Java threading Multithreaded applets Peter Mozelius DSVUCSC 1
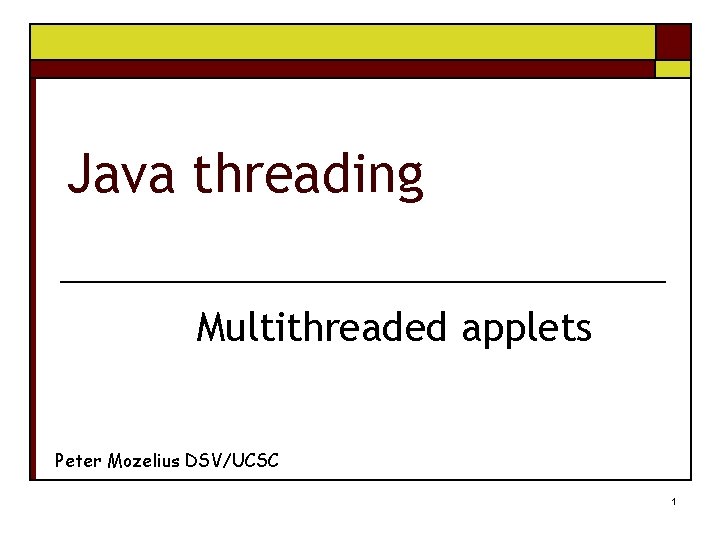
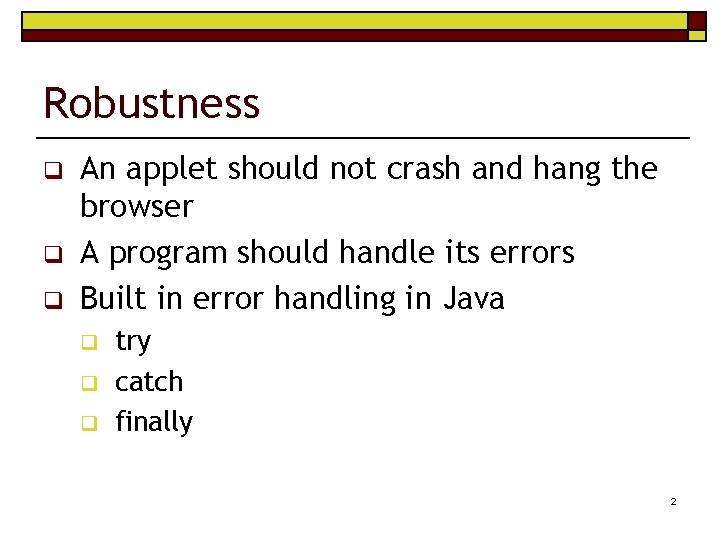
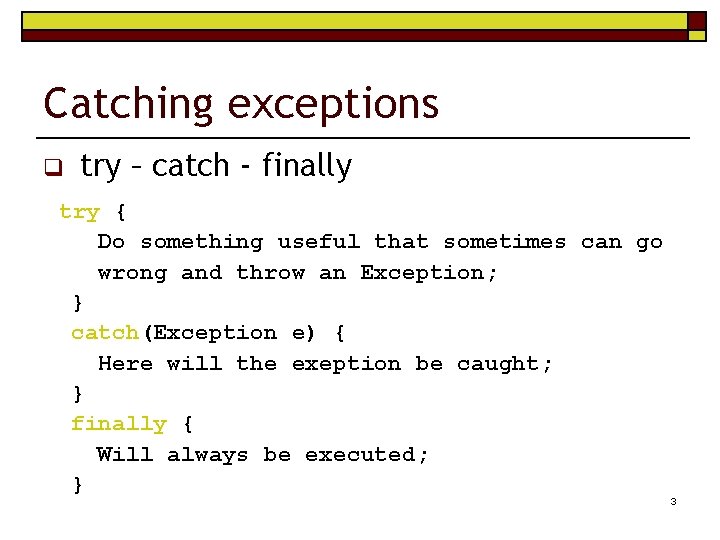
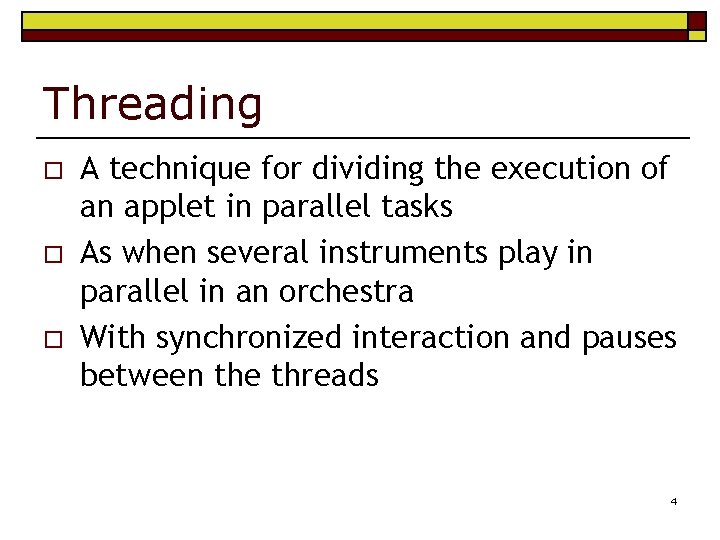
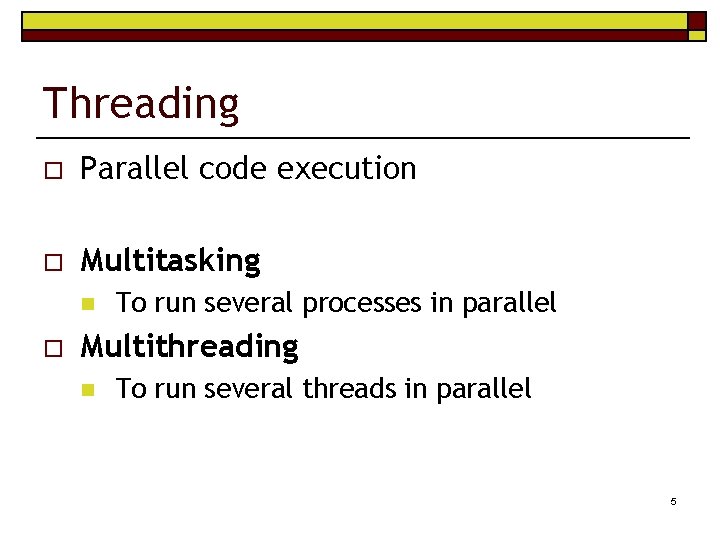
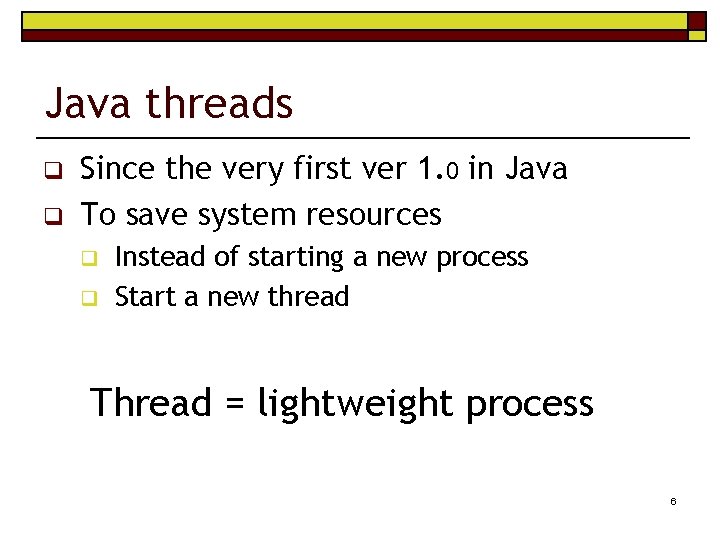
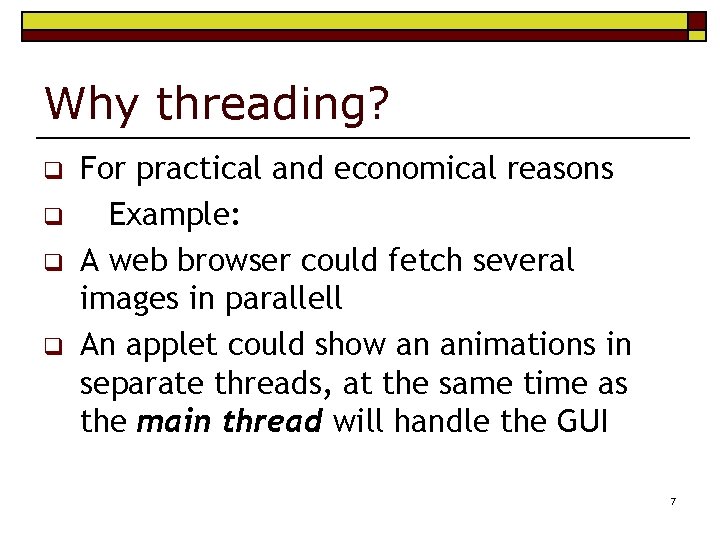
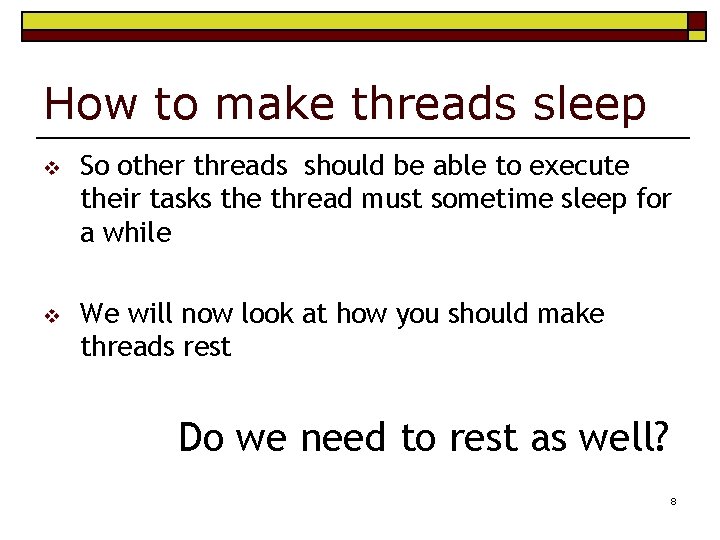
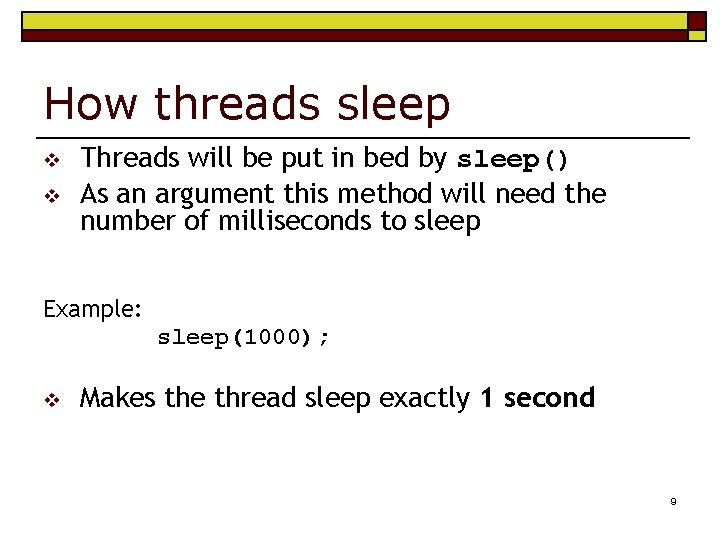
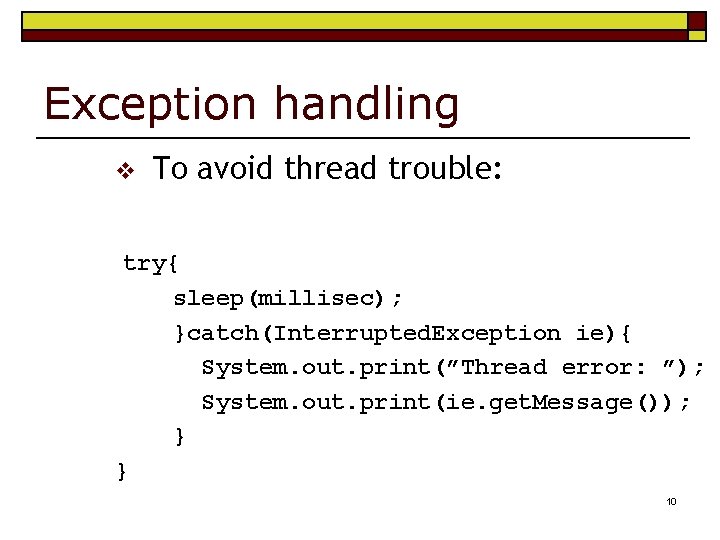
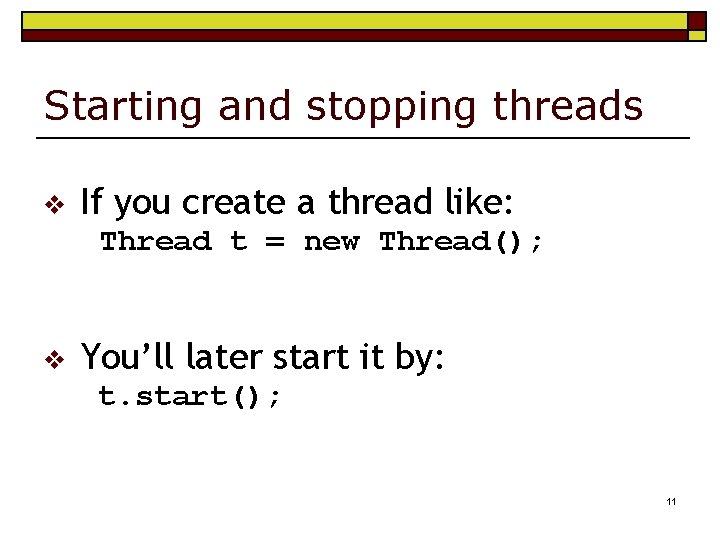
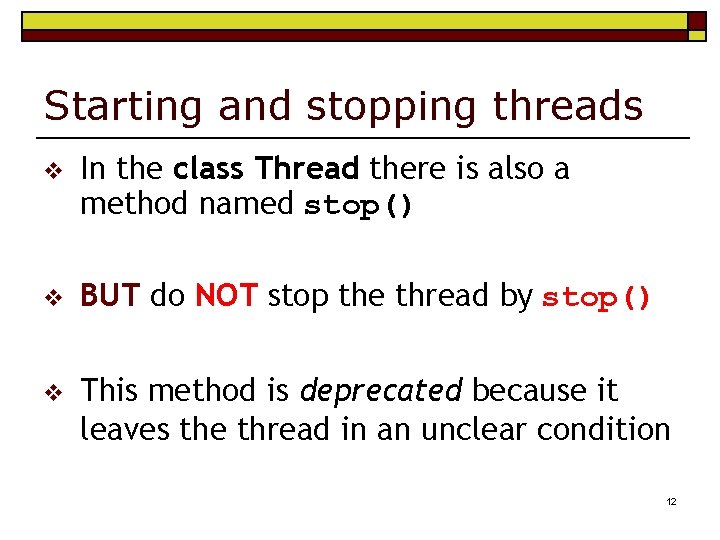
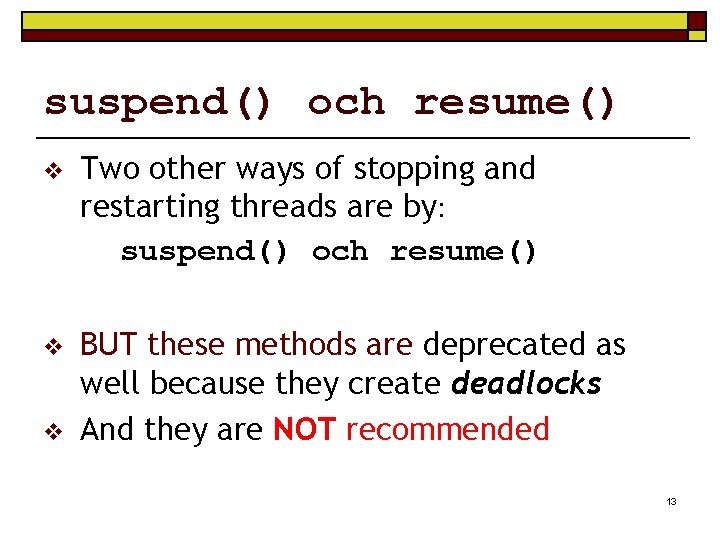
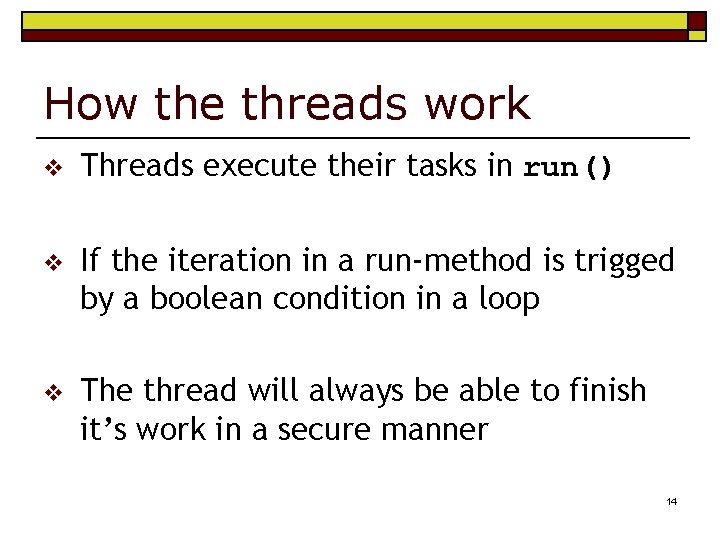
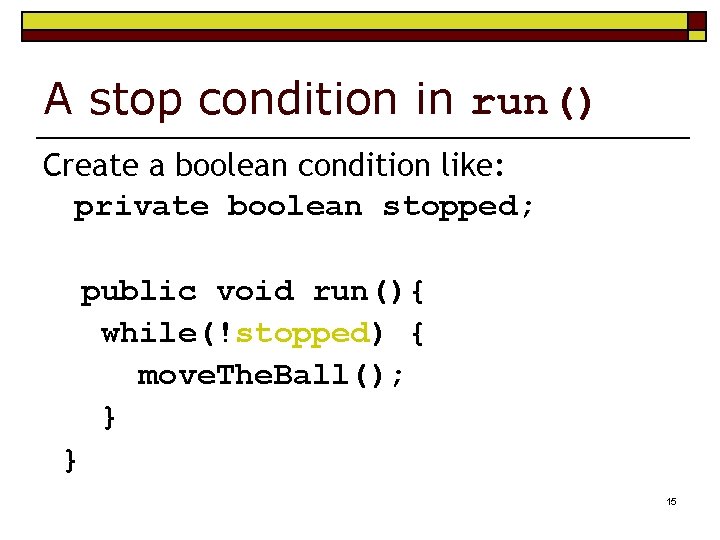
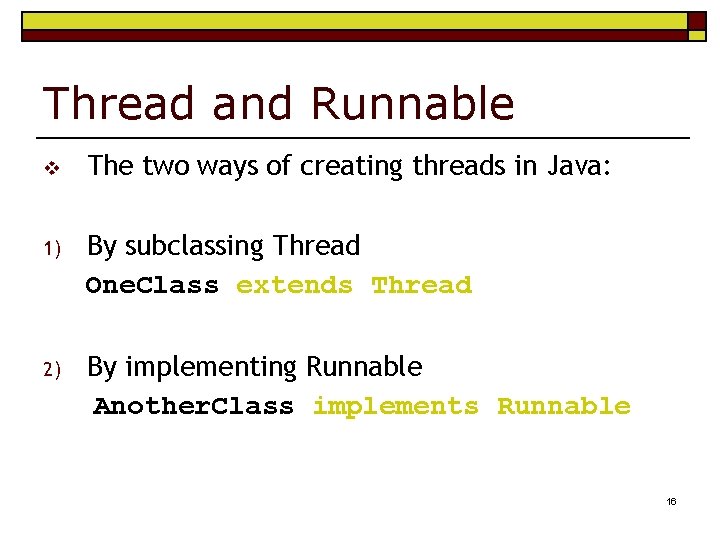
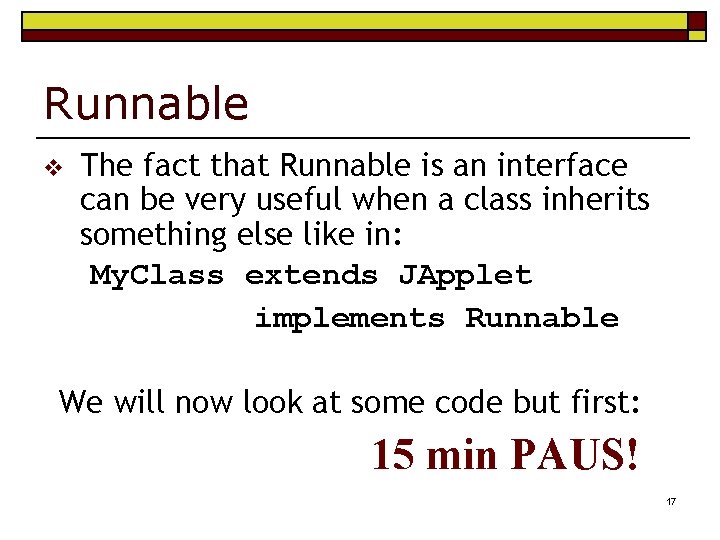
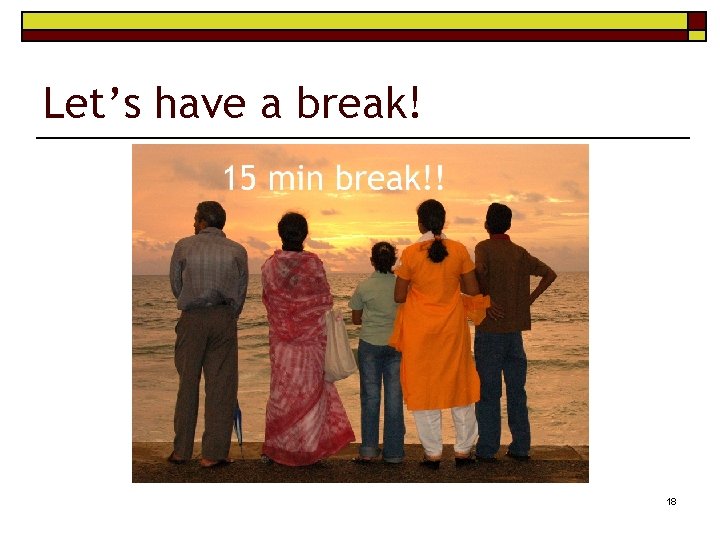
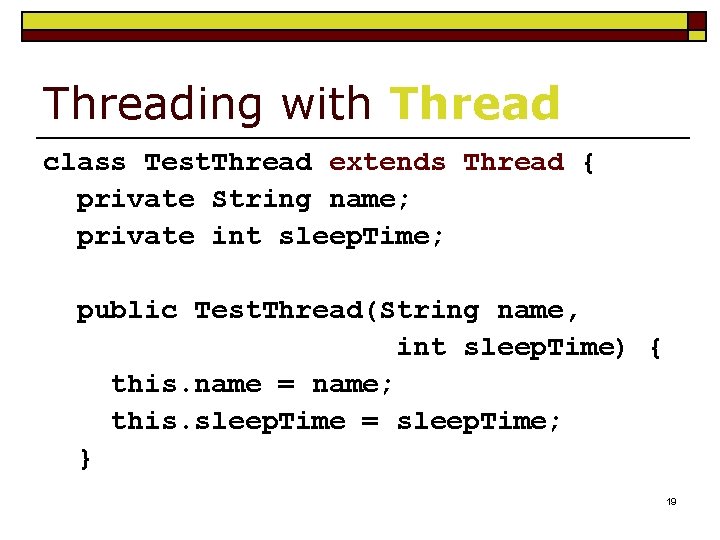
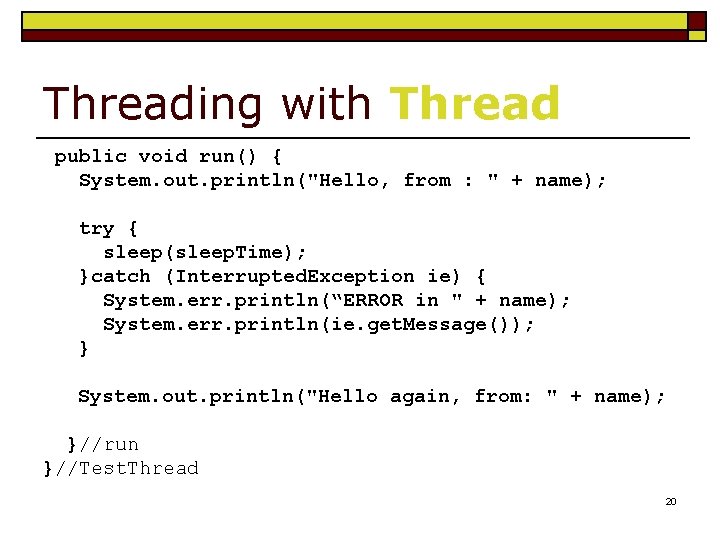
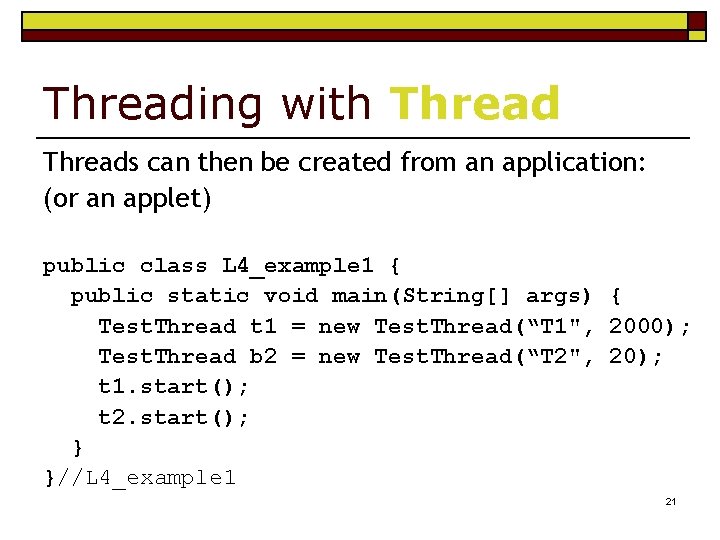
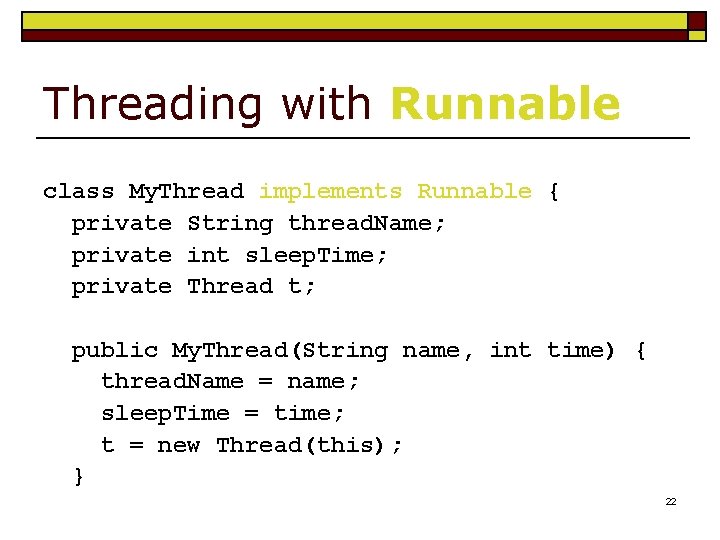
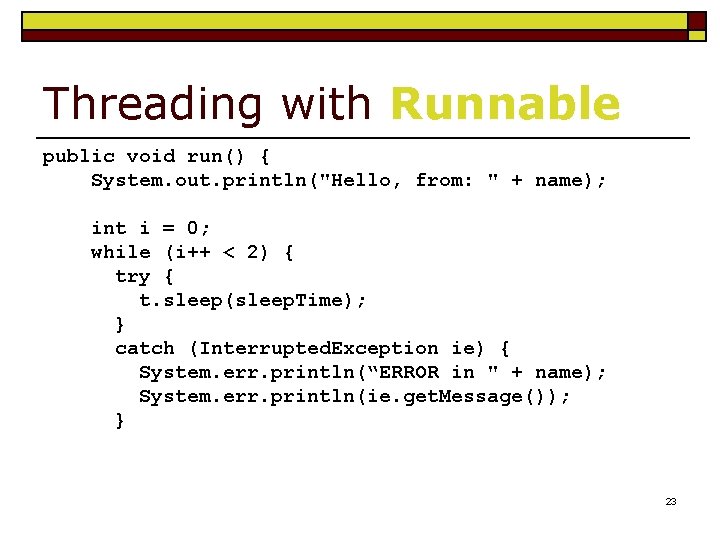
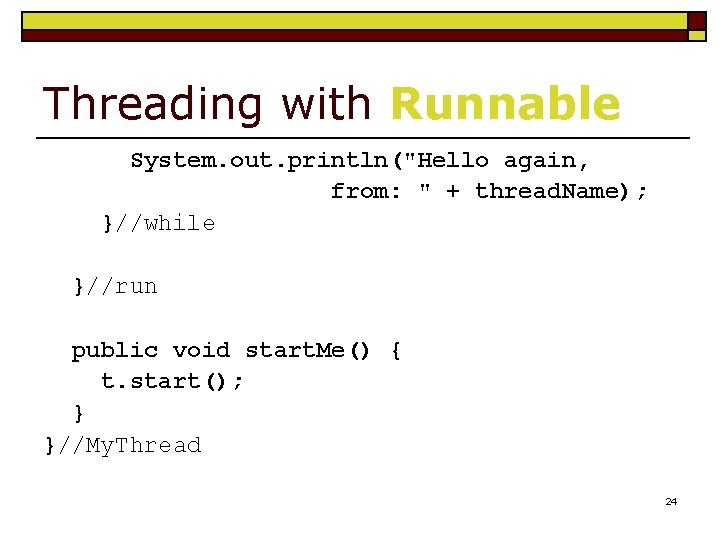
