Lecture 4 Control Structures while for and dowhile
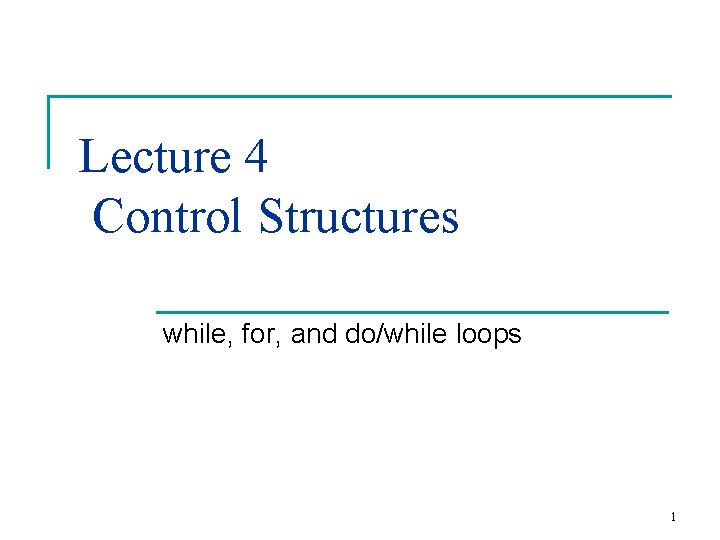
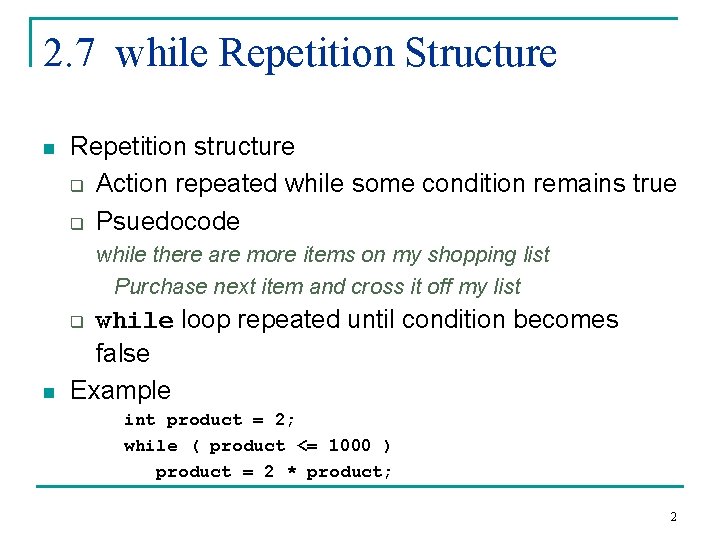
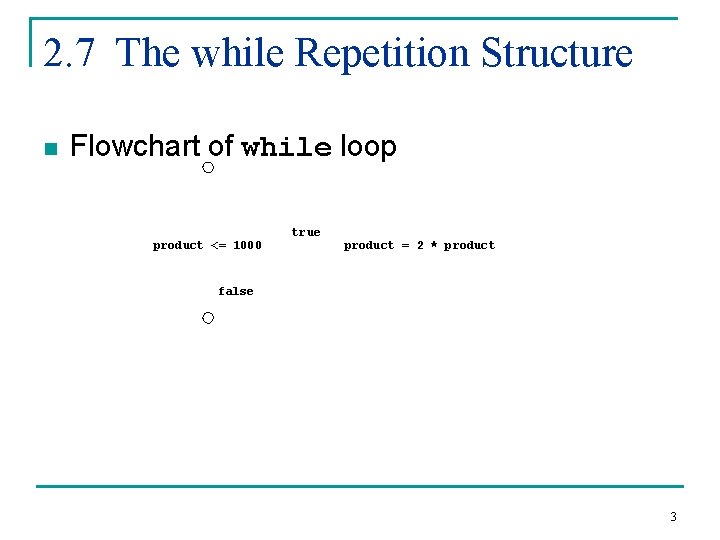
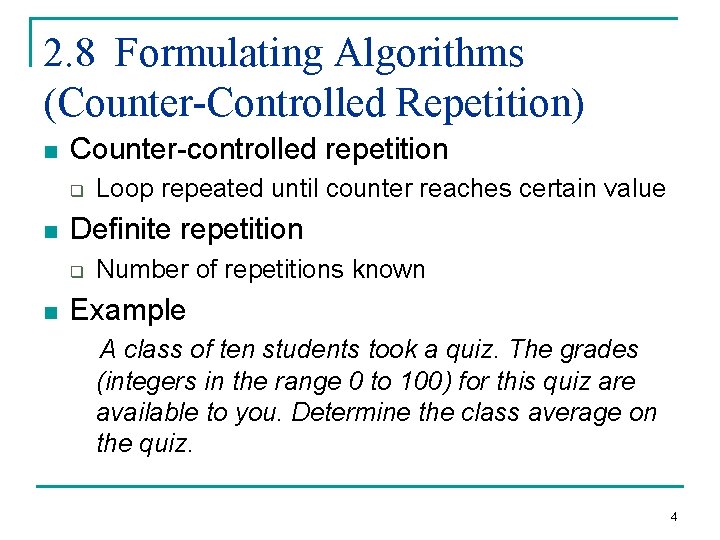
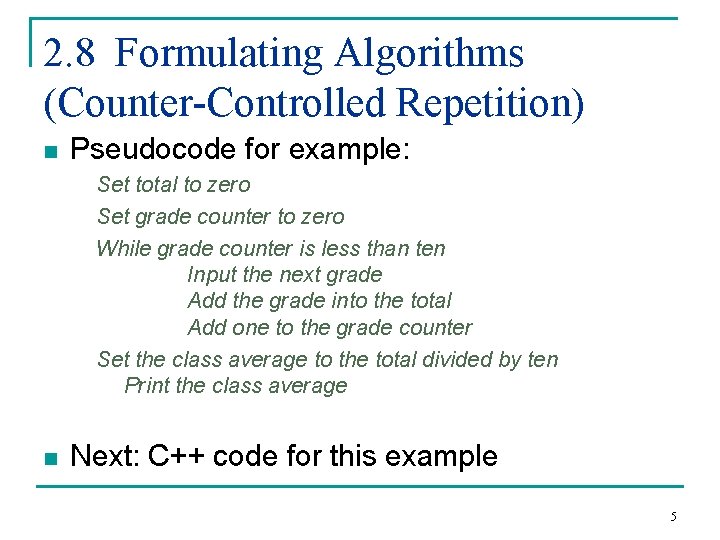
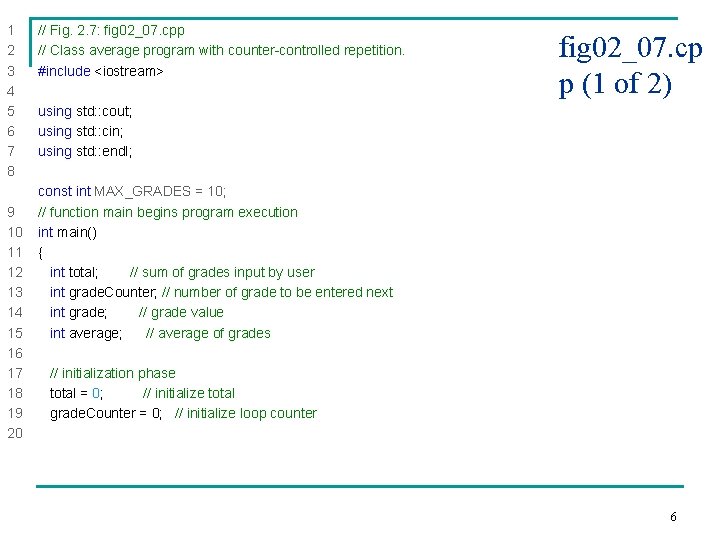
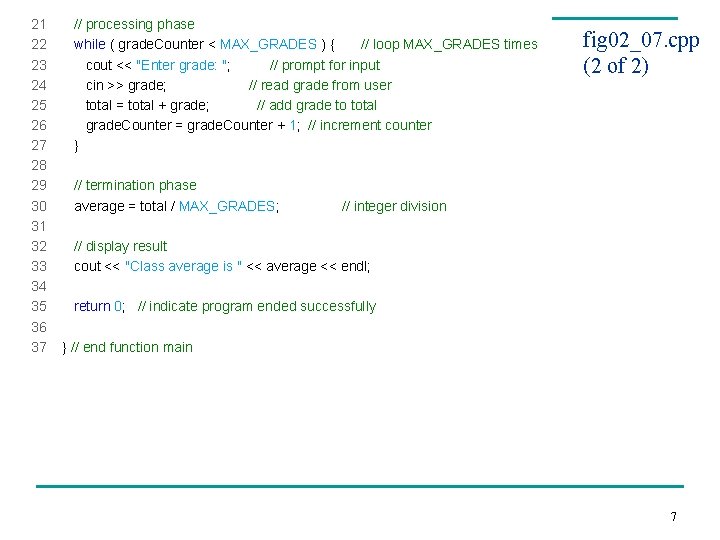
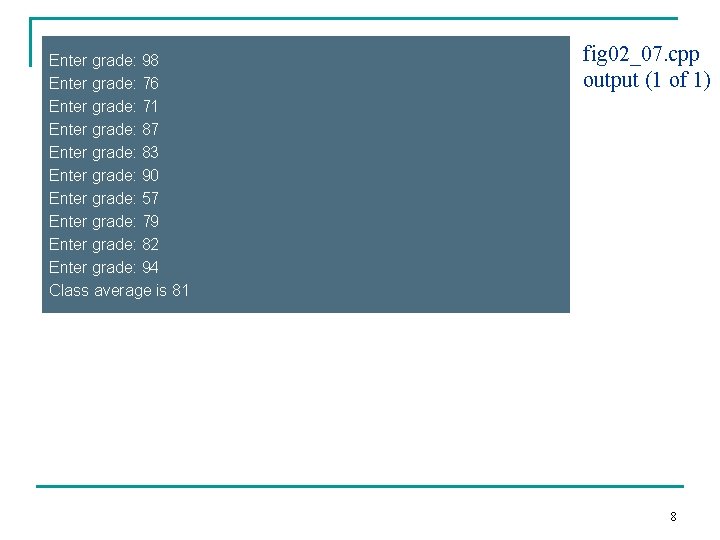
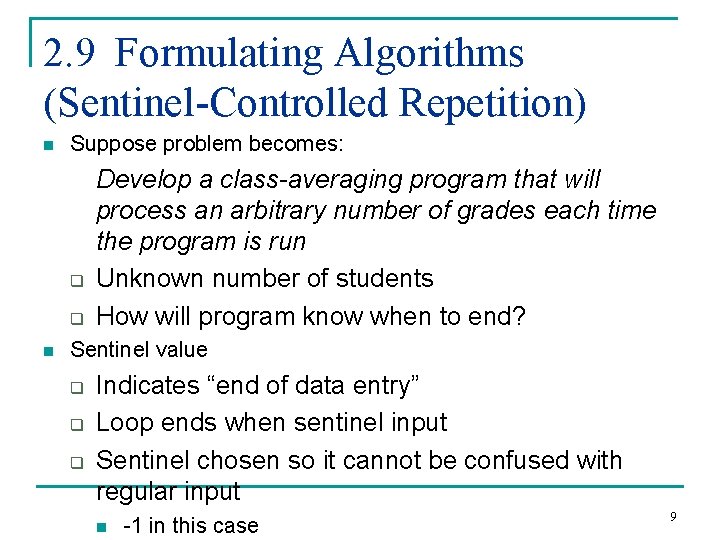
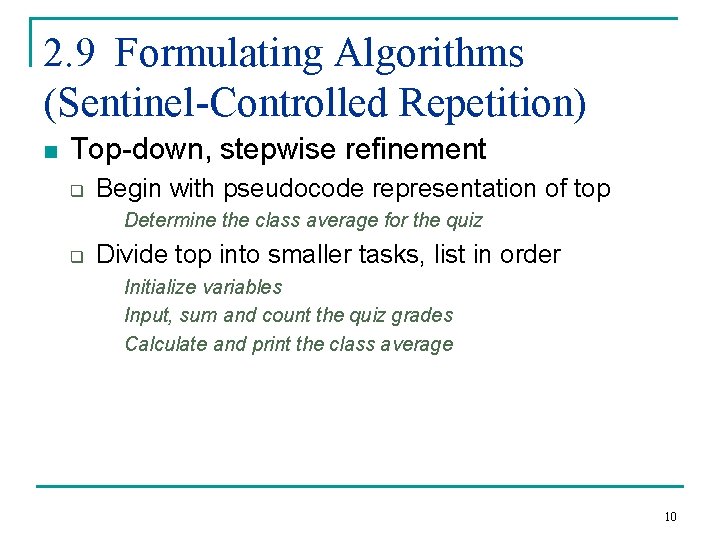
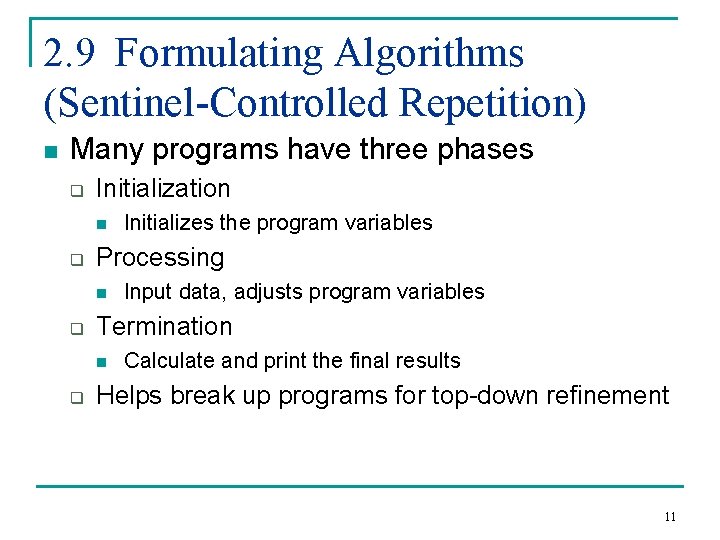
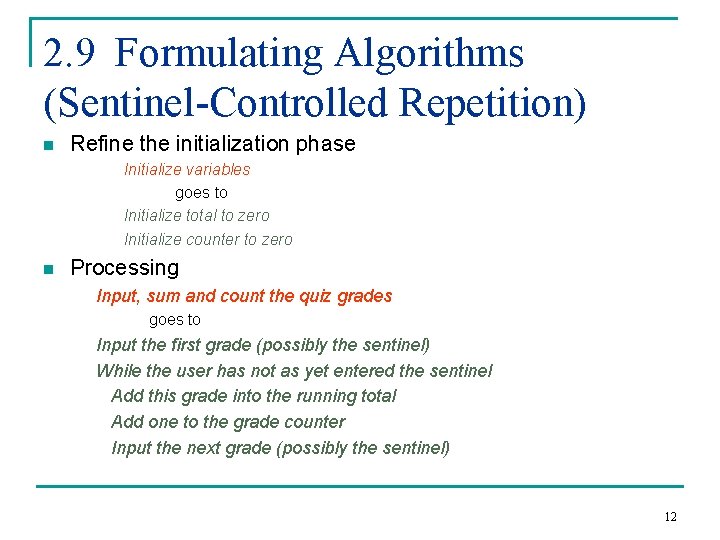
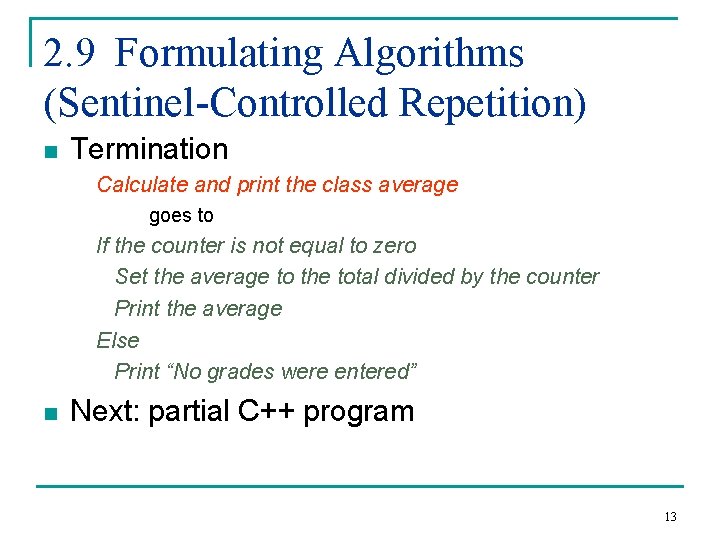
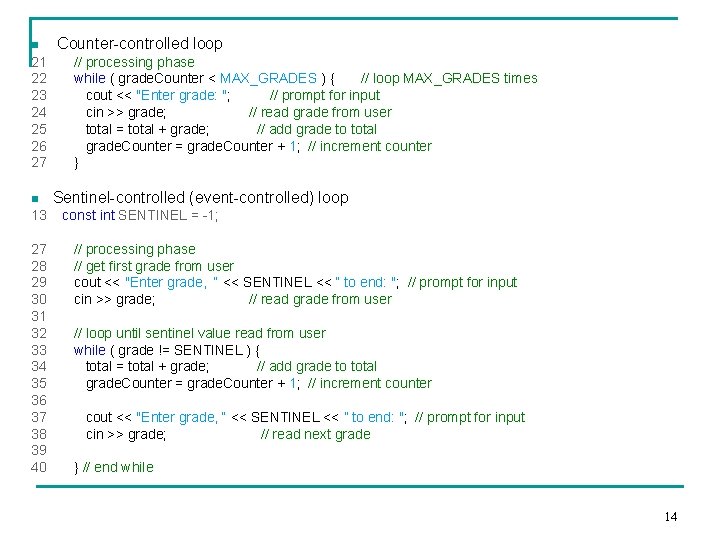
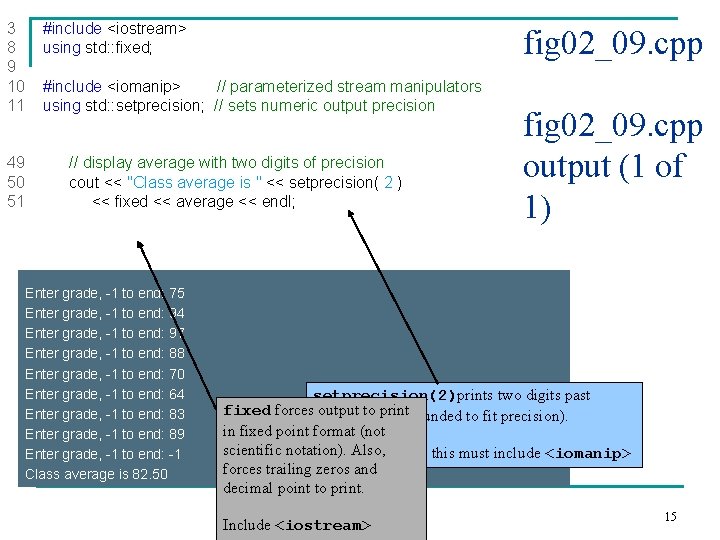
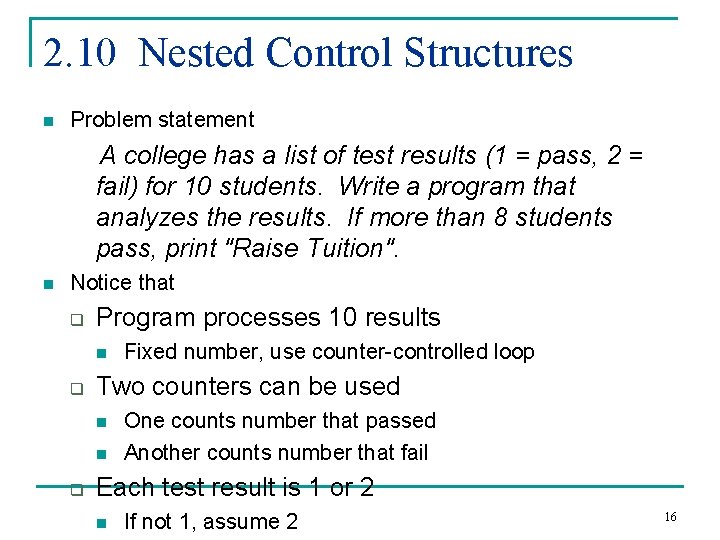
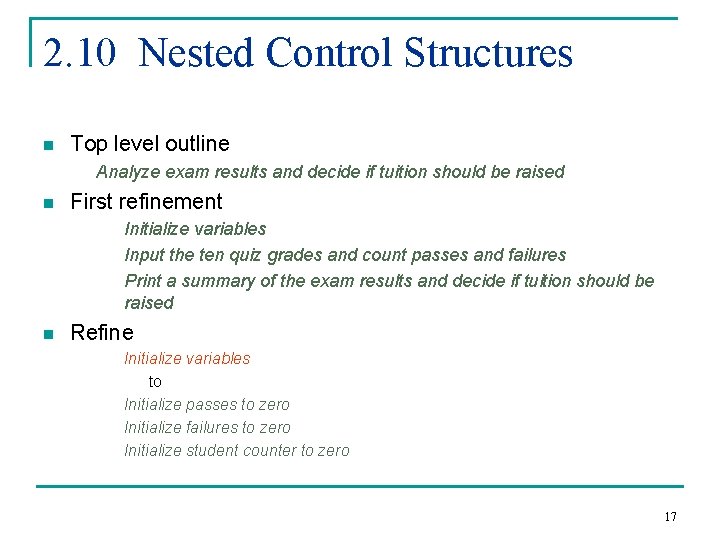
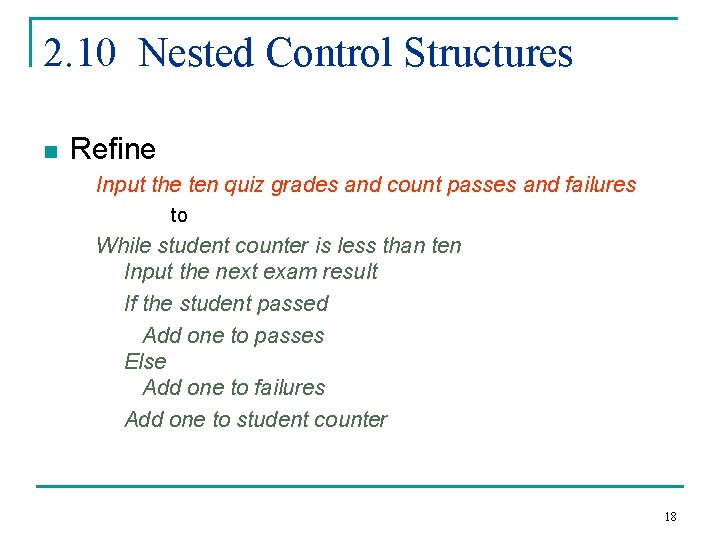
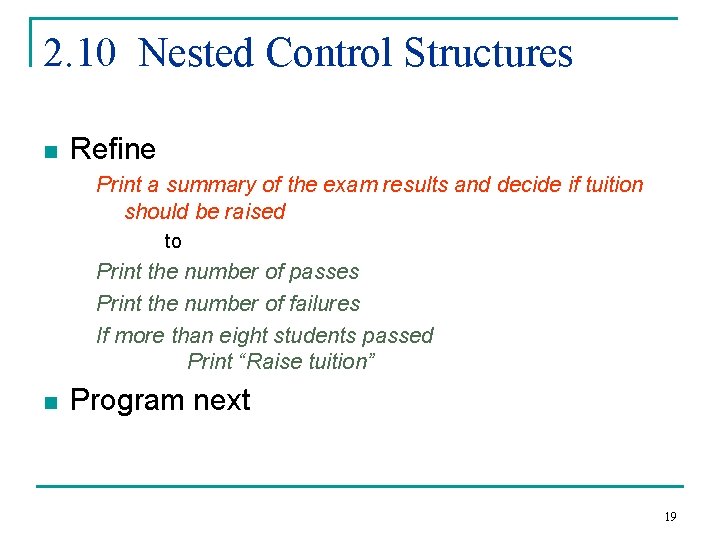
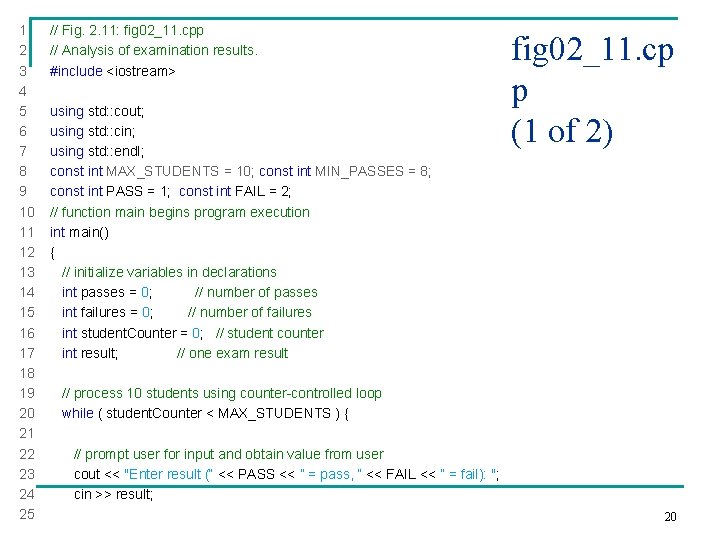
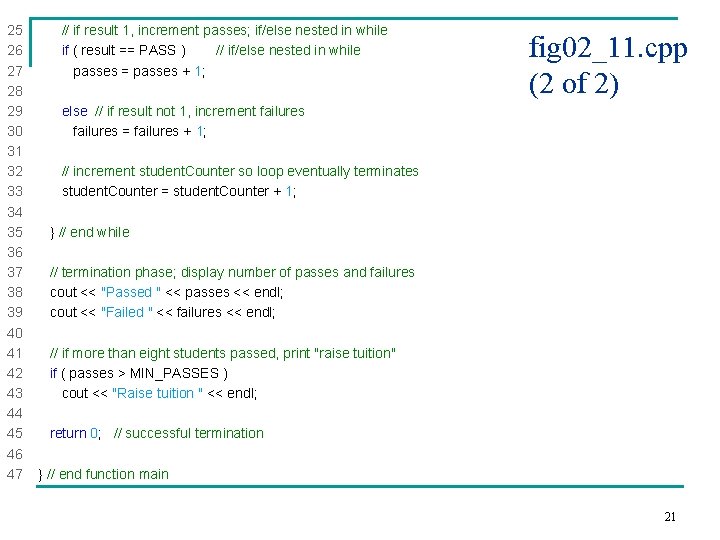
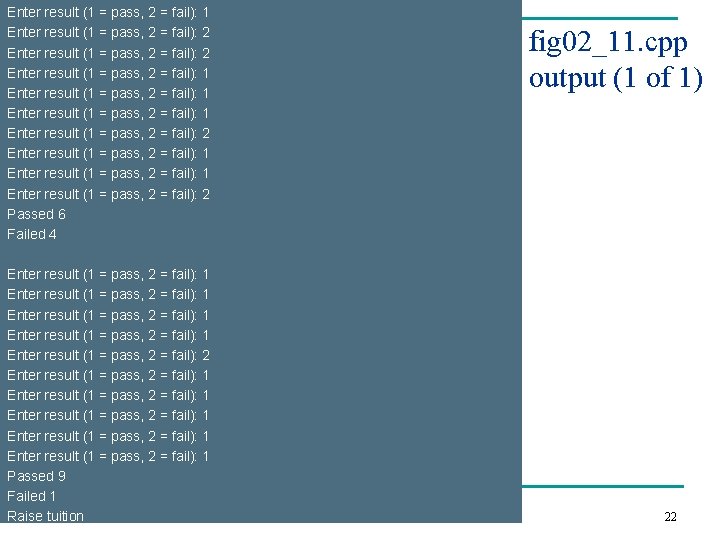
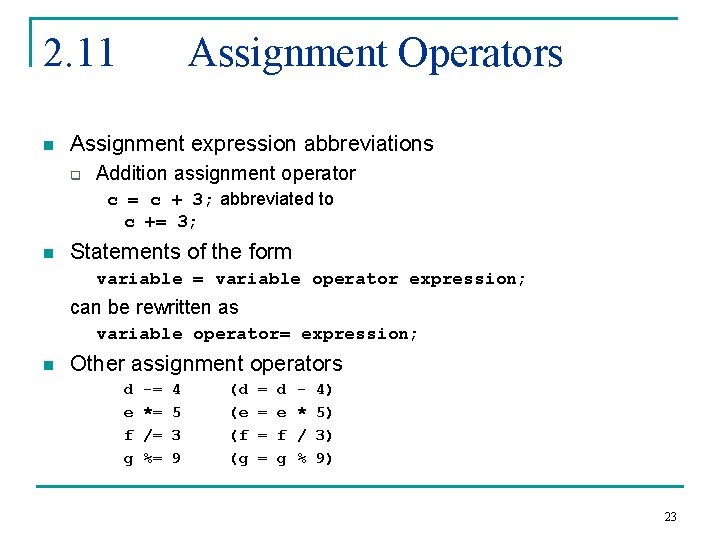
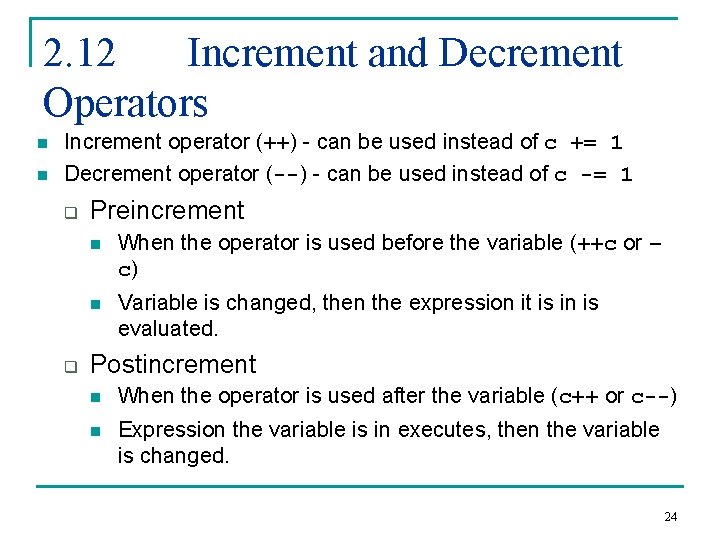
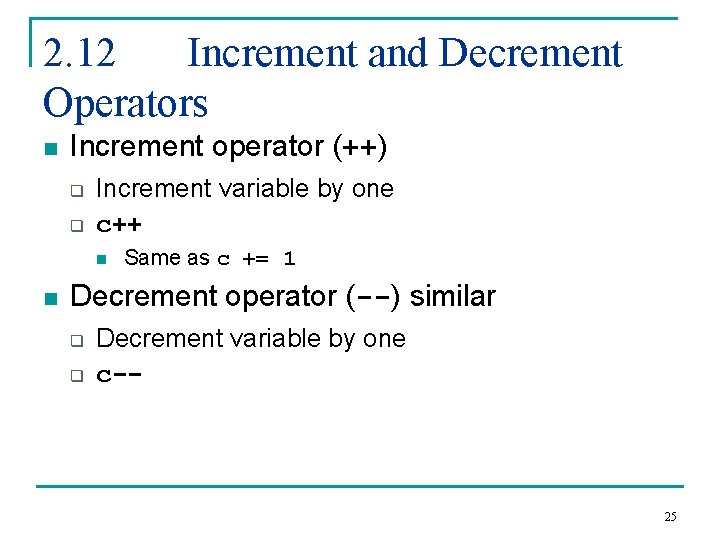
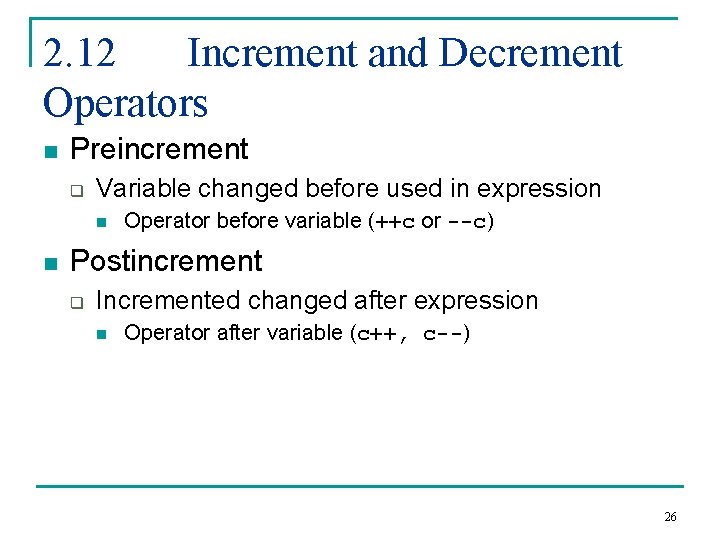
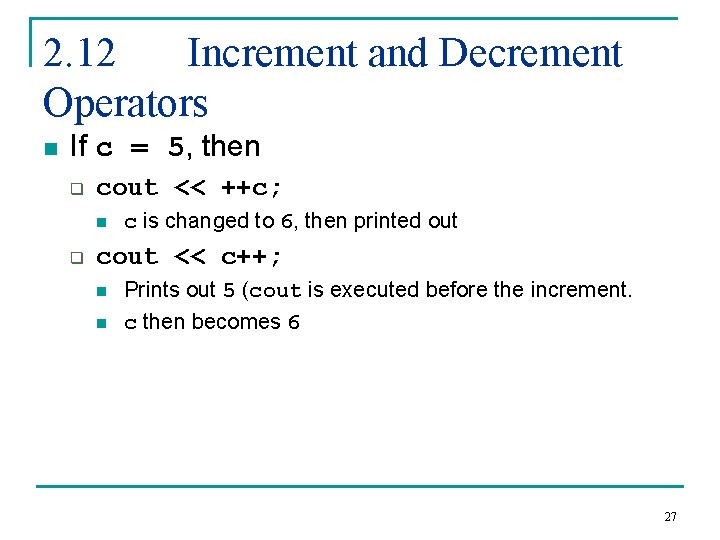
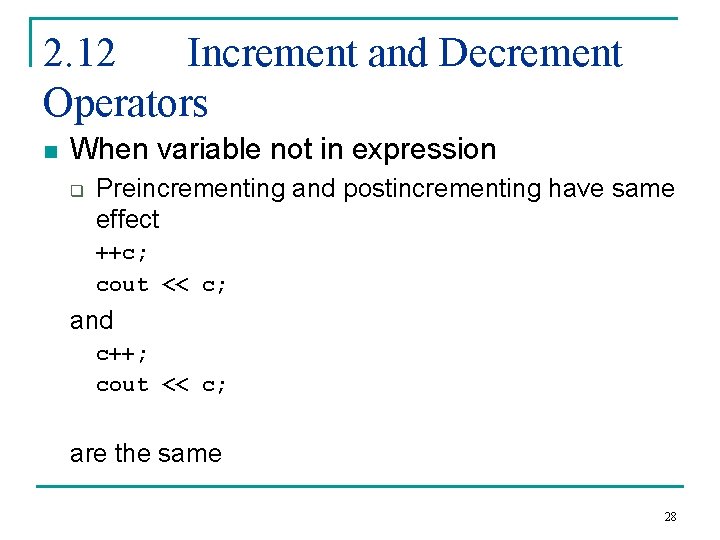
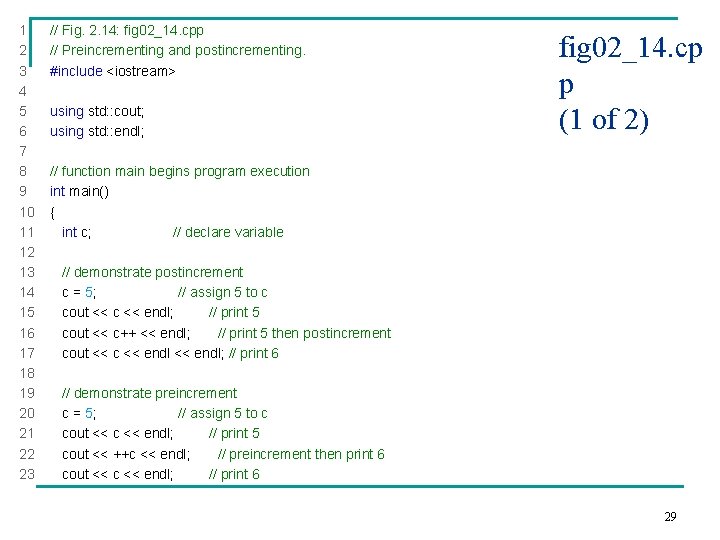
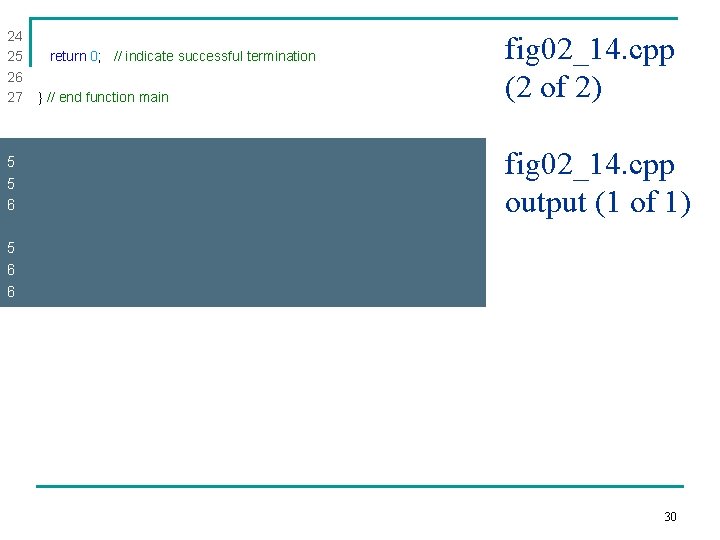
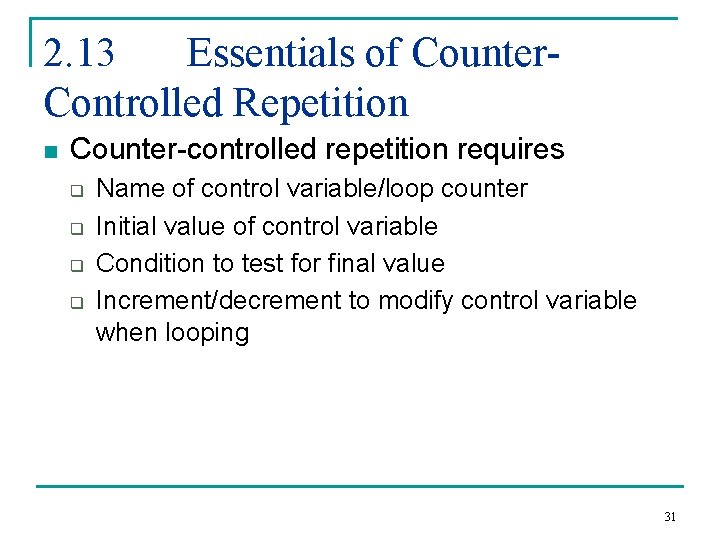
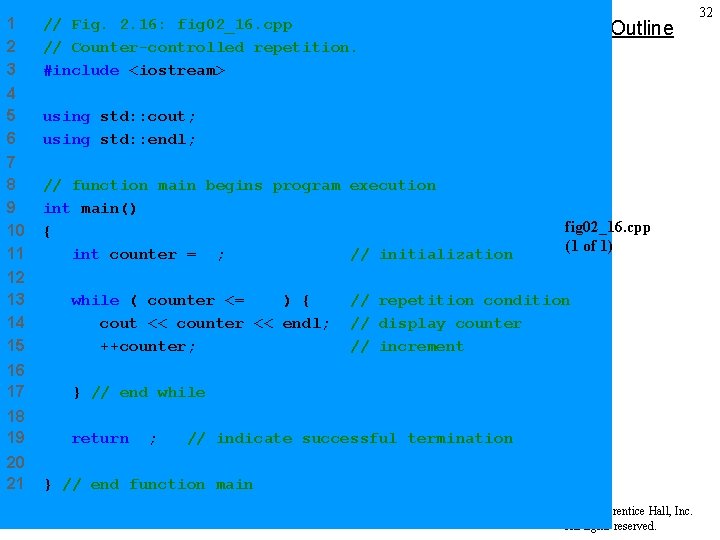
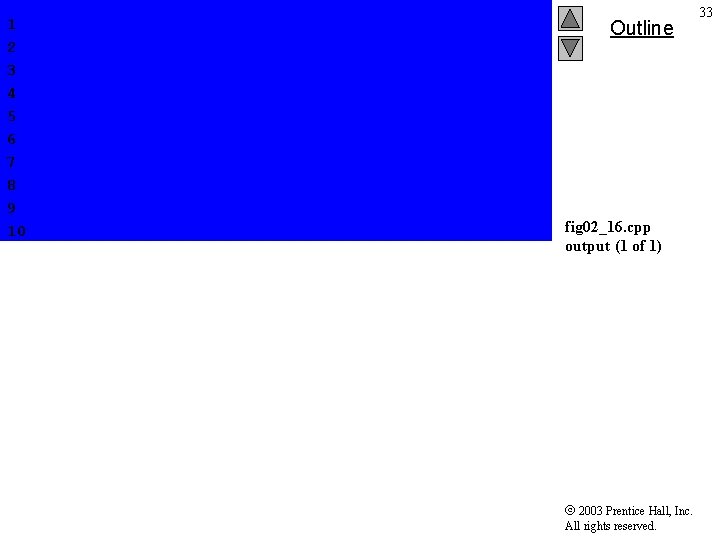
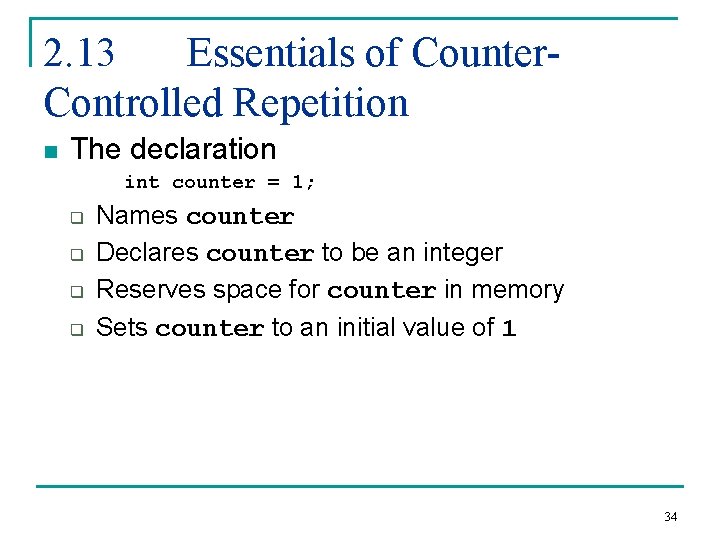
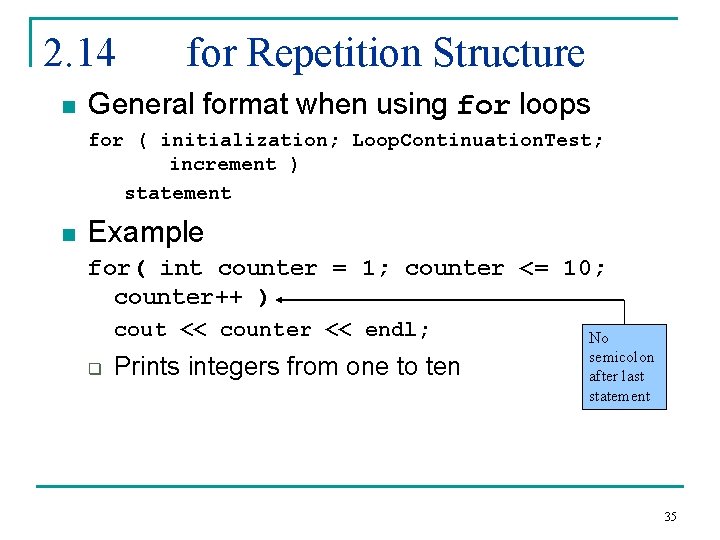
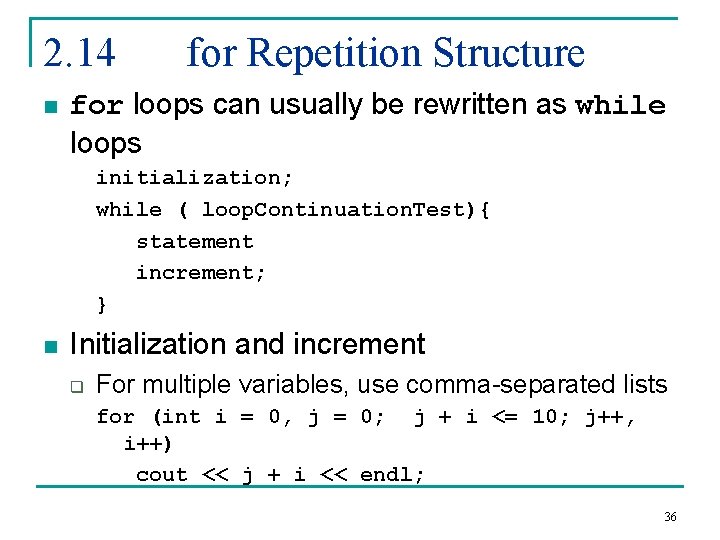
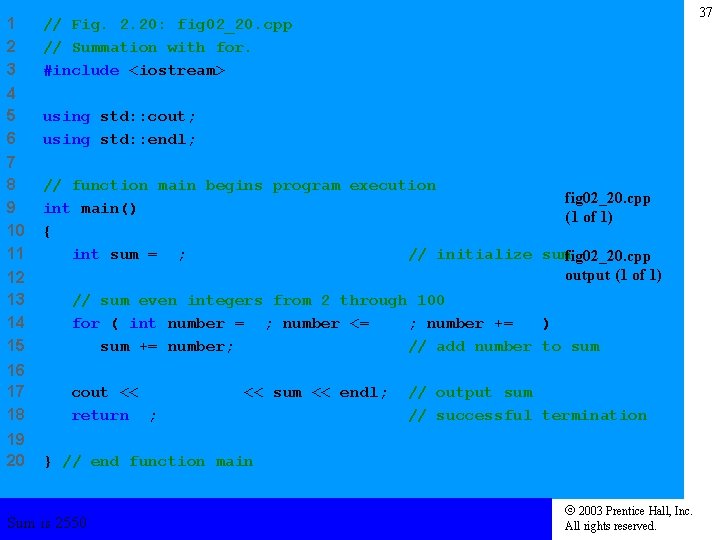
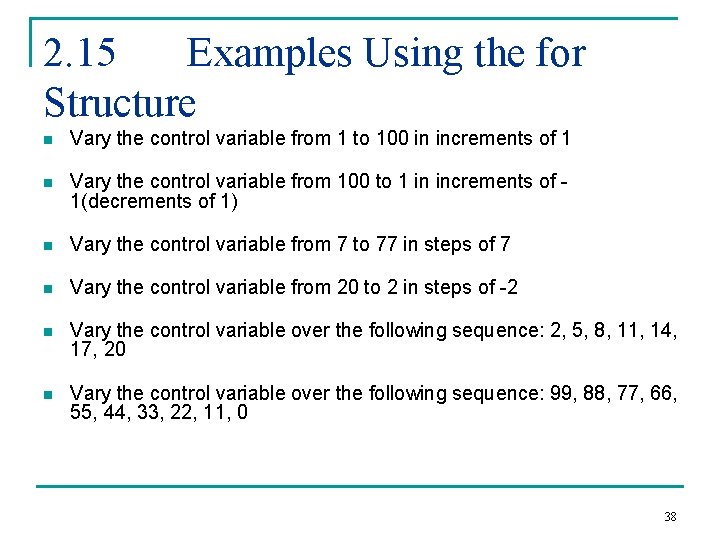
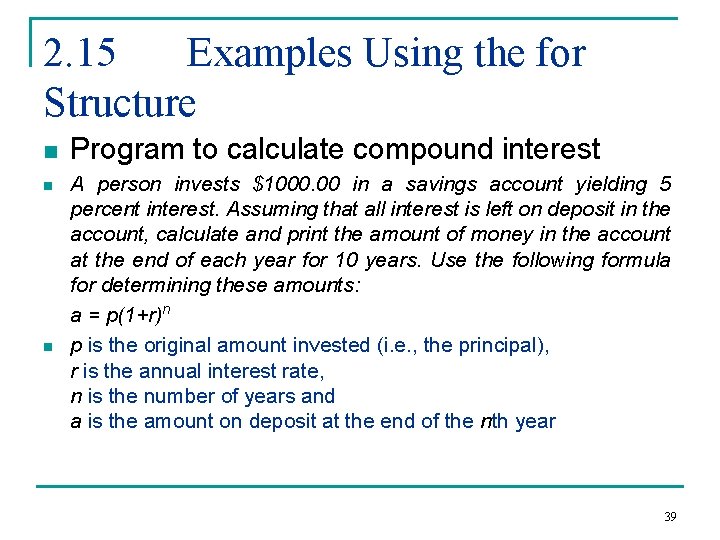
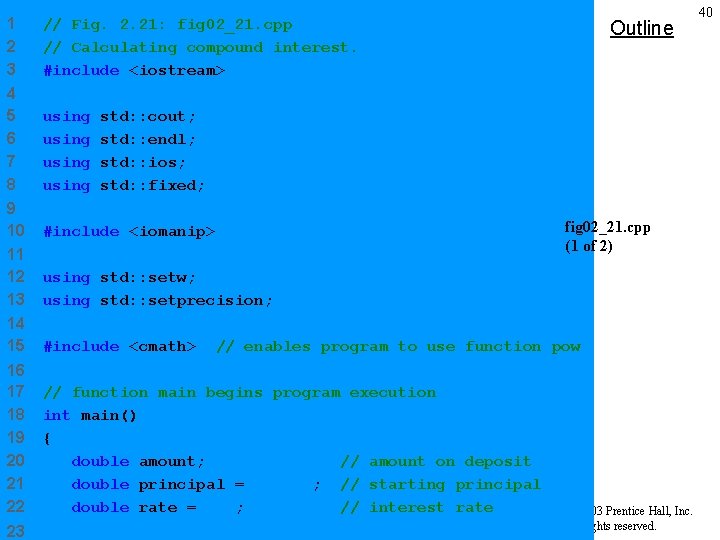
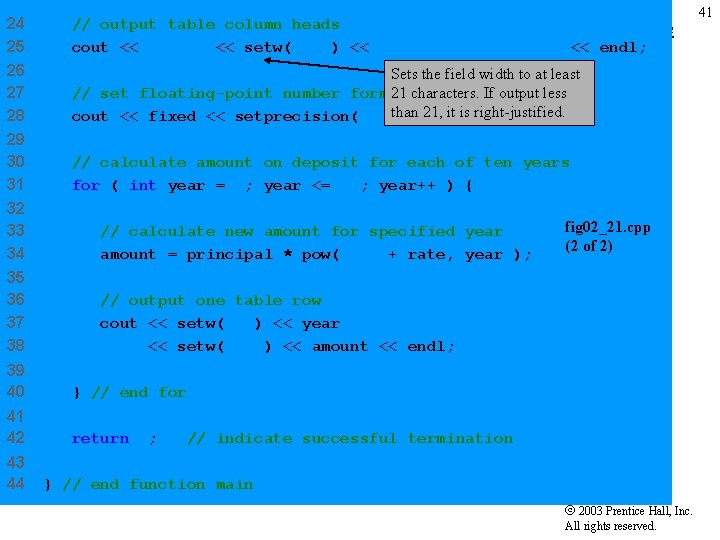
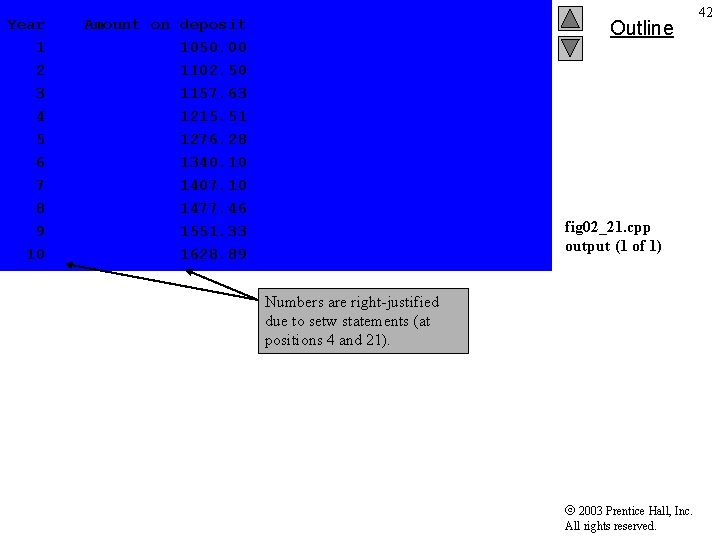
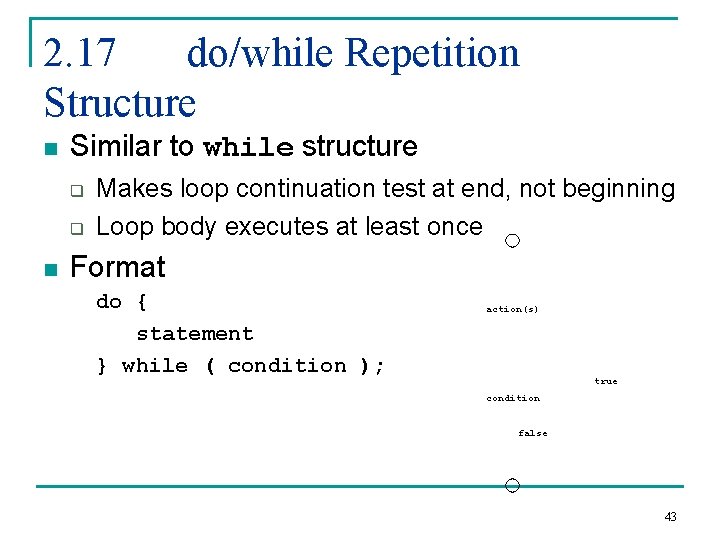
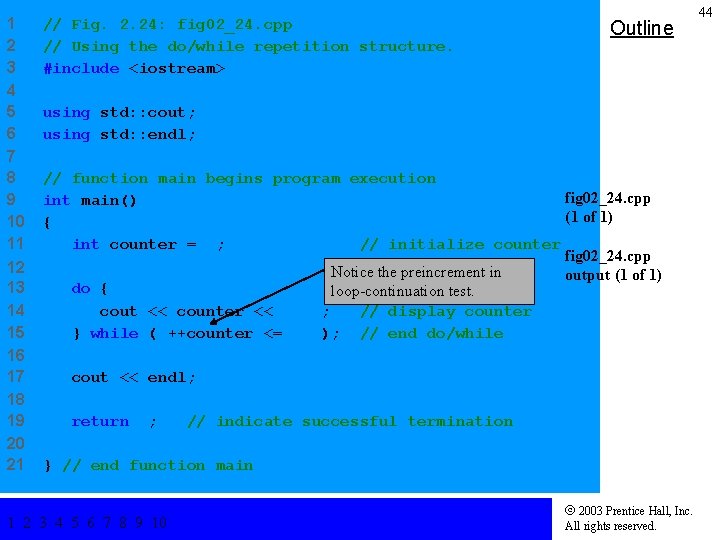
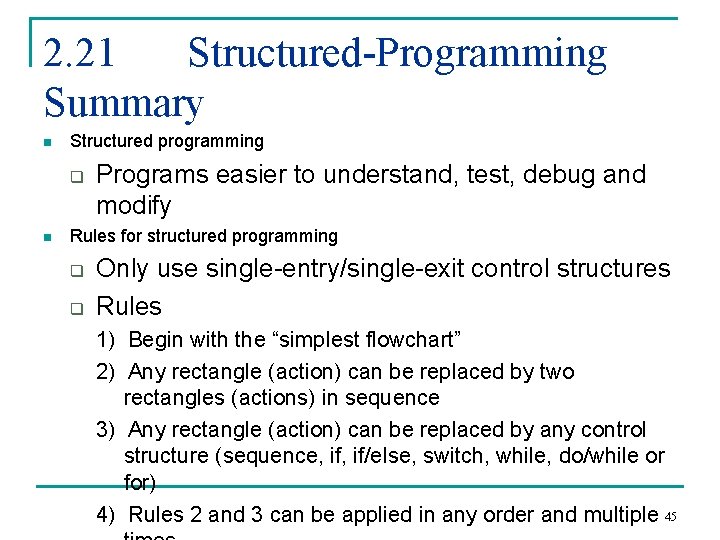
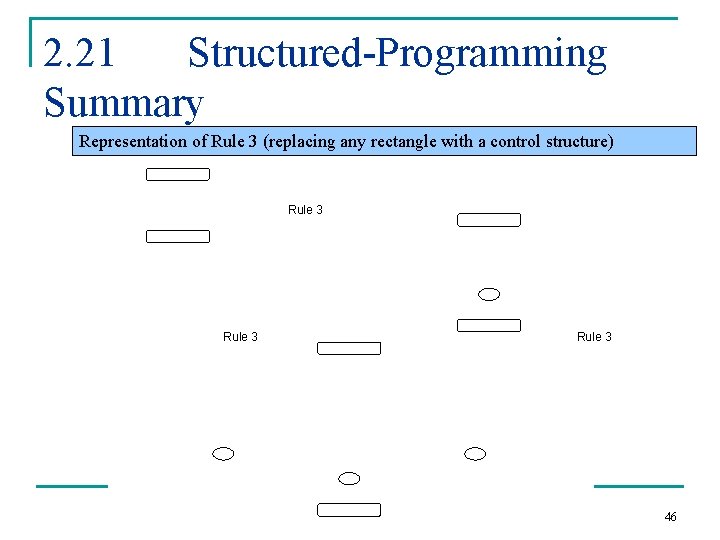
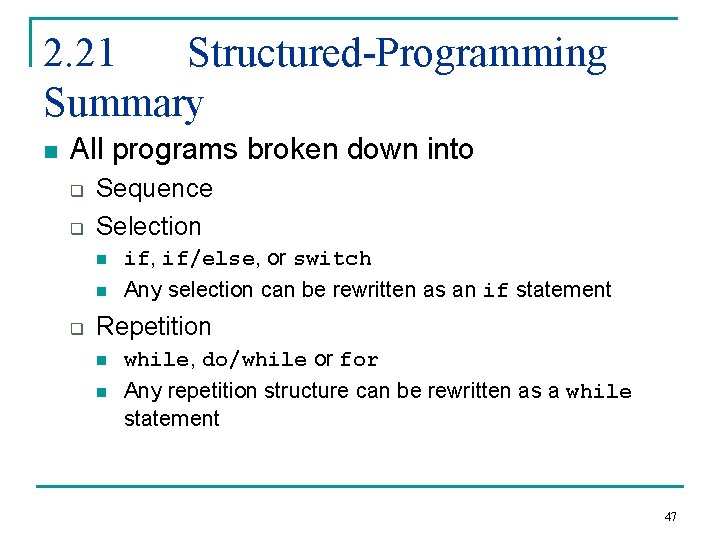
- Slides: 47
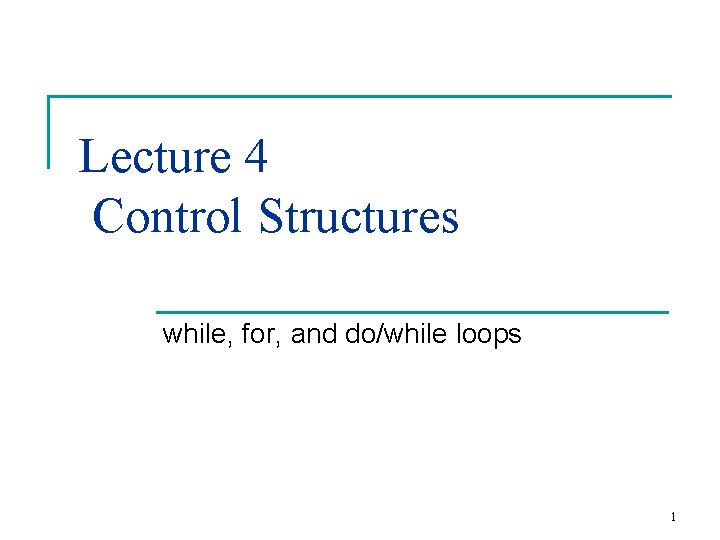
Lecture 4 Control Structures while, for, and do/while loops 1
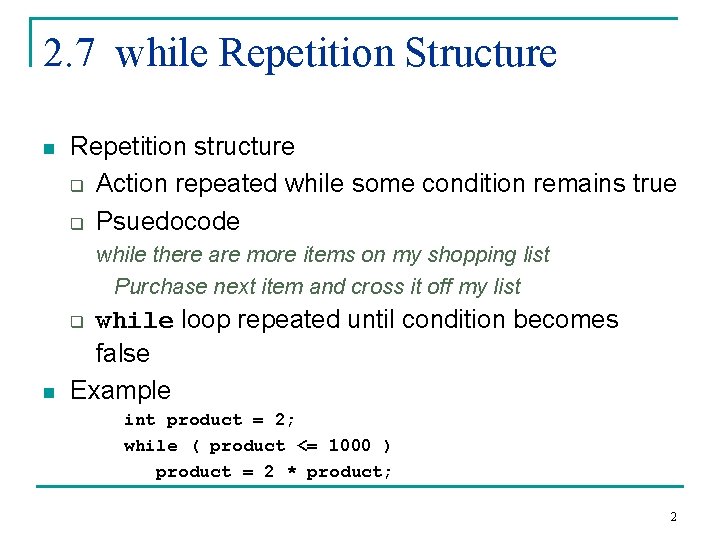
2. 7 while Repetition Structure n Repetition structure q Action repeated while some condition remains true q Psuedocode while there are more items on my shopping list Purchase next item and cross it off my list while loop repeated until condition becomes false Example q n int product = 2; while ( product <= 1000 ) product = 2 * product; 2
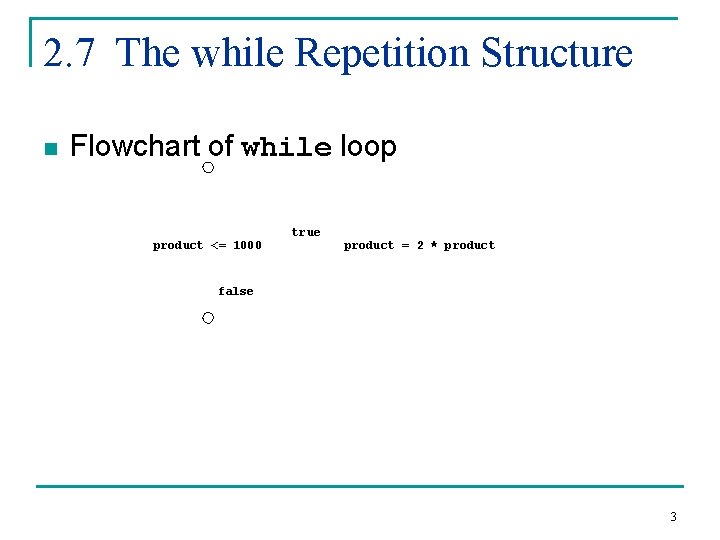
2. 7 The while Repetition Structure n Flowchart of while loop product <= 1000 true product = 2 * product false 3
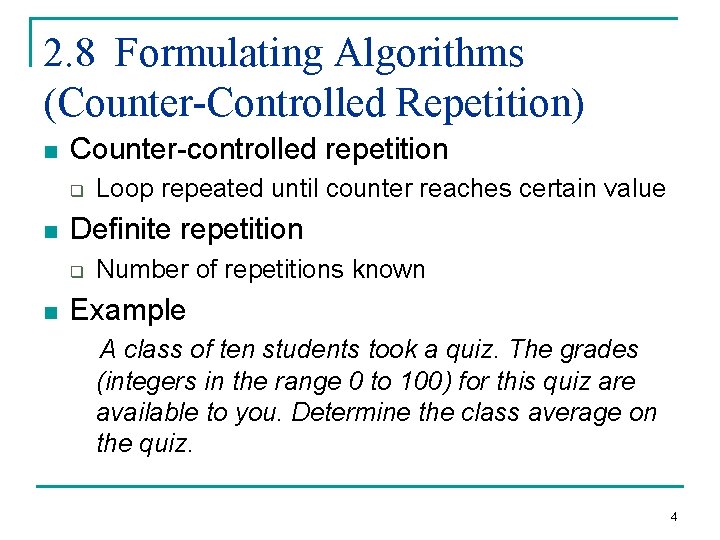
