Lecture 14 The command invocation protocol Generic Protocol
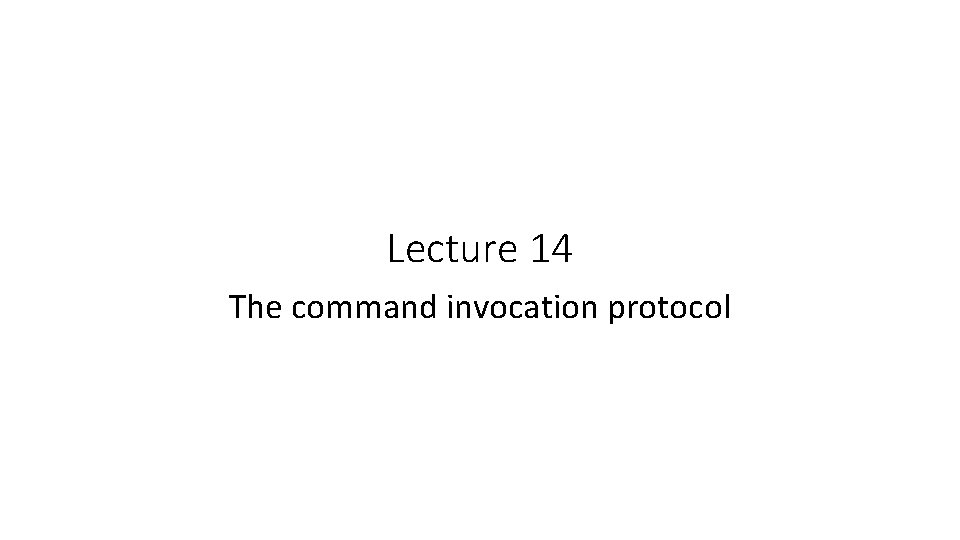
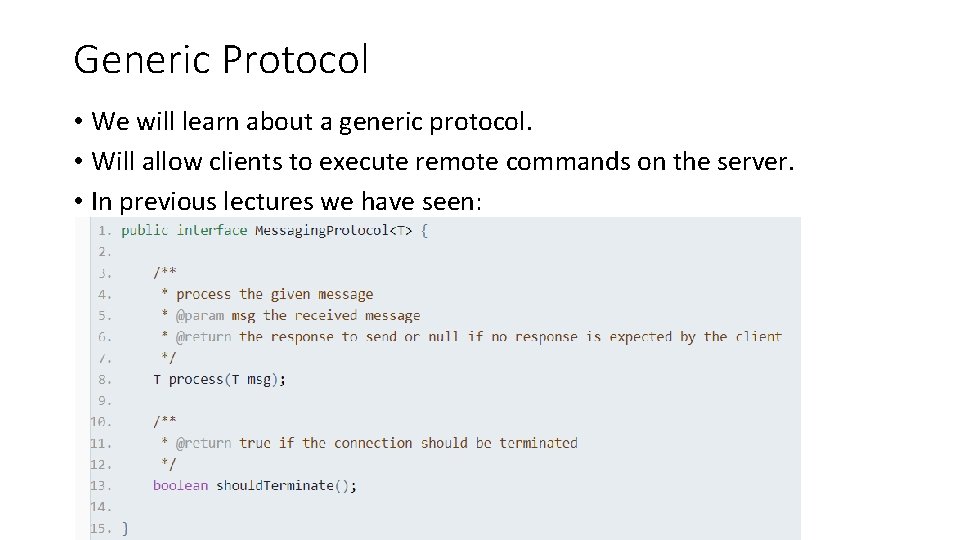
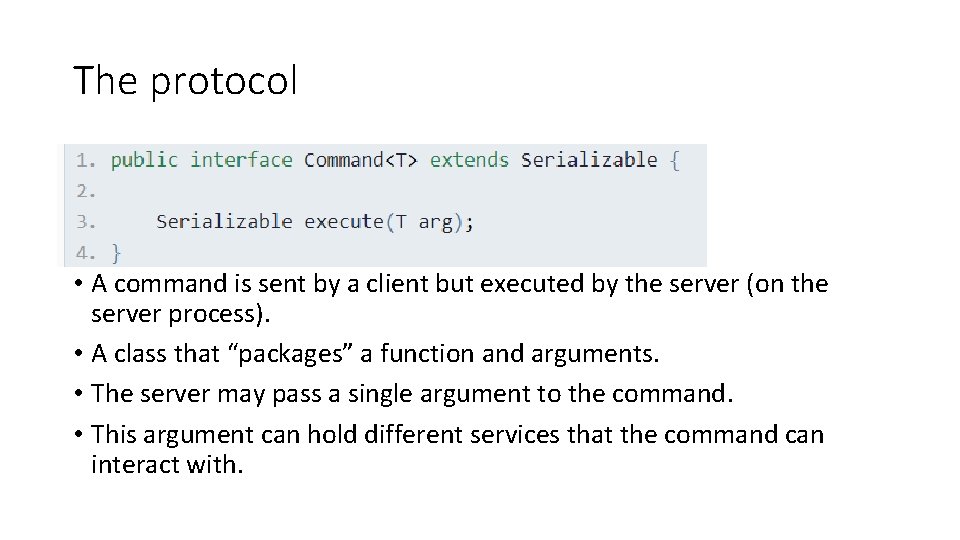
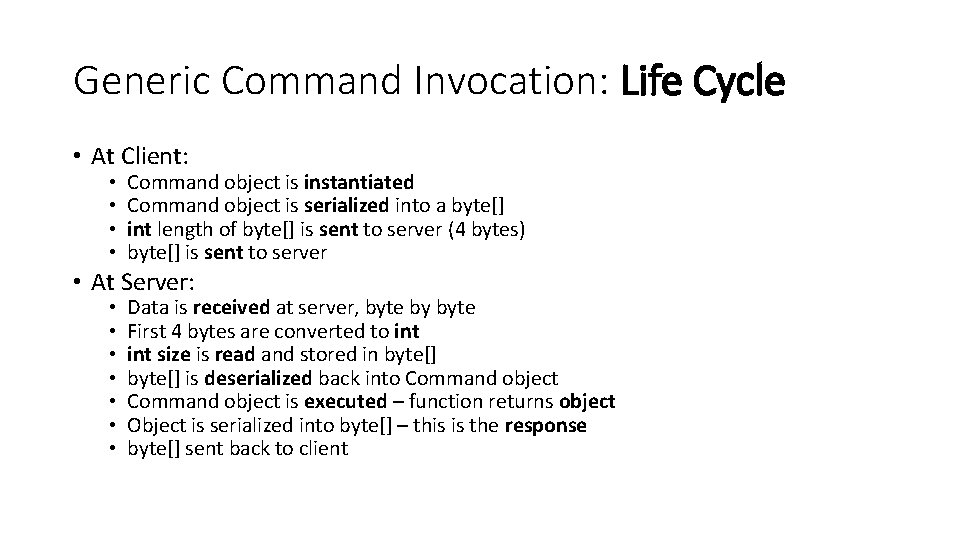
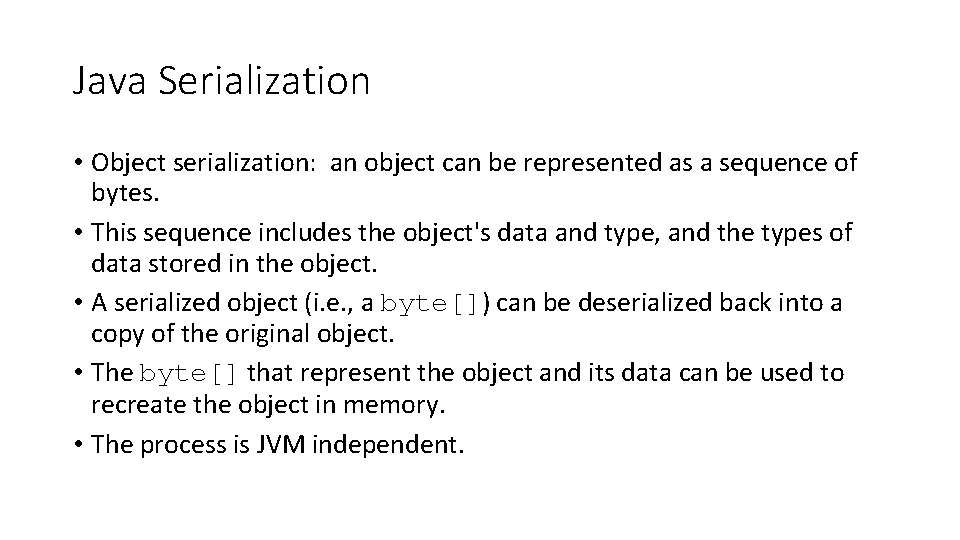
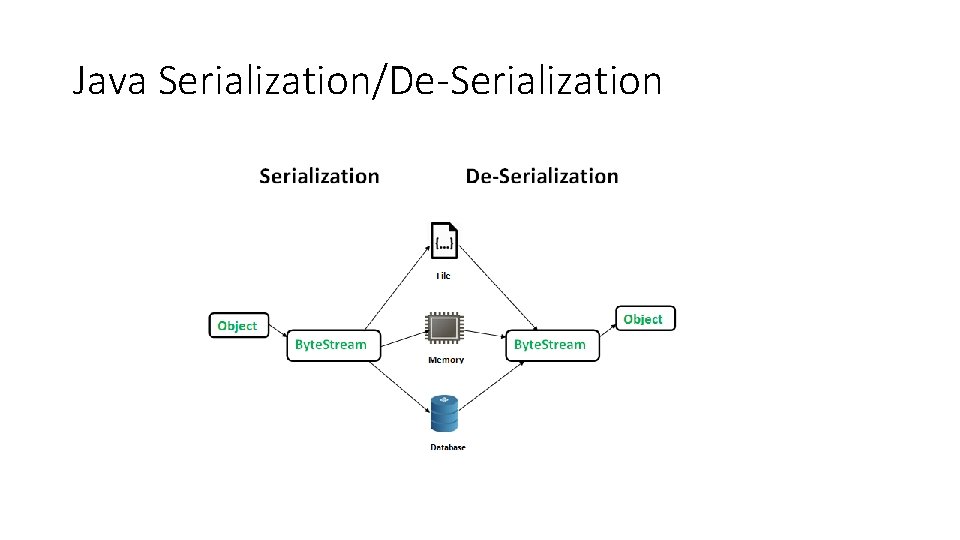
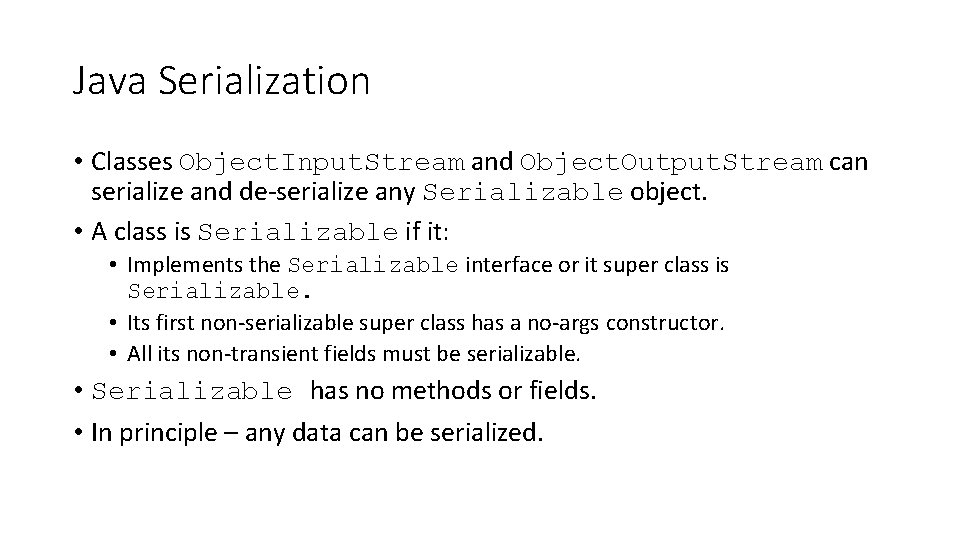
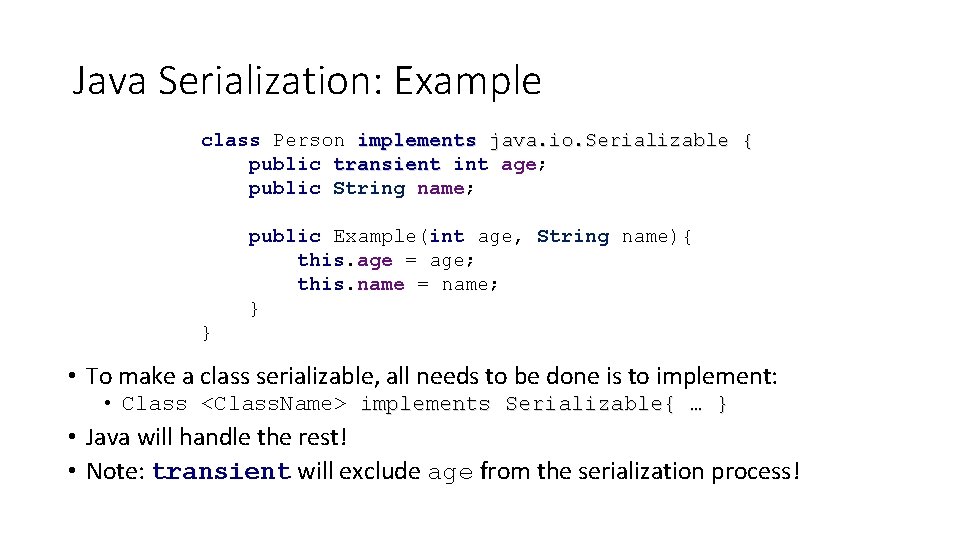
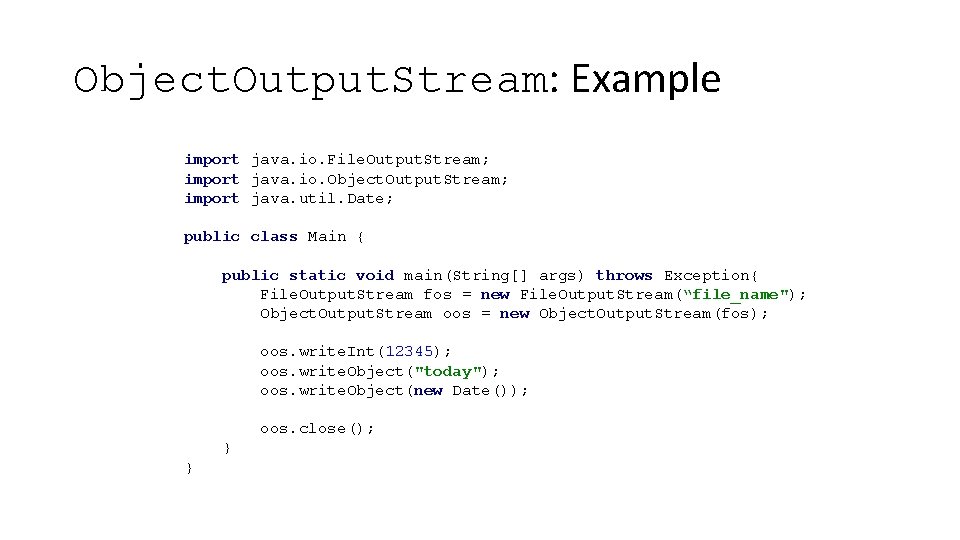
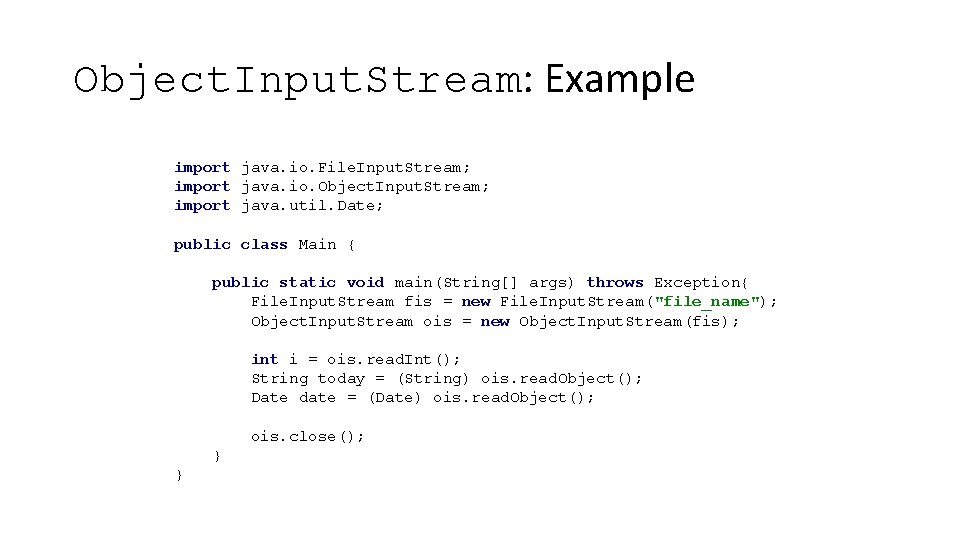
