JUnit Mockito Spring Test Context Framework Maven Junit
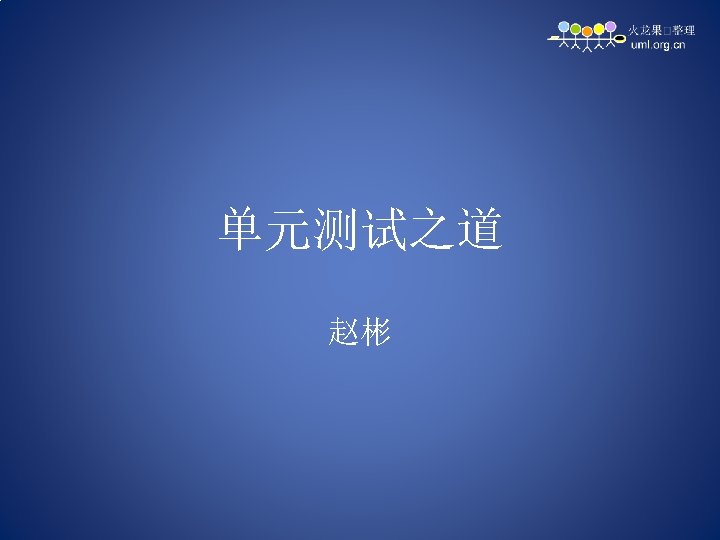
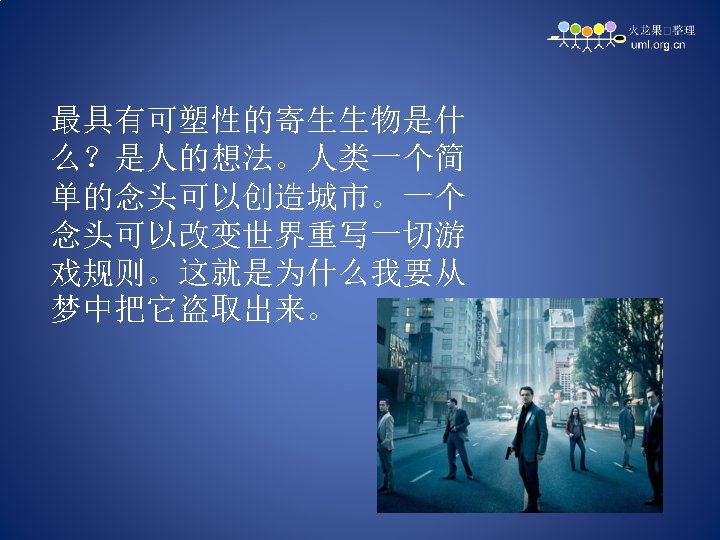
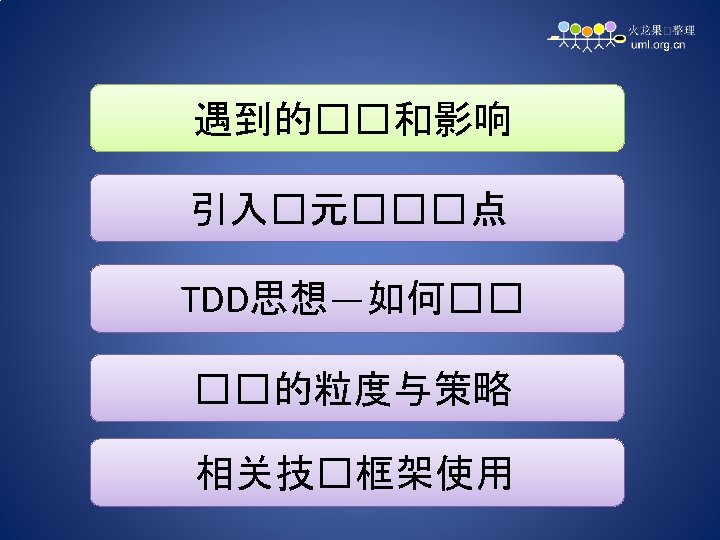
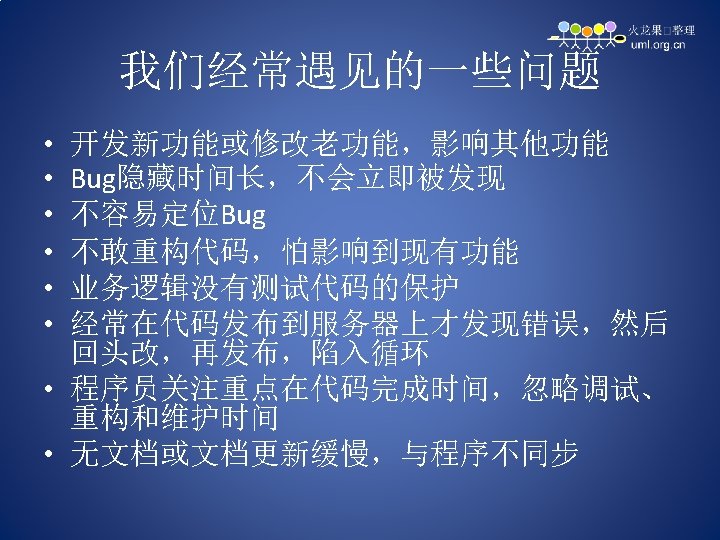
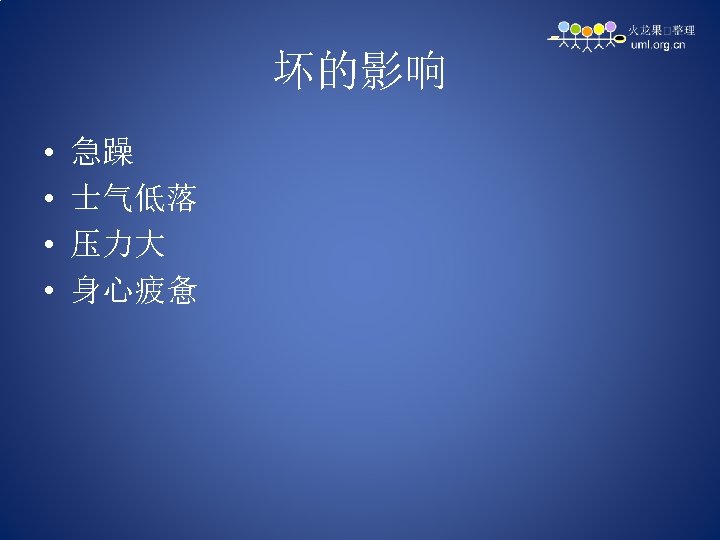
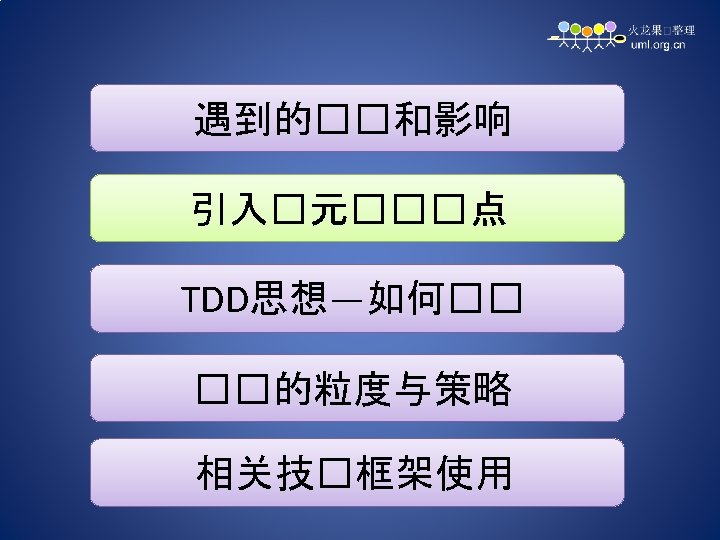
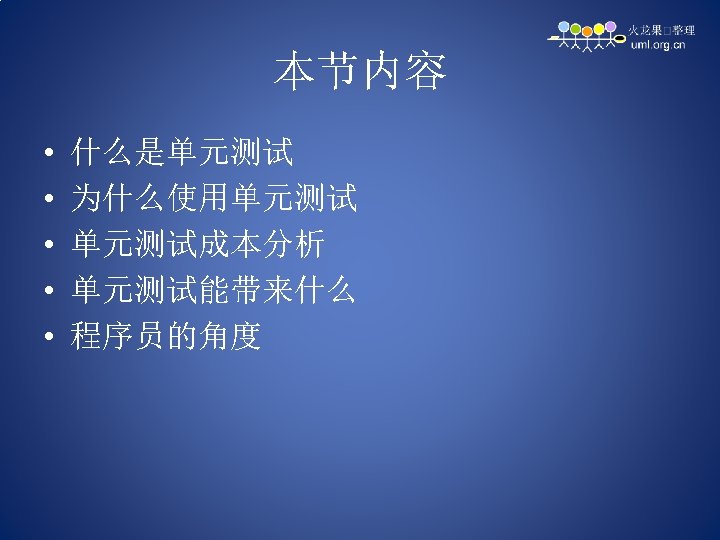
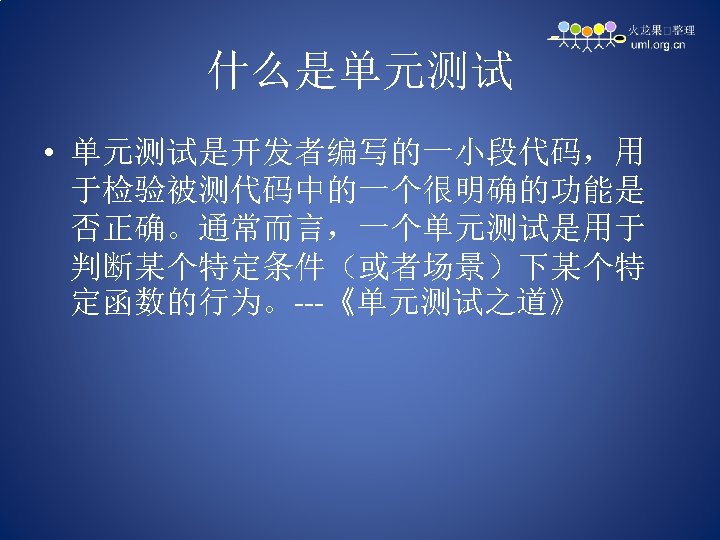
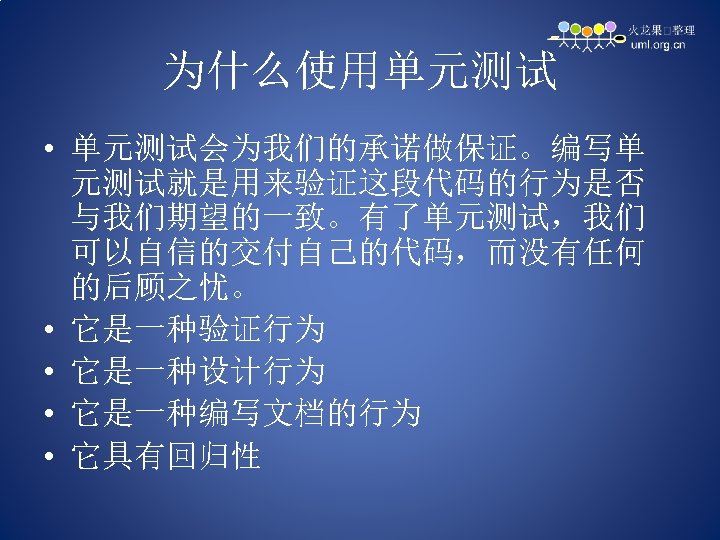
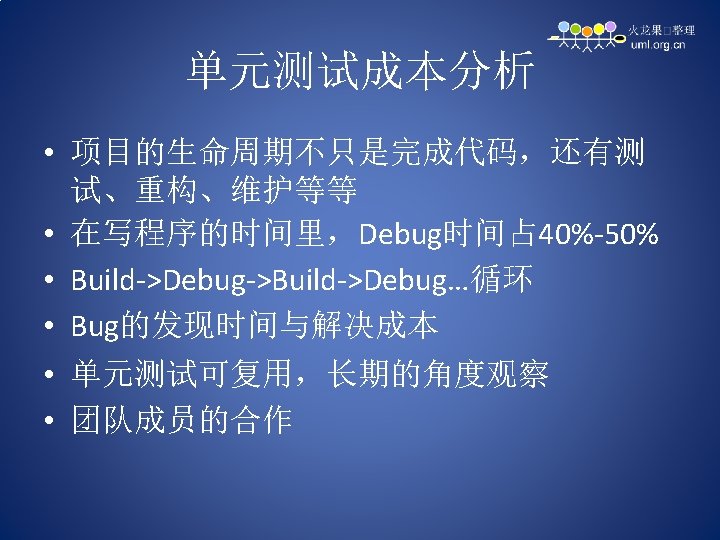
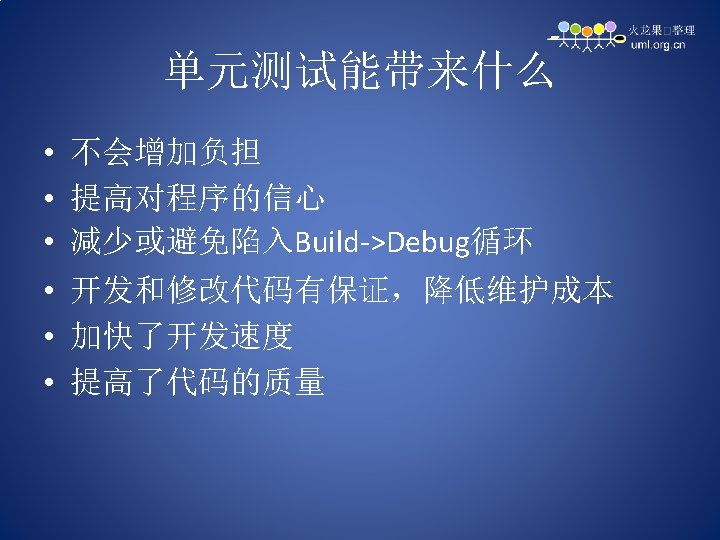
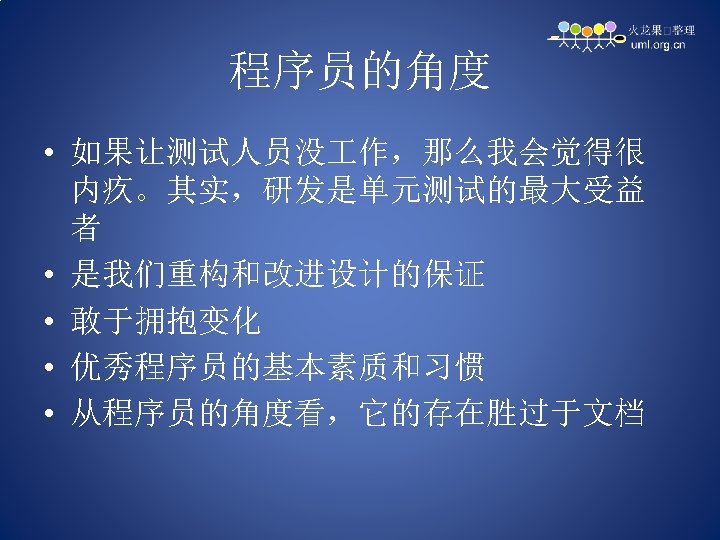
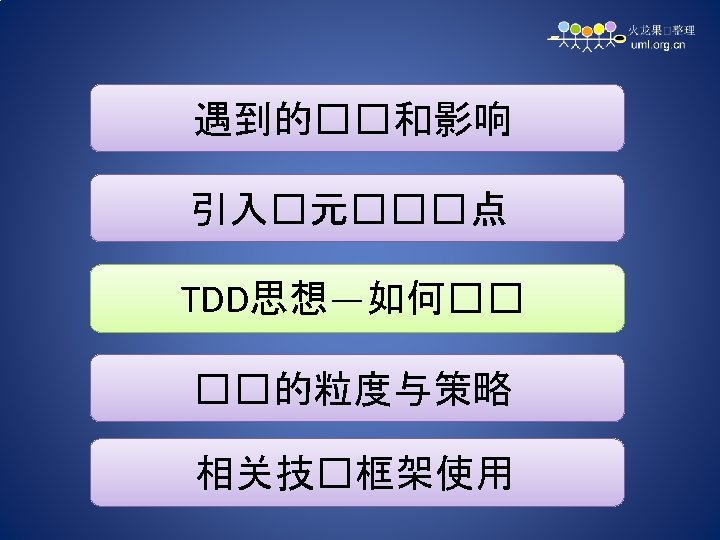
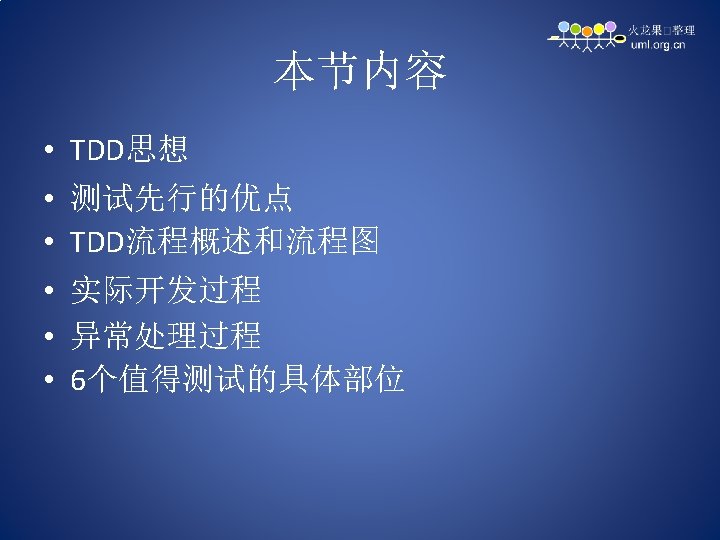
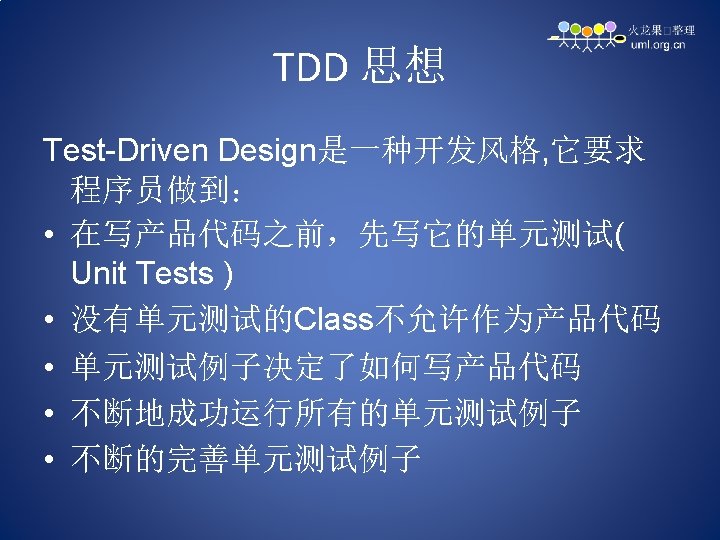
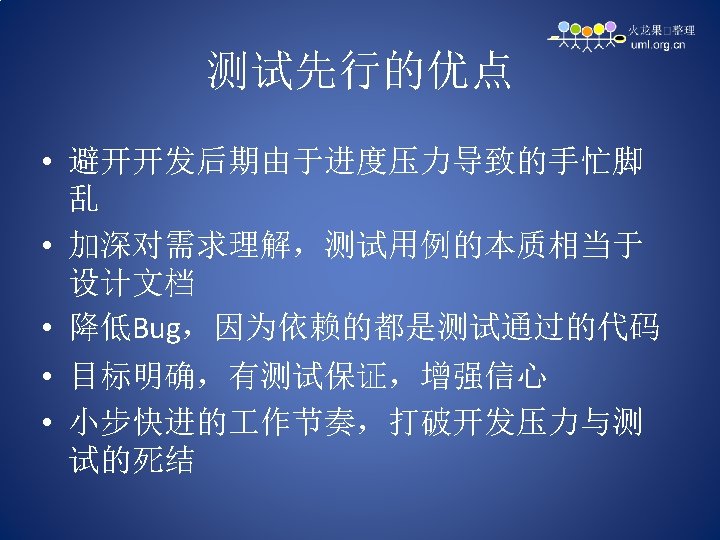
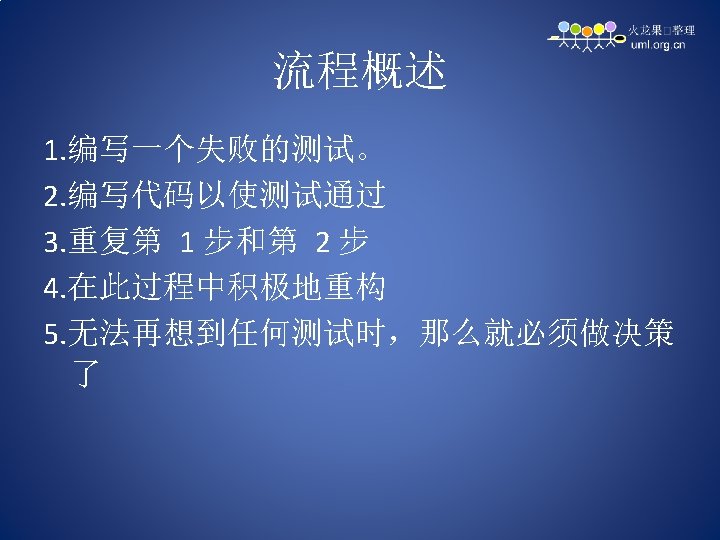
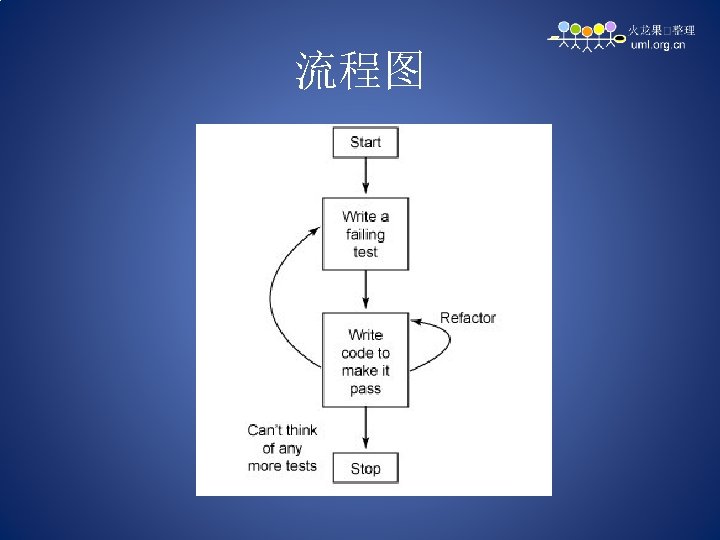
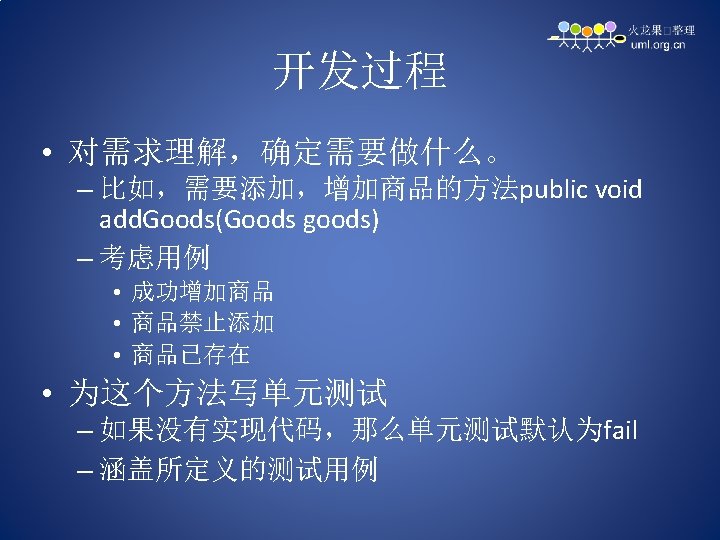
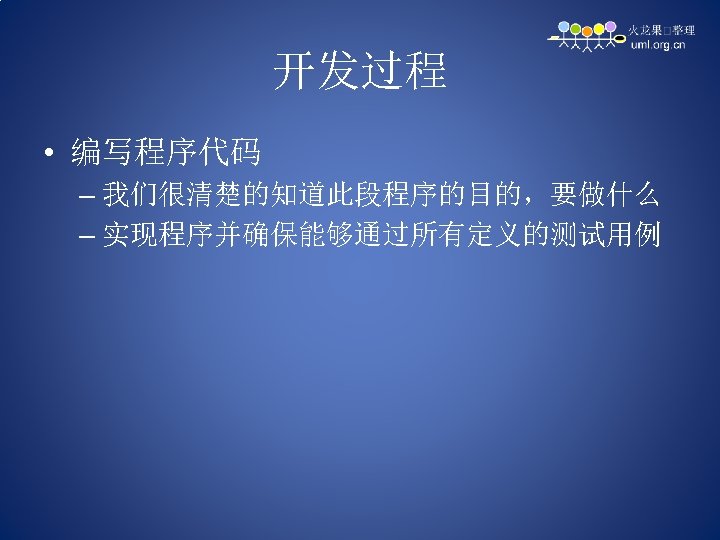
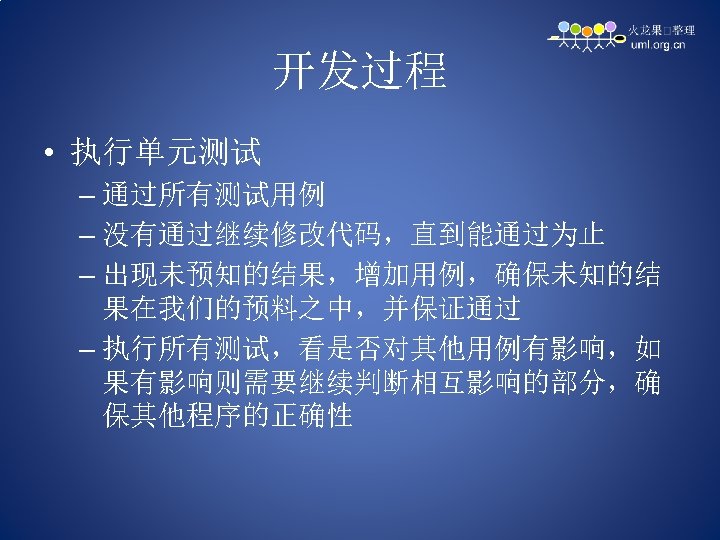
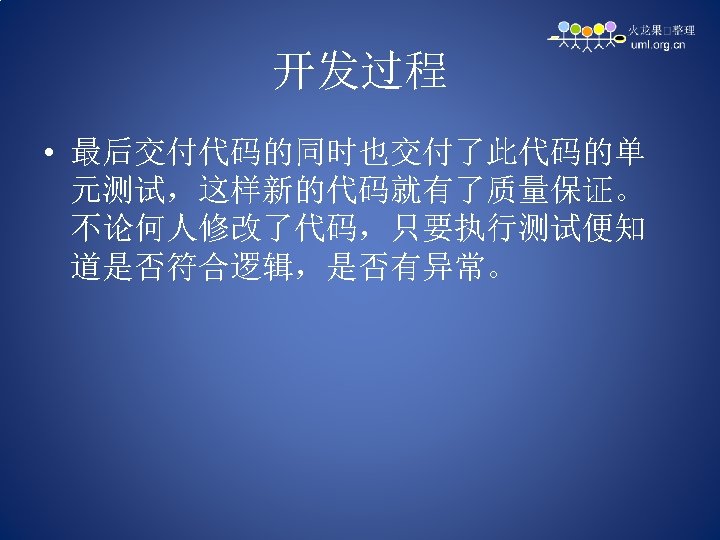
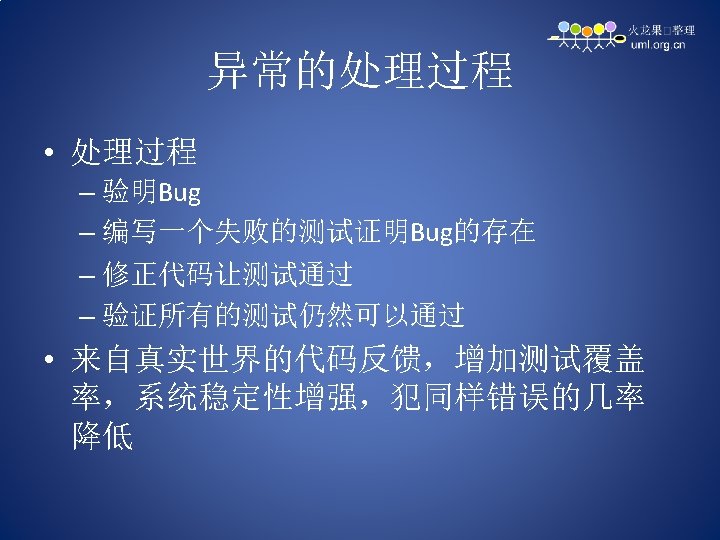
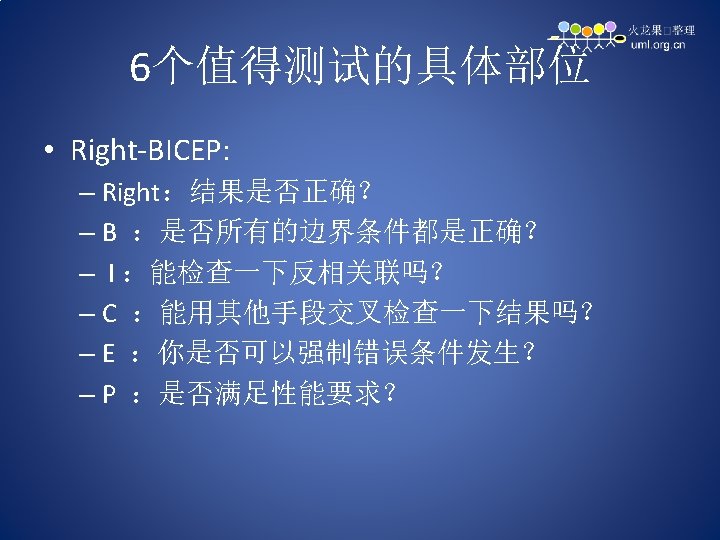
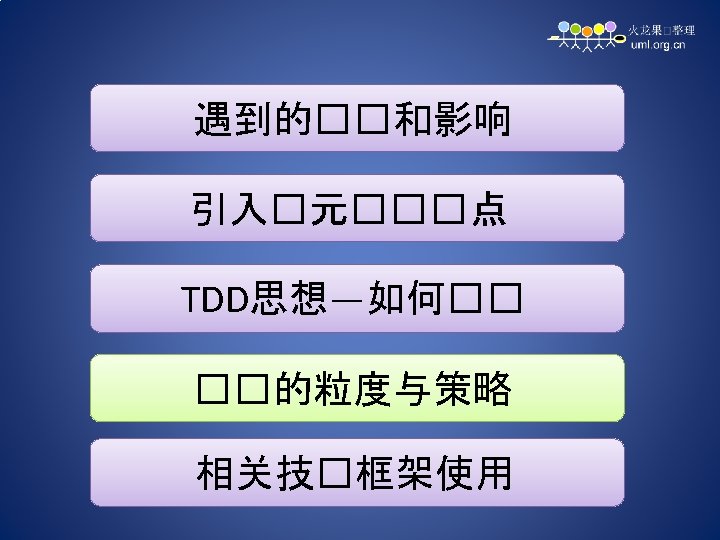
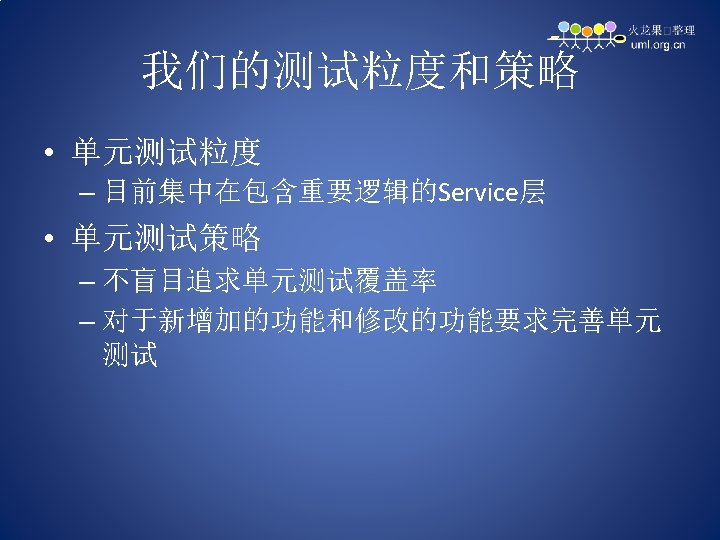
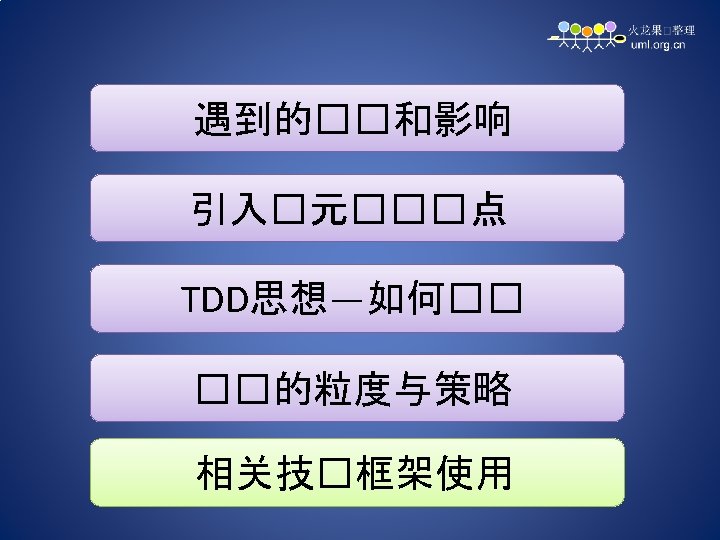
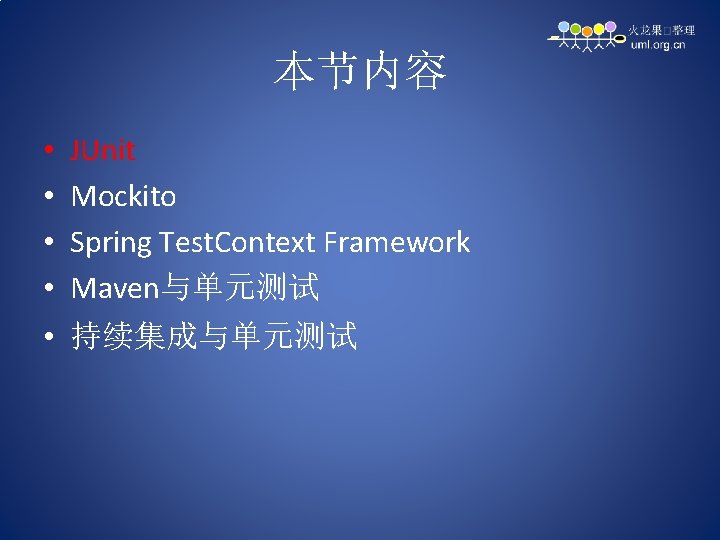
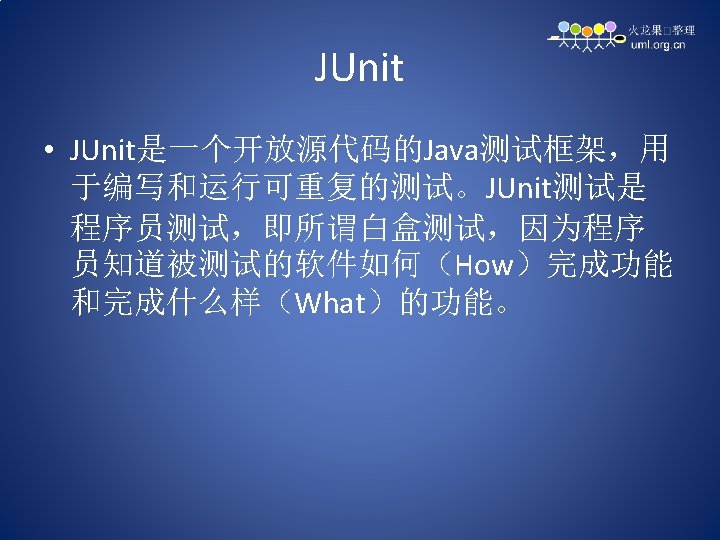
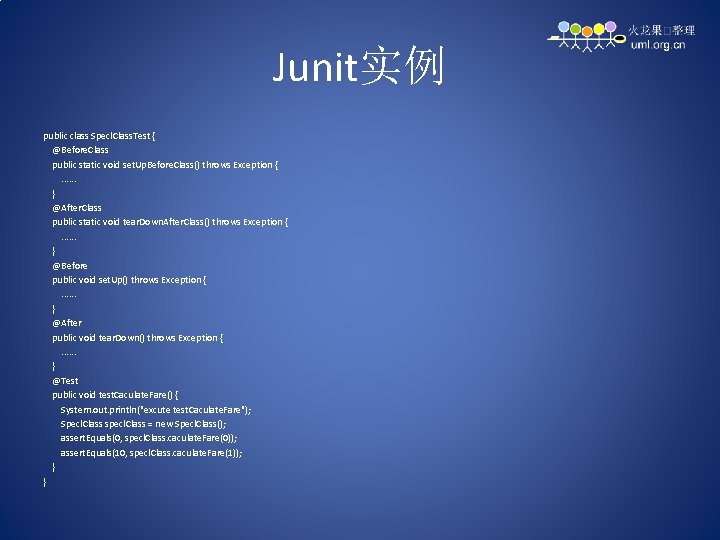
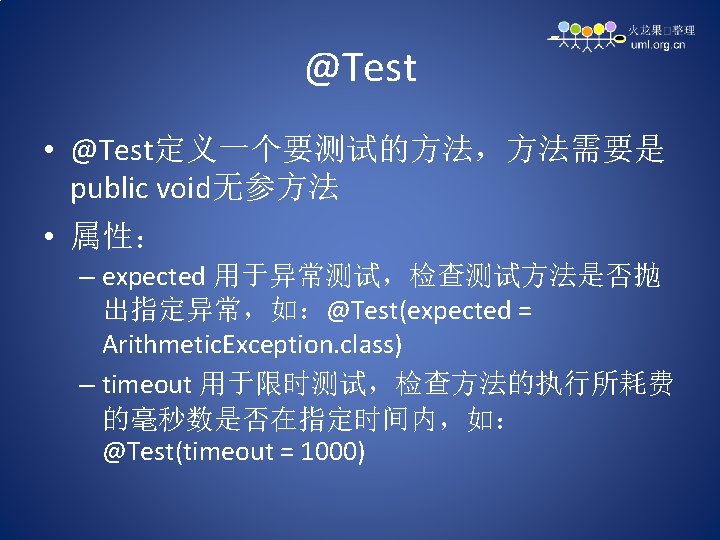
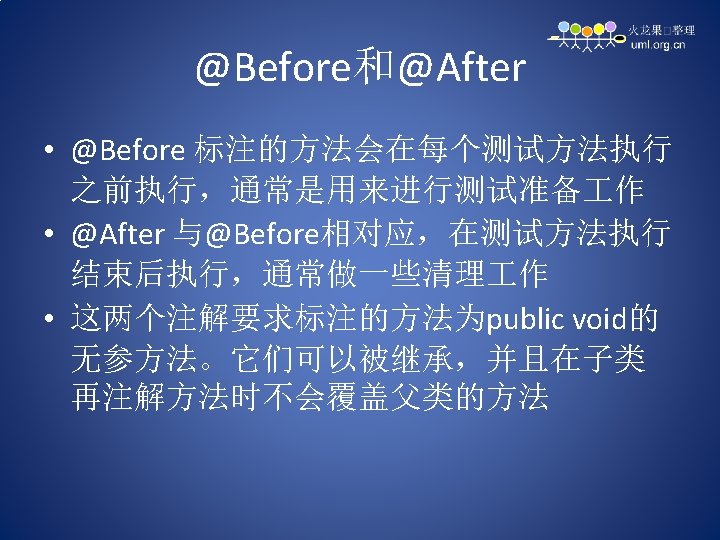
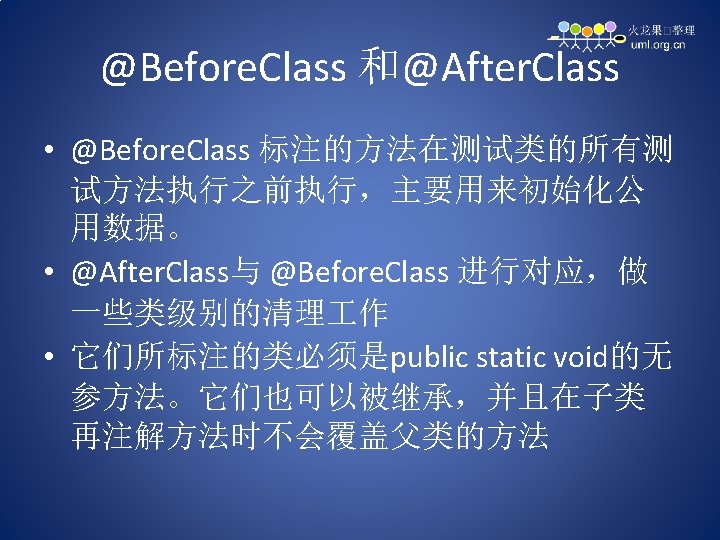
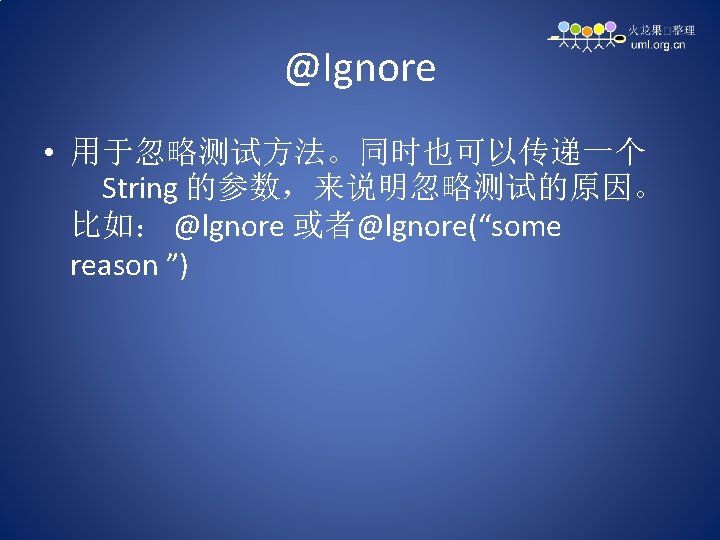
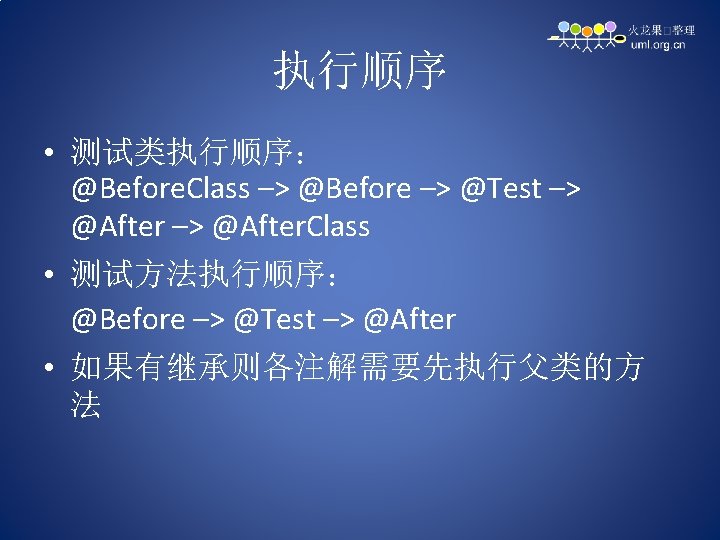
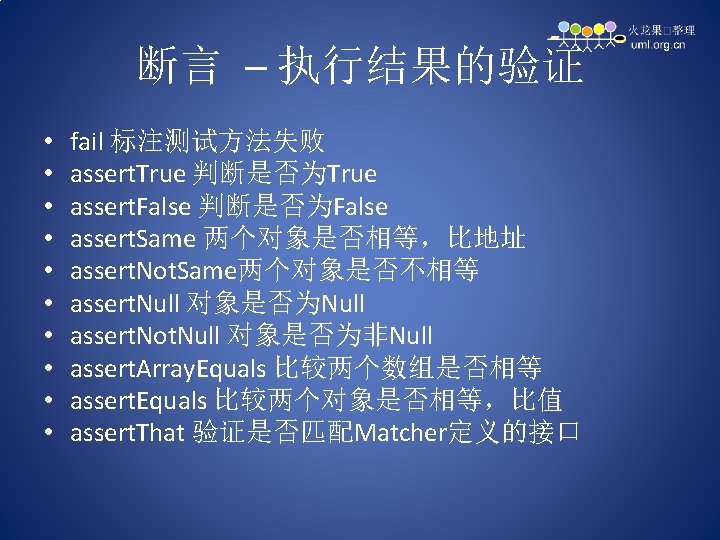
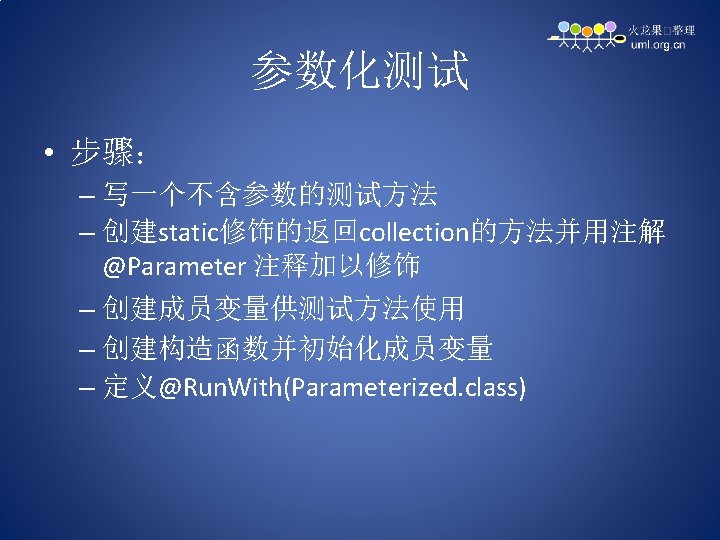
![参数化测试实例 @Run. With(Parameterized. class) public class Fibonacci. Test { @Parameters public static List<Object[]> data() 参数化测试实例 @Run. With(Parameterized. class) public class Fibonacci. Test { @Parameters public static List<Object[]> data()](https://slidetodoc.com/presentation_image_h2/84802b9ec27b46b3be9dcd68072b0711/image-38.jpg)
