Lecture 5 More Recursion EXAMPLES OF RECURSION Towers
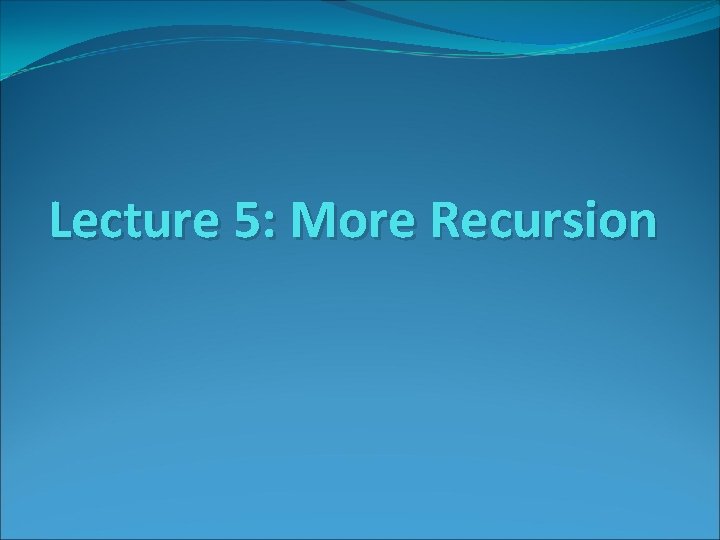
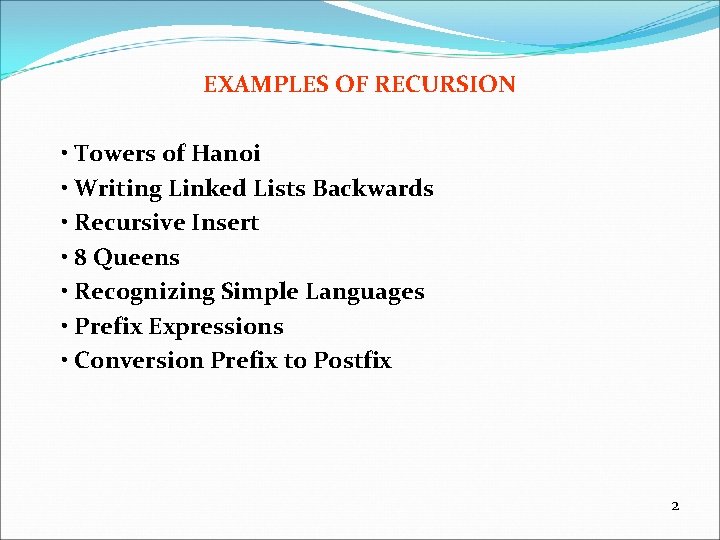
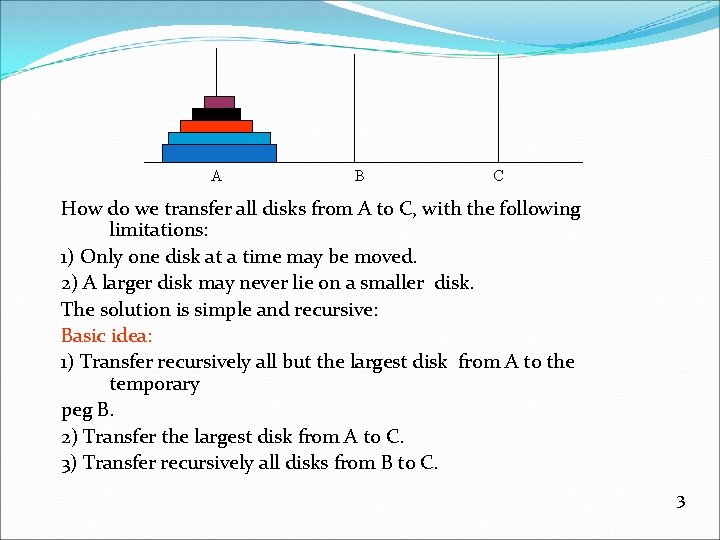
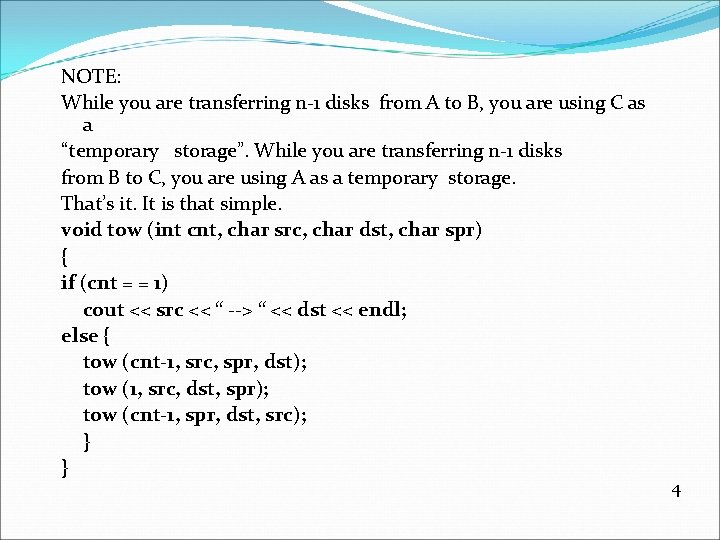
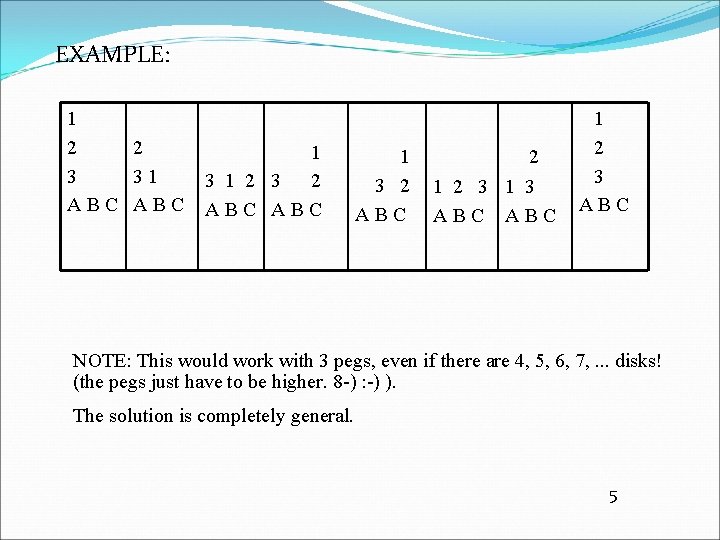
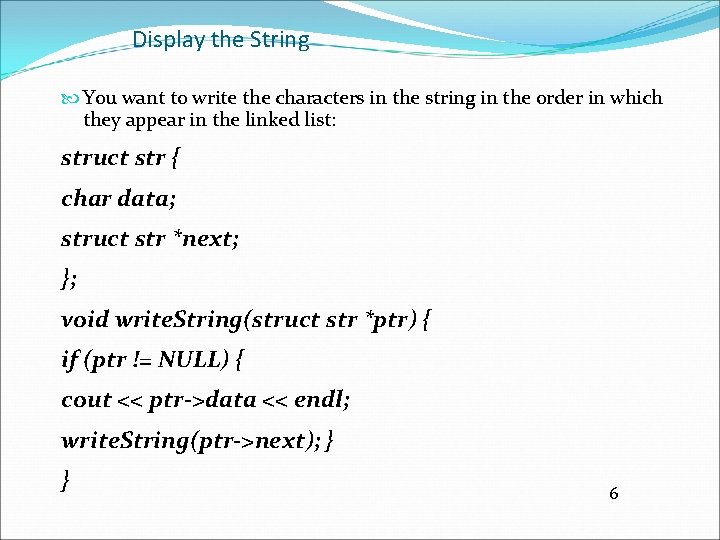
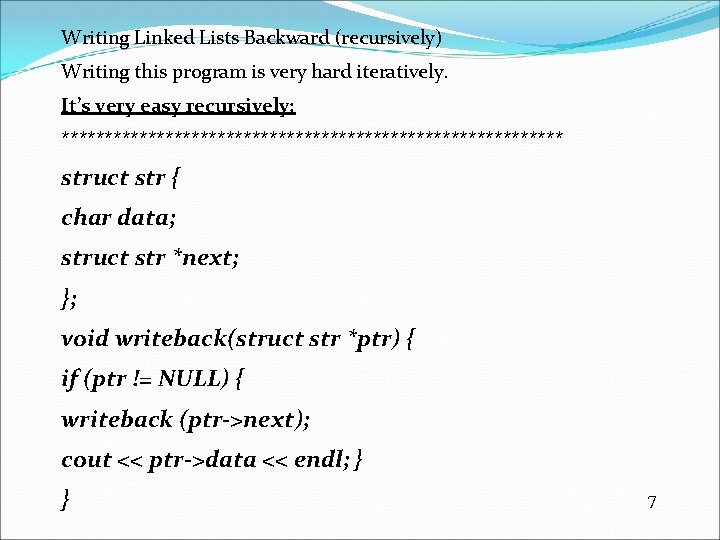
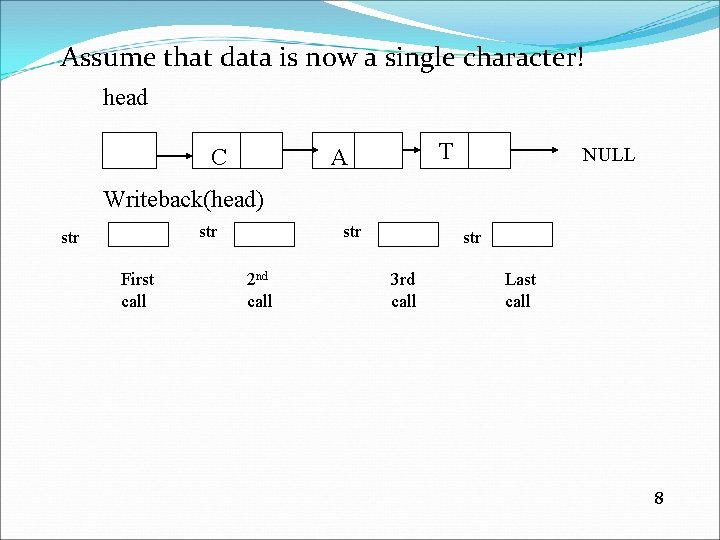
![Strip a way first character write. Backward 2(char [] s) { cout << “Enter Strip a way first character write. Backward 2(char [] s) { cout << “Enter](https://slidetodoc.com/presentation_image_h2/9cedad0492457c726ebebbb8463dfe8b/image-9.jpg)
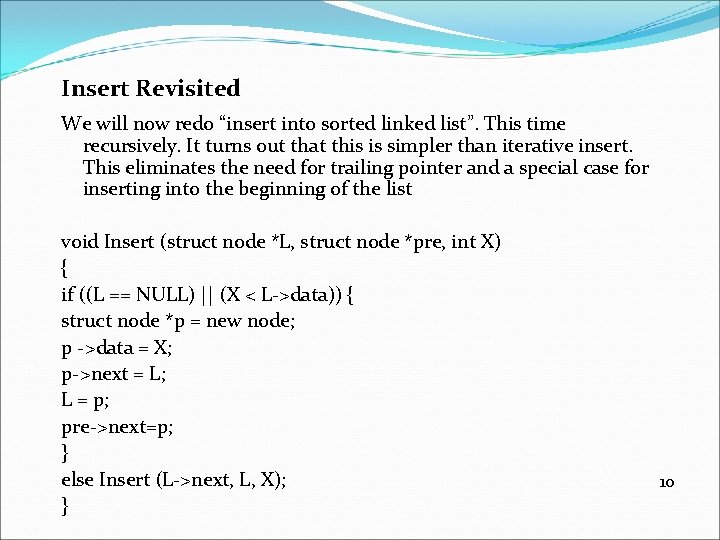
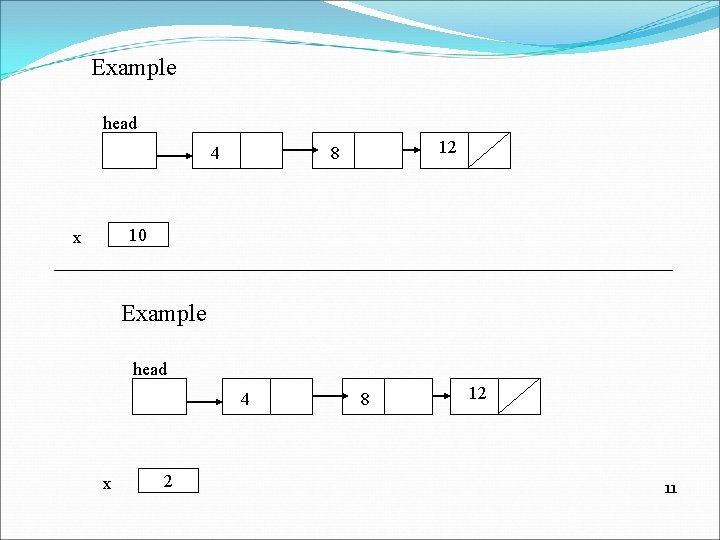
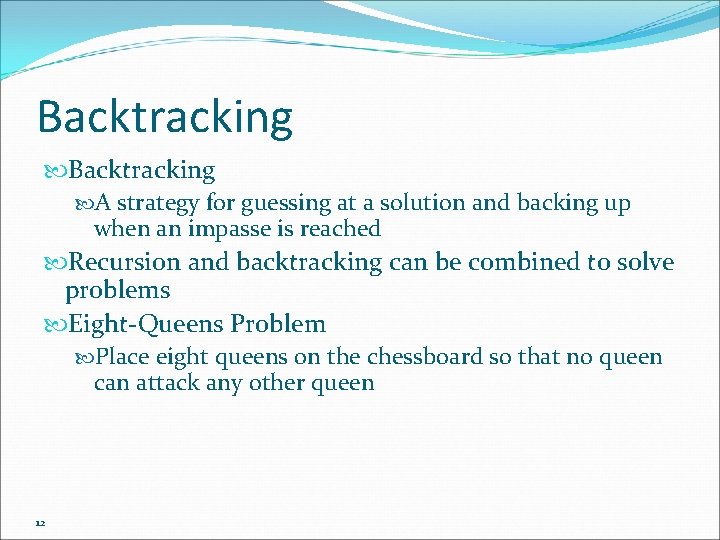
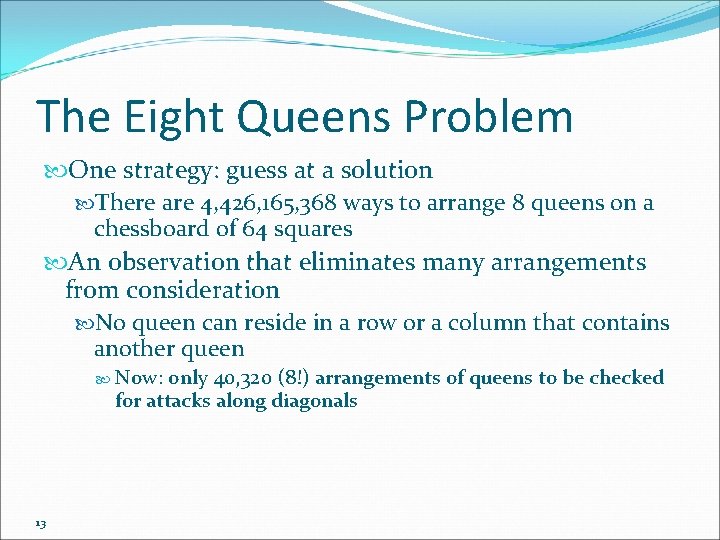
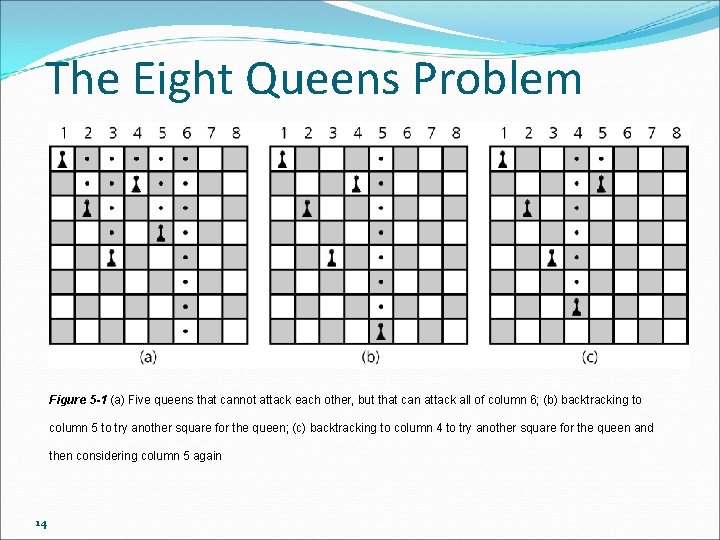
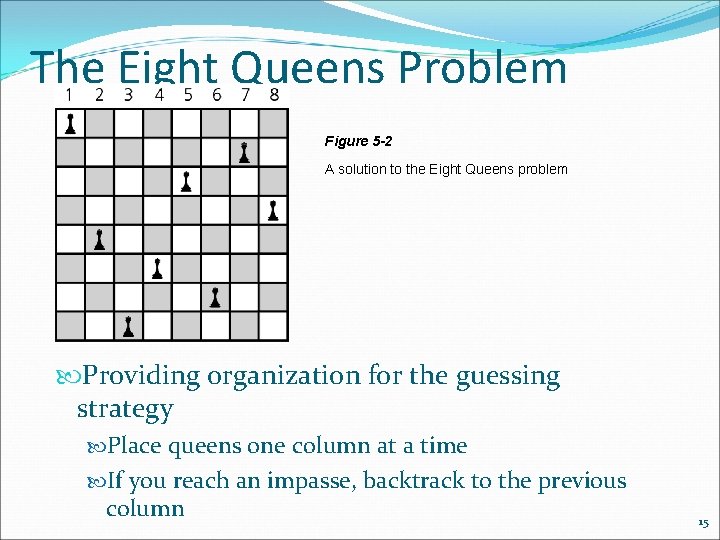
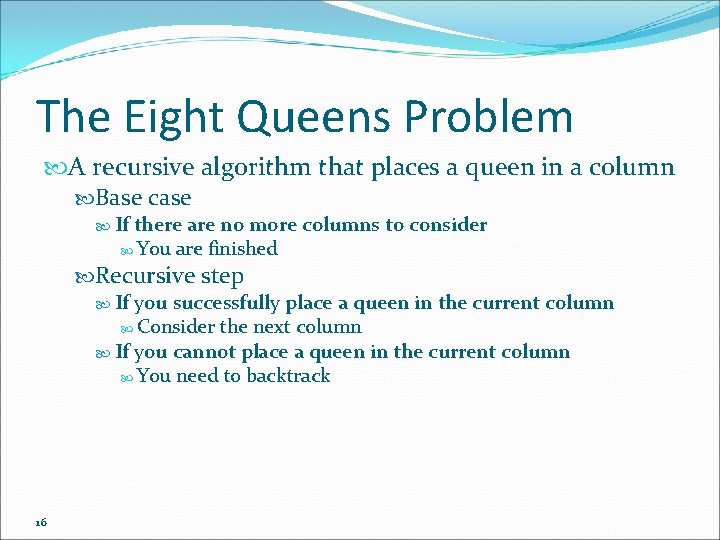
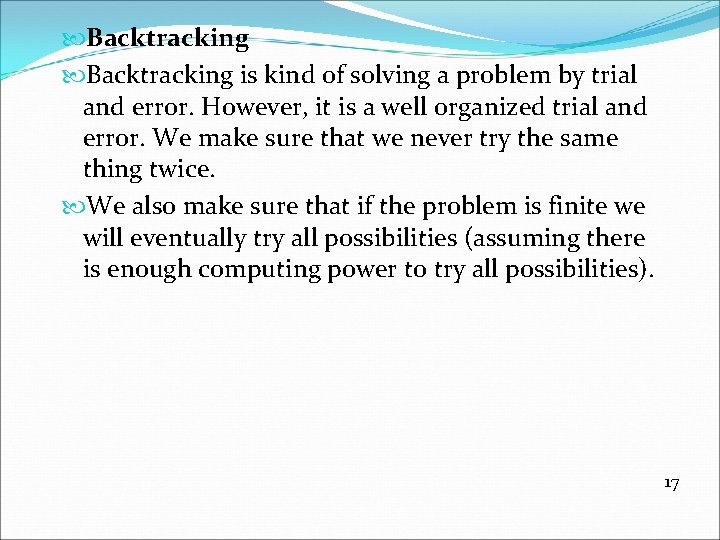
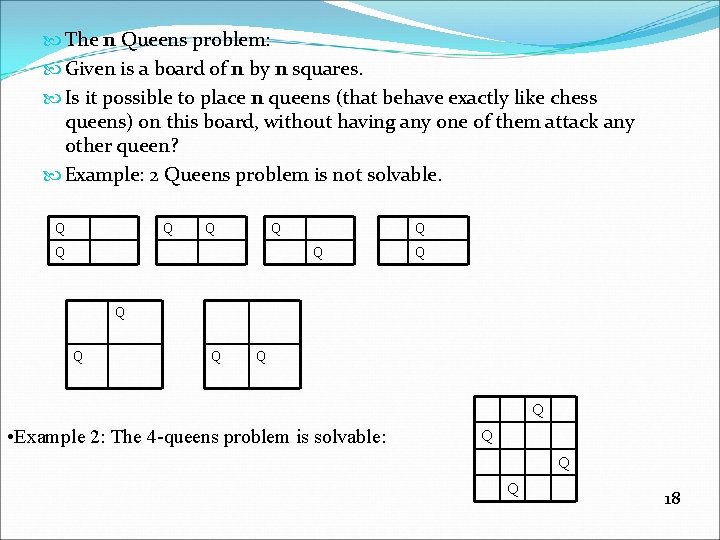
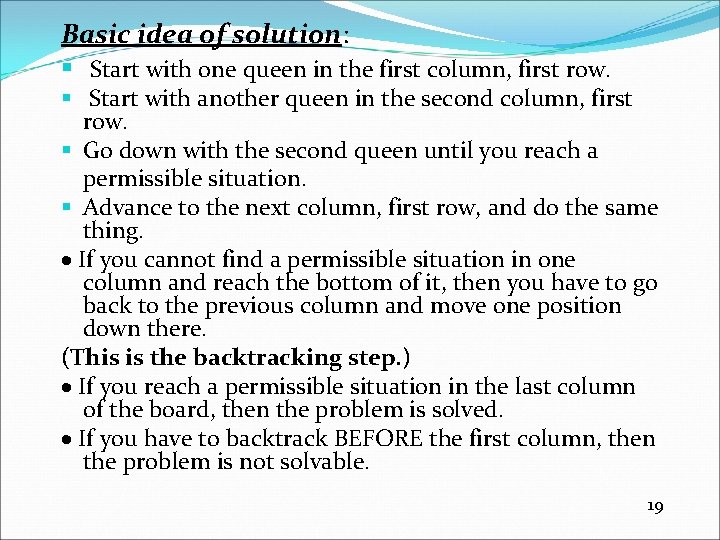
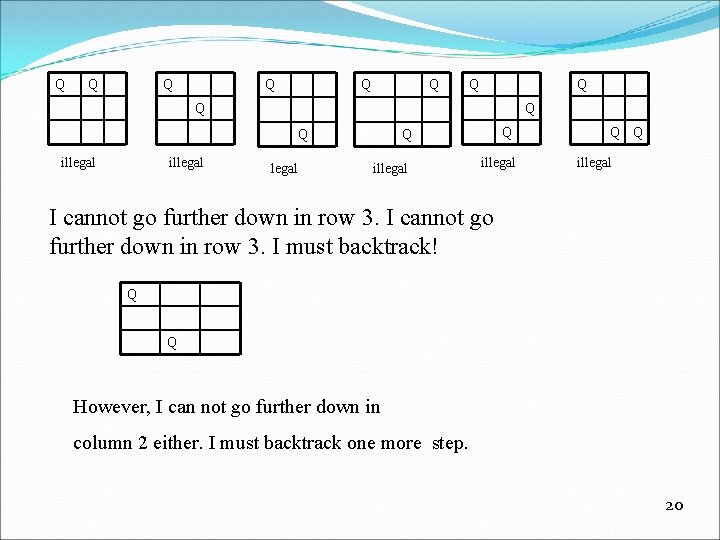
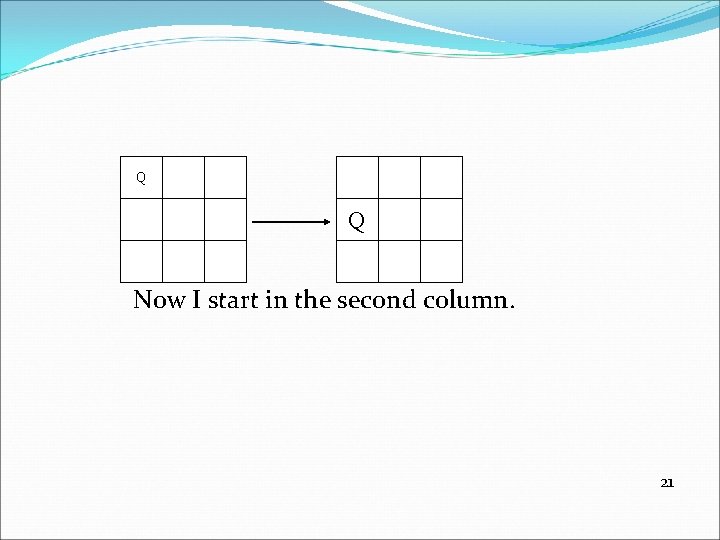
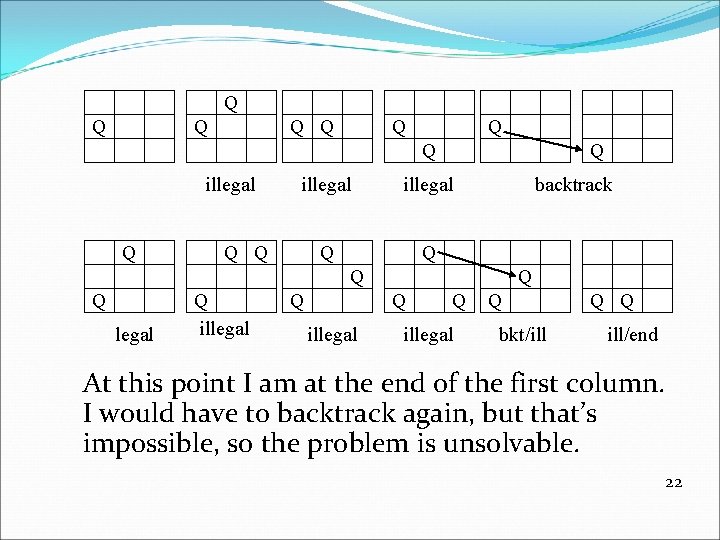
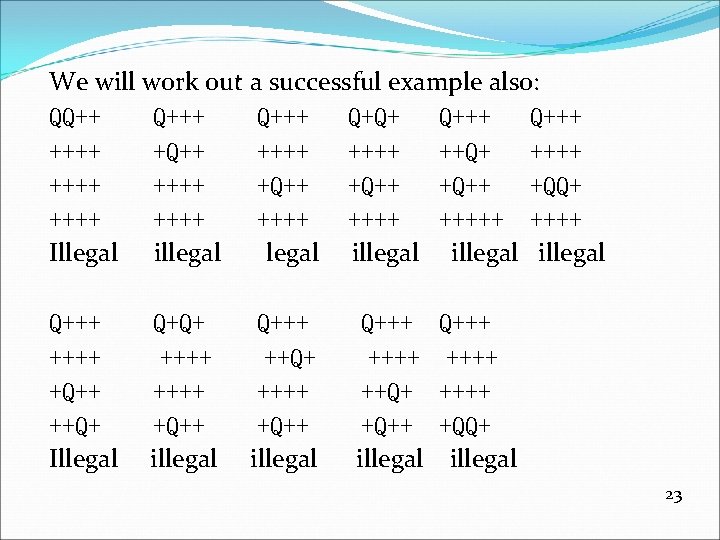
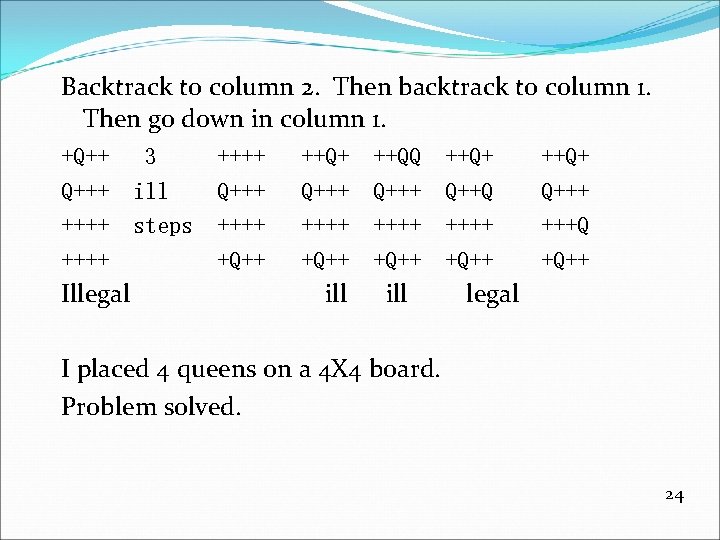
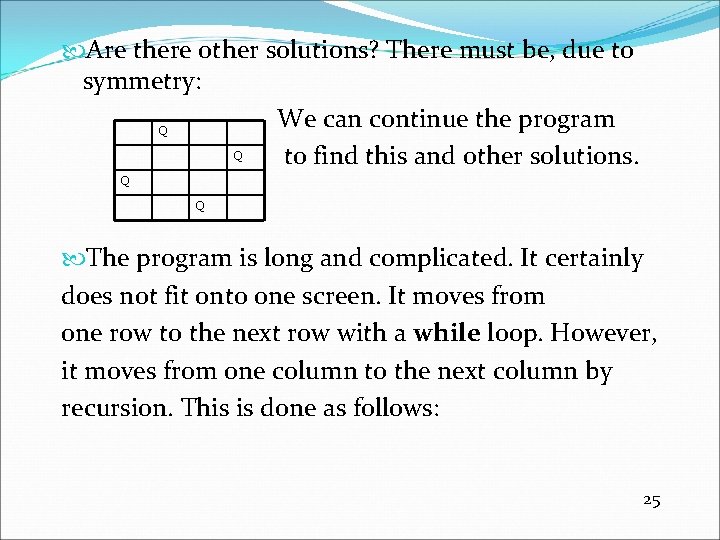
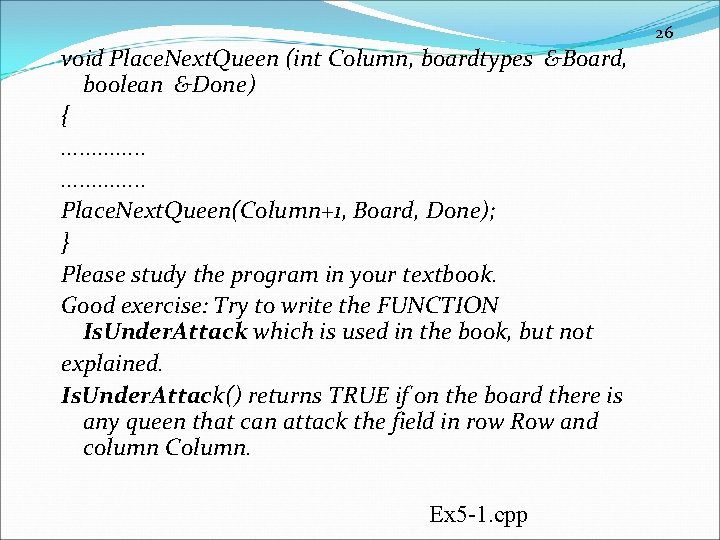
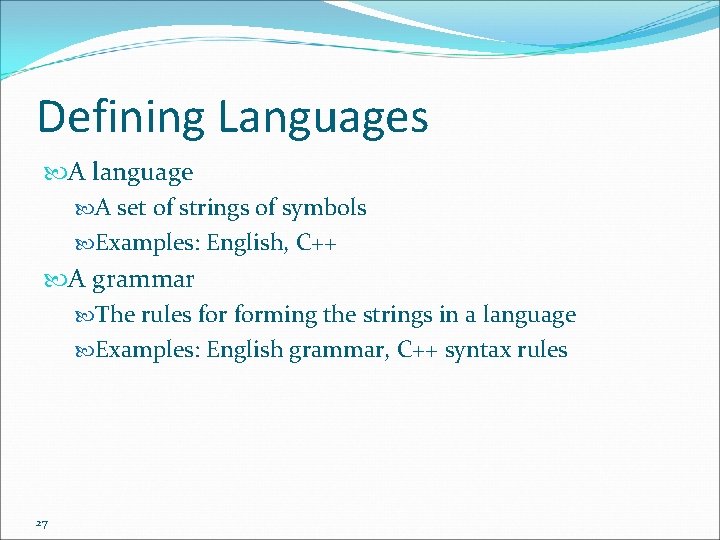
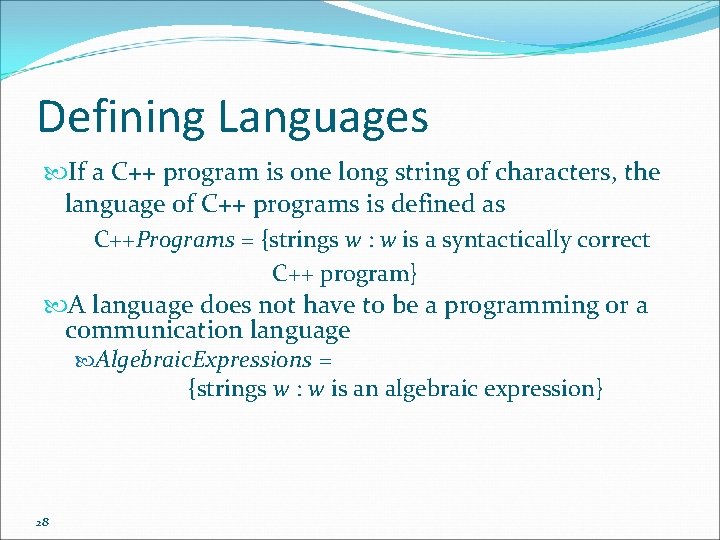
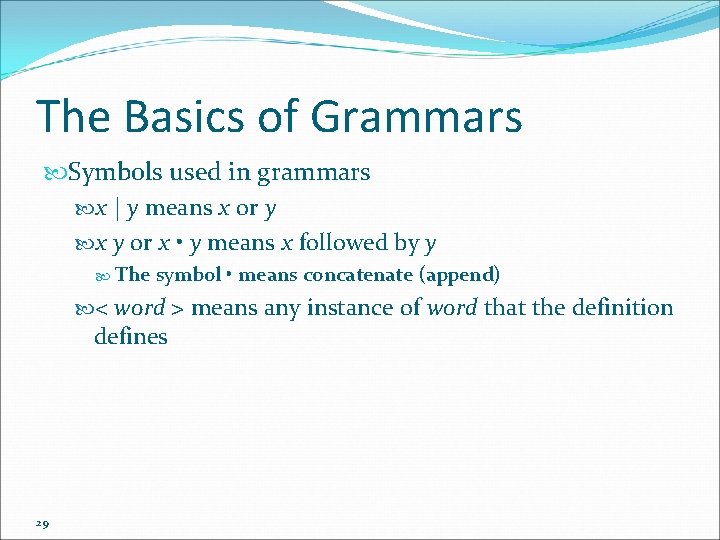
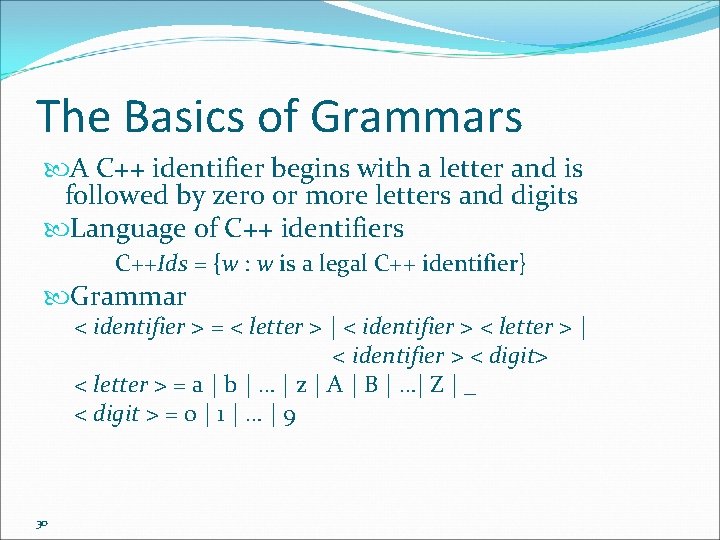
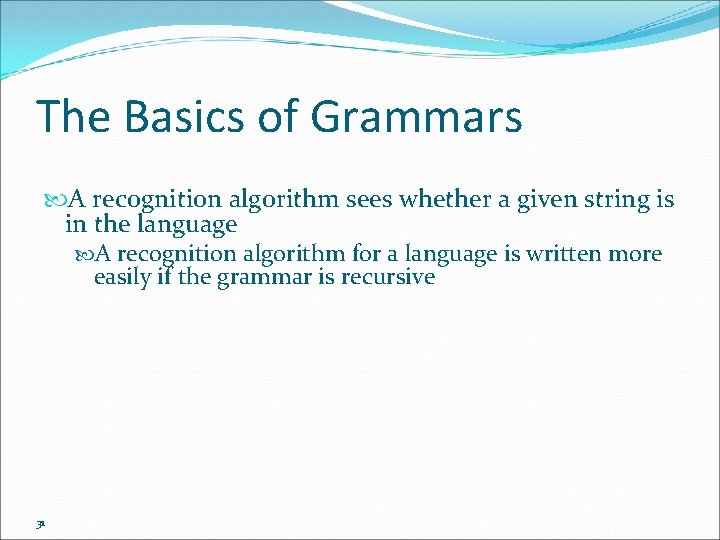
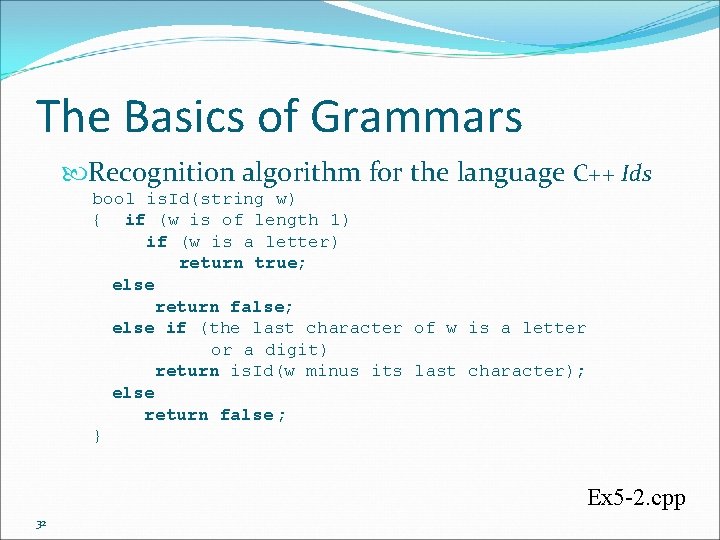
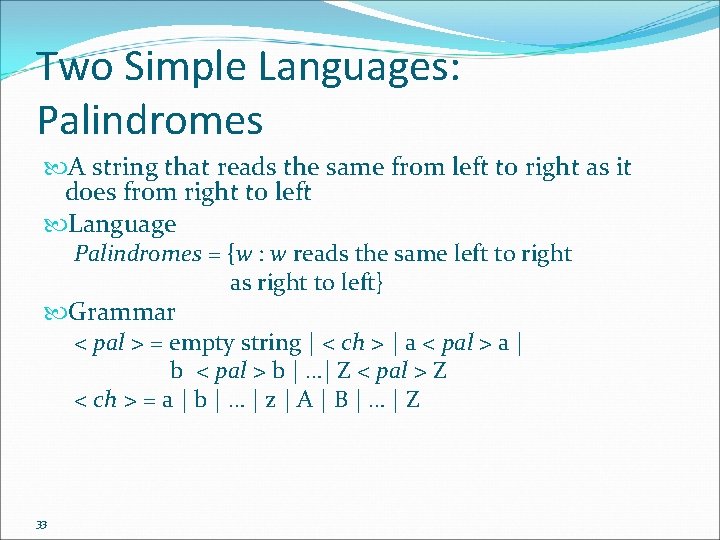
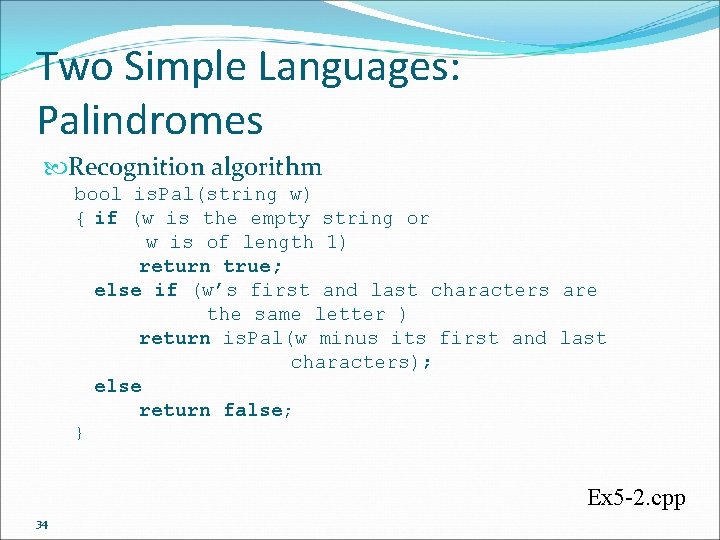
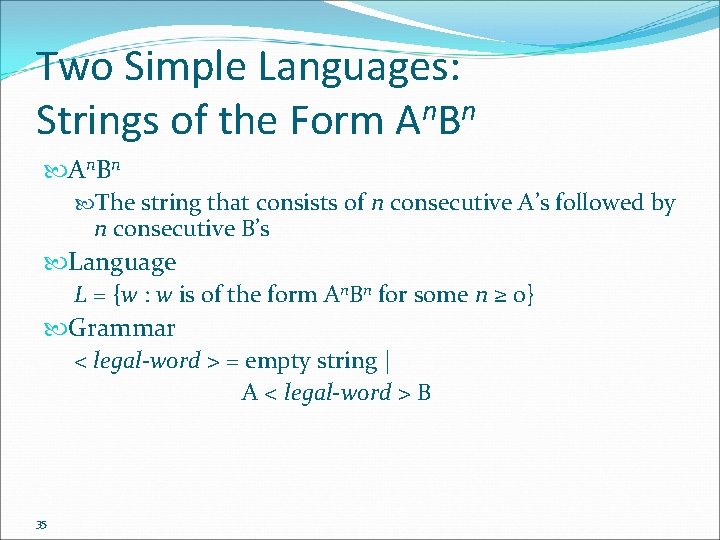
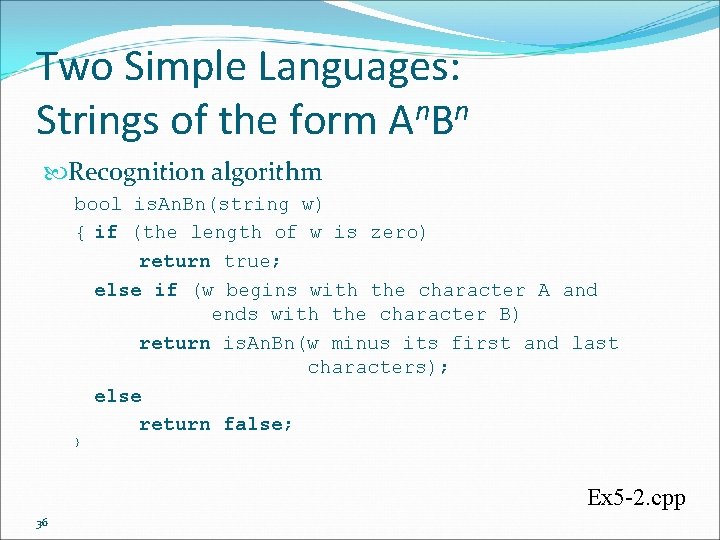
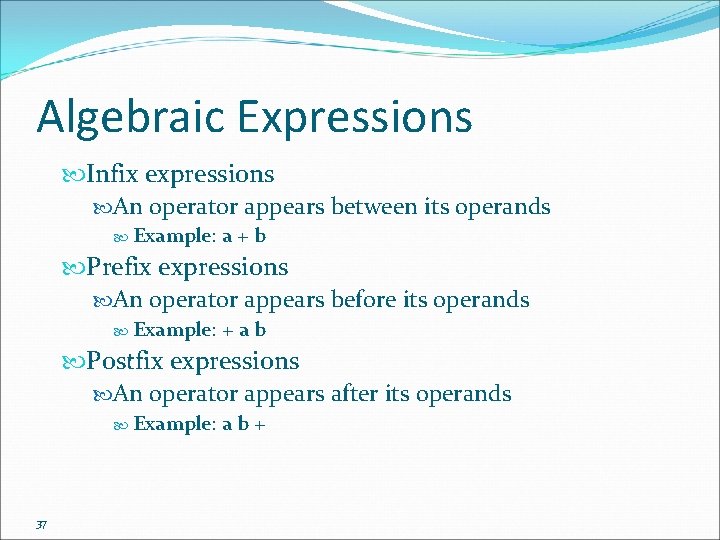
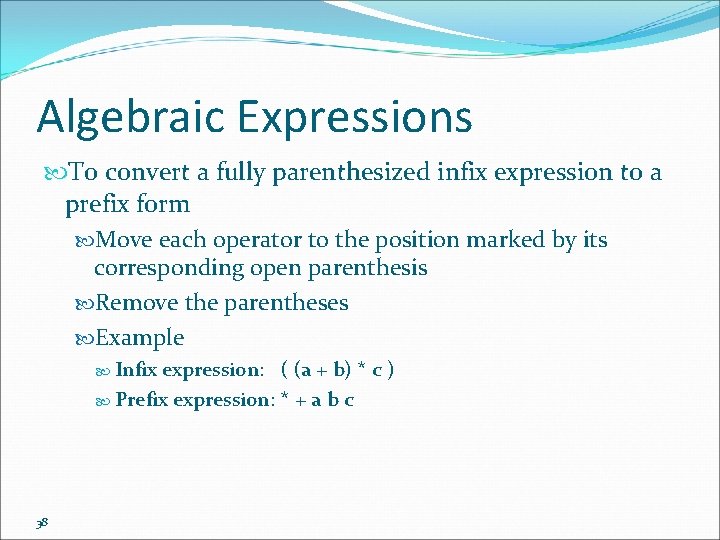
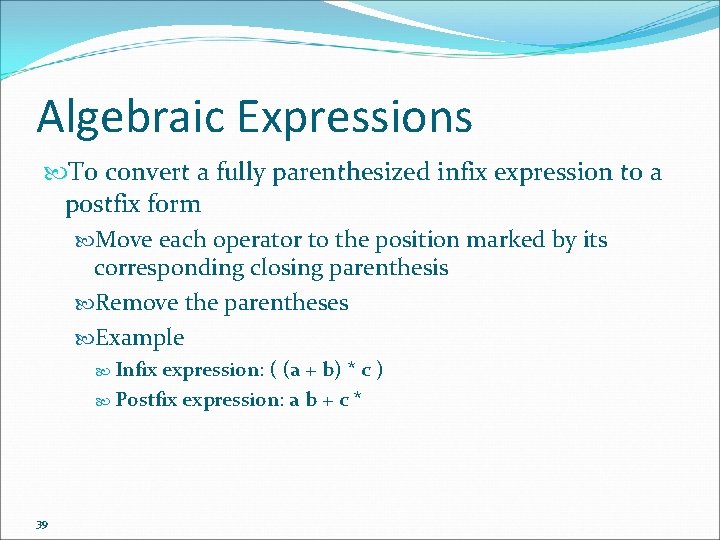
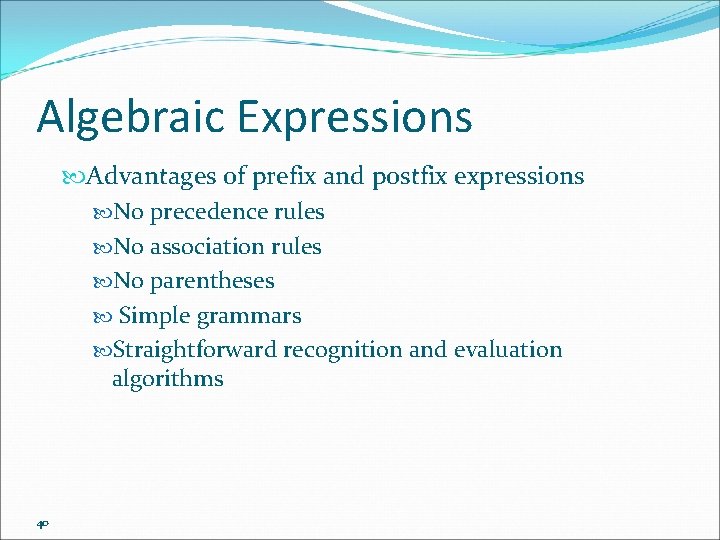
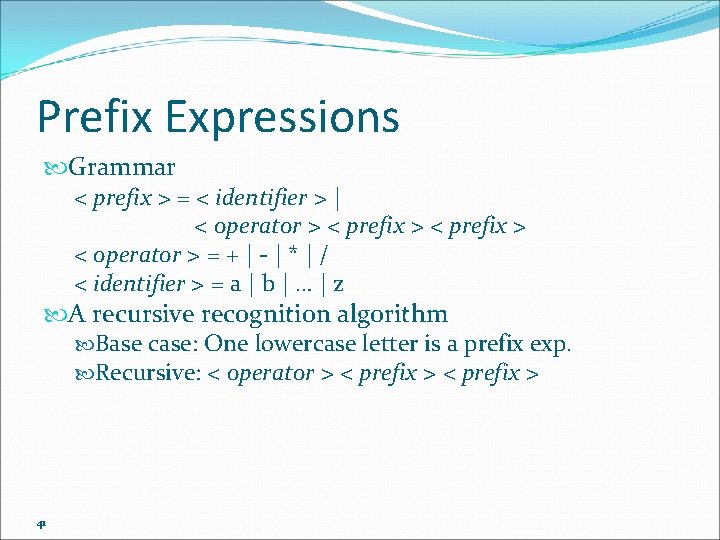
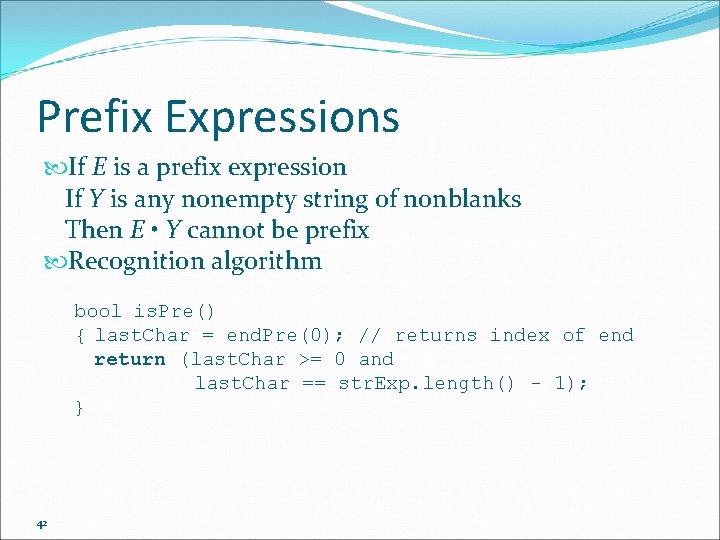
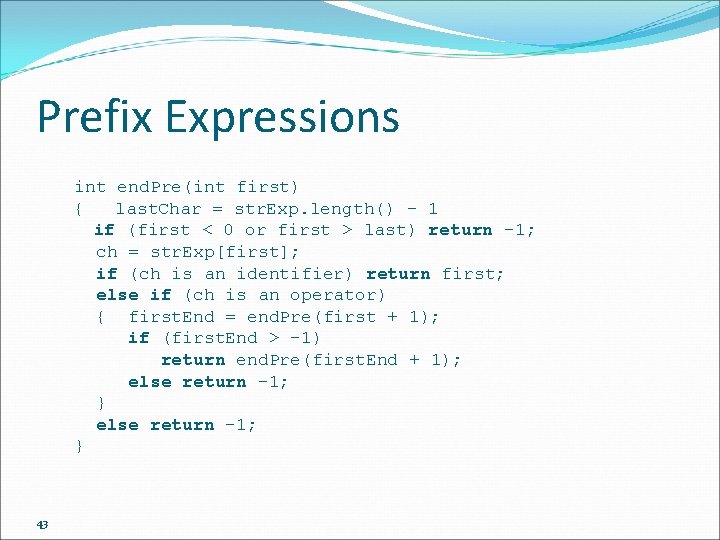
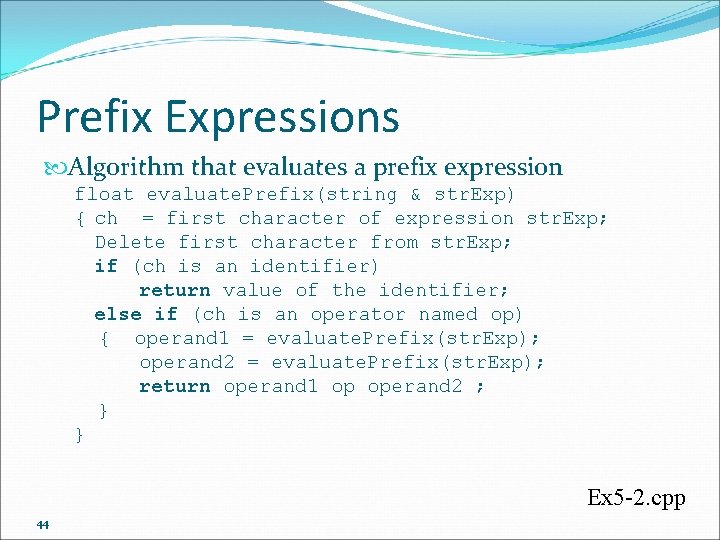
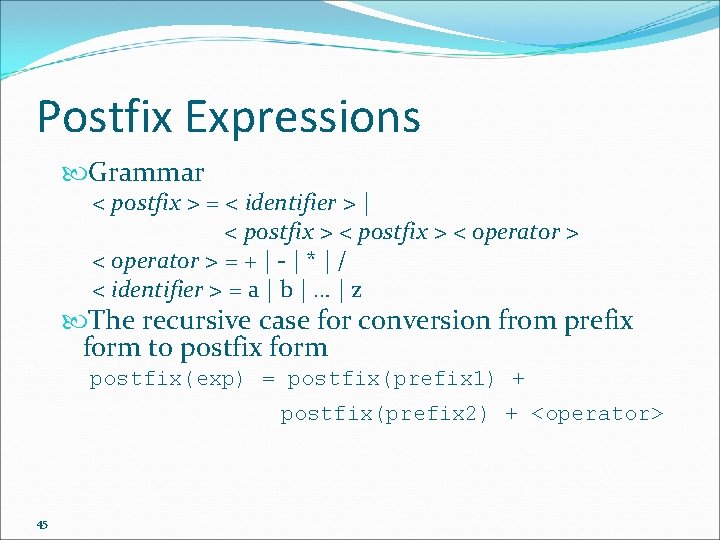
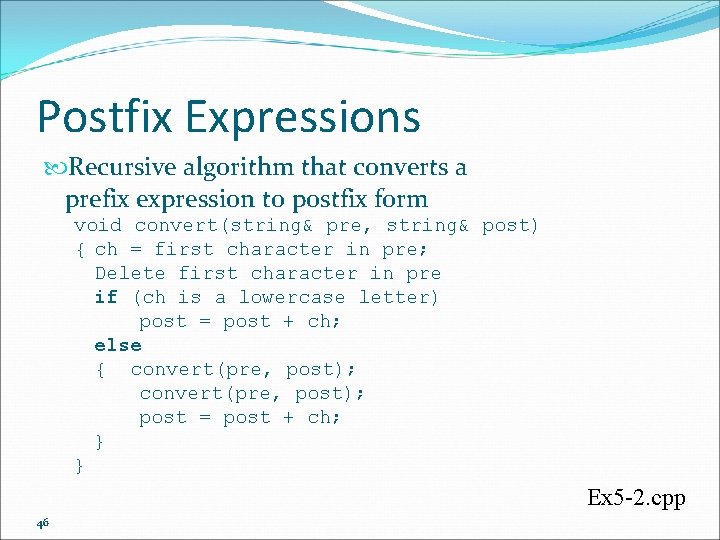
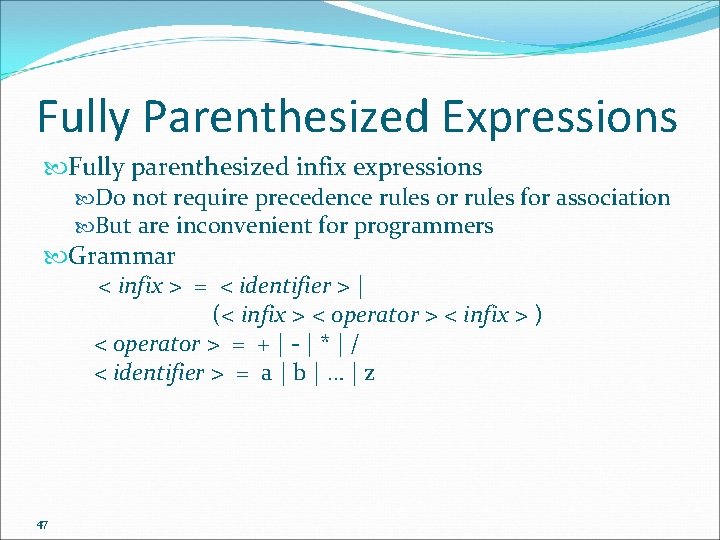
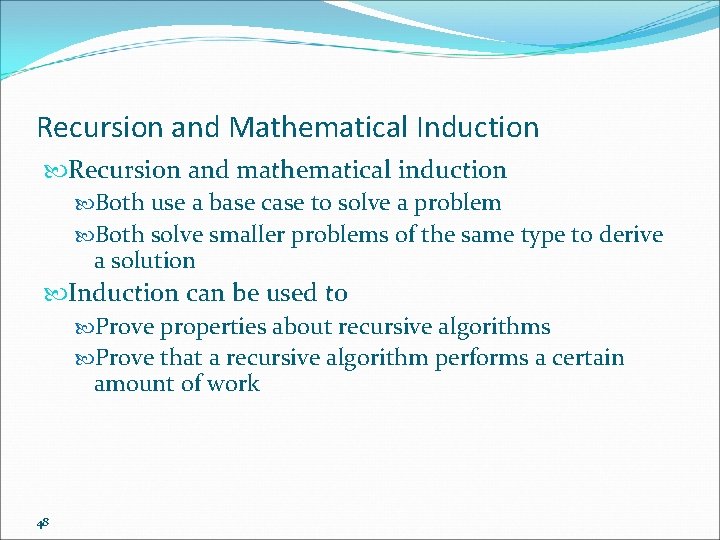
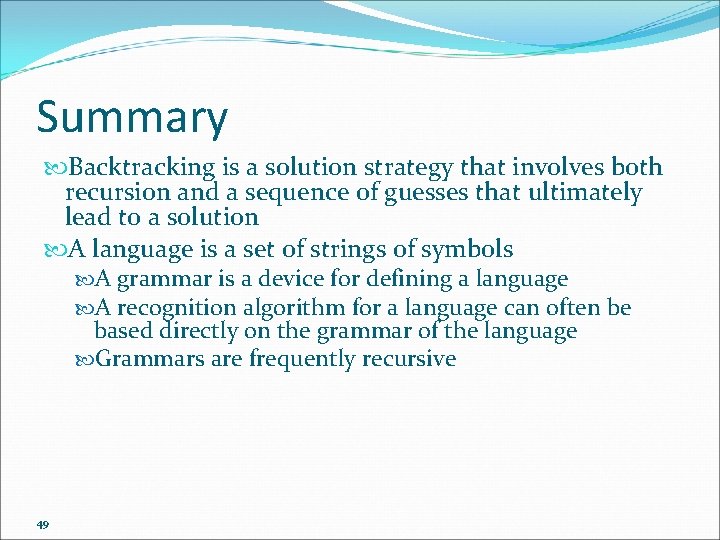
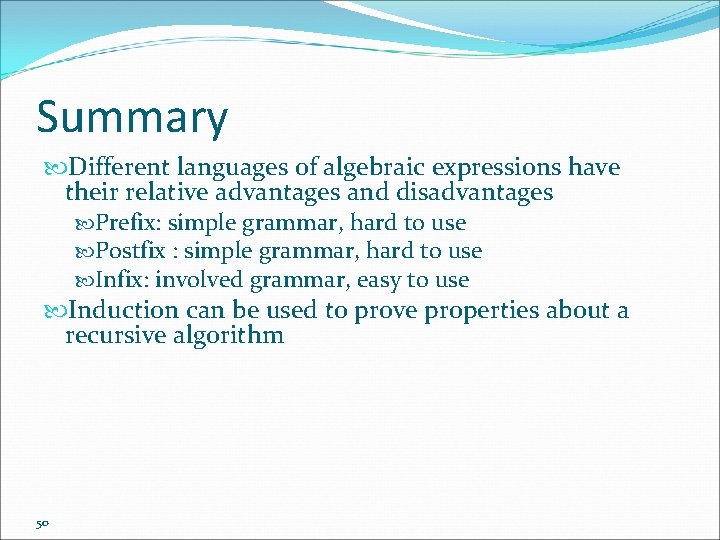
- Slides: 50
