Java Threads Multitasking and Multithreading Multitasking refers to
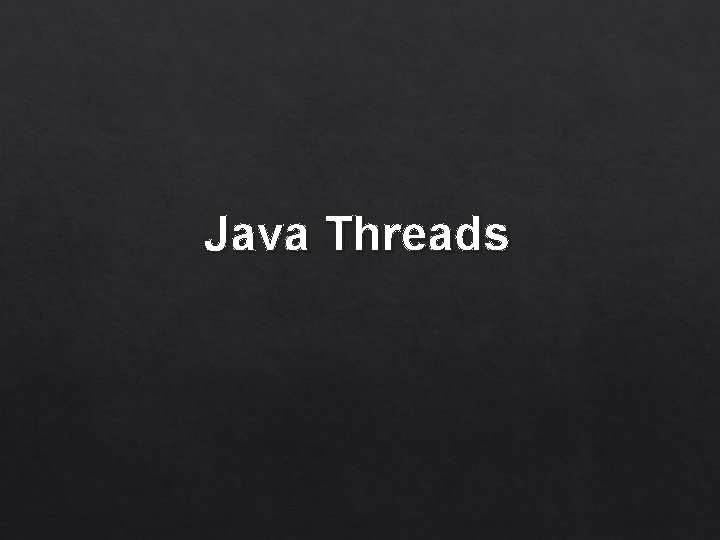
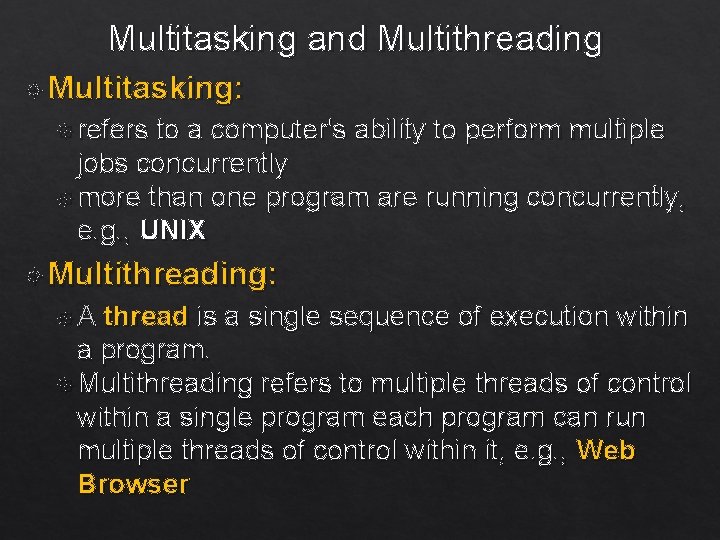
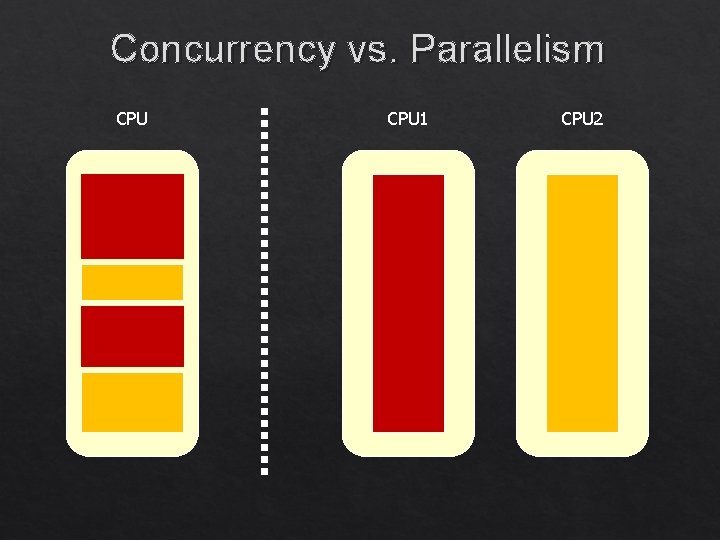
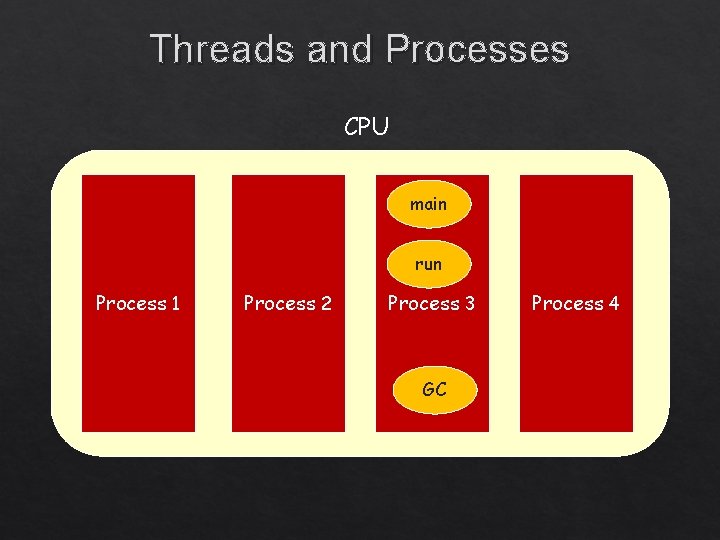
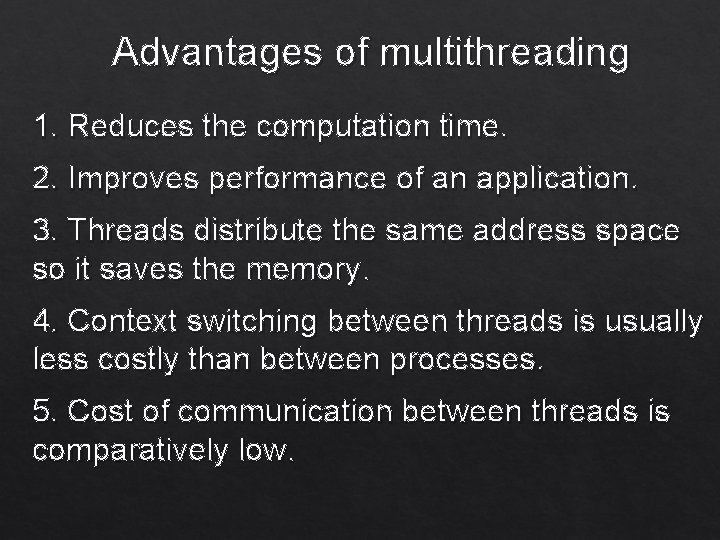
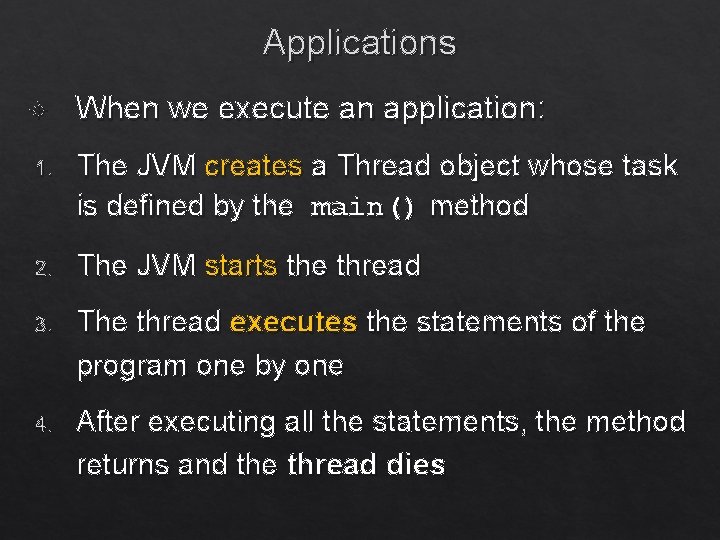
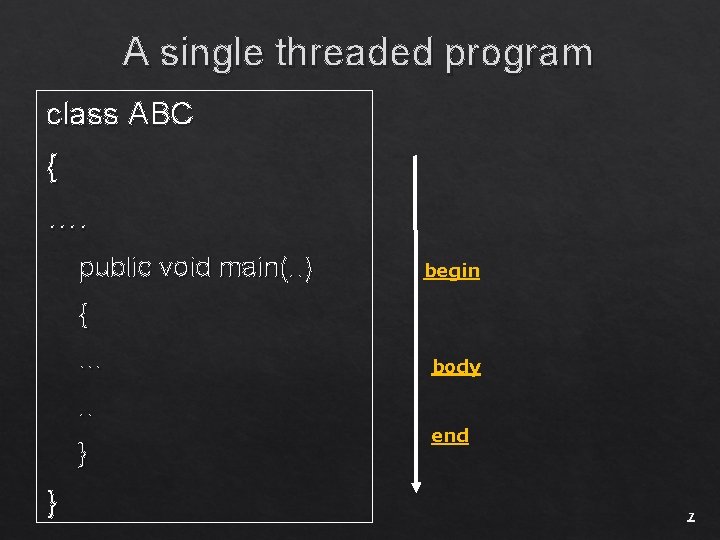
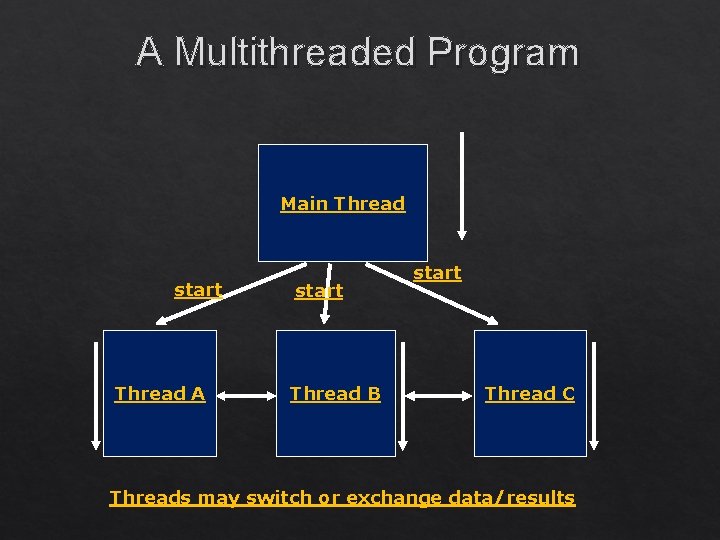
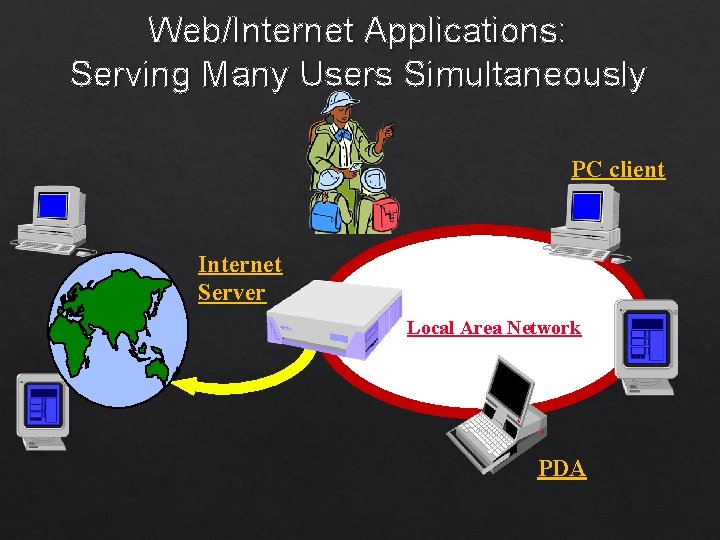
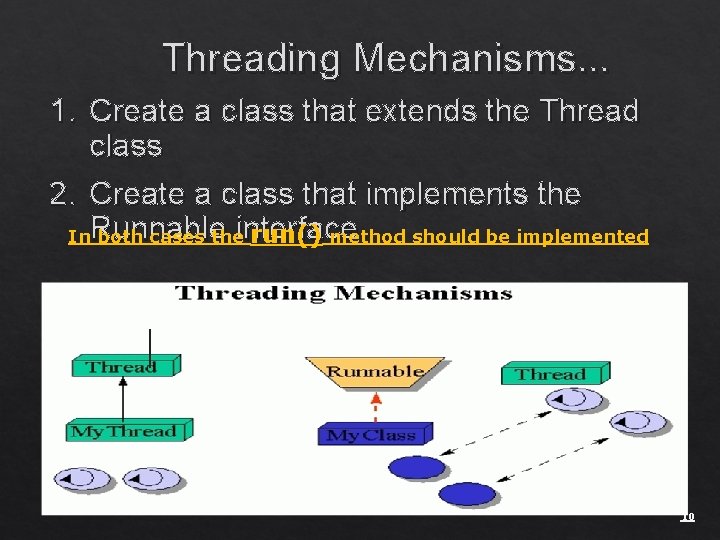
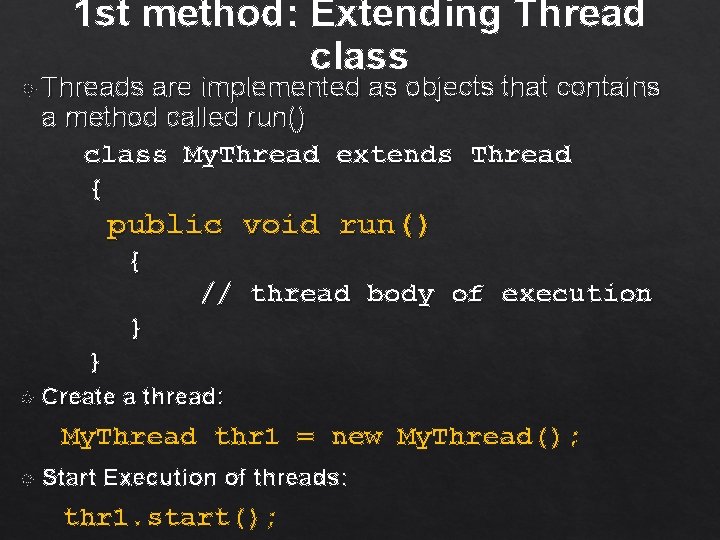
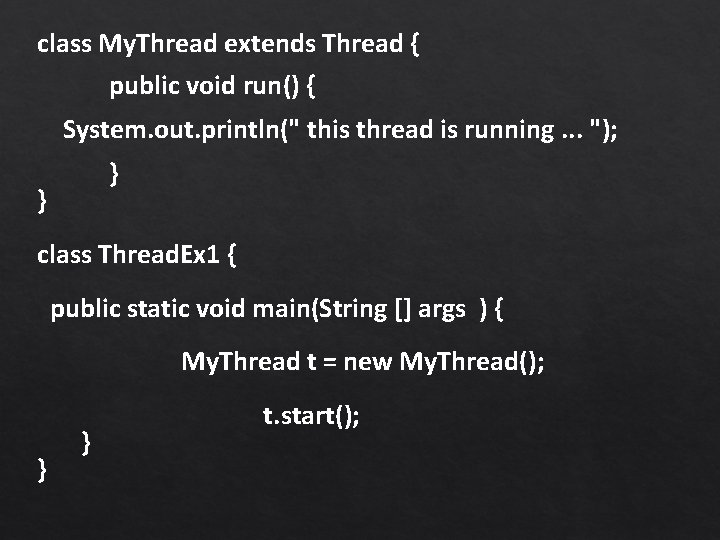
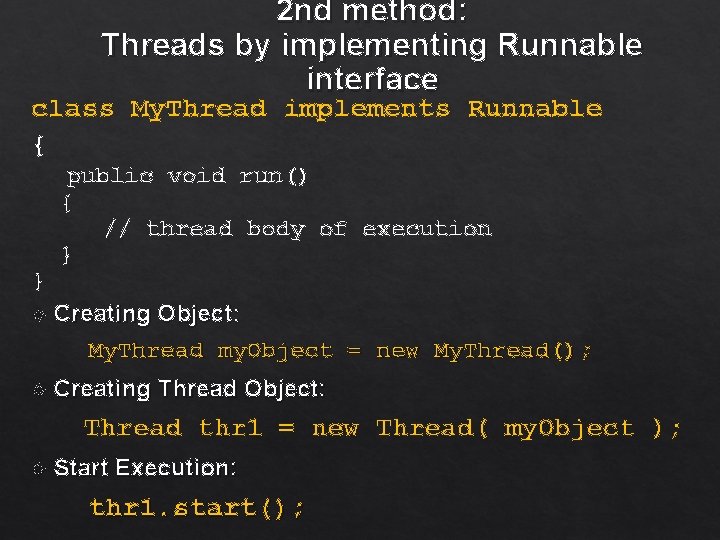
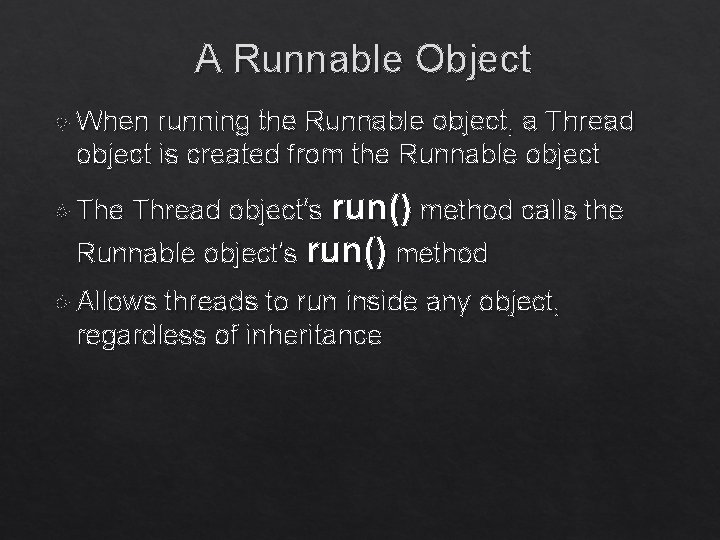
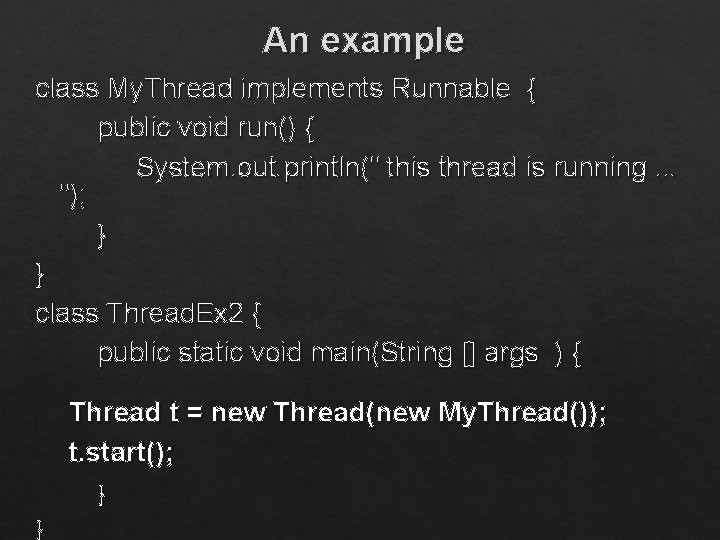
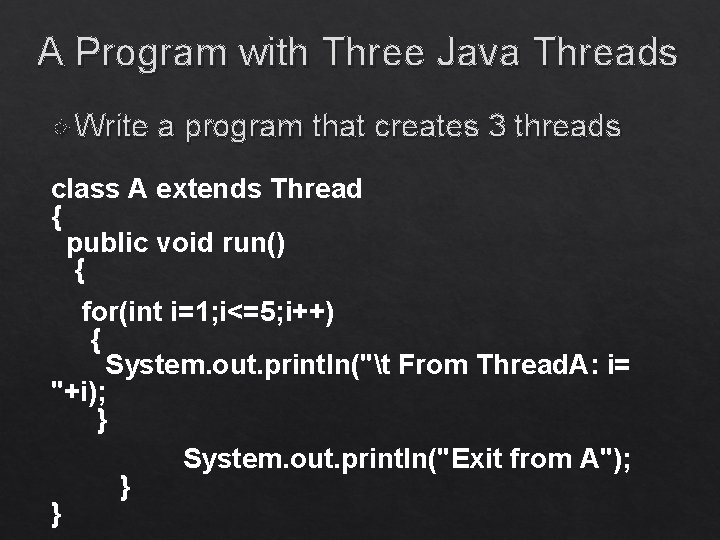
