JDOM Notes from Rusty Harolds Processing XML with
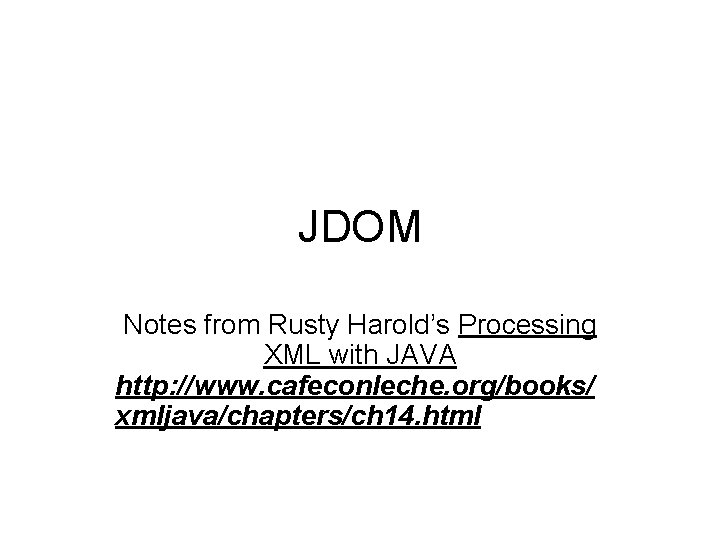
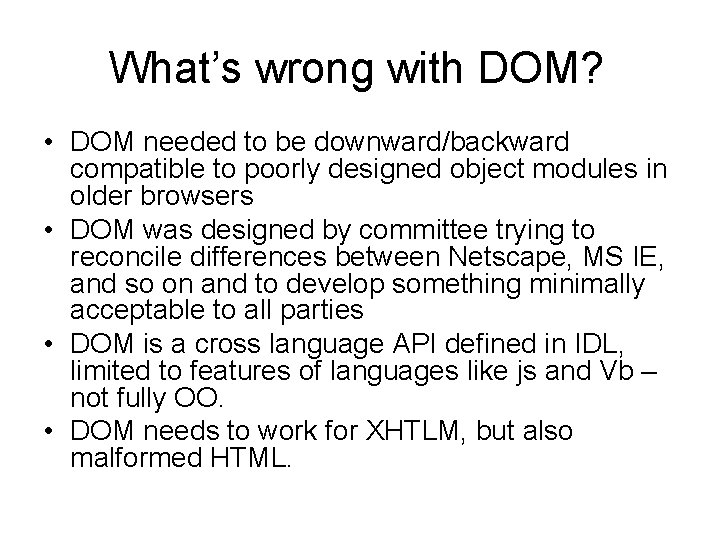
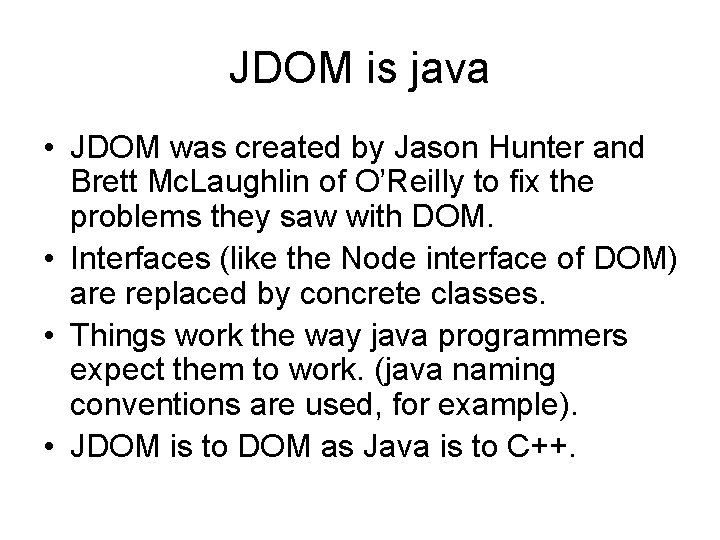
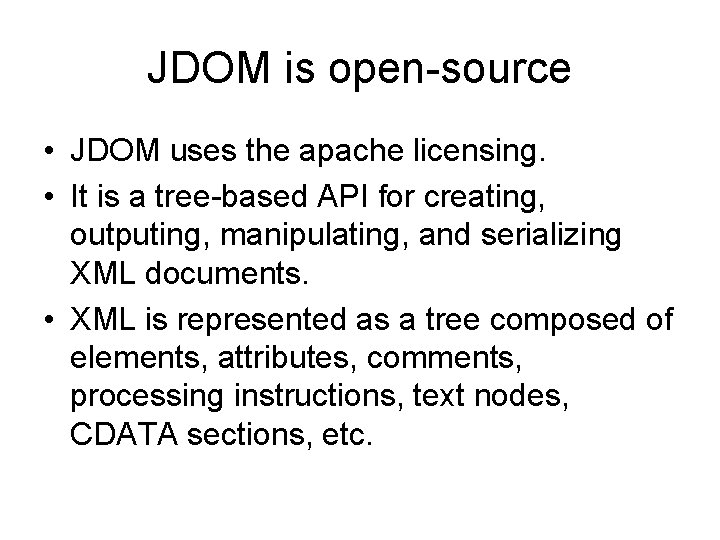
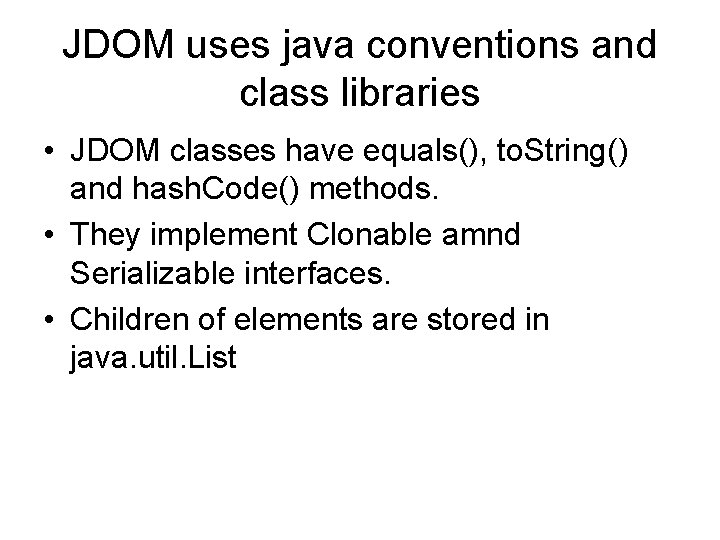
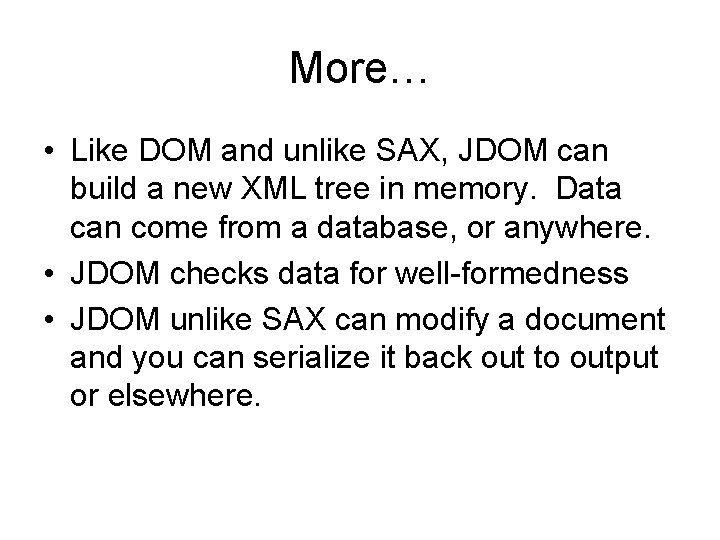
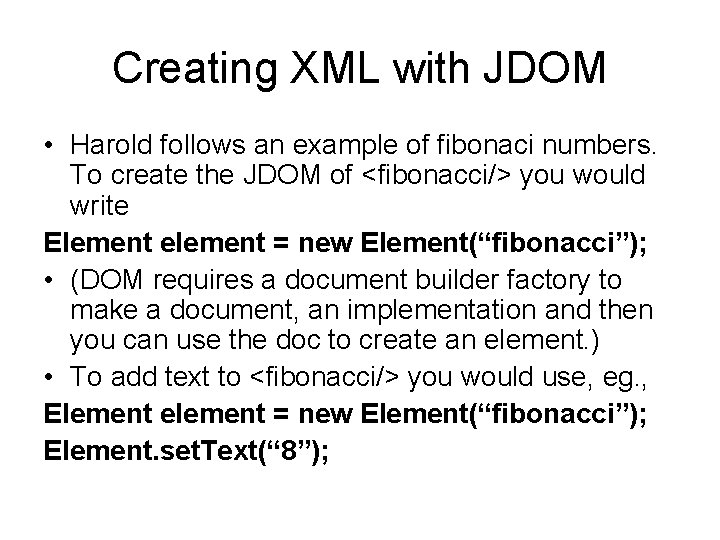
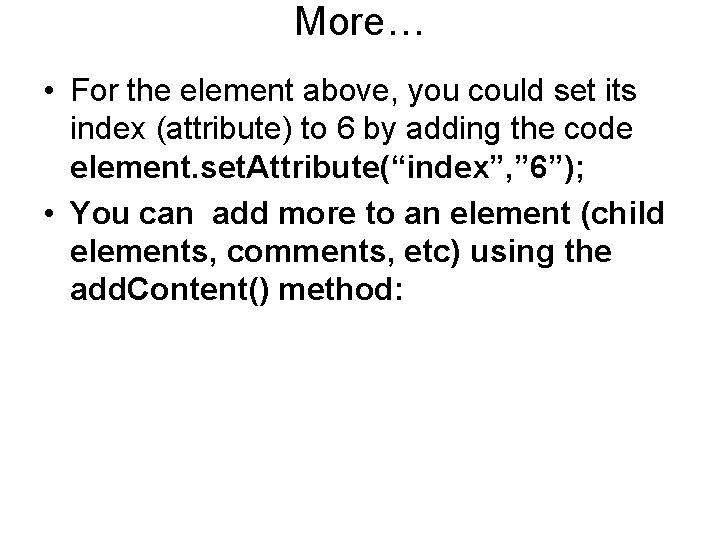
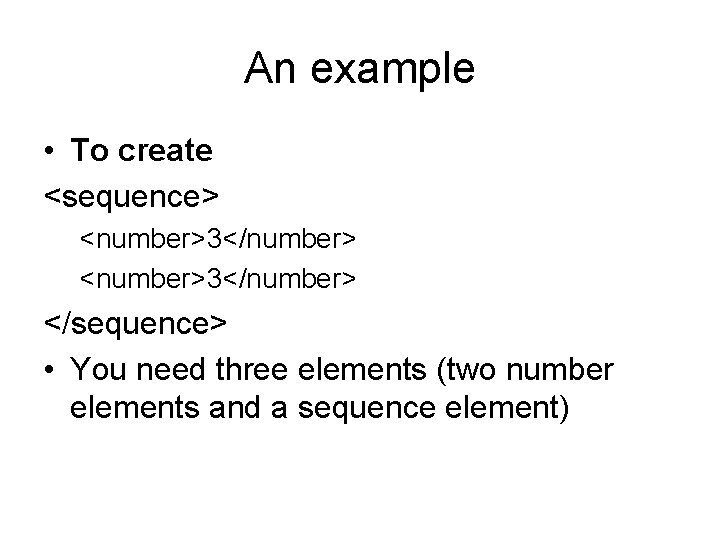
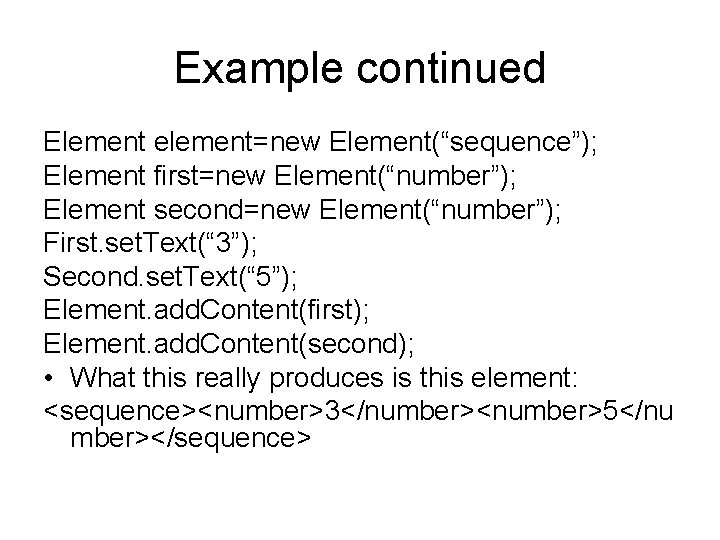
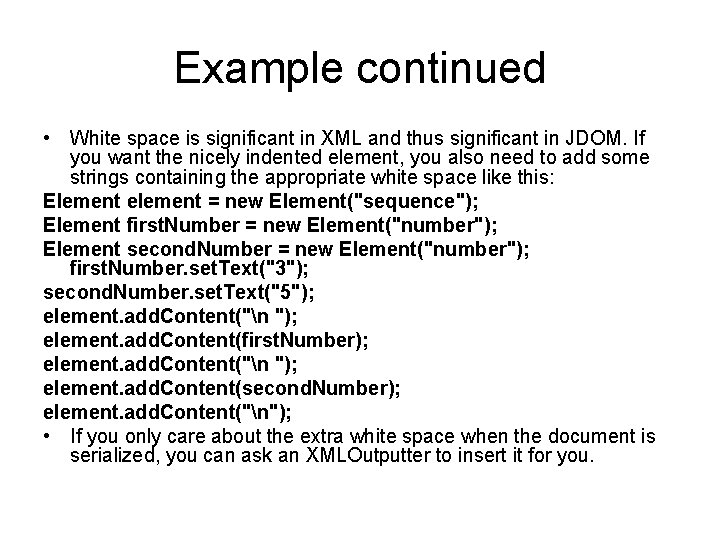
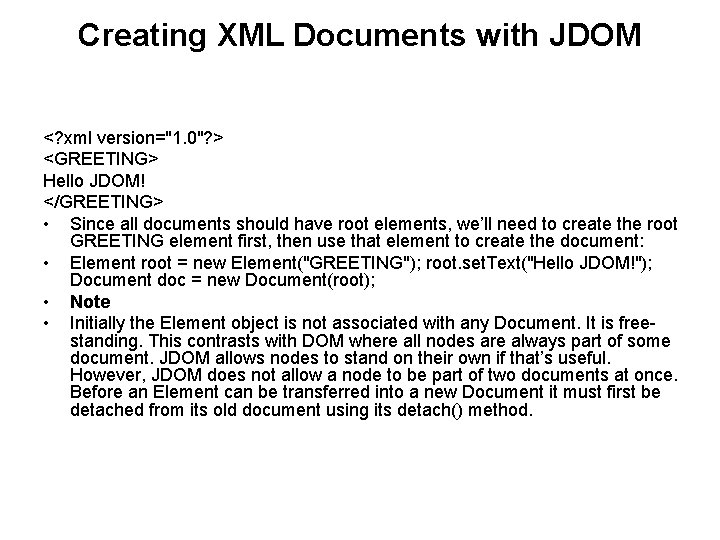
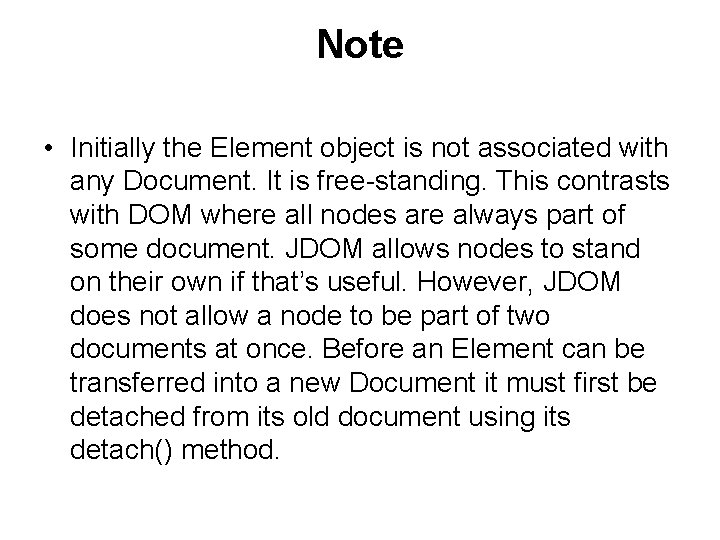
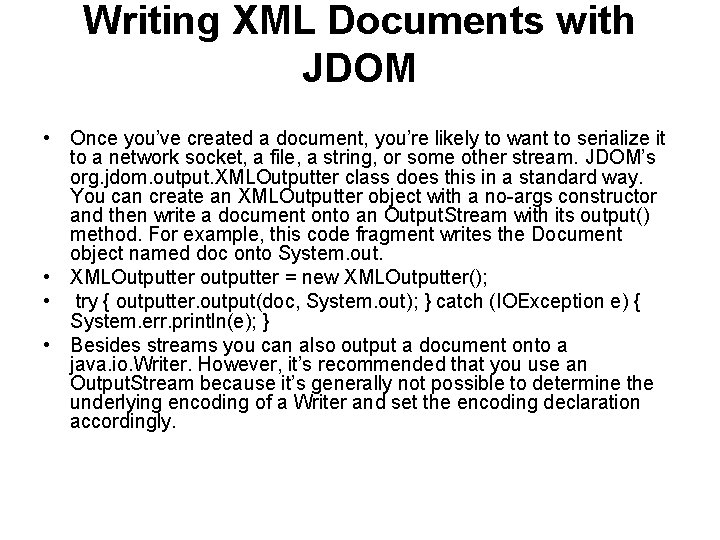
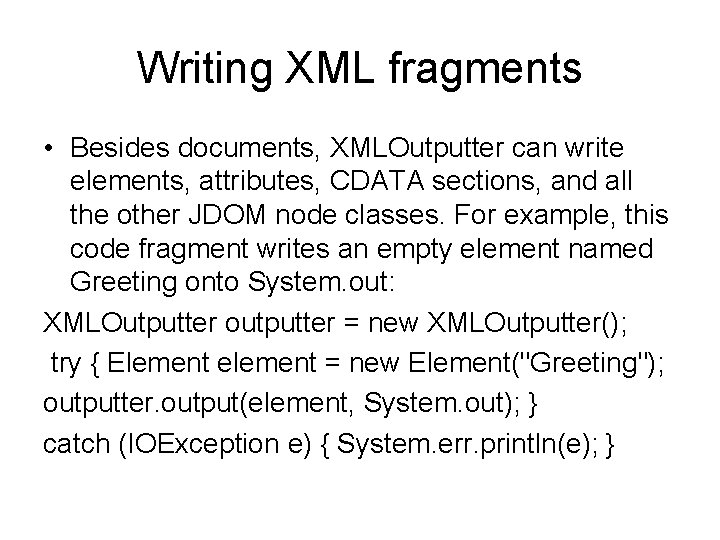
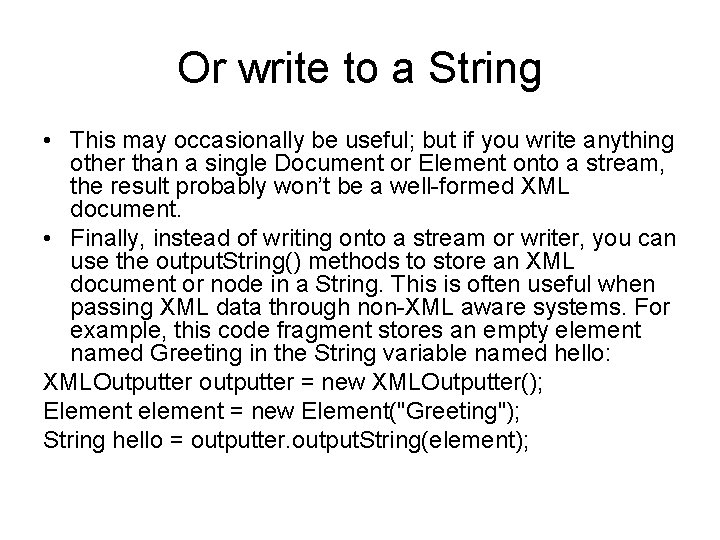
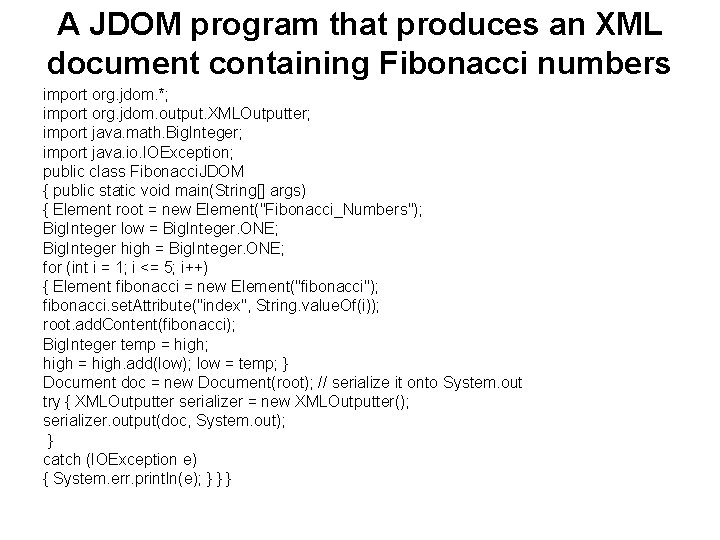
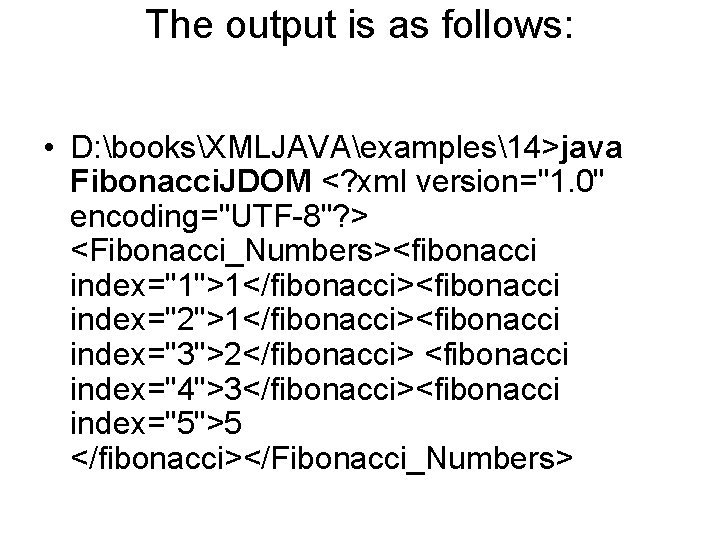
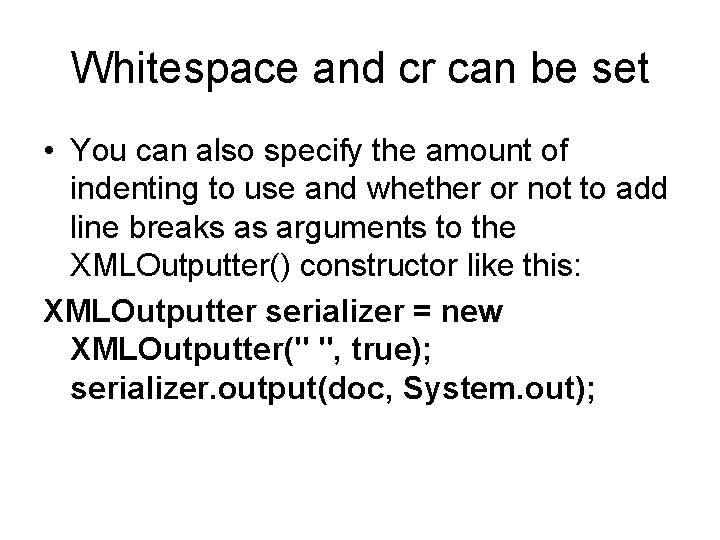
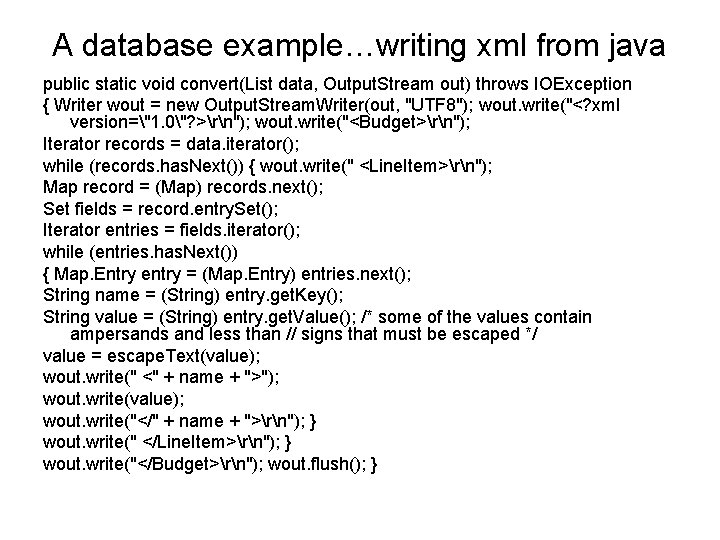
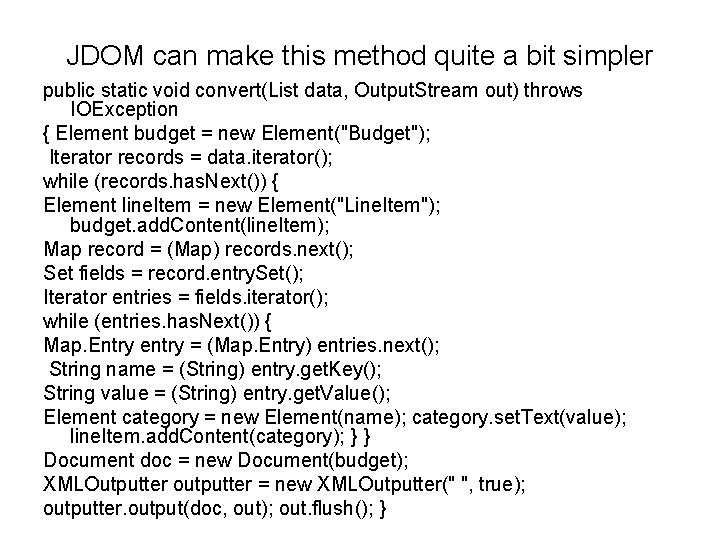
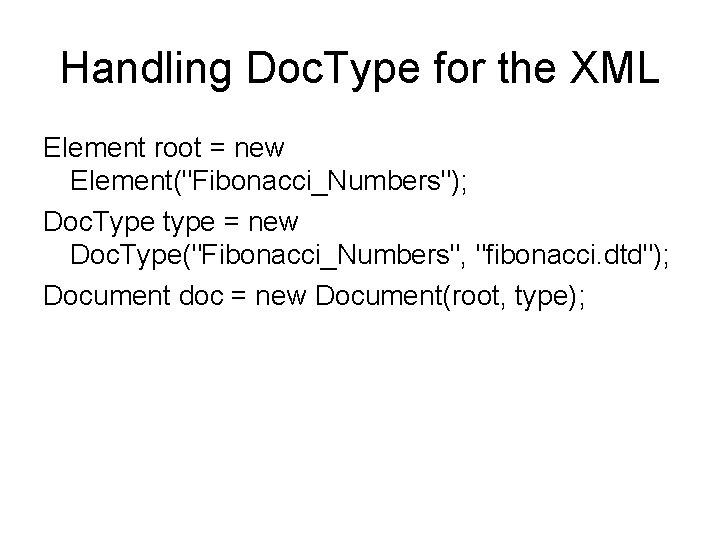
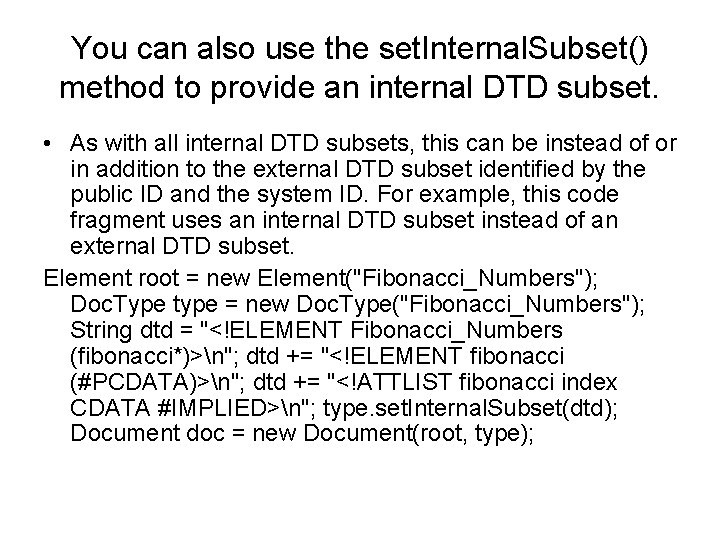
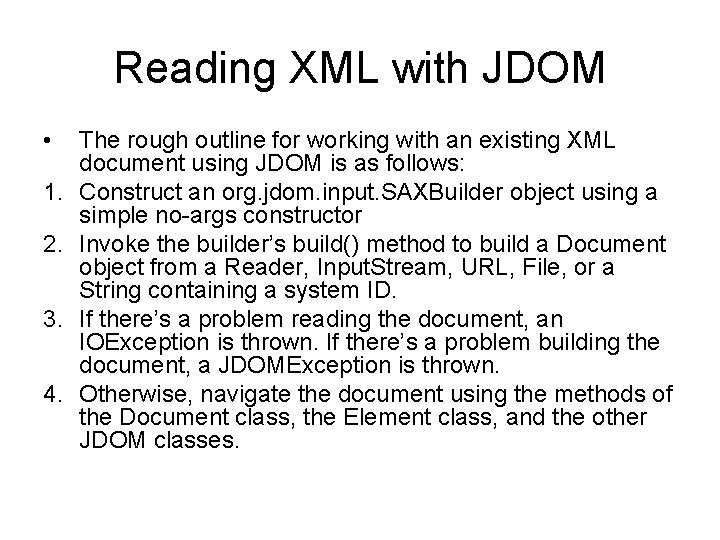
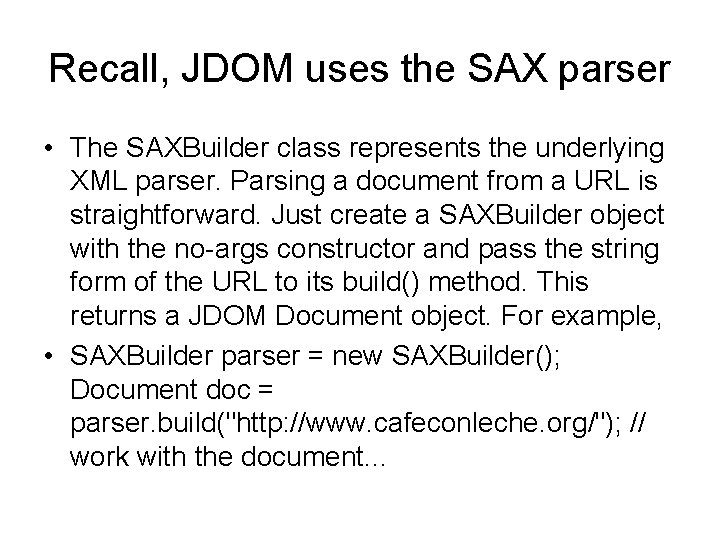
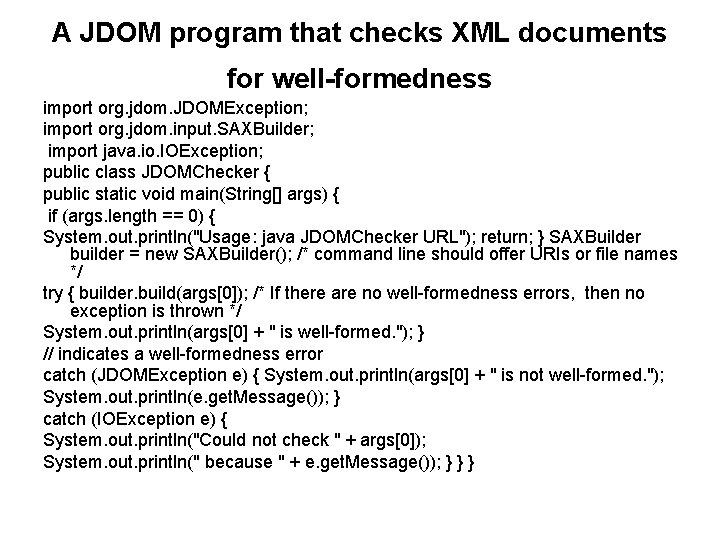
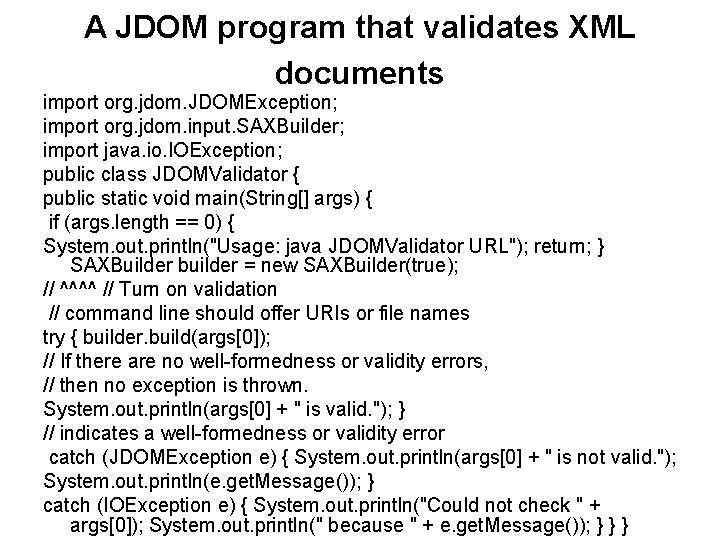
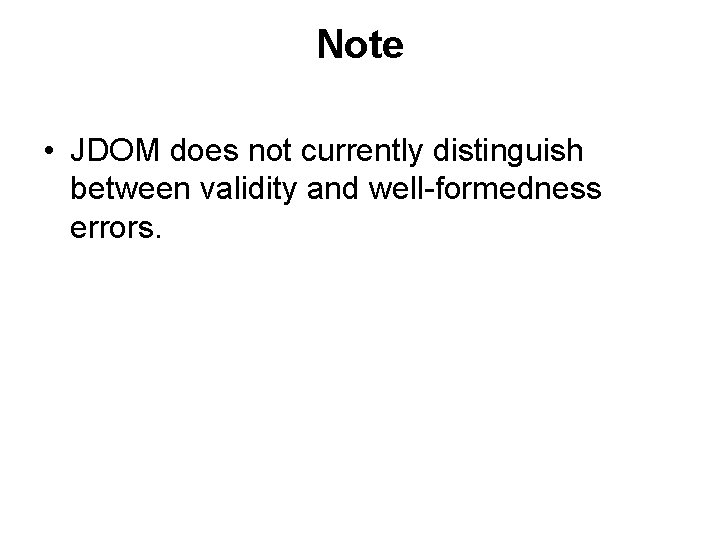
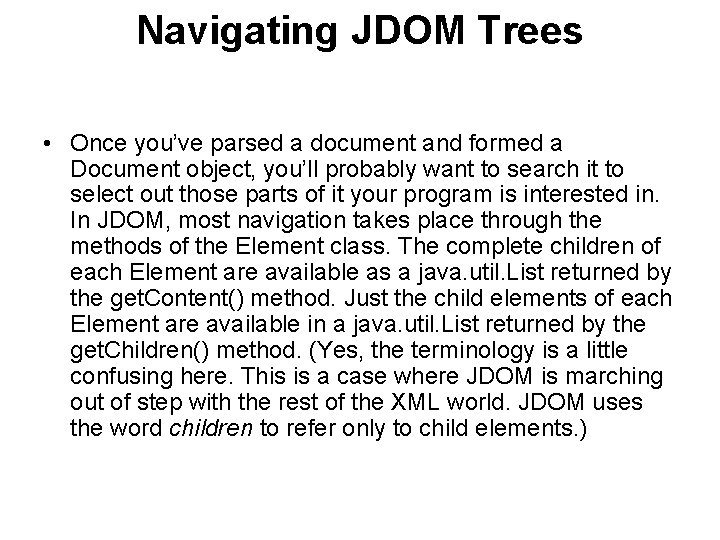
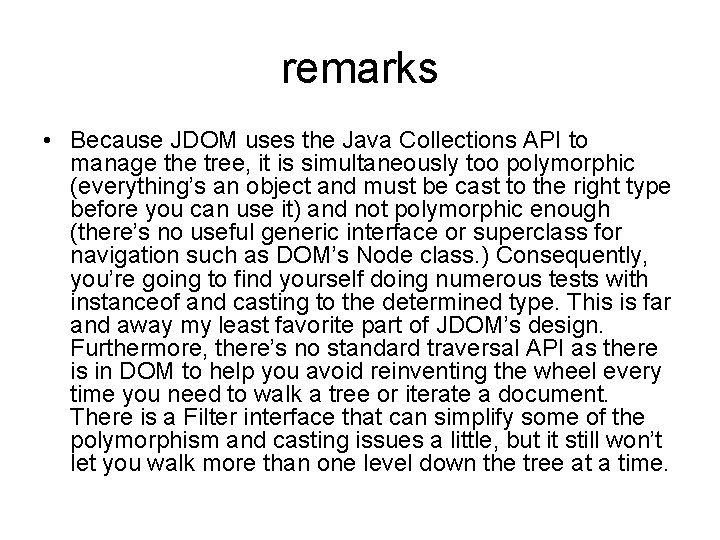
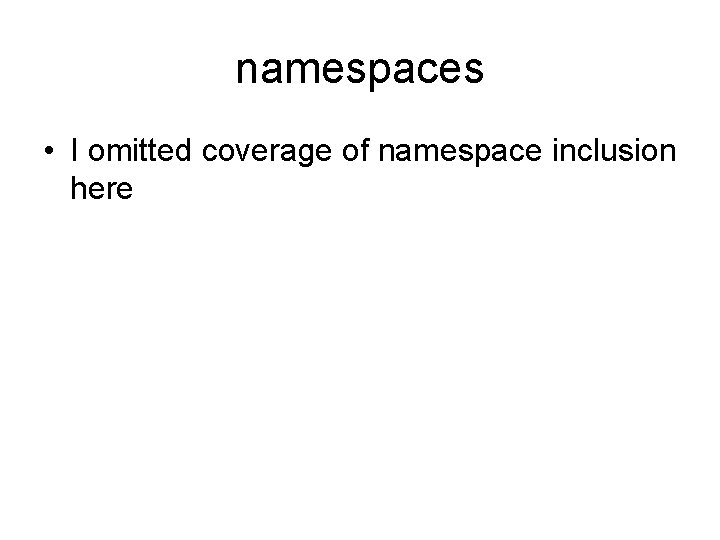
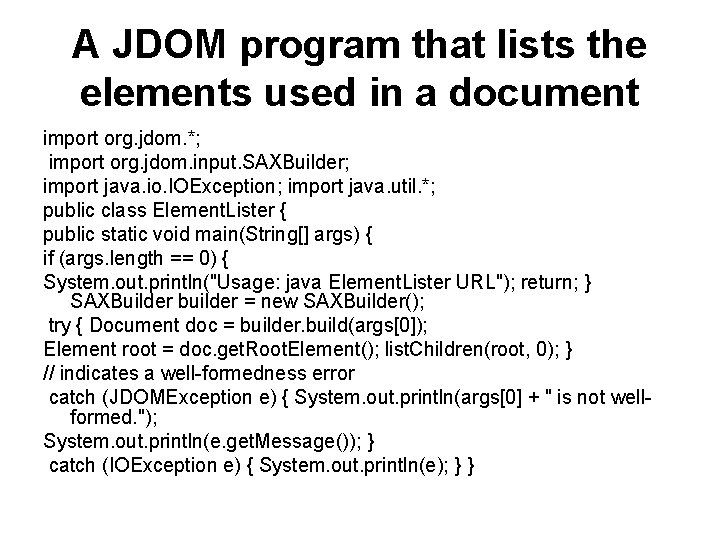
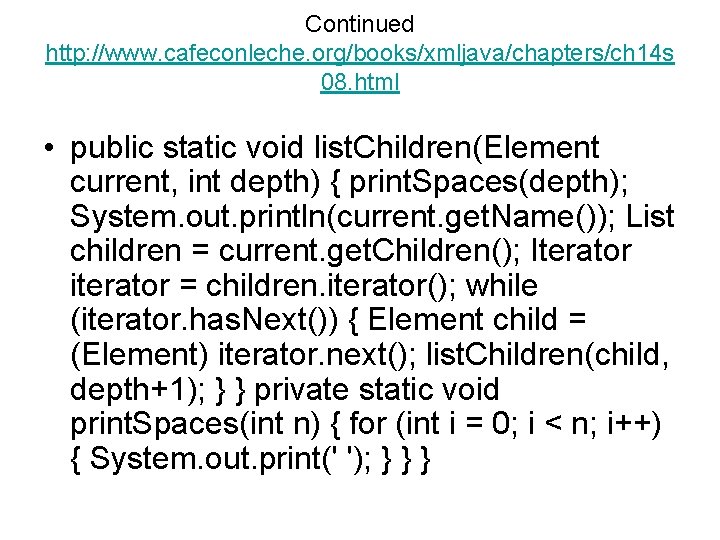
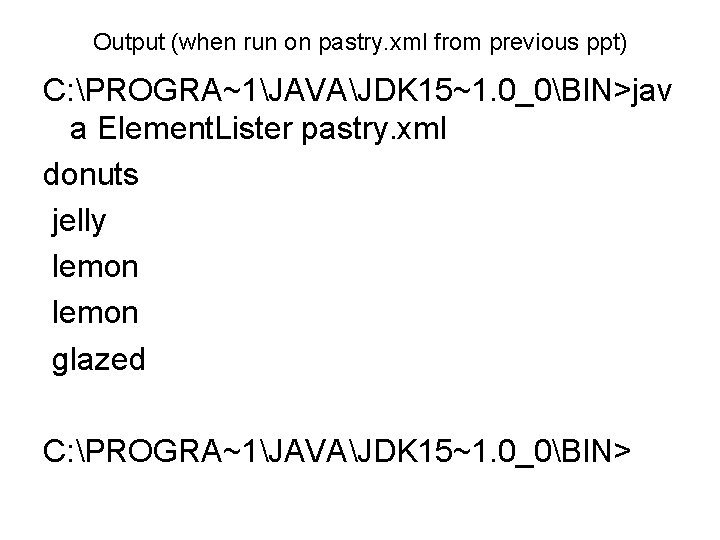
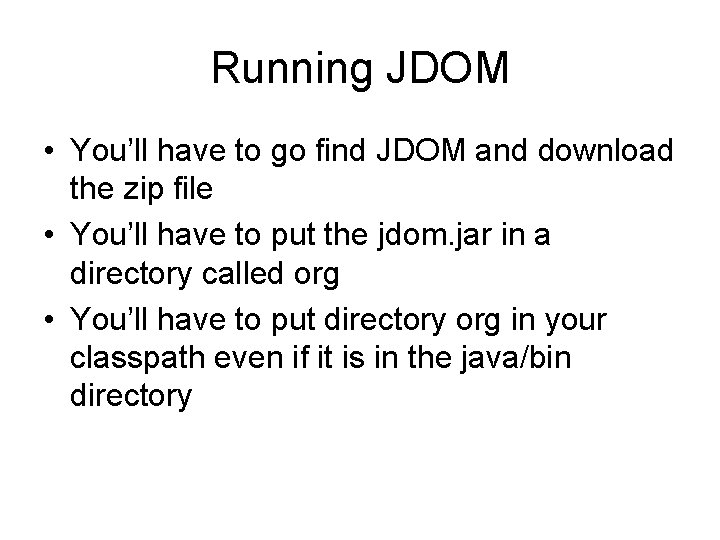
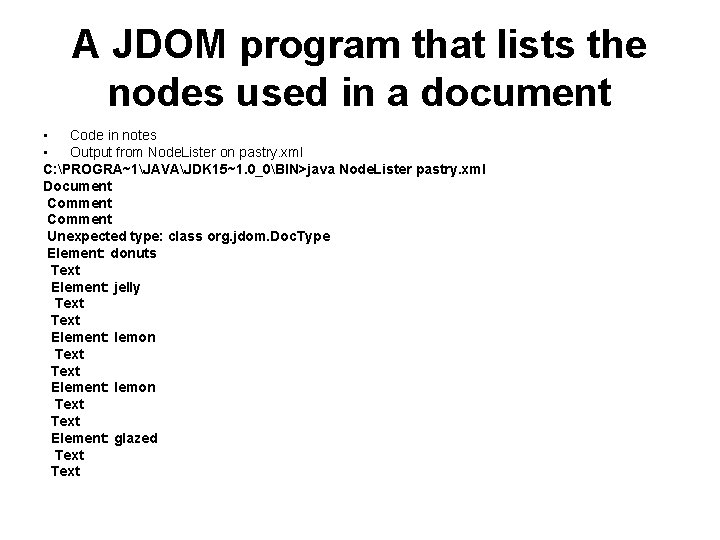
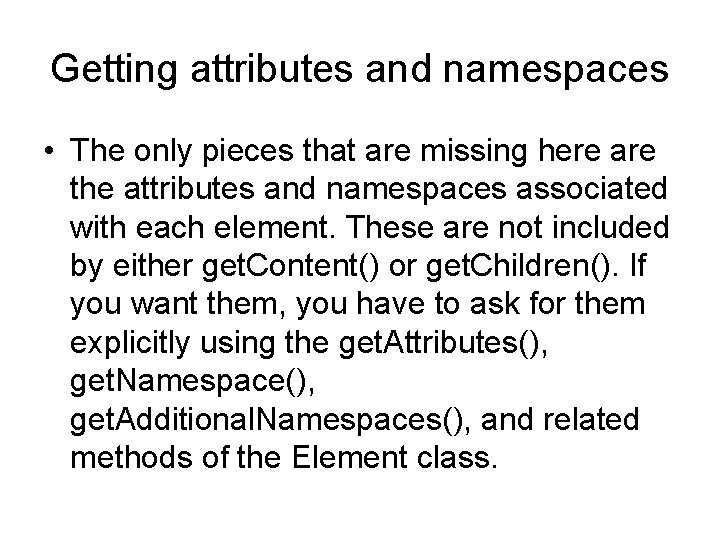
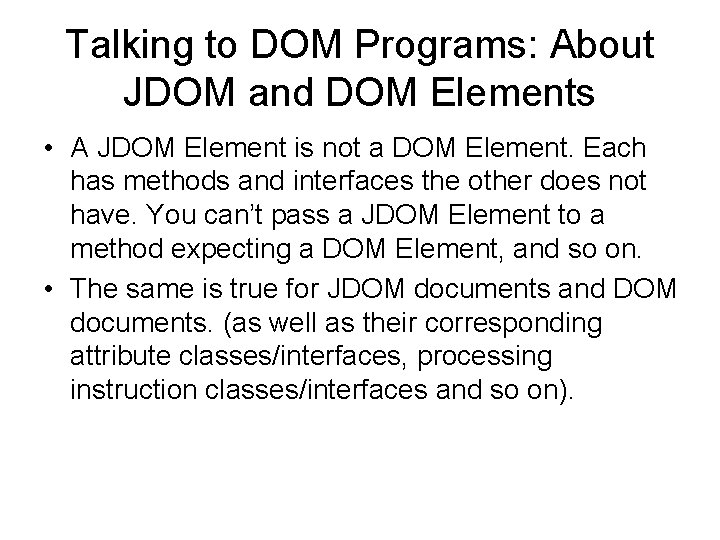
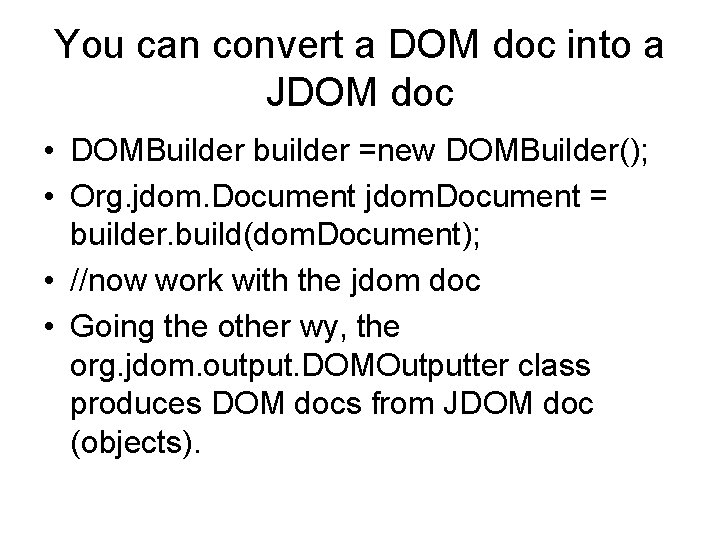
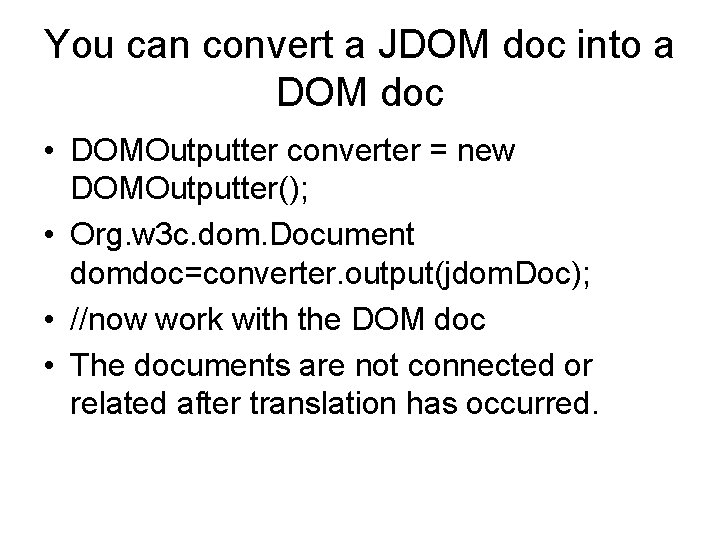
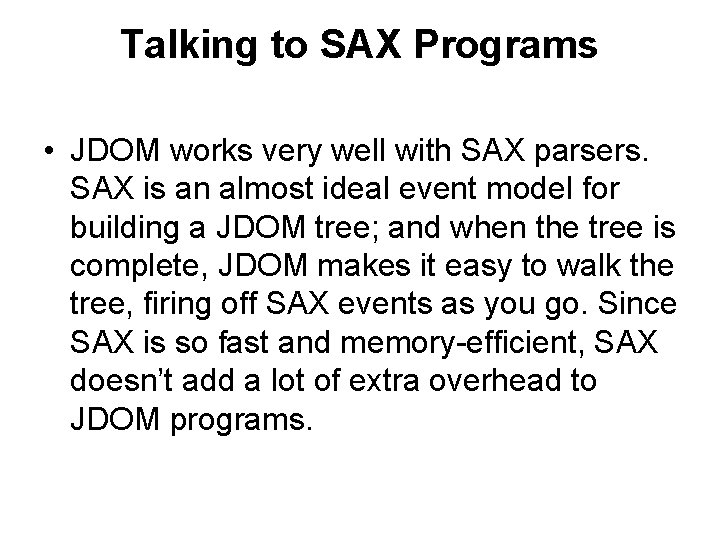
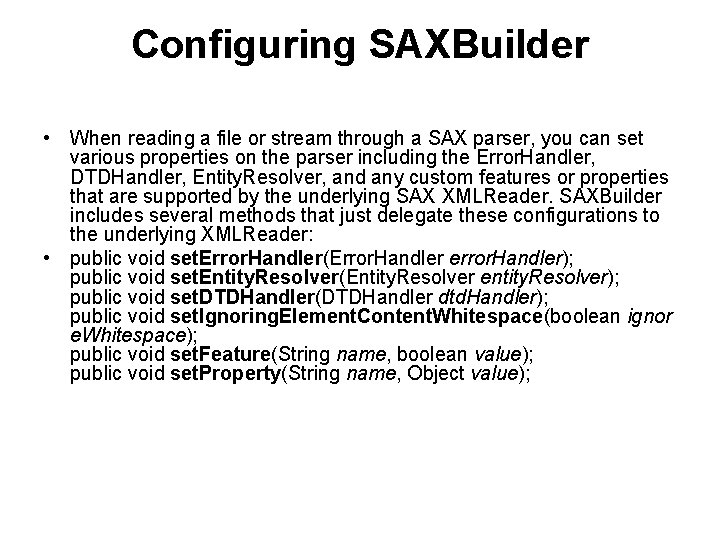
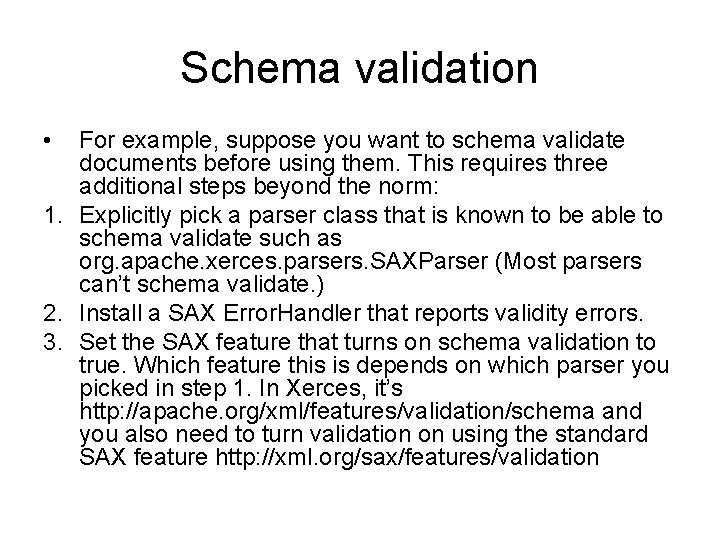
