Lecture 16 Sockets III Internet Domain Sockets in
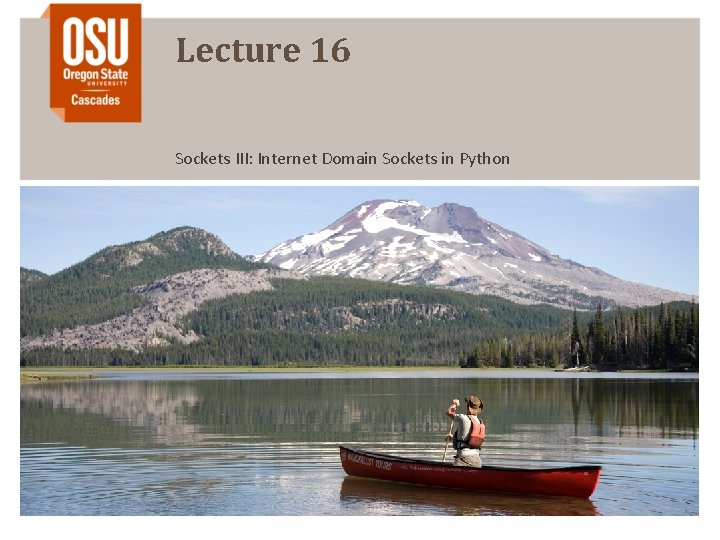
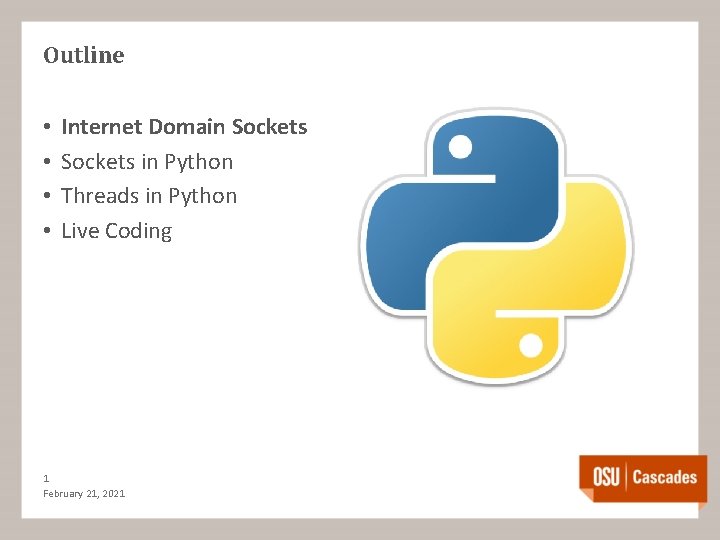
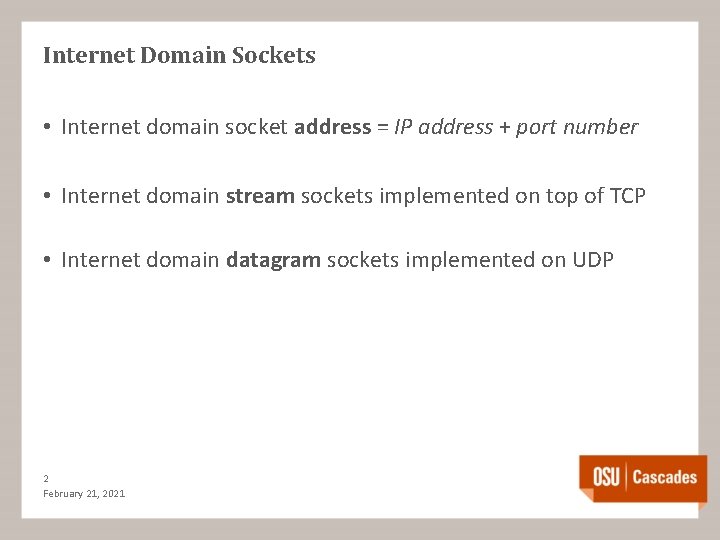
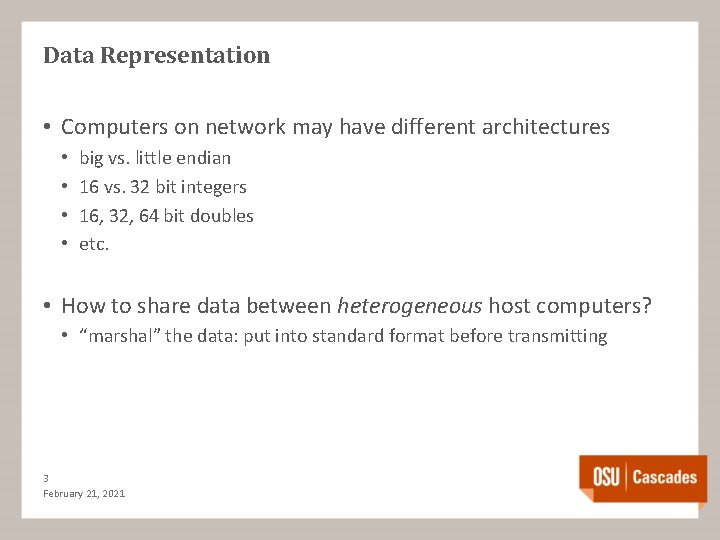
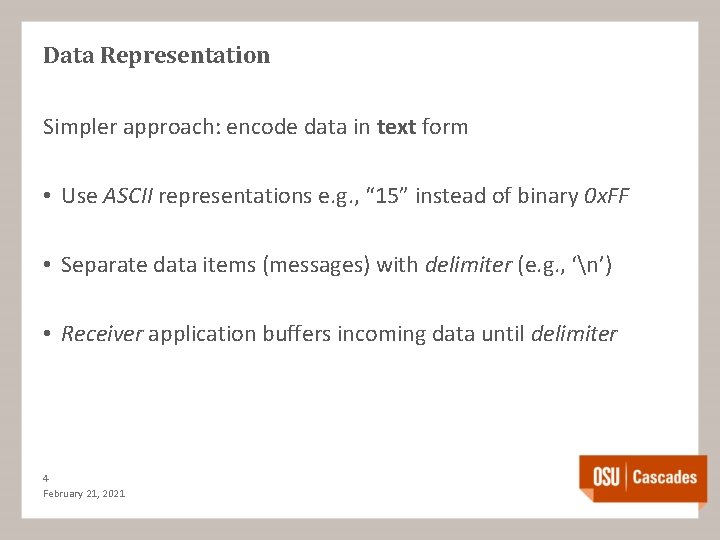
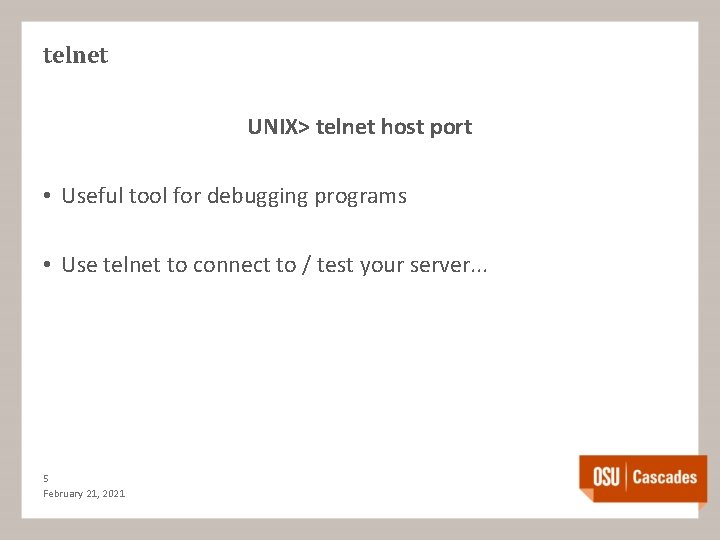
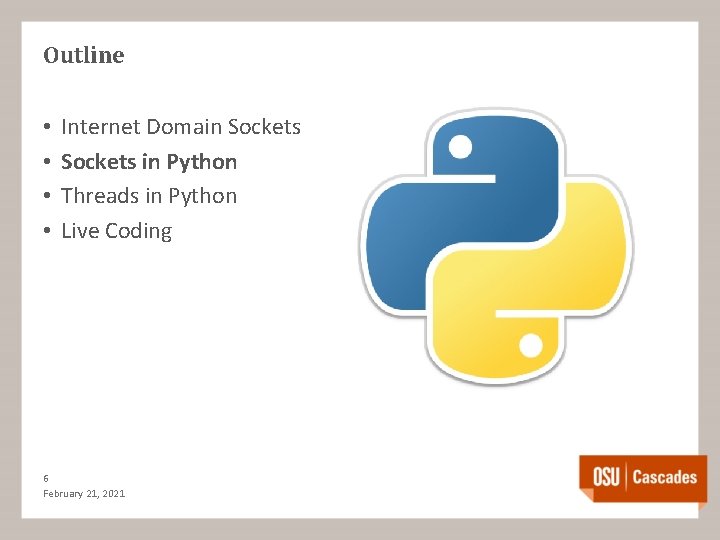
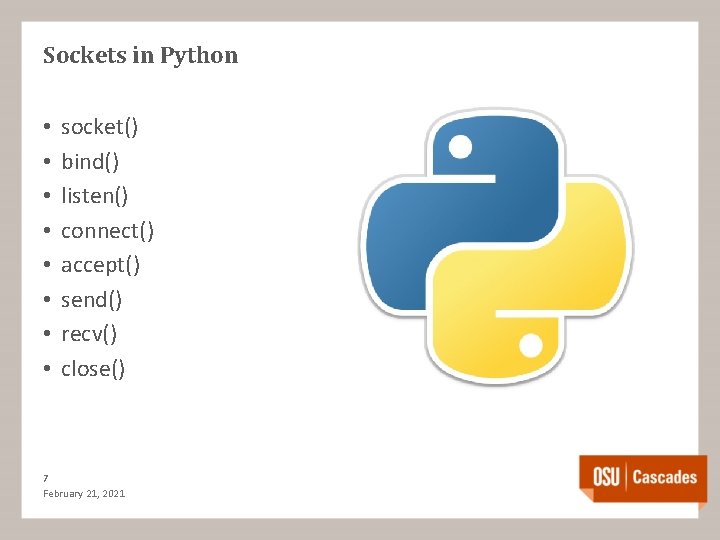
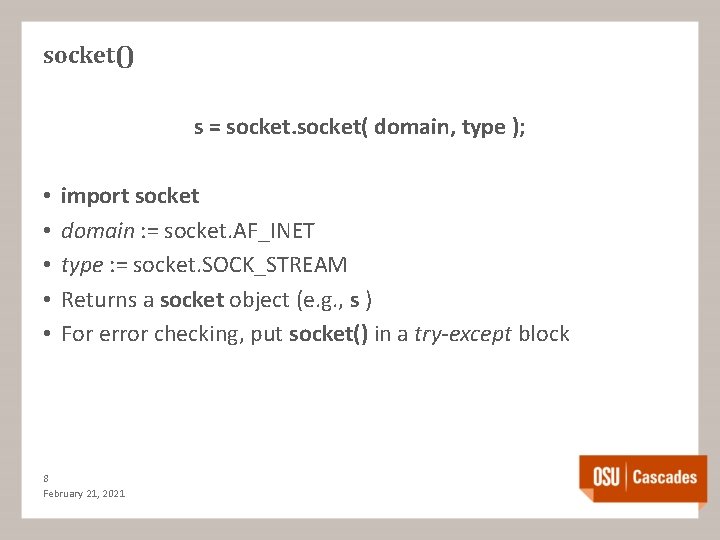
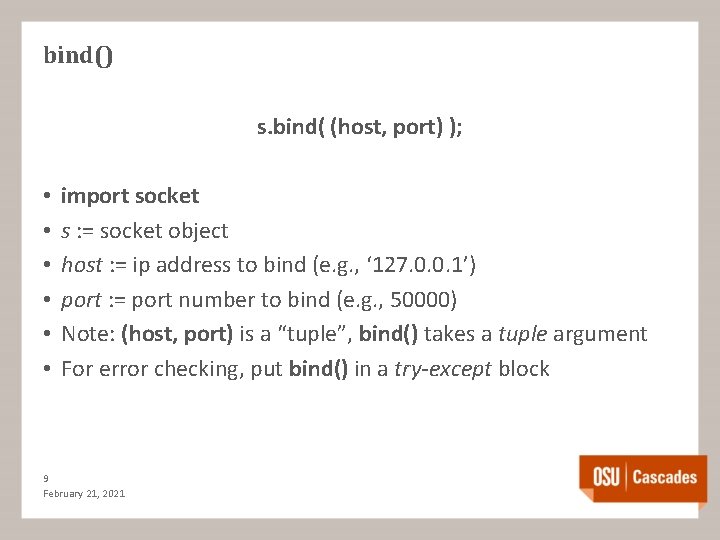
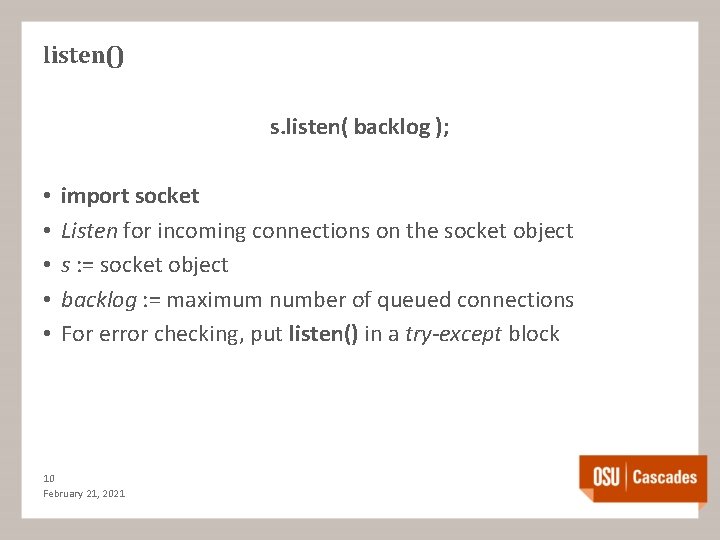
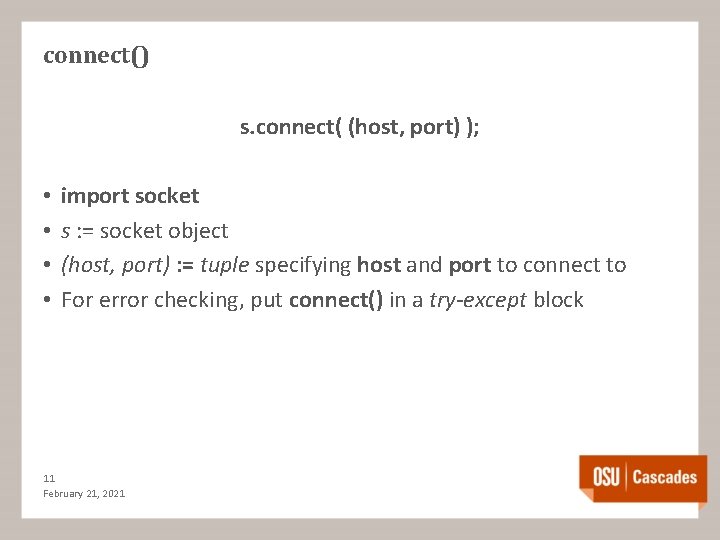
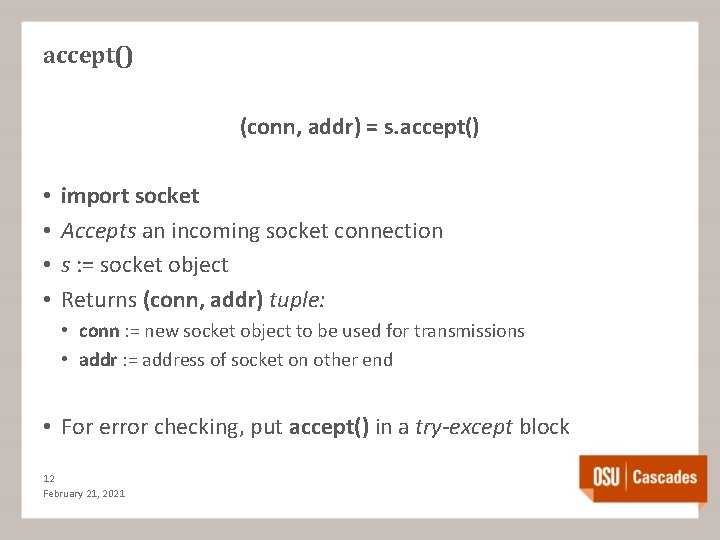
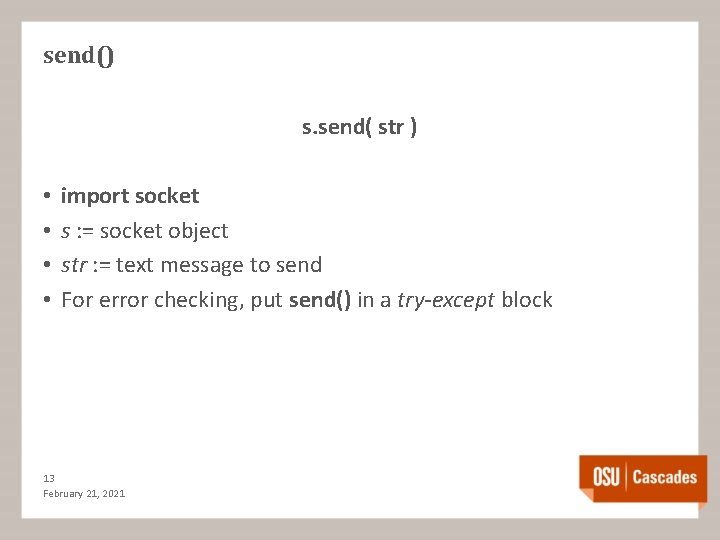
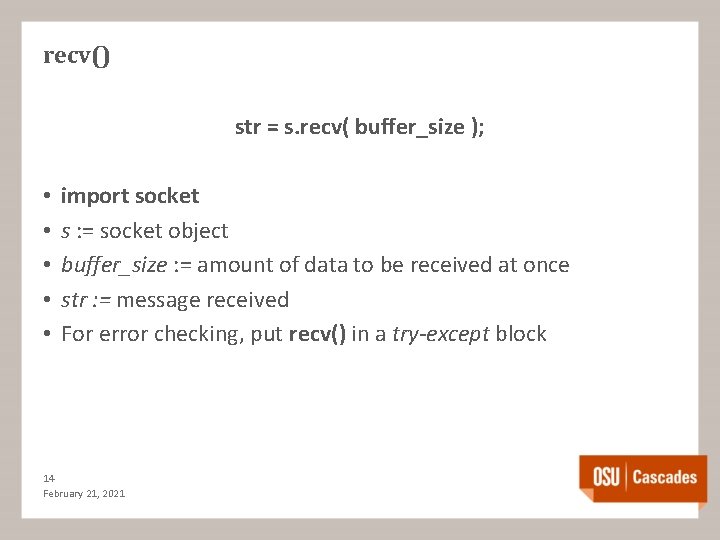
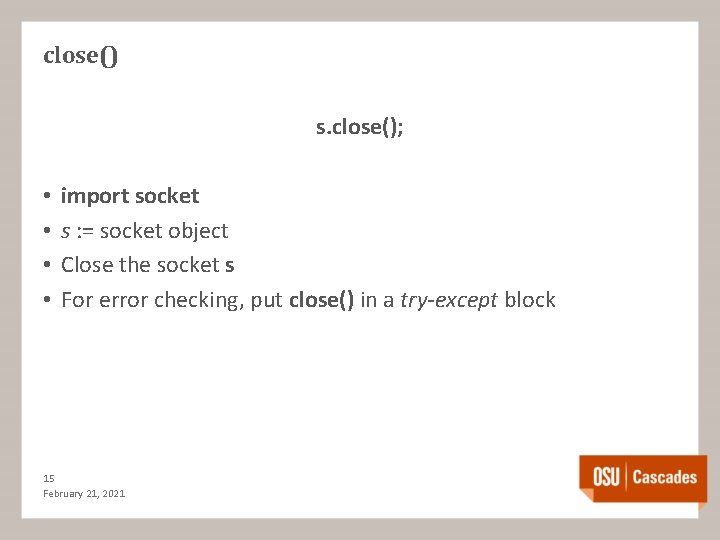
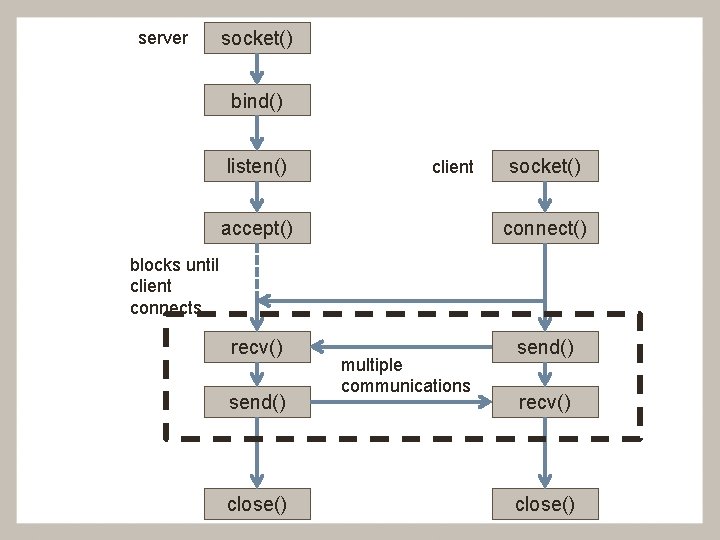
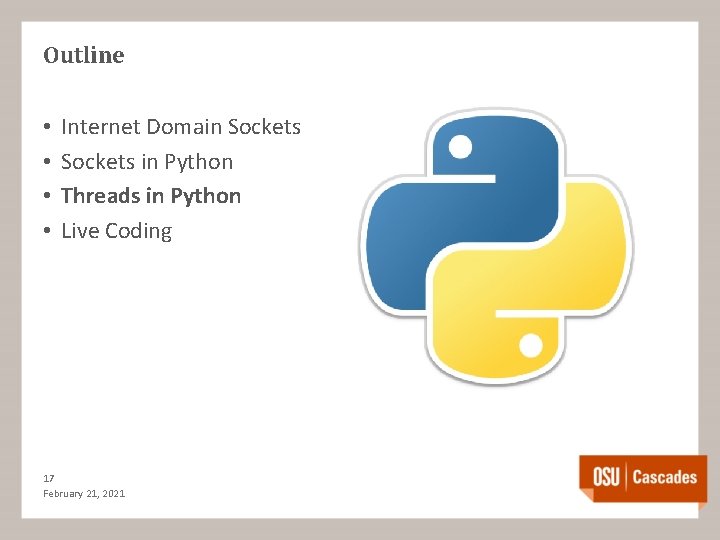
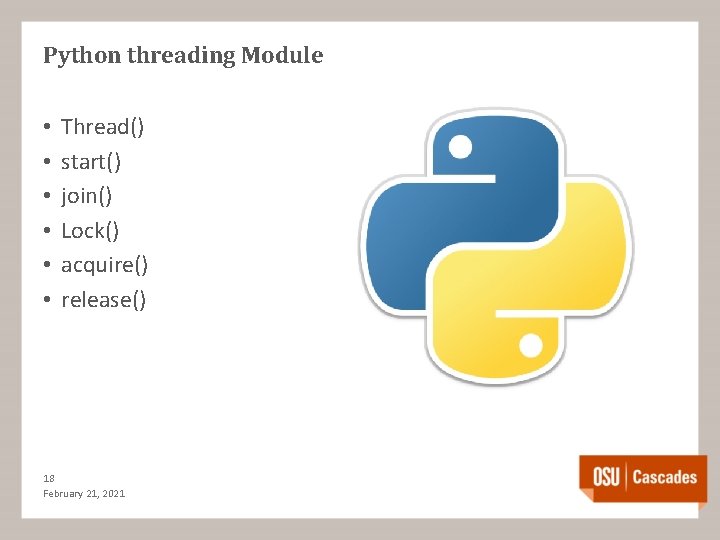
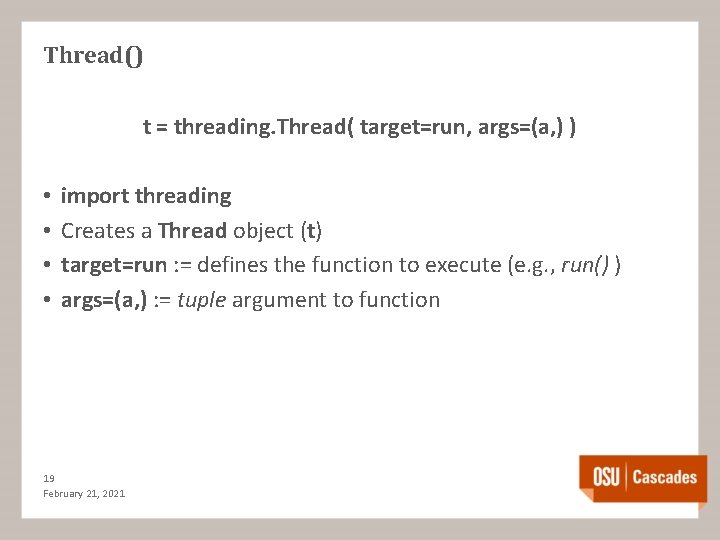
