Lecture Serverside Programming CGI Common Gateway Interface 1
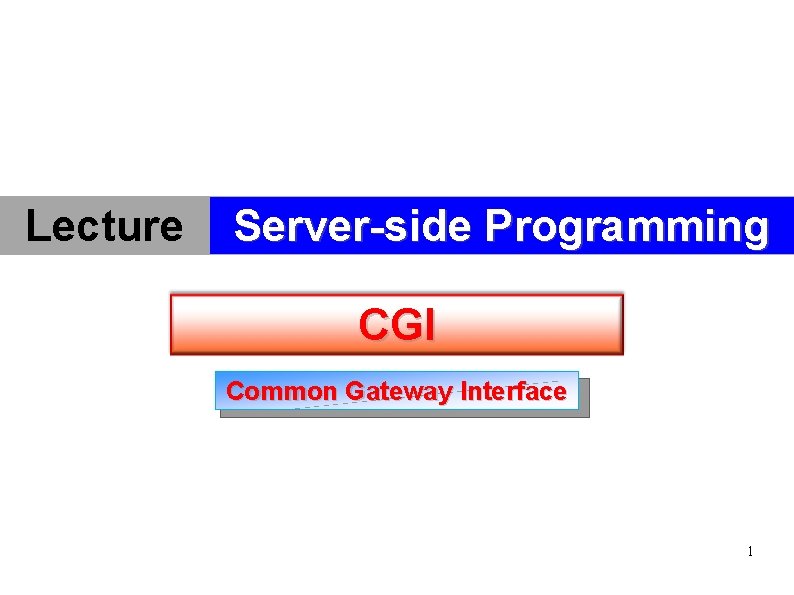
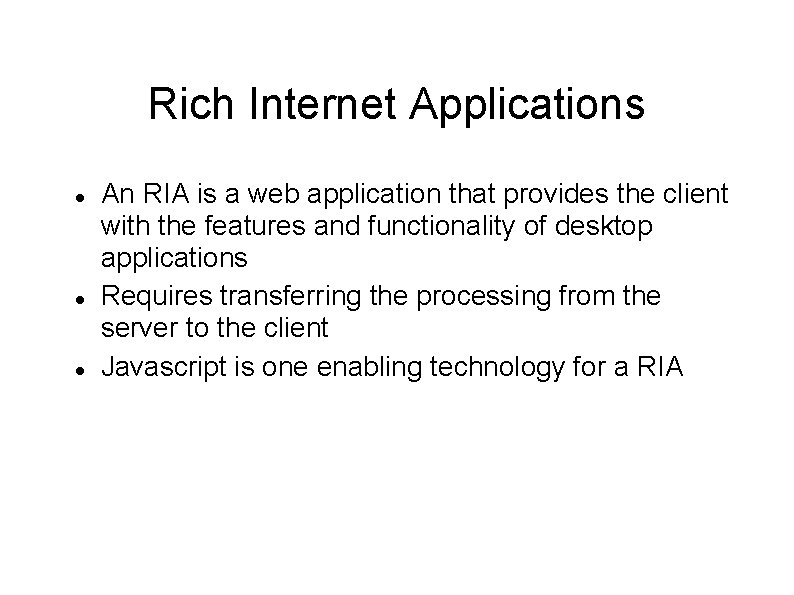
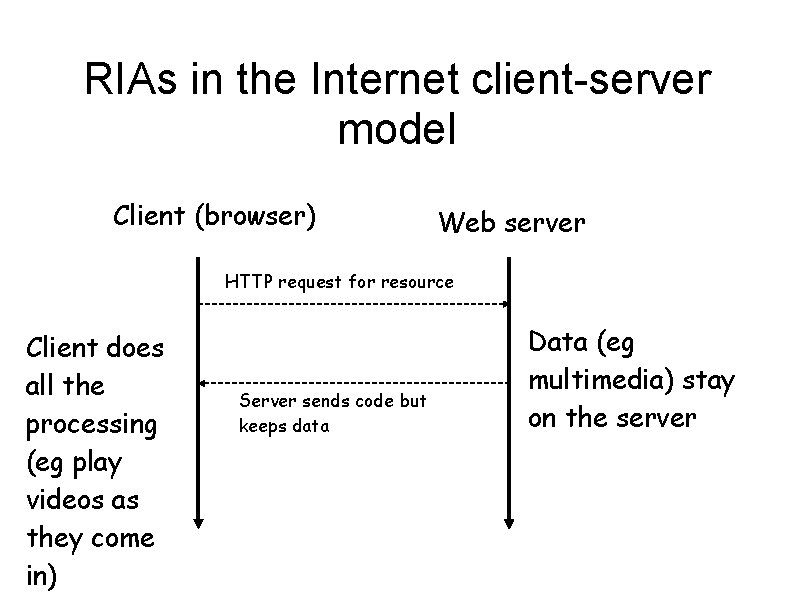
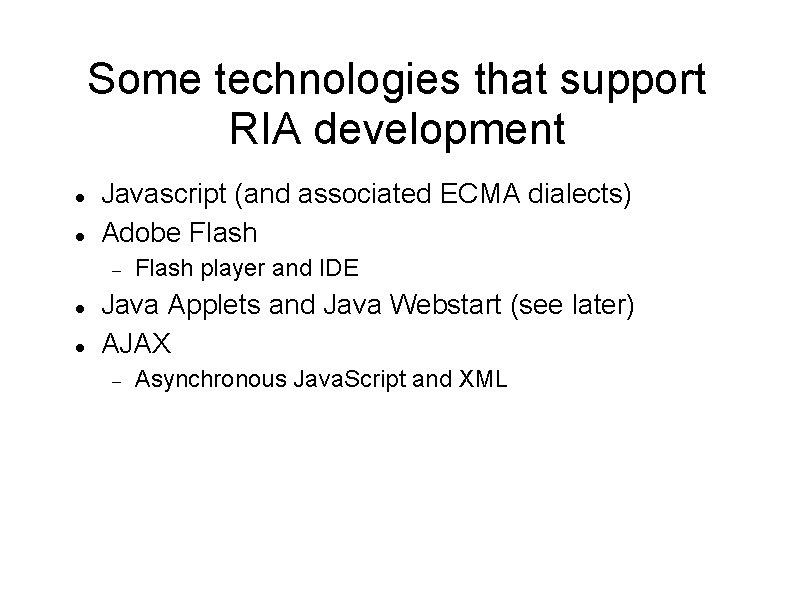
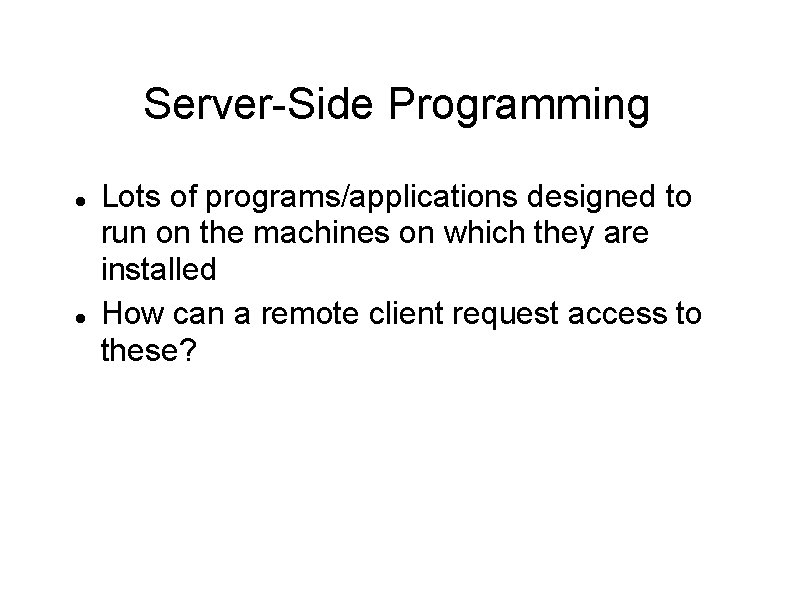
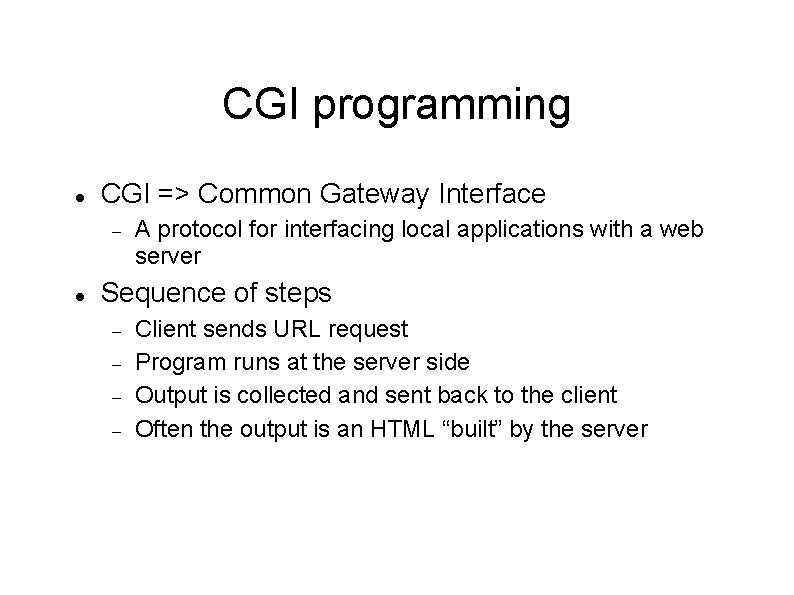
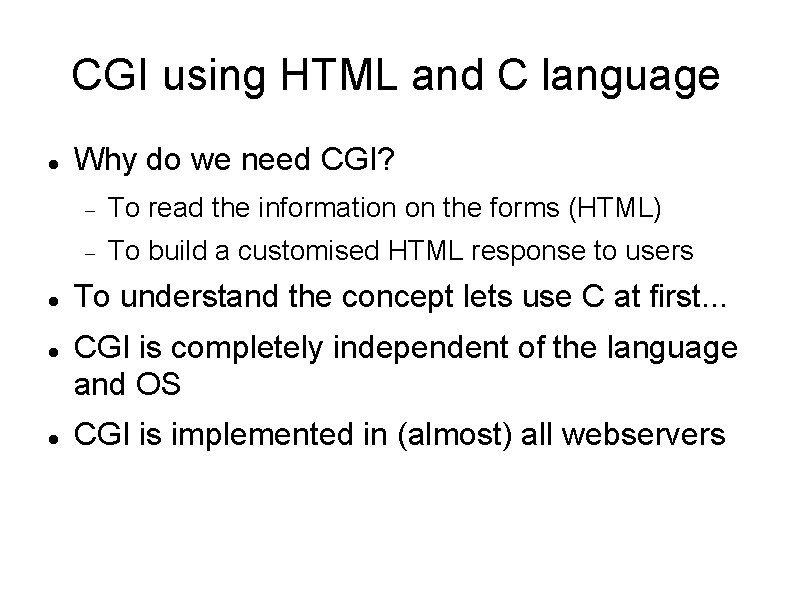
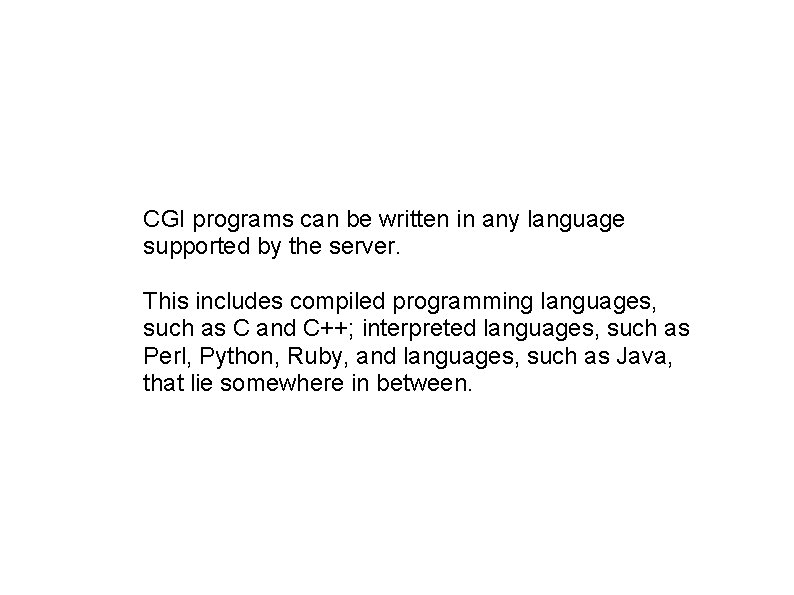
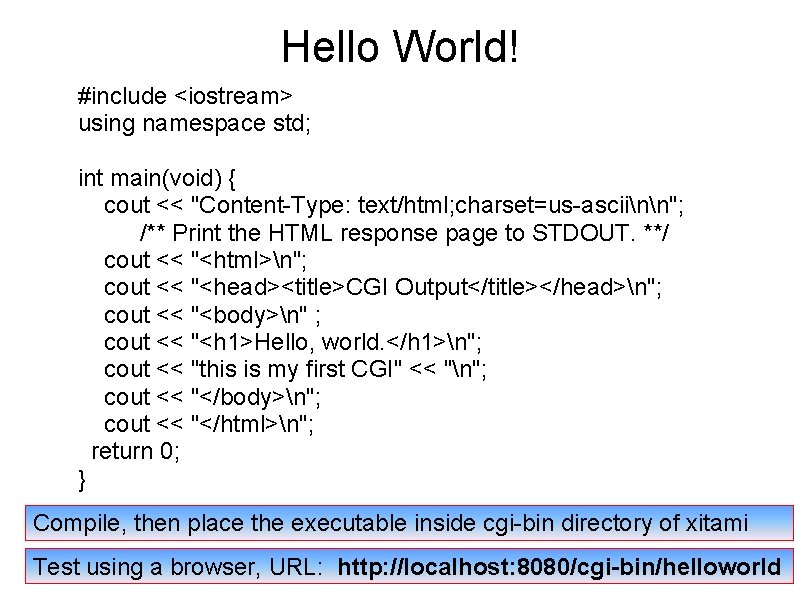
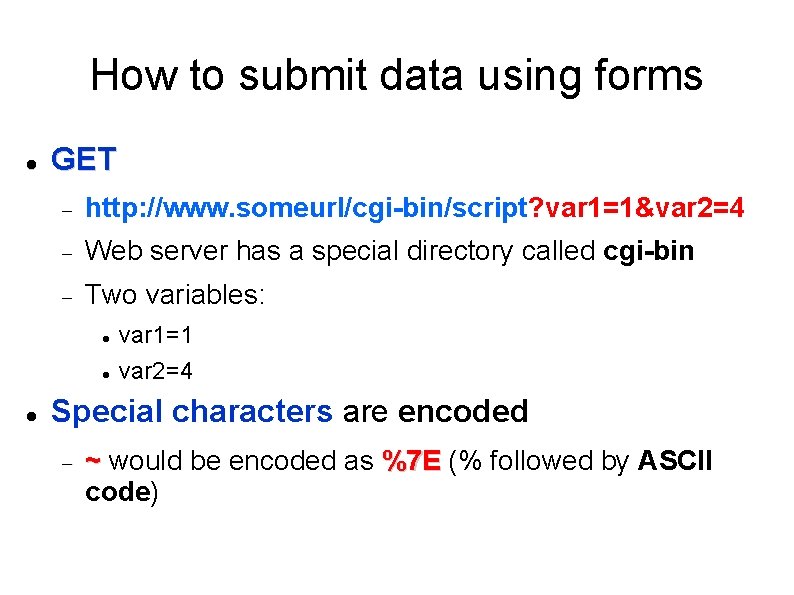
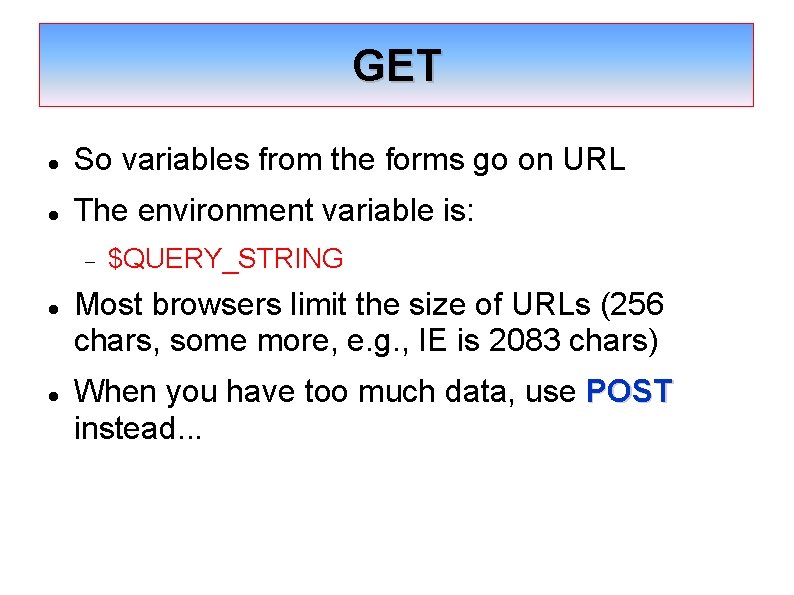
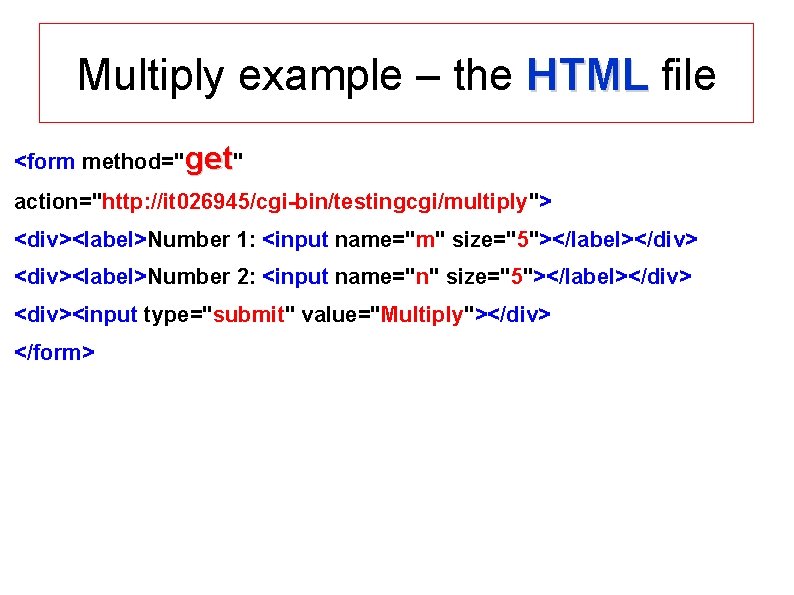
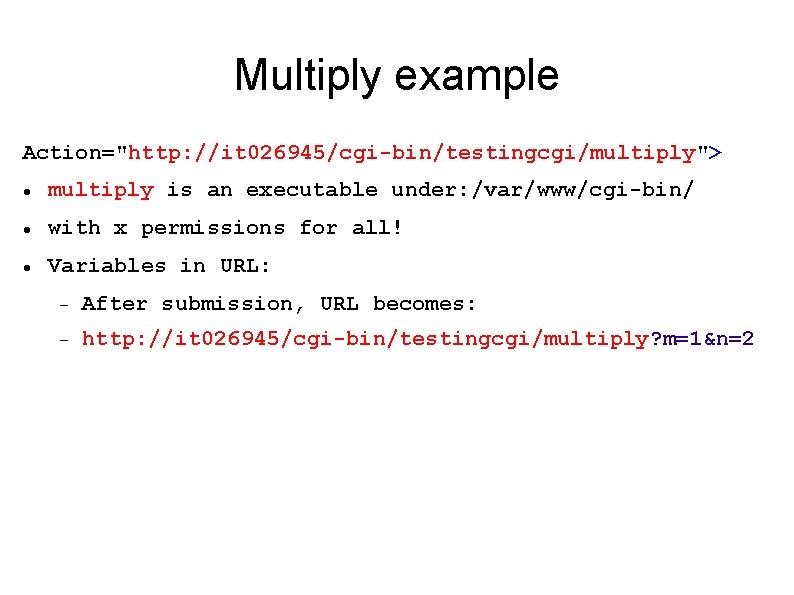
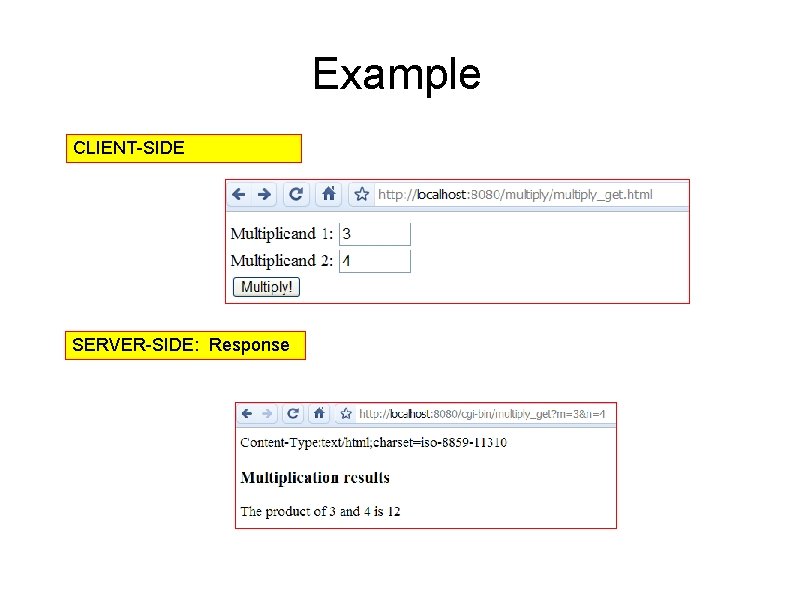
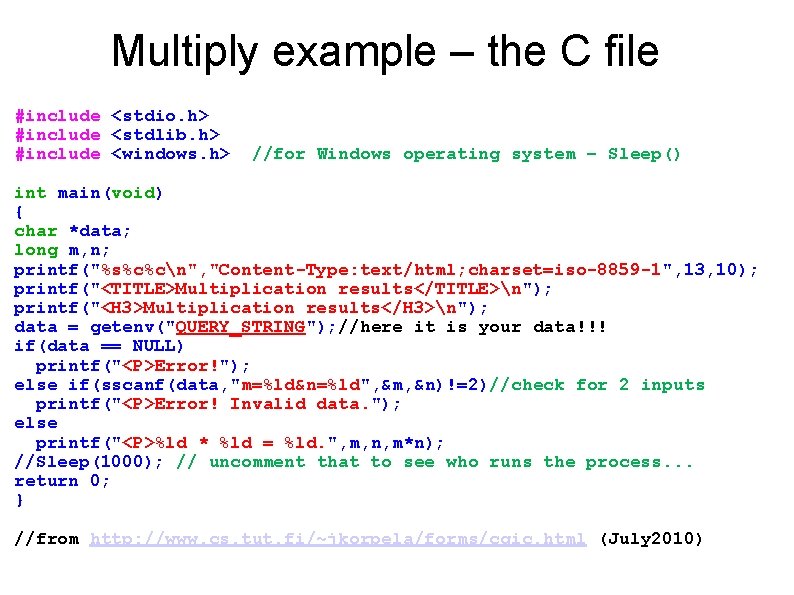