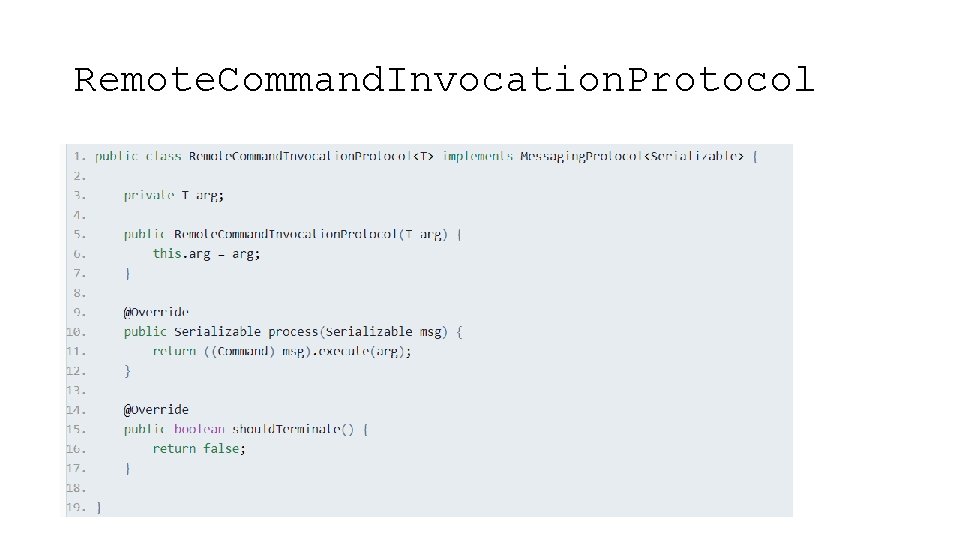
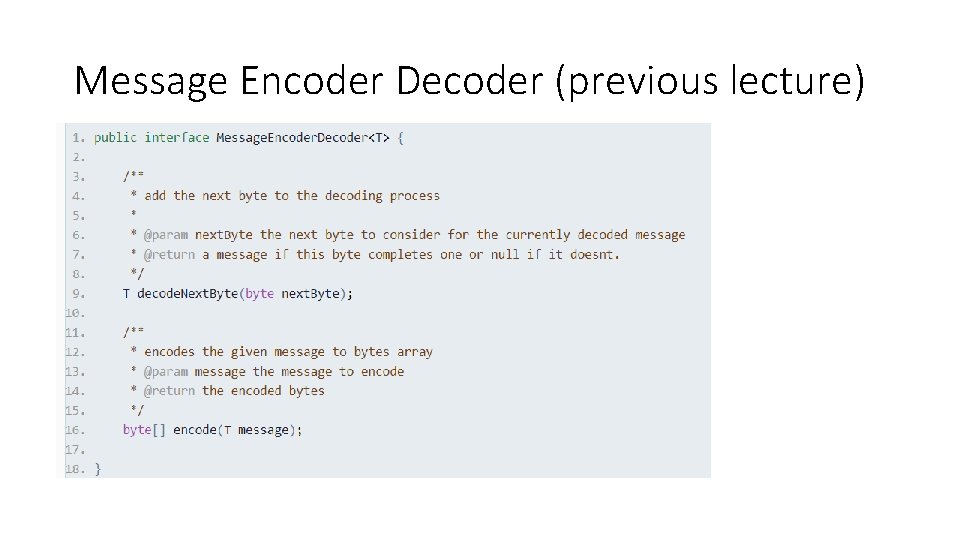
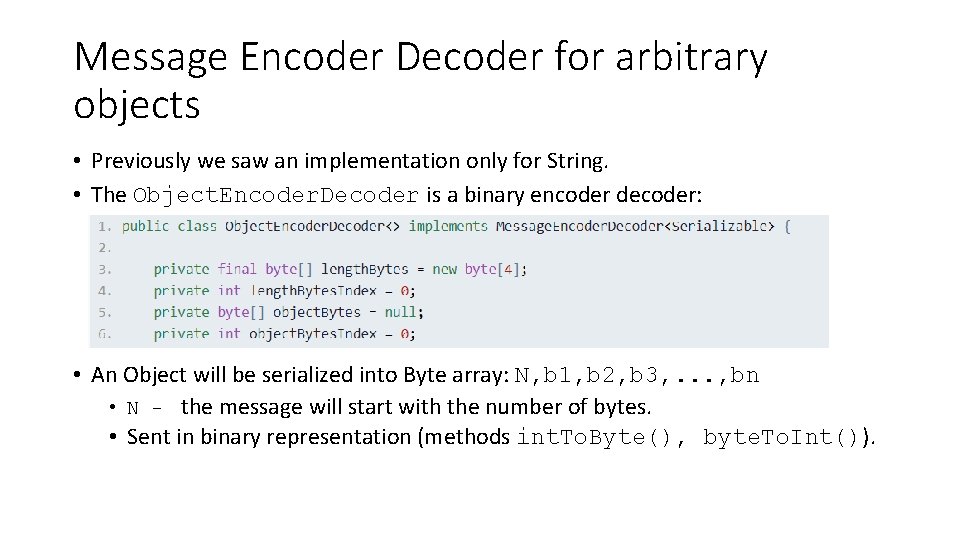
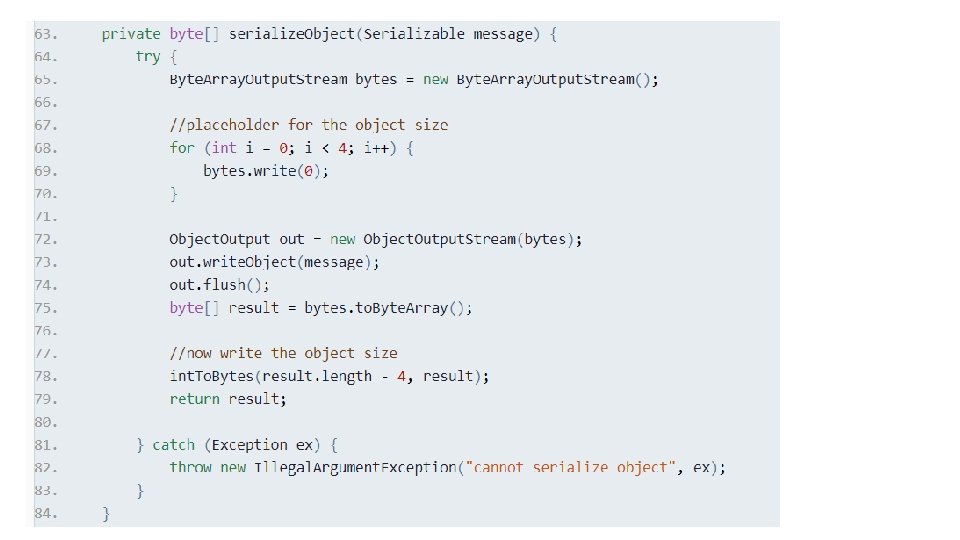
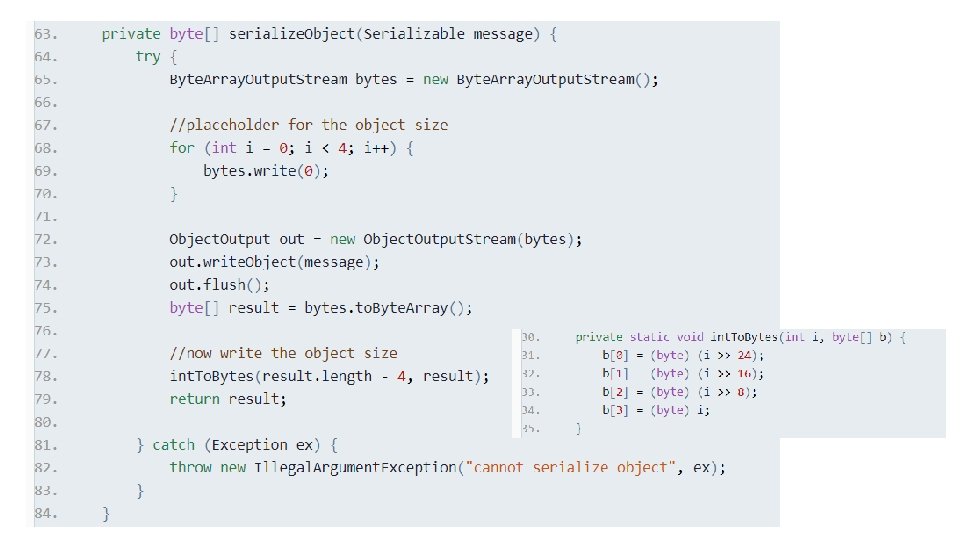
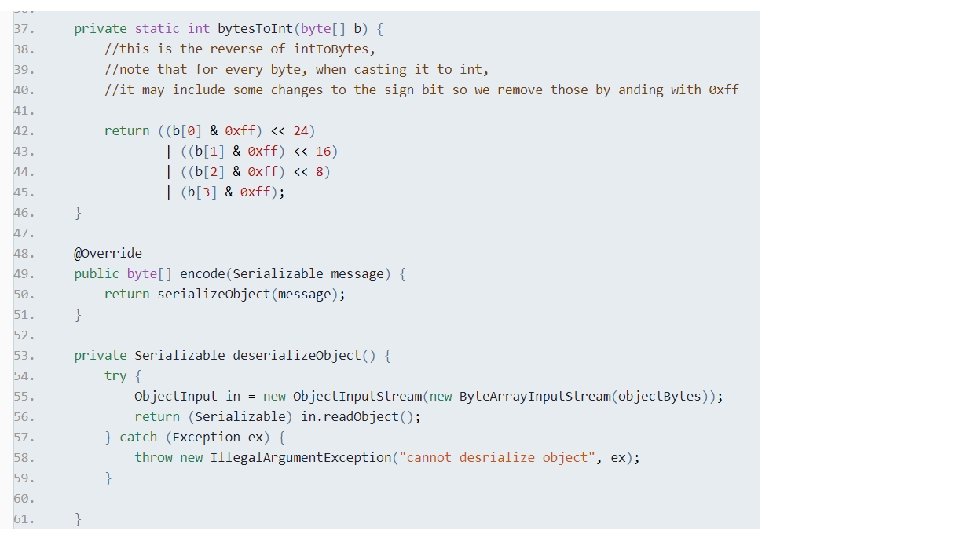
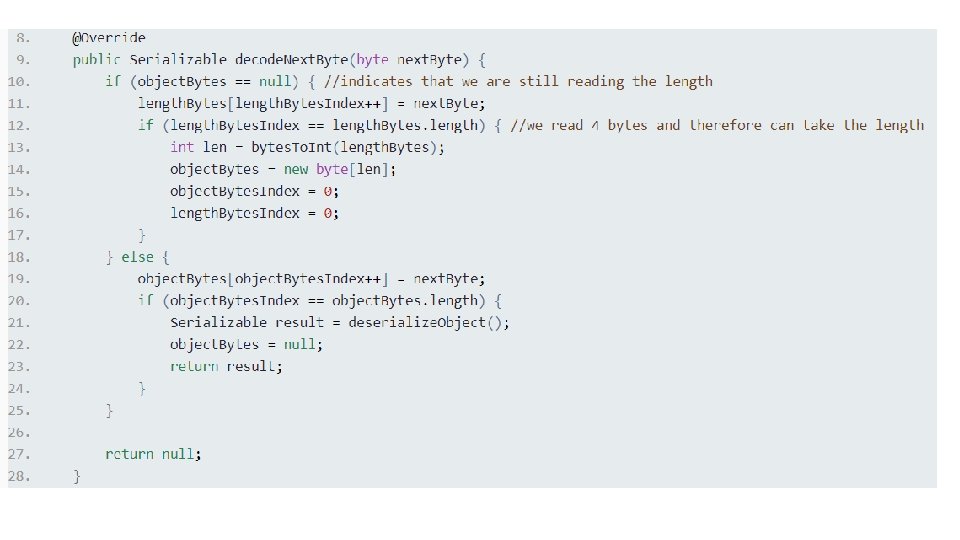
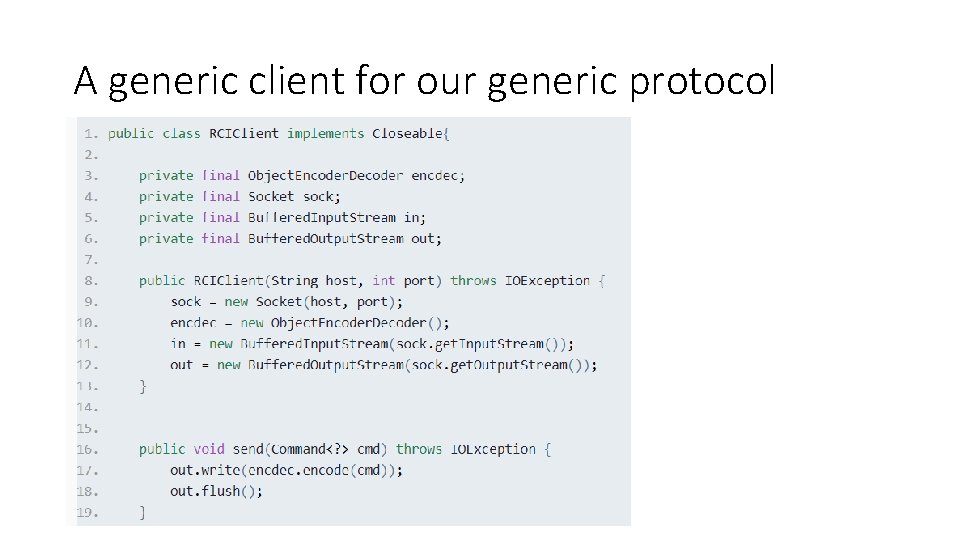
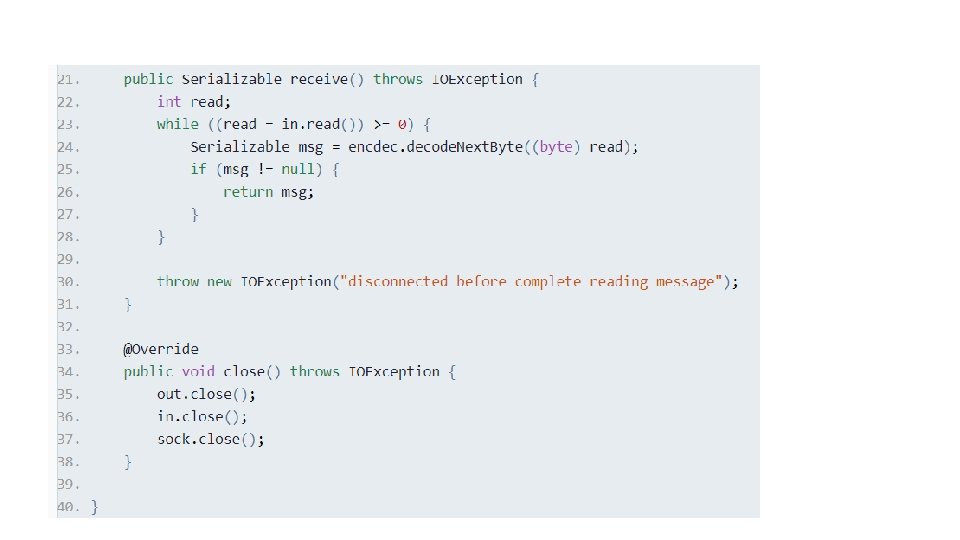
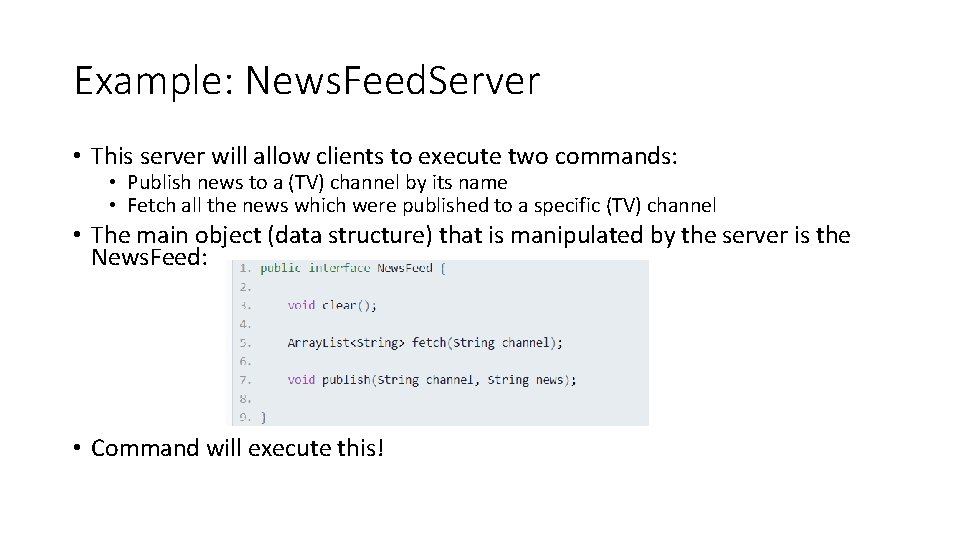
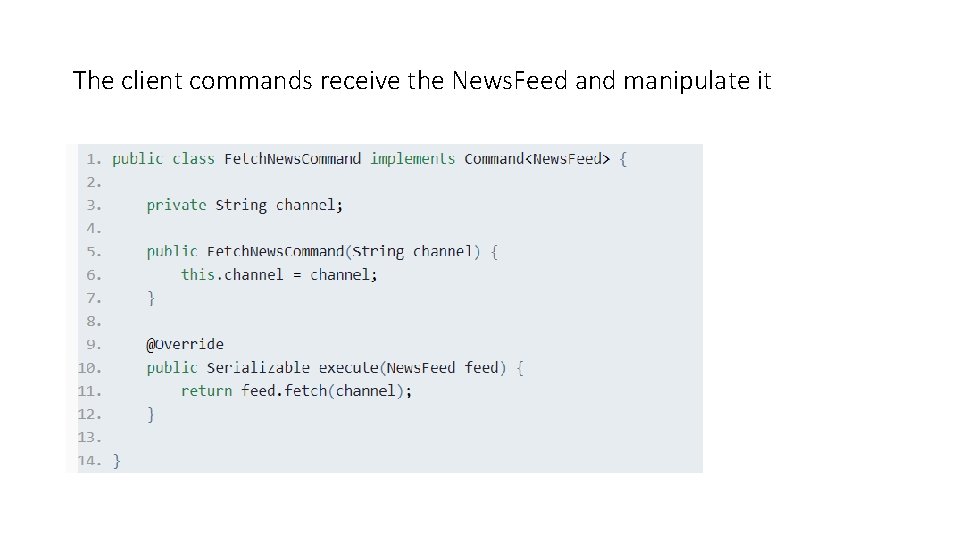
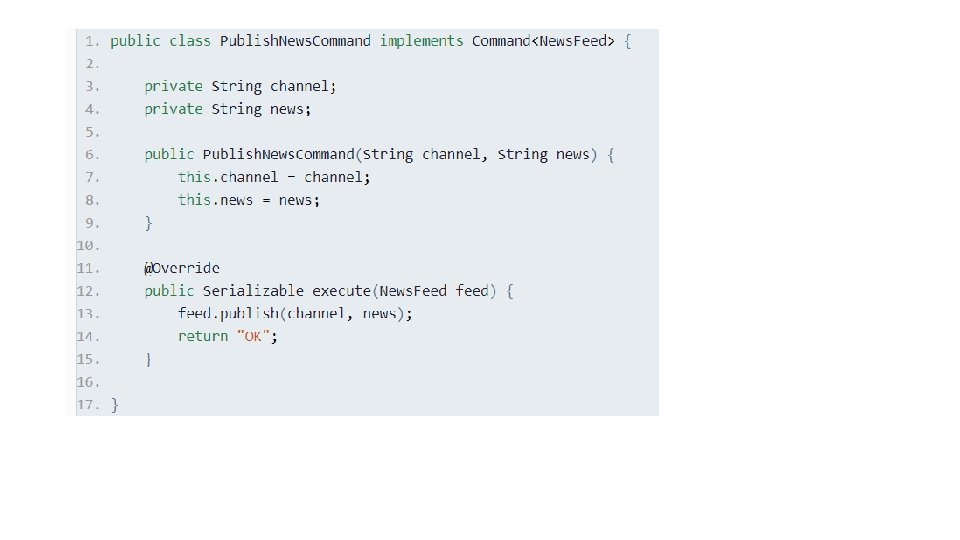