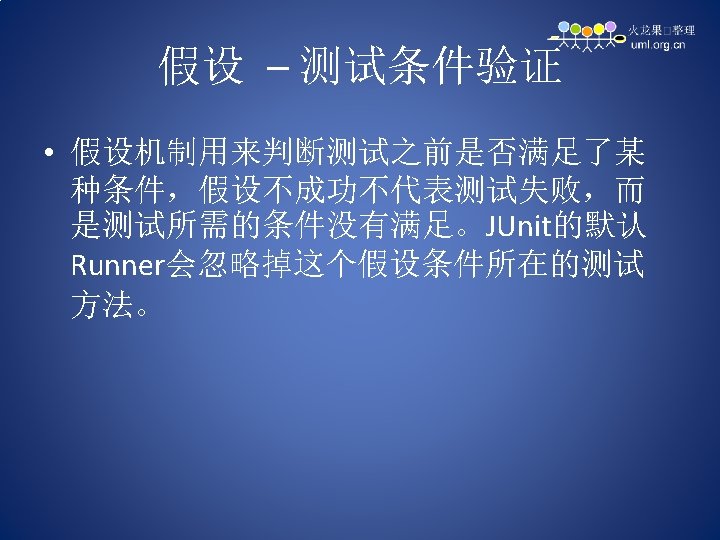
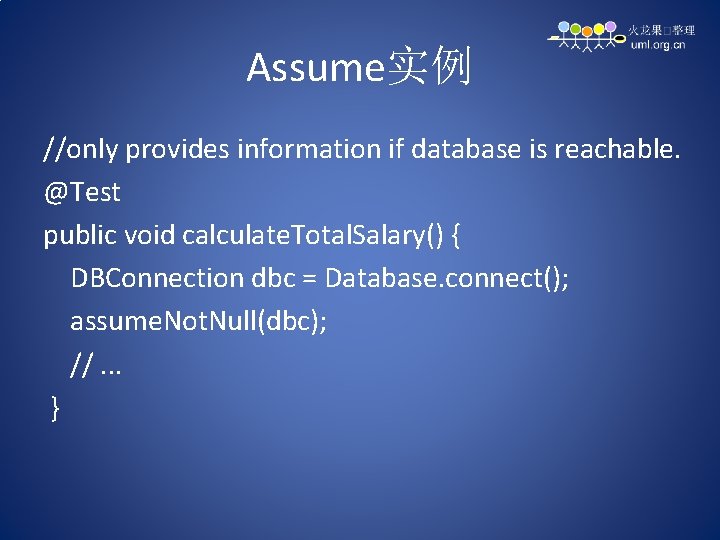
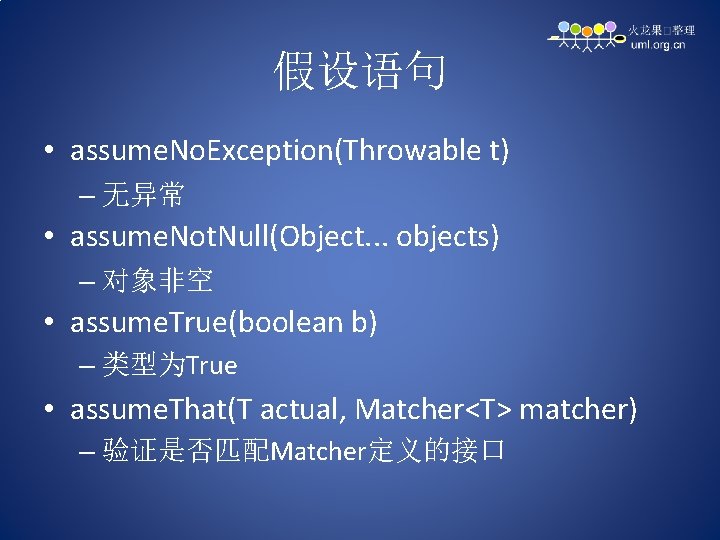
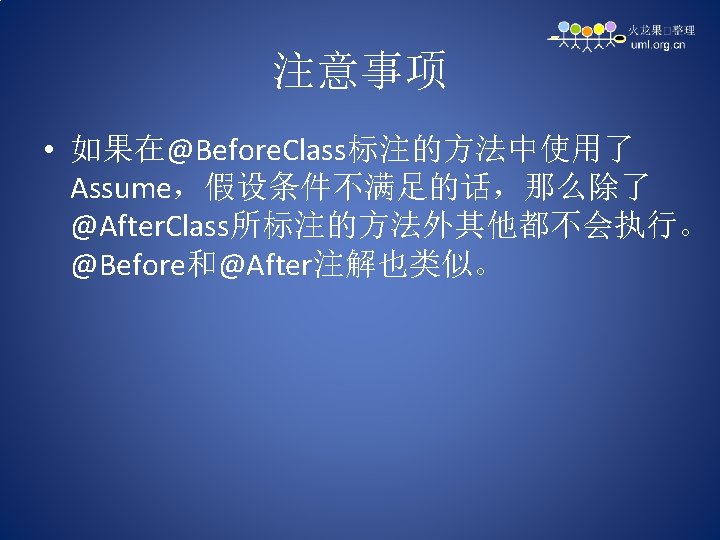
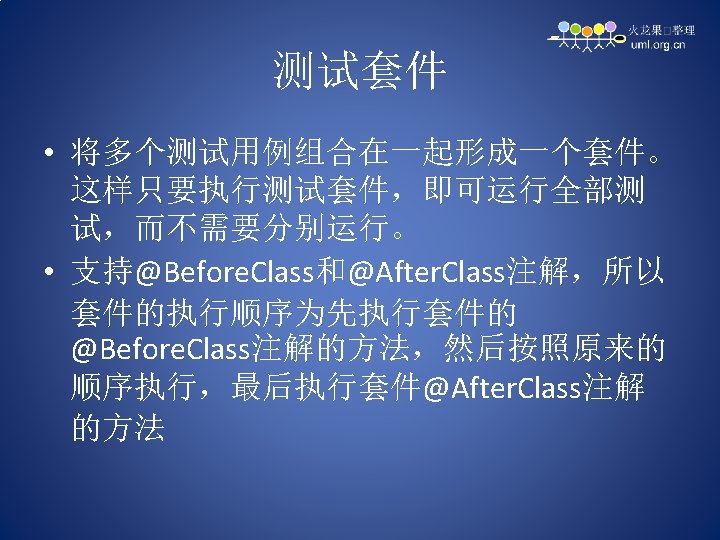
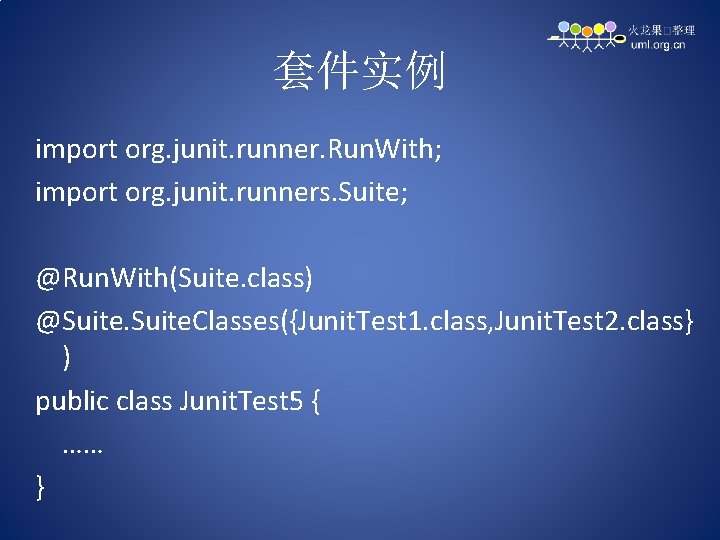
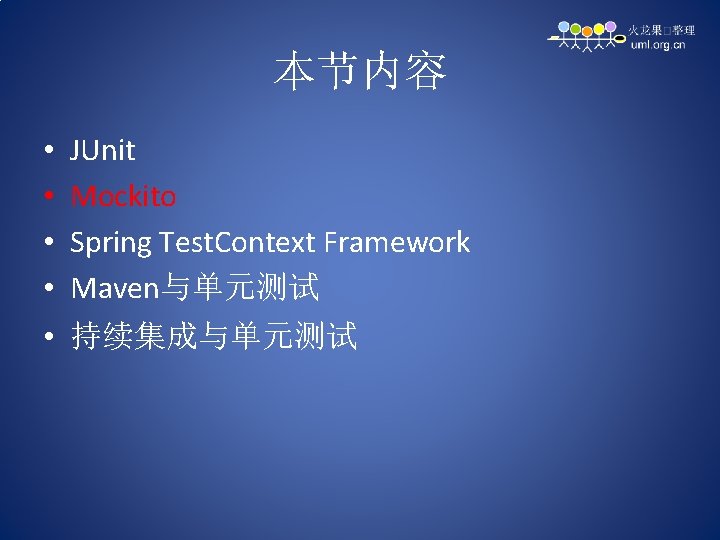
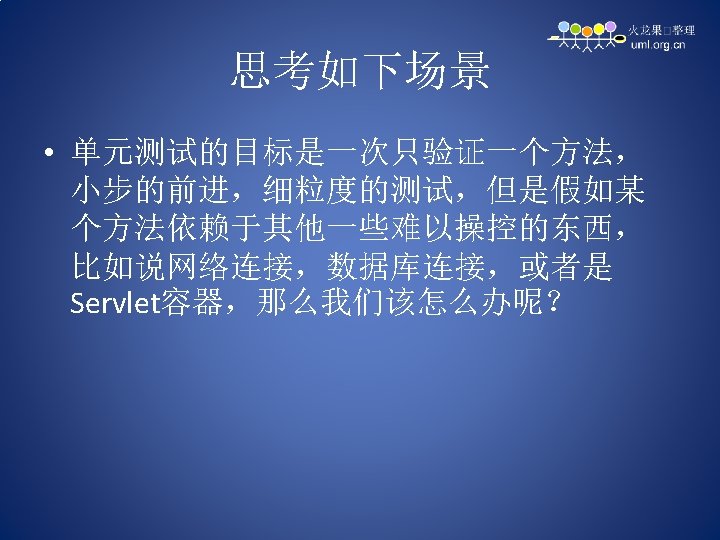
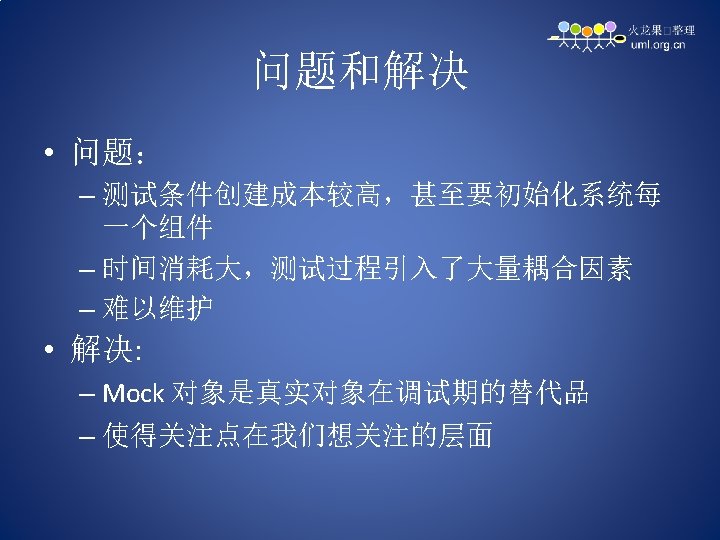
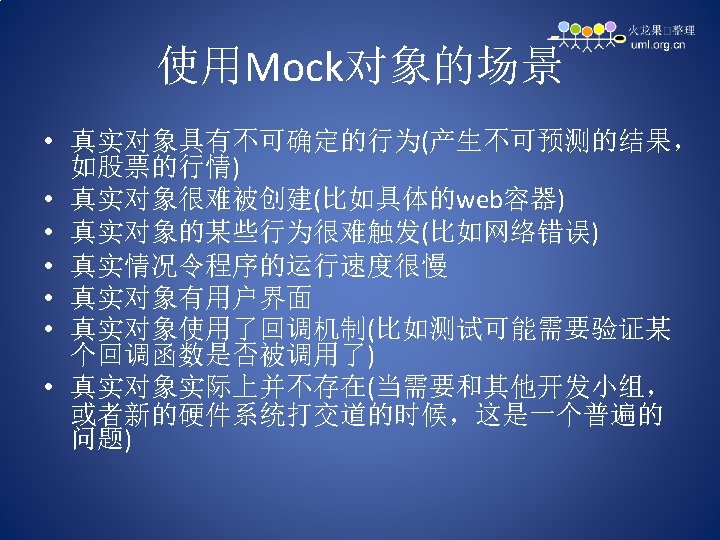
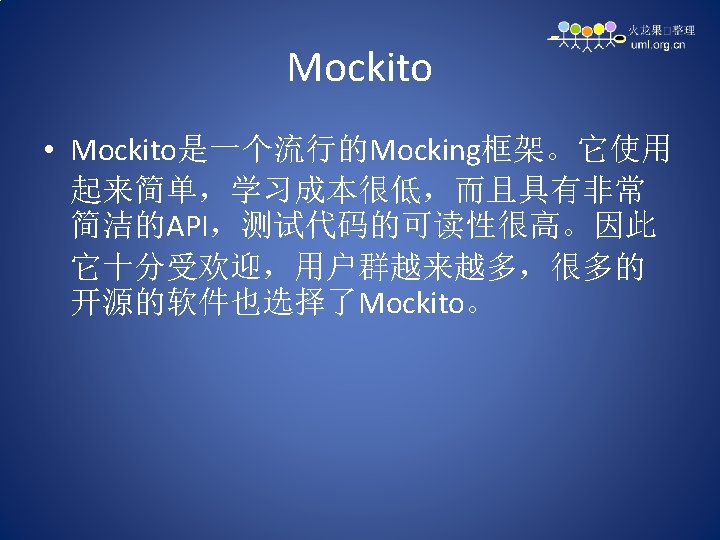
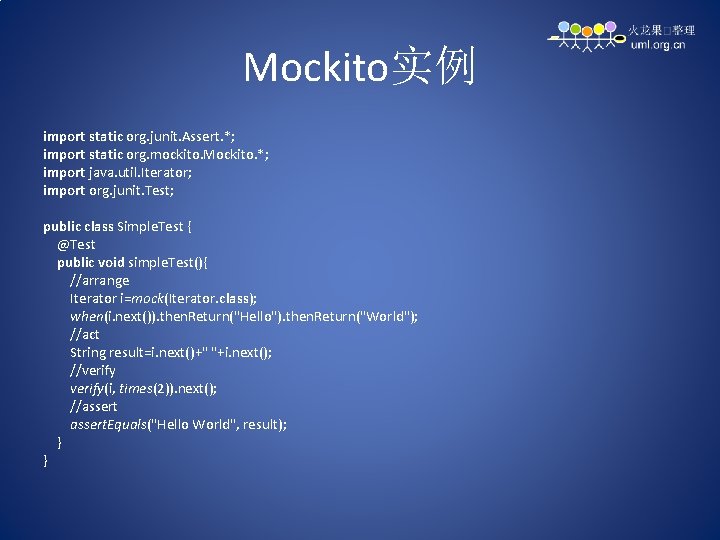
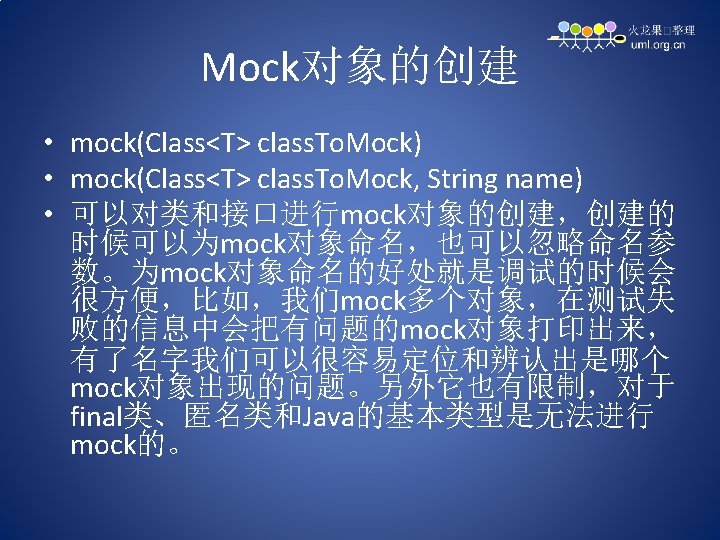
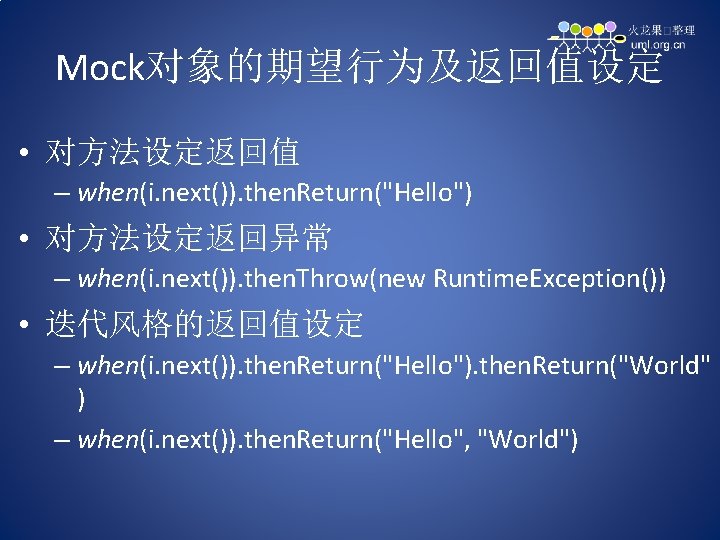
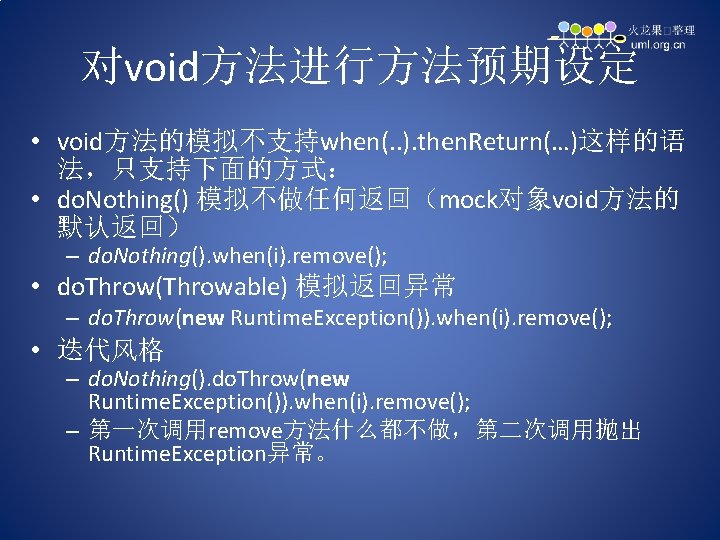
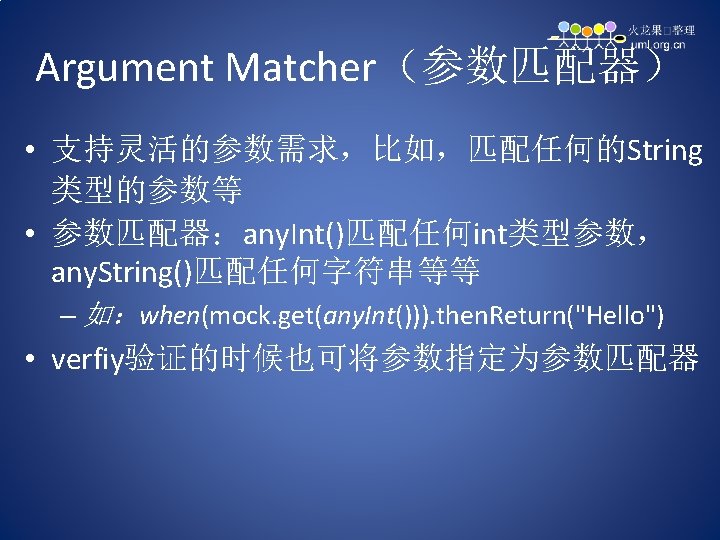
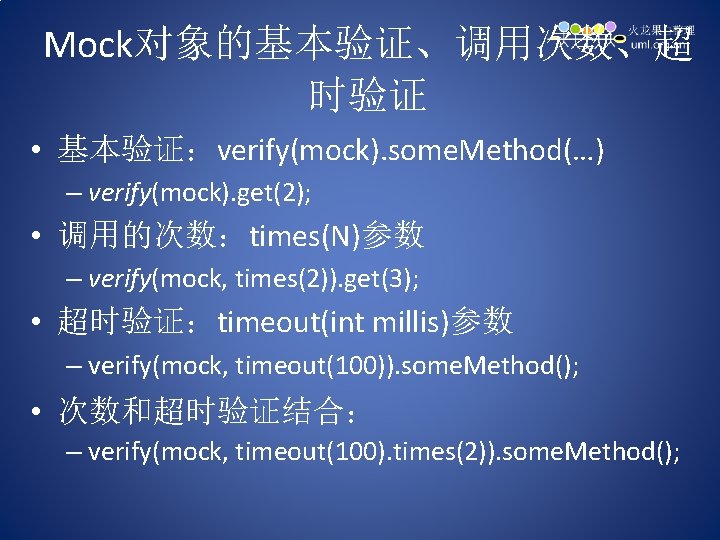
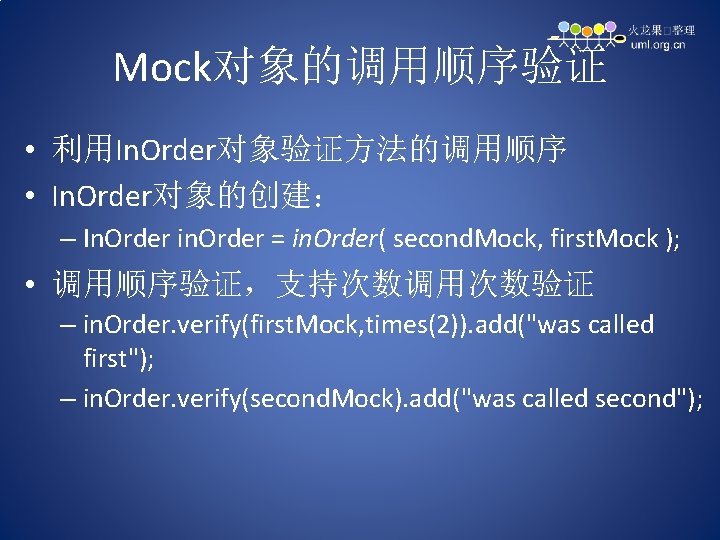
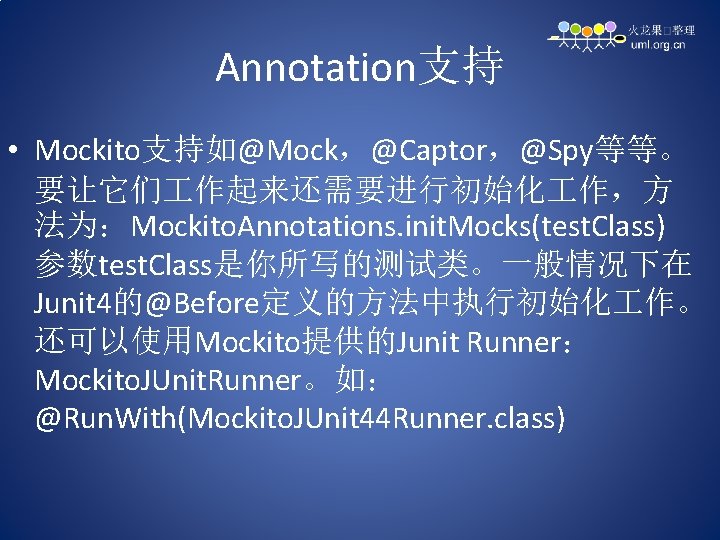
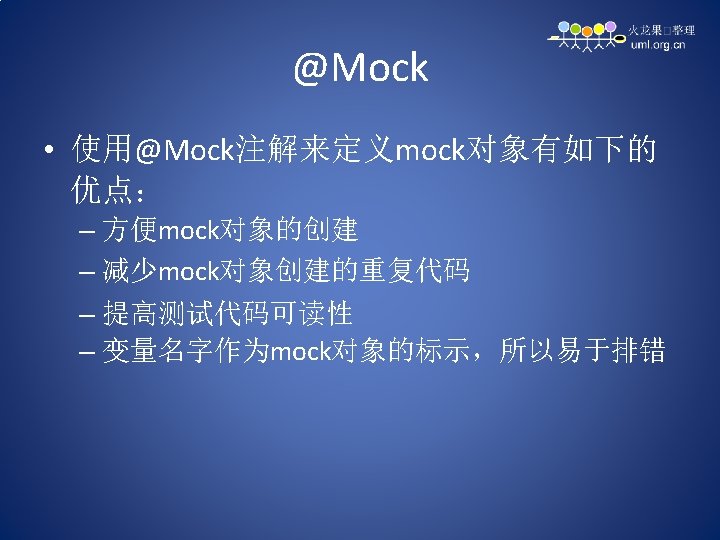
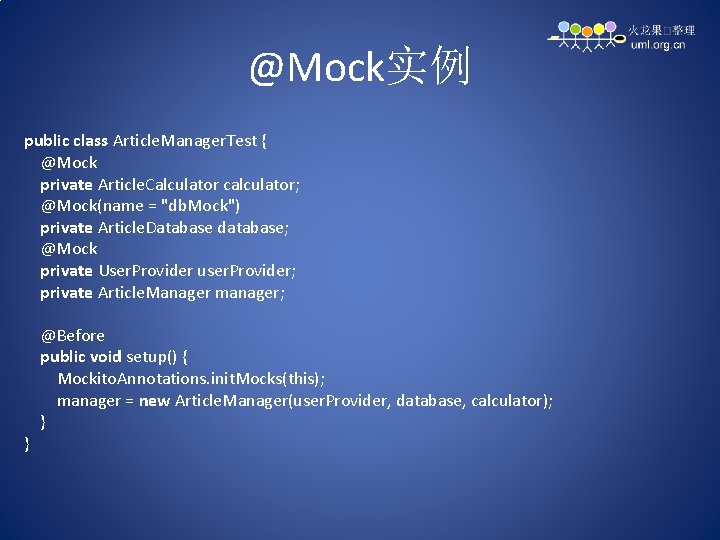
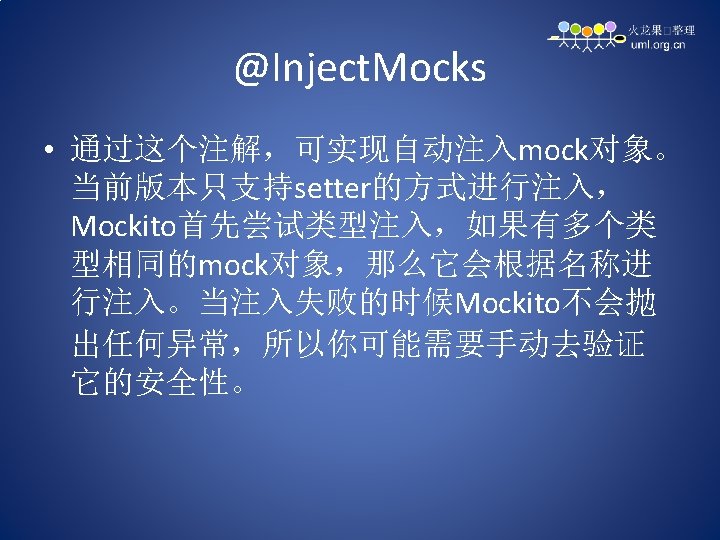
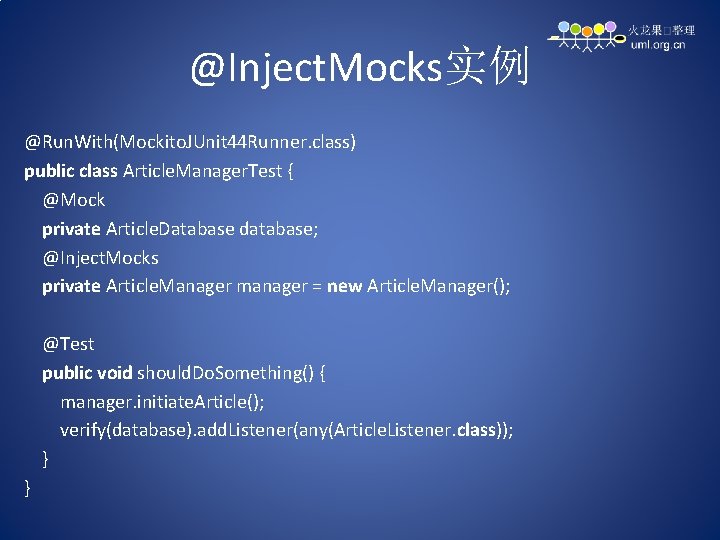
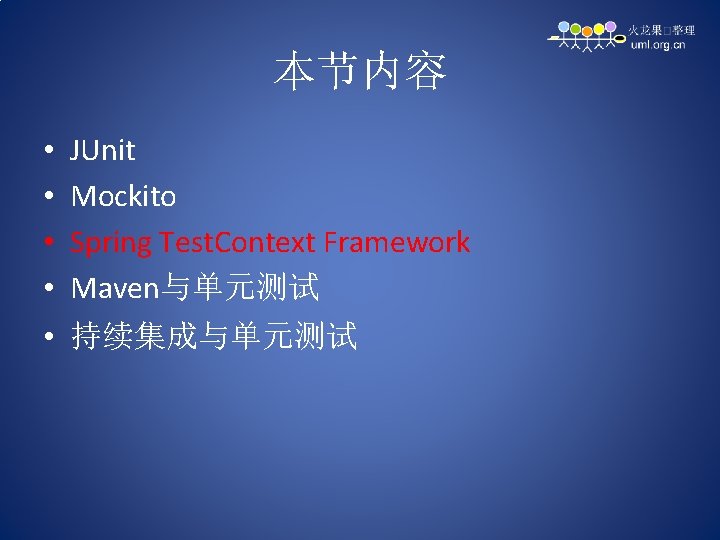
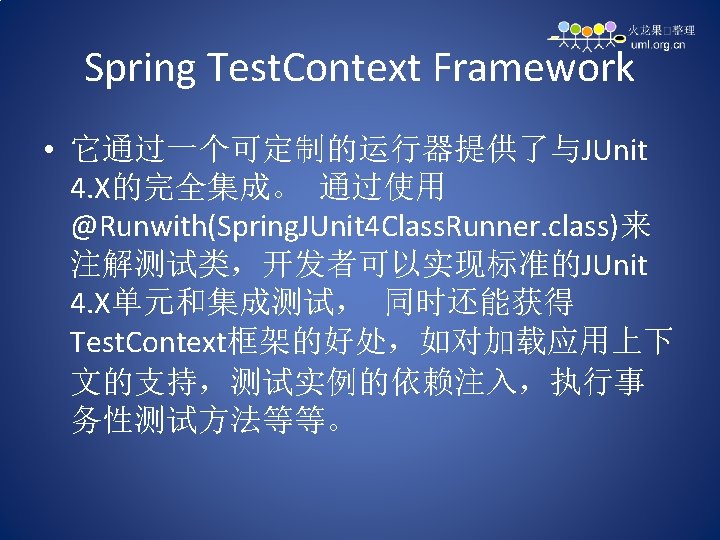
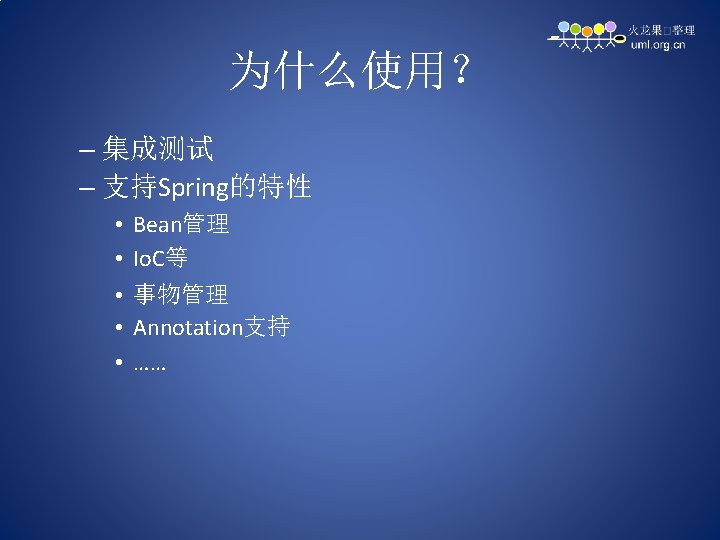
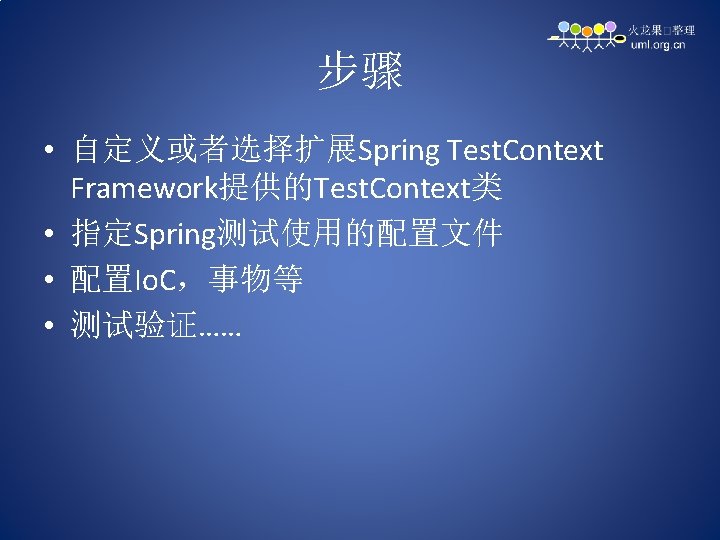
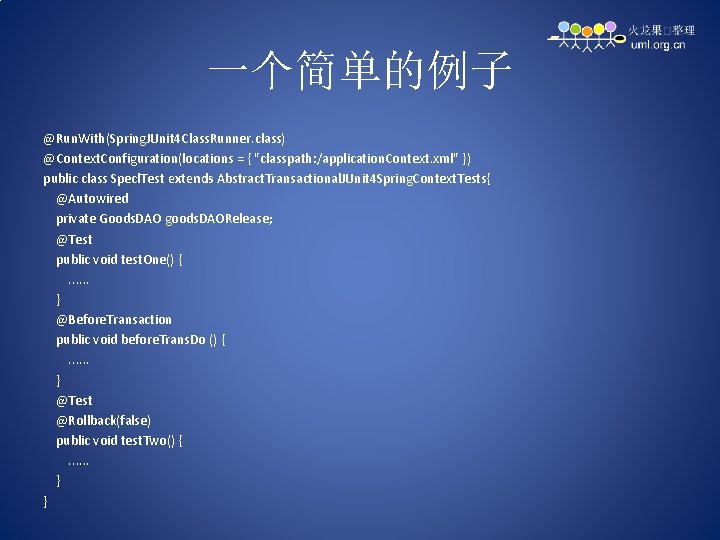
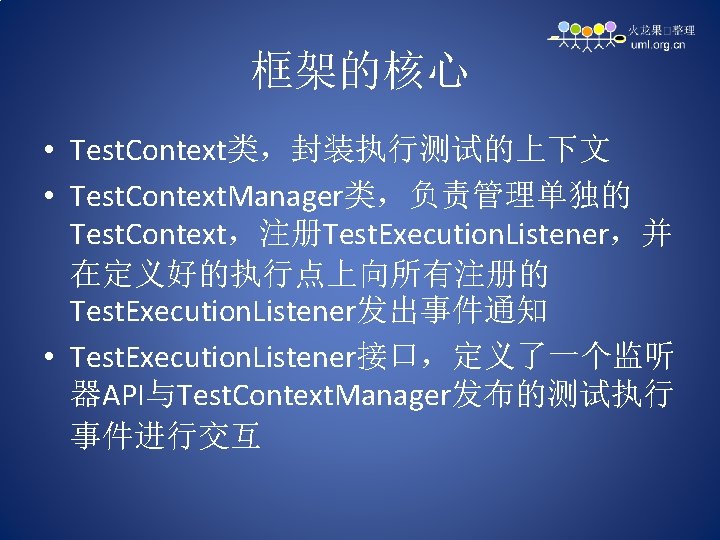
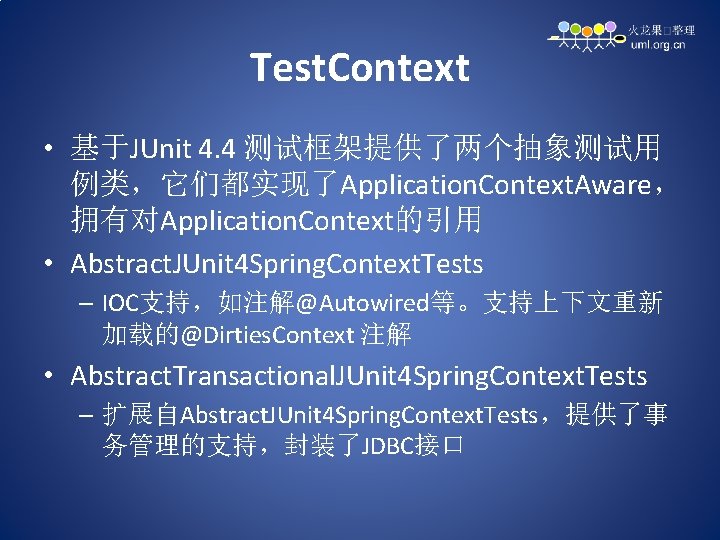
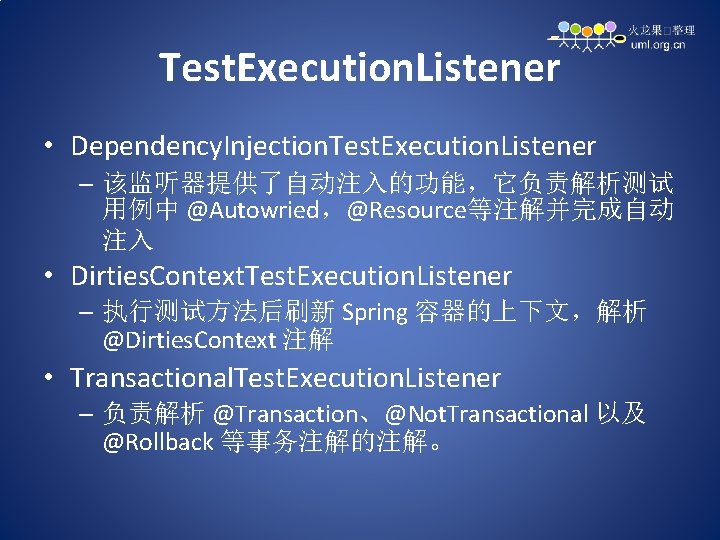
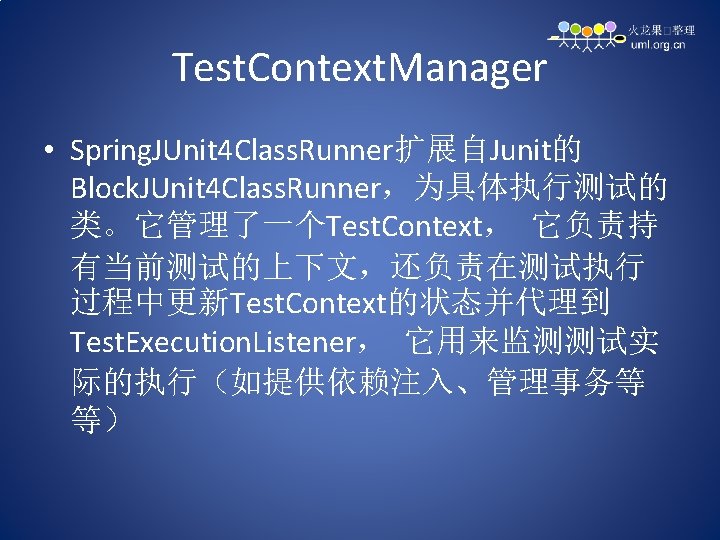
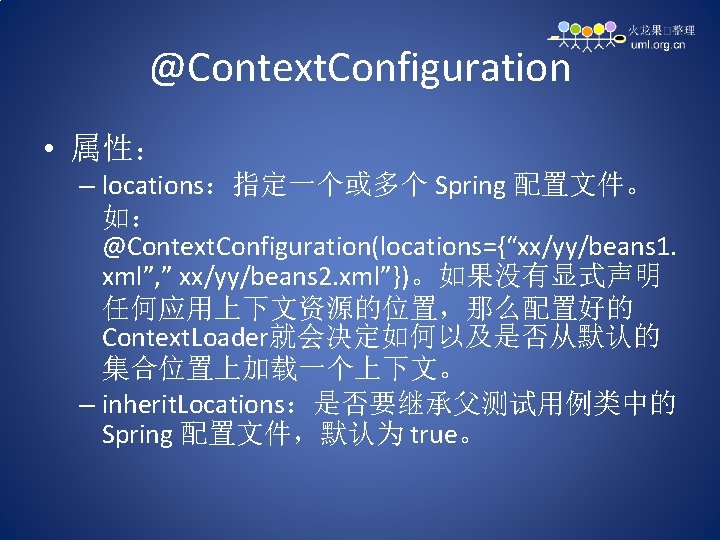
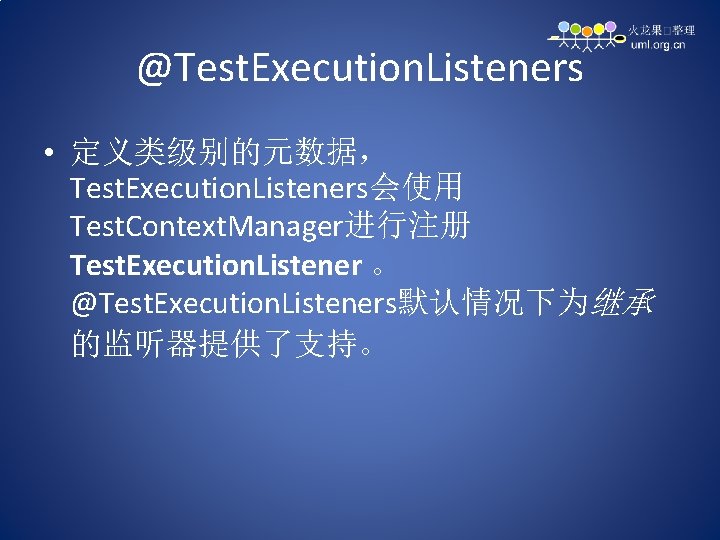
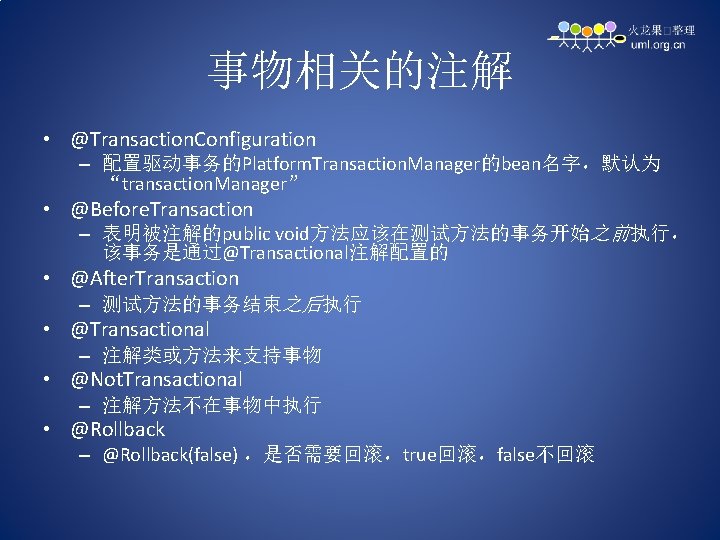
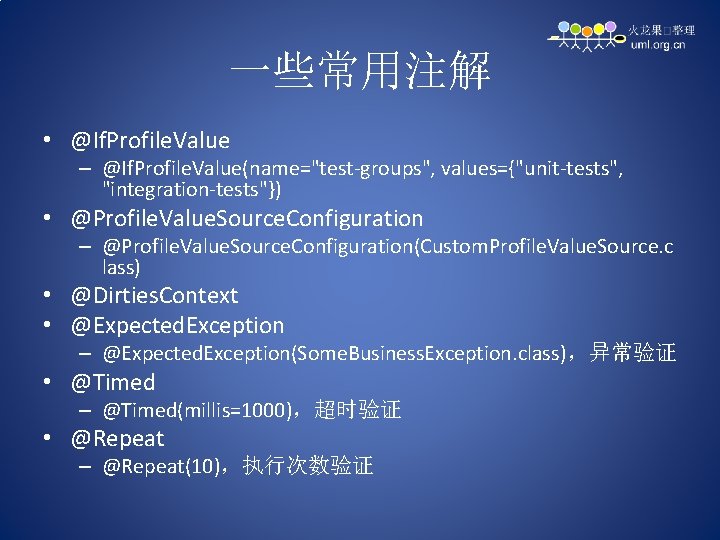
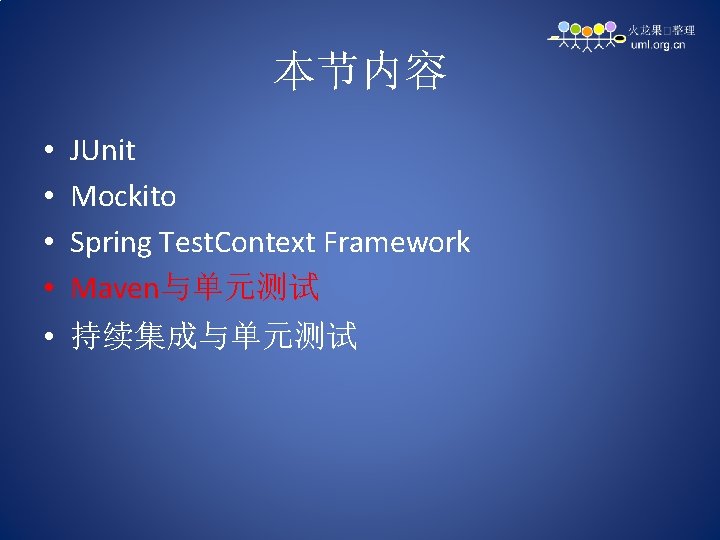
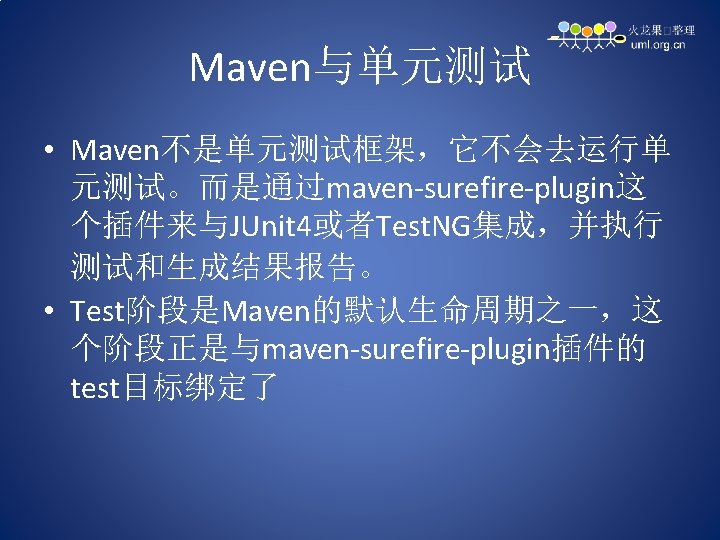
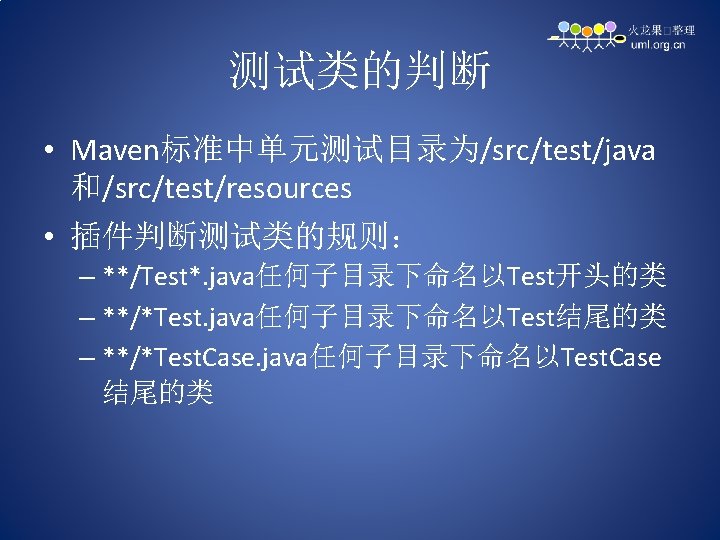
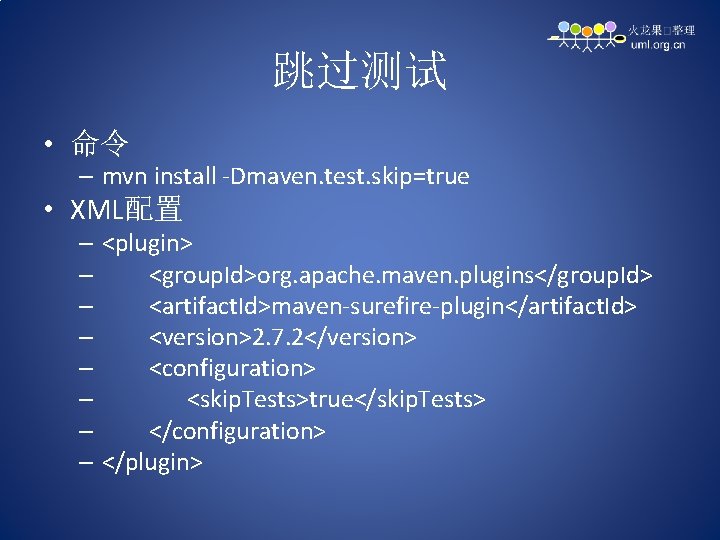
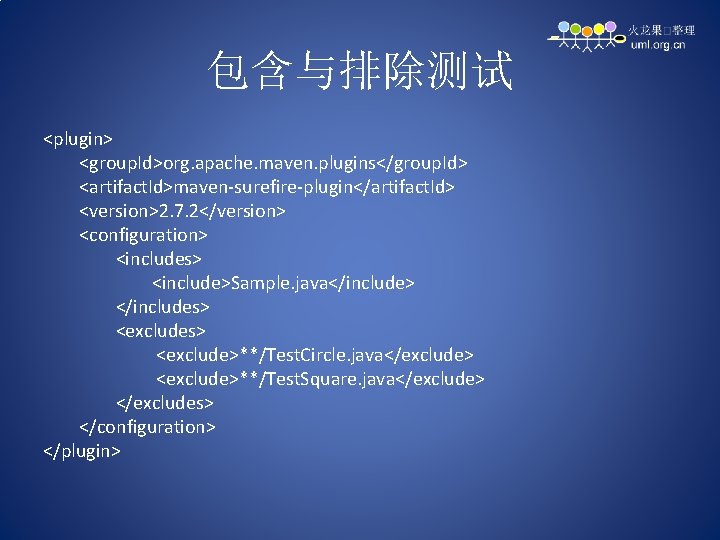
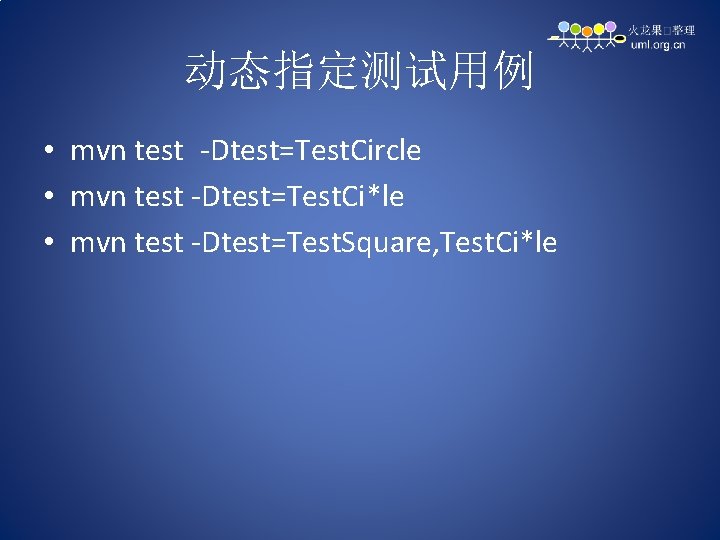
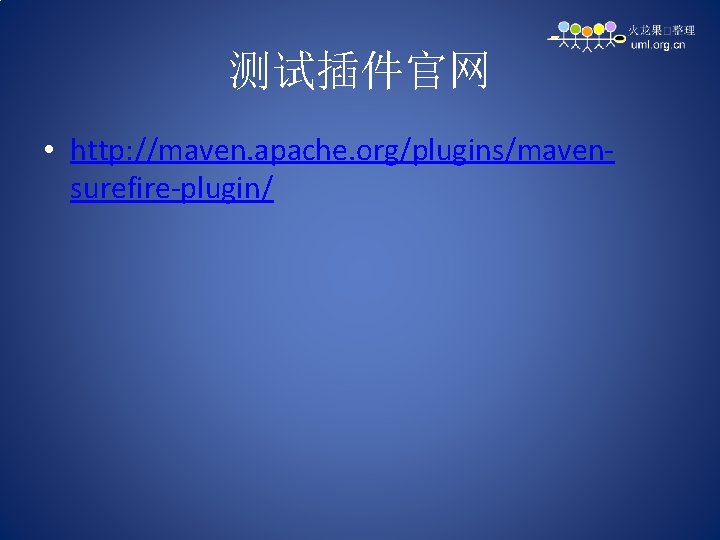
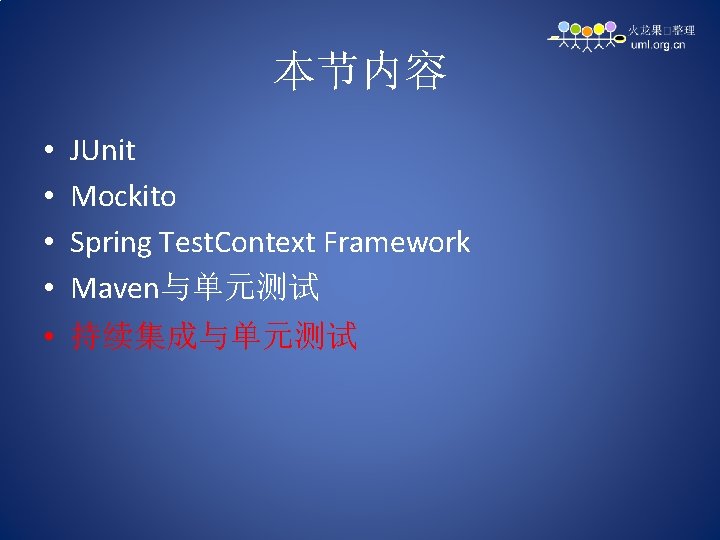
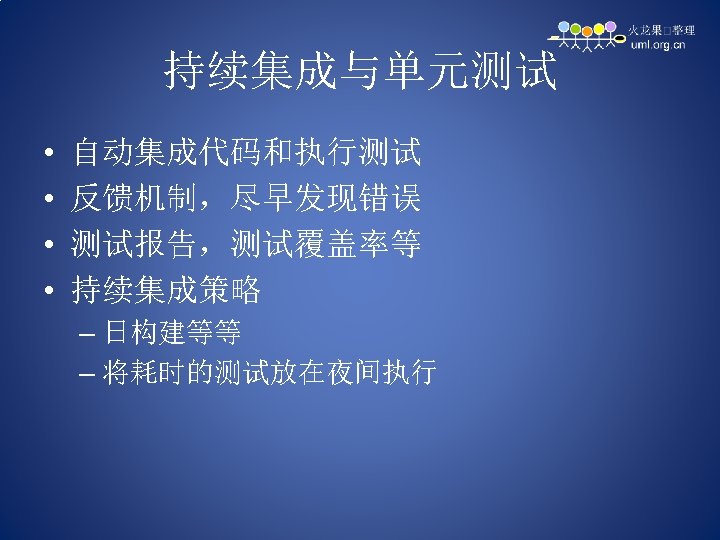
