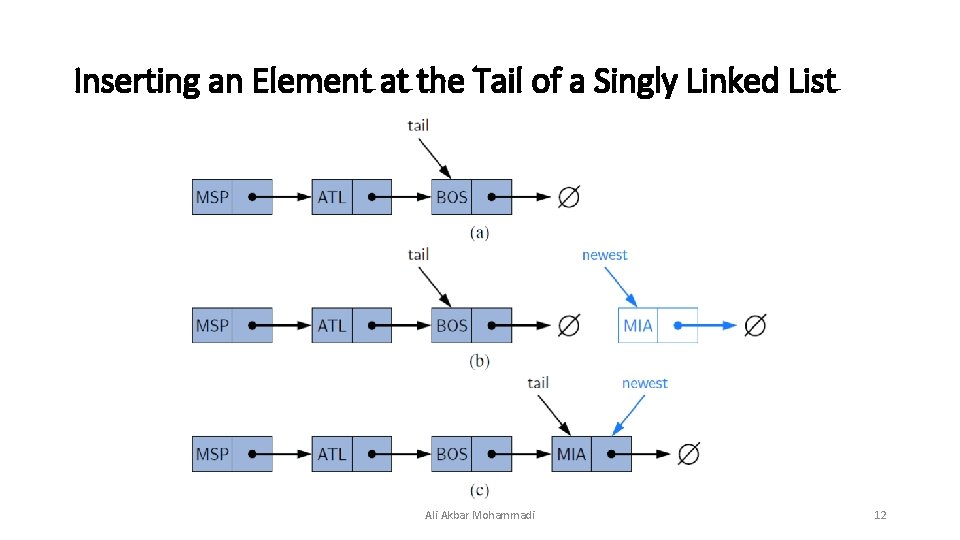
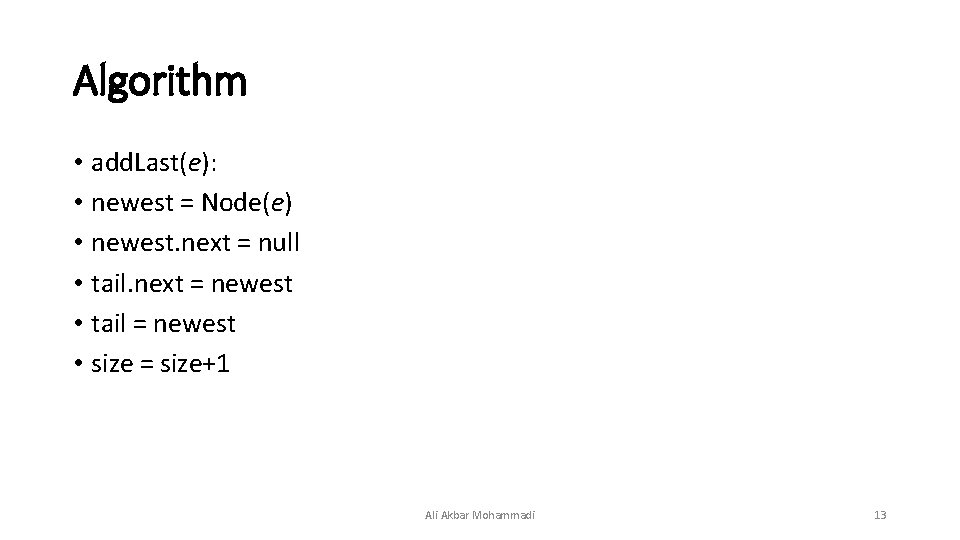
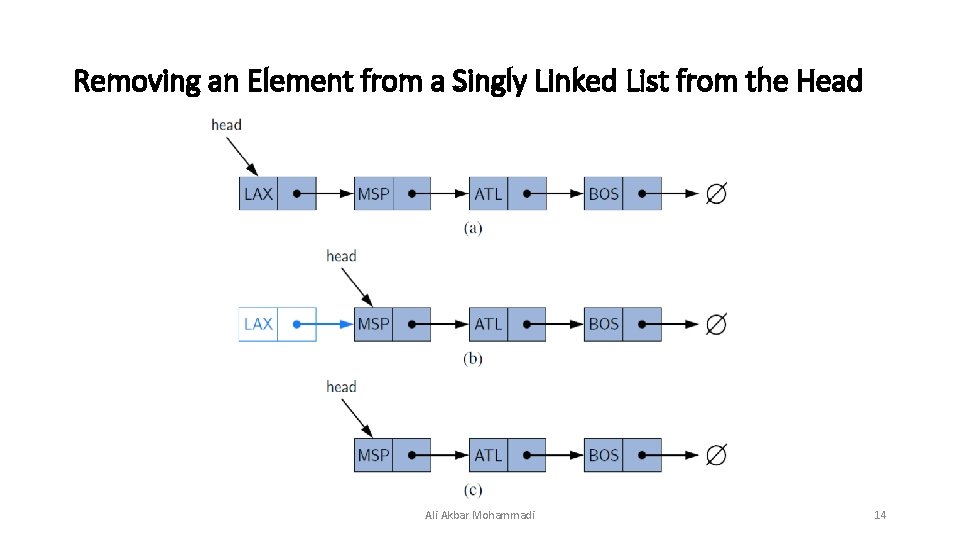
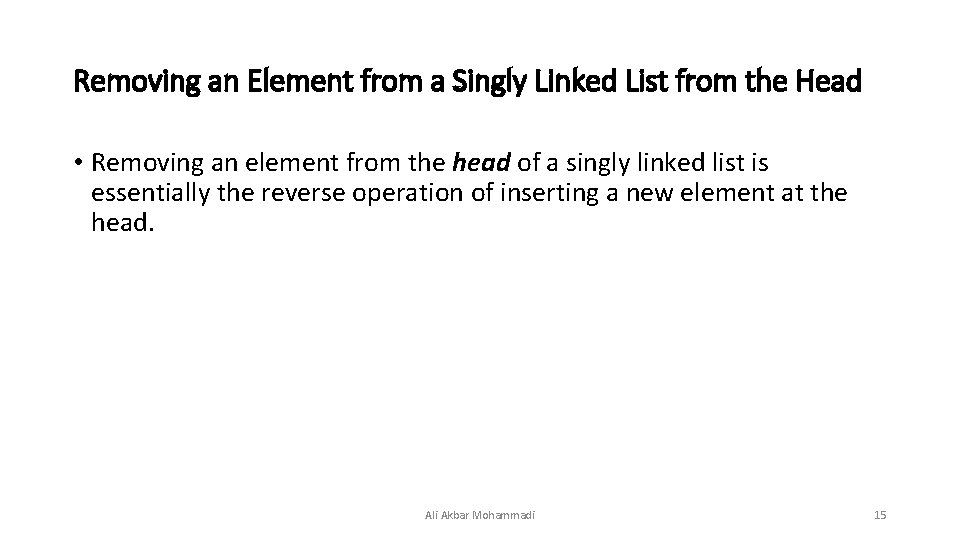
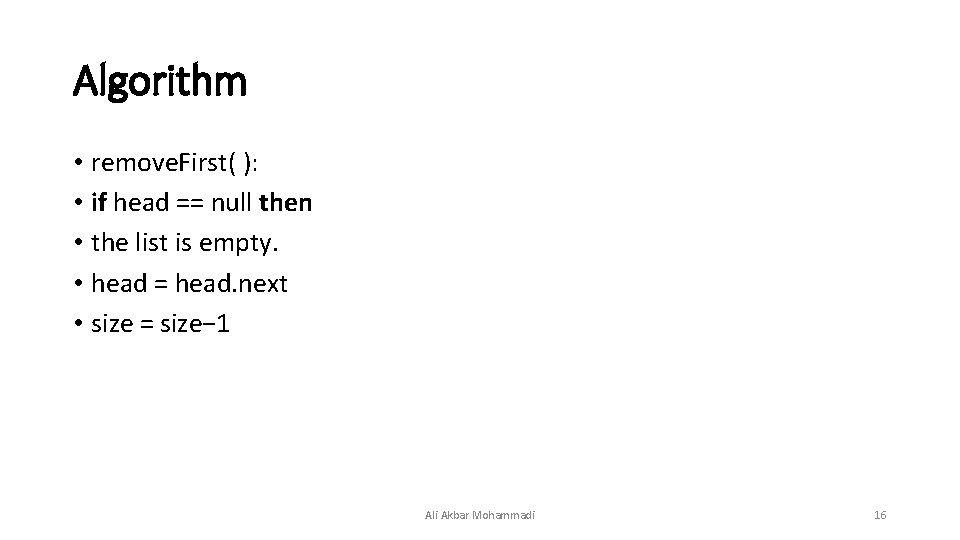
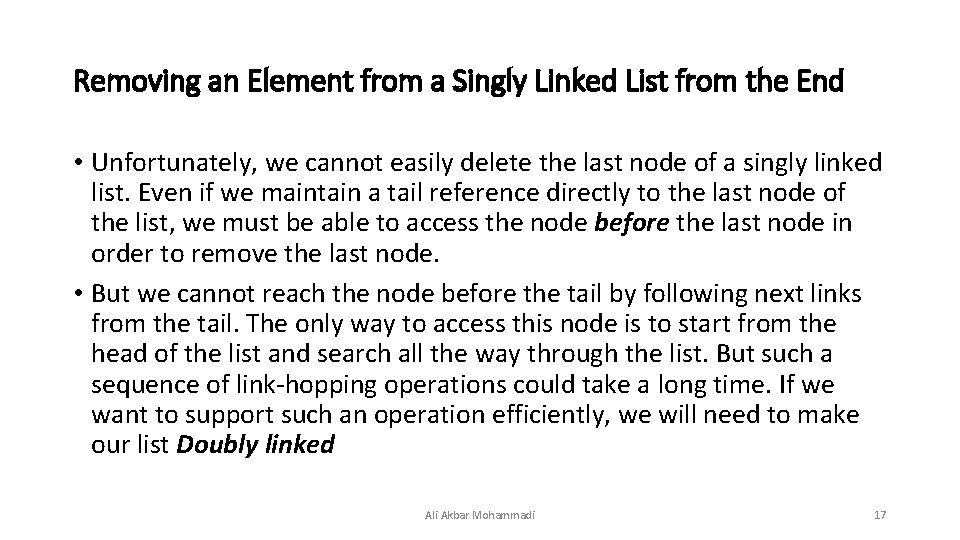
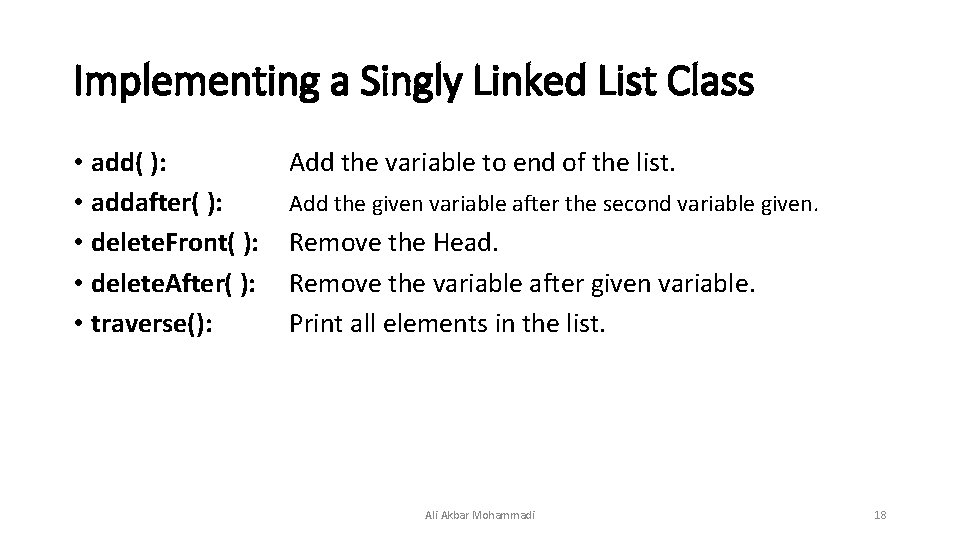
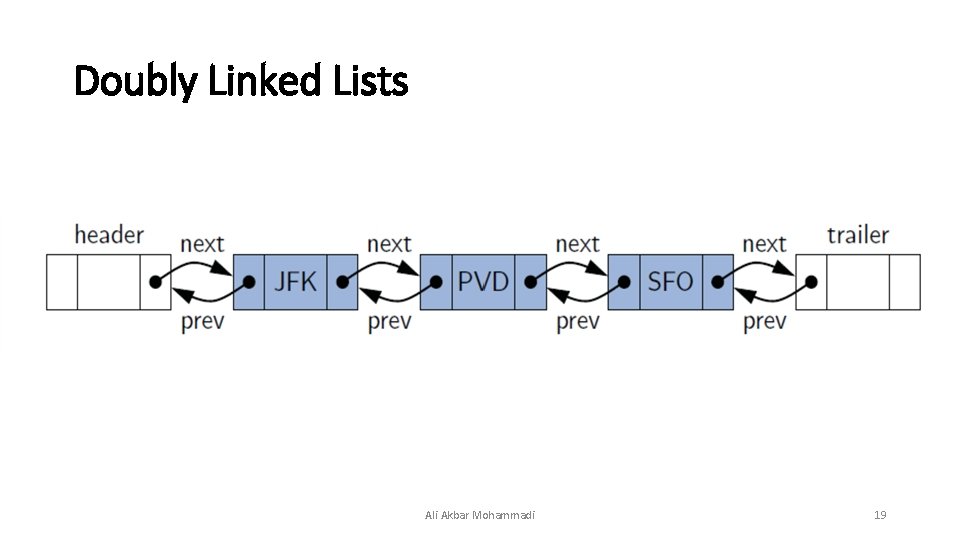
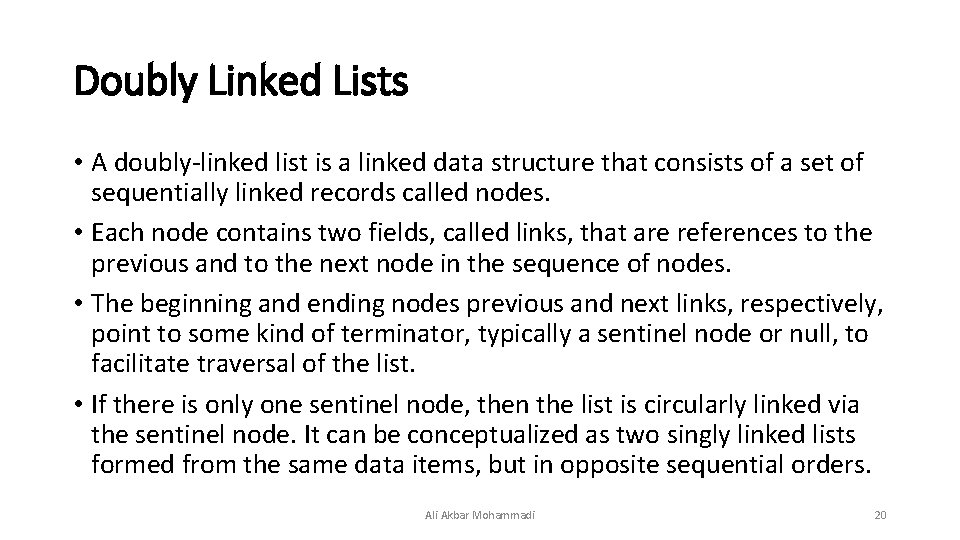
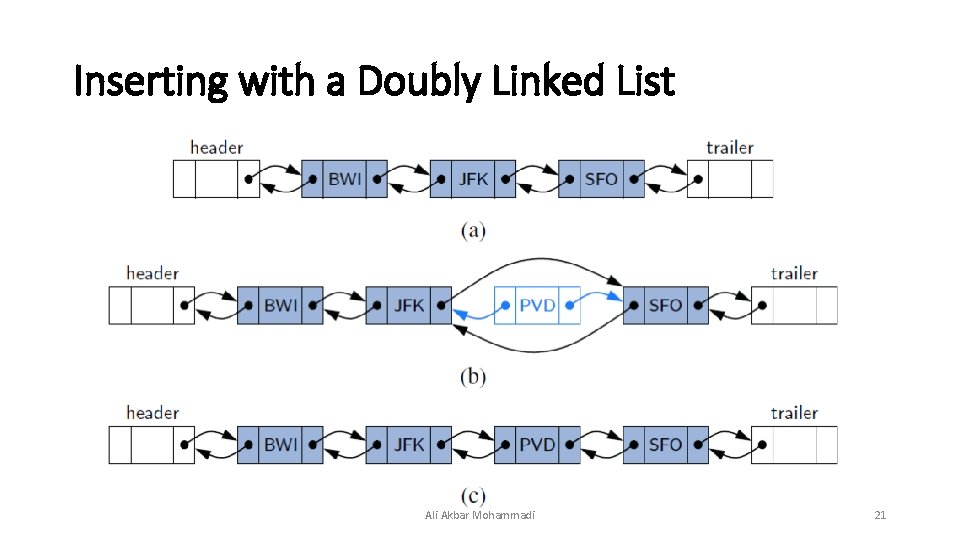
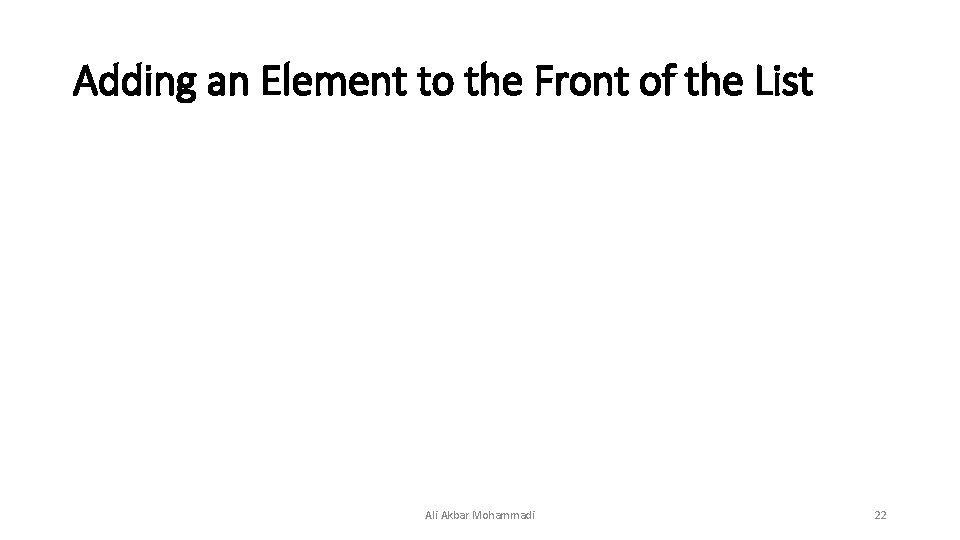
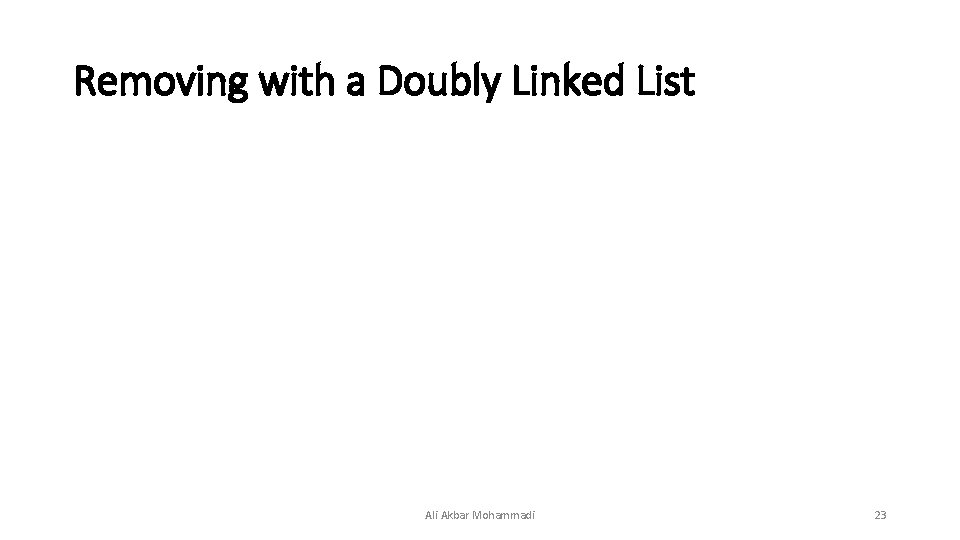
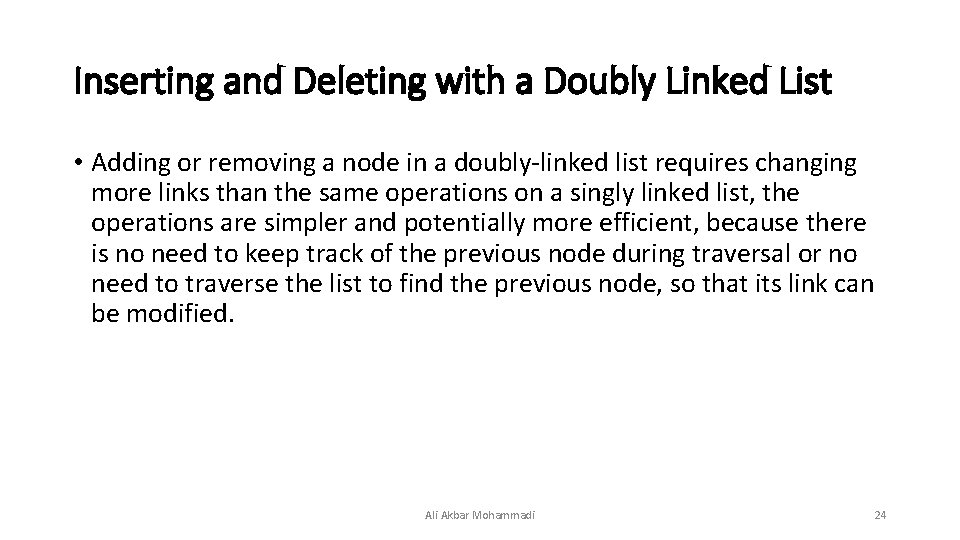
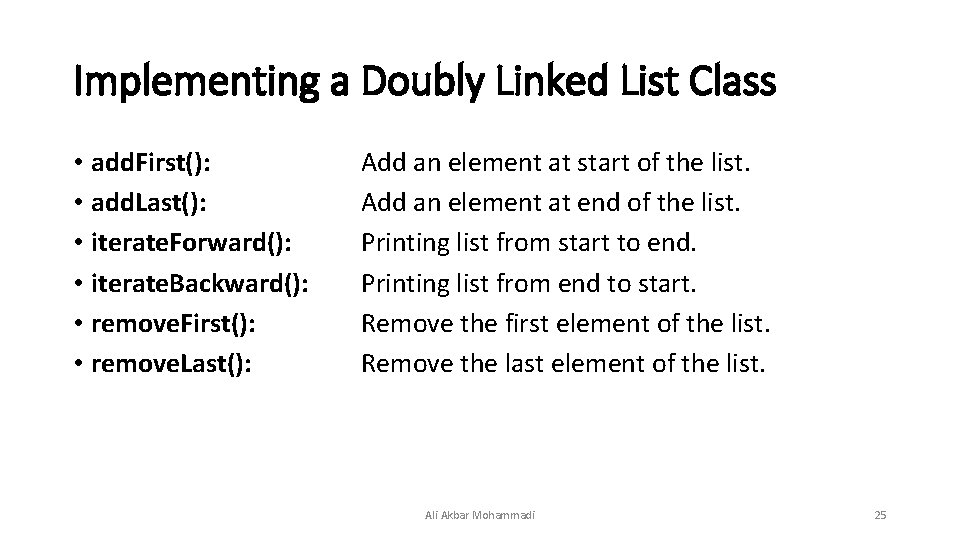
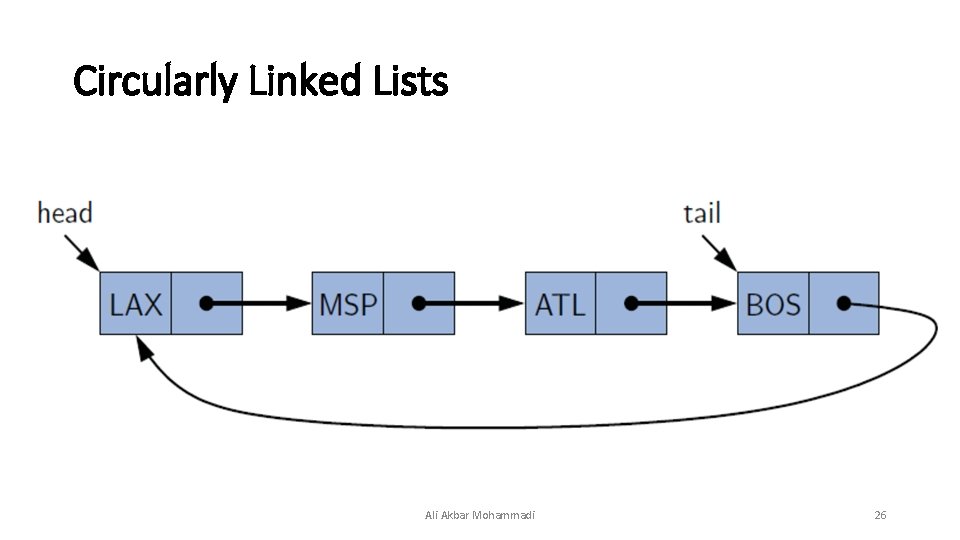
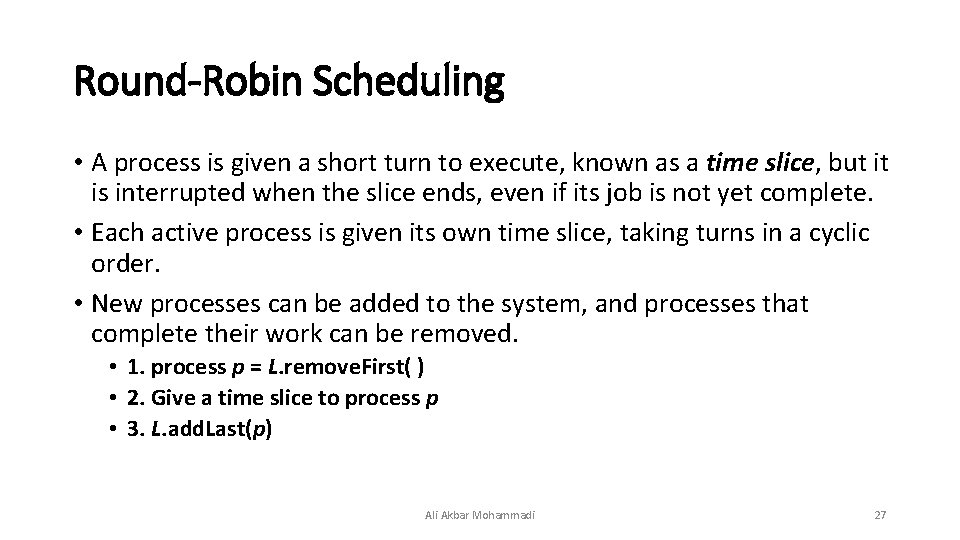
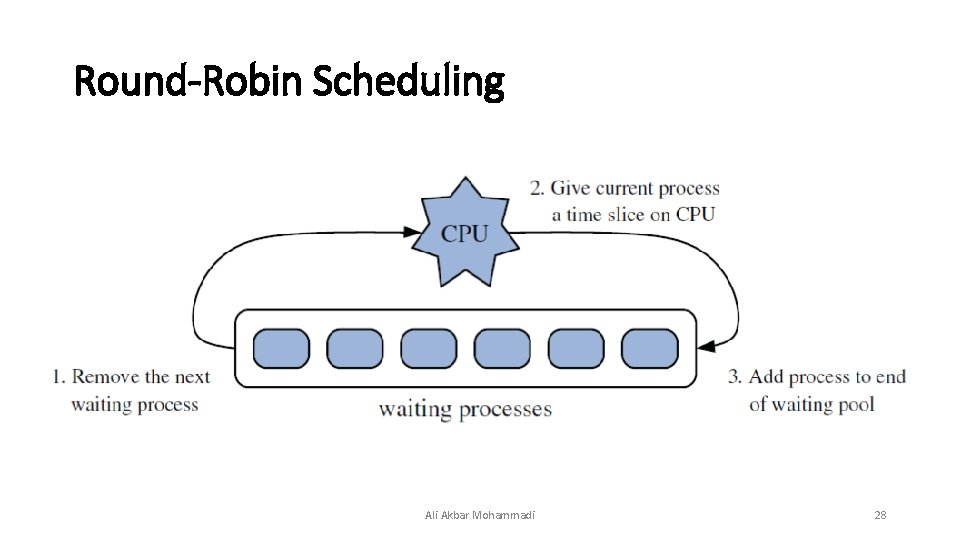
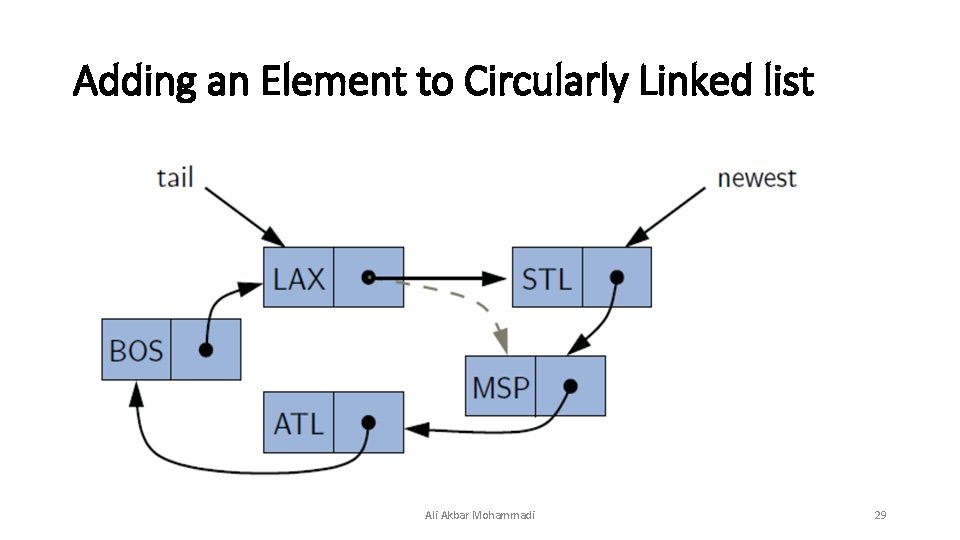
- Slides: 29
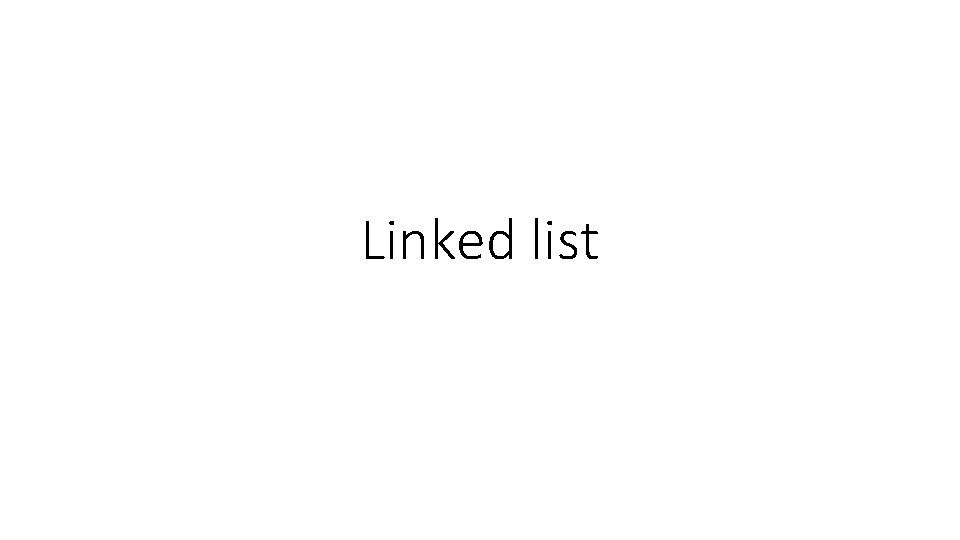
Linked list
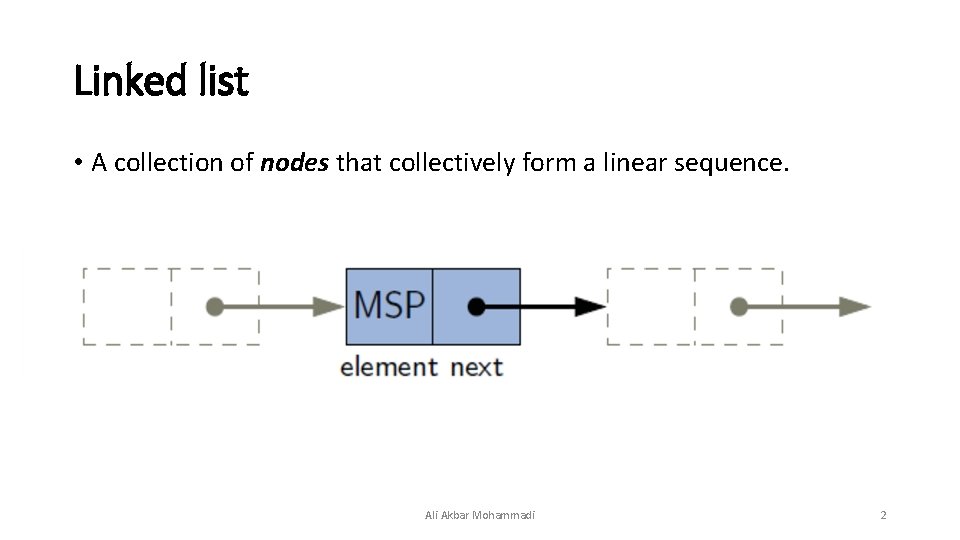
Linked list • A collection of nodes that collectively form a linear sequence. Ali Akbar Mohammadi 2
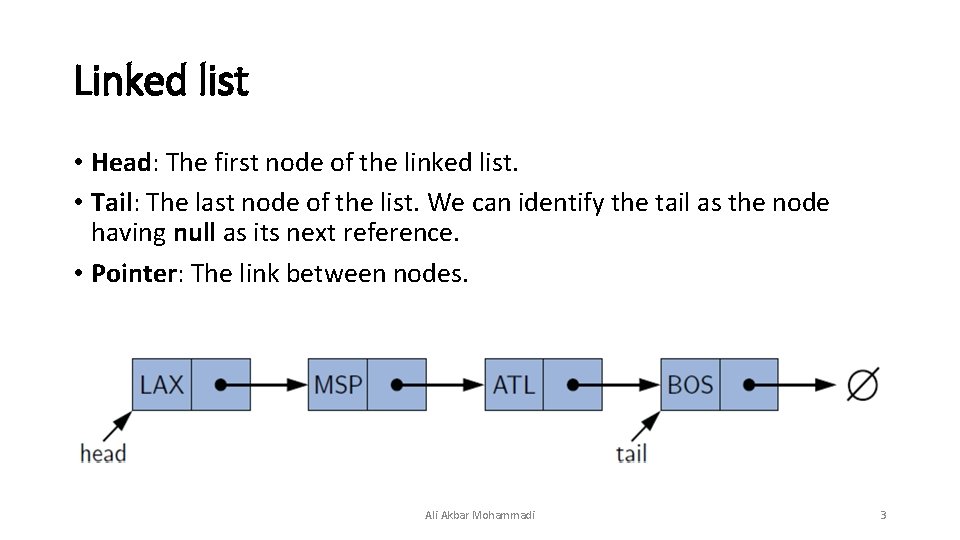
