Java Security Petr Admek Content Java Security Java
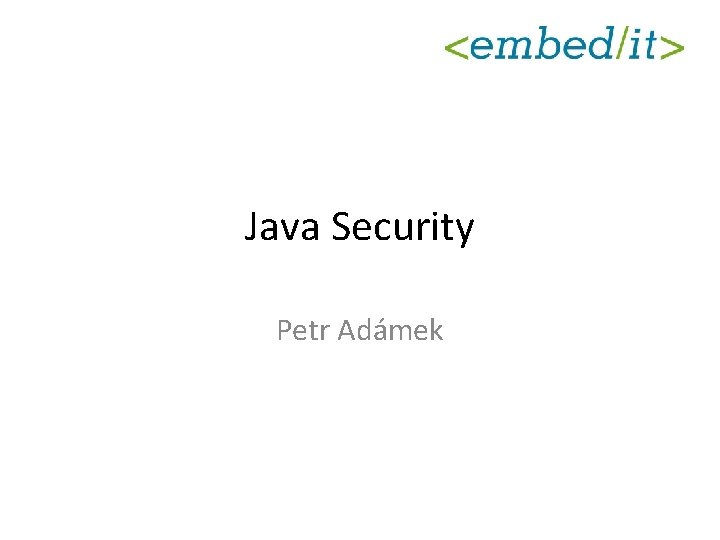
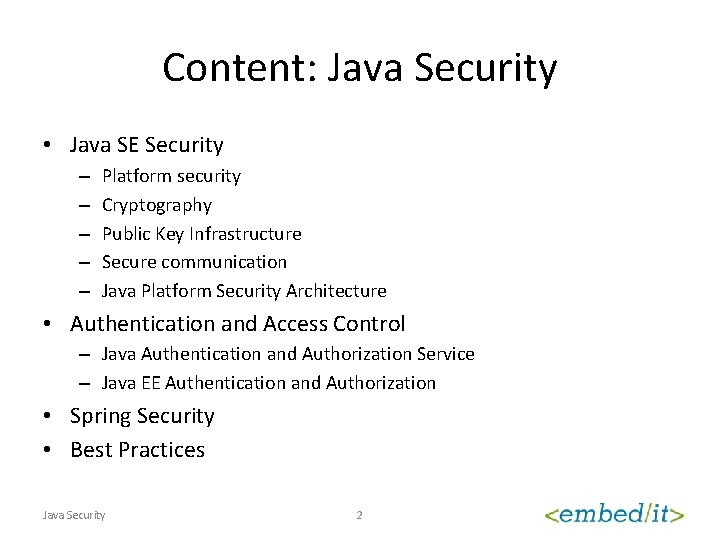
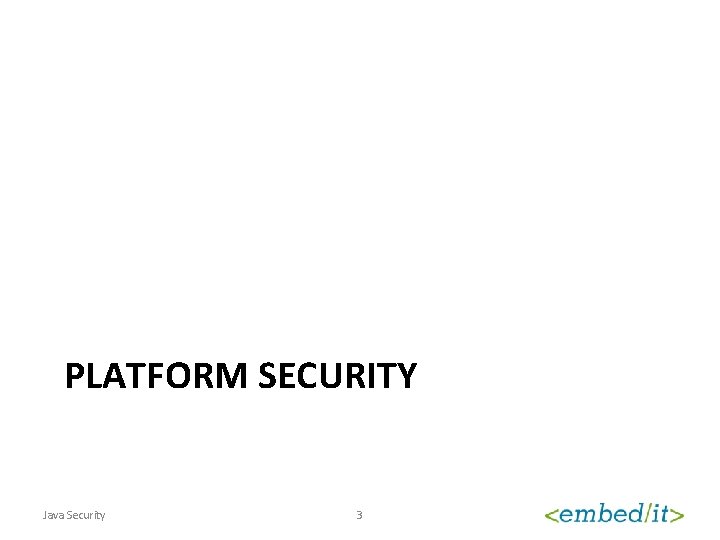
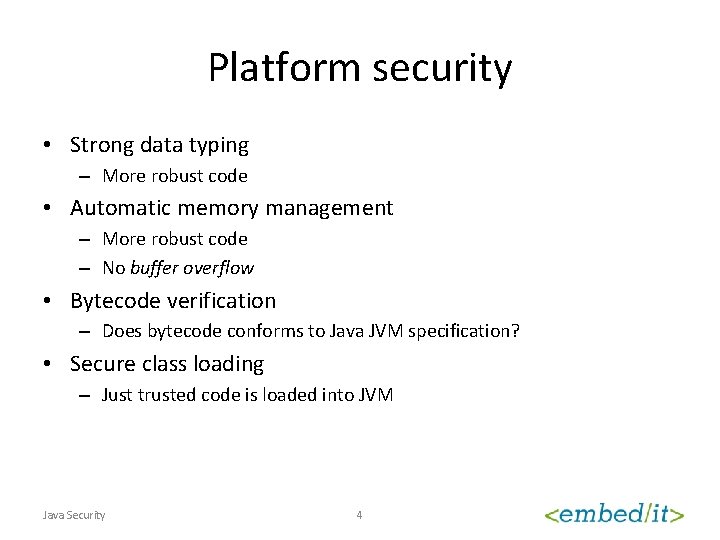
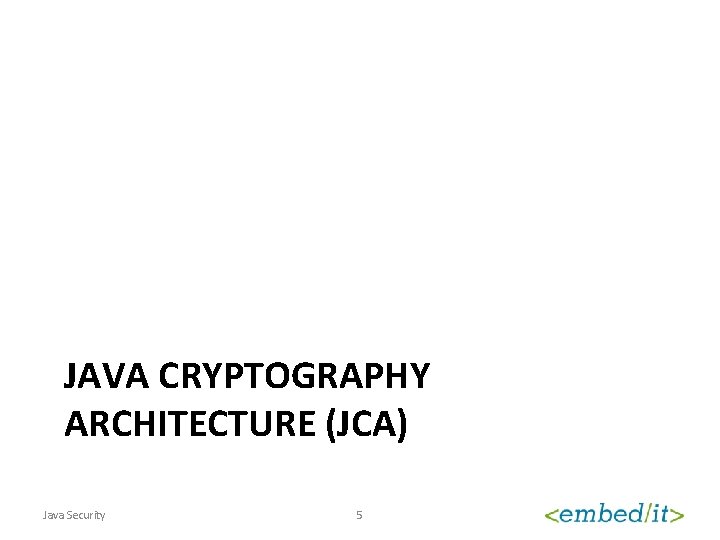
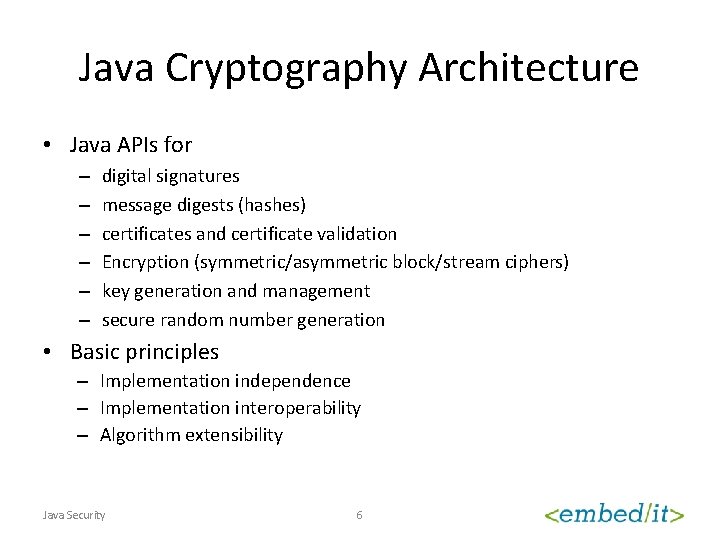
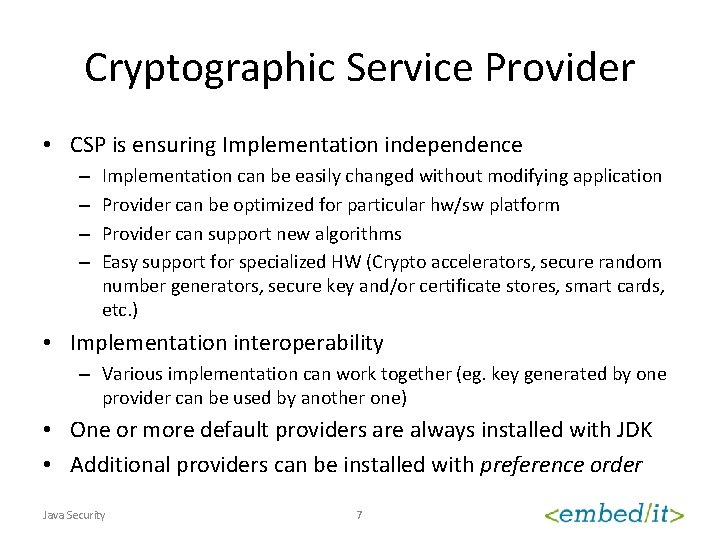
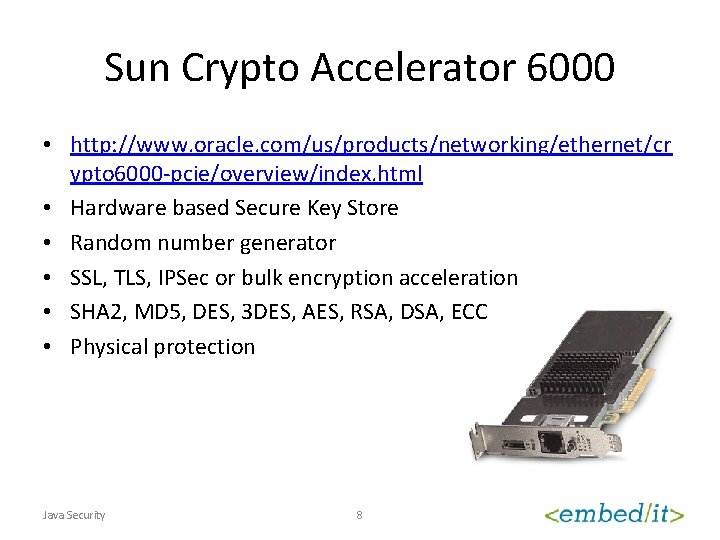
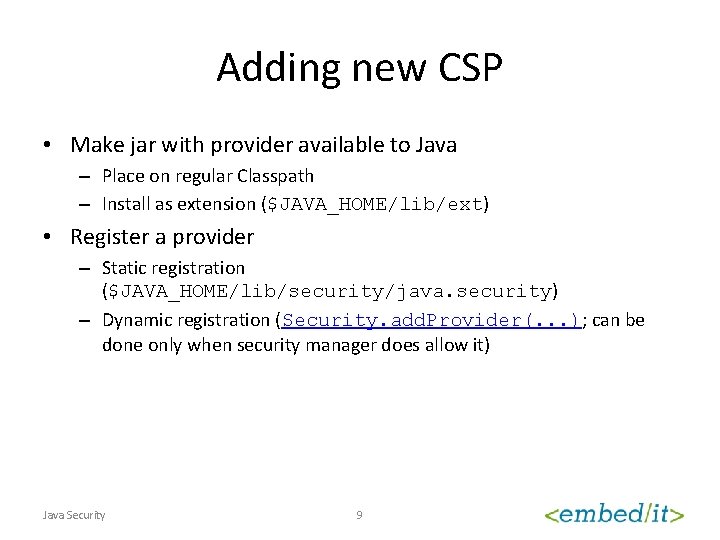
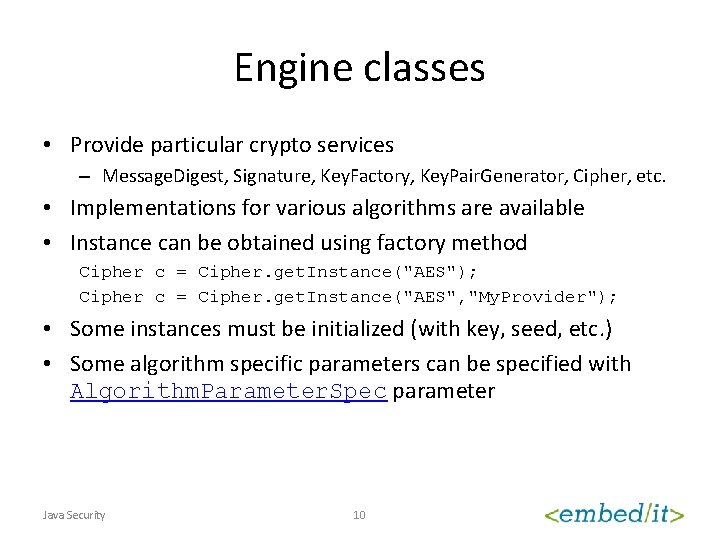
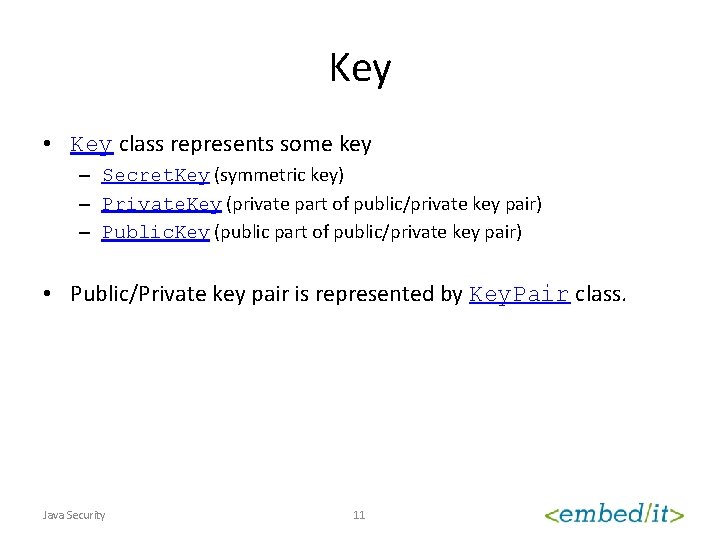
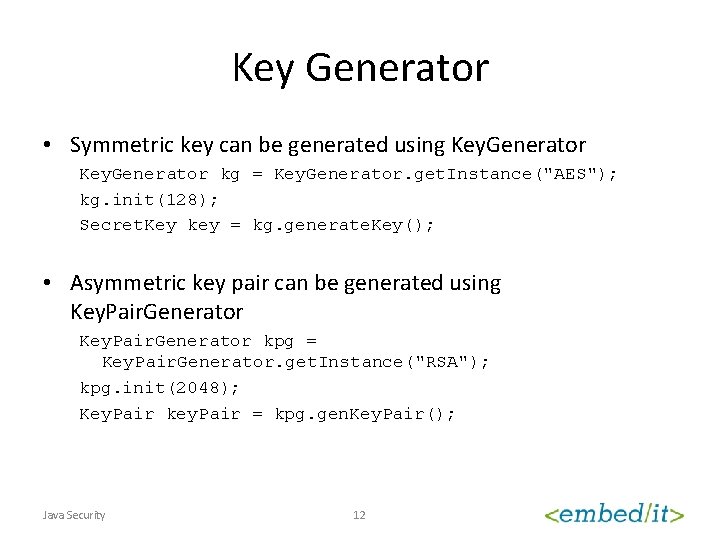
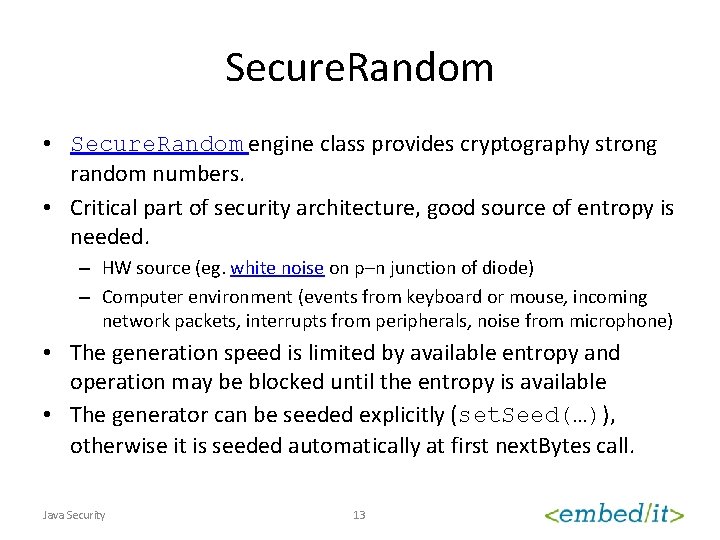
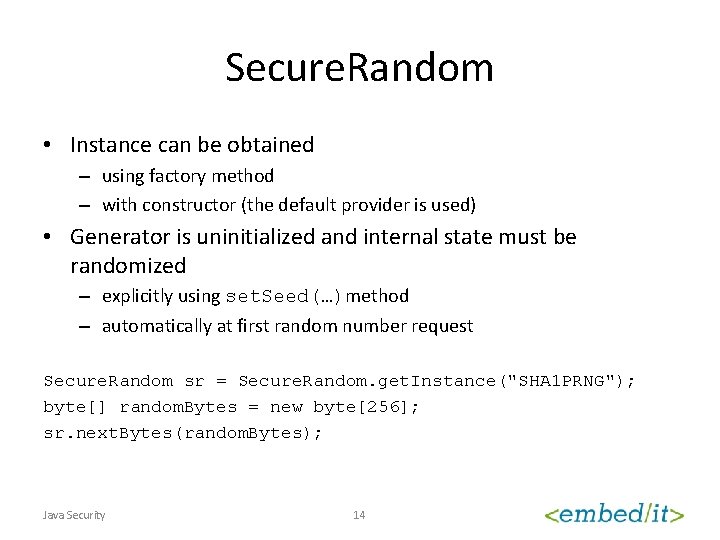
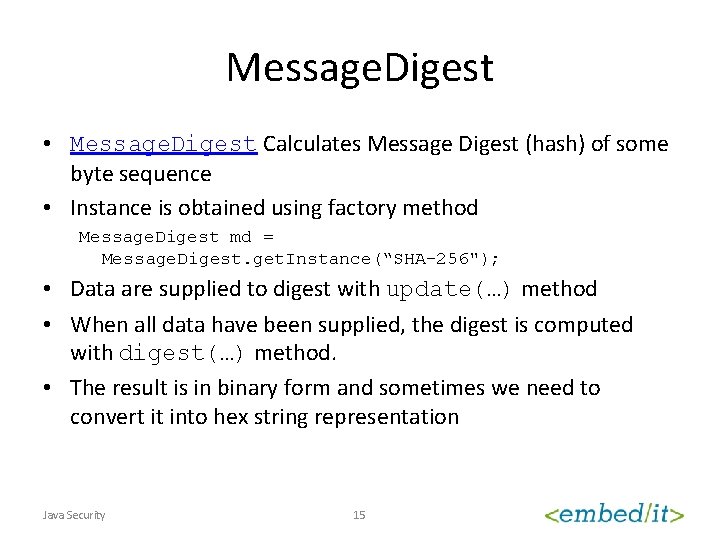
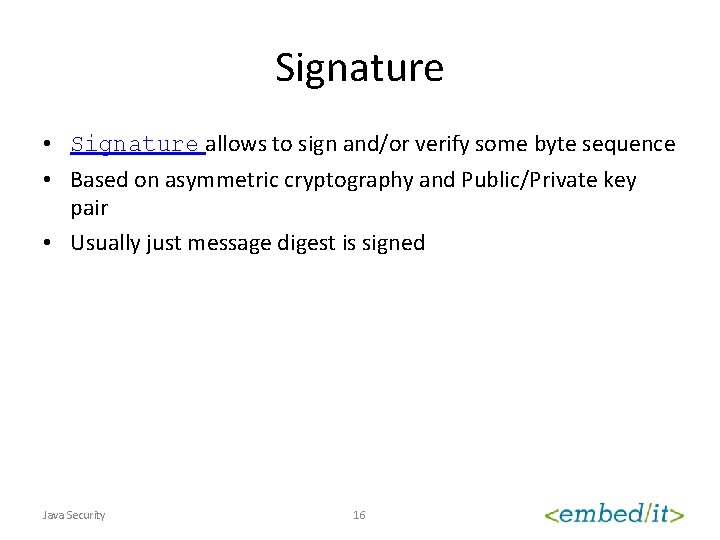
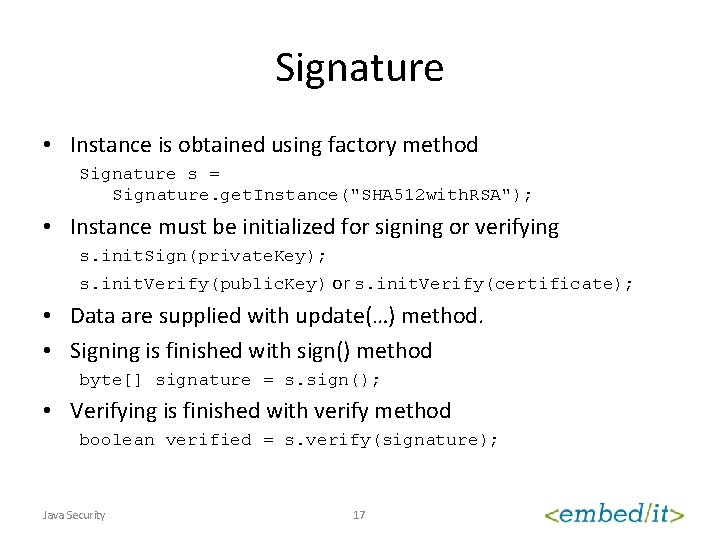
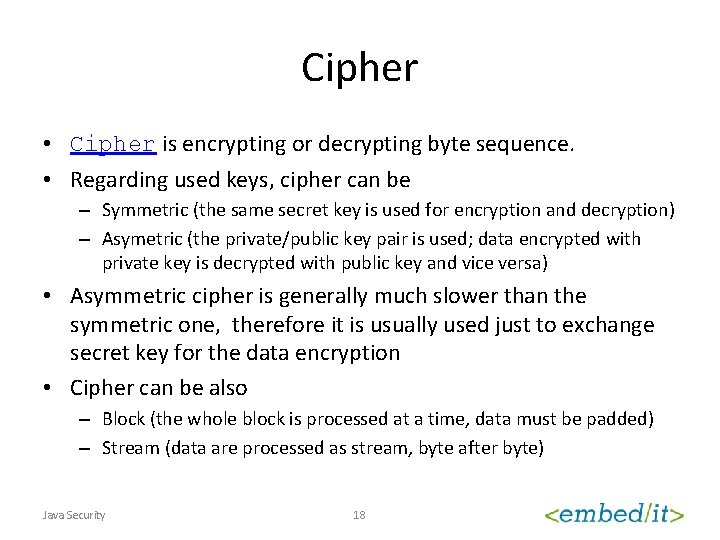
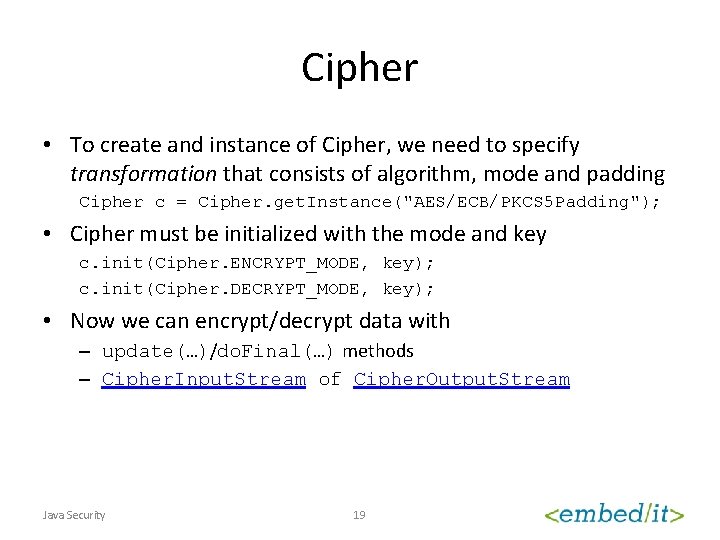
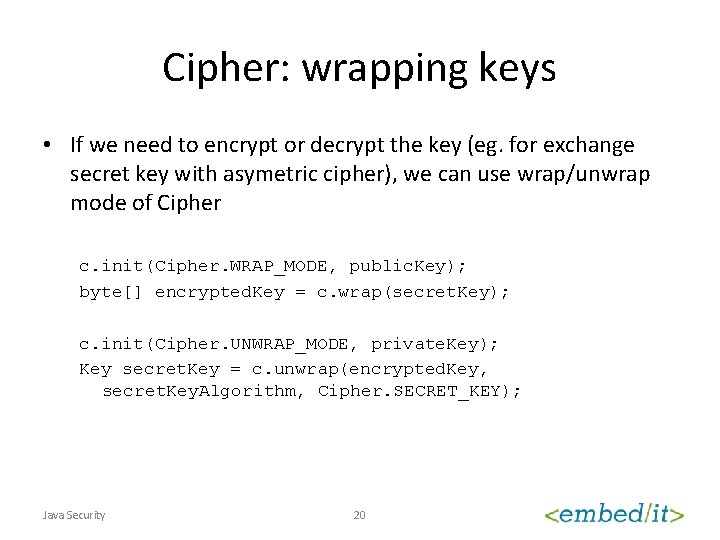
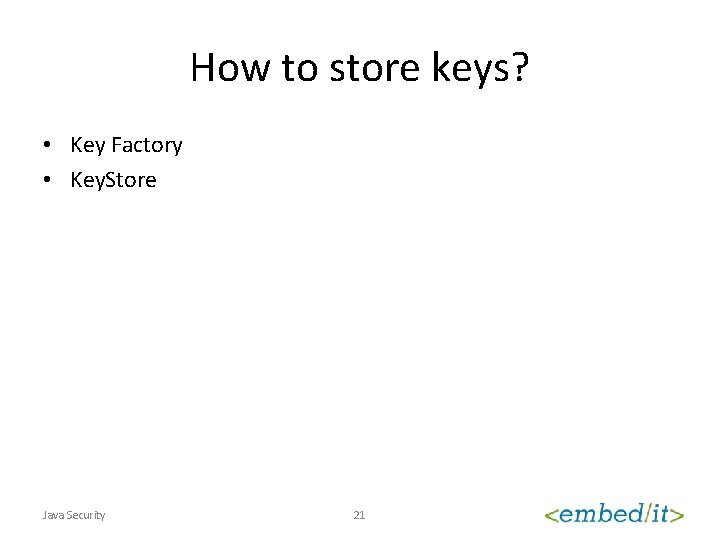
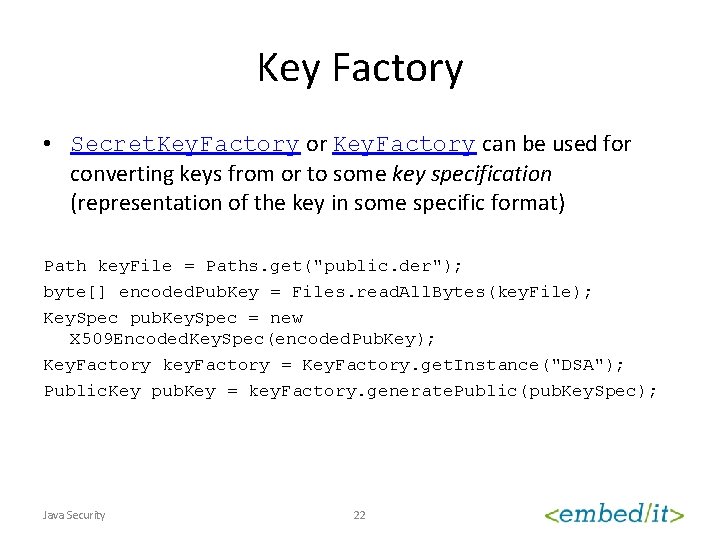
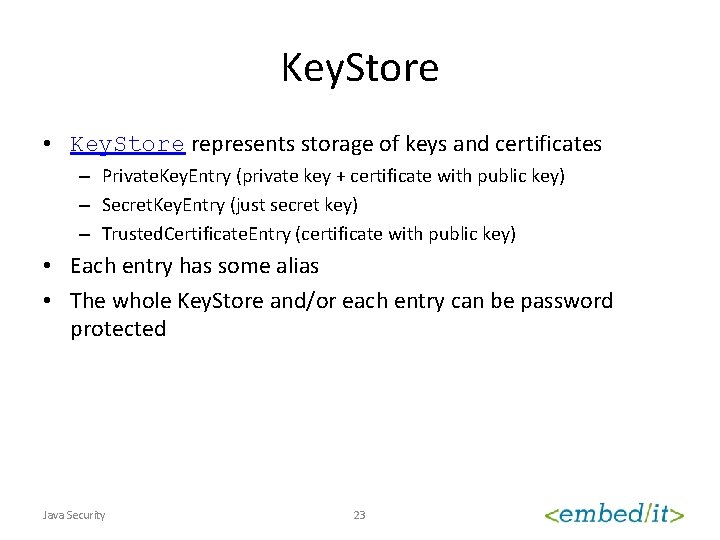
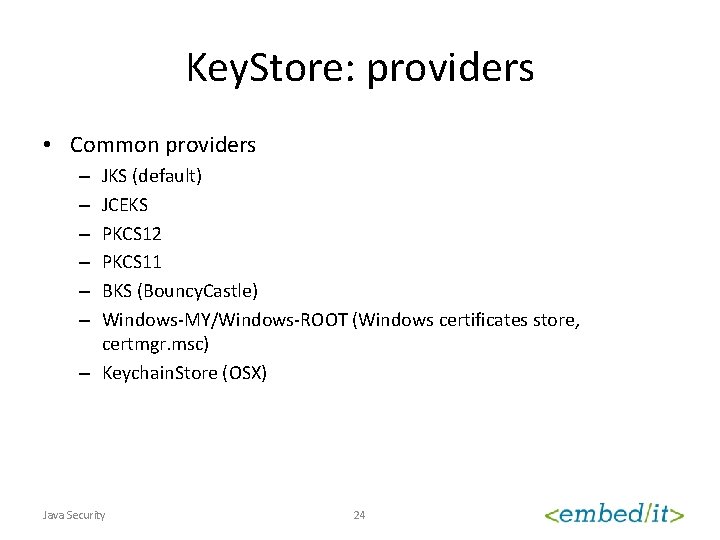
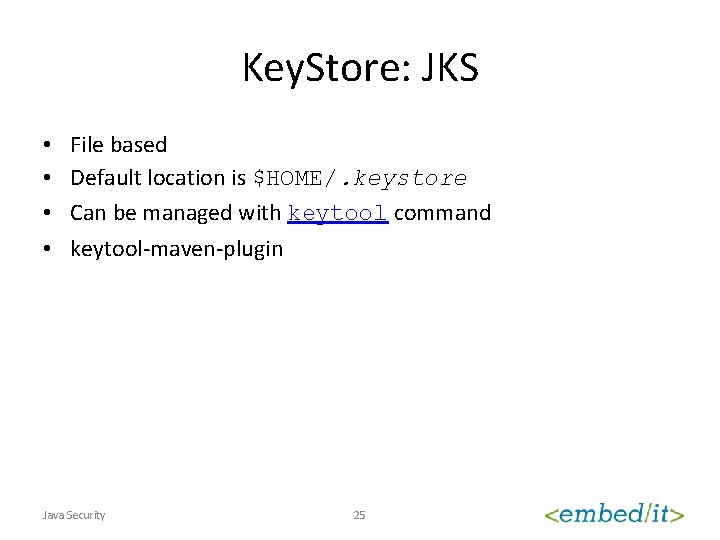
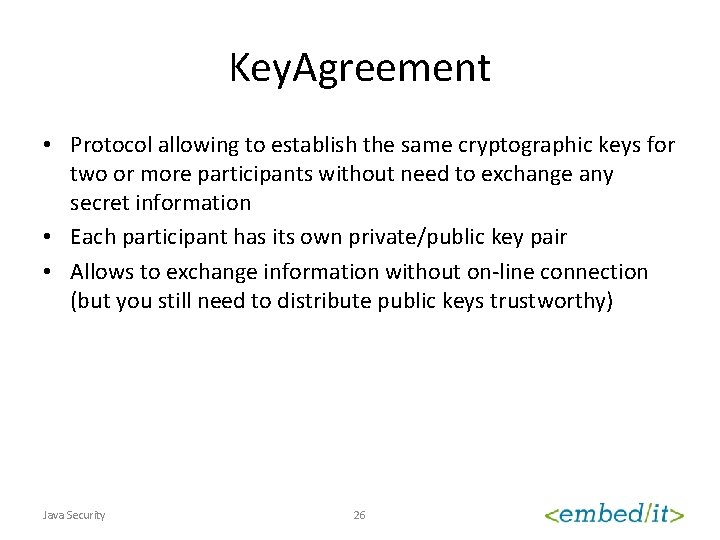
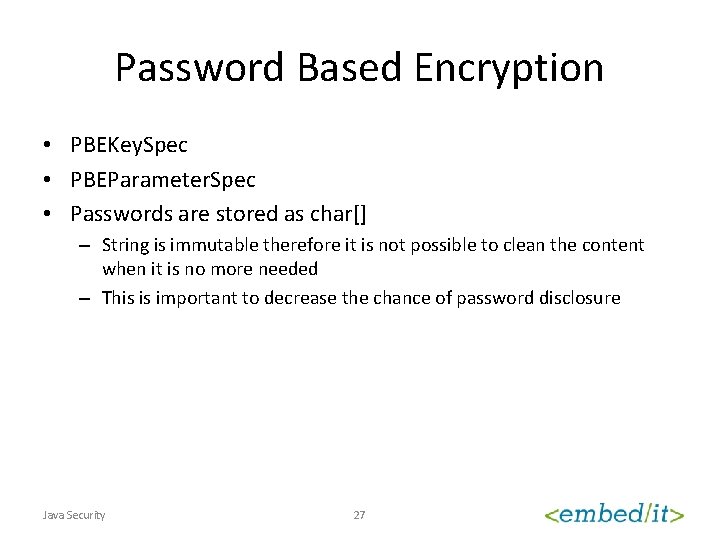
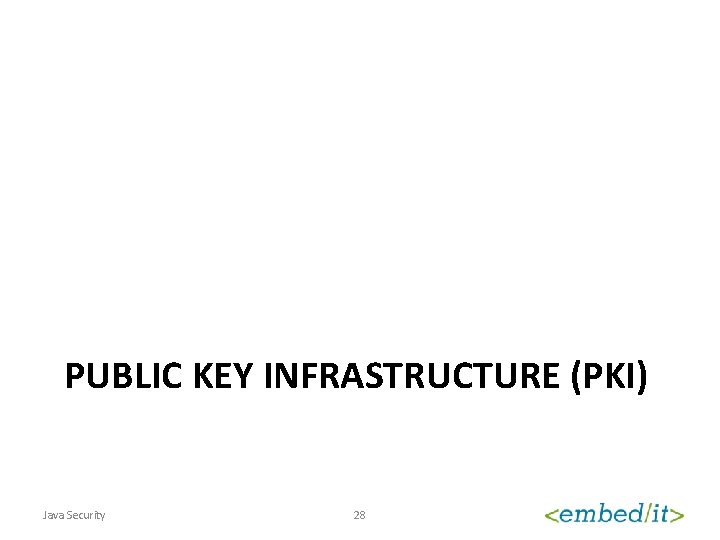
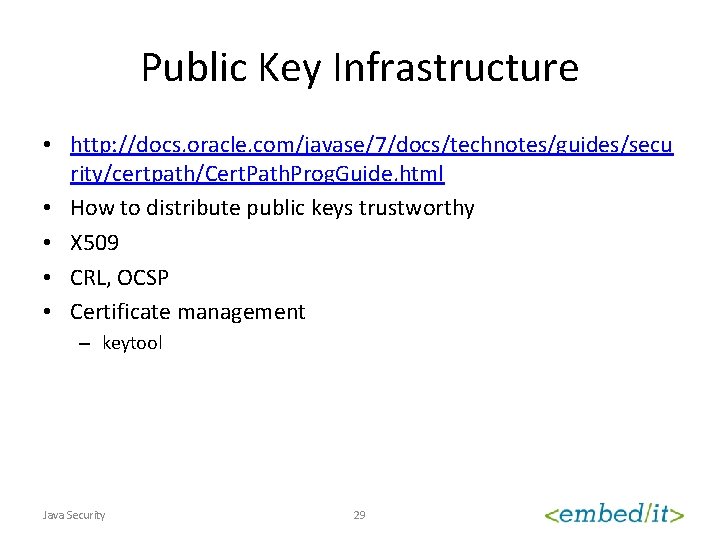
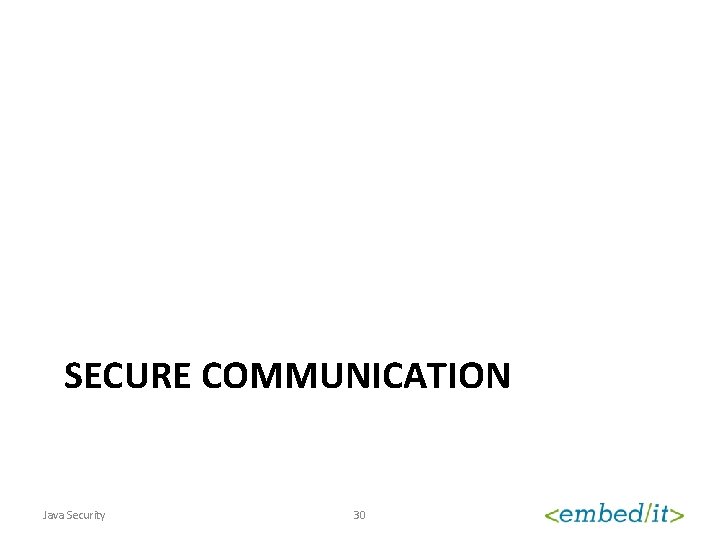
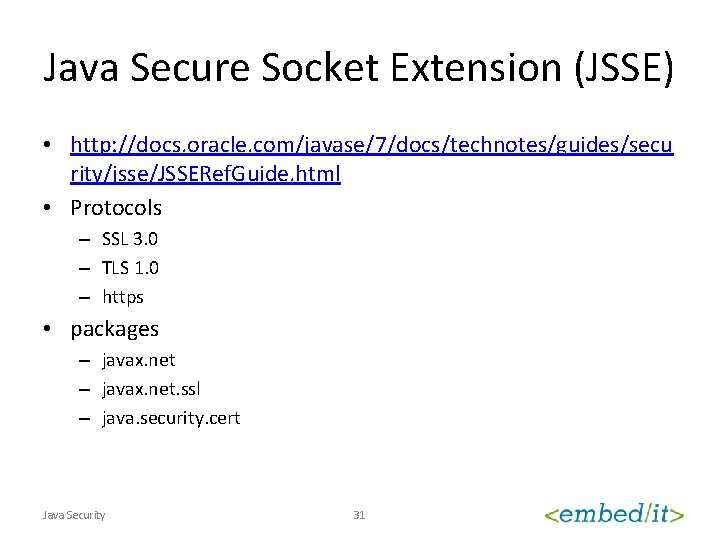
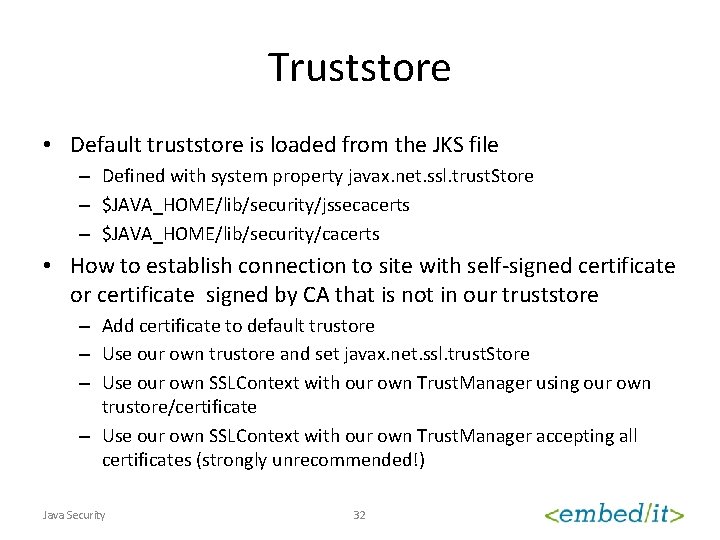
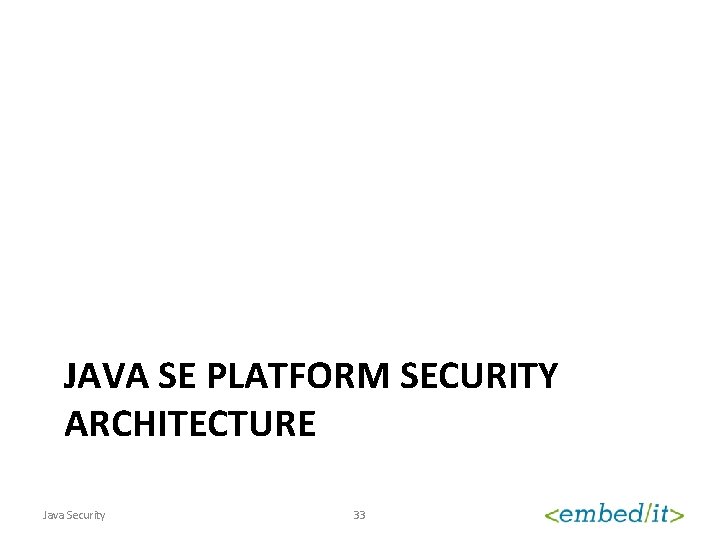
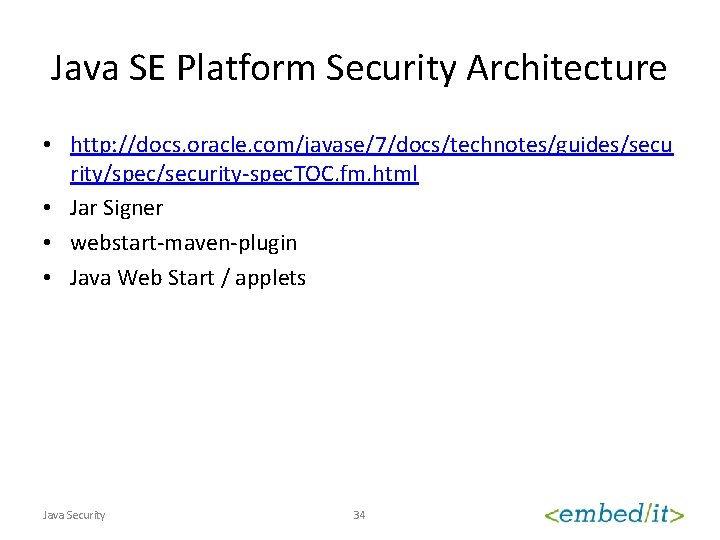
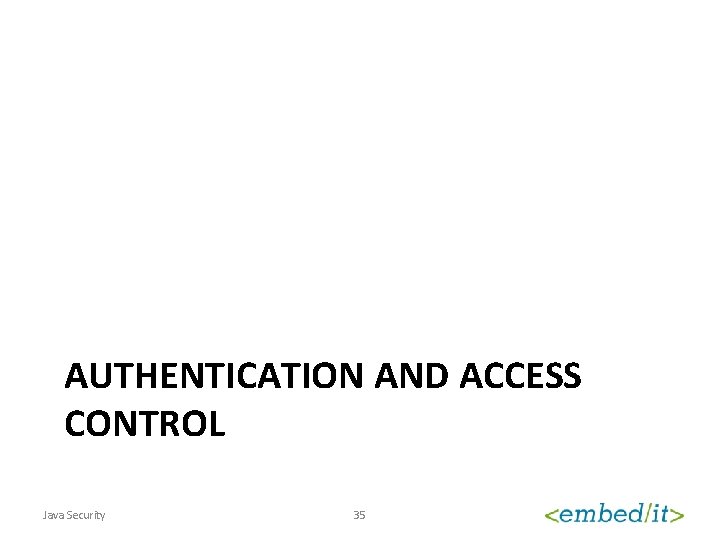
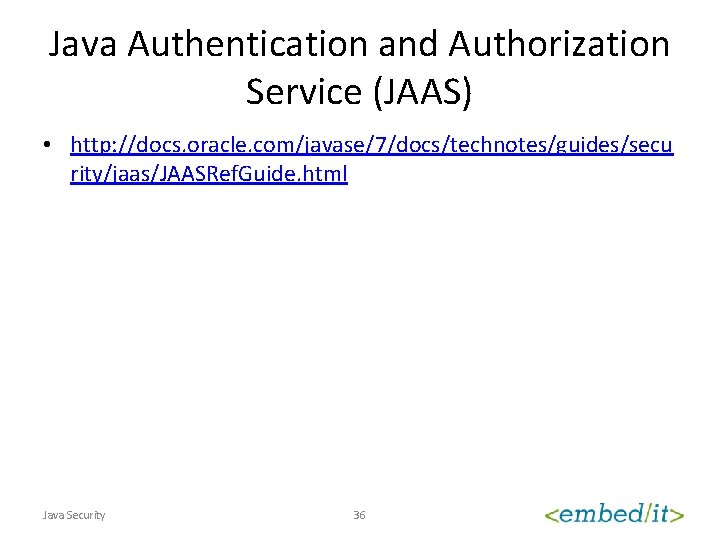
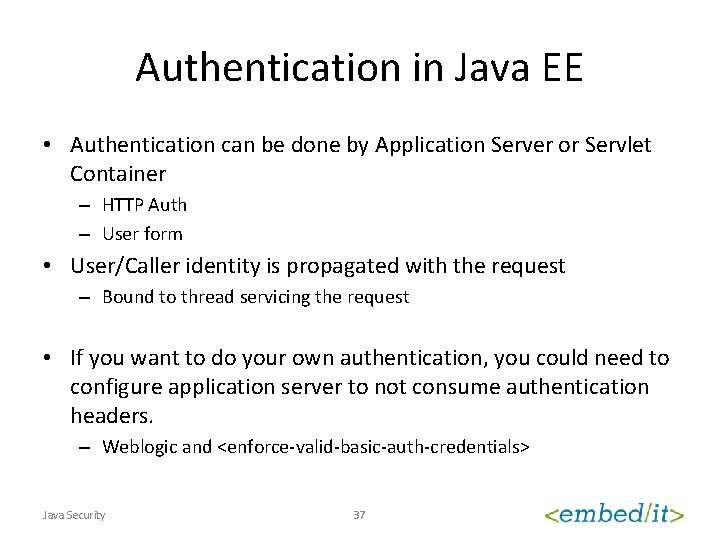
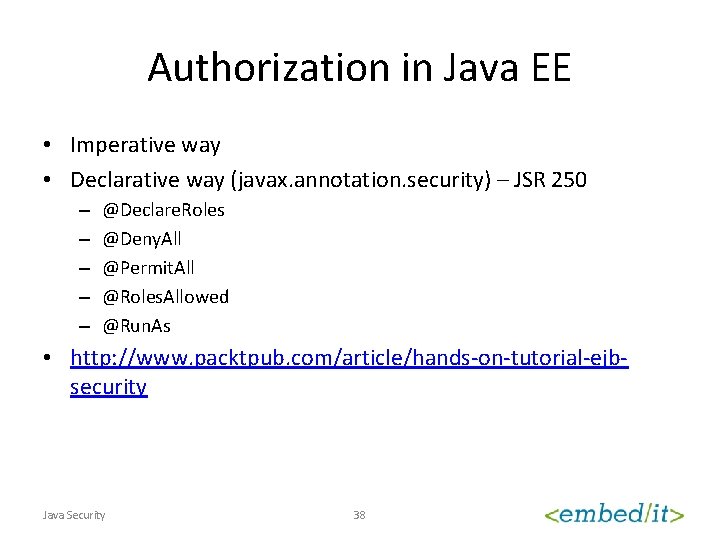
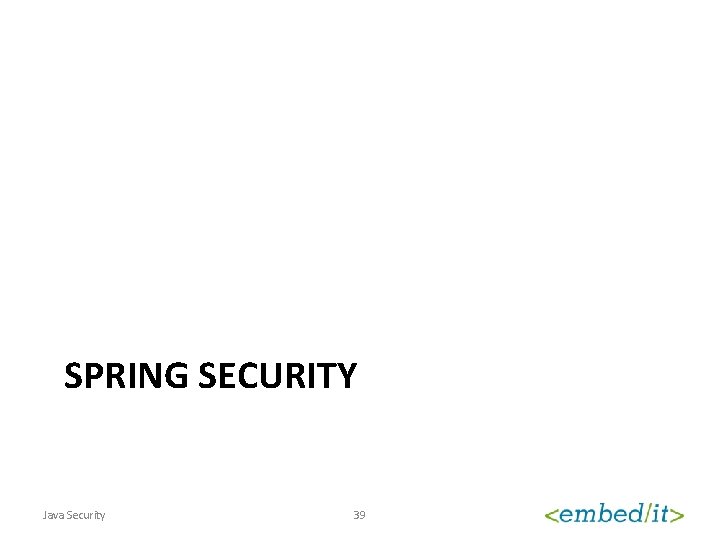
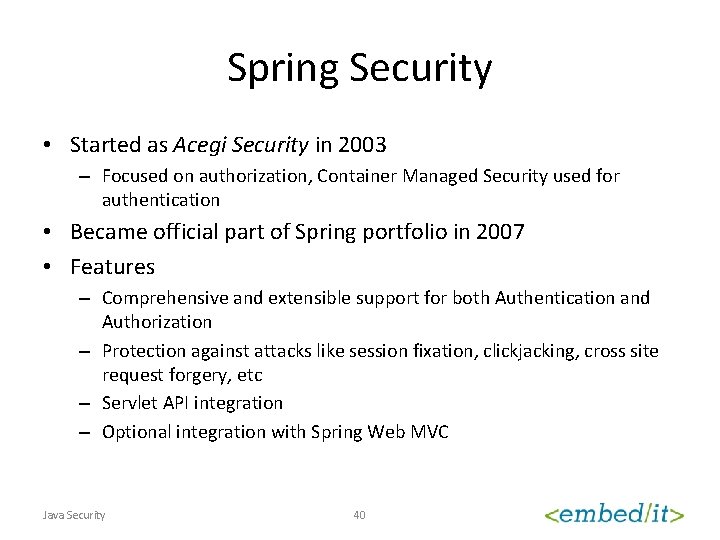
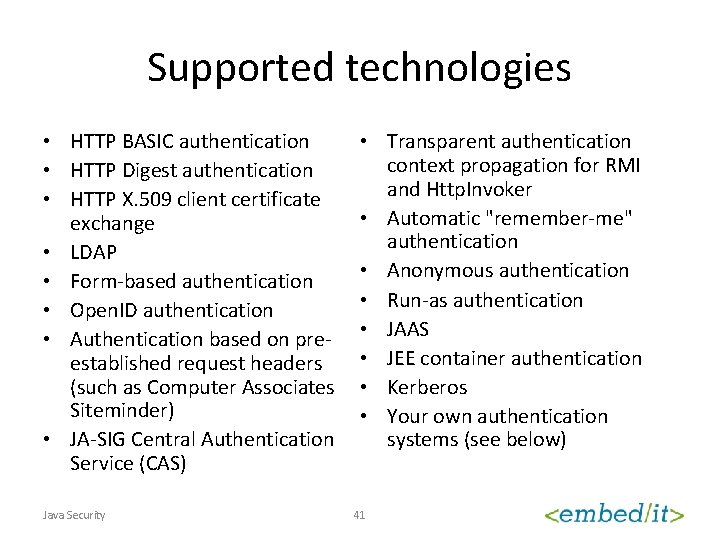
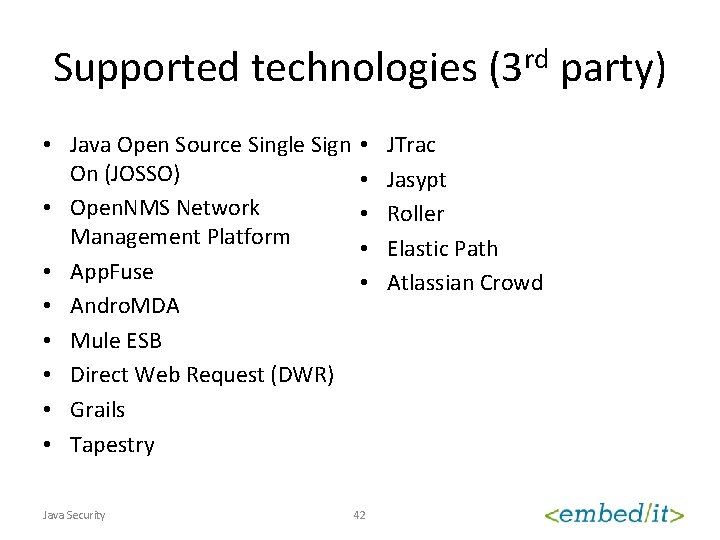
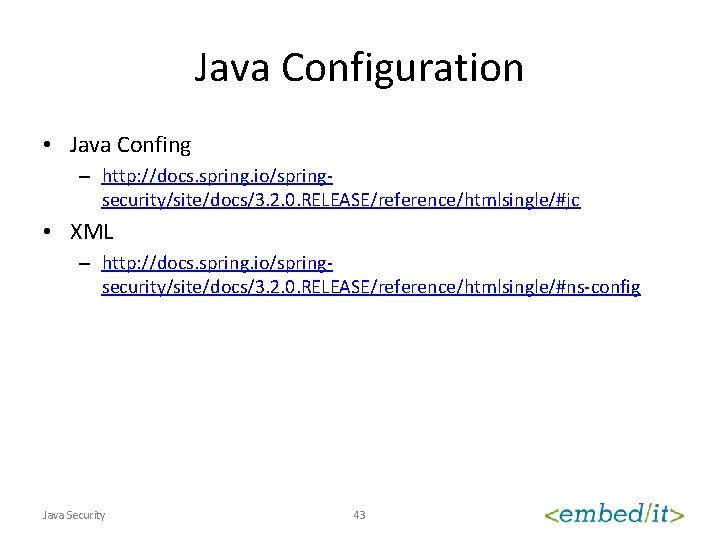
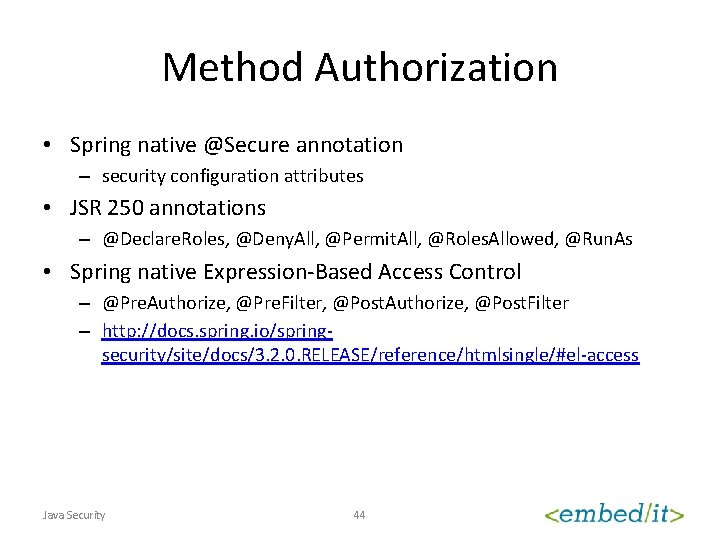
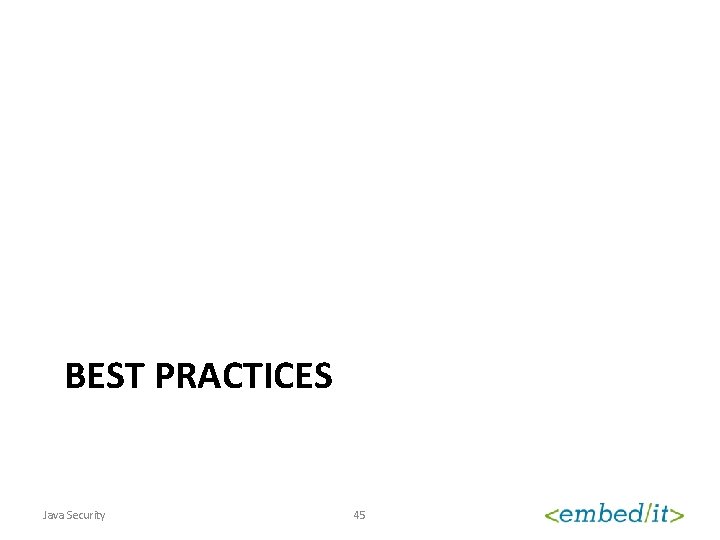
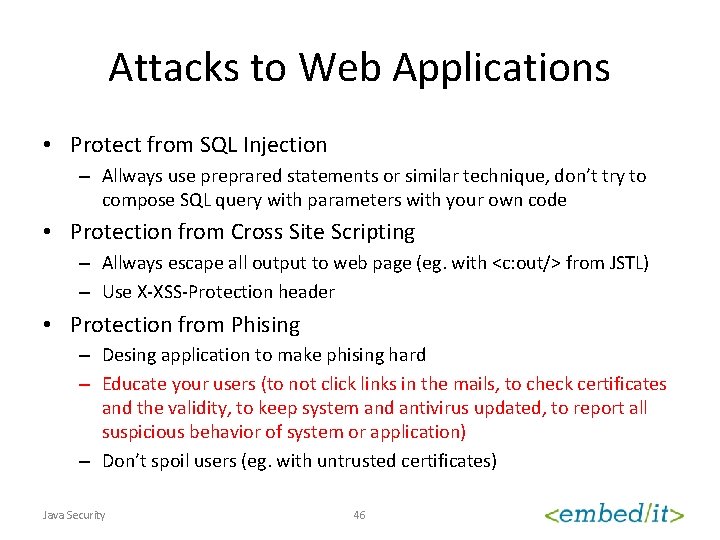
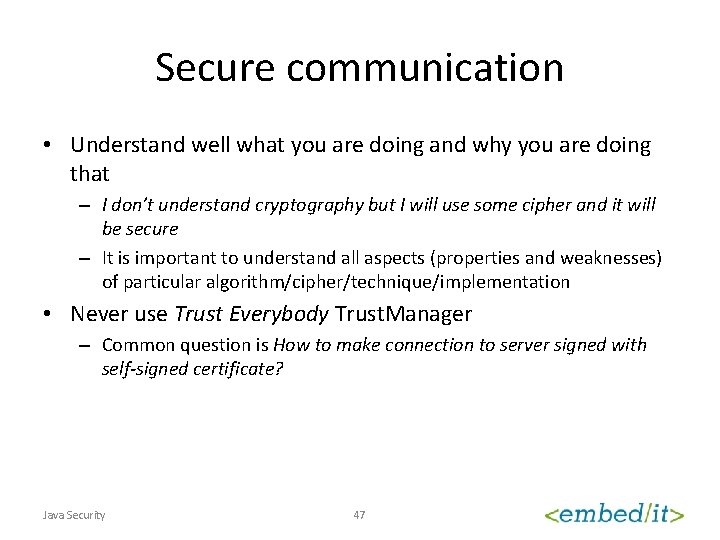
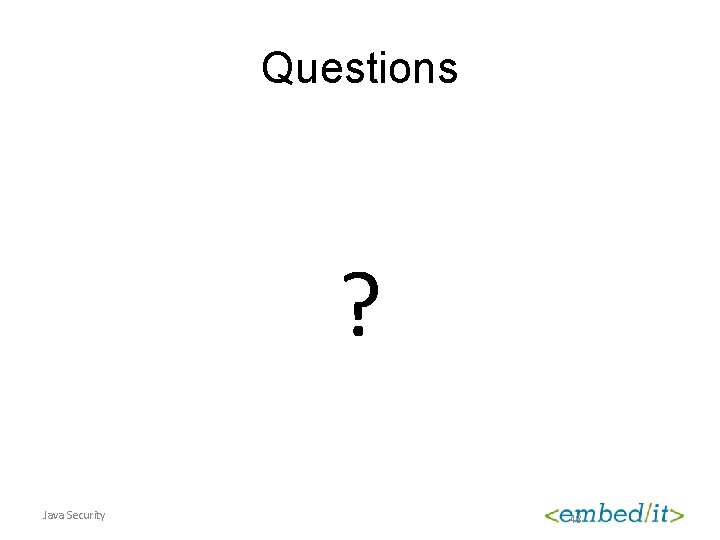
- Slides: 48
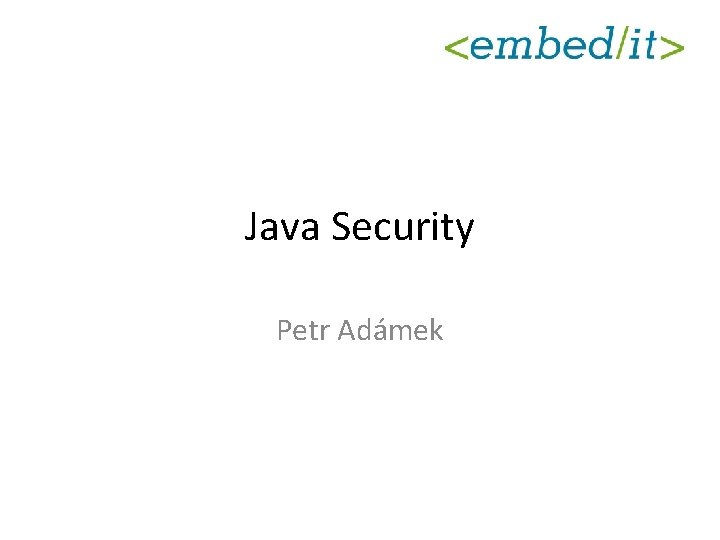
Java Security Petr Adámek
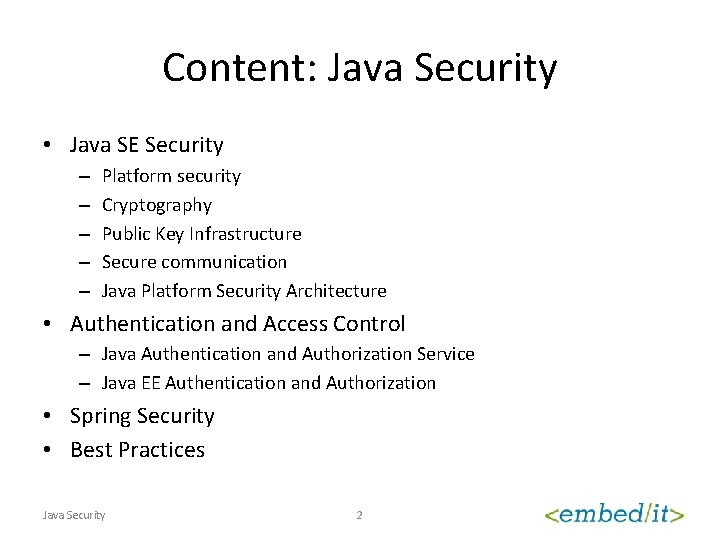
Content: Java Security • Java SE Security – – – Platform security Cryptography Public Key Infrastructure Secure communication Java Platform Security Architecture • Authentication and Access Control – Java Authentication and Authorization Service – Java EE Authentication and Authorization • Spring Security • Best Practices Java Security 2
