Lecture 1 Runtime environments Course Overview System programming
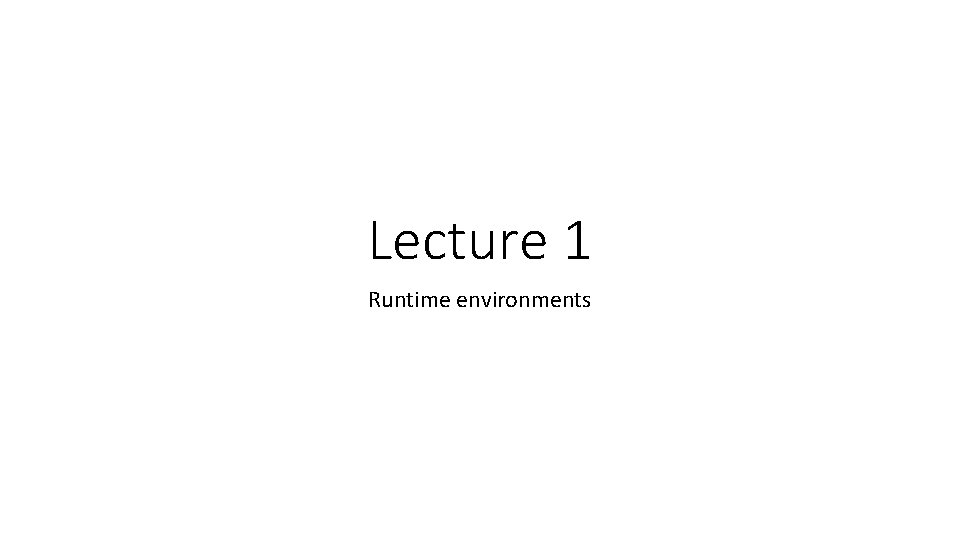
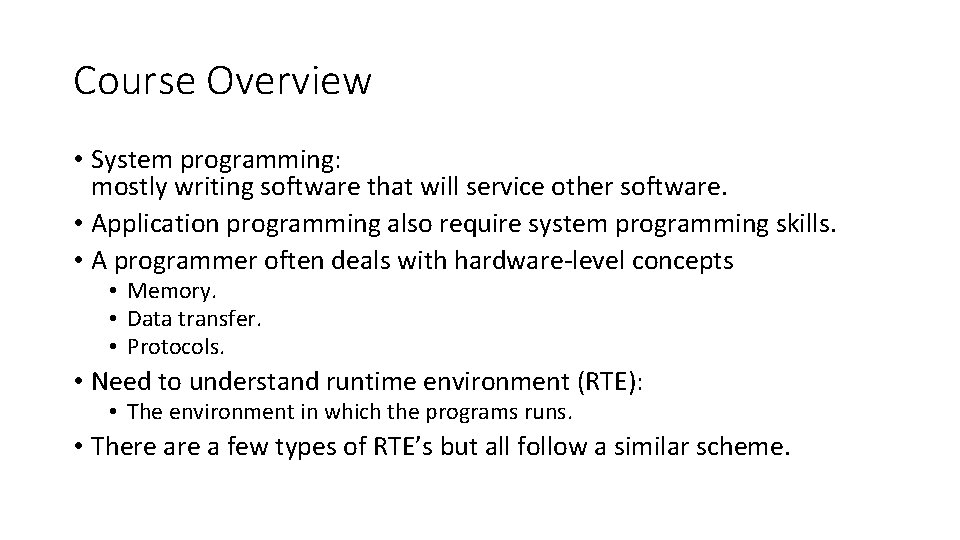
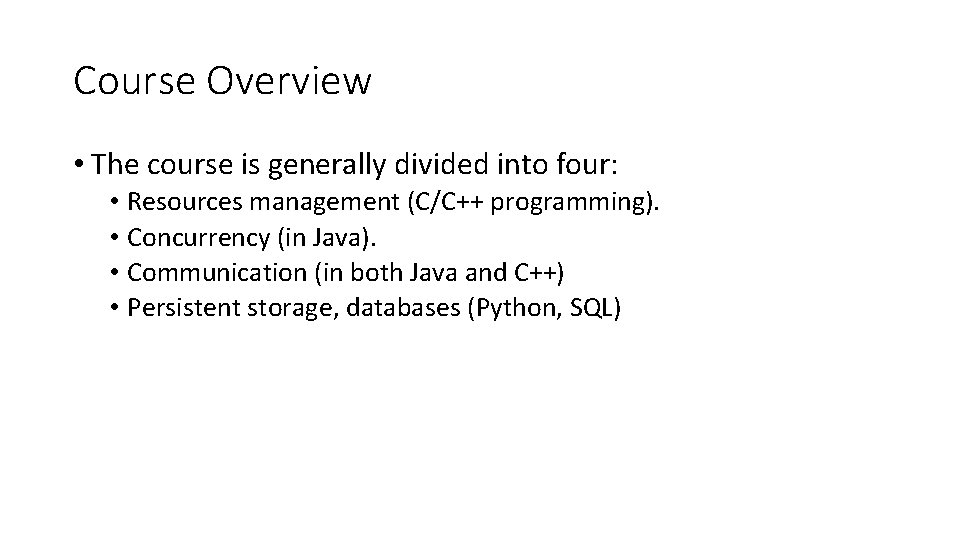
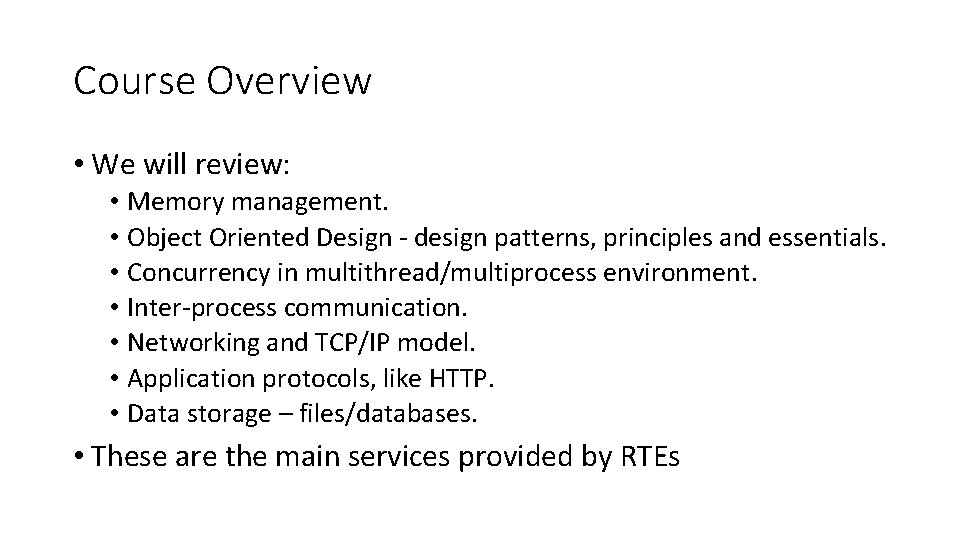
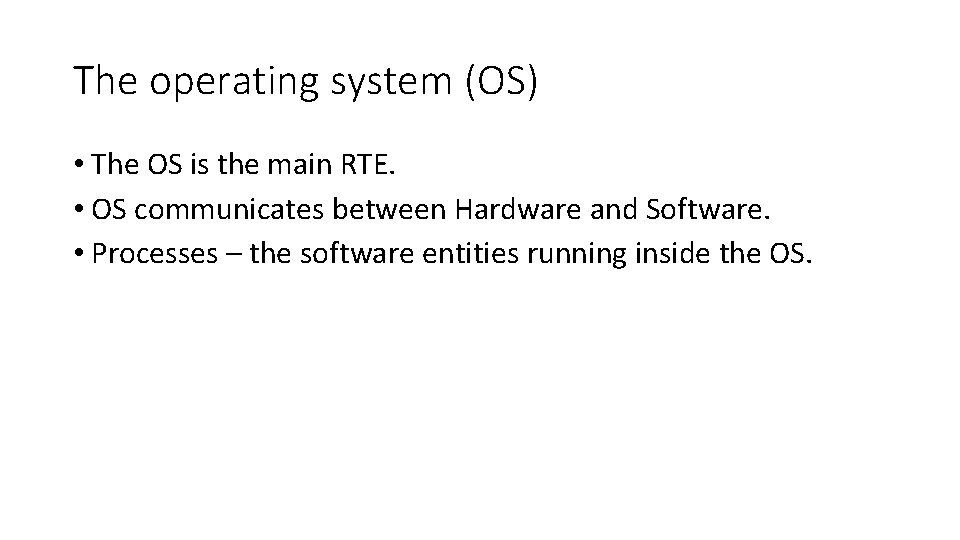
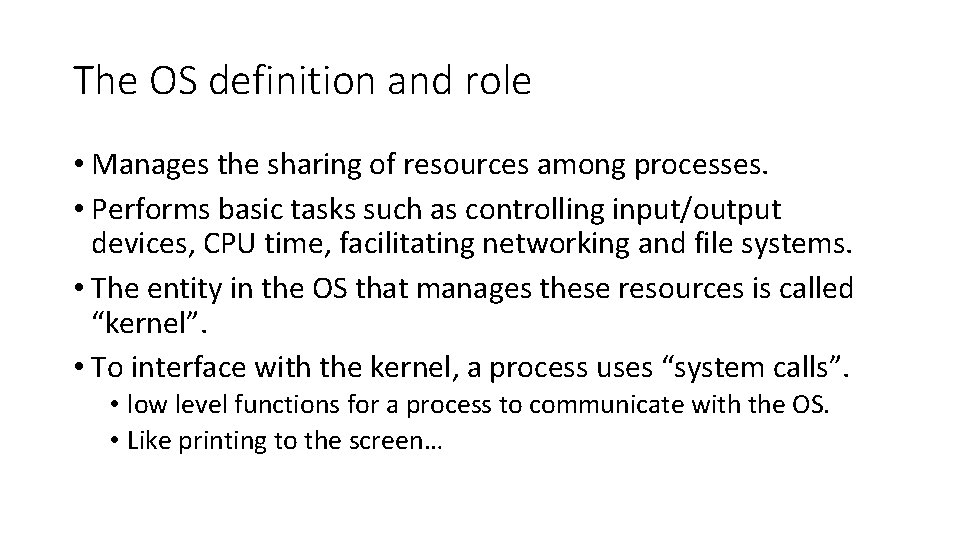
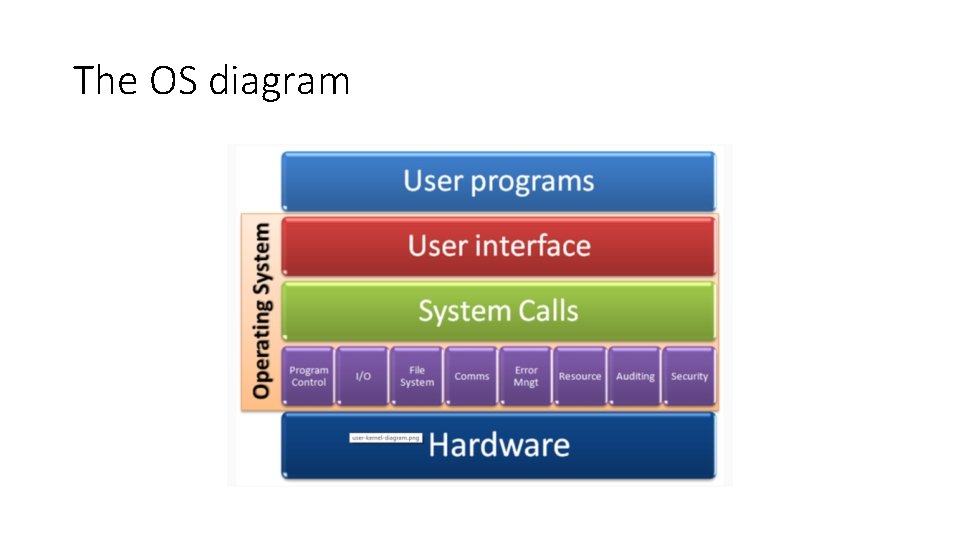
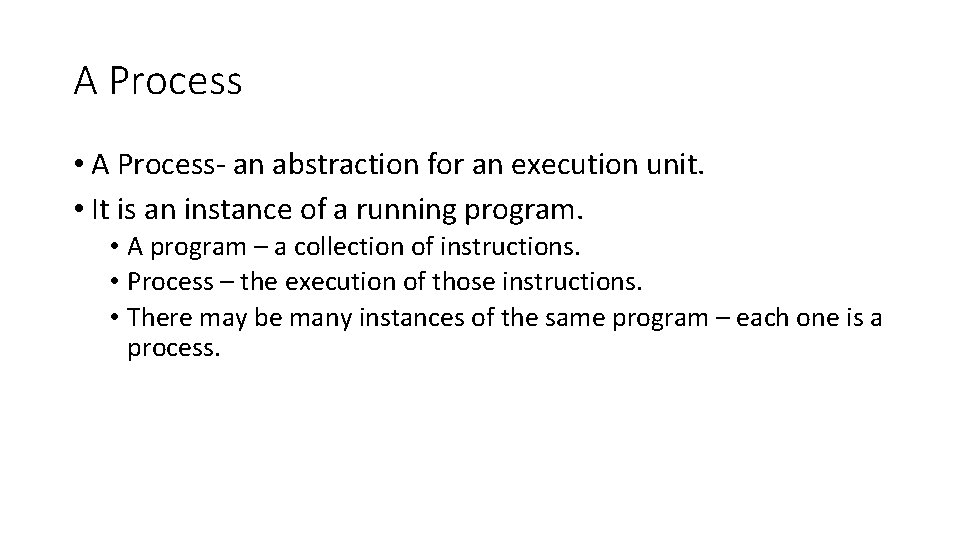
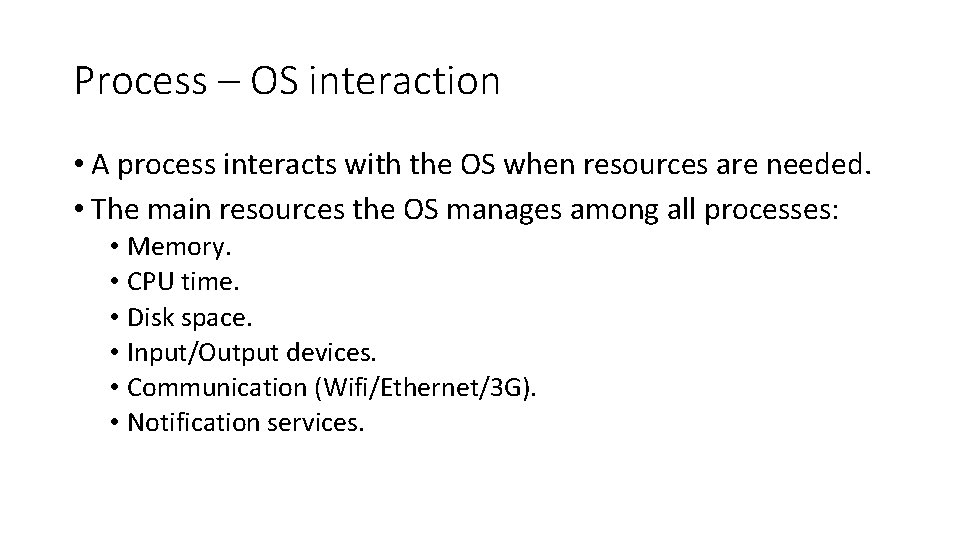