Linked list • Head: The first node of the linked list. • Tail: The last node of the list. We can identify the tail as the node having null as its next reference. • Pointer: The link between nodes. Ali Akbar Mohammadi 3
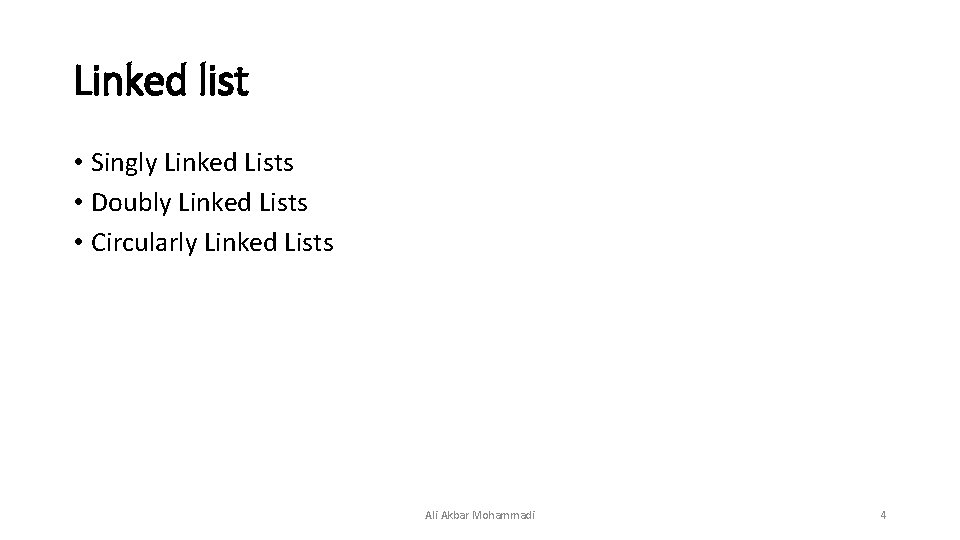
Linked list • Singly Linked Lists • Doubly Linked Lists • Circularly Linked Lists Ali Akbar Mohammadi 4
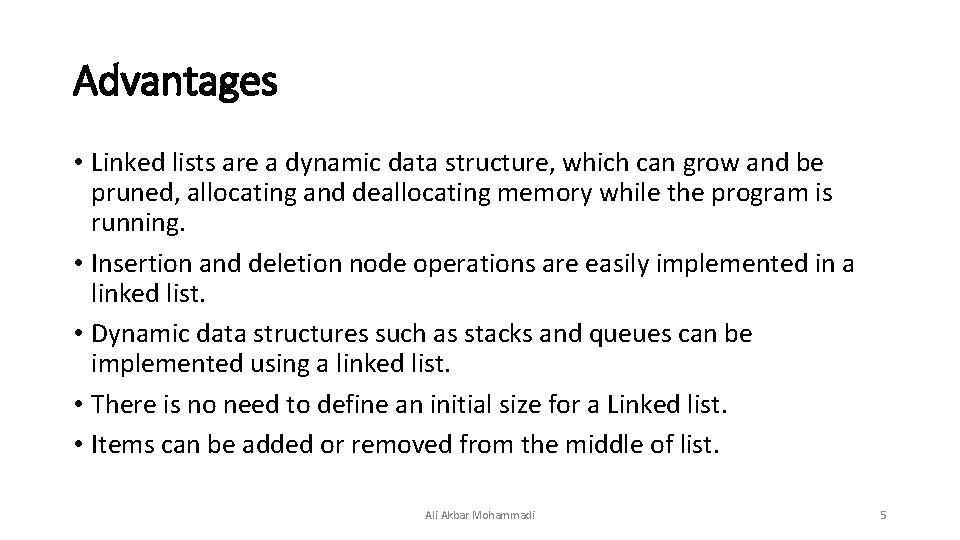
Advantages • Linked lists are a dynamic data structure, which can grow and be pruned, allocating and deallocating memory while the program is running. • Insertion and deletion node operations are easily implemented in a linked list. • Dynamic data structures such as stacks and queues can be implemented using a linked list. • There is no need to define an initial size for a Linked list. • Items can be added or removed from the middle of list. Ali Akbar Mohammadi 5
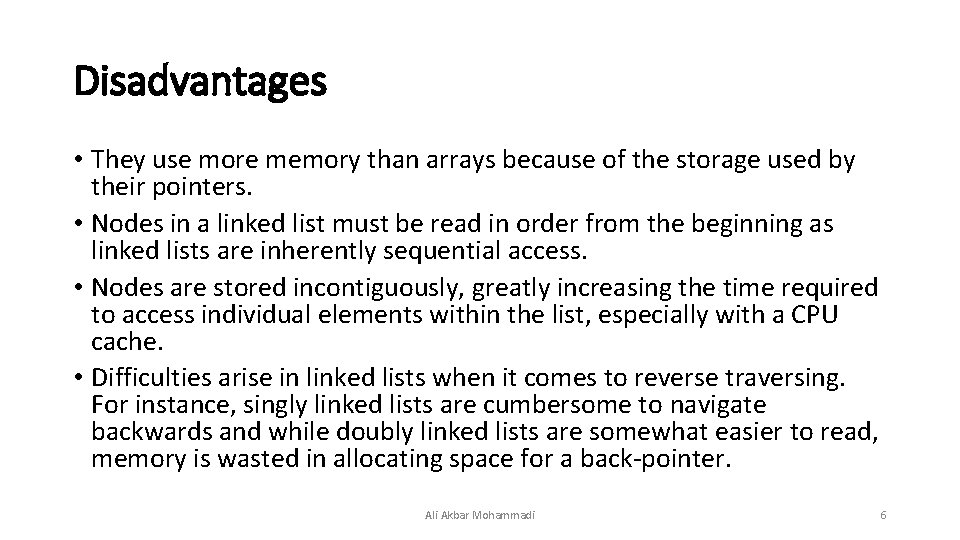
Disadvantages • They use more memory than arrays because of the storage used by their pointers. • Nodes in a linked list must be read in order from the beginning as linked lists are inherently sequential