Java Script and Ajax Java Script Arrays Week
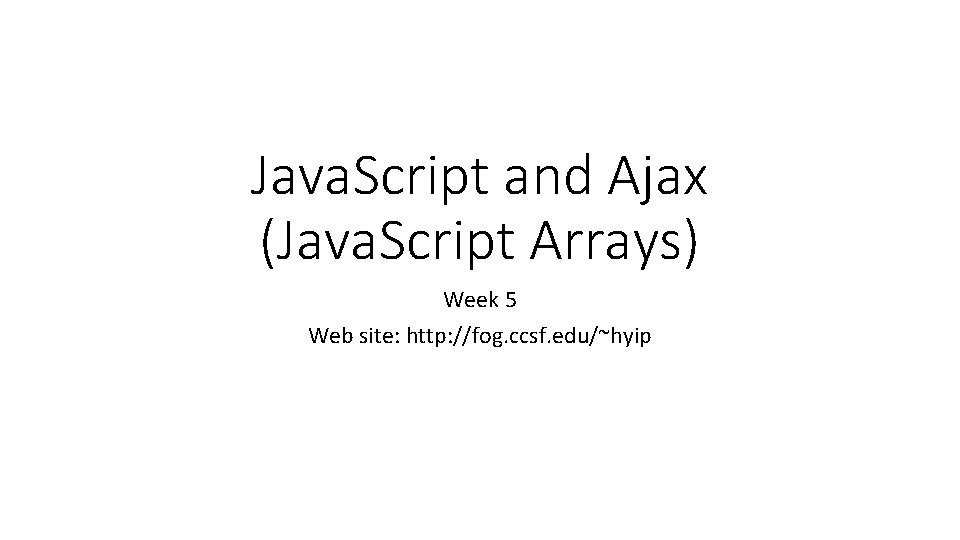
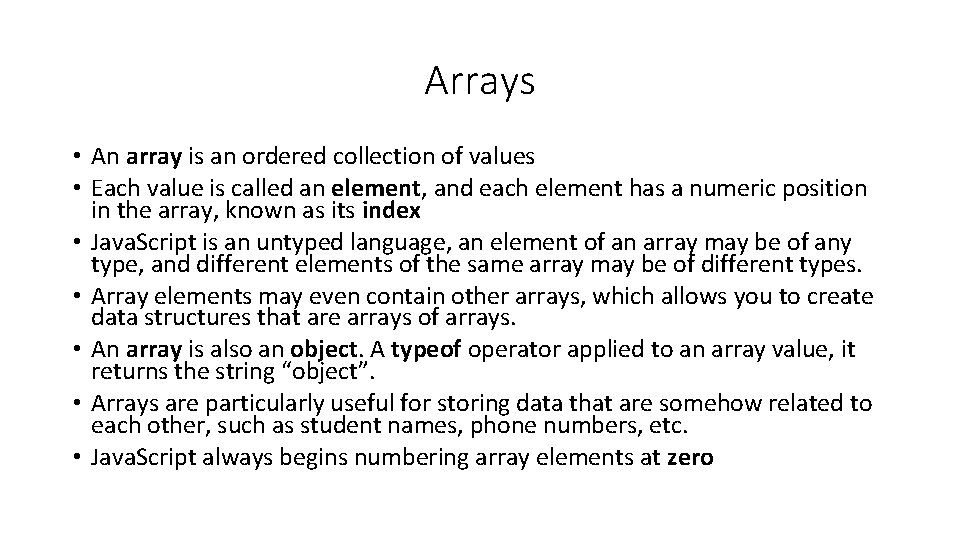
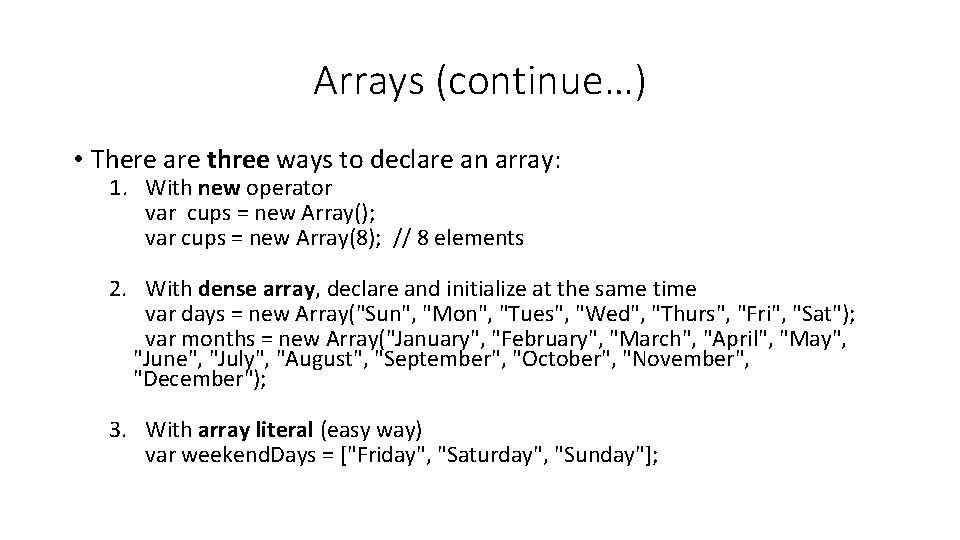
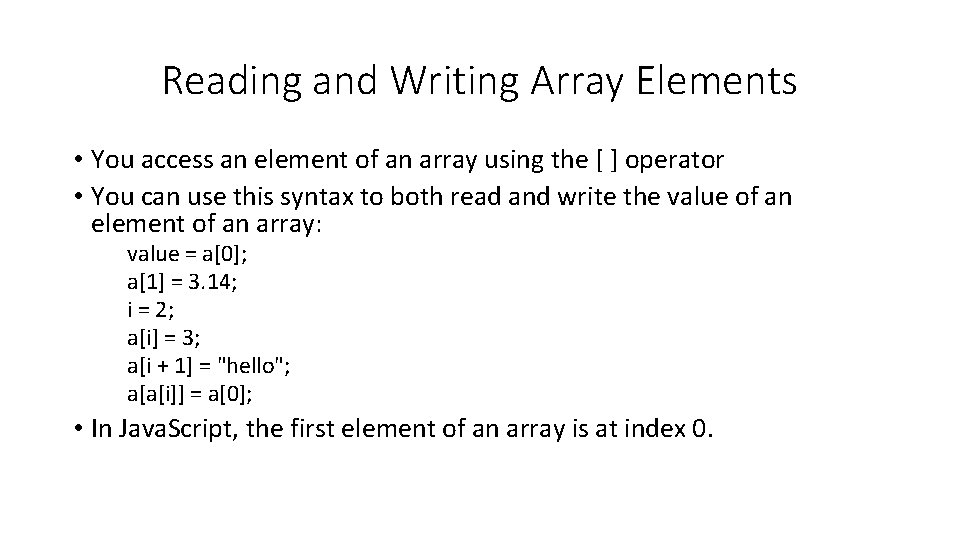
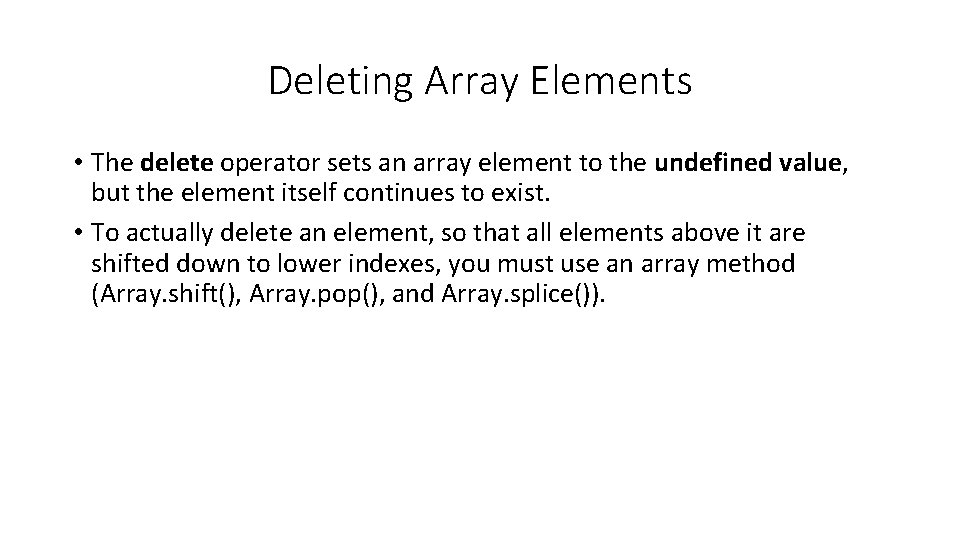
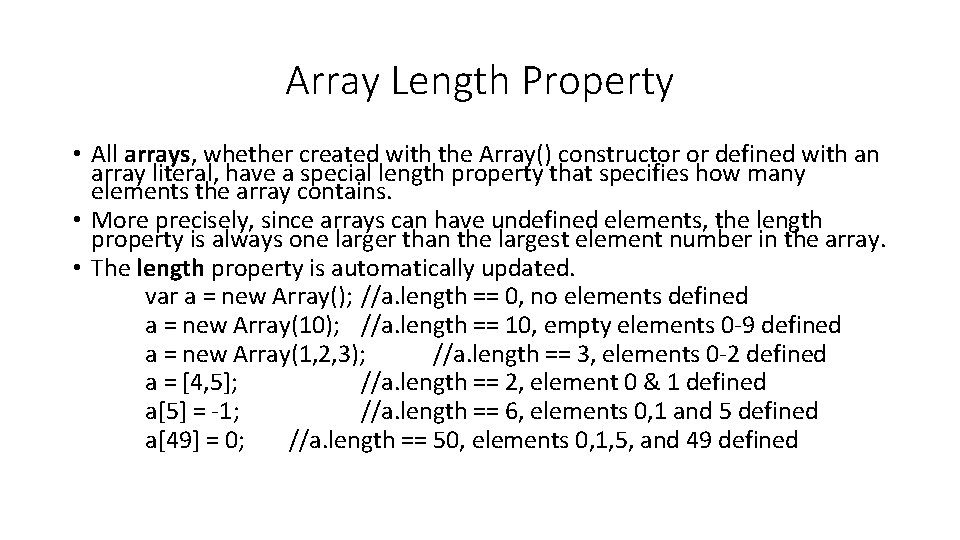
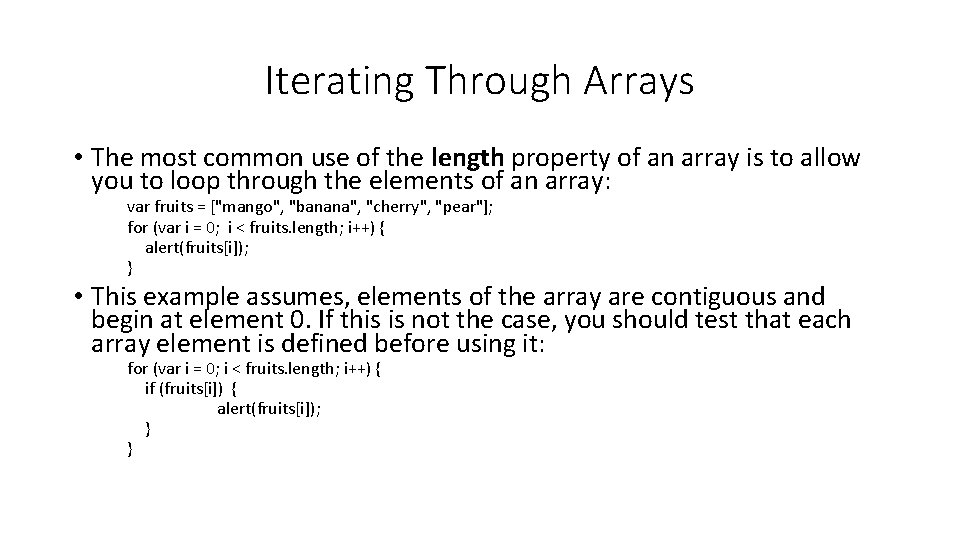
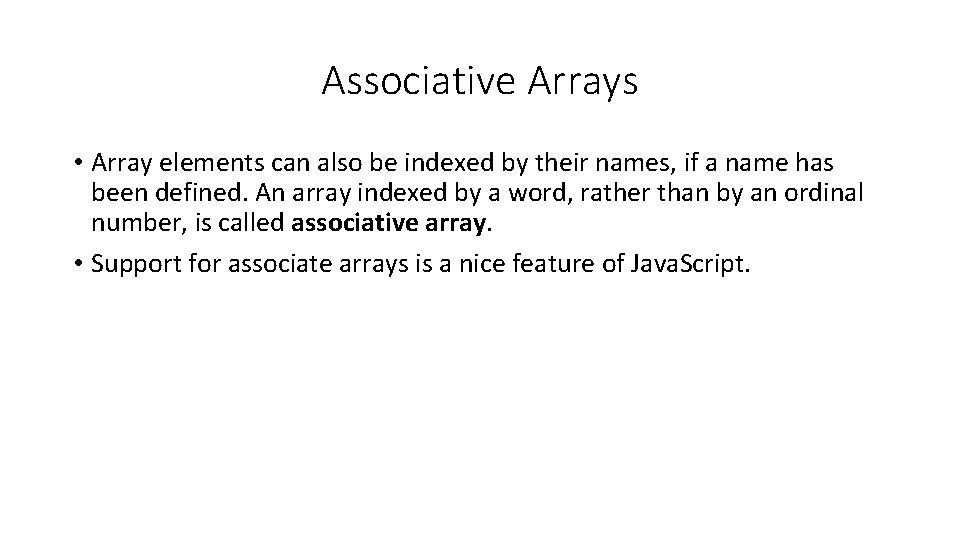
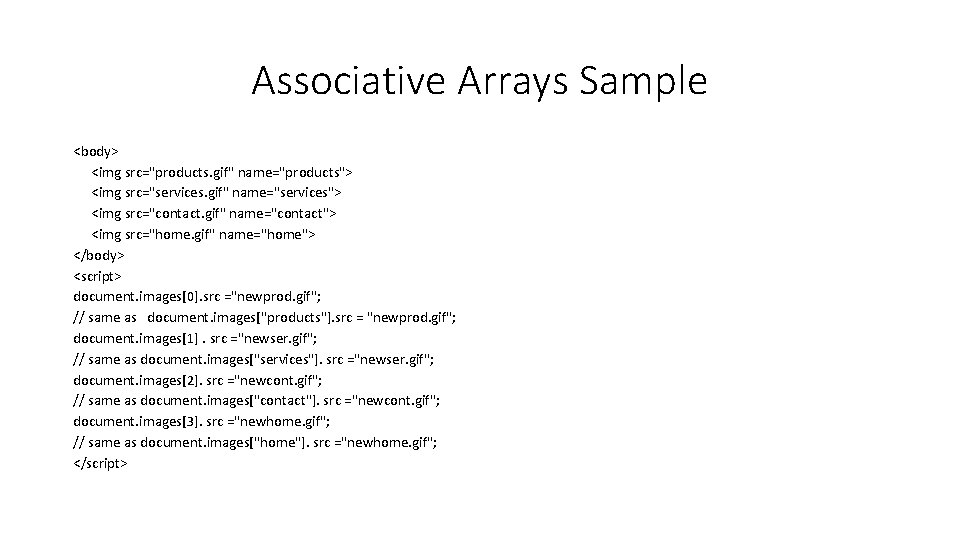
![Associative Arrays Sample (continue…) var birthdays = new Array(); birthdays["Tim"] = "May 15"; Birthdays["Mom"] Associative Arrays Sample (continue…) var birthdays = new Array(); birthdays["Tim"] = "May 