Lecture Seven Functions Functions In mathematics a function
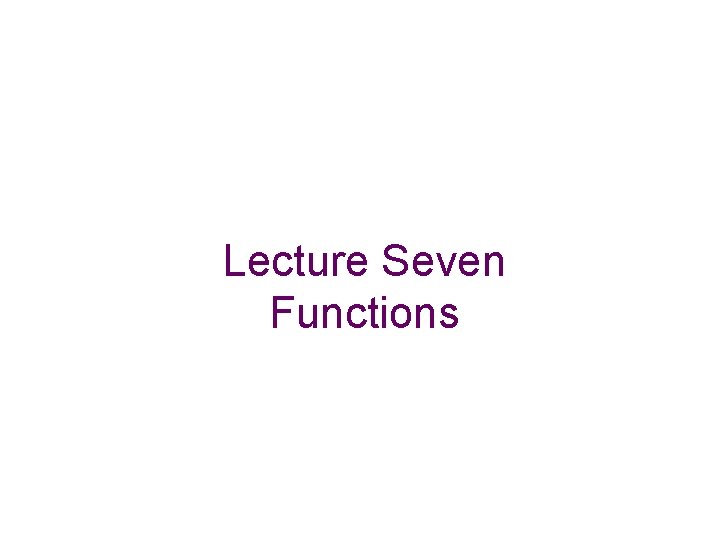
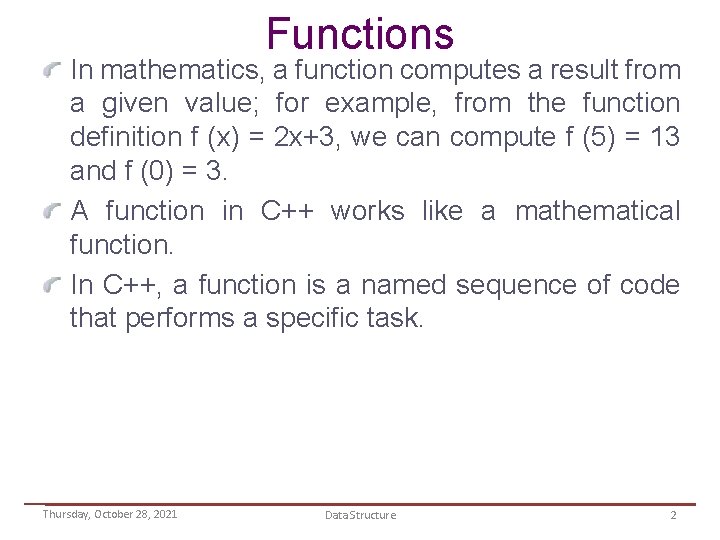
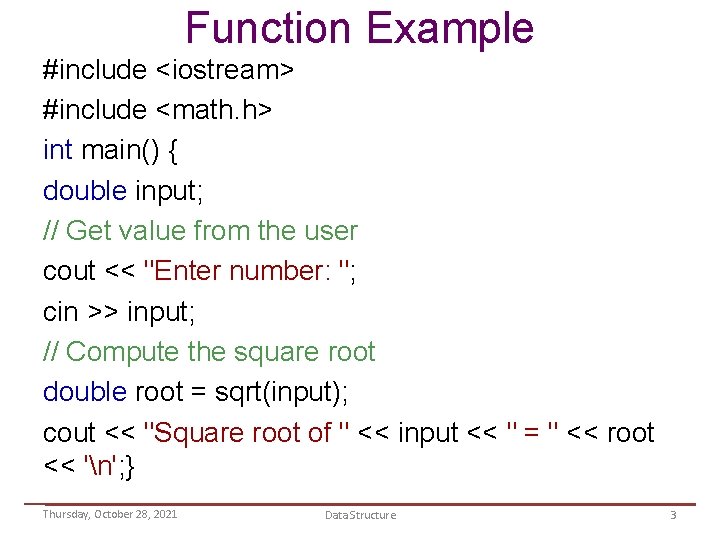
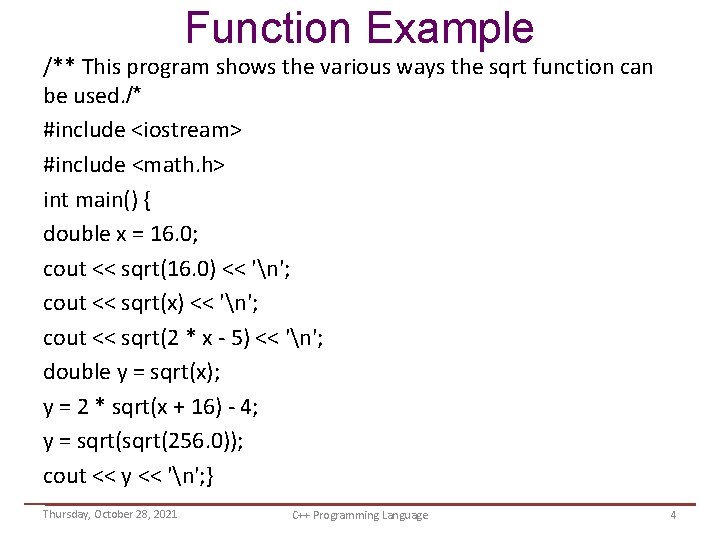
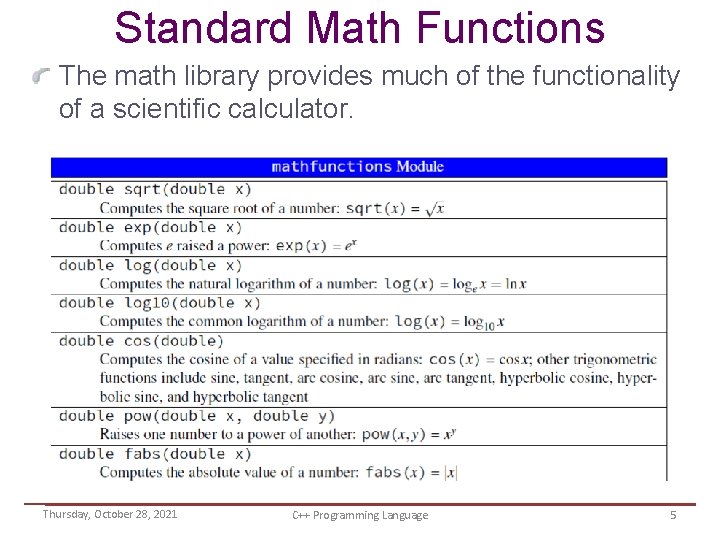
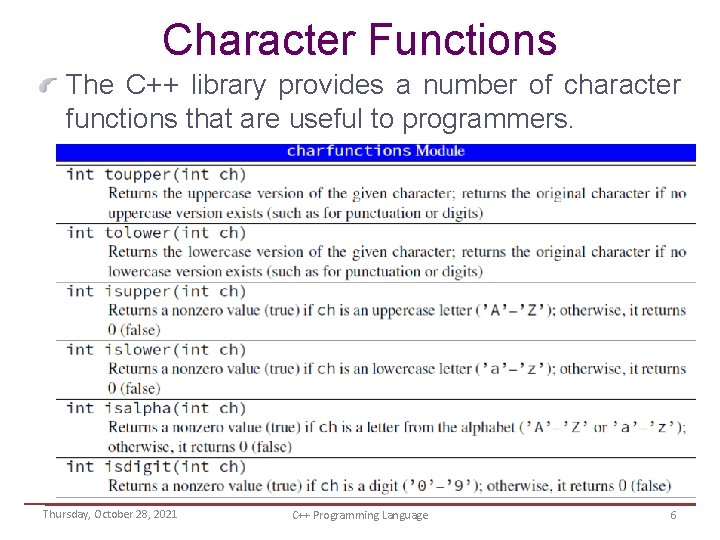
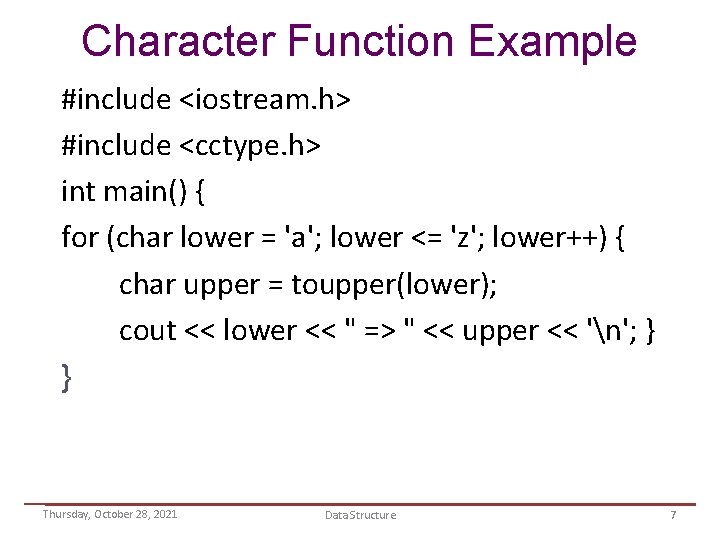
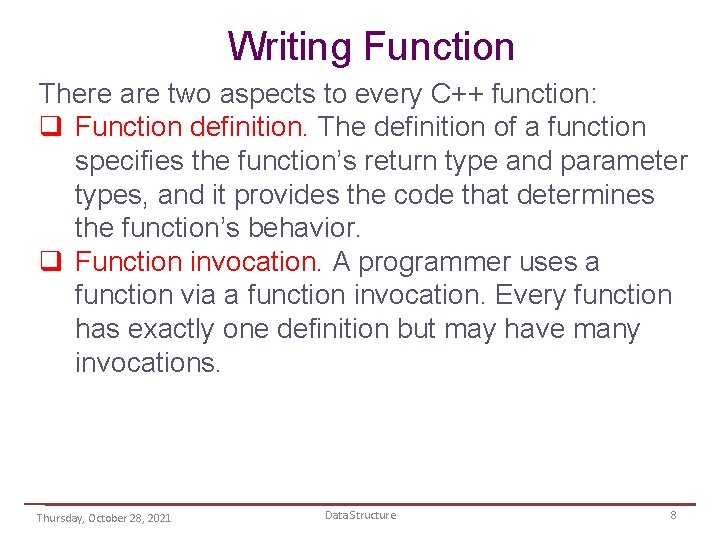
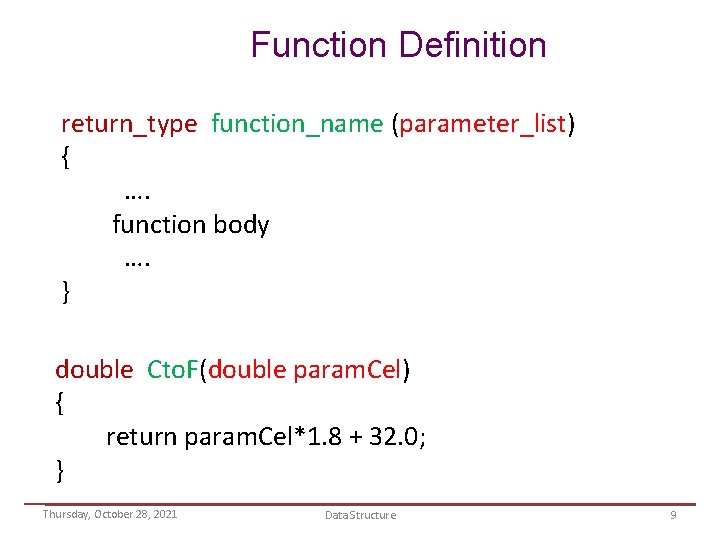
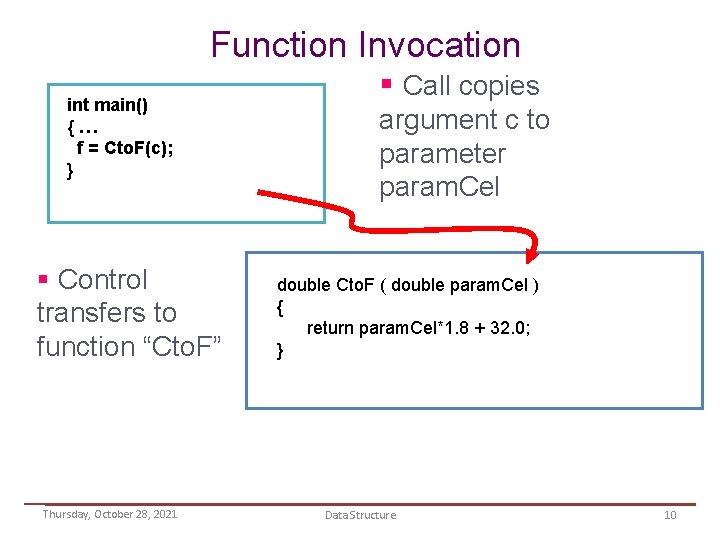
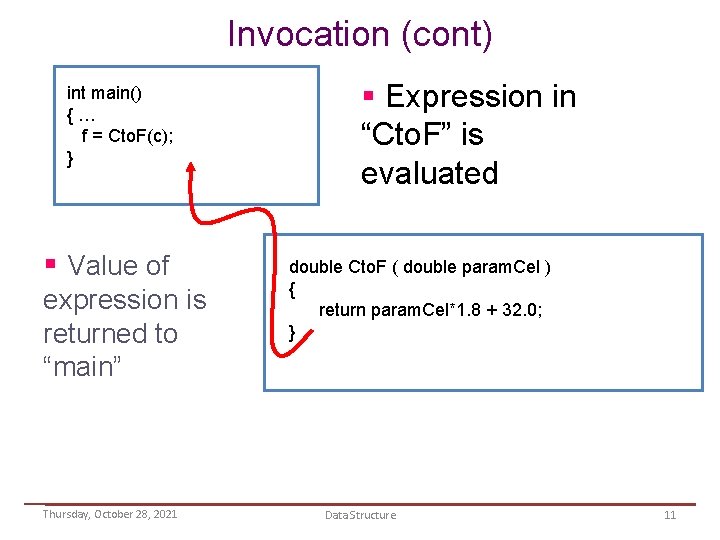
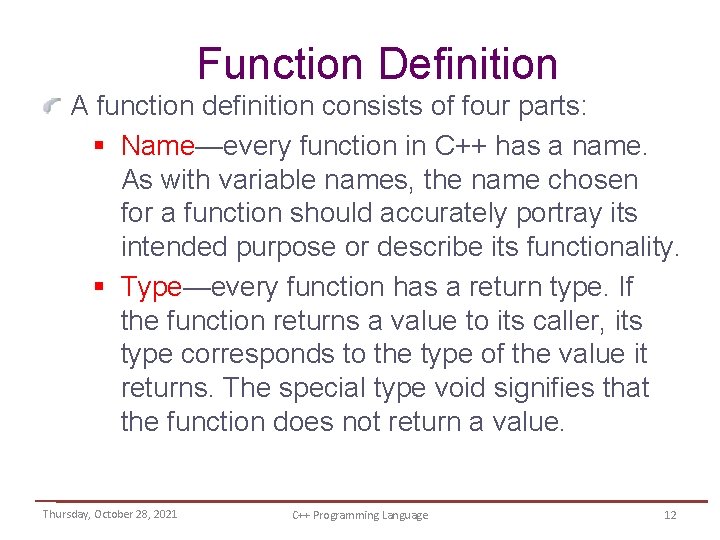
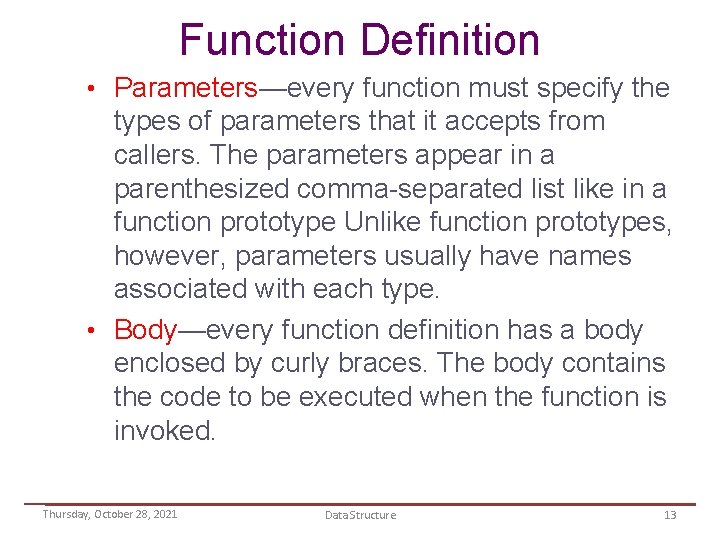
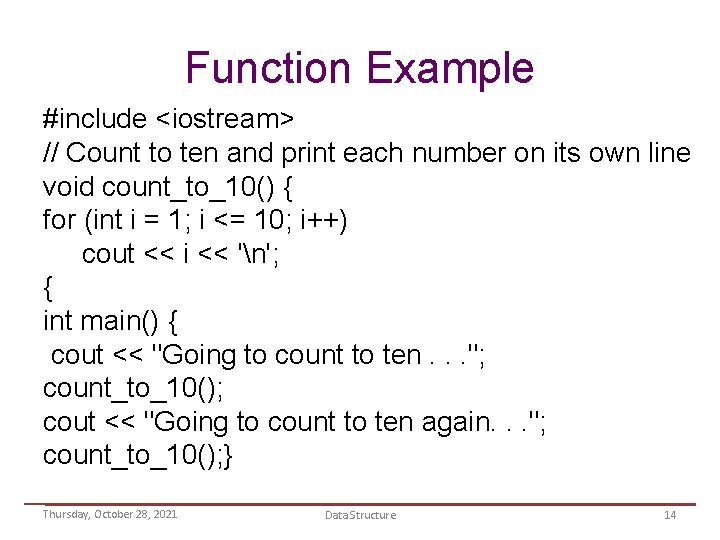
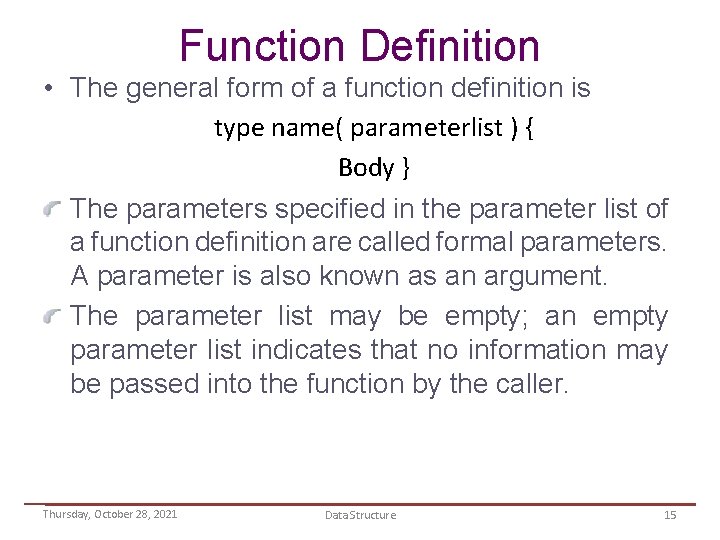
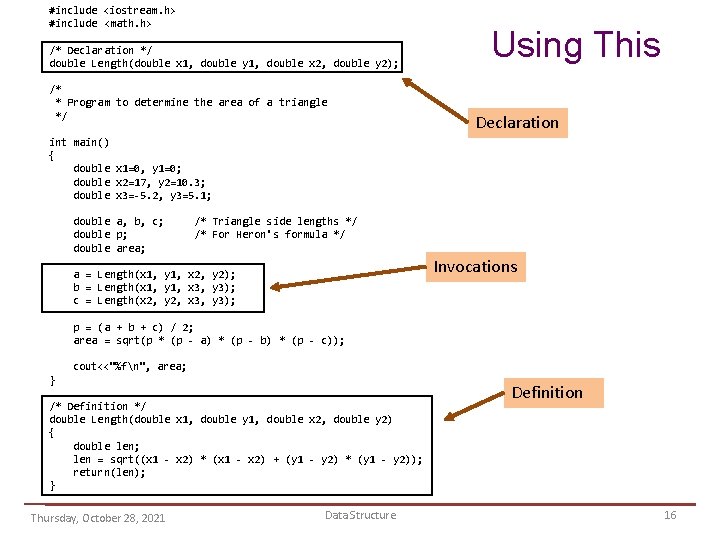
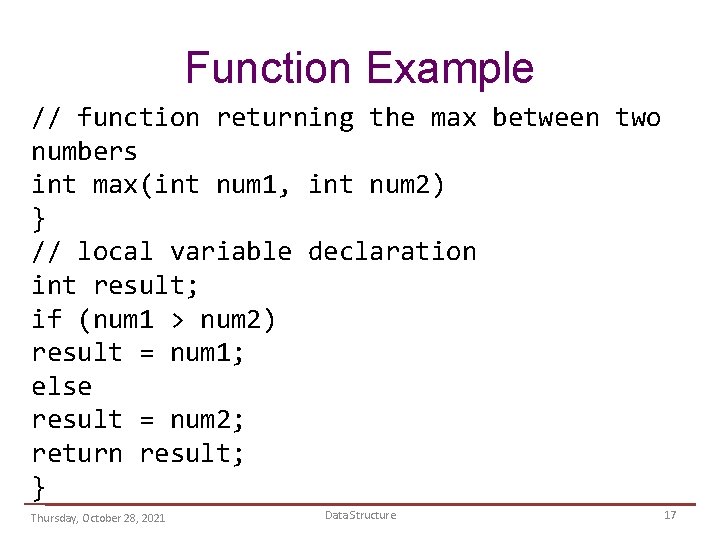
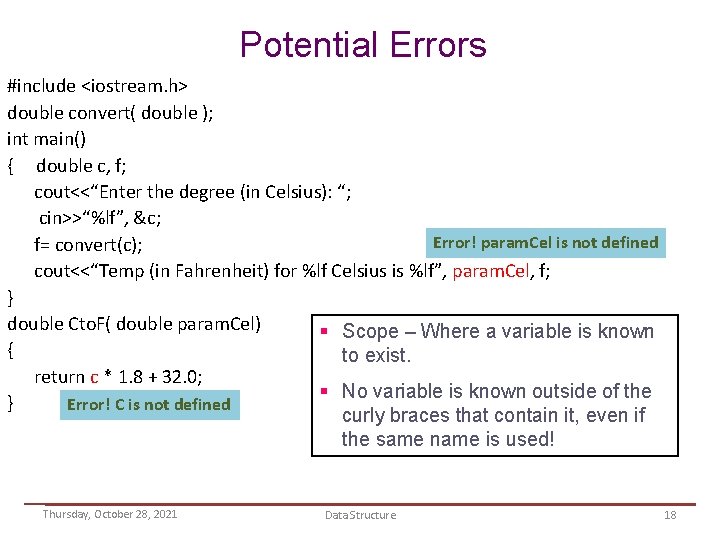
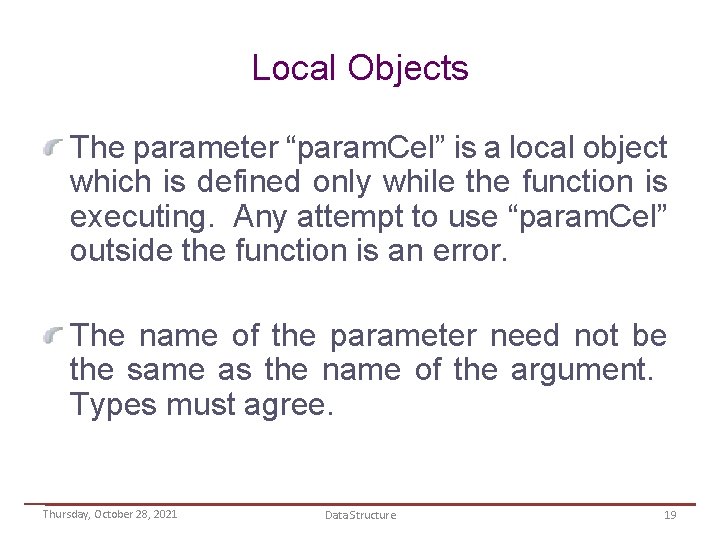
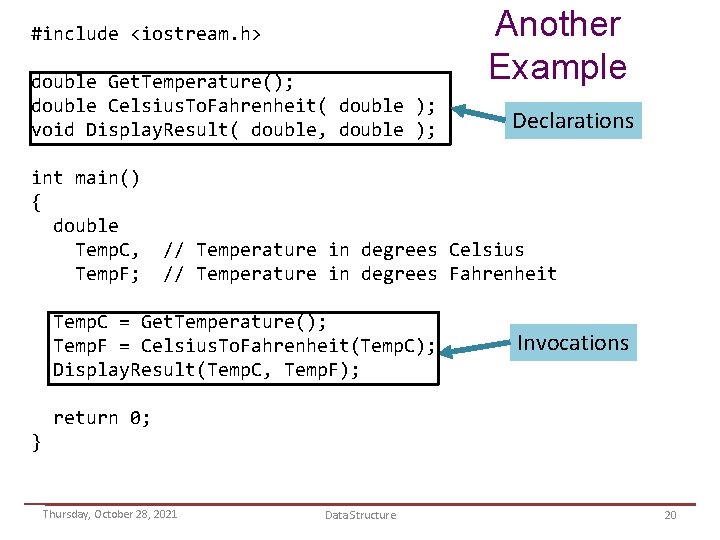
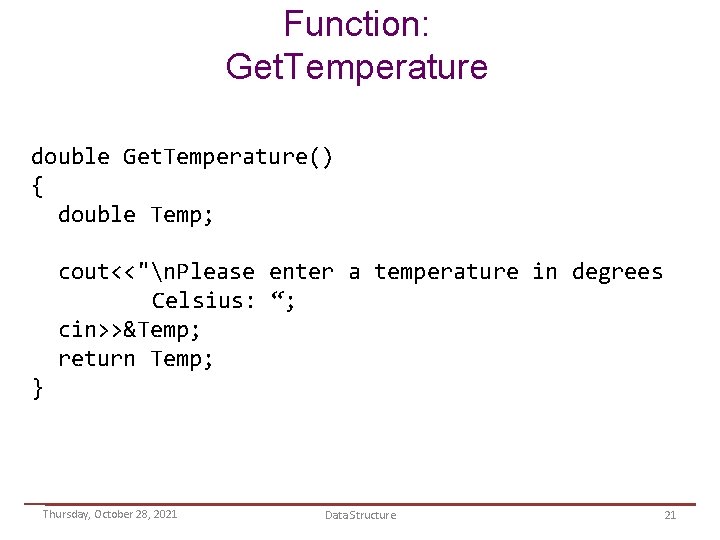
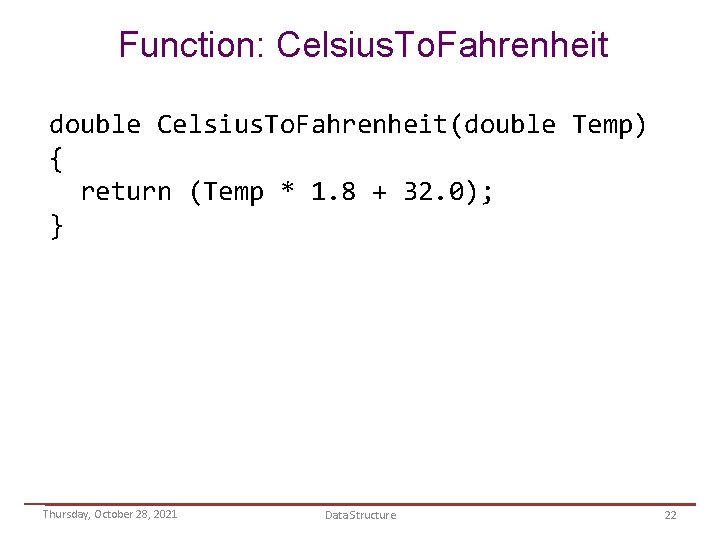
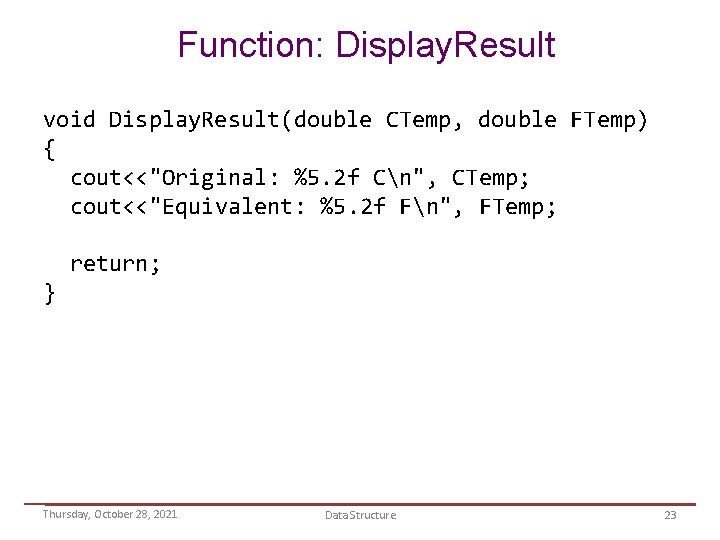
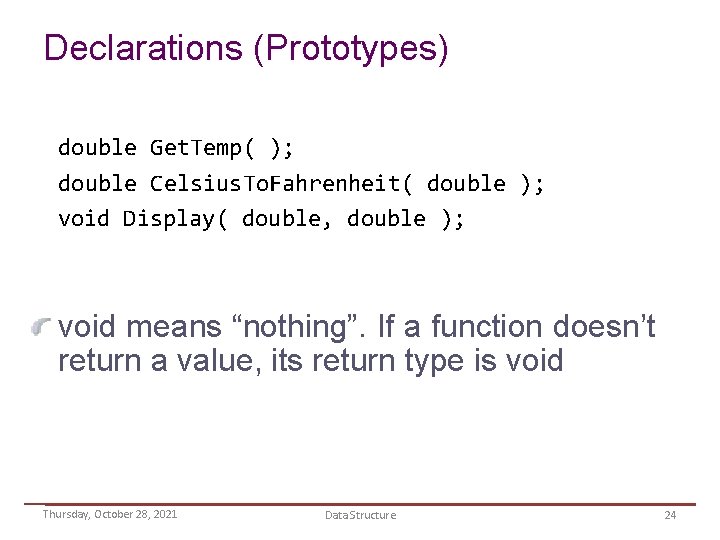
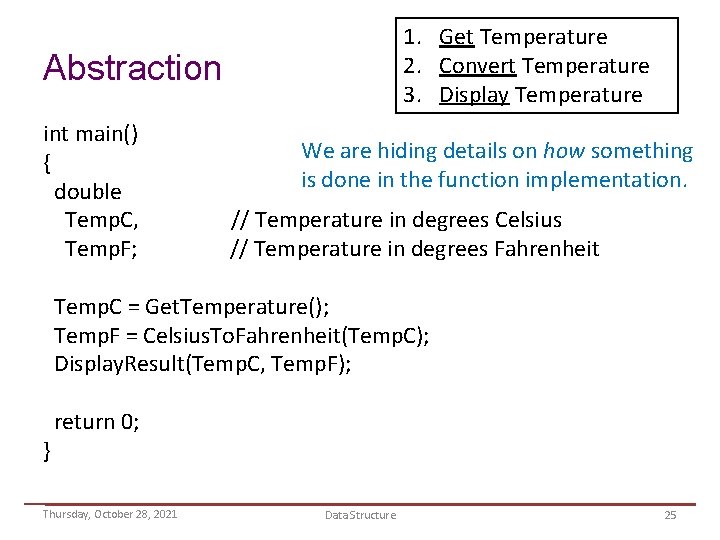
- Slides: 25
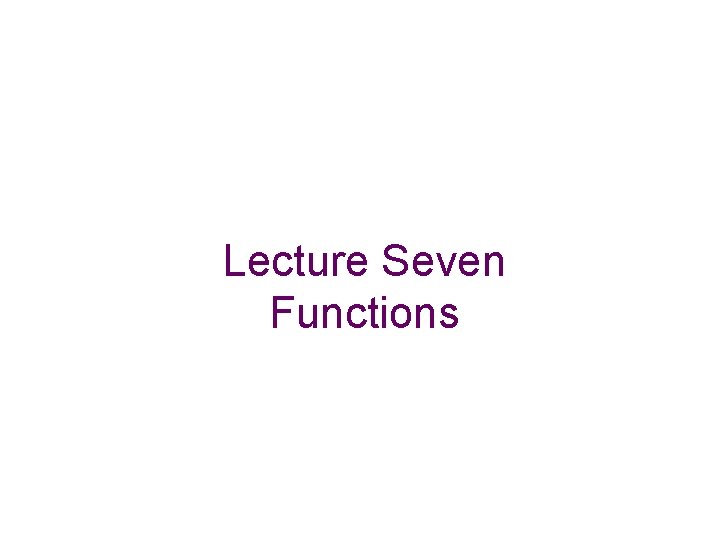
Lecture Seven Functions
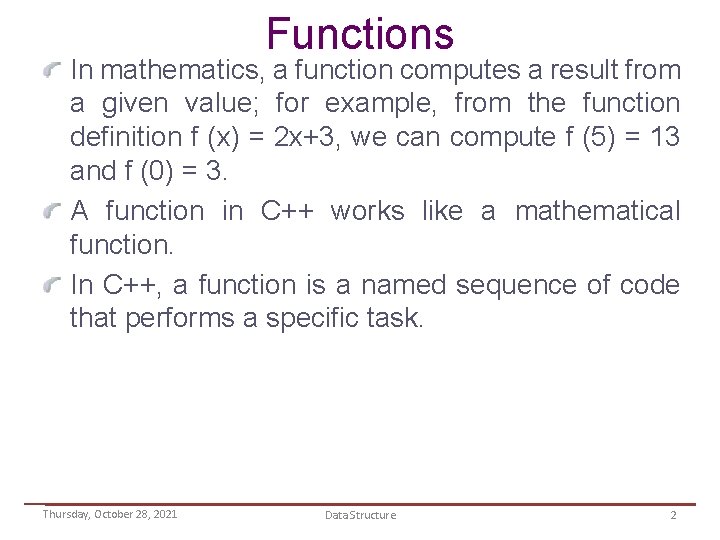
