Java Script and Ajax Java Script Form Validation
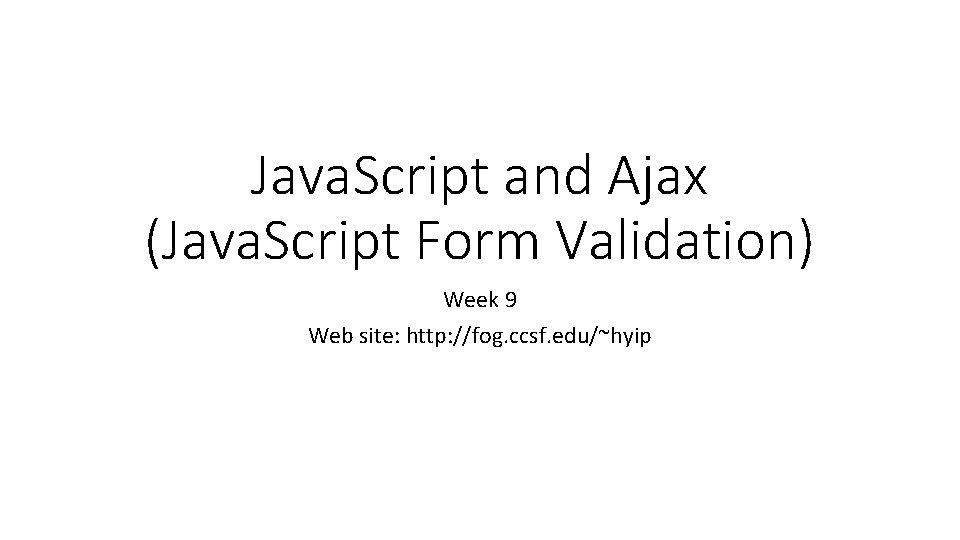
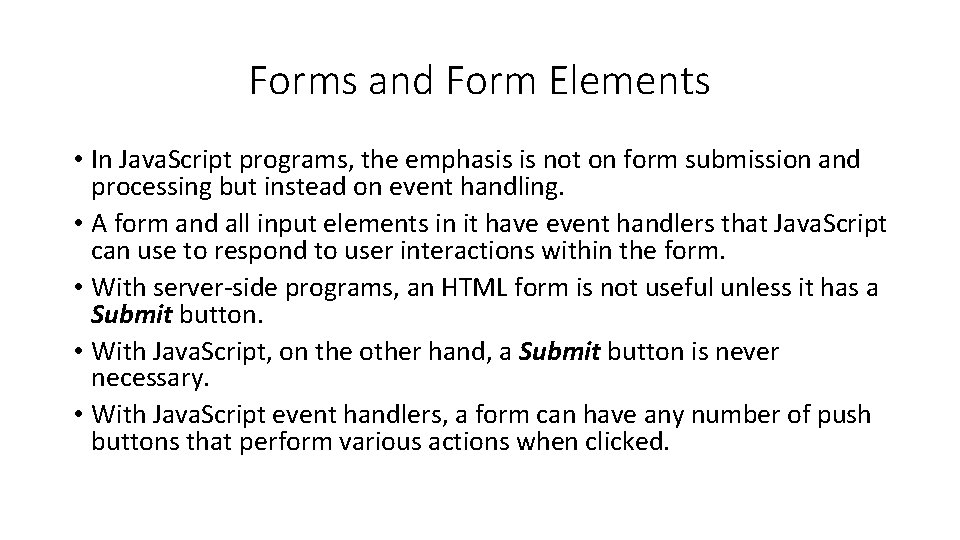
![The Form Object • Form objects are available as elements of the forms[ ] The Form Object • Form objects are available as elements of the forms[ ]](https://slidetodoc.com/presentation_image_h2/791d96c6d9215473956bda4424984fdf/image-3.jpg)
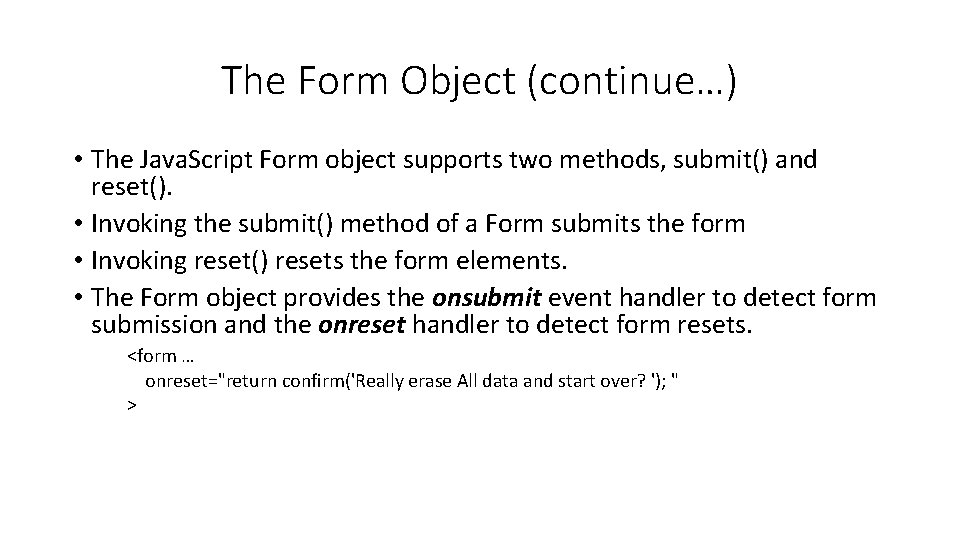
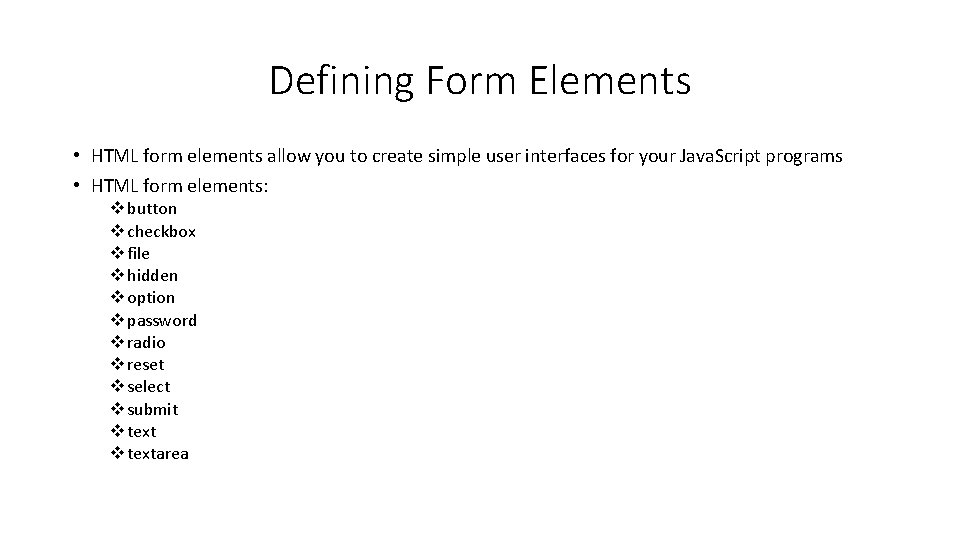
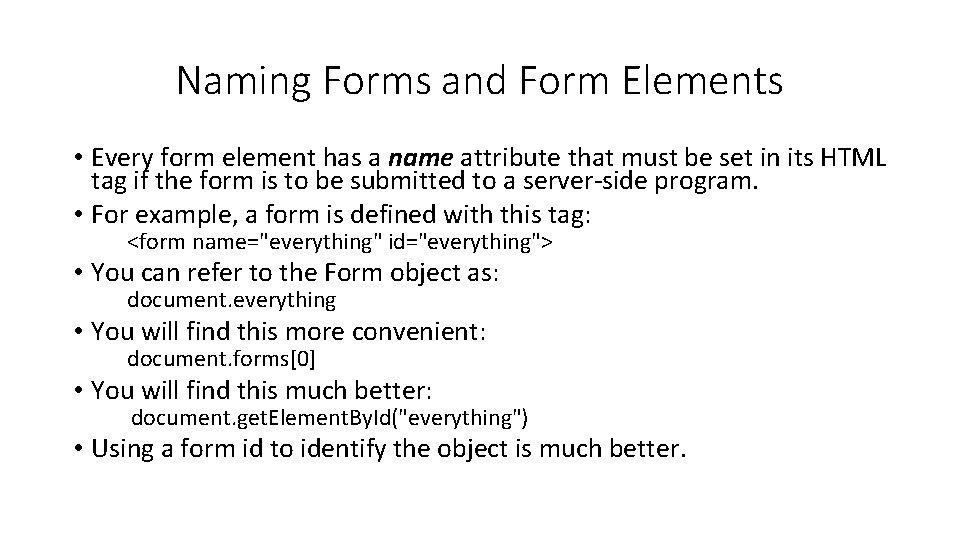
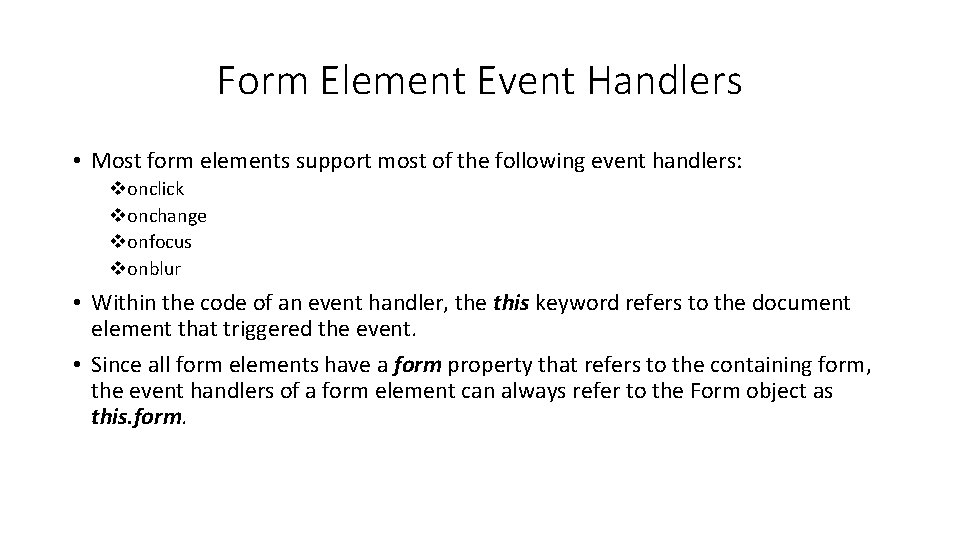
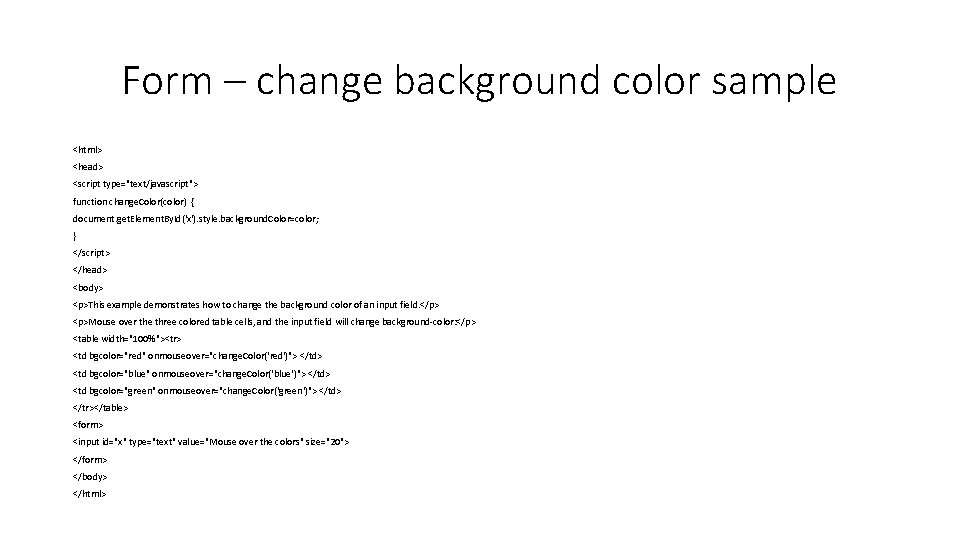
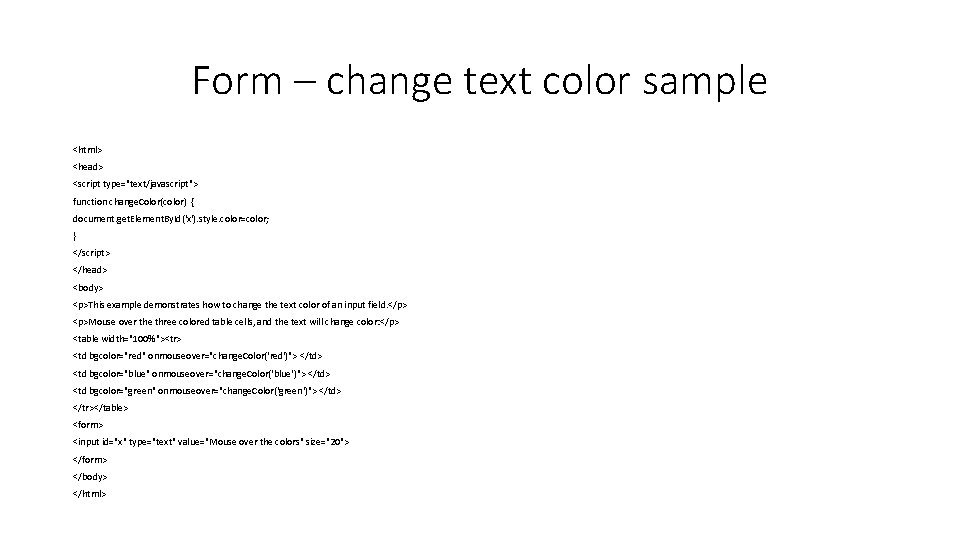