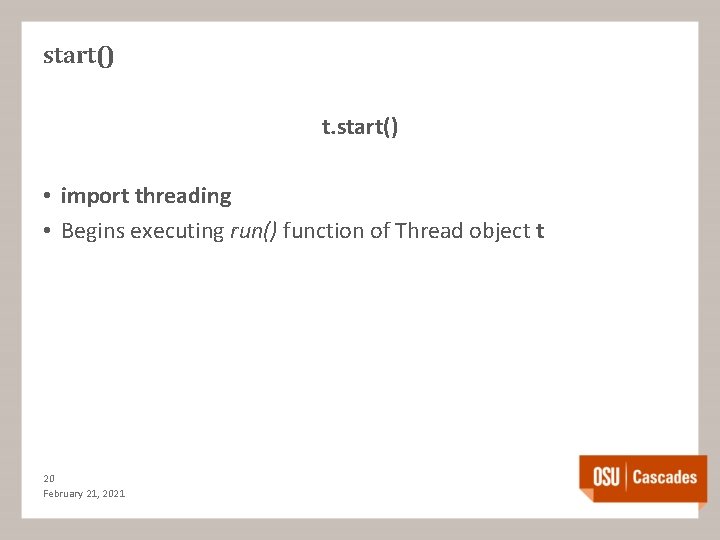
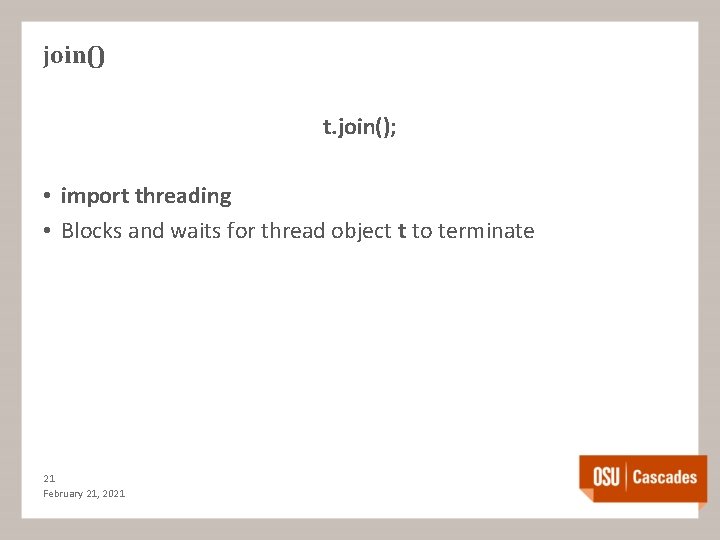
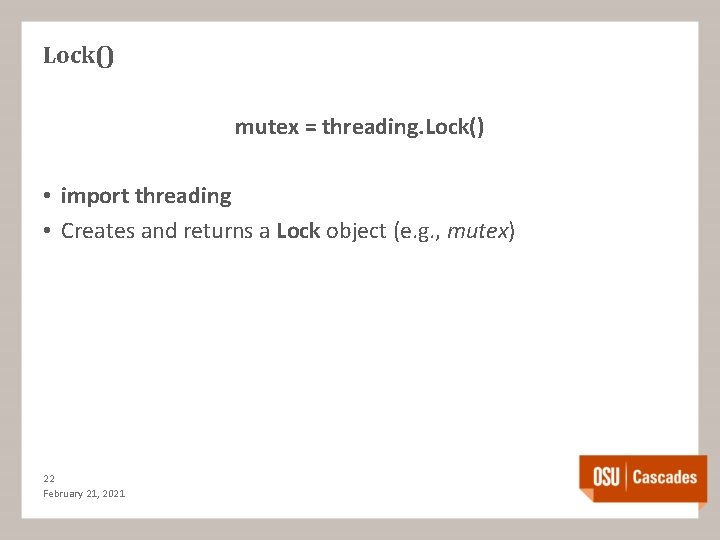
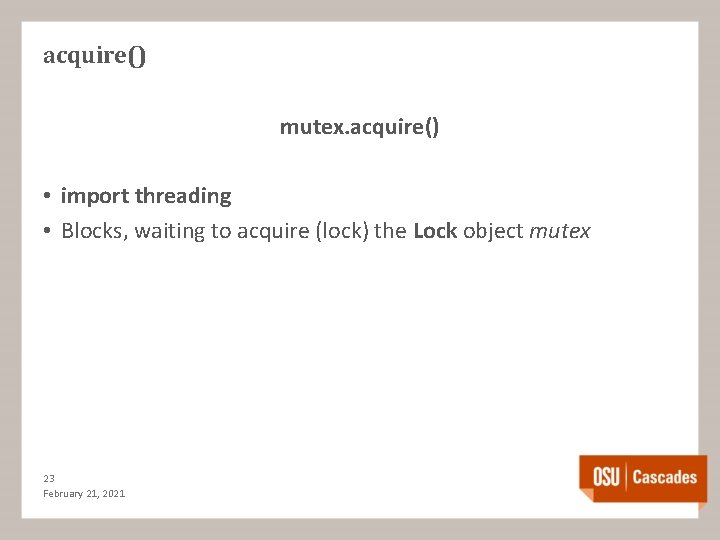
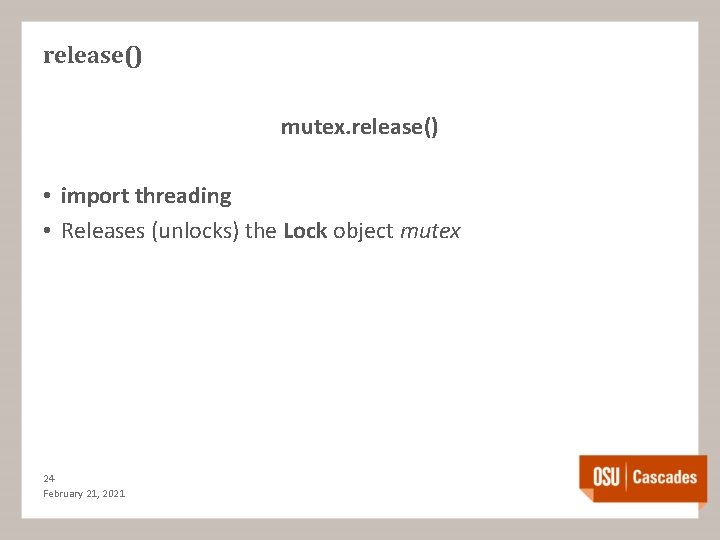
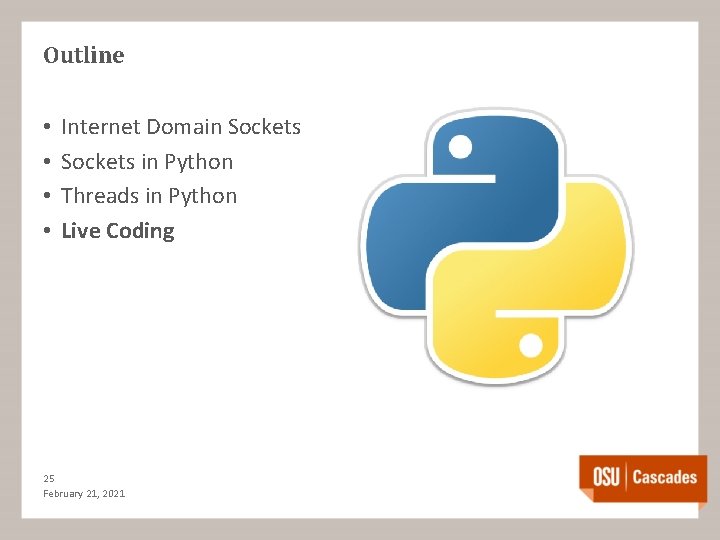
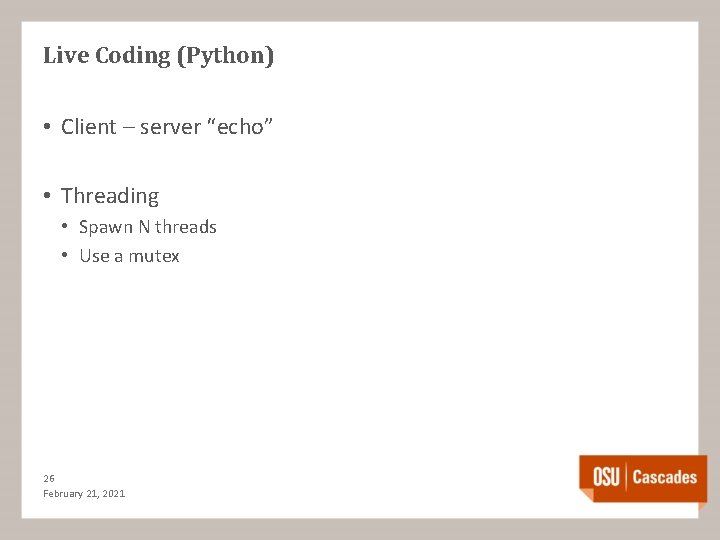
- Slides: 27
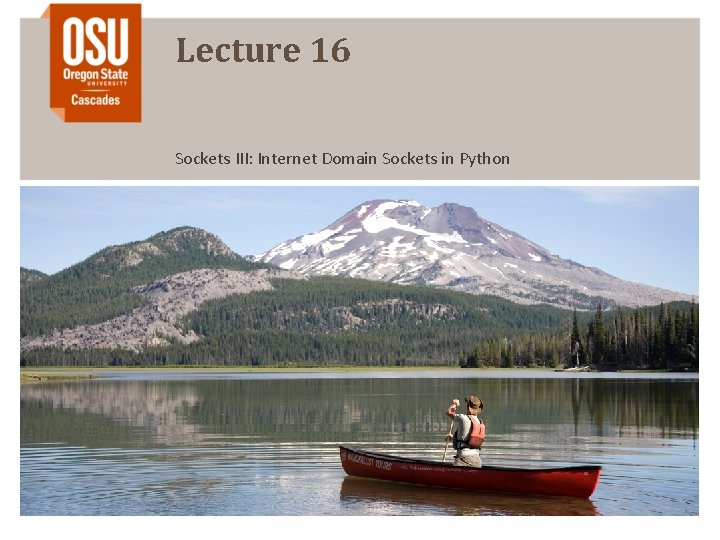
Lecture 16 Sockets III: Internet Domain Sockets in Python
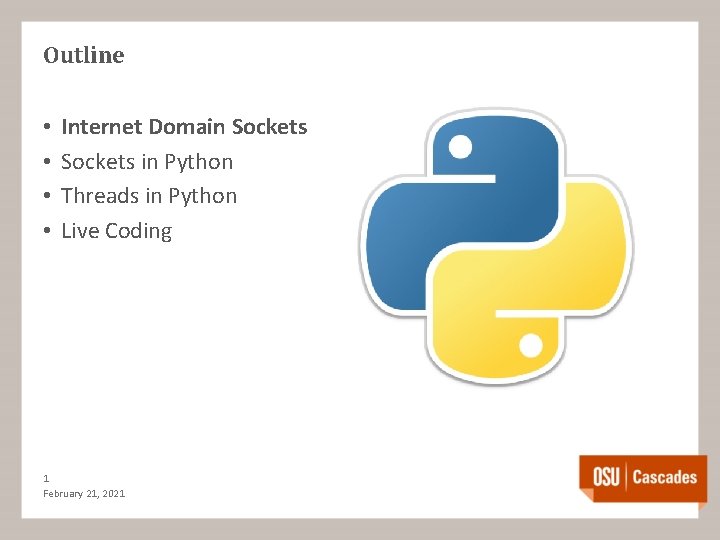
Outline • • Internet Domain Sockets in Python Threads in Python Live Coding 1 February 21, 2021
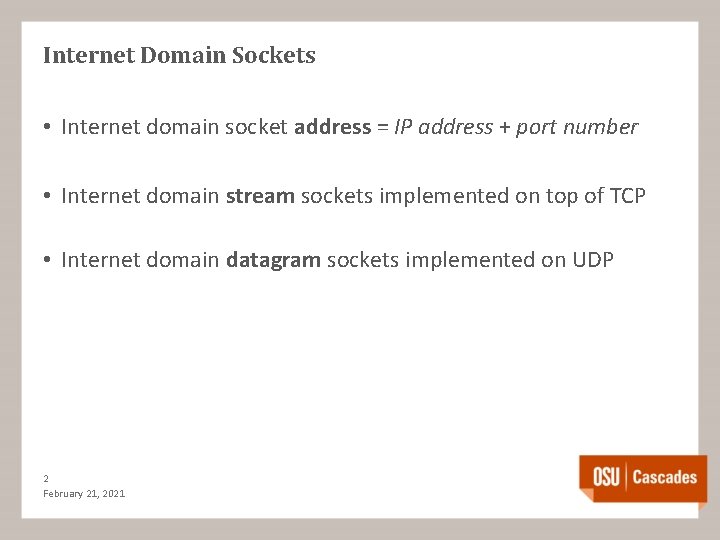
Internet Domain Sockets • Internet domain socket address = IP address + port number • Internet domain stream sockets implemented on top of TCP • Internet domain datagram sockets implemented on UDP 2 February 21, 2021
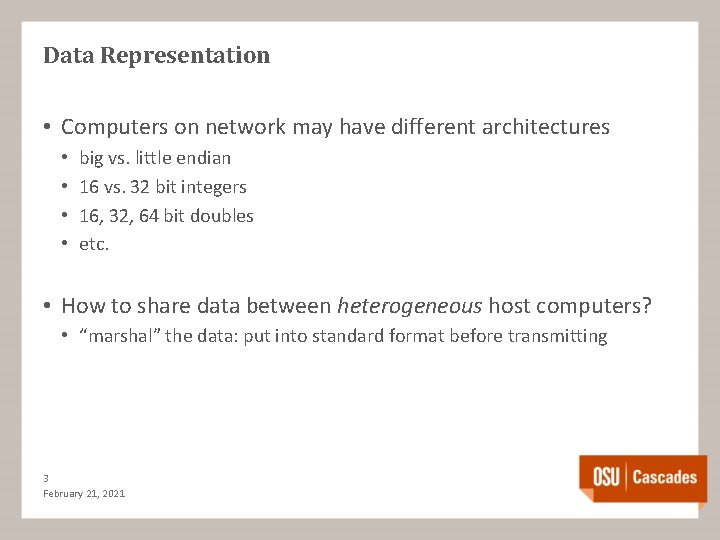