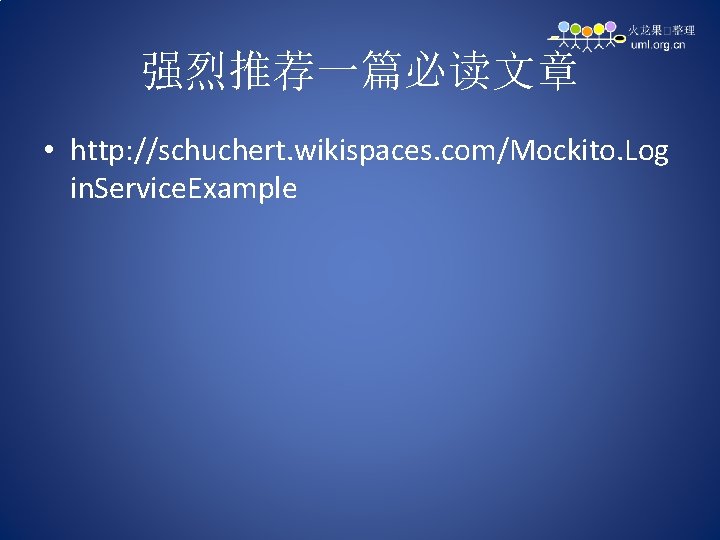
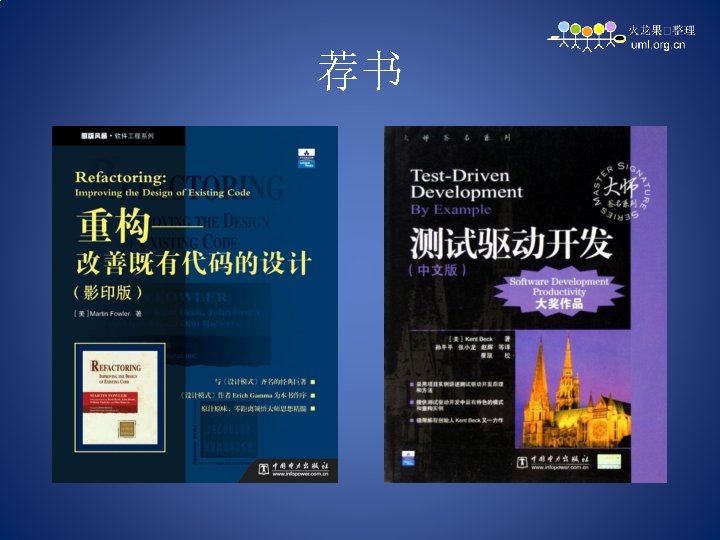
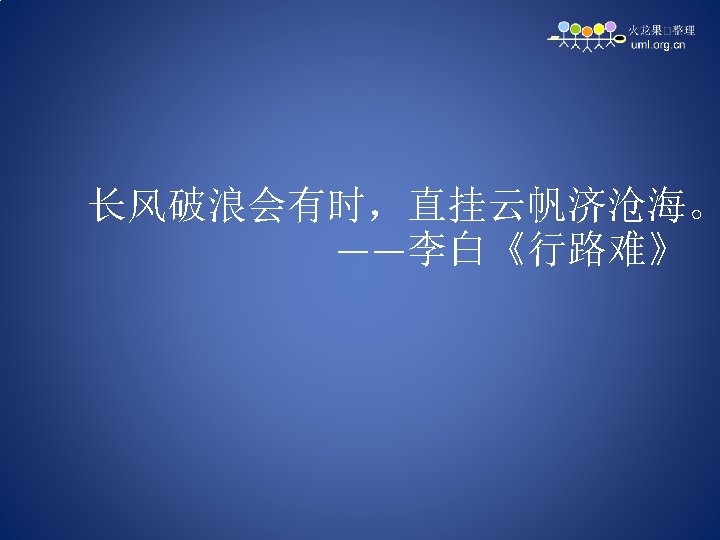
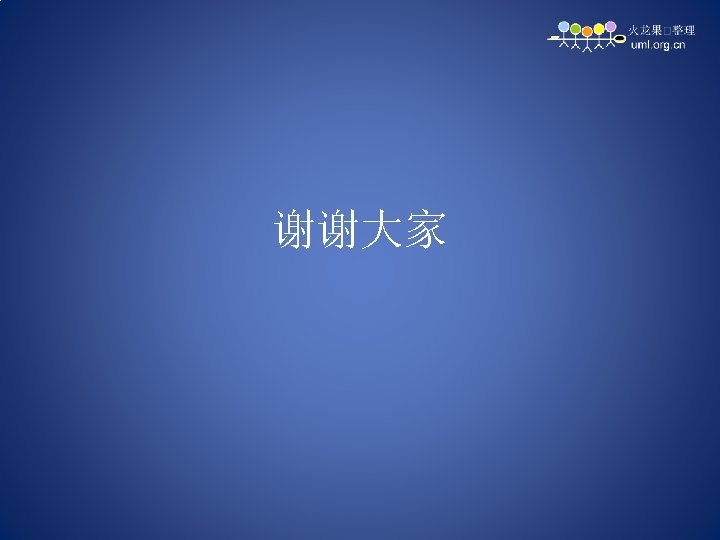
- Slides: 87
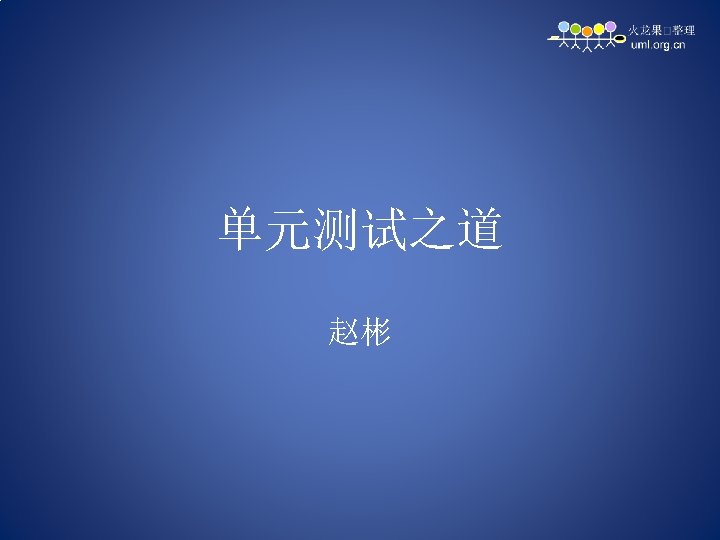
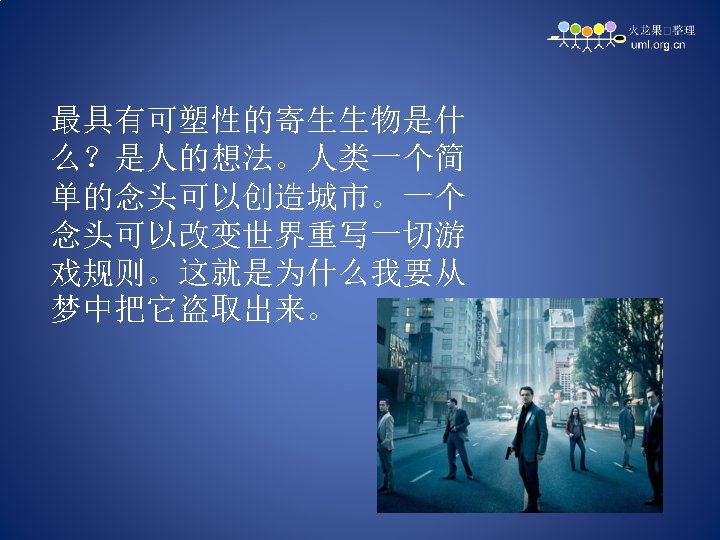
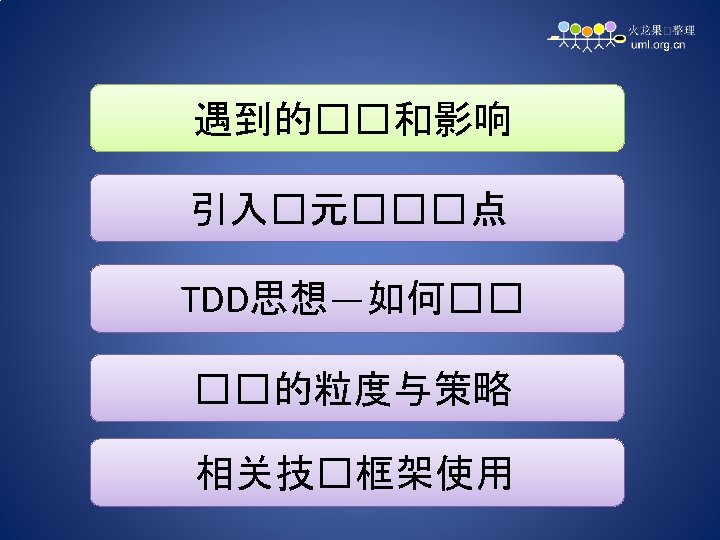
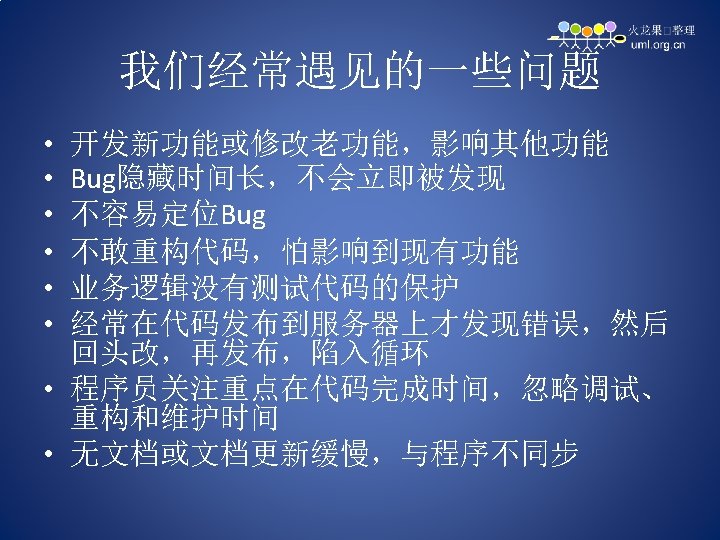
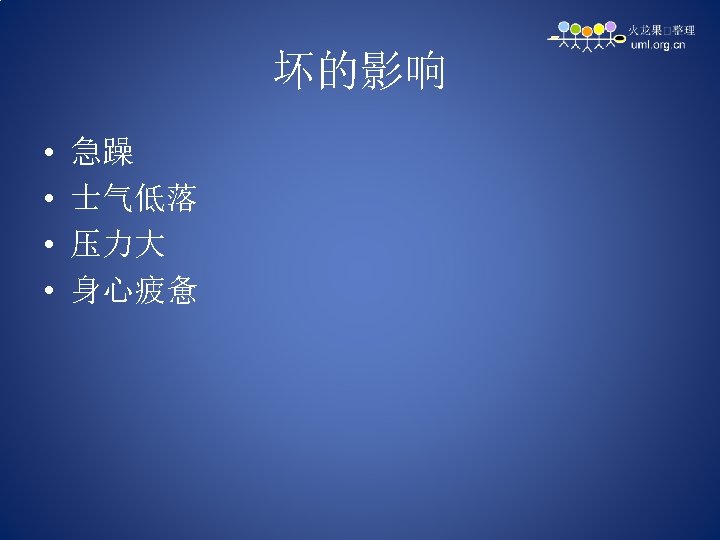
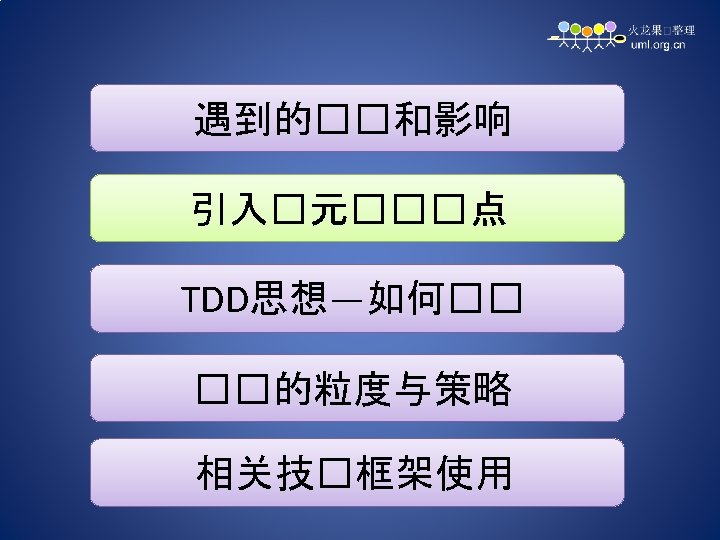
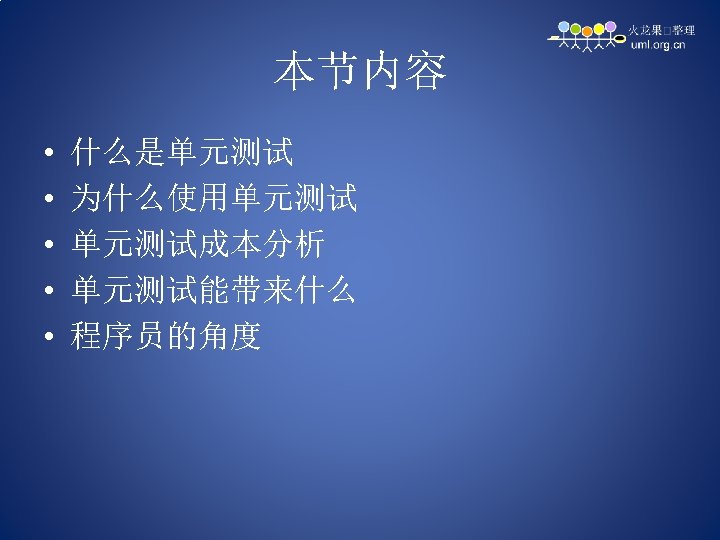
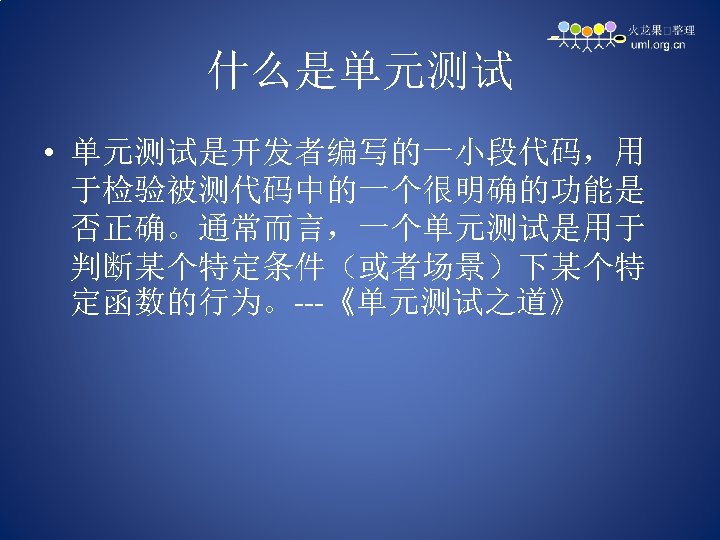
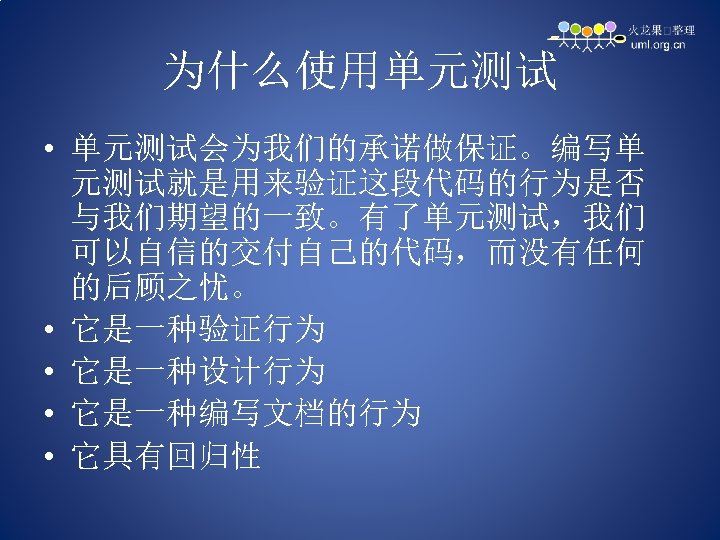
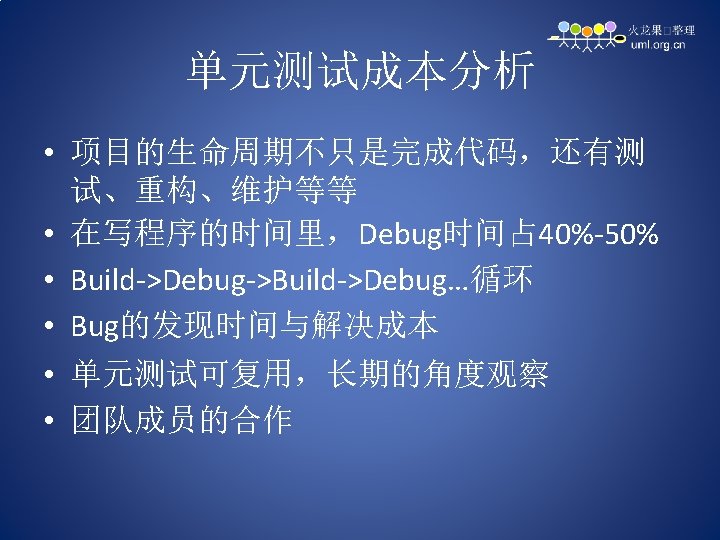
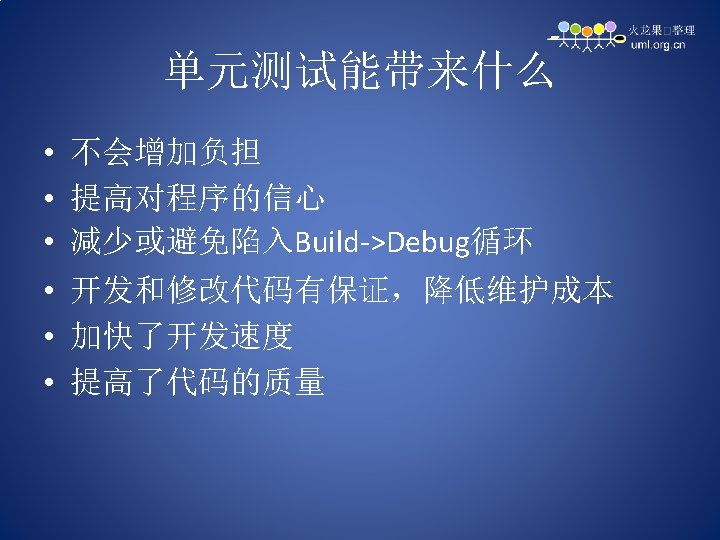
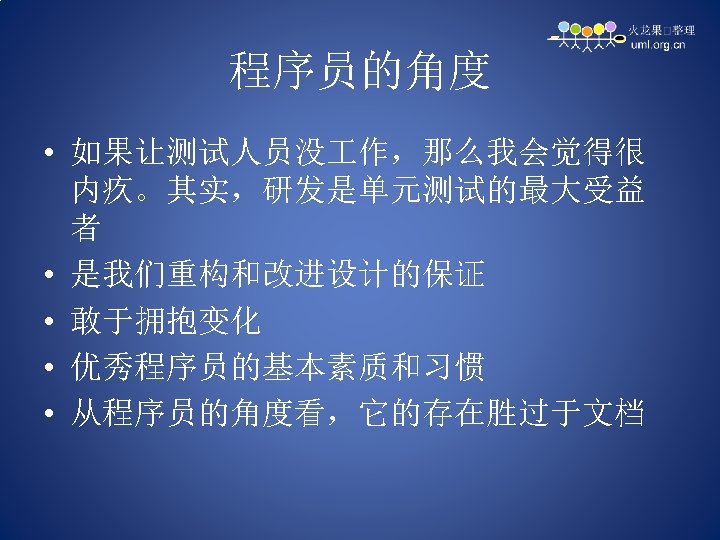
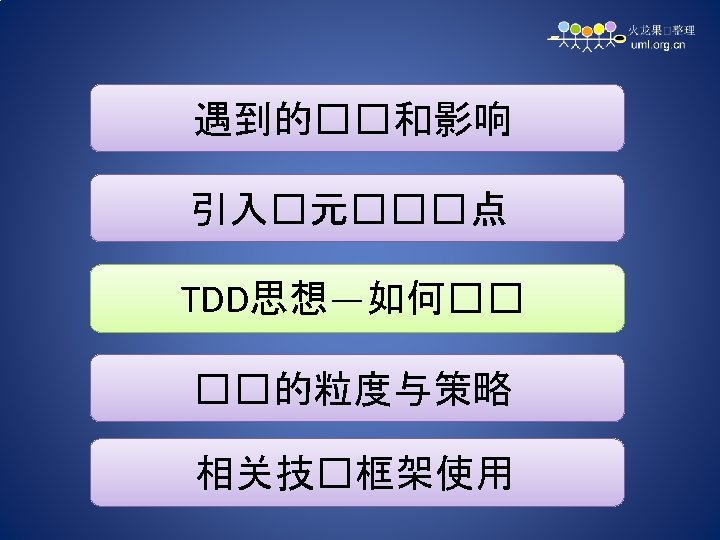
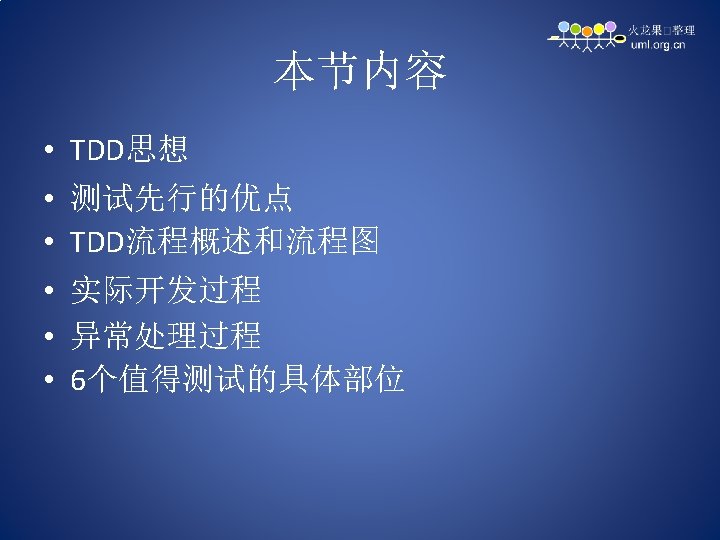
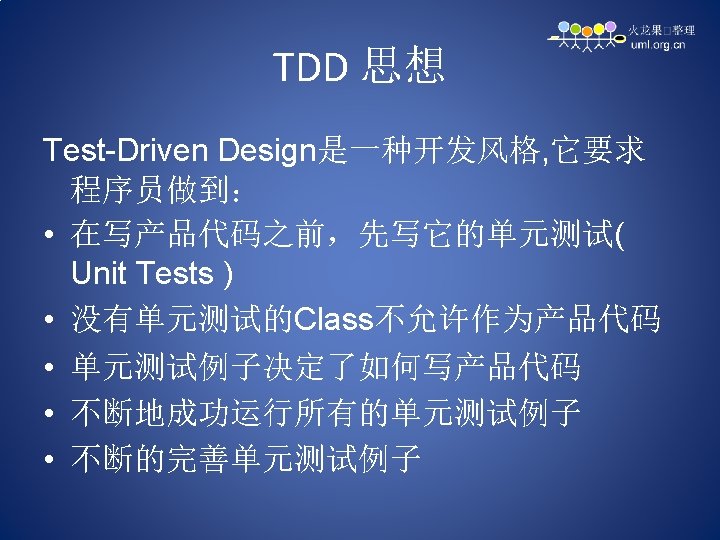
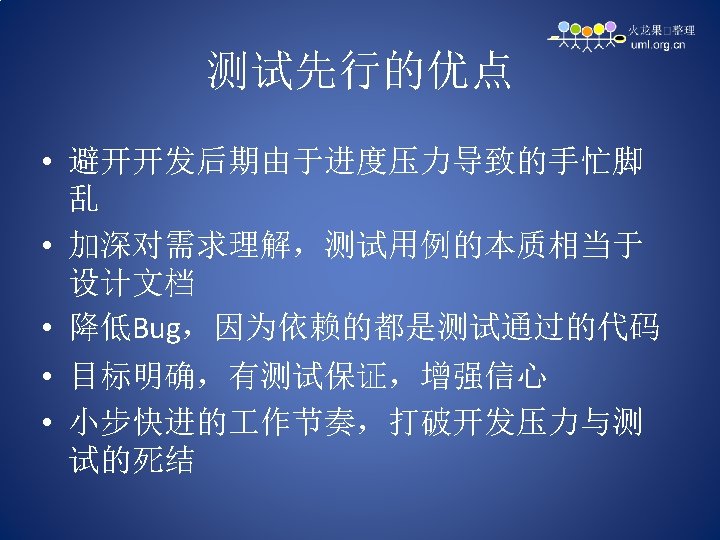
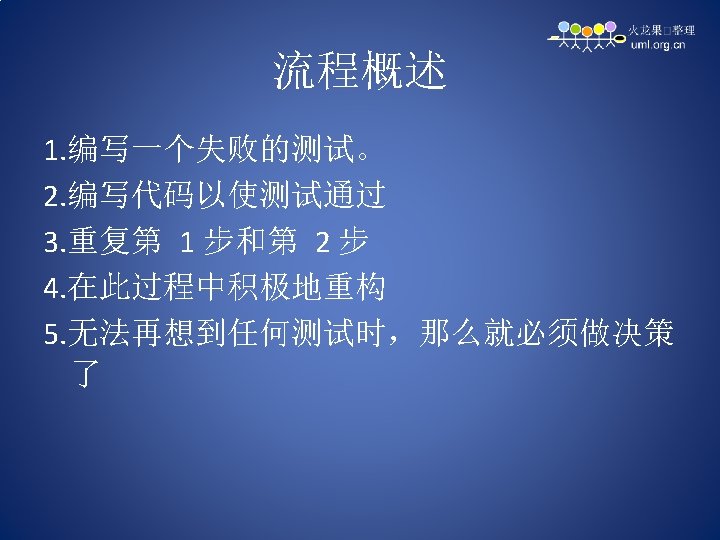
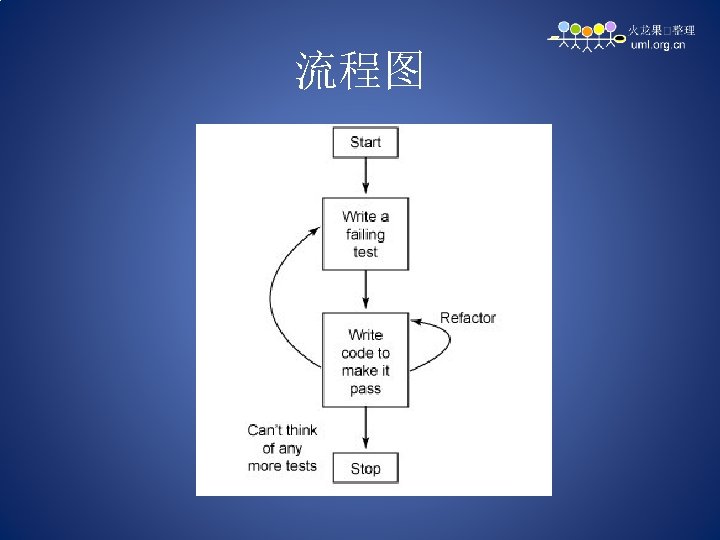
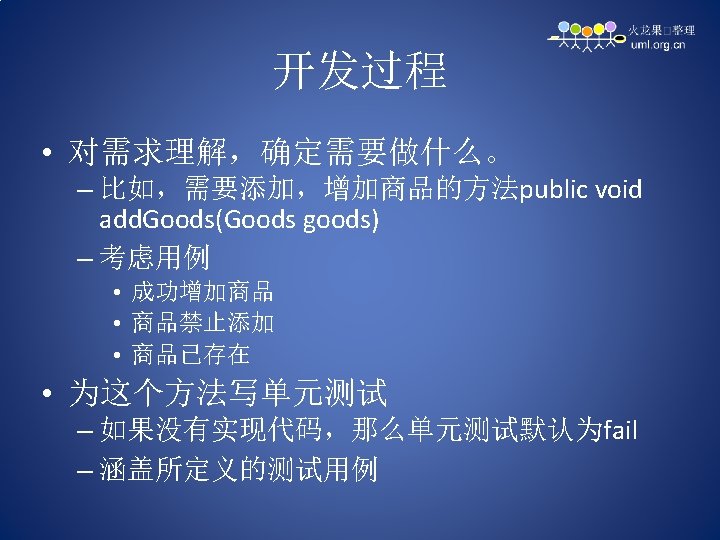
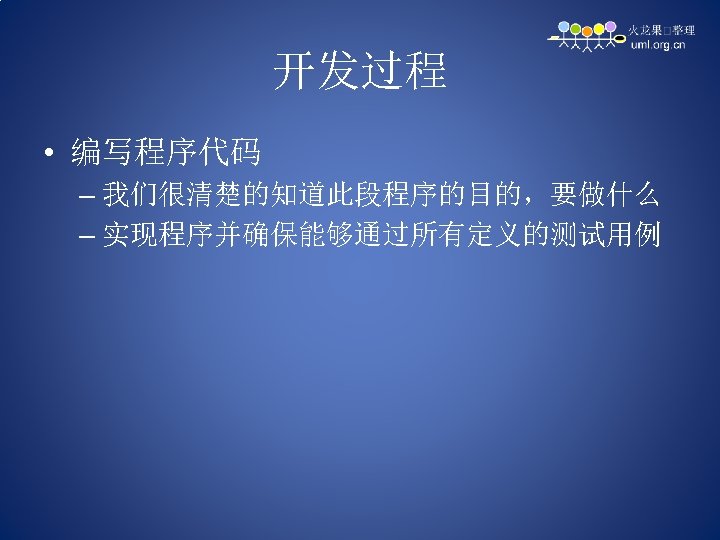
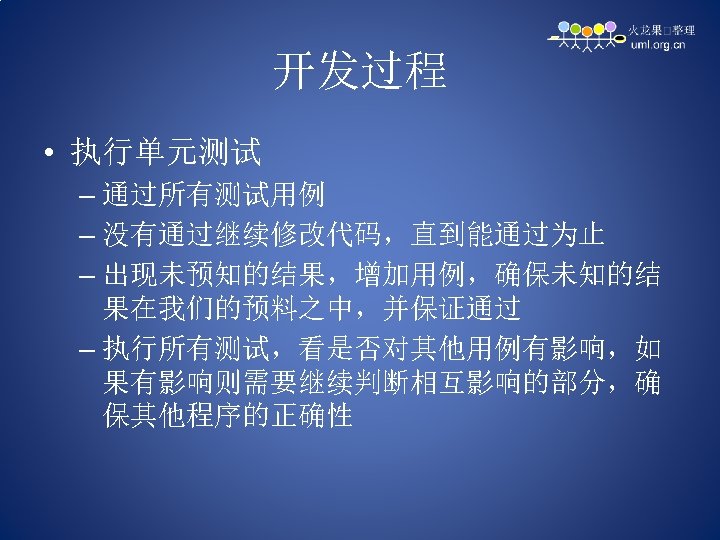
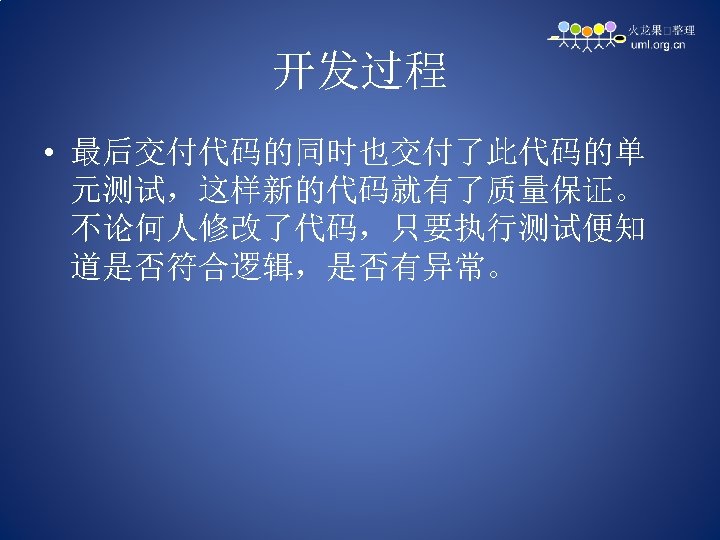
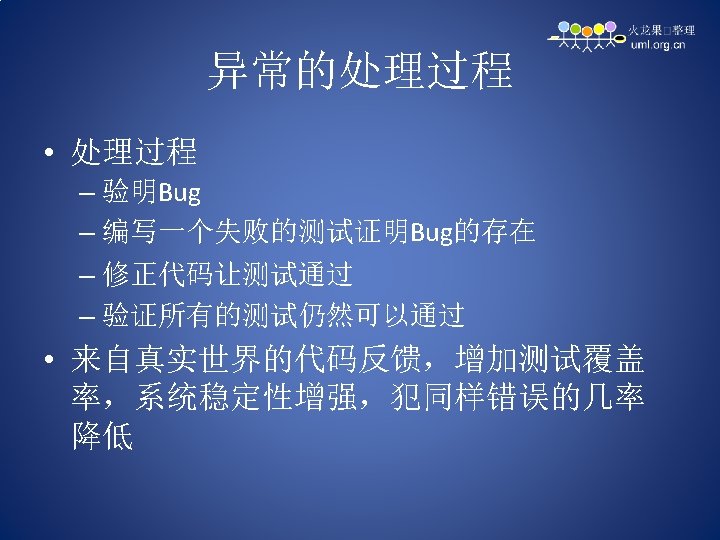
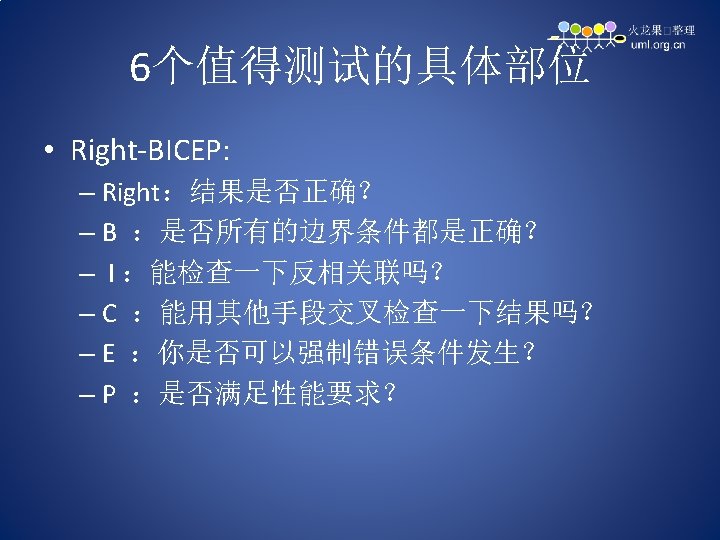
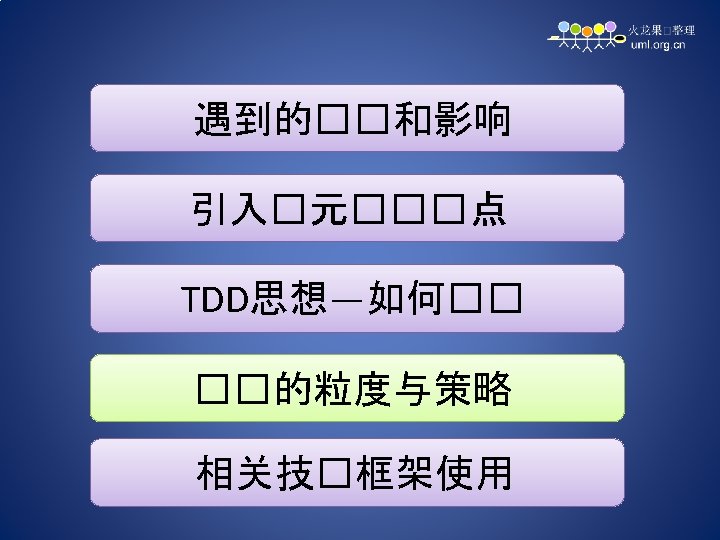
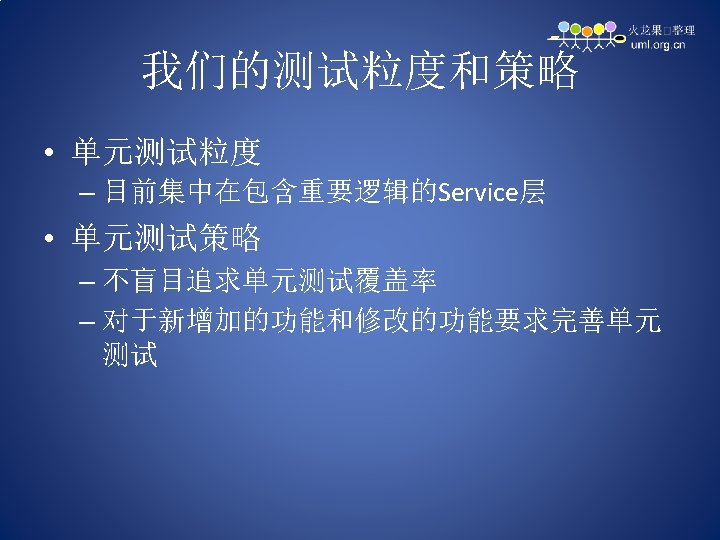
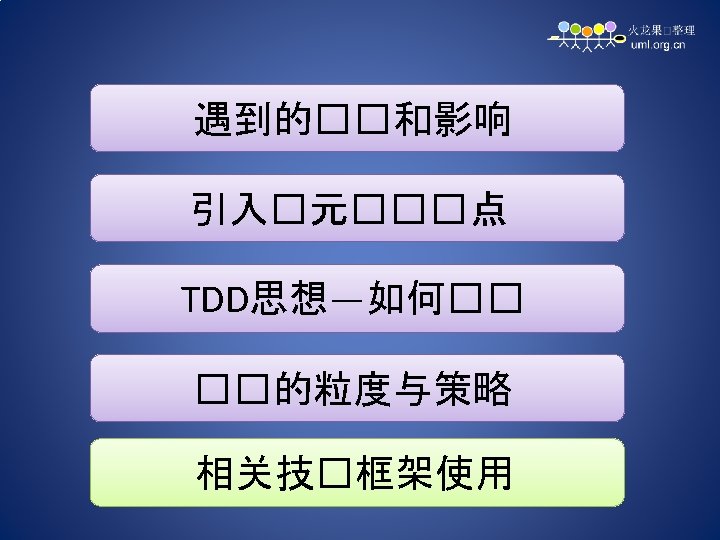
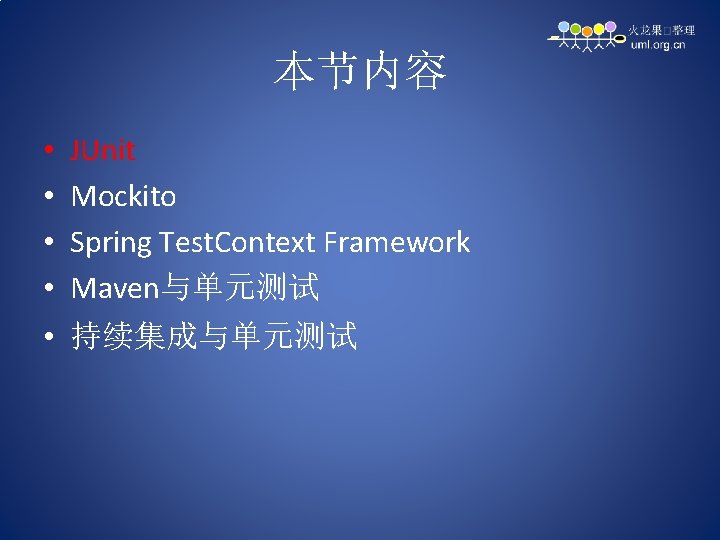
本节内容 • • JUnit Mockito Spring Test. Context Framework Maven与单元测试 • 持续集成与单元测试
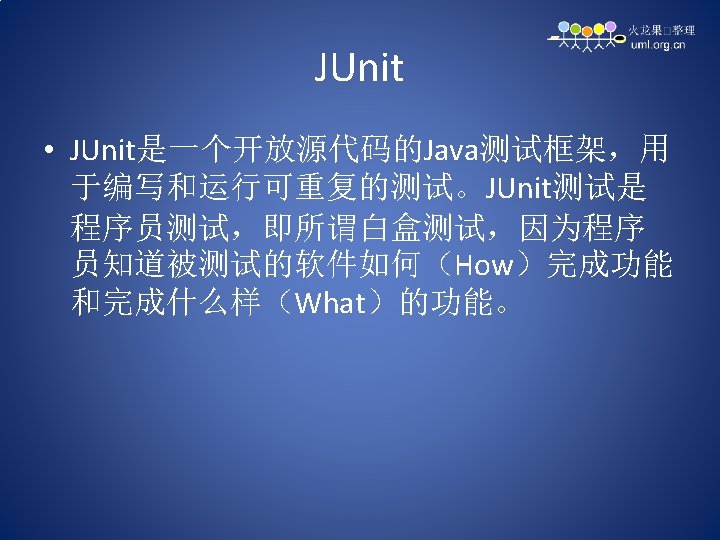
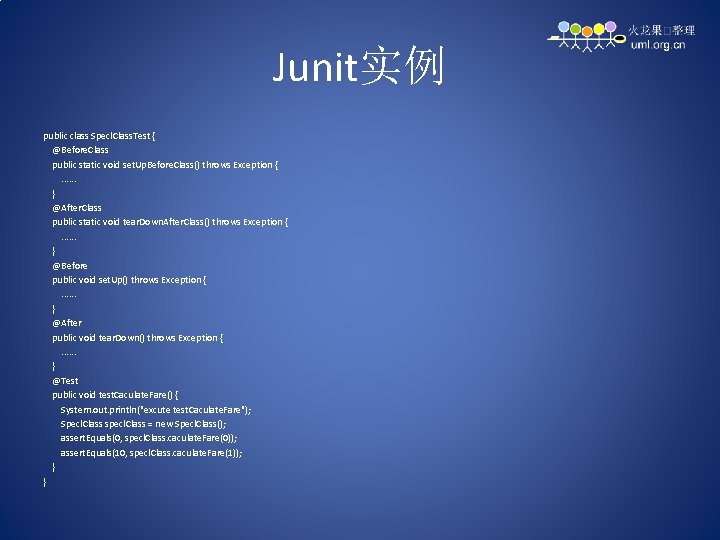
Junit实例 public class Specl. Class. Test { @Before. Class public static void set. Up. Before. Class() throws Exception {. . . } @After. Class public static void tear. Down. After. Class() throws Exception {. . . } @Before public void set. Up() throws Exception {. . . } @After public void tear. Down() throws Exception {. . . } @Test public void test. Caculate. Fare() { System. out. println("excute test. Caculate. Fare"); Specl. Class specl. Class = new Specl. Class(); assert. Equals(0, specl. Class. caculate. Fare(0)); assert. Equals(10, specl. Class. caculate. Fare(1)); } }