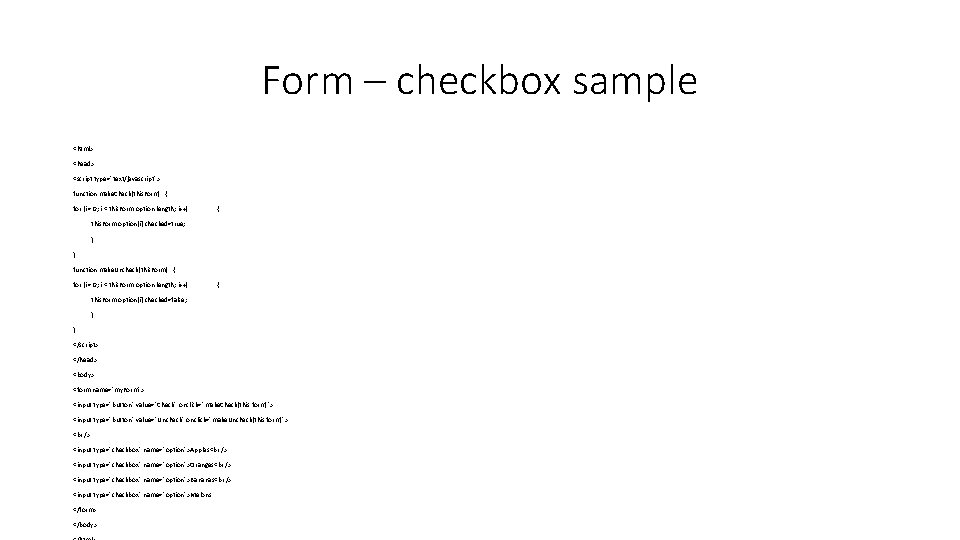
- Slides: 10
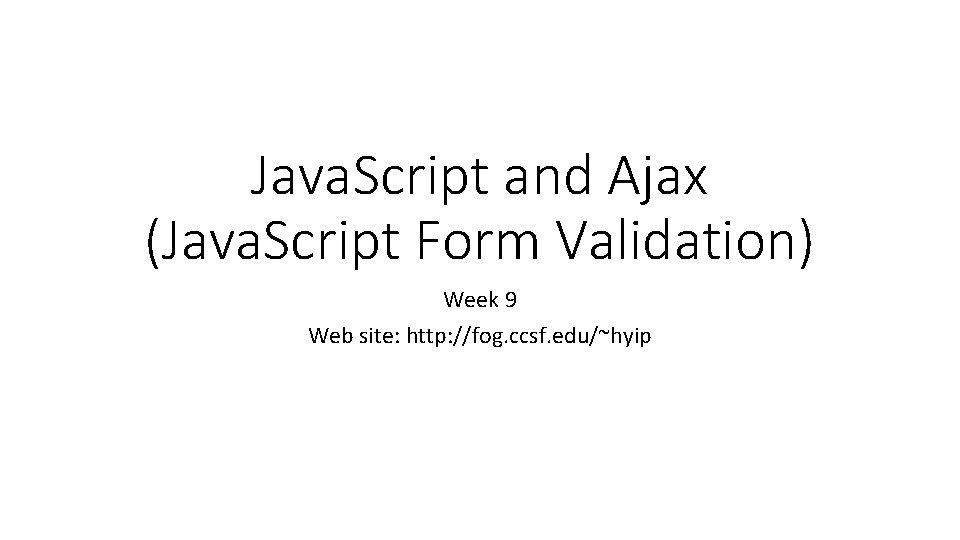
Java. Script and Ajax (Java. Script Form Validation) Week 9 Web site: http: //fog. ccsf. edu/~hyip
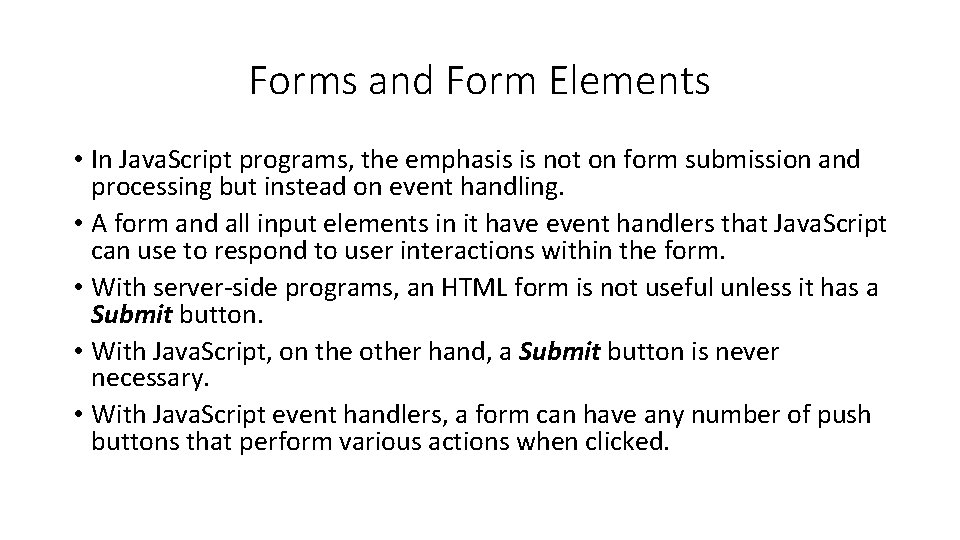
Forms and Form Elements • In Java. Script programs, the emphasis is not on form submission and processing but instead on event handling. • A form and all input elements in it have event handlers that Java. Script can use to respond to user interactions within the form. • With server-side programs, an HTML form is not useful unless it has a Submit