15"; Birthdays["Mom"]](https://slidetodoc.com/presentation_image/4fcdea33a032bd92efba2e85949f84dd/image-10.jpg)
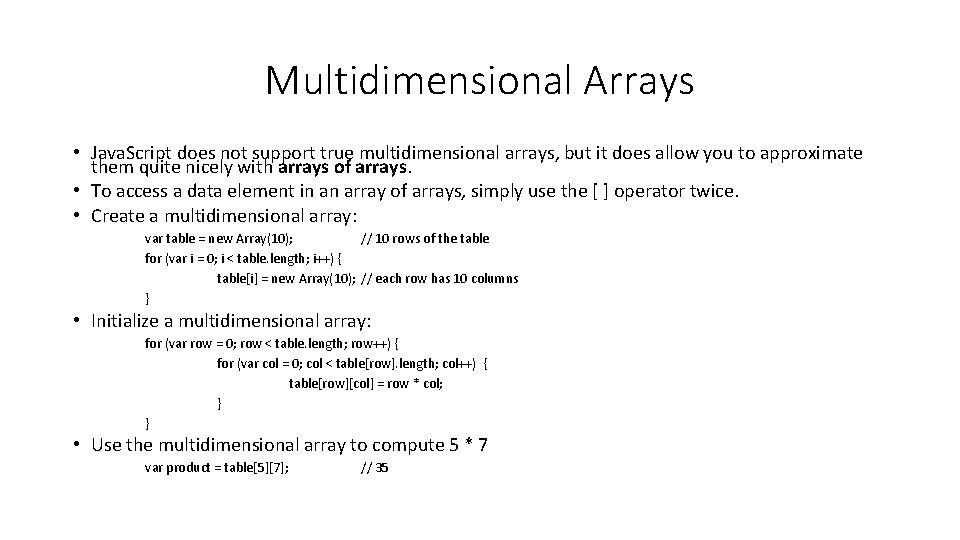
![Array Methods • In addition to the [ ] operator, arrays can be manipulated Array Methods • In addition to the [ ] operator, arrays can be manipulated](https://slidetodoc.com/presentation_image/4fcdea33a032bd92efba2e85949f84dd/image-12.jpg)
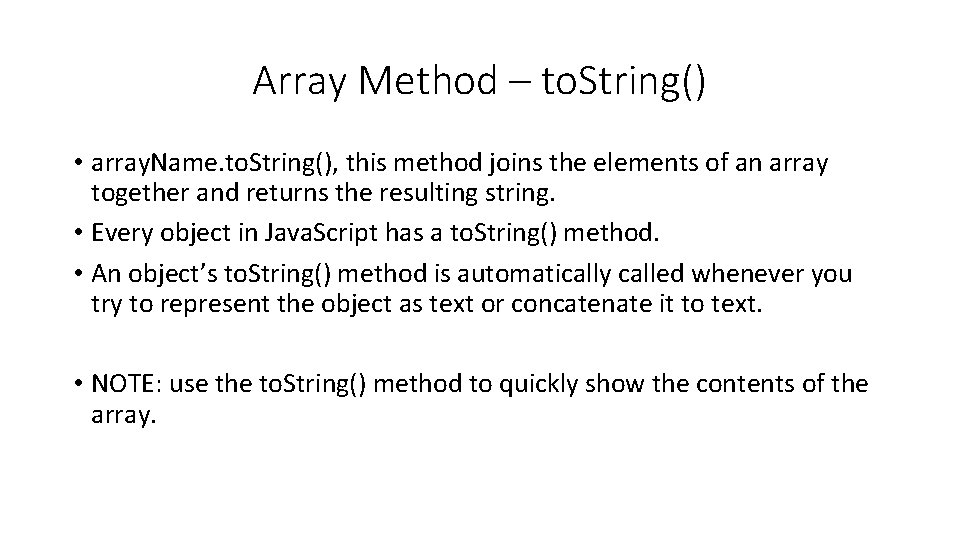
![A Summary of Array Methods Method Description concat(ary 2[, ary 3, …]) Concatenates two A Summary of Array Methods Method Description concat(ary 2[, ary 3, …]) Concatenates two](https://slidetodoc.com/presentation_image/4fcdea33a032bd92efba2e85949f84dd/image-14.jpg)