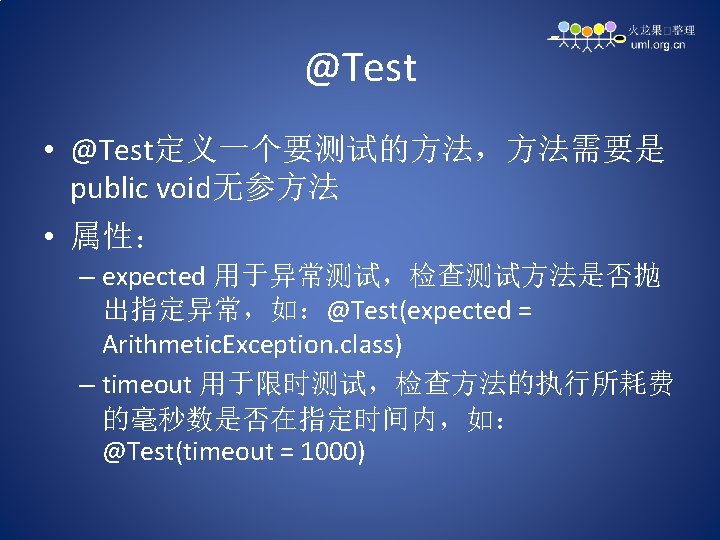
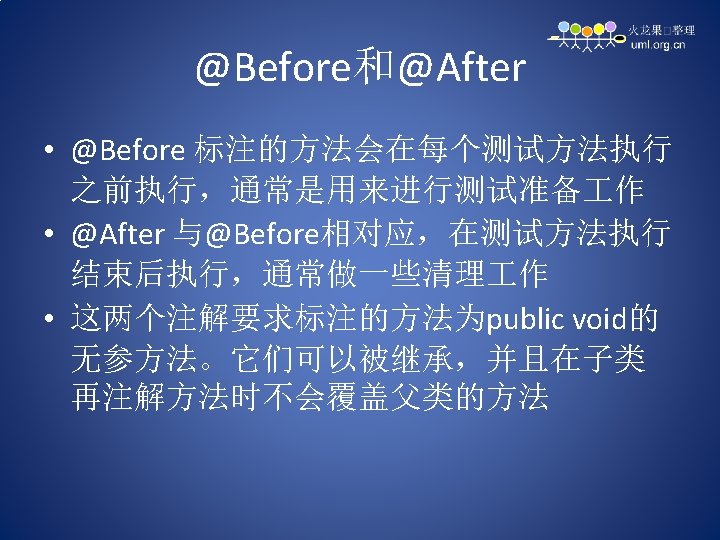
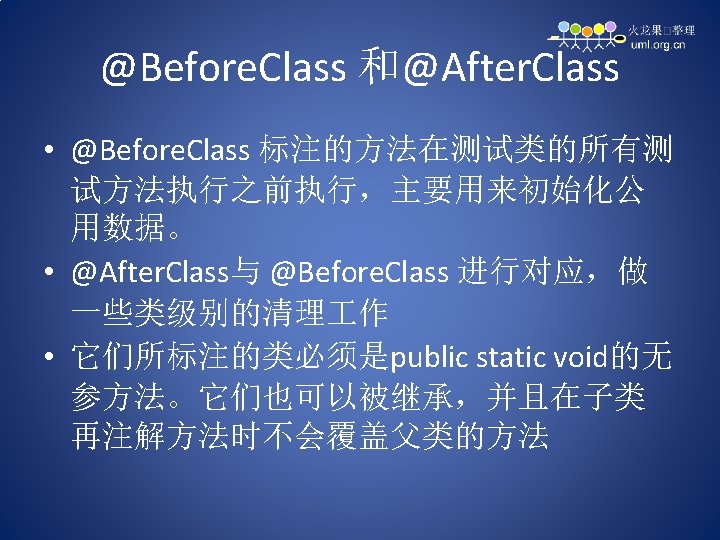
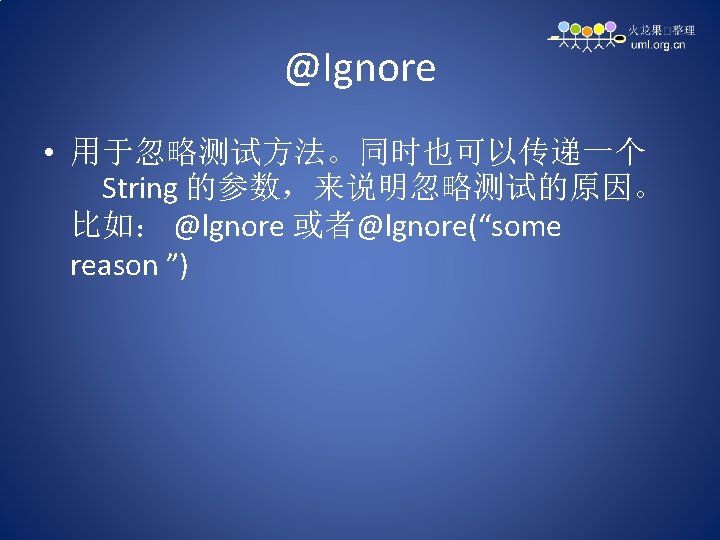
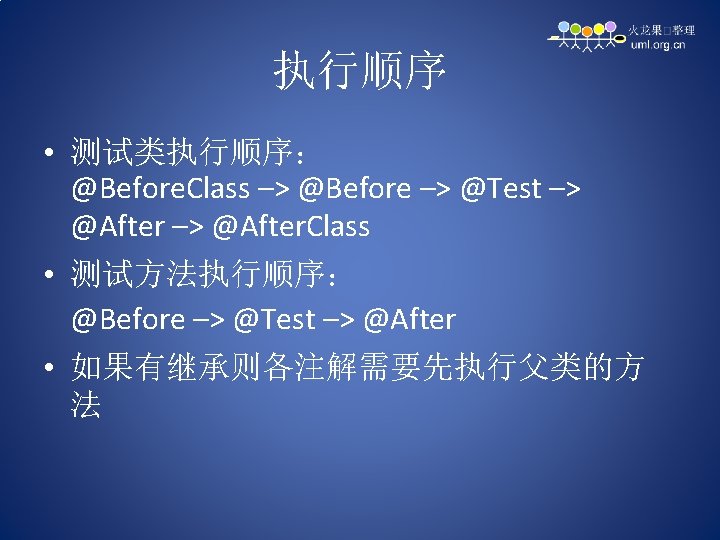
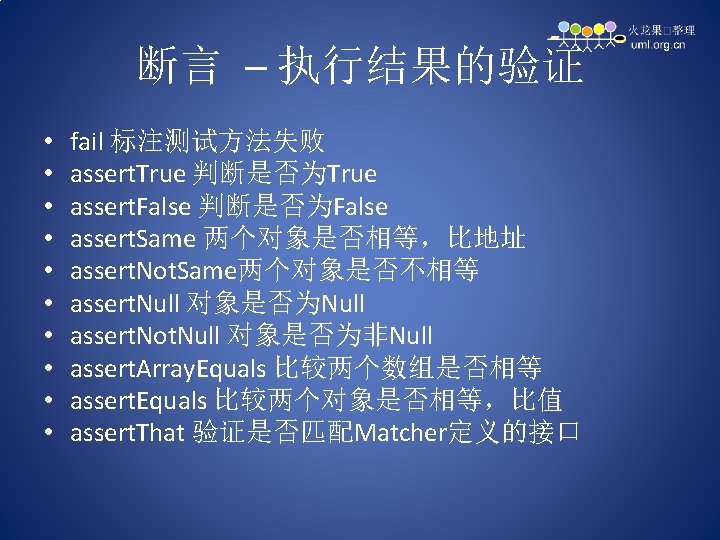
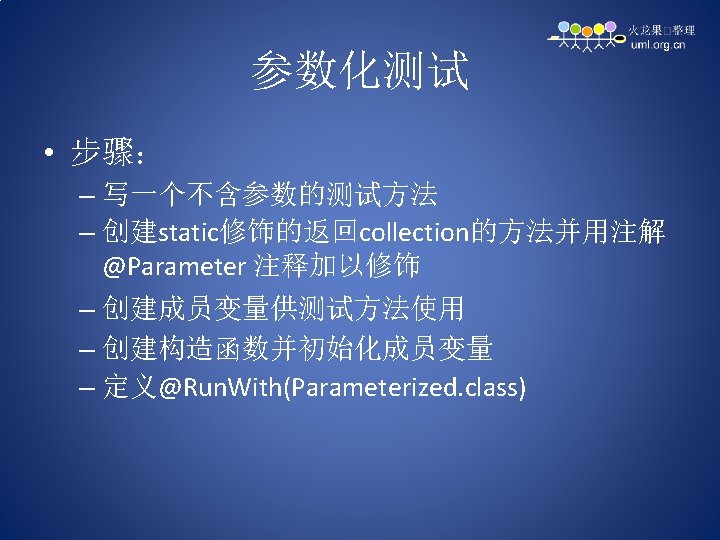
![参数化测试实例 Run WithParameterized class public class Fibonacci Test Parameters public static ListObject data 参数化测试实例 @Run. With(Parameterized. class) public class Fibonacci. Test { @Parameters public static List<Object[]> data()](https://slidetodoc.com/presentation_image_h2/84802b9ec27b46b3be9dcd68072b0711/image-38.jpg)
参数化测试实例 @Run. With(Parameterized. class) public class Fibonacci. Test { @Parameters public static List<Object[]> data() { return Arrays. as. List(new Object[][] { Fibonacci, { { 0, 0 }, { 1, 1 }, { 2, 1 }, { 3, 2 }, { 4, 3 }, { 5, 5 }, { 6, 8 } } }); } private int f. Input; private int f. Expected; public Fibonacci. Test(int input, int expected) { f. Input= input; f. Expected= expected; } @Test public void test() { assert. Equals(f. Expected, Fibonacci. compute(f. Input)); } }
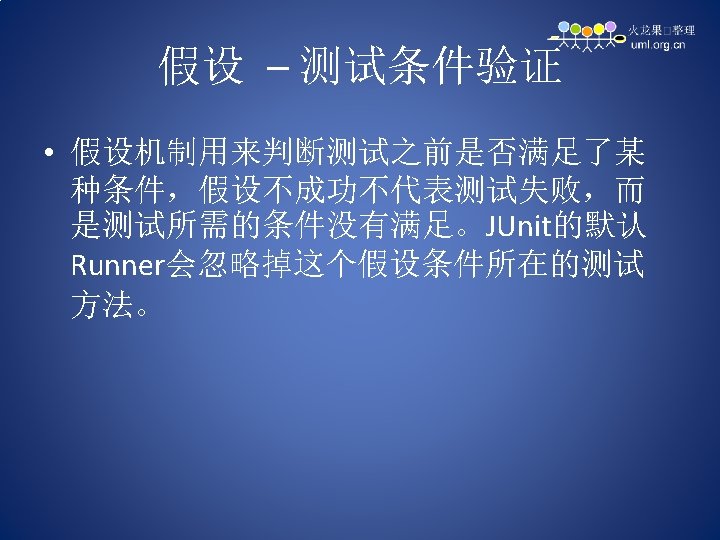
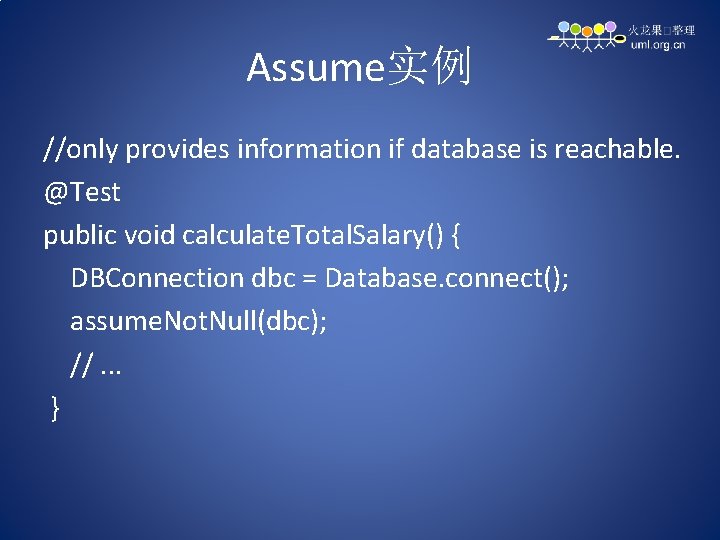
Assume实例 //only provides information if database is reachable. @Test public void calculate. Total. Salary() { DBConnection dbc = Database. connect(); assume. Not. Null(dbc); //. . . }
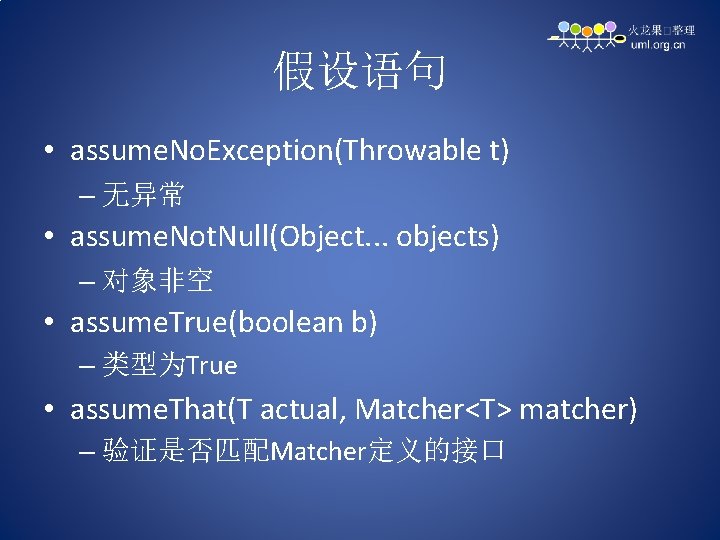
假设语句 • assume. No. Exception(Throwable t) – 无异常 • assume. Not. Null(Object. . . objects) – 对象非空 • assume. True(boolean b) – 类型为True • assume. That(T actual, Matcher<T> matcher) – 验证是否匹配Matcher定义的接口
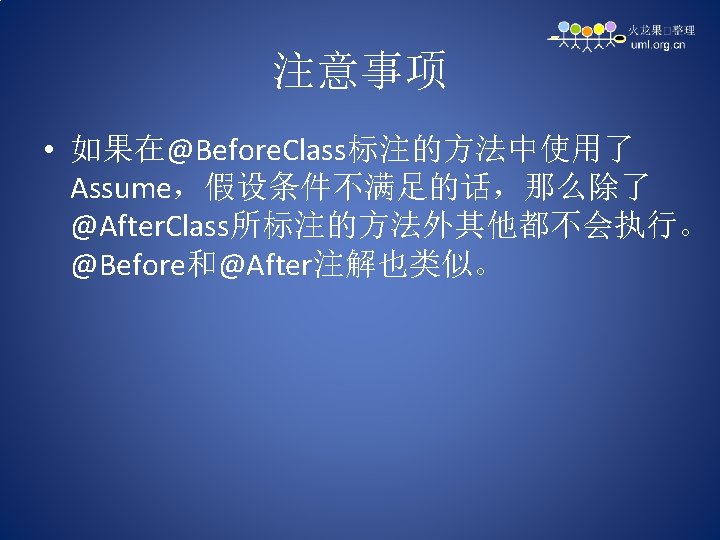
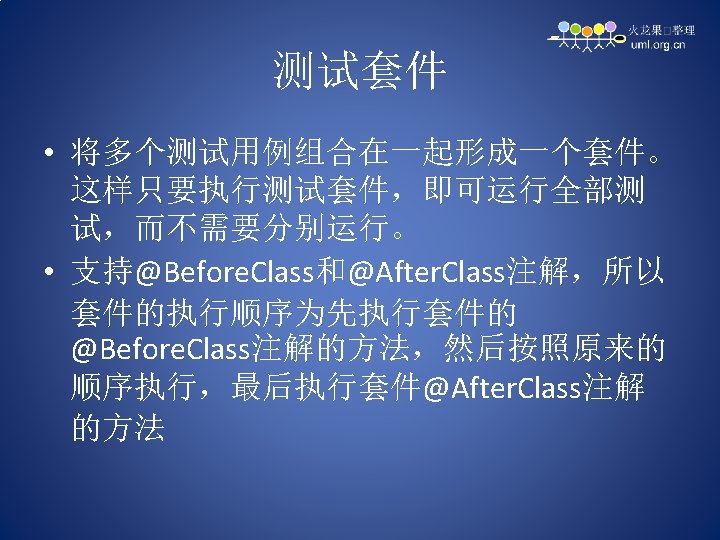