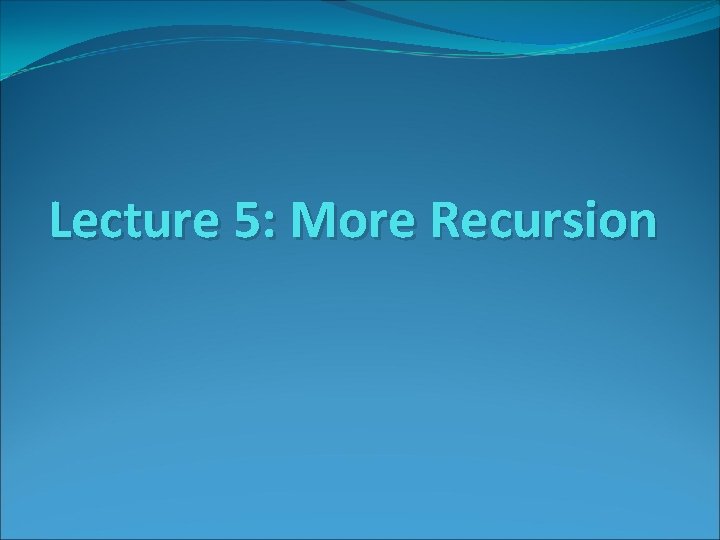
Lecture 5: More Recursion
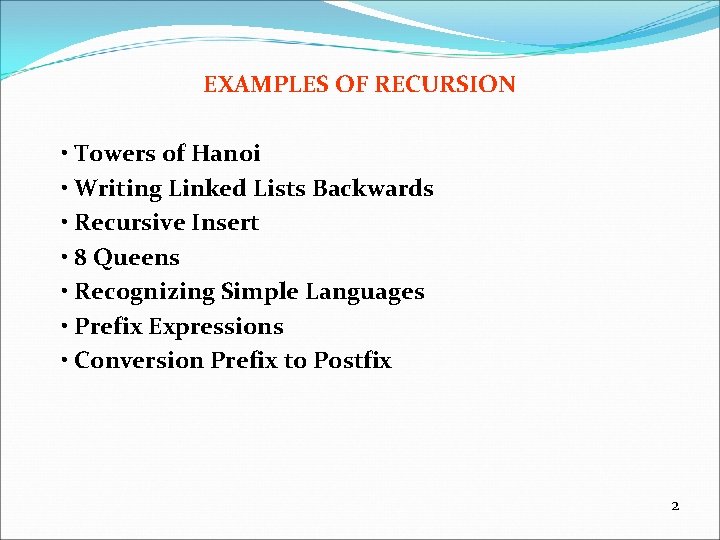
EXAMPLES OF RECURSION • Towers of Hanoi • Writing Linked Lists Backwards • Recursive Insert • 8 Queens • Recognizing Simple Languages • Prefix Expressions • Conversion Prefix to Postfix 2
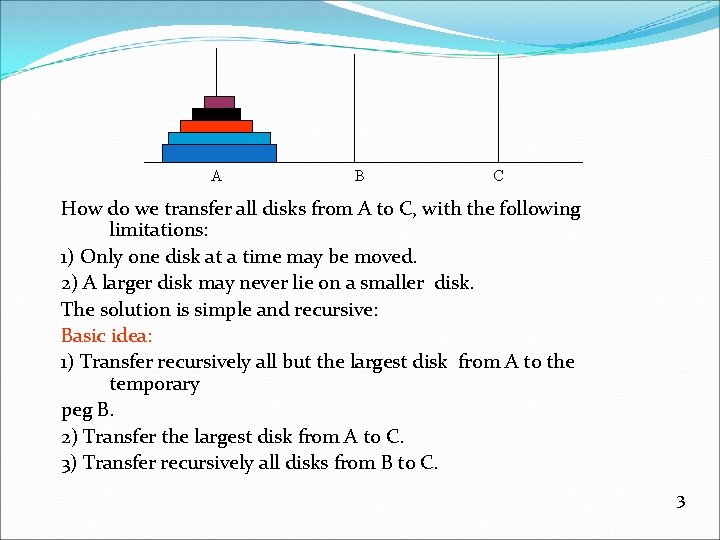
A B C How do we transfer all disks from A to C, with the following limitations: 1) Only one disk at a time may be moved. 2) A larger disk may never lie on a smaller disk. The solution is simple and recursive: Basic idea: 1) Transfer recursively all but the largest disk from A to the temporary peg B. 2) Transfer the largest disk from A to C. 3) Transfer recursively all disks from B to C. 3
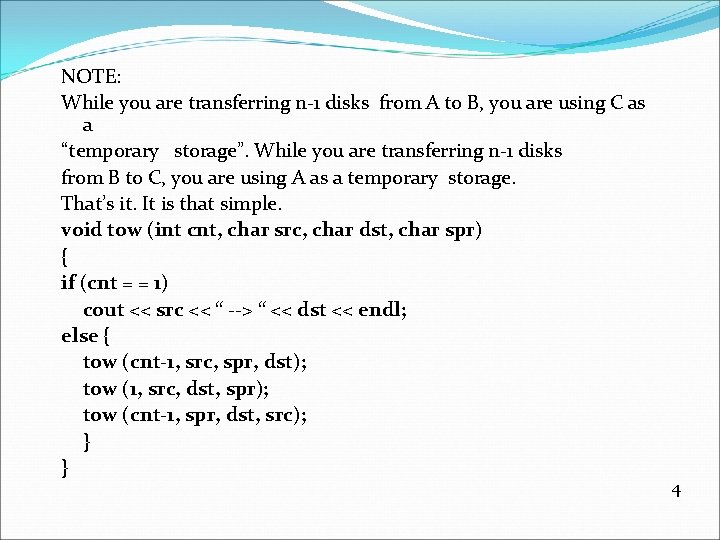
NOTE: While you are transferring n-1 disks from A to B, you are using C as a “temporary storage”. While you are transferring n-1 disks from B to C, you are using A as a temporary storage. That’s it. It is that simple. void tow (int cnt, char src, char dst, char spr) { if (cnt = = 1) cout << src << “ --> “ << dst << endl; else { tow (cnt-1, src, spr, dst); tow (1, src, dst, spr); tow (cnt-1, spr, dst, src); } } 4
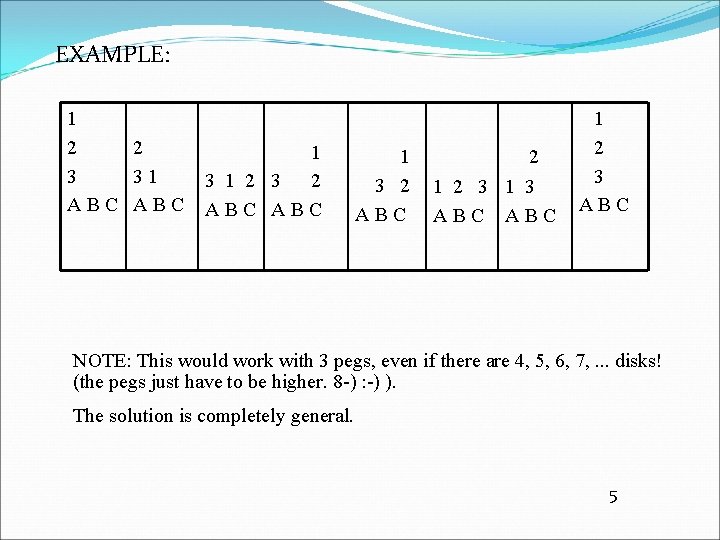
EXAMPLE: 1 2 2 3 31 ABC 1 3 1 2 3 2 ABC 1 3 2 ABC 2 1 2 3 1 3 ABC 1 2 3 ABC NOTE: This would work with 3 pegs, even if there are 4, 5, 6, 7, . . . disks! (the pegs just have to be higher. 8 -) : -) ). The solution is completely general. 5
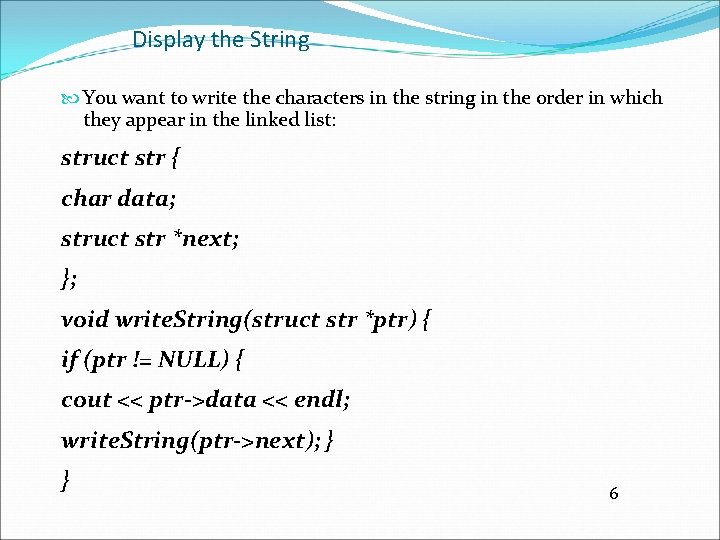
Display the String You want to write the characters in the string in the order in which they appear in the linked list: struct str { char data; struct str *next; }; void write. String(struct str *ptr) { if (ptr != NULL) { cout << ptr->data << endl; write. String(ptr->next); } } 6
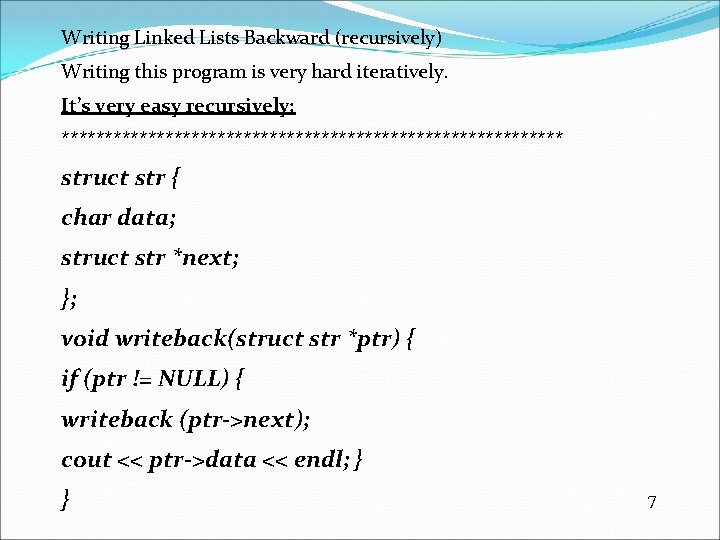
Writing Linked Lists Backward (recursively) Writing this program is very hard iteratively. It’s very easy recursively: ***************************** struct str { char data; struct str *next; }; void writeback(struct str *ptr) { if (ptr != NULL) { writeback (ptr->next); cout << ptr->data << endl; } } 7
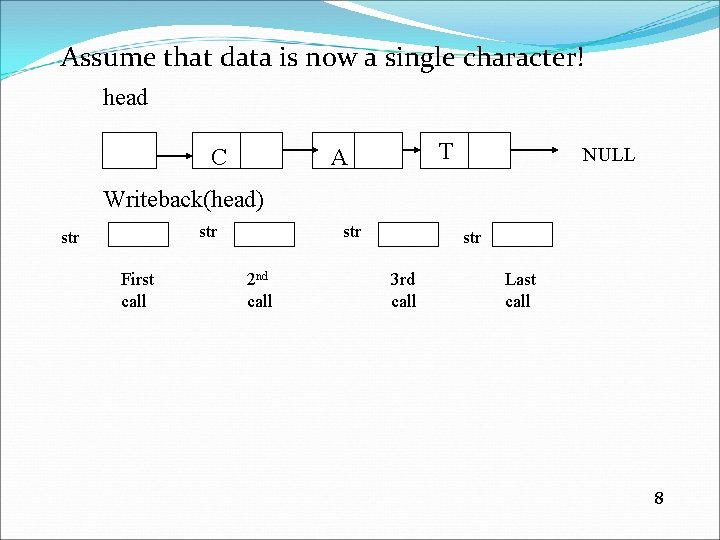
Assume that data is now a single character! head C T A NULL Writeback(head) str First call str 2 nd call str 3 rd call Last call 8
