Lecture 7 Arrays 1 Uni MAP Sem I
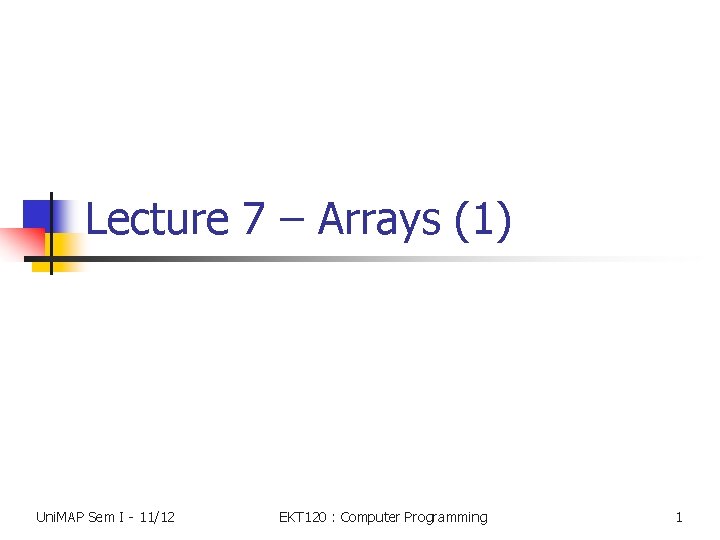
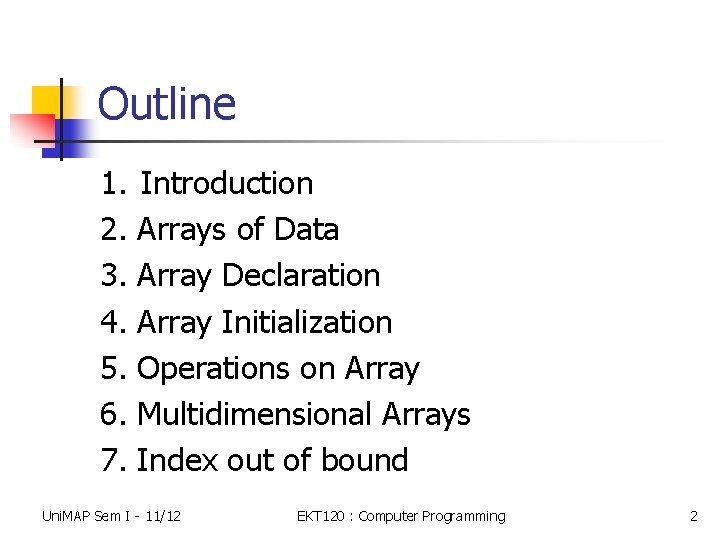
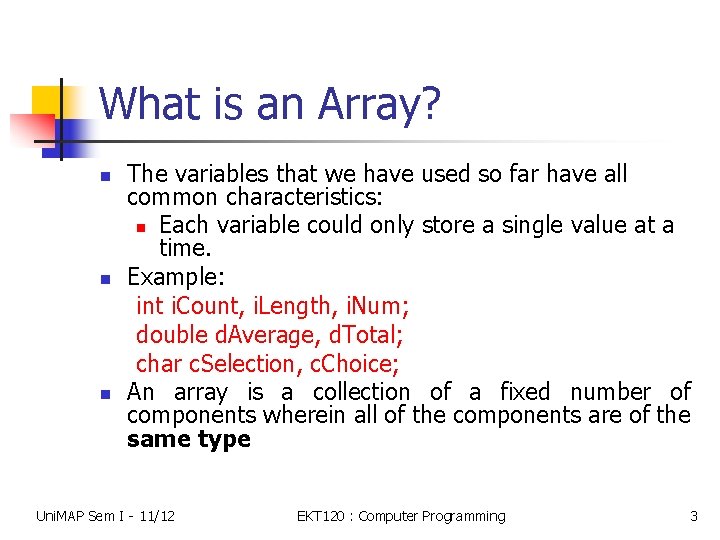
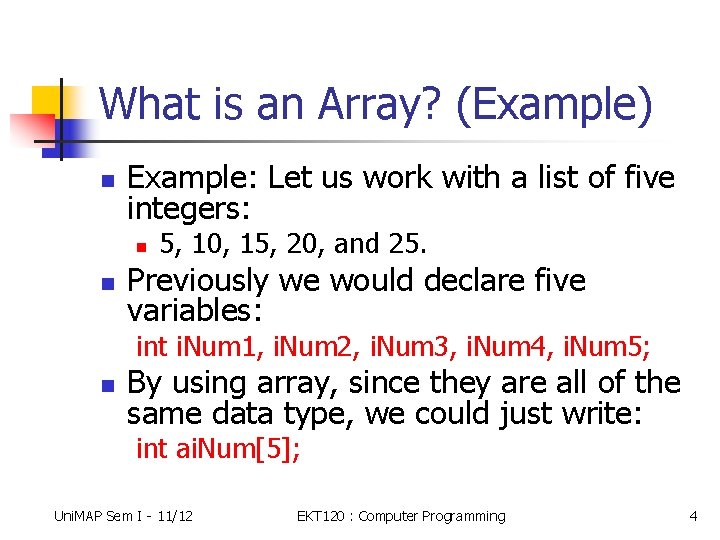
![What is an Array? (Example) ai. Num 5 ai. Num[0] 10 ai. Num[1] 15 What is an Array? (Example) ai. Num 5 ai. Num[0] 10 ai. Num[1] 15](https://slidetodoc.com/presentation_image_h2/7279efc1c73464aa9d95fe88956088b0/image-5.jpg)
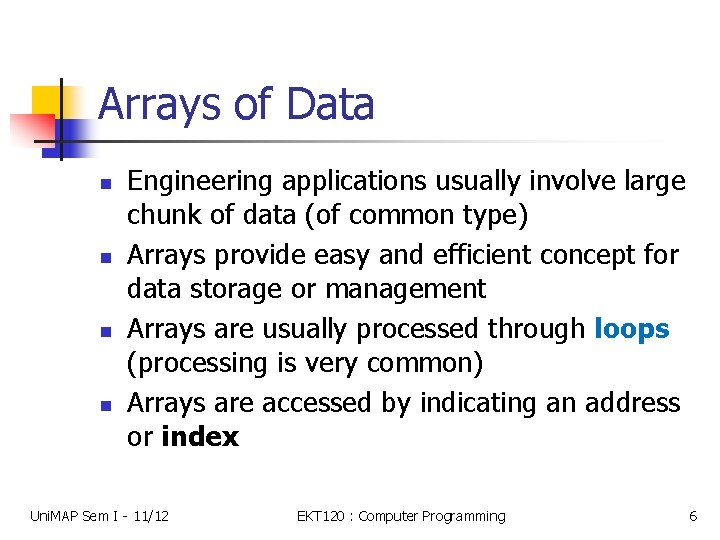
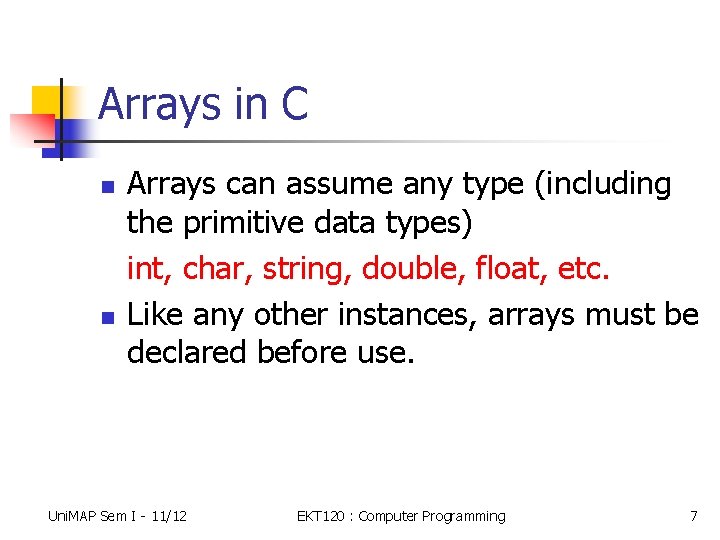
![Array Declaration Format: n n data_type array_name[int value]; Example: n n n int ai. Array Declaration Format: n n data_type array_name[int value]; Example: n n n int ai.](https://slidetodoc.com/presentation_image_h2/7279efc1c73464aa9d95fe88956088b0/image-8.jpg)
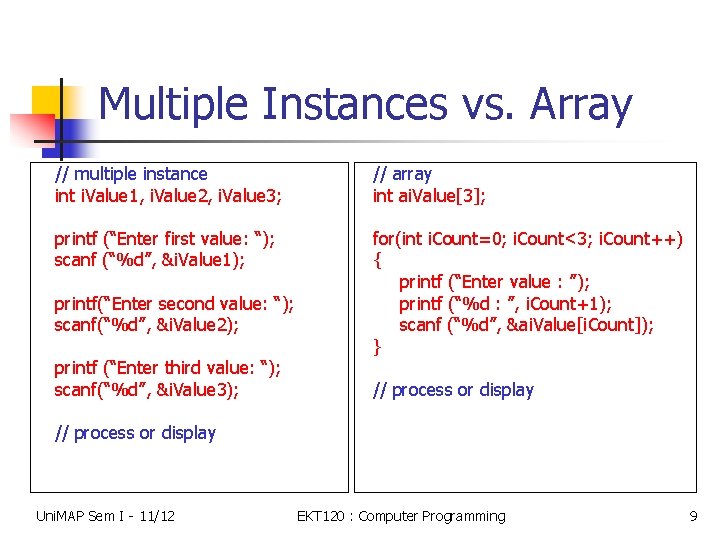
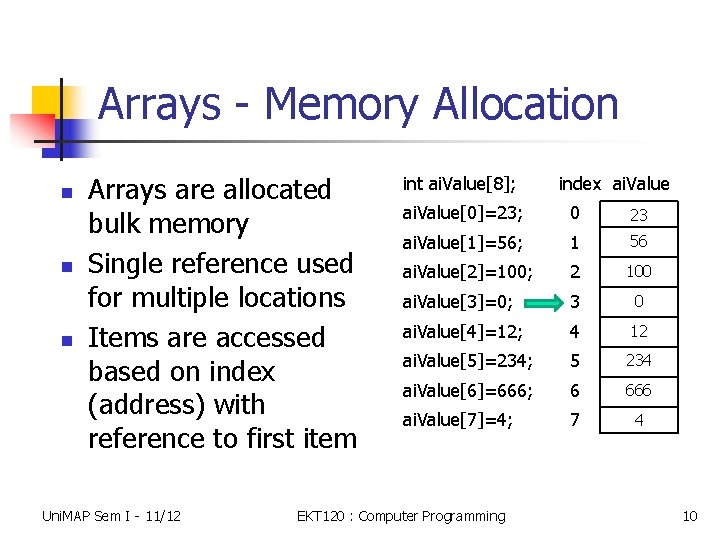
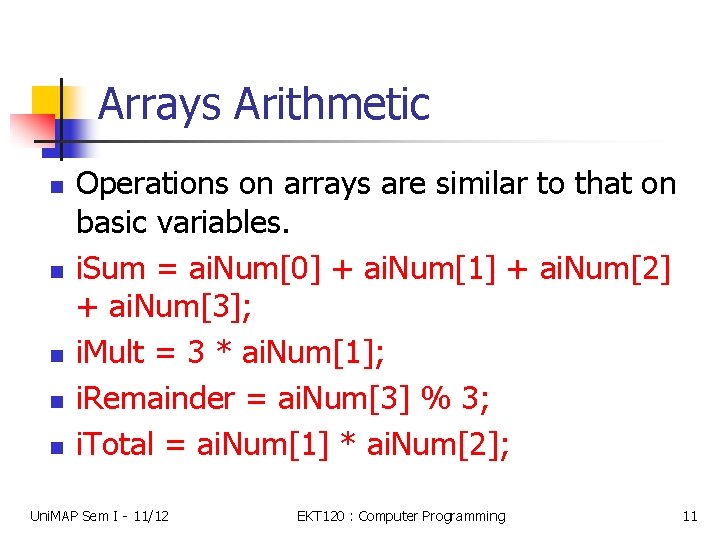
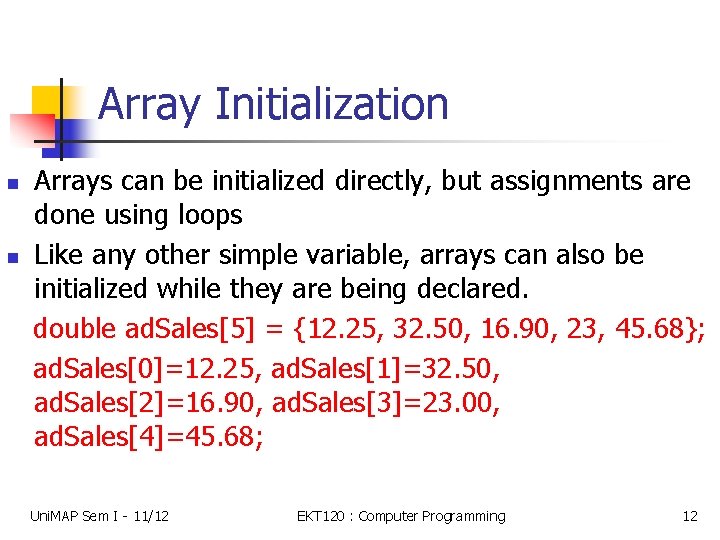
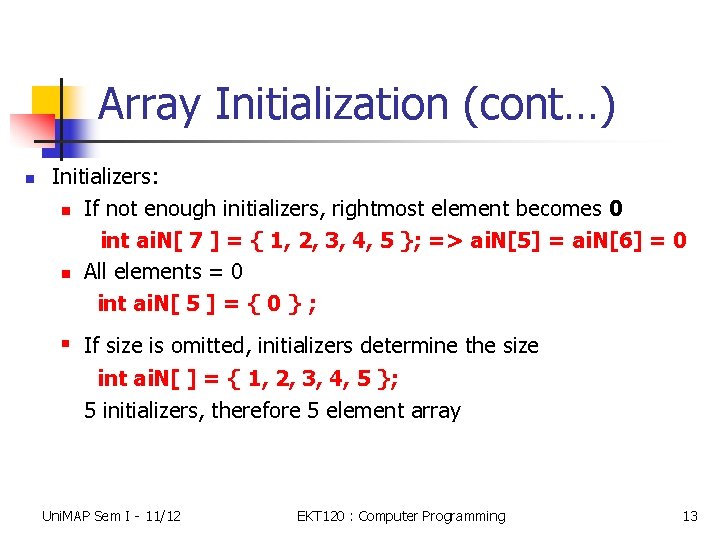
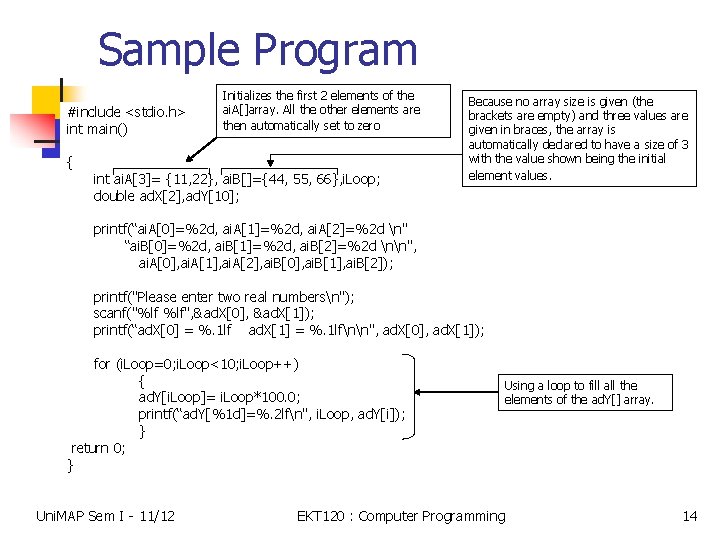
![Sample Program n Output: ai. A[0]=11, ai. A[1]=22, ai. A[2]= 0 ai. B[0]=44, ai. Sample Program n Output: ai. A[0]=11, ai. A[1]=22, ai. A[2]= 0 ai. B[0]=44, ai.](https://slidetodoc.com/presentation_image_h2/7279efc1c73464aa9d95fe88956088b0/image-15.jpg)
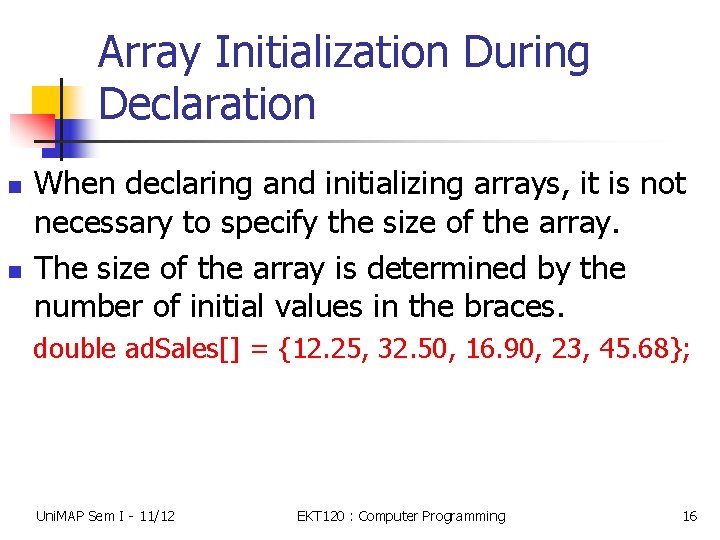
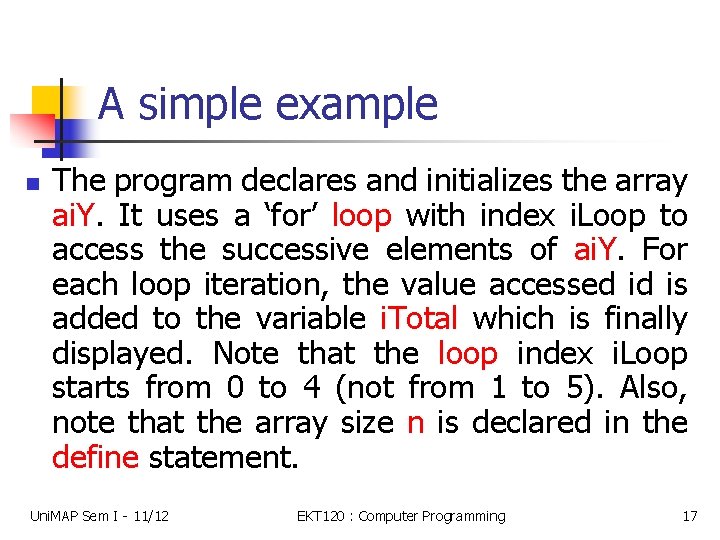
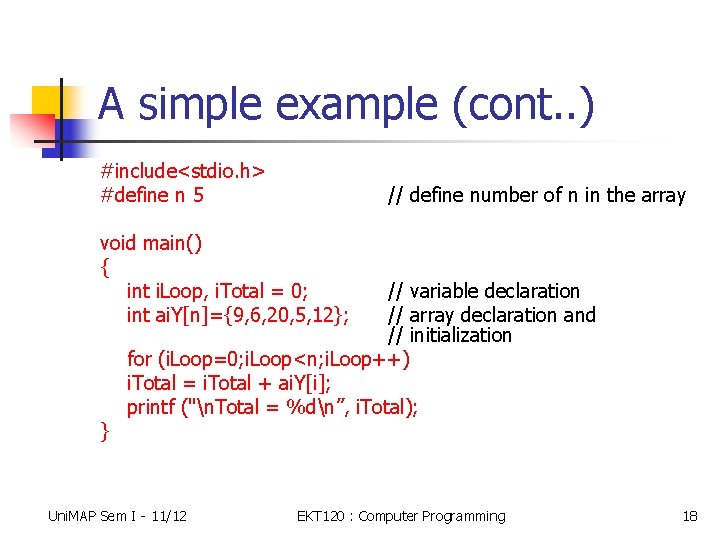
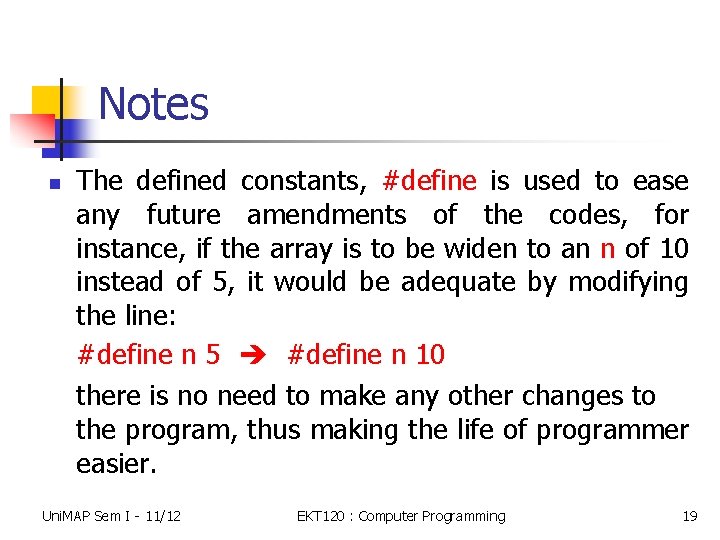
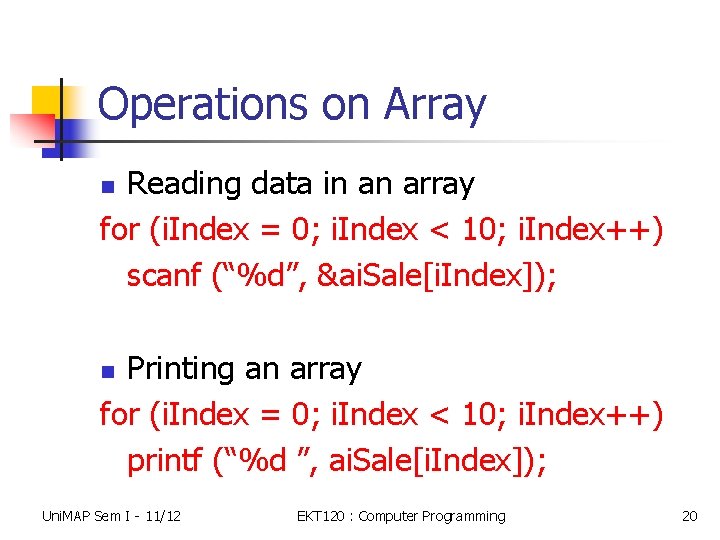
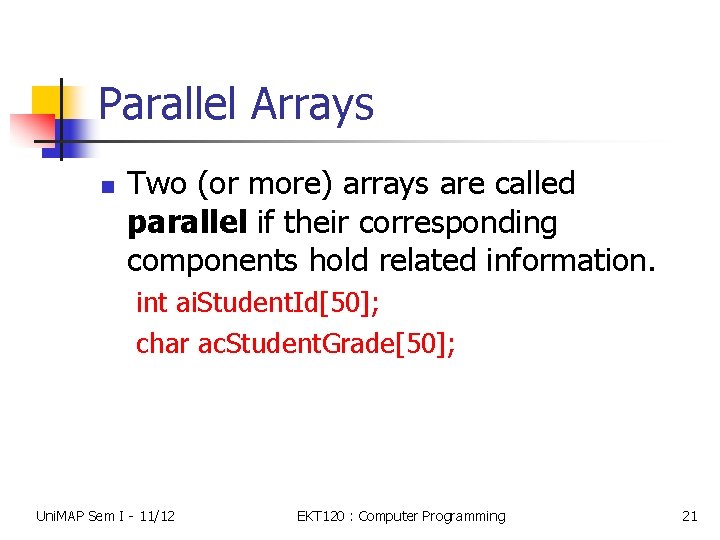
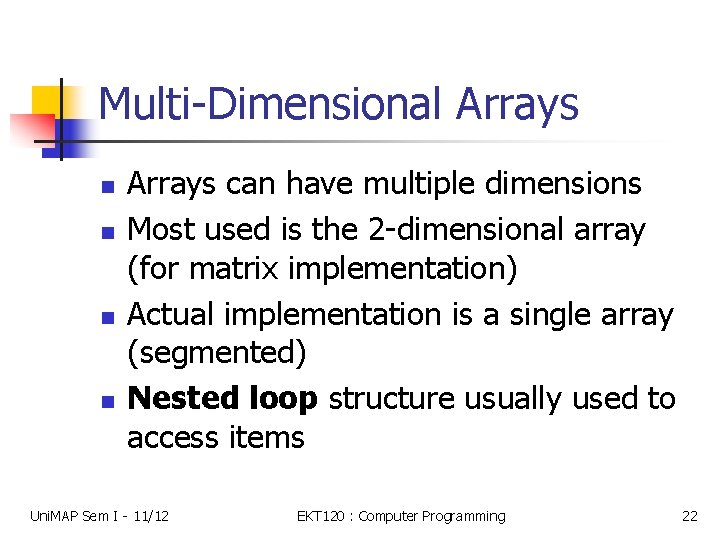
![2 -Dimensional Array (Example) int ai. Value[4][2]; ai. Value[2][1]=5; index ai. Value Row 0 2 -Dimensional Array (Example) int ai. Value[4][2]; ai. Value[2][1]=5; index ai. Value Row 0](https://slidetodoc.com/presentation_image_h2/7279efc1c73464aa9d95fe88956088b0/image-23.jpg)
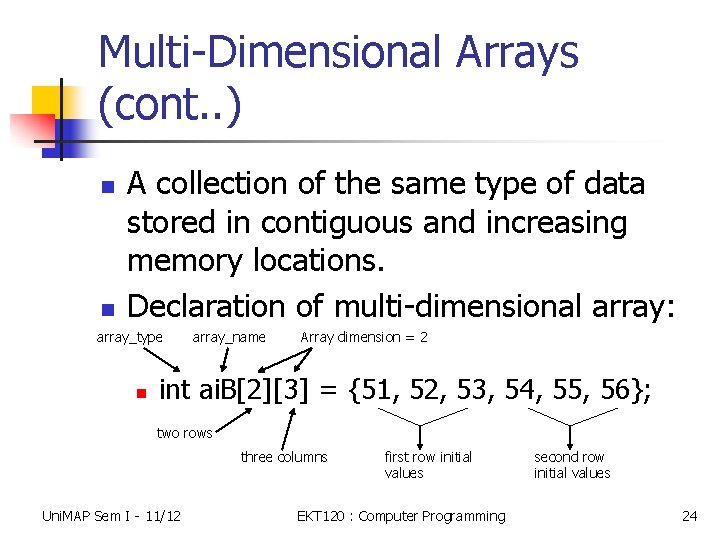