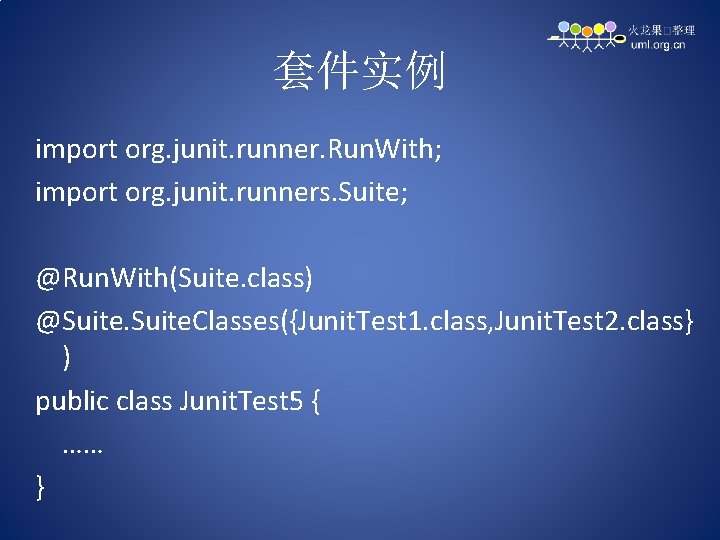
套件实例 import org. junit. runner. Run. With; import org. junit. runners. Suite; @Run. With(Suite. class) @Suite. Classes({Junit. Test 1. class, Junit. Test 2. class} ) public class Junit. Test 5 { …… }
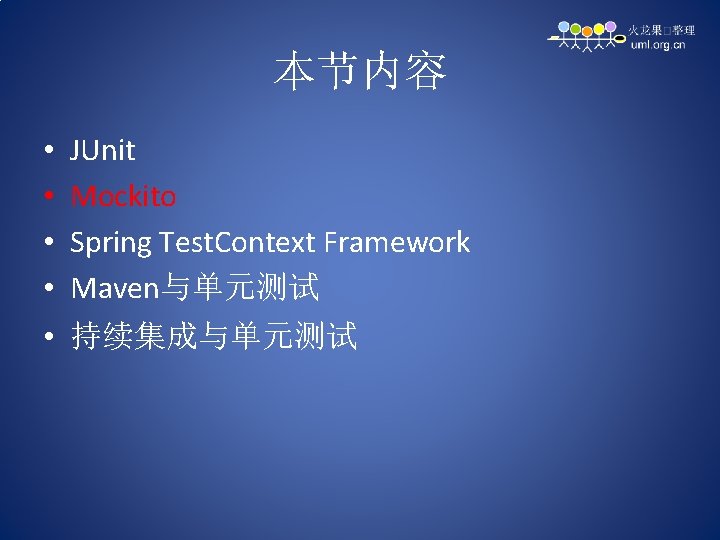
本节内容 • • JUnit Mockito Spring Test. Context Framework Maven与单元测试 • 持续集成与单元测试
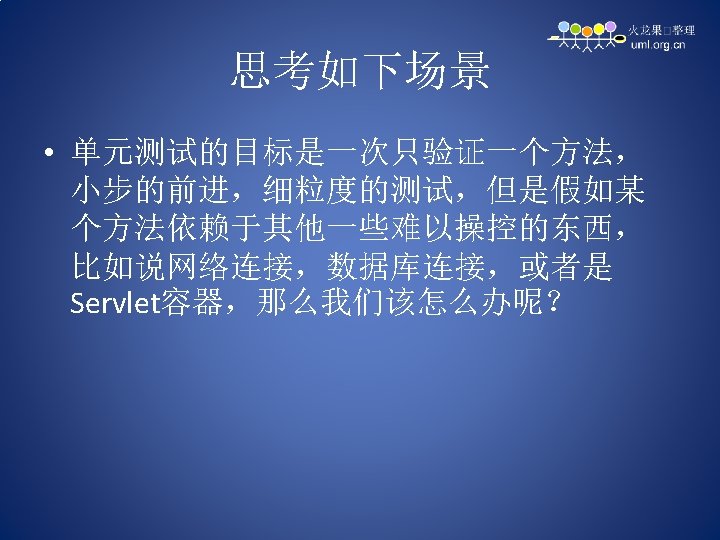
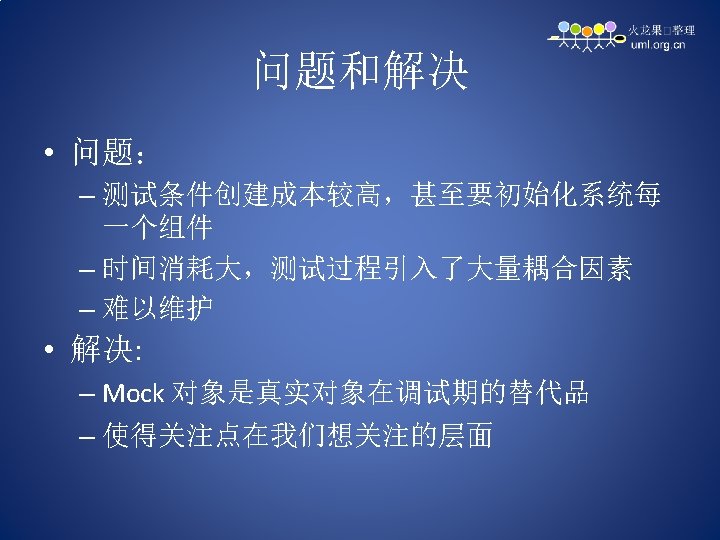
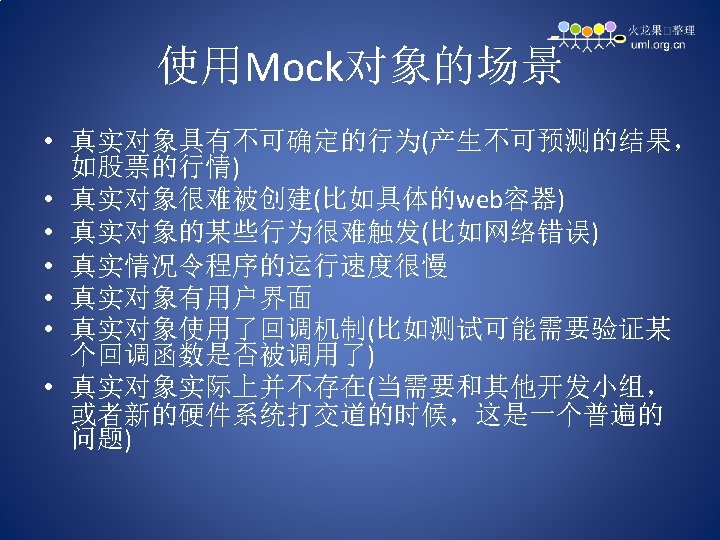
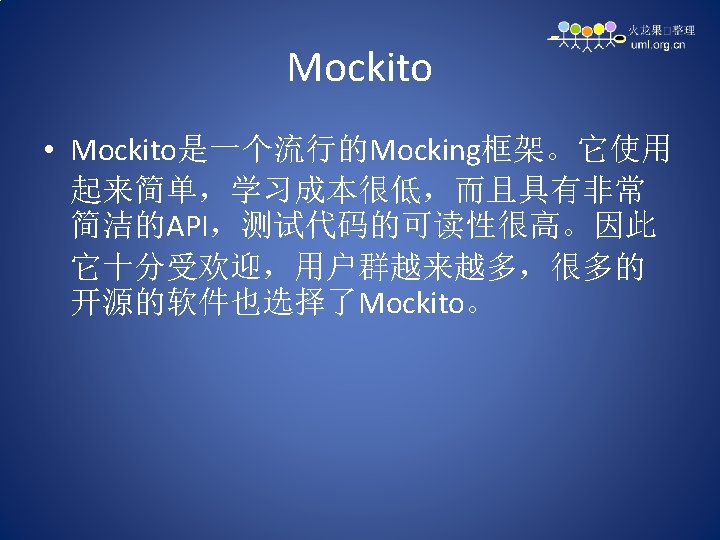
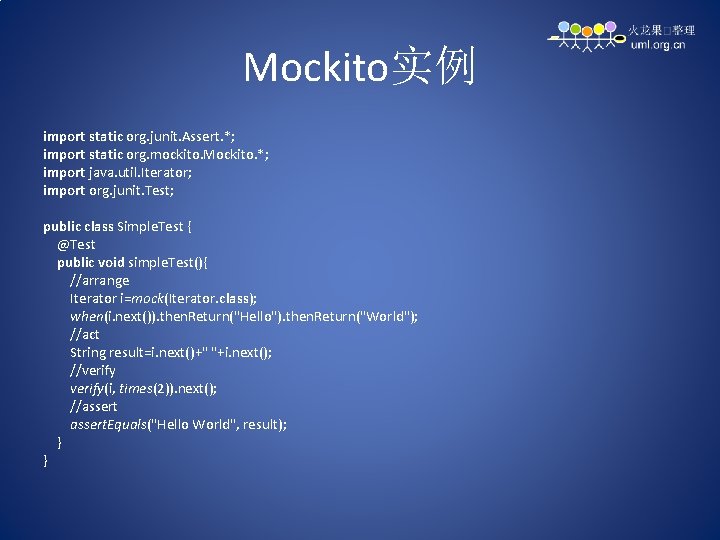
Mockito实例 import static org. junit. Assert. *; import static org. mockito. Mockito. *; import java. util. Iterator; import org. junit. Test; public class Simple. Test { @Test public void simple. Test(){ //arrange Iterator i=mock(Iterator. class); when(i. next()). then. Return("Hello"). then. Return("World"); //act String result=i. next()+" "+i. next(); //verify(i, times(2)). next(); //assert. Equals("Hello World", result); } }
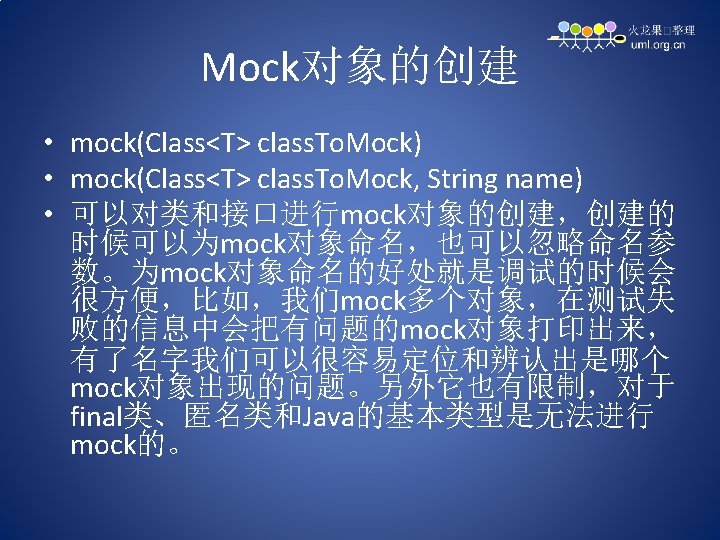
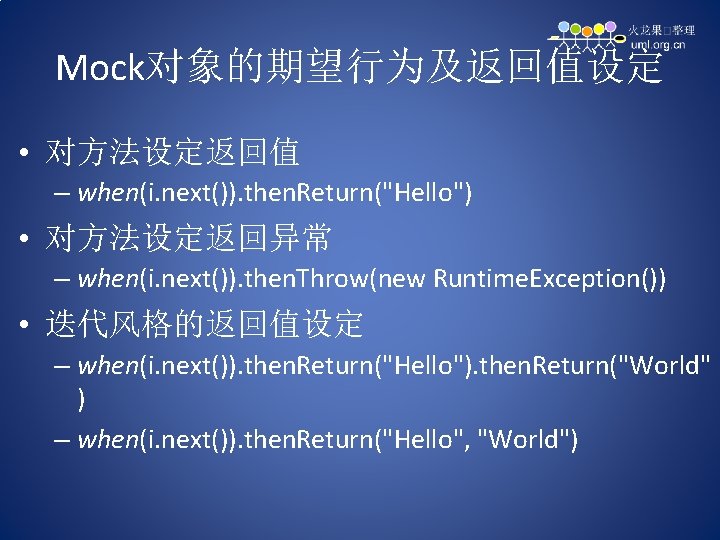
Mock对象的期望行为及返回值设定 • 对方法设定返回值 – when(i. next()). then. Return("Hello") • 对方法设定返回异常 – when(i. next()). then. Throw(new Runtime. Exception()) • 迭代风格的返回值设定 – when(i. next()). then. Return("Hello"). then. Return("World" ) – when(i. next()). then. Return("Hello", "World")
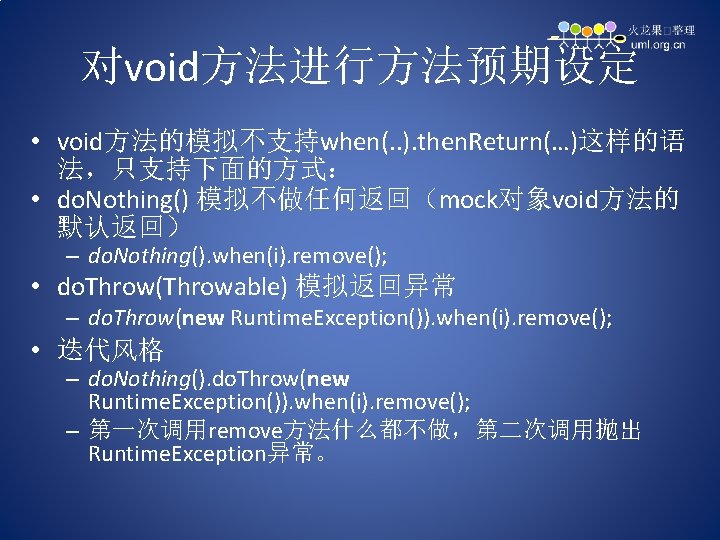
对void方法进行方法预期设定 • void方法的模拟不支持when(. . ). then. Return(…)这样的语 法,只支持下面的方式: • do. Nothing() 模拟不做任何返回(mock对象void方法的 默认返回) – do. Nothing(). when(i). remove(); • do. Throw(Throwable) 模拟返回异常 – do. Throw(new Runtime. Exception()). when(i). remove(); • 迭代风格 – do. Nothing(). do. Throw(new Runtime. Exception()). when(i). remove(); – 第一次调用remove方法什么都不做,第二次调用抛出 Runtime. Exception异常。
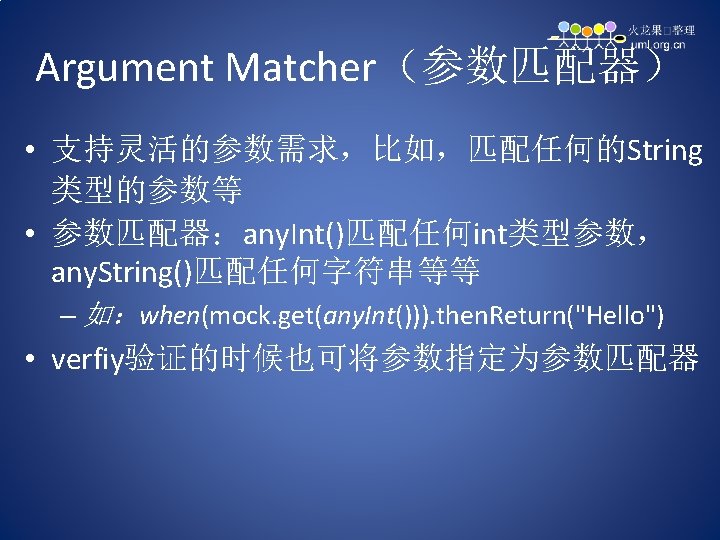
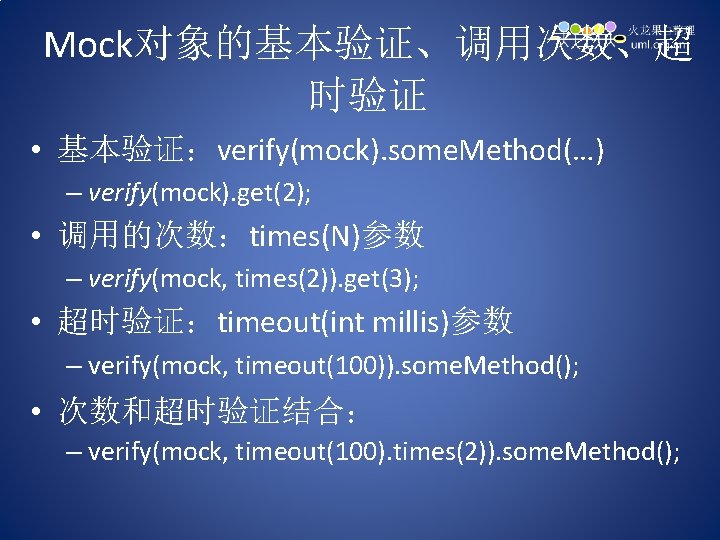