2. 8 Formulating Algorithms (Counter-Controlled Repetition) n Counter-controlled repetition q n Definite repetition q n Loop repeated until counter reaches certain value Number of repetitions known Example A class of ten students took a quiz. The grades (integers in the range 0 to 100) for this quiz are available to you. Determine the class average on the quiz. 4
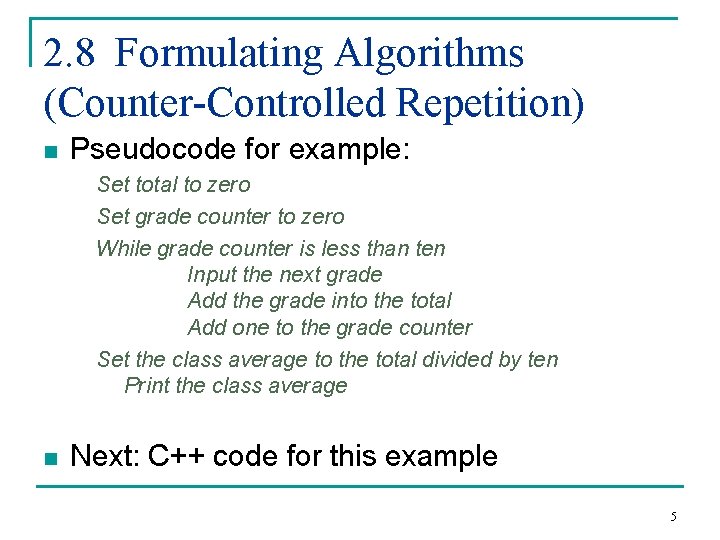
2. 8 Formulating Algorithms (Counter-Controlled Repetition) n Pseudocode for example: Set total to zero Set grade counter to zero While grade counter is less than ten Input the next grade Add the grade into the total Add one to the grade counter Set the class average to the total divided by ten Print the class average n Next: C++ code for this example 5
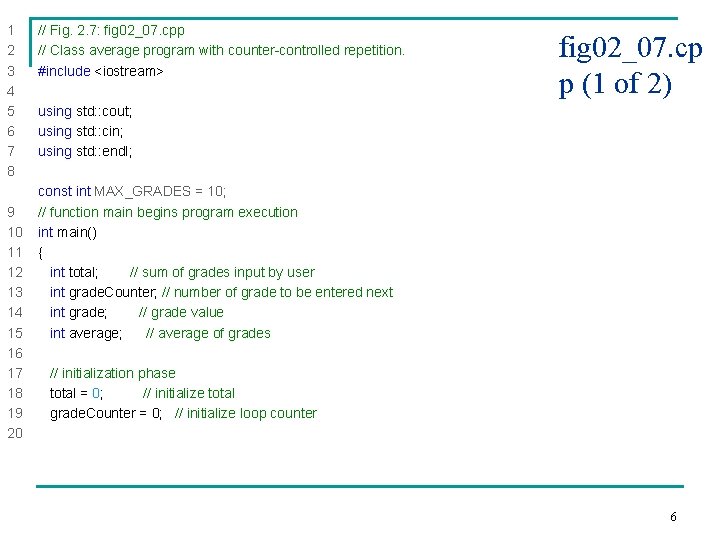
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 // Fig. 2. 7: fig 02_07. cpp // Class average program with counter-controlled repetition. #include <iostream> fig 02_07. cp p (1 of 2) using std: : cout; using std: : cin; using std: : endl; const int MAX_GRADES = 10; // function main begins program execution int main() { int total; // sum of grades input by user int grade. Counter; // number of grade to be entered next int grade; // grade value int average; // average of grades // initialization phase total = 0; // initialize total grade. Counter = 0; // initialize loop counter 6
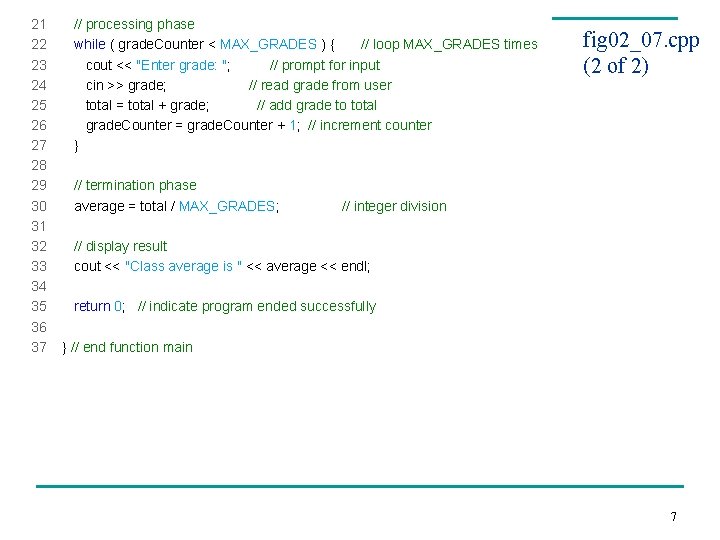
21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 // processing phase while ( grade. Counter < MAX_GRADES ) { // loop MAX_GRADES times cout << "Enter grade: "; // prompt for input cin >> grade; // read grade from user total = total + grade; // add grade to total grade. Counter = grade. Counter + 1; // increment counter } // termination phase average = total / MAX_GRADES; fig 02_07. cpp (2 of 2) // integer division // display result cout << "Class average is " << average << endl; return 0; // indicate program ended successfully } // end function main 7
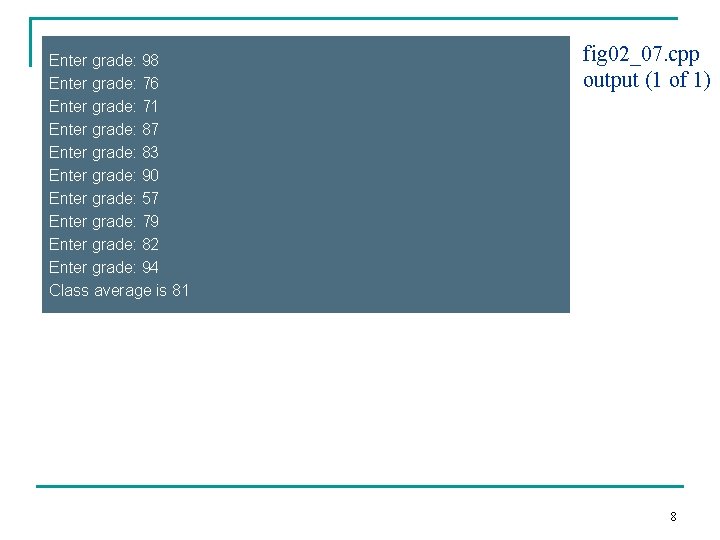
Enter grade: 98 Enter grade: 76 Enter grade: 71 Enter grade: 87 Enter grade: 83 Enter grade: 90 Enter grade: 57 Enter grade: 79 Enter grade: 82 Enter grade: 94 Class average is 81 fig 02_07. cpp output (1 of 1) 8
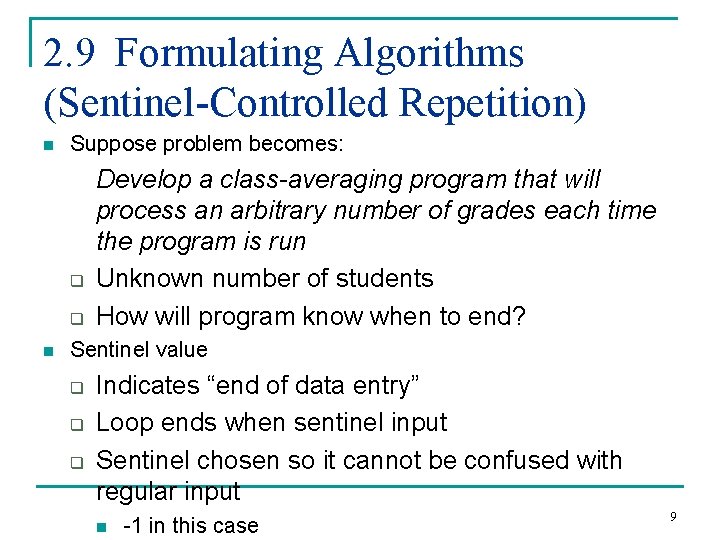
2. 9 Formulating Algorithms (Sentinel-Controlled Repetition) n Suppose problem becomes: q q n Develop a class-averaging program that will process an arbitrary number of grades each time the program is run Unknown number of students How will program know when to end? Sentinel value q q q Indicates “end of data entry” Loop ends when sentinel input Sentinel chosen so it cannot be confused with regular input n -1 in this case 9
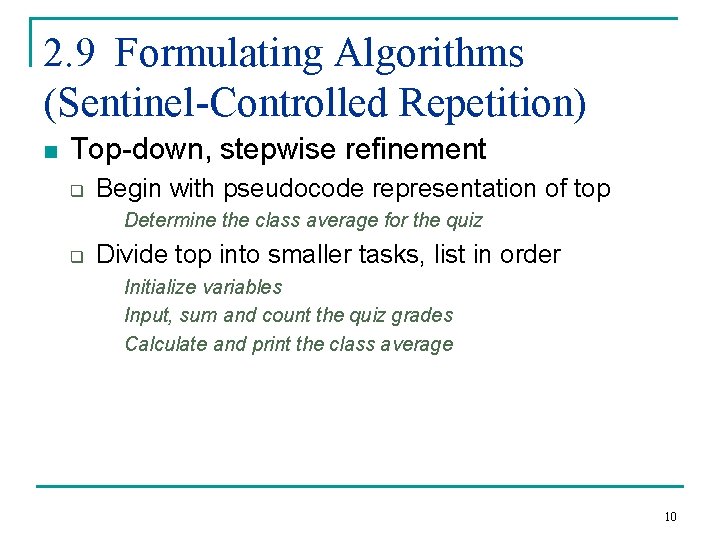
2. 9 Formulating Algorithms (Sentinel-Controlled Repetition) n Top-down, stepwise refinement q Begin with pseudocode representation of top Determine the class average for the quiz q Divide top into smaller tasks, list in order Initialize variables Input, sum and count the quiz grades Calculate and print the class average 10