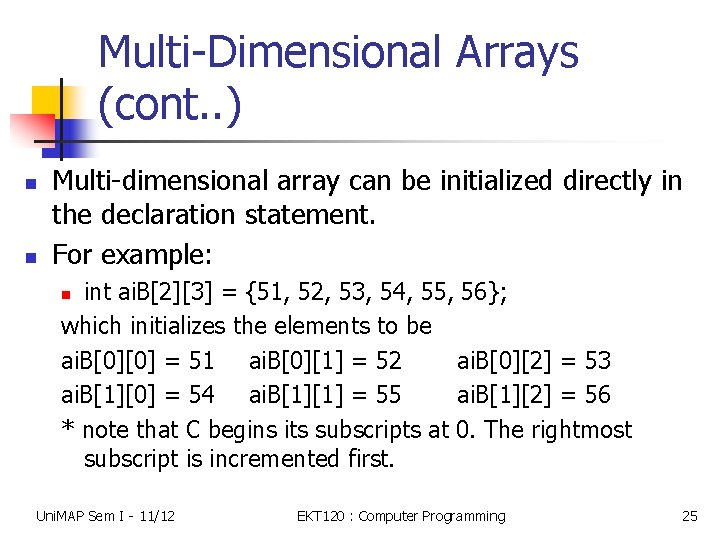
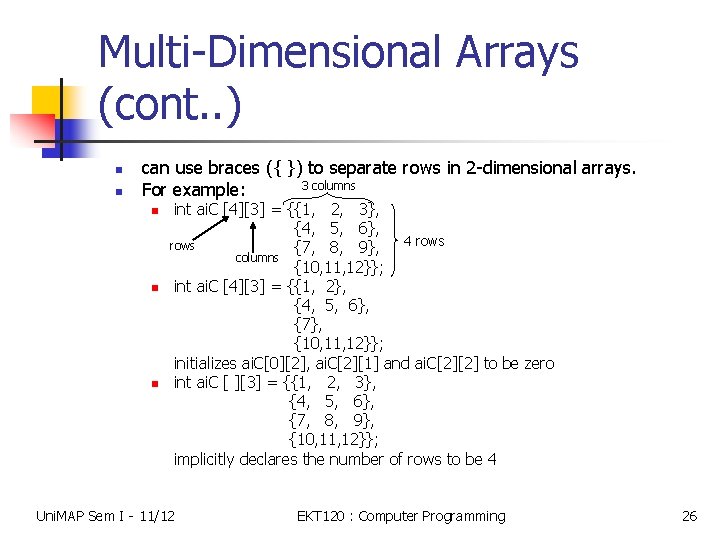
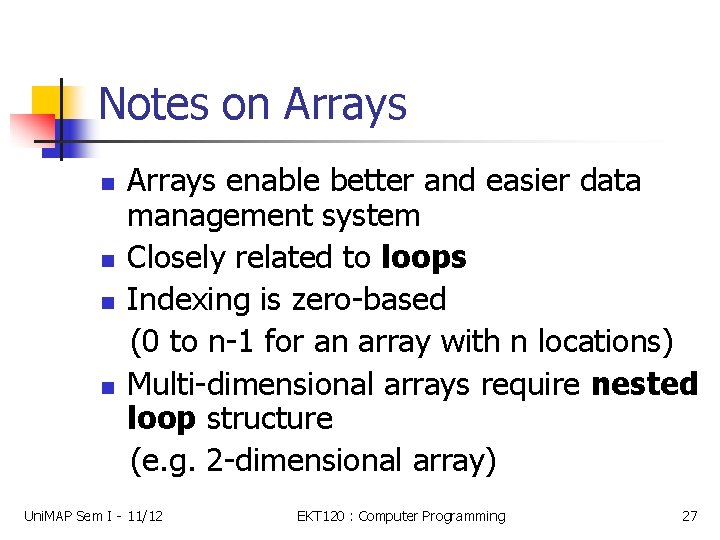
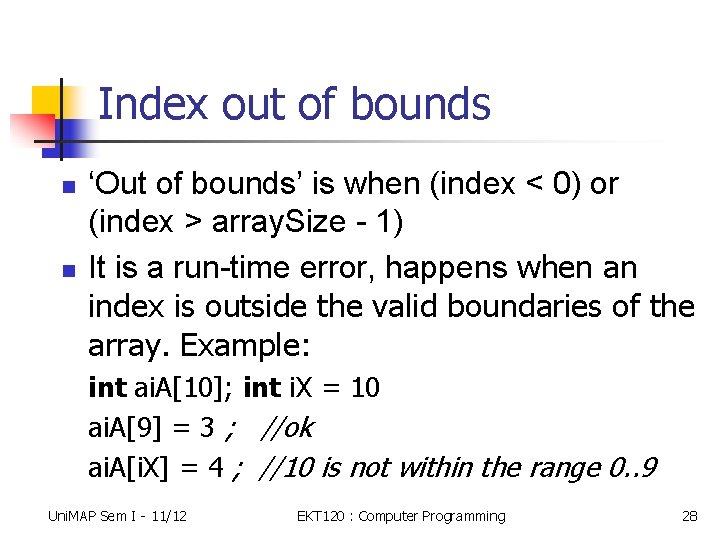
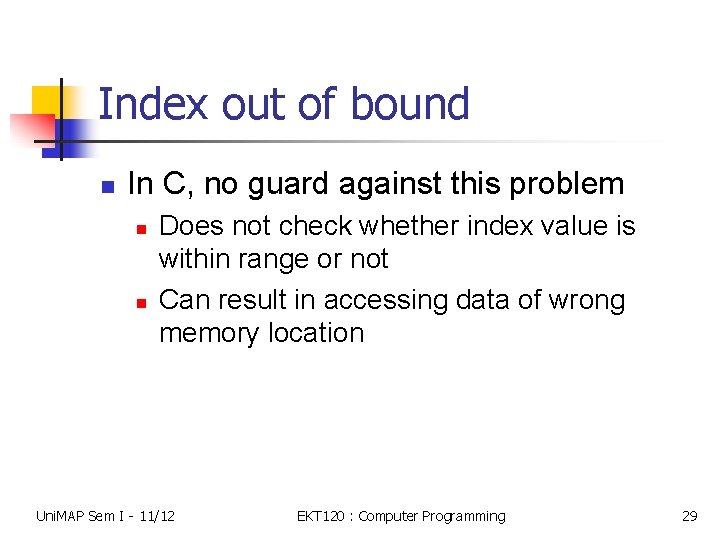
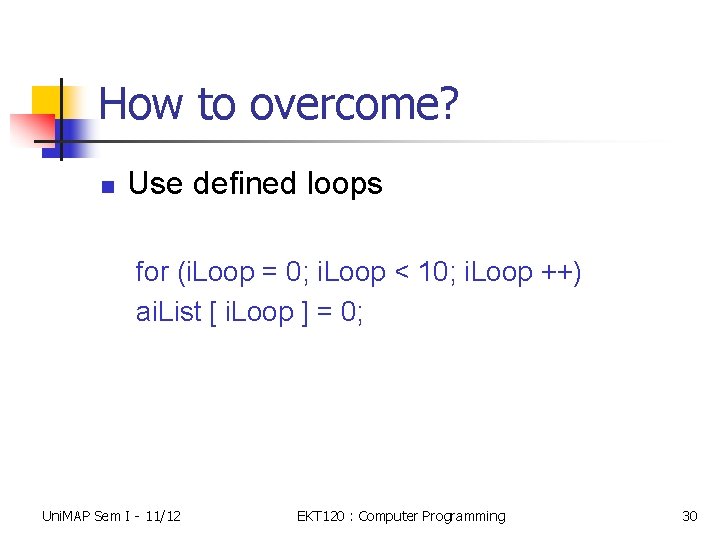
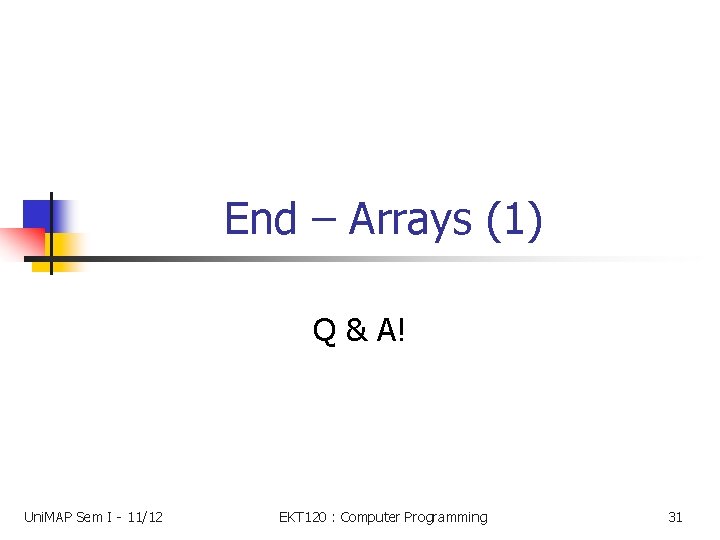
- Slides: 31
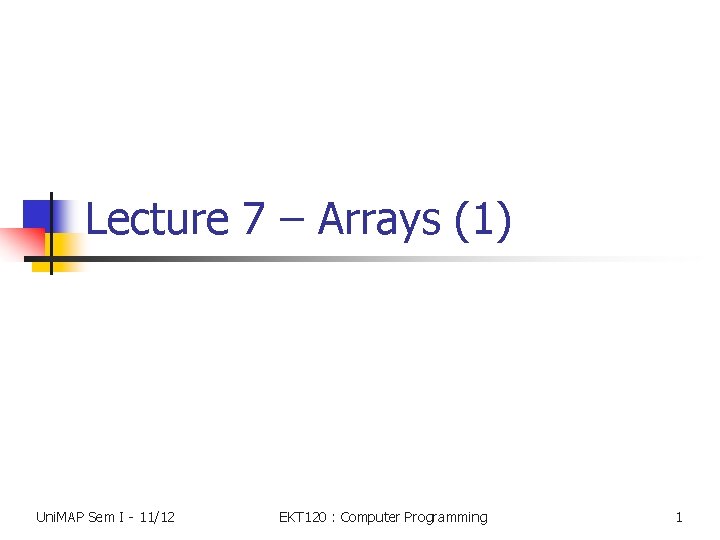
Lecture 7 – Arrays (1) Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 1
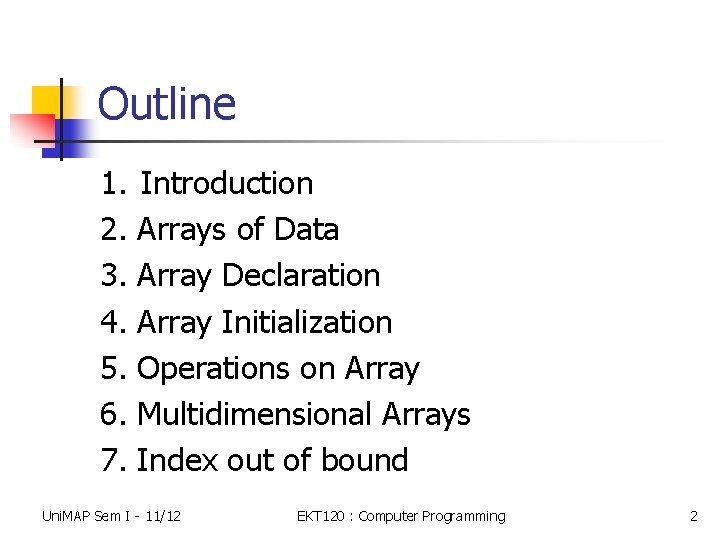
Outline 1. 2. 3. 4. 5. 6. 7. Introduction Arrays of Data Array Declaration Array Initialization Operations on Array Multidimensional Arrays Index out of bound Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 2
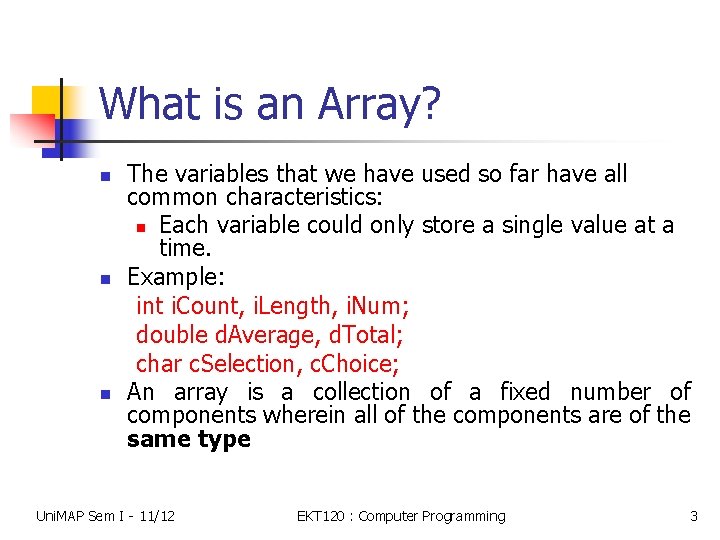
What is an Array? n n n The variables that we have used so far have all common characteristics: n Each variable could only store a single value at a time. Example: int i. Count, i. Length, i. Num; double d. Average, d. Total; char c. Selection, c. Choice; An array is a collection of a fixed number of components wherein all of the components are of the same type Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 3
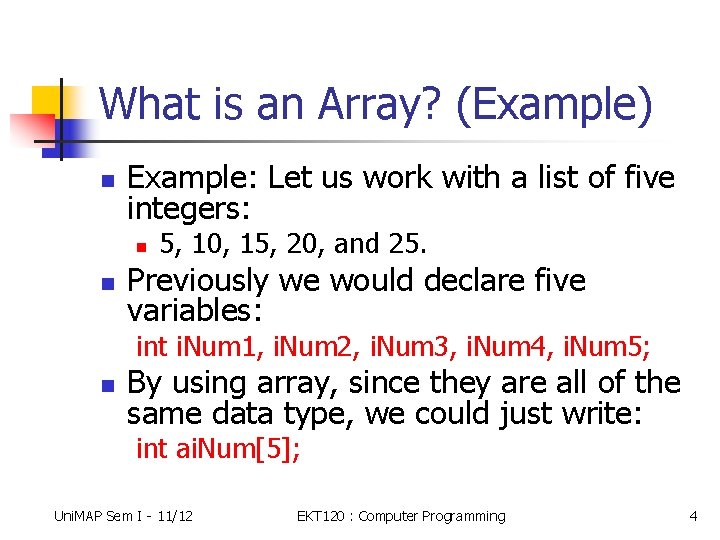
What is an Array? (Example) n Example: Let us work with a list of five integers: n n 5, 10, 15, 20, and 25. Previously we would declare five variables: int i. Num 1, i. Num 2, i. Num 3, i. Num 4, i. Num 5; n By using array, since they are all of the same data type, we could just write: int ai. Num[5]; Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 4
![What is an Array Example ai Num 5 ai Num0 10 ai Num1 15 What is an Array? (Example) ai. Num 5 ai. Num[0] 10 ai. Num[1] 15](https://slidetodoc.com/presentation_image_h2/7279efc1c73464aa9d95fe88956088b0/image-5.jpg)
What is an Array? (Example) ai. Num 5 ai. Num[0] 10 ai. Num[1] 15 20 25 ai. Num[2] n 5 components or elements in this array. n Elements are referred to index. ai. Num[3] n ai. Num[4] Uni. MAP Sem I - 11/12 Element ai. Num[2] has index 2 and value 15. EKT 120 : Computer Programming 5