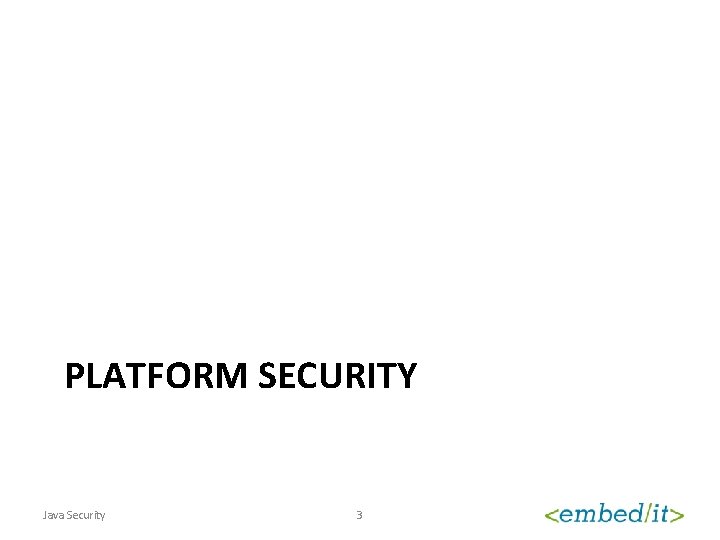
PLATFORM SECURITY Java Security 3
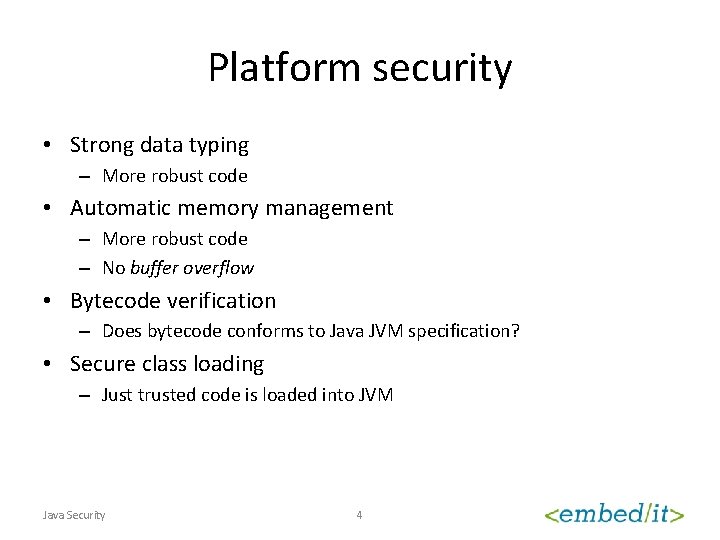
Platform security • Strong data typing – More robust code • Automatic memory management – More robust code – No buffer overflow • Bytecode verification – Does bytecode conforms to Java JVM specification? • Secure class loading – Just trusted code is loaded into JVM Java Security 4
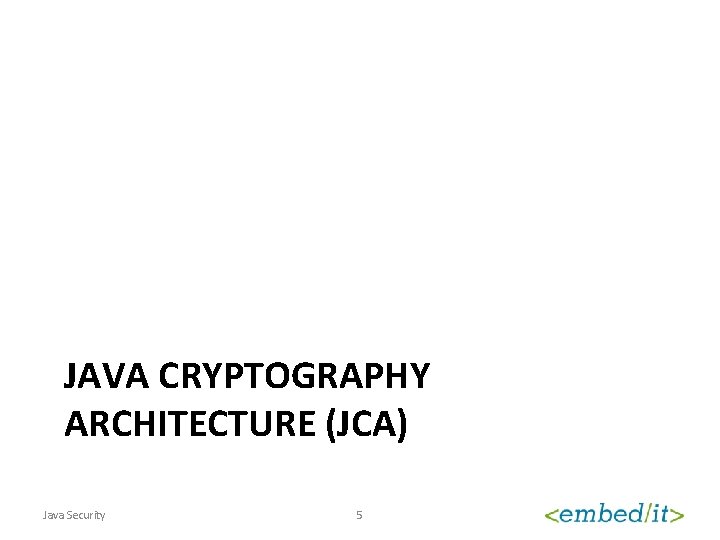
JAVA CRYPTOGRAPHY ARCHITECTURE (JCA) Java Security 5
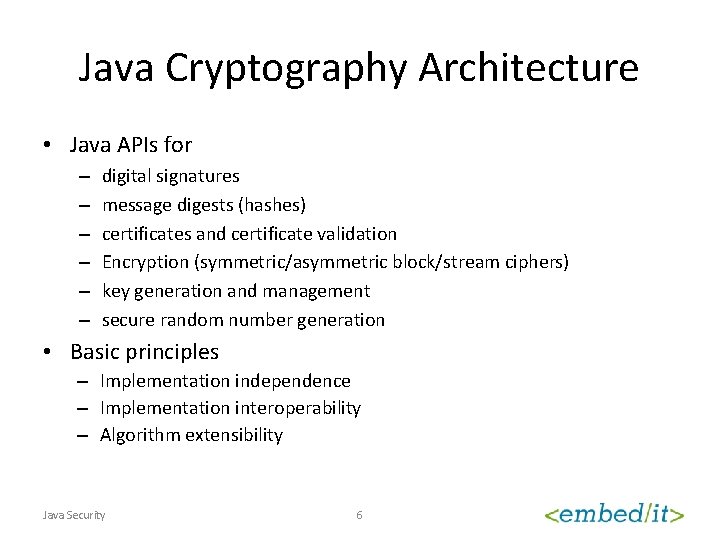
Java Cryptography Architecture • Java APIs for – – – digital signatures message digests (hashes) certificates and certificate validation Encryption (symmetric/asymmetric block/stream ciphers) key generation and management secure random number generation • Basic principles – Implementation independence – Implementation interoperability – Algorithm extensibility Java Security 6
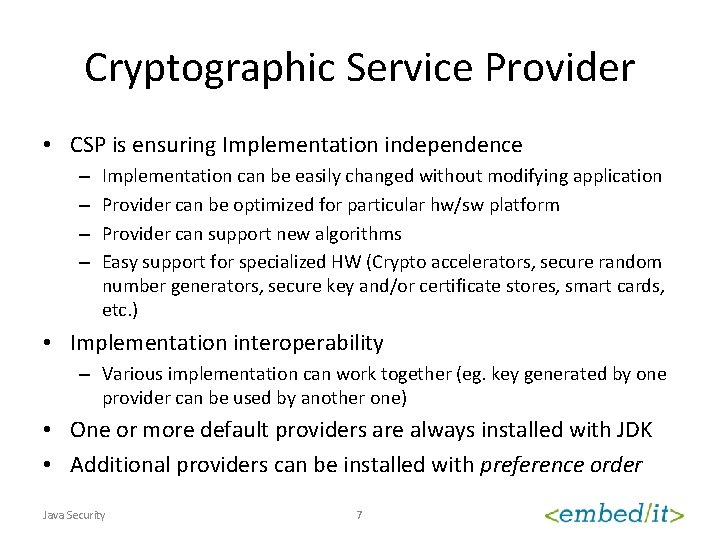
Cryptographic Service Provider • CSP is ensuring Implementation independence – – Implementation can be easily changed without modifying application Provider can be optimized for particular hw/sw platform Provider can support new algorithms Easy support for specialized HW (Crypto accelerators, secure random number generators, secure key and/or certificate stores, smart cards, etc. ) • Implementation interoperability – Various implementation can work together (eg. key generated by one provider can be used by another one) • One or more default providers are always installed with JDK • Additional providers can be installed with preference order Java Security 7
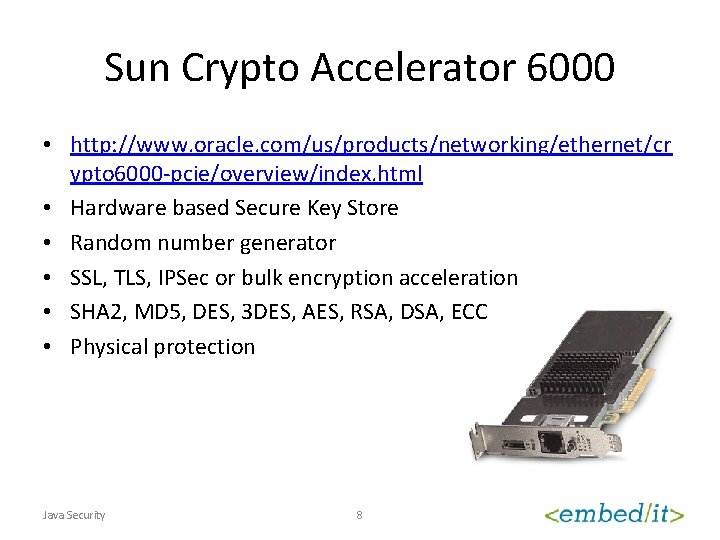
Sun Crypto Accelerator 6000 • http: //www. oracle. com/us/products/networking/ethernet/cr ypto 6000 -pcie/overview/index. html • Hardware based Secure Key Store • Random number generator • SSL, TLS, IPSec or bulk encryption acceleration • SHA 2, MD 5, DES, 3 DES, AES, RSA, DSA, ECC • Physical protection Java Security 8
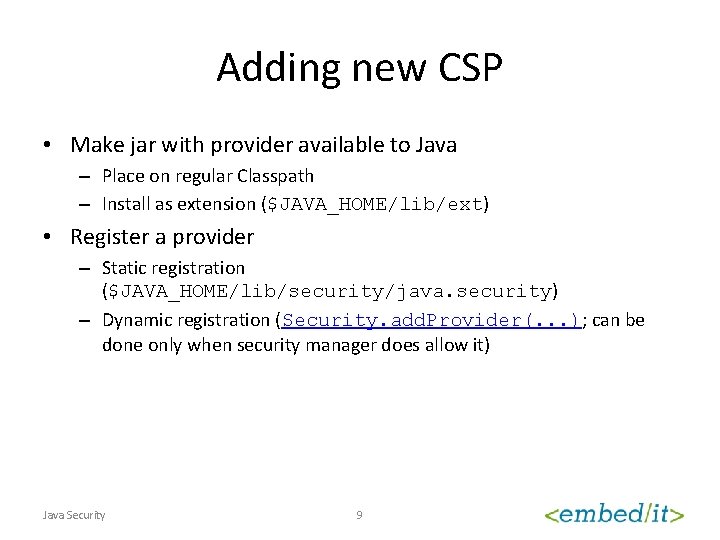