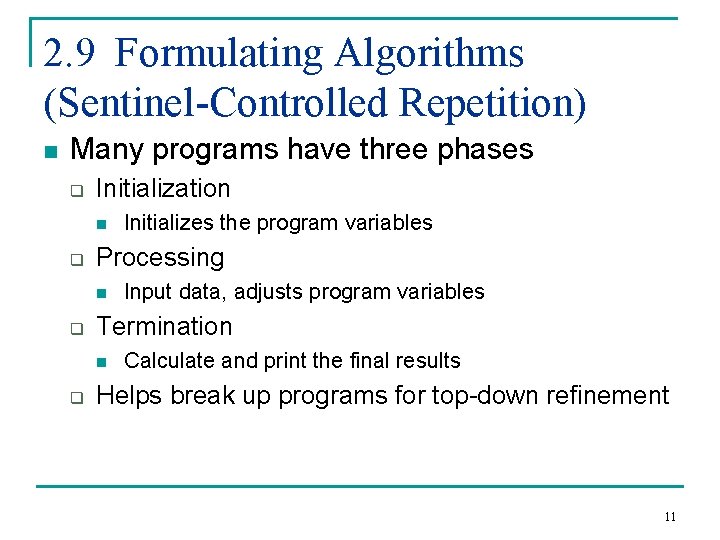
2. 9 Formulating Algorithms (Sentinel-Controlled Repetition) n Many programs have three phases q Initialization n q Processing n q Input data, adjusts program variables Termination n q Initializes the program variables Calculate and print the final results Helps break up programs for top-down refinement 11
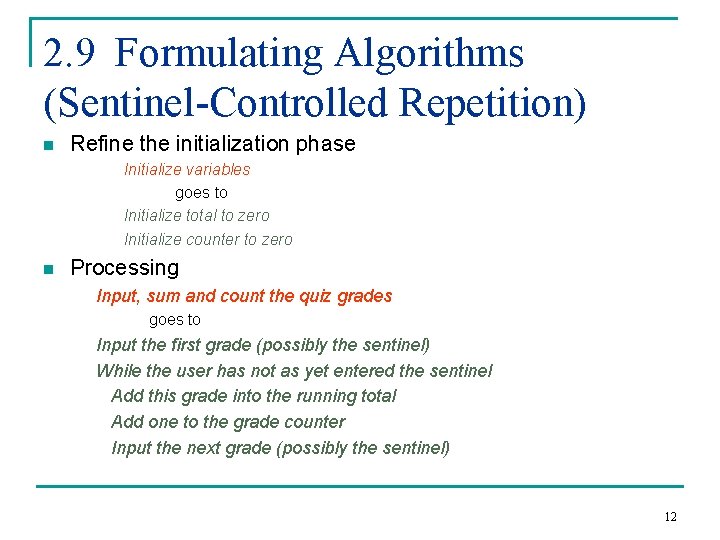
2. 9 Formulating Algorithms (Sentinel-Controlled Repetition) n Refine the initialization phase Initialize variables goes to Initialize total to zero Initialize counter to zero n Processing Input, sum and count the quiz grades goes to Input the first grade (possibly the sentinel) While the user has not as yet entered the sentinel Add this grade into the running total Add one to the grade counter Input the next grade (possibly the sentinel) 12
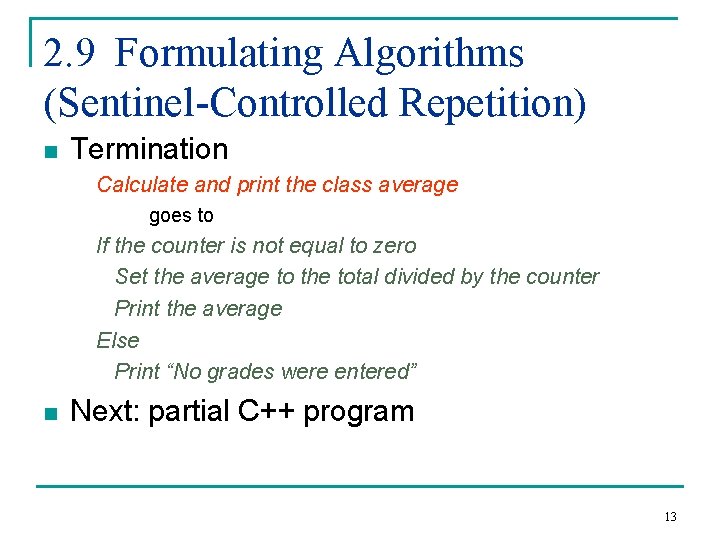
2. 9 Formulating Algorithms (Sentinel-Controlled Repetition) n Termination Calculate and print the class average goes to If the counter is not equal to zero Set the average to the total divided by the counter Print the average Else Print “No grades were entered” n Next: partial C++ program 13
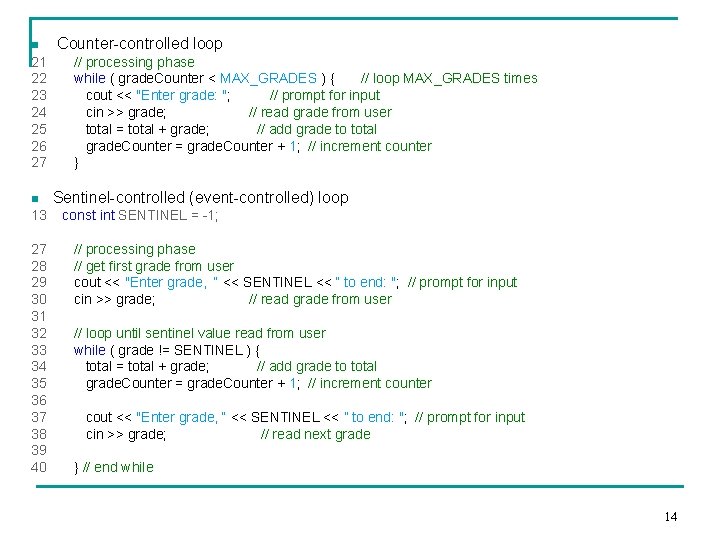
n 21 22 23 24 25 26 27 n 13 27 28 29 30 31 32 33 34 35 36 37 38 39 40 Counter-controlled loop // processing phase while ( grade. Counter < MAX_GRADES ) { // loop MAX_GRADES times cout << "Enter grade: "; // prompt for input cin >> grade; // read grade from user total = total + grade; // add grade to total grade. Counter = grade. Counter + 1; // increment counter } Sentinel-controlled (event-controlled) loop const int SENTINEL = -1; // processing phase // get first grade from user cout << "Enter grade, “ << SENTINEL << “ to end: "; // prompt for input cin >> grade; // read grade from user // loop until sentinel value read from user while ( grade != SENTINEL ) { total = total + grade; // add grade to total grade. Counter = grade. Counter + 1; // increment counter cout << "Enter grade, “ << SENTINEL << ” to end: "; // prompt for input cin >> grade; // read next grade } // end while 14
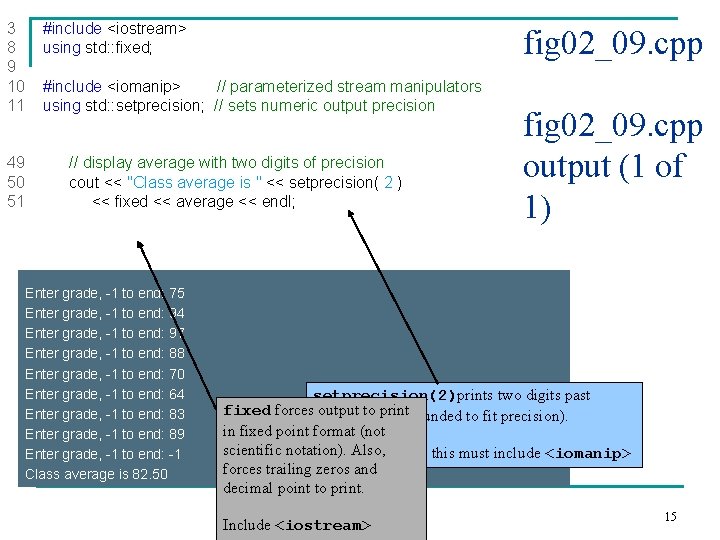
3 8 9 10 11 49 50 51 #include <iostream> using std: : fixed; fig 02_09. cpp #include <iomanip> // parameterized stream manipulators using std: : setprecision; // sets numeric output precision // display average with two digits of precision cout << "Class average is " << setprecision( 2 ) << fixed << average << endl; Enter grade, -1 to end: 75 Enter grade, -1 to end: 94 Enter grade, -1 to end: 97 Enter grade, -1 to end: 88 Enter grade, -1 to end: 70 Enter grade, -1 to end: 64 Enter grade, -1 to end: 83 Enter grade, -1 to end: 89 Enter grade, -1 to end: -1 Class average is 82. 50 fig 02_09. cpp output (1 of 1) setprecision(2)prints two digits past fixed forces output to print decimal point (rounded to fit precision). in fixed point format (not scientific notation). Also, Programs that use this must include <iomanip> forces trailing zeros and decimal point to print. Include <iostream> 15