access. • Nodes are stored incontiguously, greatly increasing the time required to access individual elements within the list, especially with a CPU cache. • Difficulties arise in linked lists when it comes to reverse traversing. For instance, singly linked lists are cumbersome to navigate backwards and while doubly linked lists are somewhat easier to read, memory is wasted in allocating space for a back-pointer. Ali Akbar Mohammadi 6
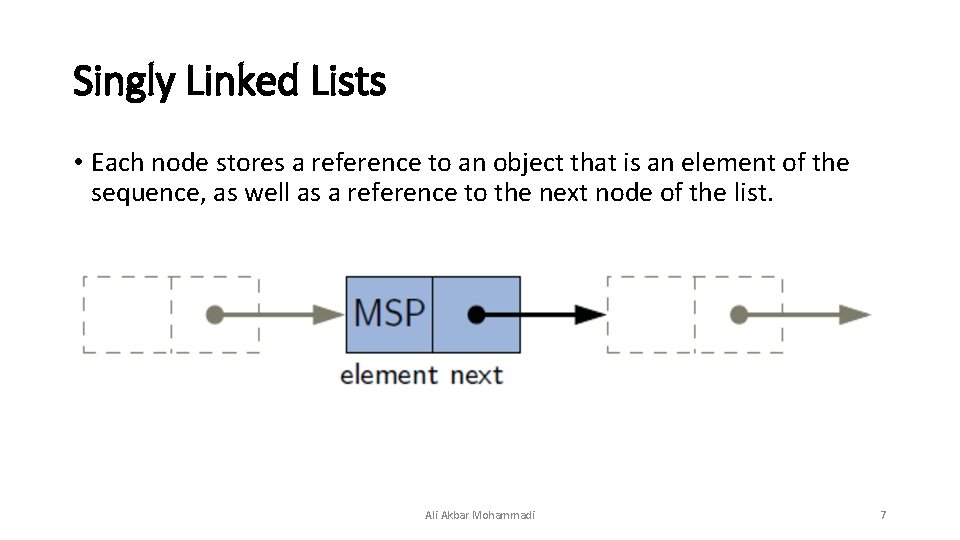
Singly Linked Lists • Each node stores a reference to an object that is an element of the sequence, as well as a reference to the next node of the list. Ali Akbar Mohammadi 7
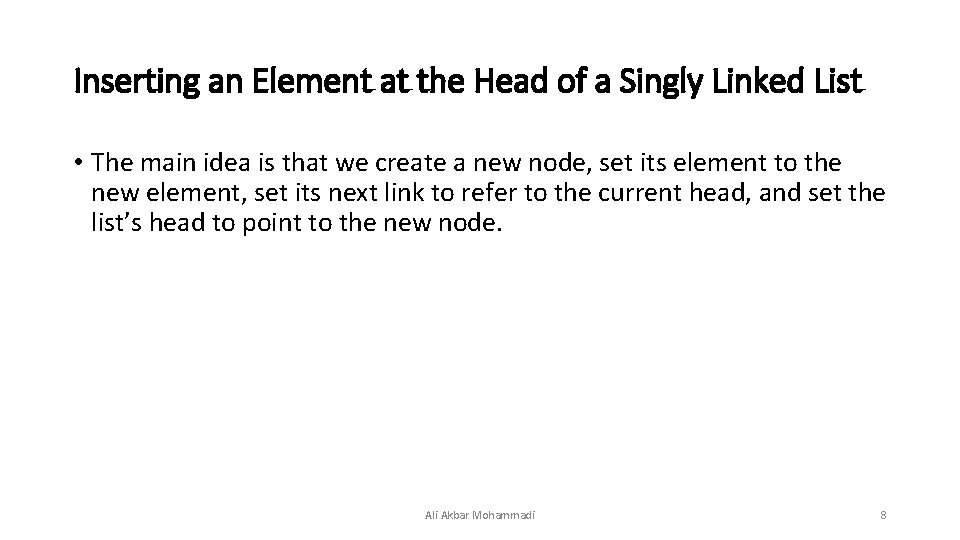
Inserting an Element at the Head of a Singly Linked List • The main idea is that we create a new node, set its element to the new element, set its next link to refer to the current head, and set the list’s head to point to the new node. Ali Akbar Mohammadi 8
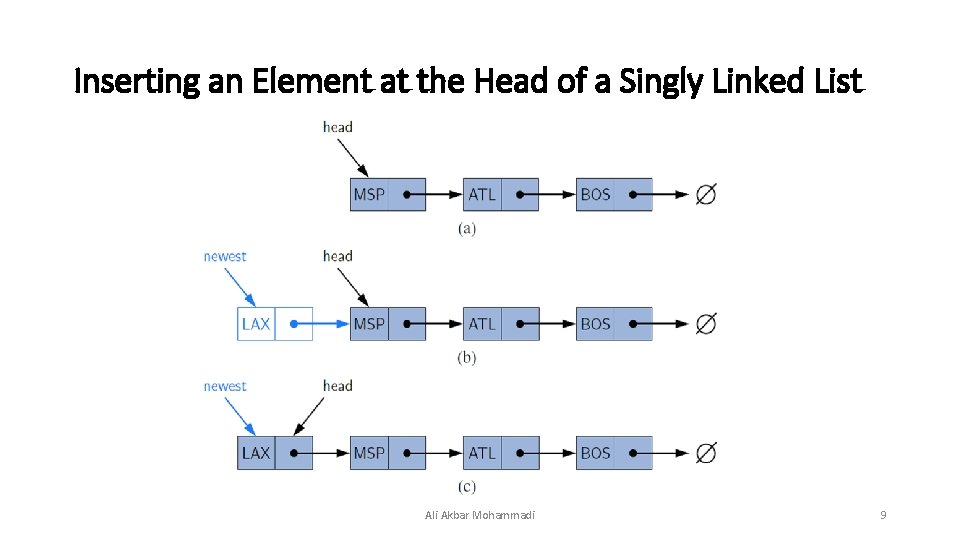
Inserting an Element at the Head of a Singly Linked List Ali Akbar Mohammadi 9