![Threading with Runnable public class L 4_example 2 { public static void main(String[] args) Threading with Runnable public class L 4_example 2 { public static void main(String[] args)](https://slidetodoc.com/presentation_image/4515ebd166267d5b3a0ad08a2a799c41/image-25.jpg)
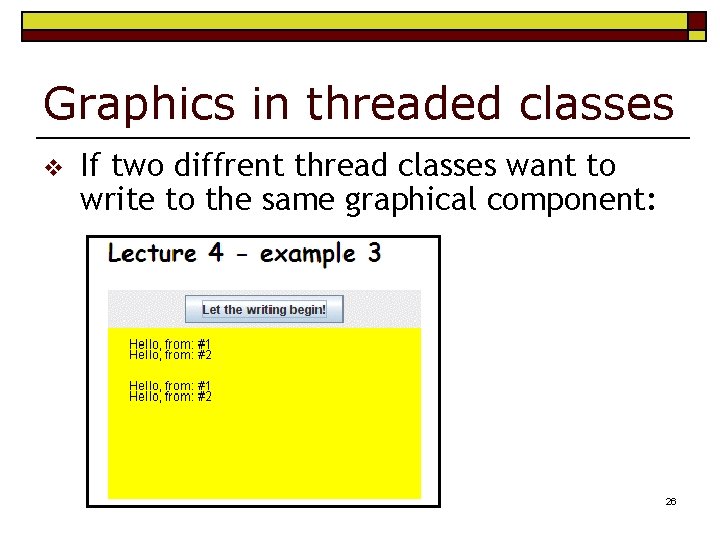
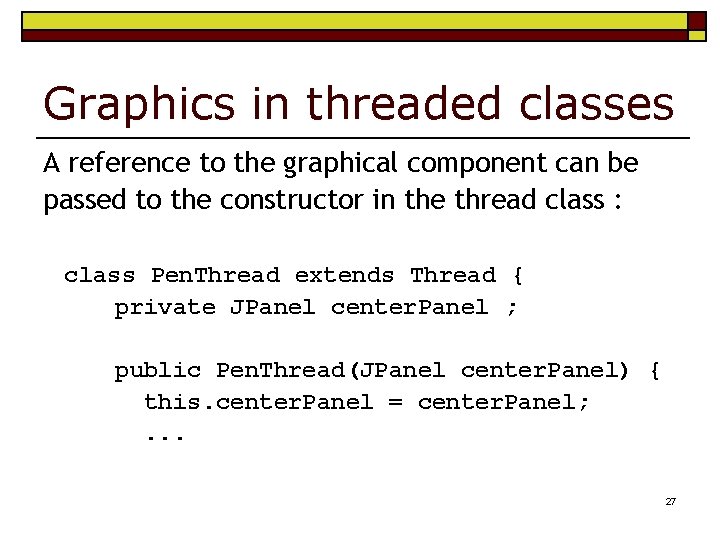
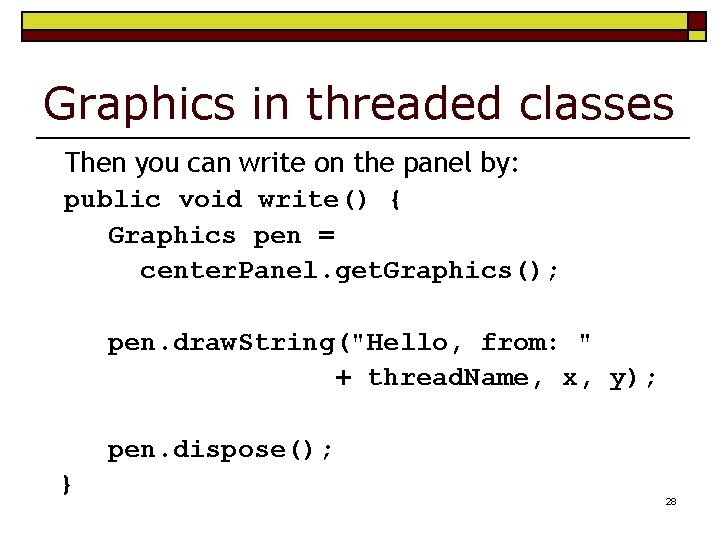
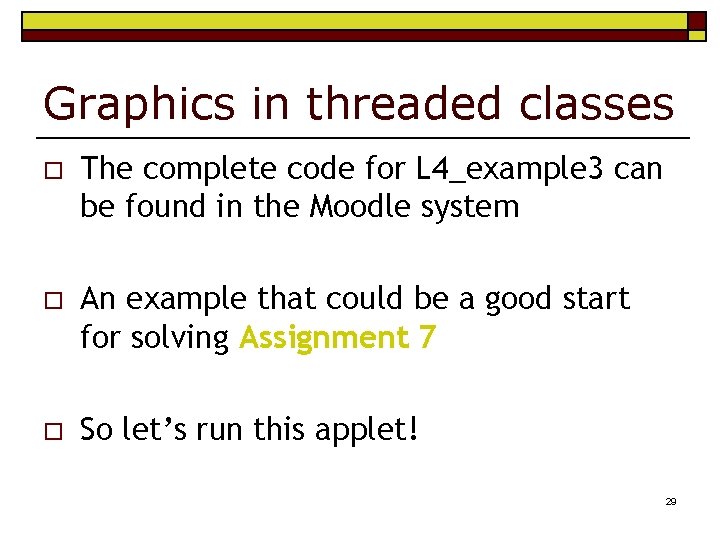
- Slides: 29
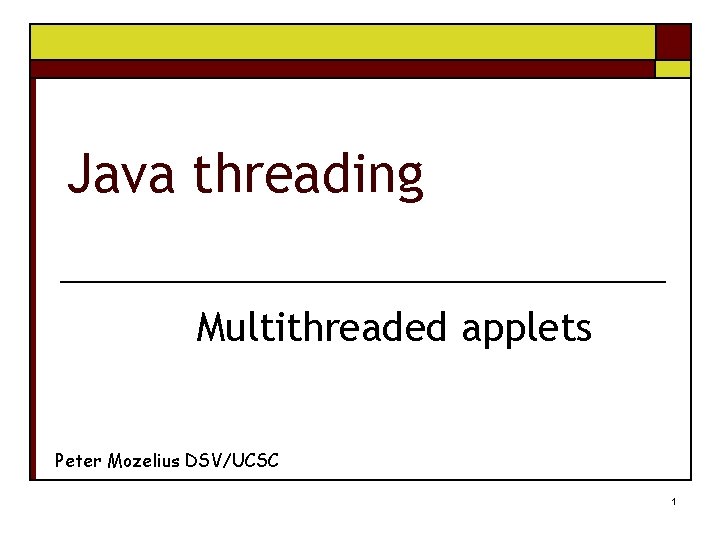
Java threading Multithreaded applets Peter Mozelius DSV/UCSC 1
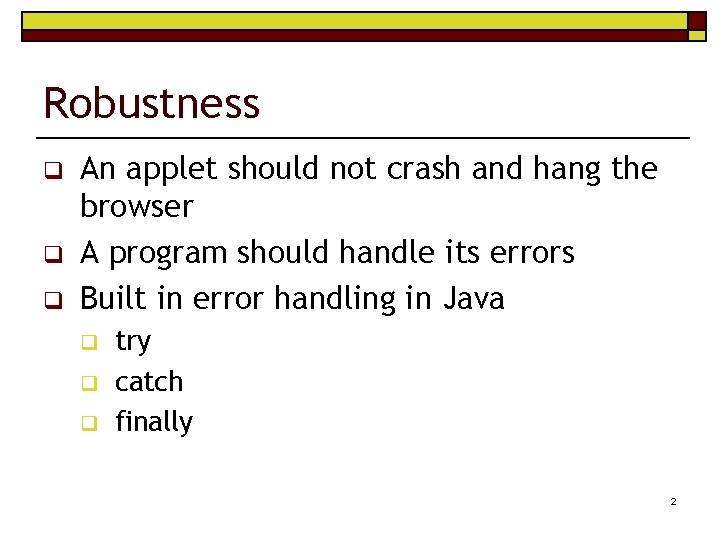
Robustness q q q An applet should not crash and hang the browser A program should handle its errors Built in error handling in Java q q q try catch finally 2
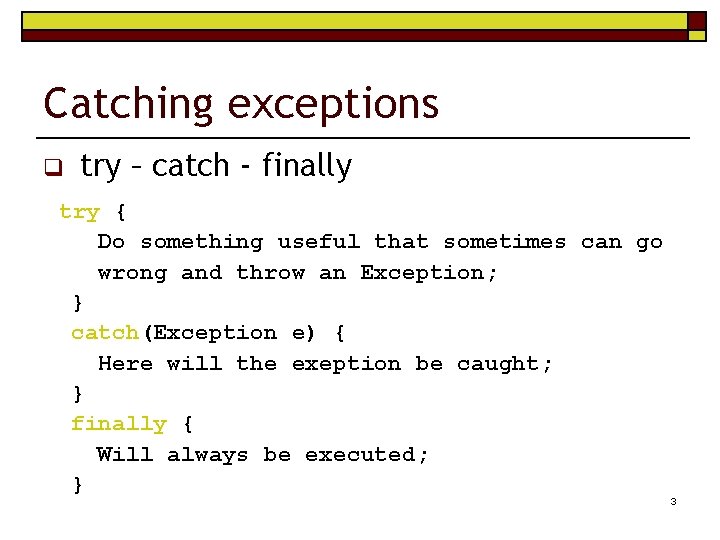
Catching exceptions q try – catch - finally try { Do something useful that sometimes can go wrong and throw an Exception; } catch(Exception e) { Here will the exeption be caught; } finally { Will always be executed; } 3
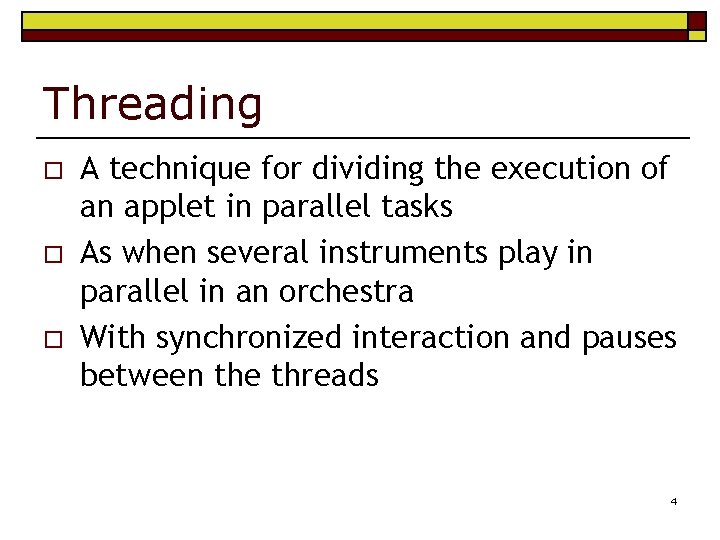
Threading o o o A technique for dividing the execution of an applet in parallel tasks As when several instruments play in parallel in an orchestra With synchronized interaction and pauses between the threads 4