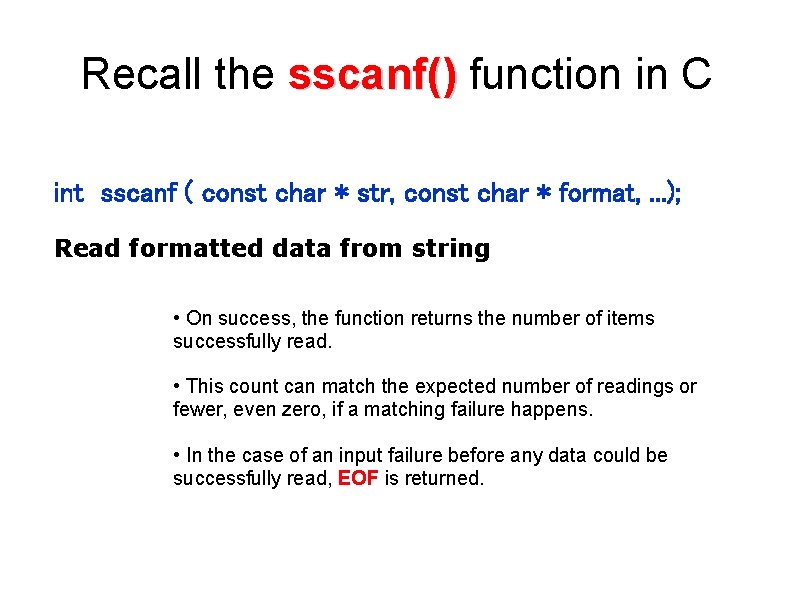
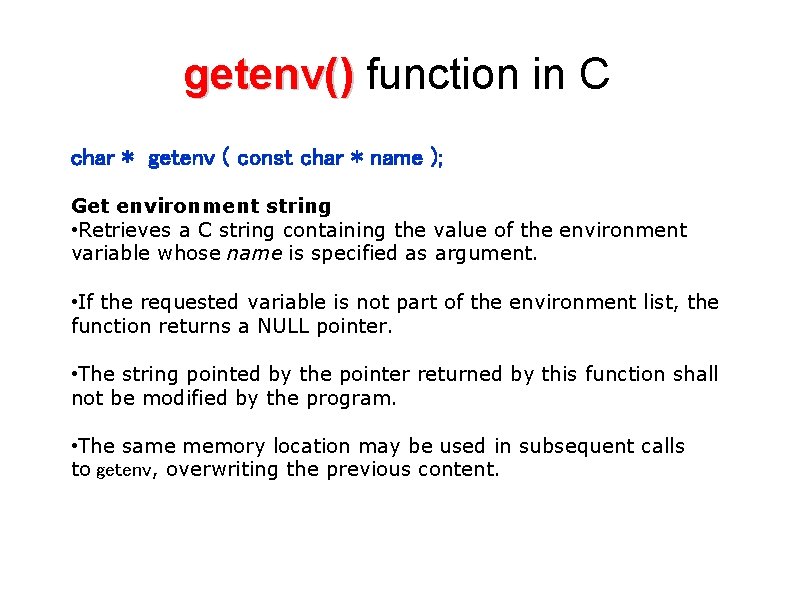
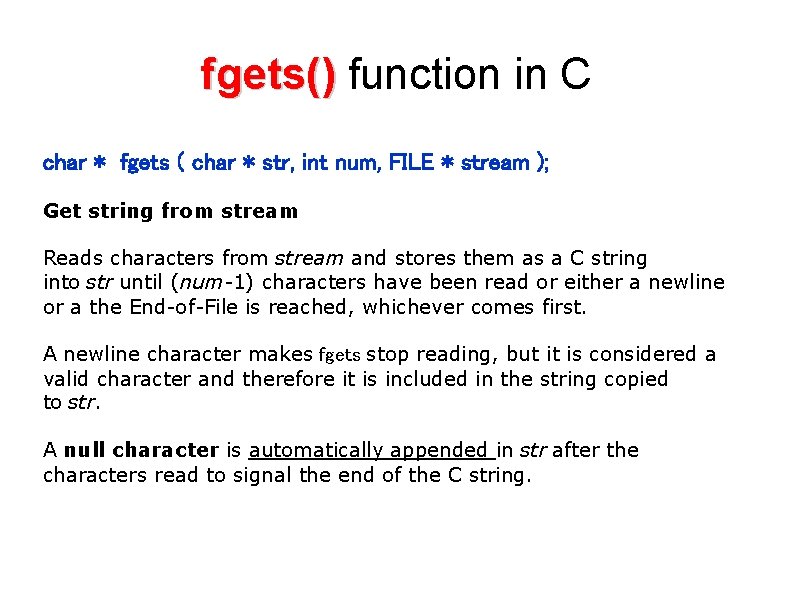
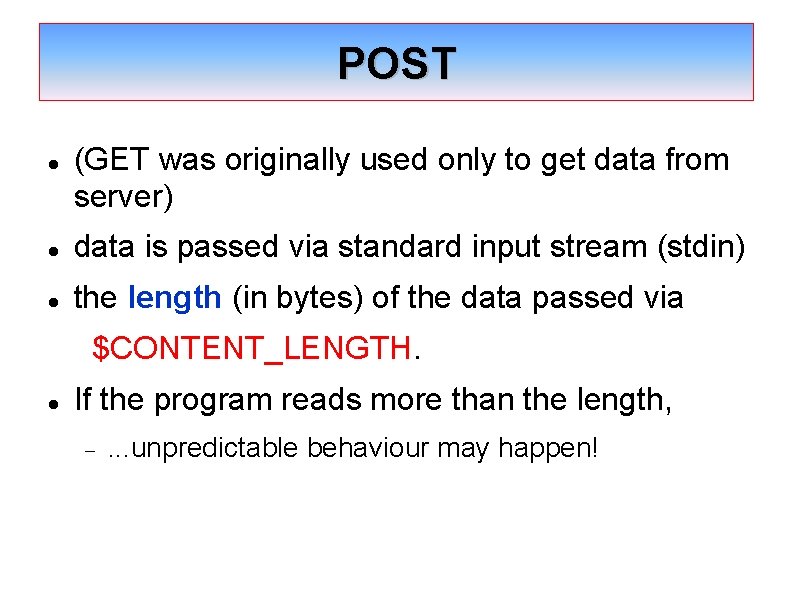
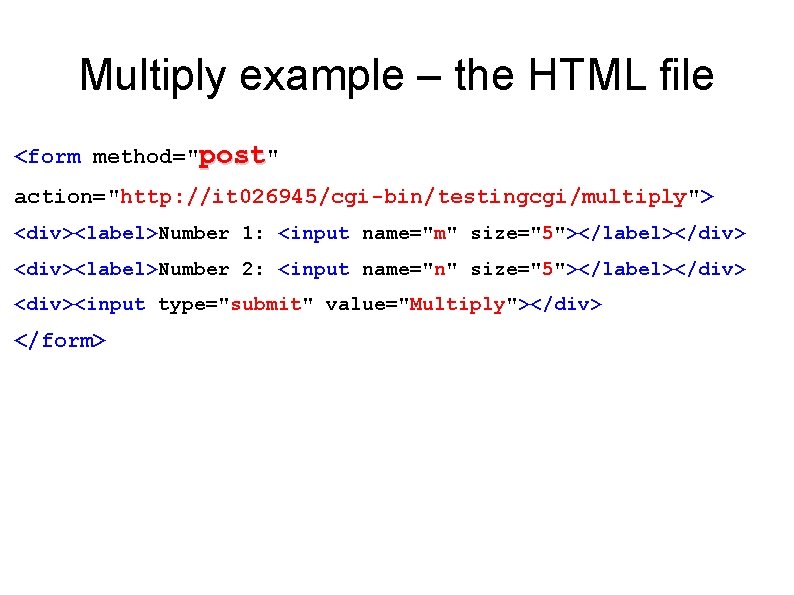
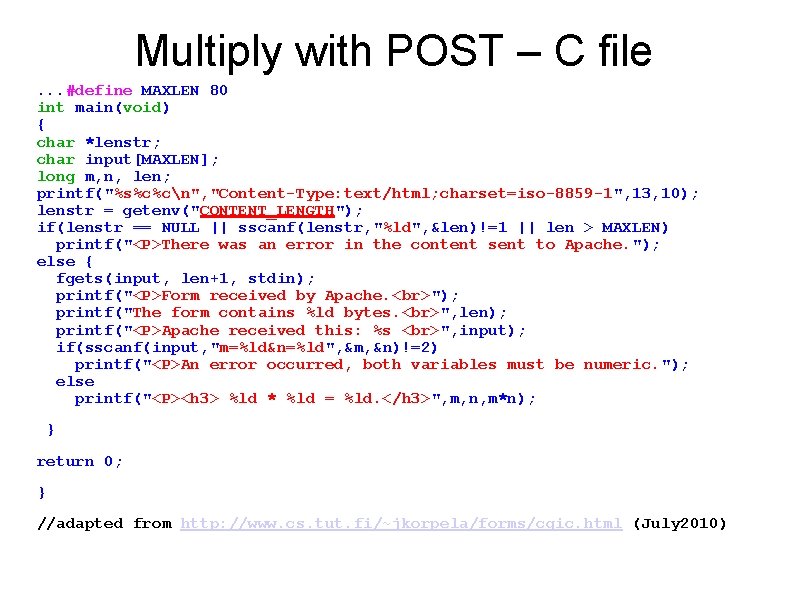
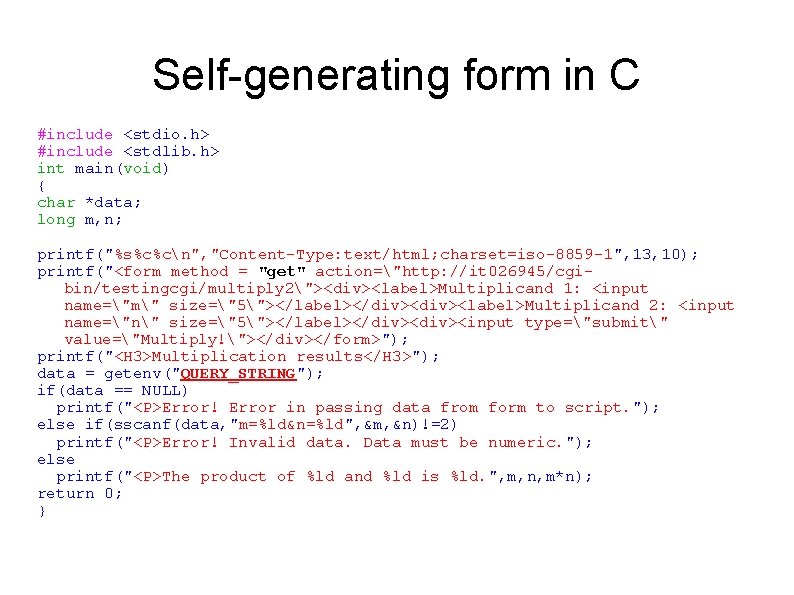
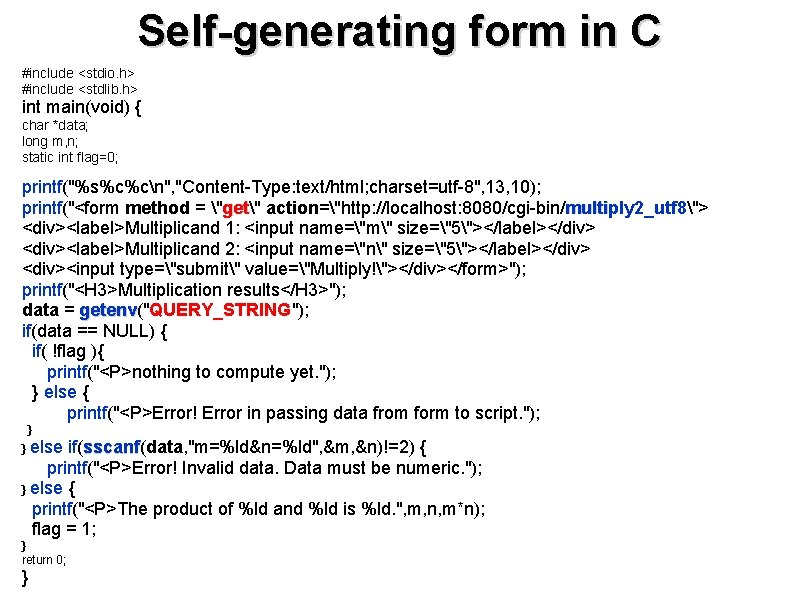
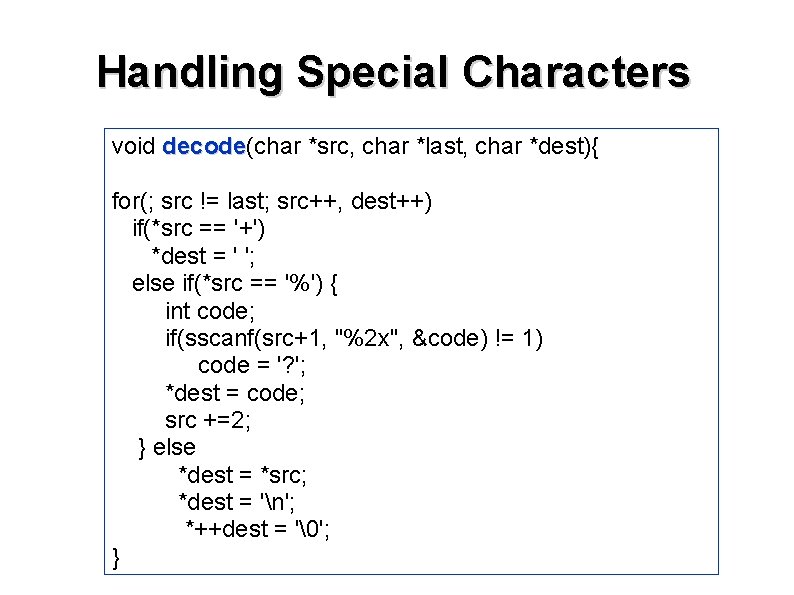
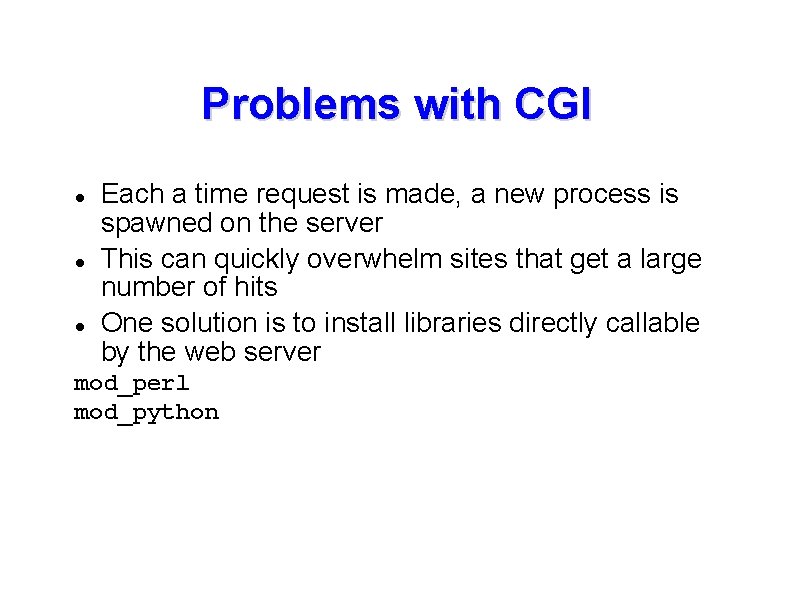
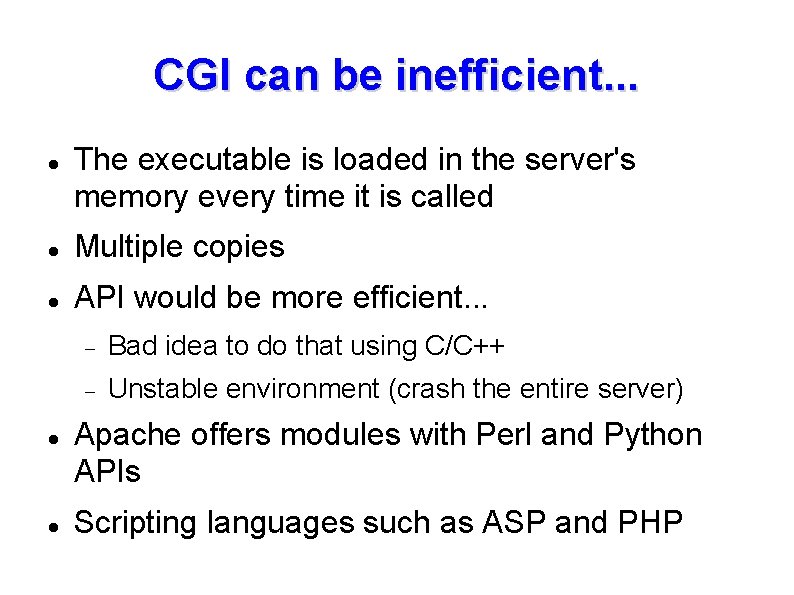
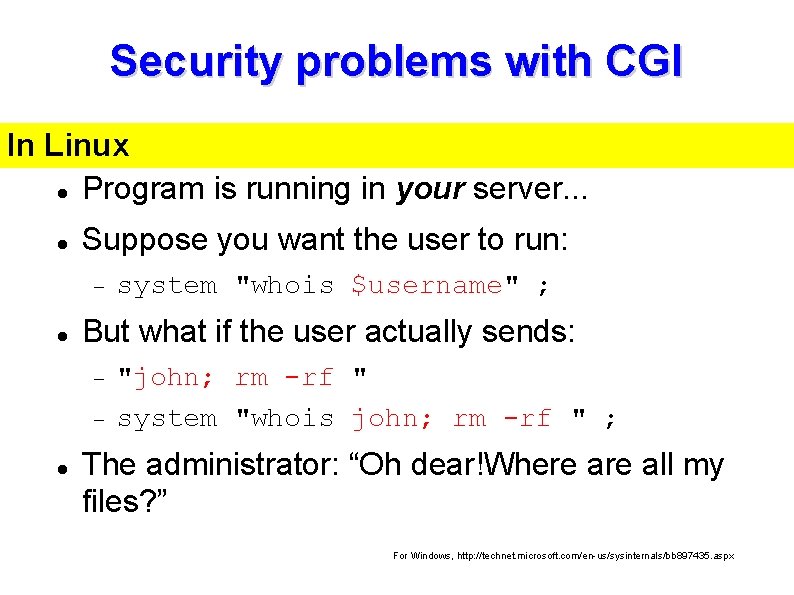
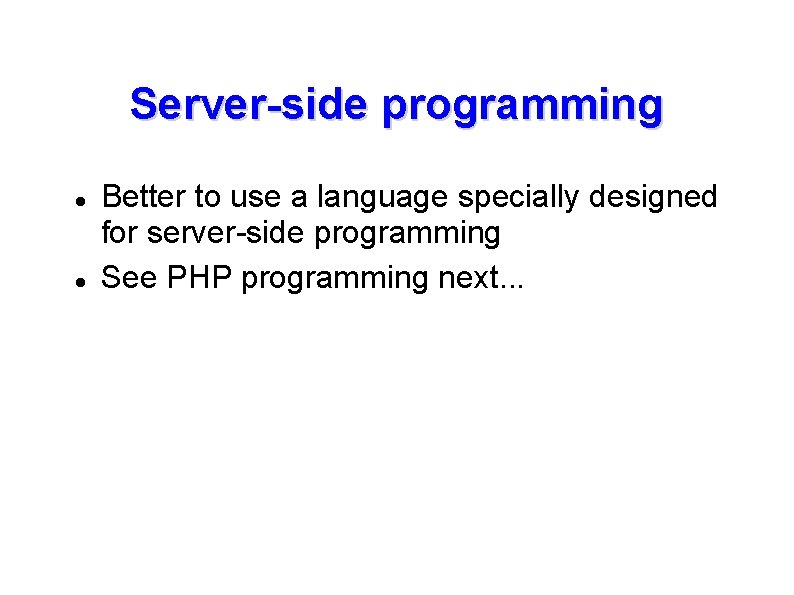
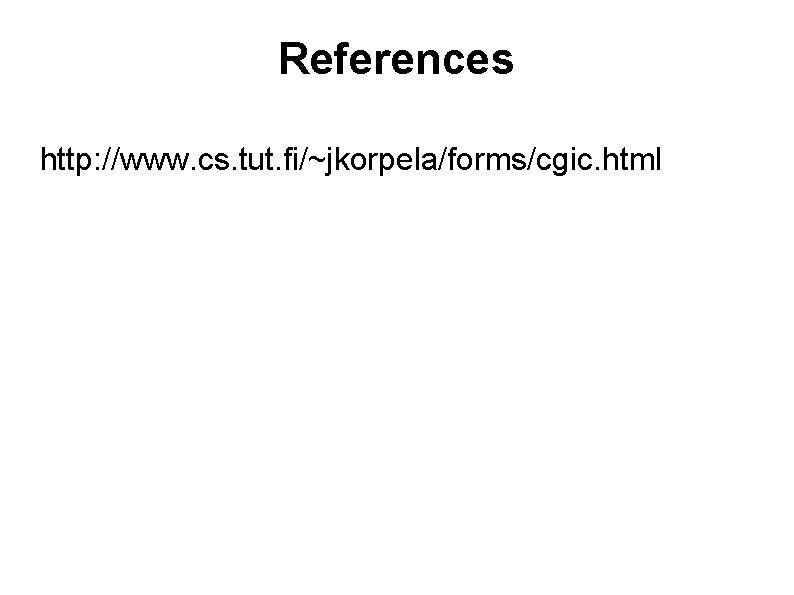
- Slides: 29
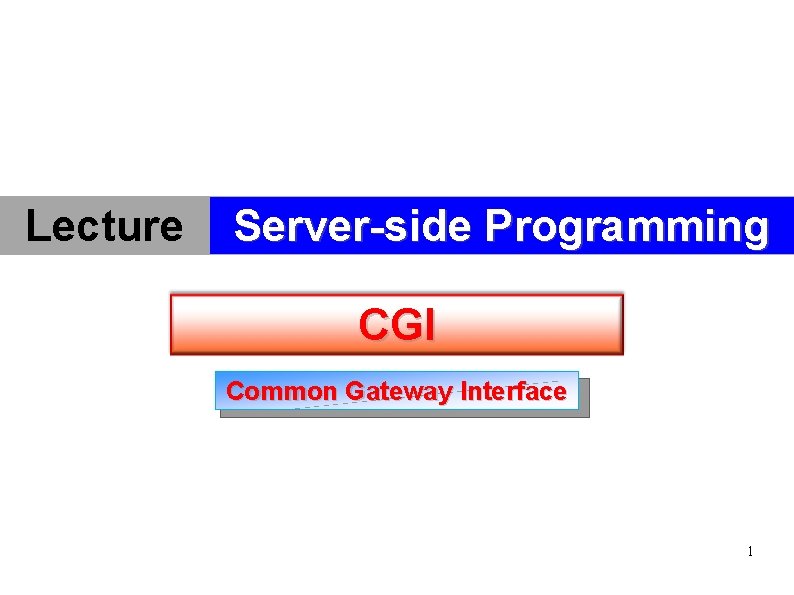