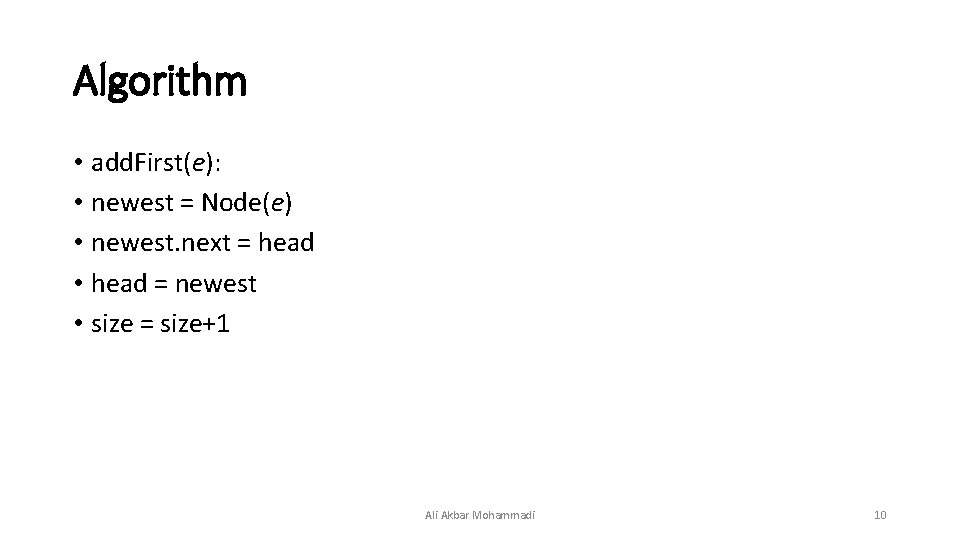
Algorithm • add. First(e): • newest = Node(e) • newest. next = head • head = newest • size = size+1 Ali Akbar Mohammadi 10
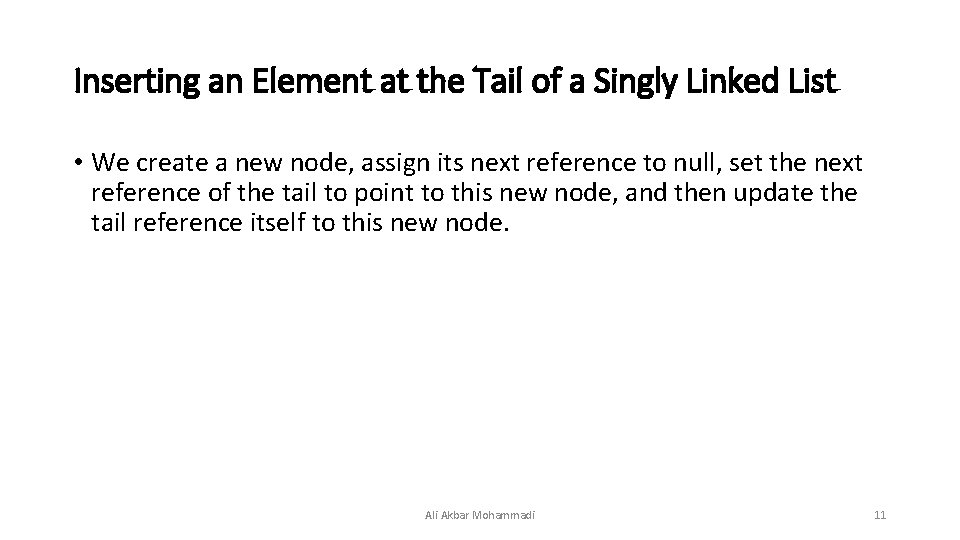
Inserting an Element at the Tail of a Singly Linked List • We create a new node, assign its next reference to null, set the next reference of the tail to point to this new node, and then update the tail reference itself to this new node. Ali Akbar Mohammadi 11
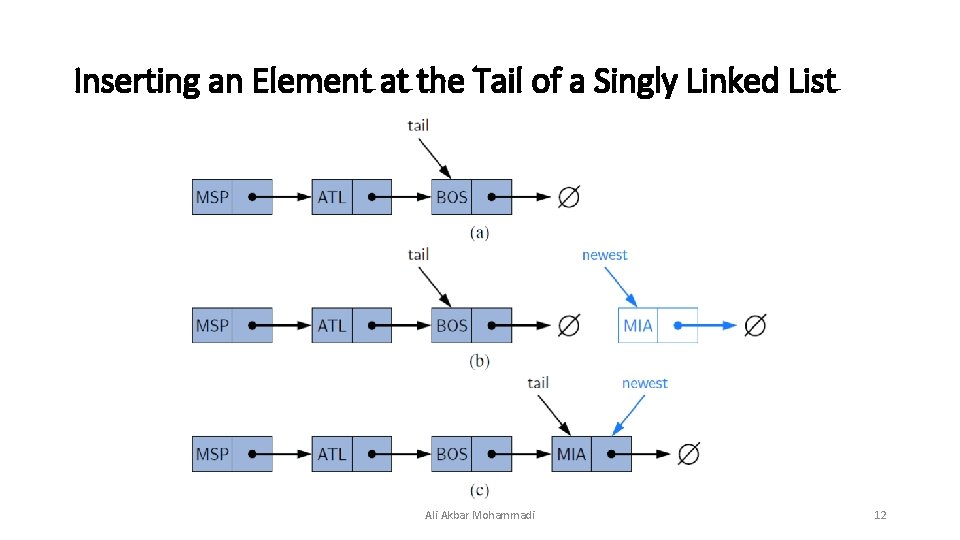
Inserting an Element at the Tail of a Singly Linked List Ali Akbar Mohammadi 12
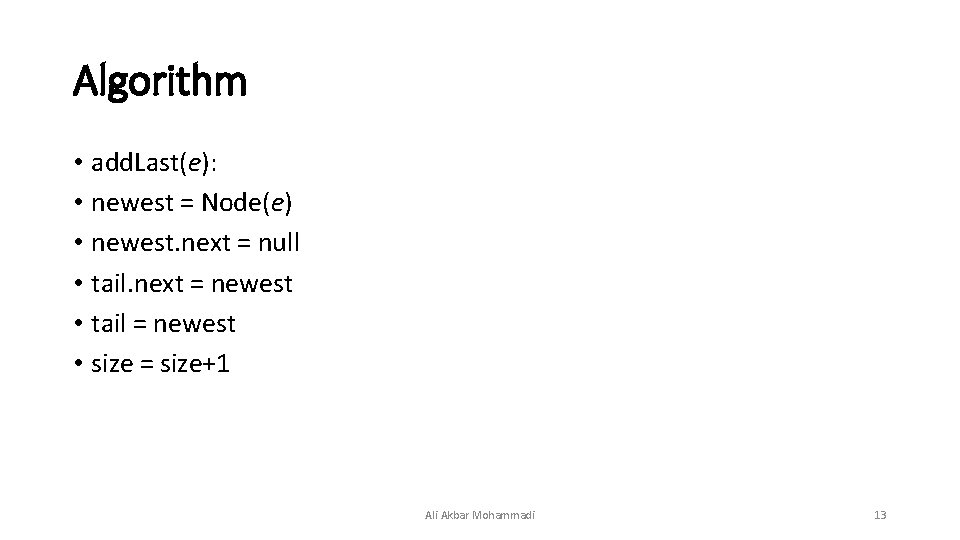
Algorithm • add. Last(e): • newest = Node(e) • newest. next = null • tail. next = newest • tail = newest • size = size+1 Ali Akbar Mohammadi 13
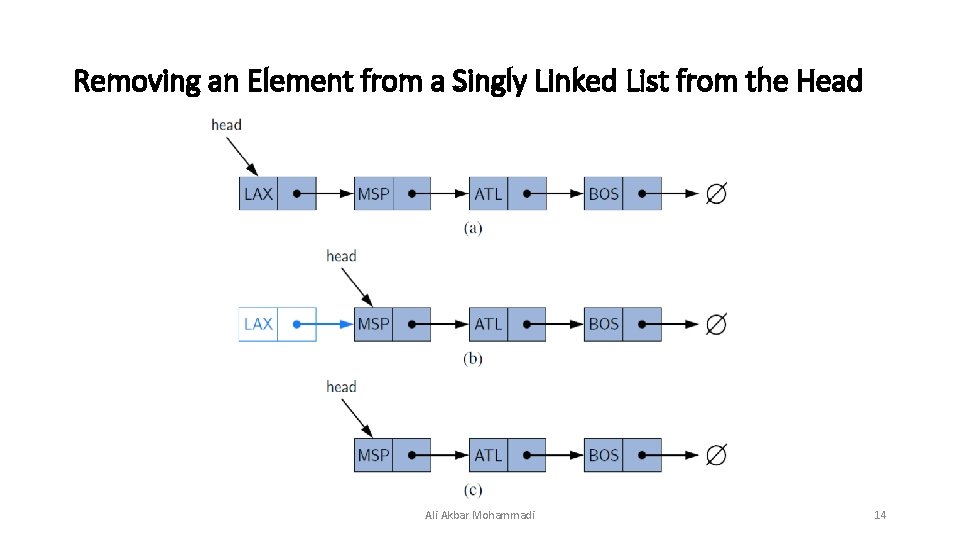
Removing an Element from a Singly Linked List from the Head Ali Akbar Mohammadi 14
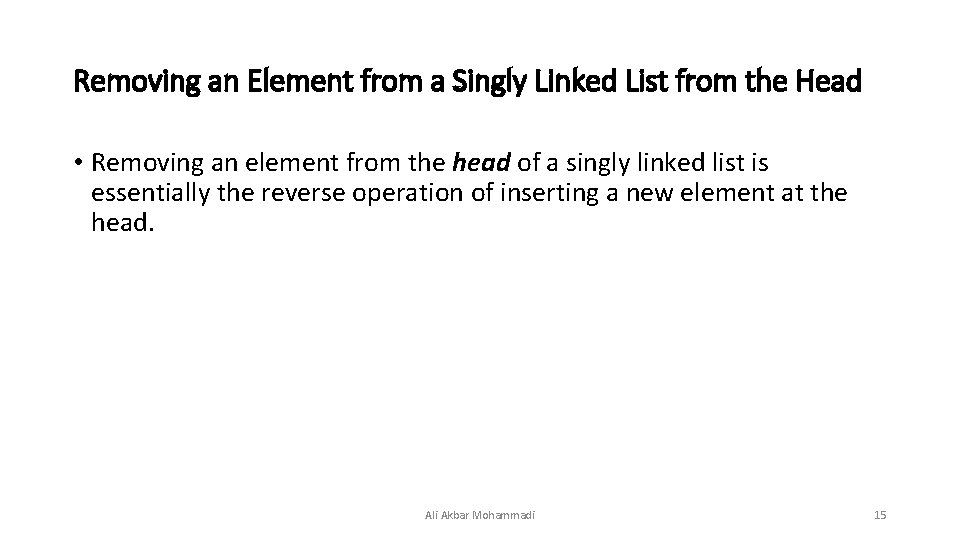