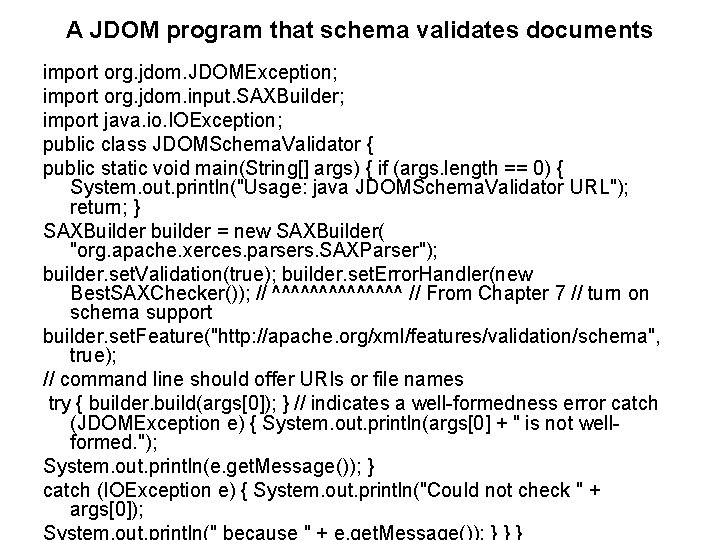
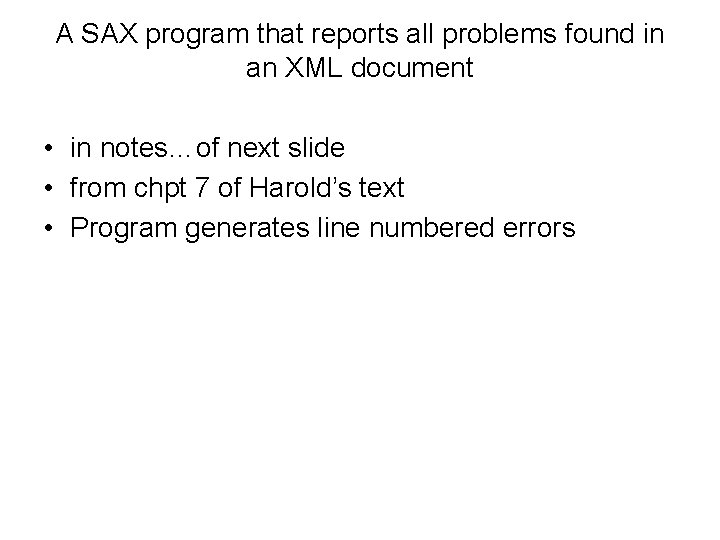
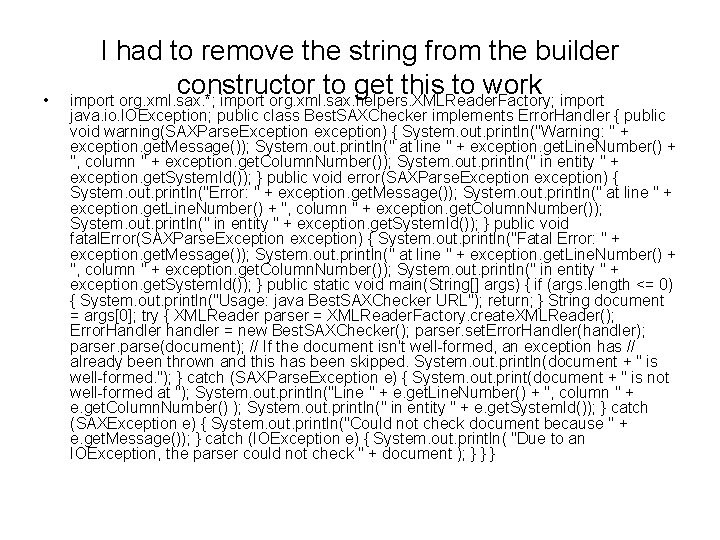
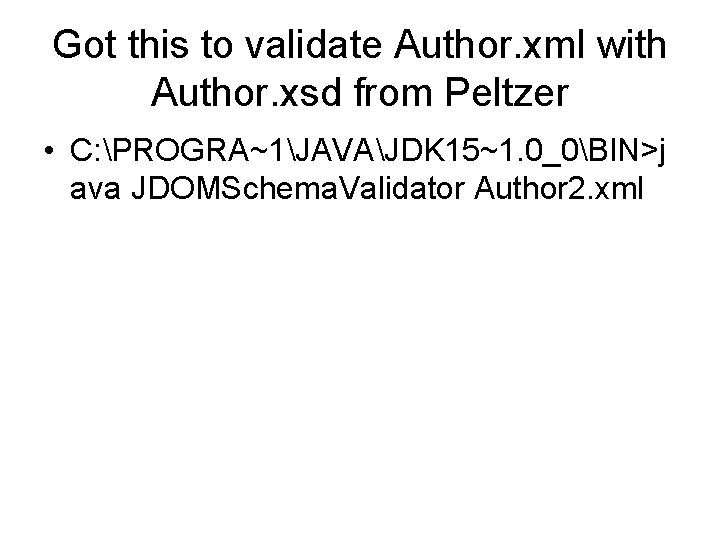
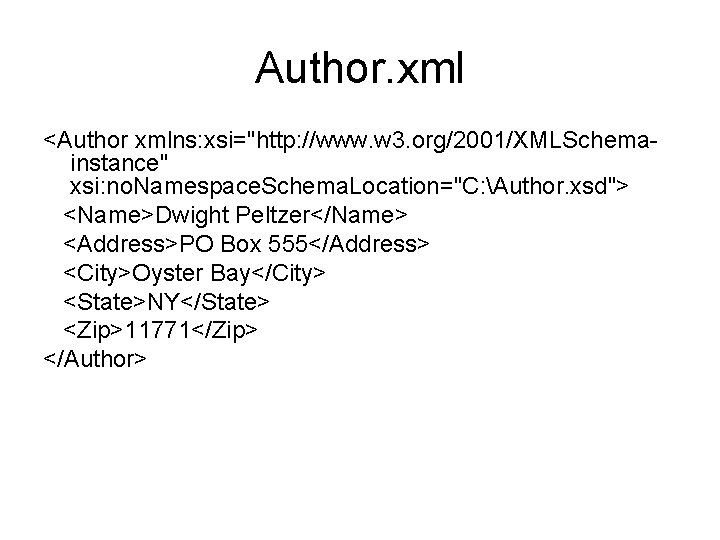
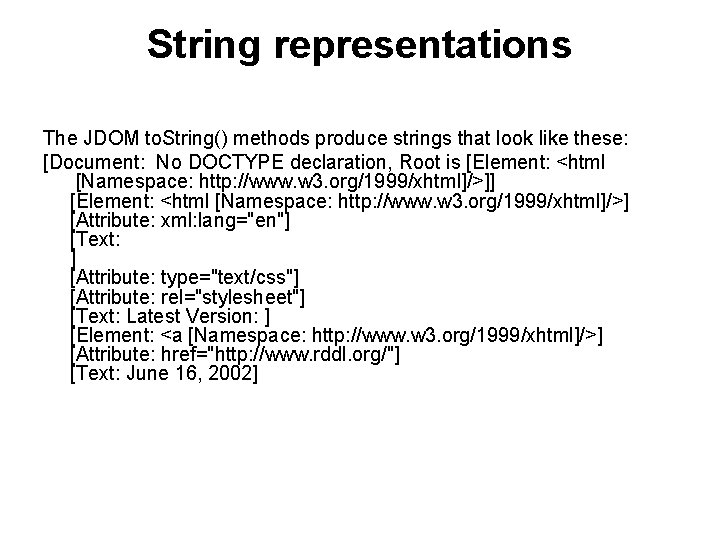
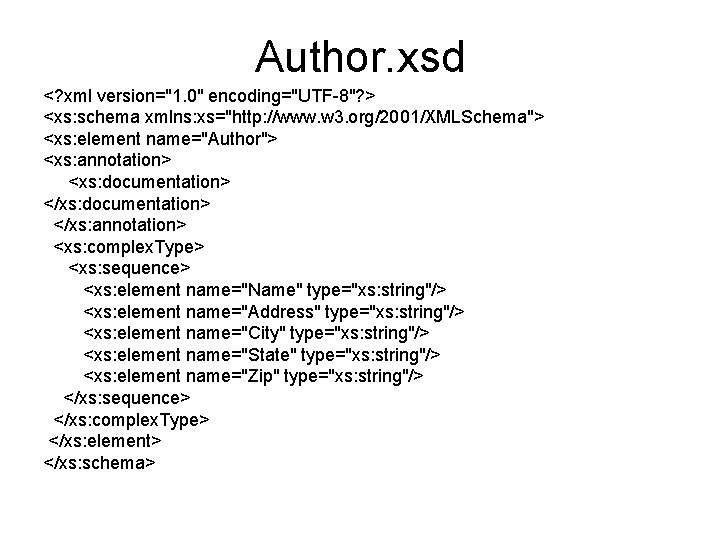
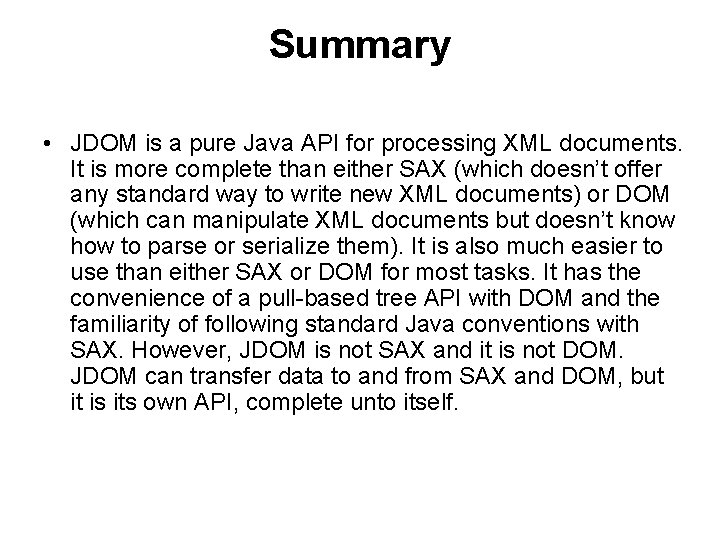
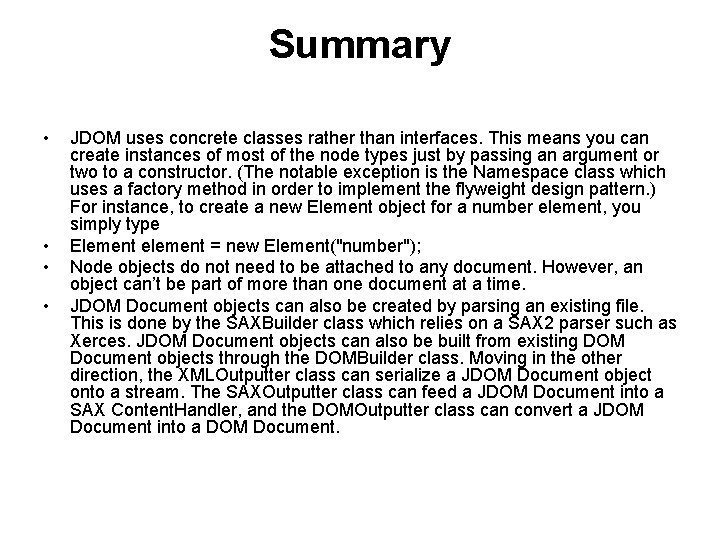
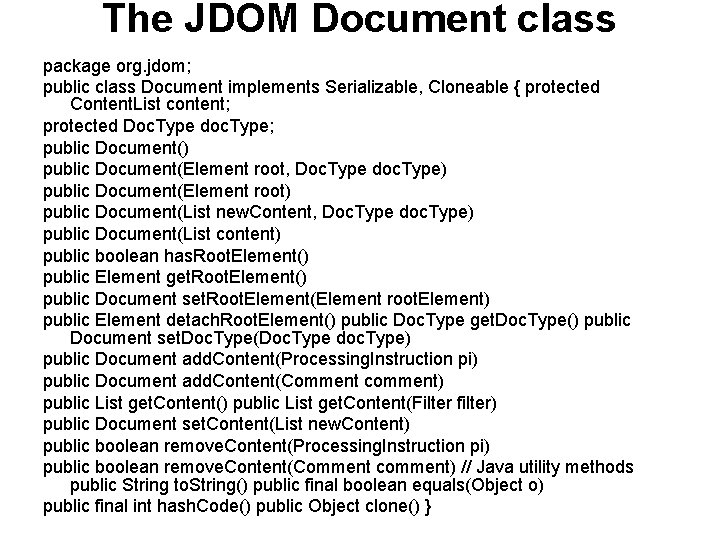
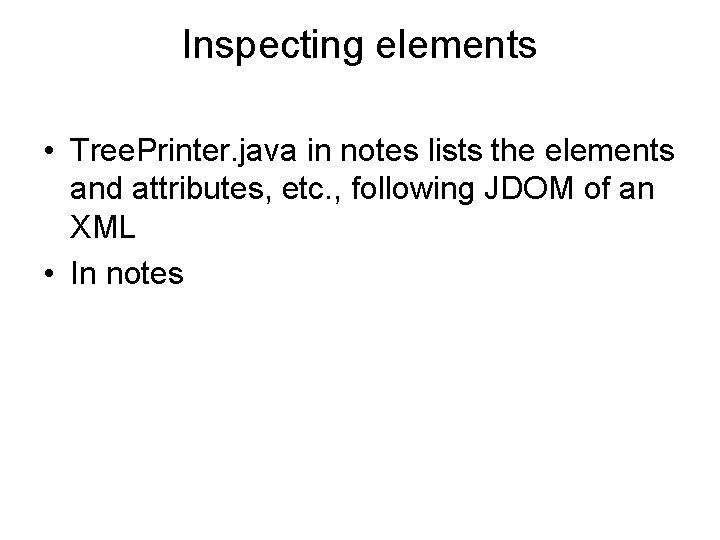
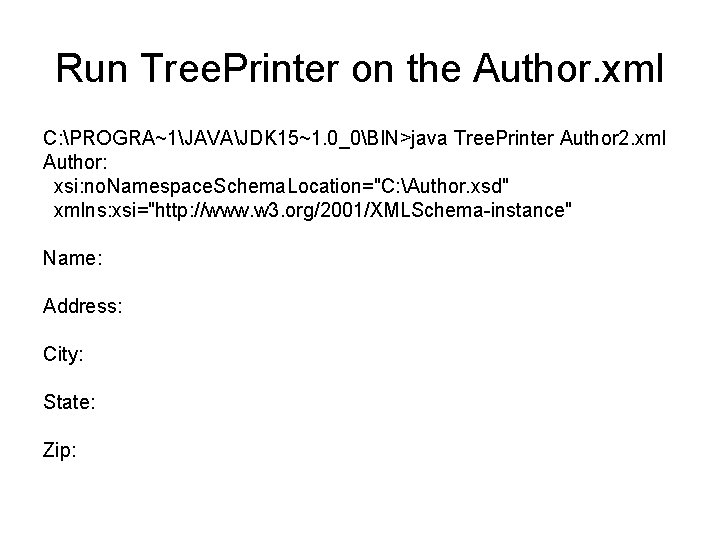
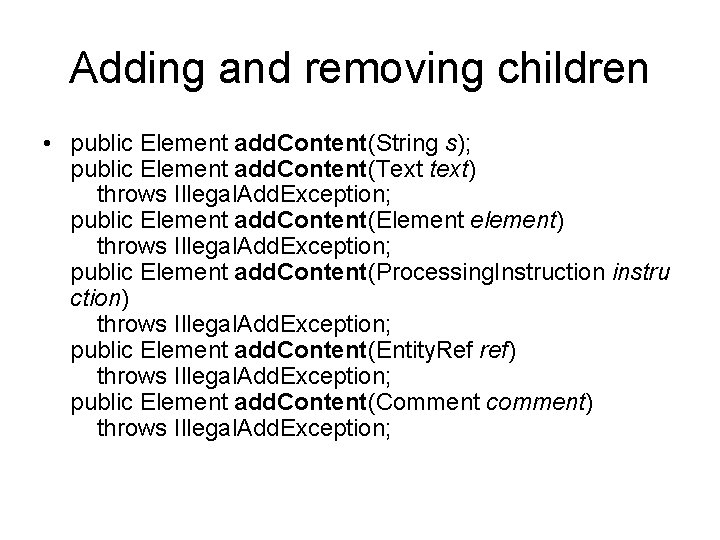
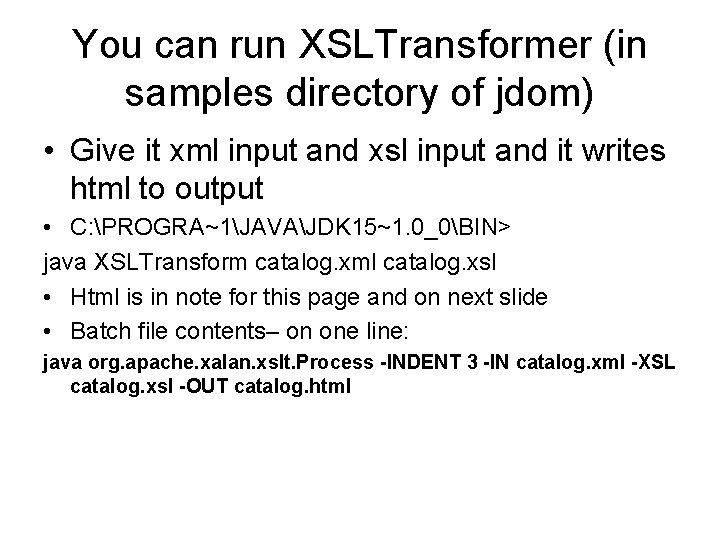
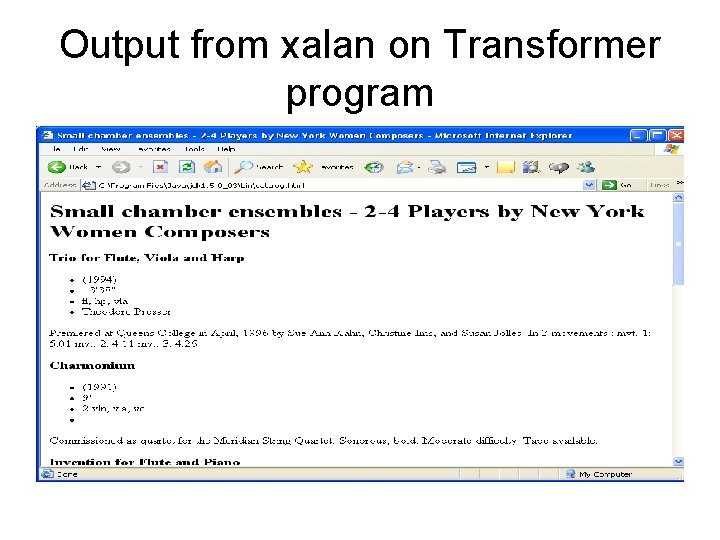
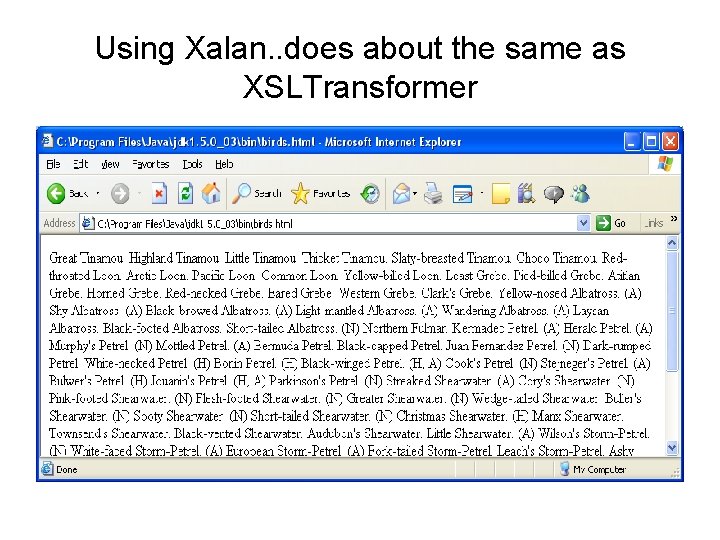
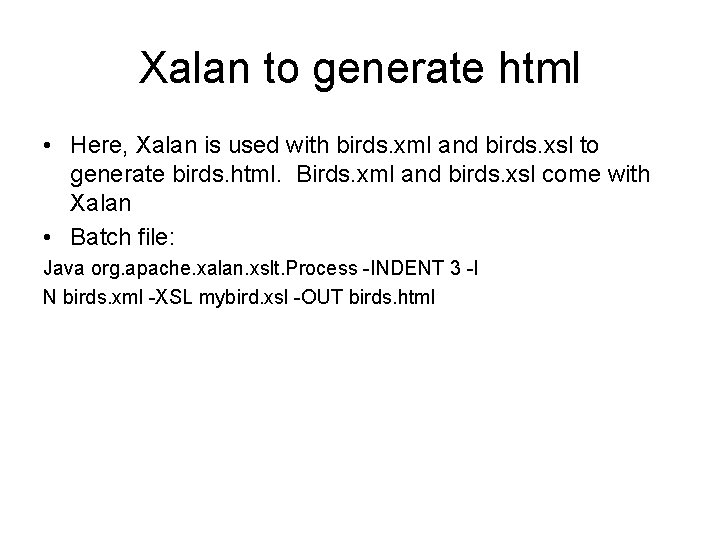
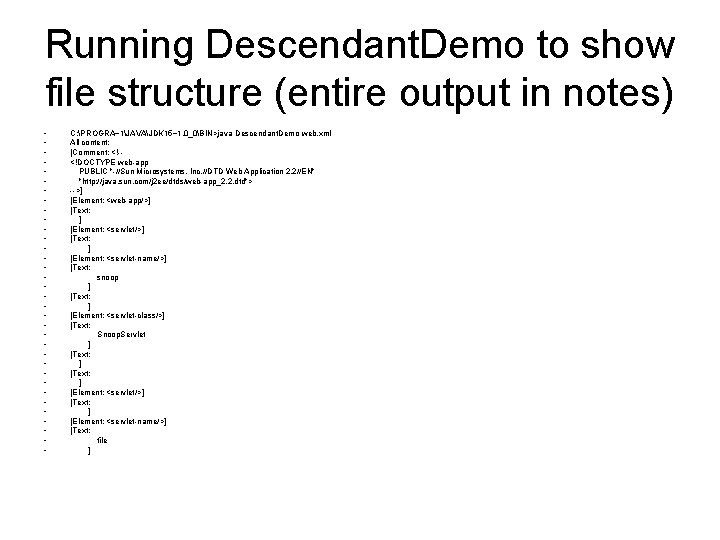
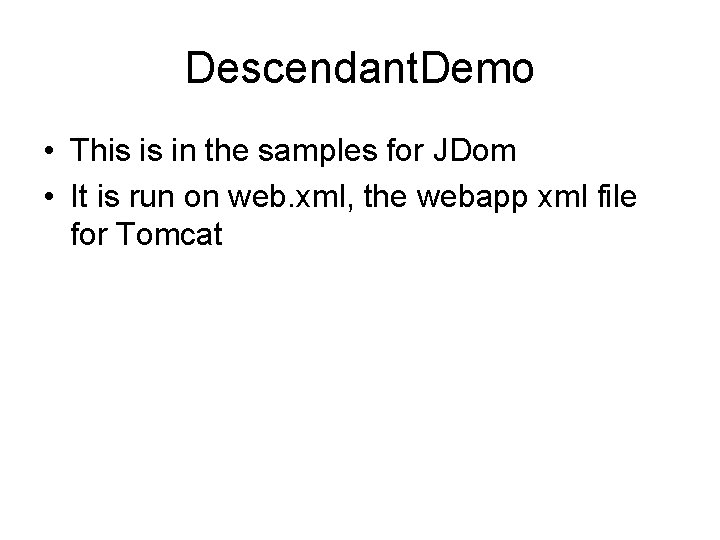
![SAXBuilder. Demo • "Usage: java SAXBuilder. Demo " + "[XML document filename] ([expand. Entities] SAXBuilder. Demo • "Usage: java SAXBuilder. Demo " + "[XML document filename] ([expand. Entities]](https://slidetodoc.com/presentation_image_h/3524ffee0431820bdee1ca8b6a3f825b/image-63.jpg)
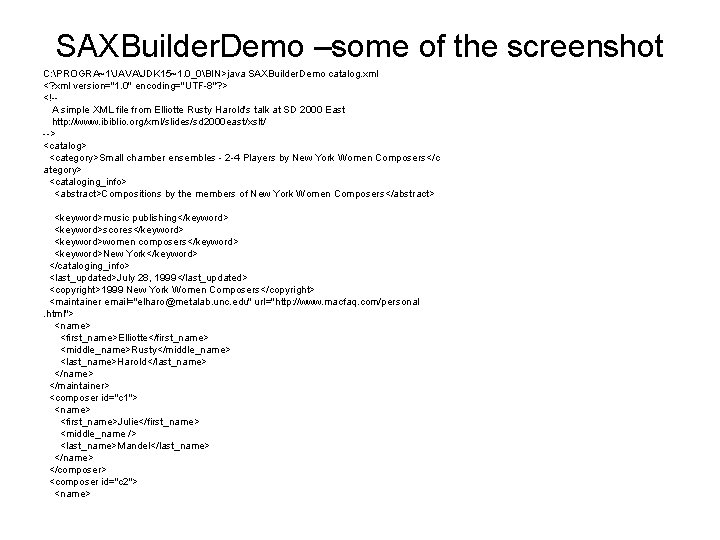
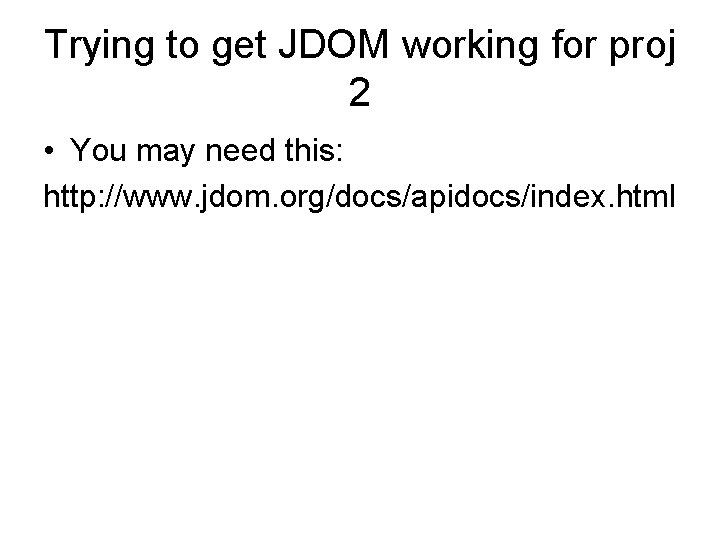
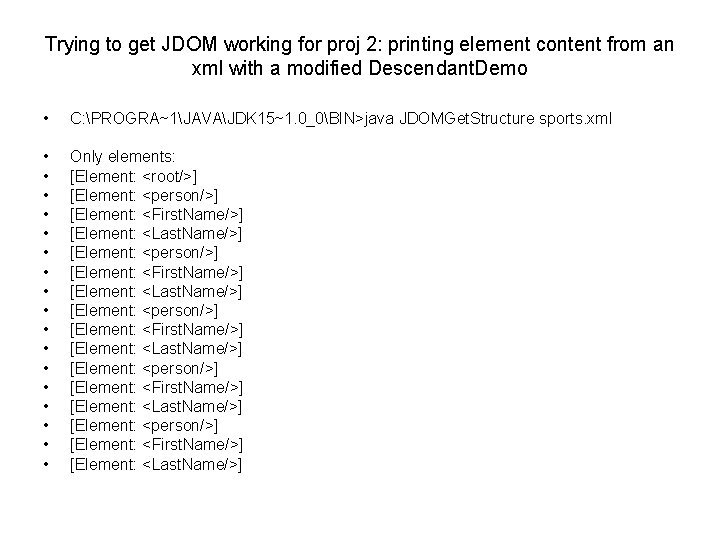
![My code (with imports omitted) public class JDOMGet. Structure { public static void main(String[] My code (with imports omitted) public class JDOMGet. Structure { public static void main(String[]](https://slidetodoc.com/presentation_image_h/3524ffee0431820bdee1ca8b6a3f825b/image-67.jpg)
- Slides: 67
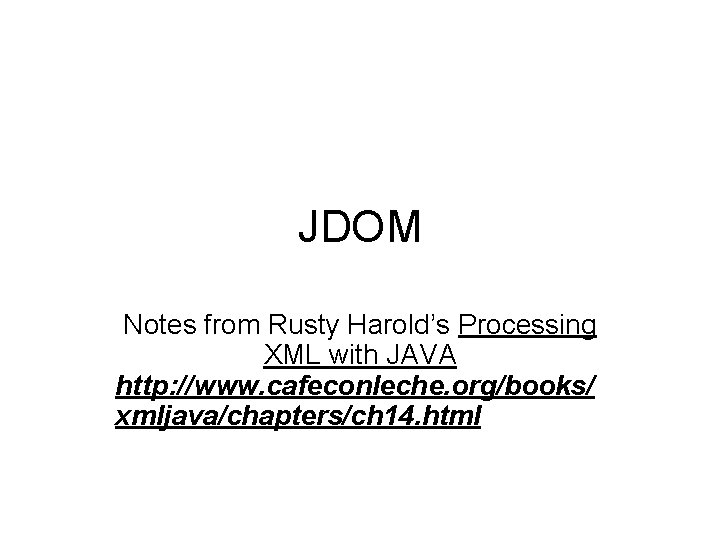
JDOM Notes from Rusty Harold’s Processing XML with JAVA http: //www. cafeconleche. org/books/ xmljava/chapters/ch 14. html
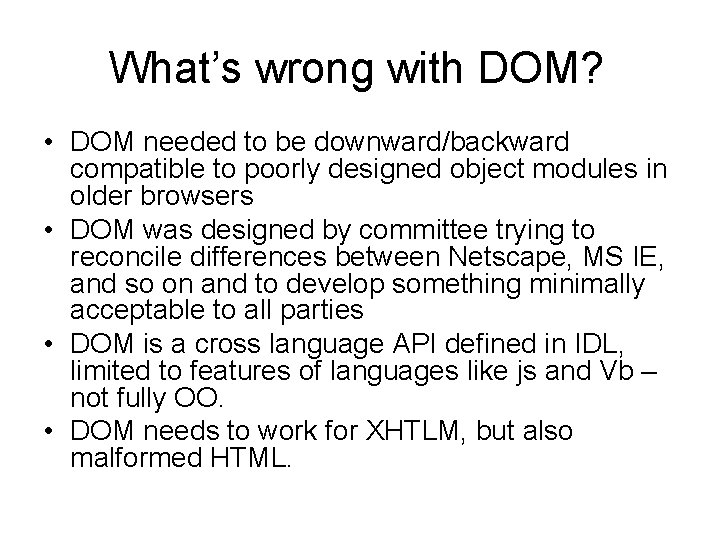