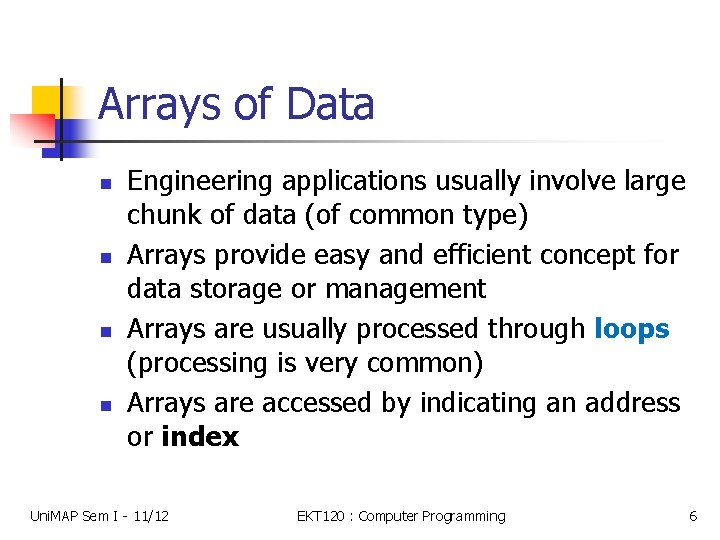
Arrays of Data n n Engineering applications usually involve large chunk of data (of common type) Arrays provide easy and efficient concept for data storage or management Arrays are usually processed through loops (processing is very common) Arrays are accessed by indicating an address or index Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 6
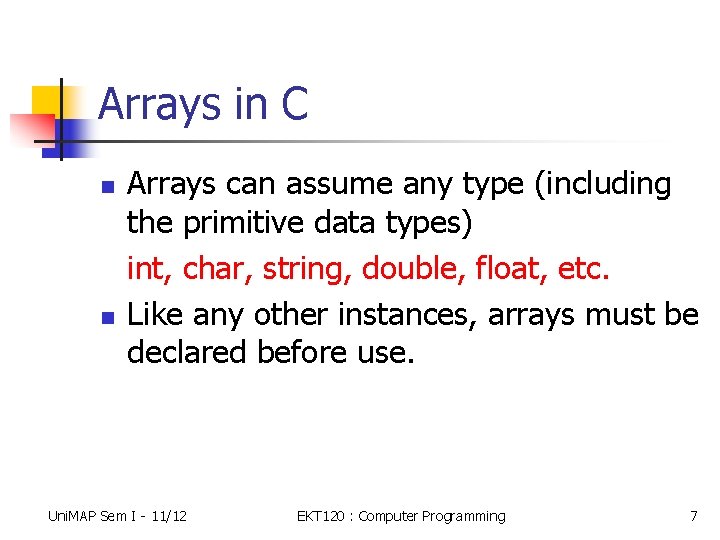
Arrays in C n n Arrays can assume any type (including the primitive data types) int, char, string, double, float, etc. Like any other instances, arrays must be declared before use. Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 7
![Array Declaration Format n n datatype arraynameint value Example n n n int ai Array Declaration Format: n n data_type array_name[int value]; Example: n n n int ai.](https://slidetodoc.com/presentation_image_h2/7279efc1c73464aa9d95fe88956088b0/image-8.jpg)
Array Declaration Format: n n data_type array_name[int value]; Example: n n n int ai. List[5]; const int Max_List_Size = 10; int ai. Hours[Max_List_Size]; const int SIZE = 100; double ad. Amount[SIZE]; const int Max_List_Size = 6; char ac. Alphas[Max_List_Size]; #define N 10 double ad. B[N]; Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 8
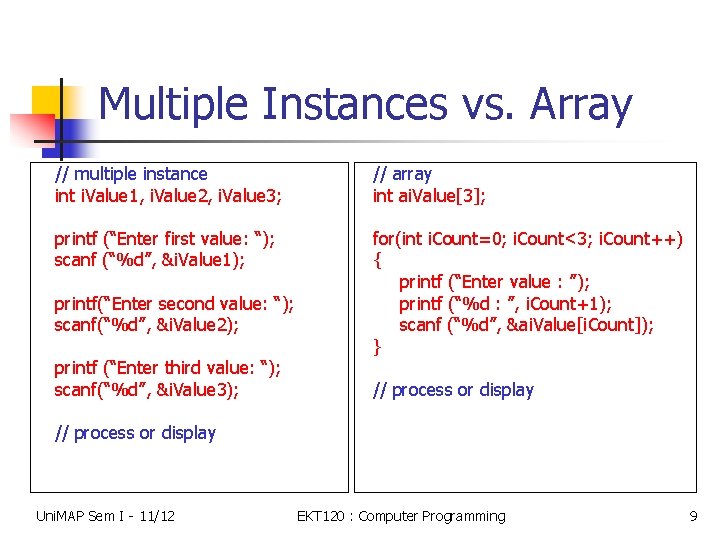
Multiple Instances vs. Array // multiple instance int i. Value 1, i. Value 2, i. Value 3; // array int ai. Value[3]; printf (“Enter first value: “); scanf (“%d”, &i. Value 1); for(int i. Count=0; i. Count<3; i. Count++) { printf (“Enter value : ”); printf (“%d : ”, i. Count+1); scanf (“%d”, &ai. Value[i. Count]); } printf(“Enter second value: “); scanf(“%d”, &i. Value 2); printf (“Enter third value: “); scanf(“%d”, &i. Value 3); // process or display Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 9