Lecture Server-side Programming CGI Common Gateway Interface 1
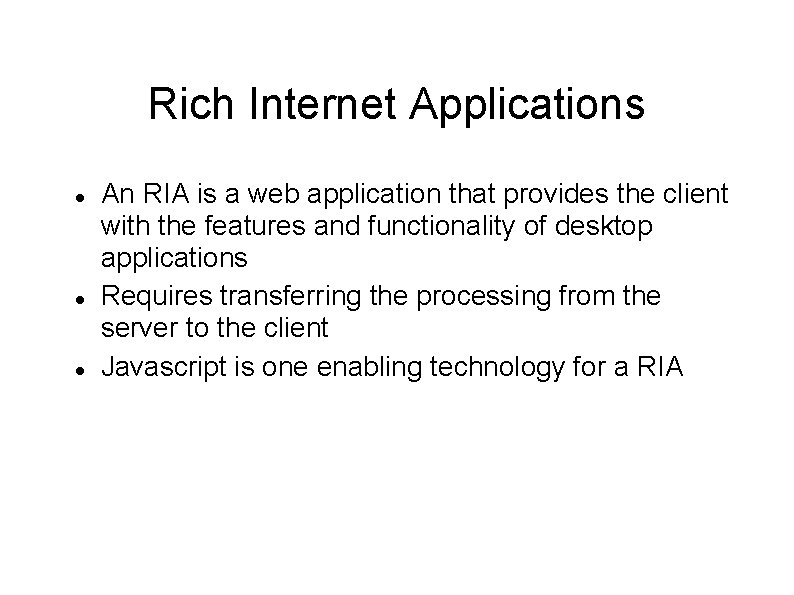
Rich Internet Applications An RIA is a web application that provides the client with the features and functionality of desktop applications Requires transferring the processing from the server to the client Javascript is one enabling technology for a RIA
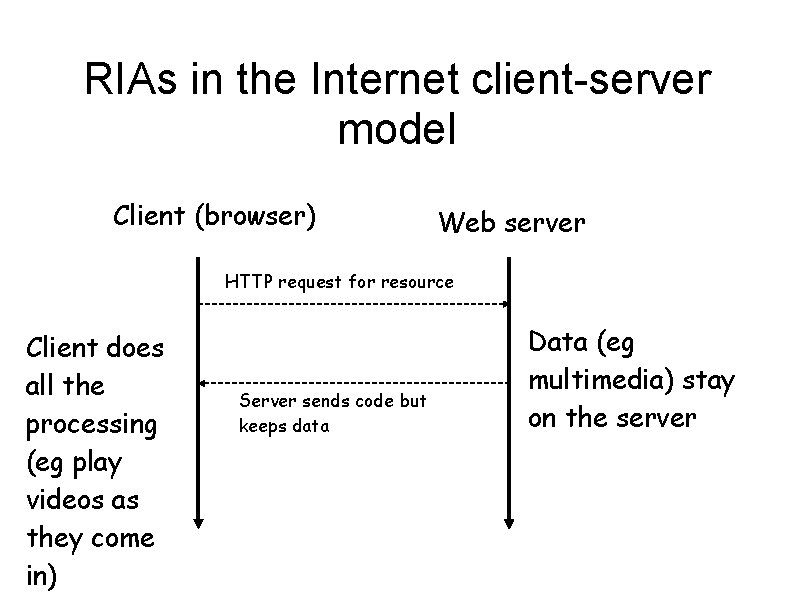
RIAs in the Internet client-server model Client (browser) Web server HTTP request for resource Client does all the processing (eg play videos as they come in) Server sends code but keeps data Data (eg multimedia) stay on the server
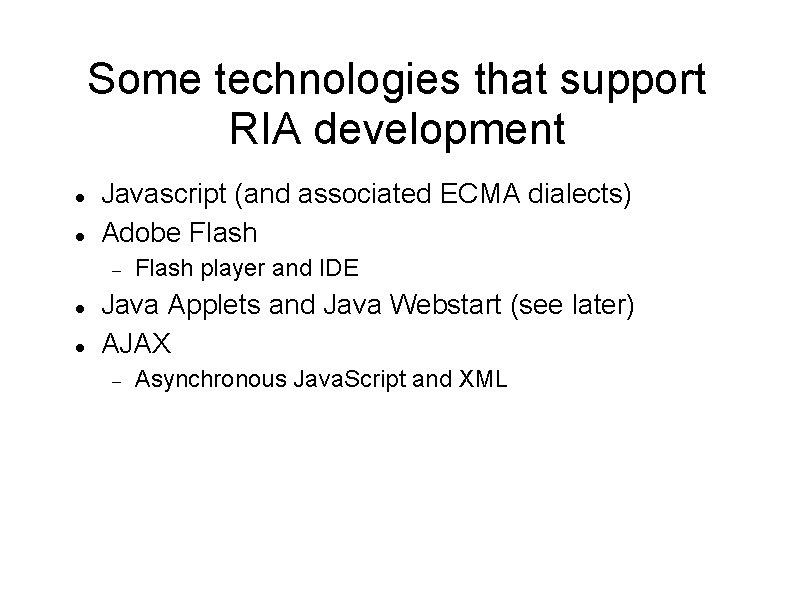