![Strip a way first character write Backward 2char s cout Enter Strip a way first character write. Backward 2(char [] s) { cout << “Enter](https://slidetodoc.com/presentation_image_h2/9cedad0492457c726ebebbb8463dfe8b/image-9.jpg)
Strip a way first character write. Backward 2(char [] s) { cout << “Enter writ. Backward 2 with string: “ << s<< endl; if (the string is empty) //Do nothing – this is the base case else { writed. Backward 2 (s minus its character ); // calling point cout << “About to write first character of string: ” << s << endl; write the first character of s } cout << “leave write. Backward 2 with string: “ << s << endl; } 9
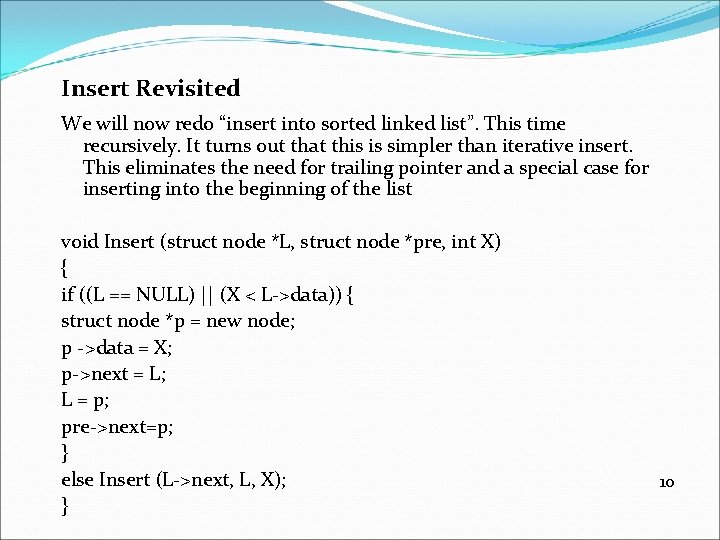
Insert Revisited We will now redo “insert into sorted linked list”. This time recursively. It turns out that this is simpler than iterative insert. This eliminates the need for trailing pointer and a special case for inserting into the beginning of the list void Insert (struct node *L, struct node *pre, int X) { if ((L == NULL) || (X < L->data)) { struct node *p = new node; p ->data = X; p->next = L; L = p; pre->next=p; } else Insert (L->next, L, X); } 10
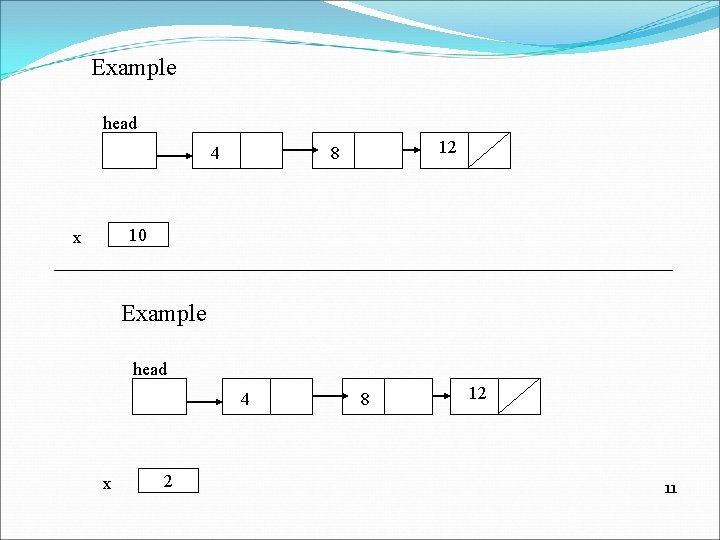
Example head 4 12 8 10 x Example head 4 x 2 8 12 11
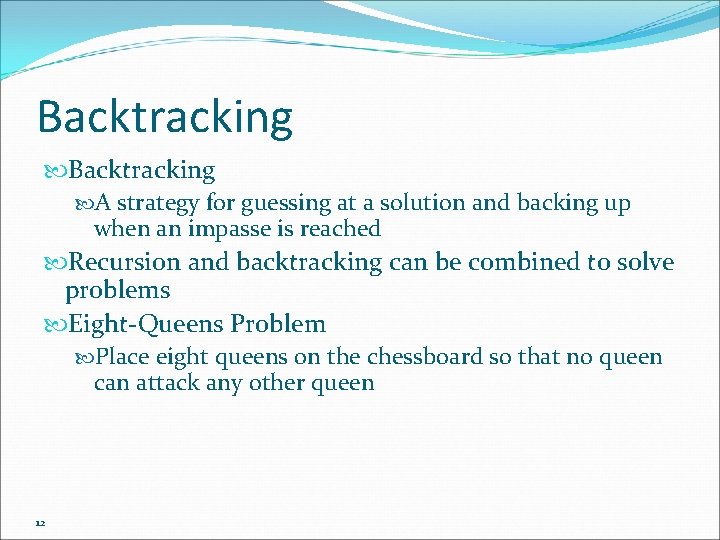
Backtracking A strategy for guessing at a solution and backing up when an impasse is reached Recursion and backtracking can be combined to solve problems Eight-Queens Problem Place eight queens on the chessboard so that no queen can attack any other queen 12
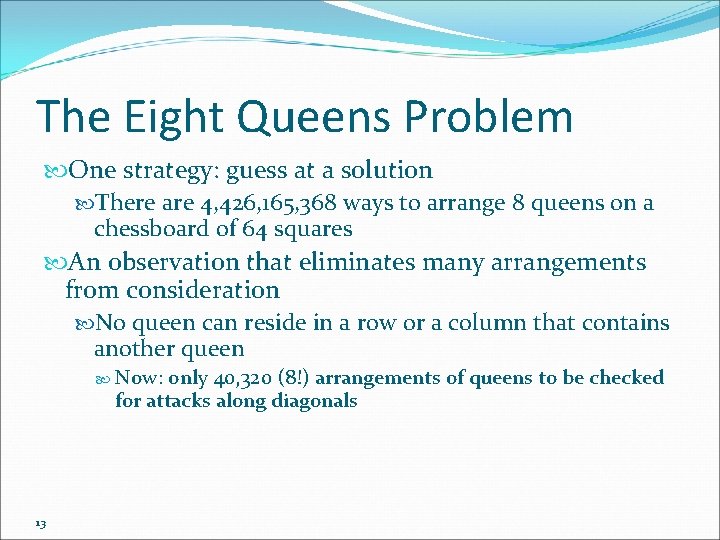
The Eight Queens Problem One strategy: guess at a solution There are 4, 426, 165, 368 ways to arrange 8 queens on a chessboard of 64 squares An observation that eliminates many arrangements from consideration No queen can reside in a row or a column that contains another queen Now: only 40, 320 (8!) arrangements of queens to be checked for attacks along diagonals 13
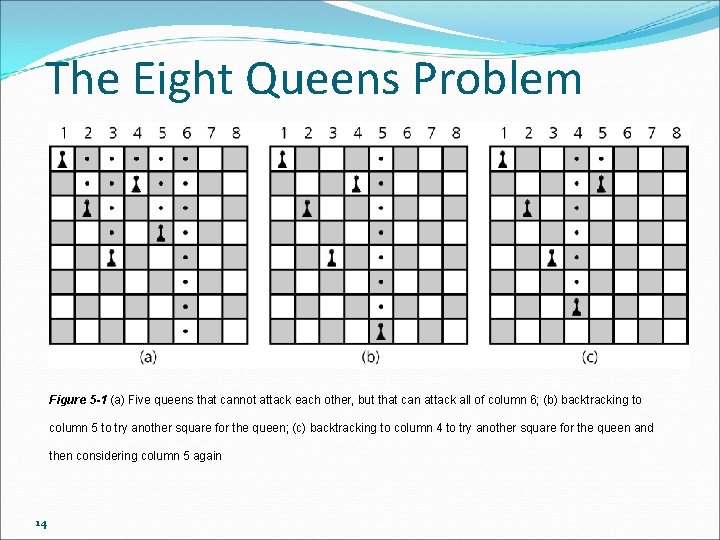
The Eight Queens Problem Figure 5 -1 (a) Five queens that cannot attack each other, but that can attack all of column 6; (b) backtracking to column 5 to try another square for the queen; (c) backtracking to column 4 to try another square for the queen and then considering column 5 again 14
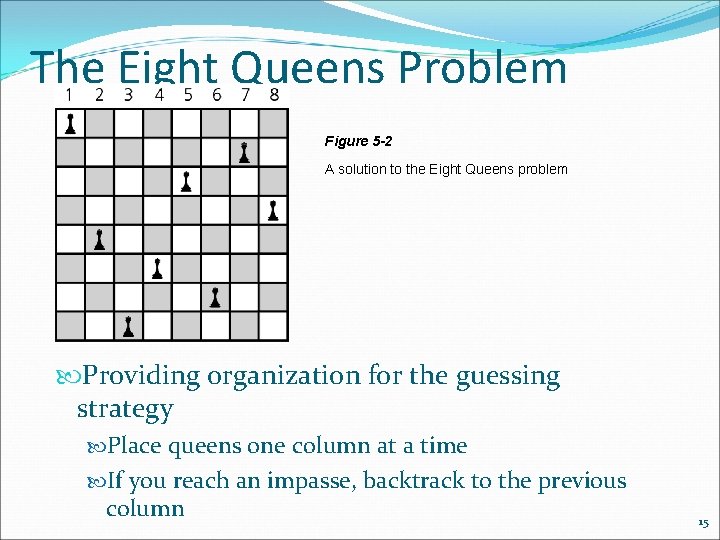