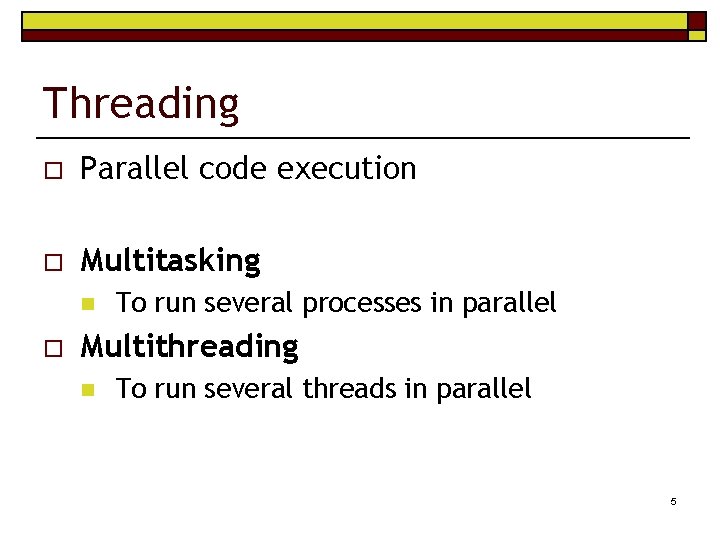
Threading o Parallel code execution o Multitasking n o To run several processes in parallel Multithreading n To run several threads in parallel 5
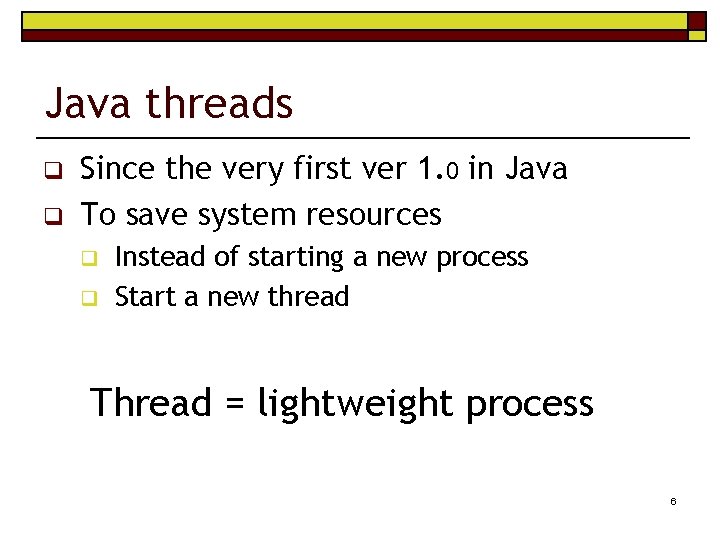
Java threads q q Since the very first ver 1. 0 in Java To save system resources q q Instead of starting a new process Start a new thread Thread = lightweight process 6
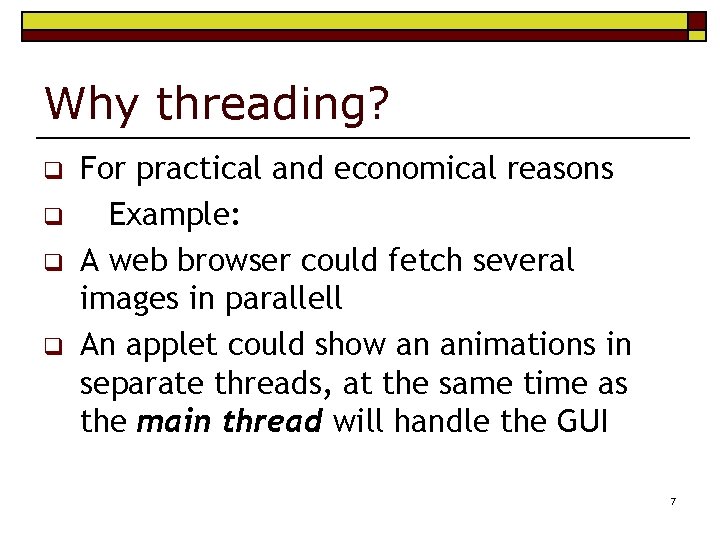
Why threading? q q For practical and economical reasons Example: A web browser could fetch several images in parallell An applet could show an animations in separate threads, at the same time as the main thread will handle the GUI 7
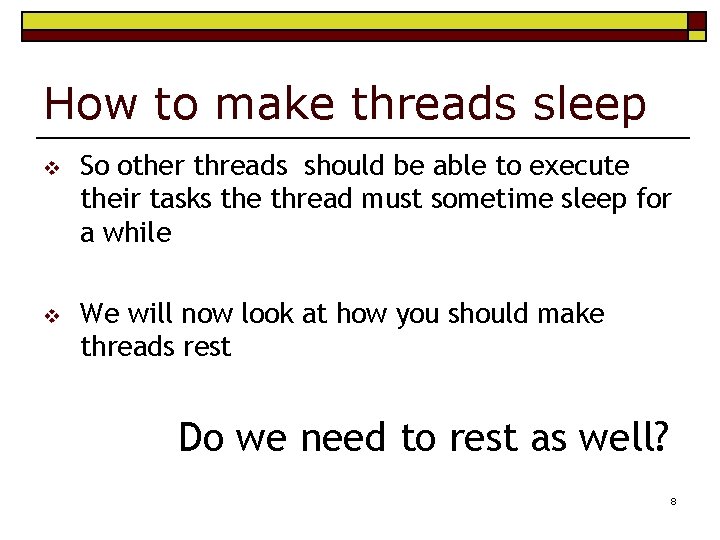
How to make threads sleep v So other threads should be able to execute their tasks the thread must sometime sleep for a while v We will now look at how you should make threads rest Do we need to rest as well? 8
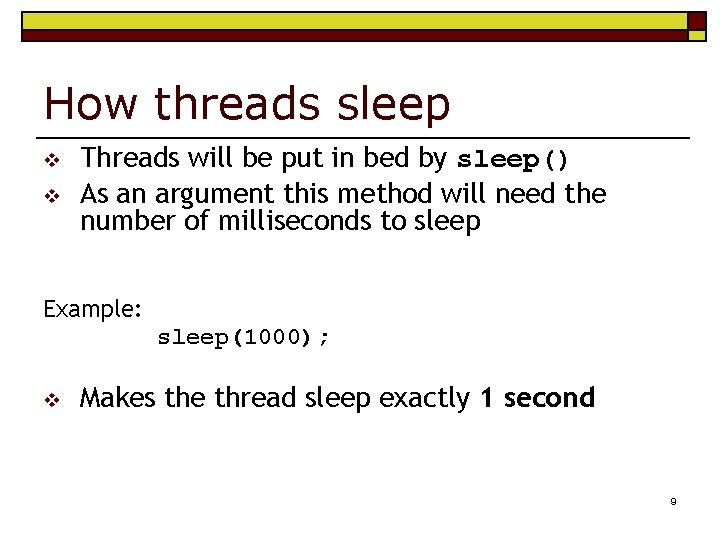
How threads sleep v v Threads will be put in bed by sleep() As an argument this method will need the number of milliseconds to sleep Example: sleep(1000); v Makes the thread sleep exactly 1 second 9