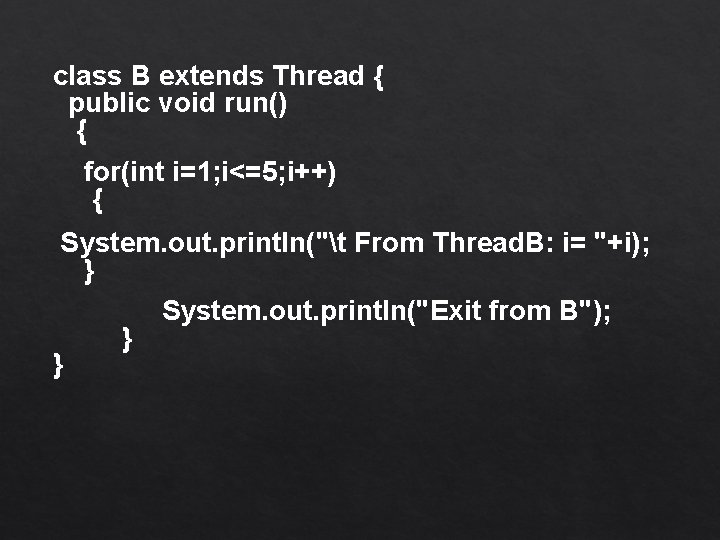
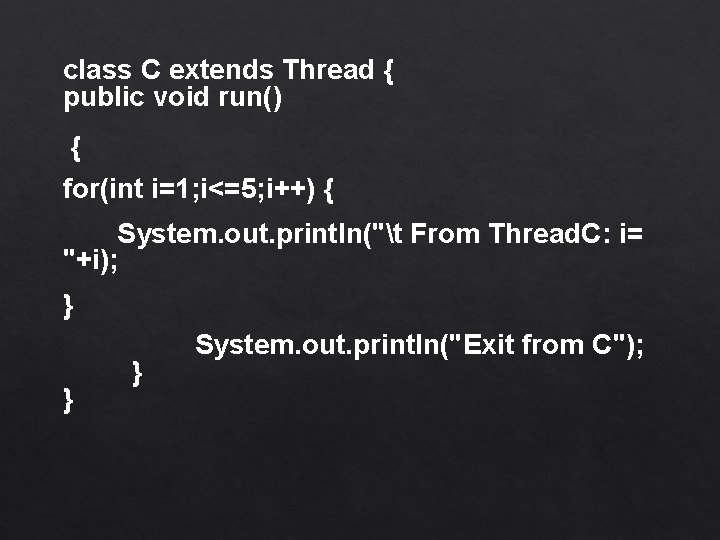
![class Thread. Test { public static void main(String args[]) { new A(). start(); new class Thread. Test { public static void main(String args[]) { new A(). start(); new](https://slidetodoc.com/presentation_image_h2/1619221d927b223805e10fa909c15984/image-19.jpg)
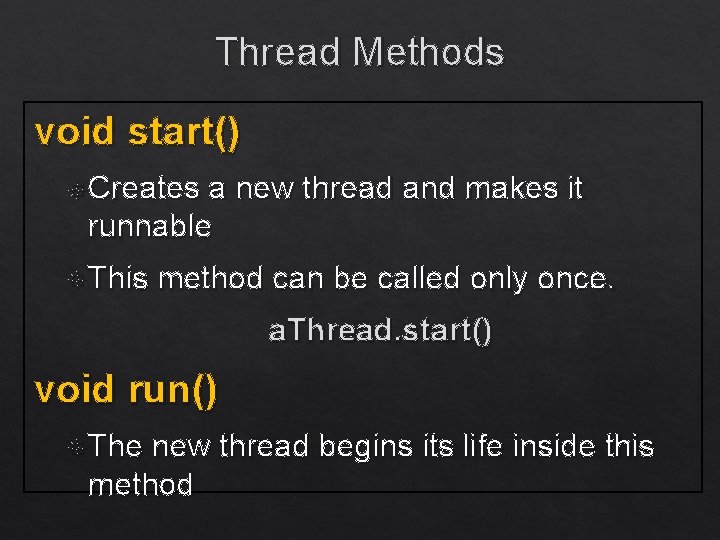
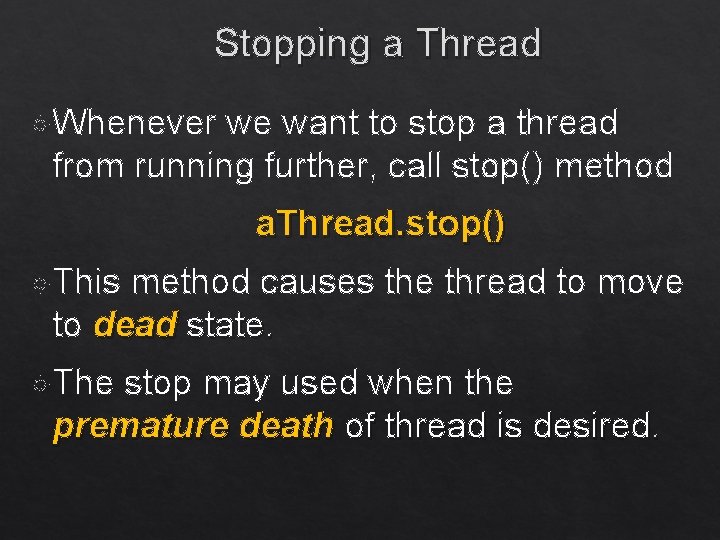
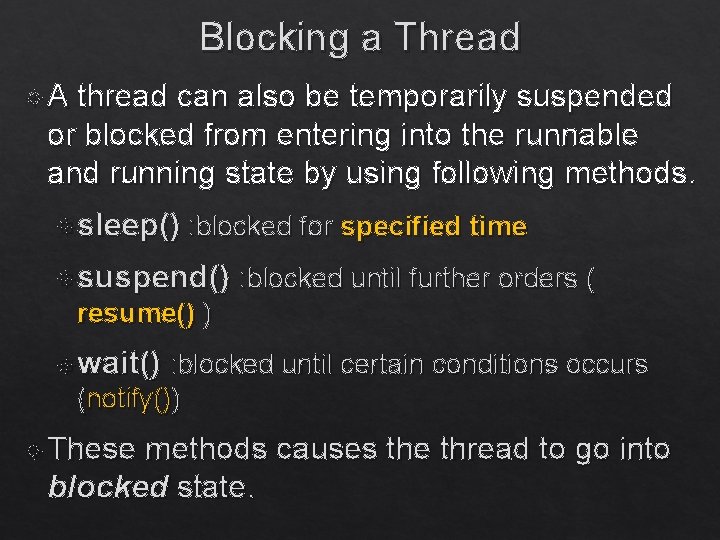
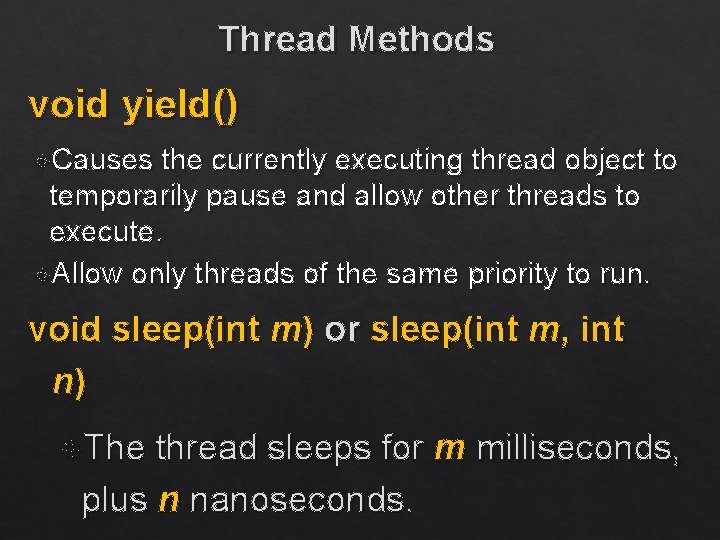
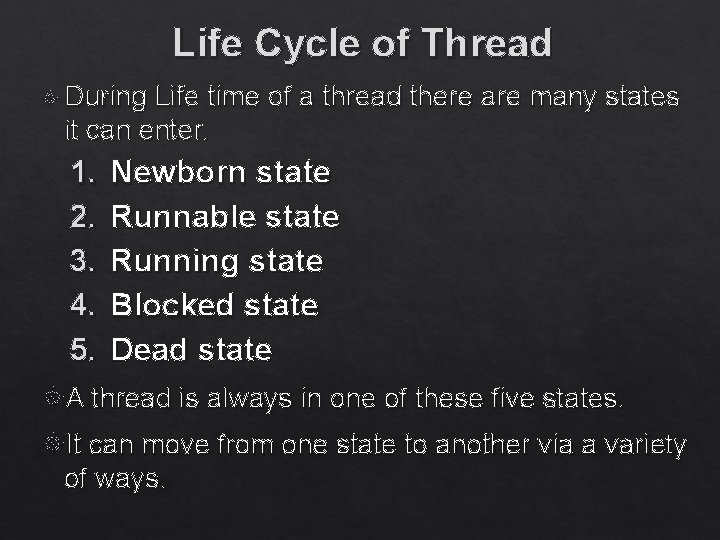
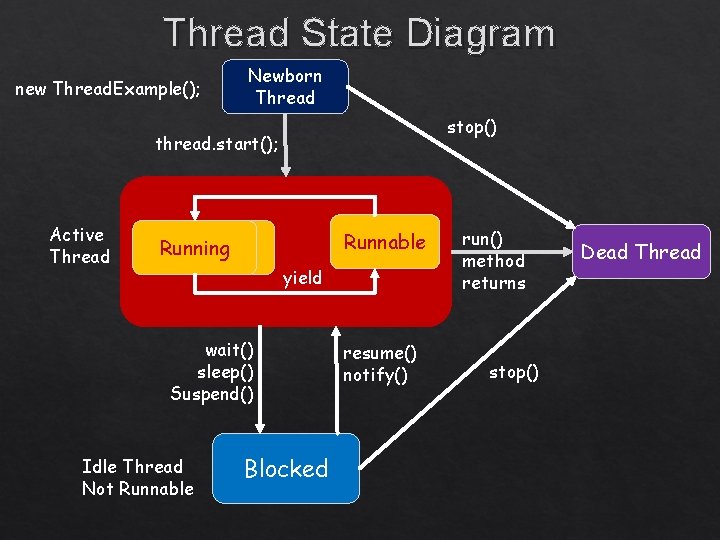
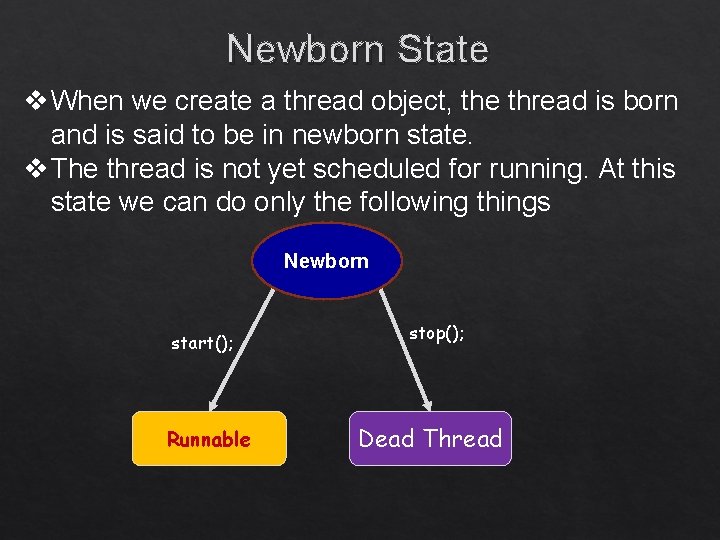
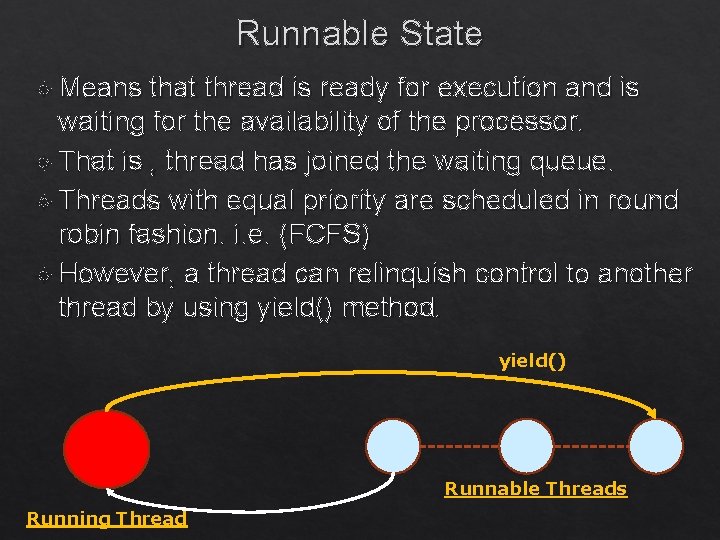
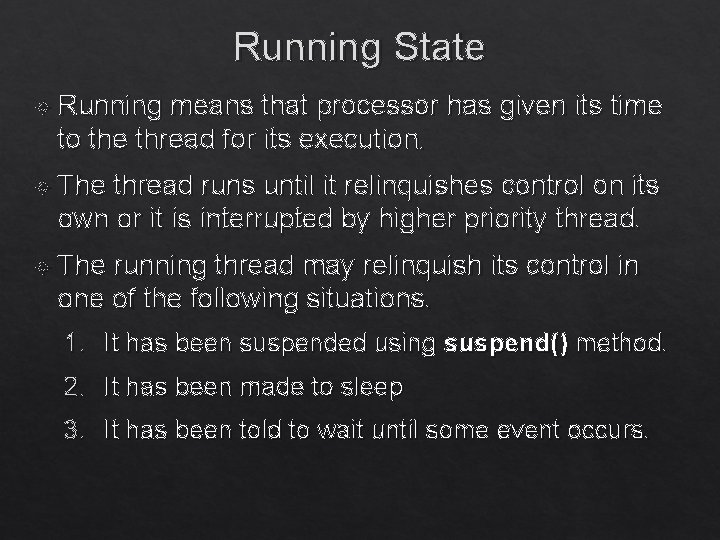