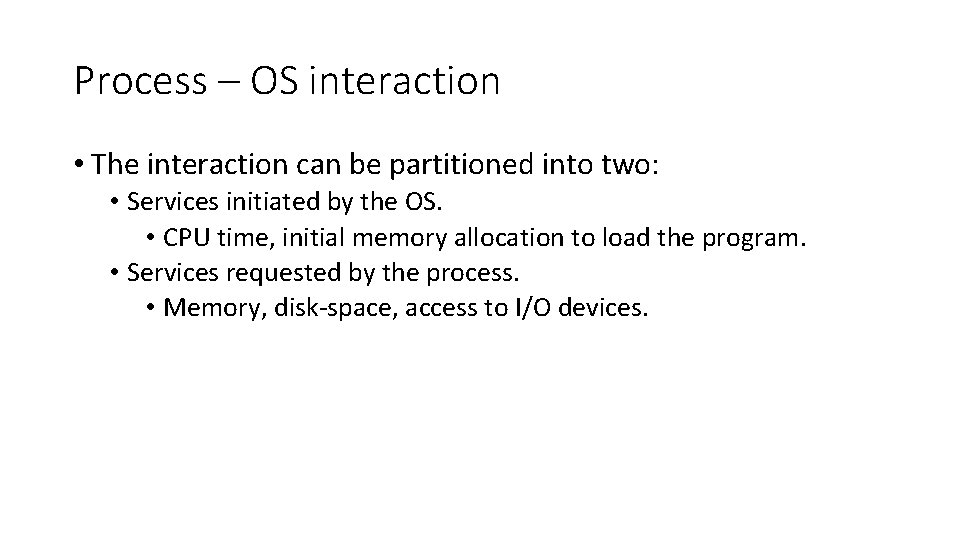
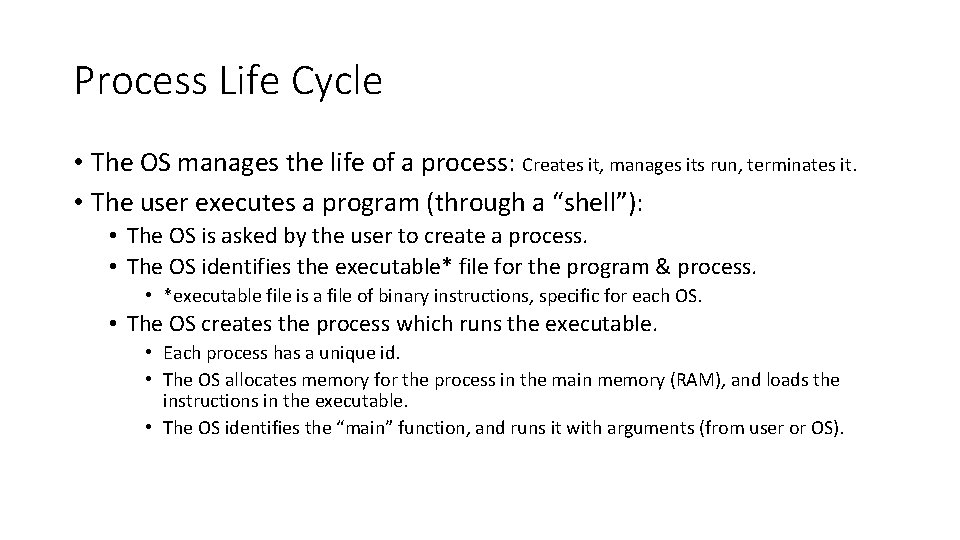
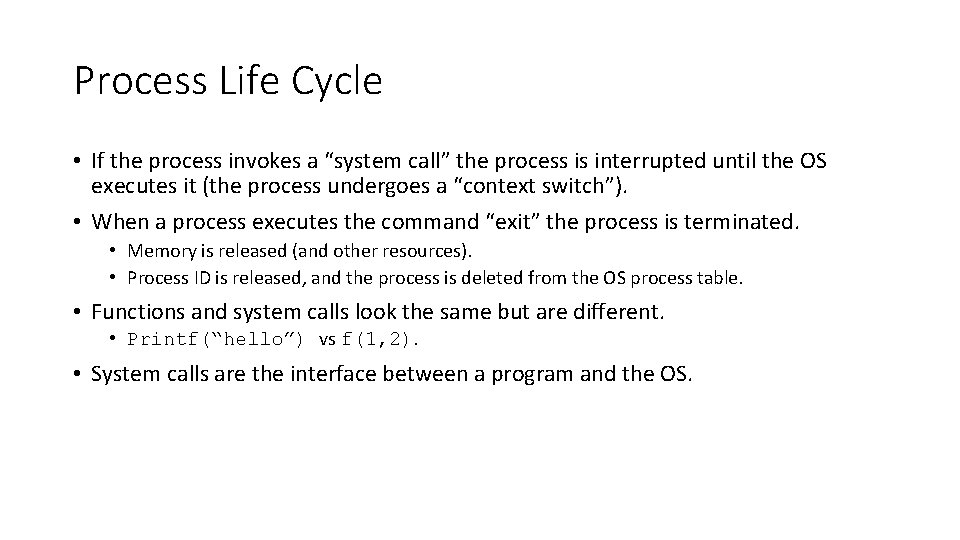
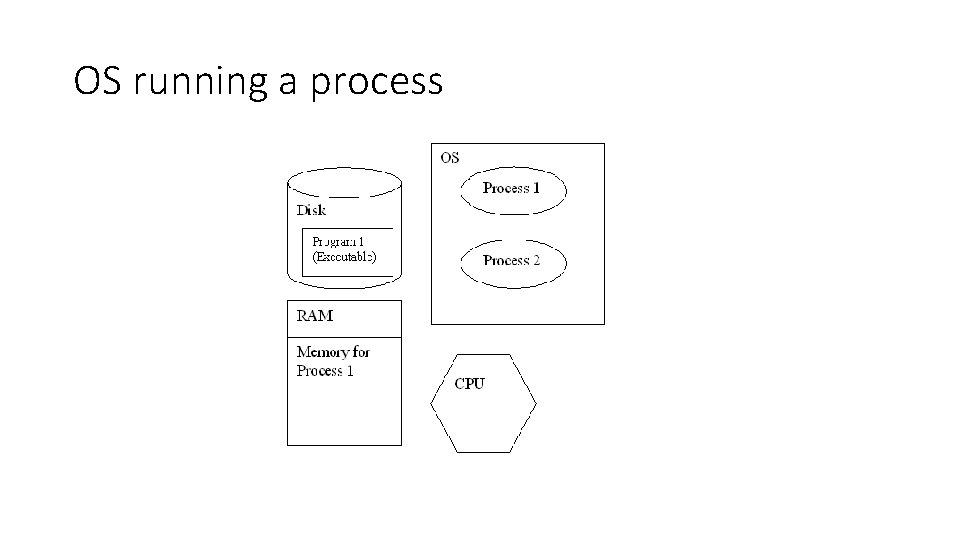
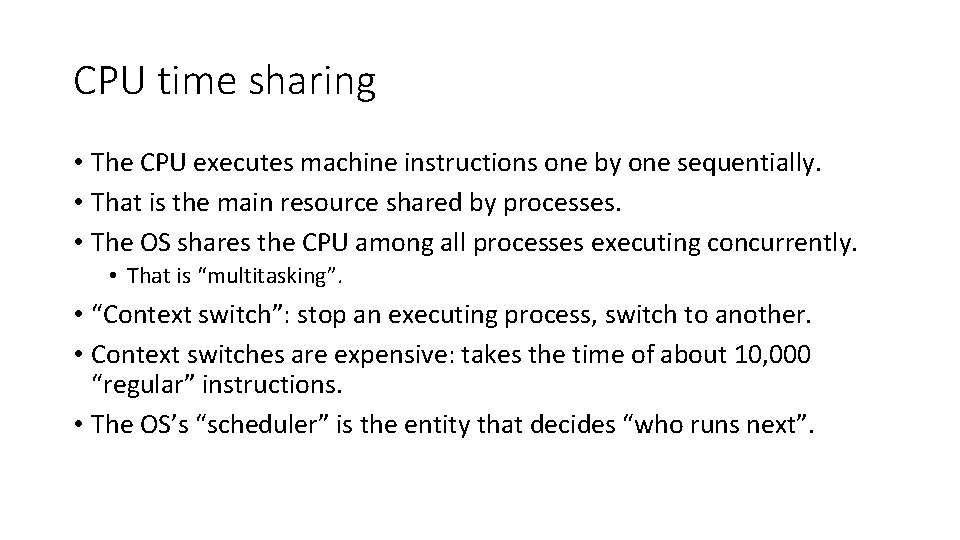
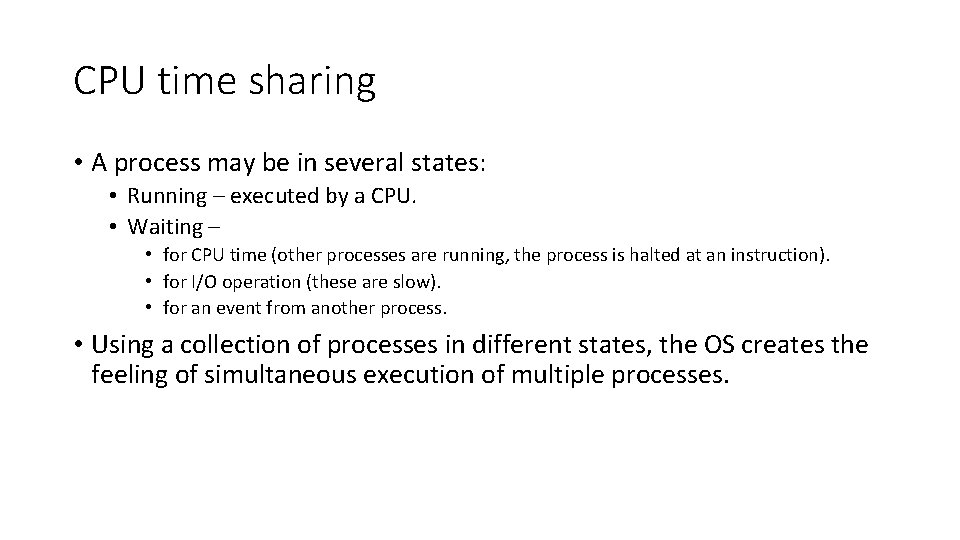
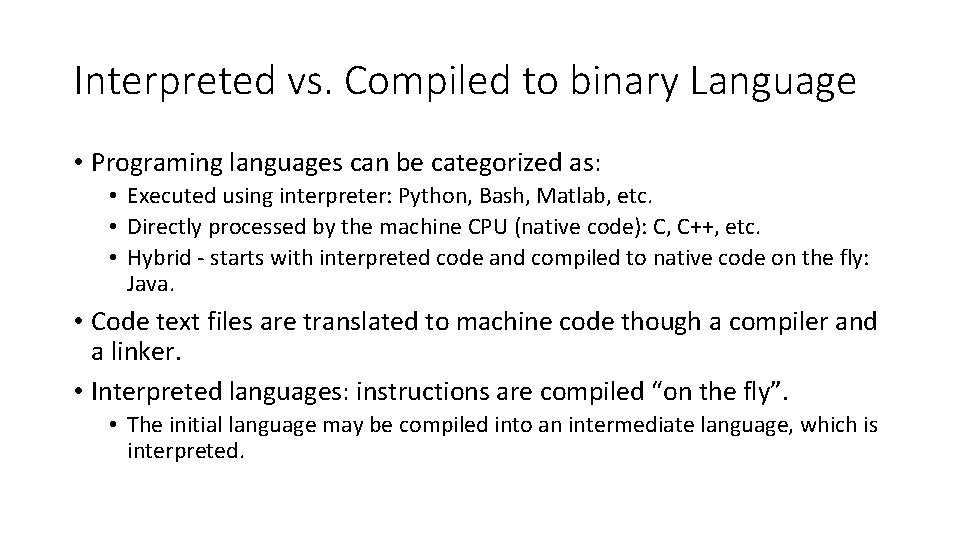
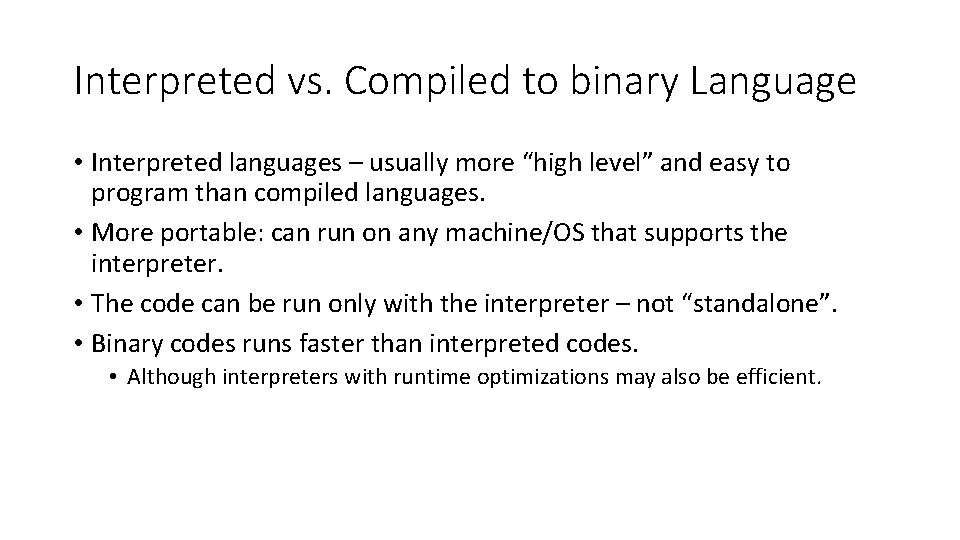
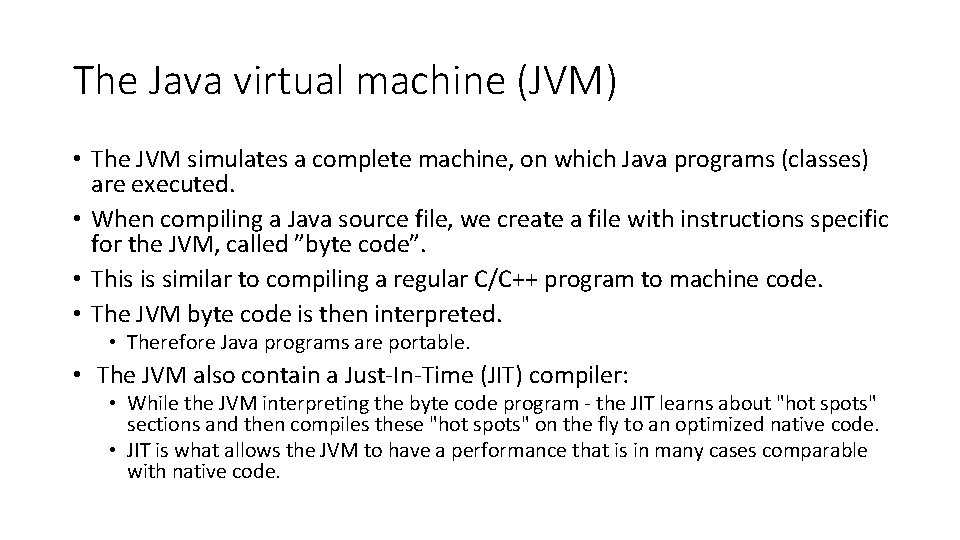
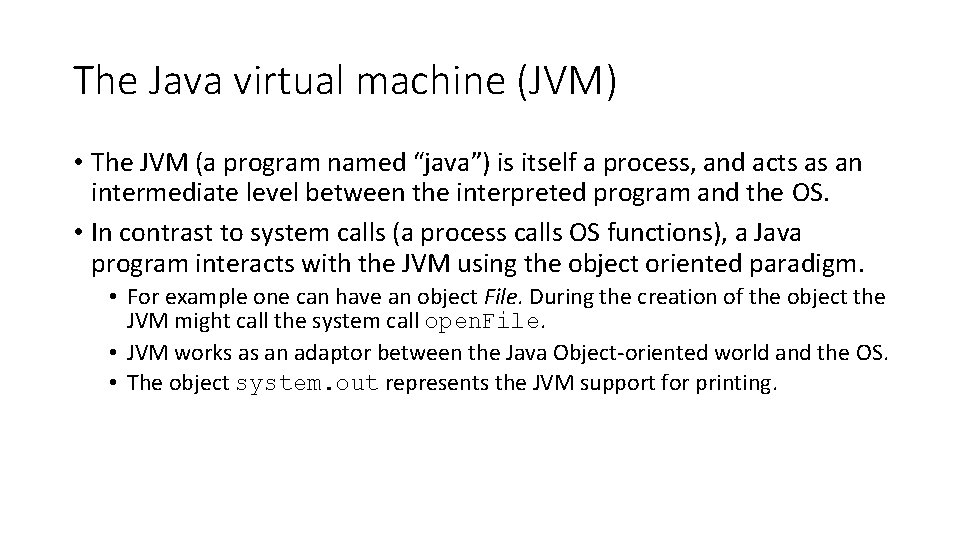