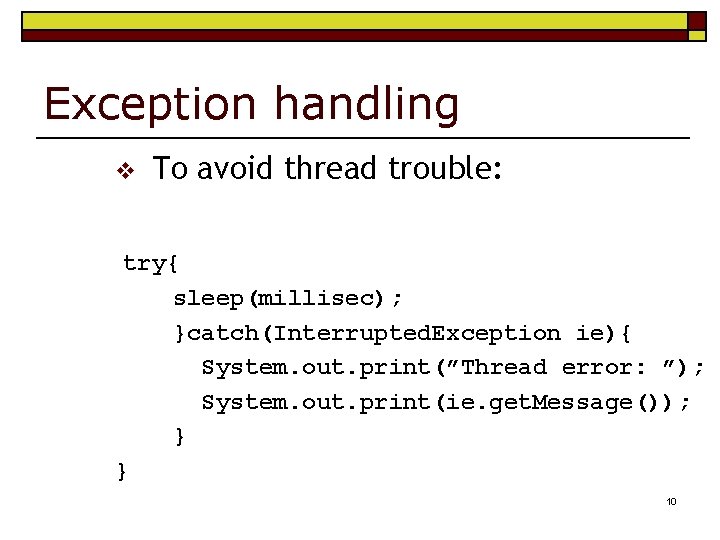
Exception handling v To avoid thread trouble: try{ sleep(millisec); }catch(Interrupted. Exception ie){ System. out. print(”Thread error: ”); System. out. print(ie. get. Message()); } } 10
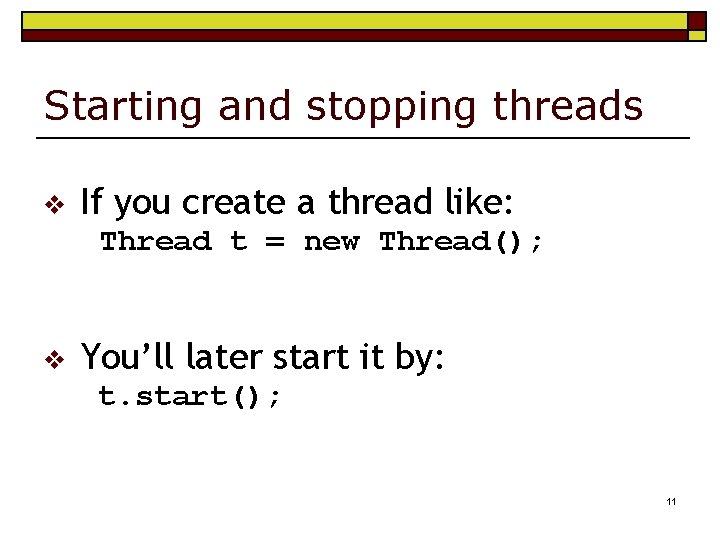
Starting and stopping threads v If you create a thread like: Thread t = new Thread(); v You’ll later start it by: t. start(); 11
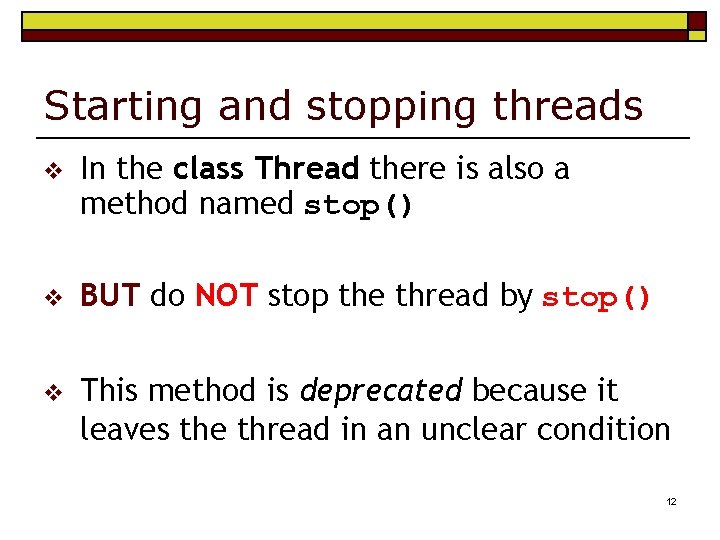
Starting and stopping threads v In the class Thread there is also a method named stop() v BUT do NOT stop the thread by stop() v This method is deprecated because it leaves the thread in an unclear condition 12
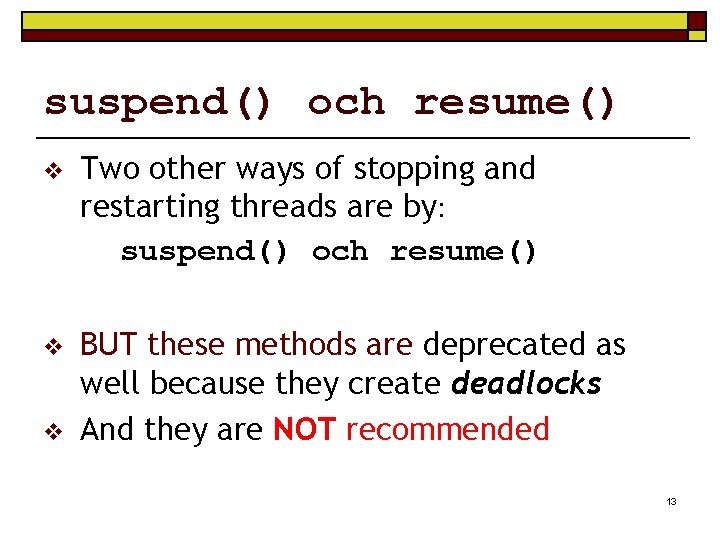
suspend() och resume() v Two other ways of stopping and restarting threads are by: suspend() och resume() v BUT these methods are deprecated as well because they create deadlocks And they are NOT recommended v 13
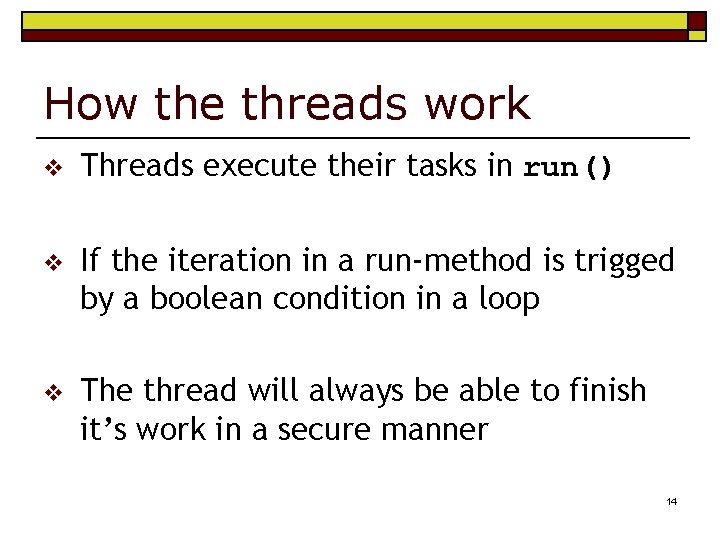
How the threads work v Threads execute their tasks in run() v If the iteration in a run-method is trigged by a boolean condition in a loop v The thread will always be able to finish it’s work in a secure manner 14