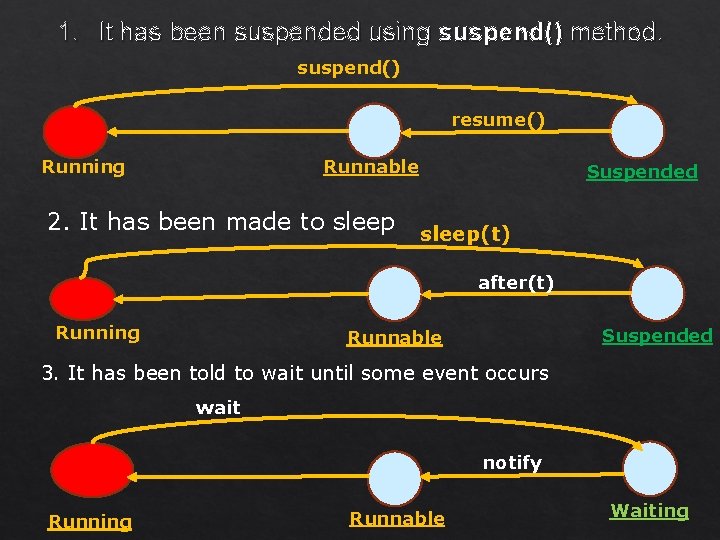
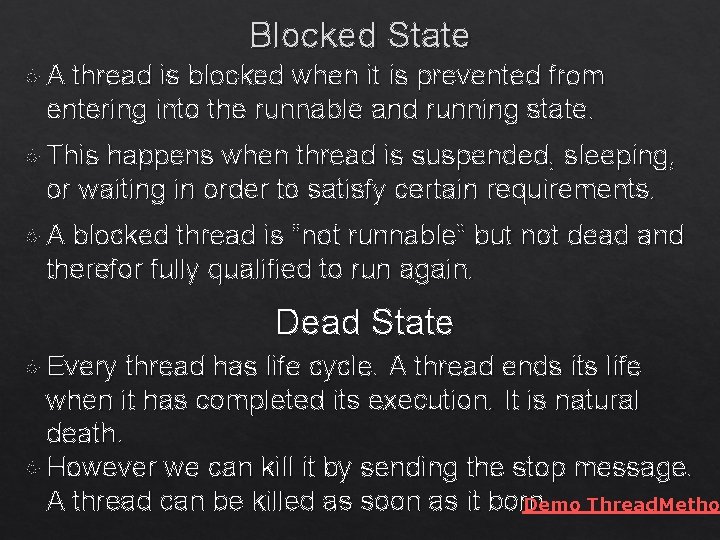
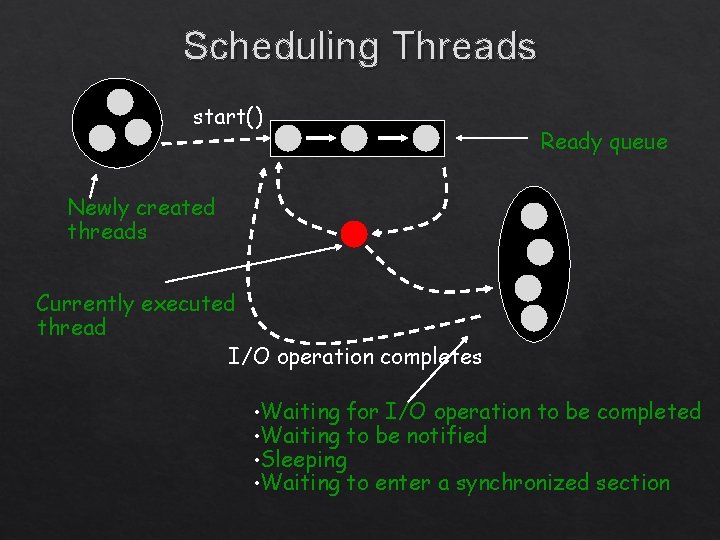
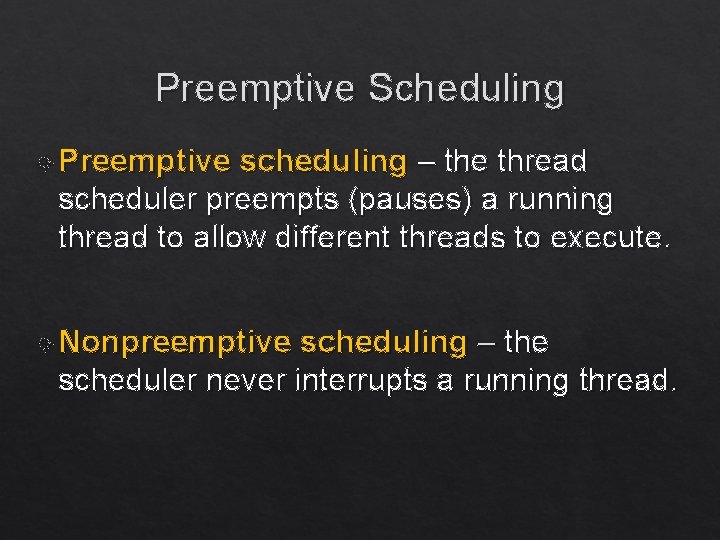
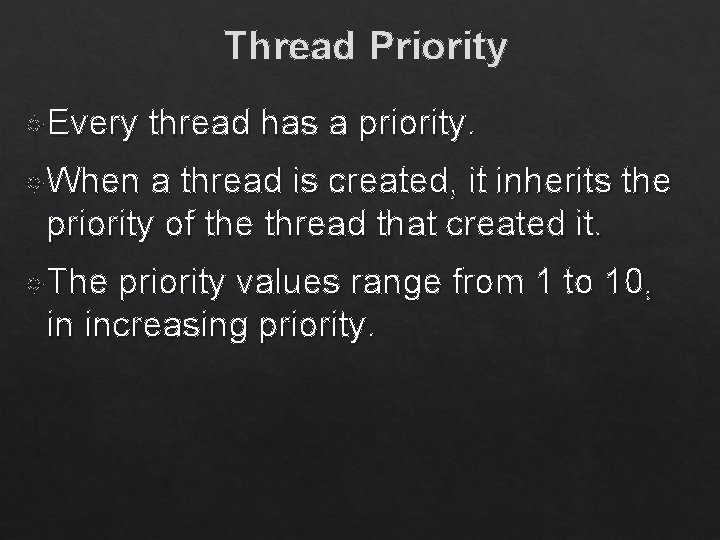
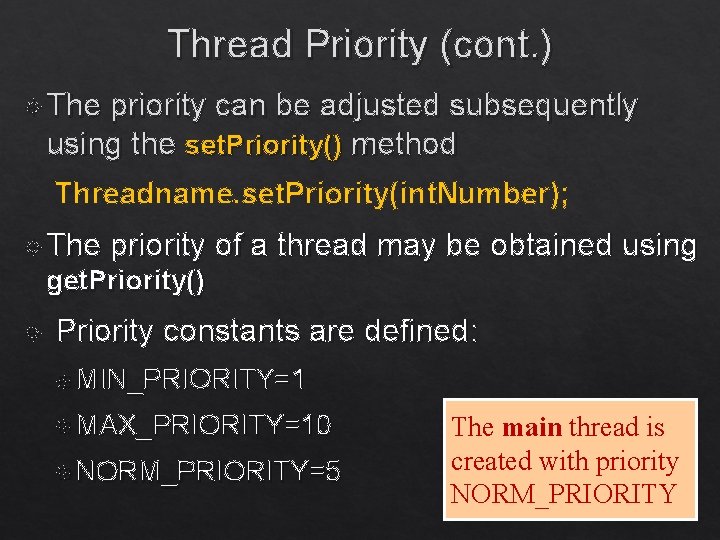
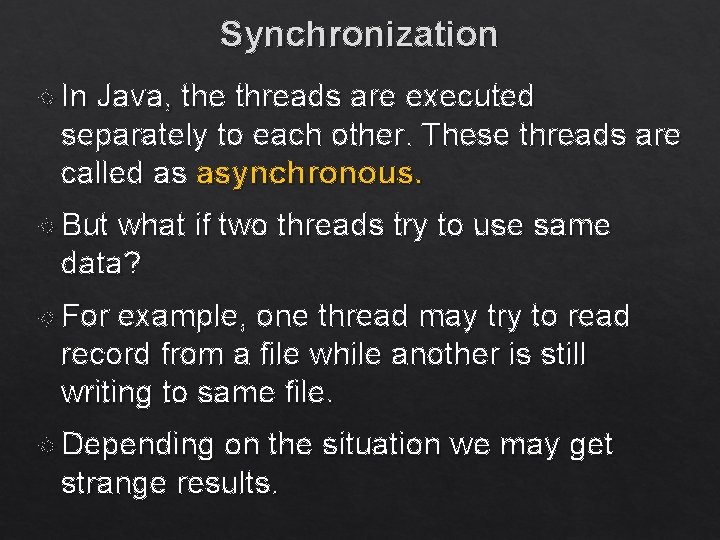
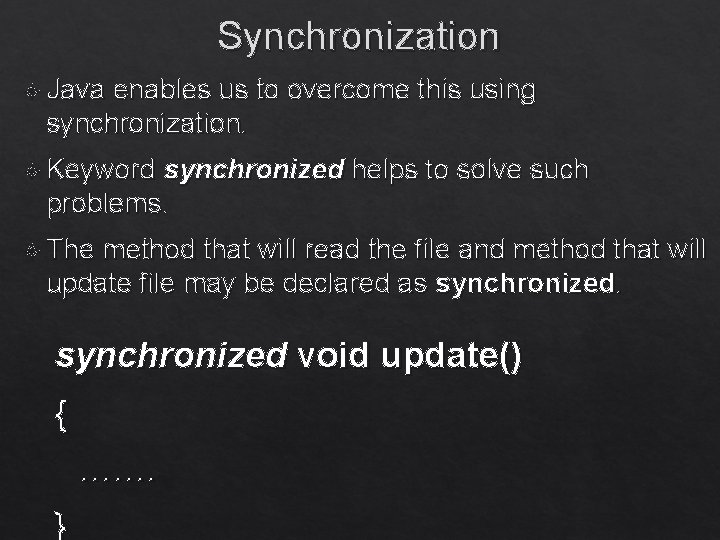
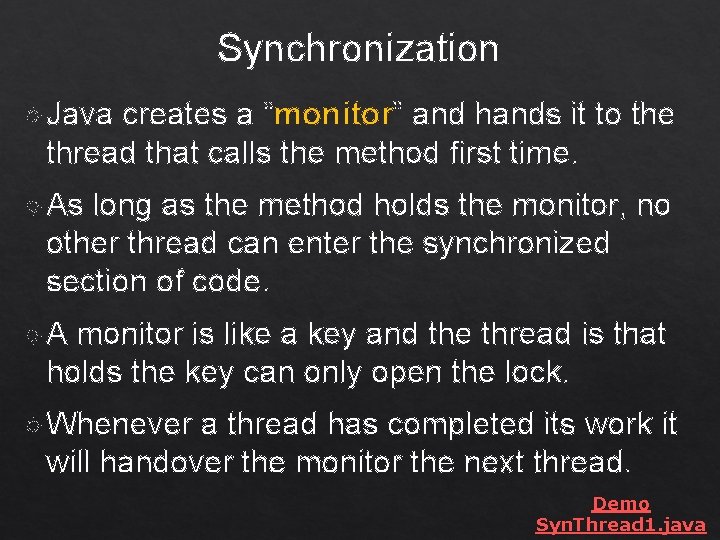
- Slides: 37
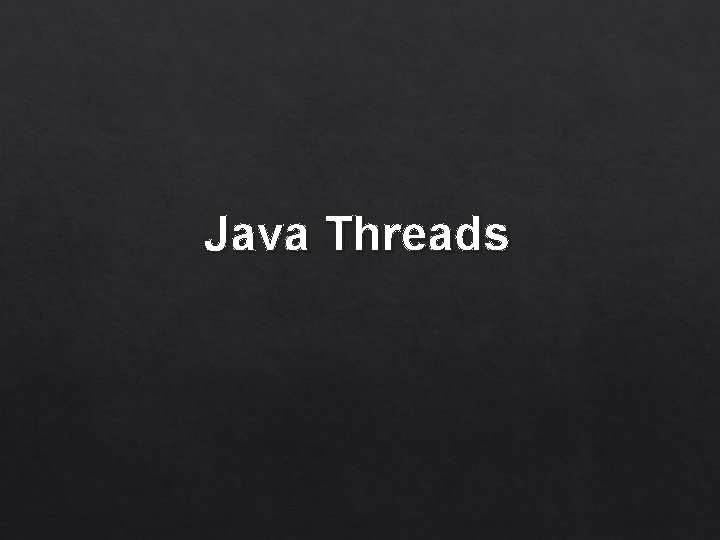
Java Threads
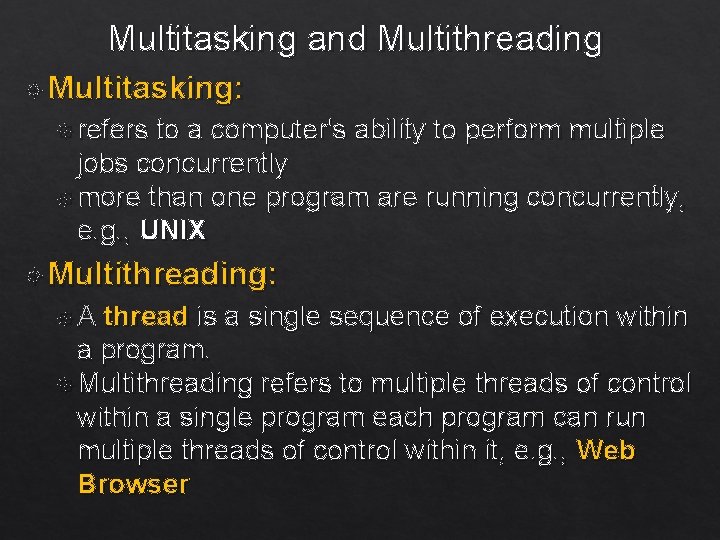
Multitasking and Multithreading Multitasking: refers to a computer's ability to perform multiple jobs concurrently more than one program are running concurrently, e. g. , UNIX Multithreading: A thread is a single sequence of execution within a