Functions In mathematics, a function computes a result from a given value; for example, from the function definition f (x) = 2 x+3, we can compute f (5) = 13 and f (0) = 3. A function in C++ works like a mathematical function. In C++, a function is a named sequence of code that performs a specific task. Thursday, October 28, 2021 Data Structure 2
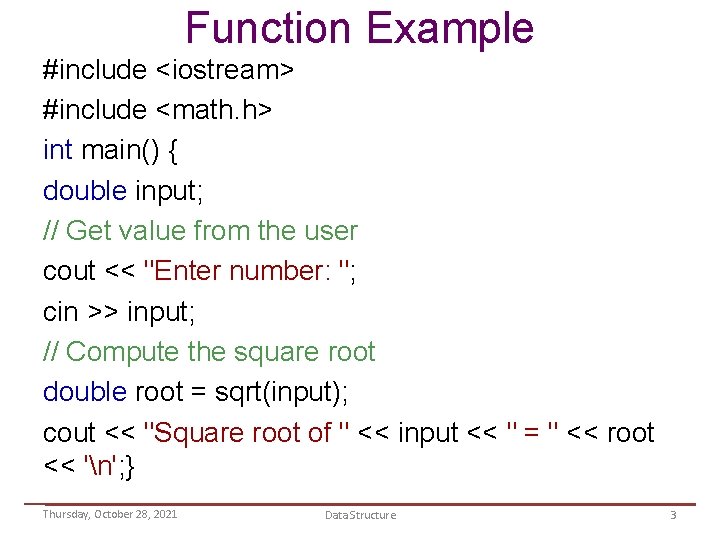
Function Example #include <iostream> #include <math. h> int main() { double input; // Get value from the user cout << "Enter number: "; cin >> input; // Compute the square root double root = sqrt(input); cout << "Square root of " << input << " = " << root << 'n'; } Thursday, October 28, 2021 Data Structure 3
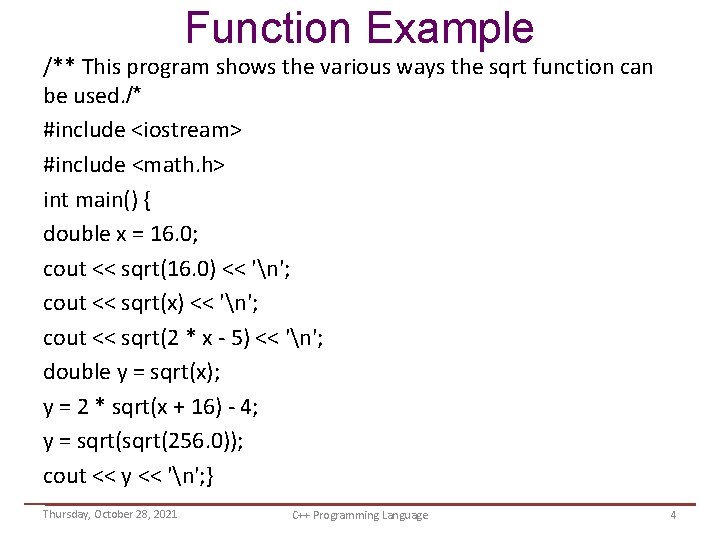
Function Example /** This program shows the various ways the sqrt function can be used. /* #include <iostream> #include <math. h> int main() { double x = 16. 0; cout << sqrt(16. 0) << 'n'; cout << sqrt(x) << 'n'; cout << sqrt(2 * x - 5) << 'n'; double y = sqrt(x); y = 2 * sqrt(x + 16) - 4; y = sqrt(256. 0)); cout << y << 'n'; } Thursday, October 28, 2021 C++ Programming Language 4
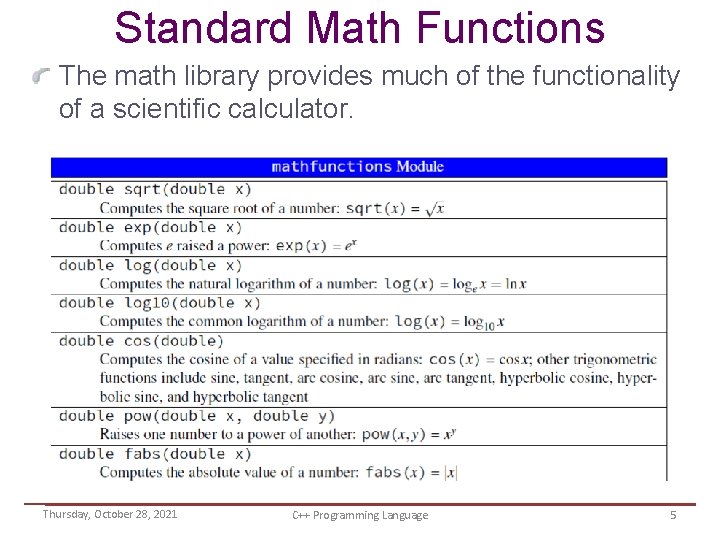
Standard Math Functions The math library provides much of the functionality of a scientific calculator. Thursday, October 28, 2021 C++ Programming Language 5
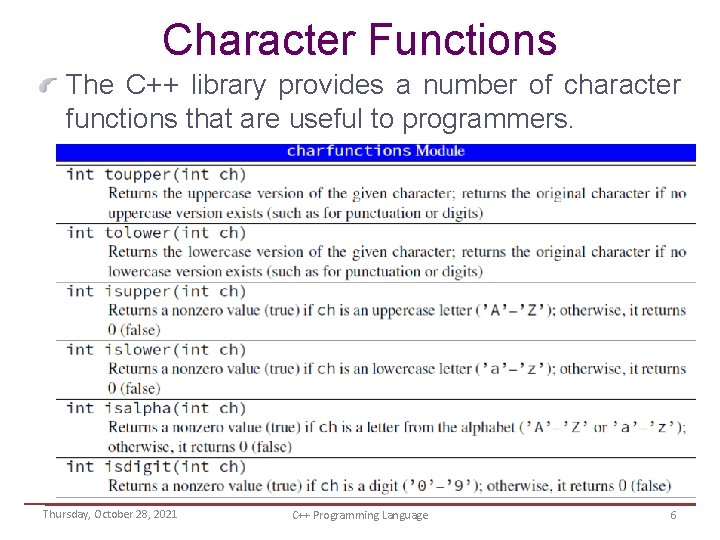