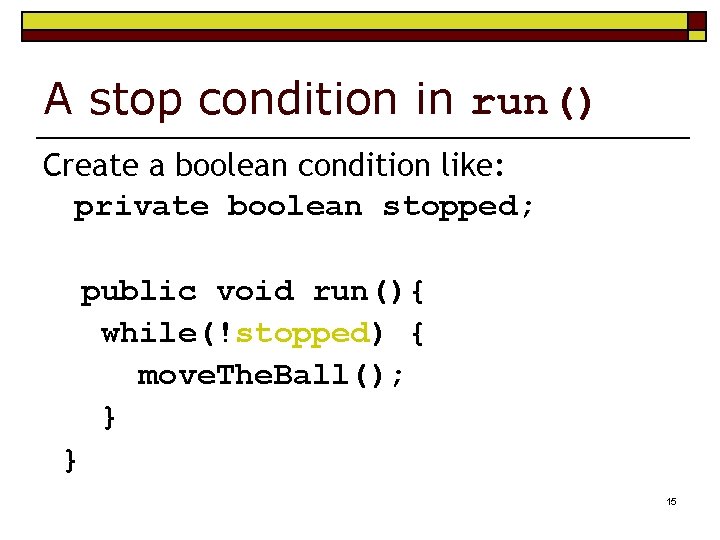
A stop condition in run() Create a boolean condition like: private boolean stopped; public void run(){ while(!stopped) { move. The. Ball(); } } 15
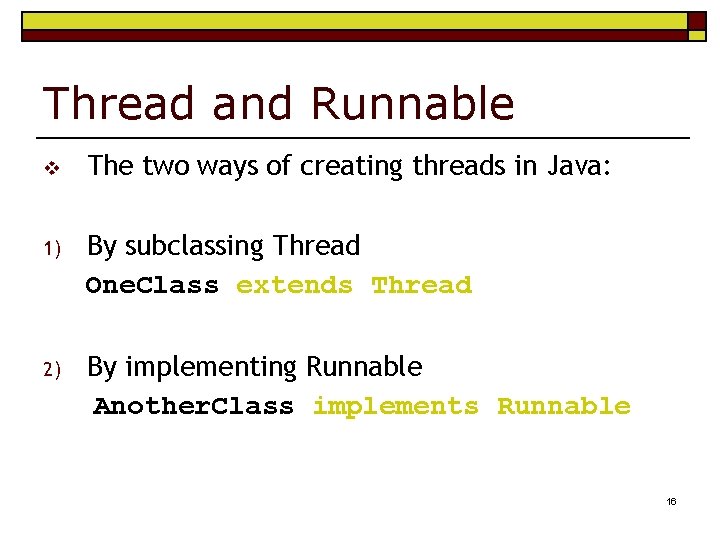
Thread and Runnable v The two ways of creating threads in Java: 1) By subclassing Thread One. Class extends Thread 2) By implementing Runnable Another. Class implements Runnable 16
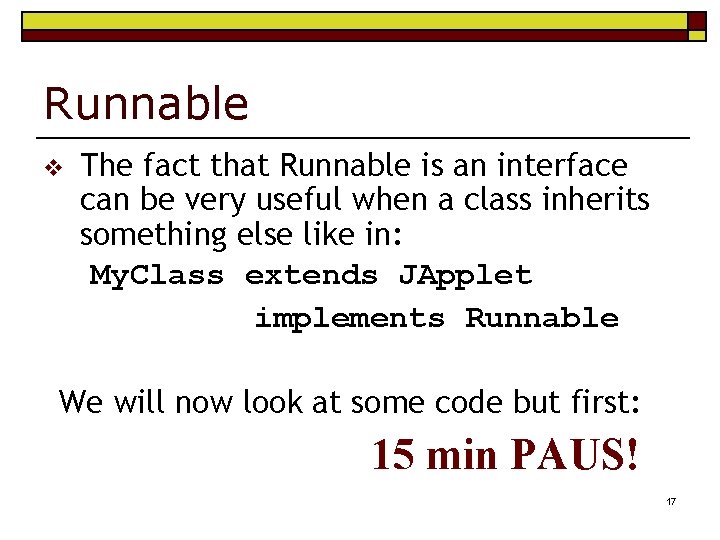
Runnable v The fact that Runnable is an interface can be very useful when a class inherits something else like in: My. Class extends JApplet implements Runnable We will now look at some code but first: 15 min PAUS! 17
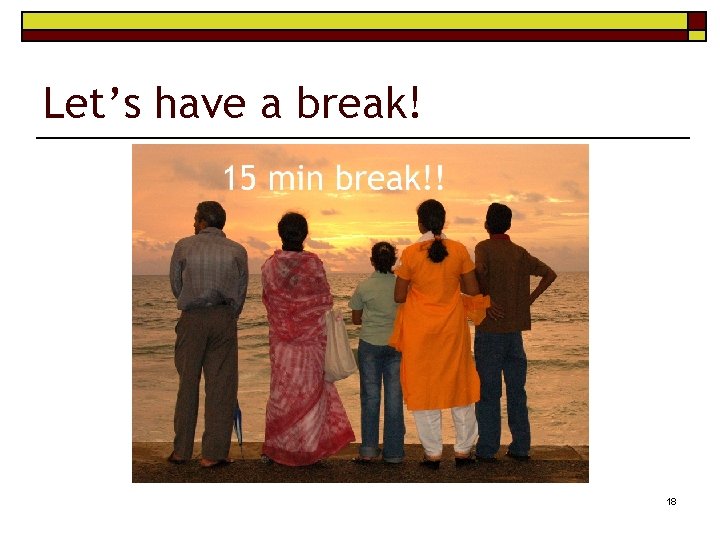
Let’s have a break! 18
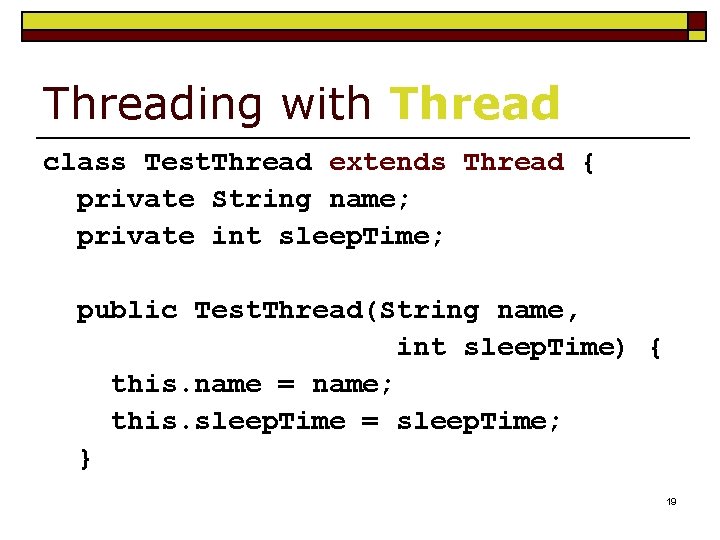
Threading with Thread class Test. Thread extends Thread { private String name; private int sleep. Time; public Test. Thread(String name, int sleep. Time) { this. name = name; this. sleep. Time = sleep. Time; } 19
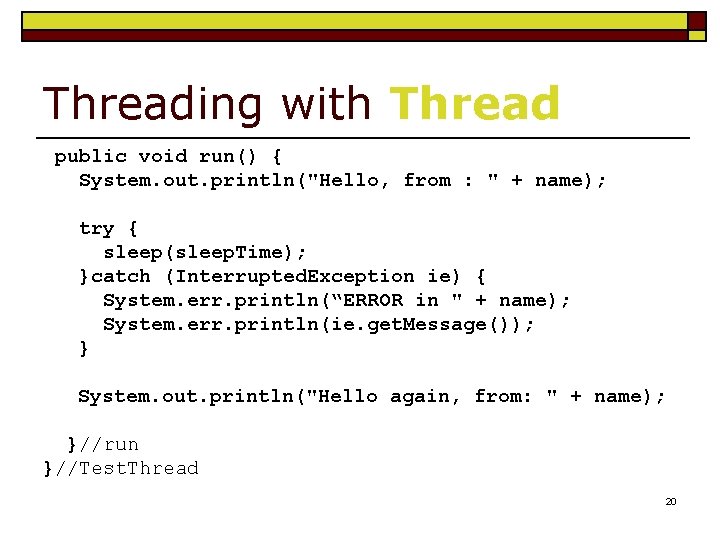