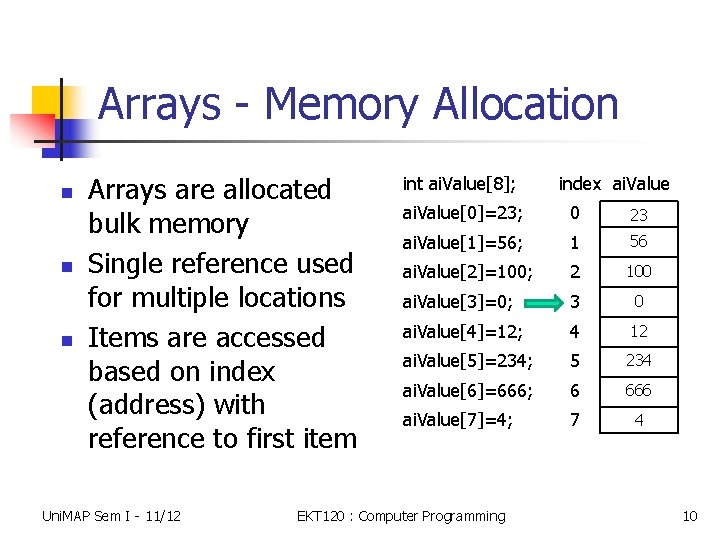
Arrays - Memory Allocation n Arrays are allocated bulk memory Single reference used for multiple locations Items are accessed based on index (address) with reference to first item Uni. MAP Sem I - 11/12 int ai. Value[8]; index ai. Value[0]=23; 0 23 ai. Value[1]=56; 1 56 ai. Value[2]=100; 2 100 ai. Value[3]=0; 3 0 ai. Value[4]=12; 4 12 ai. Value[5]=234; 5 234 ai. Value[6]=666; 6 666 ai. Value[7]=4; 7 4 EKT 120 : Computer Programming 10
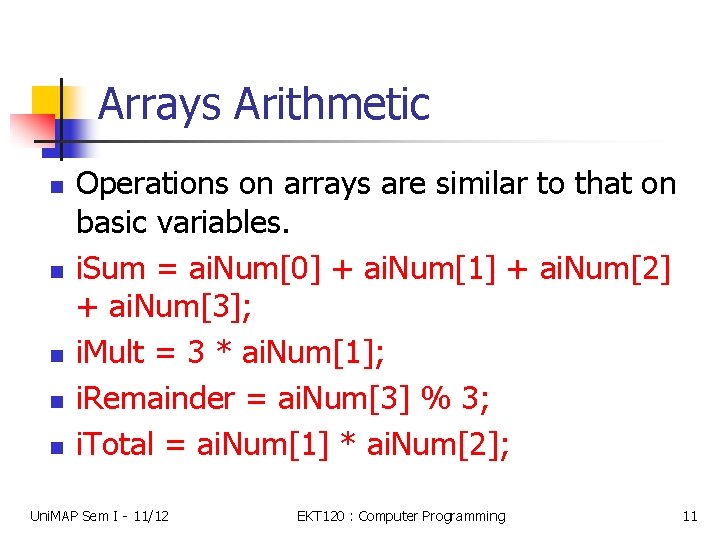
Arrays Arithmetic n n n Operations on arrays are similar to that on basic variables. i. Sum = ai. Num[0] + ai. Num[1] + ai. Num[2] + ai. Num[3]; i. Mult = 3 * ai. Num[1]; i. Remainder = ai. Num[3] % 3; i. Total = ai. Num[1] * ai. Num[2]; Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 11
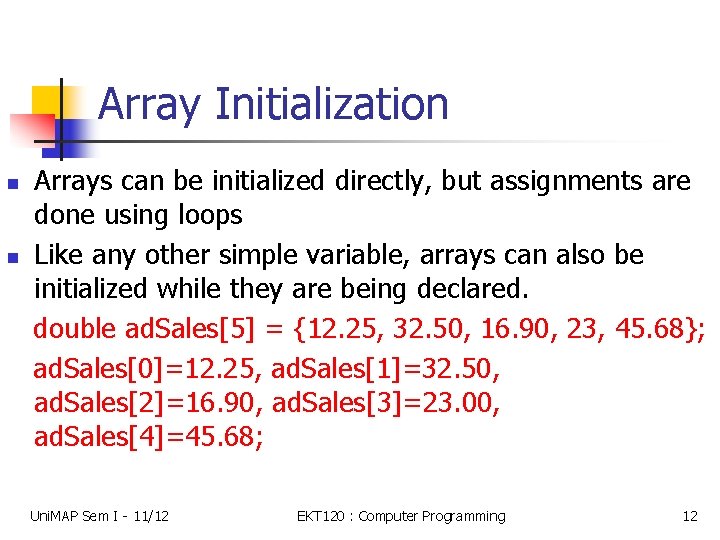
Array Initialization n n Arrays can be initialized directly, but assignments are done using loops Like any other simple variable, arrays can also be initialized while they are being declared. double ad. Sales[5] = {12. 25, 32. 50, 16. 90, 23, 45. 68}; ad. Sales[0]=12. 25, ad. Sales[1]=32. 50, ad. Sales[2]=16. 90, ad. Sales[3]=23. 00, ad. Sales[4]=45. 68; Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 12
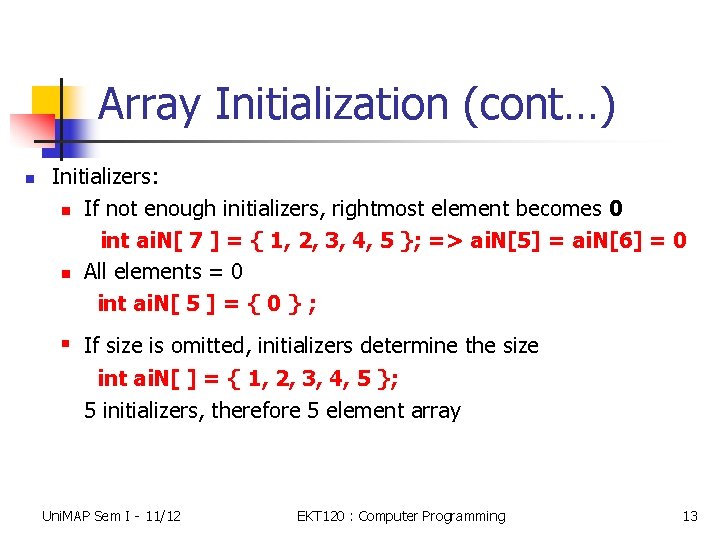
Array Initialization (cont…) n Initializers: n If not enough initializers, rightmost element becomes 0 int ai. N[ 7 ] = { 1, 2, 3, 4, 5 }; => ai. N[5] = ai. N[6] = 0 n All elements = 0 int ai. N[ 5 ] = { 0 } ; ▪ If size is omitted, initializers determine the size int ai. N[ ] = { 1, 2, 3, 4, 5 }; 5 initializers, therefore 5 element array Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 13
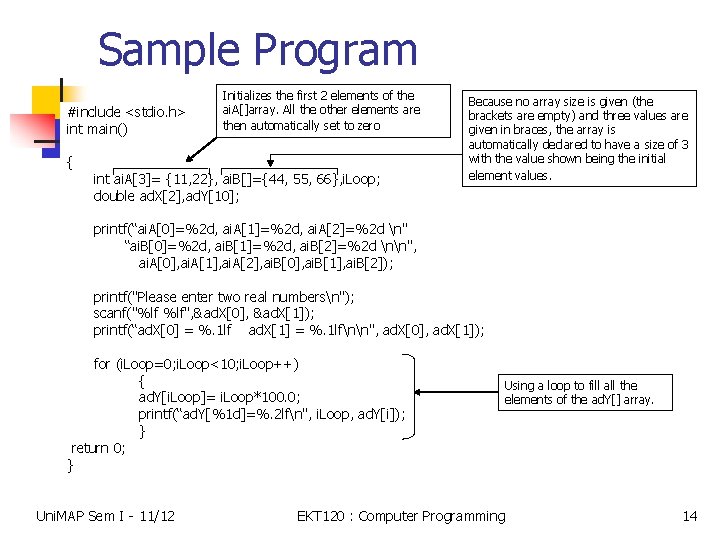