Character Functions The C++ library provides a number of character functions that are useful to programmers. Thursday, October 28, 2021 C++ Programming Language 6
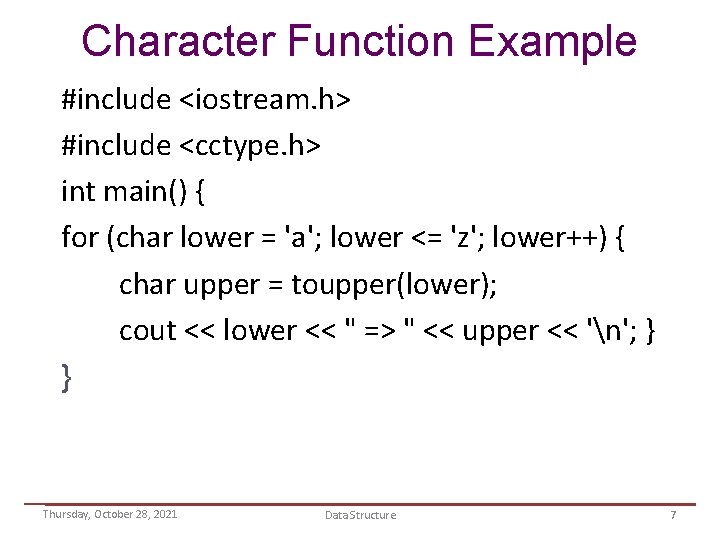
Character Function Example #include <iostream. h> #include <cctype. h> int main() { for (char lower = 'a'; lower <= 'z'; lower++) { char upper = toupper(lower); cout << lower << " => " << upper << 'n'; } } Thursday, October 28, 2021 Data Structure 7
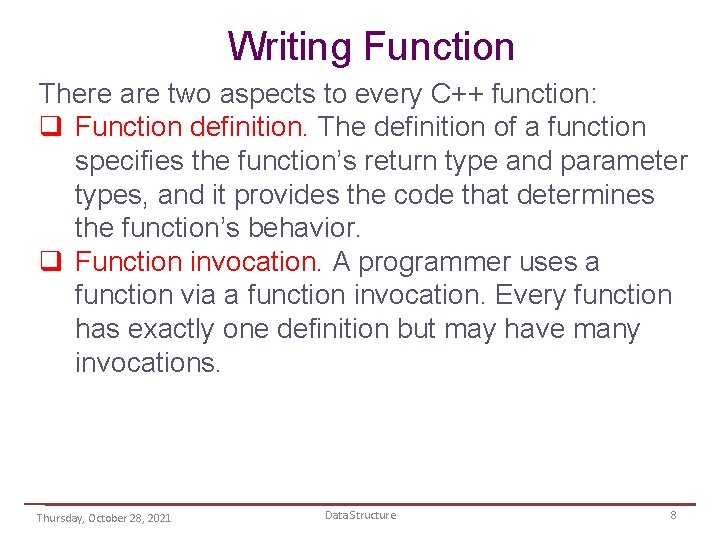
Writing Function There are two aspects to every C++ function: q Function definition. The definition of a function specifies the function’s return type and parameter types, and it provides the code that determines the function’s behavior. q Function invocation. A programmer uses a function via a function invocation. Every function has exactly one definition but may have many invocations. Thursday, October 28, 2021 Data Structure 8
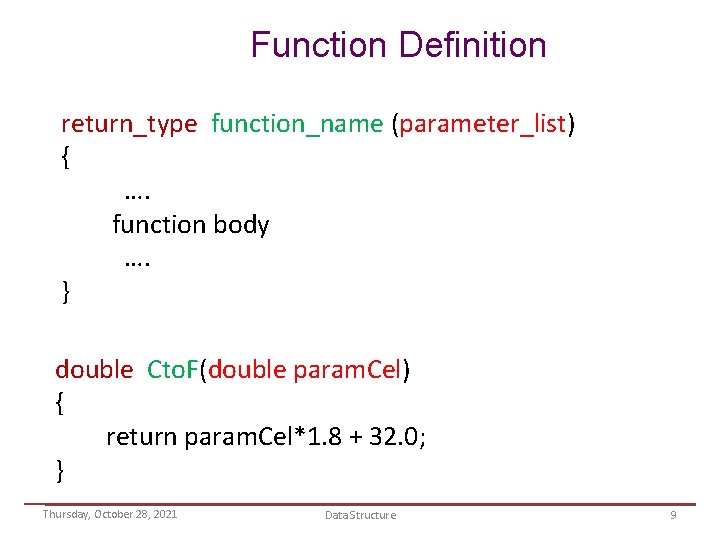
Function Definition return_type function_name (parameter_list) { …. function body …. } double Cto. F(double param. Cel) { return param. Cel*1. 8 + 32. 0; } Thursday, October 28, 2021 Data Structure 9
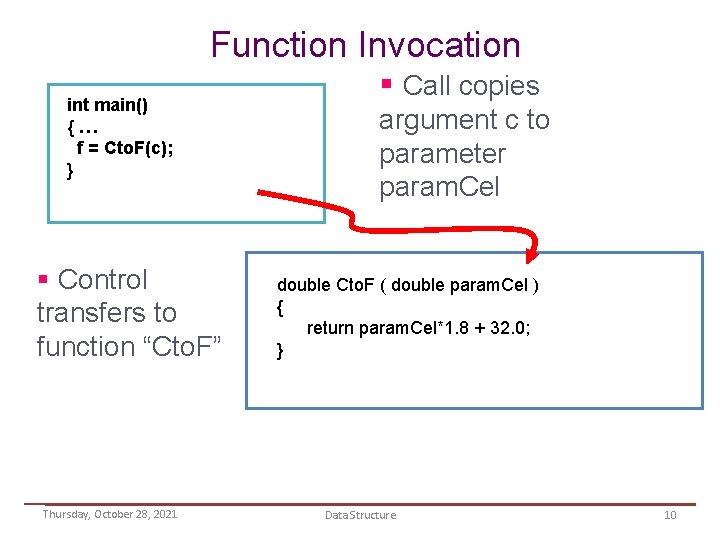