Data Representation • Computers on network may have different architectures • • big vs. little endian 16 vs. 32 bit integers 16, 32, 64 bit doubles etc. • How to share data between heterogeneous host computers? • “marshal” the data: put into standard format before transmitting 3 February 21, 2021
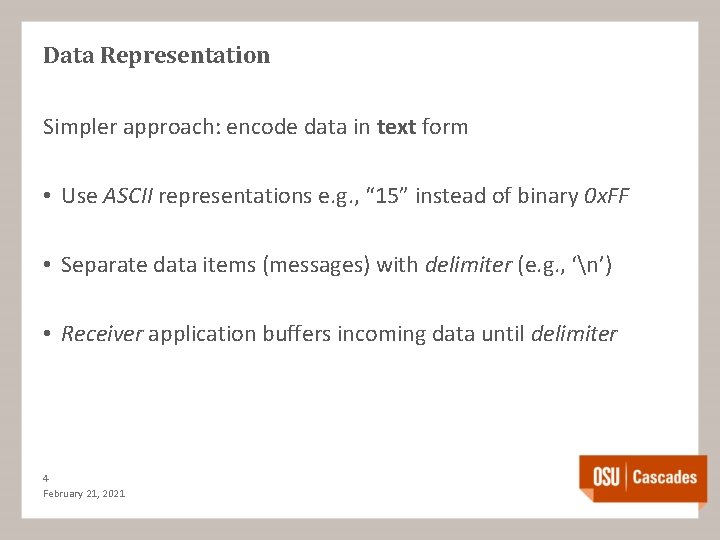
Data Representation Simpler approach: encode data in text form • Use ASCII representations e. g. , “ 15” instead of binary 0 x. FF • Separate data items (messages) with delimiter (e. g. , ‘n’) • Receiver application buffers incoming data until delimiter 4 February 21, 2021
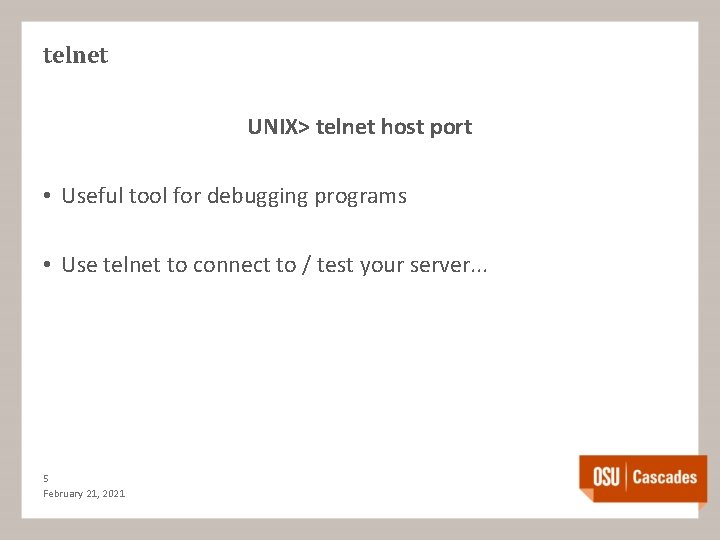
telnet UNIX> telnet host port • Useful tool for debugging programs • Use telnet to connect to / test your server. . . 5 February 21, 2021
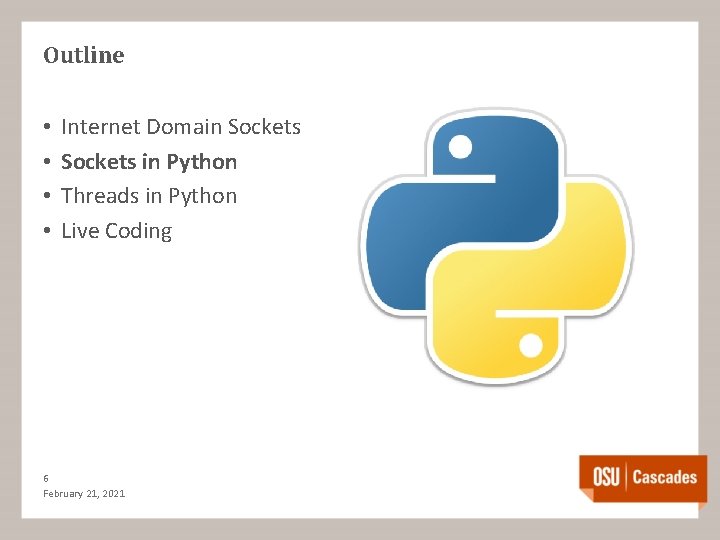