program. Multithreading refers to multiple threads of control within a single program each program can run multiple threads of control within it, e. g. , Web Browser
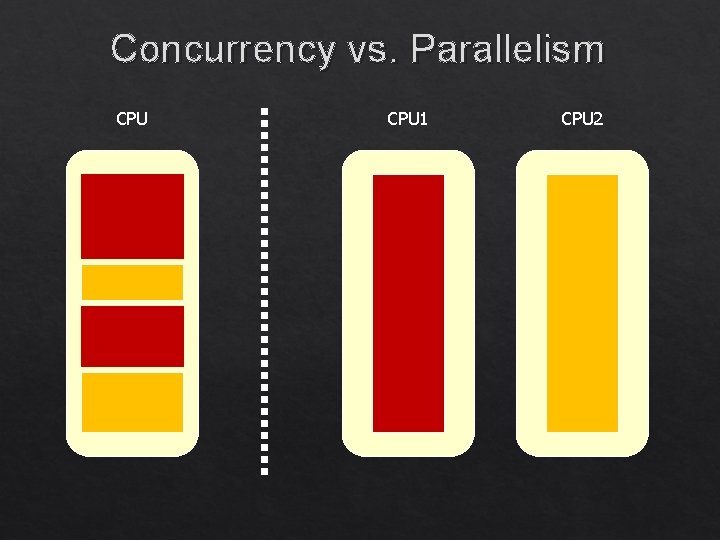
Concurrency vs. Parallelism CPU 1 CPU 2
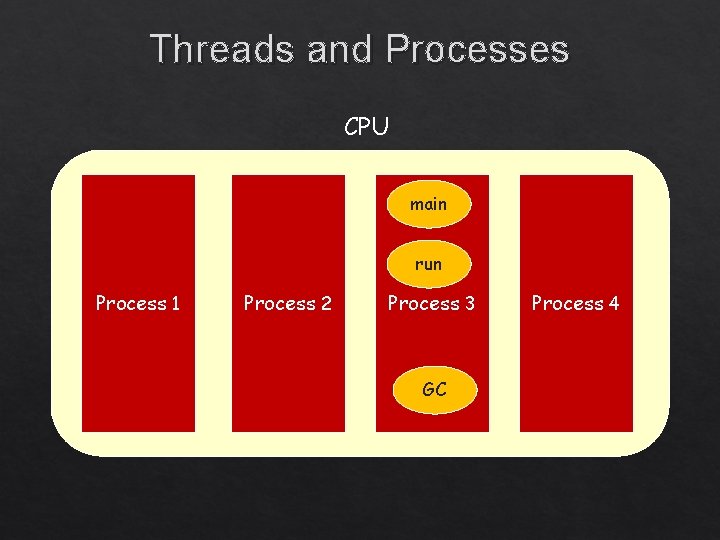
Threads and Processes CPU main run Process 1 Process 2 Process 3 GC Process 4
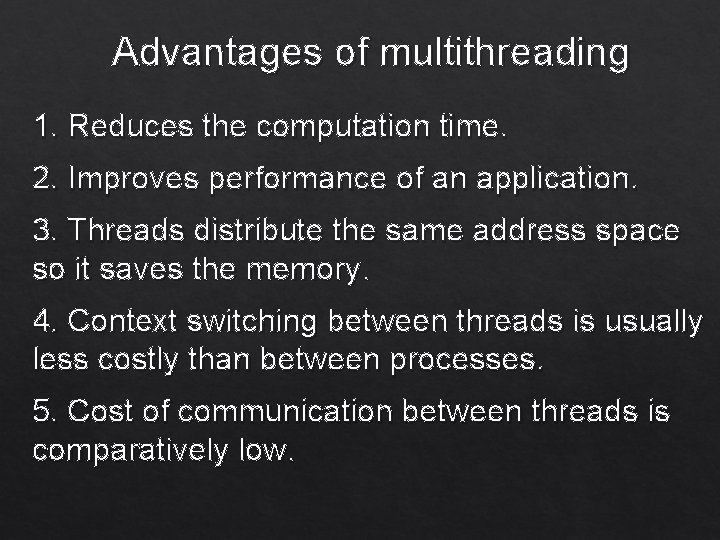
Advantages of multithreading 1. Reduces the computation time. 2. Improves performance of an application. 3. Threads distribute the same address space so it saves the memory. 4. Context switching between threads is usually less costly than between processes. 5. Cost of communication between threads is comparatively low.