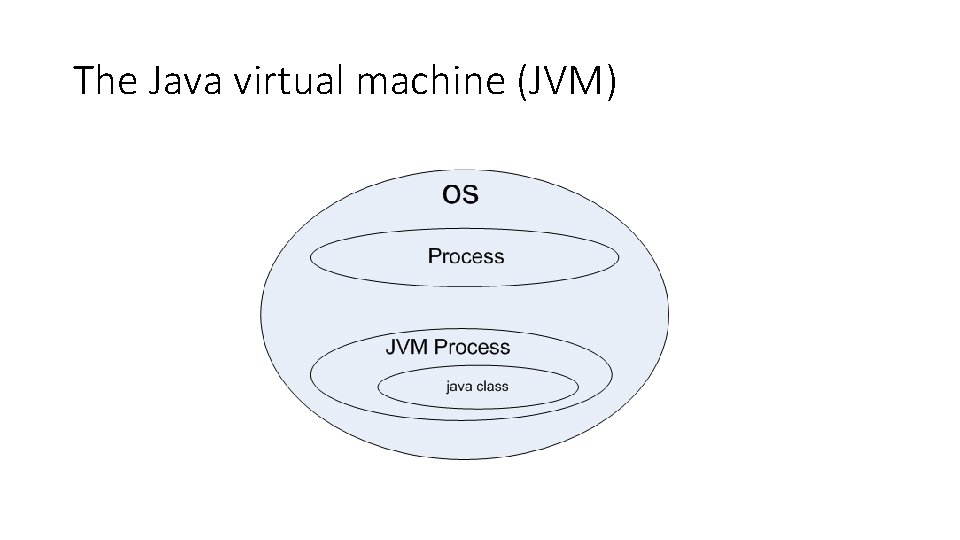
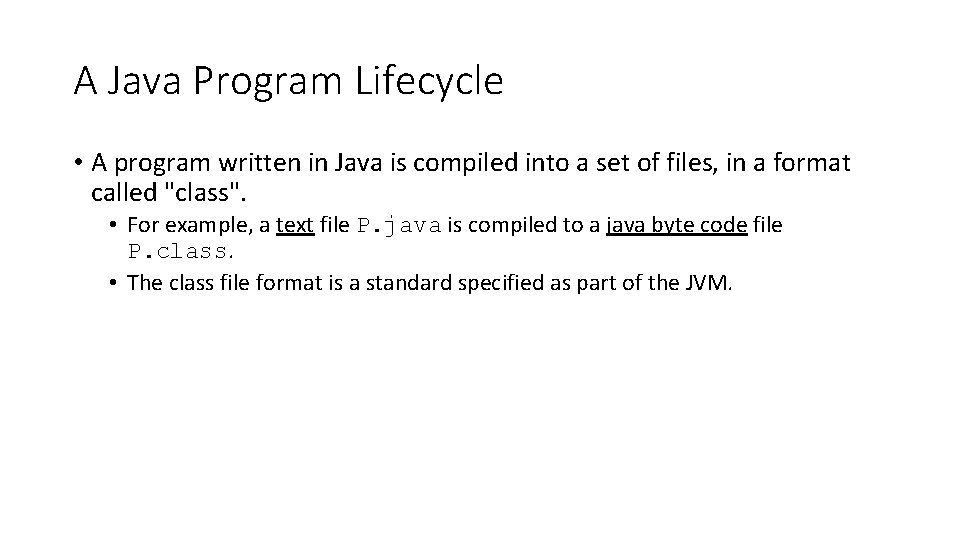
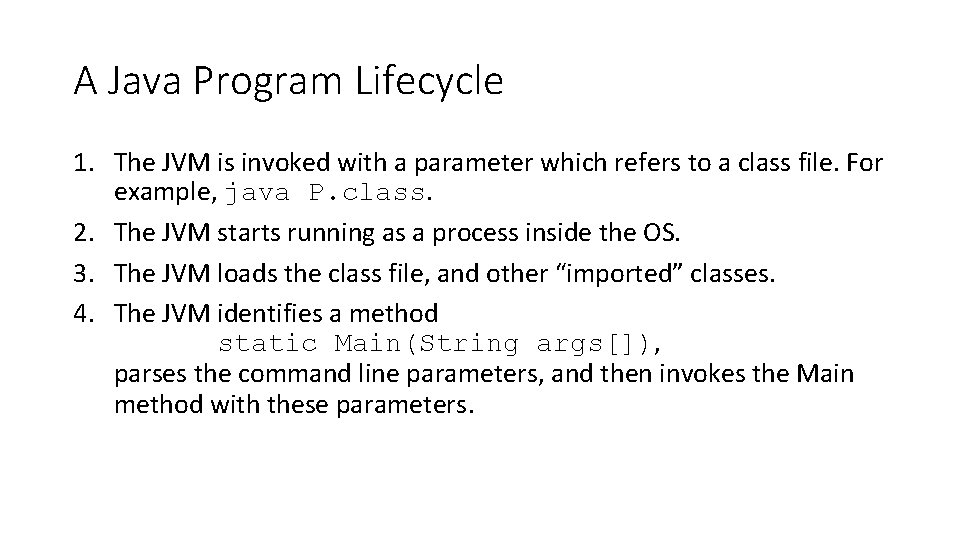
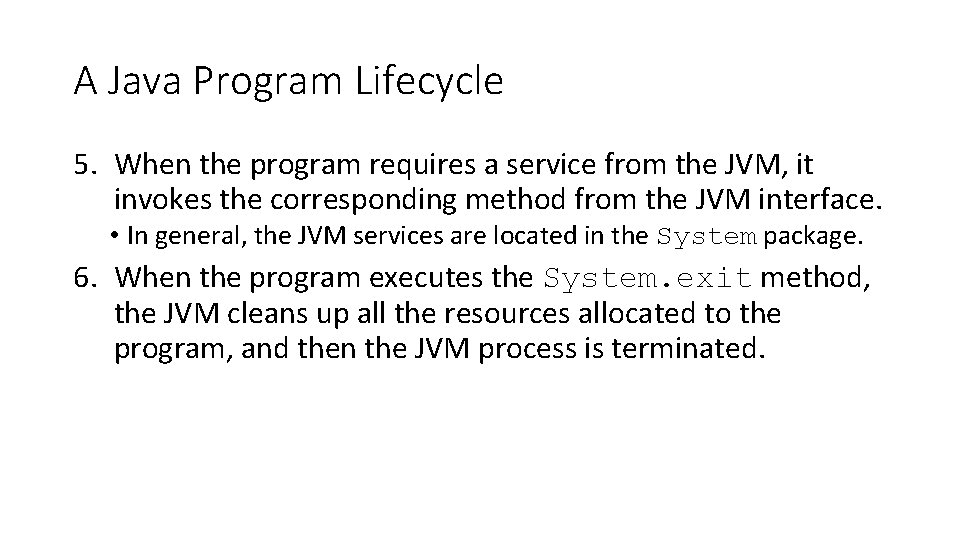
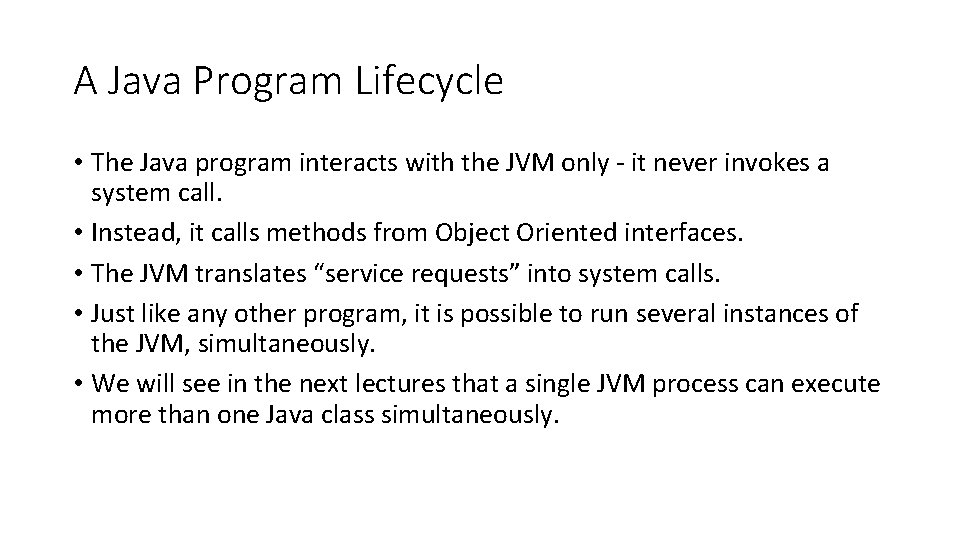
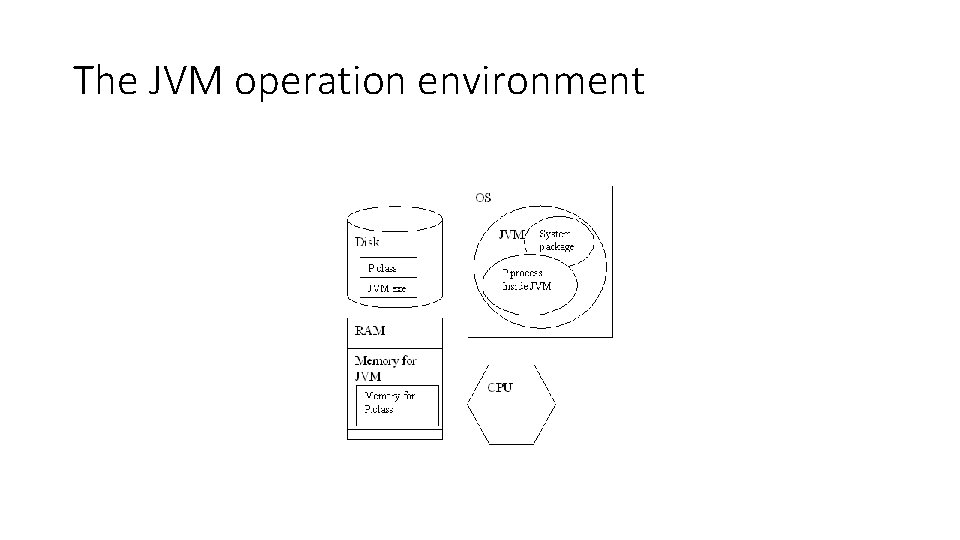
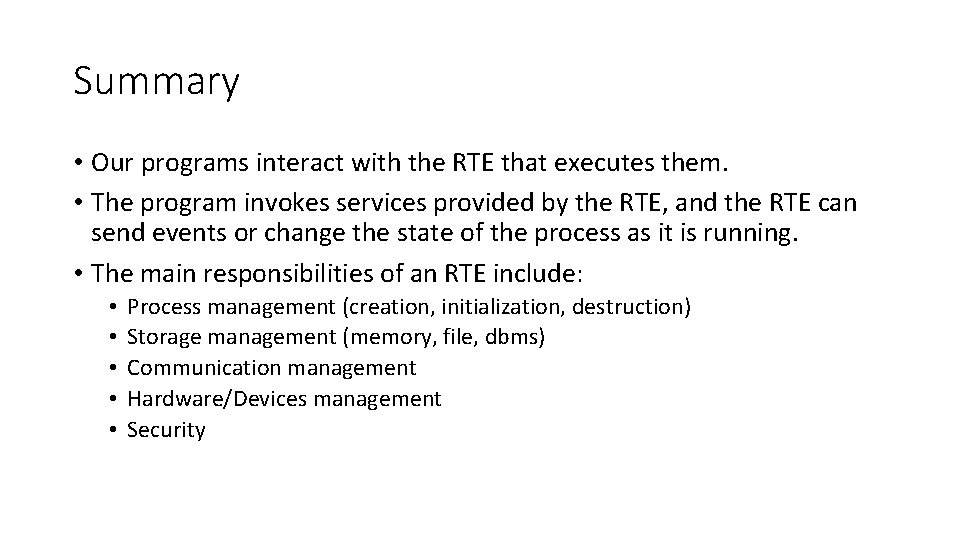
- Slides: 26
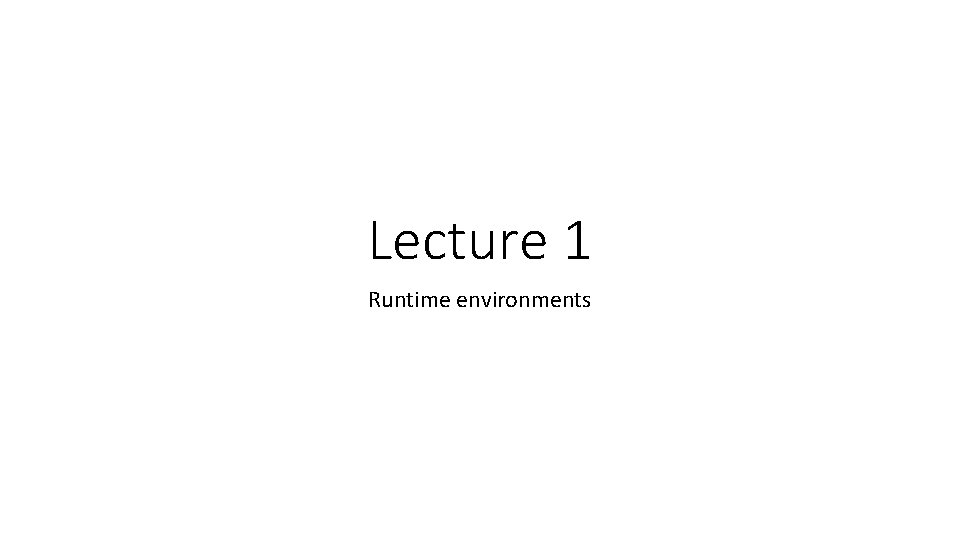
Lecture 1 Runtime environments
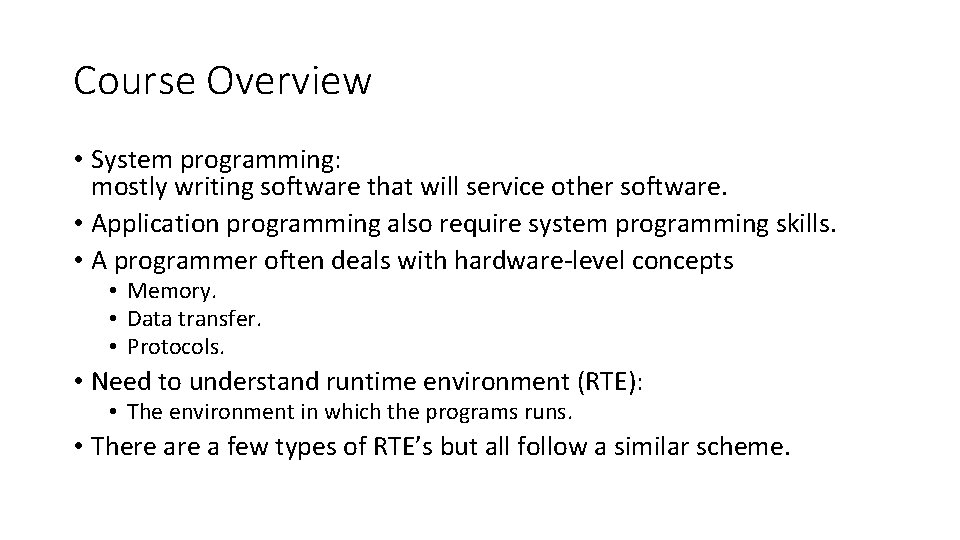
Course Overview • System programming: mostly writing software that will service other software. • Application programming also require system programming skills. • A programmer often deals with hardware-level concepts • Memory. • Data transfer. • Protocols. • Need to understand runtime environment (RTE): • The environment in which the programs runs. • There a few types of RTE’s but all follow a similar scheme.