Sample Program #include <stdio. h> int main() { Initializes the first 2 elements of the ai. A[]array. All the other elements are then automatically set to zero int ai. A[3]= {11, 22}, ai. B[]={44, 55, 66}, i. Loop; double ad. X[2], ad. Y[10]; Because no array size is given (the brackets are empty) and three values are given in braces, the array is automatically declared to have a size of 3 with the value shown being the initial element values. printf(“ai. A[0]=%2 d, ai. A[1]=%2 d, ai. A[2]=%2 d n" “ai. B[0]=%2 d, ai. B[1]=%2 d, ai. B[2]=%2 d nn", ai. A[0], ai. A[1], ai. A[2], ai. B[0], ai. B[1], ai. B[2]); printf("Please enter two real numbersn"); scanf("%lf %lf", &ad. X[0], &ad. X[1]); printf(“ad. X[0] = %. 1 lf ad. X[1] = %. 1 lfnn", ad. X[0], ad. X[1]); for (i. Loop=0; i. Loop<10; i. Loop++) { ad. Y[i. Loop]= i. Loop*100. 0; printf(“ad. Y[%1 d]=%. 2 lfn", i. Loop, ad. Y[i]); } return 0; } Uni. MAP Sem I - 11/12 Using a loop to fill all the elements of the ad. Y[] array. EKT 120 : Computer Programming 14
![Sample Program n Output ai A011 ai A122 ai A2 0 ai B044 ai Sample Program n Output: ai. A[0]=11, ai. A[1]=22, ai. A[2]= 0 ai. B[0]=44, ai.](https://slidetodoc.com/presentation_image_h2/7279efc1c73464aa9d95fe88956088b0/image-15.jpg)
Sample Program n Output: ai. A[0]=11, ai. A[1]=22, ai. A[2]= 0 ai. B[0]=44, ai. B[1]=55, ai. B[2]=66 Please enter two real numbers 77. 0 88. 0 ad. X[0] = 77. 0 ad. X[1] = 88. 0 ad. Y[0]=0. 00 ad. Y[1]=100. 00 ad. Y[2]=200. 00 ad. Y[3]=300. 00 ad. Y[4]=400. 00 ad. Y[5]=500. 00 ad. Y[6]=600. 00 ad. Y[7]=700. 00 ad. Y[8]=800. 00 ad. Y[9]=900. 00 Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 15
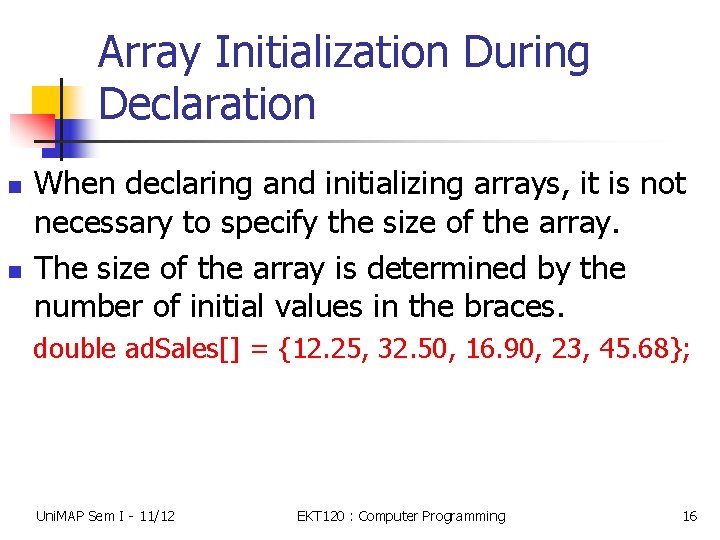
Array Initialization During Declaration n n When declaring and initializing arrays, it is not necessary to specify the size of the array. The size of the array is determined by the number of initial values in the braces. double ad. Sales[] = {12. 25, 32. 50, 16. 90, 23, 45. 68}; Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 16