Function Invocation int main() {… f = Cto. F(c); } § Control transfers to function “Cto. F” Thursday, October 28, 2021 § Call copies argument c to parameter param. Cel double Cto. F ( double param. Cel ) { return param. Cel*1. 8 + 32. 0; } Data Structure 10
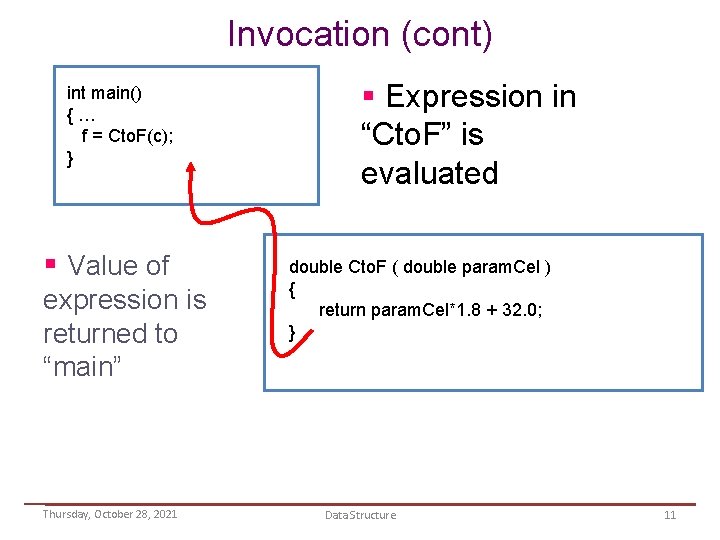
Invocation (cont) int main() {… f = Cto. F(c); } § Value of expression is returned to “main” Thursday, October 28, 2021 § Expression in “Cto. F” is evaluated double Cto. F ( double param. Cel ) { return param. Cel*1. 8 + 32. 0; } Data Structure 11
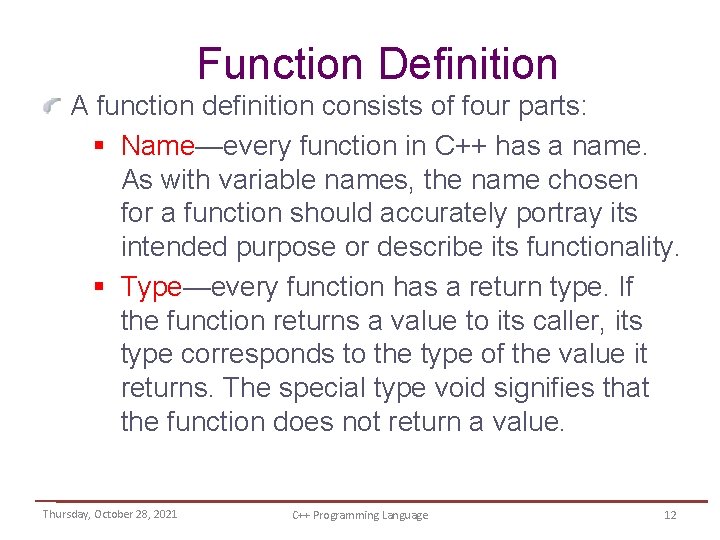
Function Definition A function definition consists of four parts: § Name—every function in C++ has a name. As with variable names, the name chosen for a function should accurately portray its intended purpose or describe its functionality. § Type—every function has a return type. If the function returns a value to its caller, its type corresponds to the type of the value it returns. The special type void signifies that the function does not return a value. Thursday, October 28, 2021 C++ Programming Language 12
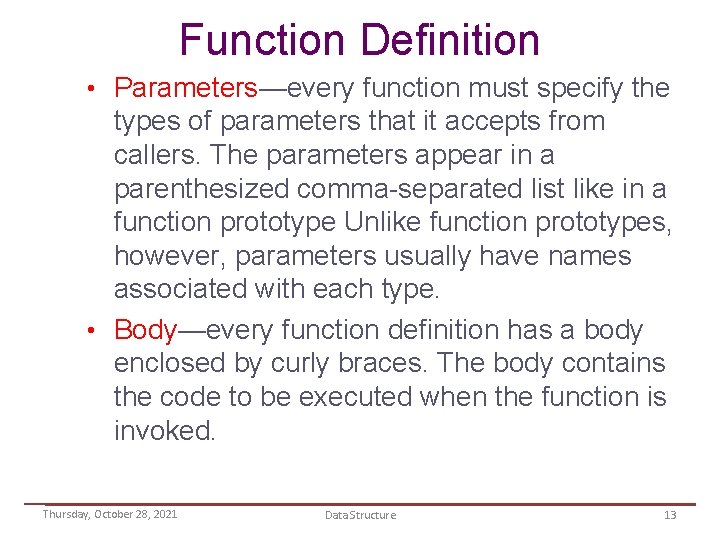
Function Definition • Parameters—every function must specify the types of parameters that it accepts from callers. The parameters appear in a parenthesized comma-separated list like in a function prototype Unlike function