Outline • • Internet Domain Sockets in Python Threads in Python Live Coding 6 February 21, 2021
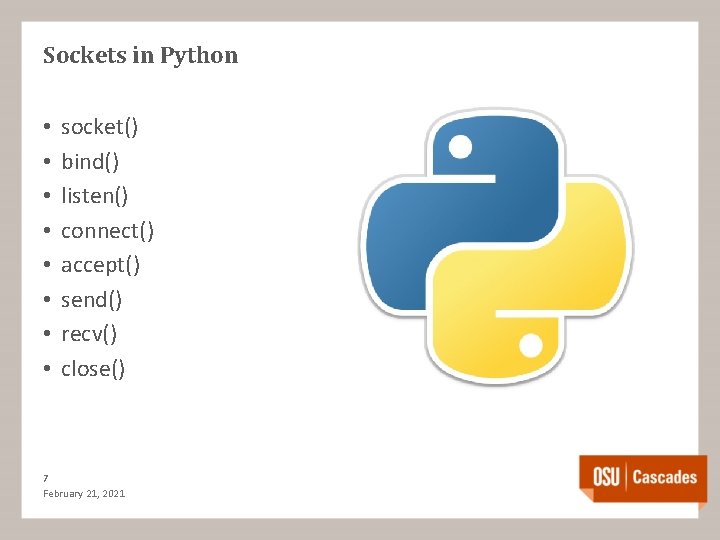
Sockets in Python • • socket() bind() listen() connect() accept() send() recv() close() 7 February 21, 2021
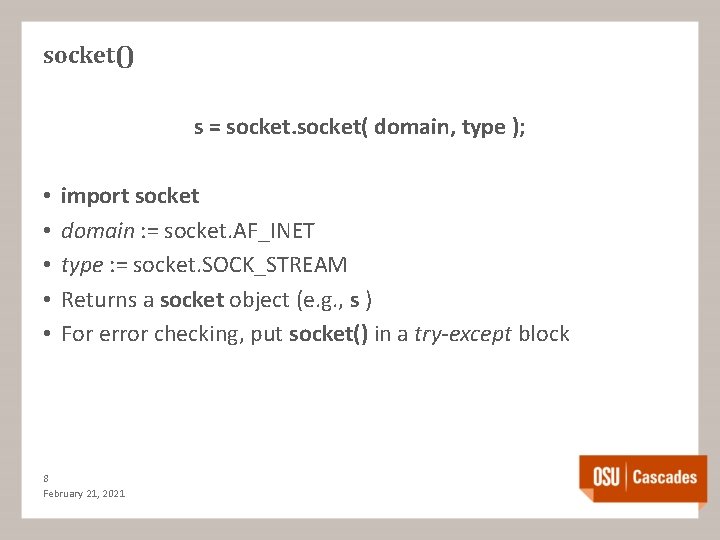
socket() s = socket( domain, type ); • • • import socket domain : = socket. AF_INET type : = socket. SOCK_STREAM Returns a socket object (e. g. , s ) For error checking, put socket() in a try-except block 8 February 21, 2021
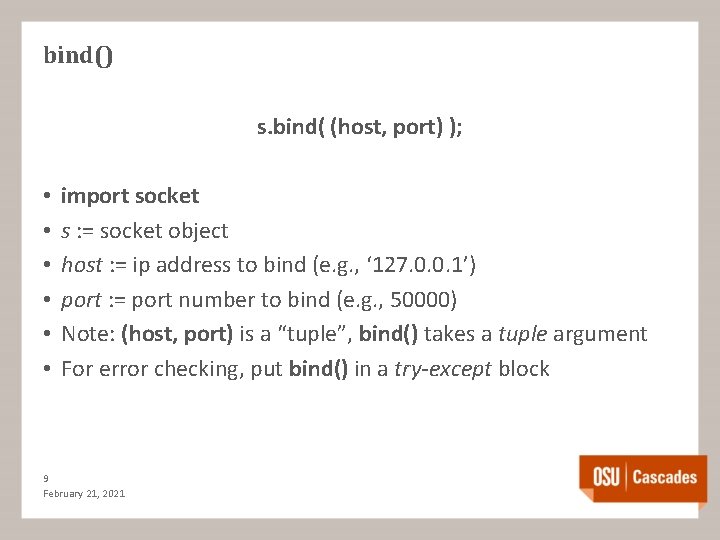
bind() s. bind( (host, port) ); • • • import socket s : = socket object host : = ip address to bind (e. g. , ‘ 127. 0. 0. 1’) port : = port number to bind (e. g. , 50000) Note: (host, port) is a “tuple”, bind() takes a tuple argument For error checking, put bind() in a try-except block 9 February 21, 2021