Mock对象的基本验证、调用次数、超 时验证 • 基本验证:verify(mock). some. Method(…) – verify(mock). get(2); • 调用的次数:times(N)参数 – verify(mock, times(2)). get(3); • 超时验证:timeout(int millis)参数 – verify(mock, timeout(100)). some. Method(); • 次数和超时验证结合: – verify(mock, timeout(100). times(2)). some. Method();
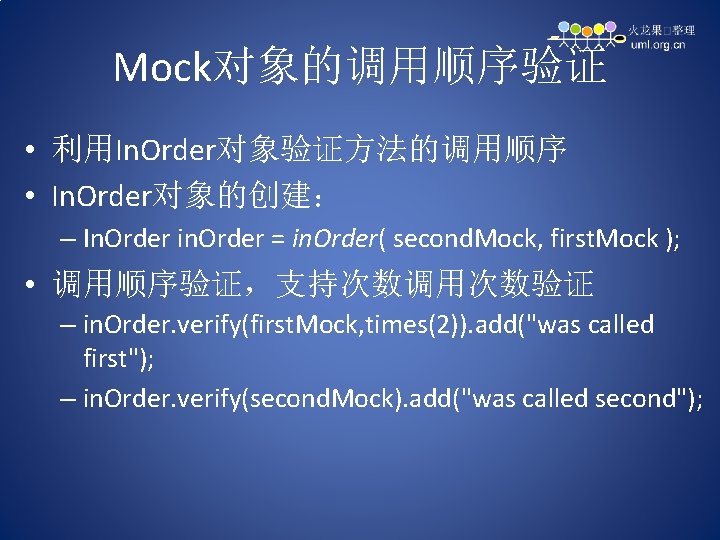
Mock对象的调用顺序验证 • 利用In. Order对象验证方法的调用顺序 • In. Order对象的创建: – In. Order in. Order = in. Order( second. Mock, first. Mock ); • 调用顺序验证,支持次数调用次数验证 – in. Order. verify(first. Mock, times(2)). add("was called first"); – in. Order. verify(second. Mock). add("was called second");
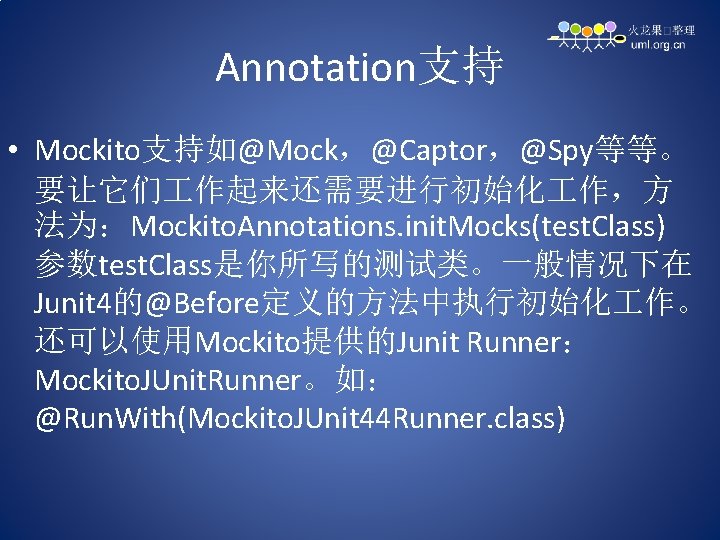
Annotation支持 • Mockito支持如@Mock,@Captor,@Spy等等。 要让它们 作起来还需要进行初始化 作,方 法为:Mockito. Annotations. init. Mocks(test. Class) 参数test. Class是你所写的测试类。一般情况下在 Junit 4的@Before定义的方法中执行初始化 作。 还可以使用Mockito提供的Junit Runner: Mockito. JUnit. Runner。如: @Run. With(Mockito. JUnit 44 Runner. class)
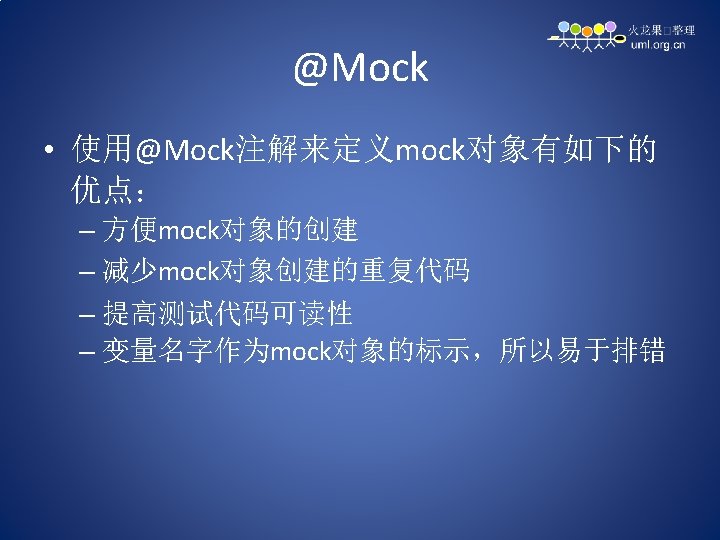
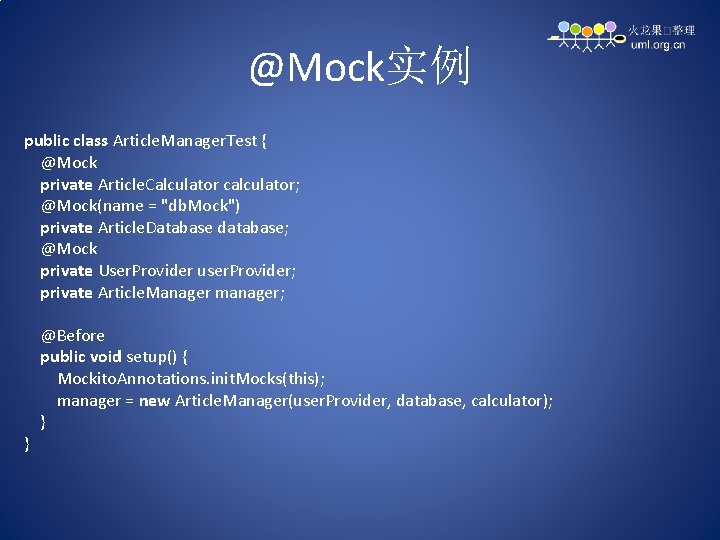
@Mock实例 public class Article. Manager. Test { @Mock private Article. Calculator calculator; @Mock(name = "db. Mock") private Article. Database database; @Mock private User. Provider user. Provider; private Article. Manager manager; } @Before public void setup() { Mockito. Annotations. init. Mocks(this); manager = new Article. Manager(user. Provider, database, calculator); }
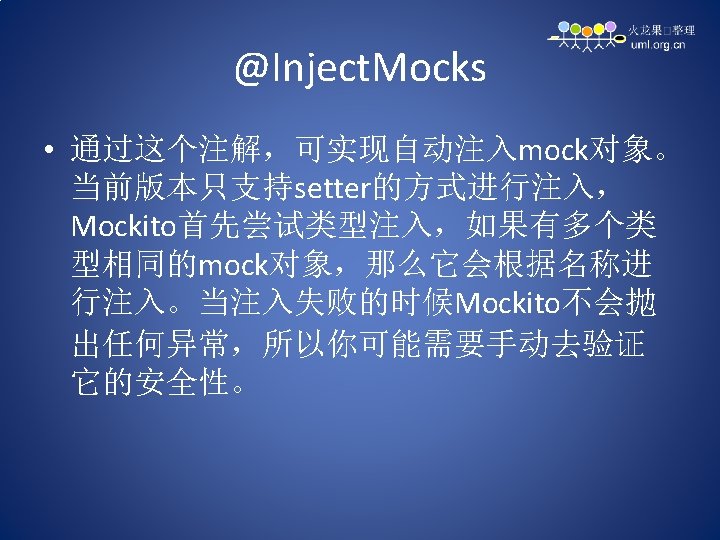
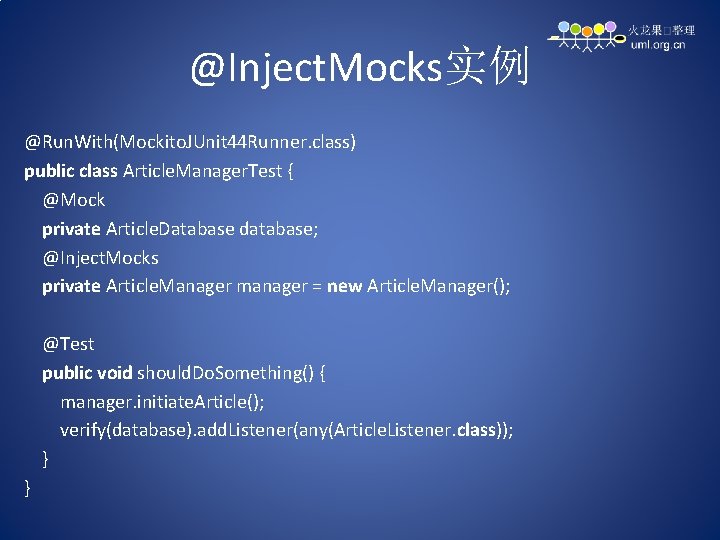
@Inject. Mocks实例 @Run. With(Mockito. JUnit 44 Runner. class) public class Article. Manager. Test { @Mock private Article. Database database; @Inject. Mocks private Article. Manager manager = new Article. Manager(); @Test public void should. Do. Something() { manager. initiate. Article(); verify(database). add. Listener(any(Article. Listener. class)); } }
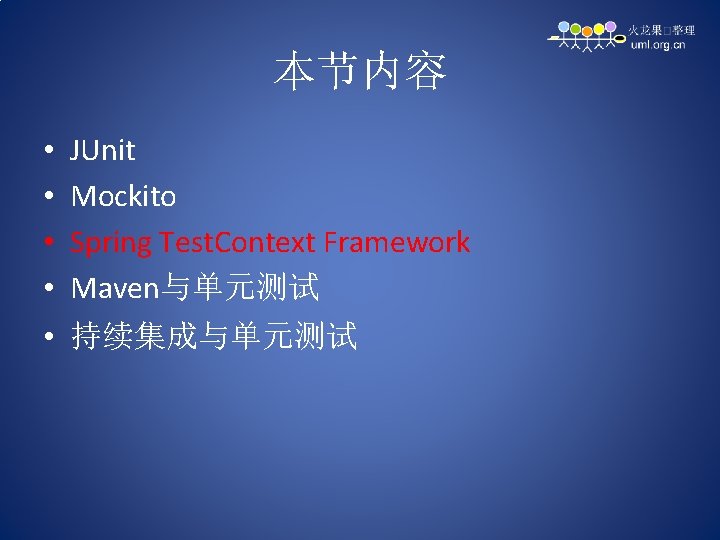
本节内容 • • JUnit Mockito Spring Test. Context Framework Maven与单元测试 • 持续集成与单元测试