button. • With Java. Script, on the other hand, a Submit button is never necessary. • With Java. Script event handlers, a form can have any number of push buttons that perform various actions when clicked.
![The Form Object Form objects are available as elements of the forms The Form Object • Form objects are available as elements of the forms[ ]](https://slidetodoc.com/presentation_image_h2/791d96c6d9215473956bda4424984fdf/image-3.jpg)
The Form Object • Form objects are available as elements of the forms[ ] array, which is a property of the Document object. You can refer to the last form in a document: document. forms[document. forms. length – 1] • The most interesting property of the Form object is the elements[ ] array, which contains Java. Script objects (of various types) that represent the various input elements of the form. document. forms[1]. elements[2] // 3 rd element 2 nd form • The remaining properties of the Form object are of less importance. The action, encoding, method, and target properties correspond directly to the action, encoding, method, and target attributes of the <form> tag.
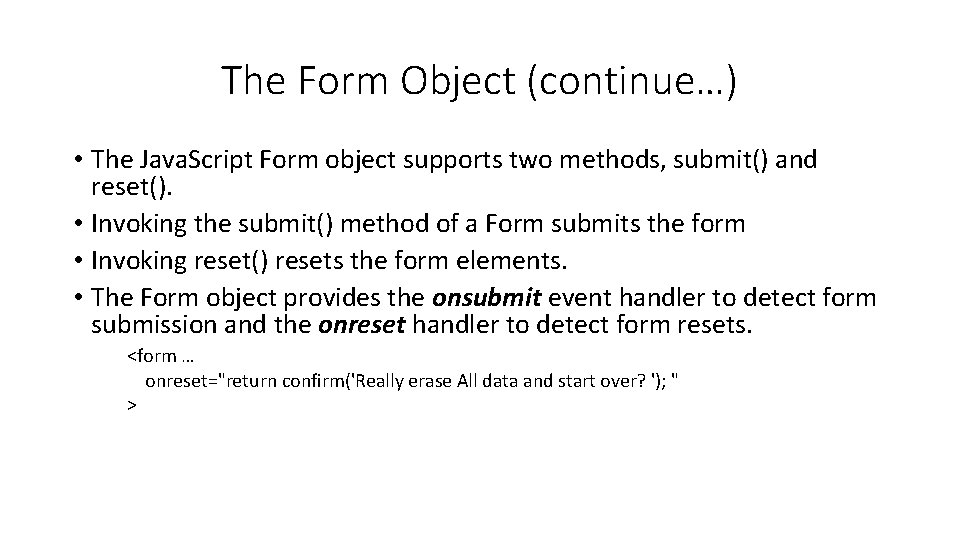