Threading with Thread public void run() { System. out. println("Hello, from : " + name); try { sleep(sleep. Time); }catch (Interrupted. Exception ie) { System. err. println(“ERROR in " + name); System. err. println(ie. get. Message()); } System. out. println("Hello again, from: " + name); }//run }//Test. Thread 20
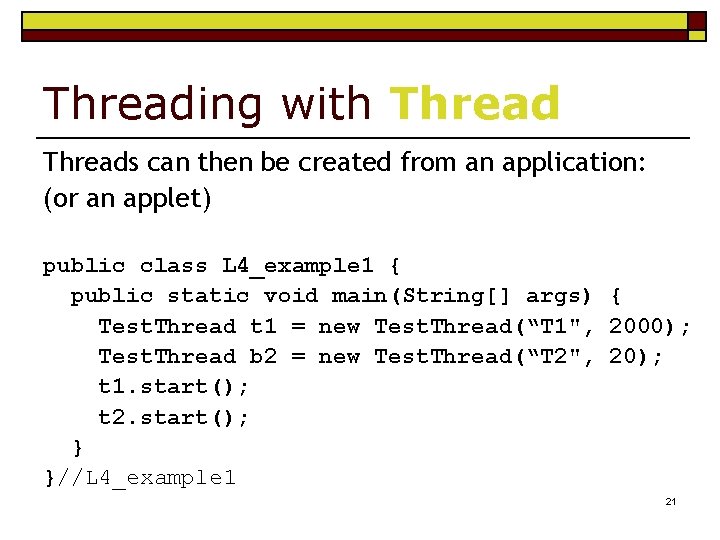
Threading with Threads can then be created from an application: (or an applet) public class L 4_example 1 { public static void main(String[] args) { Test. Thread t 1 = new Test. Thread(“T 1", 2000); Test. Thread b 2 = new Test. Thread(“T 2", 20); t 1. start(); t 2. start(); } }//L 4_example 1 21
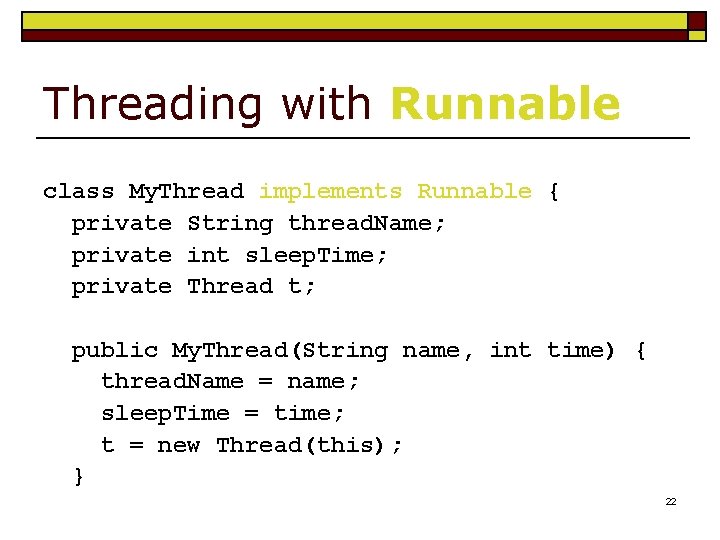
Threading with Runnable class My. Thread implements Runnable { private String thread. Name; private int sleep. Time; private Thread t; public My. Thread(String name, int time) { thread. Name = name; sleep. Time = time; t = new Thread(this); } 22
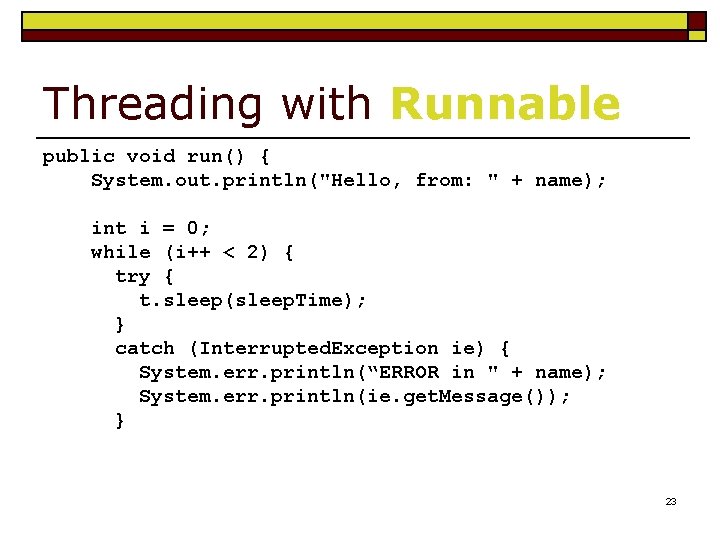
Threading with Runnable public void run() { System. out. println("Hello, from: " + name); int i = 0; while (i++ < 2) { try { t. sleep(sleep. Time); } catch (Interrupted. Exception ie) { System. err. println(“ERROR in " + name); System. err. println(ie. get. Message()); } 23