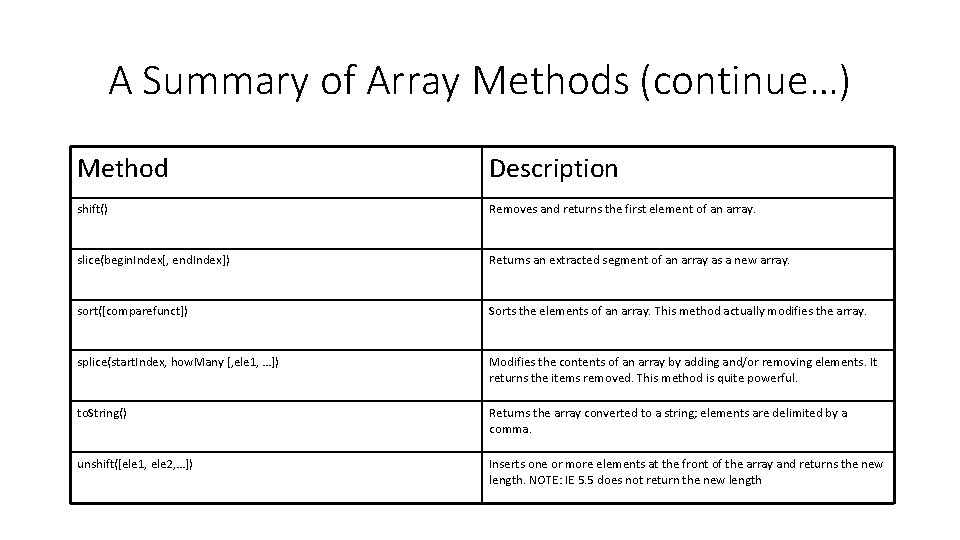
- Slides: 15
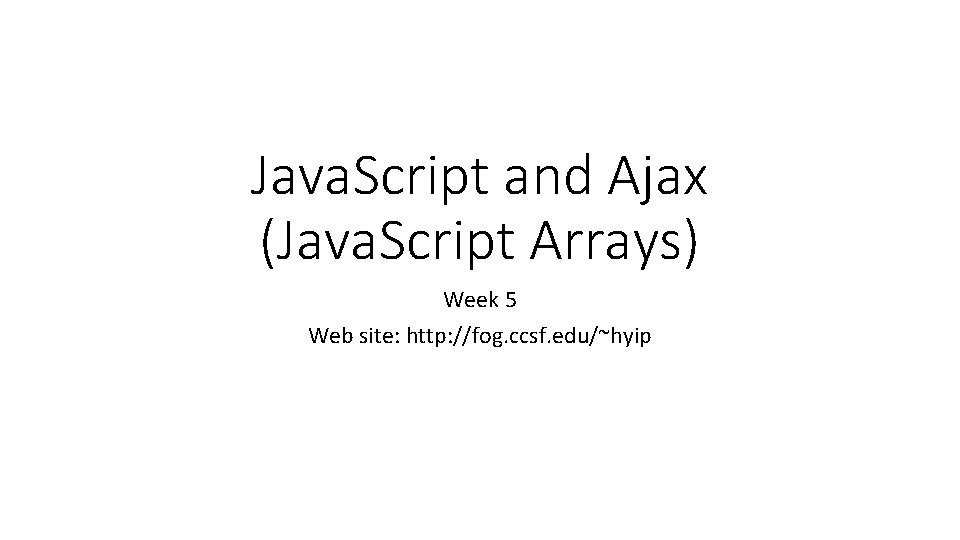
Java. Script and Ajax (Java. Script Arrays) Week 5 Web site: http: //fog. ccsf. edu/~hyip
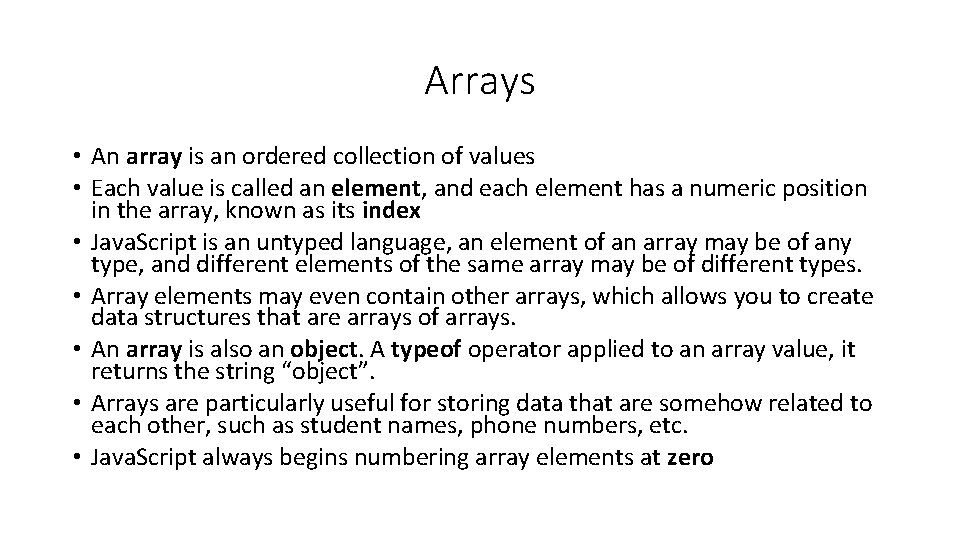
Arrays • An array is an ordered collection of values • Each value is called an element, and each element has a numeric position in the array, known as its index • Java. Script is an untyped language, an element of an array may be of any type, and different elements of the same array may be of different types. • Array elements may even