Adding new CSP • Make jar with provider available to Java – Place on regular Classpath – Install as extension ($JAVA_HOME/lib/ext) • Register a provider – Static registration ($JAVA_HOME/lib/security/java. security) – Dynamic registration (Security. add. Provider(. . . ); can be done only when security manager does allow it) Java Security 9
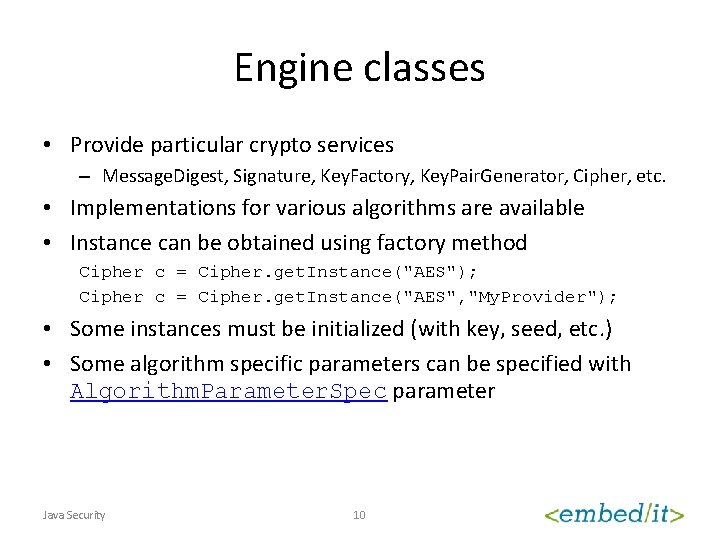
Engine classes • Provide particular crypto services – Message. Digest, Signature, Key. Factory, Key. Pair. Generator, Cipher, etc. • Implementations for various algorithms are available • Instance can be obtained using factory method Cipher c = Cipher. get. Instance("AES"); Cipher c = Cipher. get. Instance("AES", "My. Provider"); • Some instances must be initialized (with key, seed, etc. ) • Some algorithm specific parameters can be specified with Algorithm. Parameter. Spec parameter Java Security 10
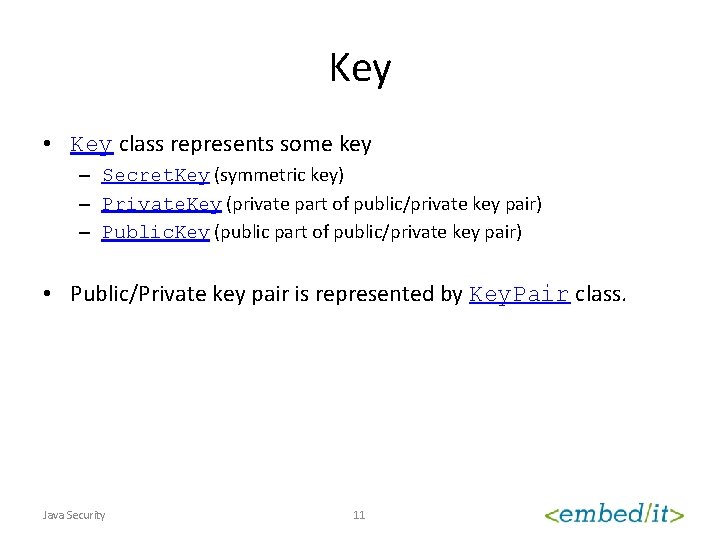
Key • Key class represents some key – Secret. Key (symmetric key) – Private. Key (private part of public/private key pair) – Public. Key (public part of public/private key pair) • Public/Private key pair is represented by Key. Pair class. Java Security 11
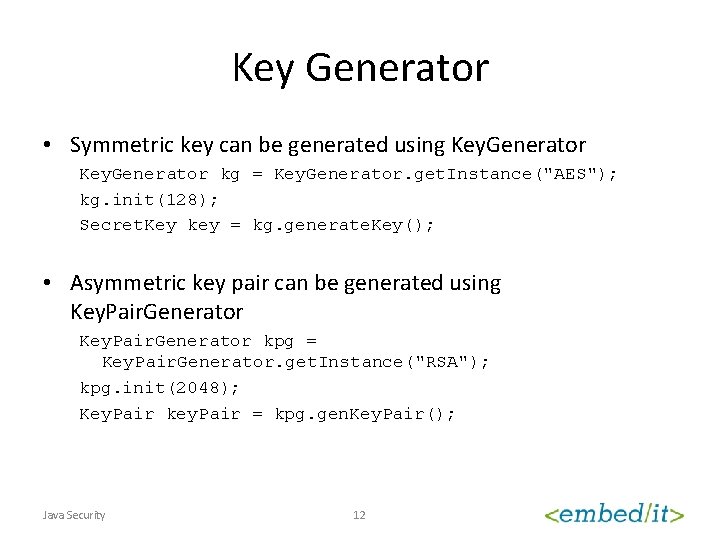
Key Generator • Symmetric key can be generated using Key. Generator kg = Key. Generator. get. Instance("AES"); kg. init(128); Secret. Key key = kg. generate. Key(); • Asymmetric key pair can be generated using Key. Pair. Generator kpg = Key. Pair. Generator. get. Instance("RSA"); kpg. init(2048); Key. Pair key. Pair = kpg. gen. Key. Pair(); Java Security 12
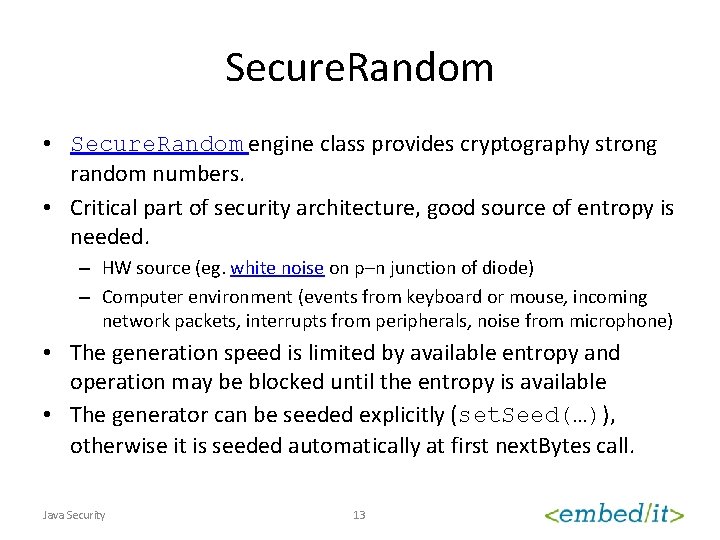
Secure. Random • Secure. Random engine class provides cryptography strong random numbers. • Critical part of security architecture, good source of entropy is needed. – HW source (eg. white noise on p–n junction of diode) – Computer environment (events from keyboard or mouse, incoming network packets, interrupts from peripherals, noise from microphone) • The generation speed is limited by available entropy and operation may be blocked until the entropy is available • The generator can be seeded explicitly (set. Seed(…)), otherwise it is seeded automatically at first next. Bytes call. Java Security 13
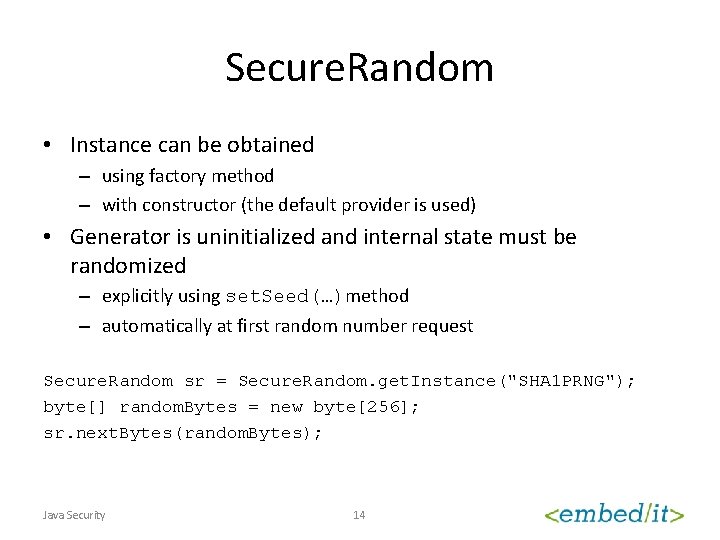