The Eight Queens Problem Figure 5 -2 A solution to the Eight Queens problem Providing organization for the guessing strategy Place queens one column at a time If you reach an impasse, backtrack to the previous column 15
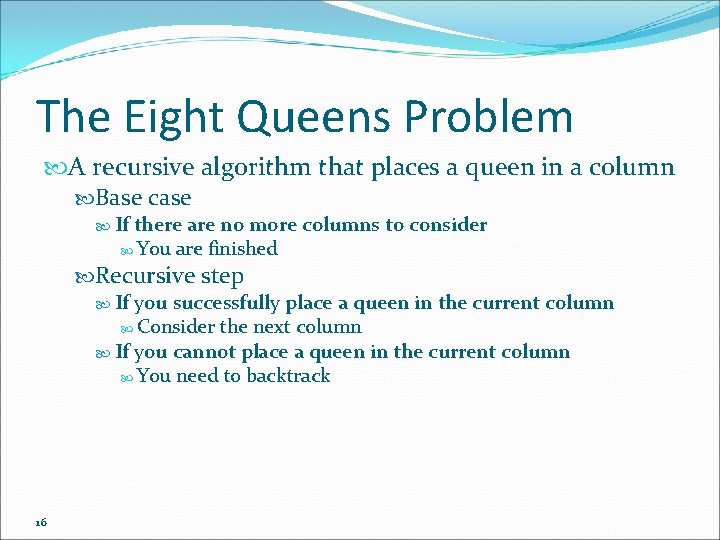
The Eight Queens Problem A recursive algorithm that places a queen in a column Base case If there are no more columns to consider You are finished Recursive step If you successfully place a queen in the current column Consider the next column If you cannot place a queen in the current column You need to backtrack 16
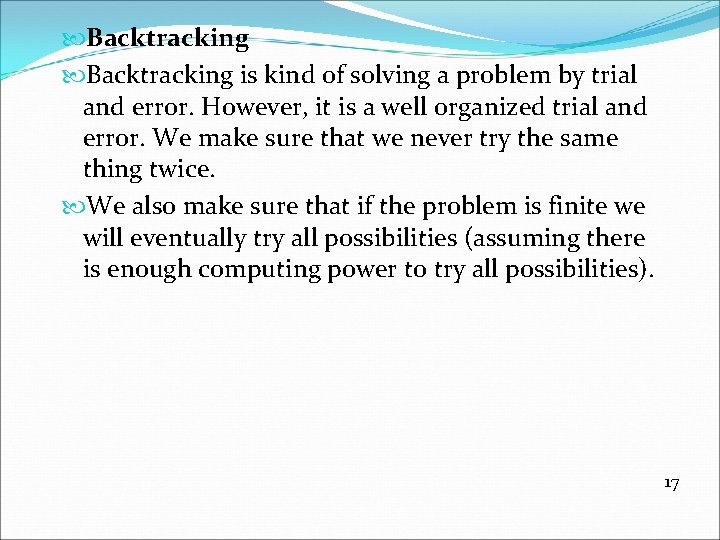
Backtracking is kind of solving a problem by trial and error. However, it is a well organized trial and error. We make sure that we never try the same thing twice. We also make sure that if the problem is finite we will eventually try all possibilities (assuming there is enough computing power to try all possibilities). 17
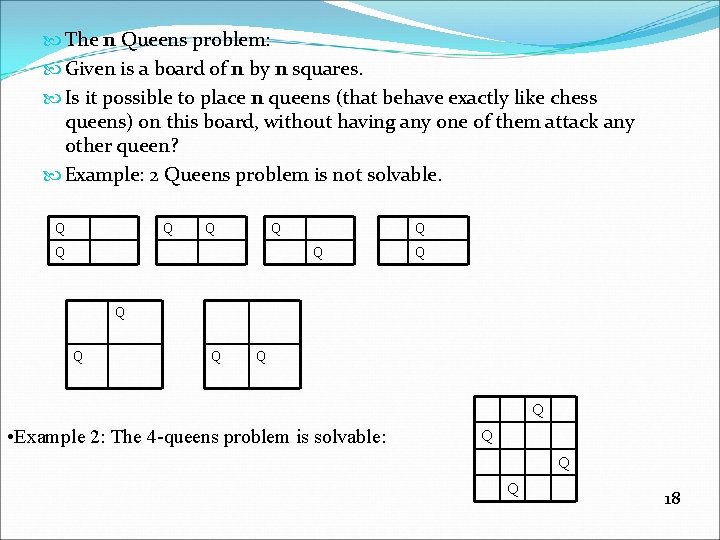
The n Queens problem: Given is a board of n by n squares. Is it possible to place n queens (that behave exactly like chess queens) on this board, without having any one of them attack any other queen? Example: 2 Queens problem is not solvable. Q Q Q Q • Example 2: The 4 -queens problem is solvable: Q Q Q 18
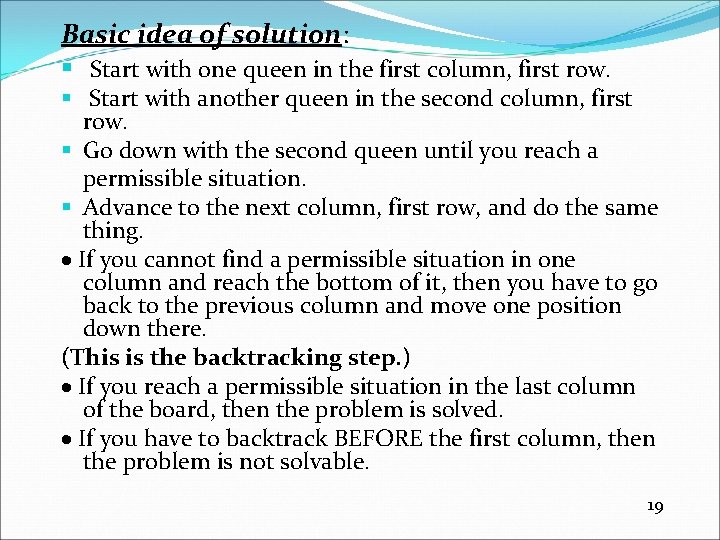
Basic idea of solution: § Start with one queen in the first column, first row. § Start with another queen in the second column, first row. § Go down with the second queen until you reach a permissible situation. § Advance to the next column, first row, and do the same thing. · If you cannot find a permissible situation in one column and reach the bottom of it, then you have to go back to the previous column and move one position down there. (This is the backtracking step. ) · If you reach a permissible situation in the last column of the board, then the problem is solved. · If you have to backtrack BEFORE the first column, then the problem is not solvable. 19
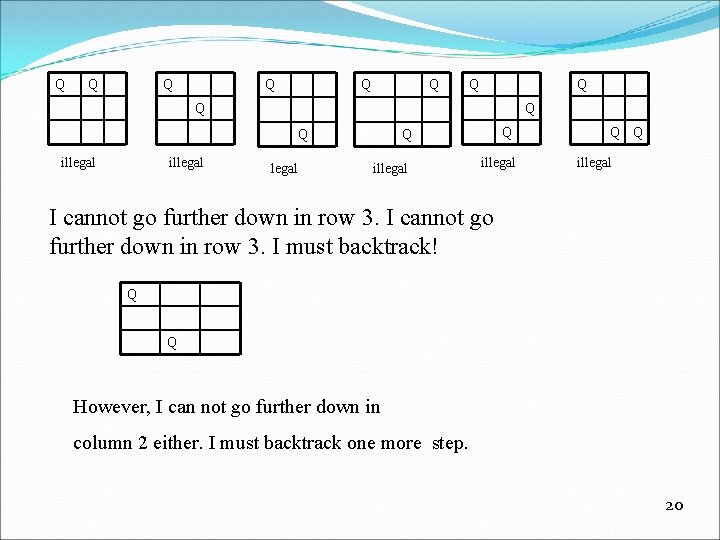
Q Q Q illegal Q illegal Q Q illegal I cannot go further down in row 3. I must backtrack! Q Q However, I can not go further down in column 2 either. I must backtrack one more step. 20
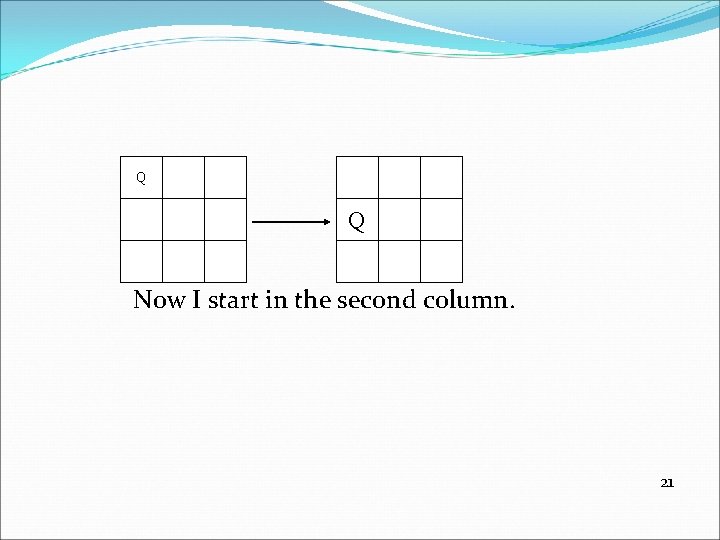
Q Q Now I start in the second column. 21
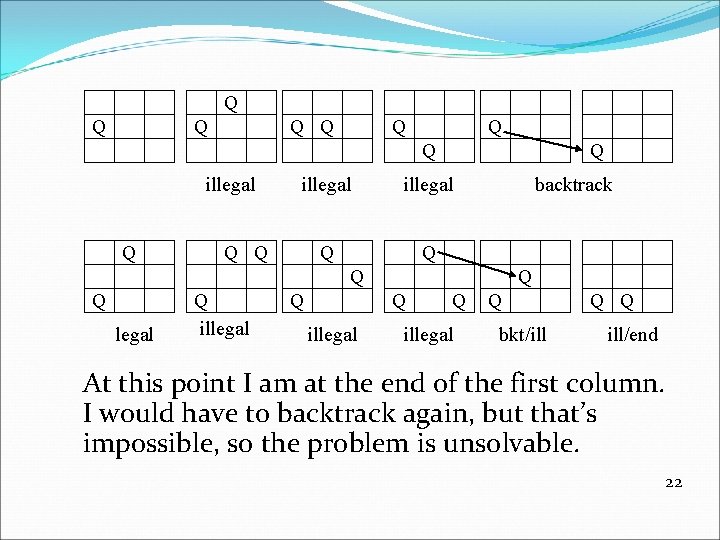