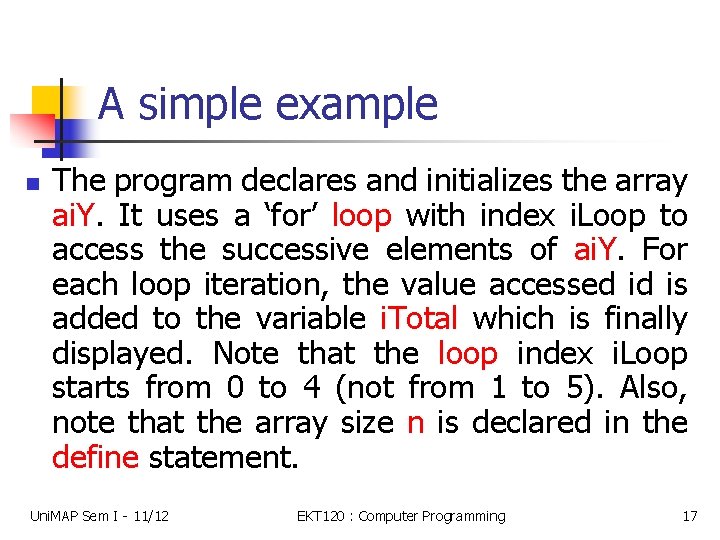
A simple example n The program declares and initializes the array ai. Y. It uses a ‘for’ loop with index i. Loop to access the successive elements of ai. Y. For each loop iteration, the value accessed id is added to the variable i. Total which is finally displayed. Note that the loop index i. Loop starts from 0 to 4 (not from 1 to 5). Also, note that the array size n is declared in the define statement. Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 17
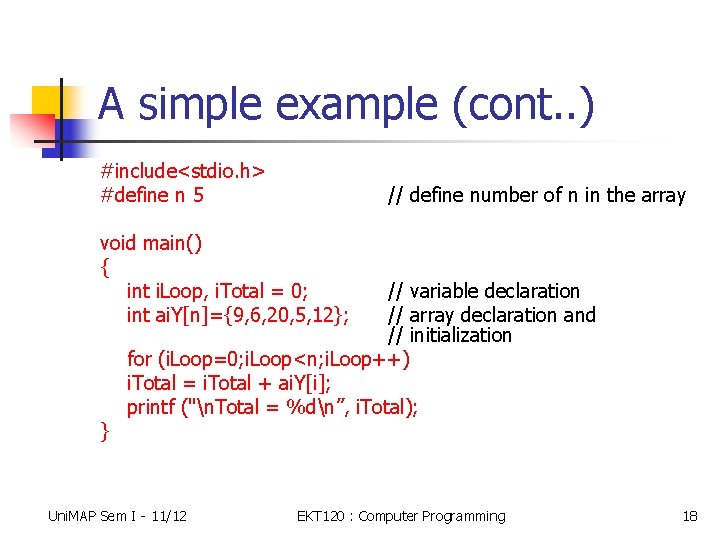
A simple example (cont. . ) #include<stdio. h> #define n 5 // define number of n in the array void main() { int i. Loop, i. Total = 0; int ai. Y[n]={9, 6, 20, 5, 12}; } // variable declaration // array declaration and // initialization for (i. Loop=0; i. Loop<n; i. Loop++) i. Total = i. Total + ai. Y[i]; printf ("n. Total = %dn”, i. Total); Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 18
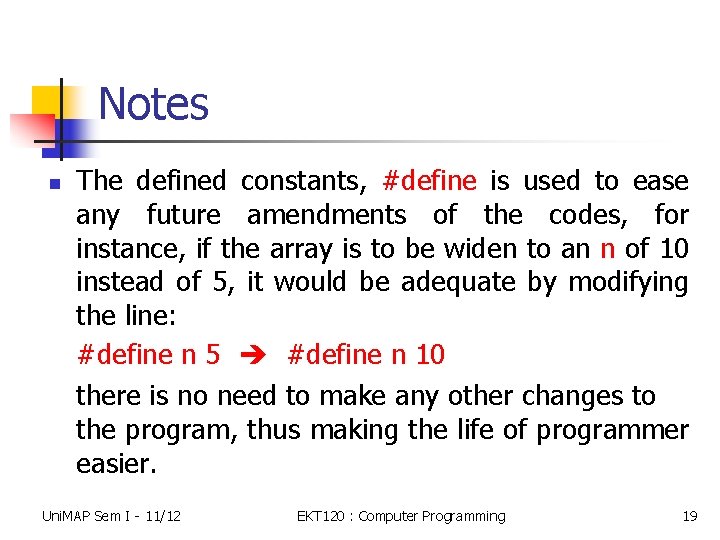
Notes n The defined constants, #define is used to ease any future amendments of the codes, for instance, if the array is to be widen to an n of 10 instead of 5, it would be adequate by modifying the line: #define n 5 #define n 10 there is no need to make any other changes to the program, thus making the life of programmer easier. Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 19
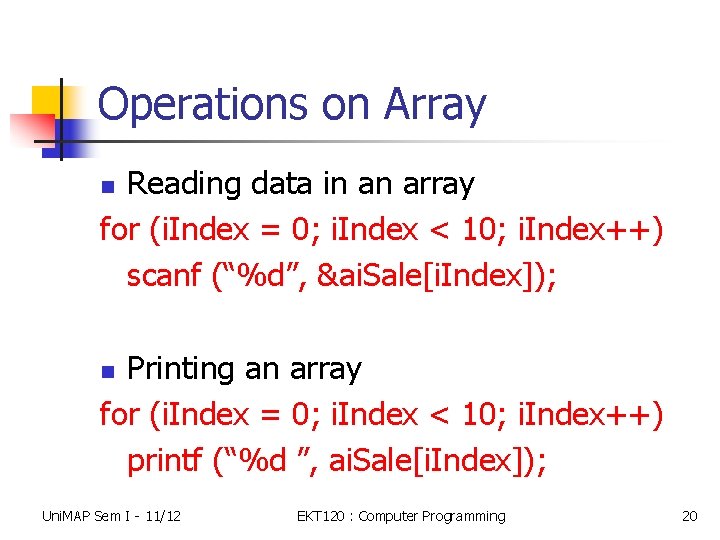
Operations on Array Reading data in an array for (i. Index = 0; i. Index < 10; i. Index++) scanf (“%d”, &ai. Sale[i. Index]); n Printing an array for (i. Index = 0; i. Index < 10; i. Index++) printf (“%d ”, ai. Sale[i. Index]); n Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 20
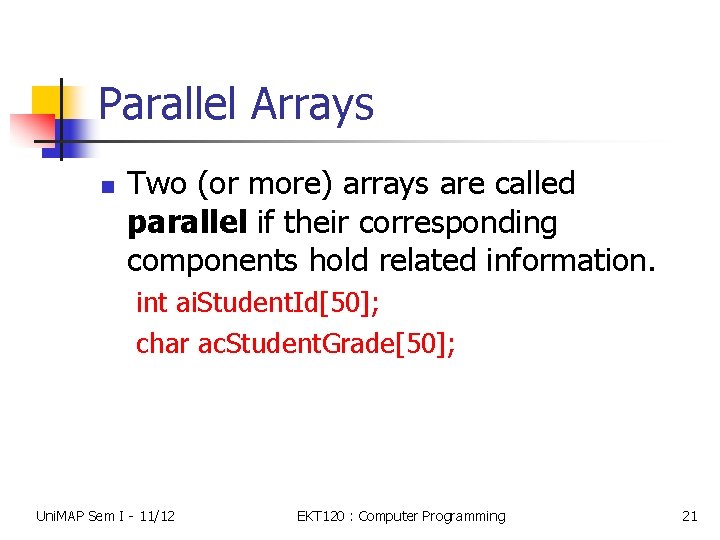