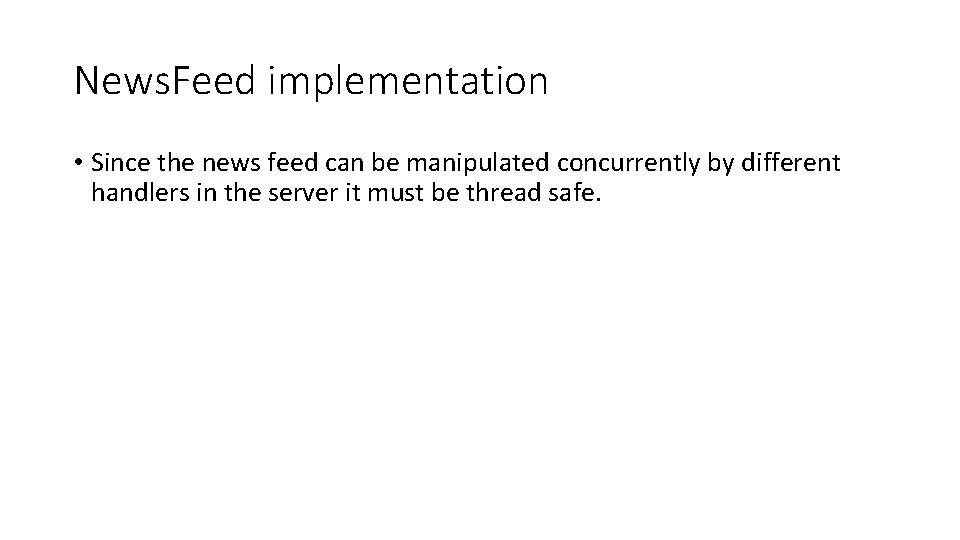
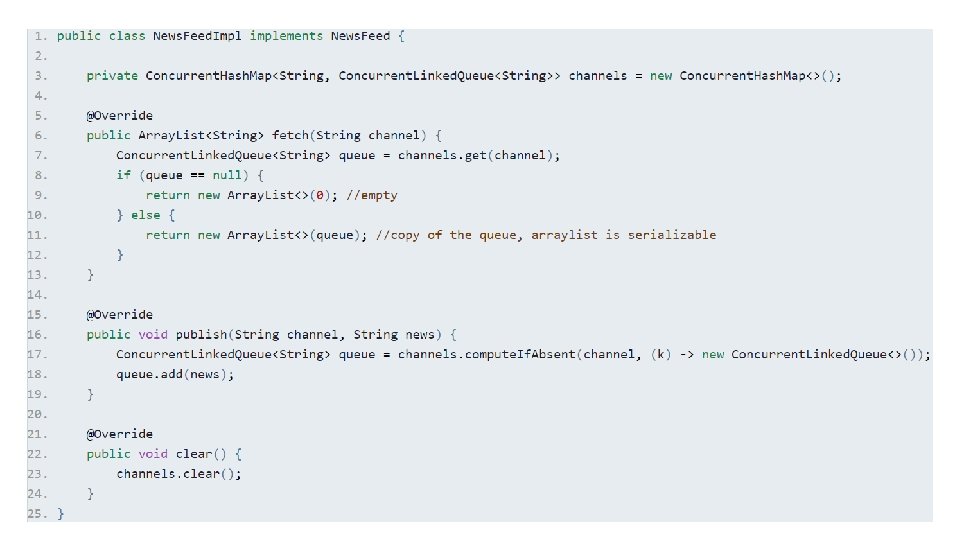
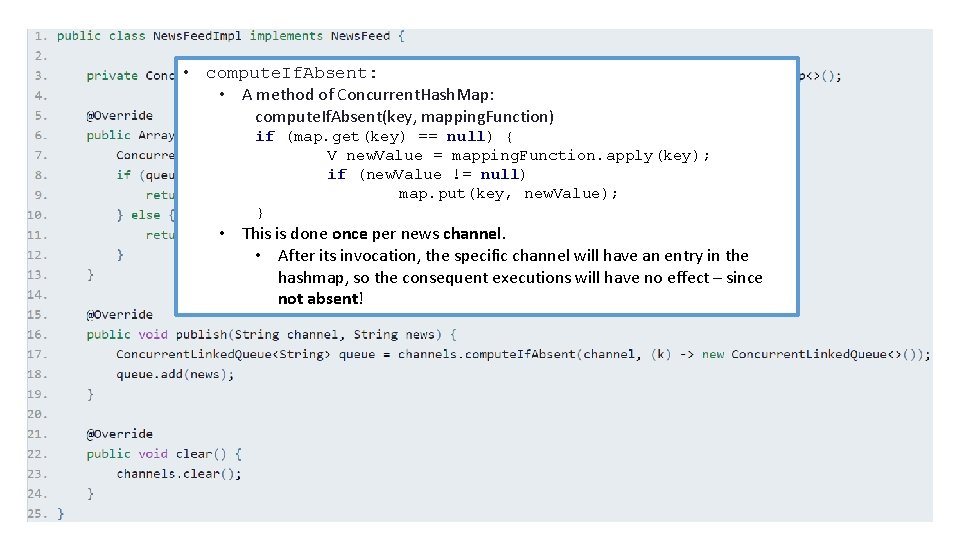
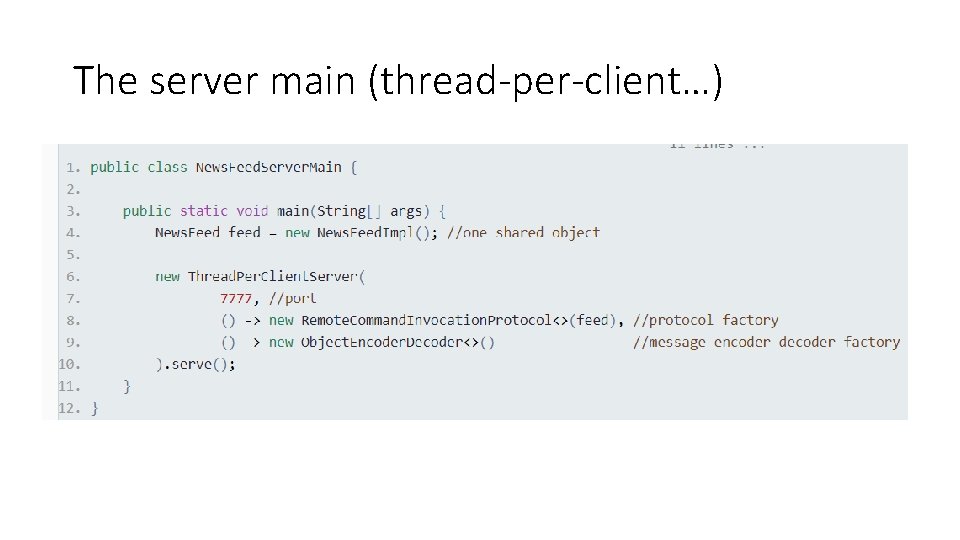
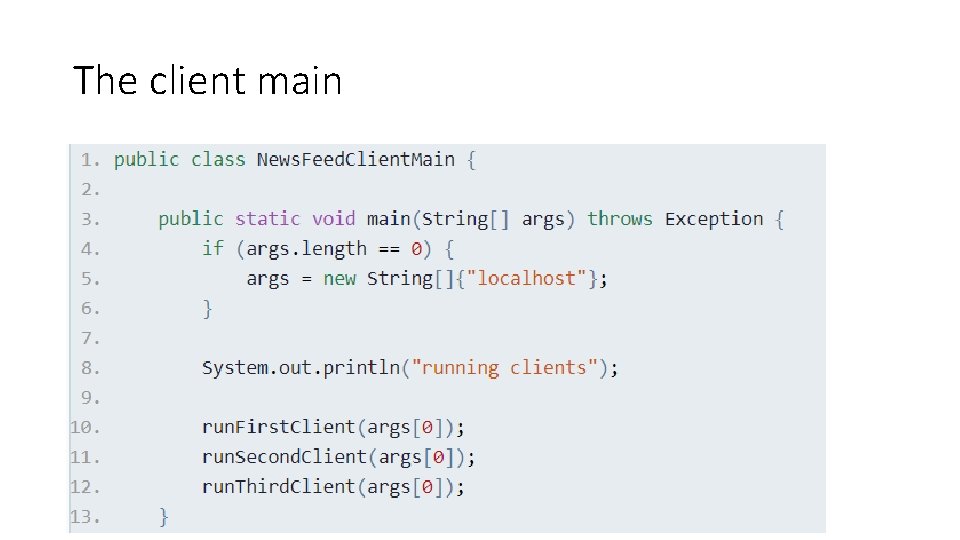
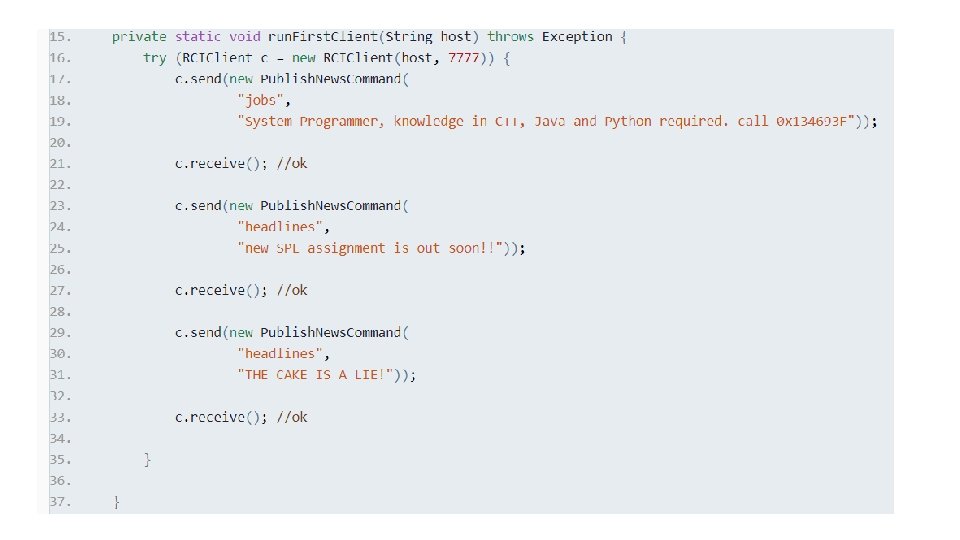
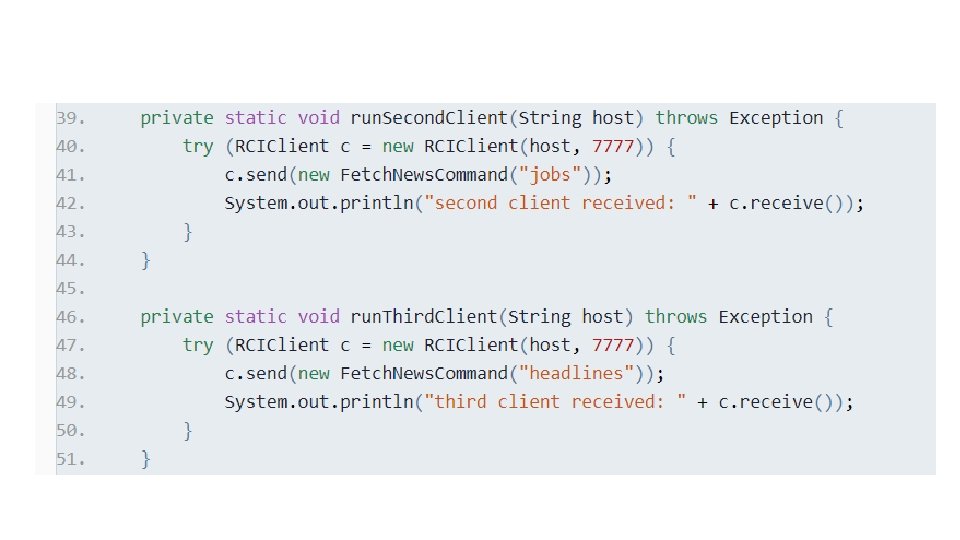
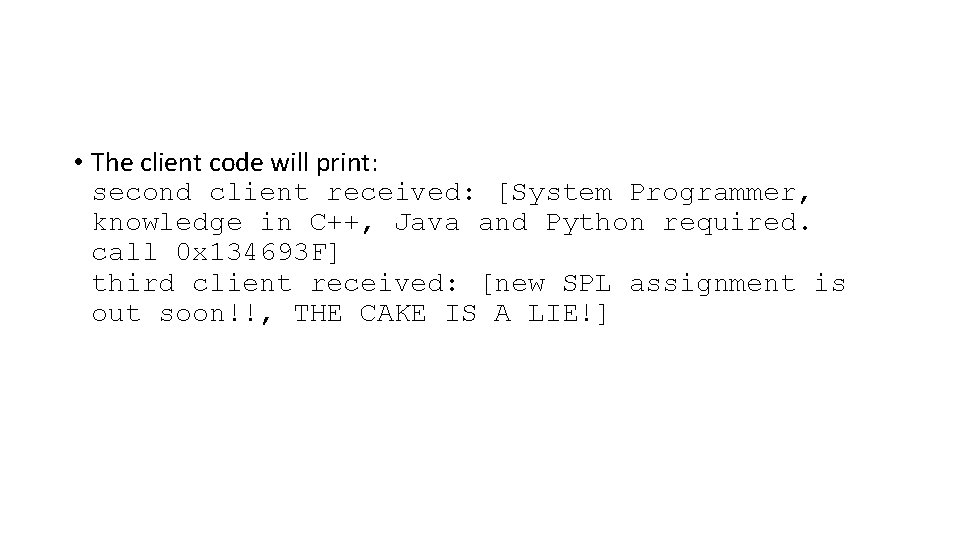
- Slides: 30
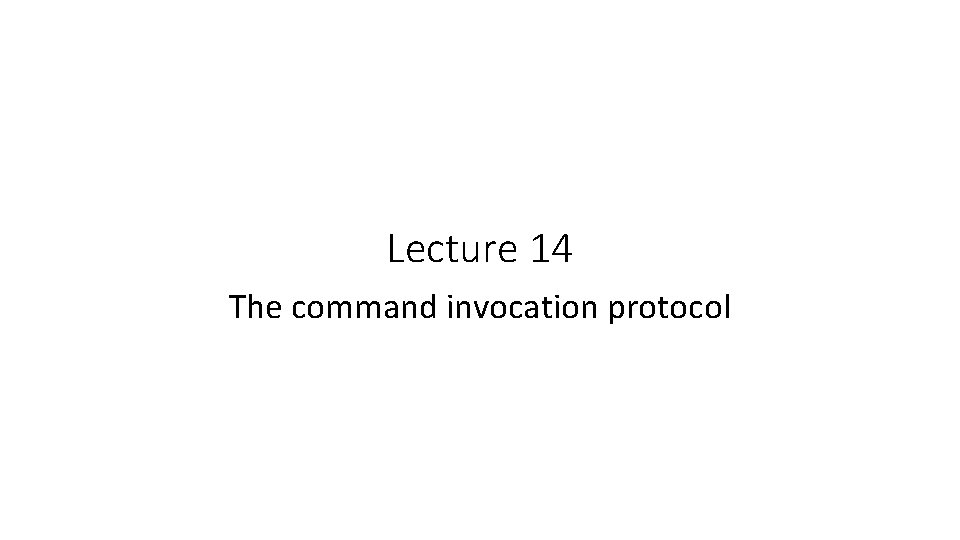
Lecture 14 The command invocation protocol
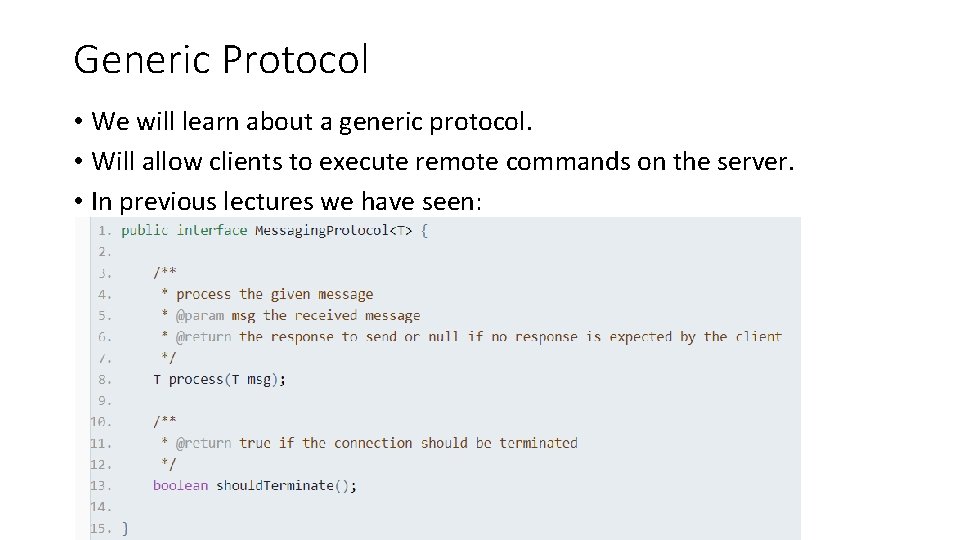