Removing an Element from a Singly Linked List from the Head • Removing an element from the head of a singly linked list is essentially the reverse operation of inserting a new element at the head. Ali Akbar Mohammadi 15
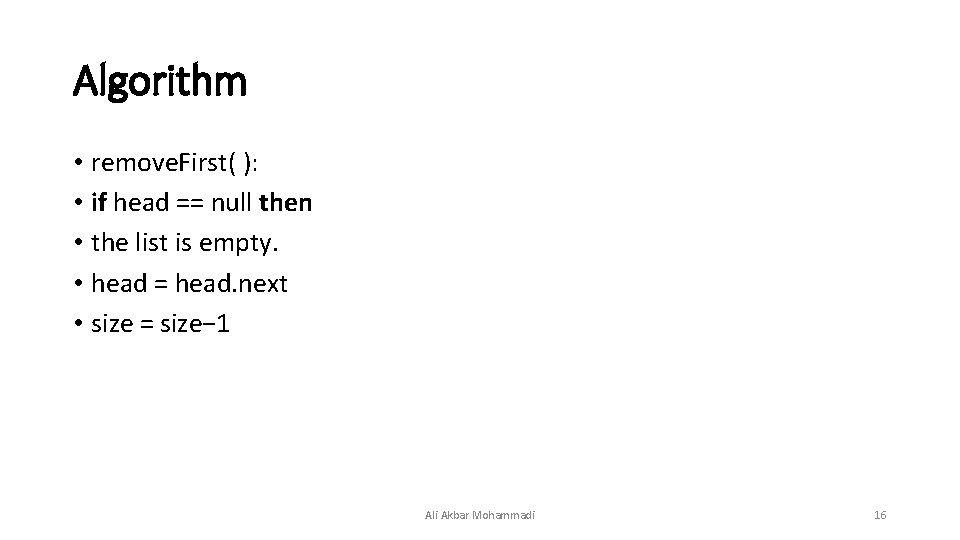
Algorithm • remove. First( ): • if head == null then • the list is empty. • head = head. next • size = size− 1 Ali Akbar Mohammadi 16
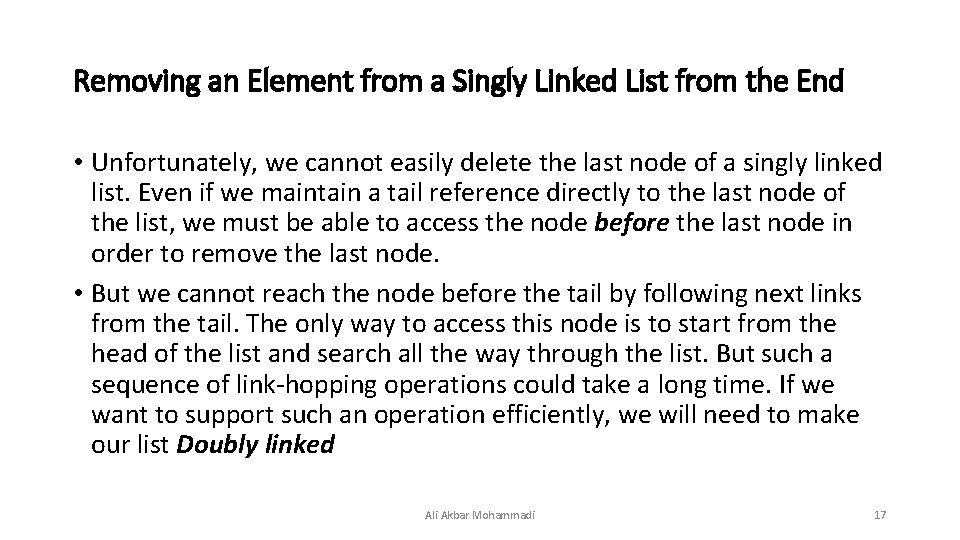
Removing an Element from a Singly Linked List from the End • Unfortunately, we cannot easily delete the last node of a singly linked list. Even if we maintain a tail reference directly to the last node of the list, we must be able to access the node before the last node in order to remove the last node. • But we cannot reach the node before the tail by following next links from the tail. The only way to access this node is to start from the head of the list and search all the way through the list. But such a sequence of link-hopping operations could take a long time. If we want to support such an operation efficiently, we will need to make our list Doubly linked Ali Akbar Mohammadi 17