prototypes, however, parameters usually have names associated with each type. • Body—every function definition has a body enclosed by curly braces. The body contains the code to be executed when the function is invoked. Thursday, October 28, 2021 Data Structure 13
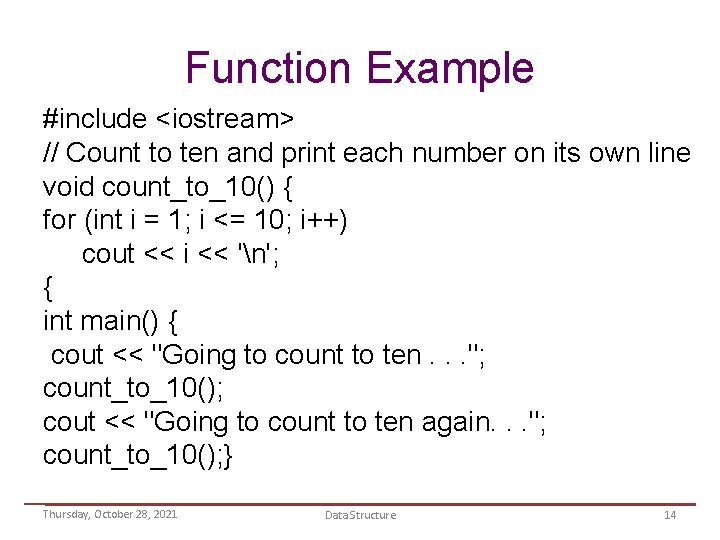
Function Example #include <iostream> // Count to ten and print each number on its own line void count_to_10() { for (int i = 1; i <= 10; i++) cout << i << 'n'; { int main() { cout << "Going to count to ten. . . "; count_to_10(); cout << "Going to count to ten again. . . "; count_to_10(); } Thursday, October 28, 2021 Data Structure 14
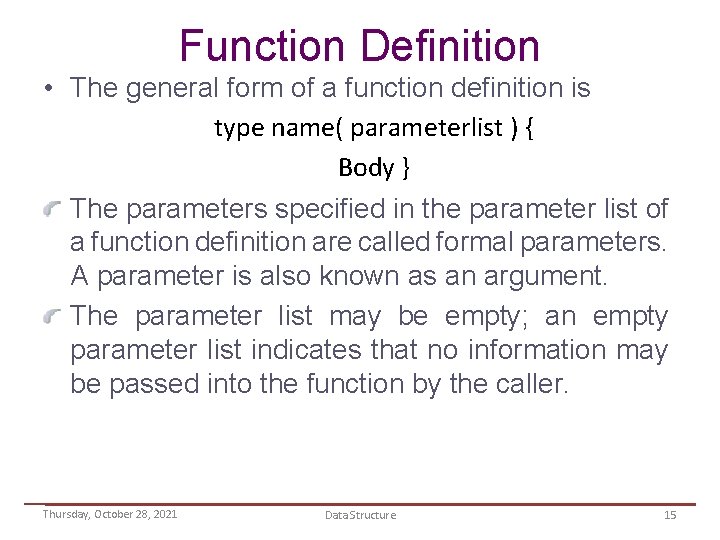
Function Definition • The general form of a function definition is type name( parameterlist ) { Body } The parameters specified in the parameter list of a function definition are called formal parameters. A parameter is also known as an argument. The parameter list may be empty; an empty parameter list indicates that no information may be passed into the function by the caller. Thursday, October 28, 2021 Data Structure 15
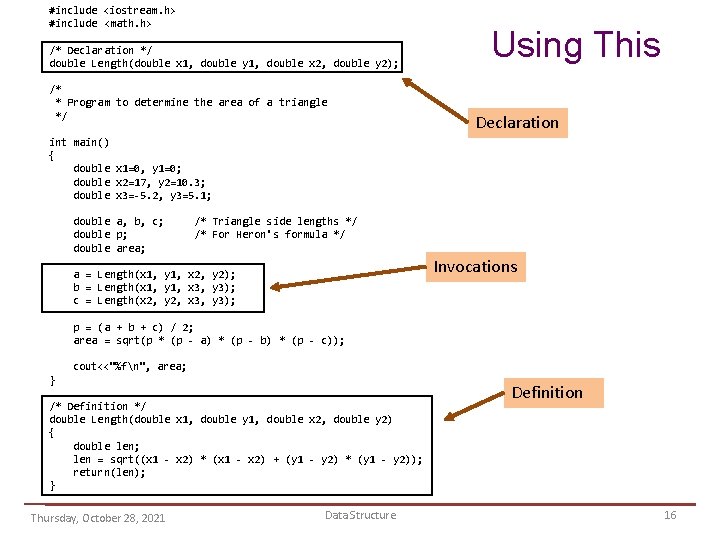