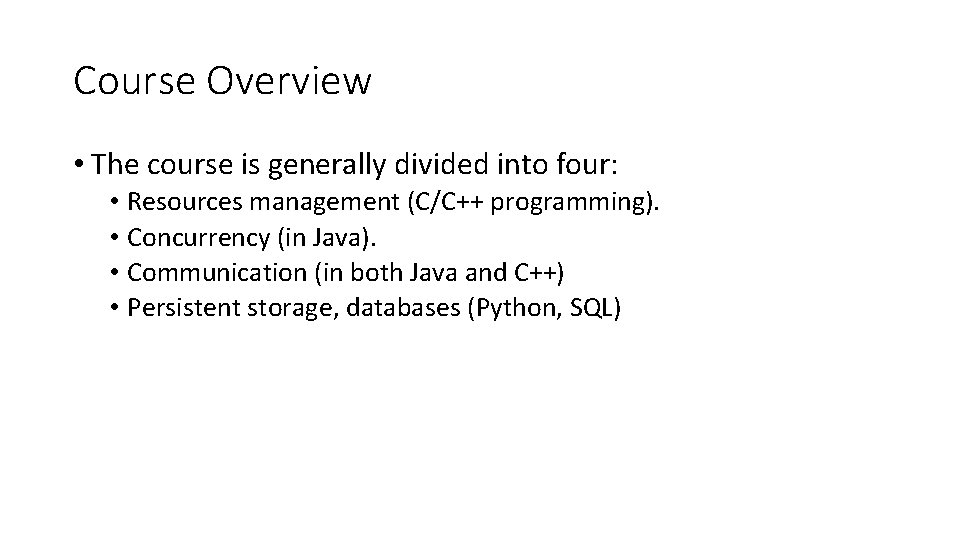
Course Overview • The course is generally divided into four: • Resources management (C/C++ programming). • Concurrency (in Java). • Communication (in both Java and C++) • Persistent storage, databases (Python, SQL)
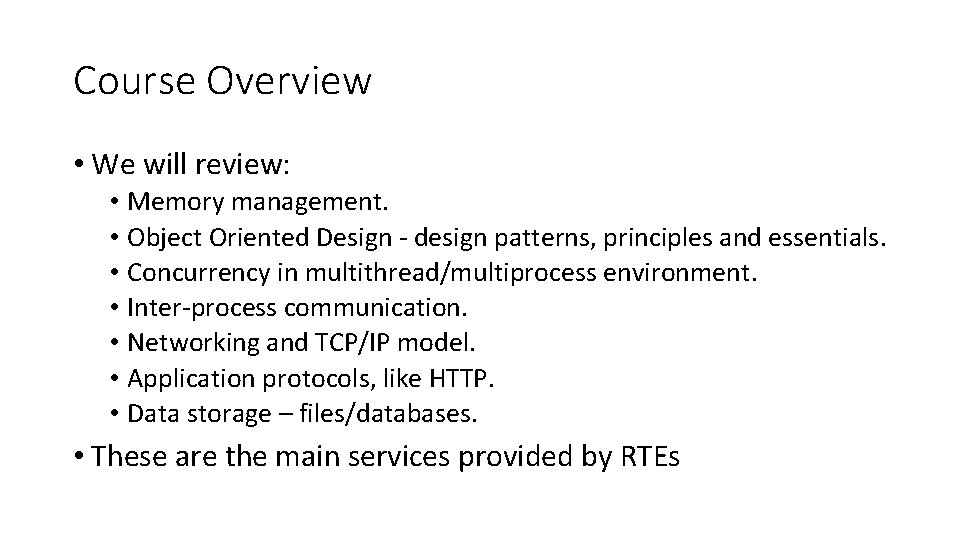
Course Overview • We will review: • Memory management. • Object Oriented Design - design patterns, principles and essentials. • Concurrency in multithread/multiprocess environment. • Inter-process communication. • Networking and TCP/IP model. • Application protocols, like HTTP. • Data storage – files/databases. • These are the main services provided by RTEs
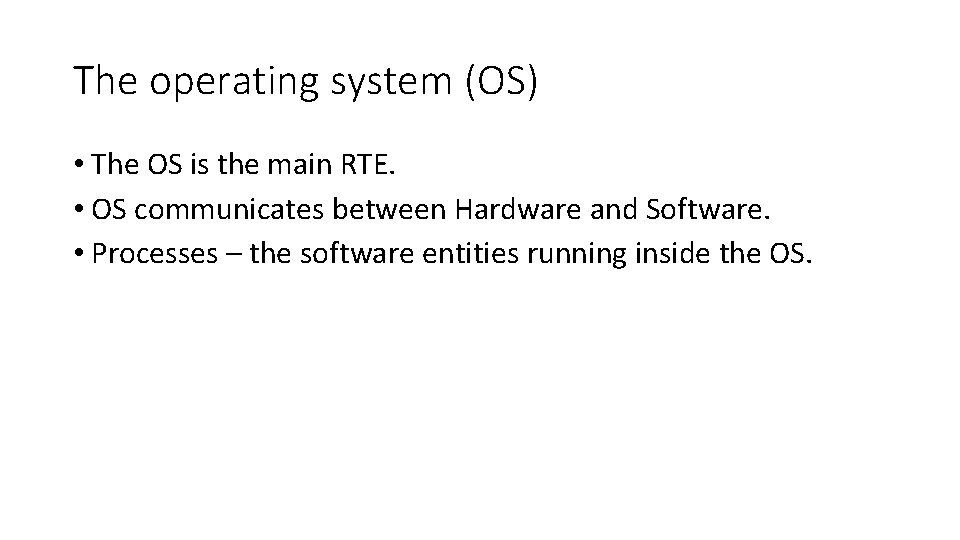
The operating system (OS) • The OS is the main RTE. • OS communicates between Hardware and Software. • Processes – the software entities running inside the OS.
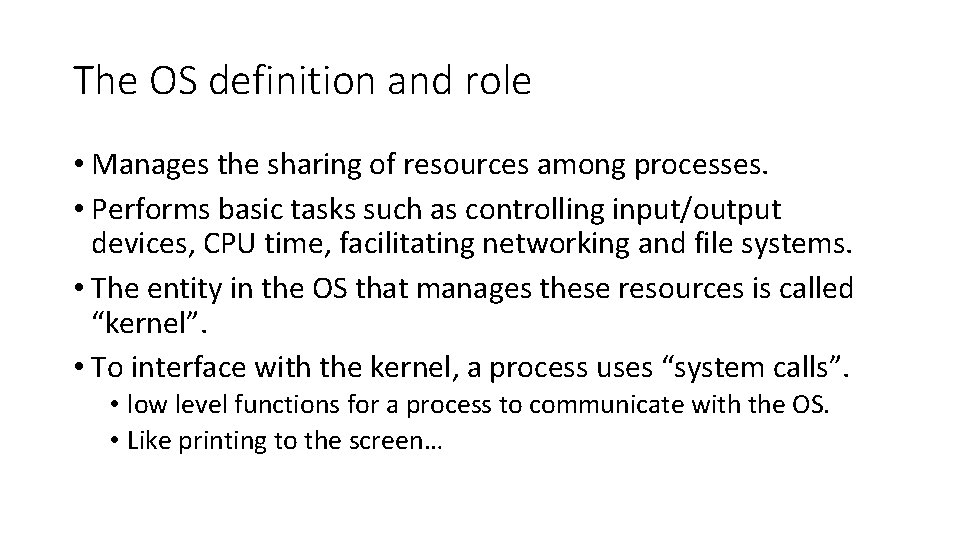
The OS definition and role • Manages the sharing of resources among processes. • Performs basic tasks such as controlling input/output devices, CPU time, facilitating networking and file systems. • The entity in the OS that manages these resources is called “kernel”. • To interface with the kernel, a process uses “system calls”. • low level functions for a process to communicate with the OS. • Like printing to the screen…
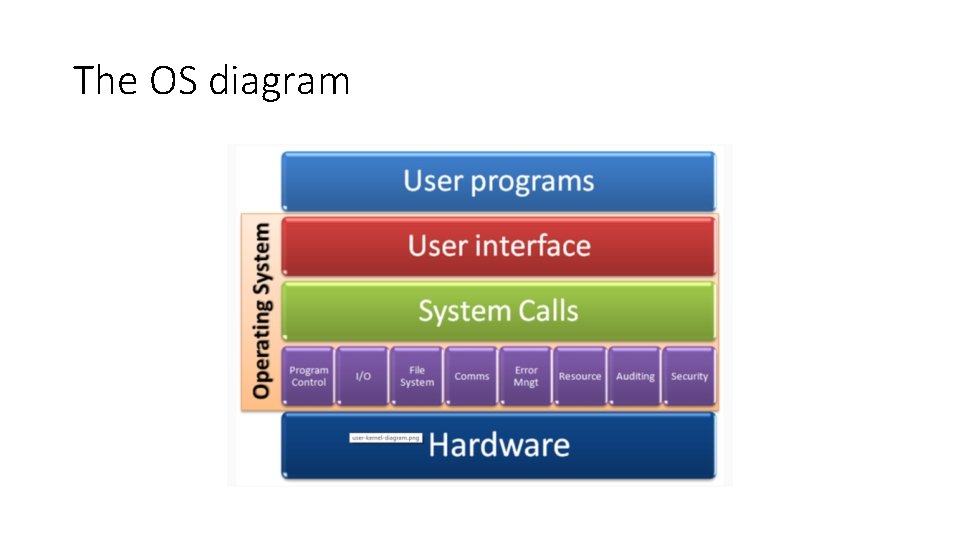
The OS diagram
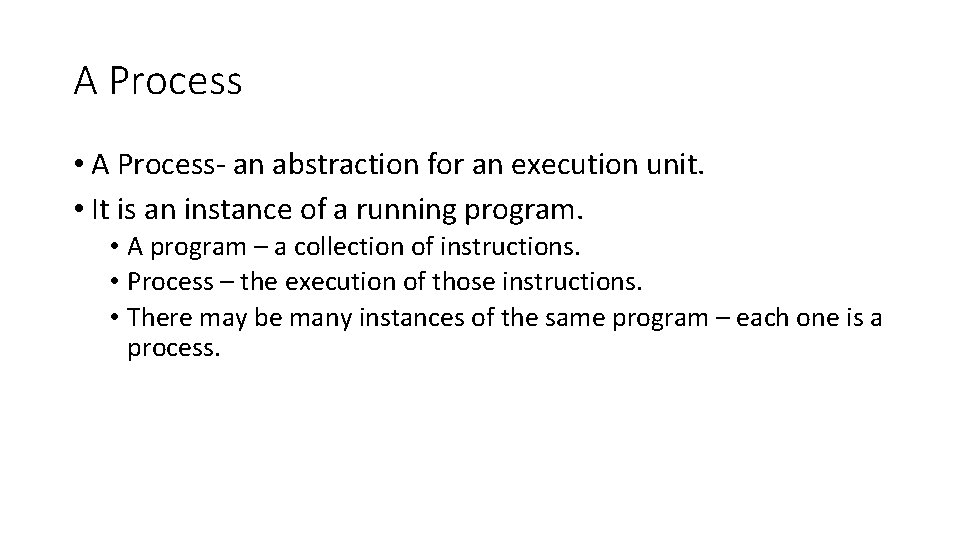
A Process • A Process- an abstraction for an execution unit. • It is an instance of a running program. • A program – a collection of instructions. • Process – the execution of those instructions. • There may be many instances of the same program – each one is a process.