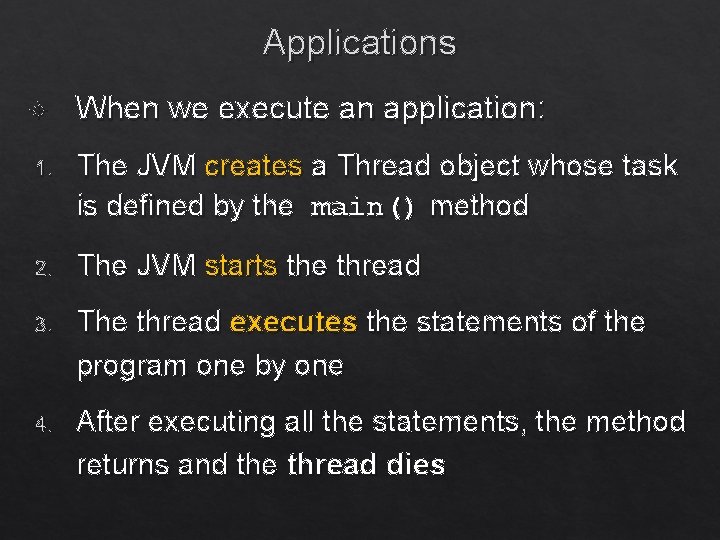
Applications When we execute an application: 1. The JVM creates a Thread object whose task is defined by the main() method 2. The JVM starts the thread 3. The thread executes the statements of the program one by one 4. After executing all the statements, the method returns and the thread dies
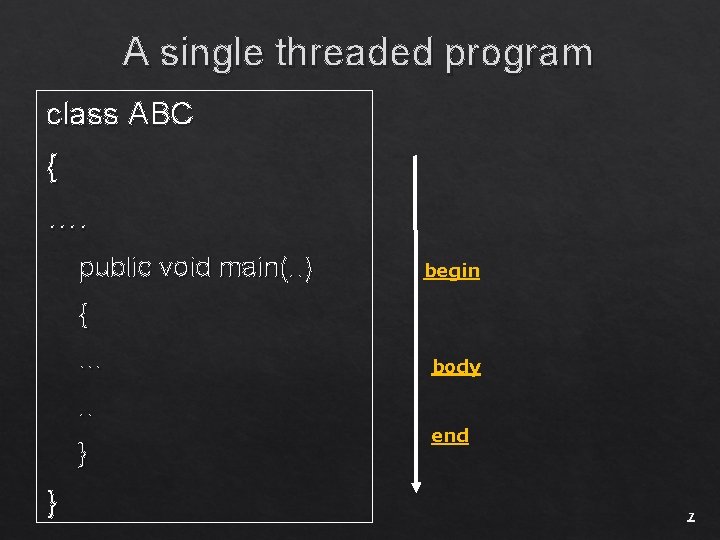
A single threaded program class ABC { …. public void main(. . ) begin { … body . . } } end 7
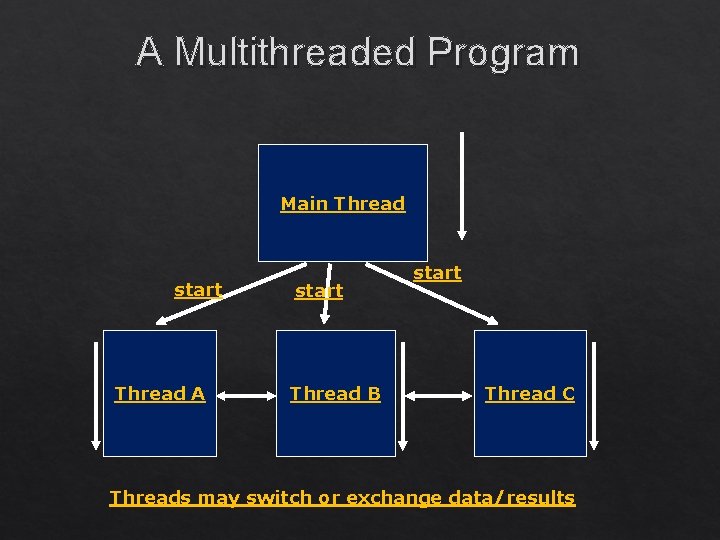
A Multithreaded Program Main Thread start Thread A start Thread B start Thread C Threads may switch or exchange data/results
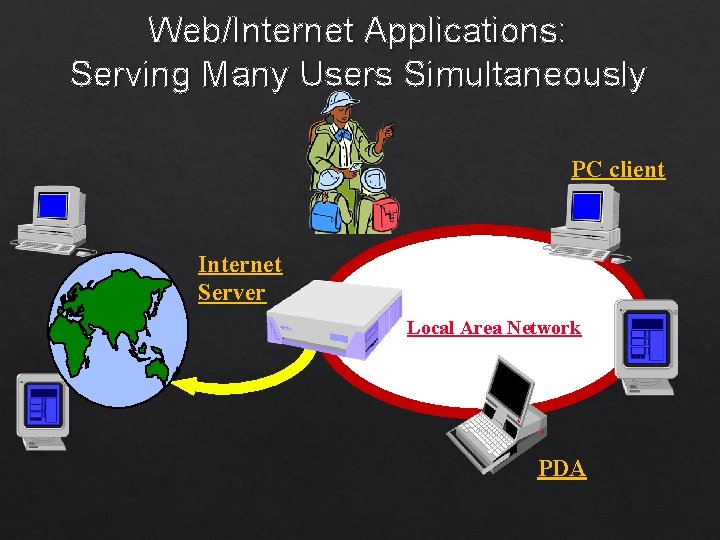
Web/Internet Applications: Serving Many Users Simultaneously PC client Internet Server Local Area Network PDA
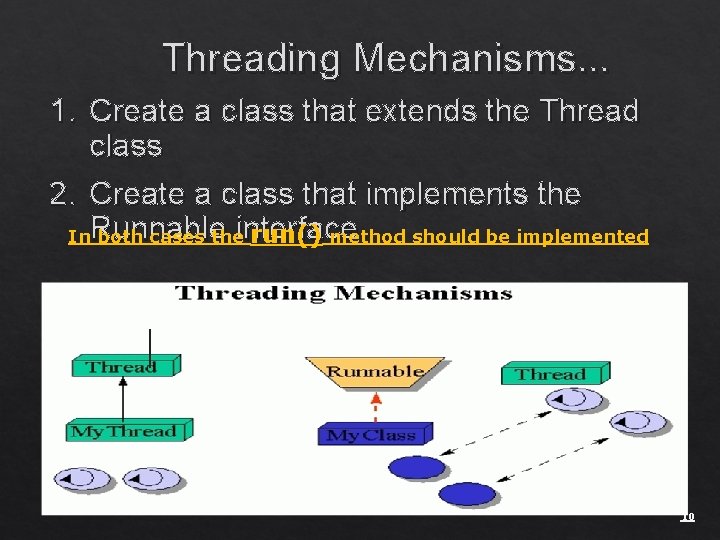
Threading Mechanisms. . . 1. Create a class that extends the Thread class 2. Create a class that implements the In. Runnable both cases theinterface run() method should be implemented 10
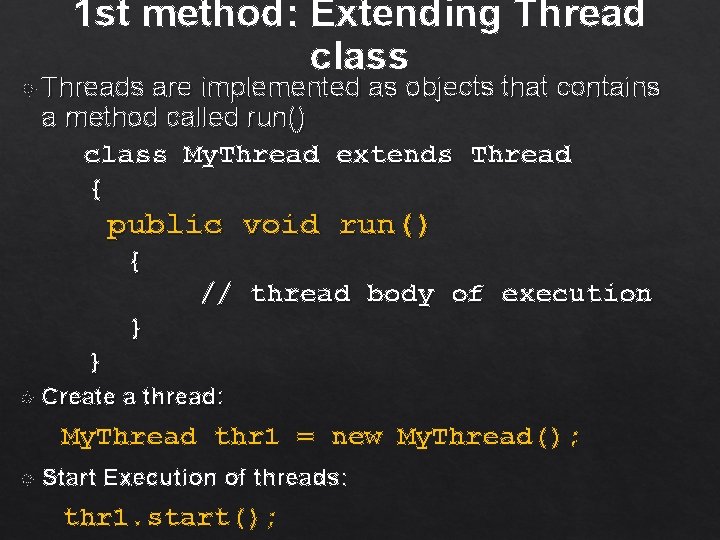
1 st method: Extending Thread class Threads are implemented as objects that contains a method called run() class My. Thread extends Thread { public void run() { // thread body of execution } } Create a thread: My. Thread thr 1 = new My. Thread(); Start Execution of threads: thr 1. start();
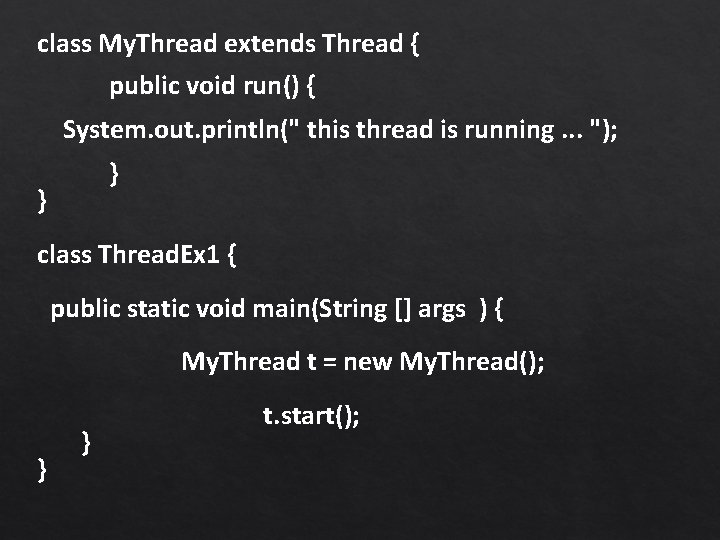
class My. Thread extends Thread { public void run() { System. out. println(" this thread is running. . . "); } } class Thread. Ex 1 { public static void main(String [] args ) { My. Thread t = new My. Thread(); } } t. start();
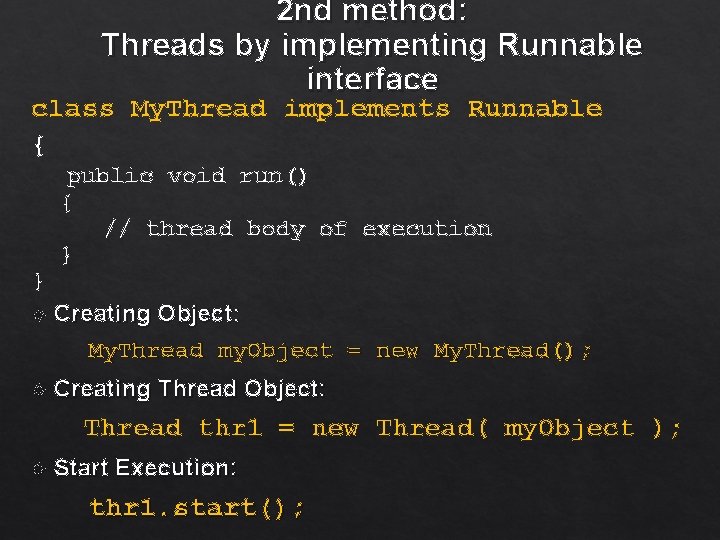
2 nd method: Threads by implementing Runnable interface class My. Thread implements Runnable { } public void run() { // thread body of execution } Creating Object: My. Thread my. Object = new My. Thread(); Creating Thread Object: Thread thr 1 = new Thread( my. Object ); Start Execution: thr 1. start();
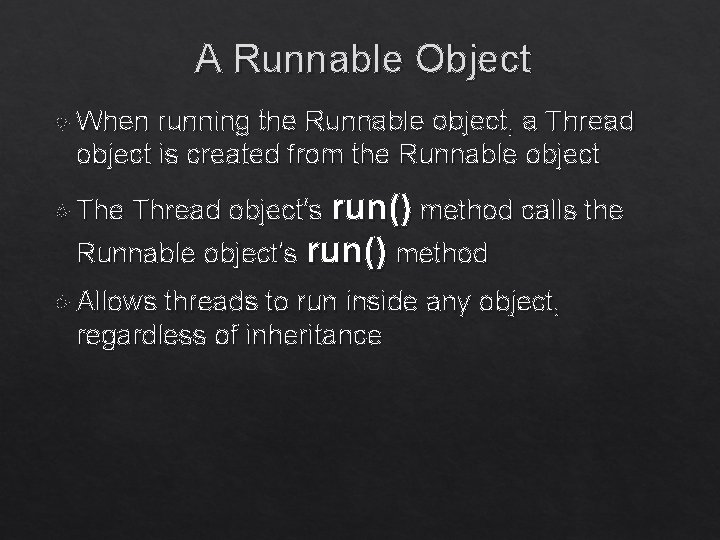