Secure. Random • Instance can be obtained – using factory method – with constructor (the default provider is used) • Generator is uninitialized and internal state must be randomized – explicitly using set. Seed(…)method – automatically at first random number request Secure. Random sr = Secure. Random. get. Instance("SHA 1 PRNG"); byte[] random. Bytes = new byte[256]; sr. next. Bytes(random. Bytes); Java Security 14
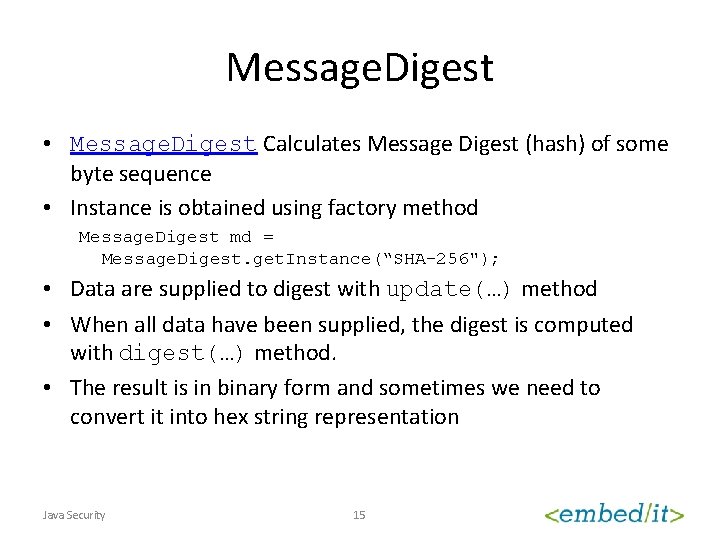
Message. Digest • Message. Digest Calculates Message Digest (hash) of some byte sequence • Instance is obtained using factory method Message. Digest md = Message. Digest. get. Instance(“SHA-256"); • Data are supplied to digest with update(…) method • When all data have been supplied, the digest is computed with digest(…) method. • The result is in binary form and sometimes we need to convert it into hex string representation Java Security 15
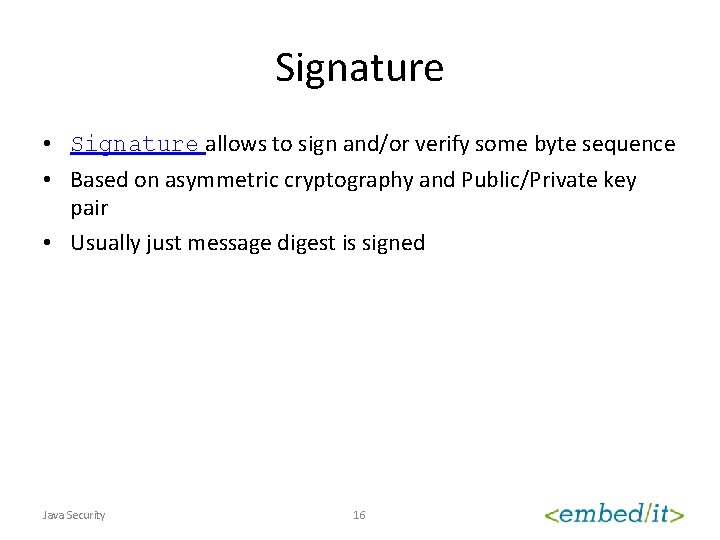
Signature • Signature allows to sign and/or verify some byte sequence • Based on asymmetric cryptography and Public/Private key pair • Usually just message digest is signed Java Security 16
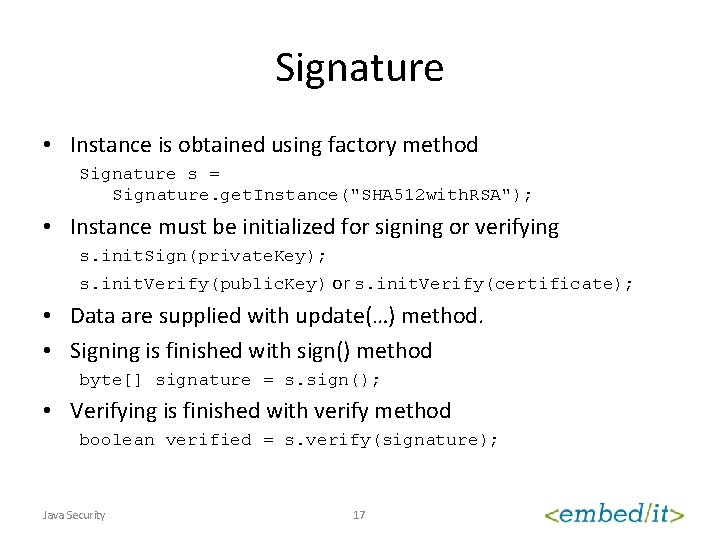
Signature • Instance is obtained using factory method Signature s = Signature. get. Instance("SHA 512 with. RSA"); • Instance must be initialized for signing or verifying s. init. Sign(private. Key); s. init. Verify(public. Key) or s. init. Verify(certificate); • Data are supplied with update(…) method. • Signing is finished with sign() method byte[] signature = s. sign(); • Verifying is finished with verify method boolean verified = s. verify(signature); Java Security 17
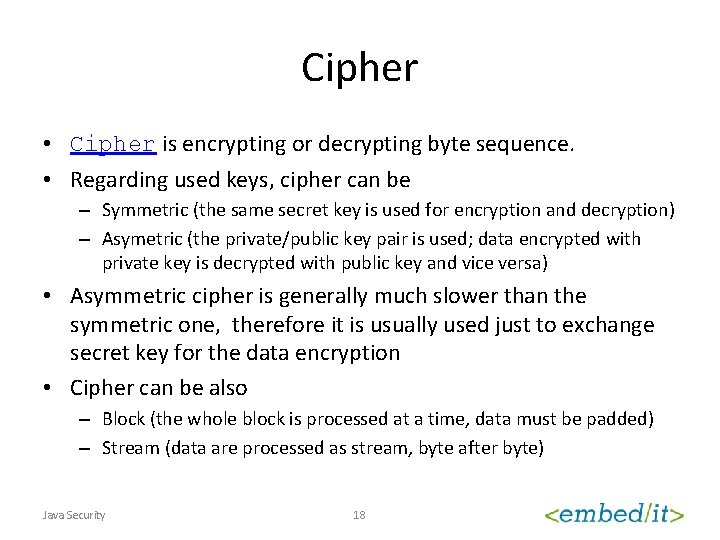
Cipher • Cipher is encrypting or decrypting byte sequence. • Regarding used keys, cipher can be – Symmetric (the same secret key is used for encryption and decryption) – Asymetric (the private/public key pair is used; data encrypted with private key is decrypted with public key and vice versa) • Asymmetric cipher is generally much slower than the symmetric one, therefore it is usually used just to exchange secret key for the data encryption • Cipher can be also – Block (the whole block is processed at a time, data must be padded) – Stream (data are processed as stream, byte after byte) Java Security 18
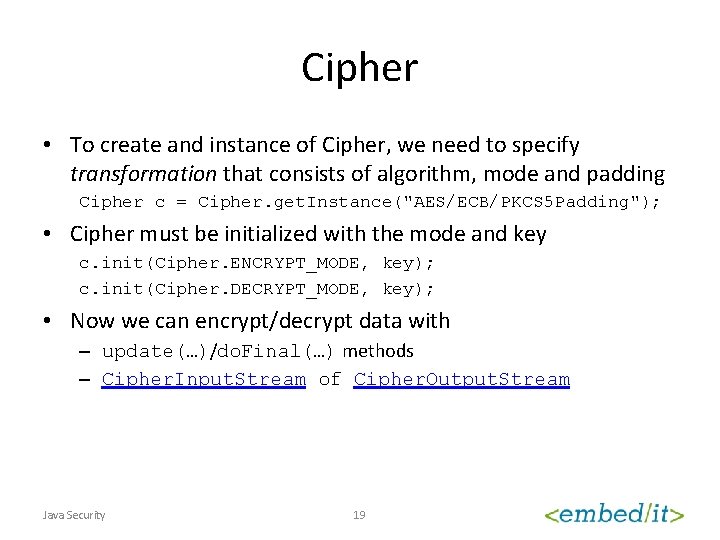