Generic Protocol • We will learn about a generic protocol. • Will allow clients to execute remote commands on the server. • In previous lectures we have seen:
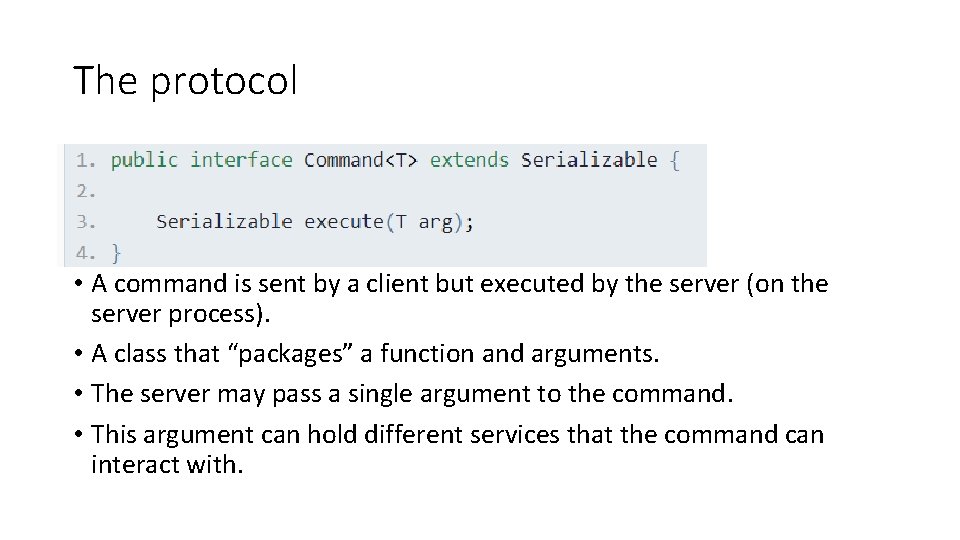
The protocol • A command is sent by a client but executed by the server (on the server process). • A class that “packages” a function and arguments. • The server may pass a single argument to the command. • This argument can hold different services that the command can interact with.