Q Q Q Q illegal Q Q backtrack Q Q legal Q illegal Q Q Q illegal Q bkt/ill Q Q ill/end At this point I am at the end of the first column. I would have to backtrack again, but that’s impossible, so the problem is unsolvable. 22
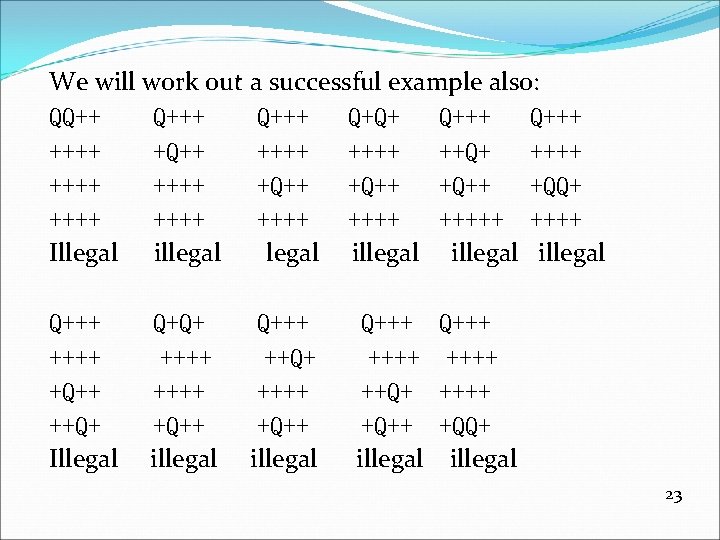
We will work out a successful example also: QQ++ Q+++ Q+Q+ Q+++ ++++ +Q++ ++++ ++Q+ ++++ +Q++ +QQ+ ++++ ++++ Illegal illegal Q+++ +Q++ ++Q+ Illegal Q+Q+ ++++ +Q++ illegal Q+++ ++Q+ +Q++ illegal Q+++ ++++ +QQ+ illegal 23
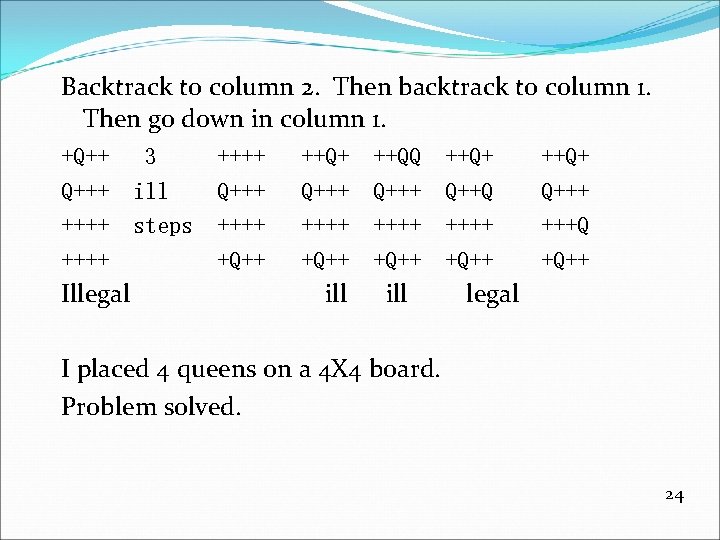
Backtrack to column 2. Then backtrack to column 1. Then go down in column 1. +Q++ Q+++ ++++ Illegal 3 ill steps ++++ Q+++ +Q++ ++Q+ Q+++ +Q++ ill ++QQ Q+++ +Q++ ill ++Q+ Q++Q ++++ +Q++ ++Q+ Q+++ +++Q +Q++ legal I placed 4 queens on a 4 X 4 board. Problem solved. 24
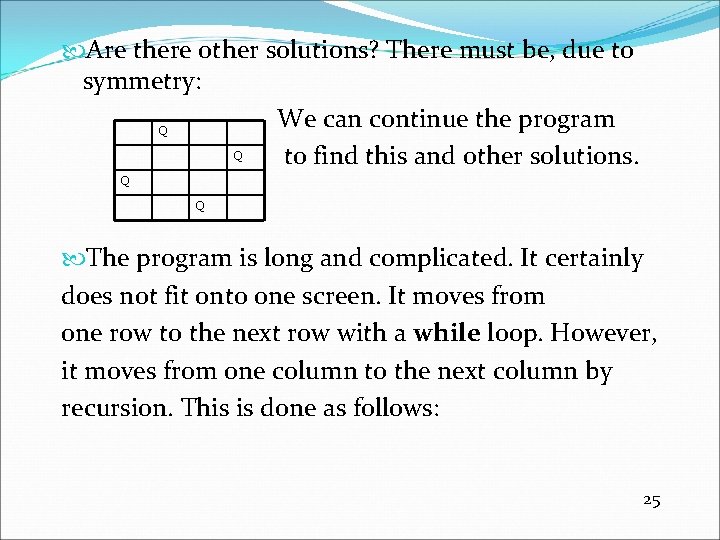
Are there other solutions? There must be, due to symmetry: We can continue the program Q Q to find this and other solutions. Q Q The program is long and complicated. It certainly does not fit onto one screen. It moves from one row to the next row with a while loop. However, it moves from one column to the next column by recursion. This is done as follows: 25
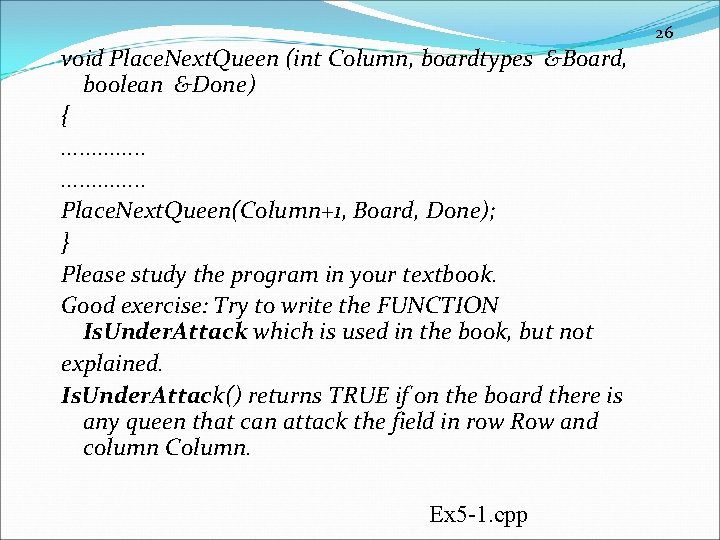
void Place. Next. Queen (int Column, boardtypes &Board, boolean &Done) {. . . . Place. Next. Queen(Column+1, Board, Done); } Please study the program in your textbook. Good exercise: Try to write the FUNCTION Is. Under. Attack which is used in the book, but not explained. Is. Under. Attack() returns TRUE if on the board there is any queen that can attack the field in row Row and column Column. Ex 5 -1. cpp 26
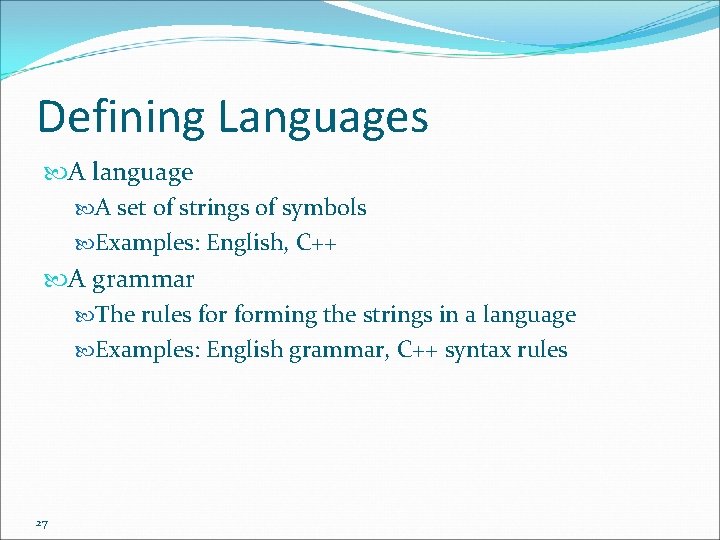
Defining Languages A language A set of strings of symbols Examples: English, C++ A grammar The rules forming the strings in a language Examples: English grammar, C++ syntax rules 27
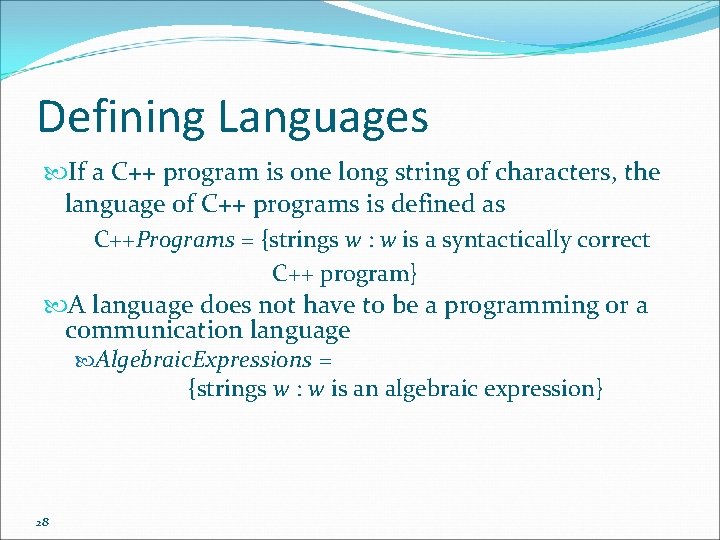
Defining Languages If a C++ program is one long string of characters, the language of C++ programs is defined as C++Programs = {strings w : w is a syntactically correct C++ program} A language does not have to be a programming or a communication language Algebraic. Expressions = {strings w : w is an algebraic expression} 28
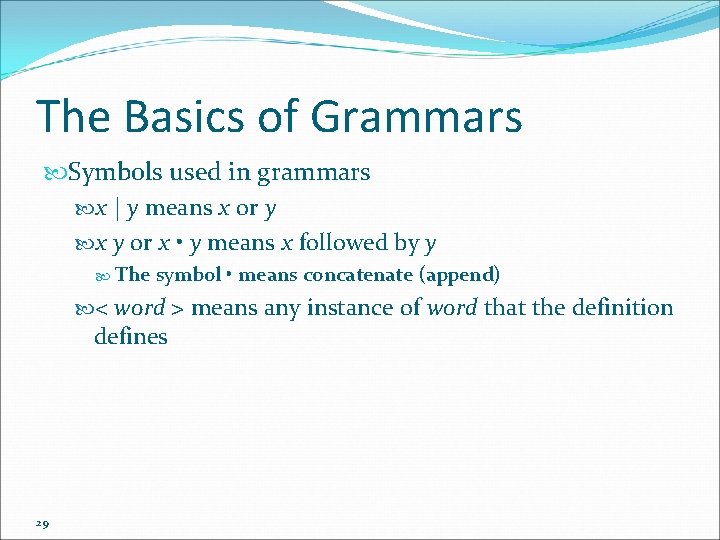
The Basics of Grammars Symbols used in grammars x | y means x or y x y or x • y means x followed by y The symbol • means concatenate (append) < word > means any instance of word that the definition defines 29