Parallel Arrays n Two (or more) arrays are called parallel if their corresponding components hold related information. int ai. Student. Id[50]; char ac. Student. Grade[50]; Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 21
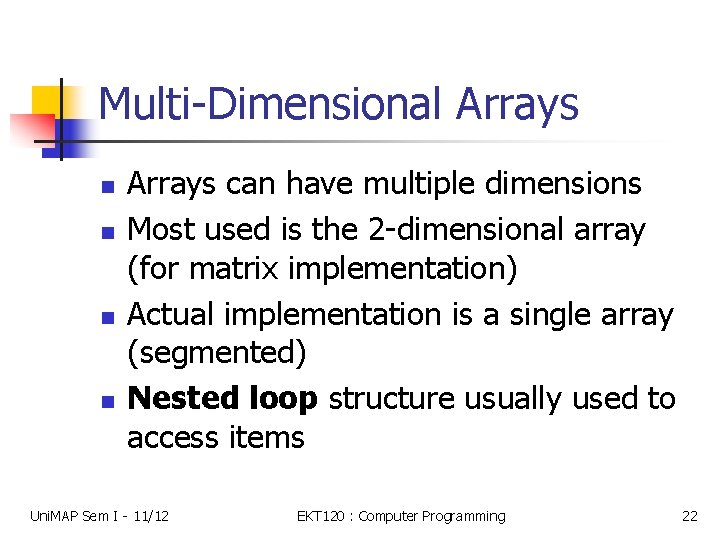
Multi-Dimensional Arrays n n Arrays can have multiple dimensions Most used is the 2 -dimensional array (for matrix implementation) Actual implementation is a single array (segmented) Nested loop structure usually used to access items Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 22
![2 Dimensional Array Example int ai Value42 ai Value215 index ai Value Row 0 2 -Dimensional Array (Example) int ai. Value[4][2]; ai. Value[2][1]=5; index ai. Value Row 0](https://slidetodoc.com/presentation_image_h2/7279efc1c73464aa9d95fe88956088b0/image-23.jpg)
2 -Dimensional Array (Example) int ai. Value[4][2]; ai. Value[2][1]=5; index ai. Value Row 0 Column 0 1 0 Row 3 1 2 3 4 1 2 Row 1 0 5 5 5 6 7 Address Resolution = Row*(Max. Col) + Col Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 23
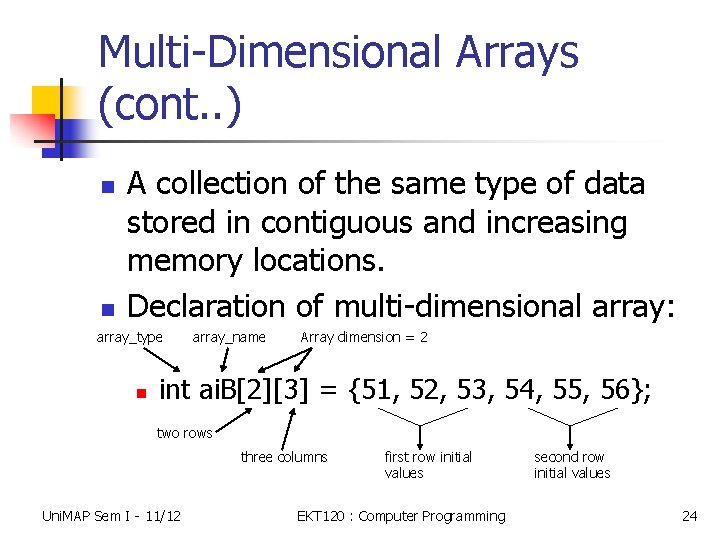
Multi-Dimensional Arrays (cont. . ) n n A collection of the same type of data stored in contiguous and increasing memory locations. Declaration of multi-dimensional array: array_type n array_name Array dimension = 2 int ai. B[2][3] = {51, 52, 53, 54, 55, 56}; two rows three columns Uni. MAP Sem I - 11/12 first row initial values EKT 120 : Computer Programming second row initial values 24
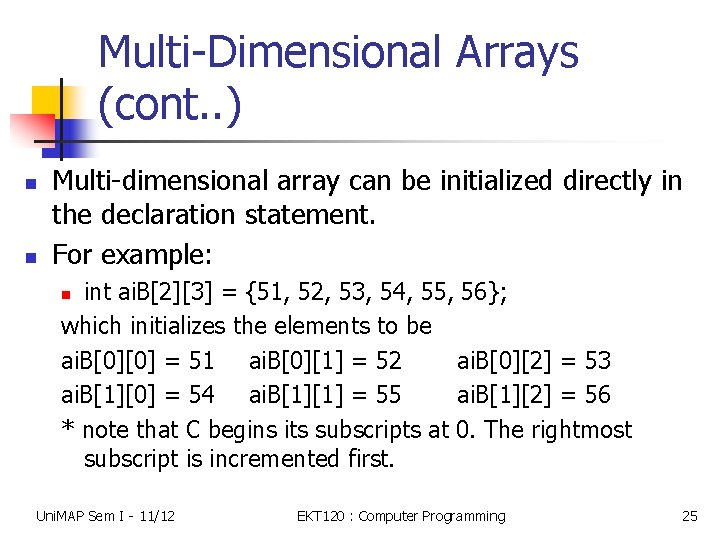
Multi-Dimensional Arrays (cont. . ) n n Multi-dimensional array can be initialized directly in the declaration statement. For example: int ai. B[2][3] = {51, 52, 53, 54, 55, 56}; which initializes the elements to be ai. B[0][0] = 51 ai. B[0][1] = 52 ai. B[0][2] = 53 ai. B[1][0] = 54 ai. B[1][1] = 55 ai. B[1][2] = 56 * note that C begins its subscripts at 0. The rightmost subscript is incremented first. n Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 25