What’s wrong with DOM? • DOM needed to be downward/backward compatible to poorly designed object modules in older browsers • DOM was designed by committee trying to reconcile differences between Netscape, MS IE, and so on and to develop something minimally acceptable to all parties • DOM is a cross language API defined in IDL, limited to features of languages like js and Vb – not fully OO. • DOM needs to work for XHTLM, but also malformed HTML.
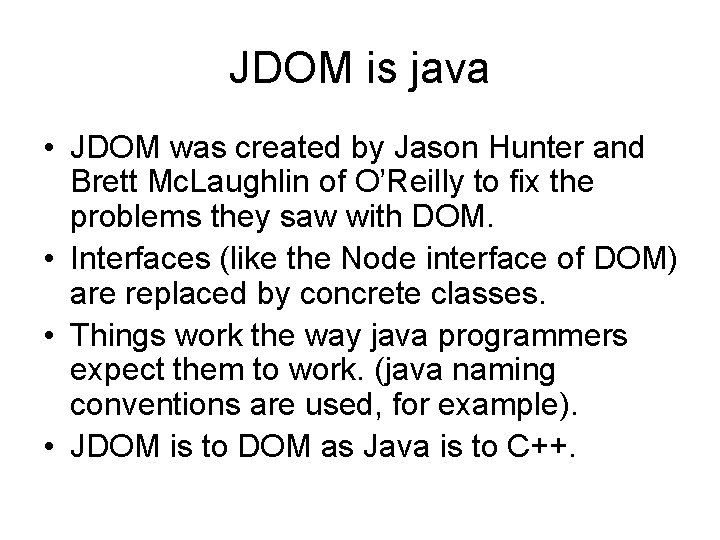
JDOM is java • JDOM was created by Jason Hunter and Brett Mc. Laughlin of O’Reilly to fix the problems they saw with DOM. • Interfaces (like the Node interface of DOM) are replaced by concrete classes. • Things work the way java programmers expect them to work. (java naming conventions are used, for example). • JDOM is to DOM as Java is to C++.
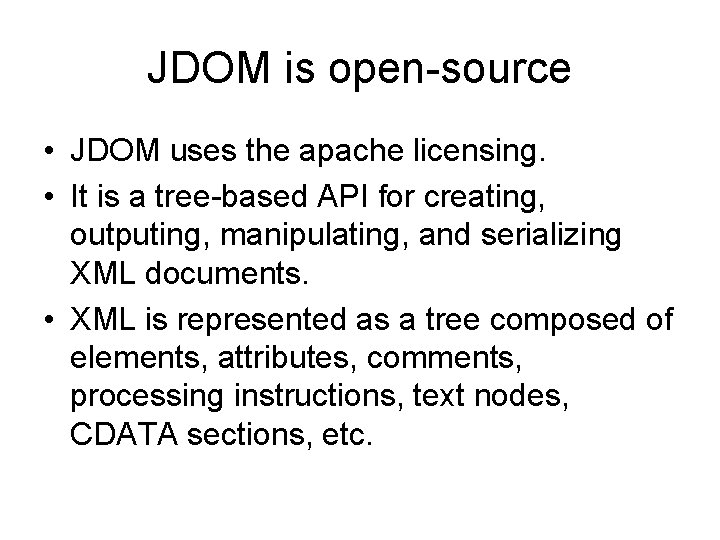
JDOM is open-source • JDOM uses the apache licensing. • It is a tree-based API for creating, outputing, manipulating, and serializing XML documents. • XML is represented as a tree composed of elements, attributes, comments, processing instructions, text nodes, CDATA sections, etc.
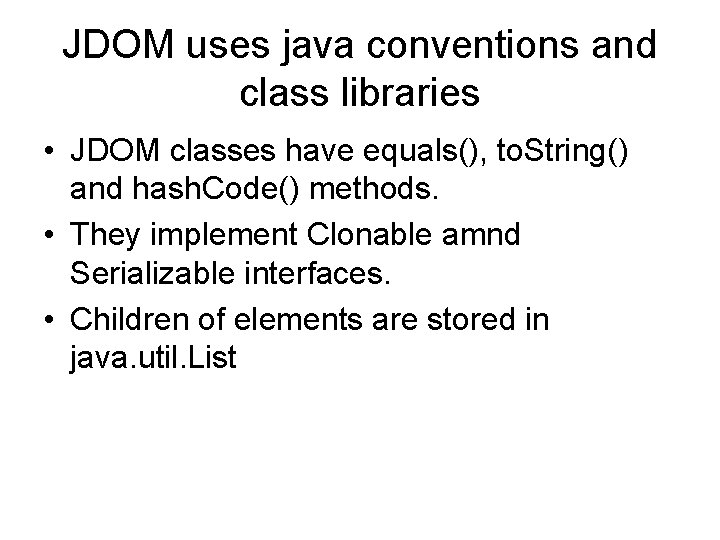
JDOM uses java conventions and class libraries • JDOM classes have equals(), to. String() and hash. Code() methods. • They implement Clonable amnd Serializable interfaces. • Children of elements are stored in java. util. List
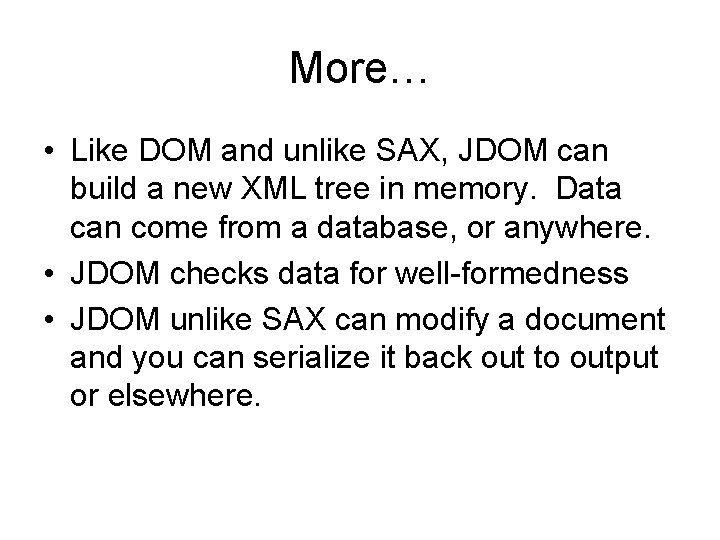
More… • Like DOM and unlike SAX, JDOM can build a new XML tree in memory. Data can come from a database, or anywhere. • JDOM checks data for well-formedness • JDOM unlike SAX can modify a document and you can serialize it back out to output or elsewhere.
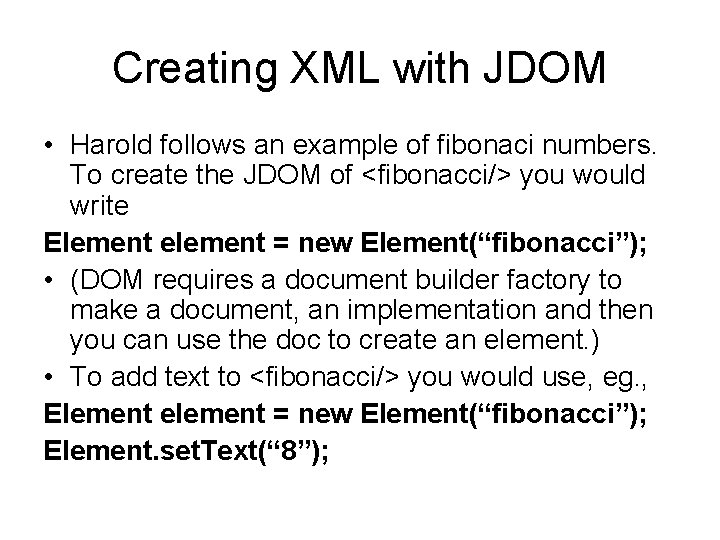