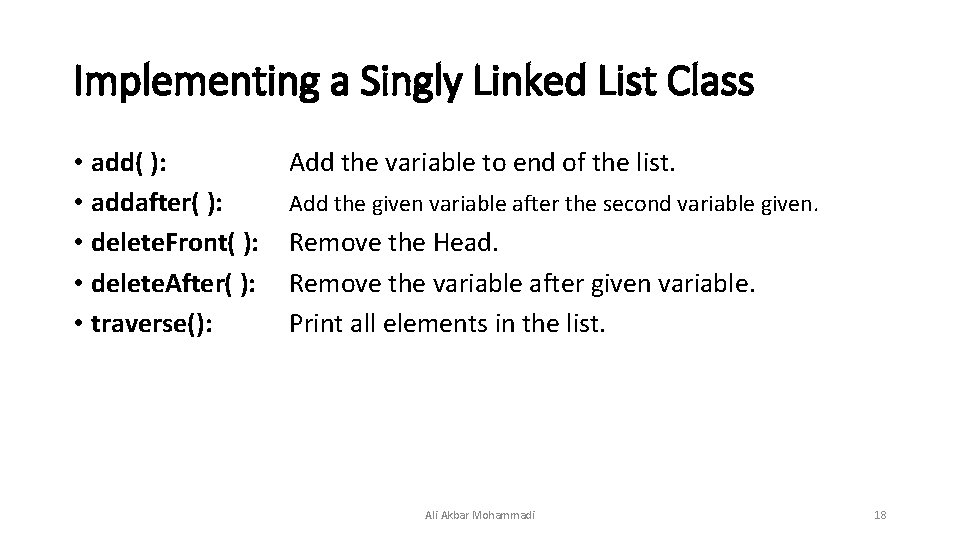
Implementing a Singly Linked List Class • add( ): • addafter( ): • delete. Front( ): • delete. After( ): • traverse(): Add the variable to end of the list. Add the given variable after the second variable given. Remove the Head. Remove the variable after given variable. Print all elements in the list. Ali Akbar Mohammadi 18
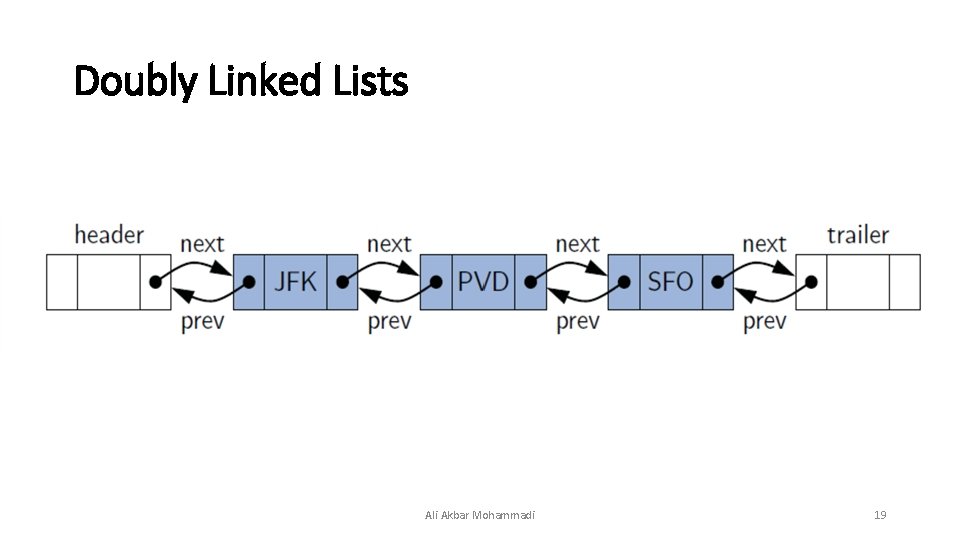
Doubly Linked Lists Ali Akbar Mohammadi 19
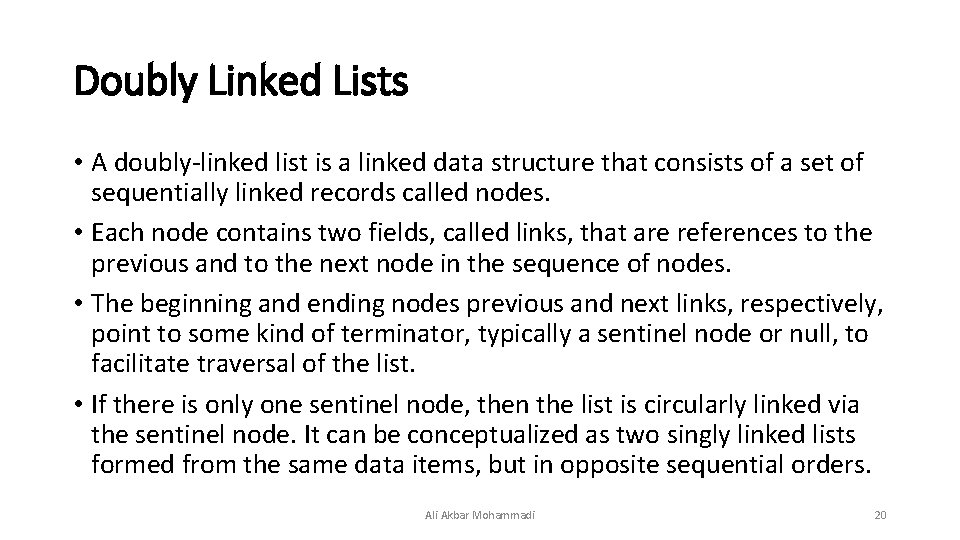
Doubly Linked Lists • A doubly-linked list is a linked data structure that consists of a set of sequentially linked records called nodes. • Each node contains two fields, called links, that are references to the previous and to the next node in the sequence of nodes. • The beginning and ending nodes previous and next links, respectively, point to some kind of terminator, typically a sentinel node or null, to facilitate traversal of the list. • If there is only one sentinel node, then the list is circularly linked via the sentinel node. It can be conceptualized as two singly linked lists formed from the same data items, but in opposite sequential orders. Ali Akbar Mohammadi 20