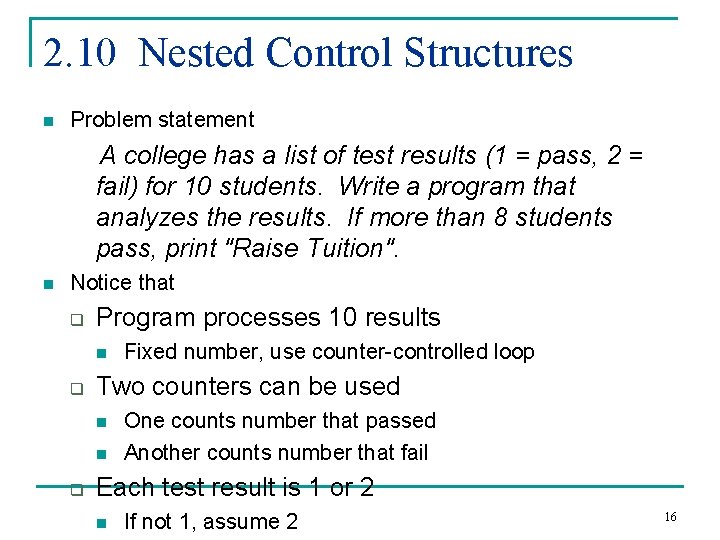
2. 10 Nested Control Structures n Problem statement A college has a list of test results (1 = pass, 2 = fail) for 10 students. Write a program that analyzes the results. If more than 8 students pass, print "Raise Tuition". n Notice that q Program processes 10 results n q Two counters can be used n n q Fixed number, use counter-controlled loop One counts number that passed Another counts number that fail Each test result is 1 or 2 n If not 1, assume 2 16
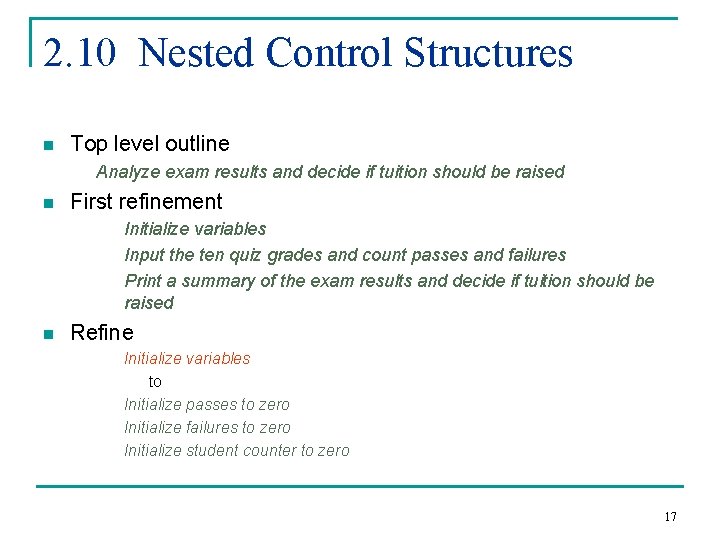
2. 10 Nested Control Structures n Top level outline Analyze exam results and decide if tuition should be raised n First refinement Initialize variables Input the ten quiz grades and count passes and failures Print a summary of the exam results and decide if tuition should be raised n Refine Initialize variables to Initialize passes to zero Initialize failures to zero Initialize student counter to zero 17
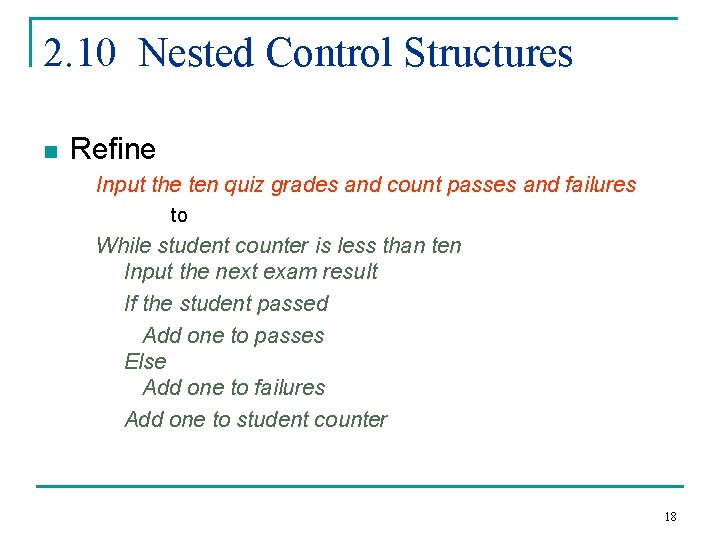
2. 10 Nested Control Structures n Refine Input the ten quiz grades and count passes and failures to While student counter is less than ten Input the next exam result If the student passed Add one to passes Else Add one to failures Add one to student counter 18
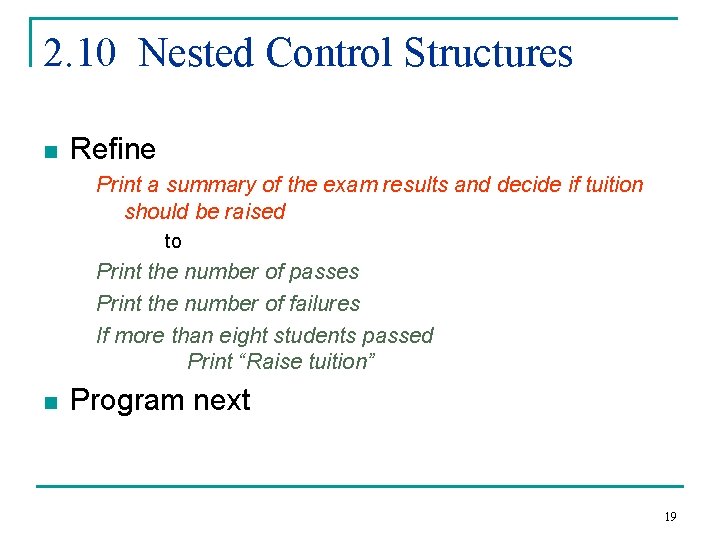
2. 10 Nested Control Structures n Refine Print a summary of the exam results and decide if tuition should be raised to Print the number of passes Print the number of failures If more than eight students passed Print “Raise tuition” n Program next 19
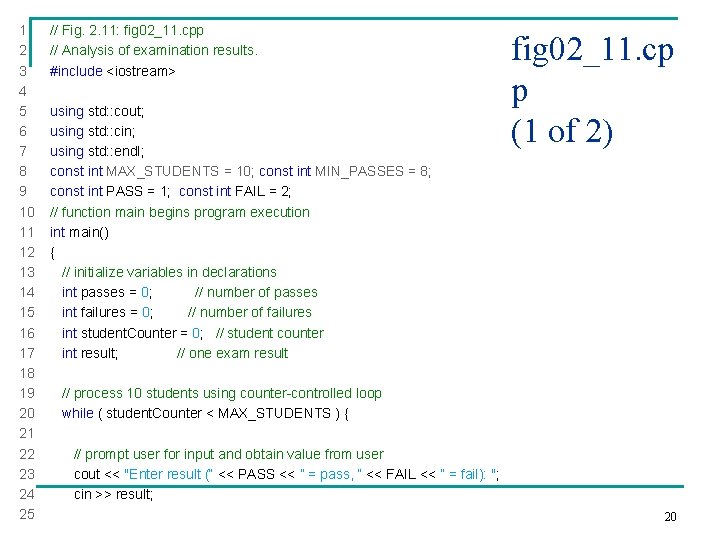
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 // Fig. 2. 11: fig 02_11. cpp // Analysis of examination results. #include <iostream> using std: : cout; using std: : cin; using std: : endl; const int MAX_STUDENTS = 10; const int MIN_PASSES = 8; const int PASS = 1; const int FAIL = 2; // function main begins program execution int main() { // initialize variables in declarations int passes = 0; // number of passes int failures = 0; // number of failures int student. Counter = 0; // student counter int result; // one exam result fig 02_11. cp p (1 of 2) // process 10 students using counter-controlled loop while ( student. Counter < MAX_STUDENTS ) { // prompt user for input and obtain value from user cout << "Enter result (“ << PASS << ” = pass, “ << FAIL << ” = fail): "; cin >> result; 20
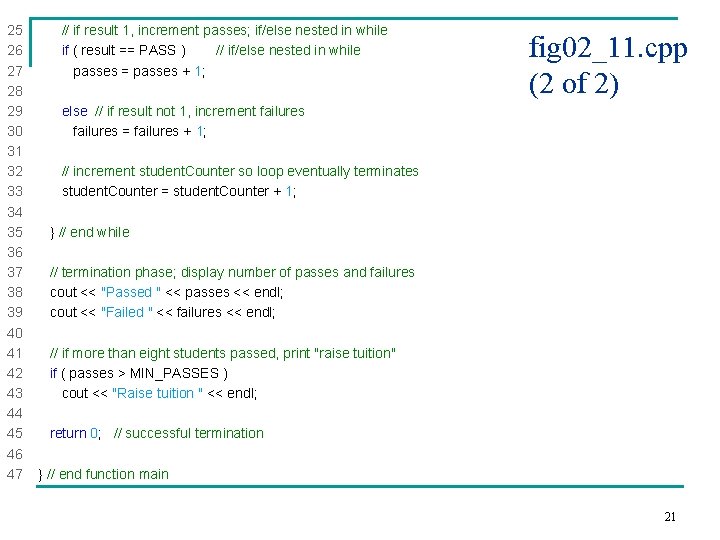