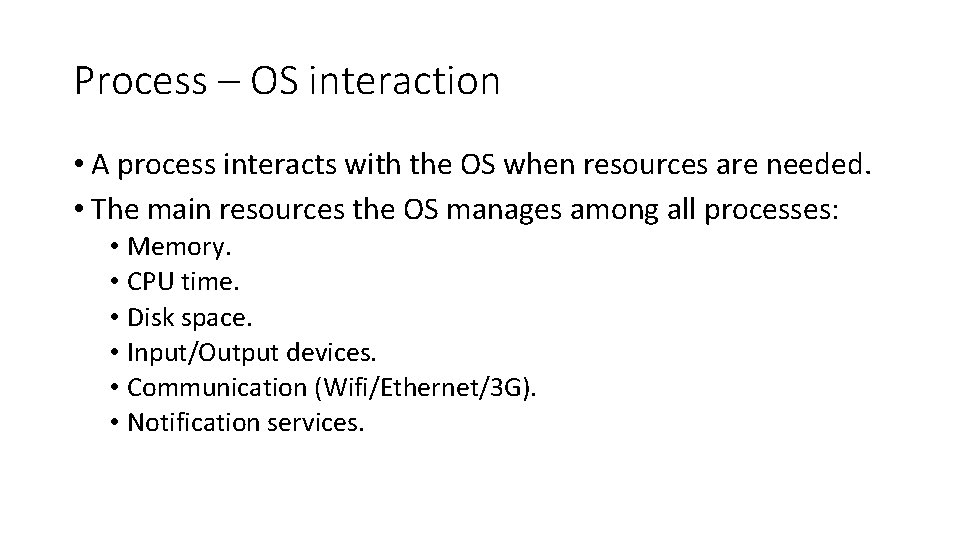
Process – OS interaction • A process interacts with the OS when resources are needed. • The main resources the OS manages among all processes: • Memory. • CPU time. • Disk space. • Input/Output devices. • Communication (Wifi/Ethernet/3 G). • Notification services.
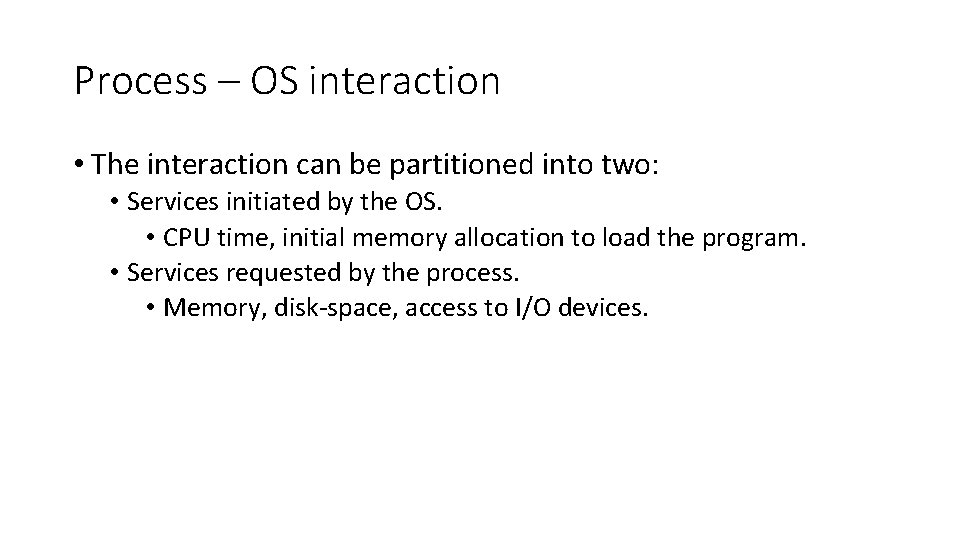
Process – OS interaction • The interaction can be partitioned into two: • Services initiated by the OS. • CPU time, initial memory allocation to load the program. • Services requested by the process. • Memory, disk-space, access to I/O devices.
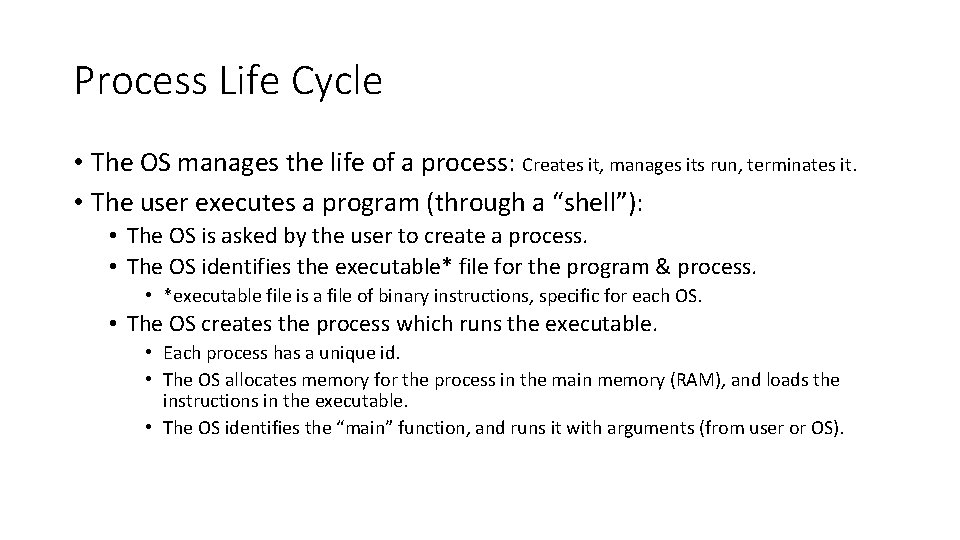
Process Life Cycle • The OS manages the life of a process: Creates it, manages its run, terminates it. • The user executes a program (through a “shell”): • The OS is asked by the user to create a process. • The OS identifies the executable* file for the program & process. • *executable file is a file of binary instructions, specific for each OS. • The OS creates the process which runs the executable. • Each process has a unique id. • The OS allocates memory for the process in the main memory (RAM), and loads the instructions in the executable. • The OS identifies the “main” function, and runs it with arguments (from user or OS).
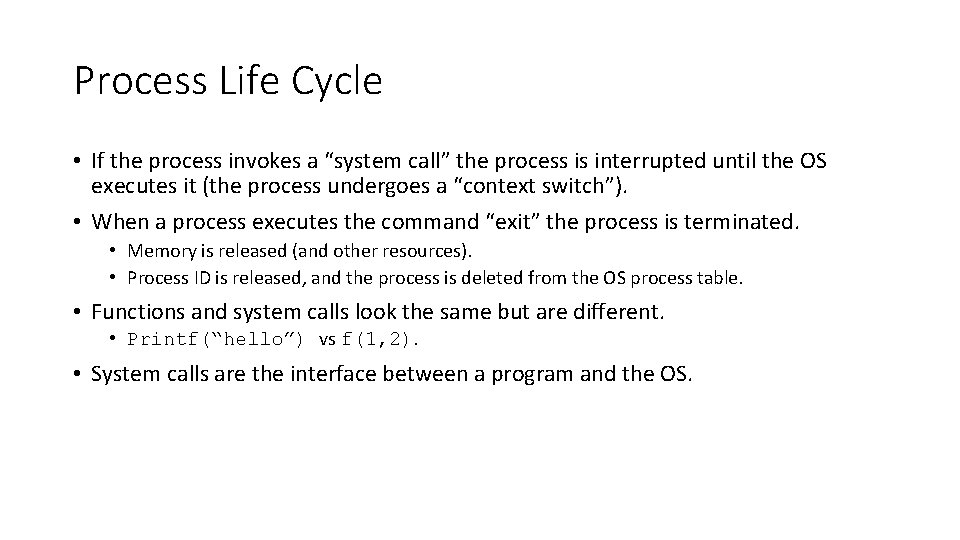
Process Life Cycle • If the process invokes a “system call” the process is interrupted until the OS executes it (the process undergoes a “context switch”). • When a process executes the command “exit” the process is terminated. • Memory is released (and other resources). • Process ID is released, and the process is deleted from the OS process table. • Functions and system calls look the same but are different. • Printf(“hello”) vs f(1, 2). • System calls are the interface between a program and the OS.
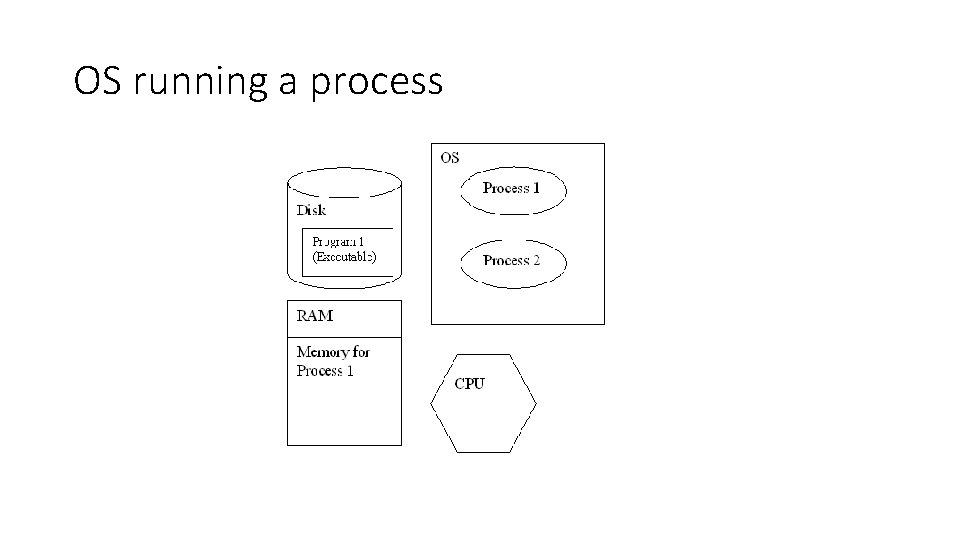
OS running a process
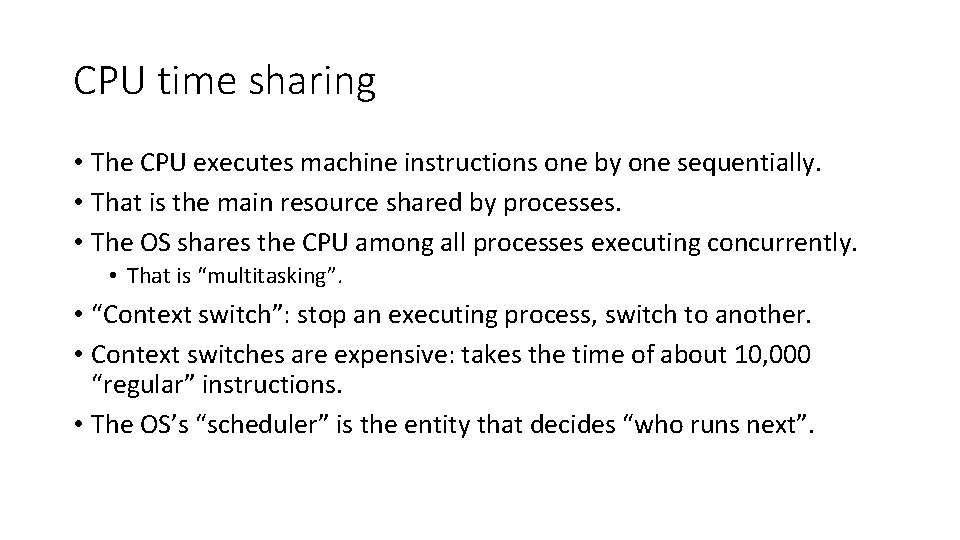
CPU time sharing • The CPU executes machine instructions one by one sequentially. • That is the main resource shared by processes. • The OS shares the CPU among all processes executing concurrently. • That is “multitasking”. • “Context switch”: stop an executing process, switch to another. • Context switches are expensive: takes the time of about 10, 000 “regular” instructions. • The OS’s “scheduler” is the entity that decides “who runs next”.