A Runnable Object When running the Runnable object, a Thread object is created from the Runnable object The Thread object’s run() method calls the Runnable object’s run() method Allows threads to run inside any object, regardless of inheritance
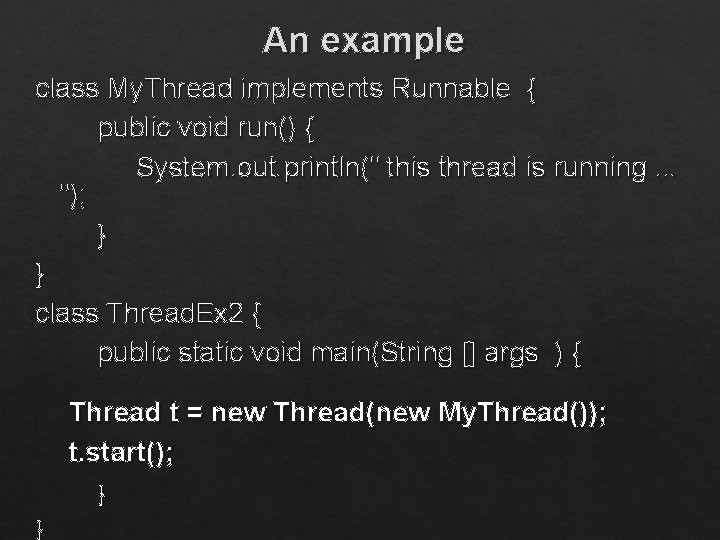
An example class My. Thread implements Runnable { public void run() { System. out. println(" this thread is running. . . "); } } class Thread. Ex 2 { public static void main(String [] args ) { Thread t = new Thread(new My. Thread()); t. start(); } }
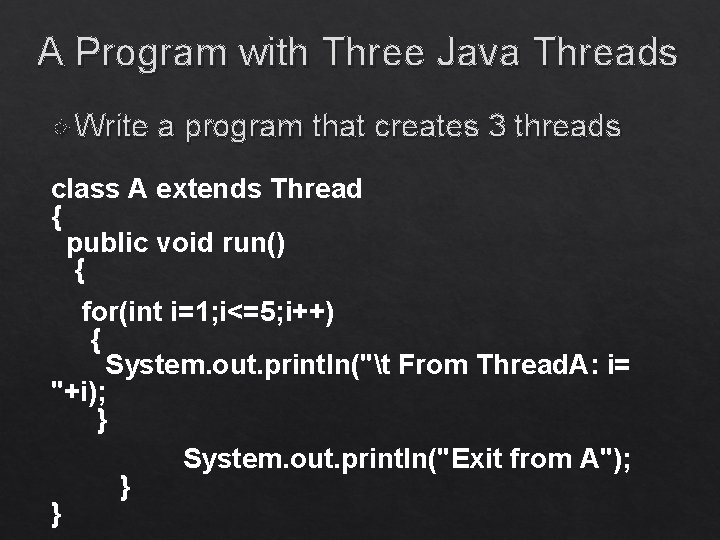
A Program with Three Java Threads Write a program that creates 3 threads class A extends Thread { public void run() { for(int i=1; i<=5; i++) { System. out. println("t From Thread. A: i= "+i); } System. out. println("Exit from A"); } }
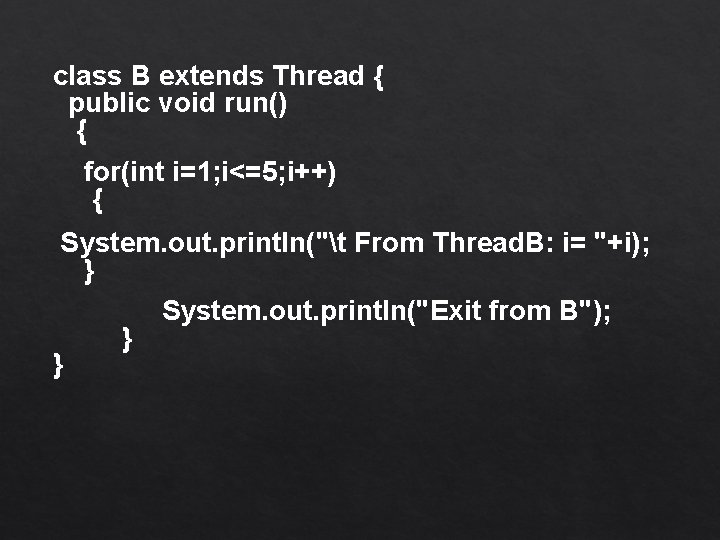
class B extends Thread { public void run() { for(int i=1; i<=5; i++) { System. out. println("t From Thread. B: i= "+i); } System. out. println("Exit from B"); } }
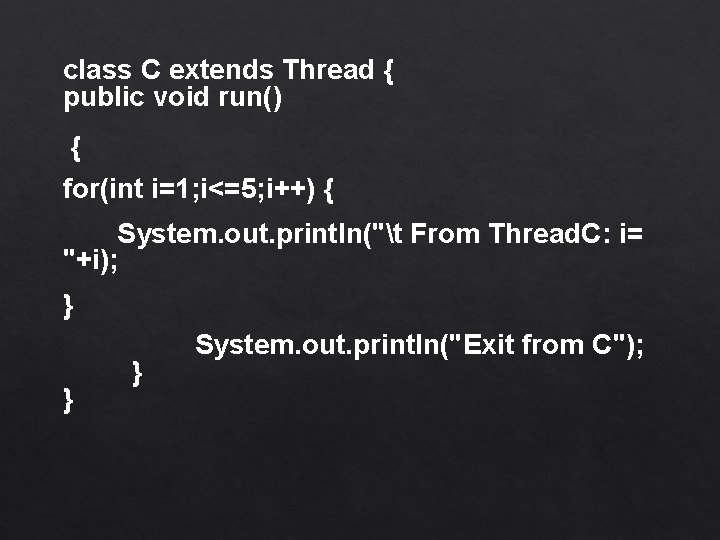
class C extends Thread { public void run() { for(int i=1; i<=5; i++) { System. out. println("t From Thread. C: i= "+i); } } } System. out. println("Exit from C");
![class Thread Test public static void mainString args new A start new class Thread. Test { public static void main(String args[]) { new A(). start(); new](https://slidetodoc.com/presentation_image_h2/1619221d927b223805e10fa909c15984/image-19.jpg)
class Thread. Test { public static void main(String args[]) { new A(). start(); new B(). start(); new C(). start(); } }
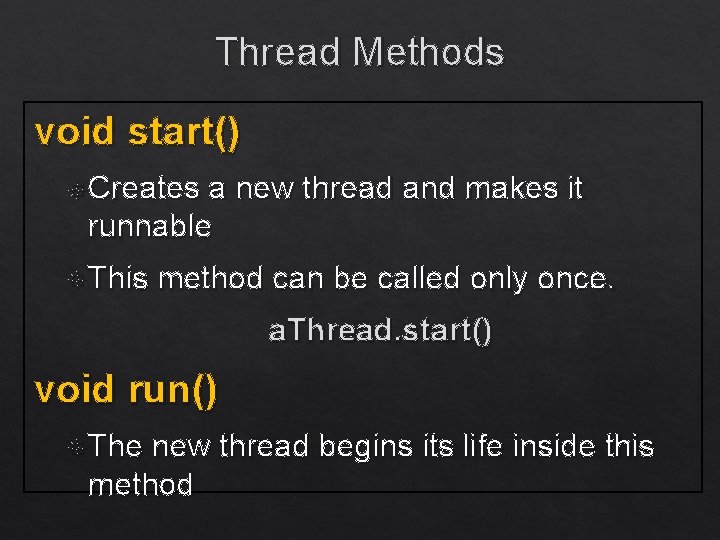
Thread Methods void start() Creates a new thread and makes it runnable This method can be called only once. a. Thread. start() void run() The new thread begins its life inside this method
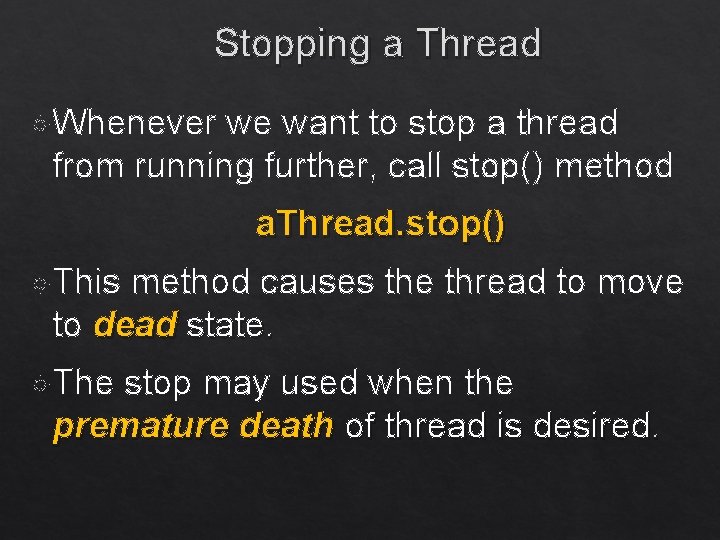
Stopping a Thread Whenever we want to stop a thread from running further, call stop() method a. Thread. stop() This method causes the thread to move to dead state. The stop may used when the premature death of thread is desired.