25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 // if result 1, increment passes; if/else nested in while if ( result == PASS ) // if/else nested in while passes = passes + 1; fig 02_11. cpp (2 of 2) else // if result not 1, increment failures = failures + 1; // increment student. Counter so loop eventually terminates student. Counter = student. Counter + 1; } // end while // termination phase; display number of passes and failures cout << "Passed " << passes << endl; cout << "Failed " << failures << endl; // if more than eight students passed, print "raise tuition" if ( passes > MIN_PASSES ) cout << "Raise tuition " << endl; return 0; // successful termination } // end function main 21
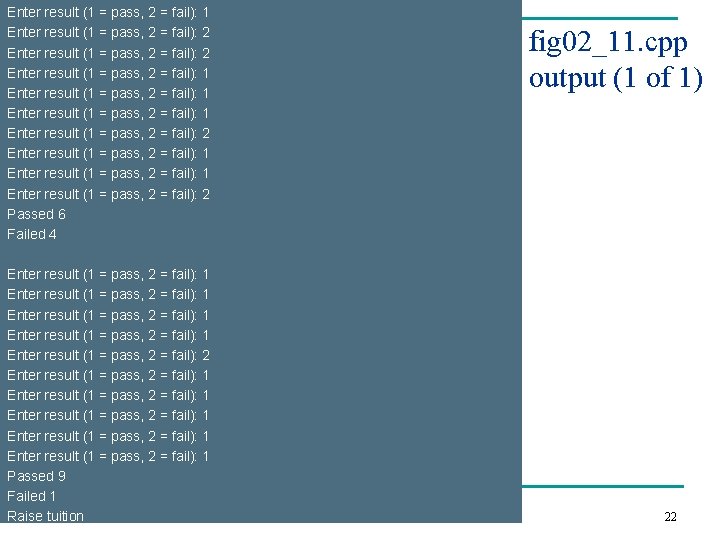
Enter result (1 = pass, 2 = fail): 1 Enter result (1 = pass, 2 = fail): 2 Enter result (1 = pass, 2 = fail): 1 Enter result (1 = pass, 2 = fail): 2 Passed 6 Failed 4 Enter result (1 = pass, 2 = fail): 1 Enter result (1 = pass, 2 = fail): 2 Enter result (1 = pass, 2 = fail): 1 Enter result (1 = pass, 2 = fail): 1 Passed 9 Failed 1 Raise tuition fig 02_11. cpp output (1 of 1) 22
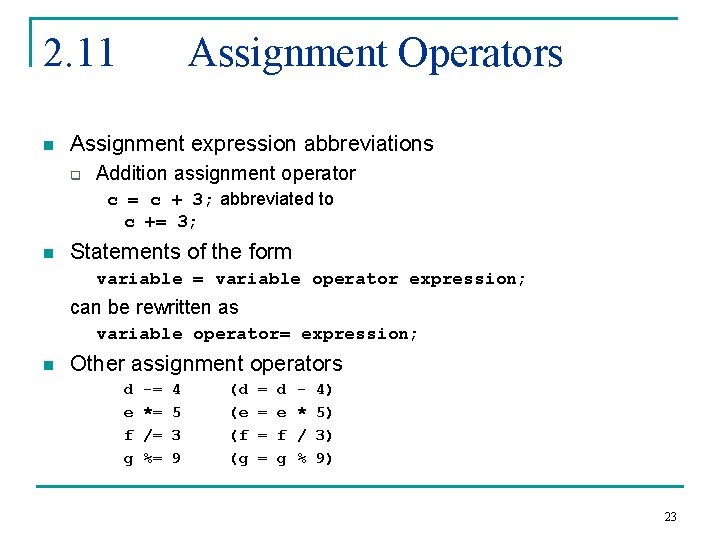
2. 11 n Assignment Operators Assignment expression abbreviations q Addition assignment operator c = c + 3; abbreviated to c += 3; n Statements of the form variable = variable operator expression; can be rewritten as variable operator= expression; n Other assignment operators d e f g -= *= /= %= 4 5 3 9 (d (e (f (g = = d e f g * / % 4) 5) 3) 9) 23
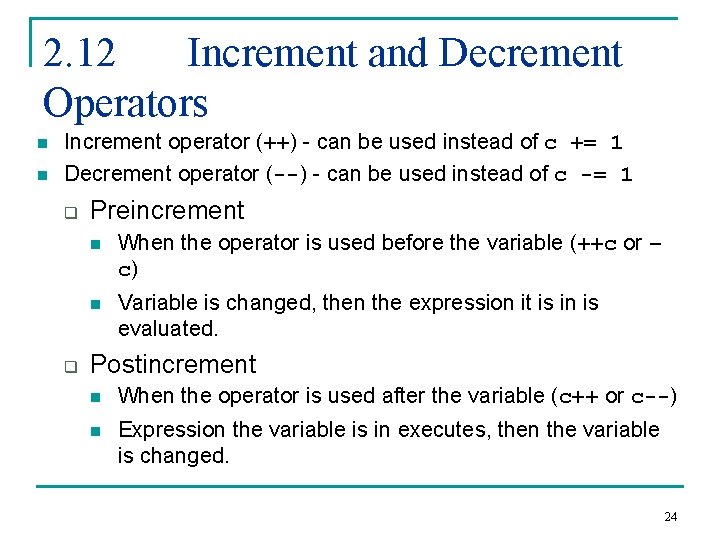
2. 12 Increment and Decrement Operators n n Increment operator (++) - can be used instead of c += 1 Decrement operator (--) - can be used instead of c -= 1 q q Preincrement n When the operator is used before the variable (++c or – c) n Variable is changed, then the expression it is in is evaluated. Postincrement n When the operator is used after the variable (c++ or c--) n Expression the variable is in executes, then the variable is changed. 24
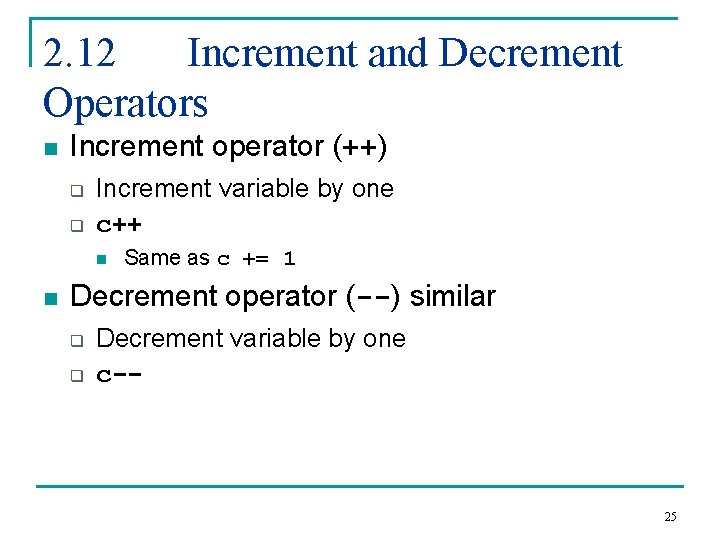
2. 12 Increment and Decrement Operators n Increment operator (++) q q Increment variable by one c++ n n Same as c += 1 Decrement operator (--) similar q q Decrement variable by one c-- 25
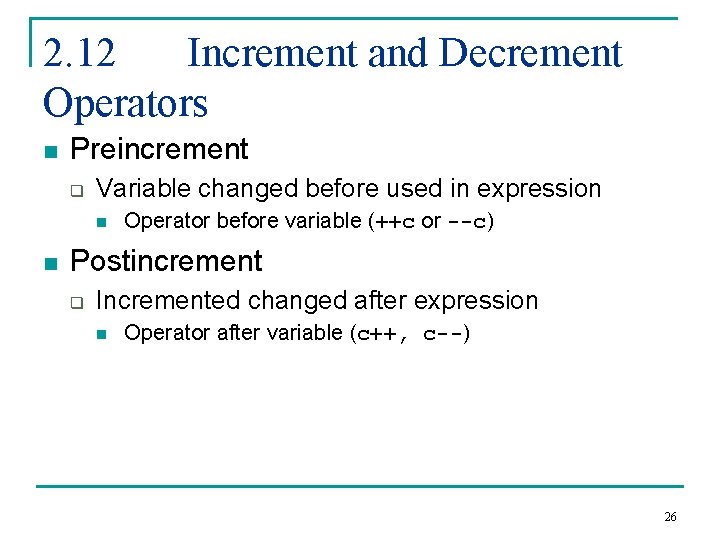
2. 12 Increment and Decrement Operators n Preincrement q Variable changed before used in expression n n Operator before variable (++c or --c) Postincrement q Incremented changed after expression n Operator after variable (c++, c--) 26
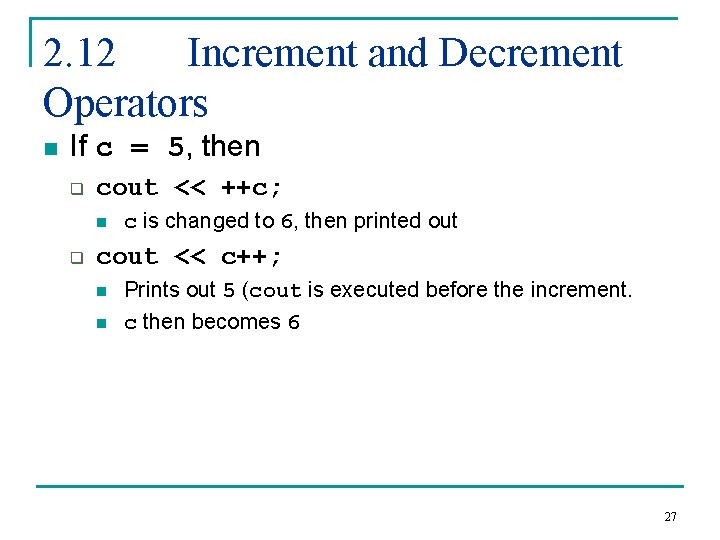
2. 12 Increment and Decrement Operators n If c = 5, then q cout << ++c; n q c is changed to 6, then printed out cout << c++; n n Prints out 5 (cout is executed before the increment. c then becomes 6 27
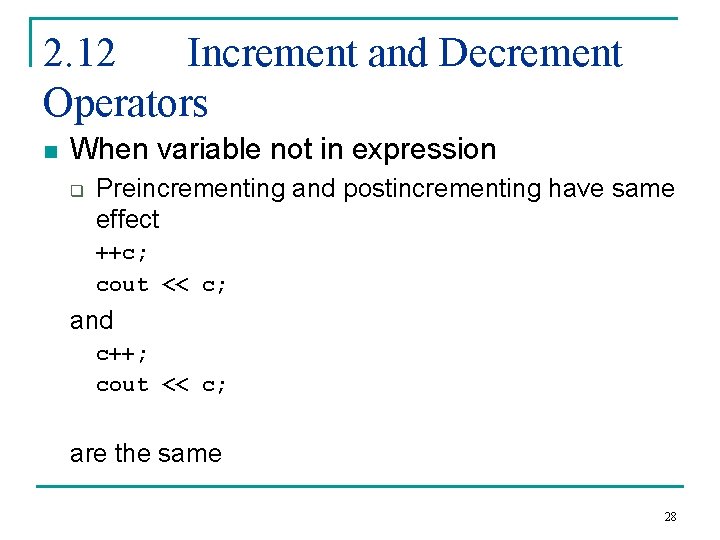