#include <iostream. h> #include <math. h> /* Declaration */ double Length(double x 1, double y 1, double x 2, double y 2); /* * Program to determine the area of a triangle */ Using This Declaration int main() { double x 1=0, y 1=0; double x 2=17, y 2=10. 3; double x 3=-5. 2, y 3=5. 1; double a, b, c; double p; double area; /* Triangle side lengths */ /* For Heron's formula */ Invocations a = Length(x 1, y 1, x 2, y 2); b = Length(x 1, y 1, x 3, y 3); c = Length(x 2, y 2, x 3, y 3); p = (a + b + c) / 2; area = sqrt(p * (p - a) * (p - b) * (p - c)); cout<<"%fn", area; } /* Definition */ double Length(double x 1, double y 1, double x 2, double y 2) { double len; len = sqrt((x 1 - x 2) * (x 1 - x 2) + (y 1 - y 2) * (y 1 - y 2)); return(len); } Thursday, October 28, 2021 Data Structure Definition 16
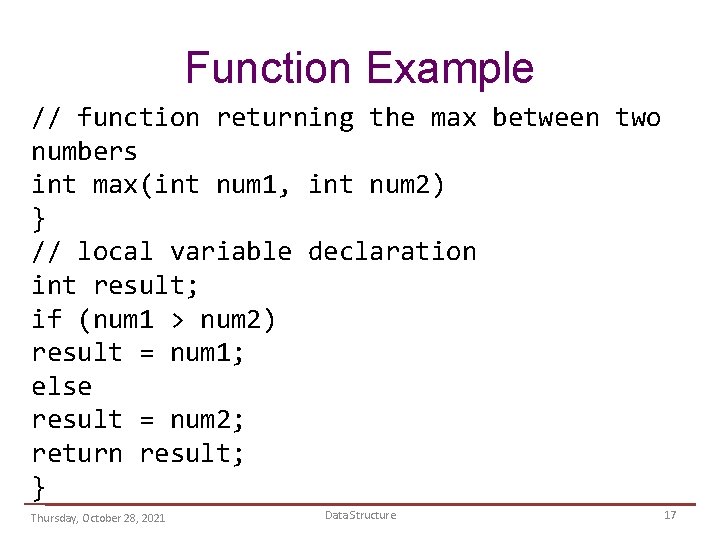
Function Example // function returning the max between two numbers int max(int num 1, int num 2) } // local variable declaration int result; if (num 1 > num 2) result = num 1; else result = num 2; return result; } Thursday, October 28, 2021 Data Structure 17
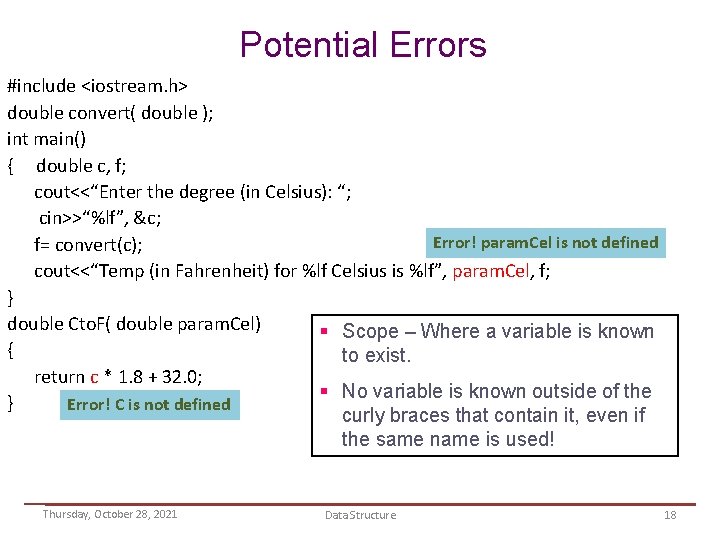
Potential Errors #include <iostream. h> double convert( double ); int main() { double c, f; cout<<“Enter the degree (in Celsius): “; cin>>“%lf”, &c; Error! param. Cel is not defined f= convert(c); cout<<“Temp (in Fahrenheit) for %lf Celsius is %lf”, param. Cel, f; } double Cto. F( double param. Cel) § Scope – Where a variable is known { to exist. return c * 1. 