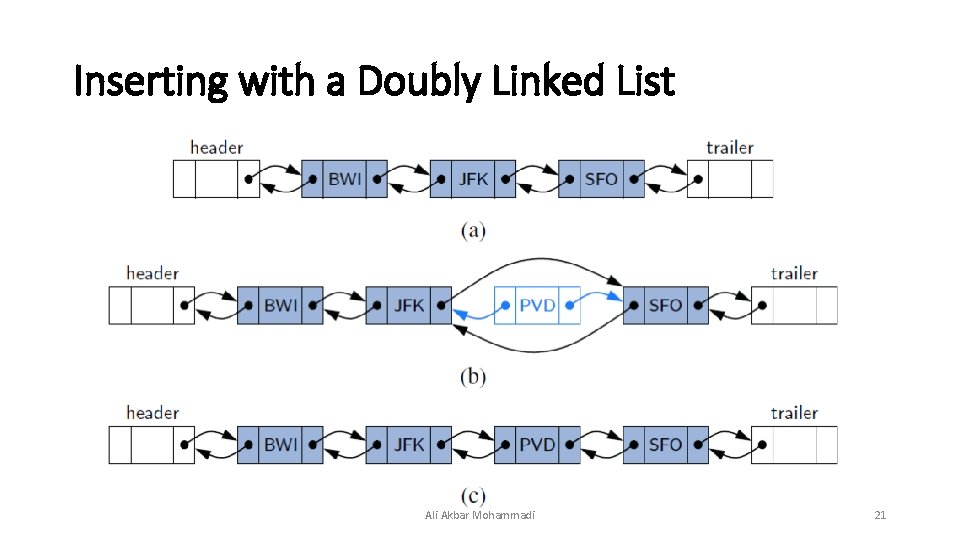
Inserting with a Doubly Linked List Ali Akbar Mohammadi 21
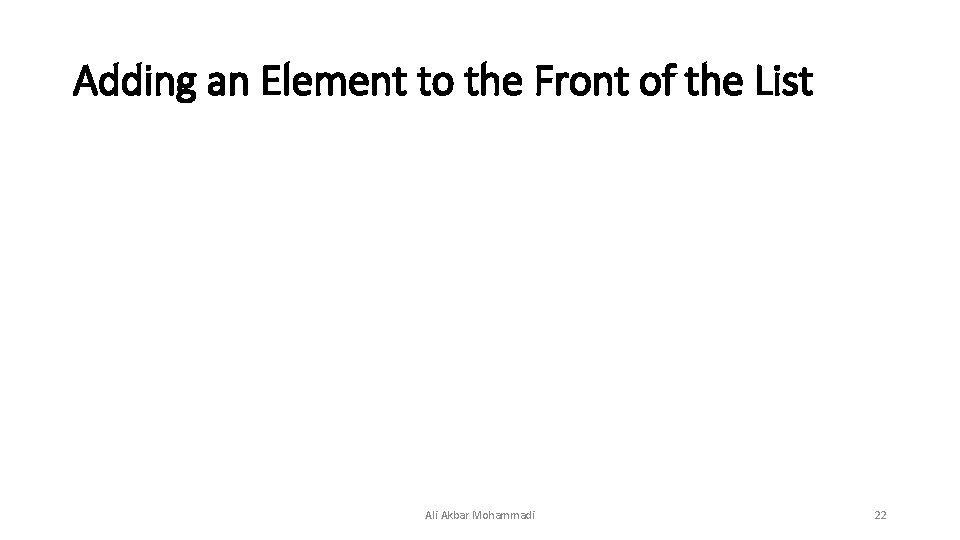
Adding an Element to the Front of the List Ali Akbar Mohammadi 22
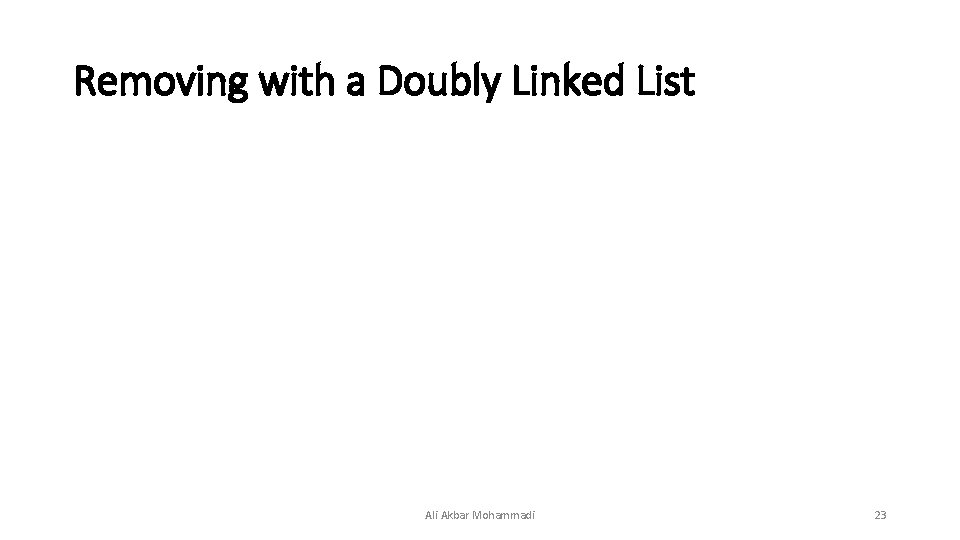
Removing with a Doubly Linked List Ali Akbar Mohammadi 23
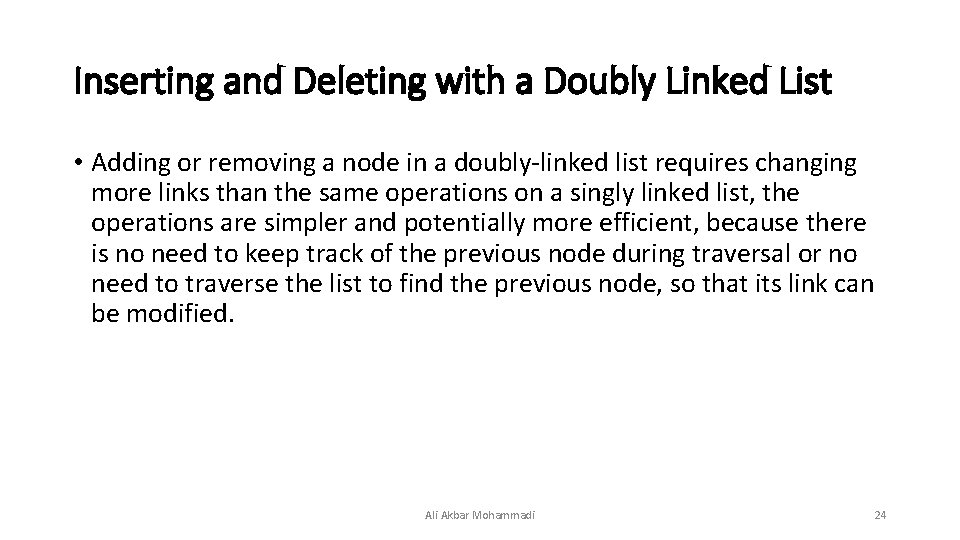
Inserting and Deleting with a Doubly Linked List • Adding or removing a node in a doubly-linked list requires changing more links than the same operations on a singly linked list, the operations are simpler and potentially more efficient, because there is no need to keep track of the previous node during traversal or no need to traverse the list to find the previous node, so that its link can be modified. Ali Akbar Mohammadi 24
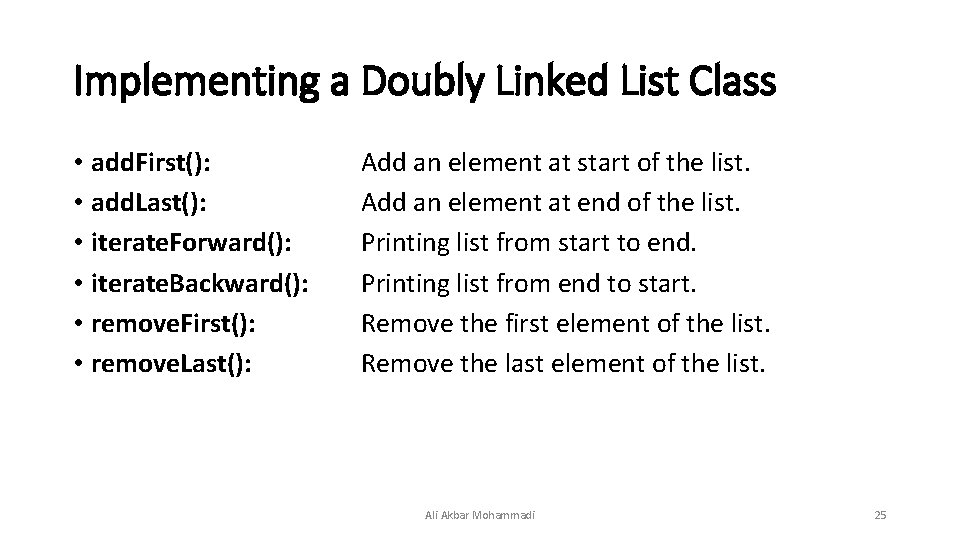