Some technologies that support RIA development Javascript (and associated ECMA dialects) Adobe Flash player and IDE Java Applets and Java Webstart (see later) AJAX Asynchronous Java. Script and XML
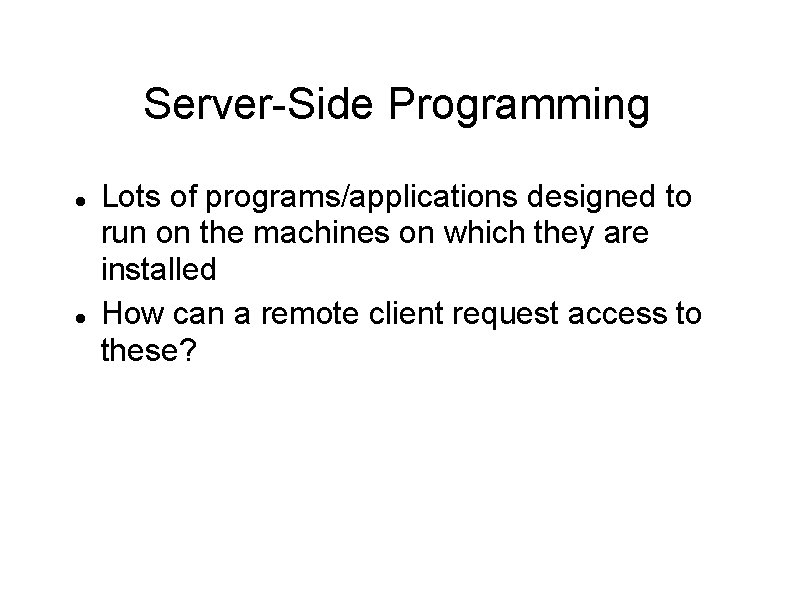
Server-Side Programming Lots of programs/applications designed to run on the machines on which they are installed How can a remote client request access to these?
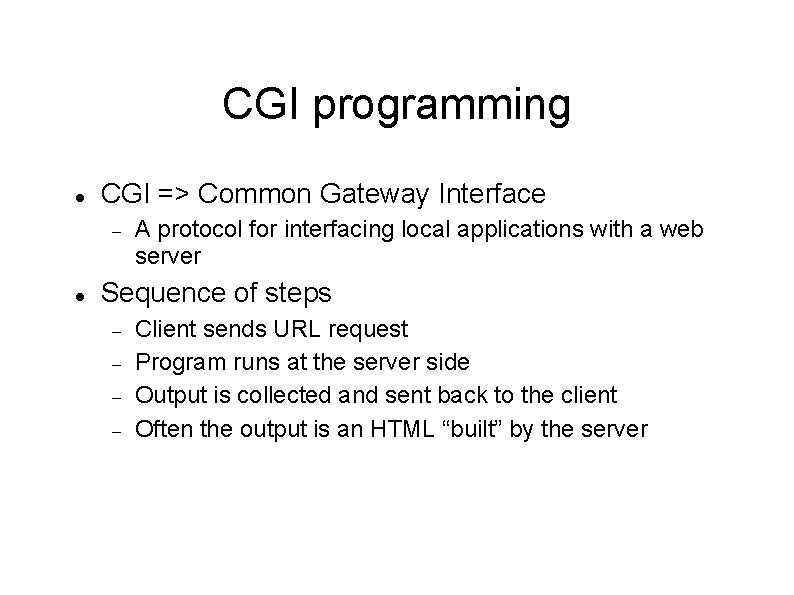
CGI programming CGI => Common Gateway Interface A protocol for interfacing local applications with a web server Sequence of steps Client sends URL request Program runs at the server side Output is collected and sent back to the client Often the output is an HTML “built” by the server