Creating XML with JDOM • Harold follows an example of fibonaci numbers. To create the JDOM of <fibonacci/> you would write Element element = new Element(“fibonacci”); • (DOM requires a document builder factory to make a document, an implementation and then you can use the doc to create an element. ) • To add text to <fibonacci/> you would use, eg. , Element element = new Element(“fibonacci”); Element. set. Text(“ 8”);
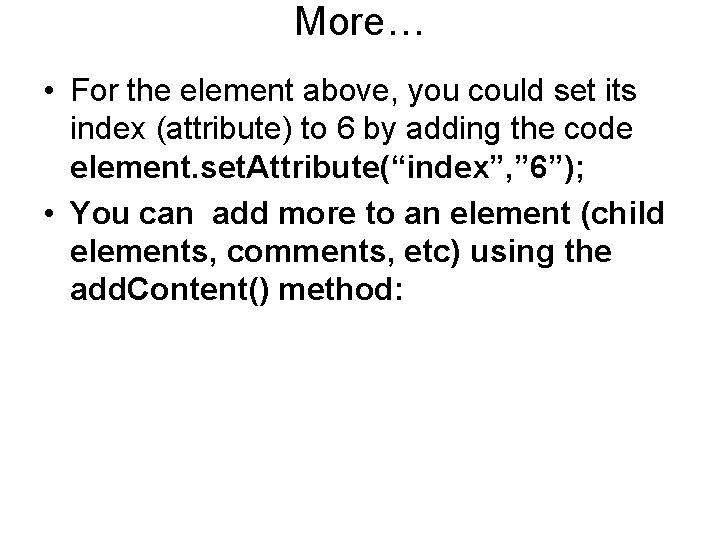
More… • For the element above, you could set its index (attribute) to 6 by adding the code element. set. Attribute(“index”, ” 6”); • You can add more to an element (child elements, comments, etc) using the add. Content() method:
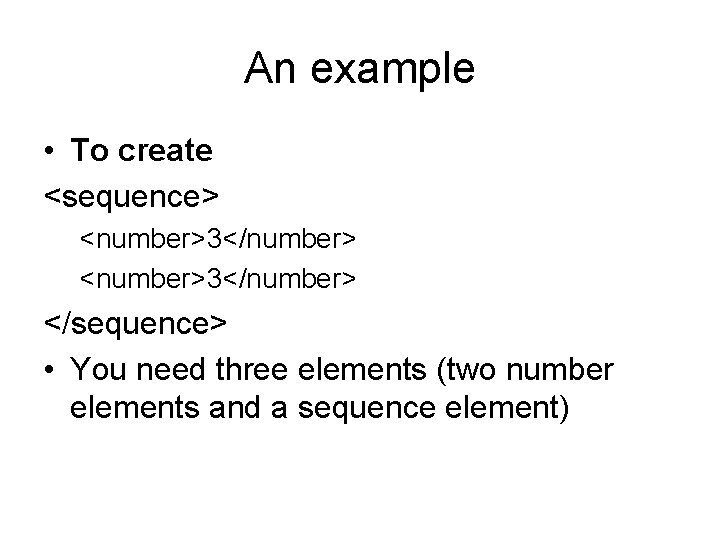
An example • To create <sequence> <number>3</number> </sequence> • You need three elements (two number elements and a sequence element)
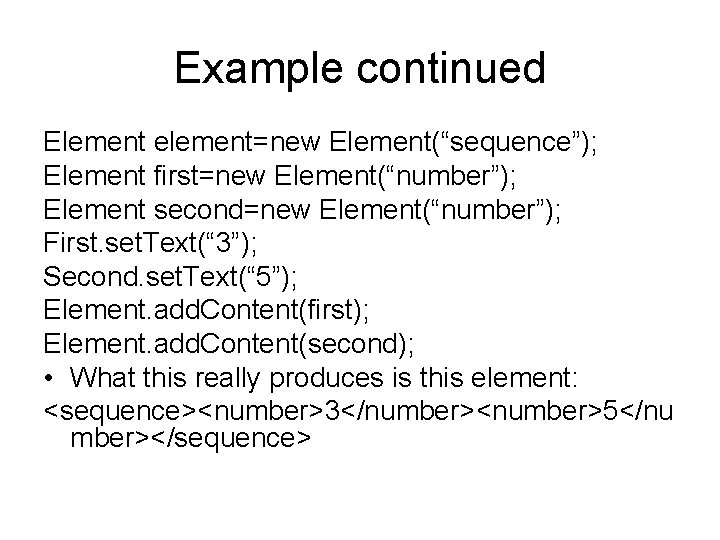
Example continued Element element=new Element(“sequence”); Element first=new Element(“number”); Element second=new Element(“number”); First. set. Text(“ 3”); Second. set. Text(“ 5”); Element. add. Content(first); Element. add. Content(second); • What this really produces is this element: <sequence><number>3</number><number>5</nu mber></sequence>
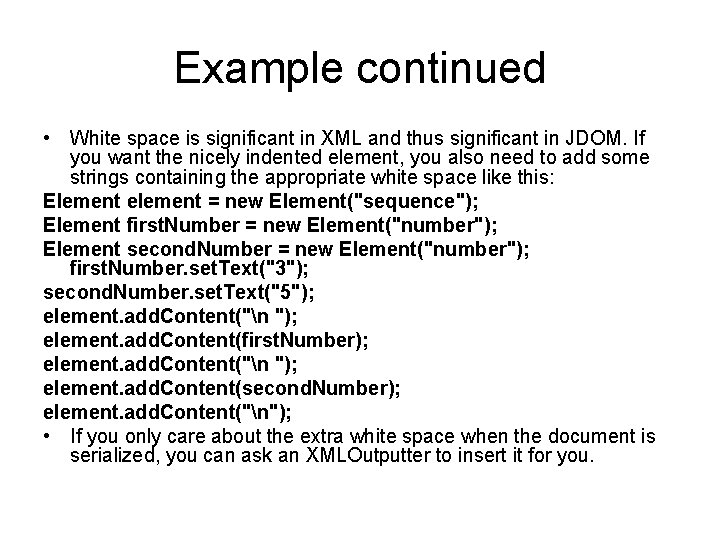
Example continued • White space is significant in XML and thus significant in JDOM. If you want the nicely indented element, you also need to add some strings containing the appropriate white space like this: Element element = new Element("sequence"); Element first. Number = new Element("number"); Element second. Number = new Element("number"); first. Number. set. Text("3"); second. Number. set. Text("5"); element. add. Content("n "); element. add. Content(first. Number); element. add. Content("n "); element. add. Content(second. Number); element. add. Content("n"); • If you only care about the extra white space when the document is serialized, you can ask an XMLOutputter to insert it for you.