contain other arrays, which allows you to create data structures that are arrays of arrays. • An array is also an object. A typeof operator applied to an array value, it returns the string “object”. • Arrays are particularly useful for storing data that are somehow related to each other, such as student names, phone numbers, etc. • Java. Script always begins numbering array elements at zero
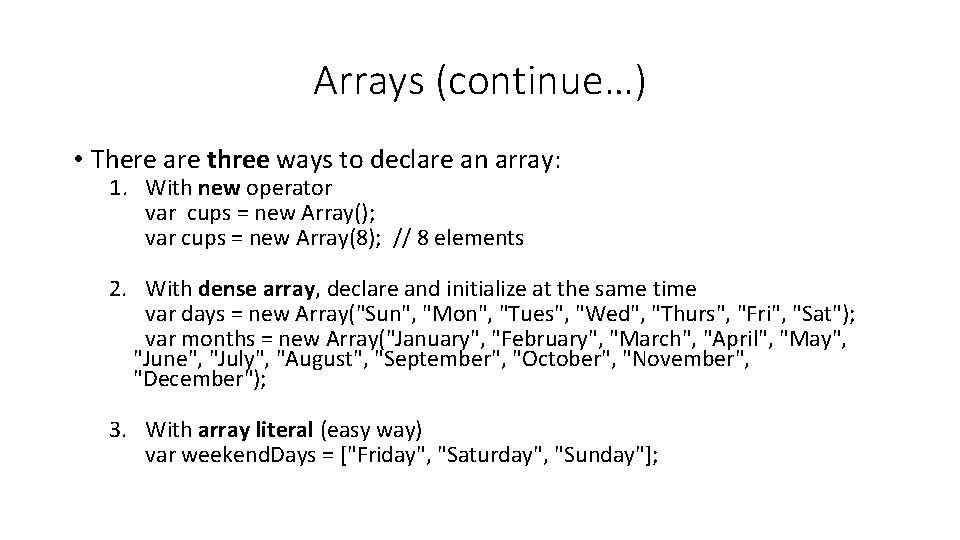
Arrays (continue…) • There are three ways to declare an array: 1. With new operator var cups = new Array(); var cups = new Array(8); // 8 elements 2. With dense array, declare and initialize at