Cipher • To create and instance of Cipher, we need to specify transformation that consists of algorithm, mode and padding Cipher c = Cipher. get. Instance("AES/ECB/PKCS 5 Padding"); • Cipher must be initialized with the mode and key c. init(Cipher. ENCRYPT_MODE, key); c. init(Cipher. DECRYPT_MODE, key); • Now we can encrypt/decrypt data with – update(…)/do. Final(…) methods – Cipher. Input. Stream of Cipher. Output. Stream Java Security 19
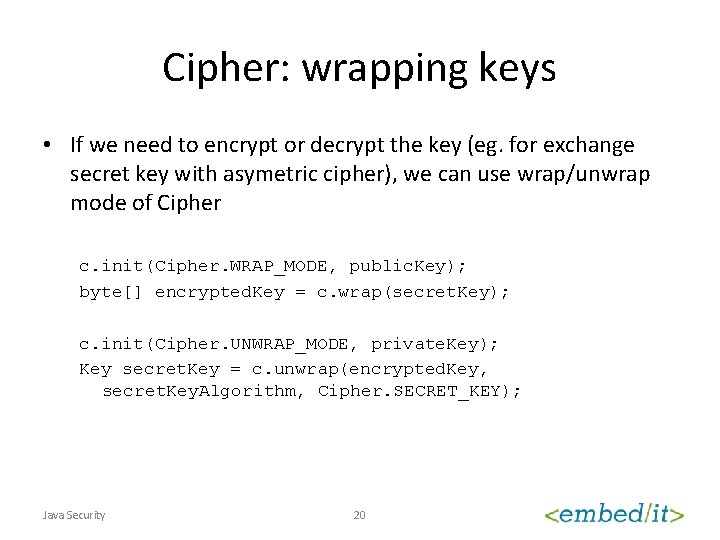
Cipher: wrapping keys • If we need to encrypt or decrypt the key (eg. for exchange secret key with asymetric cipher), we can use wrap/unwrap mode of Cipher c. init(Cipher. WRAP_MODE, public. Key); byte[] encrypted. Key = c. wrap(secret. Key); c. init(Cipher. UNWRAP_MODE, private. Key); Key secret. Key = c. unwrap(encrypted. Key, secret. Key. Algorithm, Cipher. SECRET_KEY); Java Security 20
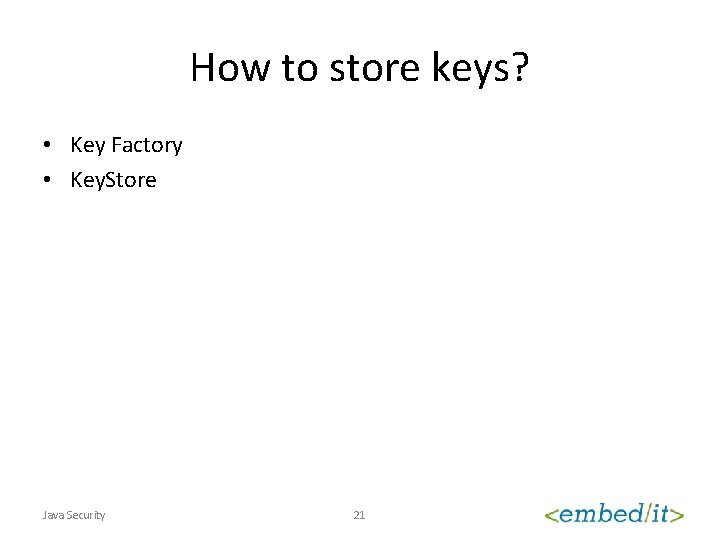
How to store keys? • Key Factory • Key. Store Java Security 21
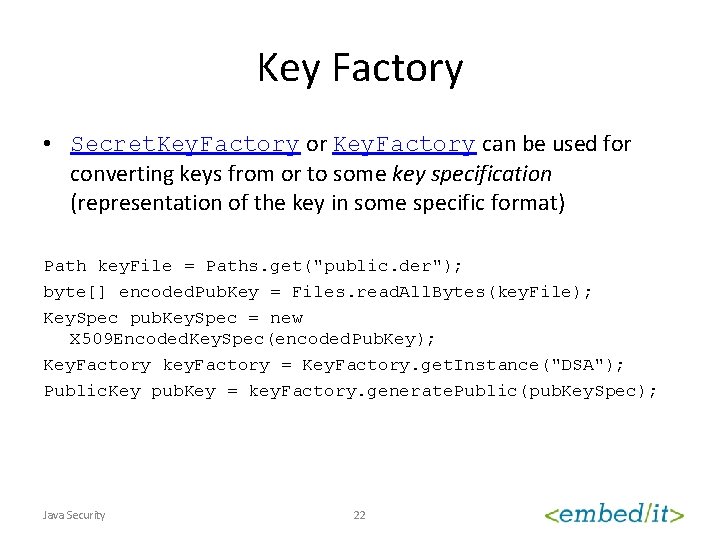
Key Factory • Secret. Key. Factory or Key. Factory can be used for converting keys from or to some key specification (representation of the key in some specific format) Path key. File = Paths. get("public. der"); byte[] encoded. Pub. Key = Files. read. All. Bytes(key. File); Key. Spec pub. Key. Spec = new X 509 Encoded. Key. Spec(encoded. Pub. Key); Key. Factory key. Factory = Key. Factory. get. Instance("DSA"); Public. Key pub. Key = key. Factory. generate. Public(pub. Key. Spec); Java Security 22
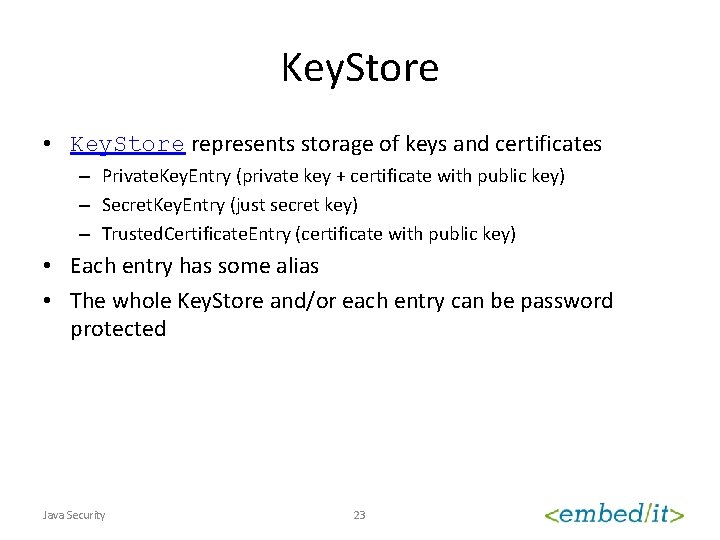
Key. Store • Key. Store represents storage of keys and certificates – Private. Key. Entry (private key + certificate with public key) – Secret. Key. Entry (just secret key) – Trusted. Certificate. Entry (certificate with public key) • Each entry has some alias • The whole Key. Store and/or each entry can be password protected Java Security 23
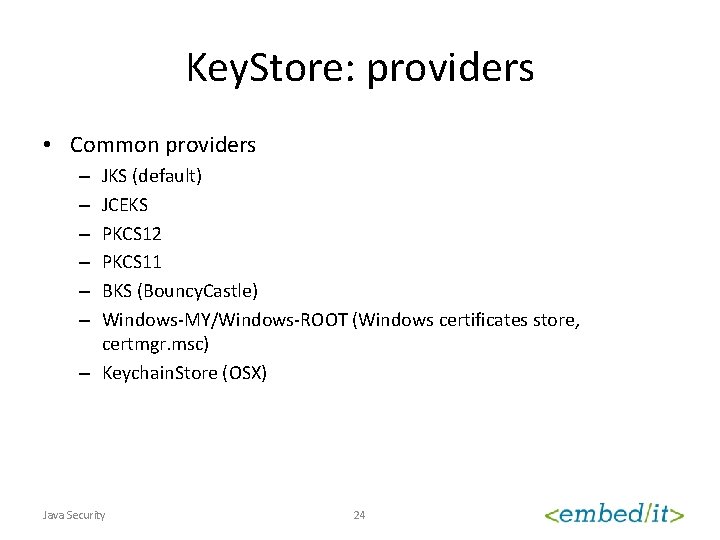
Key. Store: providers • Common providers JKS (default) JCEKS PKCS 12 PKCS 11 BKS (Bouncy. Castle) Windows-MY/Windows-ROOT (Windows certificates store, certmgr. msc) – Keychain. Store (OSX) – – – Java Security 24
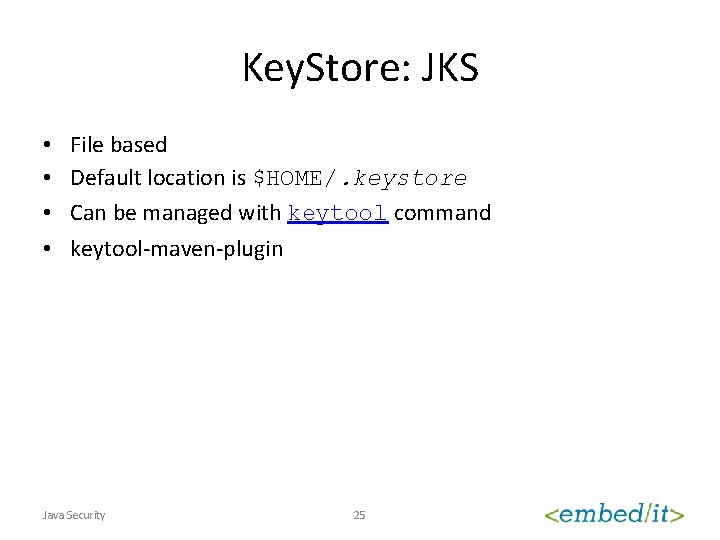
Key. Store: JKS • • File based Default location is $HOME/. keystore Can be managed with keytool command keytool-maven-plugin Java Security 25
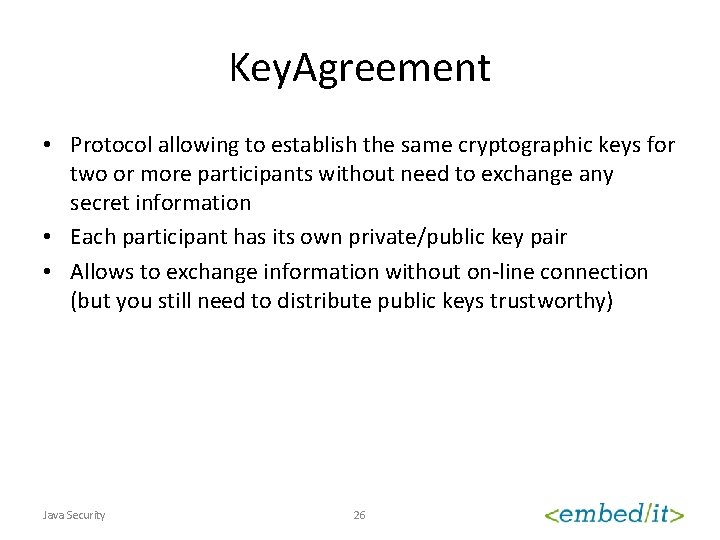