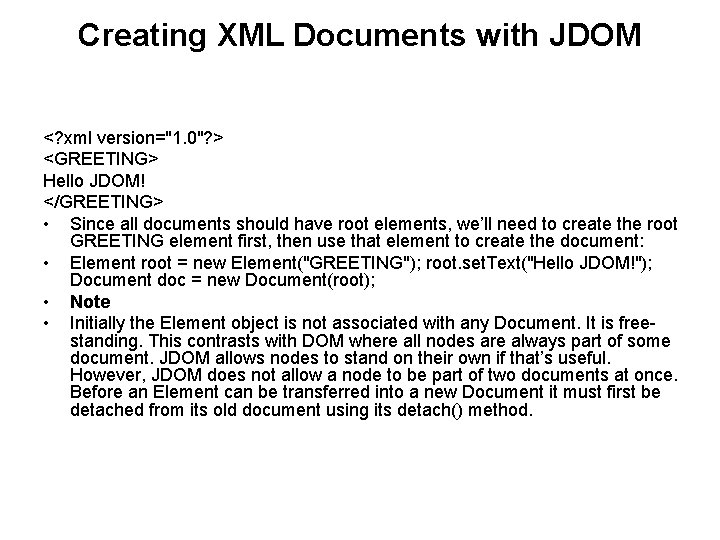
Creating XML Documents with JDOM <? xml version="1. 0"? > <GREETING> Hello JDOM! </GREETING> • Since all documents should have root elements, we’ll need to create the root GREETING element first, then use that element to create the document: • Element root = new Element("GREETING"); root. set. Text("Hello JDOM!"); Document doc = new Document(root); • Note • Initially the Element object is not associated with any Document. It is freestanding. This contrasts with DOM where all nodes are always part of some document. JDOM allows nodes to stand on their own if that’s useful. However, JDOM does not allow a node to be part of two documents at once. Before an Element can be transferred into a new Document it must first be detached from its old document using its detach() method.
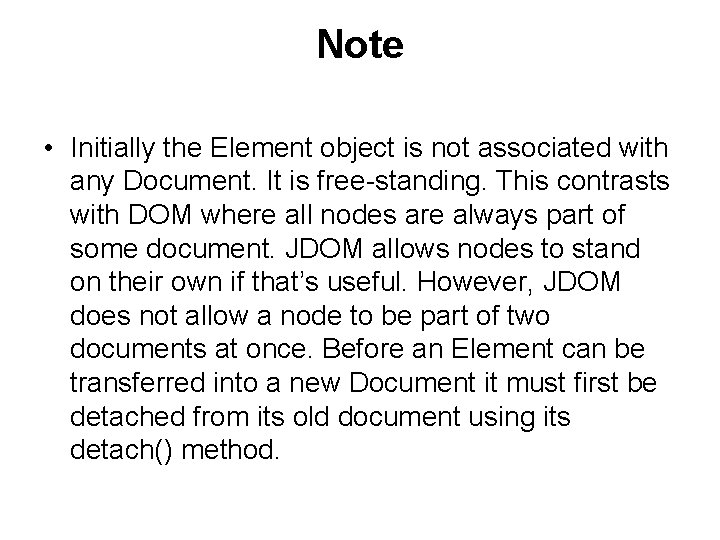
Note • Initially the Element object is not associated with any Document. It is free-standing. This contrasts with DOM where all nodes are always part of some document. JDOM allows nodes to stand on their own if that’s useful. However, JDOM does not allow a node to be part of two documents at once. Before an Element can be transferred into a new Document it must first be detached from its old document using its detach() method.
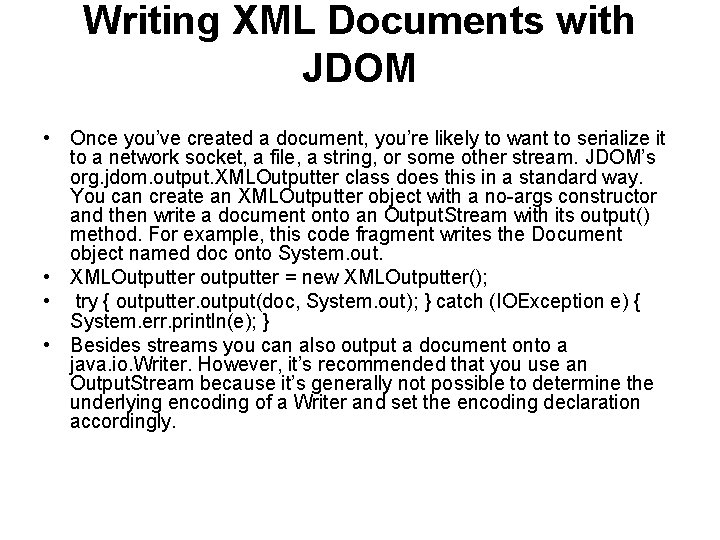
Writing XML Documents with JDOM • Once you’ve created a document, you’re likely to want to serialize it to a network socket, a file, a string, or some other stream. JDOM’s org. jdom. output. XMLOutputter class does this in a standard way. You can create an XMLOutputter object with a no-args constructor and then write a document onto an Output. Stream with its output() method. For example, this code fragment writes the Document object named doc onto System. out. • XMLOutputter outputter = new XMLOutputter(); • try { outputter. output(doc, System. out); } catch (IOException e) { System. err. println(e); } • Besides streams you can also output a document onto a java. io. Writer. However, it’s recommended that you use an Output. Stream because it’s generally not possible to determine the underlying encoding of a Writer and set the encoding declaration accordingly.
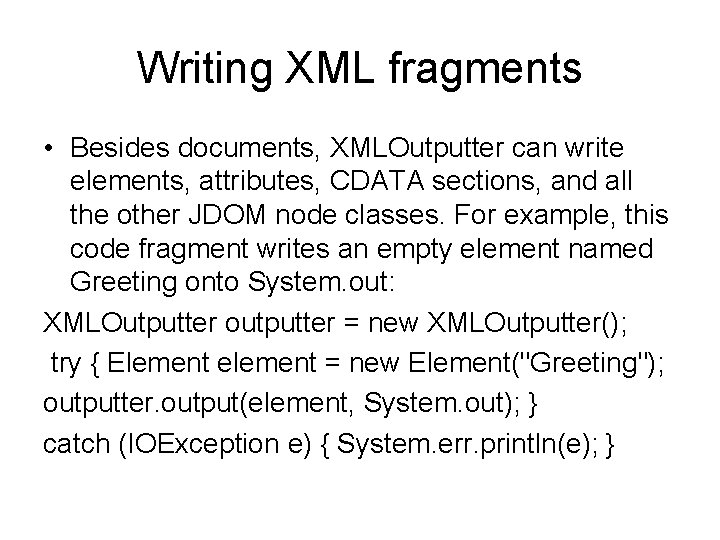
Writing XML fragments • Besides documents, XMLOutputter can write elements, attributes, CDATA sections, and all the other JDOM node classes. For example, this code fragment writes an empty element named Greeting onto System. out: XMLOutputter outputter = new XMLOutputter(); try { Element element = new Element("Greeting"); outputter. output(element, System. out); } catch (IOException e) { System. err. println(e); }
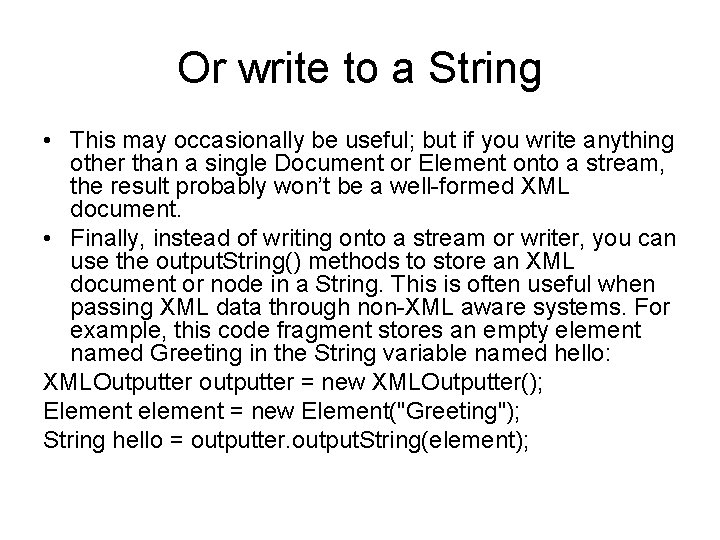
Or write to a String • This may occasionally be useful; but if you write anything other than a single Document or Element onto a stream, the result probably won’t be a well-formed XML document. • Finally, instead of writing onto a stream or writer, you can use the output. String() methods to store an XML document or node in a String. This is often useful when passing XML data through non-XML aware systems. For example, this code fragment stores an empty element named Greeting in the String variable named hello: XMLOutputter outputter = new XMLOutputter(); Element element = new Element("Greeting"); String hello = outputter. output. String(element);
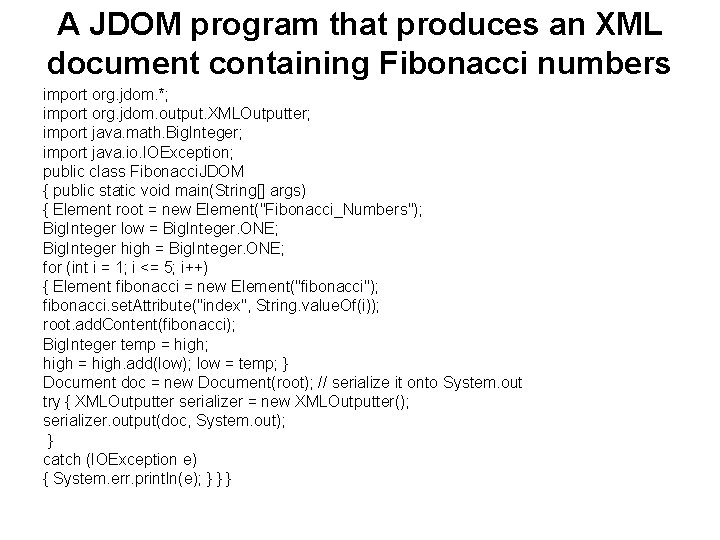
A JDOM program that produces an XML document containing Fibonacci numbers import org. jdom. *; import org. jdom. output. XMLOutputter; import java. math. Big. Integer; import java. io. IOException; public class Fibonacci. JDOM { public static void main(String[] args) { Element root = new Element("Fibonacci_Numbers"); Big. Integer low = Big. Integer. ONE; Big. Integer high = Big. Integer. ONE; for (int i = 1; i <= 5; i++) { Element fibonacci = new Element("fibonacci"); fibonacci. set. Attribute("index", String. value. Of(i)); root. add. Content(fibonacci); Big. Integer temp = high; high = high. add(low); low = temp; } Document doc = new Document(root); // serialize it onto System. out try { XMLOutputter serializer = new XMLOutputter(); serializer. output(doc, System. out); } catch (IOException e) { System. err. println(e); } } }
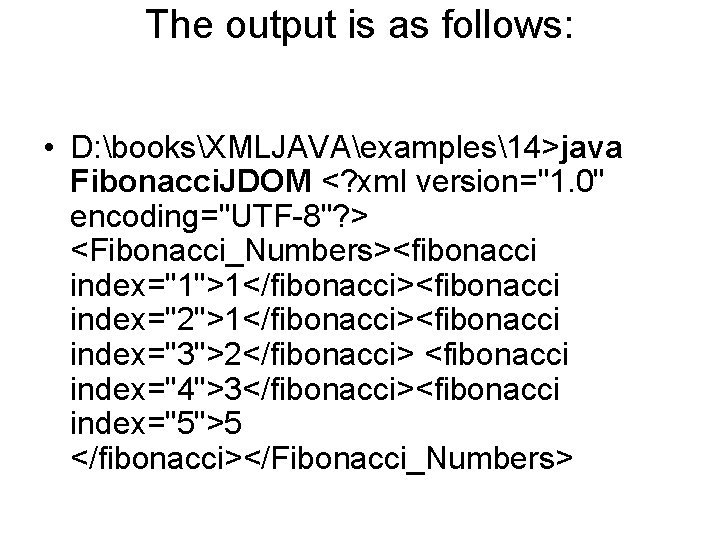
The output is as follows: • D: booksXMLJAVAexamples14>java Fibonacci. JDOM <? xml version="1. 0" encoding="UTF-8"? > <Fibonacci_Numbers><fibonacci index="1">1</fibonacci><fibonacci index="2">1</fibonacci><fibonacci index="3">2</fibonacci> <fibonacci index="4">3</fibonacci><fibonacci index="5">5 </fibonacci></Fibonacci_Numbers>
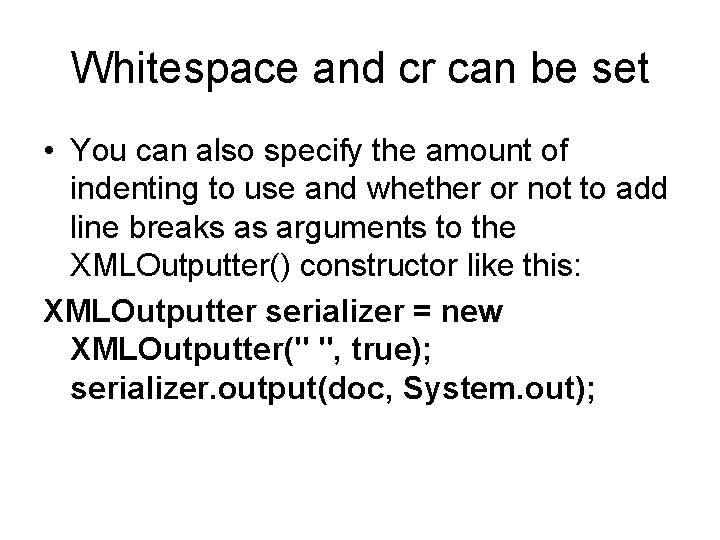
Whitespace and cr can be set • You can also specify the amount of indenting to use and whether or not to add line breaks as arguments to the XMLOutputter() constructor like this: XMLOutputter serializer = new XMLOutputter(" ", true); serializer. output(doc, System. out);
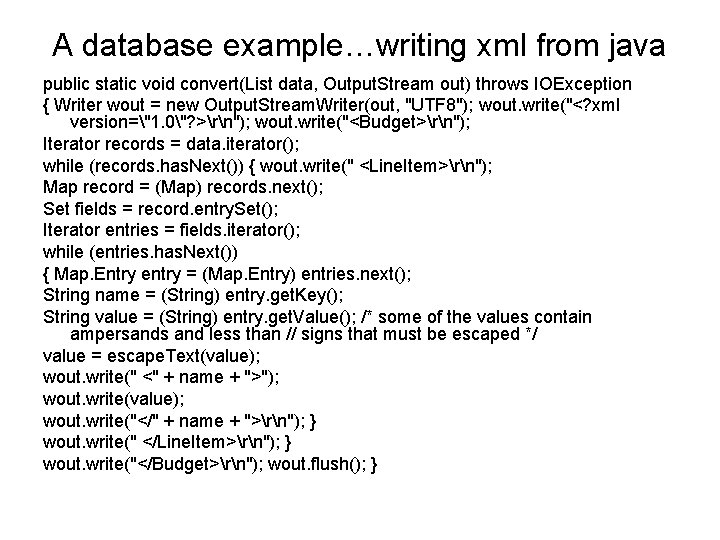
A database example…writing xml from java public static void convert(List data, Output. Stream out) throws IOException { Writer wout = new Output. Stream. Writer(out, "UTF 8"); wout. write("<? xml version="1. 0"? >rn"); wout. write("<Budget>rn"); Iterator records = data. iterator(); while (records. has. Next()) { wout. write(" <Line. Item>rn"); Map record = (Map) records. next(); Set fields = record. entry. Set(); Iterator entries = fields. iterator(); while (entries. has. Next()) { Map. Entry entry = (Map. Entry) entries. next(); String name = (String) entry. get. Key(); String value = (String) entry. get. Value(); /* some of the values contain ampersands and less than // signs that must be escaped */ value = escape. Text(value); wout. write(" <" + name + ">"); wout. write(value); wout. write("</" + name + ">rn"); } wout. write(" </Line. Item>rn"); } wout. write("</Budget>rn"); wout. flush(); }
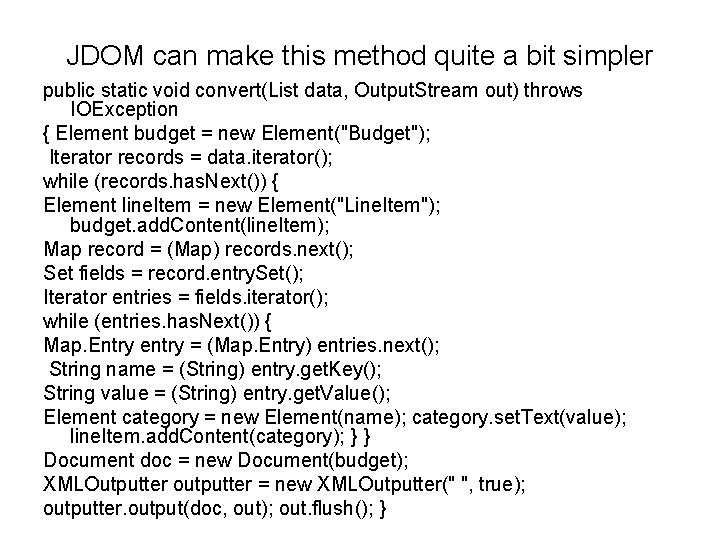
JDOM can make this method quite a bit simpler public static void convert(List data, Output. Stream out) throws IOException { Element budget = new Element("Budget"); Iterator records = data. iterator(); while (records. has. Next()) { Element line. Item = new Element("Line. Item"); budget. add. Content(line. Item); Map record = (Map) records. next(); Set fields = record. entry. Set(); Iterator entries = fields. iterator(); while (entries. has. Next()) { Map. Entry entry = (Map. Entry) entries. next(); String name = (String) entry. get. Key(); String value = (String) entry. get. Value(); Element category = new Element(name); category. set. Text(value); line. Item. add. Content(category); } } Document doc = new Document(budget); XMLOutputter outputter = new XMLOutputter(" ", true); outputter. output(doc, out); out. flush(); }
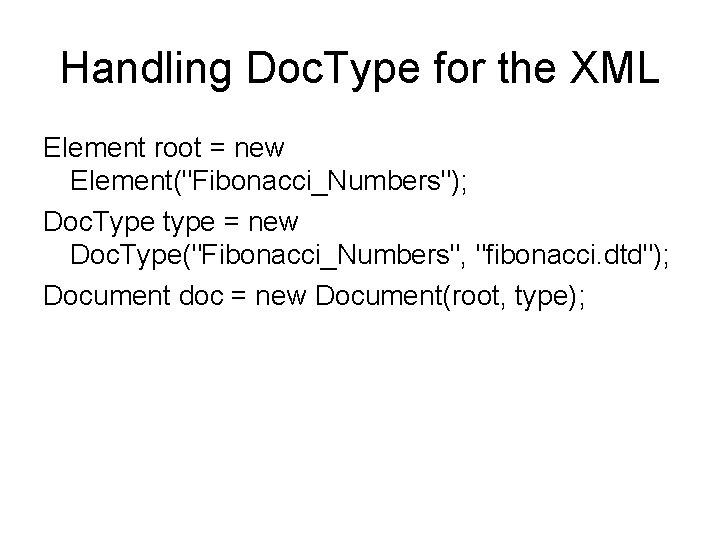
Handling Doc. Type for the XML Element root = new Element("Fibonacci_Numbers"); Doc. Type type = new Doc. Type("Fibonacci_Numbers", "fibonacci. dtd"); Document doc = new Document(root, type);
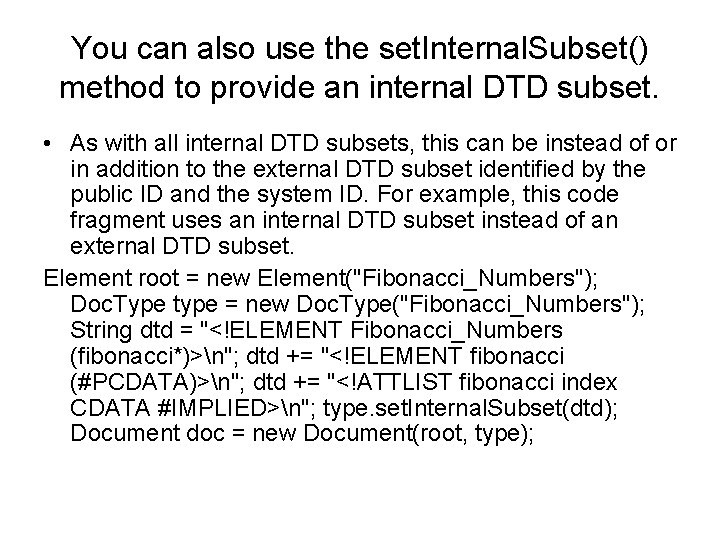
You can also use the set. Internal. Subset() method to provide an internal DTD subset. • As with all internal DTD subsets, this can be instead of or in addition to the external DTD subset identified by the public ID and the system ID. For example, this code fragment uses an internal DTD subset instead of an external DTD subset. Element root = new Element("Fibonacci_Numbers"); Doc. Type type = new Doc. Type("Fibonacci_Numbers"); String dtd = "<!ELEMENT Fibonacci_Numbers (fibonacci*)>n"; dtd += "<!ELEMENT fibonacci (#PCDATA)>n"; dtd += "<!ATTLIST fibonacci index CDATA #IMPLIED>n"; type. set. Internal. Subset(dtd); Document doc = new Document(root, type);
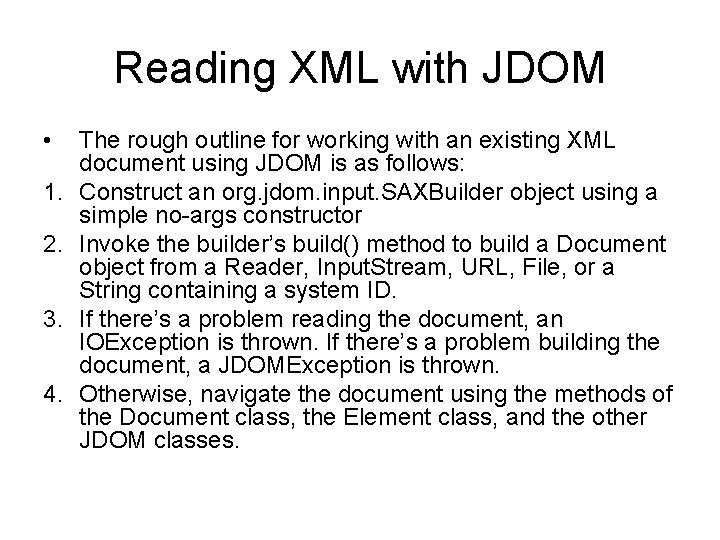
Reading XML with JDOM • 1. 2. 3. 4. The rough outline for working with an existing XML document using JDOM is as follows: Construct an org. jdom. input. SAXBuilder object using a simple no-args constructor Invoke the builder’s build() method to build a Document object from a Reader, Input. Stream, URL, File, or a String containing a system ID. If there’s a problem reading the document, an IOException is thrown. If there’s a problem building the document, a JDOMException is thrown. Otherwise, navigate the document using the methods of the Document class, the Element class, and the other JDOM classes.