8 + 32. 0; § No variable is known outside of the } Error! C is not defined curly braces that contain it, even if the same name is used! Thursday, October 28, 2021 Data Structure 18
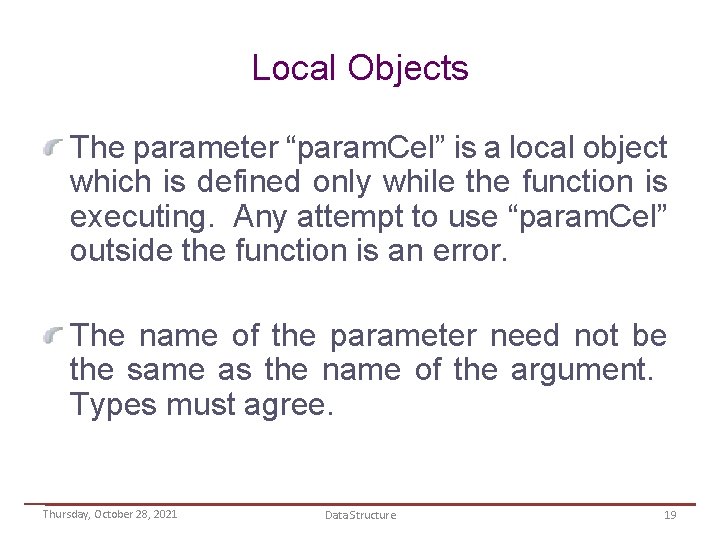
Local Objects The parameter “param. Cel” is a local object which is defined only while the function is executing. Any attempt to use “param. Cel” outside the function is an error. The name of the parameter need not be the same as the name of the argument. Types must agree. Thursday, October 28, 2021 Data Structure 19
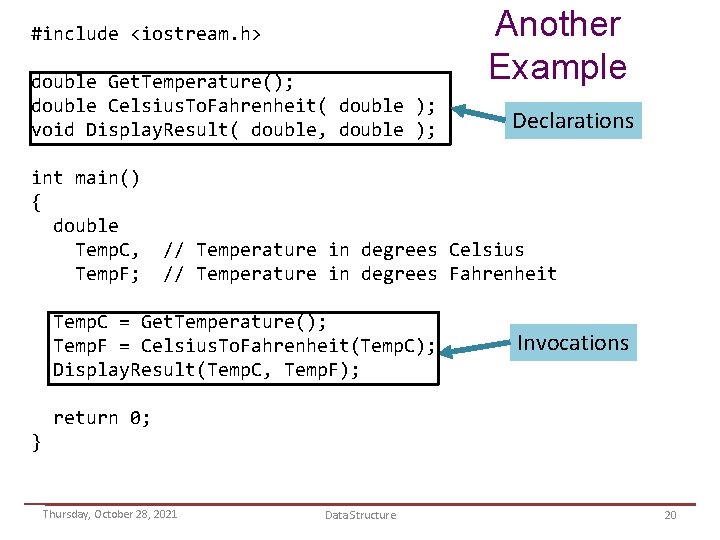
#include <iostream. h> double Get. Temperature(); double Celsius. To. Fahrenheit( double ); void Display. Result( double, double ); int main() { double Temp. C, Temp. F; Another Example Declarations // Temperature in degrees Celsius // Temperature in degrees Fahrenheit Temp. C = Get. Temperature(); Temp. F = Celsius. To. Fahrenheit(Temp. C); Display. Result(Temp. C, Temp. F); Invocations return 0; } Thursday, October 28, 2021 Data Structure 20
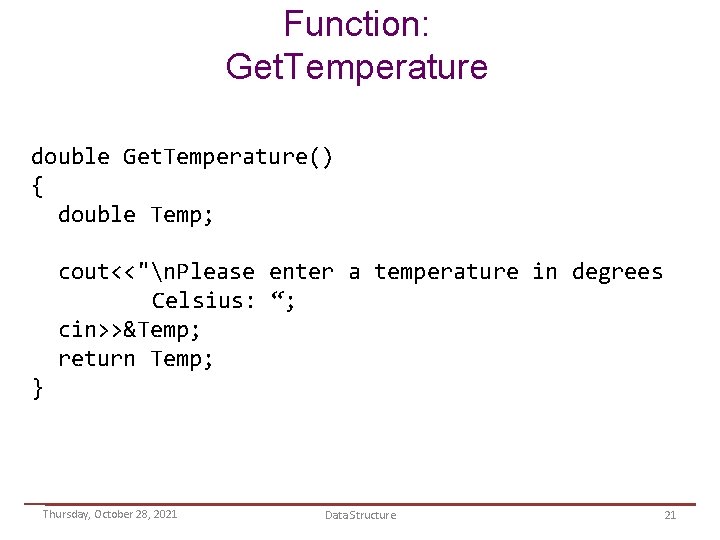
Function: Get. Temperature double Get. Temperature() { double Temp; cout<<"n. Please enter a temperature in degrees Celsius: “; cin>>&Temp; return Temp; } Thursday, October 28, 2021 Data Structure 21