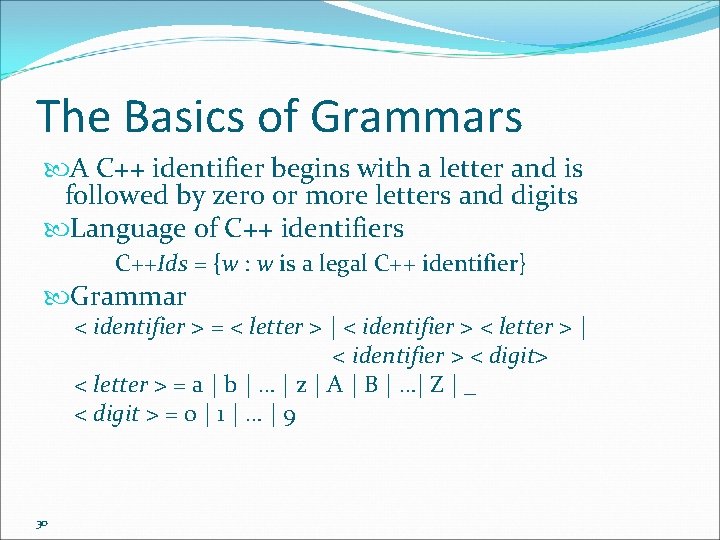
The Basics of Grammars A C++ identifier begins with a letter and is followed by zero or more letters and digits Language of C++ identifiers C++Ids = {w : w is a legal C++ identifier} Grammar < identifier > = < letter > | < identifier > < digit> < letter > = a | b | … | z | A | B | …| Z | _ < digit > = 0 | 1 | … | 9 30
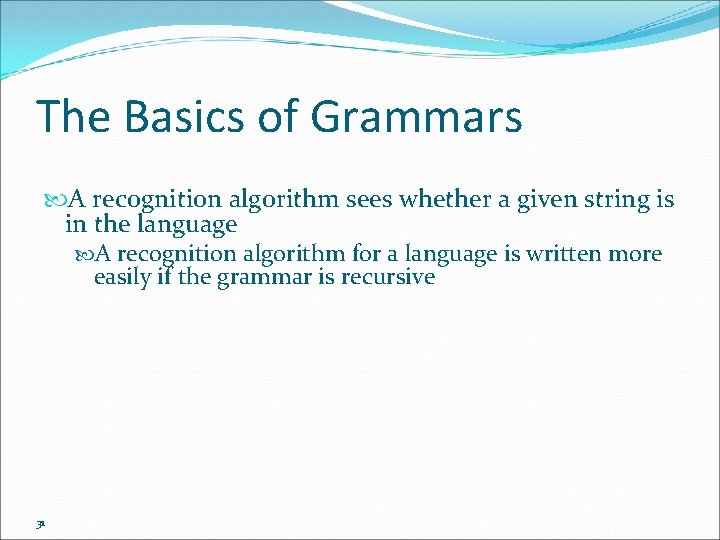
The Basics of Grammars A recognition algorithm sees whether a given string is in the language A recognition algorithm for a language is written more easily if the grammar is recursive 31
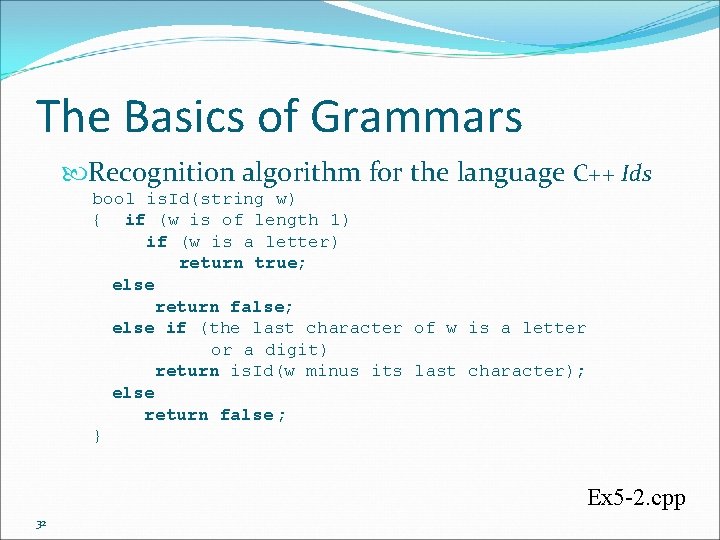
The Basics of Grammars Recognition algorithm for the language C++ Ids bool is. Id(string w) { if (w is of length 1) if (w is a letter) return true; else return false; else if (the last character of w is a letter or a digit) return is. Id(w minus its last character); else return false ; } Ex 5 -2. cpp 32
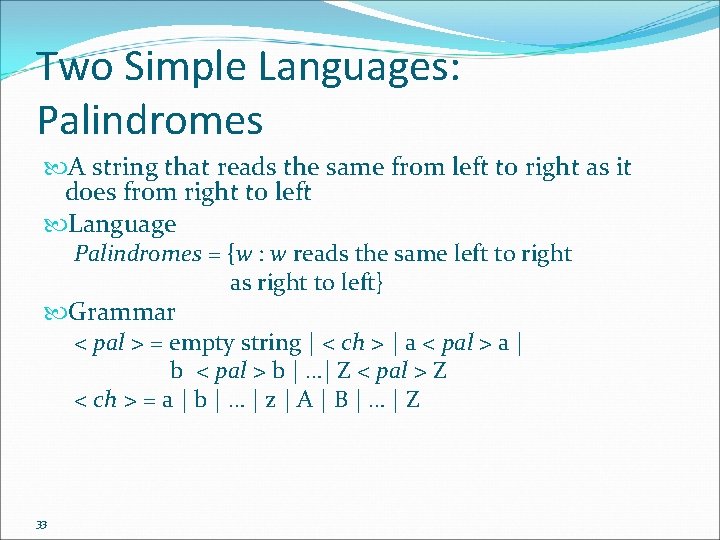
Two Simple Languages: Palindromes A string that reads the same from left to right as it does from right to left Language Palindromes = {w : w reads the same left to right as right to left} Grammar < pal > = empty string | < ch > | a < pal > a | b < pal > b | …| Z < pal > Z < ch > = a | b | … | z | A | B | … | Z 33
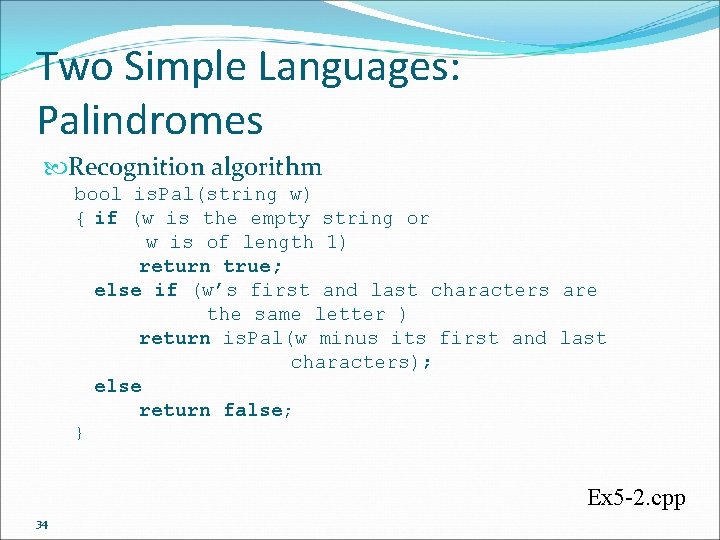
Two Simple Languages: Palindromes Recognition algorithm bool is. Pal(string w) { if (w is the empty string or w is of length 1) return true; else if (w’s first and last characters are the same letter ) return is. Pal(w minus its first and last characters); else return false; } Ex 5 -2. cpp 34
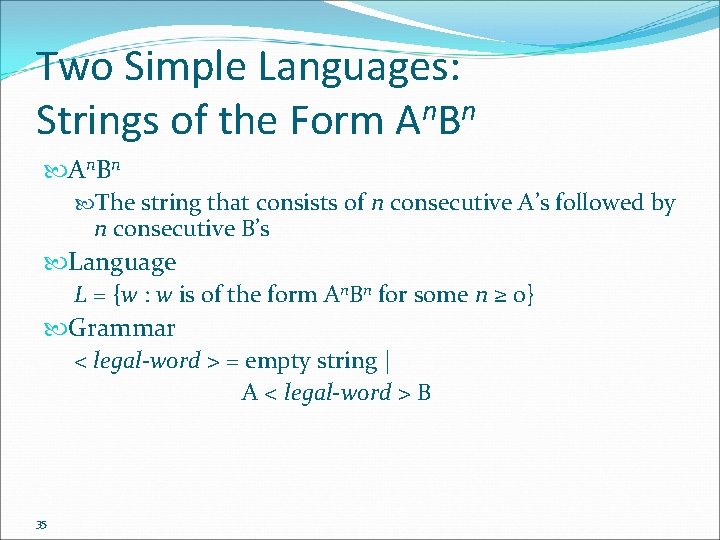
Two Simple Languages: Strings of the Form An. Bn The string that consists of n consecutive A’s followed by n consecutive B’s Language L = {w : w is of the form An. Bn for some n ≥ 0} Grammar < legal-word > = empty string | A < legal-word > B 35
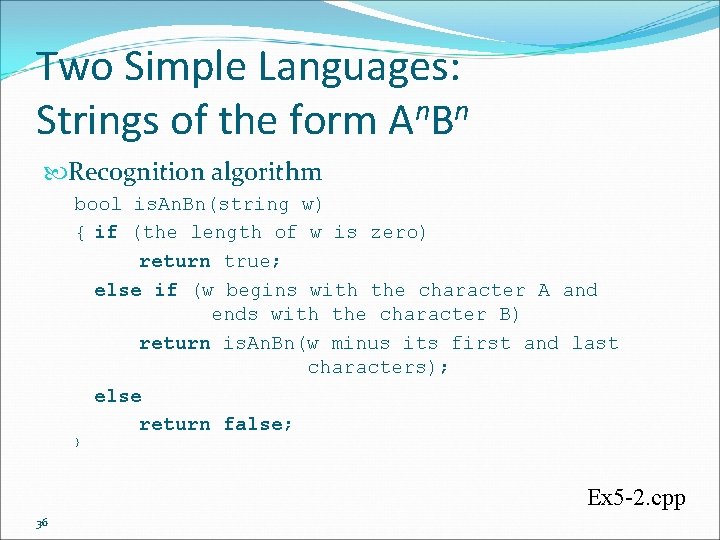
Two Simple Languages: Strings of the form An. Bn Recognition algorithm bool is. An. Bn(string w) { if (the length of w is zero) return true; else if (w begins with the character A and ends with the character B) return is. An. Bn(w minus its first and last characters); else return false; } Ex 5 -2. cpp 36