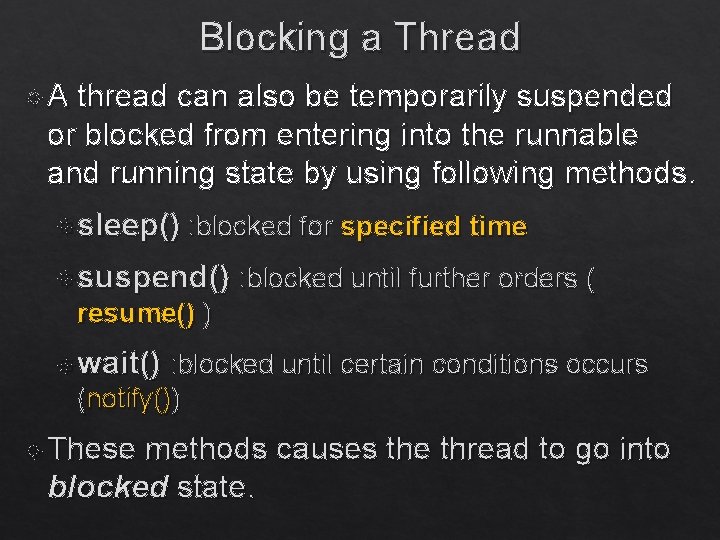
Blocking a Thread A thread can also be temporarily suspended or blocked from entering into the runnable and running state by using following methods. sleep() : blocked for specified time suspend() : blocked until further orders ( resume() ) wait() : blocked until certain conditions occurs (notify()) These methods causes the thread to go into blocked state.
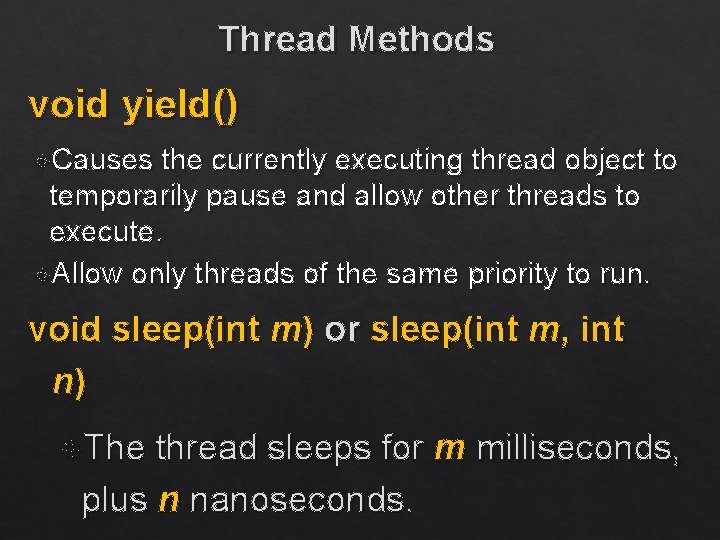
Thread Methods void yield() Causes the currently executing thread object to temporarily pause and allow other threads to execute. Allow only threads of the same priority to run. void sleep(int m) or sleep(int m, int n) The thread sleeps for m milliseconds, plus n nanoseconds.
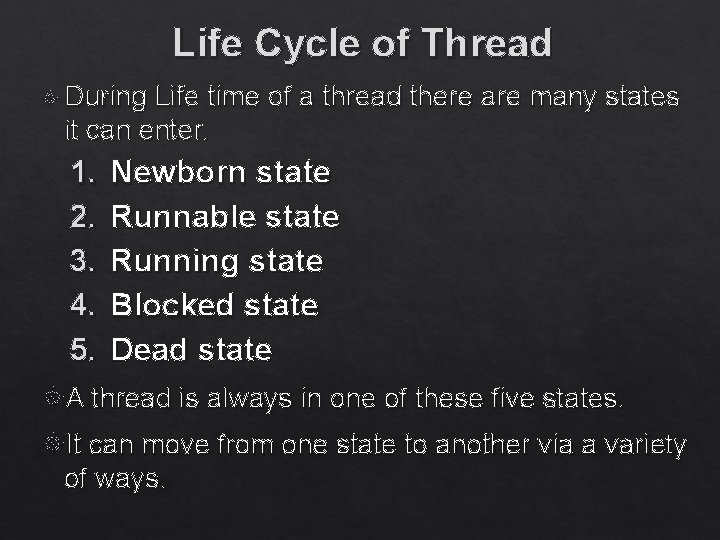
Life Cycle of Thread During Life time of a thread there are many states it can enter. 1. 2. 3. 4. 5. Newborn state Runnable state Running state Blocked state Dead state A thread is always in one of these five states. It can move from one state to another via a variety of ways.
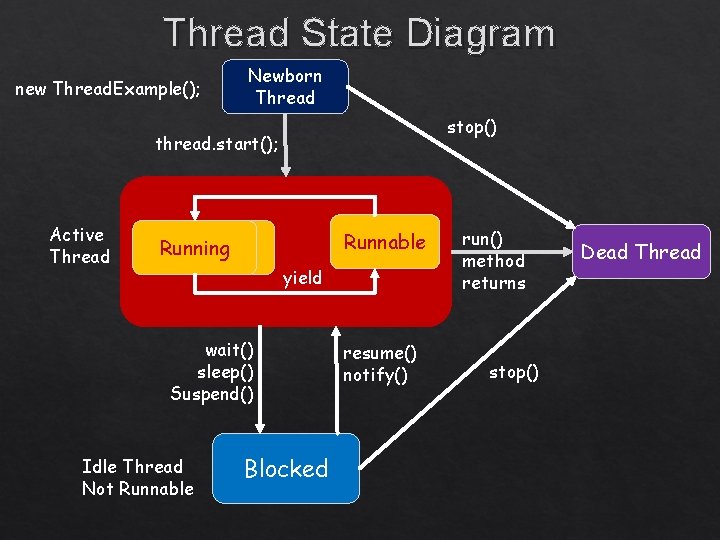
Thread State Diagram new Thread. Example(); Newborn Thread stop() thread. start(); Active Thread Runnable Running yield wait() sleep() Suspend() Idle Thread Not Runnable Blocked resume() notify() run() method returns stop() Dead Thread
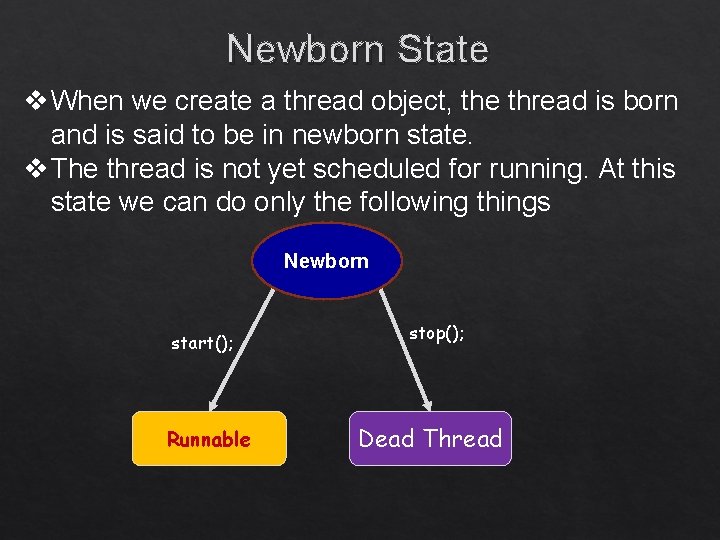
Newborn State v When we create a thread object, the thread is born and is said to be in newborn state. v The thread is not yet scheduled for running. At this state we can do only the following things Newborn start(); Runnable stop(); Dead Thread
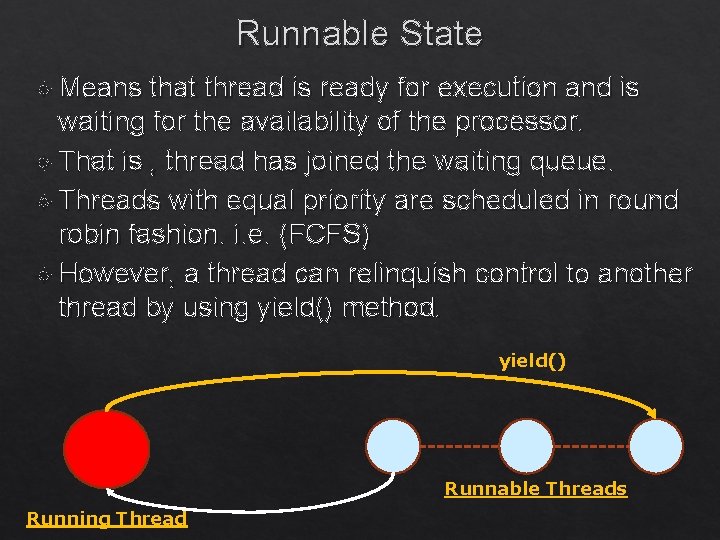
Runnable State Means that thread is ready for execution and is waiting for the availability of the processor. That is , thread has joined the waiting queue. Threads with equal priority are scheduled in round robin fashion. i. e. (FCFS) However, a thread can relinquish control to another thread by using yield() method. yield() Runnable Threads Running Thread
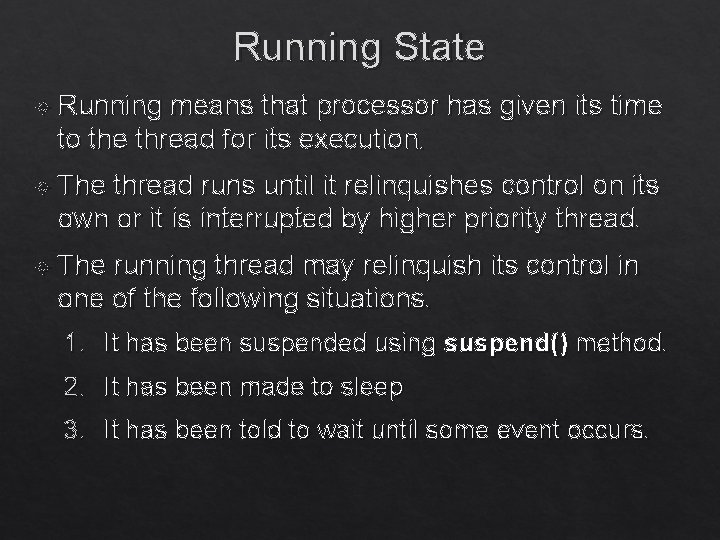
Running State Running means that processor has given its time to the thread for its execution. The thread runs until it relinquishes control on its own or it is interrupted by higher priority thread. The running thread may relinquish its control in one of the following situations. 1. It has been suspended using suspend() method. 2. It has been made to sleep 3. It has been told to wait until some event occurs.