the same time var days = new Array("Sun", "Mon", "Tues", "Wed", "Thurs", "Fri", "Sat"); var months = new Array("January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"); 3. With array literal (easy way) var weekend. Days = ["Friday", "Saturday", "Sunday"];
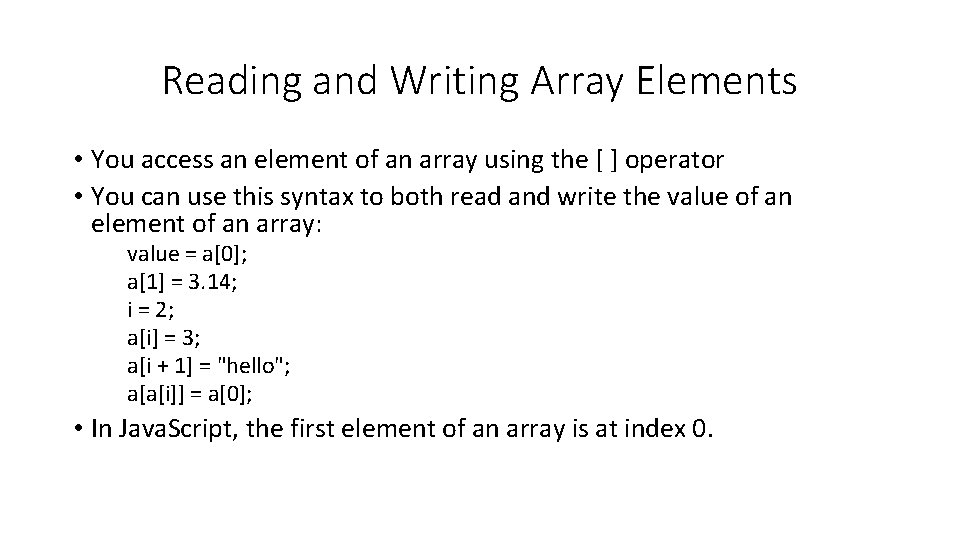
Reading and Writing Array Elements • You access an element of an array using the [ ] operator • You can use this syntax to both read and write the value of an element of an array: value = a[0]; a[1] = 3. 14; i = 2; a[i] = 3; a[i + 1] = "hello"; a[a[i]] = a[0]; • In Java. Script, the first element of an array is at index 0.