The Form Object (continue…) • The Java. Script Form object supports two methods, submit() and reset(). • Invoking the submit() method of a Form submits the form • Invoking reset() resets the form elements. • The Form object provides the onsubmit event handler to detect form submission and the onreset handler to detect form resets. <form … onreset="return confirm('Really erase All data and start over? '); " >
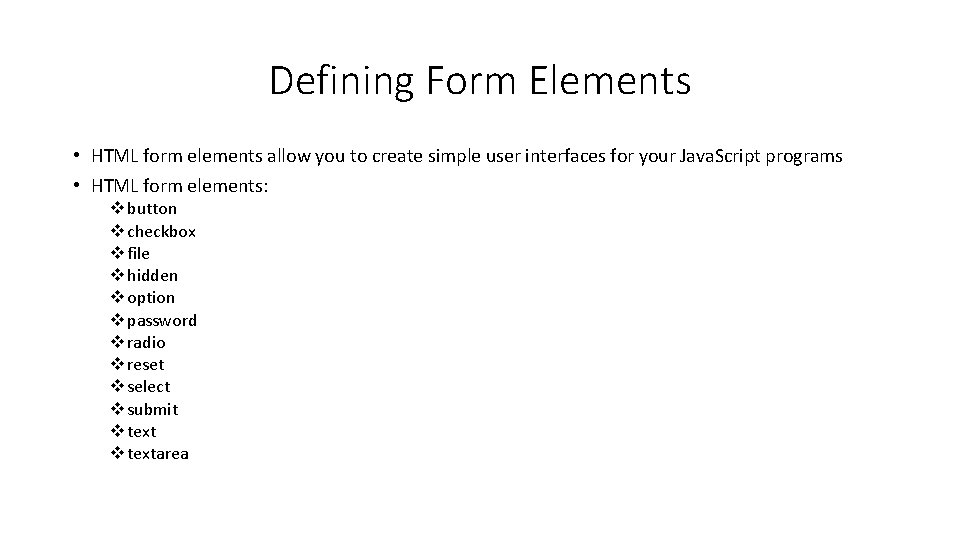
Defining Form Elements • HTML form elements allow you to create simple user interfaces for your Java. Script programs • HTML form elements: v button v checkbox v file v hidden v option v password v radio v reset v select v submit v textarea