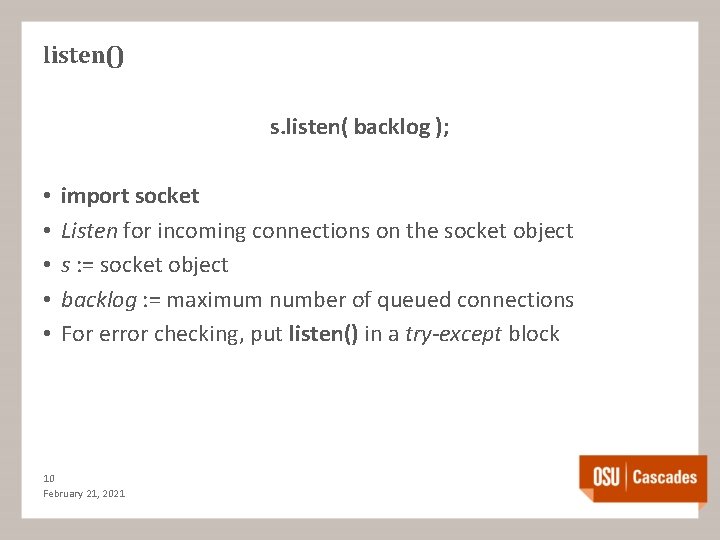
listen() s. listen( backlog ); • • • import socket Listen for incoming connections on the socket object s : = socket object backlog : = maximum number of queued connections For error checking, put listen() in a try-except block 10 February 21, 2021
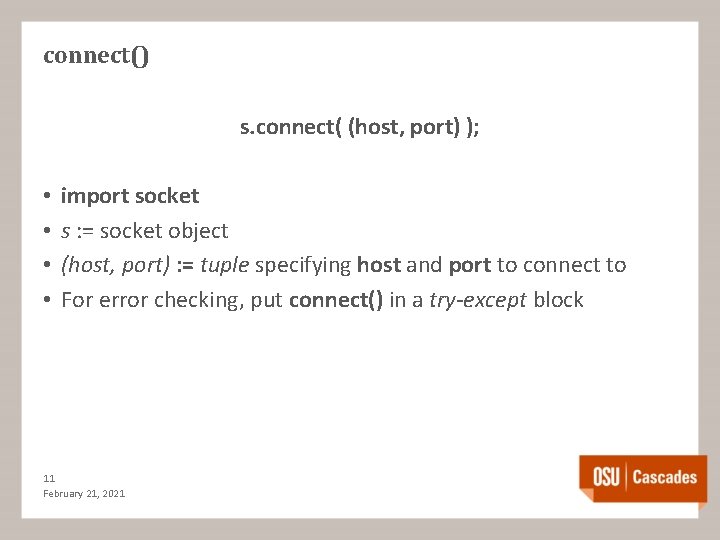
connect() s. connect( (host, port) ); • • import socket s : = socket object (host, port) : = tuple specifying host and port to connect to For error checking, put connect() in a try-except block 11 February 21, 2021
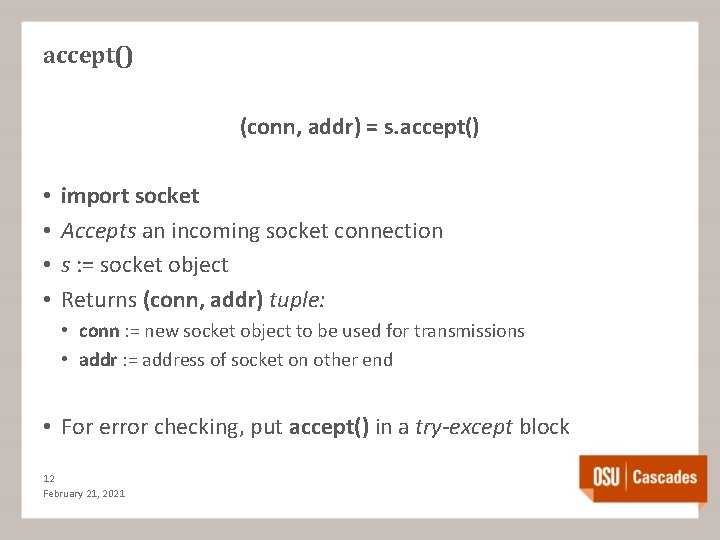
accept() (conn, addr) = s. accept() • • import socket Accepts an incoming socket connection s : = socket object Returns (conn, addr) tuple: • conn : = new socket object to be used for transmissions • addr : = address of socket on other end • For error checking, put accept() in a try-except block 12 February 21, 2021