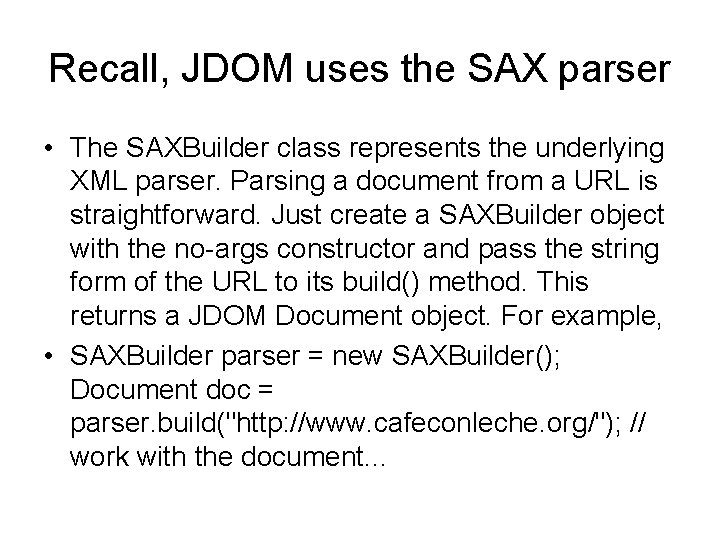
Recall, JDOM uses the SAX parser • The SAXBuilder class represents the underlying XML parser. Parsing a document from a URL is straightforward. Just create a SAXBuilder object with the no-args constructor and pass the string form of the URL to its build() method. This returns a JDOM Document object. For example, • SAXBuilder parser = new SAXBuilder(); Document doc = parser. build("http: //www. cafeconleche. org/"); // work with the document. . .
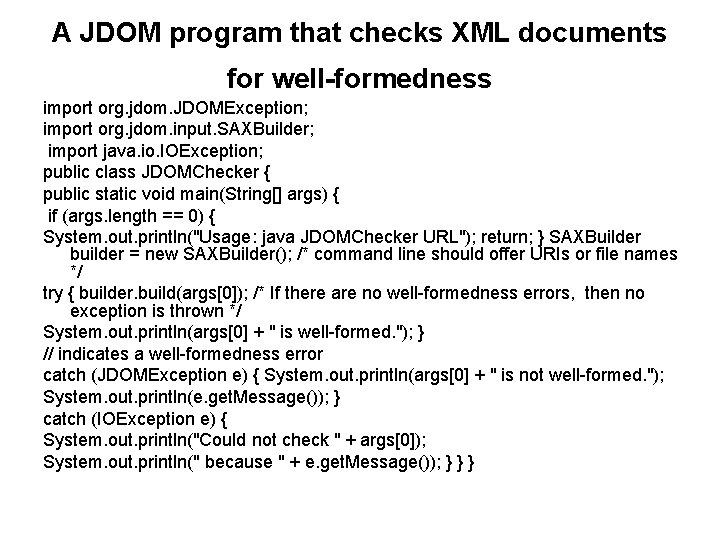
A JDOM program that checks XML documents for well-formedness import org. jdom. JDOMException; import org. jdom. input. SAXBuilder; import java. io. IOException; public class JDOMChecker { public static void main(String[] args) { if (args. length == 0) { System. out. println("Usage: java JDOMChecker URL"); return; } SAXBuilder builder = new SAXBuilder(); /* command line should offer URIs or file names */ try { builder. build(args[0]); /* If there are no well-formedness errors, then no exception is thrown */ System. out. println(args[0] + " is well-formed. "); } // indicates a well-formedness error catch (JDOMException e) { System. out. println(args[0] + " is not well-formed. "); System. out. println(e. get. Message()); } catch (IOException e) { System. out. println("Could not check " + args[0]); System. out. println(" because " + e. get. Message()); } } }
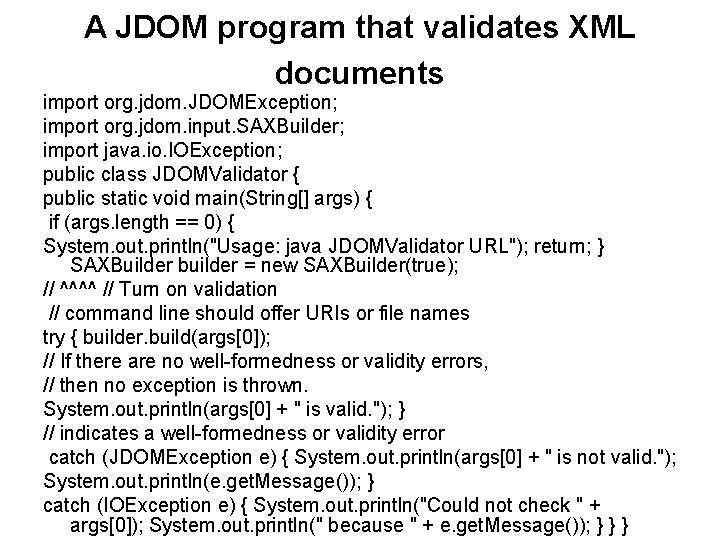
A JDOM program that validates XML documents import org. jdom. JDOMException; import org. jdom. input. SAXBuilder; import java. io. IOException; public class JDOMValidator { public static void main(String[] args) { if (args. length == 0) { System. out. println("Usage: java JDOMValidator URL"); return; } SAXBuilder builder = new SAXBuilder(true); // ^^^^ // Turn on validation // command line should offer URIs or file names try { builder. build(args[0]); // If there are no well-formedness or validity errors, // then no exception is thrown. System. out. println(args[0] + " is valid. "); } // indicates a well-formedness or validity error catch (JDOMException e) { System. out. println(args[0] + " is not valid. "); System. out. println(e. get. Message()); } catch (IOException e) { System. out. println("Could not check " + args[0]); System. out. println(" because " + e. get. Message()); } } }
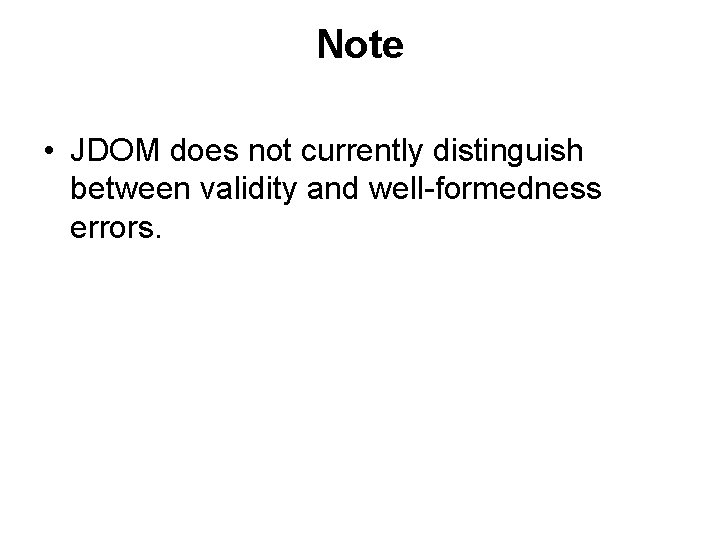
Note • JDOM does not currently distinguish between validity and well-formedness errors.
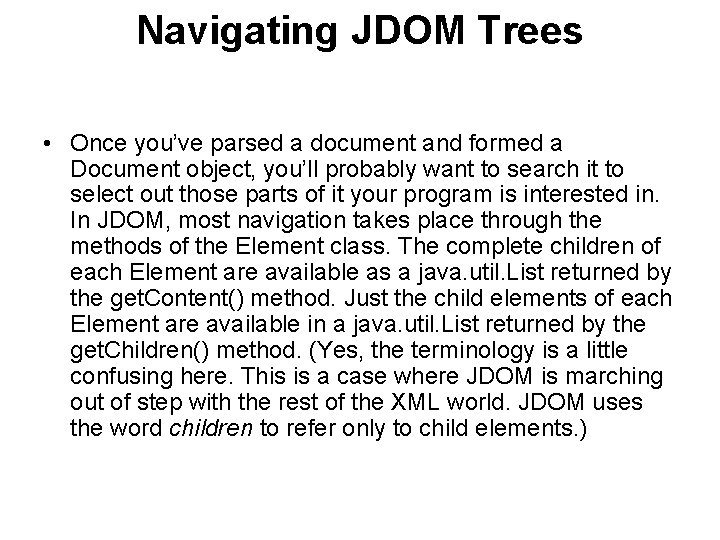
Navigating JDOM Trees • Once you’ve parsed a document and formed a Document object, you’ll probably want to search it to select out those parts of it your program is interested in. In JDOM, most navigation takes place through the methods of the Element class. The complete children of each Element are available as a java. util. List returned by the get. Content() method. Just the child elements of each Element are available in a java. util. List returned by the get. Children() method. (Yes, the terminology is a little confusing here. This is a case where JDOM is marching out of step with the rest of the XML world. JDOM uses the word children to refer only to child elements. )
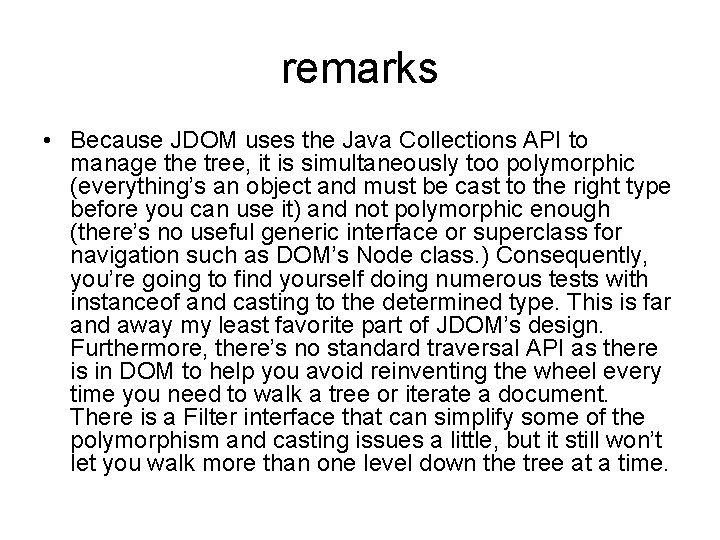
remarks • Because JDOM uses the Java Collections API to manage the tree, it is simultaneously too polymorphic (everything’s an object and must be cast to the right type before you can use it) and not polymorphic enough (there’s no useful generic interface or superclass for navigation such as DOM’s Node class. ) Consequently, you’re going to find yourself doing numerous tests with instanceof and casting to the determined type. This is far and away my least favorite part of JDOM’s design. Furthermore, there’s no standard traversal API as there is in DOM to help you avoid reinventing the wheel every time you need to walk a tree or iterate a document. There is a Filter interface that can simplify some of the polymorphism and casting issues a little, but it still won’t let you walk more than one level down the tree at a time.
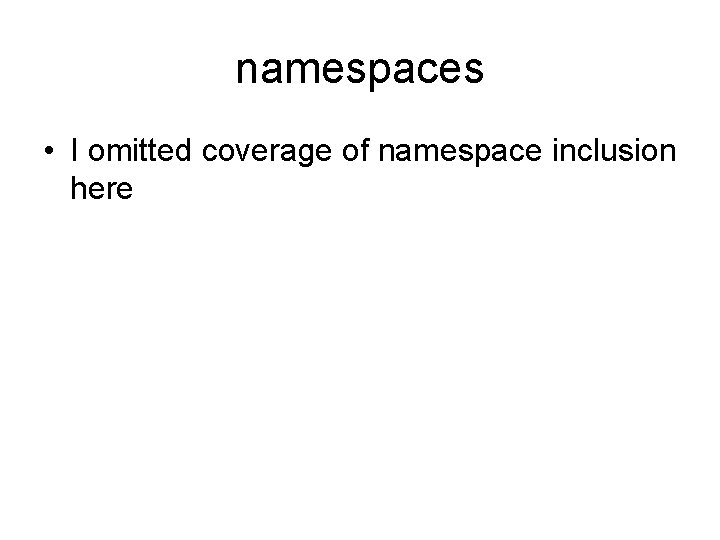
namespaces • I omitted coverage of namespace inclusion here
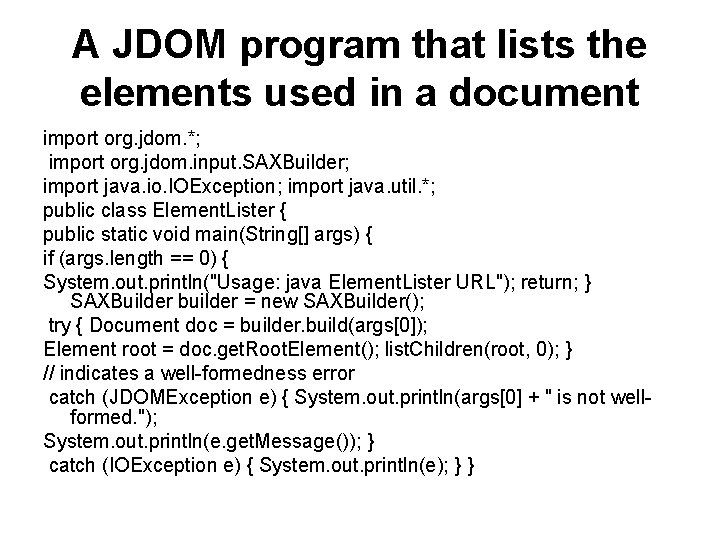
A JDOM program that lists the elements used in a document import org. jdom. *; import org. jdom. input. SAXBuilder; import java. io. IOException; import java. util. *; public class Element. Lister { public static void main(String[] args) { if (args. length == 0) { System. out. println("Usage: java Element. Lister URL"); return; } SAXBuilder builder = new SAXBuilder(); try { Document doc = builder. build(args[0]); Element root = doc. get. Root. Element(); list. Children(root, 0); } // indicates a well-formedness error catch (JDOMException e) { System. out. println(args[0] + " is not wellformed. "); System. out. println(e. get. Message()); } catch (IOException e) { System. out. println(e); } }
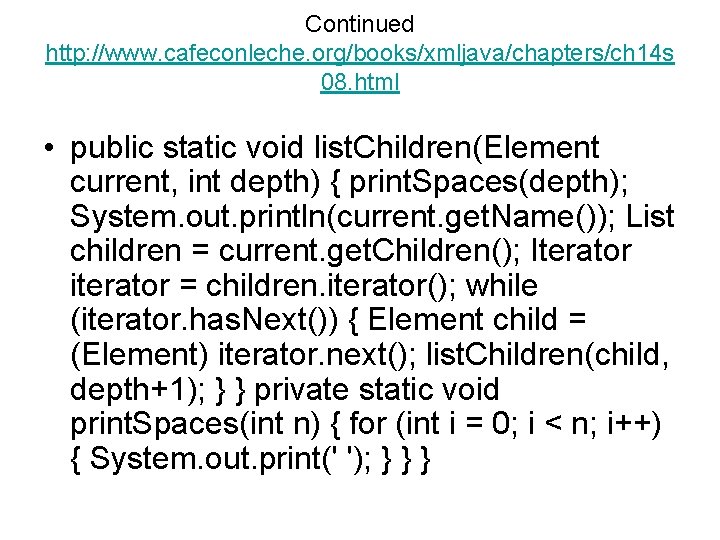
Continued http: //www. cafeconleche. org/books/xmljava/chapters/ch 14 s 08. html • public static void list. Children(Element current, int depth) { print. Spaces(depth); System. out. println(current. get. Name()); List children = current. get. Children(); Iterator iterator = children. iterator(); while (iterator. has. Next()) { Element child = (Element) iterator. next(); list. Children(child, depth+1); } } private static void print. Spaces(int n) { for (int i = 0; i < n; i++) { System. out. print(' '); } } }
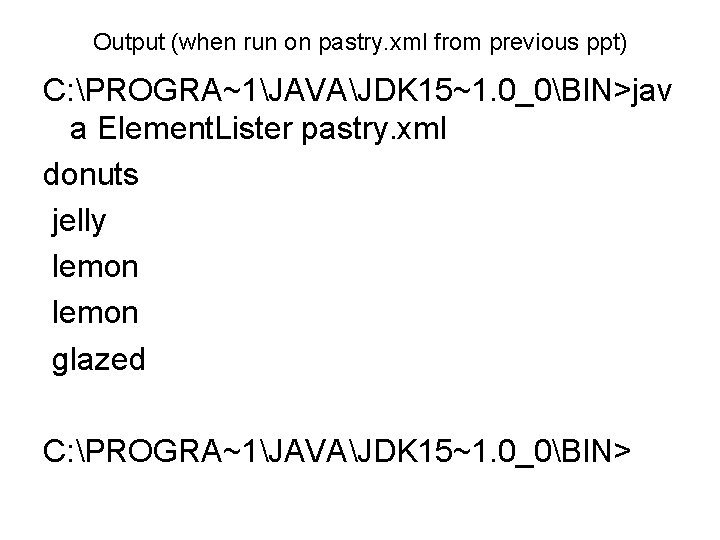
Output (when run on pastry. xml from previous ppt) C: PROGRA~1JAVAJDK 15~1. 0_0BIN>jav a Element. Lister pastry. xml donuts jelly lemon glazed C: PROGRA~1JAVAJDK 15~1. 0_0BIN>
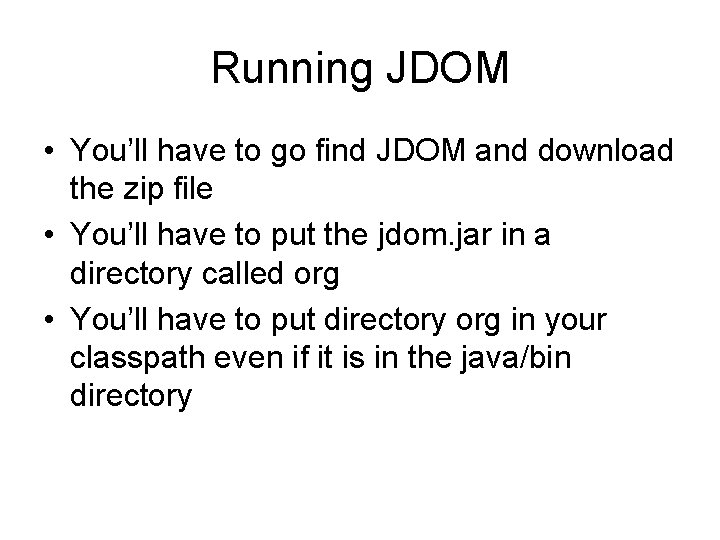
Running JDOM • You’ll have to go find JDOM and download the zip file • You’ll have to put the jdom. jar in a directory called org • You’ll have to put directory org in your classpath even if it is in the java/bin directory
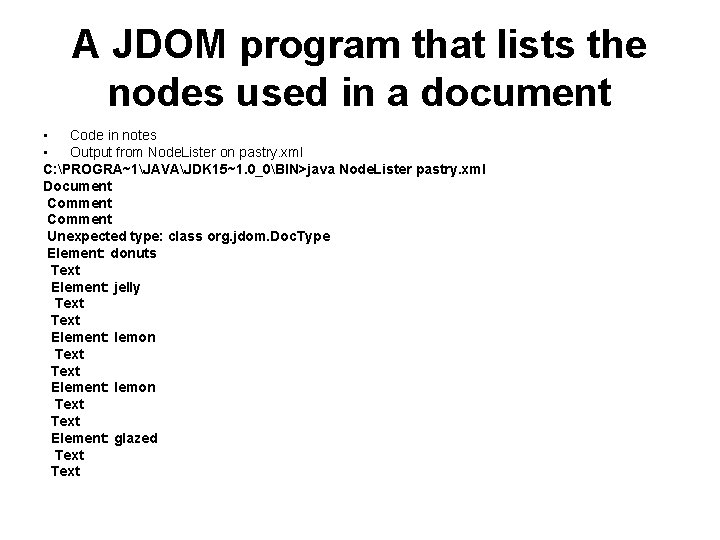
A JDOM program that lists the nodes used in a document • Code in notes • Output from Node. Lister on pastry. xml C: PROGRA~1JAVAJDK 15~1. 0_0BIN>java Node. Lister pastry. xml Document Comment Unexpected type: class org. jdom. Doc. Type Element: donuts Text Element: jelly Text Element: lemon Text Element: glazed Text
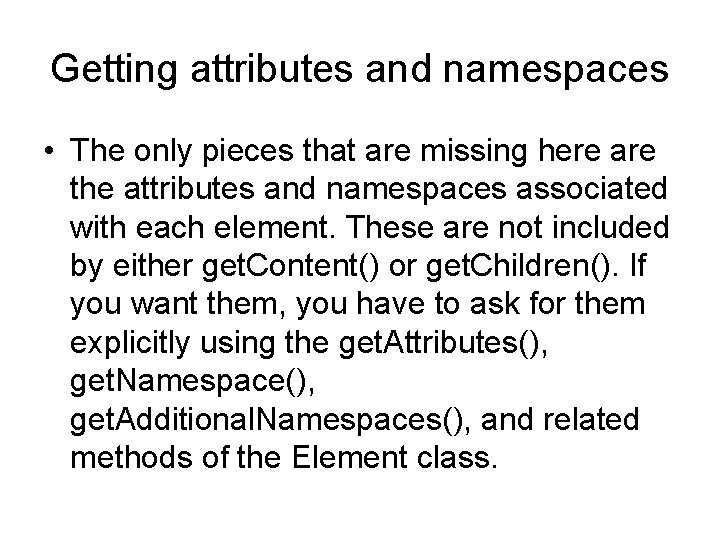
Getting attributes and namespaces • The only pieces that are missing here are the attributes and namespaces associated with each element. These are not included by either get. Content() or get. Children(). If you want them, you have to ask for them explicitly using the get. Attributes(), get. Namespace(), get. Additional. Namespaces(), and related methods of the Element class.
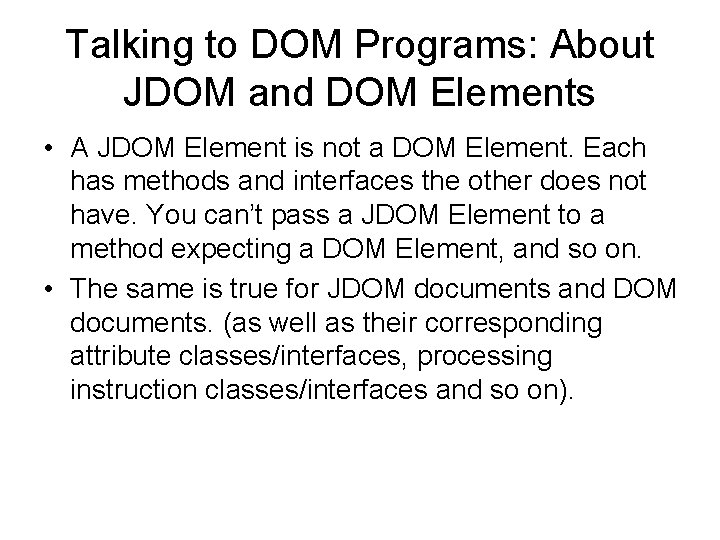
Talking to DOM Programs: About JDOM and DOM Elements • A JDOM Element is not a DOM Element. Each has methods and interfaces the other does not have. You can’t pass a JDOM Element to a method expecting a DOM Element, and so on. • The same is true for JDOM documents and DOM documents. (as well as their corresponding attribute classes/interfaces, processing instruction classes/interfaces and so on).