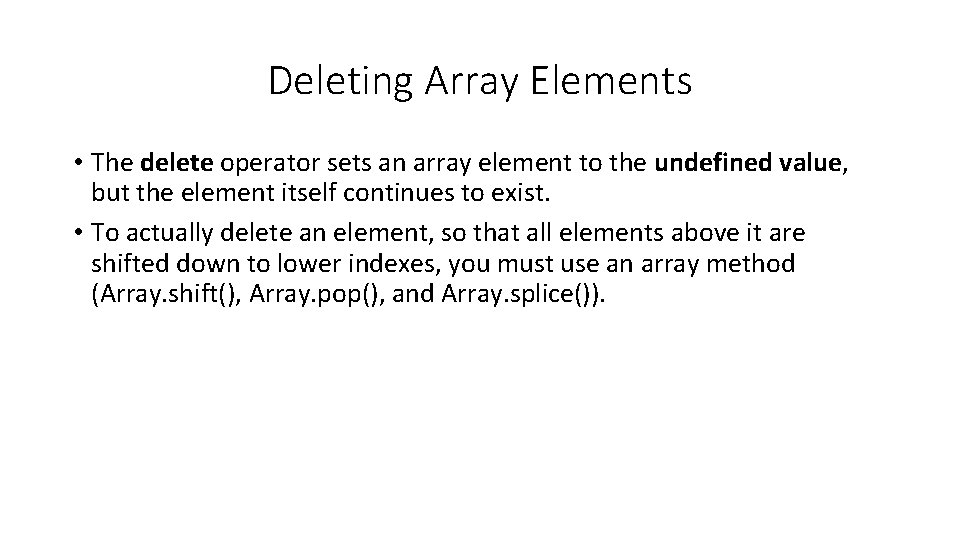
Deleting Array Elements • The delete operator sets an array element to the undefined value, but the element itself continues to exist. • To actually delete an element, so that all elements above it are shifted down to lower indexes, you must use an array method (Array. shift(), Array. pop(), and Array. splice()).
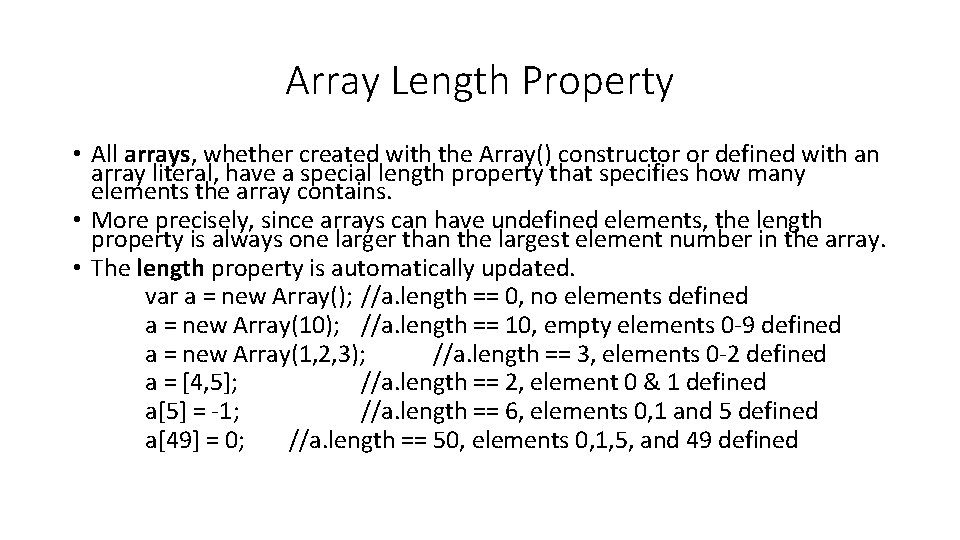
Array Length Property • All arrays, whether created with the Array() constructor or defined with an array literal, have a special length property that specifies how many elements the array contains. • More precisely, since arrays can have undefined elements, the length property is always one larger than the largest element number in the array. • The length property is automatically updated. var a = new Array(); //a. length == 0, no elements defined a = new Array(10); //a. length == 10, empty elements 0 -9 defined a = new Array(1, 2, 3); //a. length == 3, elements 0 -2 defined a = [4, 5]; //a. length == 2, element 0 & 1 defined a[5] = -1; //a. length == 6, elements 0, 1 and 5 defined a[49] = 0; //a. length == 50, elements 0, 1, 5, and 49 defined
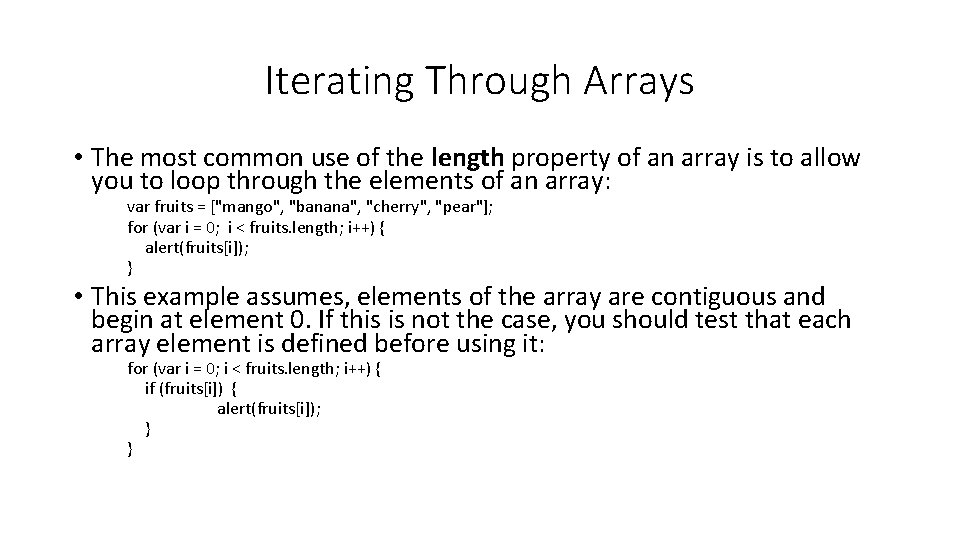
Iterating Through Arrays • The most common use of the length property of an array is to allow you to loop through the elements of an array: var fruits = ["mango", "banana", "cherry", "pear"]; for (var i = 0; i < fruits. length; i++) { alert(fruits[i]); } • This example assumes, elements of the array are contiguous and begin at element 0. If this is not the case, you should test that each array element is defined before using it: for (var i = 0; i < fruits. length; i++) { if (fruits[i]) { alert(fruits[i]); } }
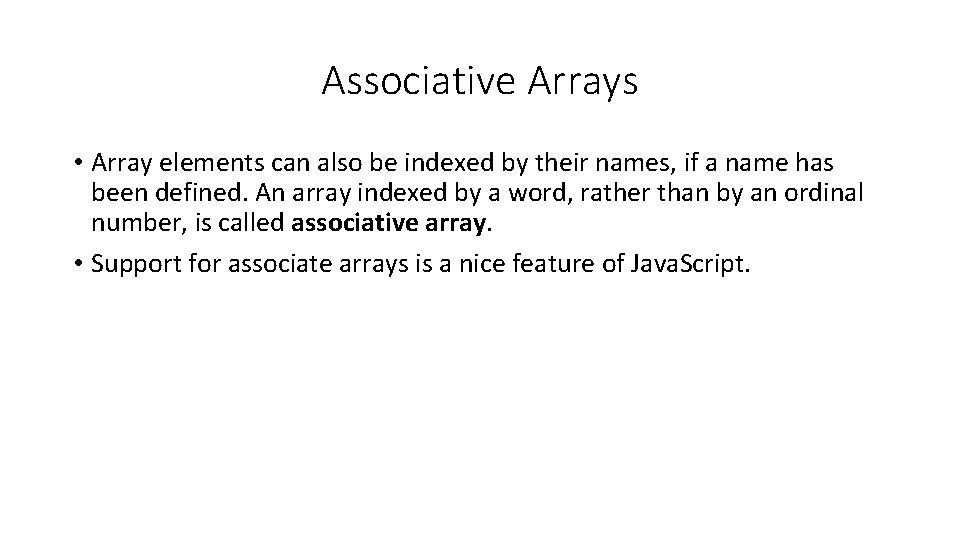
Associative Arrays • Array elements can also be indexed by their names, if a name has been defined. An array indexed by a word, rather than by an ordinal number, is called associative array. • Support for associate arrays is a nice feature of Java. Script.