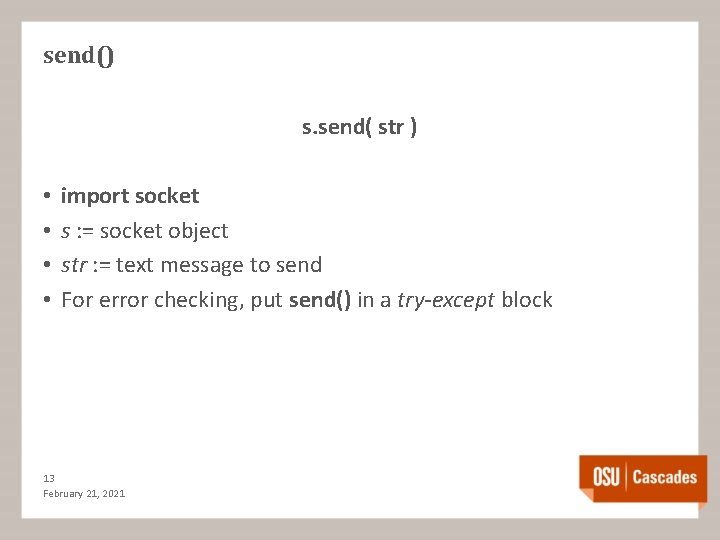
send() s. send( str ) • • import socket s : = socket object str : = text message to send For error checking, put send() in a try-except block 13 February 21, 2021
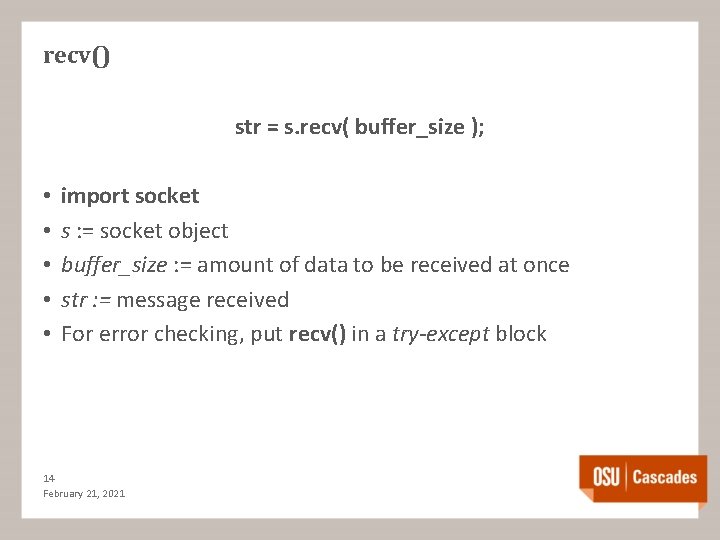
recv() str = s. recv( buffer_size ); • • • import socket s : = socket object buffer_size : = amount of data to be received at once str : = message received For error checking, put recv() in a try-except block 14 February 21, 2021
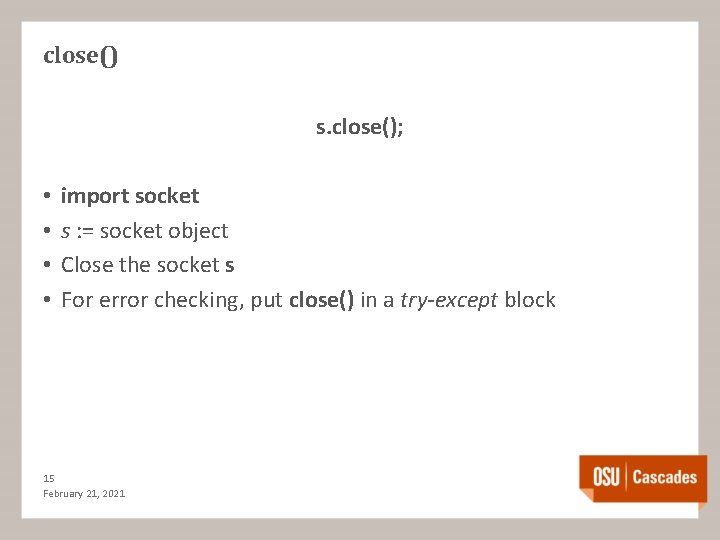
close() s. close(); • • import socket s : = socket object Close the socket s For error checking, put close() in a try-except block 15 February 21, 2021
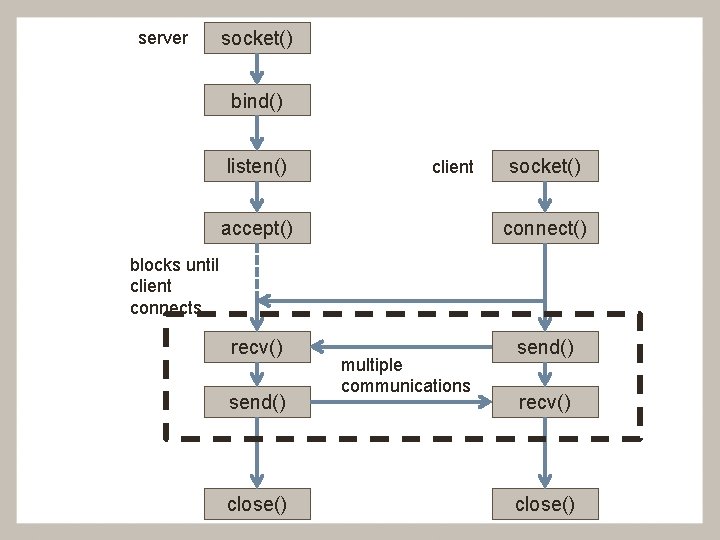
server socket() bind() listen() client socket() accept() connect() recv() send() blocks until client connects send() close() multiple communications recv() close()