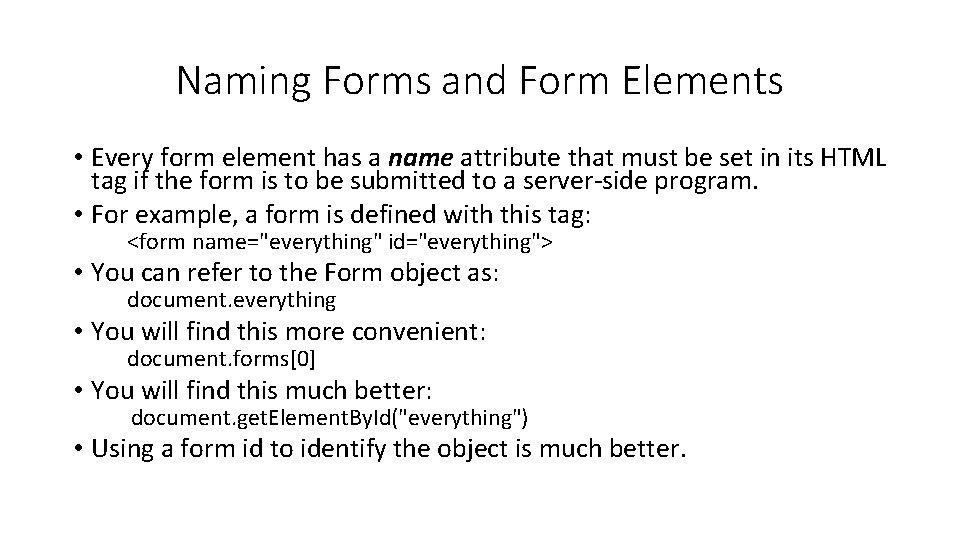
Naming Forms and Form Elements • Every form element has a name attribute that must be set in its HTML tag if the form is to be submitted to a server-side program. • For example, a form is defined with this tag: <form name="everything" id="everything"> • You can refer to the Form object as: document. everything • You will find this more convenient: document. forms[0] • You will find this much better: document. get. Element. By. Id("everything") • Using a form id to identify the object is much better.