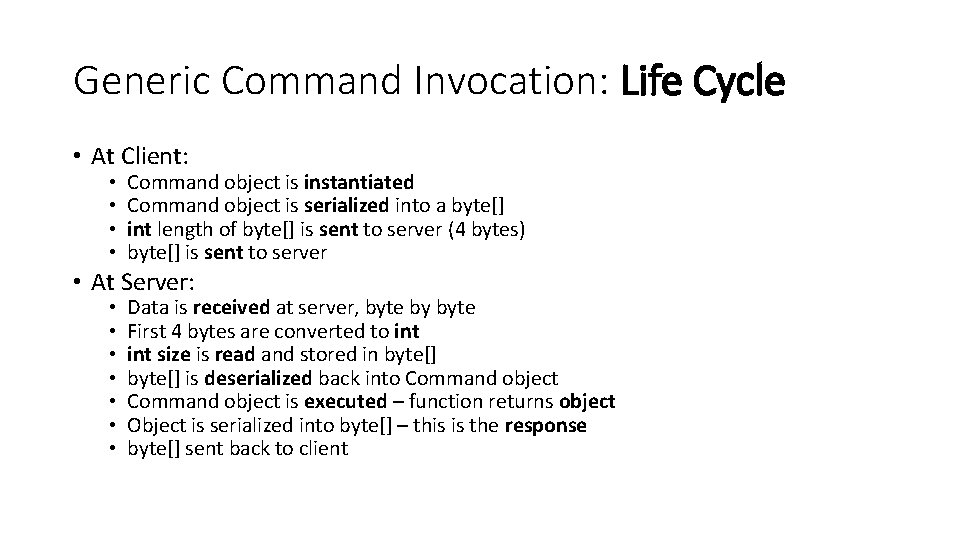
Generic Command Invocation: Life Cycle • At Client: • • Command object is instantiated Command object is serialized into a byte[] int length of byte[] is sent to server (4 bytes) byte[] is sent to server • At Server: • • Data is received at server, byte by byte First 4 bytes are converted to int size is read and stored in byte[] is deserialized back into Command object is executed – function returns object Object is serialized into byte[] – this is the response byte[] sent back to client
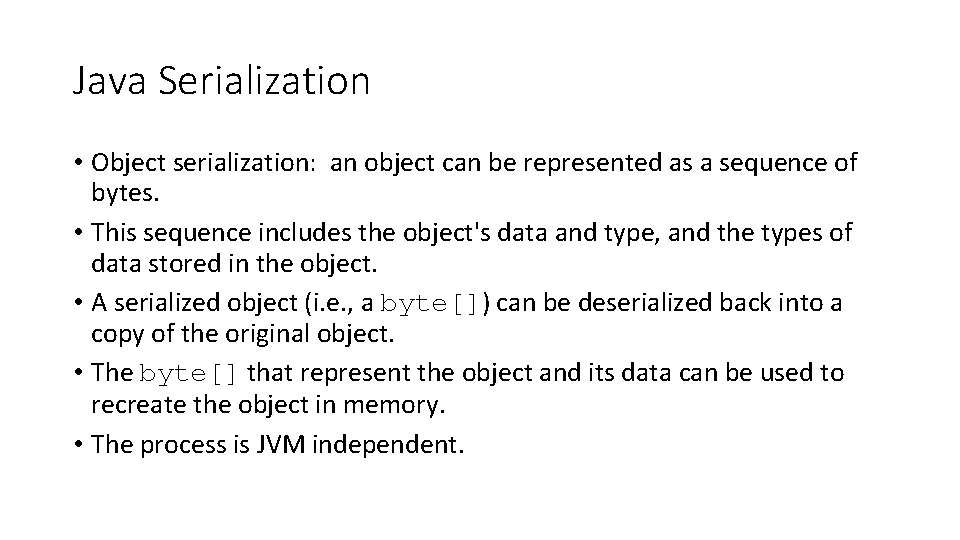
Java Serialization • Object serialization: an object can be represented as a sequence of bytes. • This sequence includes the object's data and type, and the types of data stored in the object. • A serialized object (i. e. , a byte[]) can be deserialized back into a copy of the original object. • The byte[] that represent the object and its data can be used to recreate the object in memory. • The process is JVM independent.
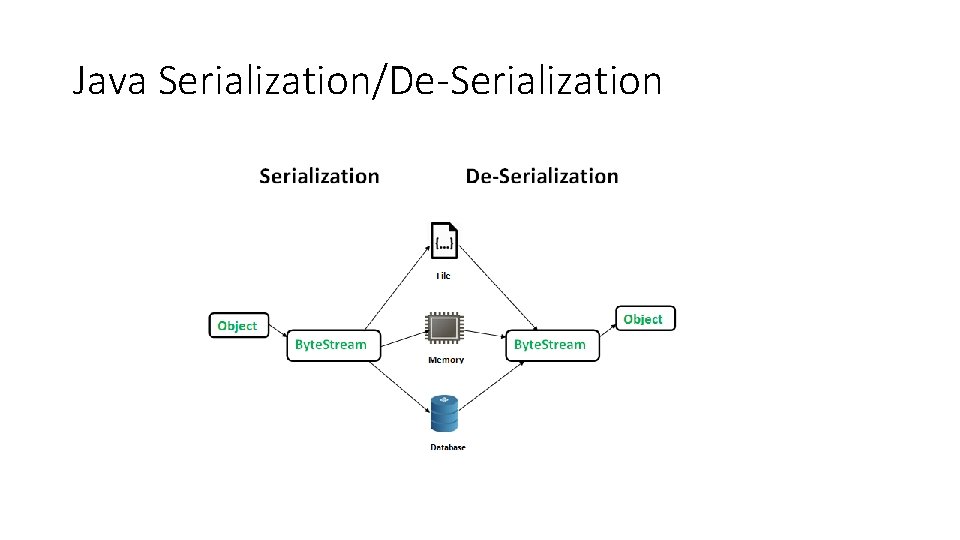
Java Serialization/De-Serialization
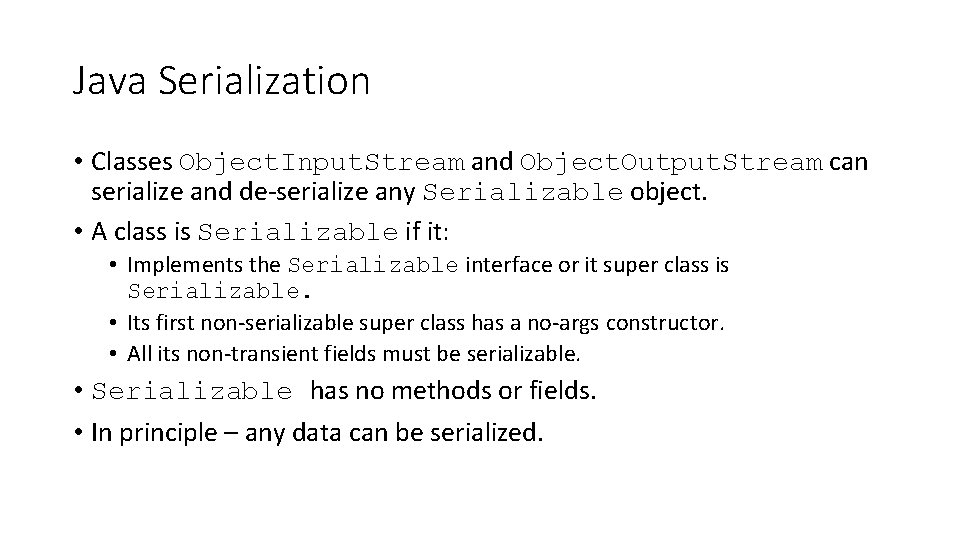
Java Serialization • Classes Object. Input. Stream and Object. Output. Stream can serialize and de-serialize any Serializable object. • A class is Serializable if it: • Implements the Serializable interface or it super class is Serializable. • Its first non-serializable super class has a no-args constructor. • All its non-transient fields must be serializable. • Serializable has no methods or fields. • In principle – any data can be serialized.
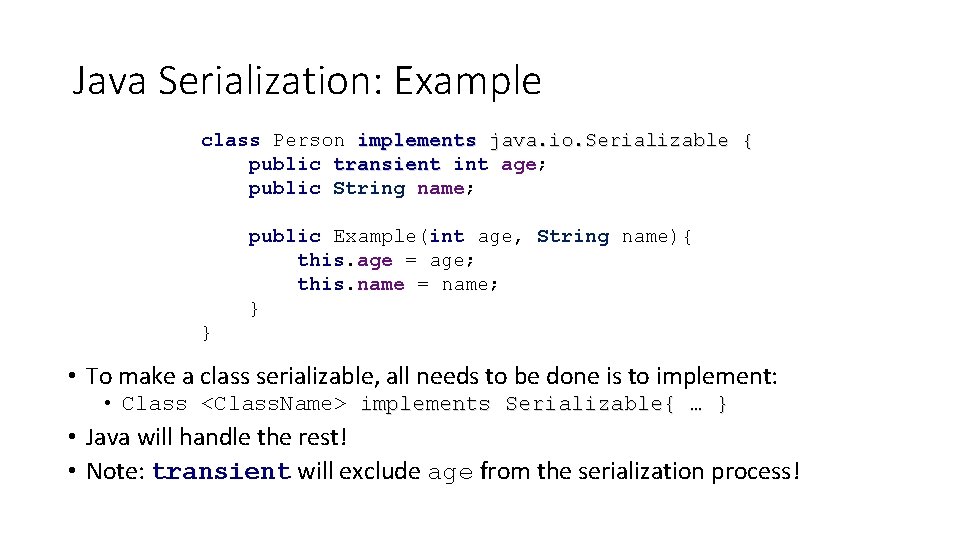
Java Serialization: Example class Person implements java. io. Serializable { public transient int age; public String name; public Example(int age, String name){ this. age = age; this. name = name; } } • To make a class serializable, all needs to be done is to implement: • Class <Class. Name> implements Serializable{ … } • Java will handle the rest! • Note: transient will exclude age from the serialization process!