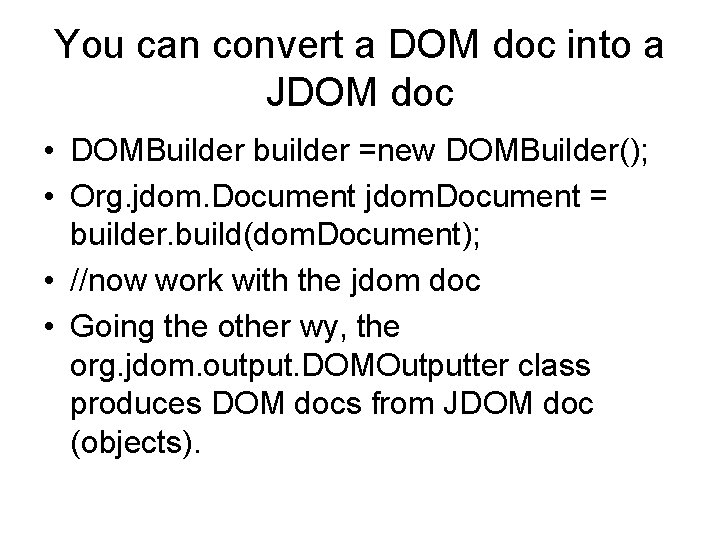
You can convert a DOM doc into a JDOM doc • DOMBuilder builder =new DOMBuilder(); • Org. jdom. Document = builder. build(dom. Document); • //now work with the jdom doc • Going the other wy, the org. jdom. output. DOMOutputter class produces DOM docs from JDOM doc (objects).
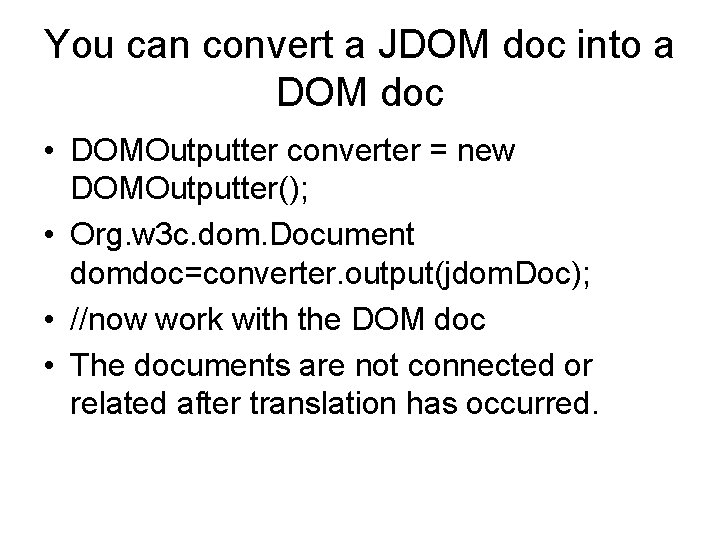
You can convert a JDOM doc into a DOM doc • DOMOutputter converter = new DOMOutputter(); • Org. w 3 c. dom. Document domdoc=converter. output(jdom. Doc); • //now work with the DOM doc • The documents are not connected or related after translation has occurred.
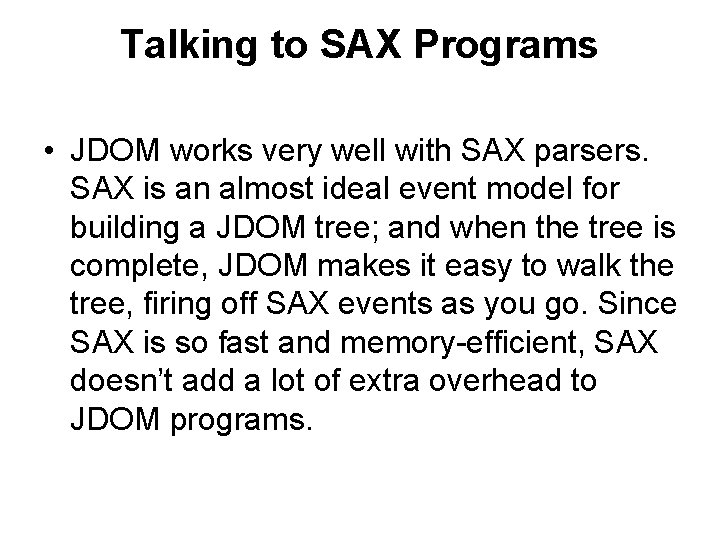
Talking to SAX Programs • JDOM works very well with SAX parsers. SAX is an almost ideal event model for building a JDOM tree; and when the tree is complete, JDOM makes it easy to walk the tree, firing off SAX events as you go. Since SAX is so fast and memory-efficient, SAX doesn’t add a lot of extra overhead to JDOM programs.
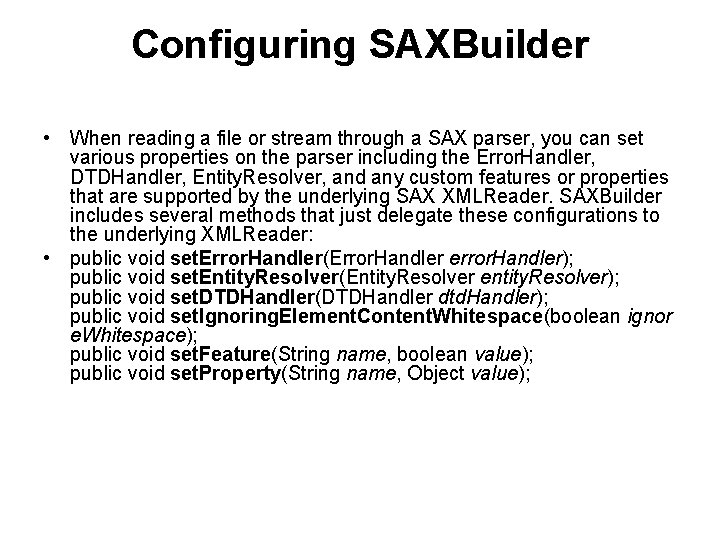
Configuring SAXBuilder • When reading a file or stream through a SAX parser, you can set various properties on the parser including the Error. Handler, DTDHandler, Entity. Resolver, and any custom features or properties that are supported by the underlying SAX XMLReader. SAXBuilder includes several methods that just delegate these configurations to the underlying XMLReader: • public void set. Error. Handler(Error. Handler error. Handler); public void set. Entity. Resolver(Entity. Resolver entity. Resolver); public void set. DTDHandler(DTDHandler dtd. Handler); public void set. Ignoring. Element. Content. Whitespace(boolean ignor e. Whitespace); public void set. Feature(String name, boolean value); public void set. Property(String name, Object value);
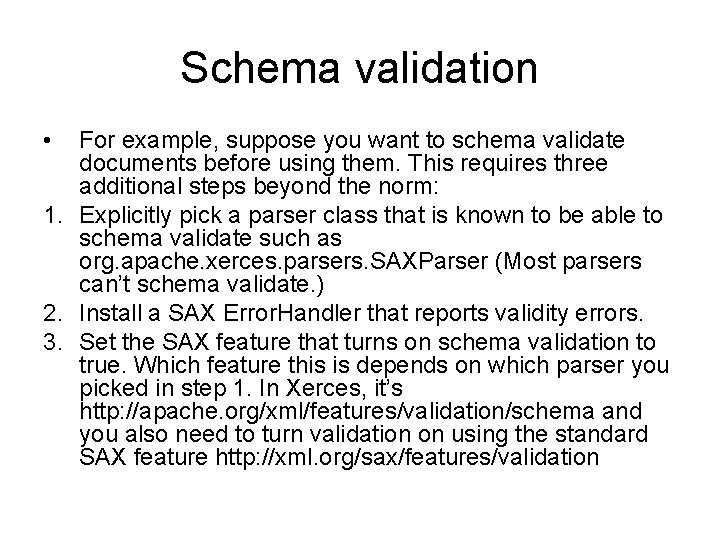
Schema validation • For example, suppose you want to schema validate documents before using them. This requires three additional steps beyond the norm: 1. Explicitly pick a parser class that is known to be able to schema validate such as org. apache. xerces. parsers. SAXParser (Most parsers can’t schema validate. ) 2. Install a SAX Error. Handler that reports validity errors. 3. Set the SAX feature that turns on schema validation to true. Which feature this is depends on which parser you picked in step 1. In Xerces, it’s http: //apache. org/xml/features/validation/schema and you also need to turn validation on using the standard SAX feature http: //xml. org/sax/features/validation
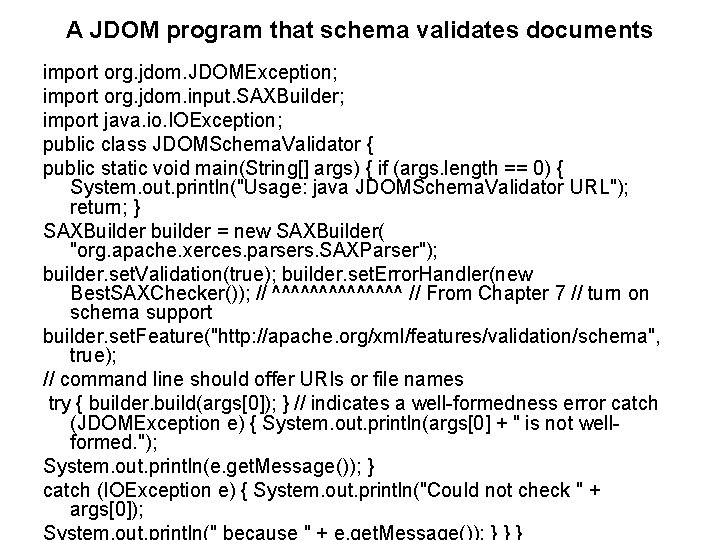
A JDOM program that schema validates documents import org. jdom. JDOMException; import org. jdom. input. SAXBuilder; import java. io. IOException; public class JDOMSchema. Validator { public static void main(String[] args) { if (args. length == 0) { System. out. println("Usage: java JDOMSchema. Validator URL"); return; } SAXBuilder builder = new SAXBuilder( "org. apache. xerces. parsers. SAXParser"); builder. set. Validation(true); builder. set. Error. Handler(new Best. SAXChecker()); // ^^^^^^^ // From Chapter 7 // turn on schema support builder. set. Feature("http: //apache. org/xml/features/validation/schema", true); // command line should offer URIs or file names try { builder. build(args[0]); } // indicates a well-formedness error catch (JDOMException e) { System. out. println(args[0] + " is not wellformed. "); System. out. println(e. get. Message()); } catch (IOException e) { System. out. println("Could not check " + args[0]); System. out. println(" because " + e. get. Message()); } } }
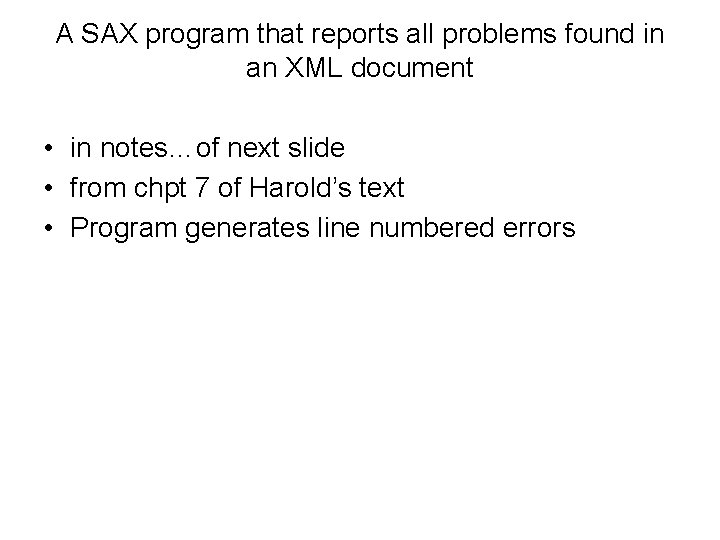
A SAX program that reports all problems found in an XML document • in notes…of next slide • from chpt 7 of Harold’s text • Program generates line numbered errors
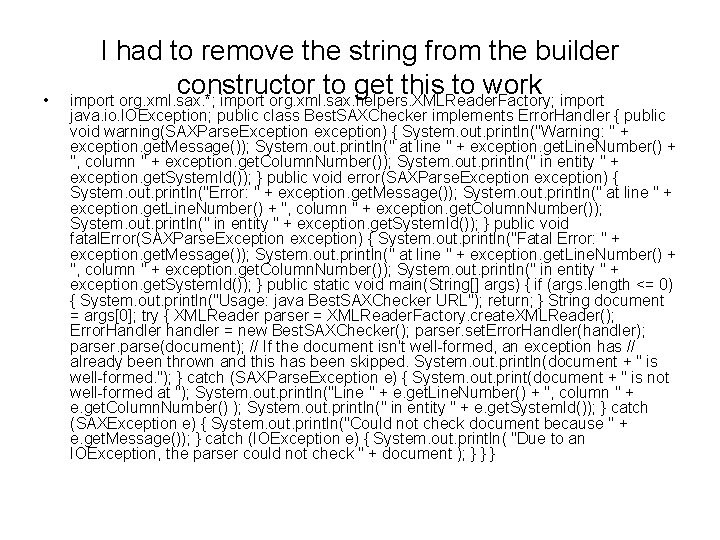
• I had to remove the string from the builder constructor to get this to work import org. xml. sax. *; import org. xml. sax. helpers. XMLReader. Factory; import java. io. IOException; public class Best. SAXChecker implements Error. Handler { public void warning(SAXParse. Exception exception) { System. out. println("Warning: " + exception. get. Message()); System. out. println(" at line " + exception. get. Line. Number() + ", column " + exception. get. Column. Number()); System. out. println(" in entity " + exception. get. System. Id()); } public void error(SAXParse. Exception exception) { System. out. println("Error: " + exception. get. Message()); System. out. println(" at line " + exception. get. Line. Number() + ", column " + exception. get. Column. Number()); System. out. println(" in entity " + exception. get. System. Id()); } public void fatal. Error(SAXParse. Exception exception) { System. out. println("Fatal Error: " + exception. get. Message()); System. out. println(" at line " + exception. get. Line. Number() + ", column " + exception. get. Column. Number()); System. out. println(" in entity " + exception. get. System. Id()); } public static void main(String[] args) { if (args. length <= 0) { System. out. println("Usage: java Best. SAXChecker URL"); return; } String document = args[0]; try { XMLReader parser = XMLReader. Factory. create. XMLReader(); Error. Handler handler = new Best. SAXChecker(); parser. set. Error. Handler(handler); parser. parse(document); // If the document isn't well-formed, an exception has // already been thrown and this has been skipped. System. out. println(document + " is well-formed. "); } catch (SAXParse. Exception e) { System. out. print(document + " is not well-formed at "); System. out. println("Line " + e. get. Line. Number() + ", column " + e. get. Column. Number() ); System. out. println(" in entity " + e. get. System. Id()); } catch (SAXException e) { System. out. println("Could not check document because " + e. get. Message()); } catch (IOException e) { System. out. println( "Due to an IOException, the parser could not check " + document ); } } }
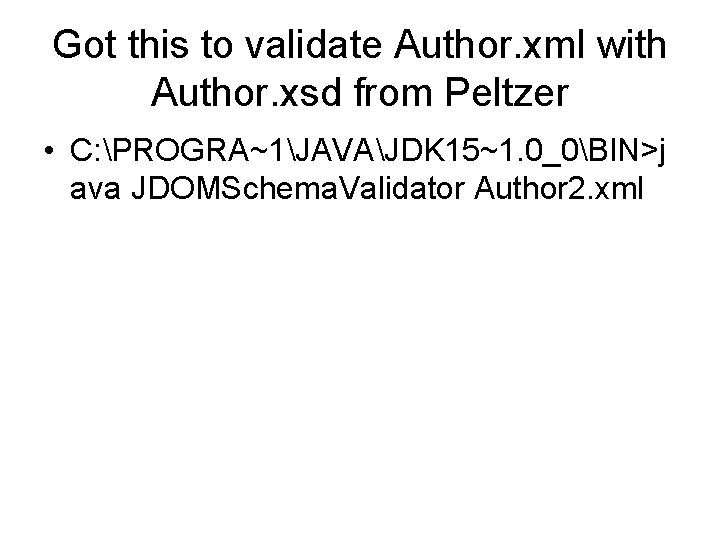
Got this to validate Author. xml with Author. xsd from Peltzer • C: PROGRA~1JAVAJDK 15~1. 0_0BIN>j ava JDOMSchema. Validator Author 2. xml
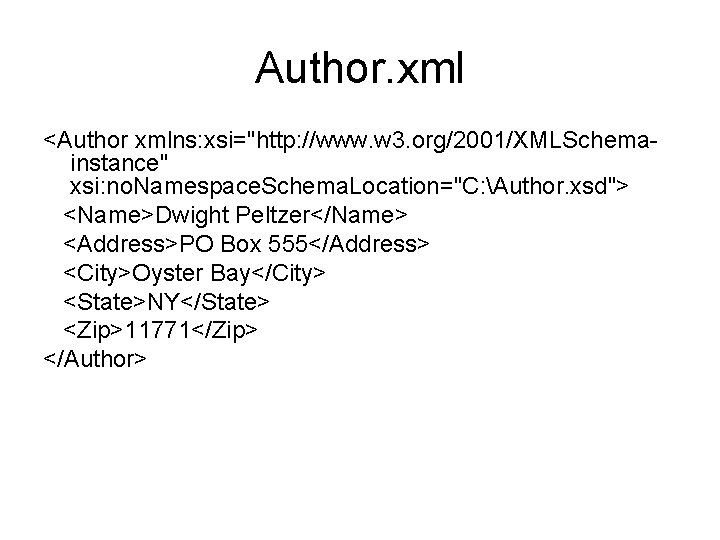
Author. xml <Author xmlns: xsi="http: //www. w 3. org/2001/XMLSchemainstance" xsi: no. Namespace. Schema. Location="C: Author. xsd"> <Name>Dwight Peltzer</Name> <Address>PO Box 555</Address> <City>Oyster Bay</City> <State>NY</State> <Zip>11771</Zip> </Author>
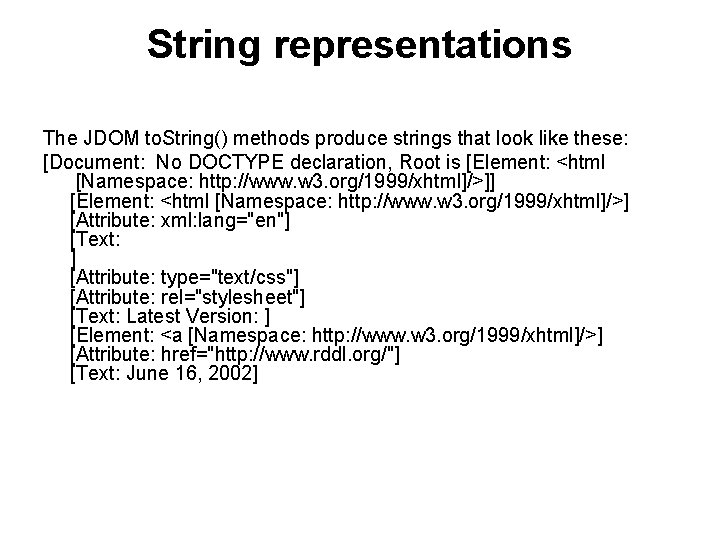
String representations The JDOM to. String() methods produce strings that look like these: [Document: No DOCTYPE declaration, Root is [Element: <html [Namespace: http: //www. w 3. org/1999/xhtml]/>]] [Element: <html [Namespace: http: //www. w 3. org/1999/xhtml]/>] [Attribute: xml: lang="en"] [Text: ] [Attribute: type="text/css"] [Attribute: rel="stylesheet"] [Text: Latest Version: ] [Element: <a [Namespace: http: //www. w 3. org/1999/xhtml]/>] [Attribute: href="http: //www. rddl. org/"] [Text: June 16, 2002]
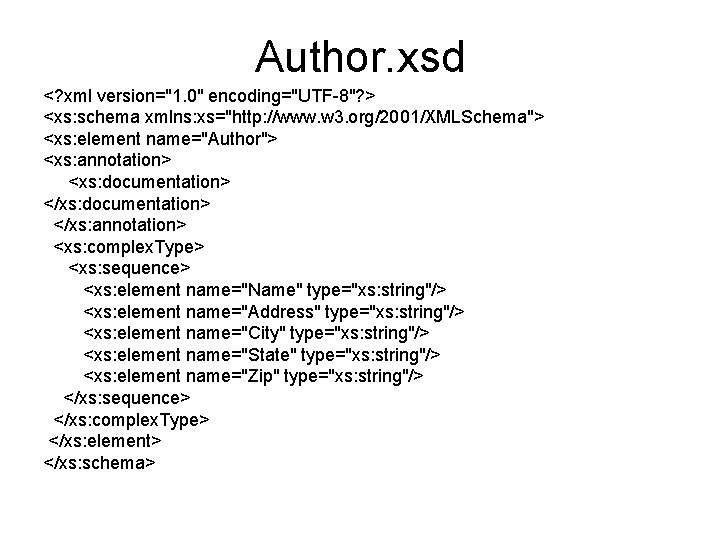
Author. xsd <? xml version="1. 0" encoding="UTF-8"? > <xs: schema xmlns: xs="http: //www. w 3. org/2001/XMLSchema"> <xs: element name="Author"> <xs: annotation> <xs: documentation> </xs: documentation> </xs: annotation> <xs: complex. Type> <xs: sequence> <xs: element name="Name" type="xs: string"/> <xs: element name="Address" type="xs: string"/> <xs: element name="City" type="xs: string"/> <xs: element name="State" type="xs: string"/> <xs: element name="Zip" type="xs: string"/> </xs: sequence> </xs: complex. Type> </xs: element> </xs: schema>
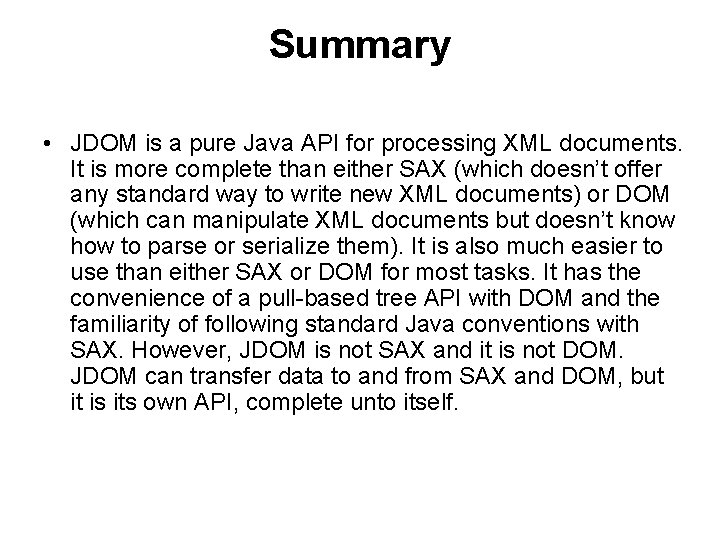
Summary • JDOM is a pure Java API for processing XML documents. It is more complete than either SAX (which doesn’t offer any standard way to write new XML documents) or DOM (which can manipulate XML documents but doesn’t know how to parse or serialize them). It is also much easier to use than either SAX or DOM for most tasks. It has the convenience of a pull-based tree API with DOM and the familiarity of following standard Java conventions with SAX. However, JDOM is not SAX and it is not DOM. JDOM can transfer data to and from SAX and DOM, but it is its own API, complete unto itself.