2. 12 Increment and Decrement Operators n When variable not in expression q Preincrementing and postincrementing have same effect ++c; cout << c; and c++; cout << c; are the same 28
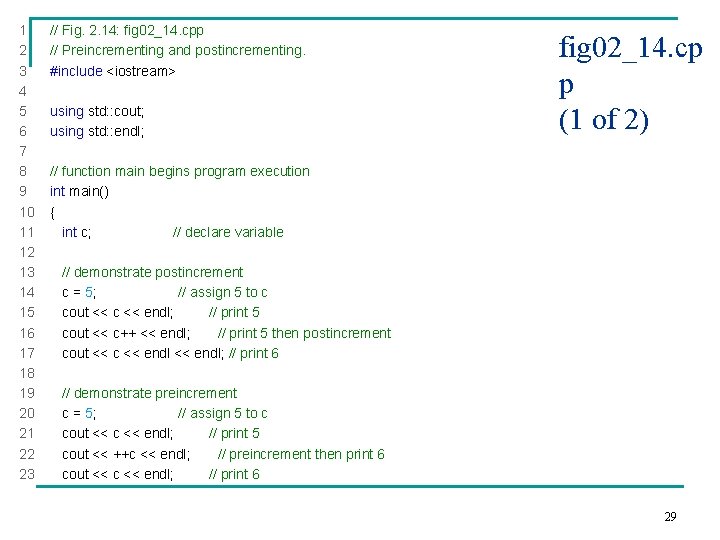
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 // Fig. 2. 14: fig 02_14. cpp // Preincrementing and postincrementing. #include <iostream> using std: : cout; using std: : endl; fig 02_14. cp p (1 of 2) // function main begins program execution int main() { int c; // declare variable // demonstrate postincrement c = 5; // assign 5 to c cout << c << endl; // print 5 cout << c++ << endl; // print 5 then postincrement cout << c << endl; // print 6 // demonstrate preincrement c = 5; // assign 5 to c cout << c << endl; // print 5 cout << ++c << endl; // preincrement then print 6 cout << c << endl; // print 6 29
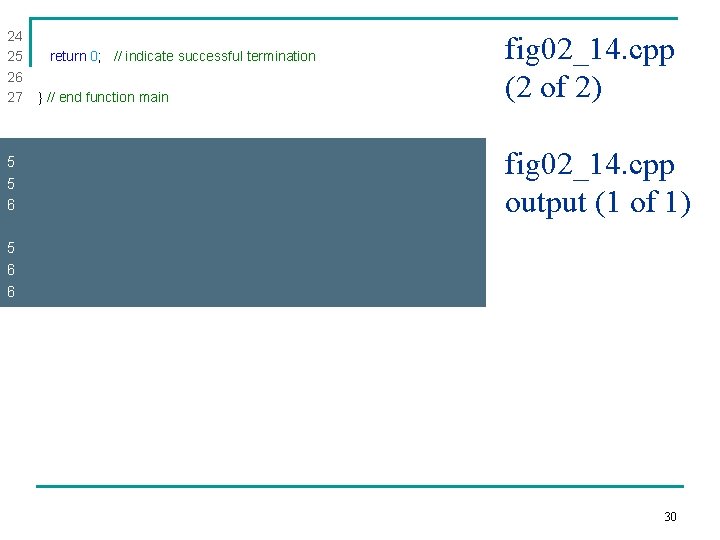
24 25 26 27 5 5 6 6 return 0; // indicate successful termination } // end function main fig 02_14. cpp (2 of 2) fig 02_14. cpp output (1 of 1) 30
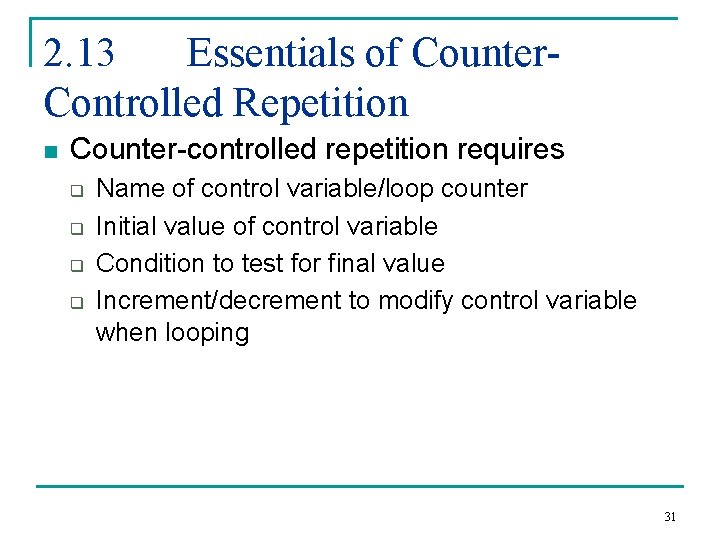
2. 13 Essentials of Counter. Controlled Repetition n Counter-controlled repetition requires q q Name of control variable/loop counter Initial value of control variable Condition to test for final value Increment/decrement to modify control variable when looping 31
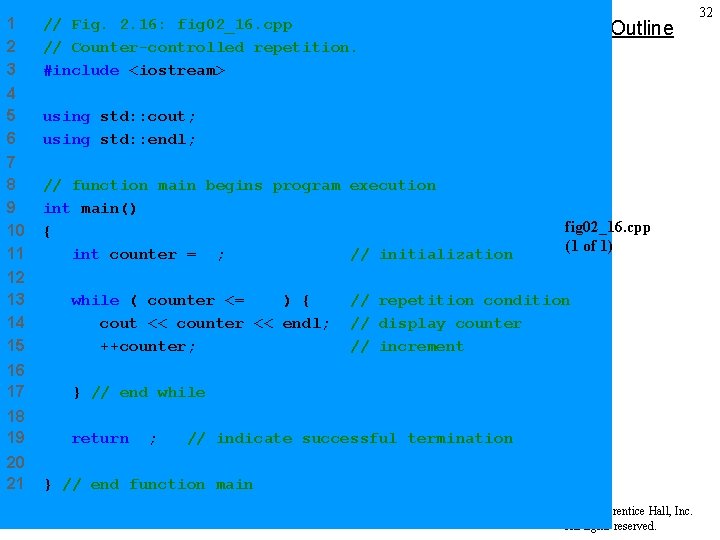
1 2 3 // Fig. 2. 16: fig 02_16. cpp // Counter-controlled repetition. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 // function main begins program execution int main() { int counter = 1; // initialization 12 13 14 15 while ( counter <= 10 ) { cout << counter << endl; ++counter; 16 17 } // end while 18 19 return 0; 20 21 Outline fig 02_16. cpp (1 of 1) // repetition condition // display counter // increment // indicate successful termination } // end function main 2003 Prentice Hall, Inc. All rights reserved. 32
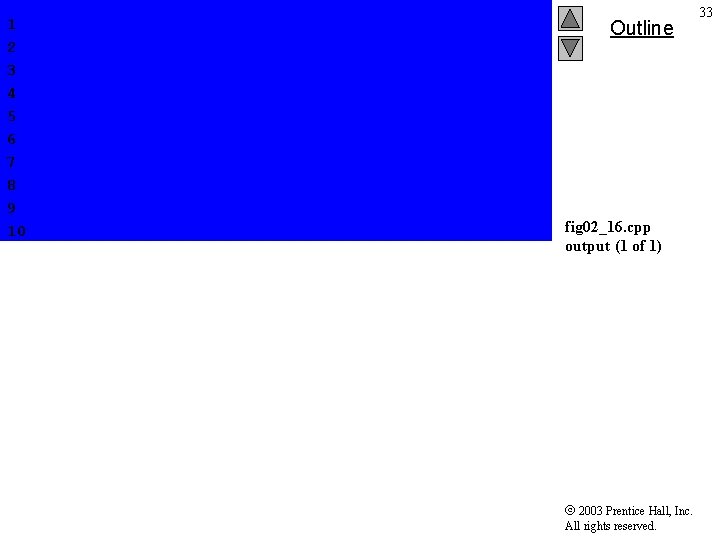
1 2 3 4 5 6 7 8 9 10 Outline fig 02_16. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 33
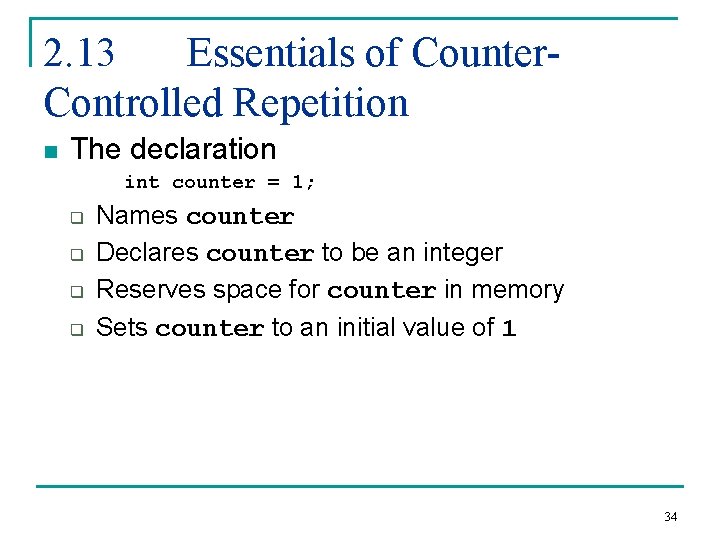
2. 13 Essentials of Counter. Controlled Repetition n The declaration int counter = 1; q q Names counter Declares counter to be an integer Reserves space for counter in memory Sets counter to an initial value of 1 34
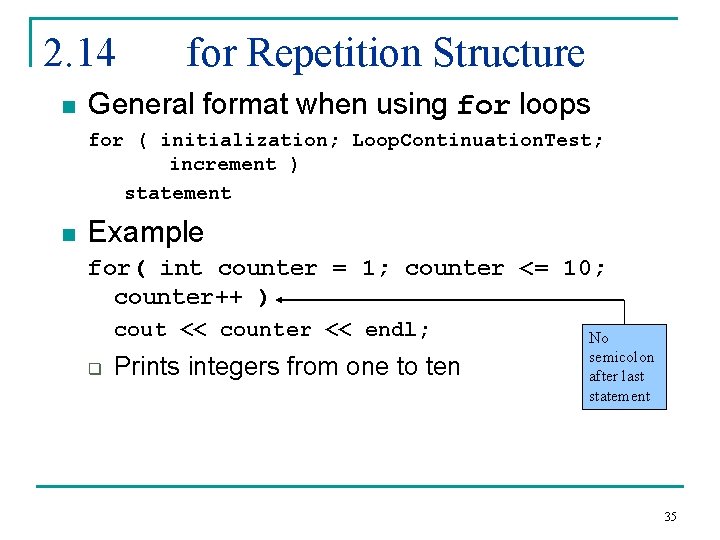
2. 14 n for Repetition Structure General format when using for loops for ( initialization; Loop. Continuation. Test; increment ) statement n Example for( int counter = 1; counter <= 10; counter++ ) cout << counter << endl; q Prints integers from one to ten No semicolon after last statement 35
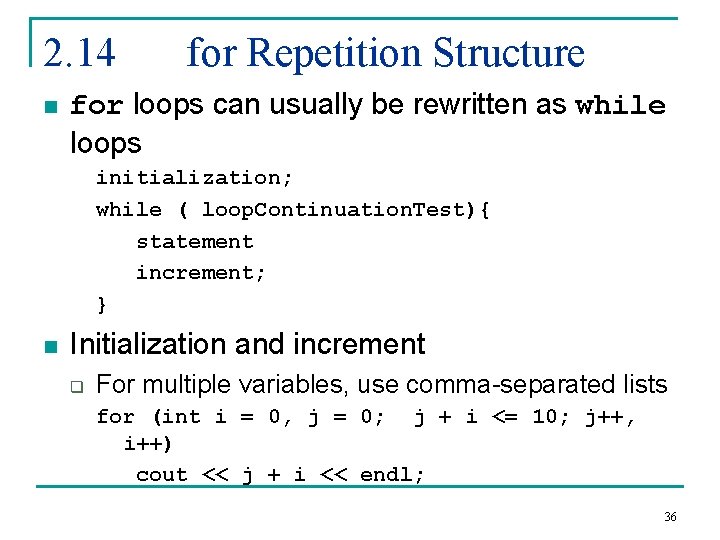
2. 14 n for Repetition Structure for loops can usually be rewritten as while loops initialization; while ( loop. Continuation. Test){ statement increment; } n Initialization and increment q For multiple variables, use comma-separated lists for (int i = 0, j = 0; j + i <= 10; j++, i++) cout << j + i << endl; 36