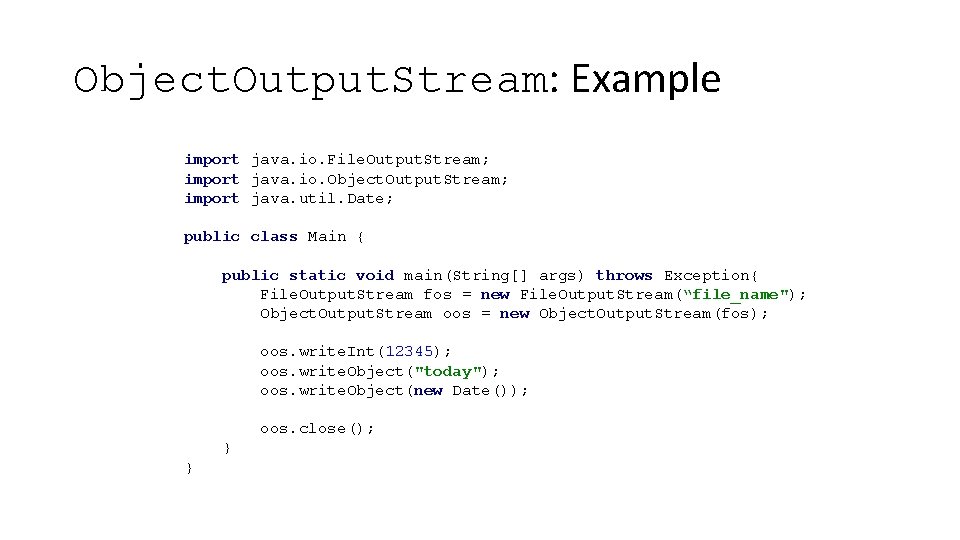
Object. Output. Stream: Example import java. io. File. Output. Stream; import java. io. Object. Output. Stream; import java. util. Date; public class Main { public static void main(String[] args) throws Exception{ File. Output. Stream fos = new File. Output. Stream(“file_name"); Object. Output. Stream oos = new Object. Output. Stream(fos); oos. write. Int(12345); oos. write. Object("today"); oos. write. Object(new Date()); oos. close(); } }
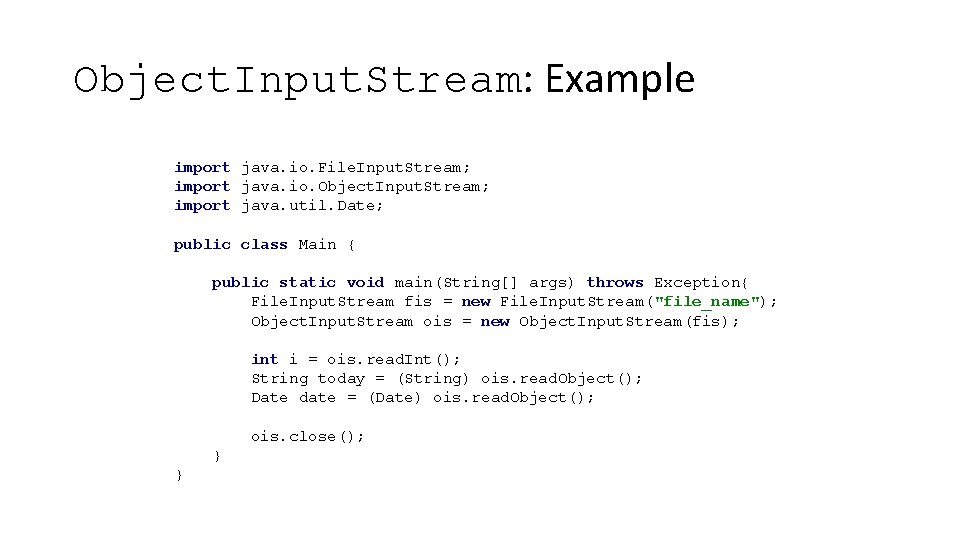
Object. Input. Stream: Example import java. io. File. Input. Stream; import java. io. Object. Input. Stream; import java. util. Date; public class Main { public static void main(String[] args) throws Exception{ File. Input. Stream fis = new File. Input. Stream("file_name"); Object. Input. Stream ois = new Object. Input. Stream(fis); int i = ois. read. Int(); String today = (String) ois. read. Object(); Date date = (Date) ois. read. Object(); ois. close(); } }
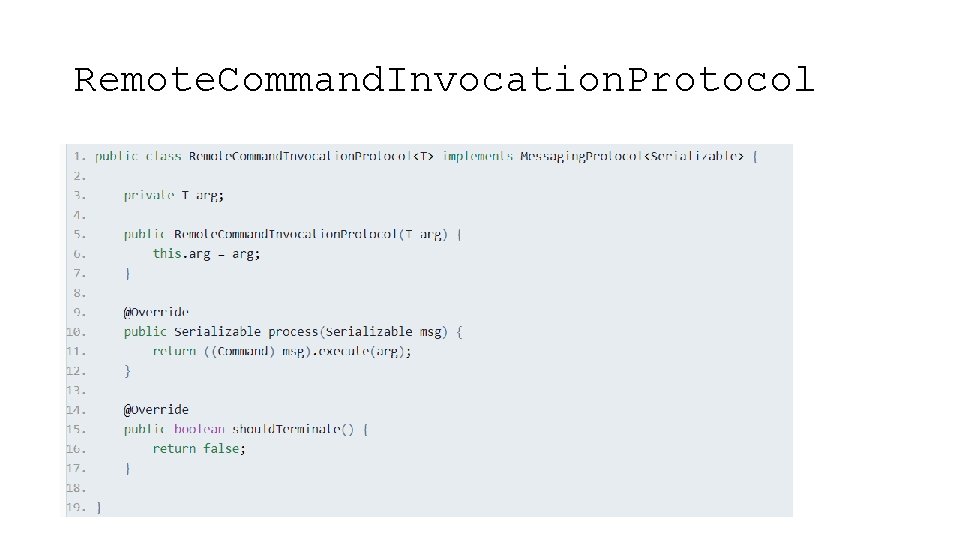
Remote. Command. Invocation. Protocol
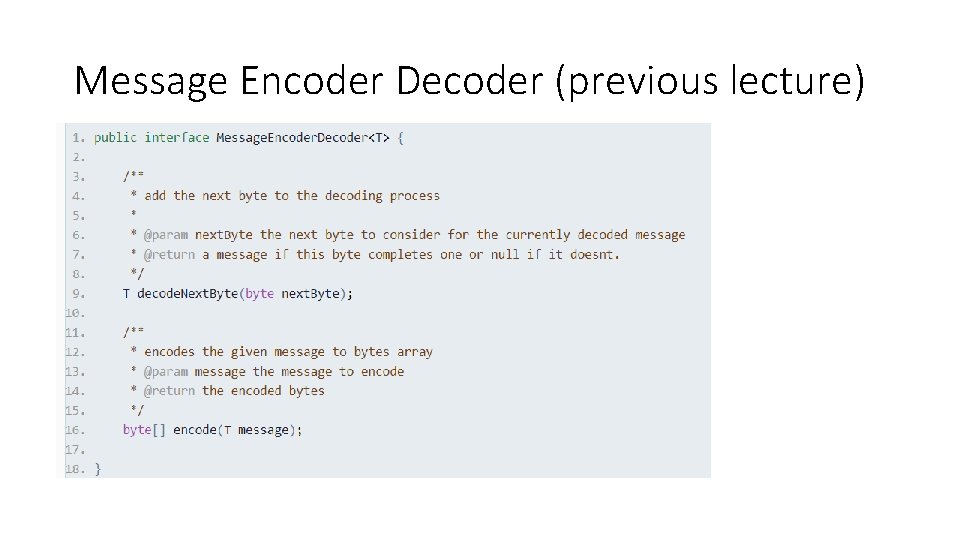
Message Encoder Decoder (previous lecture)
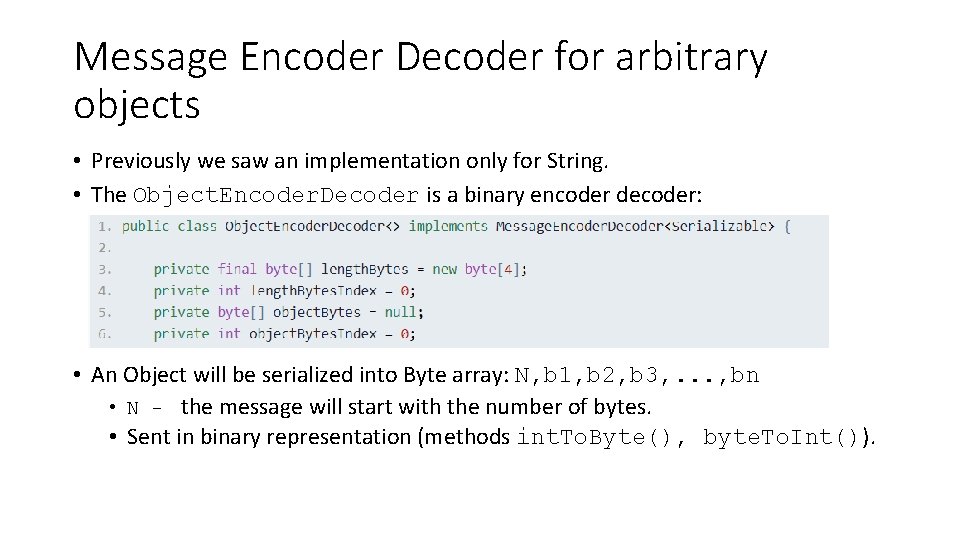
Message Encoder Decoder for arbitrary objects • Previously we saw an implementation only for String. • The Object. Encoder. Decoder is a binary encoder decoder: • An Object will be serialized into Byte array: N, b 1, b 2, b 3, . . . , bn • N - the message will start with the number of bytes. • Sent in binary representation (methods int. To. Byte(), byte. To. Int()).