Key. Agreement • Protocol allowing to establish the same cryptographic keys for two or more participants without need to exchange any secret information • Each participant has its own private/public key pair • Allows to exchange information without on-line connection (but you still need to distribute public keys trustworthy) Java Security 26
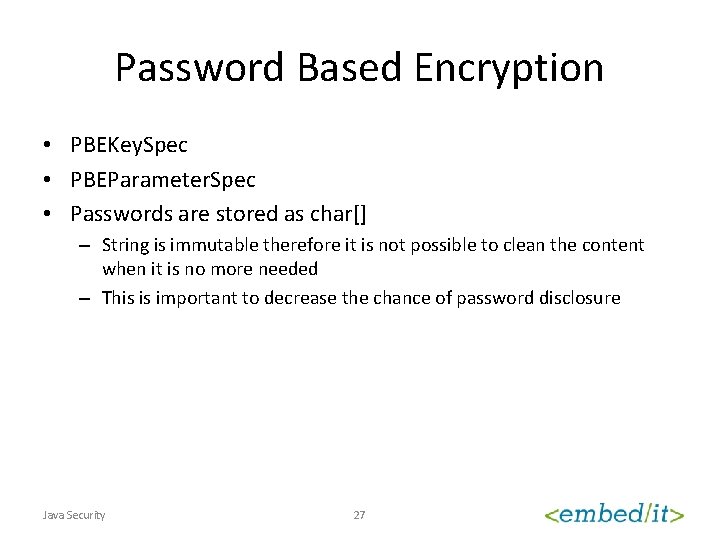
Password Based Encryption • PBEKey. Spec • PBEParameter. Spec • Passwords are stored as char[] – String is immutable therefore it is not possible to clean the content when it is no more needed – This is important to decrease the chance of password disclosure Java Security 27
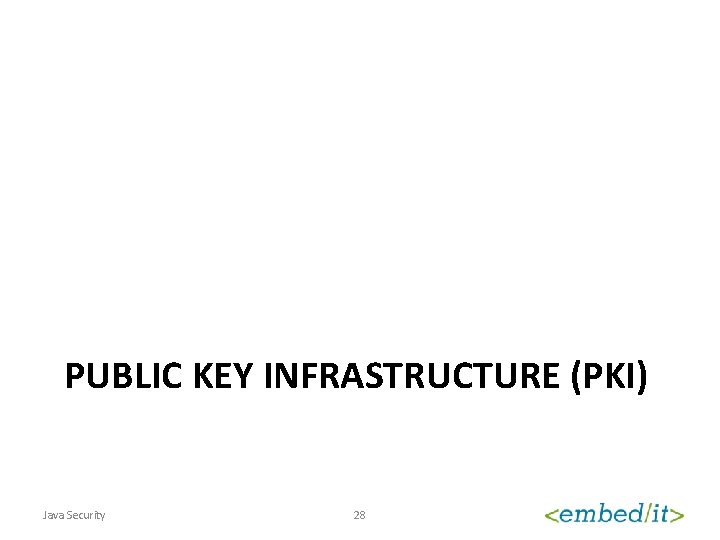
PUBLIC KEY INFRASTRUCTURE (PKI) Java Security 28
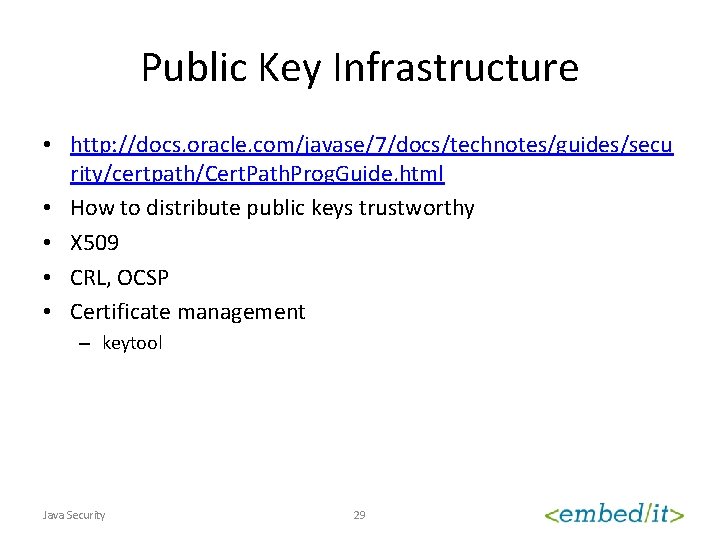
Public Key Infrastructure • http: //docs. oracle. com/javase/7/docs/technotes/guides/secu rity/certpath/Cert. Path. Prog. Guide. html • How to distribute public keys trustworthy • X 509 • CRL, OCSP • Certificate management – keytool Java Security 29
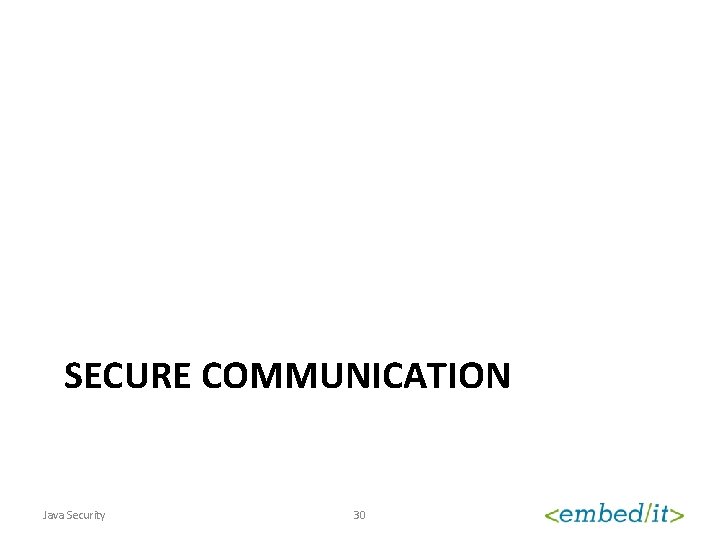
SECURE COMMUNICATION Java Security 30
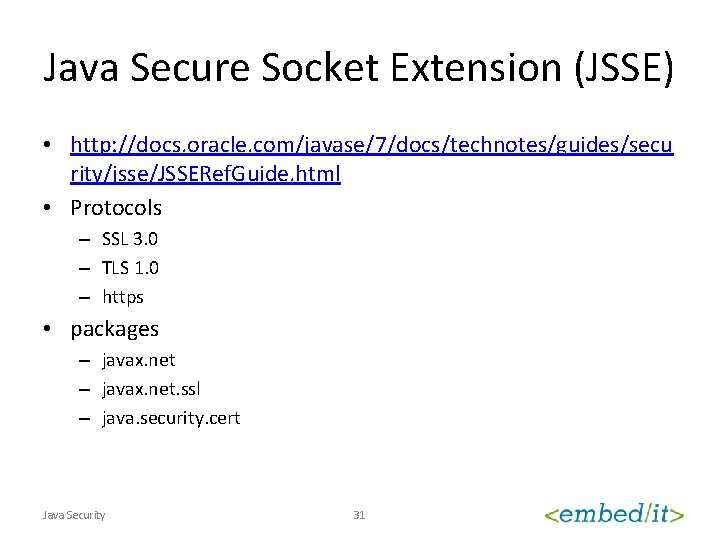
Java Secure Socket Extension (JSSE) • http: //docs. oracle. com/javase/7/docs/technotes/guides/secu rity/jsse/JSSERef. Guide. html • Protocols – SSL 3. 0 – TLS 1. 0 – https • packages – javax. net. ssl – java. security. cert Java Security 31
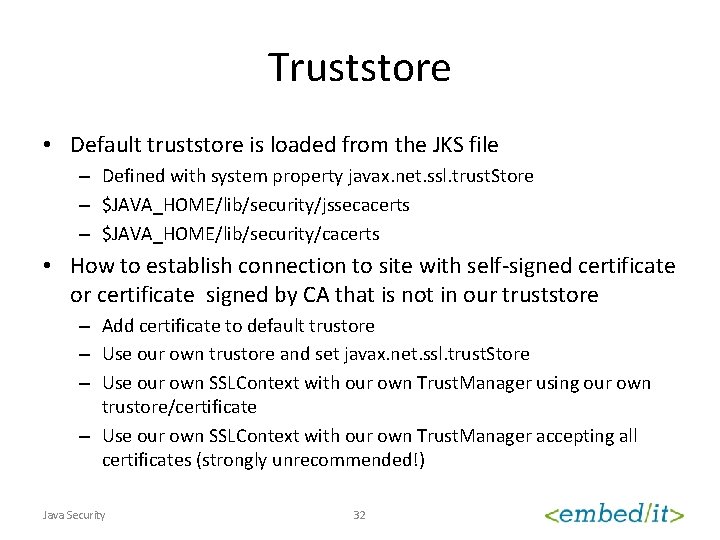
Truststore • Default truststore is loaded from the JKS file – Defined with system property javax. net. ssl. trust. Store – $JAVA_HOME/lib/security/jssecacerts – $JAVA_HOME/lib/security/cacerts • How to establish connection to site with self-signed certificate or certificate signed by CA that is not in our truststore – Add certificate to default trustore – Use our own trustore and set javax. net. ssl. trust. Store – Use our own SSLContext with our own Trust. Manager using our own trustore/certificate – Use our own SSLContext with our own Trust. Manager accepting all certificates (strongly unrecommended!) Java Security 32
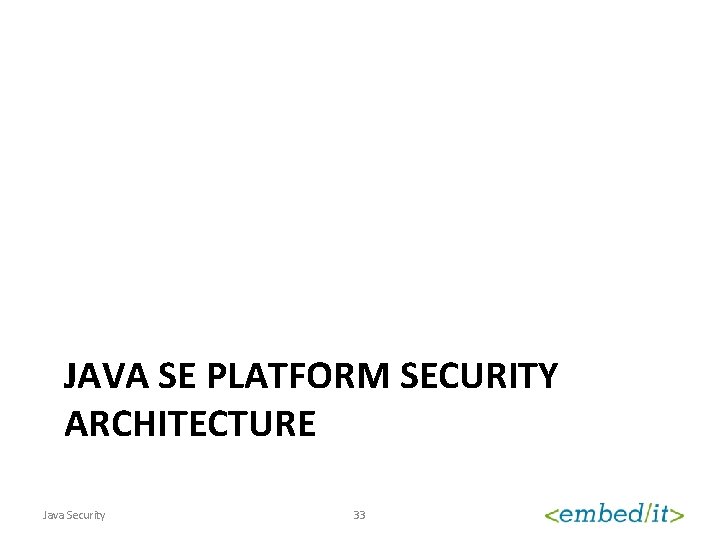
JAVA SE PLATFORM SECURITY ARCHITECTURE Java Security 33
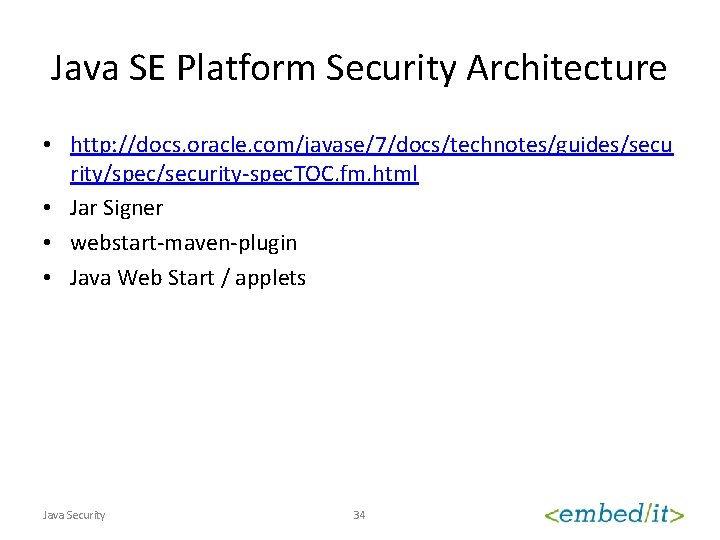
Java SE Platform Security Architecture • http: //docs. oracle. com/javase/7/docs/technotes/guides/secu rity/spec/security-spec. TOC. fm. html • Jar Signer • webstart-maven-plugin • Java Web Start / applets Java Security 34