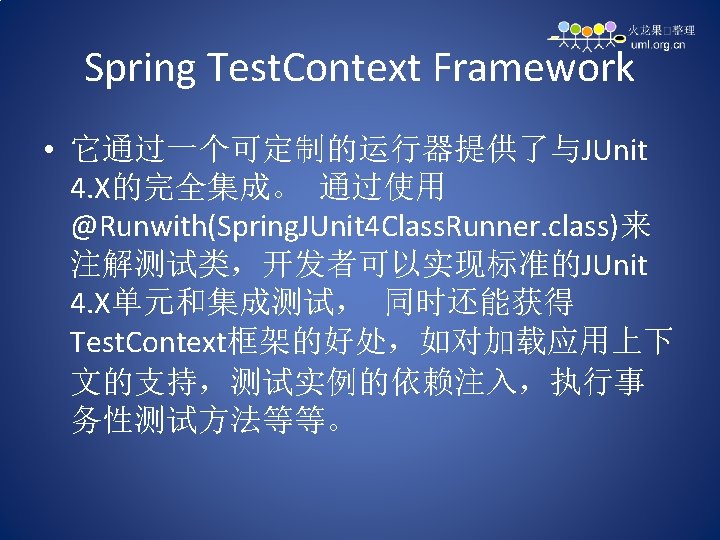
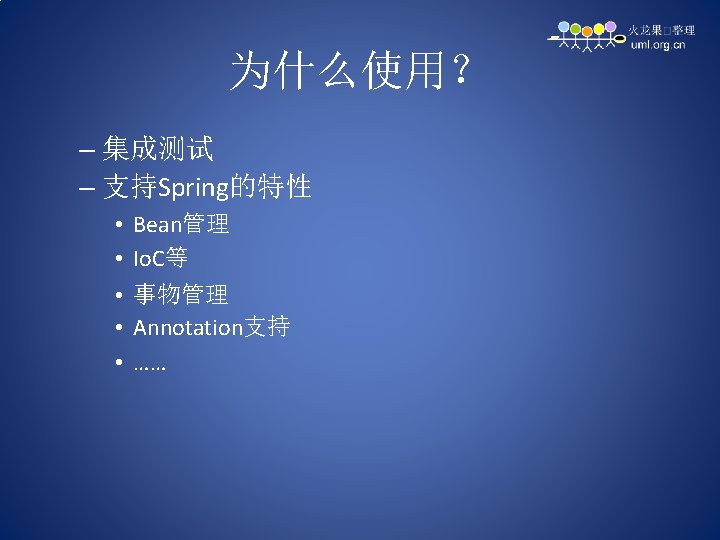
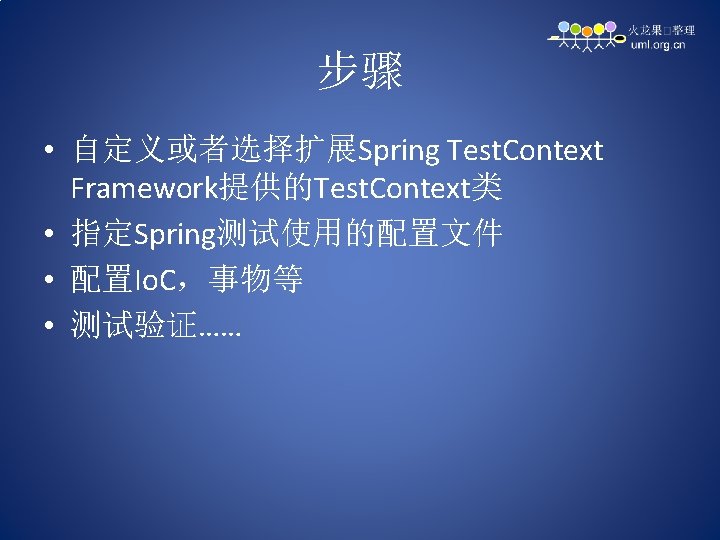
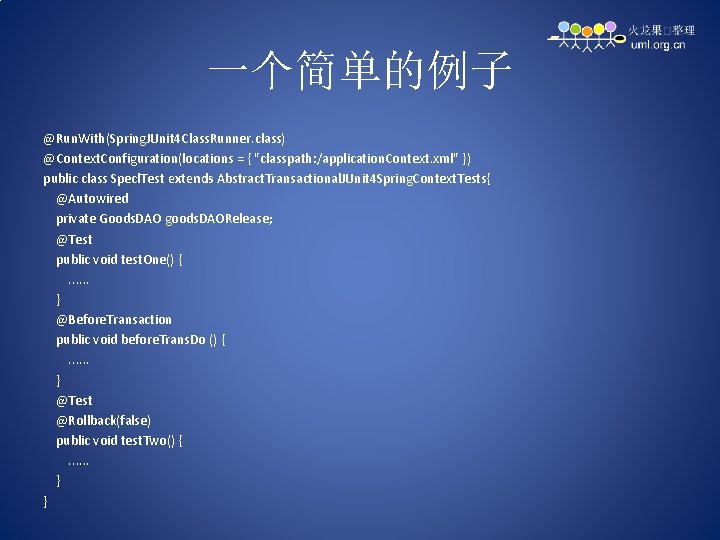
一个简单的例子 @Run. With(Spring. JUnit 4 Class. Runner. class) @Context. Configuration(locations = { "classpath: /application. Context. xml" }) public class Specl. Test extends Abstract. Transactional. JUnit 4 Spring. Context. Tests{ @Autowired private Goods. DAO goods. DAORelease; @Test public void test. One() {. . . } @Before. Transaction public void before. Trans. Do () {. . . } @Test @Rollback(false) public void test. Two() {. . . } }
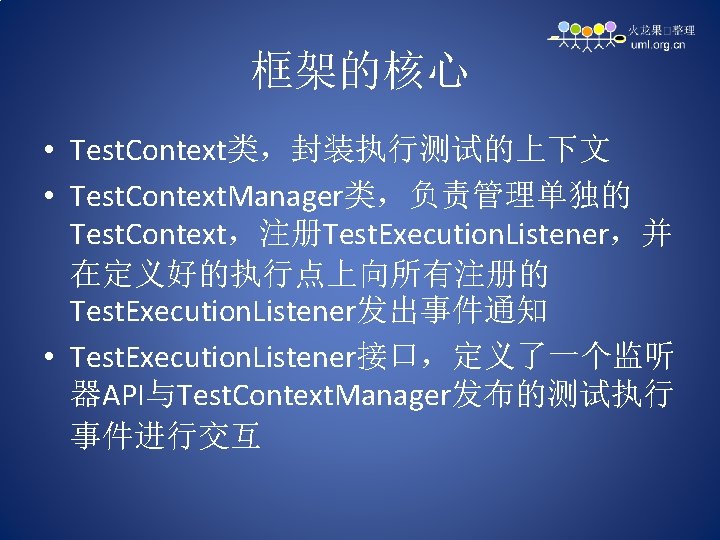
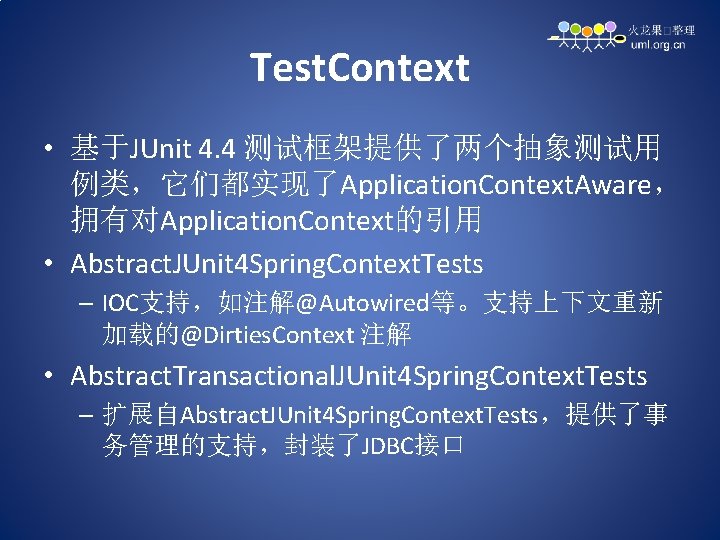
Test. Context • 基于JUnit 4. 4 测试框架提供了两个抽象测试用 例类,它们都实现了Application. Context. Aware, 拥有对Application. Context的引用 • Abstract. JUnit 4 Spring. Context. Tests – IOC支持,如注解@Autowired等。支持上下文重新 加载的@Dirties. Context 注解 • Abstract. Transactional. JUnit 4 Spring. Context. Tests – 扩展自Abstract. JUnit 4 Spring. Context. Tests,提供了事 务管理的支持,封装了JDBC接口
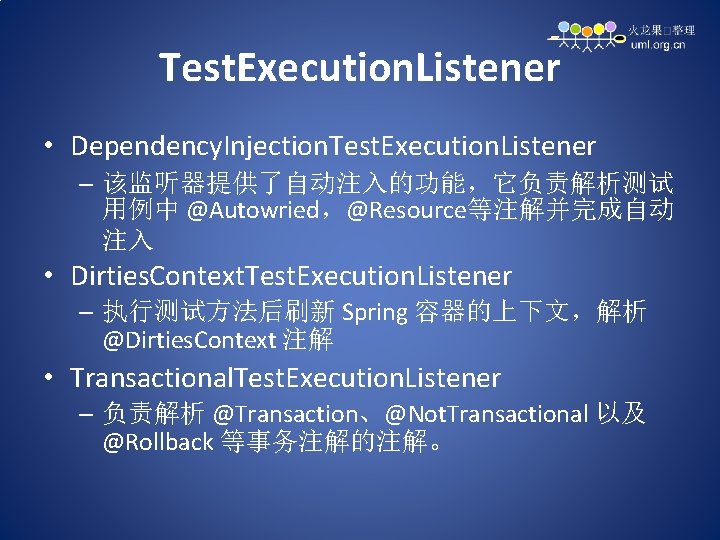
Test. Execution. Listener • Dependency. Injection. Test. Execution. Listener – 该监听器提供了自动注入的功能,它负责解析测试 用例中 @Autowried,@Resource等注解并完成自动 注入 • Dirties. Context. Test. Execution. Listener – 执行测试方法后刷新 Spring 容器的上下文,解析 @Dirties. Context 注解 • Transactional. Test. Execution. Listener – 负责解析 @Transaction、@Not. Transactional 以及 @Rollback 等事务注解的注解。
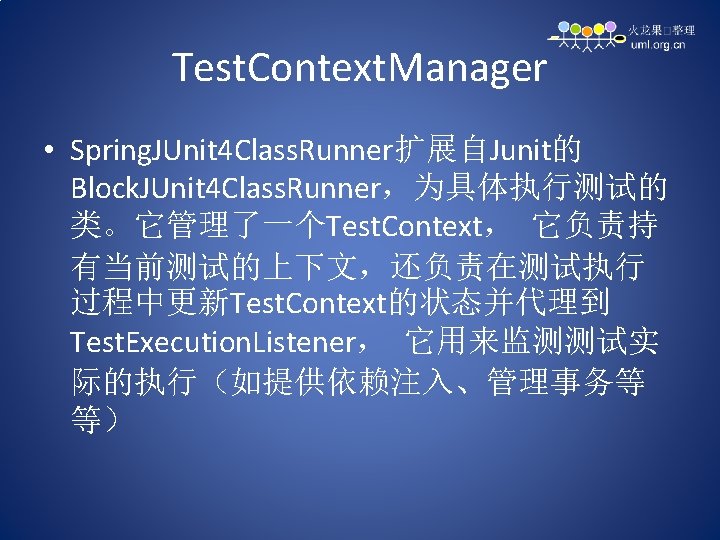
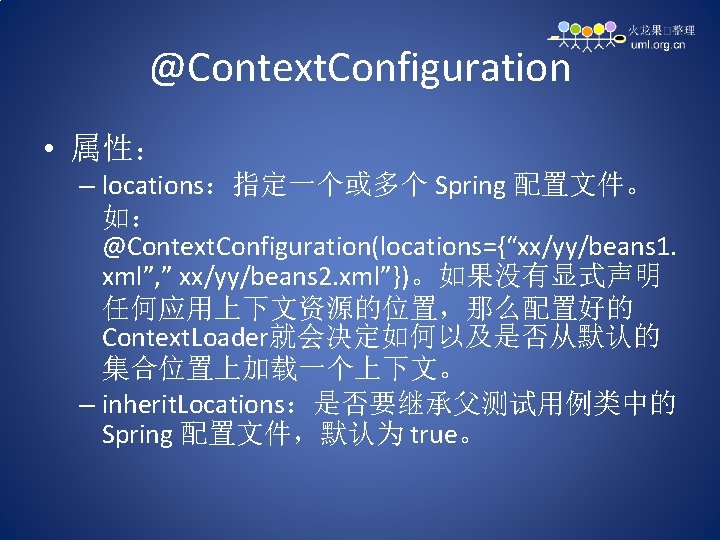
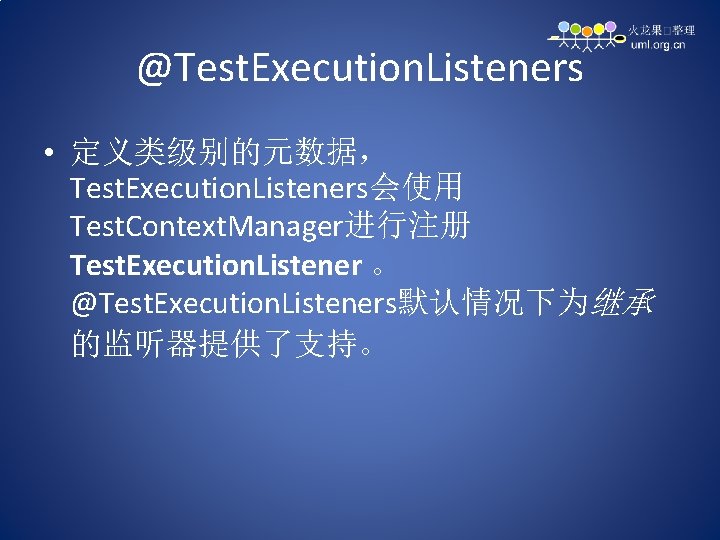
@Test. Execution. Listeners • 定义类级别的元数据, Test. Execution. Listeners会使用 Test. Context. Manager进行注册 Test. Execution. Listener 。 @Test. Execution. Listeners默认情况下为继承 的监听器提供了支持。
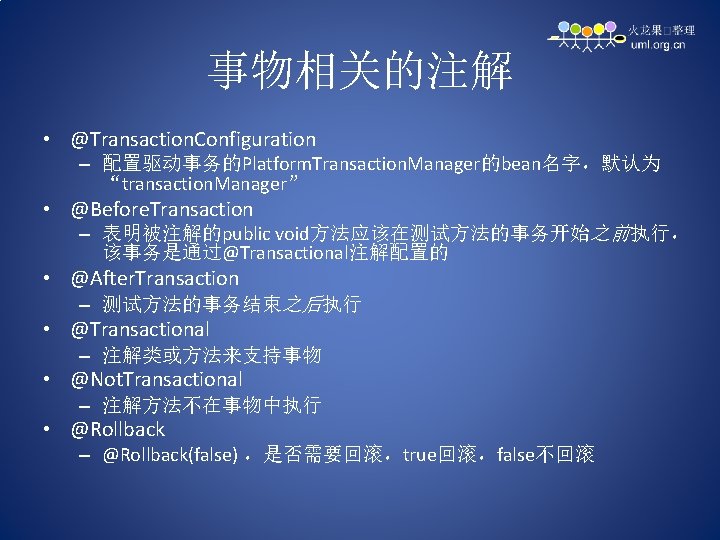
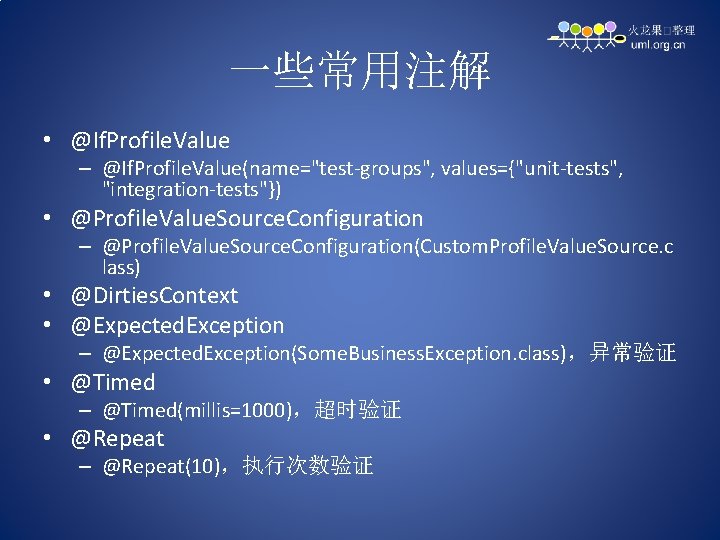