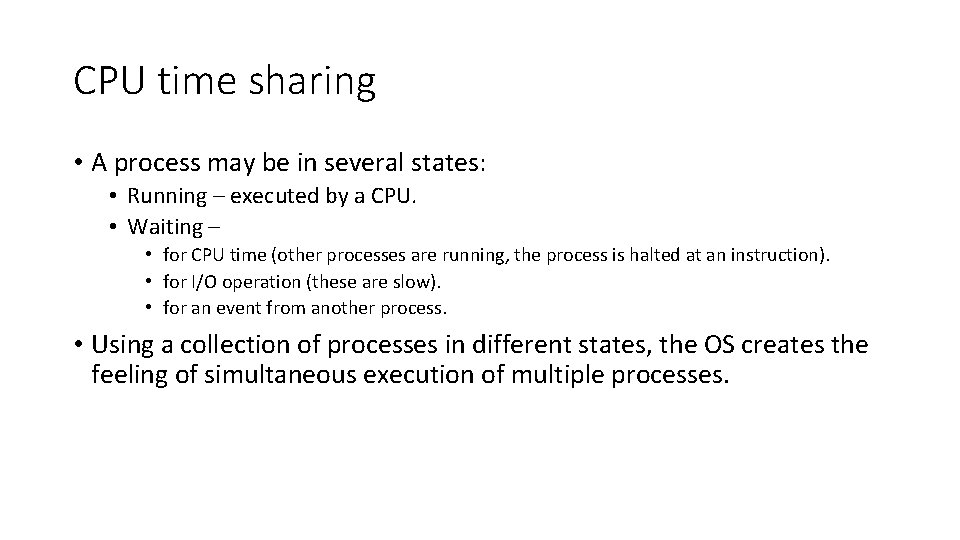
CPU time sharing • A process may be in several states: • Running – executed by a CPU. • Waiting – • for CPU time (other processes are running, the process is halted at an instruction). • for I/O operation (these are slow). • for an event from another process. • Using a collection of processes in different states, the OS creates the feeling of simultaneous execution of multiple processes.
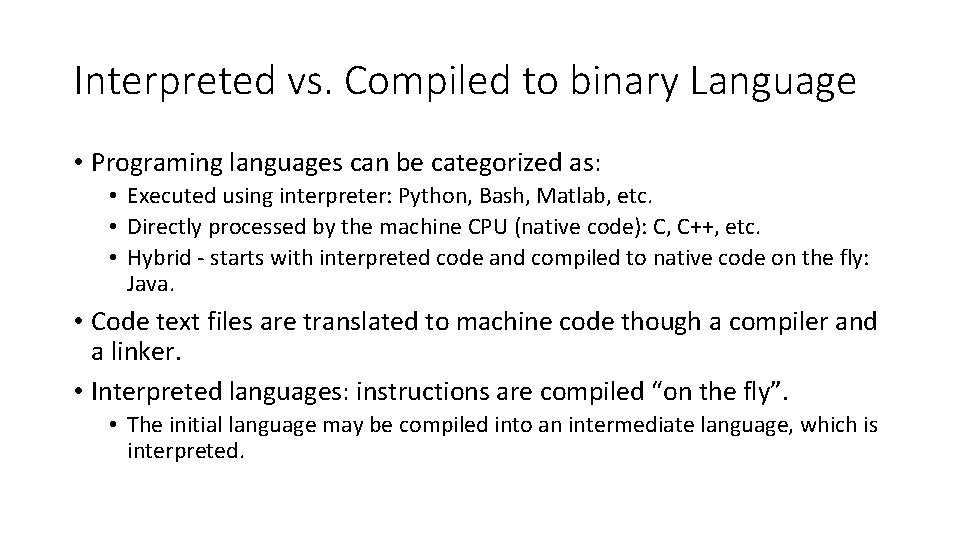
Interpreted vs. Compiled to binary Language • Programing languages can be categorized as: • Executed using interpreter: Python, Bash, Matlab, etc. • Directly processed by the machine CPU (native code): C, C++, etc. • Hybrid - starts with interpreted code and compiled to native code on the fly: Java. • Code text files are translated to machine code though a compiler and a linker. • Interpreted languages: instructions are compiled “on the fly”. • The initial language may be compiled into an intermediate language, which is interpreted.
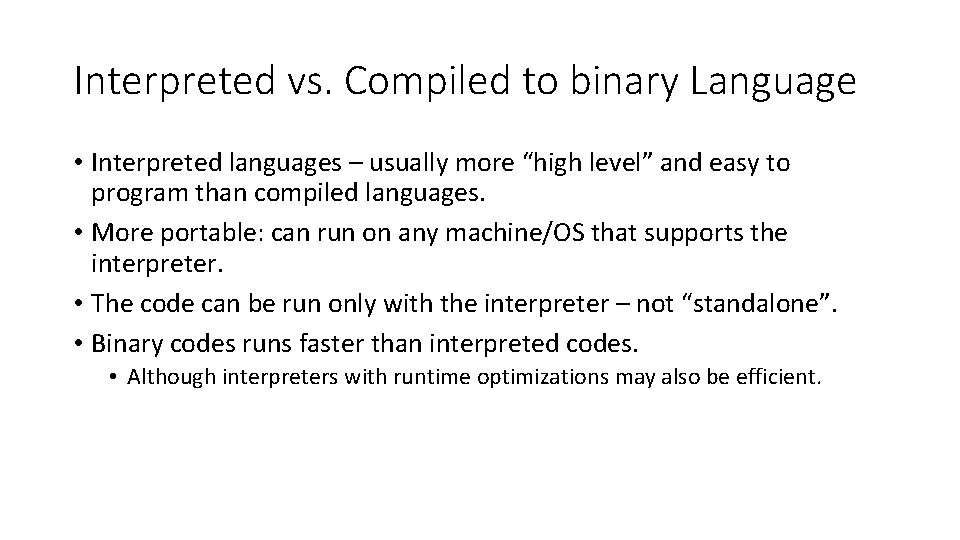
Interpreted vs. Compiled to binary Language • Interpreted languages – usually more “high level” and easy to program than compiled languages. • More portable: can run on any machine/OS that supports the interpreter. • The code can be run only with the interpreter – not “standalone”. • Binary codes runs faster than interpreted codes. • Although interpreters with runtime optimizations may also be efficient.
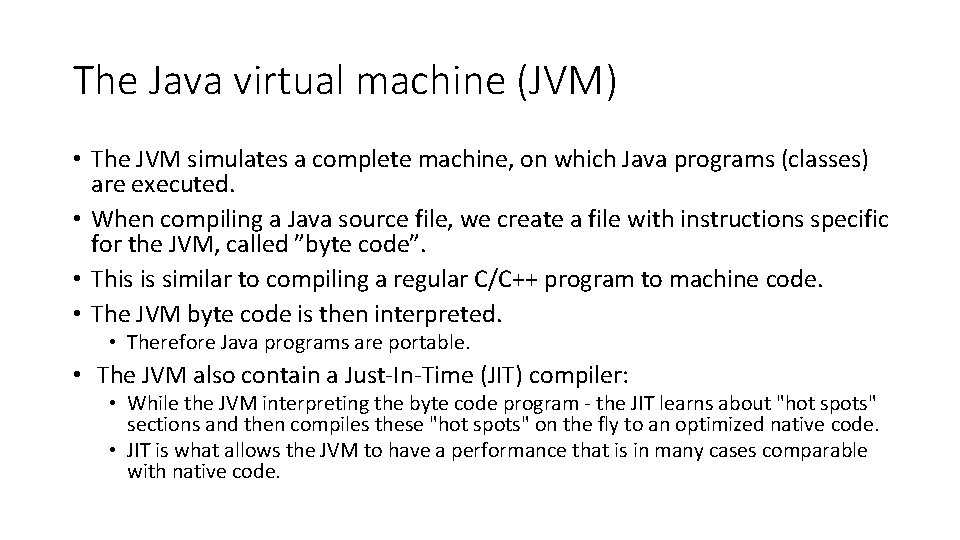
The Java virtual machine (JVM) • The JVM simulates a complete machine, on which Java programs (classes) are executed. • When compiling a Java source file, we create a file with instructions specific for the JVM, called ”byte code”. • This is similar to compiling a regular C/C++ program to machine code. • The JVM byte code is then interpreted. • Therefore Java programs are portable. • The JVM also contain a Just-In-Time (JIT) compiler: • While the JVM interpreting the byte code program - the JIT learns about "hot spots" sections and then compiles these "hot spots" on the fly to an optimized native code. • JIT is what allows the JVM to have a performance that is in many cases comparable with native code.
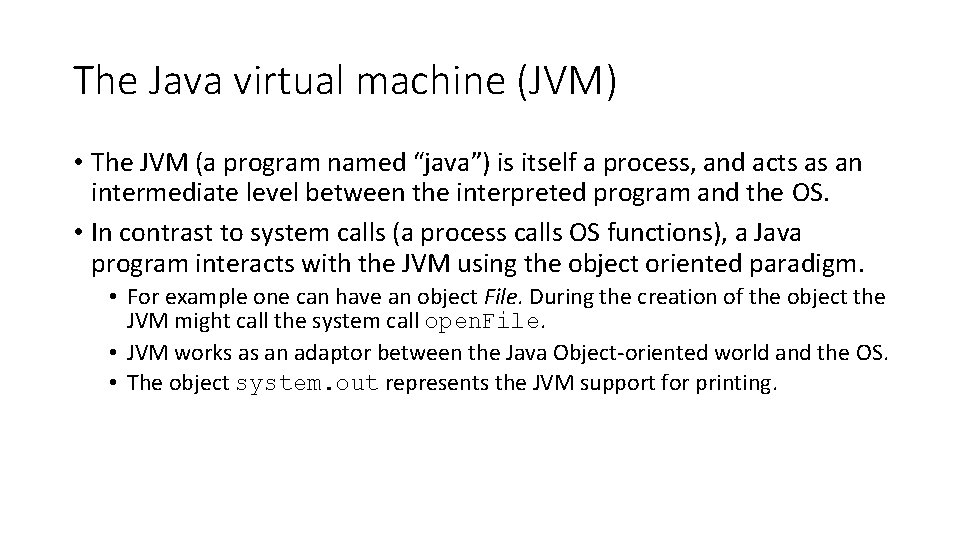
The Java virtual machine (JVM) • The JVM (a program named “java”) is itself a process, and acts as an intermediate level between the interpreted program and the OS. • In contrast to system calls (a process calls OS functions), a Java program interacts with the JVM using the object oriented paradigm. • For example one can have an object File. During the creation of the object the JVM might call the system call open. File. • JVM works as an adaptor between the Java Object-oriented world and the OS. • The object system. out represents the JVM support for printing.
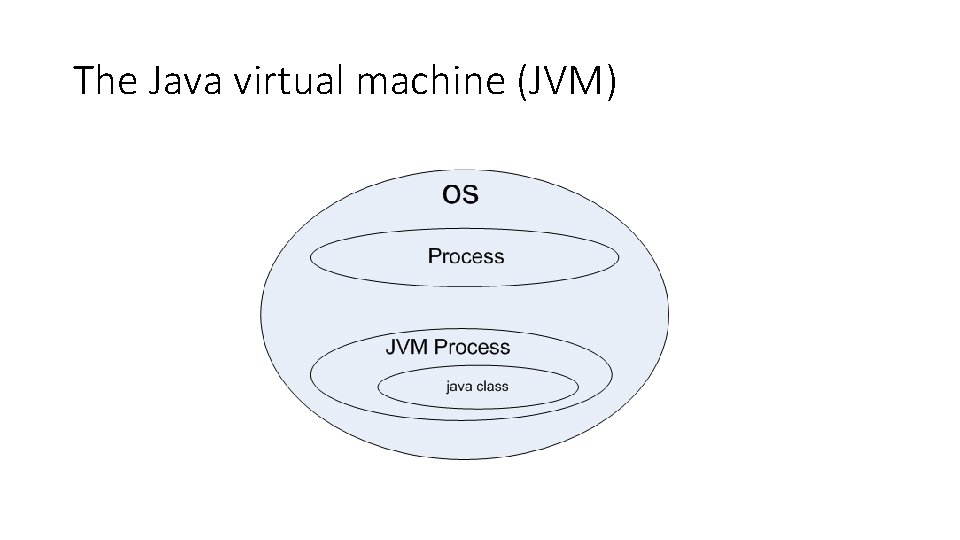
The Java virtual machine (JVM)
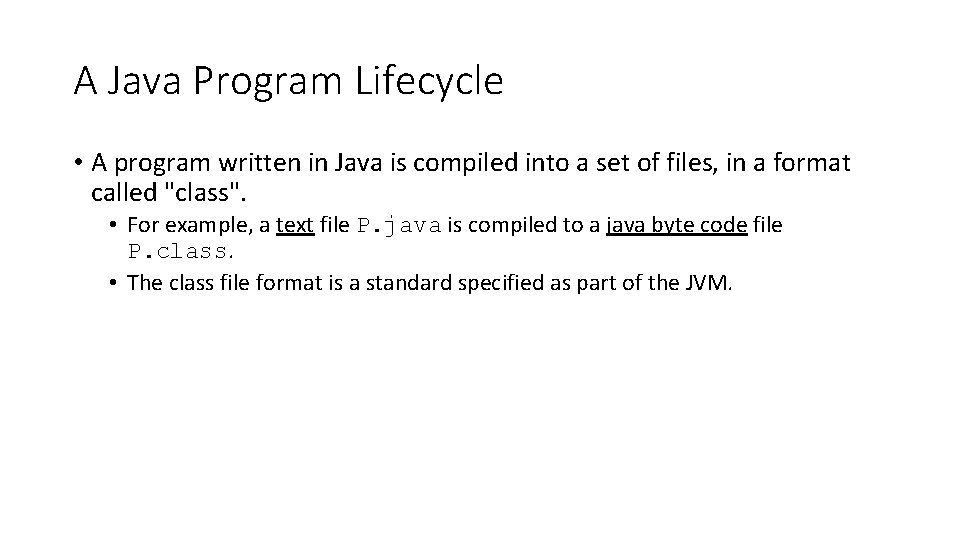
A Java Program Lifecycle • A program written in Java is compiled into a set of files, in a format called "class". • For example, a text file P. java is compiled to a java byte code file P. class. • The class file format is a standard specified as part of the JVM.