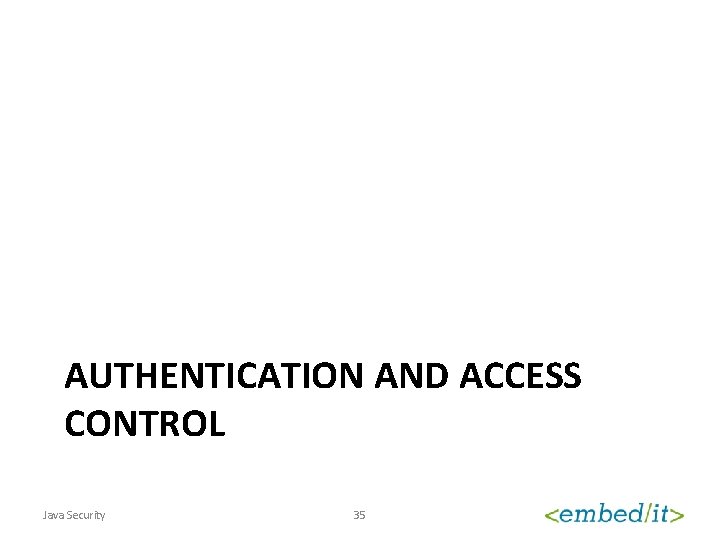
AUTHENTICATION AND ACCESS CONTROL Java Security 35
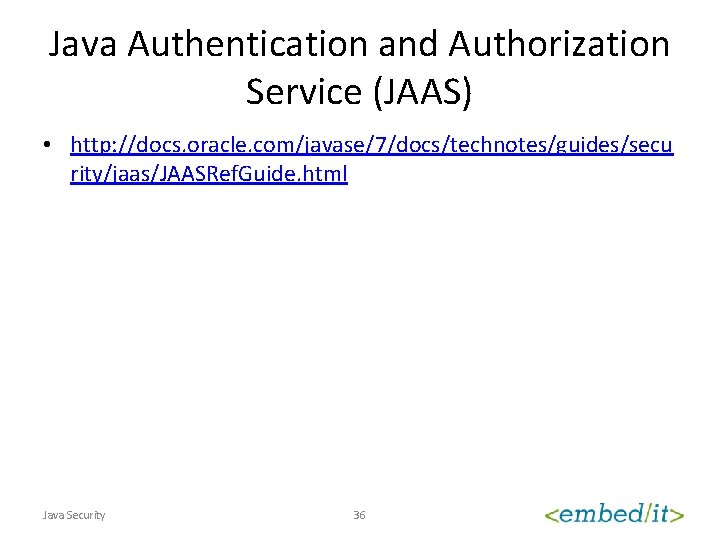
Java Authentication and Authorization Service (JAAS) • http: //docs. oracle. com/javase/7/docs/technotes/guides/secu rity/jaas/JAASRef. Guide. html Java Security 36
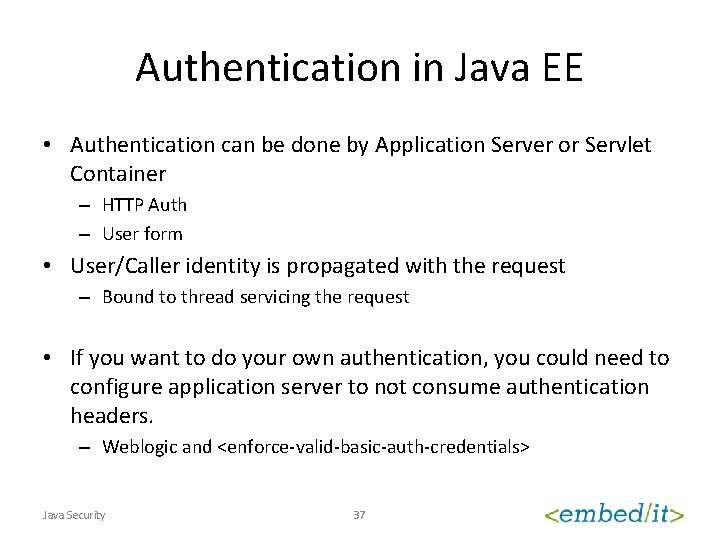
Authentication in Java EE • Authentication can be done by Application Server or Servlet Container – HTTP Auth – User form • User/Caller identity is propagated with the request – Bound to thread servicing the request • If you want to do your own authentication, you could need to configure application server to not consume authentication headers. – Weblogic and <enforce-valid-basic-auth-credentials> Java Security 37
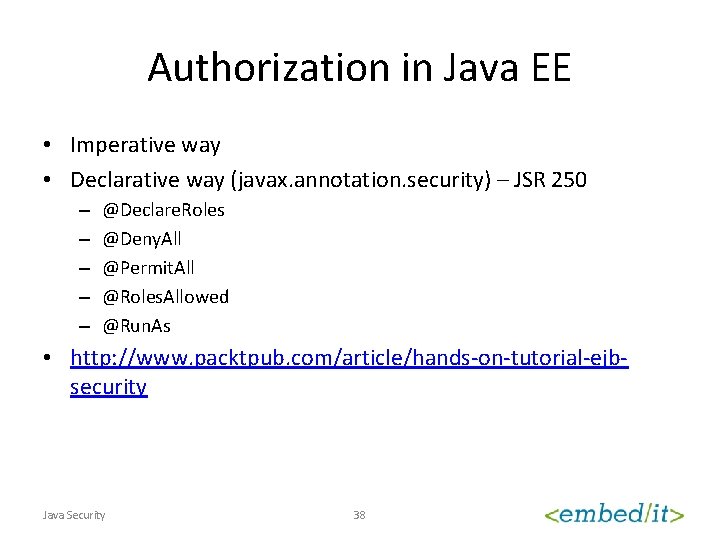
Authorization in Java EE • Imperative way • Declarative way (javax. annotation. security) – JSR 250 – – – @Declare. Roles @Deny. All @Permit. All @Roles. Allowed @Run. As • http: //www. packtpub. com/article/hands-on-tutorial-ejbsecurity Java Security 38
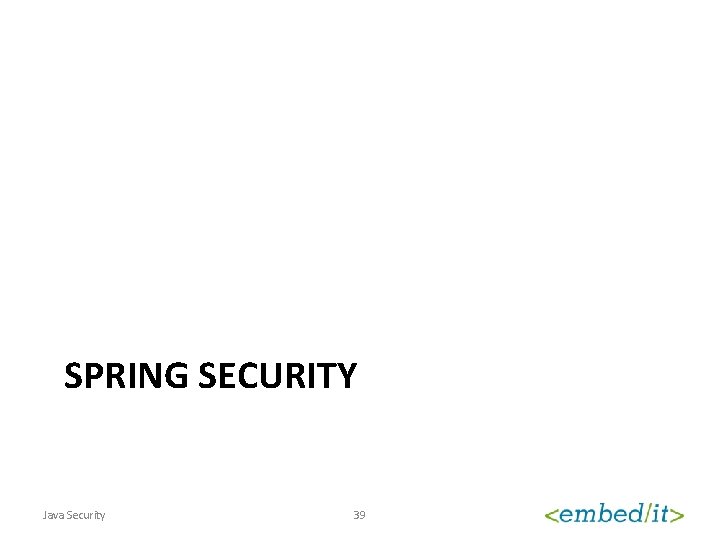
SPRING SECURITY Java Security 39
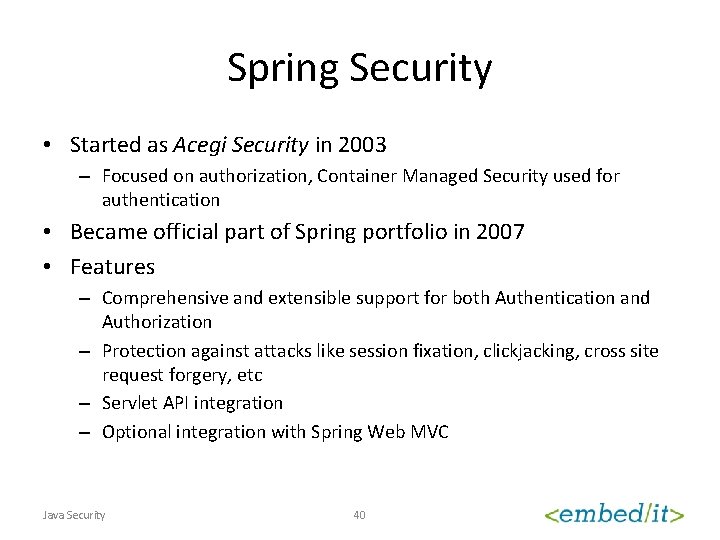
Spring Security • Started as Acegi Security in 2003 – Focused on authorization, Container Managed Security used for authentication • Became official part of Spring portfolio in 2007 • Features – Comprehensive and extensible support for both Authentication and Authorization – Protection against attacks like session fixation, clickjacking, cross site request forgery, etc – Servlet API integration – Optional integration with Spring Web MVC Java Security 40
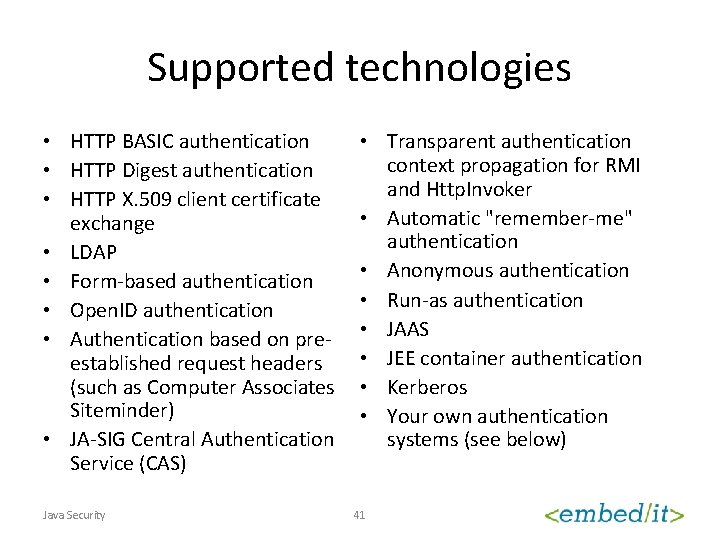
Supported technologies • HTTP BASIC authentication • HTTP Digest authentication • HTTP X. 509 client certificate exchange • LDAP • Form-based authentication • Open. ID authentication • Authentication based on preestablished request headers (such as Computer Associates Siteminder) • JA-SIG Central Authentication Service (CAS) Java Security • Transparent authentication context propagation for RMI and Http. Invoker • Automatic "remember-me" authentication • Anonymous authentication • Run-as authentication • JAAS • JEE container authentication • Kerberos • Your own authentication systems (see below) 41
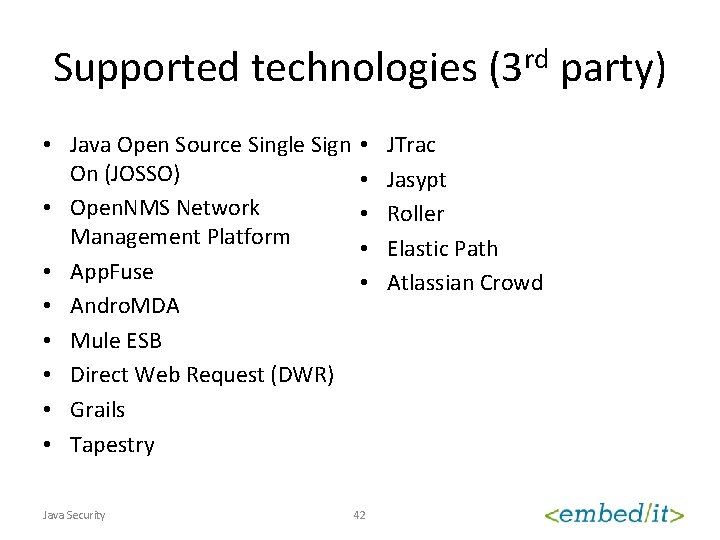