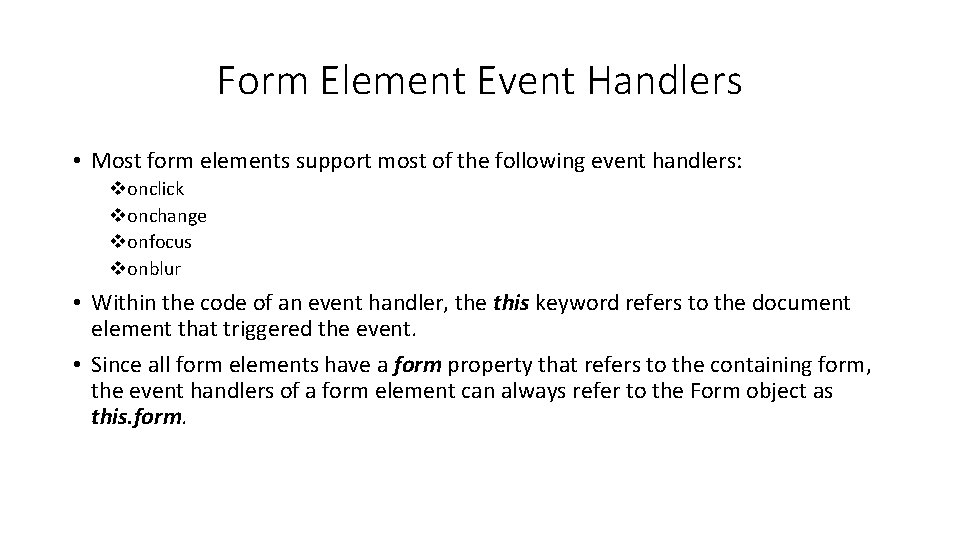
Form Element Event Handlers • Most form elements support most of the following event handlers: vonclick vonchange vonfocus vonblur • Within the code of an event handler, the this keyword refers to the document element that triggered the event. • Since all form elements have a form property that refers to the containing form, the event handlers of a form element can always refer to the Form object as this. form.
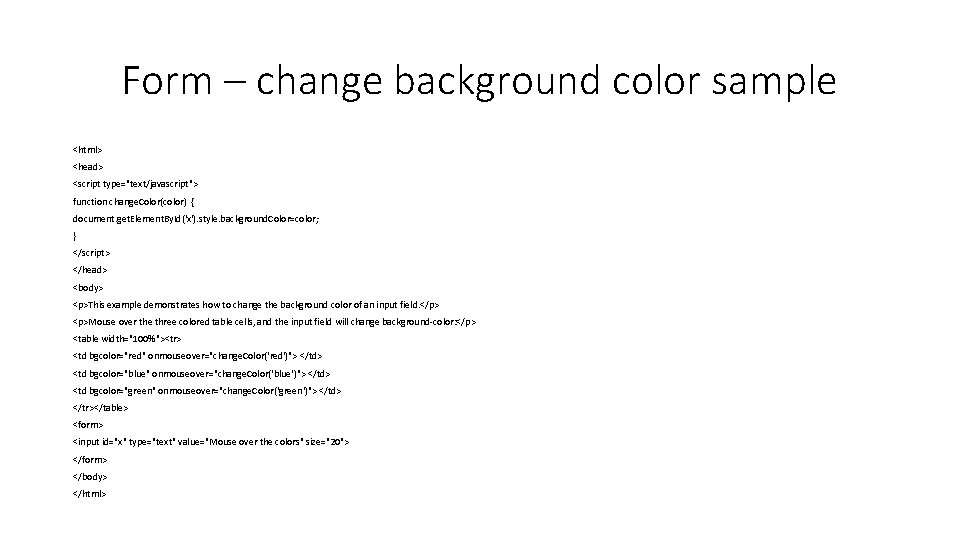
Form – change background color sample <html> <head> <script type="text/javascript"> function change. Color(color) { document. get. Element. By. Id('x'). style. background. Color=color; } </script> </head> <body> <p>This example demonstrates how to change the background color of an input field. </p> <p>Mouse over the three colored table cells, and the input field will change background-color: </p> <table width="100%"><tr> <td bgcolor="red" onmouseover="change. Color('red')"> </td> <td bgcolor="blue" onmouseover="change. Color('blue')"> </td> <td bgcolor="green" onmouseover="change. Color('green')"> </td> </tr></table> <form> <input id="x" type="text" value="Mouse over the colors" size="20"> </form> </body> </html>
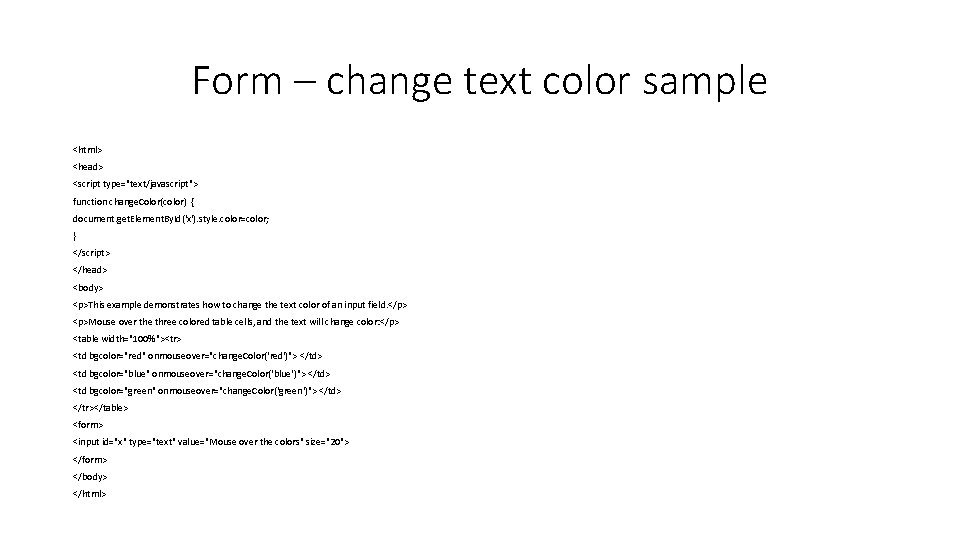