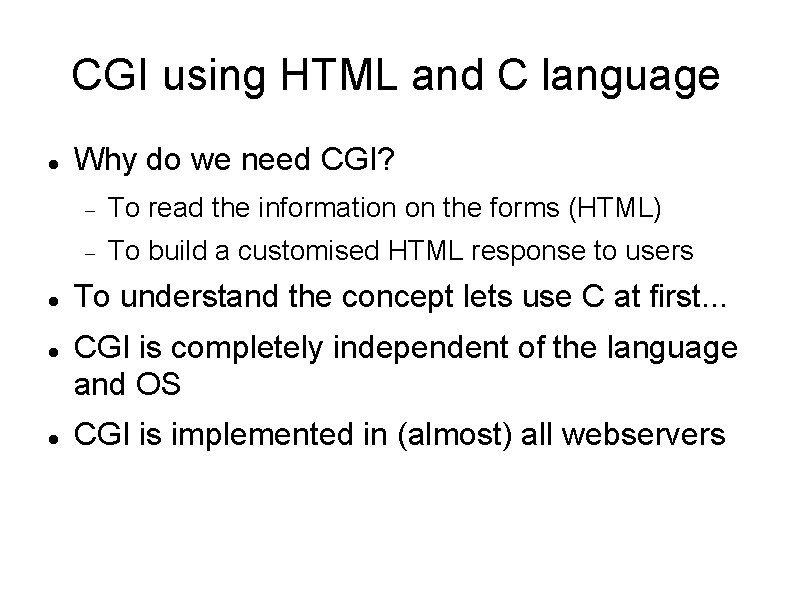
CGI using HTML and C language Why do we need CGI? To read the information on the forms (HTML) To build a customised HTML response to users To understand the concept lets use C at first. . . CGI is completely independent of the language and OS CGI is implemented in (almost) all webservers
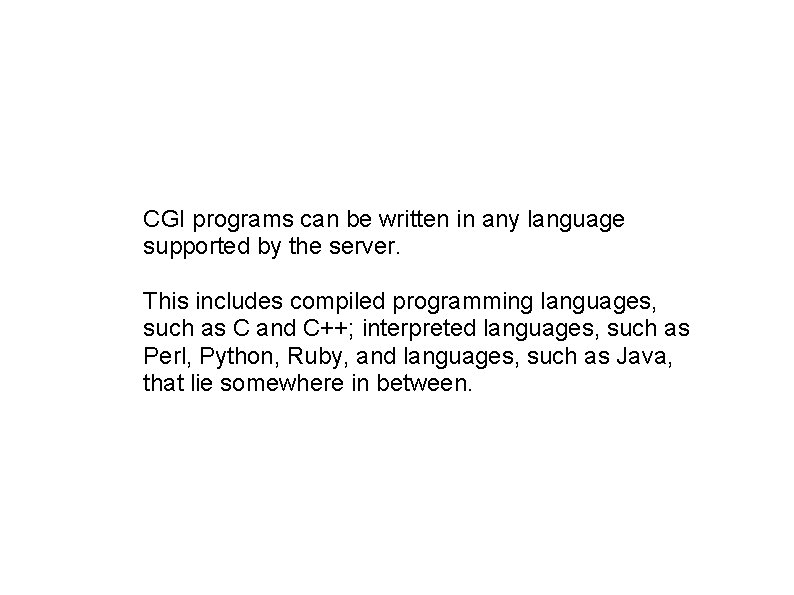
CGI programs can be written in any language supported by the server. This includes compiled programming languages, such as C and C++; interpreted languages, such as Perl, Python, Ruby, and languages, such as Java, that lie somewhere in between.