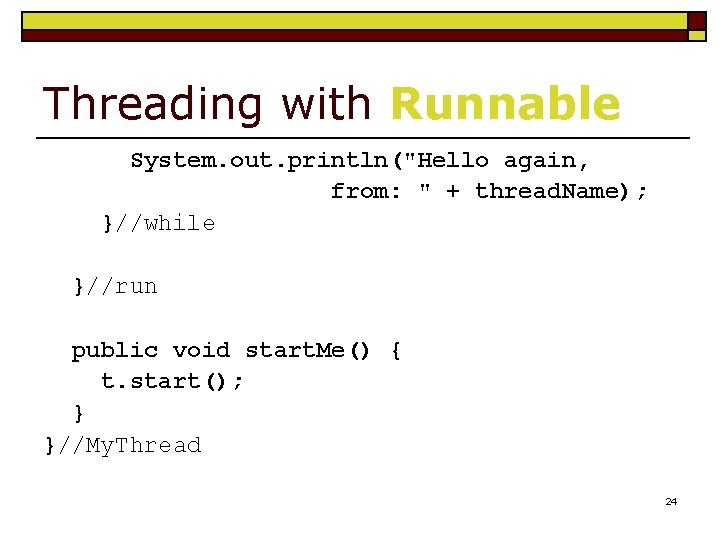
Threading with Runnable System. out. println("Hello again, from: " + thread. Name); }//while }//run public void start. Me() { t. start(); } }//My. Thread 24
![Threading with Runnable public class L 4example 2 public static void mainString args Threading with Runnable public class L 4_example 2 { public static void main(String[] args)](https://slidetodoc.com/presentation_image/4515ebd166267d5b3a0ad08a2a799c41/image-25.jpg)
Threading with Runnable public class L 4_example 2 { public static void main(String[] args) { My. Thread t 1 = new My. Thread("T 1", 2000); My. Thread t 2 = new My. Thread("T 2", 20); t 1. start. Me(); t 2. start. Me(); } }//L 4_example 2 25
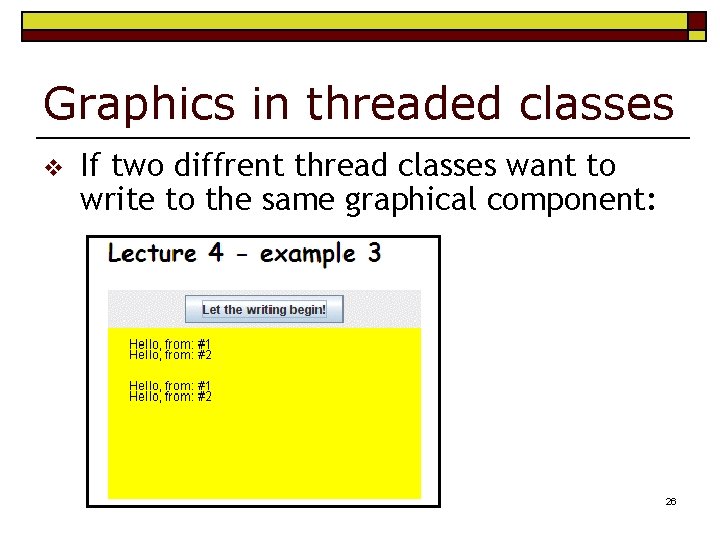
Graphics in threaded classes v If two diffrent thread classes want to write to the same graphical component: 26
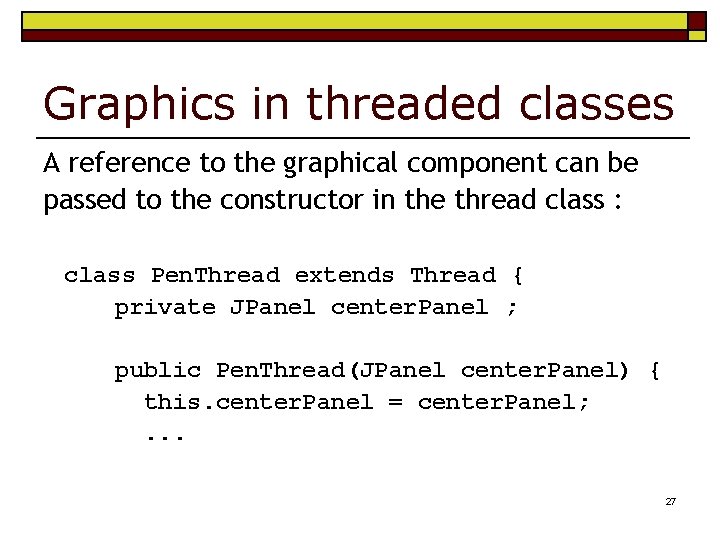
Graphics in threaded classes A reference to the graphical component can be passed to the constructor in the thread class : class Pen. Thread extends Thread { private JPanel center. Panel ; public Pen. Thread(JPanel center. Panel) { this. center. Panel = center. Panel; . . . 27