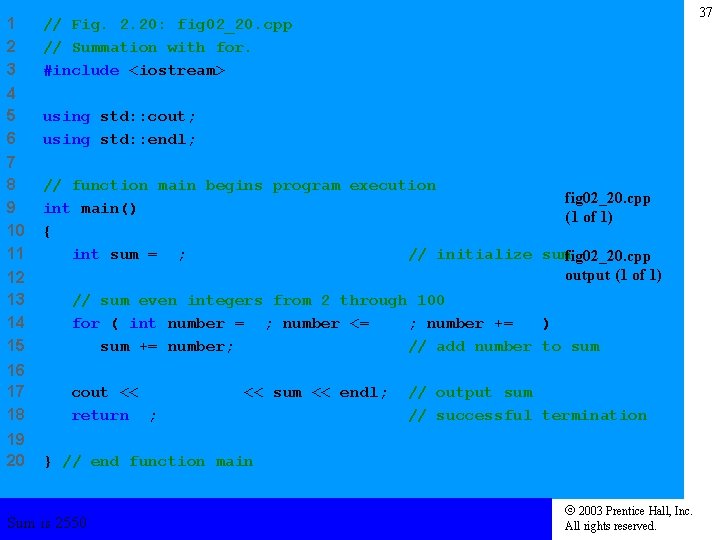
1 2 3 // Fig. 2. 20: fig 02_20. cpp // Summation with for. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 12 13 14 15 16 17 18 19 20 Outline // function main begins program execution fig 02_20. cpp int main() (1 of 1) { int sum = 0; // initialize sumfig 02_20. cpp output (1 of 1) // sum even integers from 2 through 100 for ( int number = 2; number <= 100; number += 2 ) sum += number; // add number to sum cout << "Sum is " << sum << endl; return 0; // output sum // successful termination } // end function main Sum is 2550 2003 Prentice Hall, Inc. All rights reserved. 37
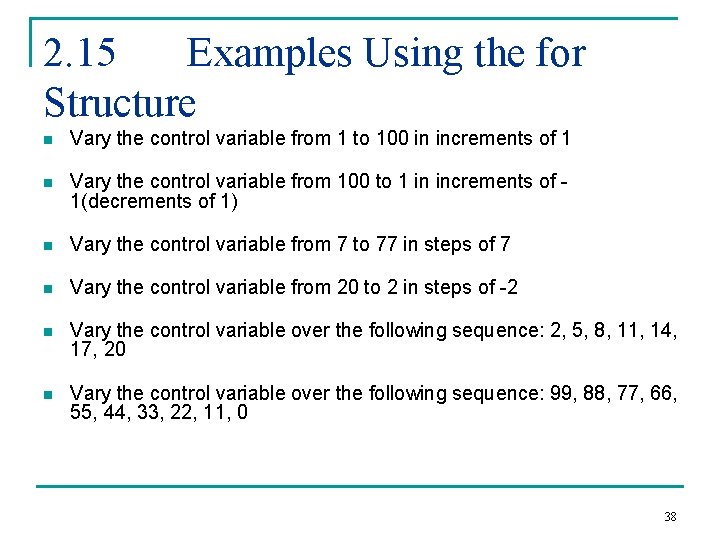
2. 15 Examples Using the for Structure n Vary the control variable from 1 to 100 in increments of 1 n Vary the control variable from 100 to 1 in increments of 1(decrements of 1) n Vary the control variable from 7 to 77 in steps of 7 n Vary the control variable from 20 to 2 in steps of -2 n Vary the control variable over the following sequence: 2, 5, 8, 11, 14, 17, 20 n Vary the control variable over the following sequence: 99, 88, 77, 66, 55, 44, 33, 22, 11, 0 38
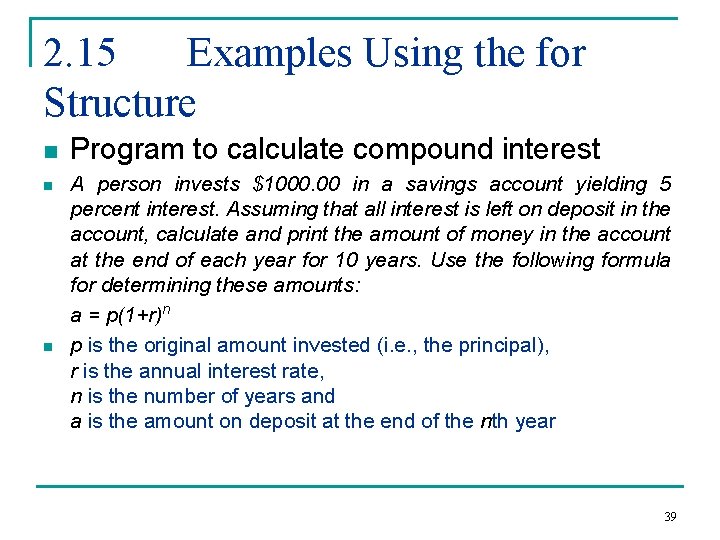
2. 15 Examples Using the for Structure n n n Program to calculate compound interest A person invests $1000. 00 in a savings account yielding 5 percent interest. Assuming that all interest is left on deposit in the account, calculate and print the amount of money in the account at the end of each year for 10 years. Use the following formula for determining these amounts: a = p(1+r)n p is the original amount invested (i. e. , the principal), r is the annual interest rate, n is the number of years and a is the amount on deposit at the end of the nth year 39
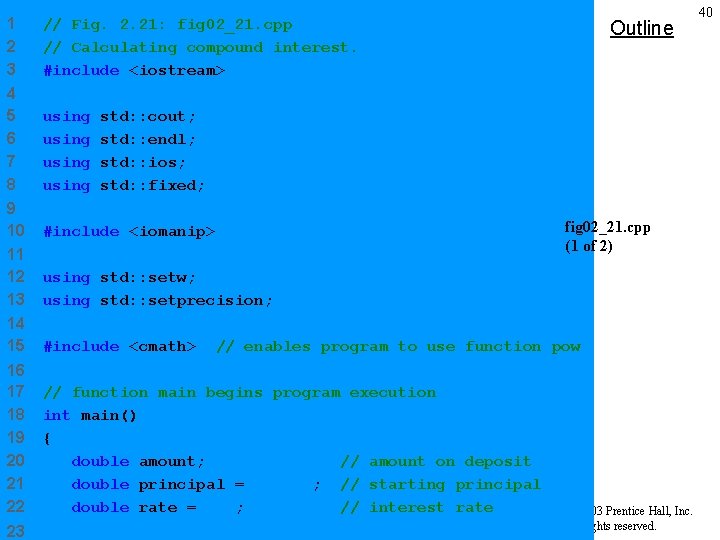
1 2 3 // Fig. 2. 21: fig 02_21. cpp // Calculating compound interest. #include <iostream> 4 5 6 7 8 using 9 10 #include <iomanip> 11 12 13 using std: : setw; using std: : setprecision; 14 15 #include <cmath> 16 17 18 19 20 21 22 // function main begins program execution int main() { double amount; // amount on deposit double principal = 1000. 0; // starting principal double rate =. 05; // interest rate 23 Outline std: : cout; std: : endl; std: : ios; std: : fixed; fig 02_21. cpp (1 of 2) // enables program to use function pow 2003 Prentice Hall, Inc. All rights reserved. 40
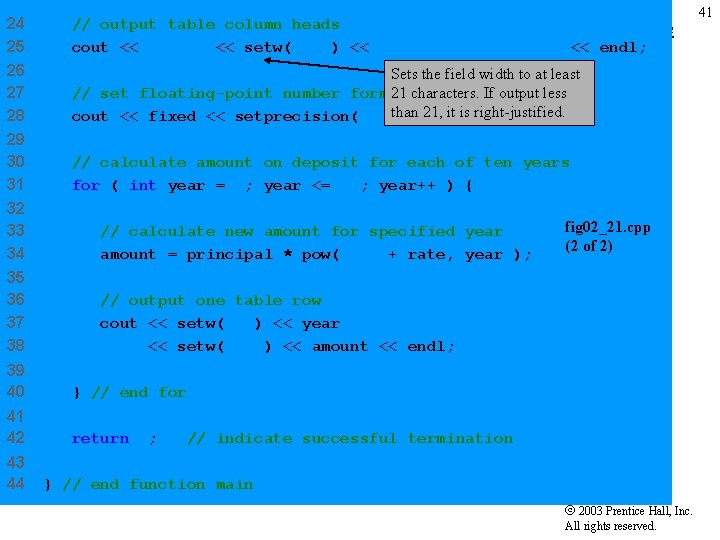