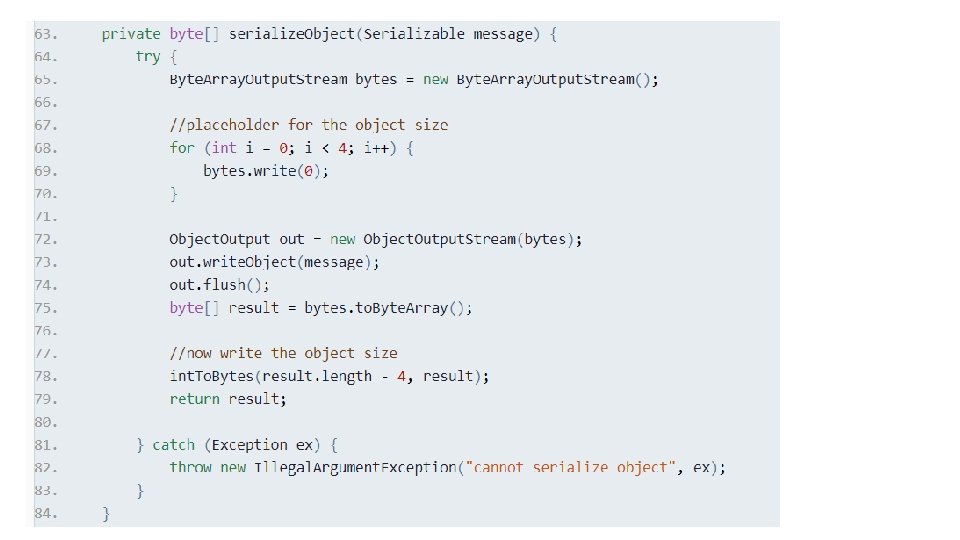
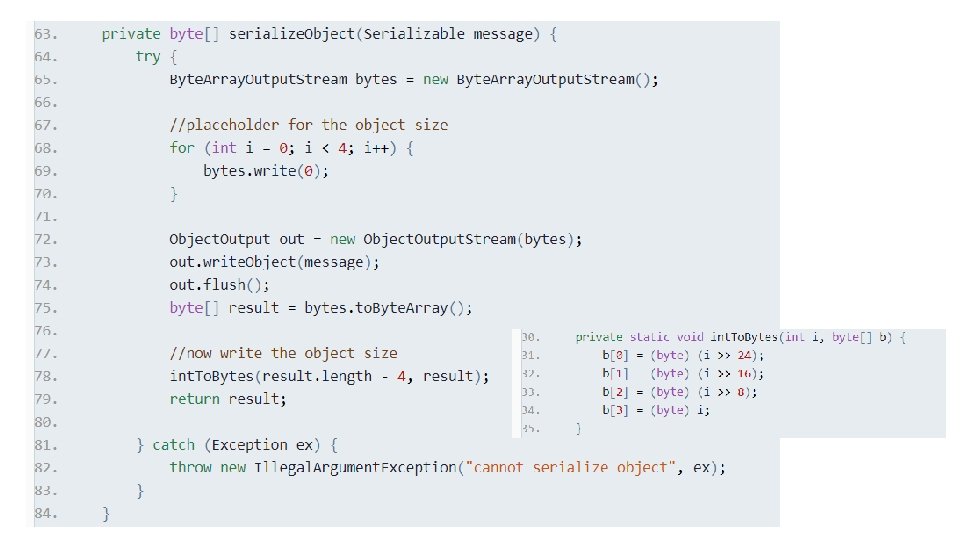
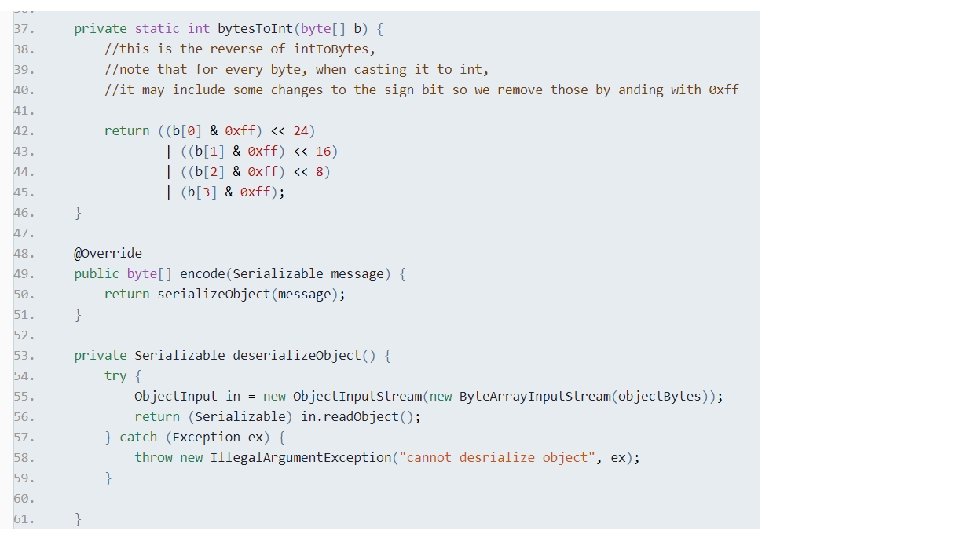
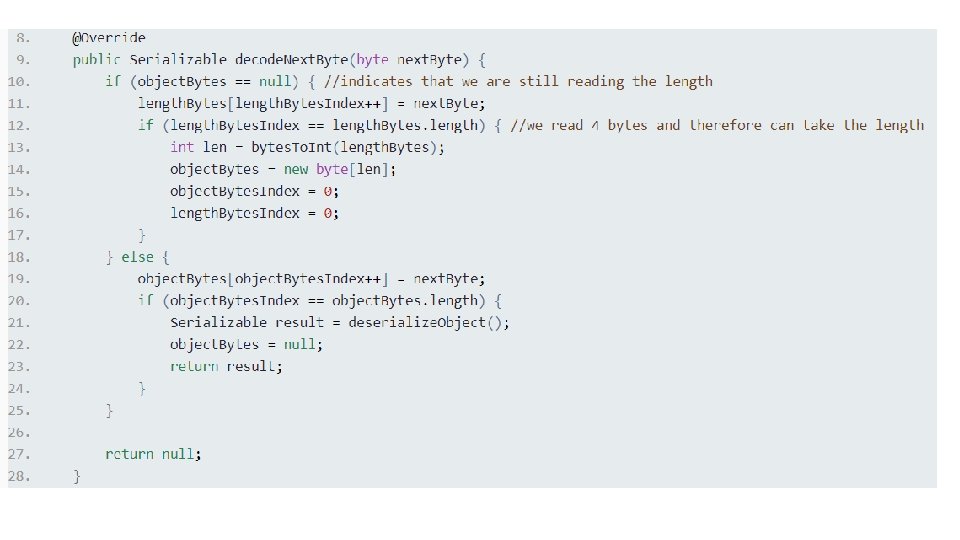
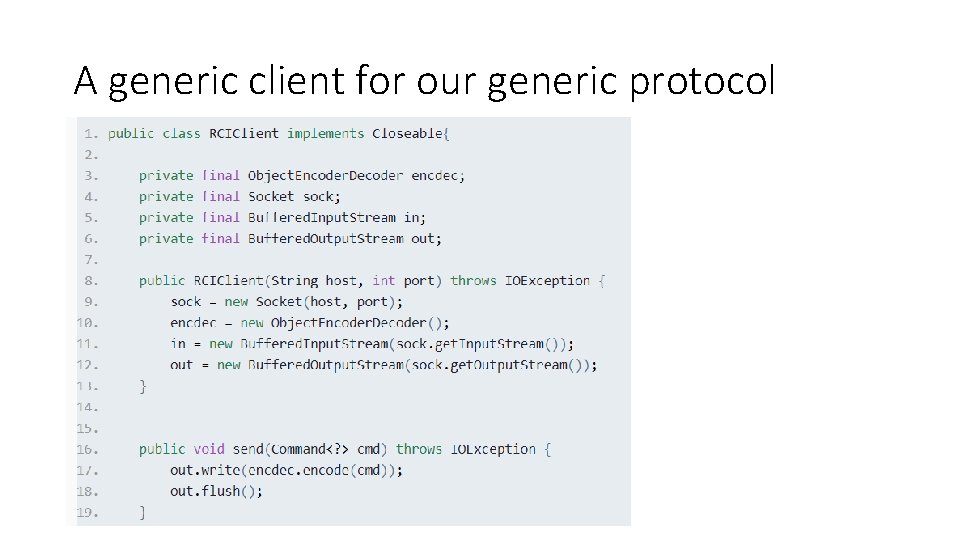
A generic client for our generic protocol
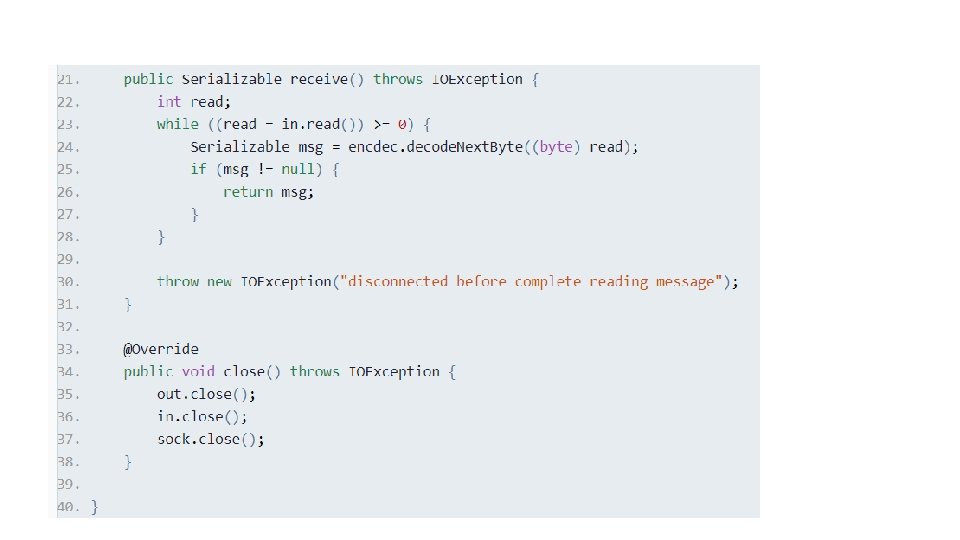
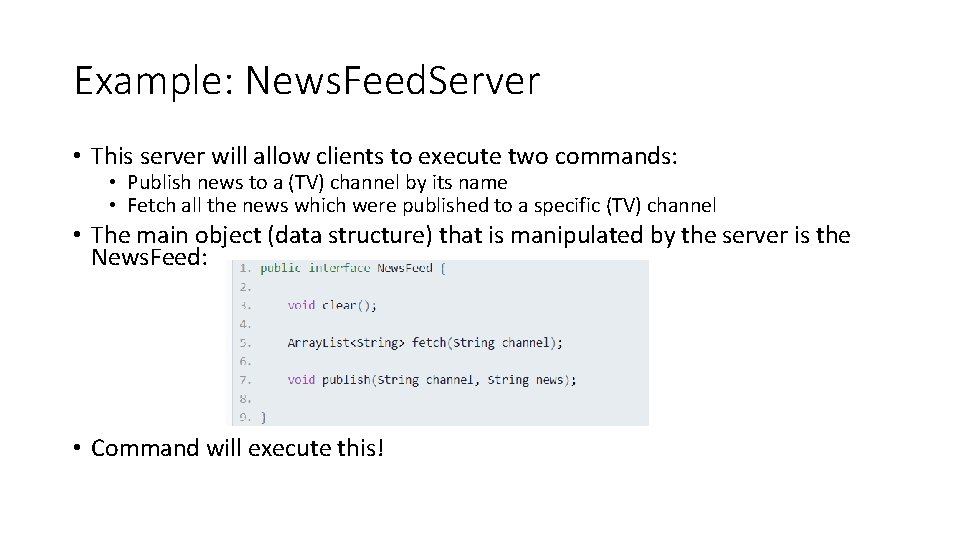
Example: News. Feed. Server • This server will allow clients to execute two commands: • Publish news to a (TV) channel by its name • Fetch all the news which were published to a specific (TV) channel • The main object (data structure) that is manipulated by the server is the News. Feed: • Command will execute this!
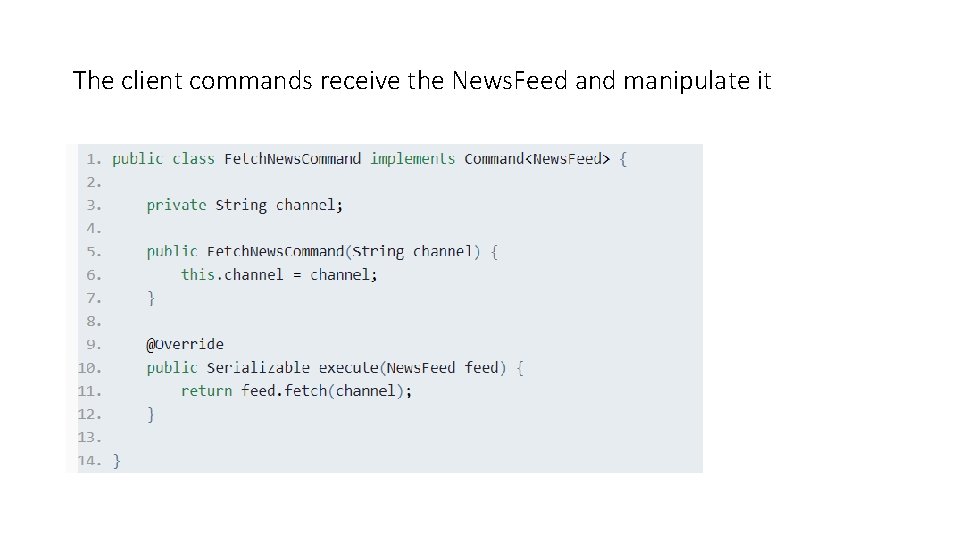
The client commands receive the News. Feed and manipulate it
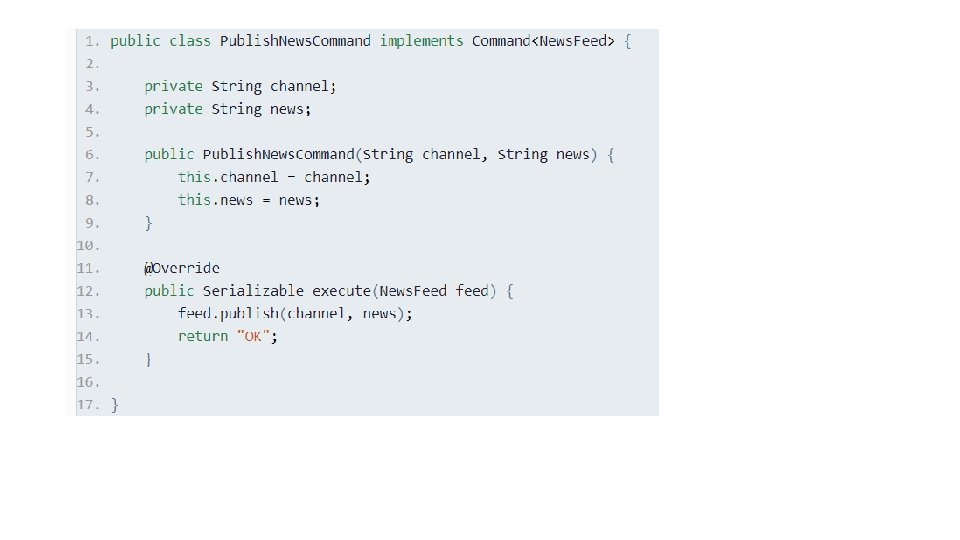
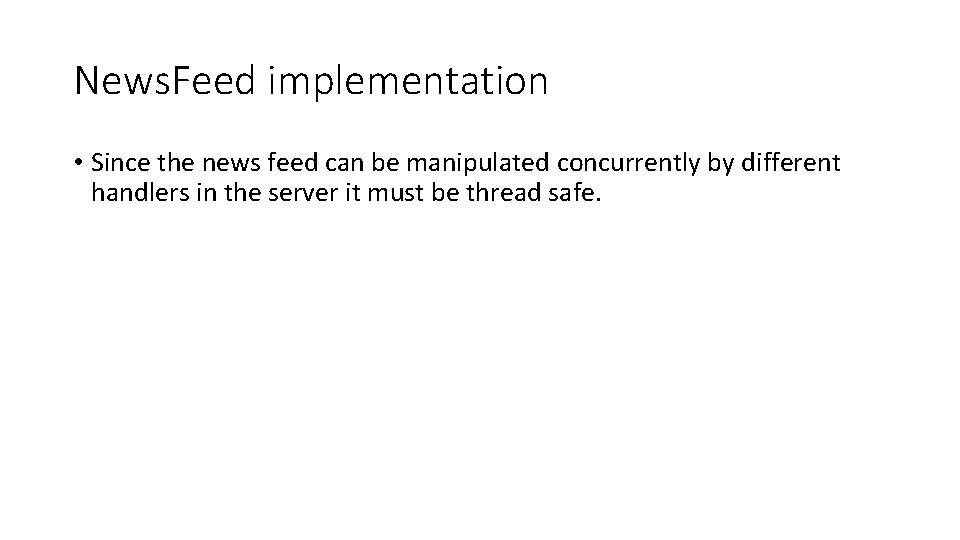
News. Feed implementation • Since the news feed can be manipulated concurrently by different handlers in the server it must be thread safe.