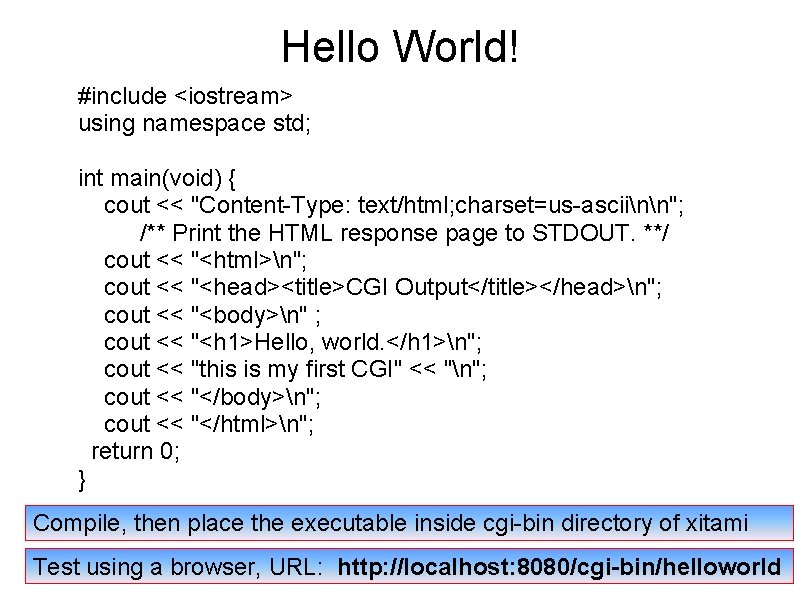
Hello World! #include <iostream> using namespace std; int main(void) { cout << "Content-Type: text/html; charset=us-asciinn"; /** Print the HTML response page to STDOUT. **/ cout << "<html>n"; cout << "<head><title>CGI Output</title></head>n"; cout << "<body>n" ; cout << "<h 1>Hello, world. </h 1>n"; cout << "this is my first CGI" << "n"; cout << "</body>n"; cout << "</html>n"; return 0; } Compile, then place the executable inside cgi-bin directory of xitami Test using a browser, URL: http: //localhost: 8080/cgi-bin/helloworld
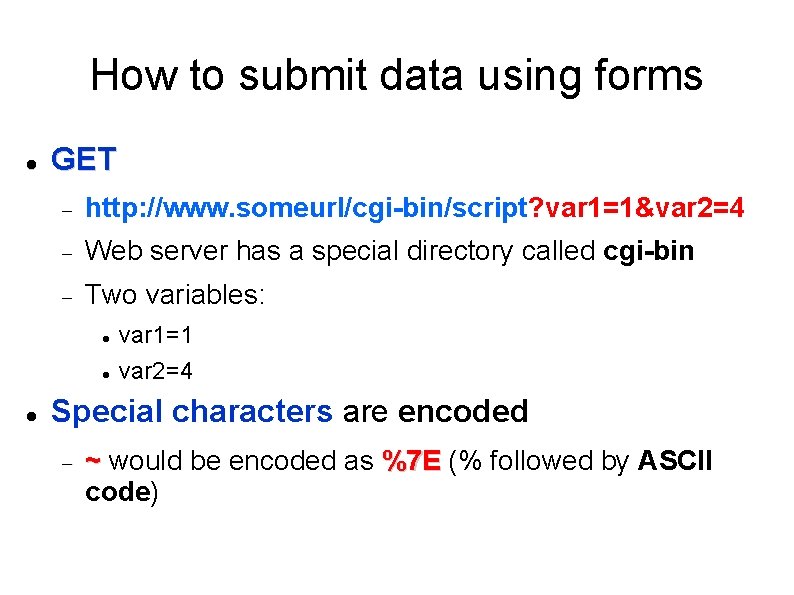
How to submit data using forms GET http: //www. someurl/cgi-bin/script? var 1=1&var 2=4 Web server has a special directory called cgi-bin Two variables: var 1=1 var 2=4 Special characters are encoded ~ would be encoded as %7 E (% followed by ASCII code)
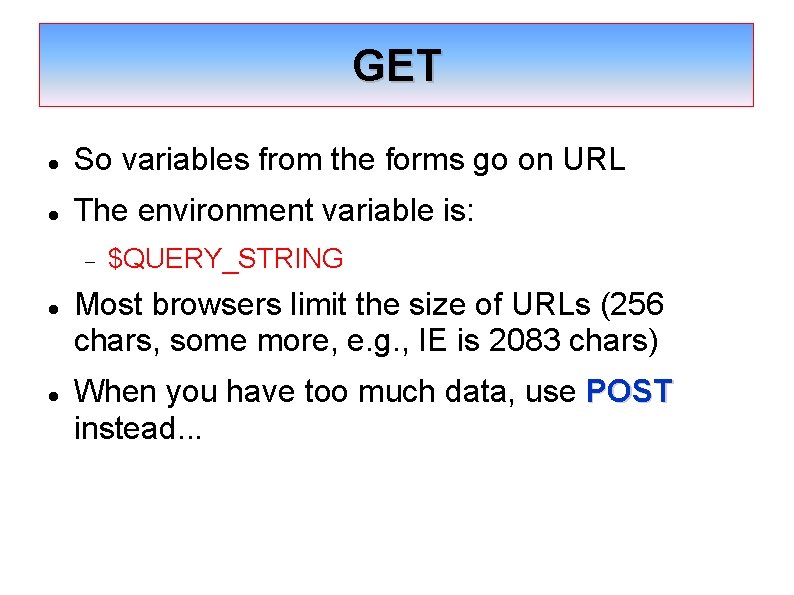
GET So variables from the forms go on URL The environment variable is: $QUERY_STRING Most browsers limit the size of URLs (256 chars, some more, e. g. , IE is 2083 chars) When you have too much data, use POST instead. . .
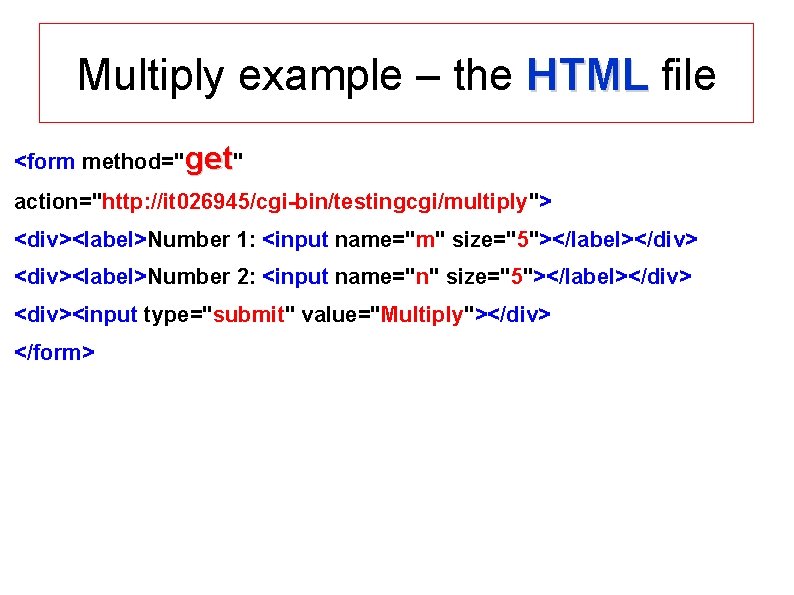
Multiply example – the HTML file <form method="get" action="http: //it 026945/cgi-bin/testingcgi/multiply"> <div><label>Number 1: <input name="m" size="5"></label></div> <div><label>Number 2: <input name="n" size="5"></label></div> <div><input type="submit" value="Multiply"></div> </form>
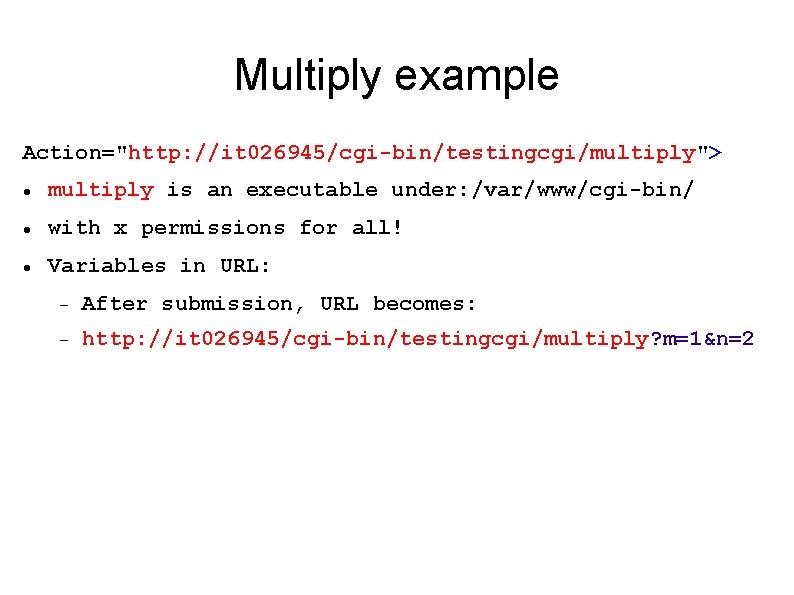
Multiply example Action="http: //it 026945/cgi-bin/testingcgi/multiply"> multiply is an executable under: /var/www/cgi-bin/ with x permissions for all! Variables in URL: After submission, URL becomes: http: //it 026945/cgi-bin/testingcgi/multiply? m=1&n=2
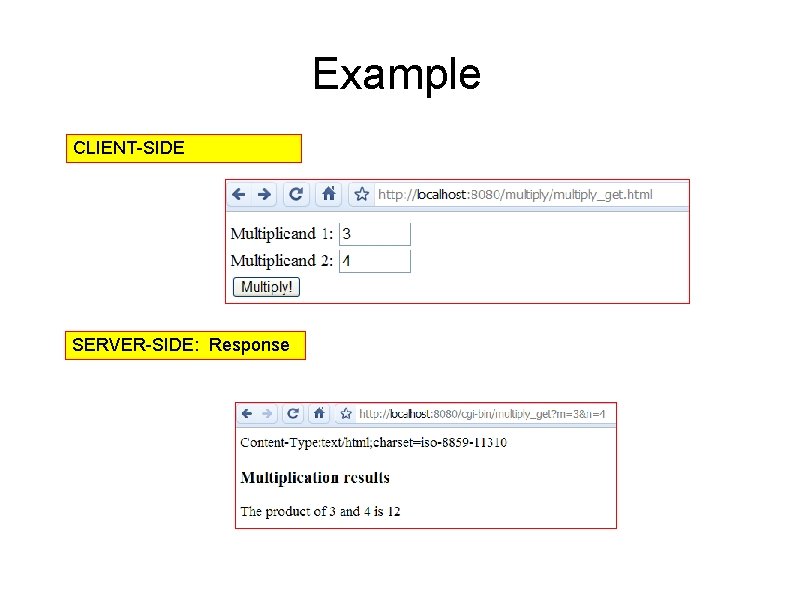
Example CLIENT-SIDE SERVER-SIDE: Response
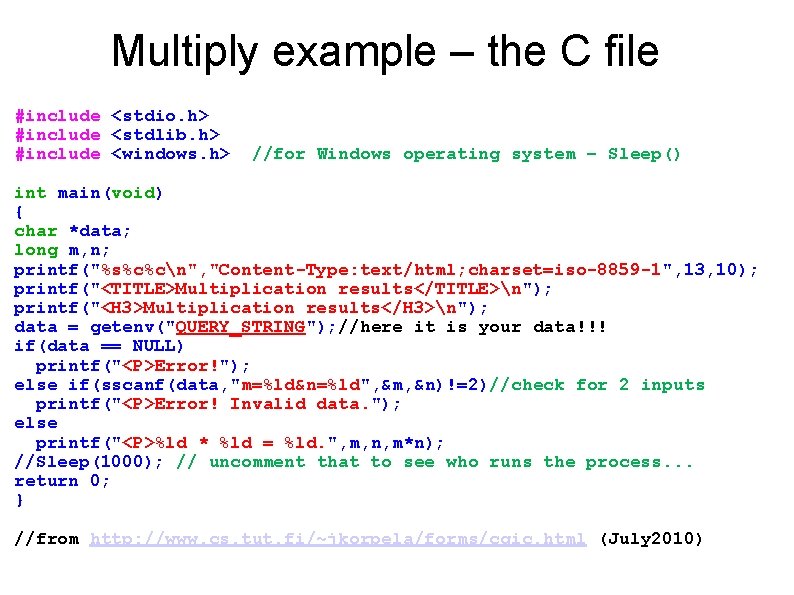
Multiply example – the C file #include <stdio. h> #include <stdlib. h> #include <windows. h> //for Windows operating system – Sleep() int main(void) { char *data; long m, n; printf("%s%c%cn", "Content-Type: text/html; charset=iso-8859 -1", 13, 10); printf("<TITLE>Multiplication results</TITLE>n"); printf("<H 3>Multiplication results</H 3>n"); data = getenv("QUERY_STRING"); //here it is your data!!! if(data == NULL) printf("<P>Error!"); else if(sscanf(data, "m=%ld&n=%ld", &m, &n)!