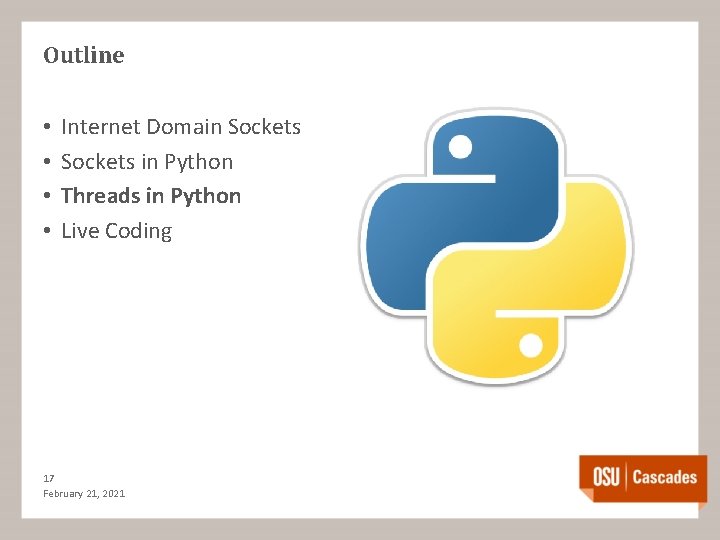
Outline • • Internet Domain Sockets in Python Threads in Python Live Coding 17 February 21, 2021
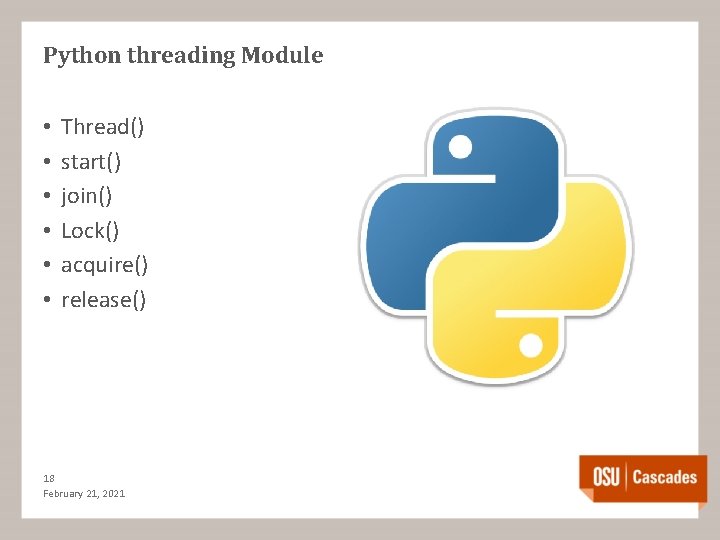
Python threading Module • • • Thread() start() join() Lock() acquire() release() 18 February 21, 2021
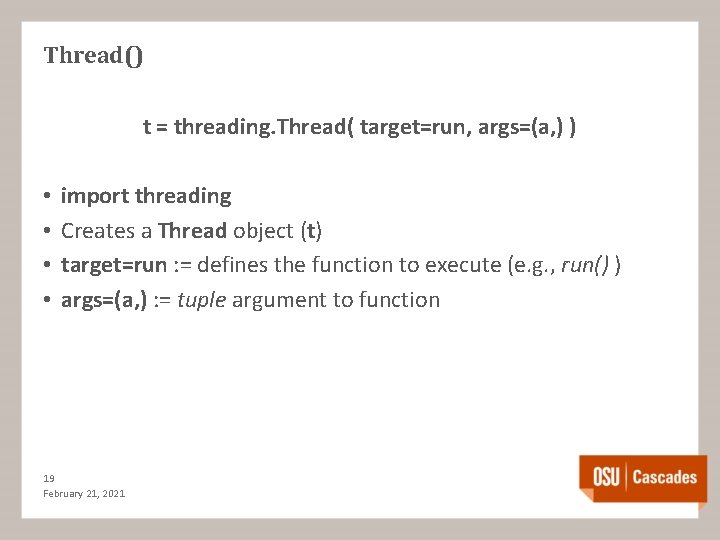
Thread() t = threading. Thread( target=run, args=(a, ) ) • • import threading Creates a Thread object (t) target=run : = defines the function to execute (e. g. , run() ) args=(a, ) : = tuple argument to function 19 February 21, 2021
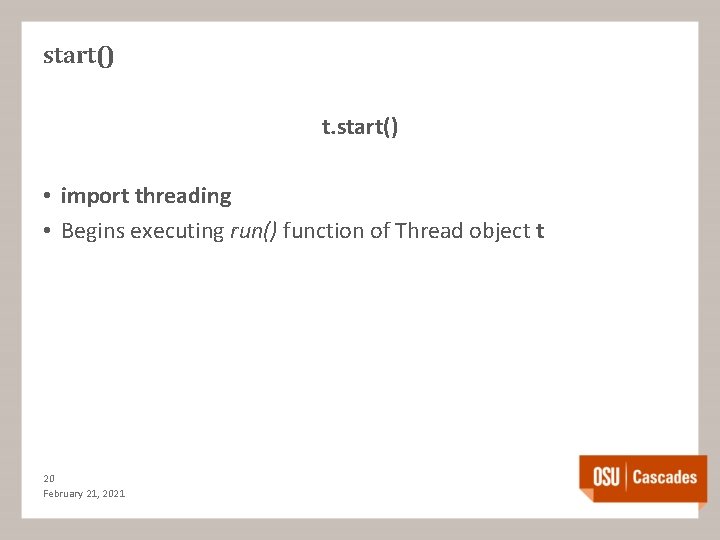
start() t. start() • import threading • Begins executing run() function of Thread object t 20 February 21, 2021
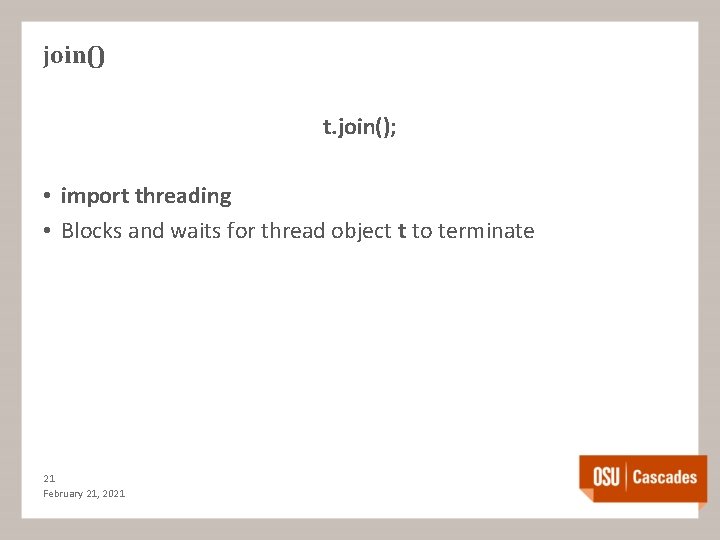