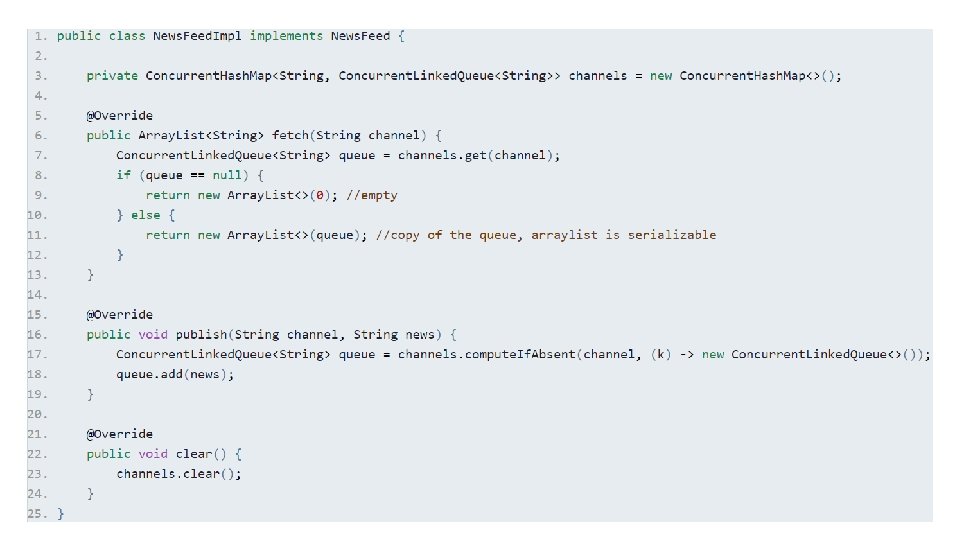
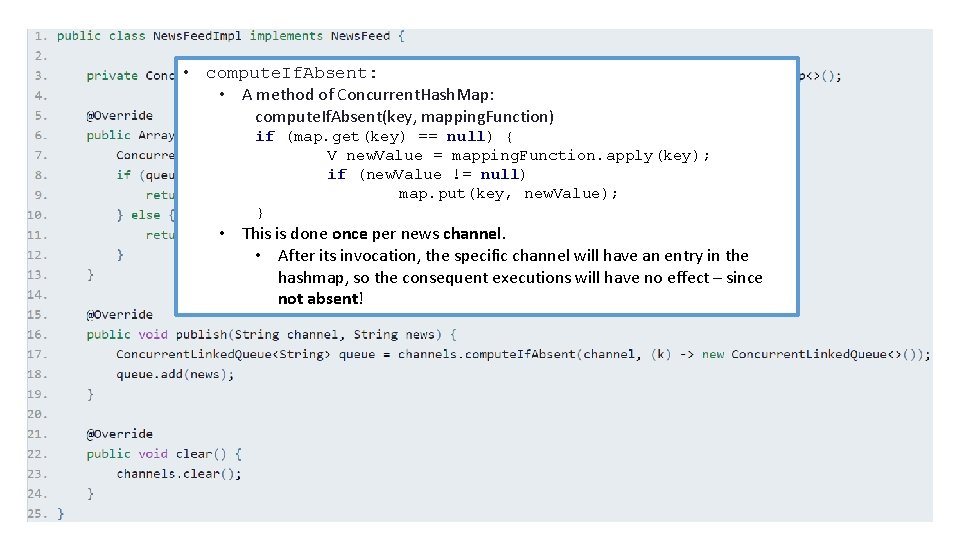
• compute. If. Absent: • A method of Concurrent. Hash. Map: compute. If. Absent(key, mapping. Function) if (map. get(key) == null) { V new. Value = mapping. Function. apply(key); if (new. Value != null) map. put(key, new. Value); } • This is done once per news channel. • After its invocation, the specific channel will have an entry in the hashmap, so the consequent executions will have no effect – since not absent!
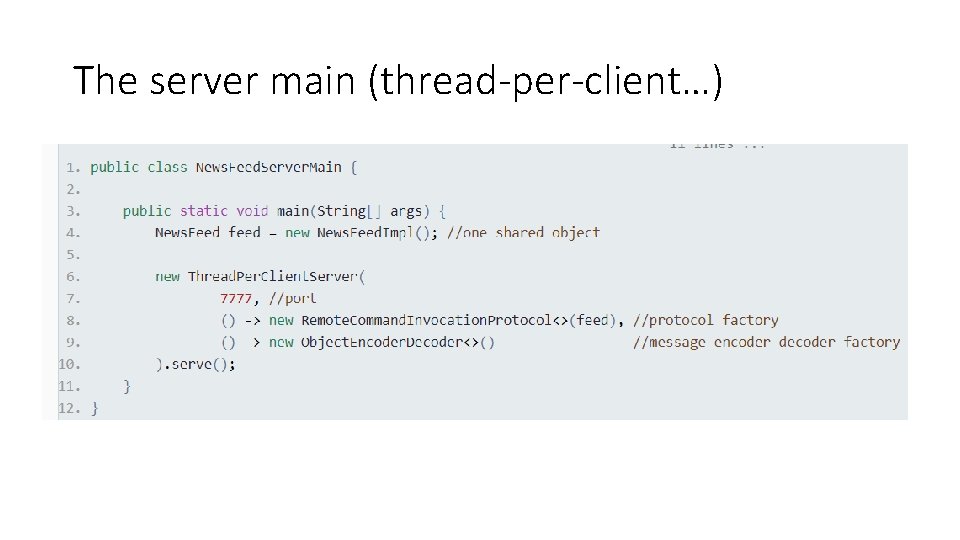
The server main (thread-per-client…)
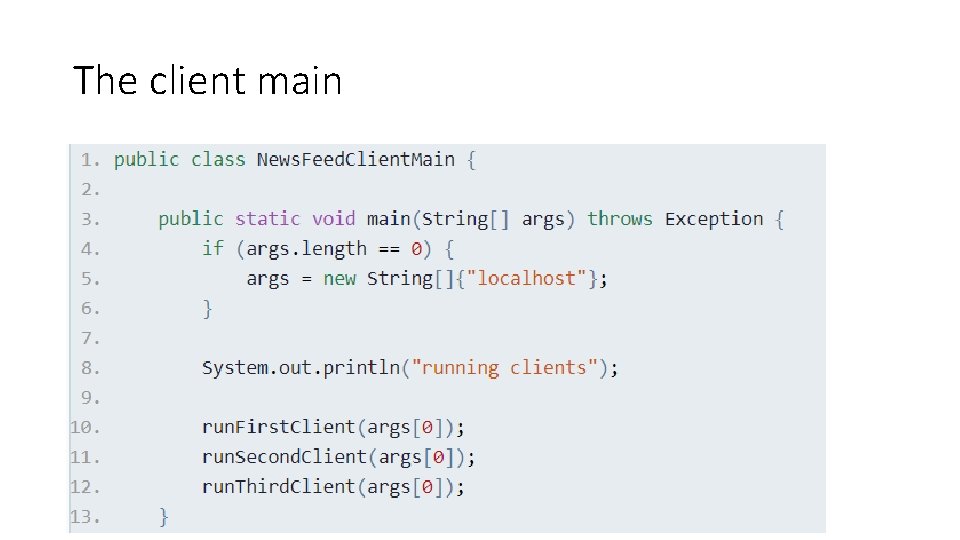
The client main
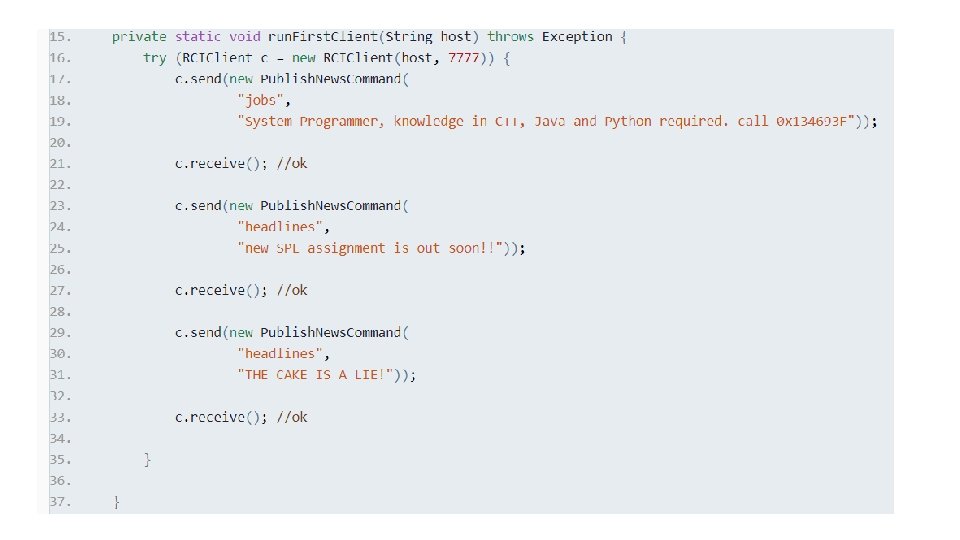
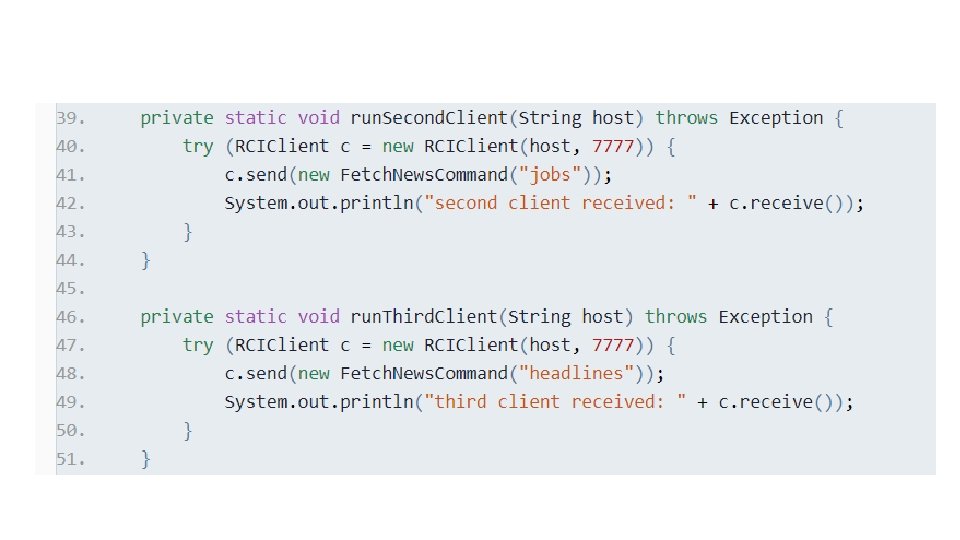
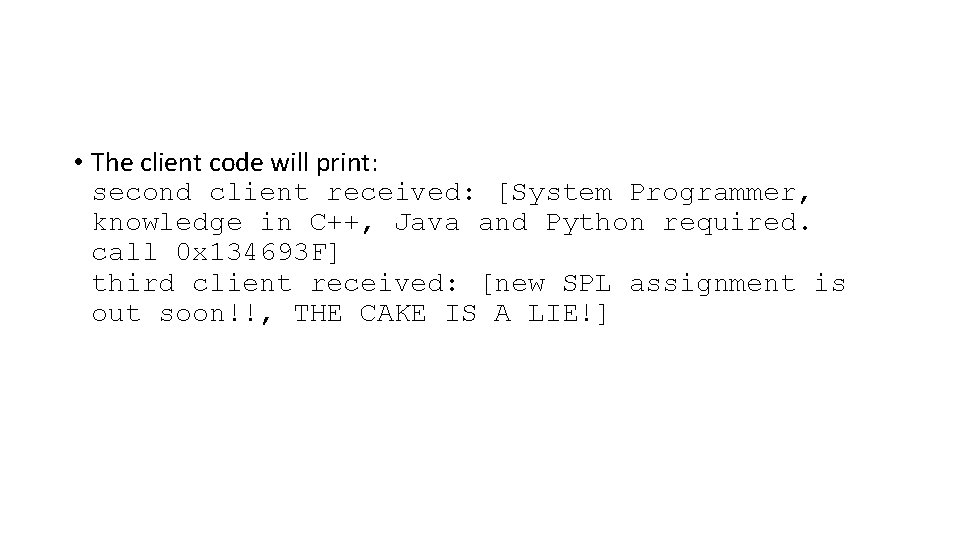
• The client code will print: second client received: [System Programmer, knowledge in C++, Java and Python required. call 0 x 134693 F] third client received: [new SPL assignment is out soon!!, THE CAKE IS A LIE!]