=2)//check for 2 inputs printf("<P>Error! Invalid data. "); else printf("<P>%ld * %ld = %ld. ", m, n, m*n); //Sleep(1000); // uncomment that to see who runs the process. . . return 0; } //from http: //www. cs. tut. fi/~jkorpela/forms/cgic. html (July 2010)
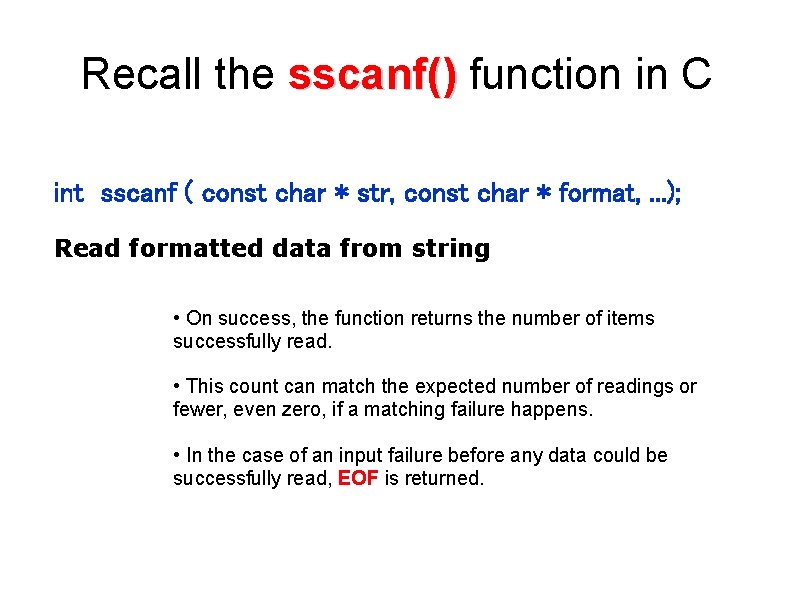
Recall the sscanf() function in C int sscanf ( const char * str, const char * format, . . . ); Read formatted data from string • On success, the function returns the number of items successfully read. • This count can match the expected number of readings or fewer, even zero, if a matching failure happens. • In the case of an input failure before any data could be successfully read, EOF is returned.
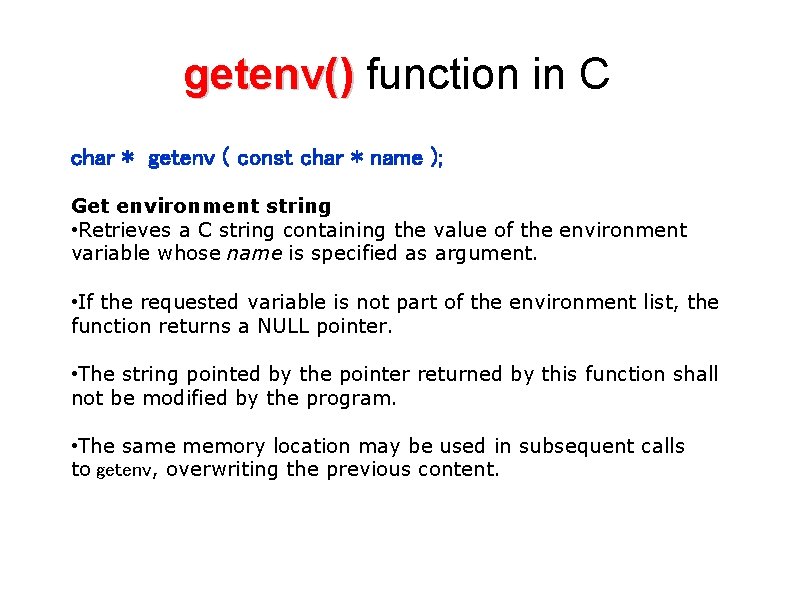
getenv() function in C char * getenv ( const char * name ); Get environment string • Retrieves a C string containing the value of the environment variable whose name is specified as argument. • If the requested variable is not part of the environment list, the function returns a NULL pointer. • The string pointed by the pointer returned by this function shall not be modified by the program. • The same memory location may be used in subsequent calls to getenv, overwriting the previous content.
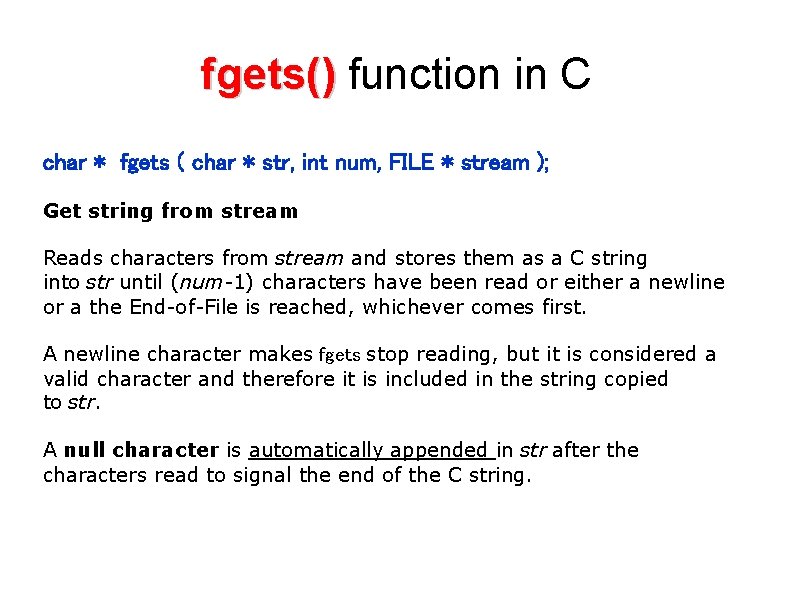
fgets() function in C char * fgets ( char * str, int num, FILE * stream ); Get string from stream Reads characters from stream and stores them as a C string into str until (num-1) characters have been read or either a newline or a the End-of-File is reached, whichever comes first. A newline character makes fgets stop reading, but it is considered a valid character and therefore it is included in the string copied to str. A null character is automatically appended in str after the characters read to signal the end of the C string.
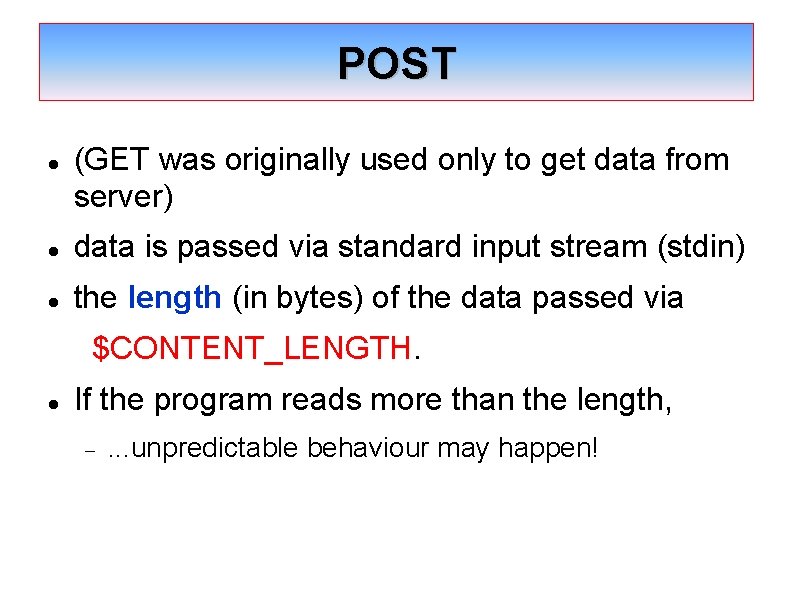
POST (GET was originally used only to get data from server) data is passed via standard input stream (stdin) the length (in bytes) of the data passed via $CONTENT_LENGTH. If the program reads more than the length, . . . unpredictable behaviour may happen!