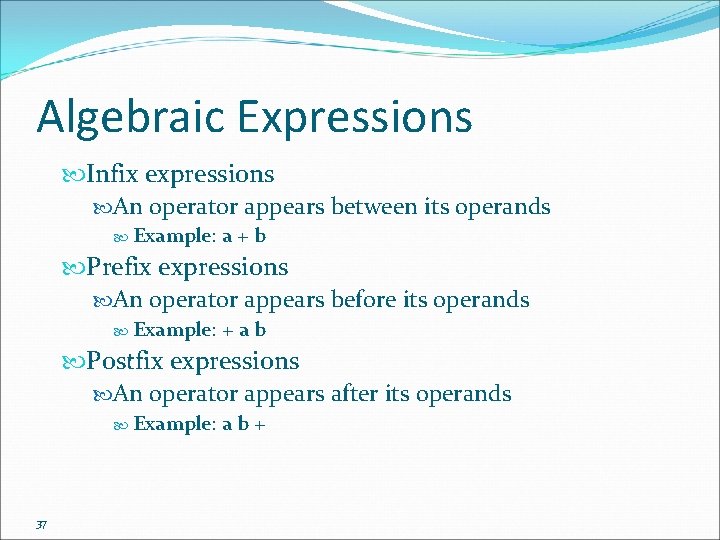
Algebraic Expressions Infix expressions An operator appears between its operands Example: a+b Prefix expressions An operator appears before its operands Example: +ab Postfix expressions An operator appears after its operands Example: 37 ab+
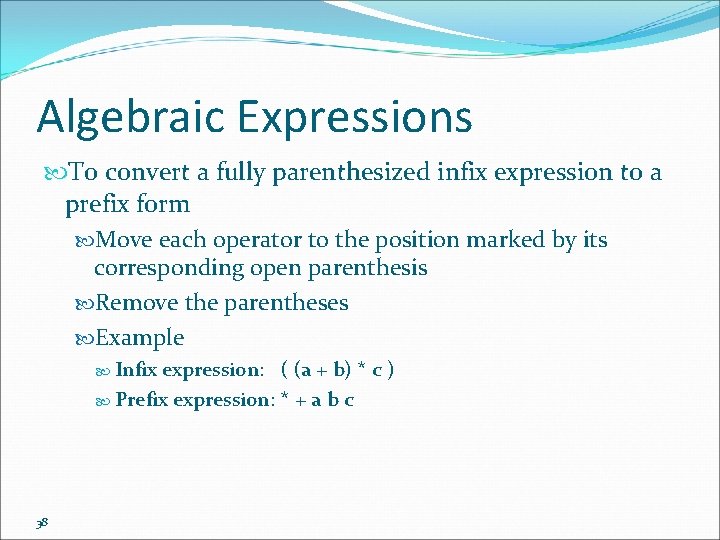
Algebraic Expressions To convert a fully parenthesized infix expression to a prefix form Move each operator to the position marked by its corresponding open parenthesis Remove the parentheses Example Infix expression: ( (a + b) * c ) Prefix expression: * + a b c 38
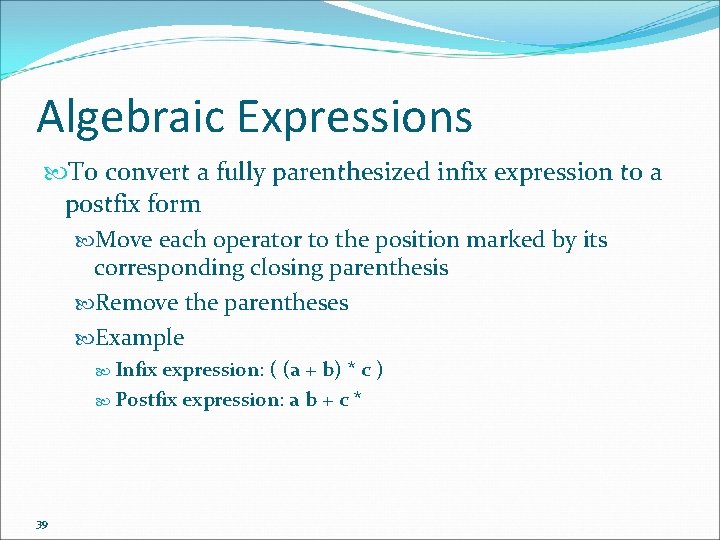
Algebraic Expressions To convert a fully parenthesized infix expression to a postfix form Move each operator to the position marked by its corresponding closing parenthesis Remove the parentheses Example Infix expression: ( (a + b) * c ) Postfix expression: a b + c * 39
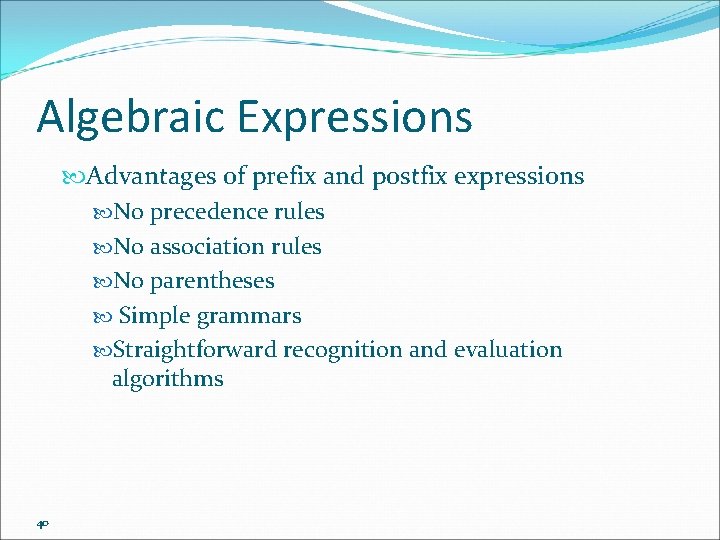
Algebraic Expressions Advantages of prefix and postfix expressions No precedence rules No association rules No parentheses Simple grammars Straightforward recognition and evaluation algorithms 40
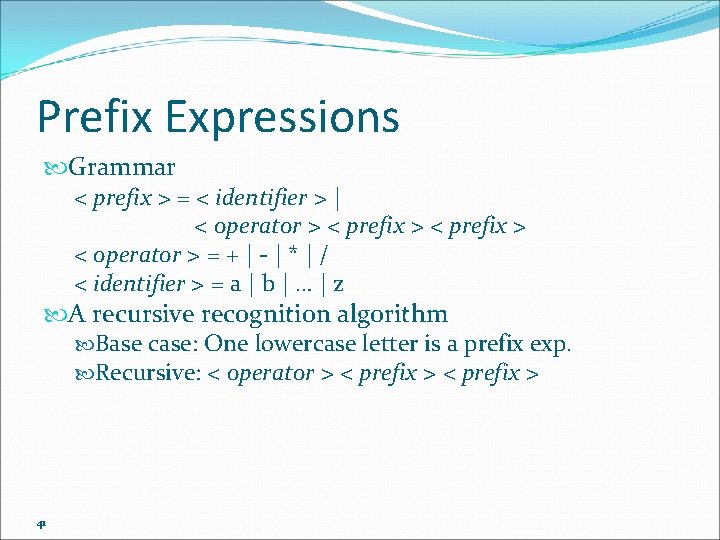
Prefix Expressions Grammar < prefix > = < identifier > | < operator > < prefix > < operator > = + | - | * | / < identifier > = a | b | … | z A recursive recognition algorithm Base case: One lowercase letter is a prefix exp. Recursive: < operator > < prefix > 41
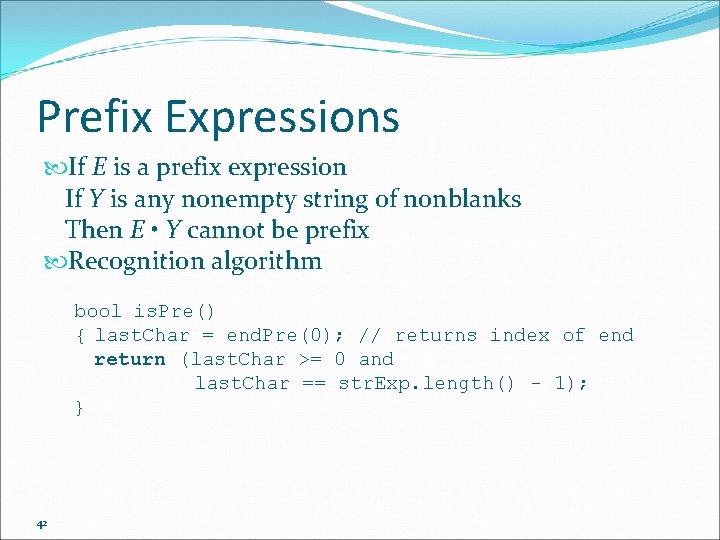
Prefix Expressions If E is a prefix expression If Y is any nonempty string of nonblanks Then E • Y cannot be prefix Recognition algorithm bool is. Pre() { last. Char = end. Pre(0); // returns index of end return (last. Char >= 0 and last. Char == str. Exp. length() - 1); } 42
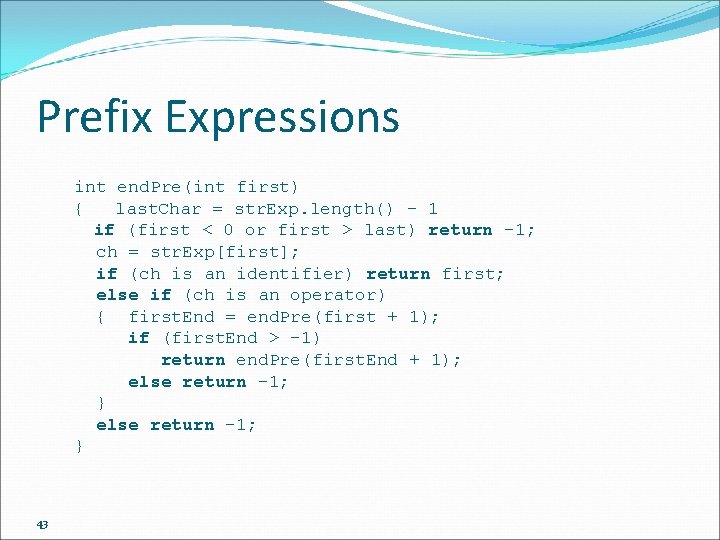
Prefix Expressions int end. Pre(int first) { last. Char = str. Exp. length() - 1 if (first < 0 or first > last) return -1; ch = str. Exp[first]; if (ch is an identifier) return first; else if (ch is an operator) { first. End = end. Pre(first + 1); if (first. End > -1) return end. Pre(first. End + 1); else return -1; } 43
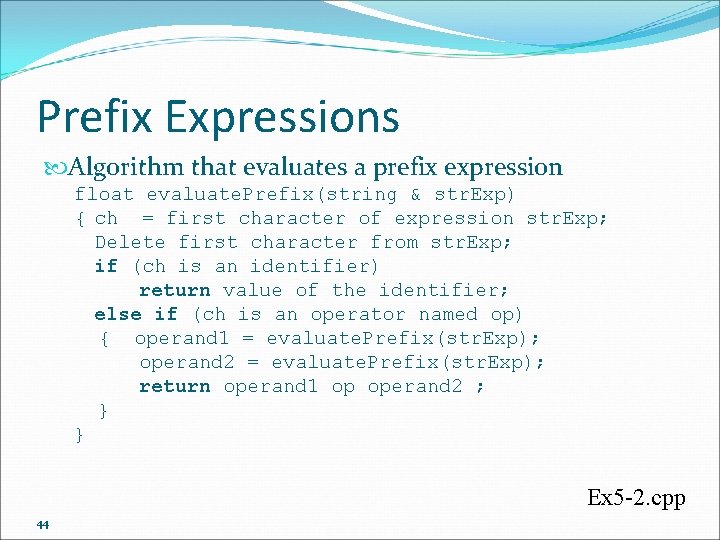