Supported technologies (3 rd party) • Java Open Source Single Sign • On (JOSSO) • • Open. NMS Network • Management Platform • • App. Fuse • • Andro. MDA • Mule ESB • Direct Web Request (DWR) • Grails • Tapestry Java Security 42 JTrac Jasypt Roller Elastic Path Atlassian Crowd
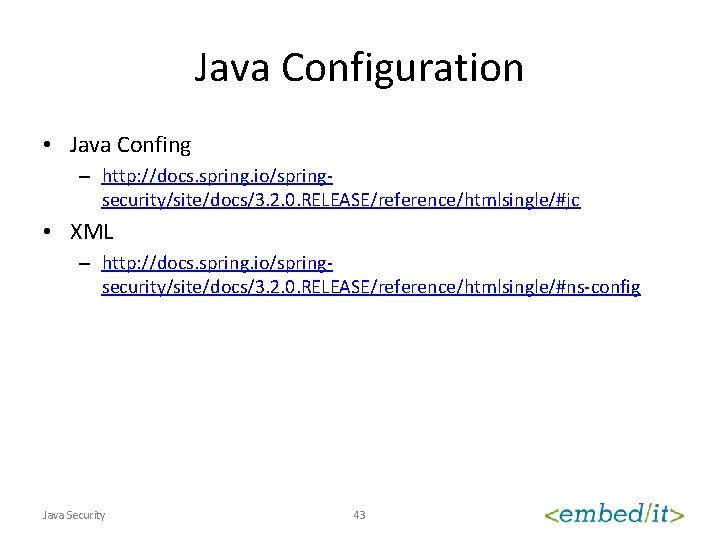
Java Configuration • Java Confing – http: //docs. spring. io/springsecurity/site/docs/3. 2. 0. RELEASE/reference/htmlsingle/#jc • XML – http: //docs. spring. io/springsecurity/site/docs/3. 2. 0. RELEASE/reference/htmlsingle/#ns-config Java Security 43
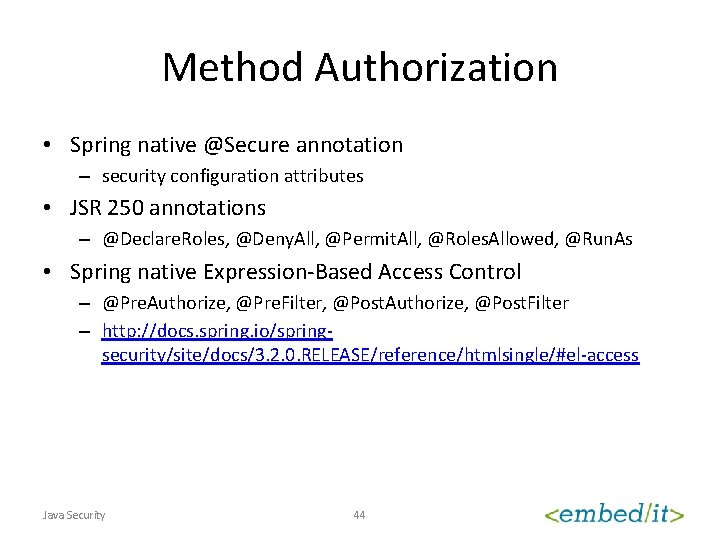
Method Authorization • Spring native @Secure annotation – security configuration attributes • JSR 250 annotations – @Declare. Roles, @Deny. All, @Permit. All, @Roles. Allowed, @Run. As • Spring native Expression-Based Access Control – @Pre. Authorize, @Pre. Filter, @Post. Authorize, @Post. Filter – http: //docs. spring. io/springsecurity/site/docs/3. 2. 0. RELEASE/reference/htmlsingle/#el-access Java Security 44
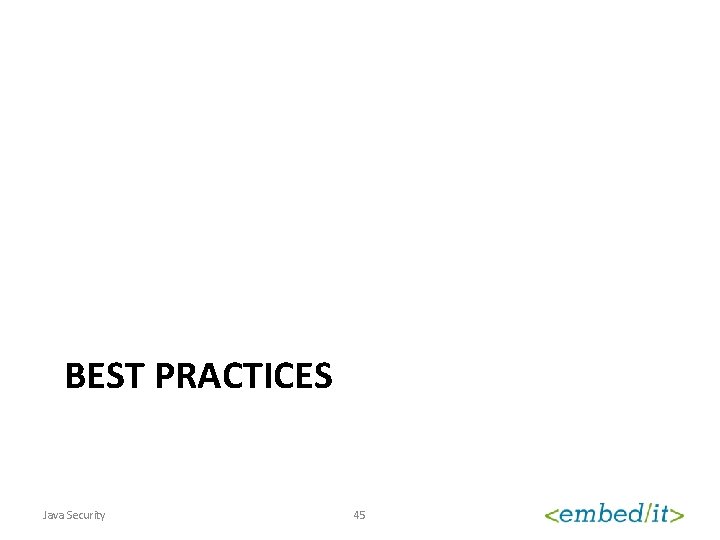
BEST PRACTICES Java Security 45
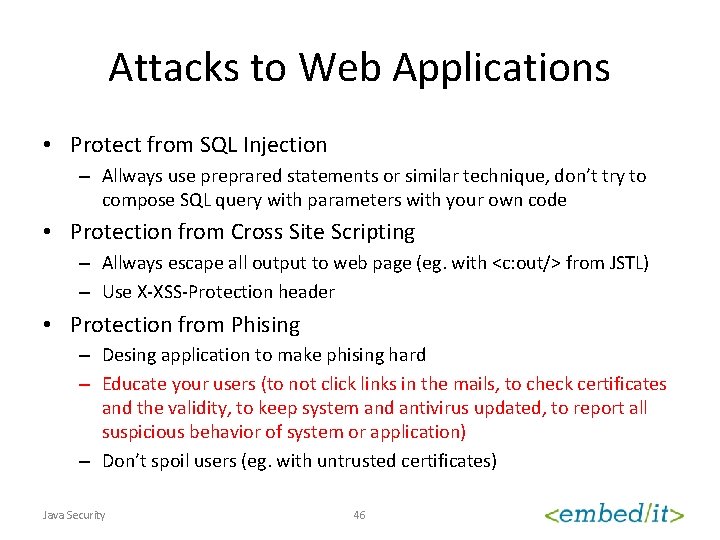
Attacks to Web Applications • Protect from SQL Injection – Allways use preprared statements or similar technique, don’t try to compose SQL query with parameters with your own code • Protection from Cross Site Scripting – Allways escape all output to web page (eg. with <c: out/> from JSTL) – Use X-XSS-Protection header • Protection from Phising – Desing application to make phising hard – Educate your users (to not click links in the mails, to check certificates and the validity, to keep system and antivirus updated, to report all suspicious behavior of system or application) – Don’t spoil users (eg. with untrusted certificates) Java Security 46
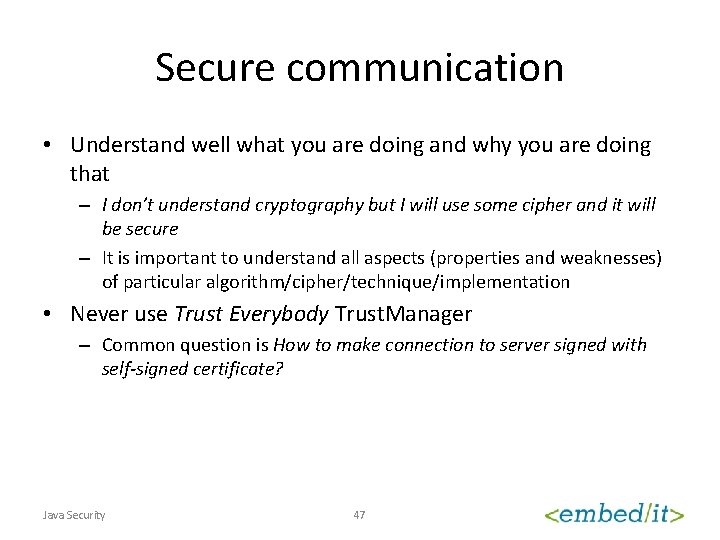
Secure communication • Understand well what you are doing and why you are doing that – I don’t understand cryptography but I will use some cipher and it will be secure – It is important to understand all aspects (properties and weaknesses) of particular algorithm/cipher/technique/implementation • Never use Trust Everybody Trust. Manager – Common question is How to make connection to server signed with self-signed certificate? Java Security 47
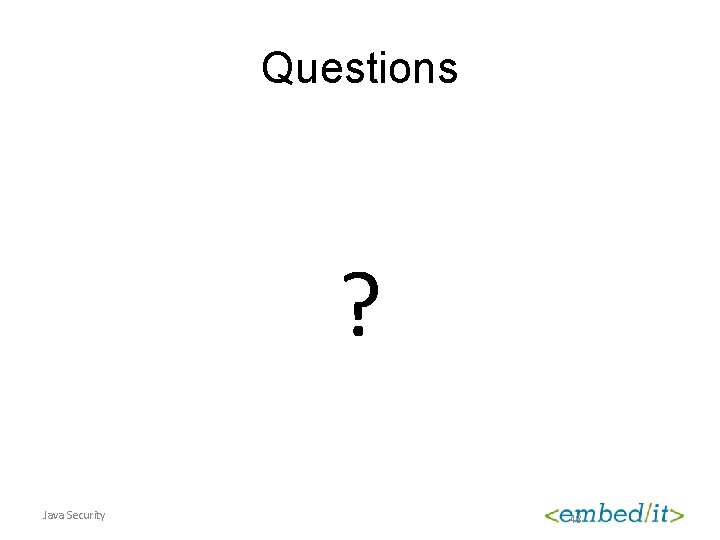
Questions ? Java Security 48