一些常用注解 • @If. Profile. Value – @If. Profile. Value(name="test-groups", values={"unit-tests", "integration-tests"}) • @Profile. Value. Source. Configuration – @Profile. Value. Source. Configuration(Custom. Profile. Value. Source. c lass) • @Dirties. Context • @Expected. Exception – @Expected. Exception(Some. Business. Exception. class),异常验证 • @Timed – @Timed(millis=1000),超时验证 • @Repeat – @Repeat(10),执行次数验证
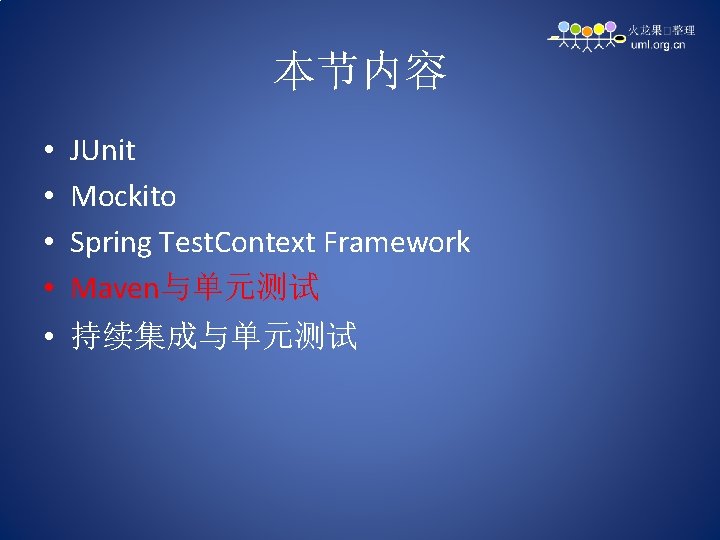
本节内容 • • JUnit Mockito Spring Test. Context Framework Maven与单元测试 • 持续集成与单元测试
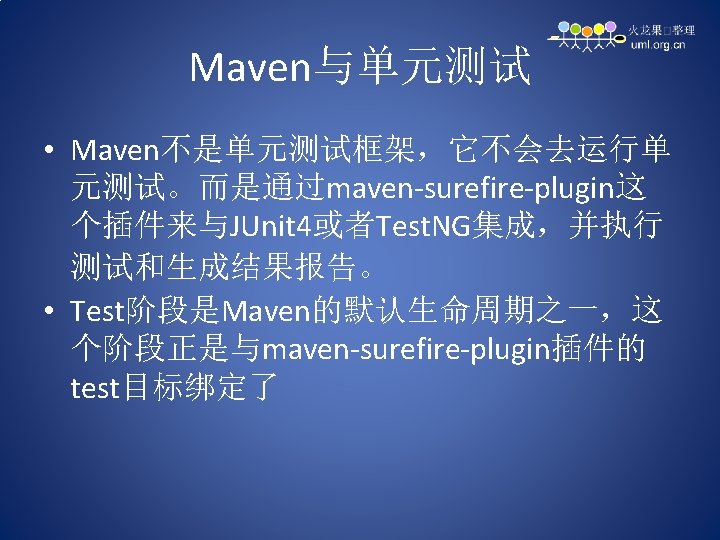
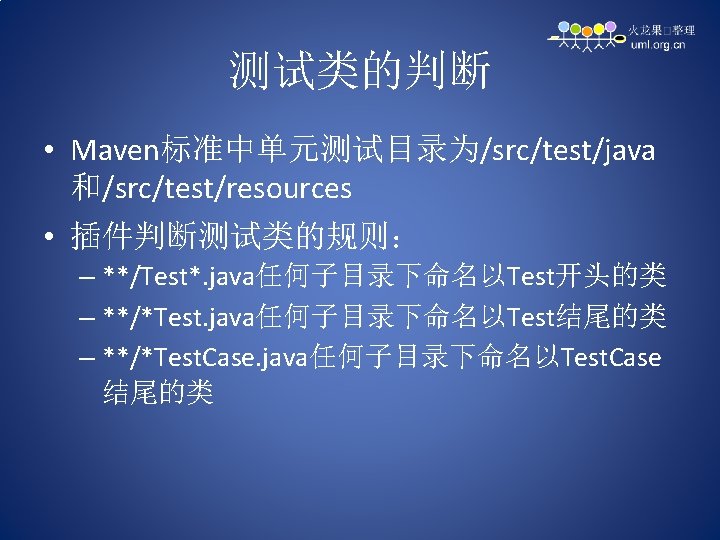
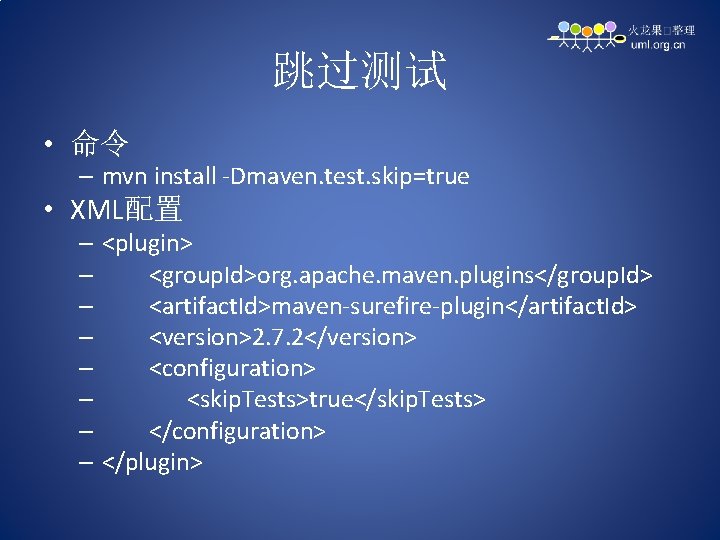
跳过测试 • 命令 – mvn install -Dmaven. test. skip=true • XML配置 – <plugin> – <group. Id>org. apache. maven. plugins</group. Id> – <artifact. Id>maven-surefire-plugin</artifact. Id> – <version>2. 7. 2</version> – <configuration> – <skip. Tests>true</skip. Tests> – </configuration> – </plugin>
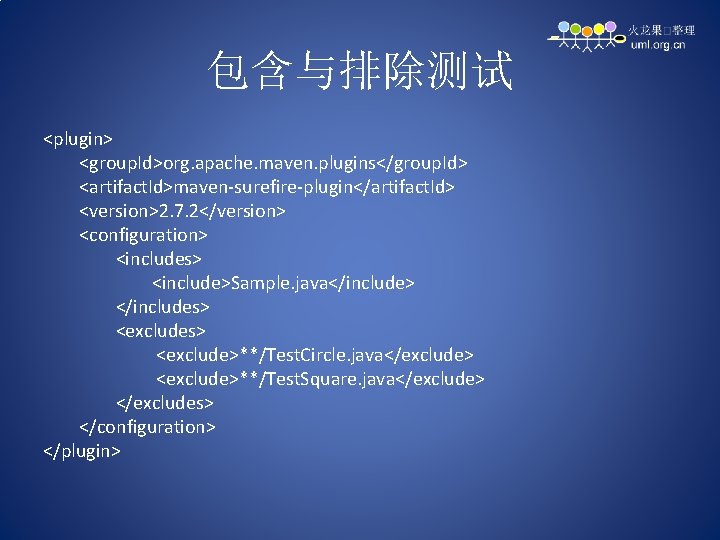
包含与排除测试 <plugin> <group. Id>org. apache. maven. plugins</group. Id> <artifact. Id>maven-surefire-plugin</artifact. Id> <version>2. 7. 2</version> <configuration> <includes> <include>Sample. java</include> </includes> <exclude>**/Test. Circle. java</exclude> <exclude>**/Test. Square. java</exclude> </excludes> </configuration> </plugin>
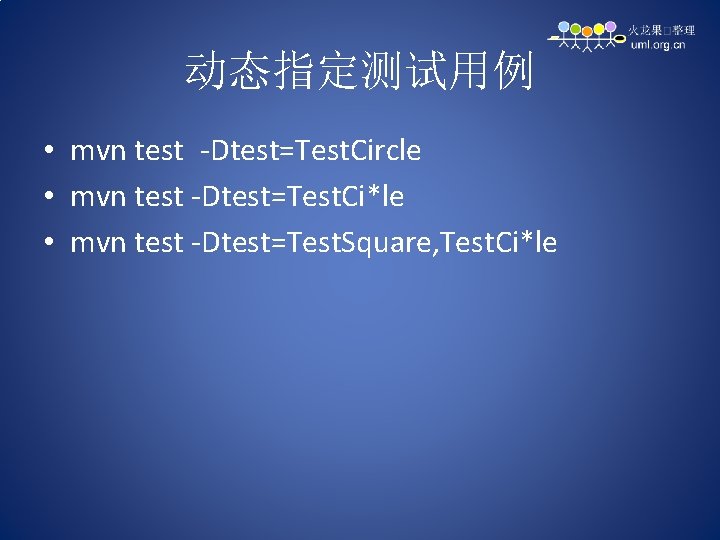
动态指定测试用例 • mvn test -Dtest=Test. Circle • mvn test -Dtest=Test. Ci*le • mvn test -Dtest=Test. Square, Test. Ci*le
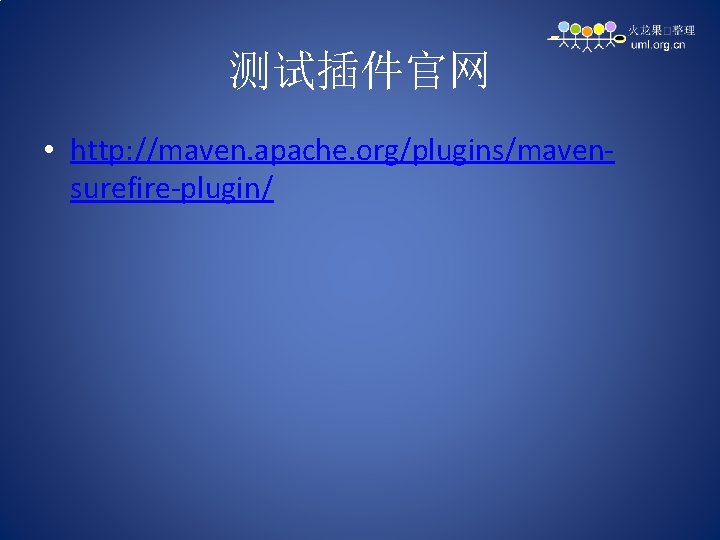
测试插件官网 • http: //maven. apache. org/plugins/mavensurefire-plugin/
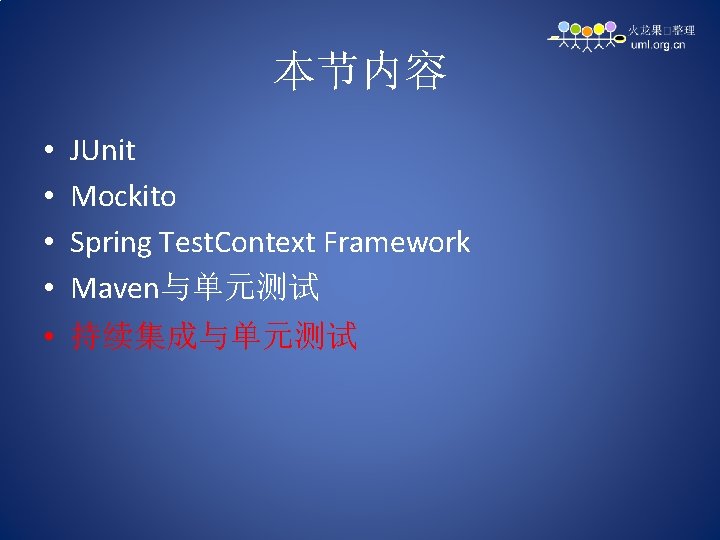
本节内容 • • JUnit Mockito Spring Test. Context Framework Maven与单元测试 • 持续集成与单元测试
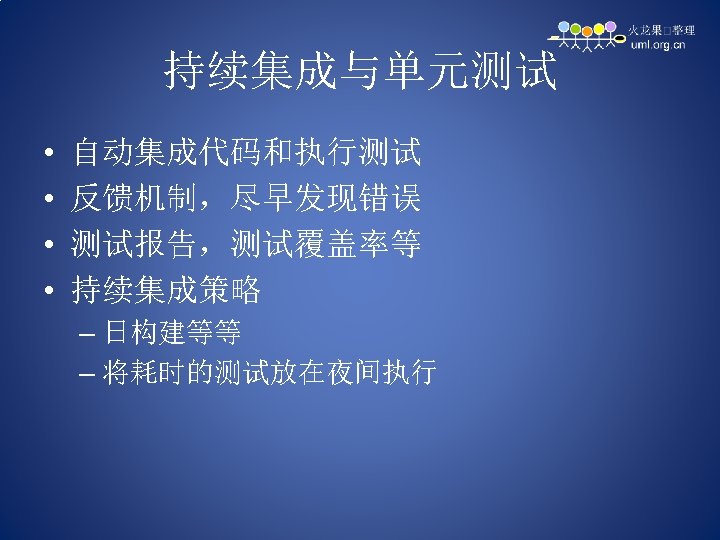
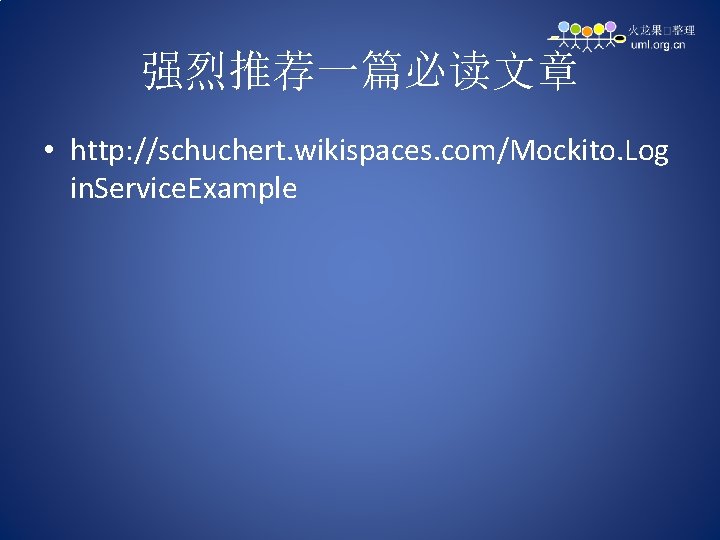
强烈推荐一篇必读文章 • http: //schuchert. wikispaces. com/Mockito. Log in. Service. Example
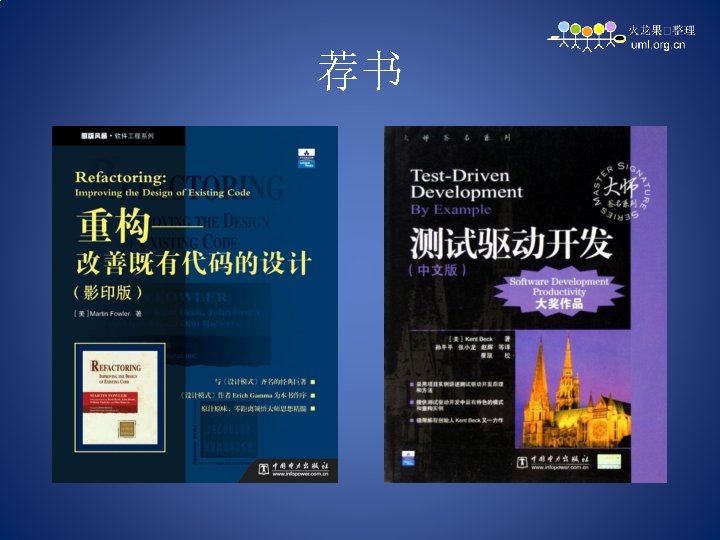
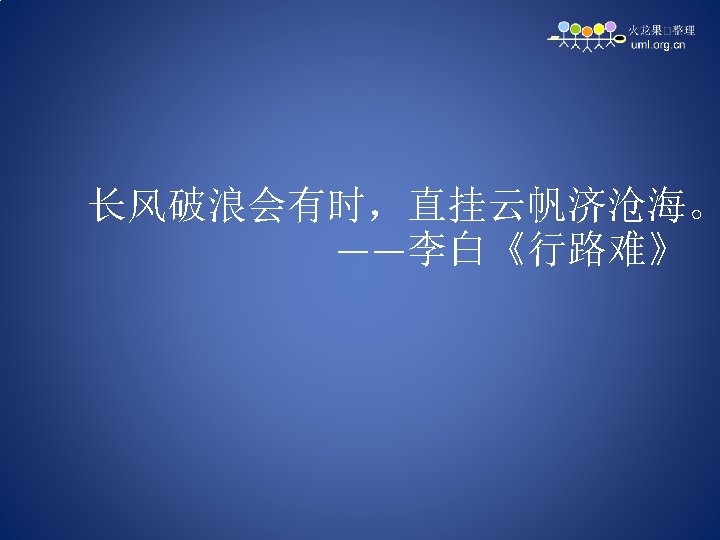
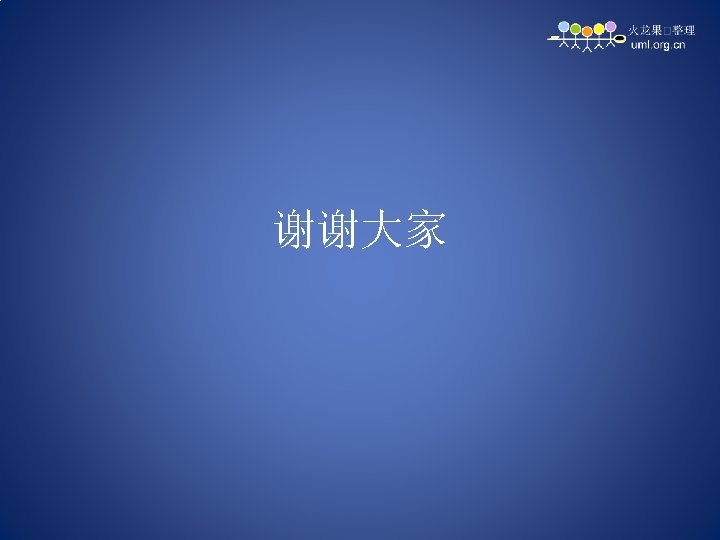