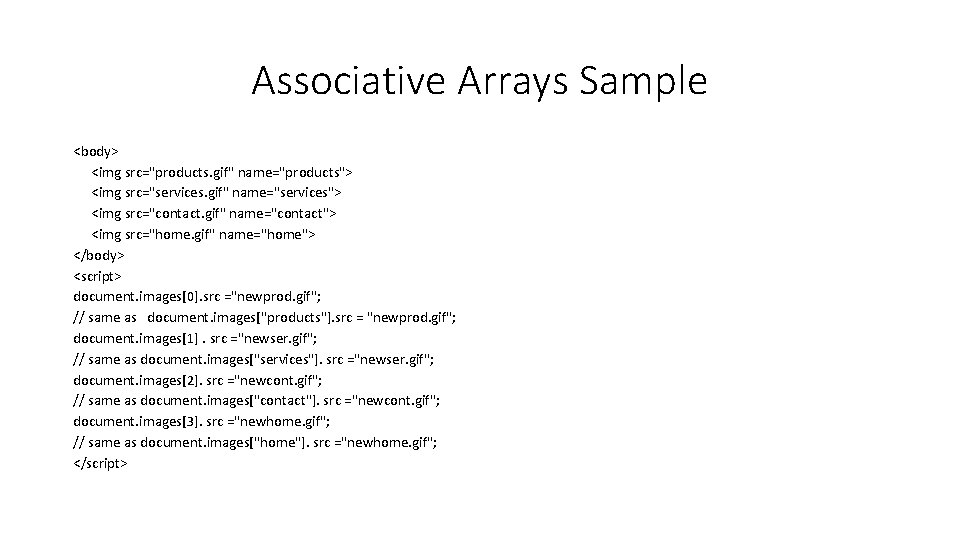
Associative Arrays Sample <body> <img src="products. gif" name="products"> <img src="services. gif" name="services"> <img src="contact. gif" name="contact"> <img src="home. gif" name="home"> </body> <script> document. images[0]. src ="newprod. gif"; // same as document. images["products"]. src = "newprod. gif"; document. images[1]. src ="newser. gif"; // same as document. images["services"]. src ="newser. gif"; document. images[2]. src ="newcont. gif"; // same as document. images["contact"]. src ="newcont. gif"; document. images[3]. src ="newhome. gif"; // same as document. images["home"]. src ="newhome. gif"; </script>
![Associative Arrays Sample continue var birthdays new Array birthdaysTim May 15 BirthdaysMom Associative Arrays Sample (continue…) var birthdays = new Array(); birthdays["Tim"] = "May 15"; Birthdays["Mom"]](https://slidetodoc.com/presentation_image/4fcdea33a032bd92efba2e85949f84dd/image-10.jpg)
Associative Arrays Sample (continue…) var birthdays = new Array(); birthdays["Tim"] = "May 15"; Birthdays["Mom"] = "Mar 22"; birthdays["Jean"] = "Feb 18"; birthdays["Paul"] = "Jan 25"; document. write("Tim’s birthday is ", birthdays["Tim"], " ");
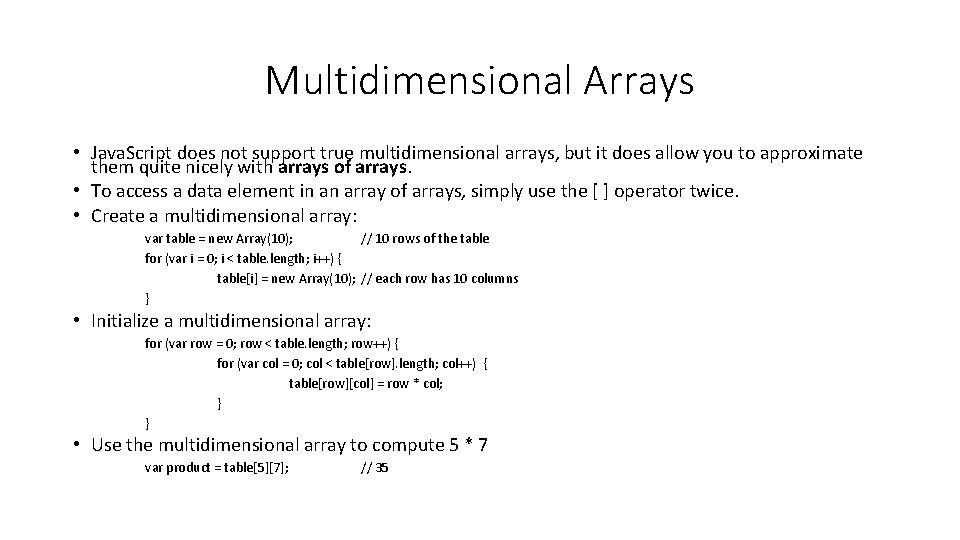
Multidimensional Arrays • Java. Script does not support true multidimensional arrays, but it does allow you to approximate them quite nicely with arrays of arrays. • To access a data element in an array of arrays, simply use the [ ] operator twice. • Create a multidimensional array: var table = new Array(10); // 10 rows of the table for (var i = 0; i < table. length; i++) { table[i] = new Array(10); // each row has 10 columns } • Initialize a multidimensional array: for (var row = 0; row < table. length; row++) { for (var col = 0; col < table[row]. length; col++) { table[row][col] = row * col; } } • Use the multidimensional array to compute 5 * 7 var product = table[5][7]; // 35
![Array Methods In addition to the operator arrays can be manipulated Array Methods • In addition to the [ ] operator, arrays can be manipulated](https://slidetodoc.com/presentation_image/4fcdea33a032bd92efba2e85949f84dd/image-12.jpg)