24 25 // output table column heads Outline cout << "Year" << setw( 21 ) << "Amount on deposit" << endl; 26 27 28 Sets the field width to at least // set floating-point number format 21 characters. If output less than 21, it is right-justified. cout << fixed << setprecision( 2 ); 29 30 31 // calculate amount on deposit for each of ten years for ( int year = 1; year <= 10; year++ ) { 32 33 34 // calculate new amount for specified year amount = principal * pow( 1. 0 + rate, year ); 35 36 37 38 // output one table row cout << setw( 4 ) << year << setw( 21 ) << amount << endl; 39 40 } // end for 41 42 return 0; 43 44 fig 02_21. cpp (2 of 2) // indicate successful termination } // end function main 2003 Prentice Hall, Inc. All rights reserved. 41
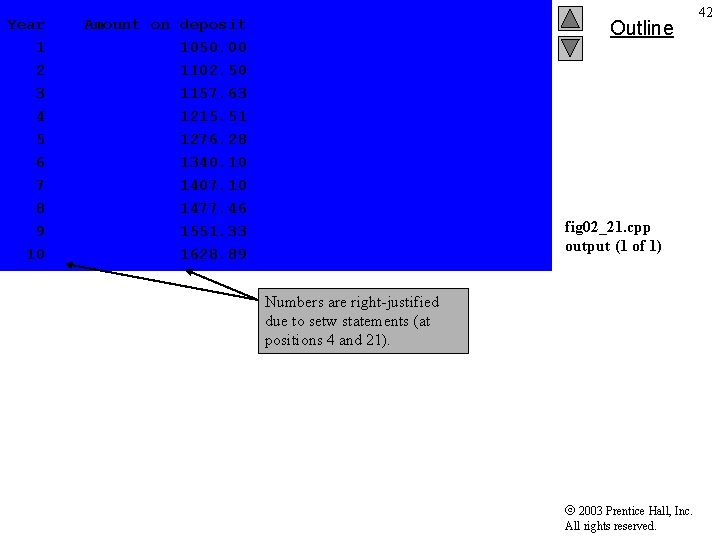
Year 1 2 3 4 5 6 7 8 9 10 Amount on deposit 1050. 00 1102. 50 1157. 63 1215. 51 1276. 28 1340. 10 1407. 10 1477. 46 1551. 33 1628. 89 Outline fig 02_21. cpp output (1 of 1) Numbers are right-justified due to setw statements (at positions 4 and 21). 2003 Prentice Hall, Inc. All rights reserved. 42
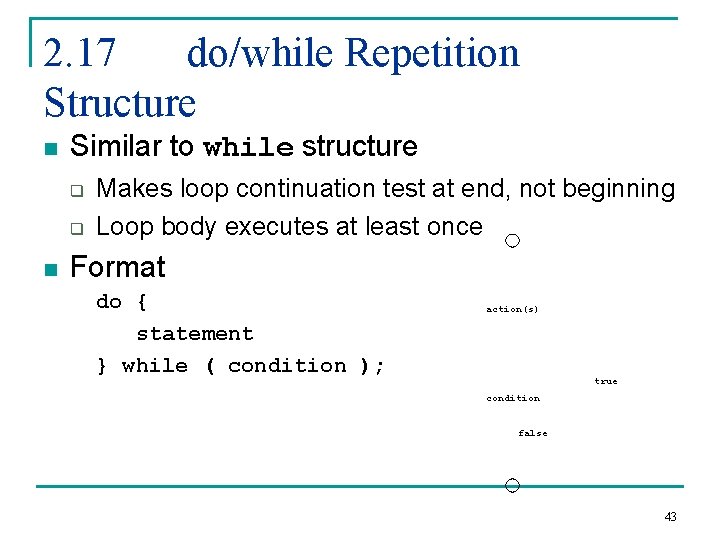
2. 17 do/while Repetition Structure n Similar to while structure q q n Makes loop continuation test at end, not beginning Loop body executes at least once Format do { statement } while ( condition ); action(s) true condition false 43
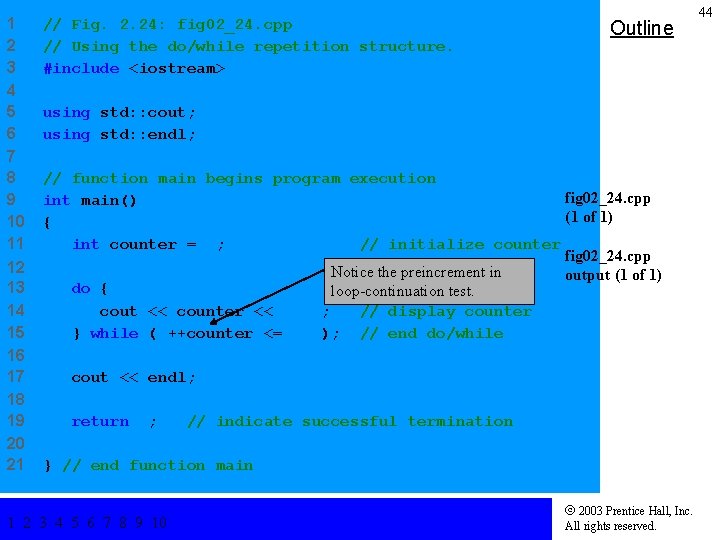
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 // Fig. 2. 24: fig 02_24. cpp // Using the do/while repetition structure. #include <iostream> Outline using std: : cout; using std: : endl; // function main begins program execution fig 02_24. cpp int main() (1 of 1) { int counter = 1; // initialize counter fig 02_24. cpp Notice the preincrement in output (1 of 1) do { loop-continuation test. cout << counter << " "; // display counter } while ( ++counter <= 10 ); // end do/while cout << endl; return 0; // indicate successful termination } // end function main 1 2 3 4 5 6 7 8 9 10 2003 Prentice Hall, Inc. All rights reserved. 44
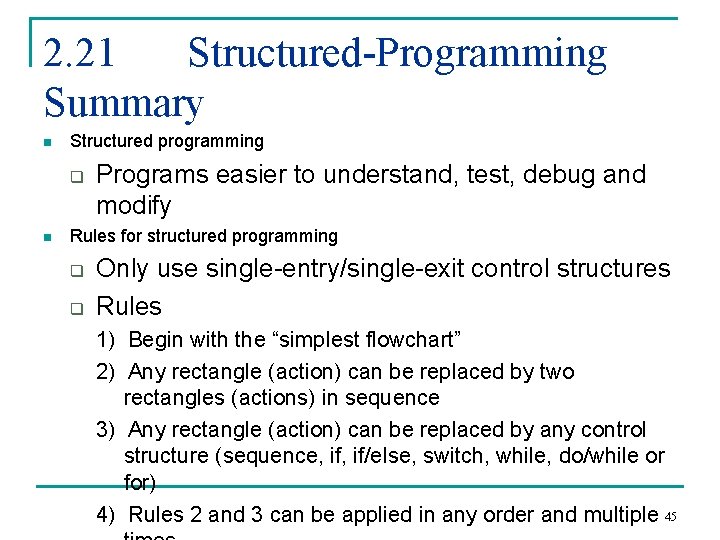
2. 21 Structured-Programming Summary n Structured programming q n Programs easier to understand, test, debug and modify Rules for structured programming q q Only use single-entry/single-exit control structures Rules 1) Begin with the “simplest flowchart” 2) Any rectangle (action) can be replaced by two rectangles (actions) in sequence 3) Any rectangle (action) can be replaced by any control structure (sequence, if/else, switch, while, do/while or for) 4) Rules 2 and 3 can be applied in any order and multiple 45
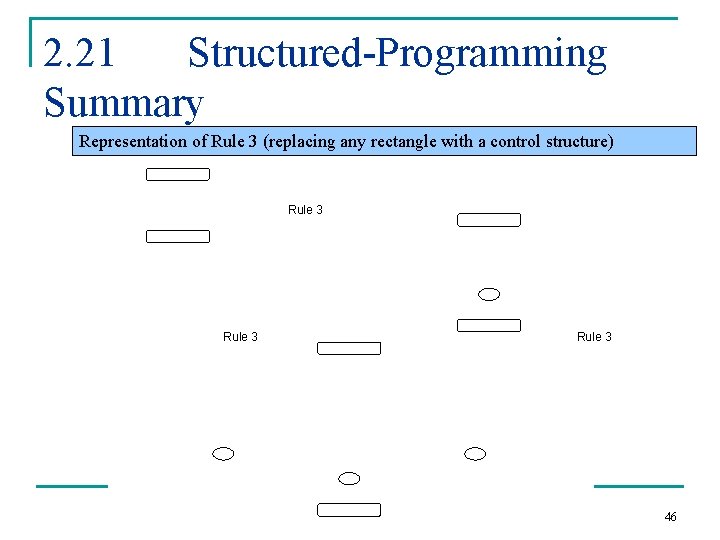
2. 21 Structured-Programming Summary Representation of Rule 3 (replacing any rectangle with a control structure) Rule 3 46
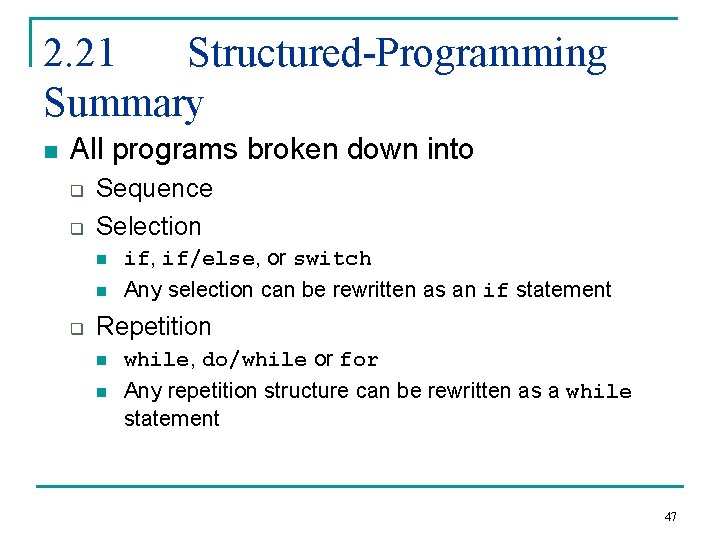
2. 21 Structured-Programming Summary n All programs broken down into q q Sequence Selection n n q if, if/else, or switch Any selection can be rewritten as an if statement Repetition n n while, do/while or for Any repetition structure can be rewritten as a while statement 47