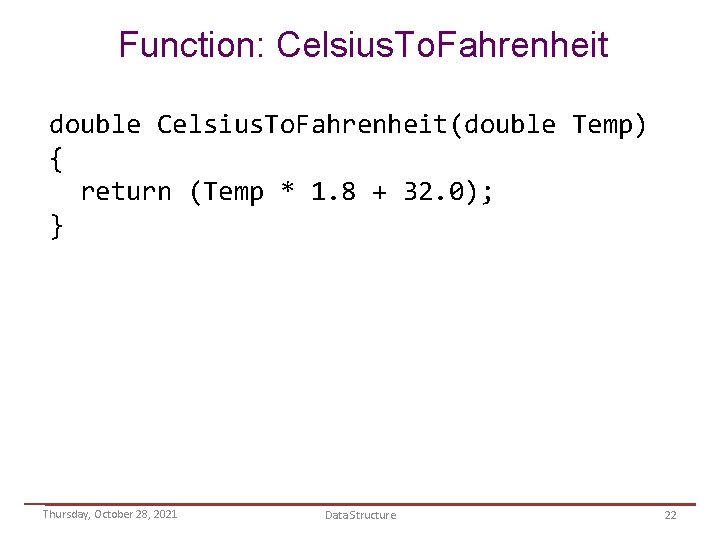
Function: Celsius. To. Fahrenheit double Celsius. To. Fahrenheit(double Temp) { return (Temp * 1. 8 + 32. 0); } Thursday, October 28, 2021 Data Structure 22
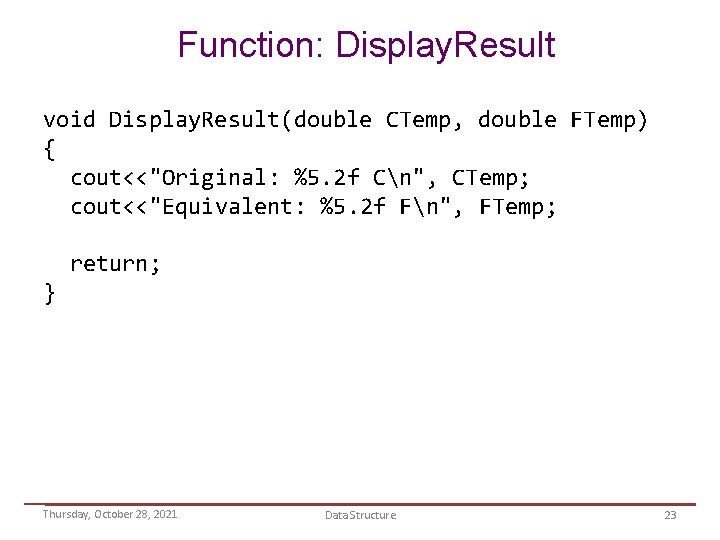
Function: Display. Result void Display. Result(double CTemp, double FTemp) { cout<<"Original: %5. 2 f Cn", CTemp; cout<<"Equivalent: %5. 2 f Fn", FTemp; return; } Thursday, October 28, 2021 Data Structure 23
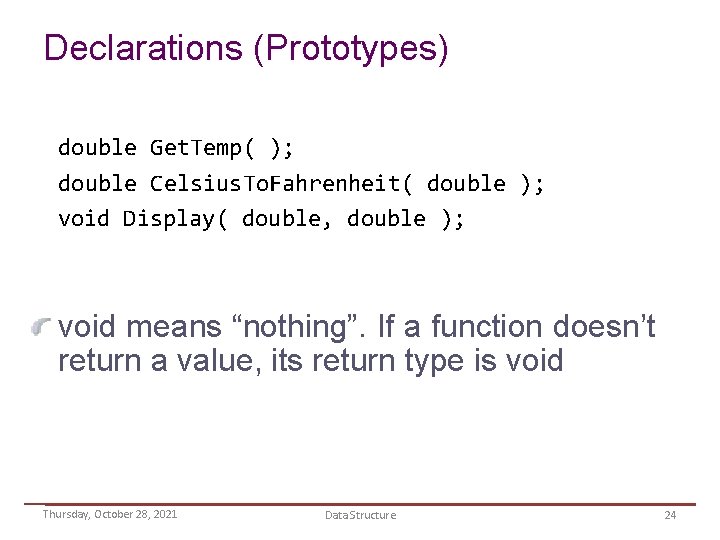
Declarations (Prototypes) double Get. Temp( ); double Celsius. To. Fahrenheit( double ); void Display( double, double ); void means “nothing”. If a function doesn’t return a value, its return type is void Thursday, October 28, 2021 Data Structure 24
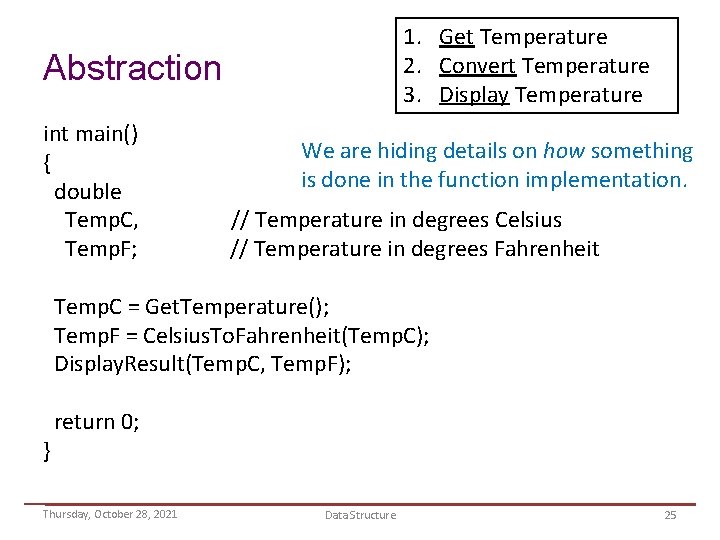
1. Get Temperature 2. Convert Temperature 3. Display Temperature Abstraction int main() { double Temp. C, Temp. F; We are hiding details on how something is done in the function implementation. // Temperature in degrees Celsius // Temperature in degrees Fahrenheit Temp. C = Get. Temperature(); Temp. F = Celsius. To. Fahrenheit(Temp. C); Display. Result(Temp. C, Temp. F); } return 0; Thursday, October 28, 2021 Data Structure 25