Array Methods • In addition to the [ ] operator, arrays can be manipulated through various methods provided by the Array class. • join() • reverse() • sort() • concat() • slice() • splice() • push() and pop() • unshift() and shift() • to. String() and to. Locale. String()
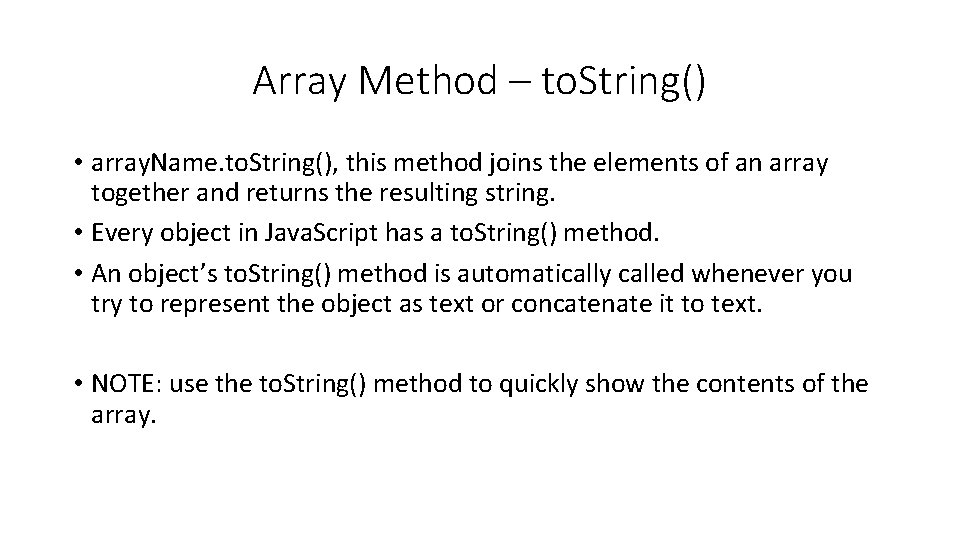
Array Method – to. String() • array. Name. to. String(), this method joins the elements of an array together and returns the resulting string. • Every object in Java. Script has a to. String() method. • An object’s to. String() method is automatically called whenever you try to represent the object as text or concatenate it to text. • NOTE: use the to. String() method to quickly show the contents of the array.
![A Summary of Array Methods Method Description concatary 2 ary 3 Concatenates two A Summary of Array Methods Method Description concat(ary 2[, ary 3, …]) Concatenates two](https://slidetodoc.com/presentation_image/4fcdea33a032bd92efba2e85949f84dd/image-14.jpg)
A Summary of Array Methods Method Description concat(ary 2[, ary 3, …]) Concatenates two or more arrays and returns the result as a new array. join([separator]) Returns all of the elements of an array joined together in a single string delimited by an optional separator, if no separator is specified, a comma is inserted between elements. pop() Removes and returns the last element of an array. push(ele 1[, ele 2, …]) Appends one or more elements on to the end of an array and returns the array’s new length. reverse() Reverses the order of an array. This method actually modifies the array.
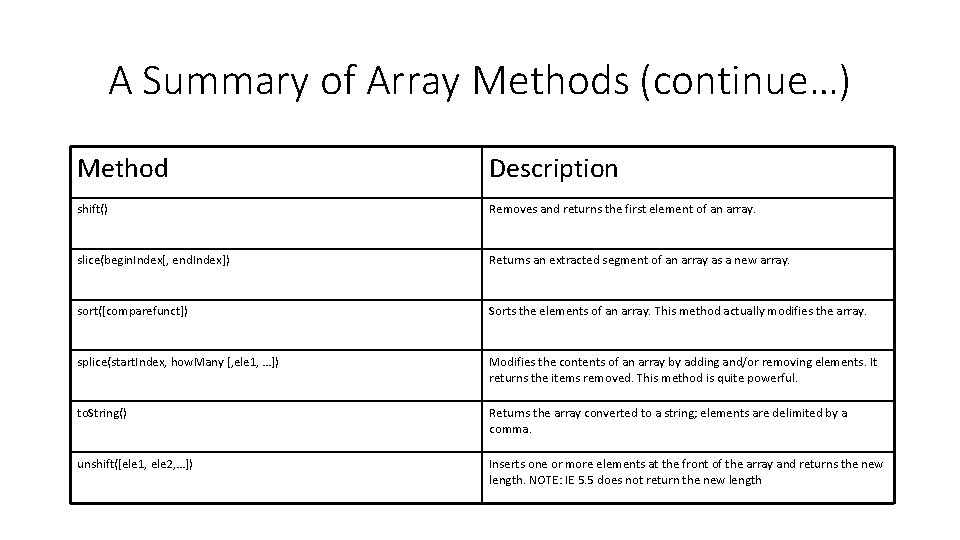
A Summary of Array Methods (continue…) Method Description shift() Removes and returns the first element of an array. slice(begin. Index[, end. Index]) Returns an extracted segment of an array as a new array. sort([comparefunct]) Sorts the elements of an array. This method actually modifies the array. splice(start. Index, how. Many [, ele 1, …]) Modifies the contents of an array by adding and/or removing elements. It returns the items removed. This method is quite powerful. to. String() Returns the array converted to a string; elements are delimited by a comma. unshift([ele 1, ele 2, …]) Inserts one or more elements at the front of the array and returns the new length. NOTE: IE 5. 5 does not return the new length