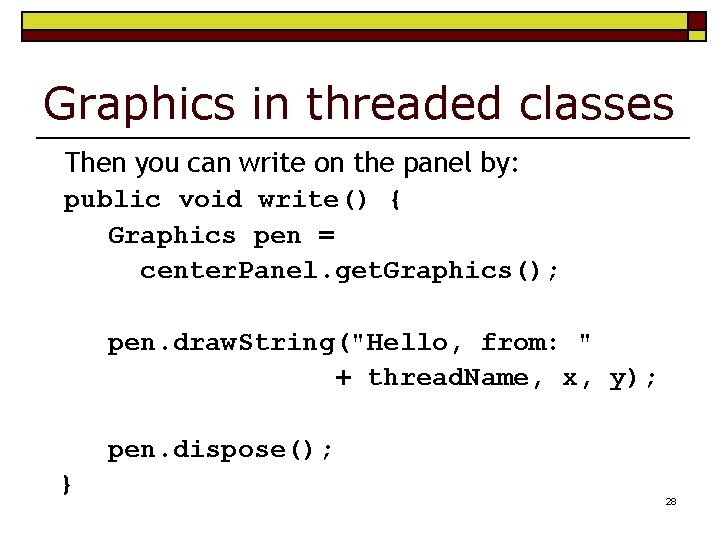
Graphics in threaded classes Then you can write on the panel by: public void write() { Graphics pen = center. Panel. get. Graphics(); pen. draw. String("Hello, from: " + thread. Name, x, y); pen. dispose(); } 28
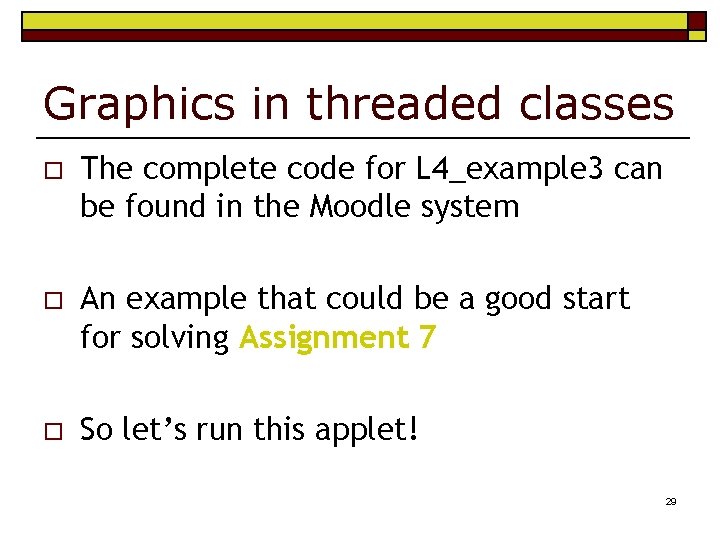
Graphics in threaded classes o The complete code for L 4_example 3 can be found in the Moodle system o An example that could be a good start for solving Assignment 7 o So let’s run this applet! 29
Peter mozelius
Wisweb applets
Bouwgeschillen oplossen
Rossmanchance
Erik poll
Jumlah level dalam gambar ini adalah . . . .
Multithreaded programming languages
Multithreaded games
Multithreaded algorithms
Apt multithreaded
Movable plate that covers the shuttle and bobbin case
What are the cutting tools
Cnabd
Hyper threading
Frunnable
Microprocessor without interlocked pipeline stages
Com threading model
Huber
Hyper threading
Reaming process
Hyper threading
Hyper threading
Java import java.util.*
Import java.util.*
Import java.awt.* import java.applet.*
Import java.util.*
Import java.util.*
Java import java.util.*
Java random
Import java.io.*