Form – change text color sample <html> <head> <script type="text/javascript"> function change. Color(color) { document. get. Element. By. Id('x'). style. color=color; } </script> </head> <body> <p>This example demonstrates how to change the text color of an input field. </p> <p>Mouse over the three colored table cells, and the text will change color: </p> <table width="100%"><tr> <td bgcolor="red" onmouseover="change. Color('red')"> </td> <td bgcolor="blue" onmouseover="change. Color('blue')"> </td> <td bgcolor="green" onmouseover="change. Color('green')"> </td> </tr></table> <form> <input id="x" type="text" value="Mouse over the colors" size="20"> </form> </body> </html>
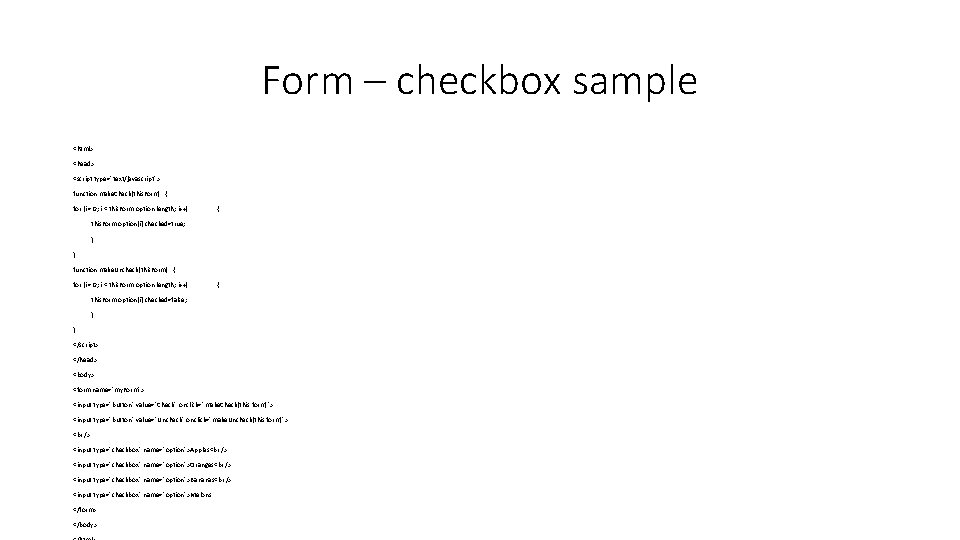
Form – checkbox sample <html> <head> <script type="text/javascript"> function make. Check(this. Form) { for (i = 0; i < this. Form. option. length; i++) { this. Form. option[i]. checked=true; } } function make. Uncheck(this. Form) { for (i = 0; i < this. Form. option. length; i++) { this. Form. option[i]. checked=false; } } </script> </head> <body> <form name="my. Form"> <input type="button" value="Check" onclick="make. Check(this. form)"> <input type="button" value="Uncheck" onclick="make. Uncheck(this. form)"> <input type="checkbox" name="option">Apples <input type="checkbox" name="option">Oranges <input type="checkbox" name="option">Bananas <input type="checkbox" name="option">Melons </form> </body>