Prefix Expressions Algorithm that evaluates a prefix expression float evaluate. Prefix(string & str. Exp) { ch = first character of expression str. Exp; Delete first character from str. Exp; if (ch is an identifier) return value of the identifier; else if (ch is an operator named op) { operand 1 = evaluate. Prefix(str. Exp); operand 2 = evaluate. Prefix(str. Exp); return operand 1 op operand 2 ; } } Ex 5 -2. cpp 44
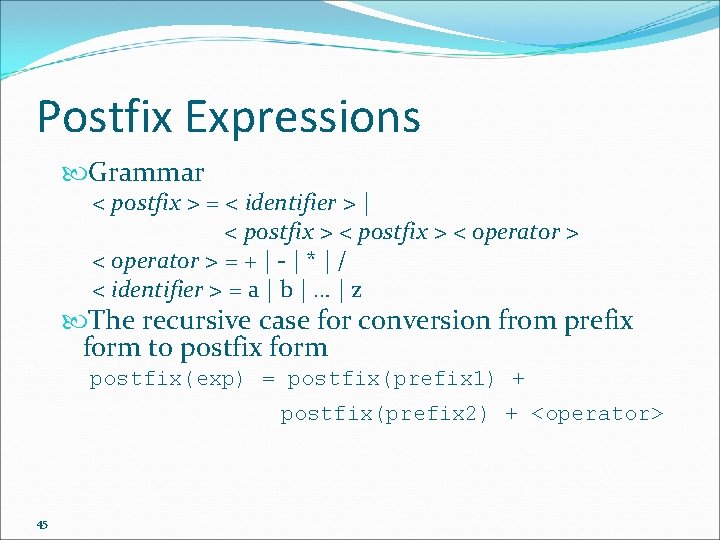
Postfix Expressions Grammar < postfix > = < identifier > | < postfix > < operator > = + | - | * | / < identifier > = a | b | … | z The recursive case for conversion from prefix form to postfix form postfix(exp) = postfix(prefix 1) + postfix(prefix 2) + <operator> 45
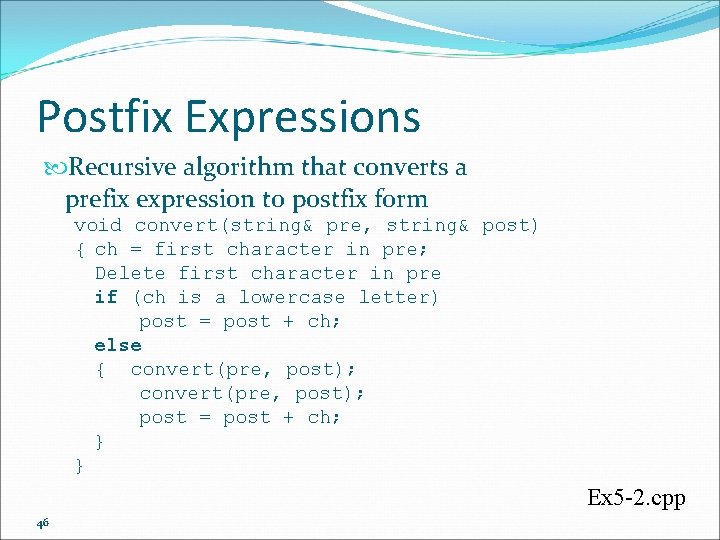
Postfix Expressions Recursive algorithm that converts a prefix expression to postfix form void convert(string& pre, string& post) { ch = first character in pre; Delete first character in pre if (ch is a lowercase letter) post = post + ch; else { convert(pre, post); post = post + ch; } } Ex 5 -2. cpp 46
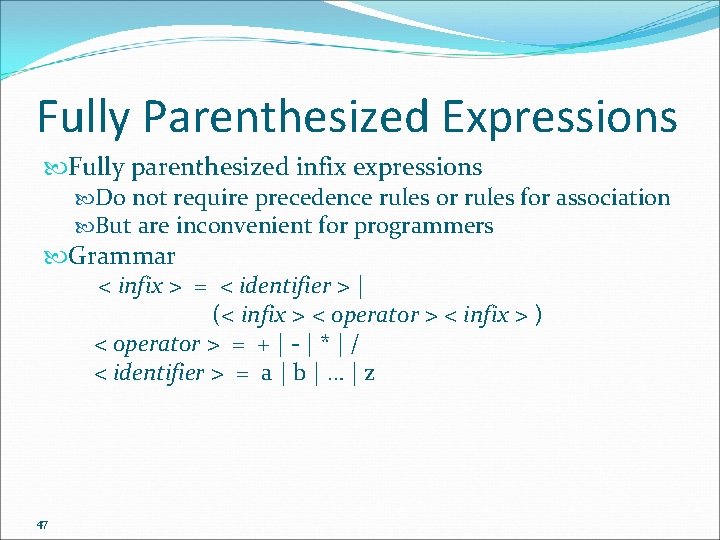
Fully Parenthesized Expressions Fully parenthesized infix expressions Do not require precedence rules or rules for association But are inconvenient for programmers Grammar < infix > = < identifier > | (< infix > < operator > < infix > ) < operator > = + | - | * | / < identifier > = a | b | … | z 47
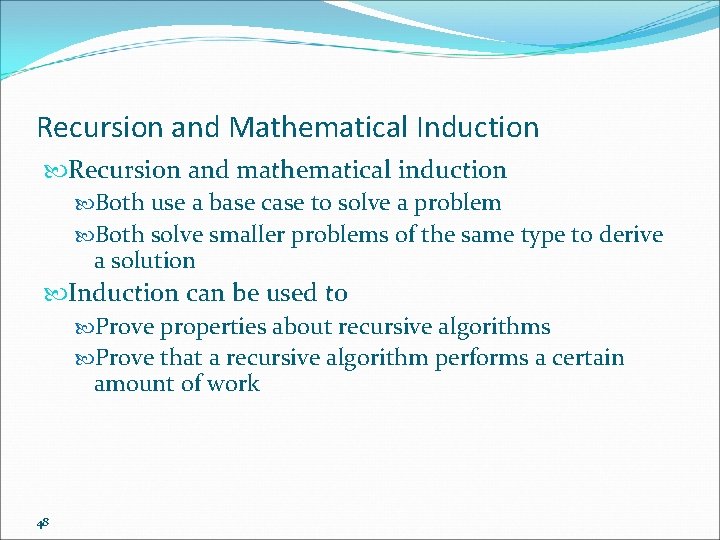
Recursion and Mathematical Induction Recursion and mathematical induction Both use a base case to solve a problem Both solve smaller problems of the same type to derive a solution Induction can be used to Prove properties about recursive algorithms Prove that a recursive algorithm performs a certain amount of work 48
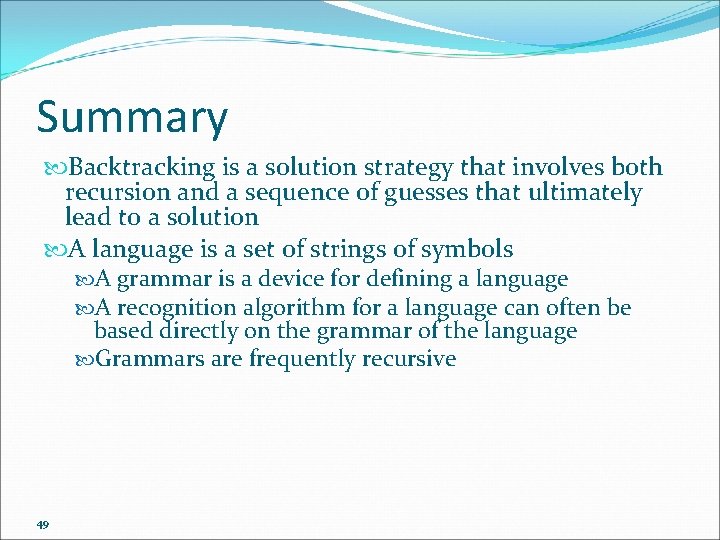
Summary Backtracking is a solution strategy that involves both recursion and a sequence of guesses that ultimately lead to a solution A language is a set of strings of symbols A grammar is a device for defining a language A recognition algorithm for a language can often be based directly on the grammar of the language Grammars are frequently recursive 49
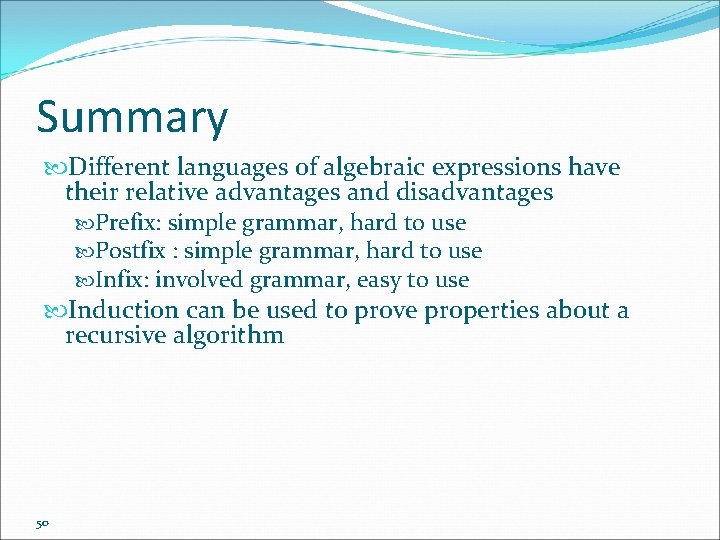
Summary Different languages of algebraic expressions have their relative advantages and disadvantages Prefix: simple grammar, hard to use Postfix : simple grammar, hard to use Infix: involved grammar, easy to use Induction can be used to prove properties about a recursive algorithm 50