join() t. join(); • import threading • Blocks and waits for thread object t to terminate 21 February 21, 2021
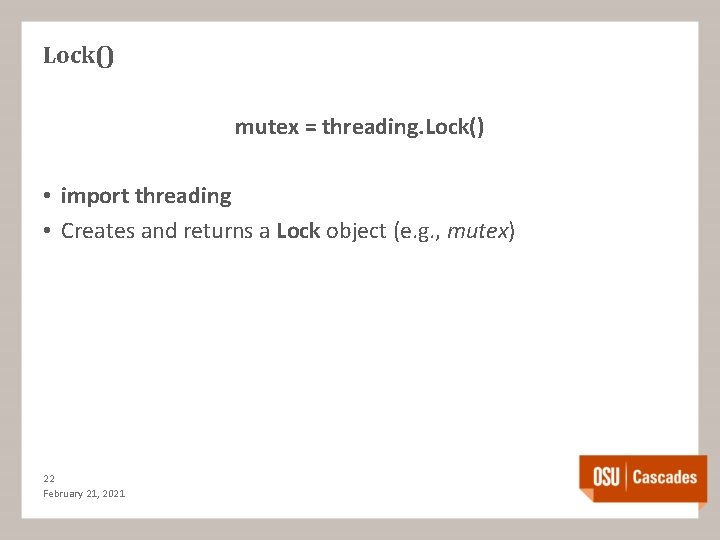
Lock() mutex = threading. Lock() • import threading • Creates and returns a Lock object (e. g. , mutex) 22 February 21, 2021
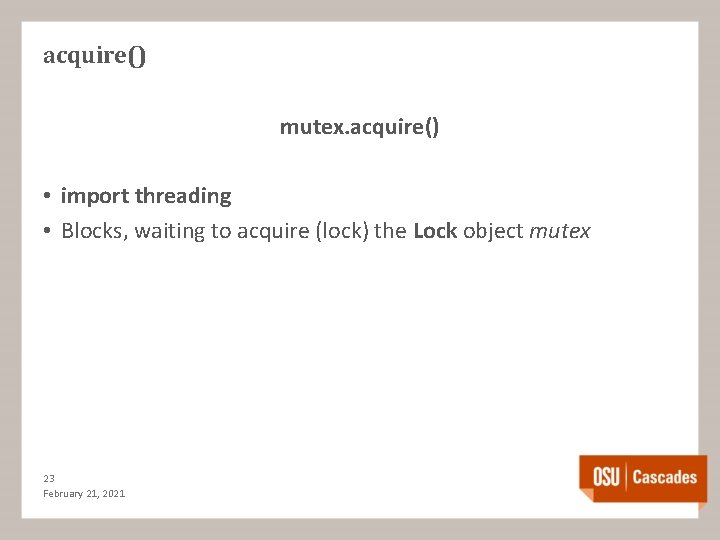
acquire() mutex. acquire() • import threading • Blocks, waiting to acquire (lock) the Lock object mutex 23 February 21, 2021
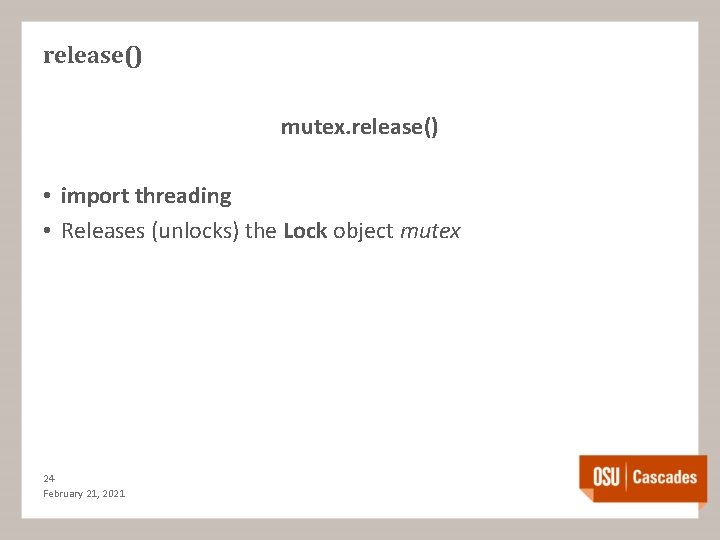
release() mutex. release() • import threading • Releases (unlocks) the Lock object mutex 24 February 21, 2021
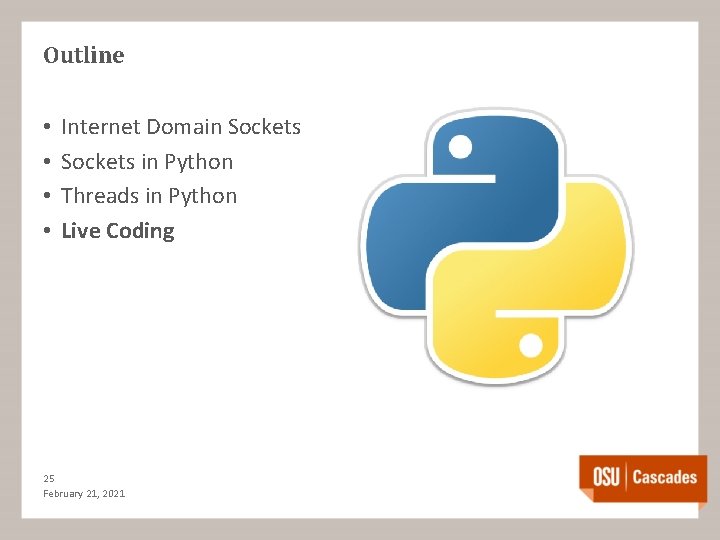
Outline • • Internet Domain Sockets in Python Threads in Python Live Coding 25 February 21, 2021
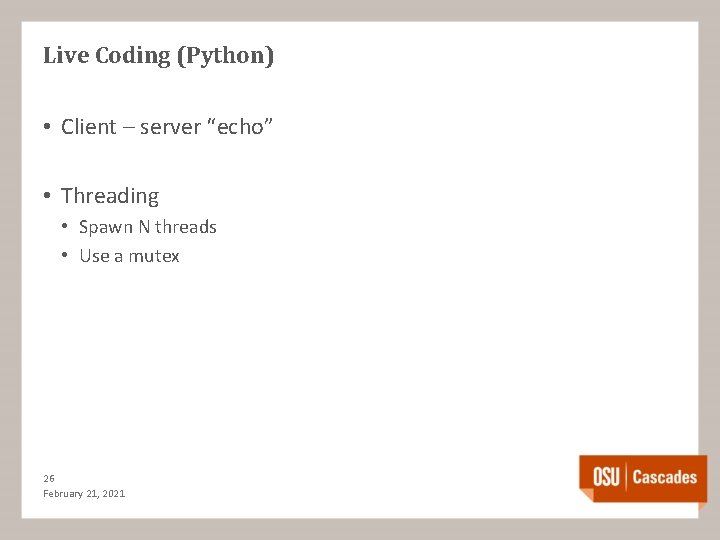
Live Coding (Python) • Client – server “echo” • Threading • Spawn N threads • Use a mutex 26 February 21, 2021