Implementing a Doubly Linked List Class • add. First(): • add. Last(): • iterate. Forward(): • iterate. Backward(): • remove. First(): • remove. Last(): Add an element at start of the list. Add an element at end of the list. Printing list from start to end. Printing list from end to start. Remove the first element of the list. Remove the last element of the list. Ali Akbar Mohammadi 25
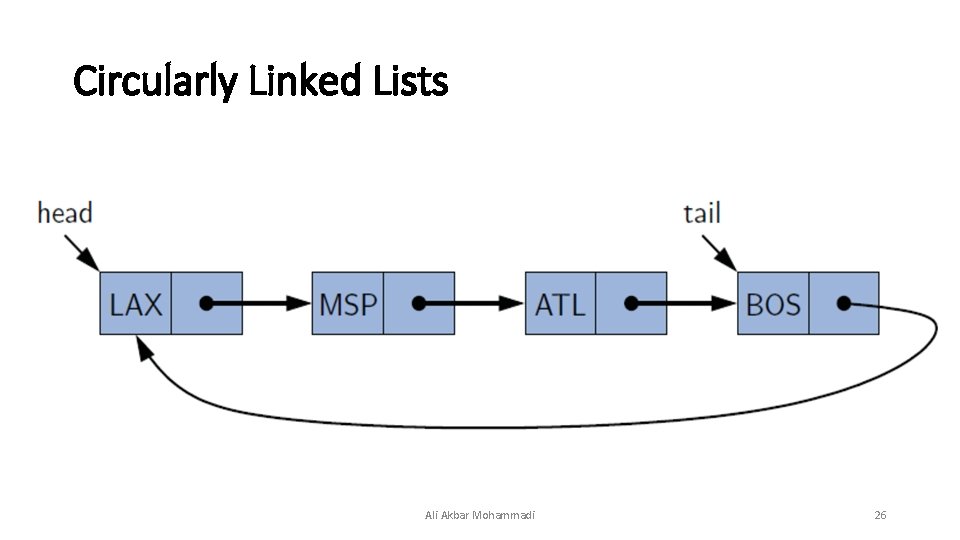
Circularly Linked Lists Ali Akbar Mohammadi 26
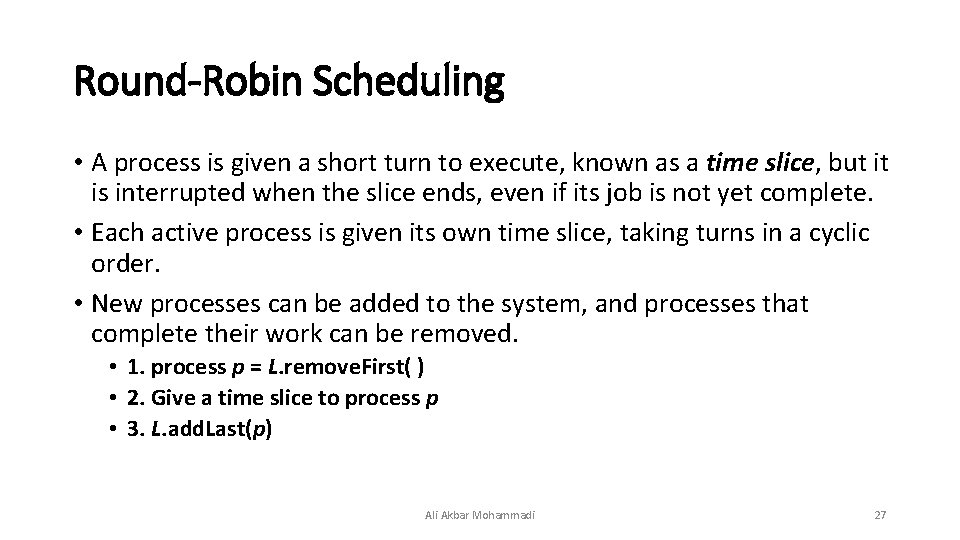
Round-Robin Scheduling • A process is given a short turn to execute, known as a time slice, but it is interrupted when the slice ends, even if its job is not yet complete. • Each active process is given its own time slice, taking turns in a cyclic order. • New processes can be added to the system, and processes that complete their work can be removed. • 1. process p = L. remove. First( ) • 2. Give a time slice to process p • 3. L. add. Last(p) Ali Akbar Mohammadi 27
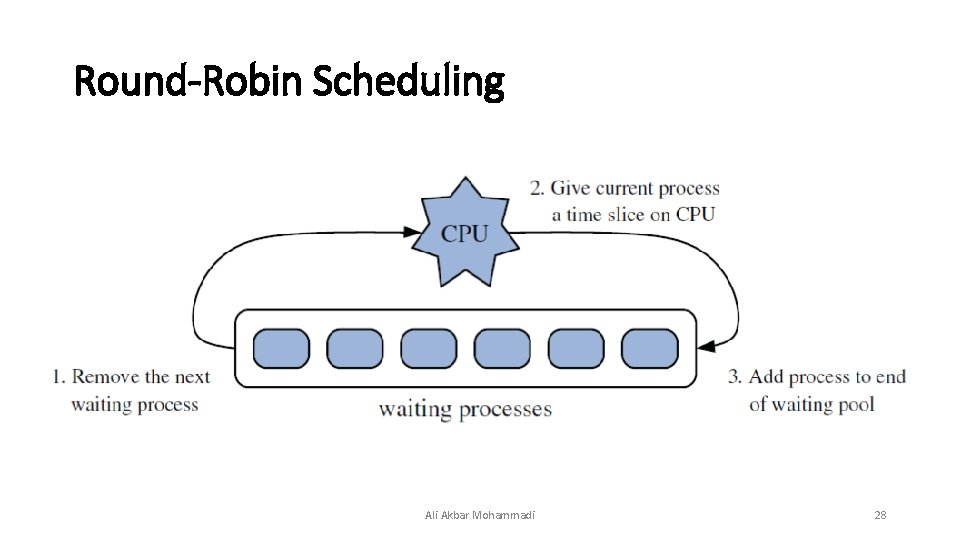
Round-Robin Scheduling Ali Akbar Mohammadi 28
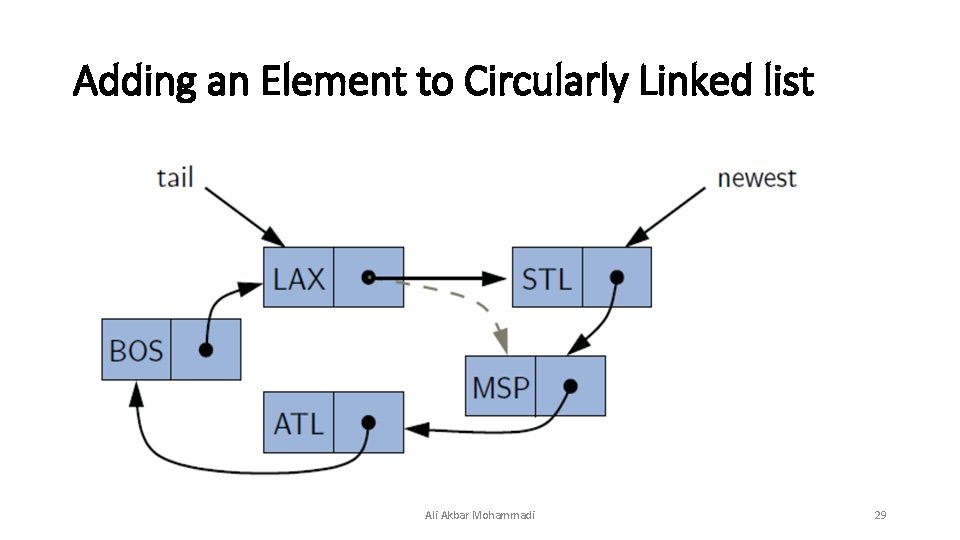
Adding an Element to Circularly Linked list Ali Akbar Mohammadi 29