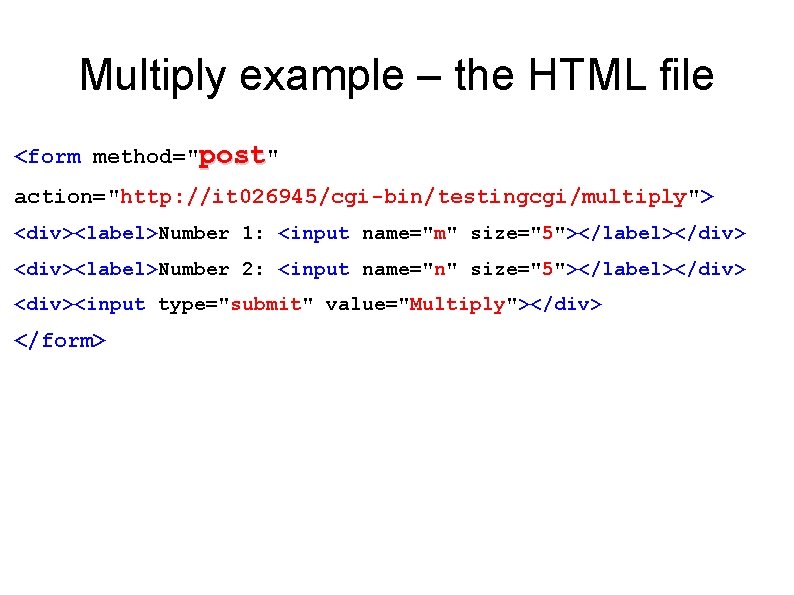
Multiply example – the HTML file <form method="post" action="http: //it 026945/cgi-bin/testingcgi/multiply"> <div><label>Number 1: <input name="m" size="5"></label></div> <div><label>Number 2: <input name="n" size="5"></label></div> <div><input type="submit" value="Multiply"></div> </form>
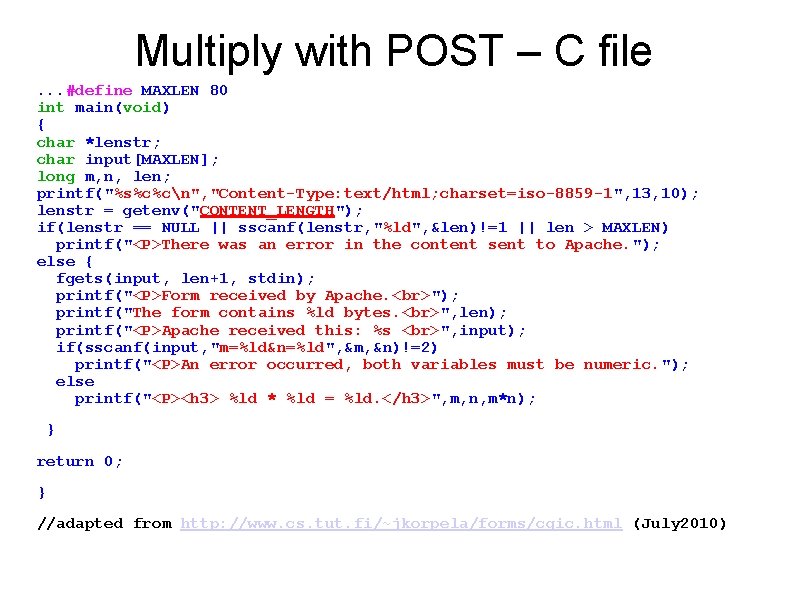
Multiply with POST – C file. . . #define MAXLEN 80 int main(void) { char *lenstr; char input[MAXLEN]; long m, n, len; printf("%s%c%cn", "Content-Type: text/html; charset=iso-8859 -1", 13, 10); lenstr = getenv("CONTENT_LENGTH"); if(lenstr == NULL || sscanf(lenstr, "%ld", &len)!=1 || len > MAXLEN) printf("<P>There was an error in the content sent to Apache. "); else { fgets(input, len+1, stdin); printf("<P>Form received by Apache. "); printf("The form contains %ld bytes. ", len); printf("<P>Apache received this: %s ", input); if(sscanf(input, "m=%ld&n=%ld", &m, &n)!=2) printf("<P>An error occurred, both variables must be numeric. "); else printf("<P><h 3> %ld * %ld = %ld. </h 3>", m, n, m*n); } return 0; } //adapted from http: //www. cs. tut. fi/~jkorpela/forms/cgic. html (July 2010)
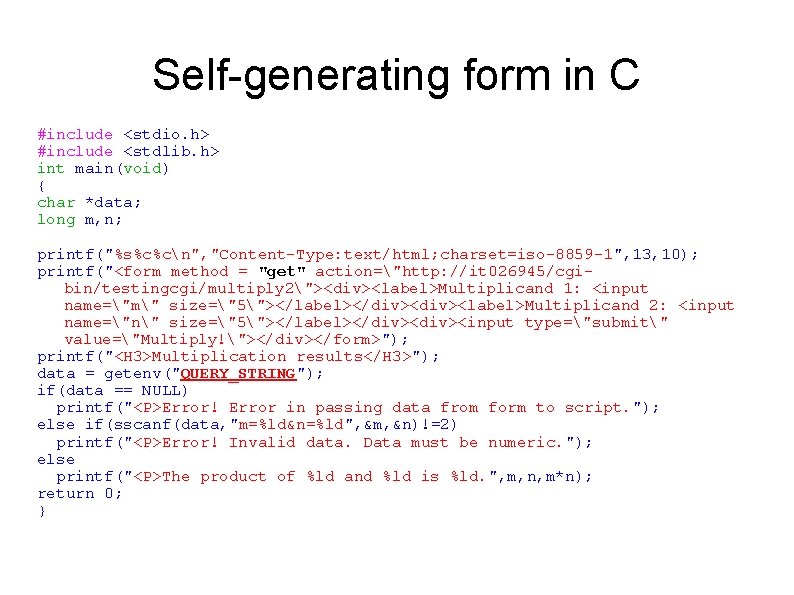
Self-generating form in C #include <stdio. h> #include <stdlib. h> int main(void) { char *data; long m, n; printf("%s%c%cn", "Content-Type: text/html; charset=iso-8859 -1", 13, 10); printf("<form method = "get" action="http: //it 026945/cgibin/testingcgi/multiply 2"><div><label>Multiplicand 1: <input name="m" size="5"></label></div><label>Multiplicand 2: <input name="n" size="5"></label></div><input type="submit" value="Multiply!"></div></form>"); printf("<H 3>Multiplication results</H 3>"); data = getenv("QUERY_STRING"); if(data == NULL) printf("<P>Error! Error in passing data from form to script. "); else if(sscanf(data, "m=%ld&n=%ld", &m, &n)!=2) printf("<P>Error! Invalid data. Data must be numeric. "); else printf("<P>The product of %ld and %ld is %ld. ", m, n, m*n); return 0; }
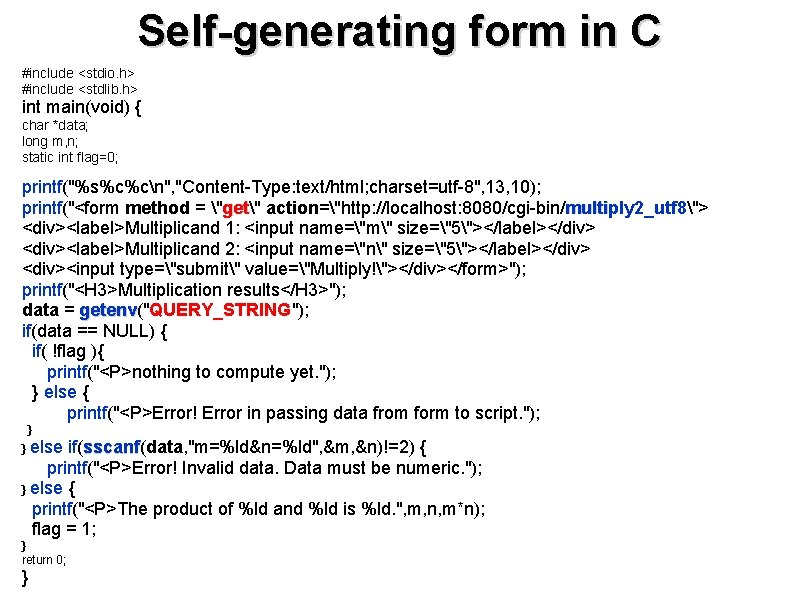
Self-generating form in C #include <stdio. h> #include <stdlib. h> int main(void) { char *data; long m, n; static int flag=0; printf("%s%c%cn", "Content-Type: text/html; charset=utf-8", 13, 10); printf("<form method = "get" get action="http: //localhost: 8080/cgi-bin/multiply 2_utf 8"> multiply 2_utf 8 <div><label>Multiplicand 1: <input name="m" size="5"></label></div> <div><label>Multiplicand 2: <input name="n" size="5"></label></div> <div><input type="submit" value="Multiply!"></div></form>"); printf("<H 3>Multiplication results</H 3>"); data = getenv("QUERY_STRING"); getenv if(data == NULL) { if( !flag ){ printf("<P>nothing to compute yet. "); } else { printf("<P>Error! Error in passing data from form to script. "); } } else if(sscanf(data, "m=%ld&n=%ld", &m, &n)!=2) { sscanf printf("<P>Error! Invalid data. Data must be numeric. "); } else { printf("<P>The product of %ld and %ld is %ld. ", m, n, m*n); flag = 1; } return 0; }
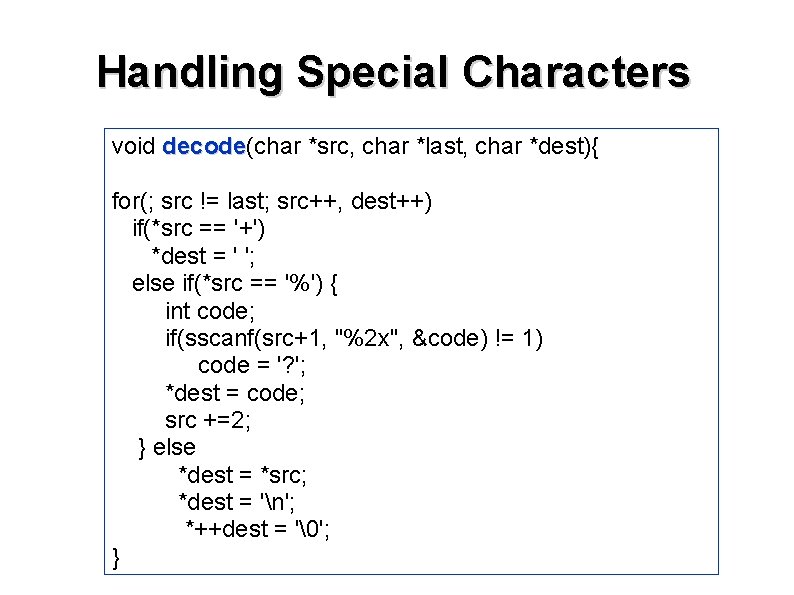
Handling Special Characters void decode(char *src, char *last, char *dest){ decode for(; src != last; src++, dest++) if(*src == '+') *dest = ' '; else if(*src == '%') { int code; if(sscanf(src+1, "%2 x", &code) != 1) code = '? '; *dest = code; src +=2; } else *dest = *src; *dest = 'n'; *++dest = '