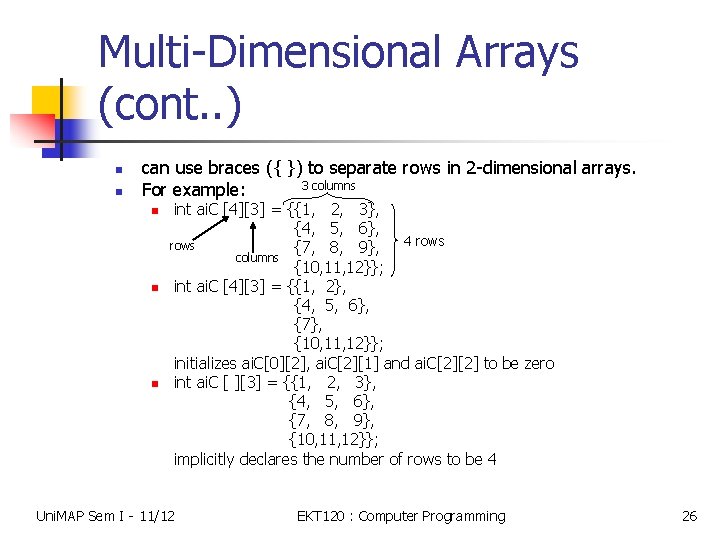
Multi-Dimensional Arrays (cont. . ) n n can use braces ({ }) to separate rows in 2 -dimensional arrays. 3 columns For example: n n n int ai. C [4][3] = {{1, 2, 3}, {4, 5, 6}, rows {7, 8, 9}, 4 rows columns {10, 11, 12}}; int ai. C [4][3] = {{1, 2}, {4, 5, 6}, {7}, {10, 11, 12}}; initializes ai. C[0][2], ai. C[2][1] and ai. C[2][2] to be zero int ai. C [ ][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}, {10, 11, 12}}; implicitly declares the number of rows to be 4 Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 26
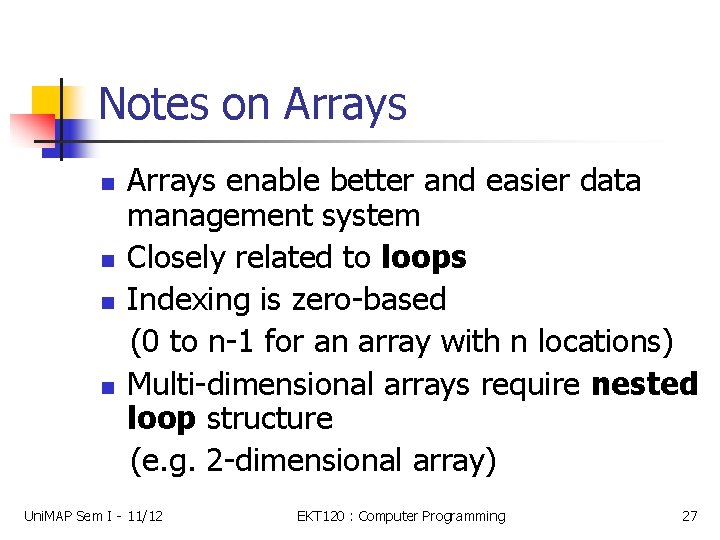
Notes on Arrays n n Arrays enable better and easier data management system Closely related to loops Indexing is zero-based (0 to n-1 for an array with n locations) Multi-dimensional arrays require nested loop structure (e. g. 2 -dimensional array) Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 27
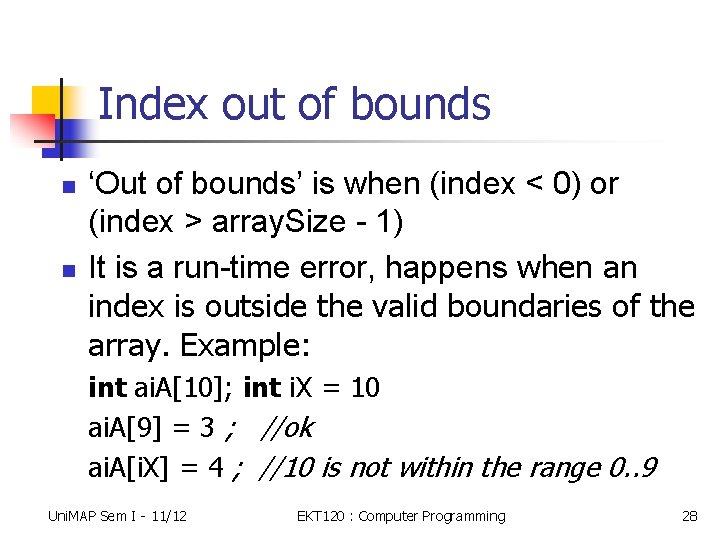
Index out of bounds n n ‘Out of bounds’ is when (index < 0) or (index > array. Size - 1) It is a run-time error, happens when an index is outside the valid boundaries of the array. Example: int ai. A[10]; int i. X = 10 ai. A[9] = 3 ; //ok ai. A[i. X] = 4 ; //10 is not within the range 0. . 9 Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 28
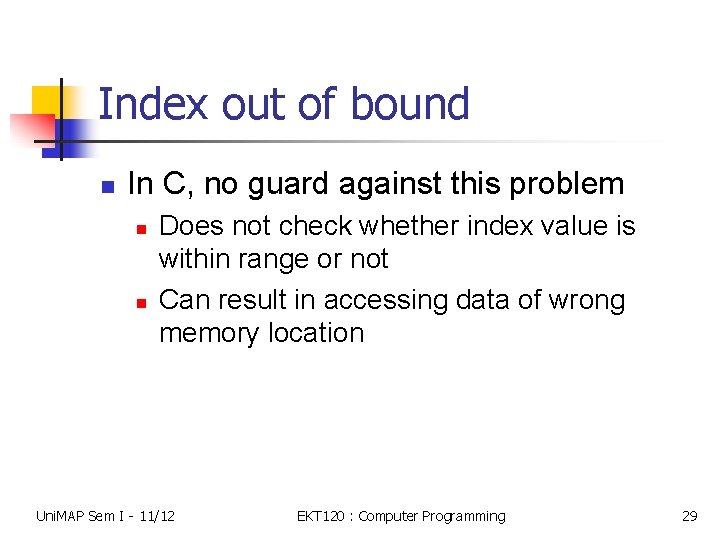
Index out of bound n In C, no guard against this problem n n Does not check whether index value is within range or not Can result in accessing data of wrong memory location Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 29
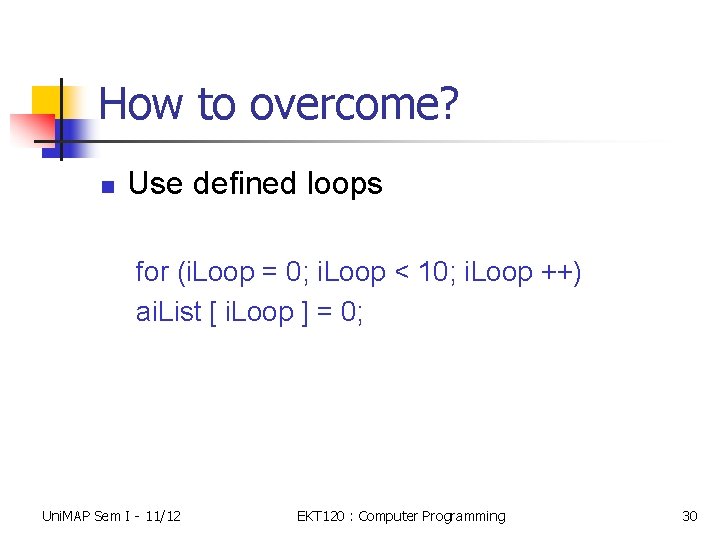
How to overcome? n Use defined loops for (i. Loop = 0; i. Loop < 10; i. Loop ++) ai. List [ i. Loop ] = 0; Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 30
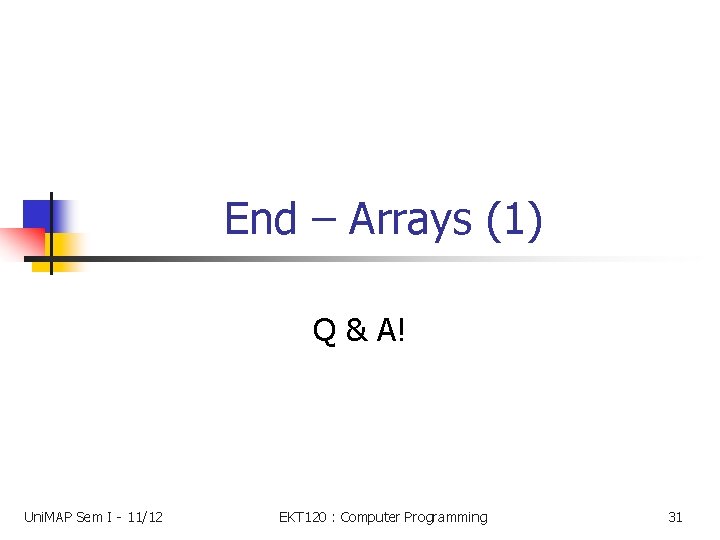
End – Arrays (1) Q & A! Uni. MAP Sem I - 11/12 EKT 120 : Computer Programming 31