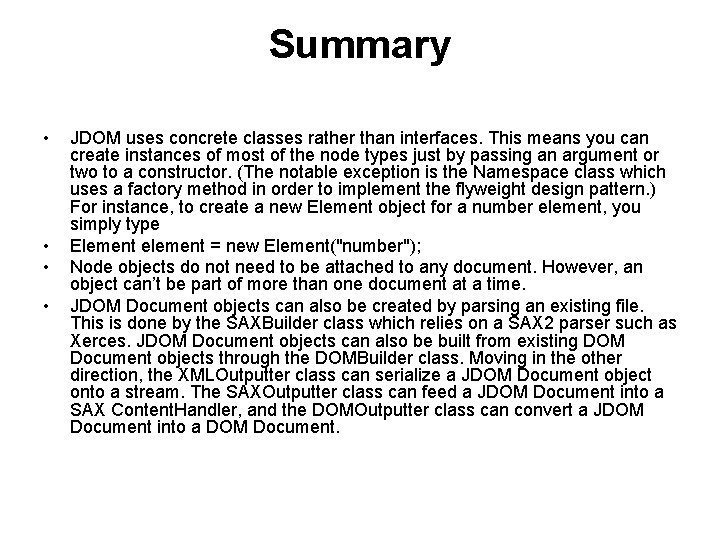
Summary • • JDOM uses concrete classes rather than interfaces. This means you can create instances of most of the node types just by passing an argument or two to a constructor. (The notable exception is the Namespace class which uses a factory method in order to implement the flyweight design pattern. ) For instance, to create a new Element object for a number element, you simply type Element element = new Element("number"); Node objects do not need to be attached to any document. However, an object can’t be part of more than one document at a time. JDOM Document objects can also be created by parsing an existing file. This is done by the SAXBuilder class which relies on a SAX 2 parser such as Xerces. JDOM Document objects can also be built from existing DOM Document objects through the DOMBuilder class. Moving in the other direction, the XMLOutputter class can serialize a JDOM Document object onto a stream. The SAXOutputter class can feed a JDOM Document into a SAX Content. Handler, and the DOMOutputter class can convert a JDOM Document into a DOM Document.
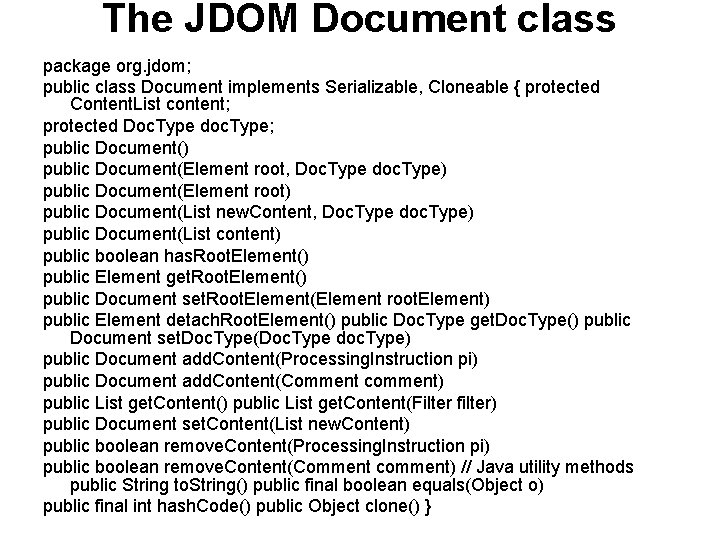
The JDOM Document class package org. jdom; public class Document implements Serializable, Cloneable { protected Content. List content; protected Doc. Type doc. Type; public Document() public Document(Element root, Doc. Type doc. Type) public Document(Element root) public Document(List new. Content, Doc. Type doc. Type) public Document(List content) public boolean has. Root. Element() public Element get. Root. Element() public Document set. Root. Element(Element root. Element) public Element detach. Root. Element() public Doc. Type get. Doc. Type() public Document set. Doc. Type(Doc. Type doc. Type) public Document add. Content(Processing. Instruction pi) public Document add. Content(Comment comment) public List get. Content(Filter filter) public Document set. Content(List new. Content) public boolean remove. Content(Processing. Instruction pi) public boolean remove. Content(Comment comment) // Java utility methods public String to. String() public final boolean equals(Object o) public final int hash. Code() public Object clone() }
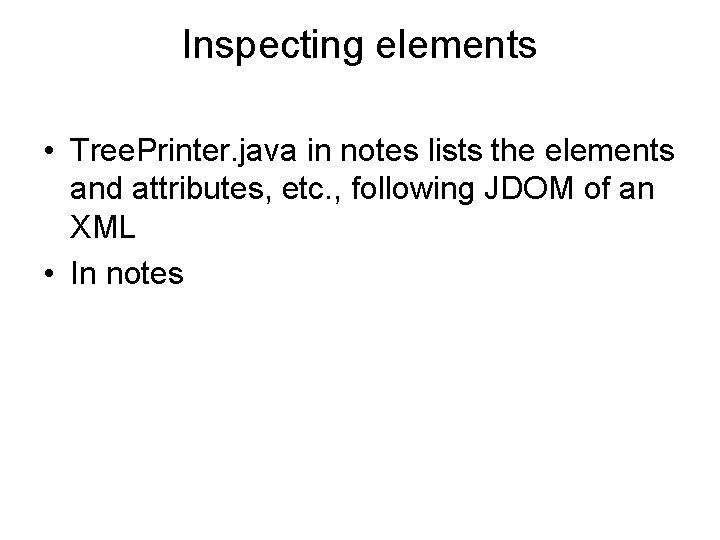
Inspecting elements • Tree. Printer. java in notes lists the elements and attributes, etc. , following JDOM of an XML • In notes
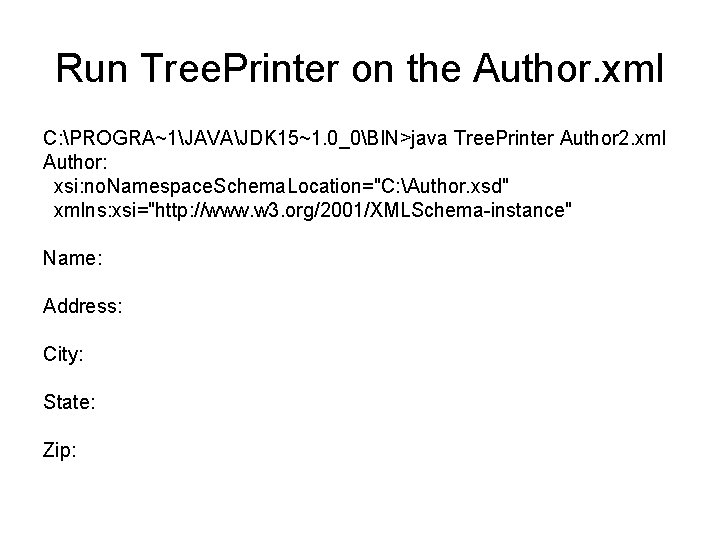
Run Tree. Printer on the Author. xml C: PROGRA~1JAVAJDK 15~1. 0_0BIN>java Tree. Printer Author 2. xml Author: xsi: no. Namespace. Schema. Location="C: Author. xsd" xmlns: xsi="http: //www. w 3. org/2001/XMLSchema-instance" Name: Address: City: State: Zip:
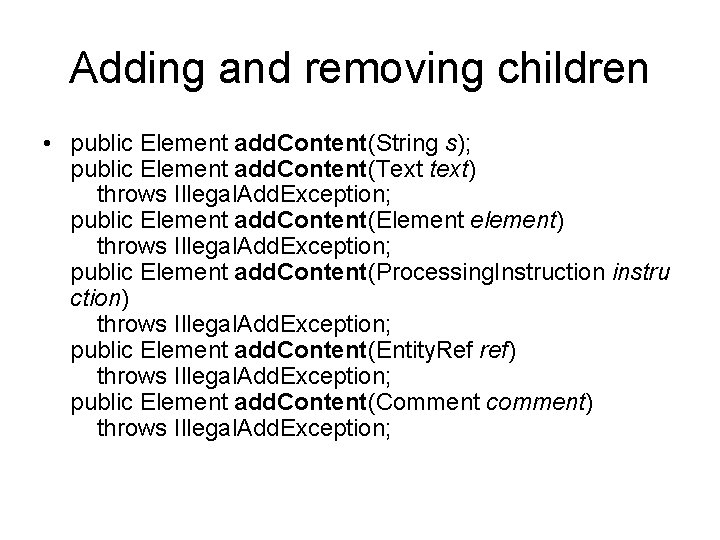
Adding and removing children • public Element add. Content(String s); public Element add. Content(Text text) throws Illegal. Add. Exception; public Element add. Content(Element element) throws Illegal. Add. Exception; public Element add. Content(Processing. Instruction instru ction) throws Illegal. Add. Exception; public Element add. Content(Entity. Ref ref) throws Illegal. Add. Exception; public Element add. Content(Comment comment) throws Illegal. Add. Exception;
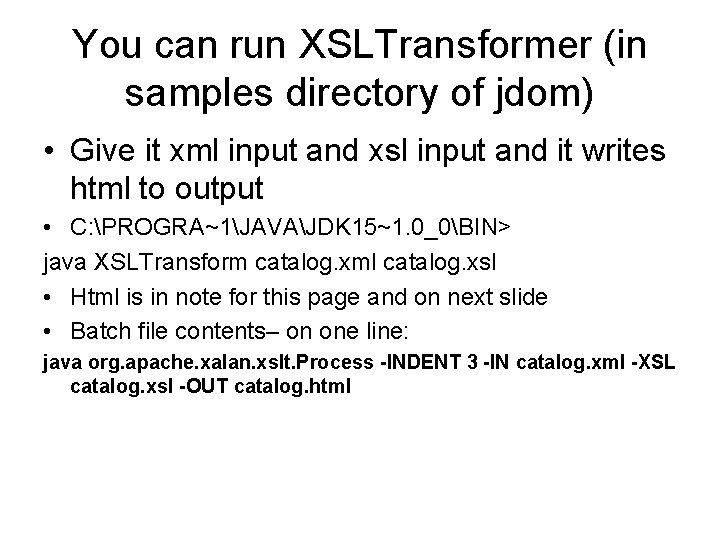
You can run XSLTransformer (in samples directory of jdom) • Give it xml input and xsl input and it writes html to output • C: PROGRA~1JAVAJDK 15~1. 0_0BIN> java XSLTransform catalog. xml catalog. xsl • Html is in note for this page and on next slide • Batch file contents– on one line: java org. apache. xalan. xslt. Process -INDENT 3 -IN catalog. xml -XSL catalog. xsl -OUT catalog. html
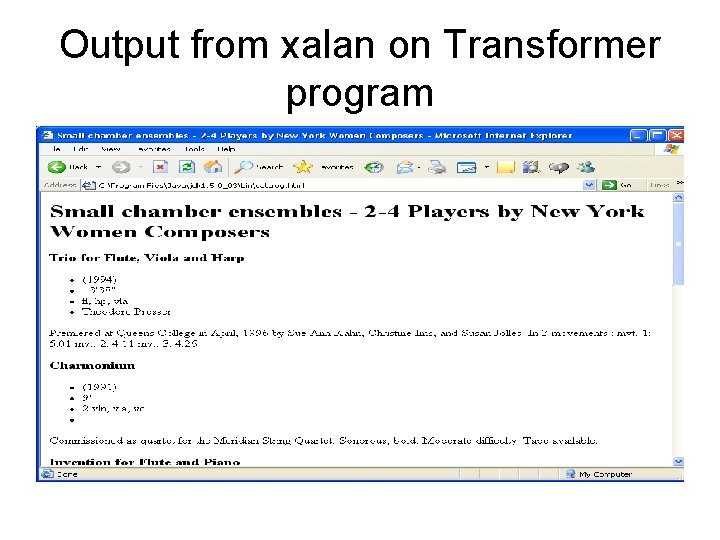
Output from xalan on Transformer program
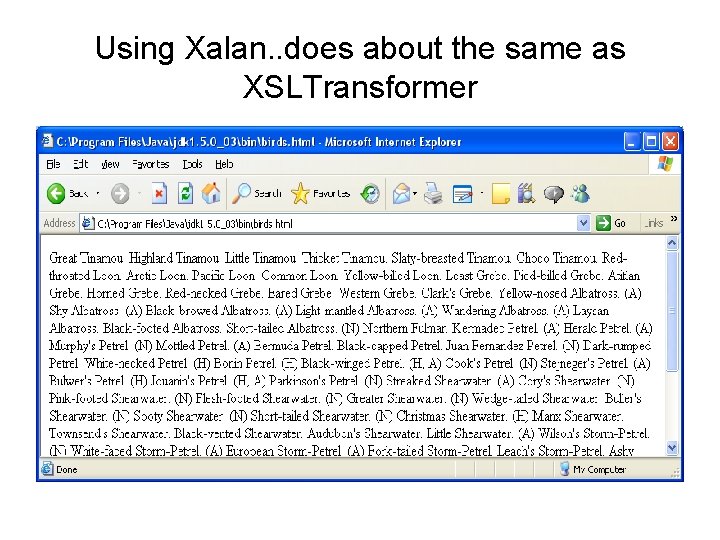
Using Xalan. . does about the same as XSLTransformer
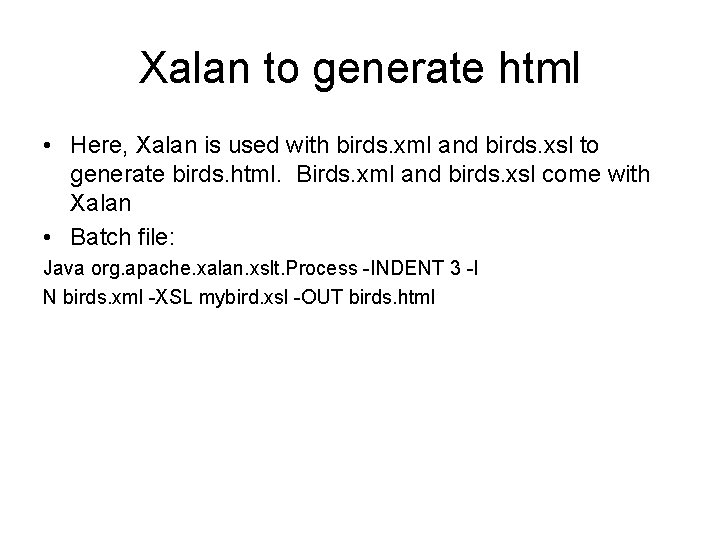
Xalan to generate html • Here, Xalan is used with birds. xml and birds. xsl to generate birds. html. Birds. xml and birds. xsl come with Xalan • Batch file: Java org. apache. xalan. xslt. Process -INDENT 3 -I N birds. xml -XSL mybird. xsl -OUT birds. html
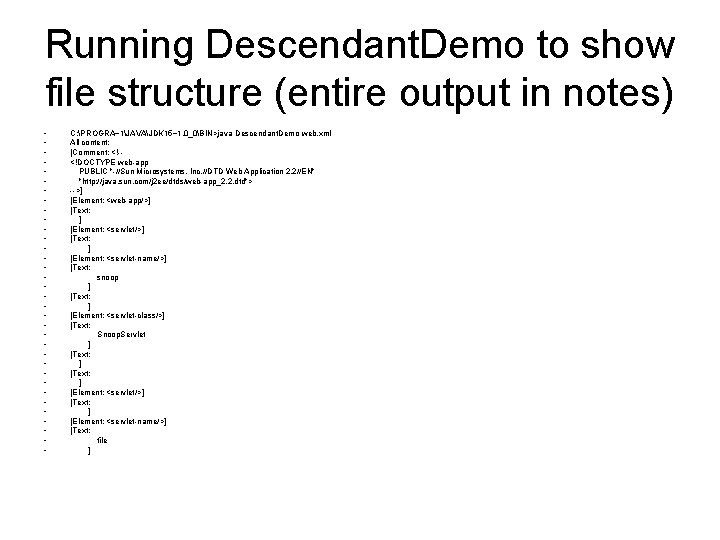
Running Descendant. Demo to show file structure (entire output in notes) • • • • • • • • • C: PROGRA~1JAVAJDK 15~1. 0_0BIN>java Descendant. Demo web. xml All content: [Comment: <!-<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc. //DTD Web Application 2. 2//EN" "http: //java. sun. com/j 2 ee/dtds/web-app_2. 2. dtd"> -->] [Element: <web-app/>] [Text: ] [Element: <servlet/>] [Text: ] [Element: <servlet-name/>] [Text: snoop ] [Text: ] [Element: <servlet-class/>] [Text: Snoop. Servlet ] [Text: ] [Element: <servlet/>] [Text: ] [Element: <servlet-name/>] [Text: file ]
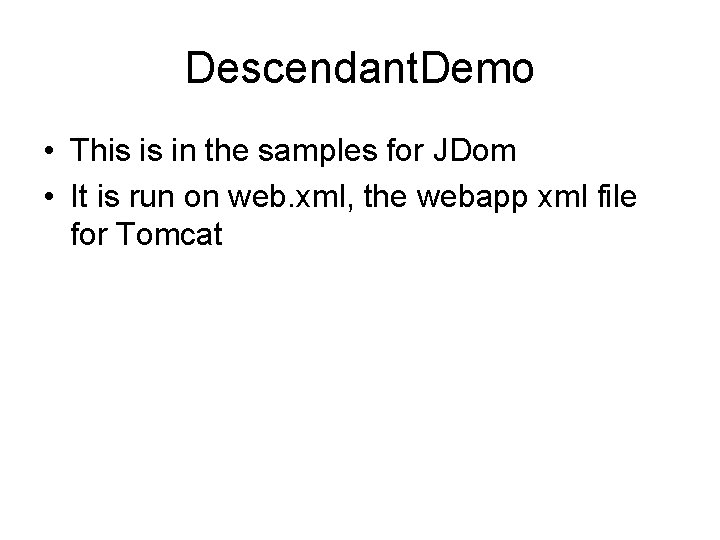
Descendant. Demo • This is in the samples for JDom • It is run on web. xml, the webapp xml file for Tomcat
![SAXBuilder Demo Usage java SAXBuilder Demo XML document filename expand Entities SAXBuilder. Demo • "Usage: java SAXBuilder. Demo " + "[XML document filename] ([expand. Entities]](https://slidetodoc.com/presentation_image_h/3524ffee0431820bdee1ca8b6a3f825b/image-63.jpg)
SAXBuilder. Demo • "Usage: java SAXBuilder. Demo " + "[XML document filename] ([expand. Entities] [SAX Driver Class])"); • Builds a JDOM from SAX parser • Provides a template for setting up JDOM • Code in notes
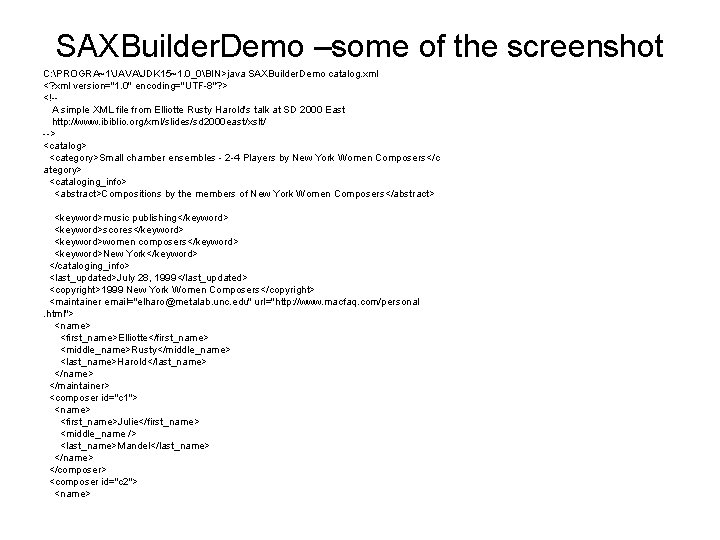
SAXBuilder. Demo –some of the screenshot C: PROGRA~1JAVAJDK 15~1. 0_0BIN>java SAXBuilder. Demo catalog. xml <? xml version="1. 0" encoding="UTF-8"? > <!- A simple XML file from Elliotte Rusty Harold's talk at SD 2000 East http: //www. ibiblio. org/xml/slides/sd 2000 east/xslt/ --> <catalog> <category>Small chamber ensembles - 2 -4 Players by New York Women Composers</c ategory> <cataloging_info> <abstract>Compositions by the members of New York Women Composers</abstract> <keyword>music publishing</keyword> <keyword>scores</keyword> <keyword>women composers</keyword> <keyword>New York</keyword> </cataloging_info> <last_updated>July 28, 1999</last_updated> <copyright>1999 New York Women Composers</copyright> <maintainer email="elharo@metalab. unc. edu" url="http: //www. macfaq. com/personal. html"> <name> <first_name>Elliotte</first_name> <middle_name>Rusty</middle_name> <last_name>Harold</last_name> </name> </maintainer> <composer id="c 1"> <name> <first_name>Julie</first_name> <middle_name /> <last_name>Mandel</last_name> </name> </composer> <composer id="c 2"> <name>
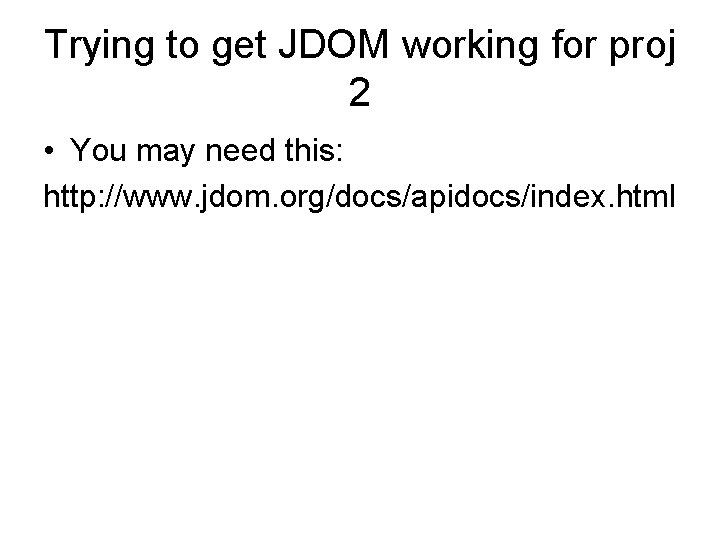
Trying to get JDOM working for proj 2 • You may need this: http: //www. jdom. org/docs/apidocs/index. html
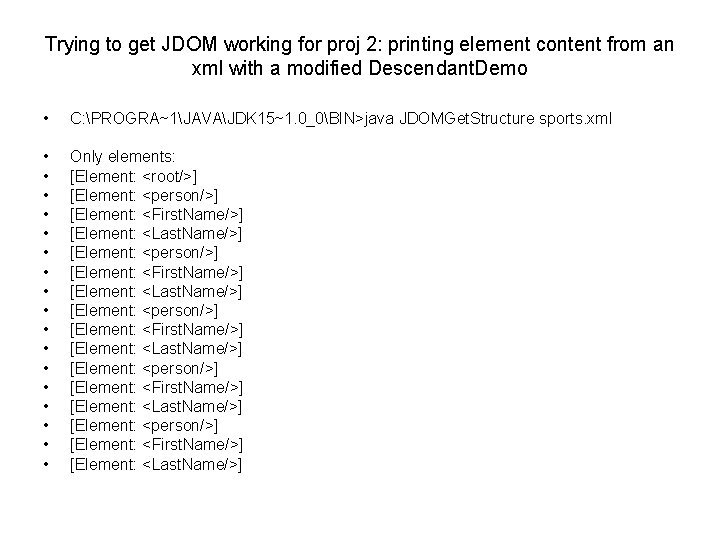
Trying to get JDOM working for proj 2: printing element content from an xml with a modified Descendant. Demo • C: PROGRA~1JAVAJDK 15~1. 0_0BIN>java JDOMGet. Structure sports. xml • • • • • Only elements: [Element: <root/>] [Element: <person/>] [Element: <First. Name/>] [Element: <Last. Name/>]
![My code with imports omitted public class JDOMGet Structure public static void mainString My code (with imports omitted) public class JDOMGet. Structure { public static void main(String[]](https://slidetodoc.com/presentation_image_h/3524ffee0431820bdee1ca8b6a3f825b/image-67.jpg)
My code (with imports omitted) public class JDOMGet. Structure { public static void main(String[] args) throws Exception { if (args. length != 1) { System. err. println("Usage: java Descendant. Demo [web. xml]"); return; } SAXBuilder builder = new SAXBuilder(); Document doc = builder. build(args[0]); System. out. println(); System. out. println("Only elements: "); Iterator itr = doc. get. Descendants(new Element. Filter()); while (itr. has. Next()) { Content c = (Content) itr. next(); System. out. println(c); } } }