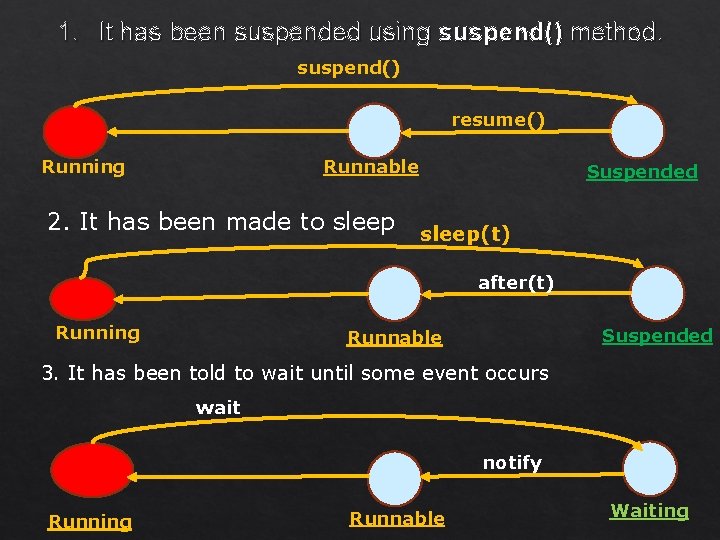
1. It has been suspended using suspend() method. suspend() resume() Running Runnable 2. It has been made to sleep Suspended sleep(t) after(t) Running Suspended Runnable 3. It has been told to wait until some event occurs wait notify Running Runnable Waiting
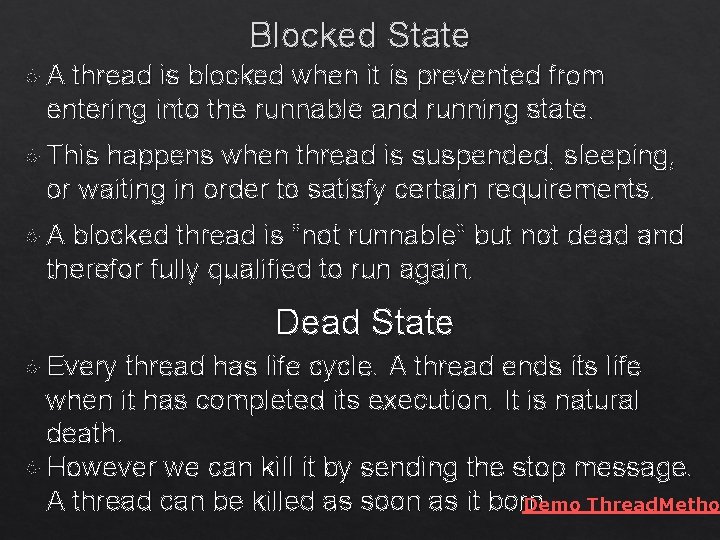
Blocked State A thread is blocked when it is prevented from entering into the runnable and running state. This happens when thread is suspended, sleeping, or waiting in order to satisfy certain requirements. A blocked thread is “not runnable” but not dead and therefor fully qualified to run again. Dead State Every thread has life cycle. A thread ends its life when it has completed its execution. It is natural death. However we can kill it by sending the stop message. A thread can be killed as soon as it born. Demo Thread. Method
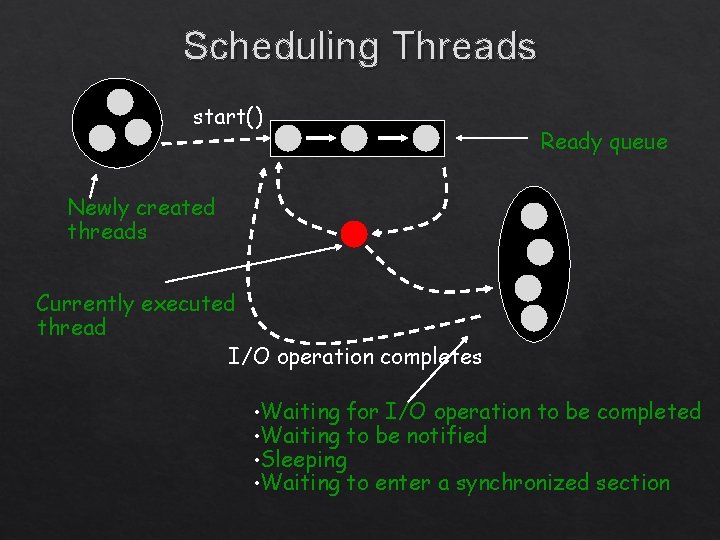
Scheduling Threads start() Ready queue Newly created threads Currently executed thread I/O operation completes • Waiting for I/O operation to be • Waiting to be notified • Sleeping • Waiting to enter a synchronized completed section
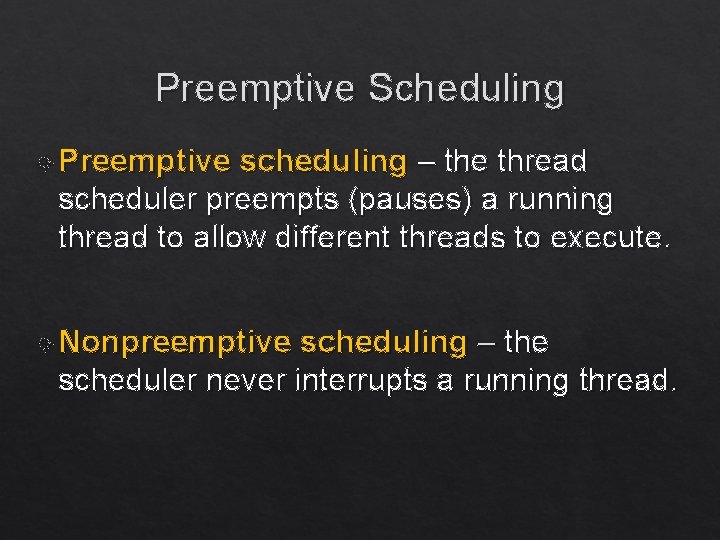
Preemptive Scheduling Preemptive scheduling – the thread scheduler preempts (pauses) a running thread to allow different threads to execute. Nonpreemptive scheduling – the scheduler never interrupts a running thread.
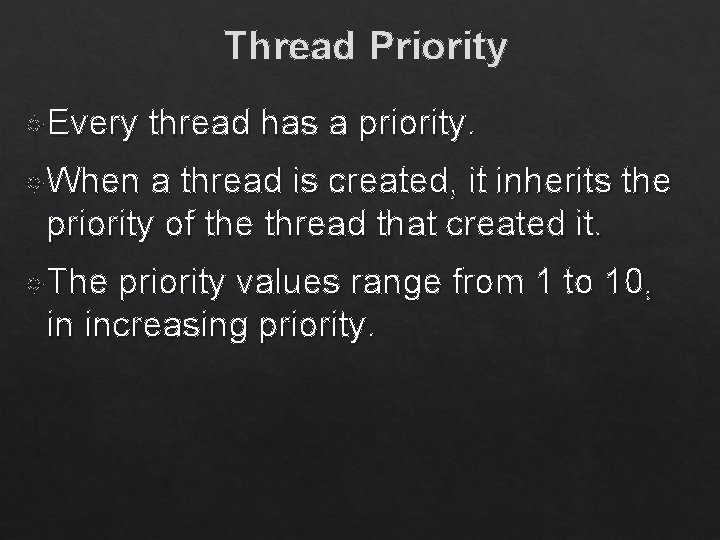
Thread Priority Every thread has a priority. When a thread is created, it inherits the priority of the thread that created it. The priority values range from 1 to 10, in increasing priority.
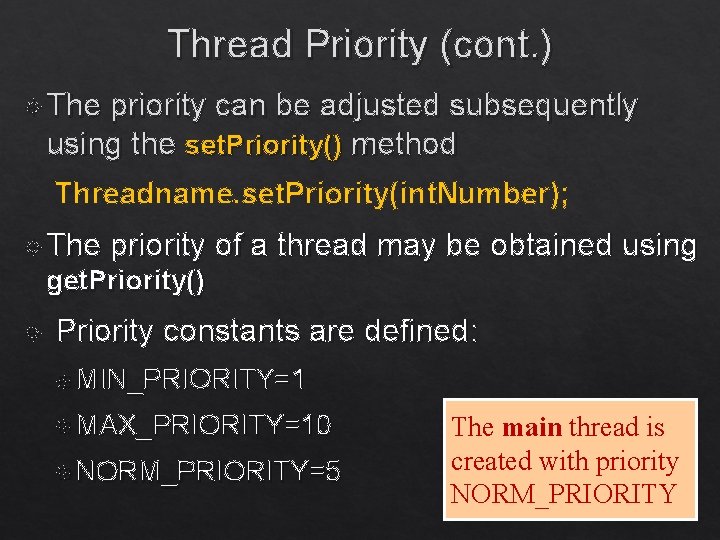
Thread Priority (cont. ) The priority can be adjusted subsequently using the set. Priority() method Threadname. set. Priority(int. Number); The priority of a thread may be obtained using get. Priority() Priority constants are defined: MIN_PRIORITY=1 MAX_PRIORITY=10 NORM_PRIORITY=5 The main thread is created with priority NORM_PRIORITY
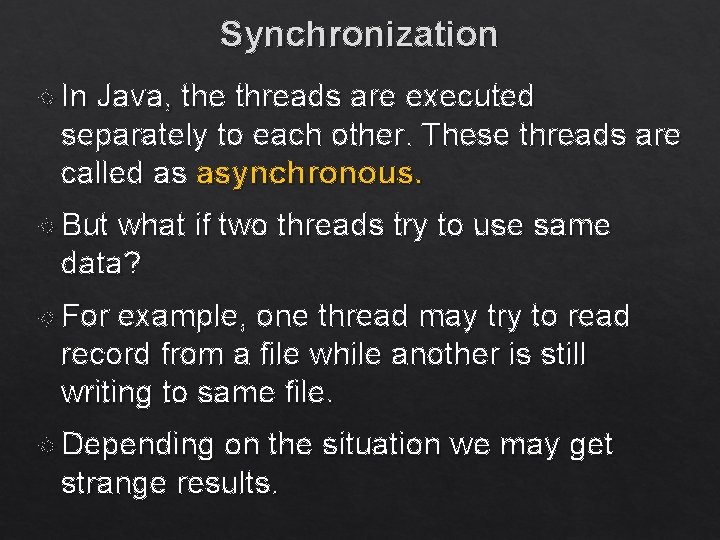
Synchronization In Java, the threads are executed separately to each other. These threads are called as asynchronous. But what if two threads try to use same data? For example, one thread may try to read record from a file while another is still writing to same file. Depending on the situation we may get strange results.
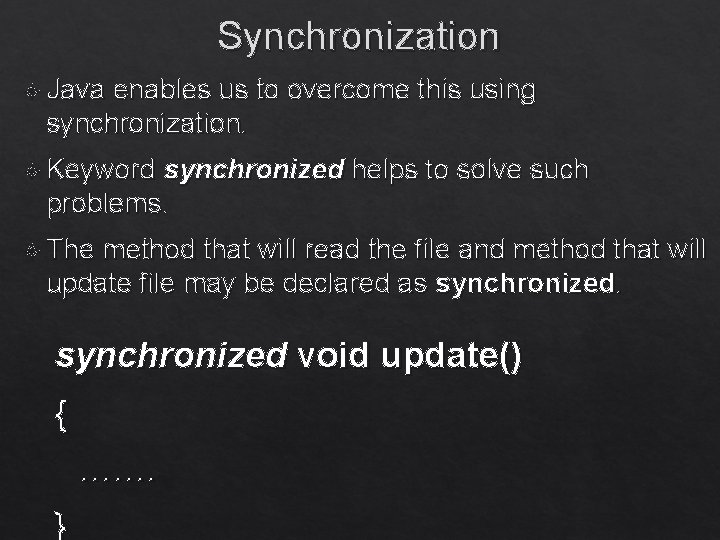
Synchronization Java enables us to overcome this using synchronization. Keyword synchronized helps to solve such problems. The method that will read the file and method that will update file may be declared as synchronized void update() { ……. }
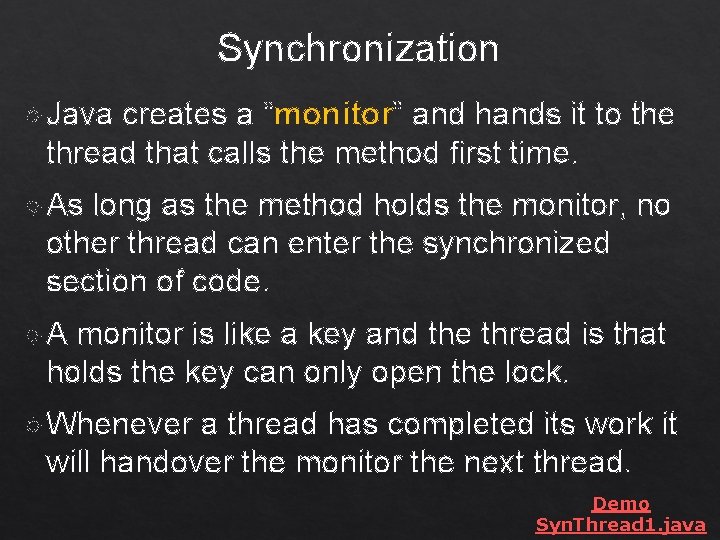
Synchronization Java creates a “monitor” and hands it to the thread that calls the method first time. As long as the method holds the monitor, no other thread can enter the synchronized section of code. A monitor is like a key and the thread is that holds the key can only open the lock. Whenever a thread has completed its work it will handover the monitor the next thread. Demo Syn. Thread 1. java