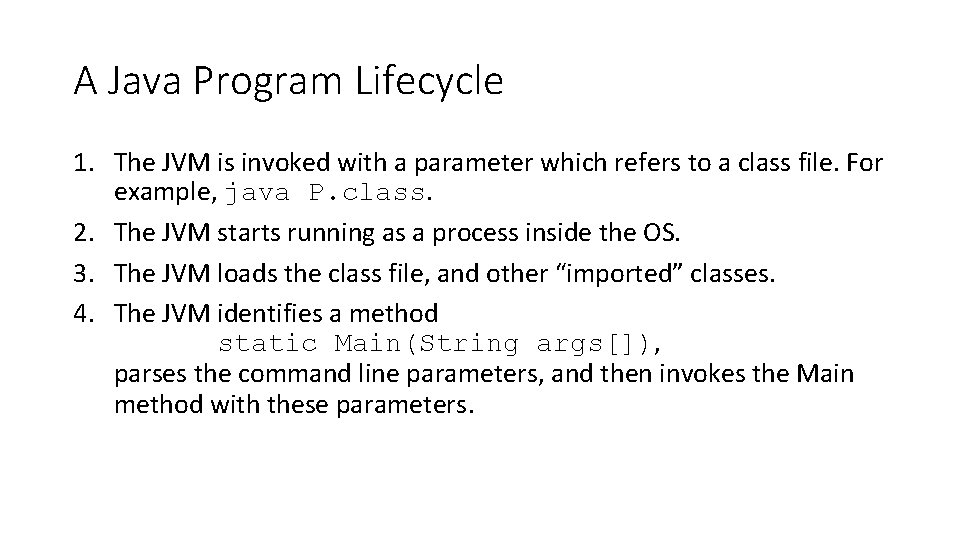
A Java Program Lifecycle 1. The JVM is invoked with a parameter which refers to a class file. For example, java P. class. 2. The JVM starts running as a process inside the OS. 3. The JVM loads the class file, and other “imported” classes. 4. The JVM identifies a method static Main(String args[]), parses the command line parameters, and then invokes the Main method with these parameters.
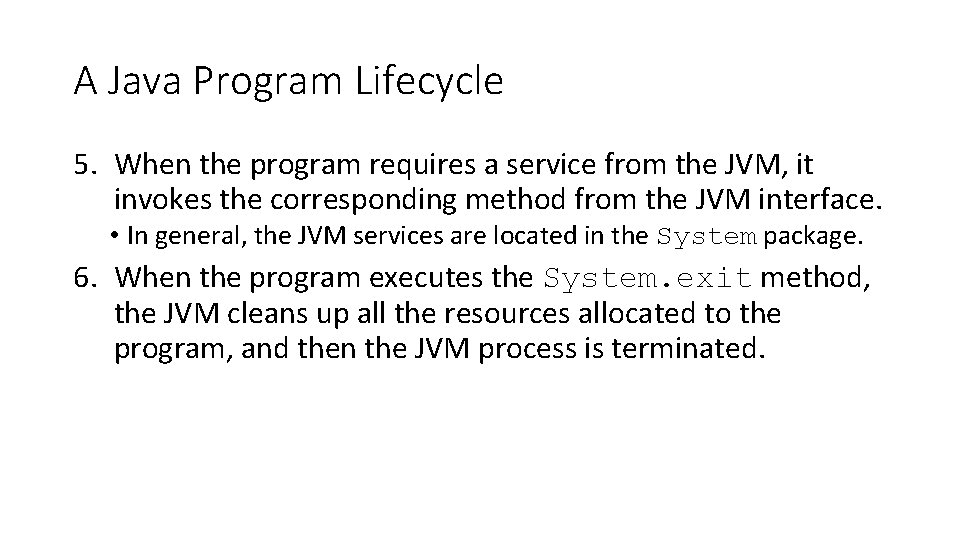
A Java Program Lifecycle 5. When the program requires a service from the JVM, it invokes the corresponding method from the JVM interface. • In general, the JVM services are located in the System package. 6. When the program executes the System. exit method, the JVM cleans up all the resources allocated to the program, and then the JVM process is terminated.
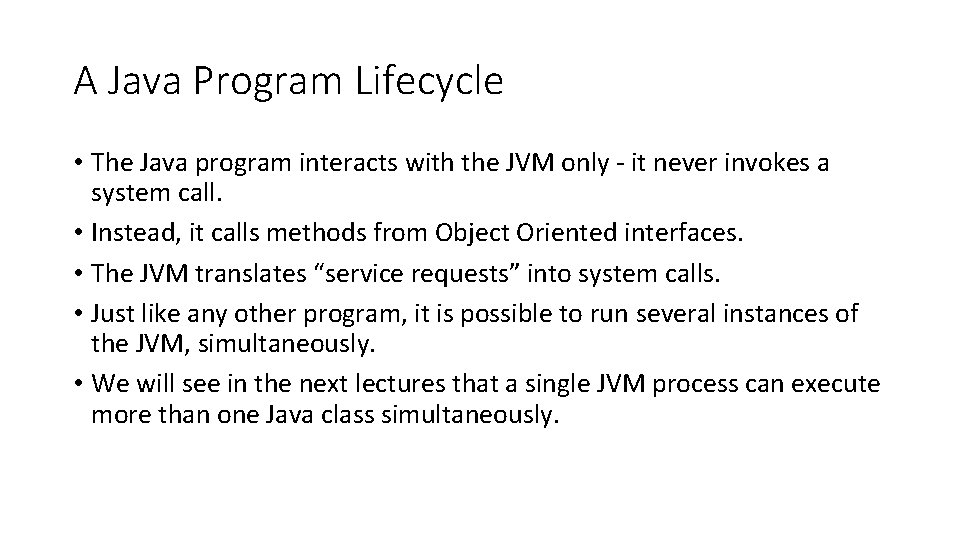
A Java Program Lifecycle • The Java program interacts with the JVM only - it never invokes a system call. • Instead, it calls methods from Object Oriented interfaces. • The JVM translates “service requests” into system calls. • Just like any other program, it is possible to run several instances of the JVM, simultaneously. • We will see in the next lectures that a single JVM process can execute more than one Java class simultaneously.
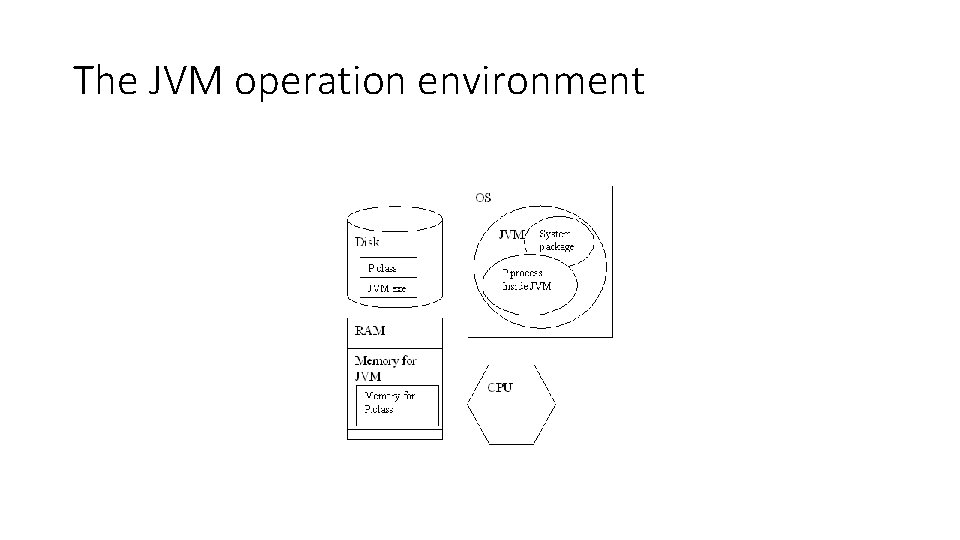
The JVM operation environment
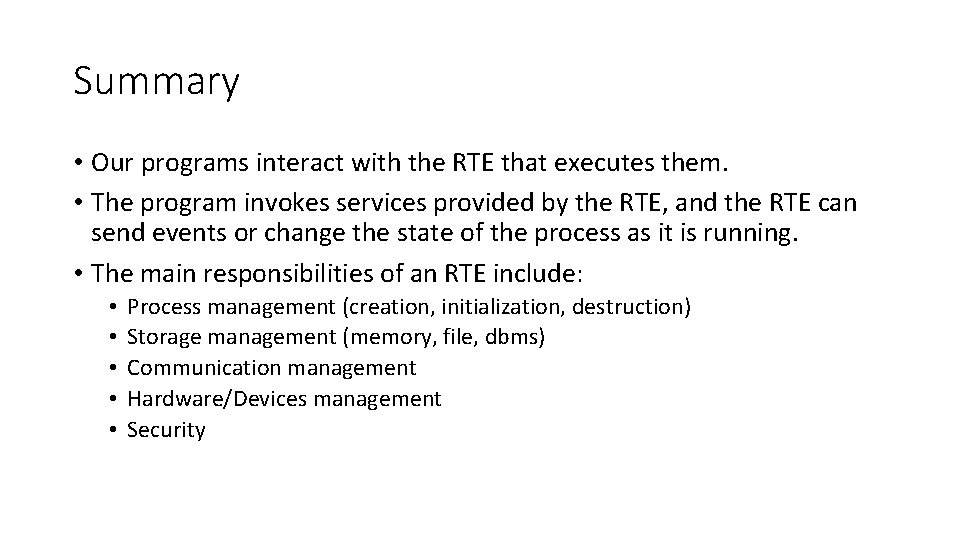
Summary • Our programs interact with the RTE that executes them. • The program invokes services provided by the RTE, and the RTE can send events or change the state of the process as it is running. • The main responsibilities of an RTE include: • • • Process management (creation, initialization, destruction) Storage management (memory, file, dbms) Communication management Hardware/Devices management Security
Windows 10 system programming, part 1
01:640:244 lecture notes - lecture 15: plat, idah, farad
Android programming overview
C programming lecture
One and half brick wall
Course title and course number
Course interne moyenne externe
Integer programming course
Ai2.appinventor.mit.edu emulator
Color 03222006
Java ee 101
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Linear vs integer programming
Definisi integer
Subdivision of runtime memory
Openplc arduino
Difference between compile time and runtime
Kinect for windows speech recognition language pack
Constructor java
Heapify runtime
Matrix multiplication runtime
Runtime error index out of bounds
Dynamic code generation
Runtime environment in compiler design
Ford fulkerson runtime
Prims algorithm runtime